Java Programming Tutorial Java TM Programming Tutorial Lanrel
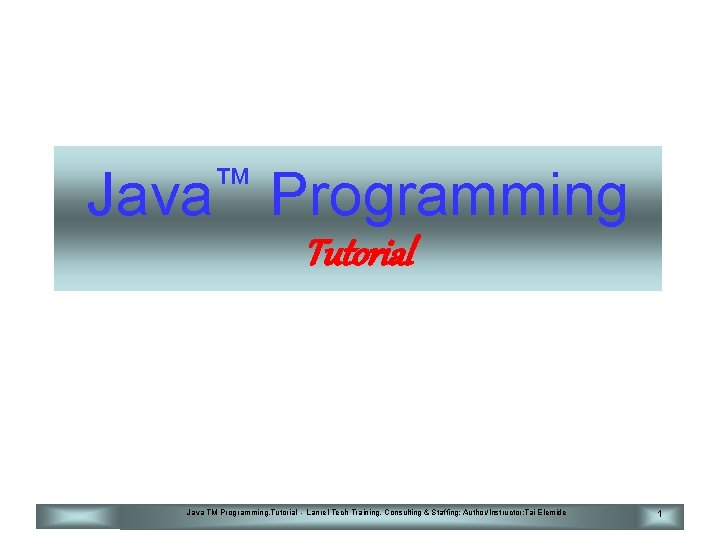
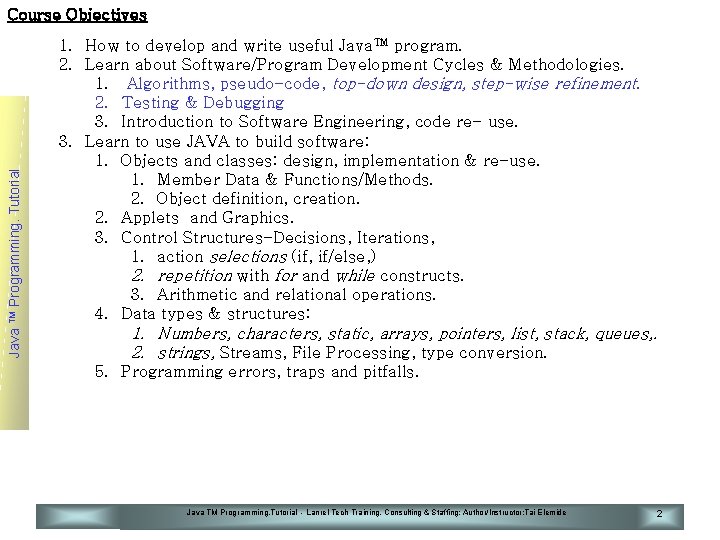
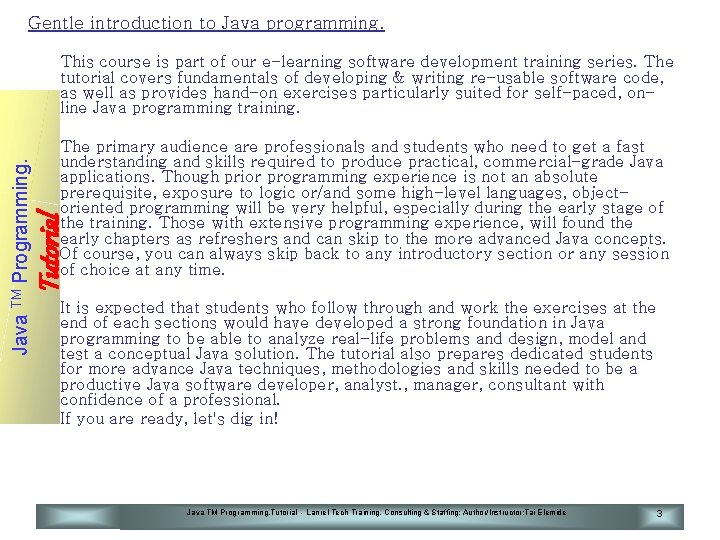
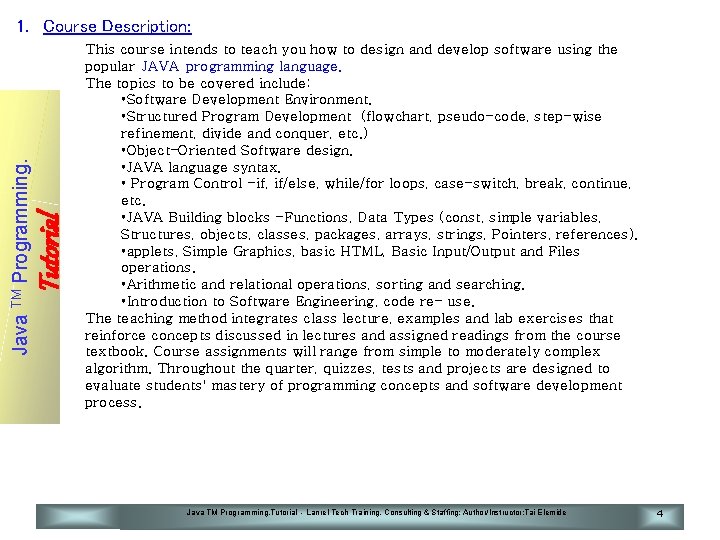
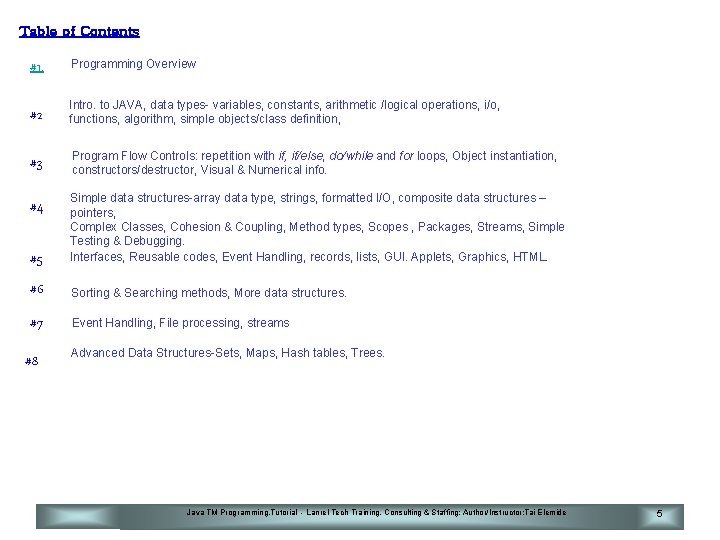
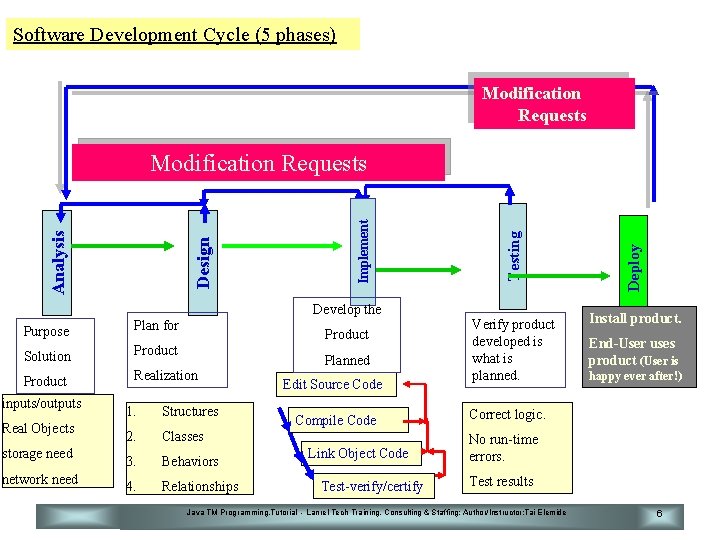
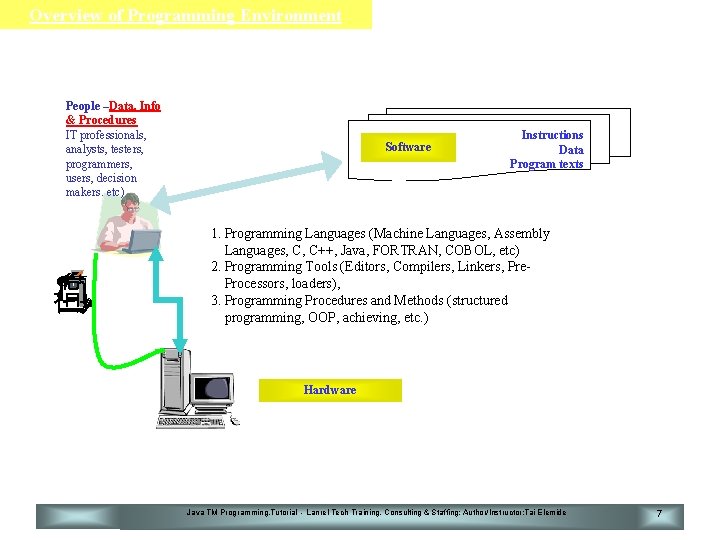
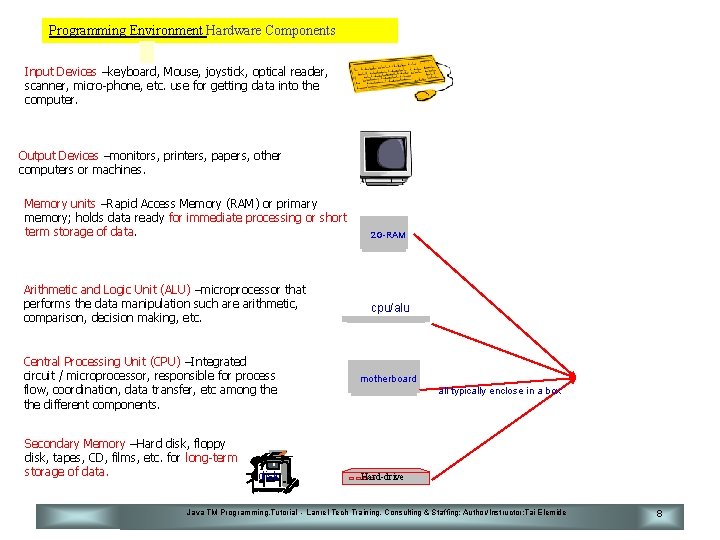
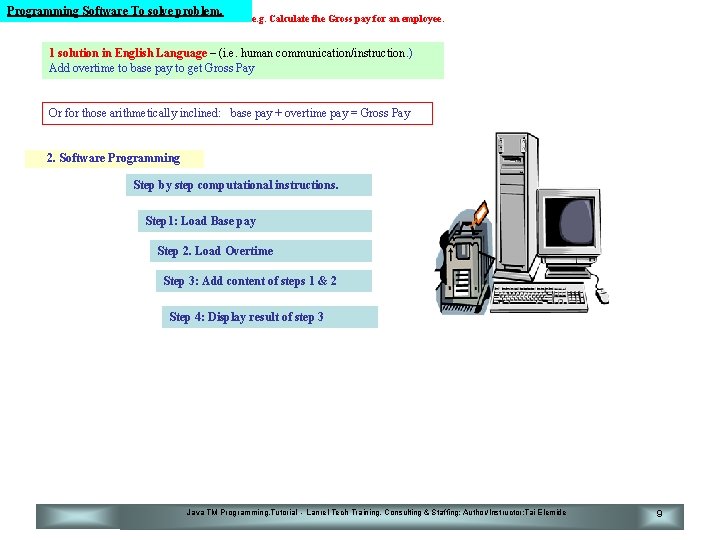
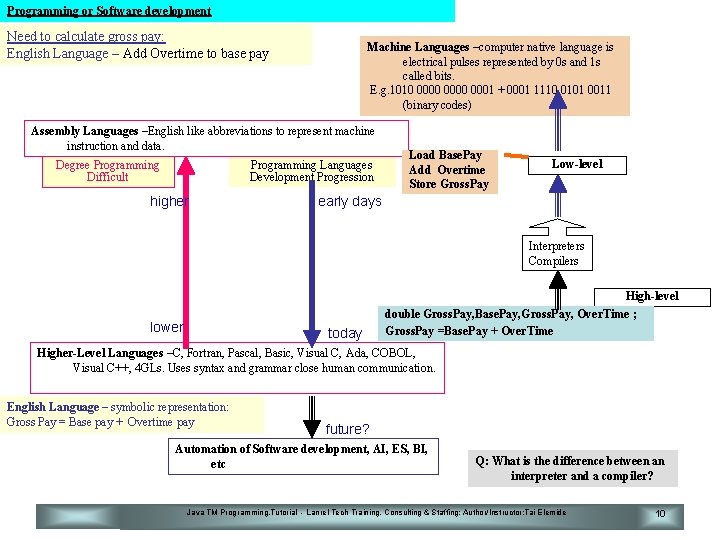
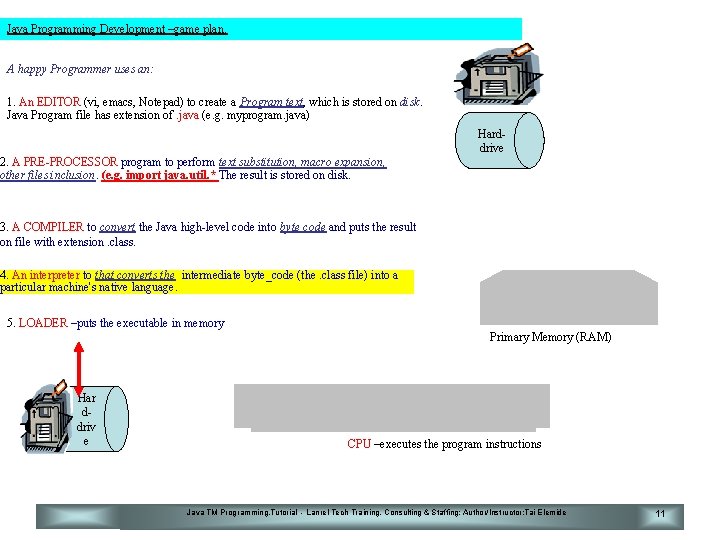
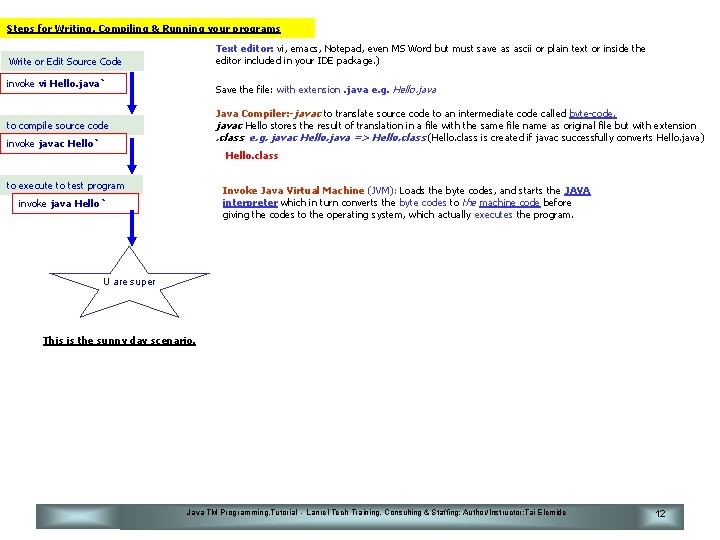
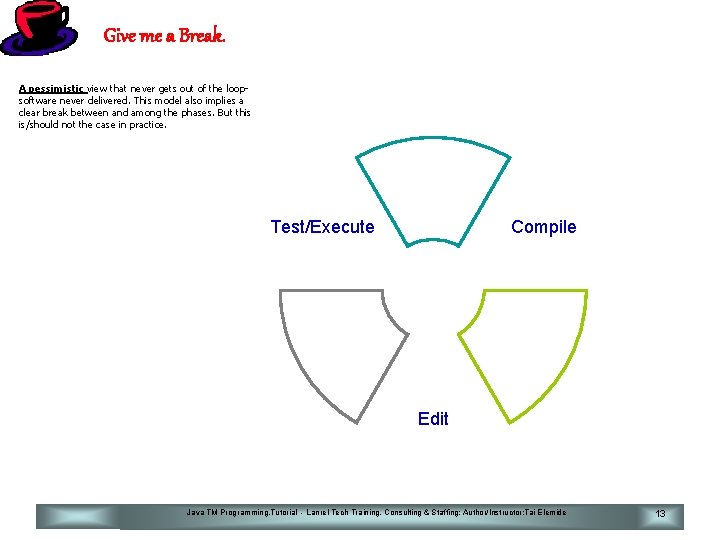
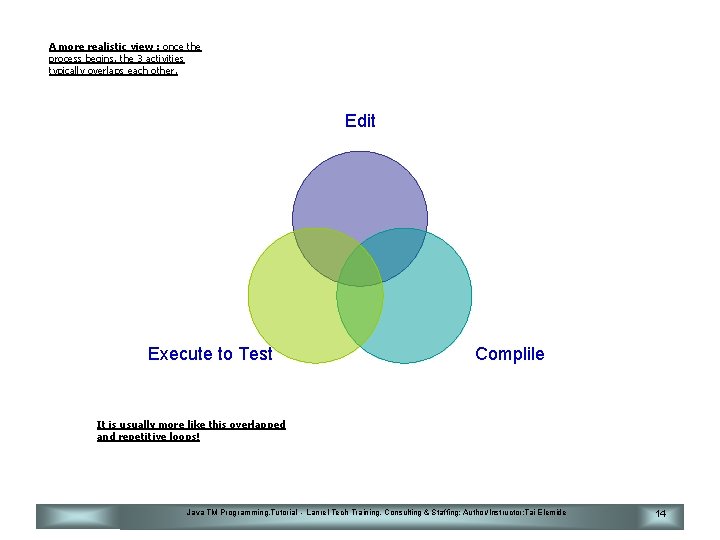
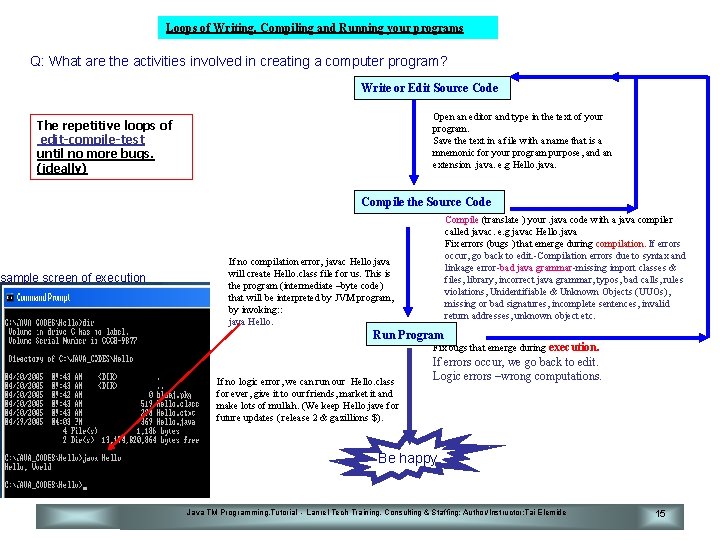
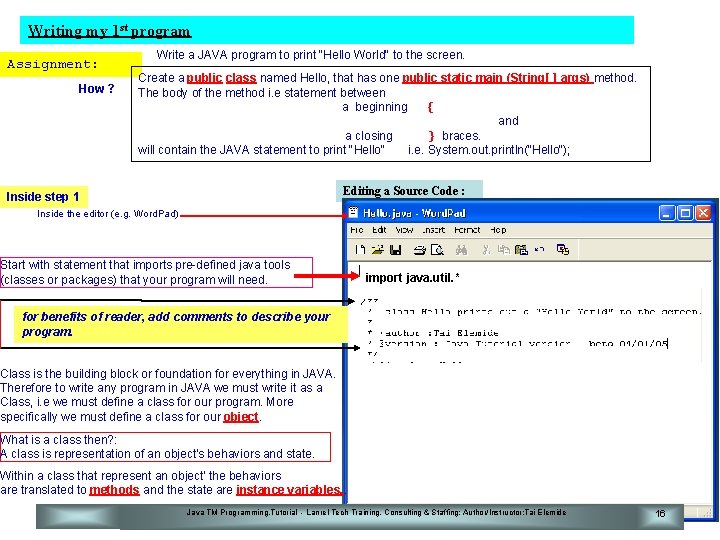
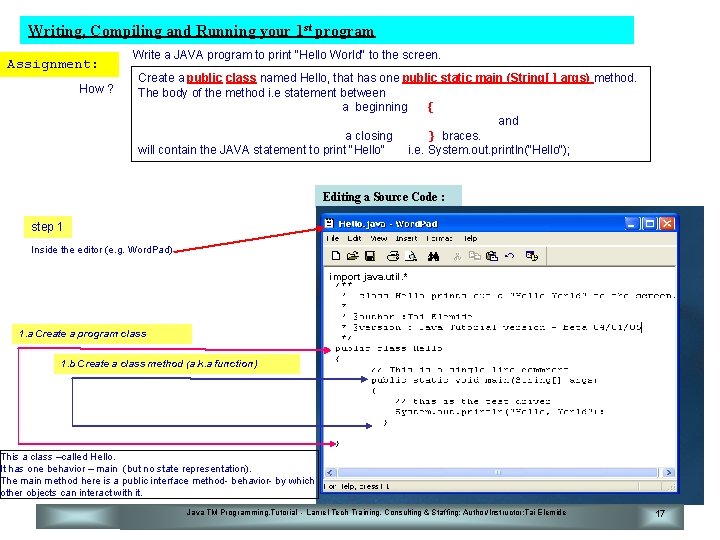
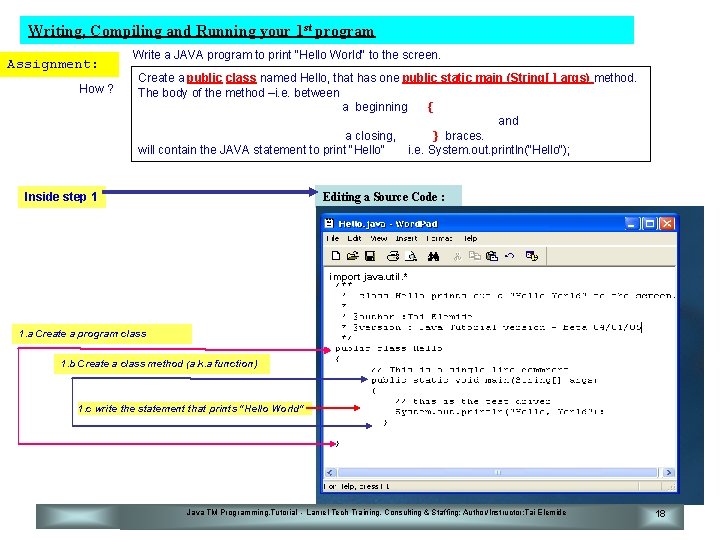
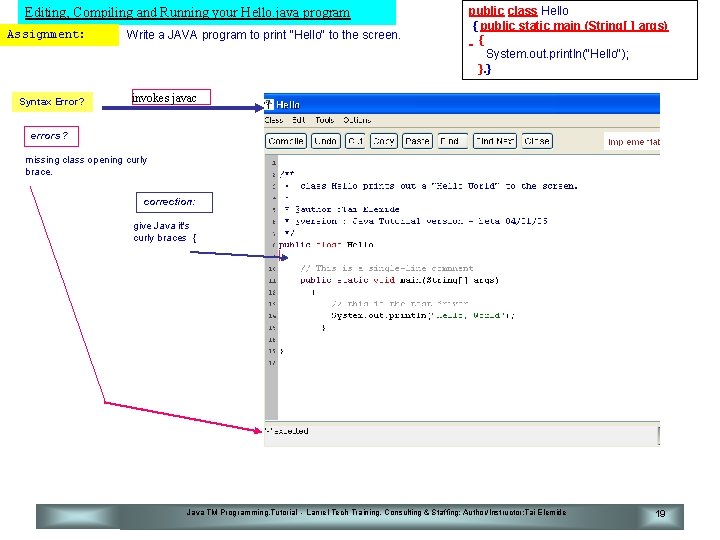
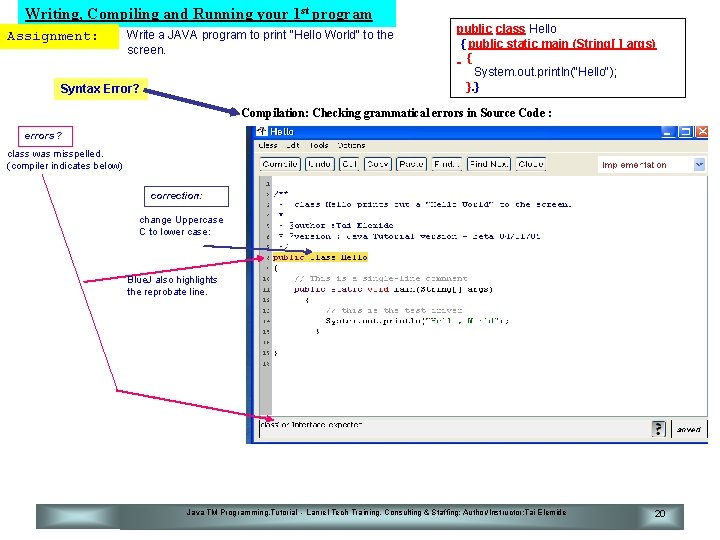
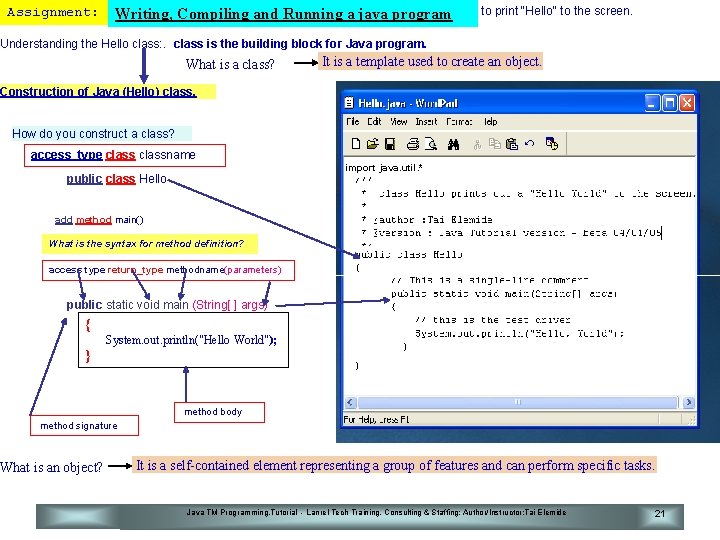
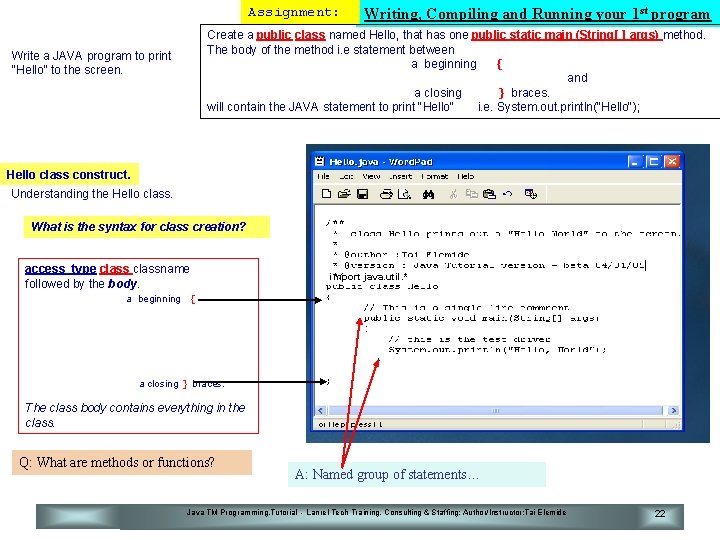
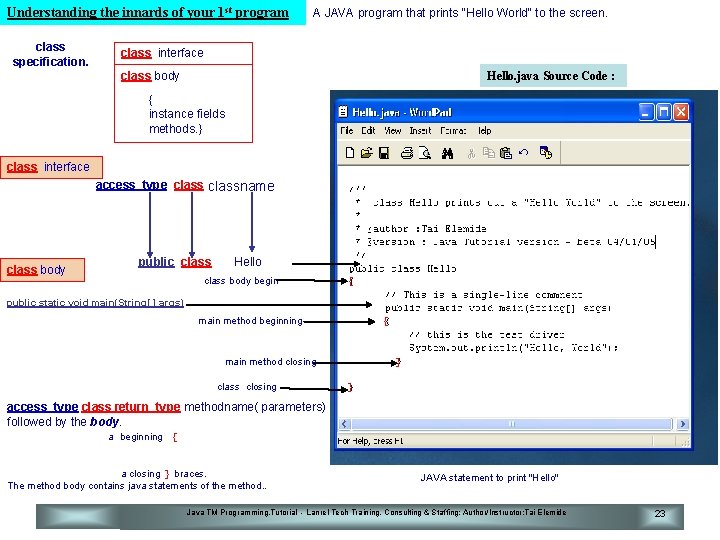
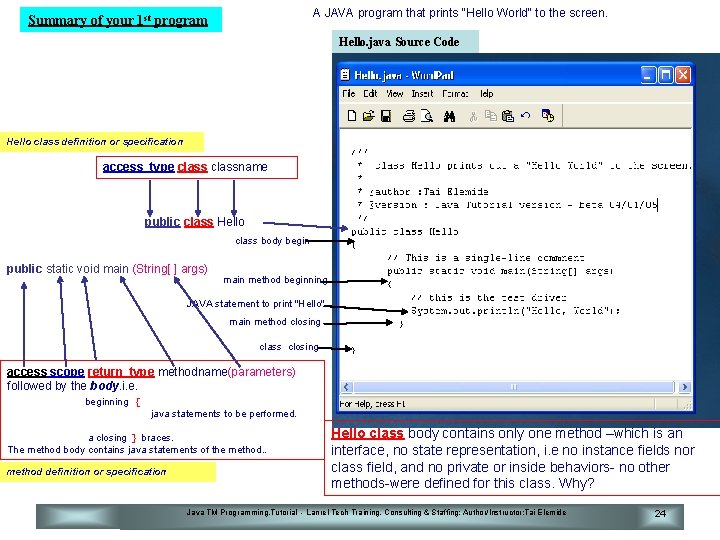
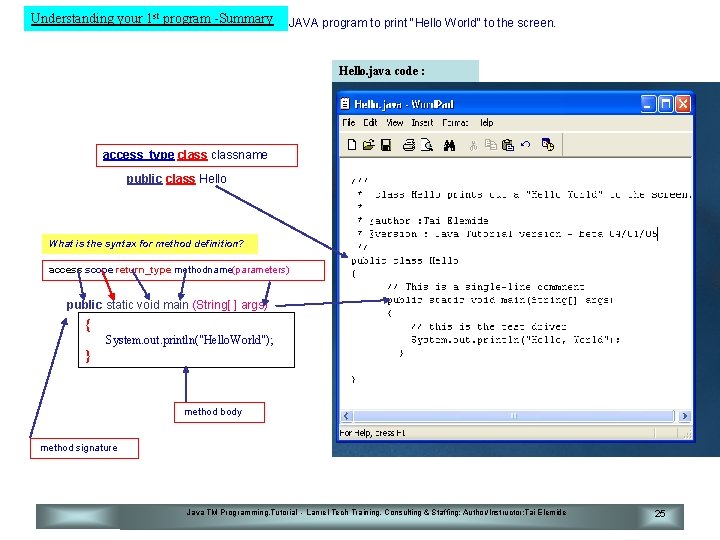
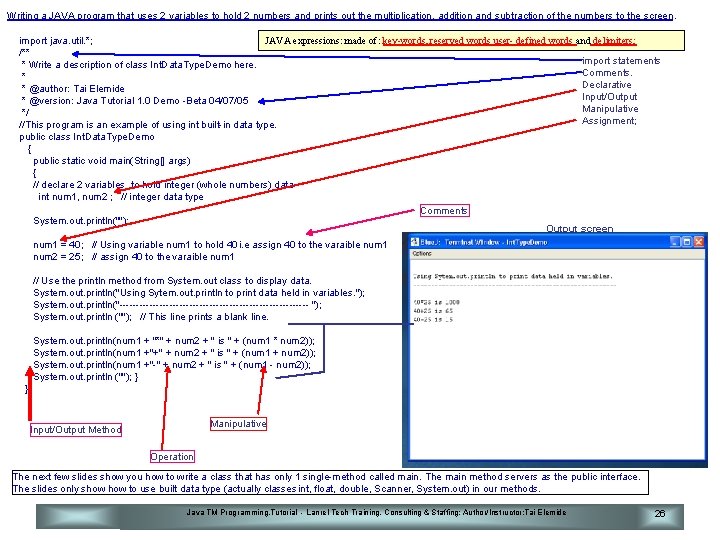
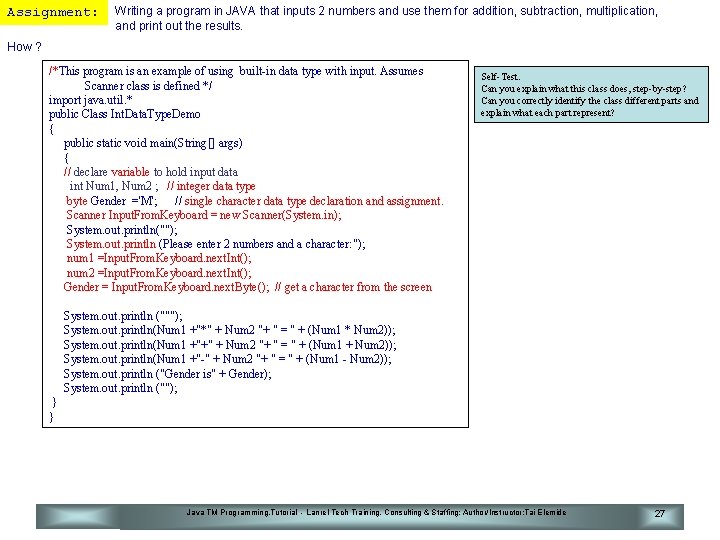
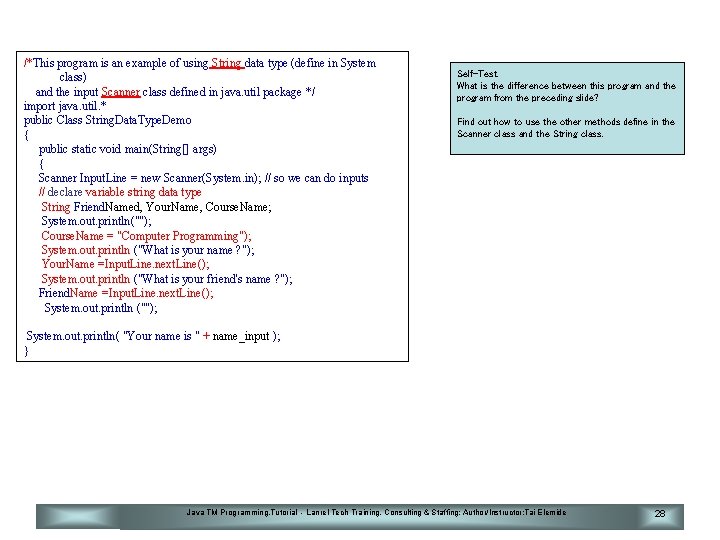
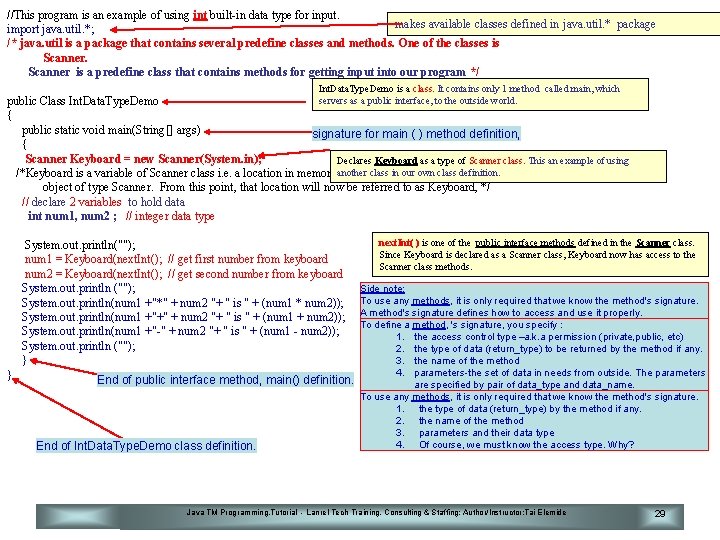
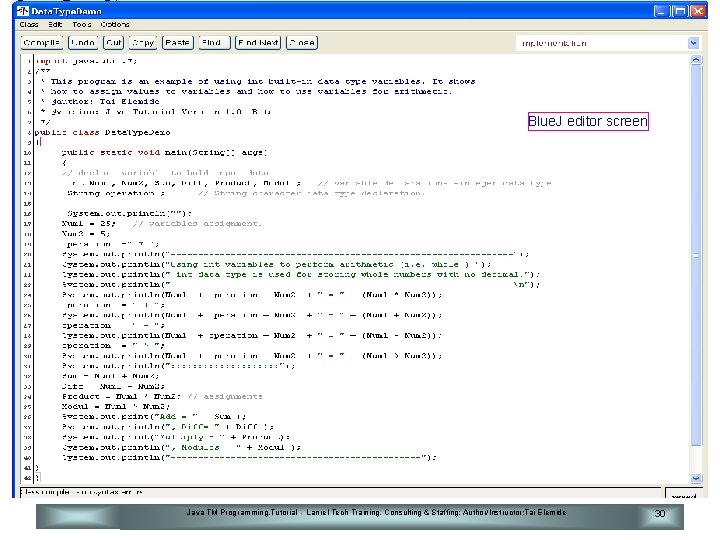
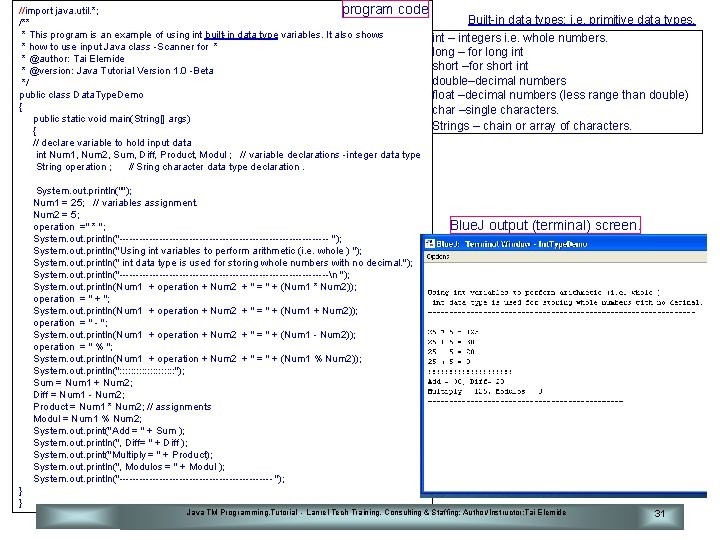
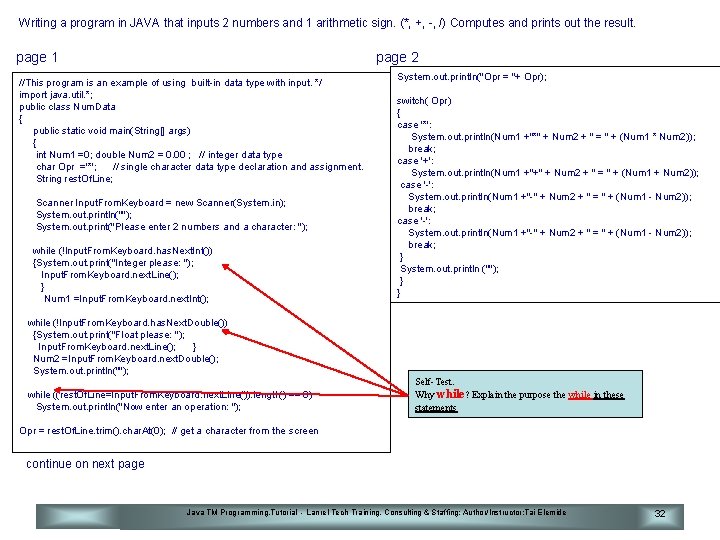
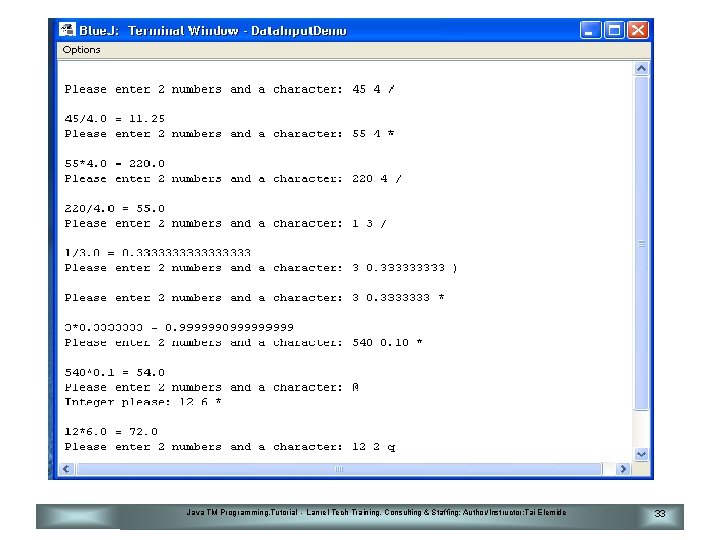
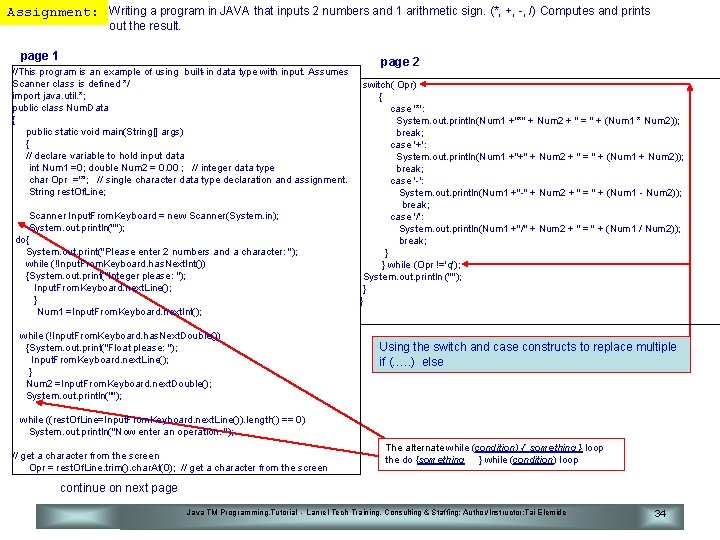
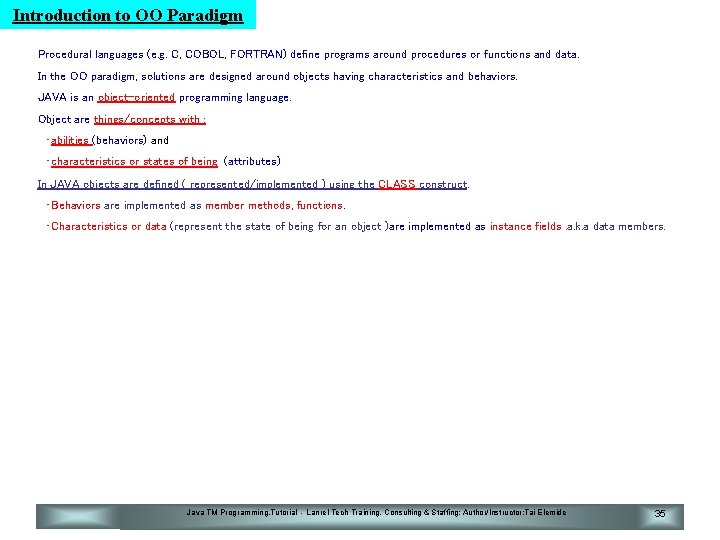
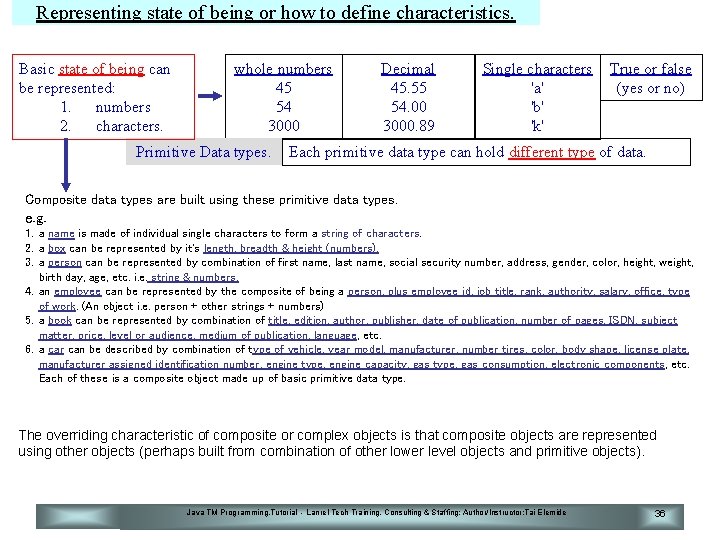
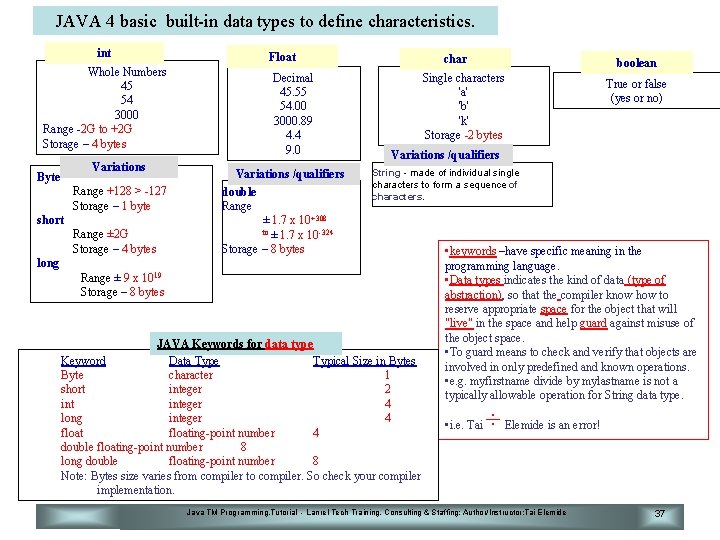
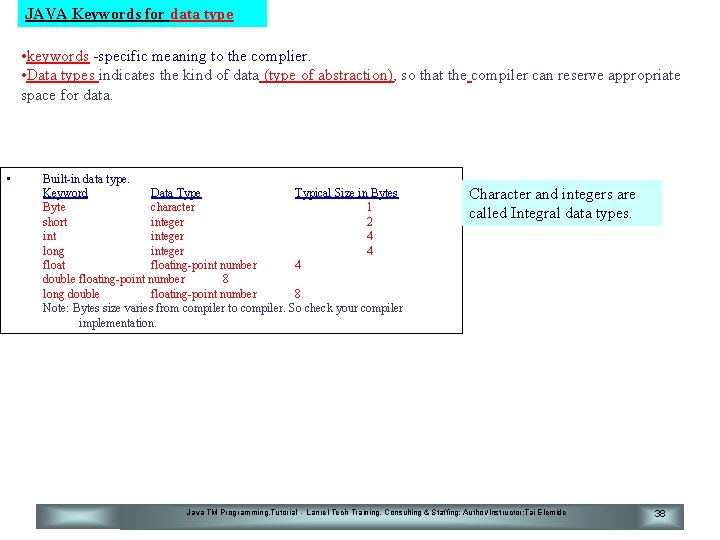
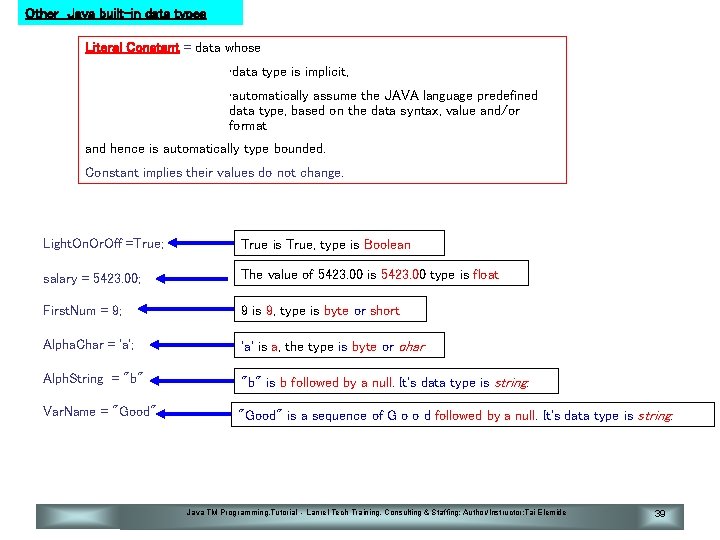
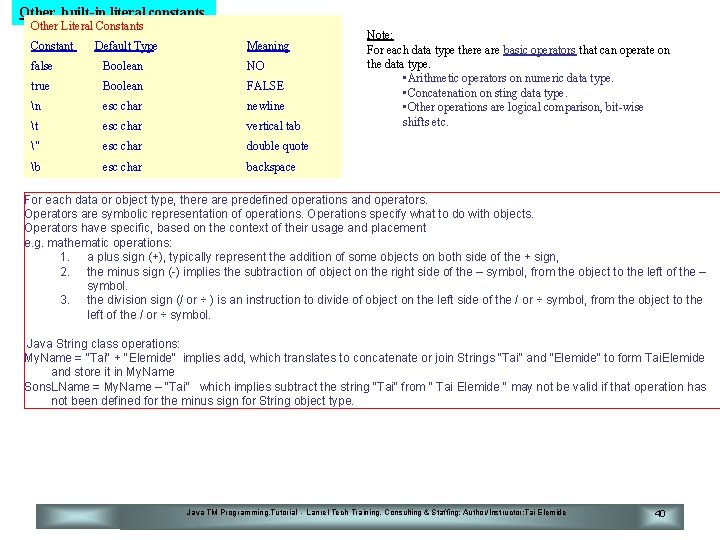
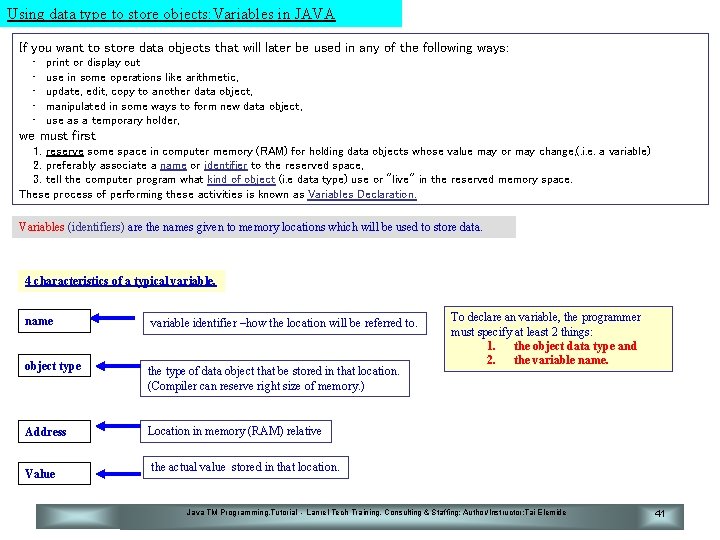
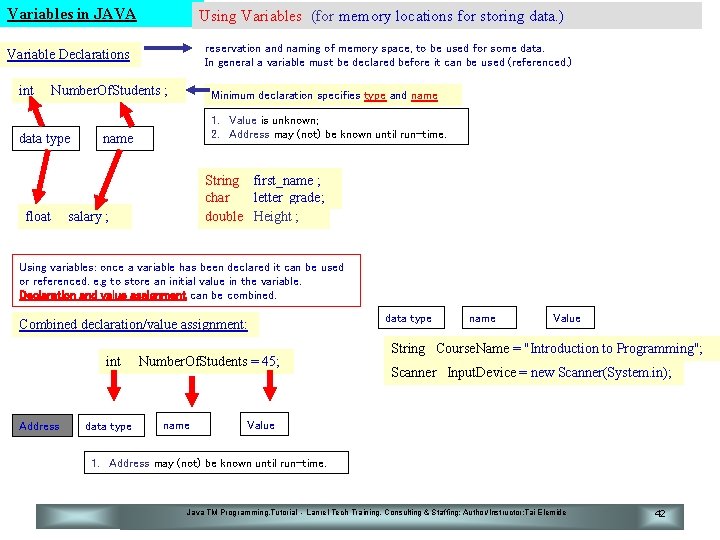
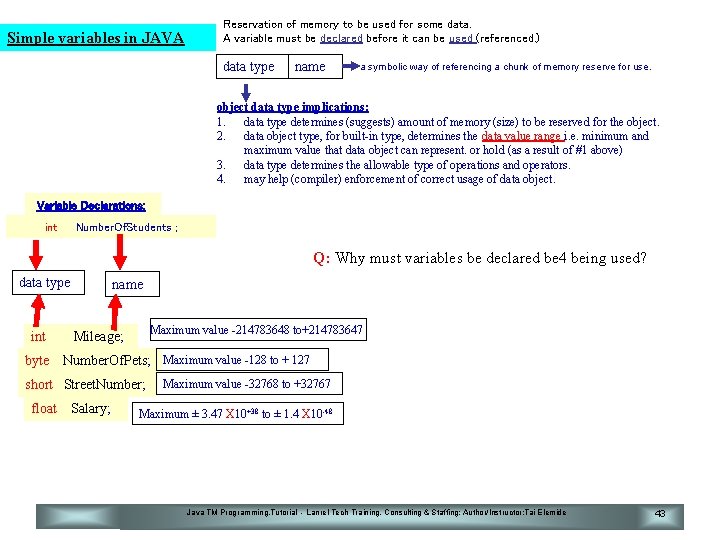
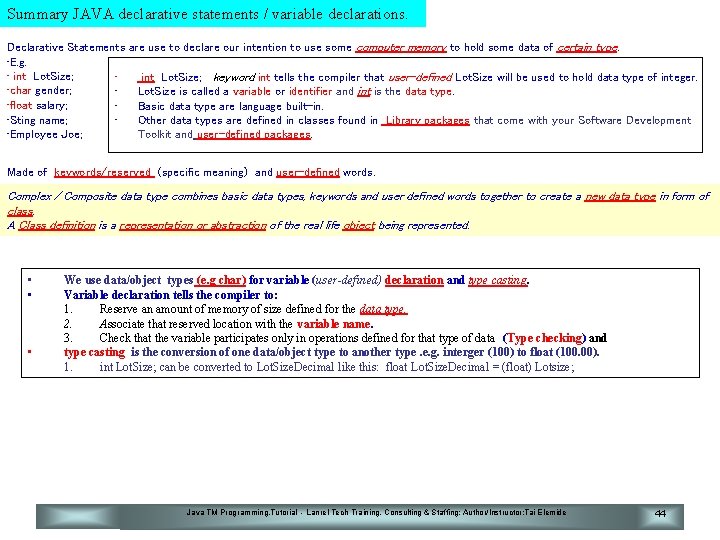
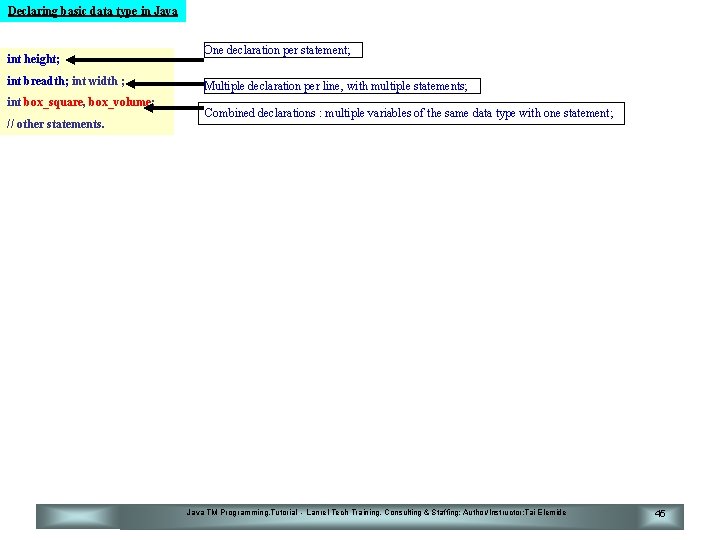
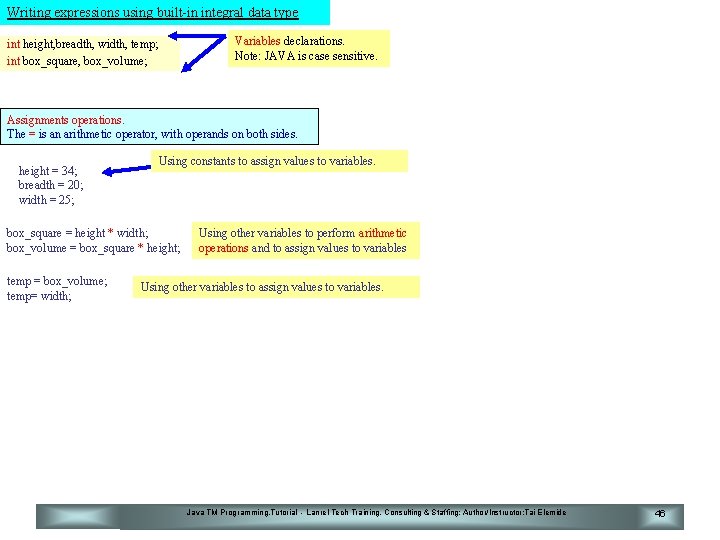
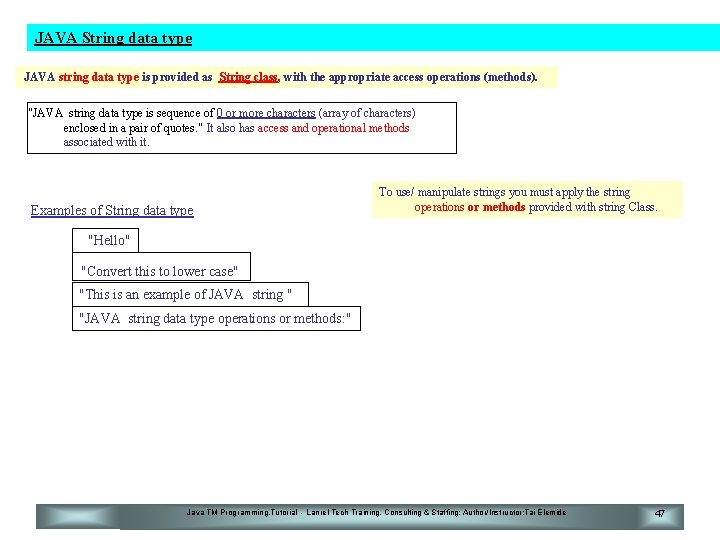
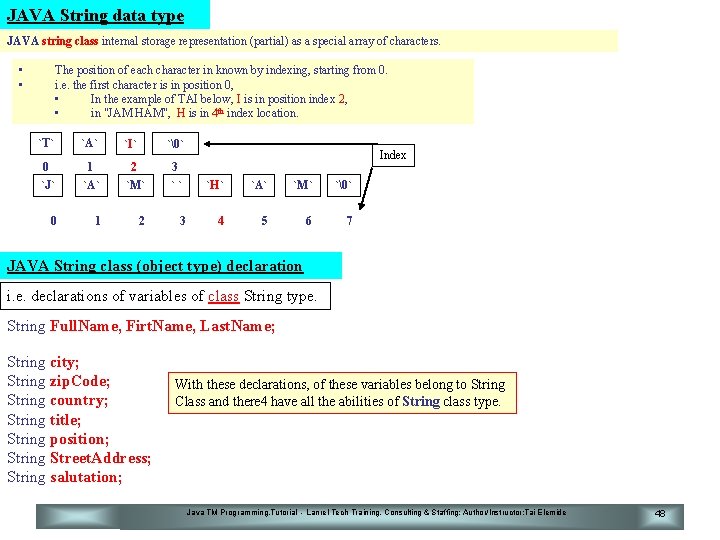
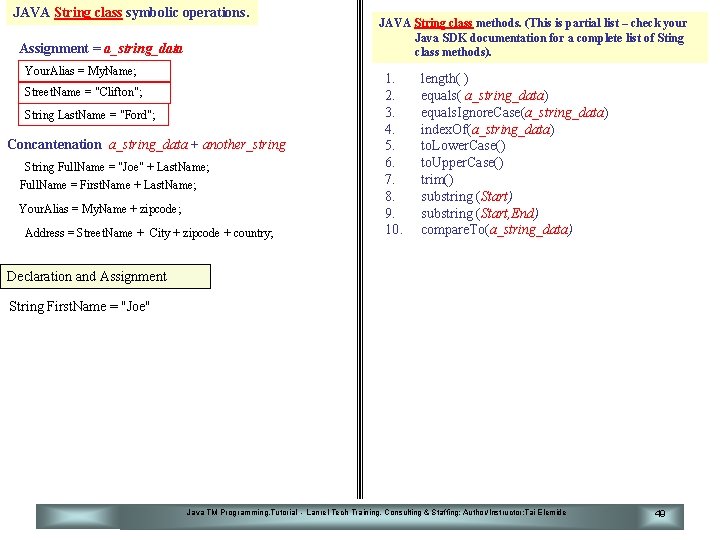
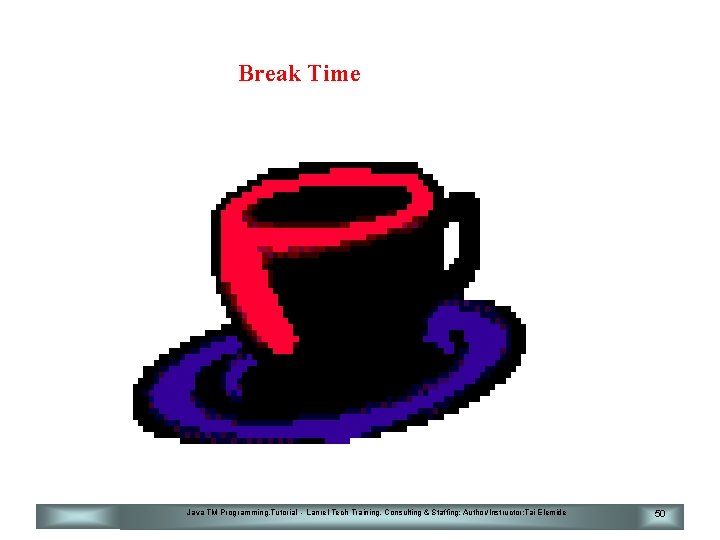
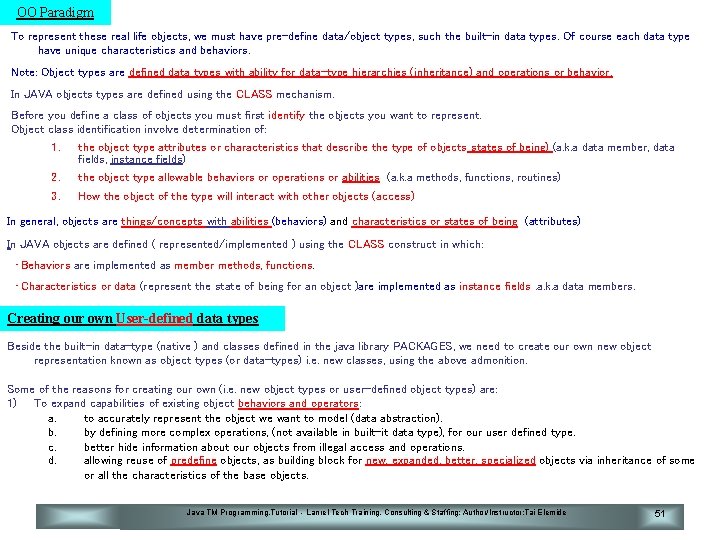
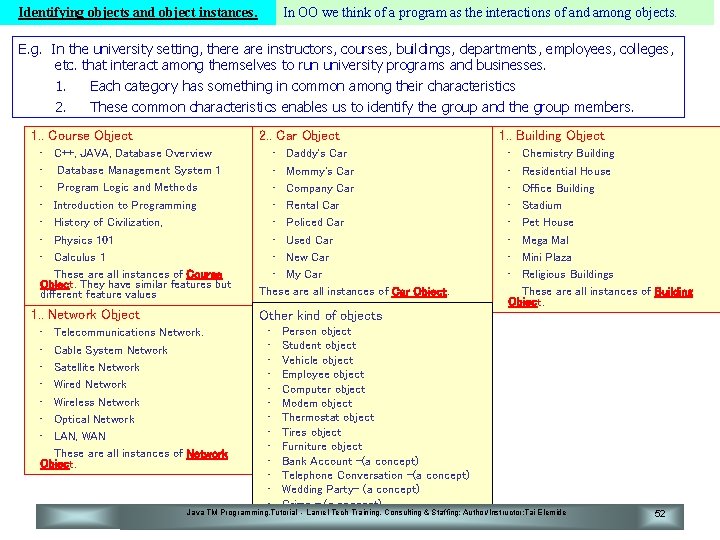
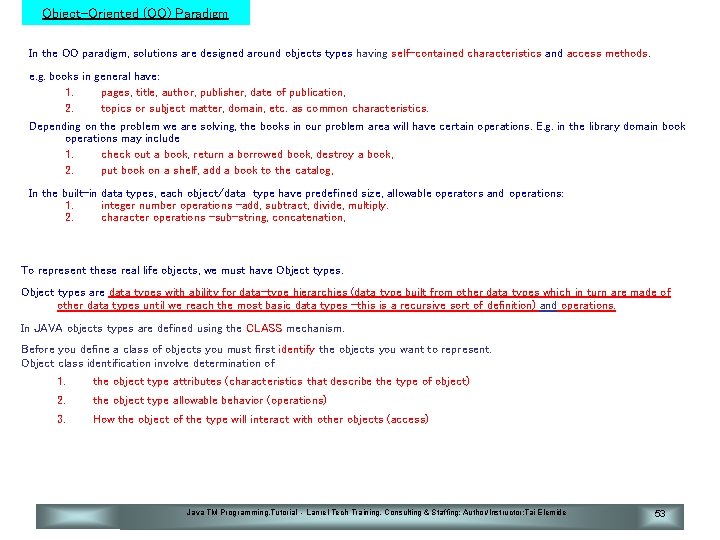
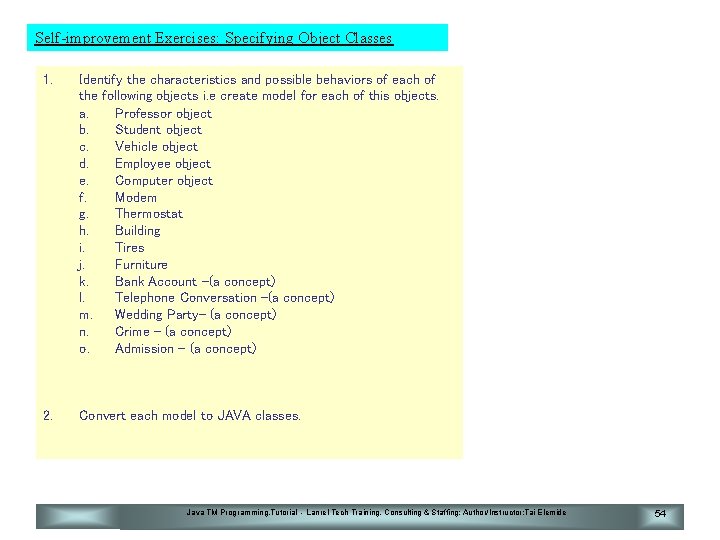
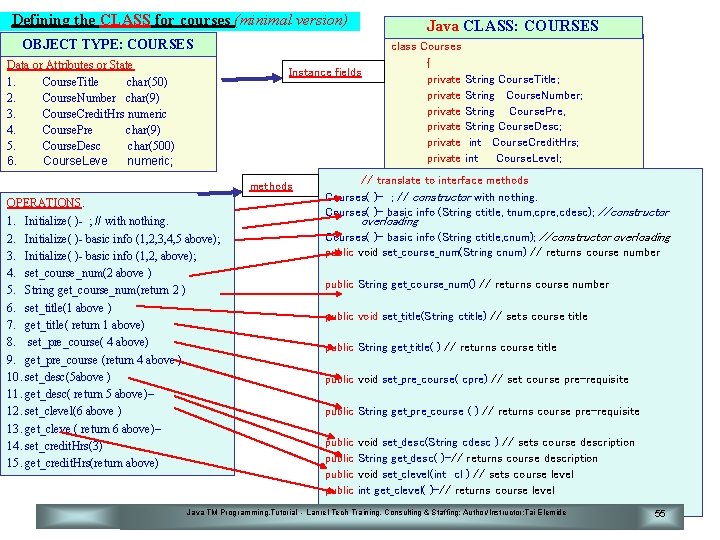
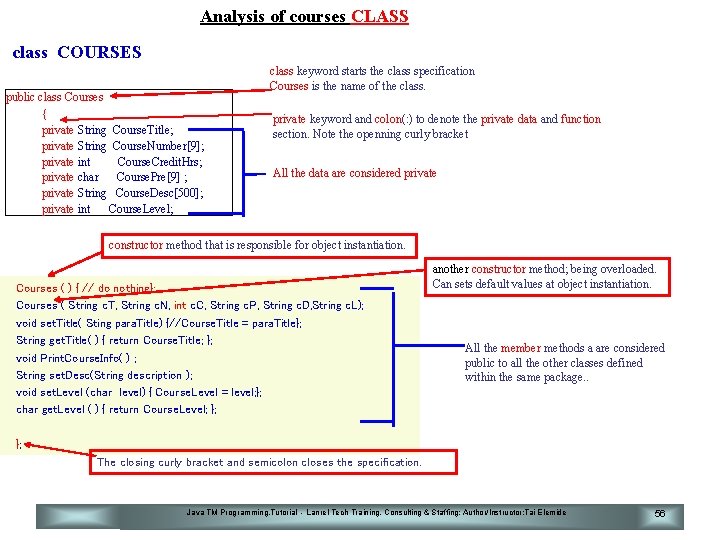
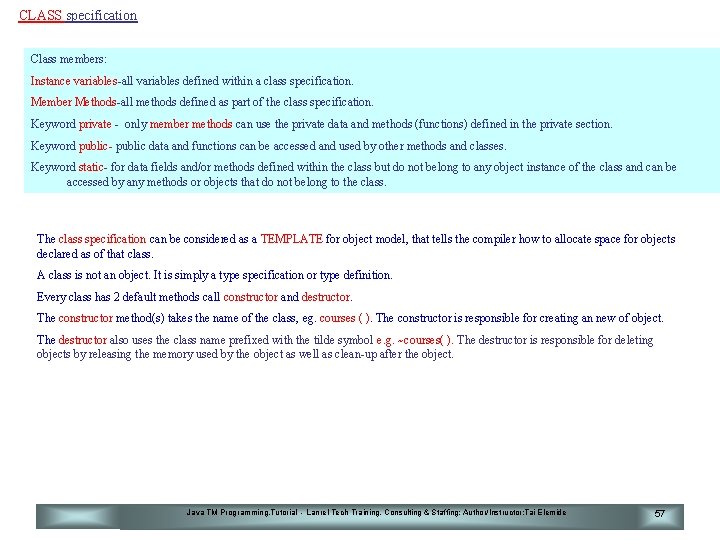
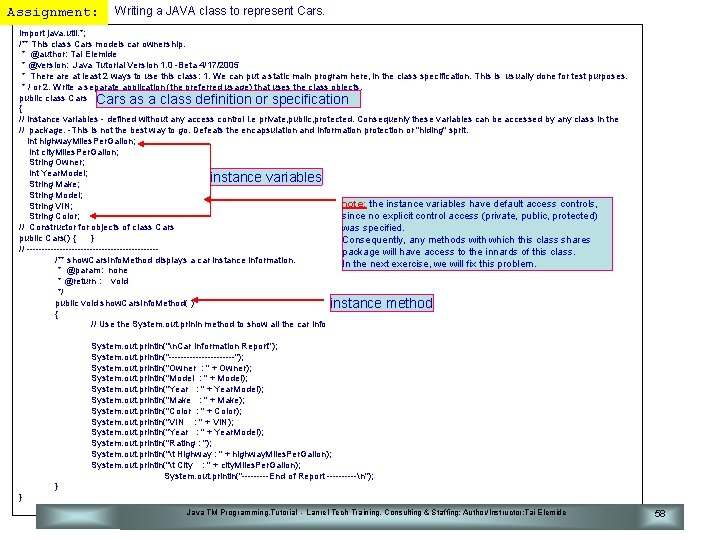
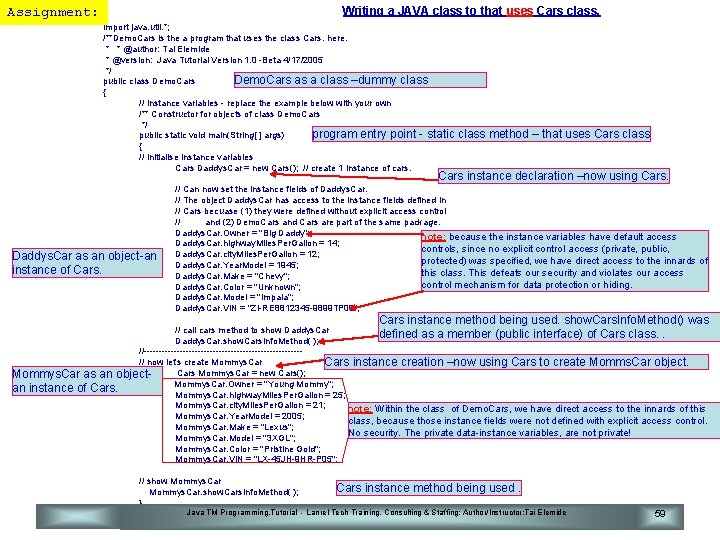
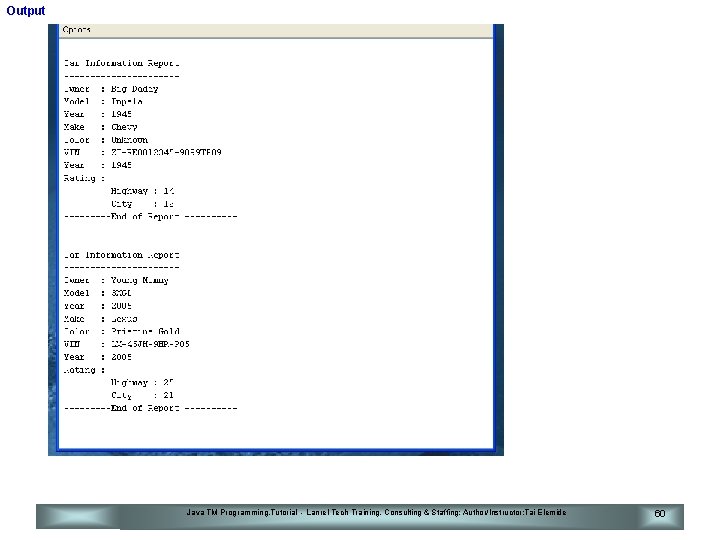
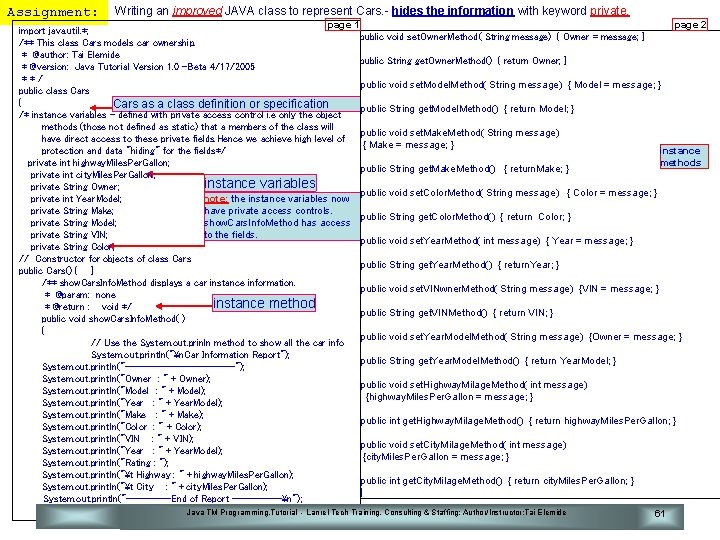
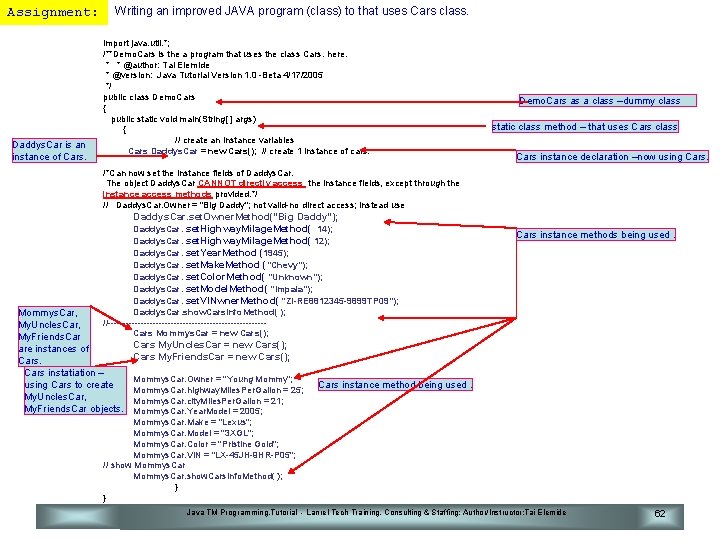
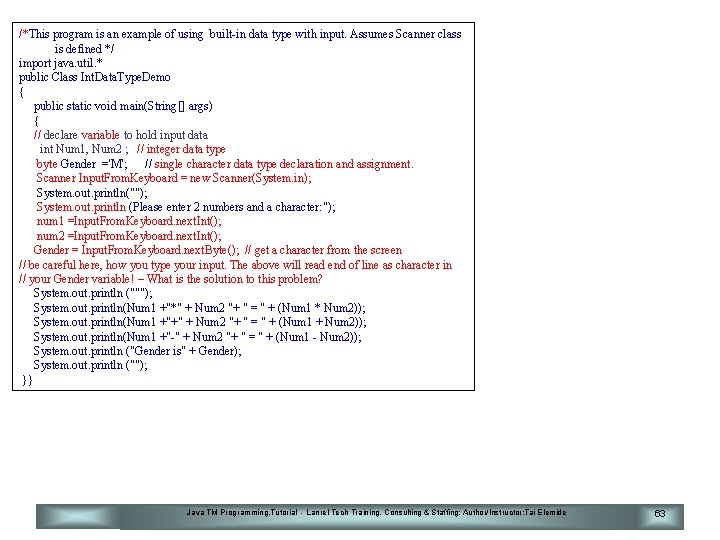
- Slides: 63
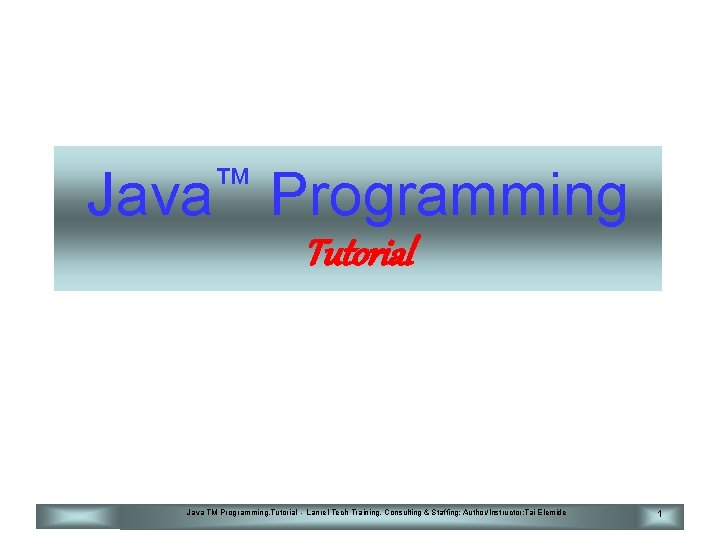
™ Java Programming Tutorial Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 1
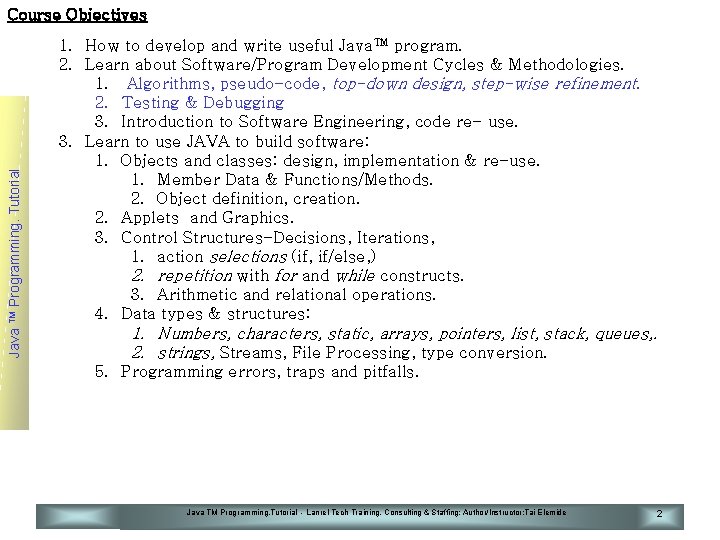
Java TM Programming. Tutorial Course Objectives 1. How to develop and write useful Java™ program. 2. Learn about Software/Program Development Cycles & Methodologies. 1. Algorithms, pseudo-code, top-down design, step-wise refinement. 2. Testing & Debugging 3. Introduction to Software Engineering, code re- use. 3. Learn to use JAVA to build software: 1. Objects and classes: design, implementation & re-use. 1. Member Data & Functions/Methods. 2. Object definition, creation. 2. Applets and Graphics. 3. Control Structures-Decisions, Iterations, 1. action selections (if, if/else, ) 2. repetition with for and while constructs. 3. Arithmetic and relational operations. 4. Data types & structures: 1. Numbers, characters, static, arrays, pointers, list, stack, queues, . 2. strings, Streams, File Processing, type conversion. 5. Programming errors, traps and pitfalls. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 2
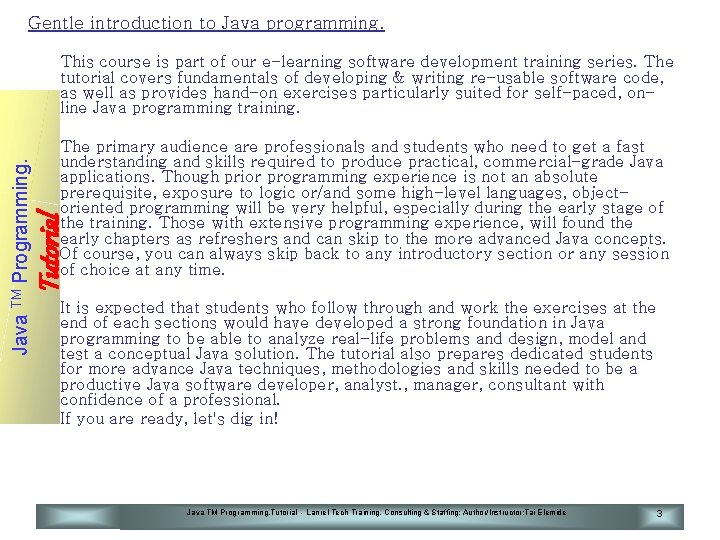
Gentle introduction to Java programming. The primary audience are professionals and students who need to get a fast understanding and skills required to produce practical, commercial-grade Java applications. Though prior programming experience is not an absolute prerequisite, exposure to logic or/and some high-level languages, objectoriented programming will be very helpful, especially during the early stage of the training. Those with extensive programming experience, will found the early chapters as refreshers and can skip to the more advanced Java concepts. Of course, you can always skip back to any introductory section or any session of choice at any time. Tutorial Java TM Programming. This course is part of our e-learning software development training series. The tutorial covers fundamentals of developing & writing re-usable software code, as well as provides hand-on exercises particularly suited for self-paced, online Java programming training. It is expected that students who follow through and work the exercises at the end of each sections would have developed a strong foundation in Java programming to be able to analyze real-life problems and design, model and test a conceptual Java solution. The tutorial also prepares dedicated students for more advance Java techniques, methodologies and skills needed to be a productive Java software developer, analyst. , manager, consultant with confidence of a professional. If you are ready, let's dig in! Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 3
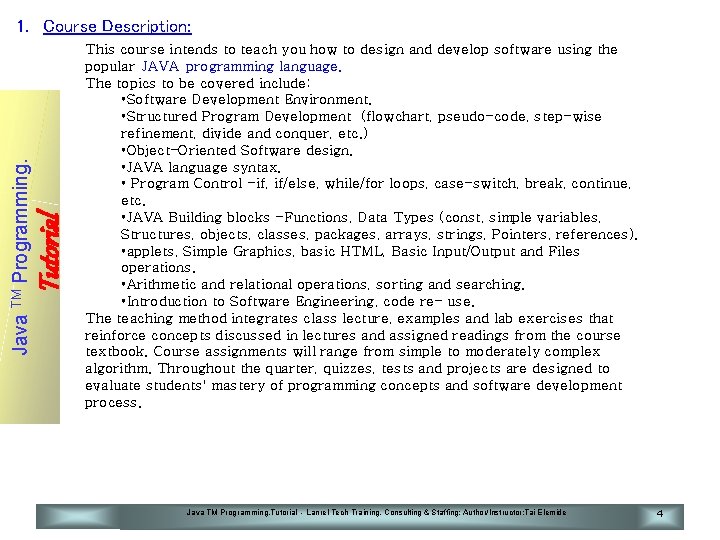
Tutorial Java TM Programming. 1. Course Description: This course intends to teach you how to design and develop software using the popular JAVA programming language. The topics to be covered include: • Software Development Environment. • Structured Program Development (flowchart, pseudo-code, step-wise refinement, divide and conquer, etc. ) • Object-Oriented Software design. • JAVA language syntax. • Program Control -if, if/else, while/for loops, case-switch, break, continue, etc. • JAVA Building blocks -Functions, Data Types (const, simple variables, Structures, objects, classes, packages, arrays, strings, Pointers, references). • applets, Simple Graphics, basic HTML, Basic Input/Output and Files operations. • Arithmetic and relational operations, sorting and searching. • Introduction to Software Engineering, code re- use. The teaching method integrates class lecture, examples and lab exercises that reinforce concepts discussed in lectures and assigned readings from the course textbook. Course assignments will range from simple to moderately complex algorithm. Throughout the quarter, quizzes, tests and projects are designed to evaluate students' mastery of programming concepts and software development process. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 4
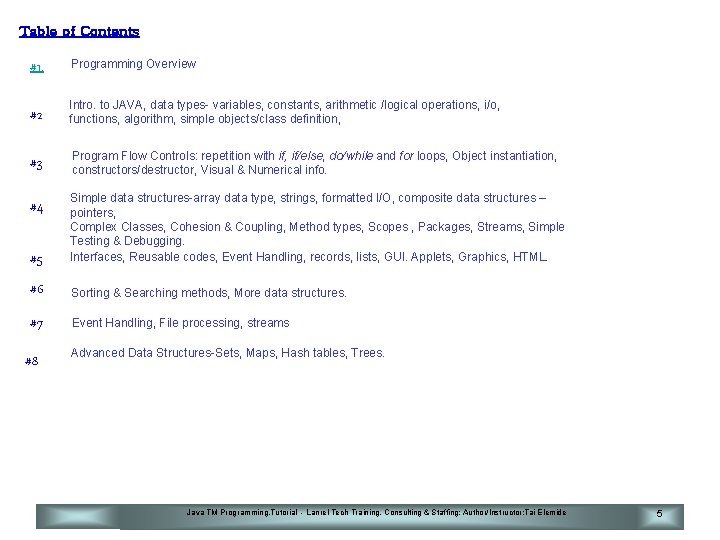
Table of Contents #1. Programming Overview #2 Intro. to JAVA, data types- variables, constants, arithmetic /logical operations, i/o, functions, algorithm, simple objects/class definition, #3 Program Flow Controls: repetition with if, if/else, do/while and for loops, Object instantiation, constructors/destructor, Visual & Numerical info. #5 Simple data structures-array data type, strings, formatted I/O, composite data structures – pointers, Complex Classes, Cohesion & Coupling, Method types, Scopes , Packages, Streams, Simple Testing & Debugging. Interfaces, Reusable codes, Event Handling, records, lists, GUI. Applets, Graphics, HTML. #6 Sorting & Searching methods, More data structures. #7 Event Handling, File processing, streams #4 #8 Advanced Data Structures-Sets, Maps, Hash tables, Trees. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 5
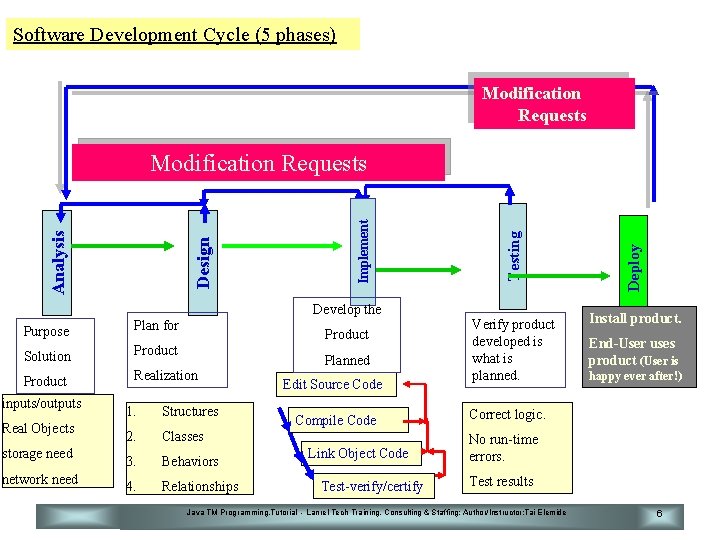
Software Development Cycle (5 phases) Modification Requests Develop the Purpose Plan for Solution Product Realization inputs/outputs Real Objects storage need network need Product Planned 1. Structures 2. Classes 3. Behaviors 4. Relationships Edit Source Code Compile Code Link Object Code Test-verify/certify Verify product developed is what is planned. Deploy Testing Implement Design Analysis Modification Requests Install product. End-User uses product (User is happy ever after!) Correct logic. No run-time errors. Test results Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 6
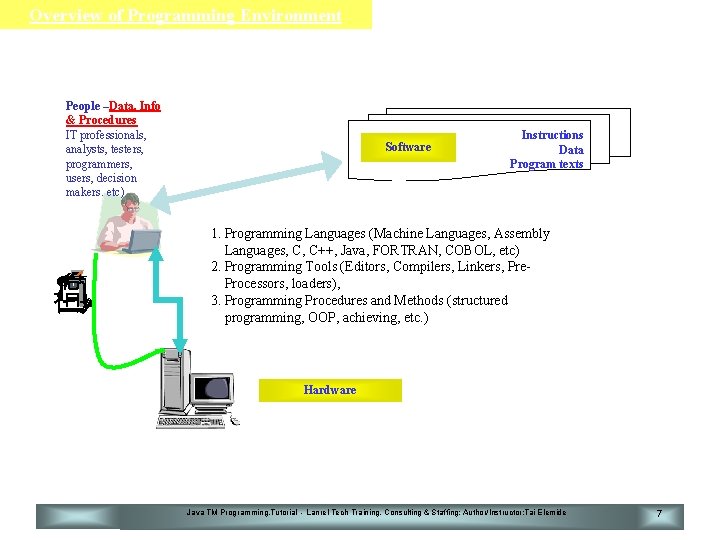
Overview of Programming Environment People –Data, Info & Procedures IT professionals, analysts, testers, programmers, users, decision makers. etc) Software Instructions Data Program texts 1. Programming Languages (Machine Languages, Assembly Languages, C, C++, Java, FORTRAN, COBOL, etc) 2. Programming Tools (Editors, Compilers, Linkers, Pre. Processors, loaders), 3. Programming Procedures and Methods (structured programming, OOP, achieving, etc. ) Hardware Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 7
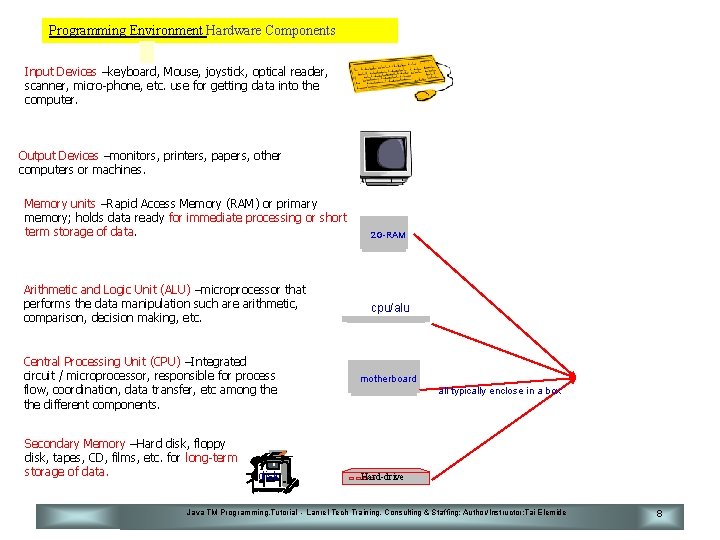
Programming Environment Hardware Components Input Devices –keyboard, Mouse, joystick, optical reader, scanner, micro-phone, etc. use for getting data into the computer. Output Devices –monitors, printers, papers, other computers or machines. Memory units –Rapid Access Memory (RAM) or primary memory; holds data ready for immediate processing or short term storage of data. Arithmetic and Logic Unit (ALU) –microprocessor that performs the data manipulation such are arithmetic, comparison, decision making, etc. Central Processing Unit (CPU) –Integrated circuit / microprocessor, responsible for process flow, coordination, data transfer, etc among the different components. Secondary Memory –Hard disk, floppy disk, tapes, CD, films, etc. for long-term storage of data. disk 2 G-RAM cpu/alu motherboard all typically enclose in a box Hard-drive Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 8
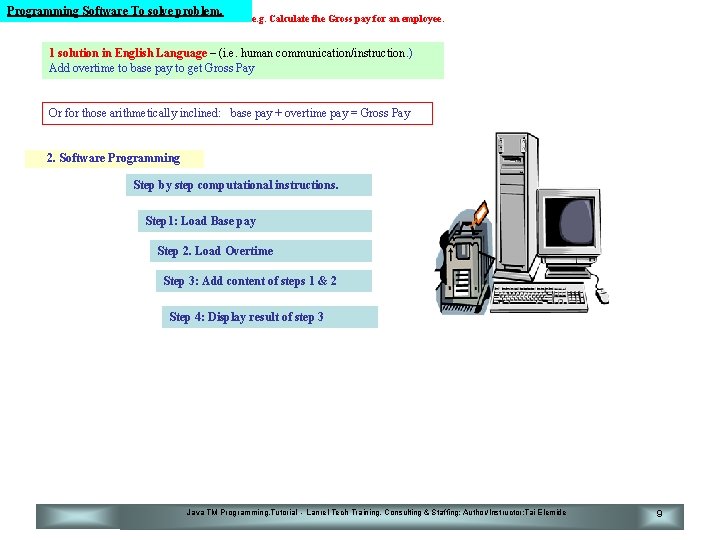
Programming Software To solve problem. e. g. Calculate the Gross pay for an employee. 1 solution in English Language – (i. e. human communication/instruction. ) Add overtime to base pay to get Gross Pay Or for those arithmetically inclined: base pay + overtime pay = Gross Pay 2. Software Programming Step by step computational instructions. Step 1: Load Base pay Step 2. Load Overtime Step 3: Add content of steps 1 & 2 Step 4: Display result of step 3 Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 9
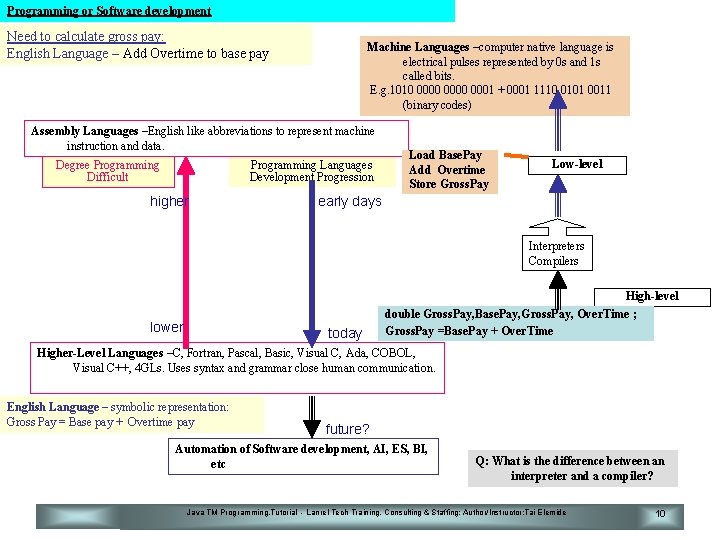
Programming or Software development Need to calculate gross pay: English Language – Add Overtime to base pay Machine Languages –computer native language is electrical pulses represented by 0 s and 1 s called bits. E. g. 1010 0000 0001 + 0001 1110 0101 0011 (binary codes) Assembly Languages –English like abbreviations to represent machine instruction and data. Degree Programming Difficult Programming Languages Development Progression higher Load Base. Pay Add Overtime Store Gross. Pay Low-level early days Interpreters Compilers lower today High-level double Gross. Pay, Base. Pay, Gross. Pay, Over. Time ; Gross. Pay =Base. Pay + Over. Time Higher-Level Languages –C, Fortran, Pascal, Basic, Visual C, Ada, COBOL, Visual C++, 4 GLs. Uses syntax and grammar close human communication. English Language – symbolic representation: Gross Pay = Base pay + Overtime pay future? Automation of Software development, AI, ES, BI, etc Q: What is the difference between an interpreter and a compiler? Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 10
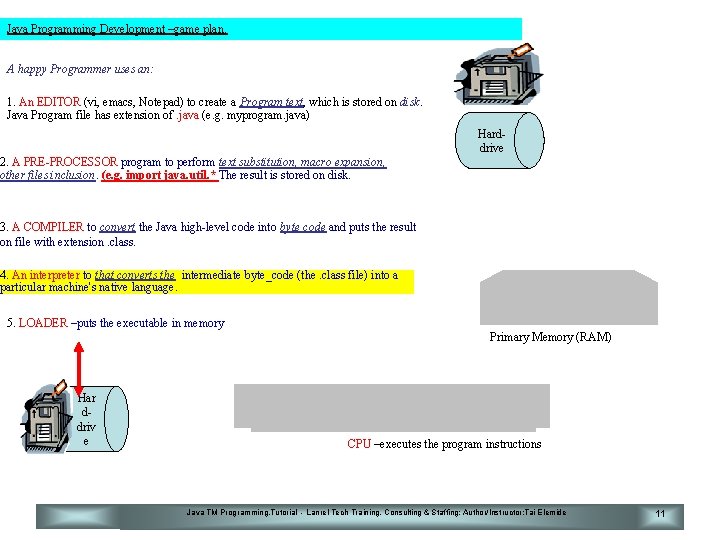
Java Programming Development –game plan. A happy Programmer uses an: 1. An EDITOR (vi, emacs, Notepad) to create a Program text, which is stored on disk. Java Program file has extension of. java (e. g. myprogram. java) Harddrive 2. A PRE-PROCESSOR program to perform text substitution, macro expansion, other files inclusion. (e. g. import java. util. * The result is stored on disk. 3. A COMPILER to convert the Java high-level code into byte code and puts the result on file with extension. class. 4. An interpreter to that converts the intermediate byte_code (the. class file) into a particular machine's native language. 5. LOADER –puts the executable in memory Primary Memory (RAM) Har ddriv e CPU –executes the program instructions Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 11
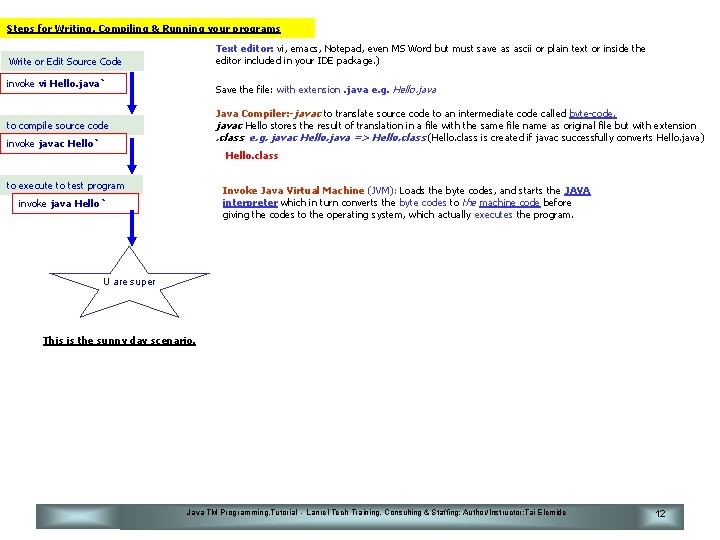
Steps for Writing, Compiling & Running your programs Text editor: vi, emacs, Notepad, even MS Word but must save as ascii or plain text or inside the editor included in your IDE package. ) Write or Edit Source Code invoke vi Hello. java` Save the file: with extension. java e. g. Hello. java Java Compiler: -javac to translate source code to an intermediate code called byte-code. javac Hello stores the result of translation in a file with the same file name as original file but with extension. class e. g. javac Hello. java => Hello. class (Hello. class is created if javac successfully converts Hello. java) to compile source code invoke javac Hello` Hello. class to execute to test program Invoke Java Virtual Machine (JVM): Loads the byte codes, and starts the JAVA interpreter which in turn converts the byte codes to the machine code before giving the codes to the operating system, which actually executes the program. invoke java Hello` U are super This is the sunny day scenario. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 12
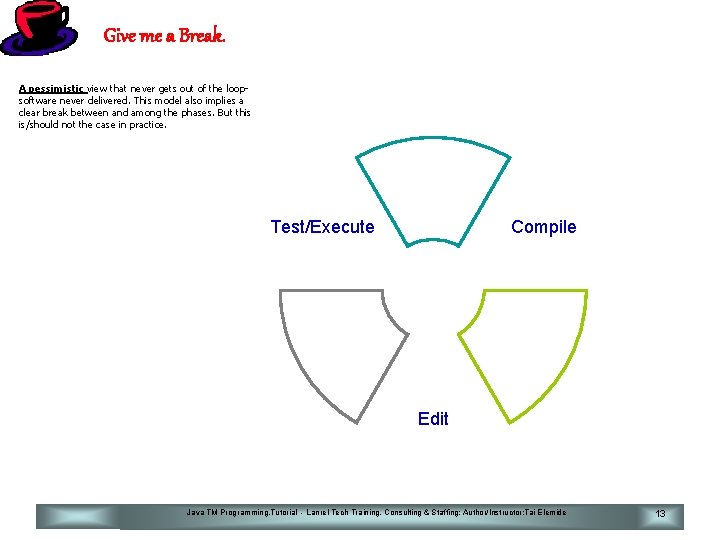
Give me a Break. A pessimistic view that never gets out of the loopsoftware never delivered. This model also implies a clear break between and among the phases. But this is/should not the case in practice. Compile Test/Execute Edit Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 13
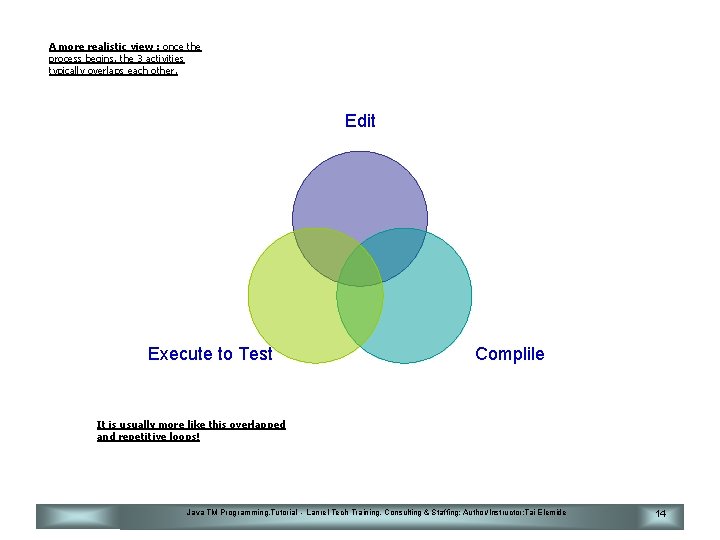
A more realistic view : once the process begins, the 3 activities typically overlaps each other. Edit Execute to Test Complile It is usually more like this overlapped and repetitive loops! Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 14
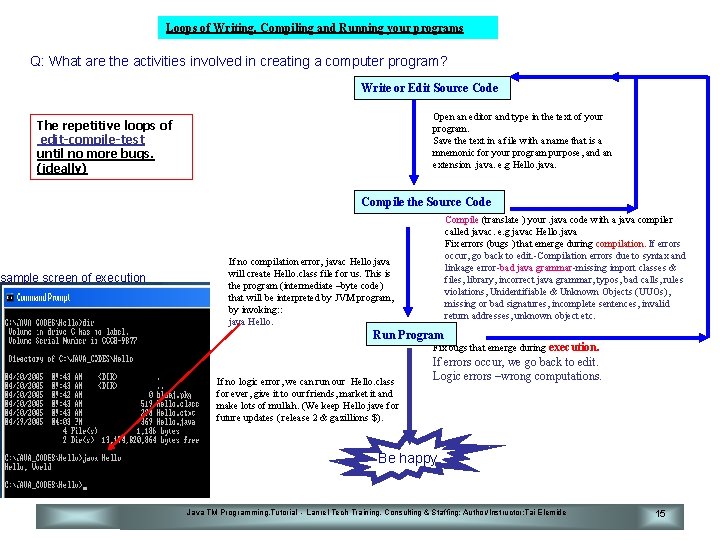
Loops of Writing, Compiling and Running your programs Q: What are the activities involved in creating a computer program? Write or Edit Source Code Open an editor and type in the text of your program. Save the text in a file with a name that is a mnemonic for your program purpose, and an extension. java. e. g Hello. java. The repetitive loops of edit-compile-test until no more bugs. (ideally) sample screen of execution Compile the Source Code Compile (translate ) your. java code with a java compiler called javac. e. g javac Hello. java Fix errors (bugs ) that emerge during compilation. If errors occur, go back to edit. -Compilation errors due to syntax and linkage error-bad java grammar-missing import classes & files, library, incorrect java grammar, typos, bad calls, rules violations, Unidentifiable & Unknown Objects (UUOs), missing or bad signatures, incomplete sentences, invalid return addresses, unknown object etc. If no compilation error, javac Hello. java will create Hello. class file for us. This is the program (intermediate –byte code) that will be interpreted by JVM program, by invoking: : java Hello. Run Program Fix bugs that emerge during execution. If no logic error, we can run our Hello. class for ever, give it to our friends, market it and make lots of mullah. (We keep Hello. jave for future updates ( release 2 & gazillions $). If errors occur, we go back to edit. Logic errors –wrong computations. Be happy Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 15
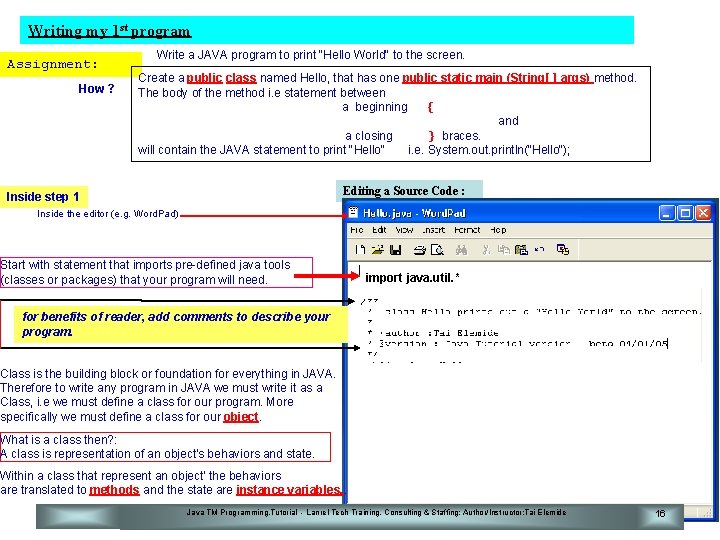
Writing my 1 st program Assignment: How ? Write a JAVA program to print "Hello World" to the screen. Create a public class named Hello, that has one public static main (String[ ] args) method. The body of the method i. e statement between a beginning { and a closing } braces. will contain the JAVA statement to print "Hello" i. e. System. out. println("Hello"); Editing a Source Code : Inside step 1 Inside the editor (e. g. Word. Pad) Start with statement that imports pre-defined java tools (classes or packages) that your program will need. import java. util. * for benefits of reader, add comments to describe your program. Class is the building block or foundation for everything in JAVA. Therefore to write any program in JAVA we must write it as a Class, i. e we must define a class for our program. More specifically we must define a class for our object. What is a class then? : A class is representation of an object's behaviors and state. Within a class that represent an object' the behaviors are translated to methods and the state are instance variables. . Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 16
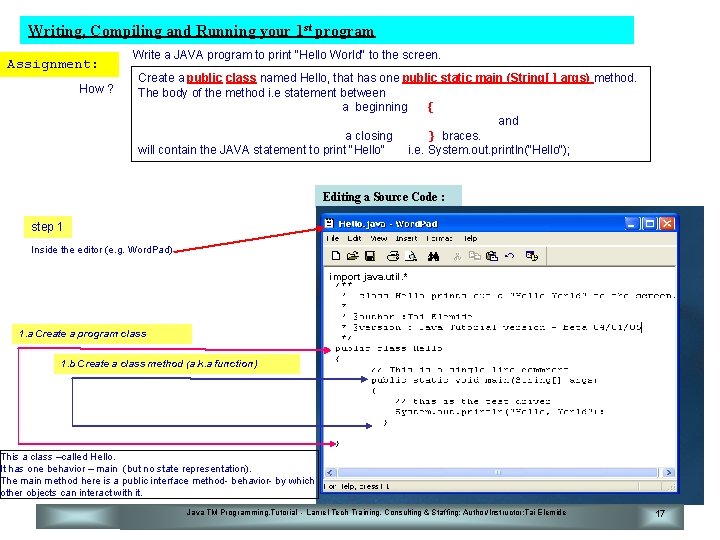
Writing, Compiling and Running your 1 st program Assignment: How ? Write a JAVA program to print "Hello World" to the screen. Create a public class named Hello, that has one public static main (String[ ] args) method. The body of the method i. e statement between a beginning { and a closing } braces. will contain the JAVA statement to print "Hello" i. e. System. out. println("Hello"); Editing a Source Code : step 1 Inside the editor (e. g. Word. Pad) import java. util. * 1. a Create a program class 1. b Create a class method (a. k. a function) This a class –called Hello. It has one behavior – main (but no state representation). The main method here is a public interface method- behavior- by which other objects can interact with it. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 17
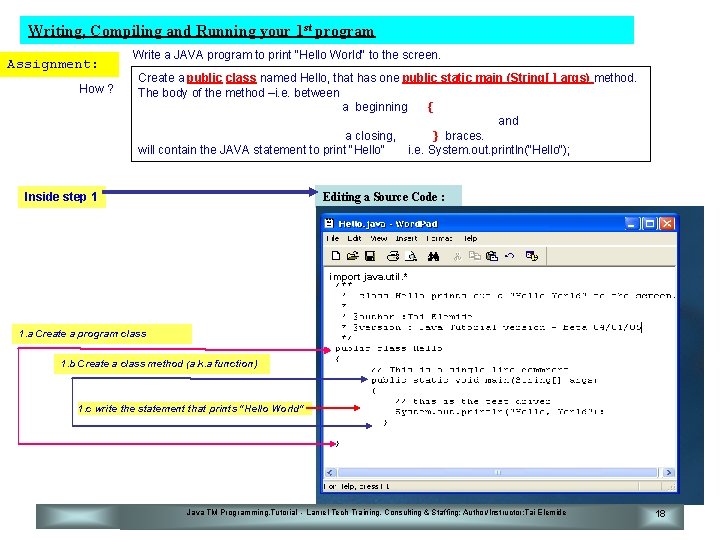
Writing, Compiling and Running your 1 st program Assignment: How ? Write a JAVA program to print "Hello World" to the screen. Create a public class named Hello, that has one public static main (String[ ] args) method. The body of the method –i. e. between a beginning { and a closing, } braces. will contain the JAVA statement to print "Hello" i. e. System. out. println("Hello"); Editing a Source Code : Inside step 1 import java. util. * 1. a Create a program class 1. b Create a class method (a. k. a function) 1. c write the statement that prints "Hello World" Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 18
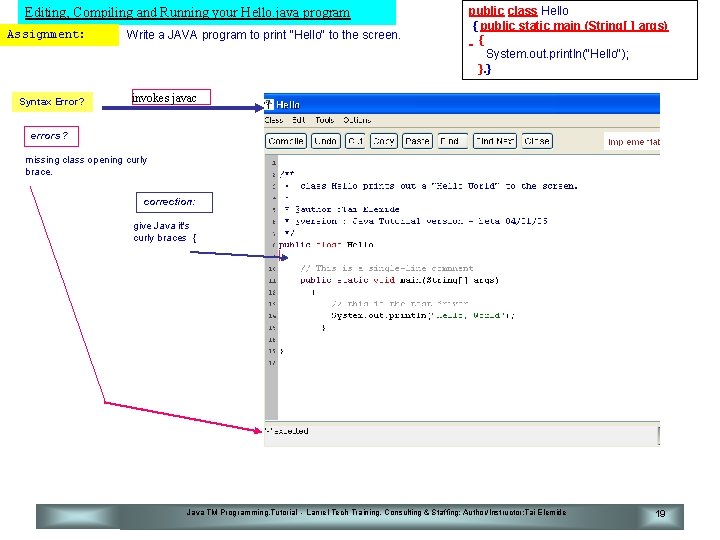
Editing, Compiling and Running your Hello. java program Assignment: Syntax Error? Write a JAVA program to print "Hello" to the screen. public class Hello { public static main (String[ ] args) { System. out. println("Hello"); }. } invokes javac errors ? missing class opening curly brace. correction: give Java it's curly braces { Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 19
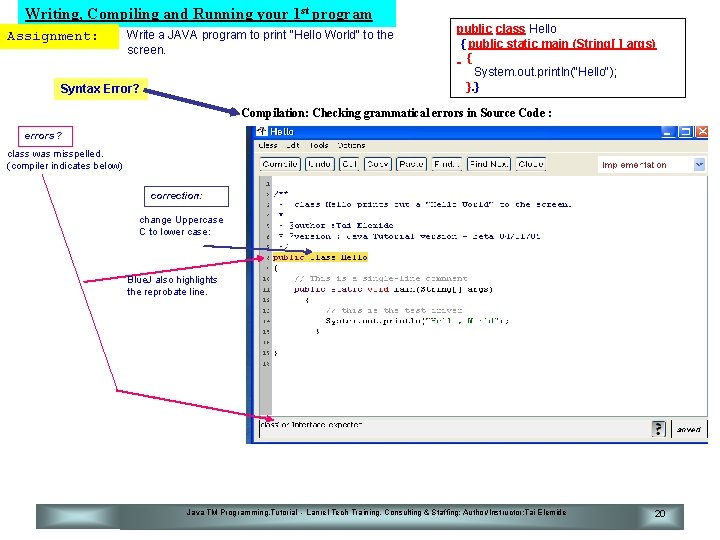
Writing, Compiling and Running your 1 st program Assignment: Write a JAVA program to print "Hello World" to the screen. Syntax How Error? ? public class Hello { public static main (String[ ] args) { System. out. println("Hello"); }. } Compilation: Checking grammatical errors in Source Code : errors ? class was misspelled. (compiler indicates below) correction: change Uppercase C to lower case: Blue. J also highlights the reprobate line. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 20
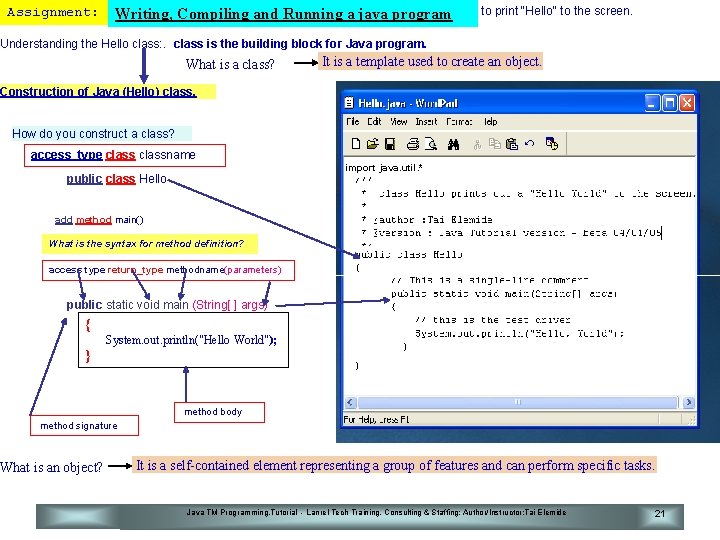
Assignment: Writing, Compiling and Running a java program to print "Hello" to the screen. Understanding the Hello class: . class is the building block for Java program. What is a class? It is a template used to create an object. Construction of Java (Hello) class. How do you construct a class? access_type classname import java. util. * public class Hello add method main() What is the syntax for method definition? access type return_type methodname(parameters) public static void main (String[ ] args) { System. out. println("Hello World"); } method body method signature What is an object? It is a self-contained element representing a group of features and can perform specific tasks. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 21
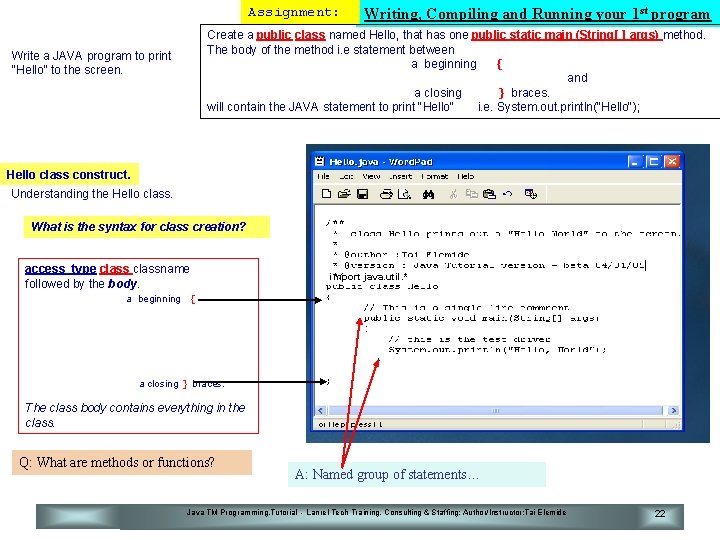
Assignment: Writing, Compiling and Running your 1 st program Create a public class named Hello, that has one public static main (String[ ] args) method. The body of the method i. e statement between a beginning { and a closing } braces. will contain the JAVA statement to print "Hello" i. e. System. out. println("Hello"); Write a JAVA program to print "Hello" to the screen. Hello class construct. Understanding the Hello class. What is the syntax for class creation? access_type classname followed by the body. a beginning import java. util. * { a closing } braces. The class body contains everything in the class. Q: What are methods or functions? A: Named group of statements… Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 22
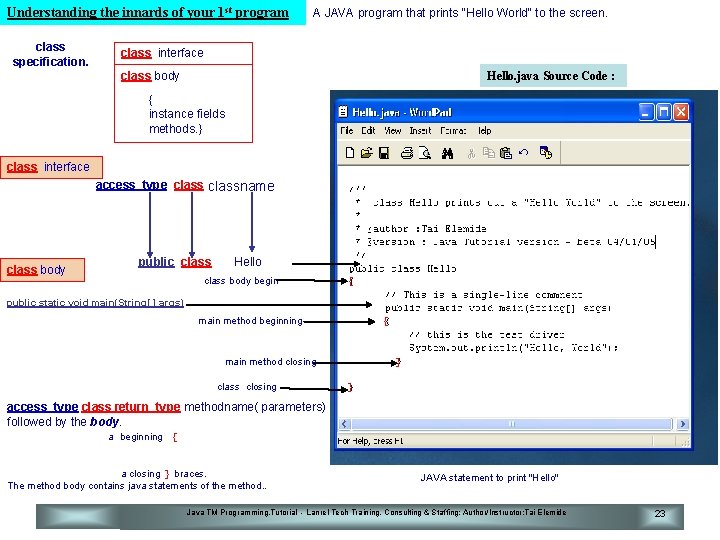
Understanding the innards of your 1 st program class specification. A JAVA program that prints "Hello World" to the screen. class interface class body Hello. java Source Code : { instance fields methods. } class interface access_type classname class body public class Hello class body begin { public static void main(String[ ] args) main method beginning { main method closing class closing } } access_type class return_type methodname( parameters) followed by the body. a beginning { a closing } braces. The method body contains java statements of the method. . JAVA statement to print "Hello" Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 23
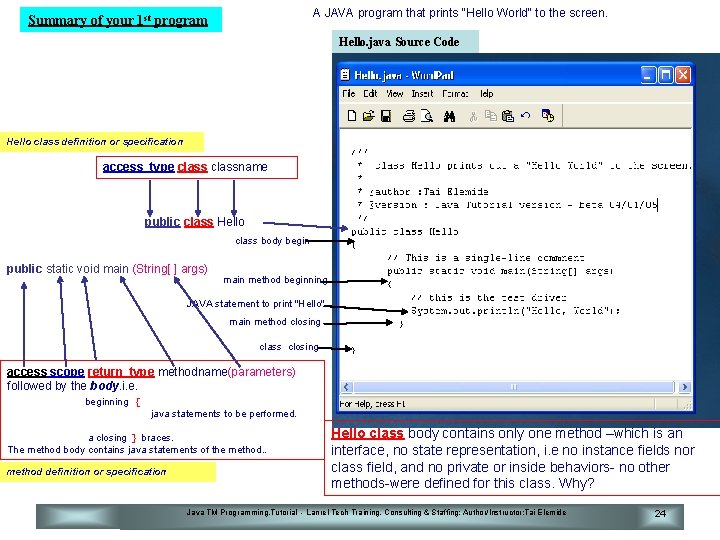
A JAVA program that prints "Hello World" to the screen. Summary of your 1 st program Hello. java Source Code Hello class definition or specification access_type classname public class Hello class body begin public static void main (String[ ] args) main method beginning JAVA statement to print "Hello" main method closing class closing access scope return_type methodname(parameters) followed by the body. i. e. beginning { java statements to be performed. a closing } braces. The method body contains java statements of the method. . method definition or specification Hello class body contains only one method –which is an interface, no state representation, i. e no instance fields nor class field, and no private or inside behaviors- no other methods-were defined for this class. Why? Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 24
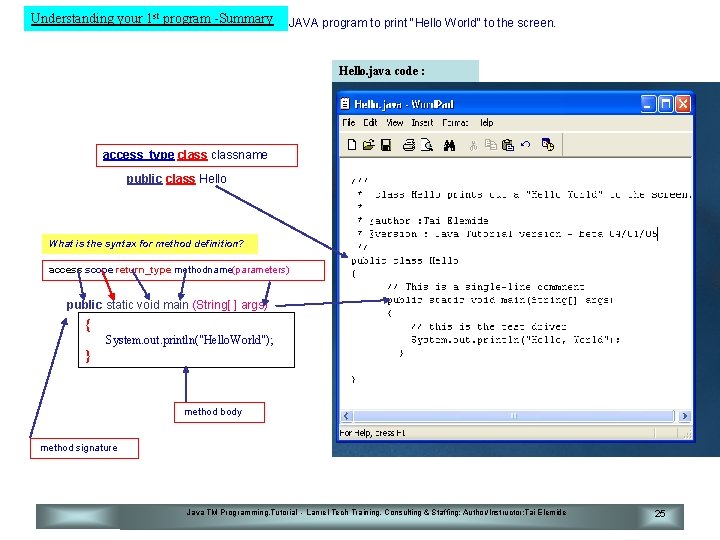
Understanding your 1 st program -Summary JAVA program to print "Hello World" to the screen. Hello. java code : access_type classname public class Hello What is the syntax for method definition? access scope return_type methodname(parameters) public static void main (String[ ] args) { System. out. println("Hello. World"); } method body method signature Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 25
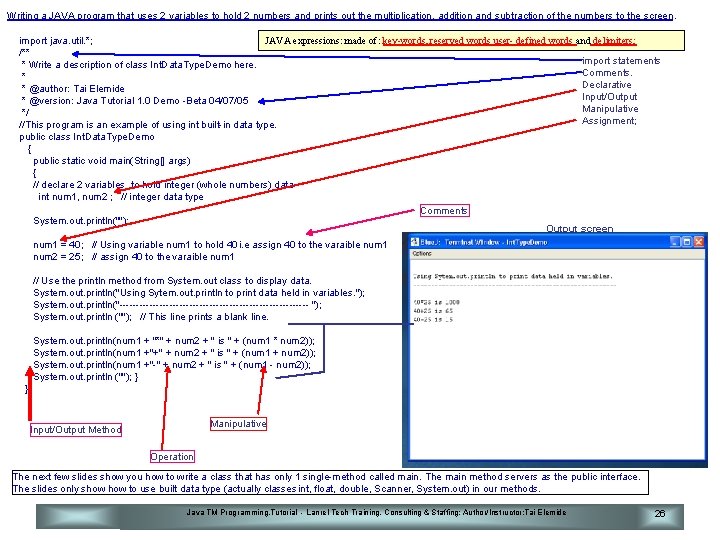
Writing a JAVA program that uses 2 variables to hold 2 numbers and prints out the multiplication, addition and subtraction of the numbers to the screen. JAVA expressions: made of: key-words, reserved words user- defined words and delimiters; import java. util. *; /** import statements * Write a description of class Int. Data. Type. Demo here. Comments. * Declarative * @author: Tai Elemide Input/Output * @version: Java Tutorial 1. 0 Demo -Beta 04/07/05 Manipulative */ Assignment; //This program is an example of using int built-in data type. public class Int. Data. Type. Demo { public static void main(String[] args) { // declare 2 variables to hold integer (whole numbers) data int num 1, num 2 ; // integer data type Comments System. out. println(""); Output screen num 1 = 40; // Using variable num 1 to hold 40 i. e assign 40 to the varaible num 1 num 2 = 25; // assign 40 to the varaible num 1 // Use the println method from System. out class to display data. System. out. println("Using Sytem. out. println to print data held in variables. "); System. out. println("----------------------------- "); System. out. println (""); // This line prints a blank line. System. out. println(num 1 + "*" + num 2 + " is " + (num 1 * num 2)); System. out. println(num 1 +"+" + num 2 + " is " + (num 1 + num 2)); System. out. println(num 1 +"-" + num 2 + " is " + (num 1 - num 2)); System. out. println (""); } } Manipulative Input/Output Method Operation The next few slides show you how to write a class that has only 1 single-method called main. The main method servers as the public interface. The slides only show to use built data type (actually classes int, float, double, Scanner, System. out) in our methods. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 26
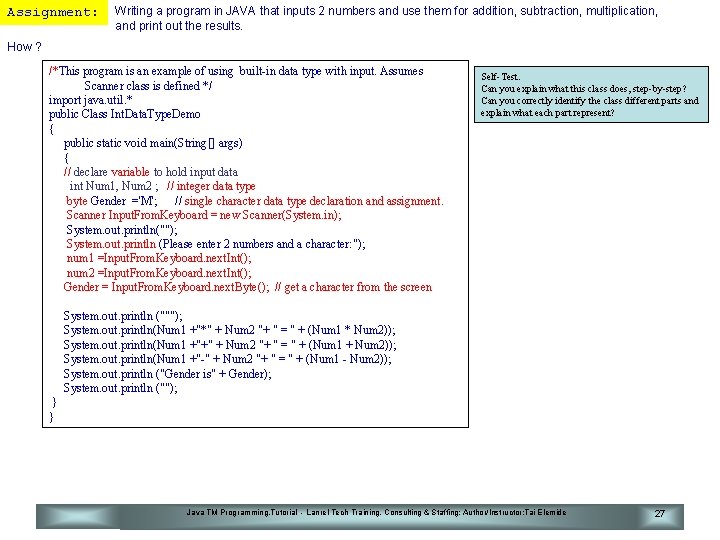
Assignment: Writing a program in JAVA that inputs 2 numbers and use them for addition, subtraction, multiplication, and print out the results. How ? /*This program is an example of using built-in data type with input. Assumes Scanner class is defined */ import java. util. * public Class Int. Data. Type. Demo { public static void main(String[] args) { // declare variable to hold input data int Num 1, Num 2 ; // integer data type byte Gender ='M'; // single character data type declaration and assignment. Scanner Input. From. Keyboard = new Scanner(System. in); System. out. println(""); System. out. println (Please enter 2 numbers and a character: "); num 1 =Input. From. Keyboard. next. Int(); num 2 =Input. From. Keyboard. next. Int(); Gender = Input. From. Keyboard. next. Byte(); // get a character from the screen Self-Test. Can you explain what this class does, step-by-step? Can you correctly identify the class different parts and explain what each part represent? System. out. println ("""); System. out. println(Num 1 +"*" + Num 2 "+ " = " + (Num 1 * Num 2)); System. out. println(Num 1 +"+" + Num 2 "+ " = " + (Num 1 + Num 2)); System. out. println(Num 1 +"-" + Num 2 "+ " = " + (Num 1 - Num 2)); System. out. println ("Gender is" + Gender); System. out. println (""); } } Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 27
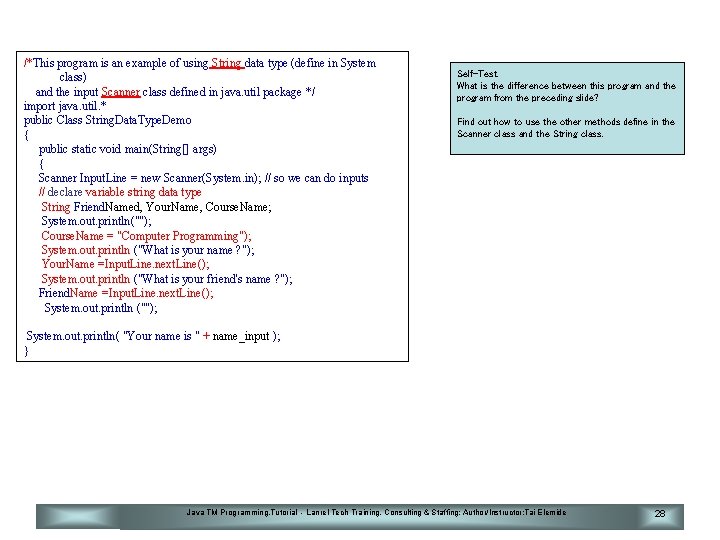
/*This program is an example of using String data type (define in System class) and the input Scanner class defined in java. util package */ import java. util. * public Class String. Data. Type. Demo { public static void main(String[] args) { Scanner Input. Line = new Scanner(System. in); // so we can do inputs // declare variable string data type String Friend. Named, Your. Name, Course. Name; System. out. println(""); Course. Name = "Computer Programming"); System. out. println ("What is your name ? "); Your. Name =Input. Line. next. Line(); System. out. println ("What is your friend's name ? "); Friend. Name =Input. Line. next. Line(); System. out. println (""); Self-Test. What is the difference between this program and the program from the preceding slide? Find out how to use the other methods define in the Scanner class and the String class. System. out. println( "Your name is " + name_input ); } Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 28
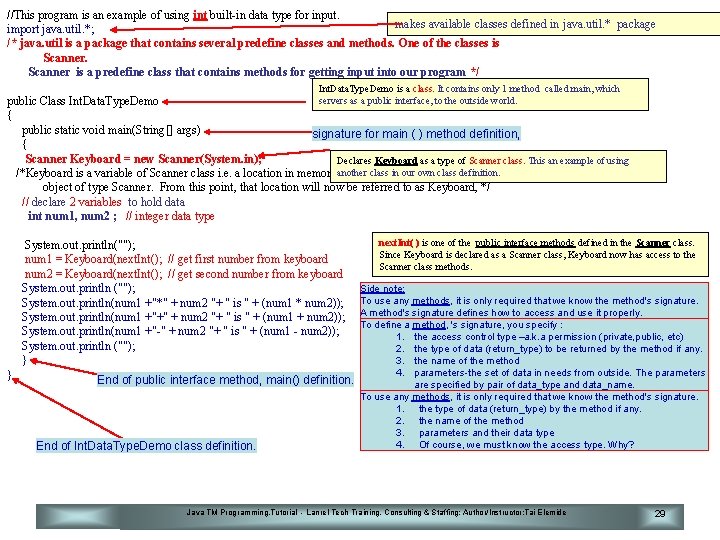
//This program is an example of using int built-in data type for input. makes available classes defined in java. util. * package import java. util. *; /* java. util is a package that contains several predefine classes and methods. One of the classes is Scanner is a predefine class that contains methods for getting input into our program */ Int. Data. Type. Demo is a class. It contains only 1 method called main, which servers as a public interface, to the outside world. public Class Int. Data. Type. Demo { public static void main(String[] args) signature for main ( ) method definition, { Scanner Keyboard = new Scanner(System. in); Declares Keyboard as a type of Scanner class. This an example of using in our own class definition. /*Keyboard is a variable of Scanner class i. e. a location in memory, another that isclass now reserved to hold object of type Scanner. From this point, that location will now be referred to as Keyboard, */ // declare 2 variables to hold data int num 1, num 2 ; // integer data type System. out. println(""); num 1 = Keyboard(next. Int(); // get first number from keyboard num 2 = Keyboard(next. Int(); // get second number from keyboard System. out. println (""); System. out. println(num 1 +"*" + num 2 "+ " is " + (num 1 * num 2)); System. out. println(num 1 +"+" + num 2 "+ " is " + (num 1 + num 2)); System. out. println(num 1 +"-" + num 2 "+ " is " + (num 1 - num 2)); System. out. println (""); } } End of public interface method, main() definition. End of Int. Data. Type. Demo class definition. next. Int( ) is one of the public interface methods defined in the Scanner class. Since Keyboard is declared as a Scanner class, Keyboard now has access to the Scanner class methods. Side note: To use any methods, it is only required that we know the method's signature. A method's signature defines how to access and use it properly. To define a method, 's signature, you specify : 1. the access control type –a. k. a permission (private, public, etc) 2. the type of data (return_type) to be returned by the method if any. 3. the name of the method 4. parameters-the set of data in needs from outside. The parameters are specified by pair of data_type and data_name. To use any methods, it is only required that we know the method's signature. 1. the type of data (return_type) by the method if any. 2. the name of the method 3. parameters and their data type 4. Of course, we must know the access type. Why? Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 29
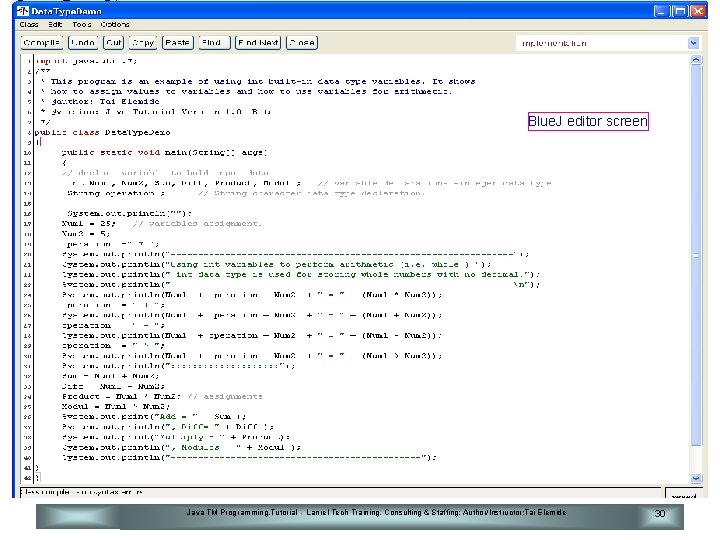
Blue. J editor screen Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 30
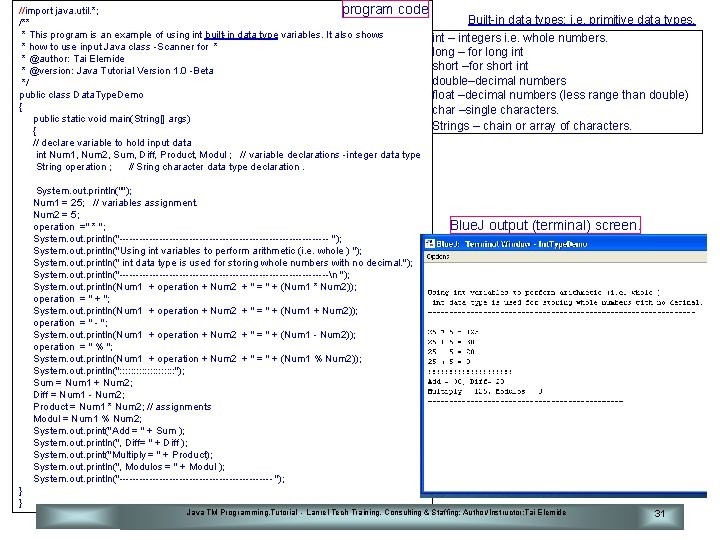
program code //import java. util. *; /** * This program is an example of using int built-in data type variables. It also shows * how to use input Java class -Scanner for * * @author: Tai Elemide * @version: Java Tutorial Version 1. 0 -Beta */ public class Data. Type. Demo { public static void main(String[] args) { // declare variable to hold input data int Num 1, Num 2, Sum, Diff, Product, Modul ; // variable declarations -integer data type String operation ; // Sring character data type declaration. System. out. println(""); Num 1 = 25; // variables assignment. Num 2 = 5; operation =" * "; System. out. println("-------------------------------- "); System. out. println("Using int variables to perform arithmetic (i. e. whole ) "); System. out. println(" int data type is used for storing whole numbers with no decimal. "); System. out. println("--------------------------------n "); System. out. println(Num 1 + operation + Num 2 + " = " + (Num 1 * Num 2)); operation = " + "; System. out. println(Num 1 + operation + Num 2 + " = " + (Num 1 + Num 2)); operation = " - "; System. out. println(Num 1 + operation + Num 2 + " = " + (Num 1 - Num 2)); operation = " % "; System. out. println(Num 1 + operation + Num 2 + " = " + (Num 1 % Num 2)); System. out. println(": : : : : "); Sum = Num 1 + Num 2; Diff = Num 1 - Num 2; Product = Num 1 * Num 2; // assignments Modul = Num 1 % Num 2; System. out. print("Add = " + Sum ); System. out. println(", Diff= " + Diff ); System. out. print("Multiply = " + Product); System. out. println(", Modulos = " + Modul ); System. out. println("----------------------- "); Built-in data types: i. e. primitive data types. int – integers i. e. whole numbers. long – for long int short –for short int double–decimal numbers float –decimal numbers (less range than double) char –single characters. Strings – chain or array of characters. Blue. J output (terminal) screen. } } Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 31
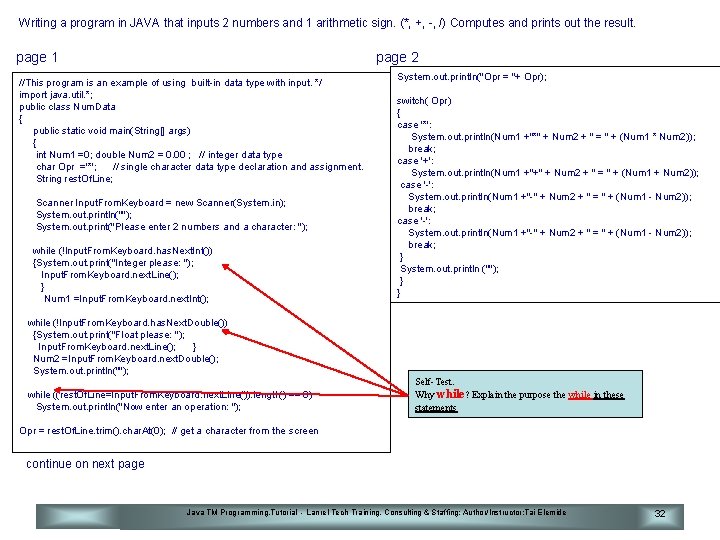
Writing a program in JAVA that inputs 2 numbers and 1 arithmetic sign. (*, +, -, /) Computes and prints out the result. page 1 page 2 //This program is an example of using built-in data type with input. */ import java. util. *; public class Num. Data { public static void main(String[] args) { int Num 1 =0; double Num 2 = 0. 00 ; // integer data type char Opr ='*'; // single character data type declaration and assignment. String rest. Of. Line; Scanner Input. From. Keyboard = new Scanner(System. in); System. out. println(""); System. out. print("Please enter 2 numbers and a character: "); while (!Input. From. Keyboard. has. Next. Int()) {System. out. print("Integer please: "); Input. From. Keyboard. next. Line(); } Num 1 =Input. From. Keyboard. next. Int(); while (!Input. From. Keyboard. has. Next. Double()) {System. out. print("Float please: "); Input. From. Keyboard. next. Line(); } Num 2 =Input. From. Keyboard. next. Double(); System. out. println(""); while ((rest. Of. Line=Input. From. Keyboard. next. Line()). length() == 0) System. out. println("Now enter an operation: "); System. out. println("Opr = "+ Opr); switch( Opr) { case '*': System. out. println(Num 1 +"*" + Num 2 + " = " + (Num 1 * Num 2)); break; case '+': System. out. println(Num 1 +"+" + Num 2 + " = " + (Num 1 + Num 2)); case '-': System. out. println(Num 1 +"-" + Num 2 + " = " + (Num 1 - Num 2)); break; } System. out. println (""); } } Self-Test. Why while? Explain the purpose the while in these statements Opr = rest. Of. Line. trim(). char. At(0); // get a character from the screen continue on next page Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 32
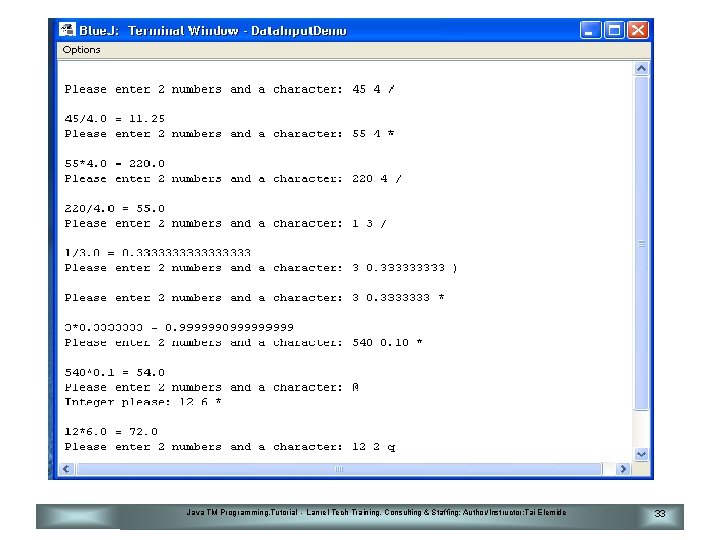
Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 33
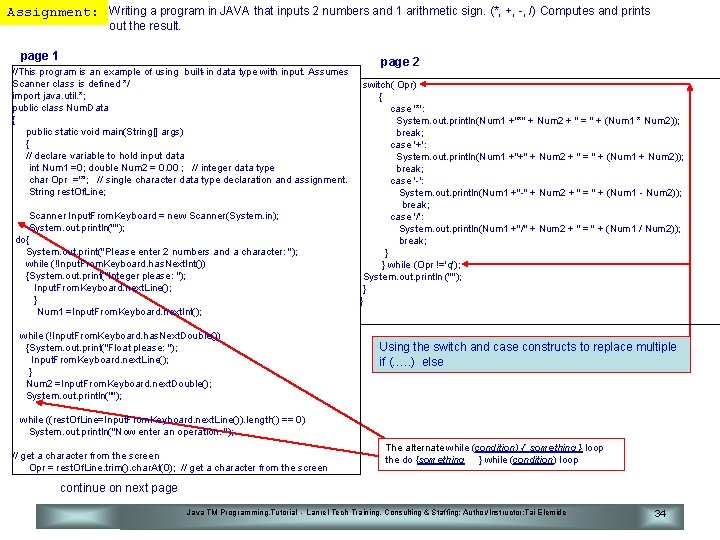
Assignment: Writing a program in JAVA that inputs 2 numbers and 1 arithmetic sign. (*, +, -, /) Computes and prints out the result. page 1 //This program is an example of using built-in data type with input. Assumes Scanner class is defined */ import java. util. *; public class Num. Data { public static void main(String[] args) { // declare variable to hold input data int Num 1 =0; double Num 2 = 0. 00 ; // integer data type char Opr ='*'; // single character data type declaration and assignment. String rest. Of. Line; Scanner Input. From. Keyboard = new Scanner(System. in); System. out. println(""); do{ System. out. print("Please enter 2 numbers and a character: "); while (!Input. From. Keyboard. has. Next. Int()) {System. out. print("Integer please: "); Input. From. Keyboard. next. Line(); } Num 1 =Input. From. Keyboard. next. Int(); while (!Input. From. Keyboard. has. Next. Double()) {System. out. print("Float please: "); Input. From. Keyboard. next. Line(); } Num 2 =Input. From. Keyboard. next. Double(); System. out. println(""); page 2 switch( Opr) { case '*': System. out. println(Num 1 +"*" + Num 2 + " = " + (Num 1 * Num 2)); break; case '+': System. out. println(Num 1 +"+" + Num 2 + " = " + (Num 1 + Num 2)); break; case '-': System. out. println(Num 1 +"-" + Num 2 + " = " + (Num 1 - Num 2)); break; case '/': System. out. println(Num 1 +"/" + Num 2 + " = " + (Num 1 / Num 2)); break; } } while (Opr !='q'); System. out. println (""); } } Using the switch and case constructs to replace multiple if (…. . ) else while ((rest. Of. Line=Input. From. Keyboard. next. Line()). length() == 0) System. out. println("Now enter an operation: "); // get a character from the screen Opr = rest. Of. Line. trim(). char. At(0); // get a character from the screen The alternate while (condition) { something } loop the do {something } while (condition) loop continue on next page Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 34
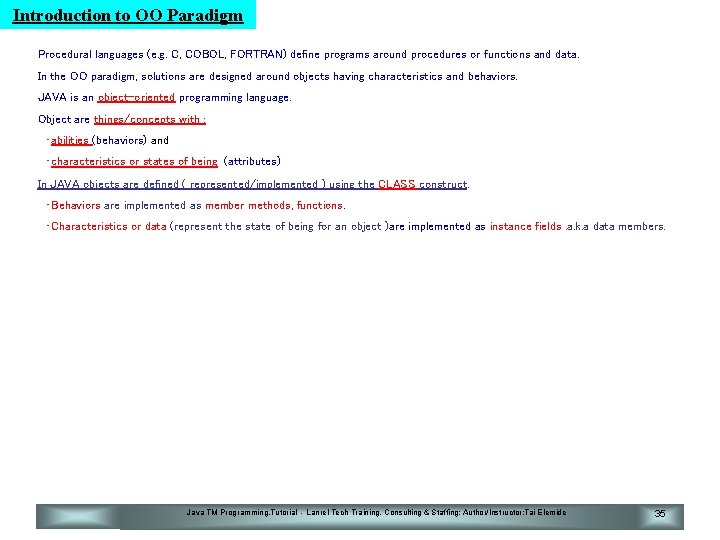
Introduction to OO Paradigm Procedural languages (e. g. C, COBOL, FORTRAN) define programs around procedures or functions and data. In the OO paradigm, solutions are designed around objects having characteristics and behaviors. JAVA is an object-oriented programming language. Object are things/concepts with : • abilities (behaviors) and • characteristics or states of being (attributes) In JAVA objects are defined ( represented/implemented ) using the CLASS construct. • Behaviors are implemented as member methods, functions. • Characteristics or data (represent the state of being for an object )are implemented as instance fields. a. k. a data members. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 35
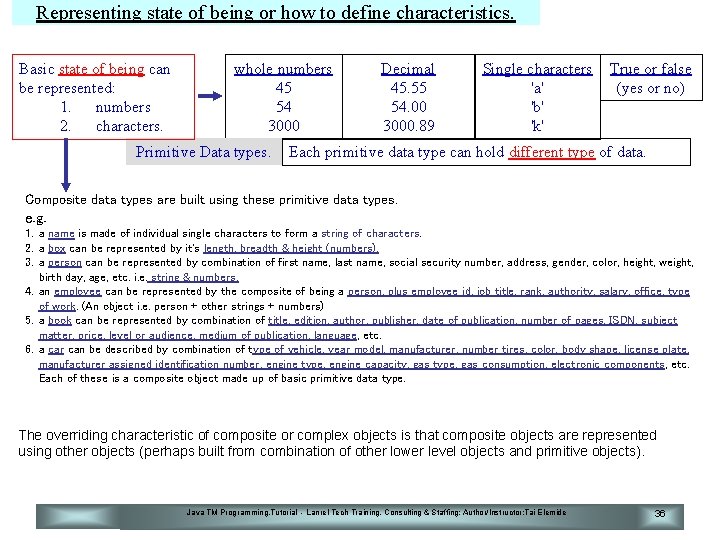
Representing state of being or how to define characteristics. Basic state of being can be represented: 1. numbers 2. characters. whole numbers 45 54 3000 Primitive Data types. Decimal 45. 55 54. 00 3000. 89 Single characters 'a' 'b' 'k' True or false (yes or no) Each primitive data type can hold different type of data. Composite data types are built using these primitive data types. e. g. 1. a name is made of individual single characters to form a string of characters. 2. a box can be represented by it's length, breadth & height (numbers). 3. a person can be represented by combination of first name, last name, social security number, address, gender, color, height, weight, birth day, age, etc. i. e. string & numbers. 4. an employee can be represented by the composite of being a person, plus employee id, job title, rank, authority, salary, office, type of work. (An object i. e. person + other strings + numbers) 5. a book can be represented by combination of title, edition, author, publisher, date of publication, number of pages, ISDN, subject matter, price, level or audience, medium of publication, language, etc. 6. a car can be described by combination of type of vehicle, year model, manufacturer, number tires, color, body shape, license plate, manufacturer assigned identification number, engine type, engine capacity, gas type, gas consumption, electronic components, etc. Each of these is a composite object made up of basic primitive data type. The overriding characteristic of composite or complex objects is that composite objects are represented using other objects (perhaps built from combination of other lower level objects and primitive objects). Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 36
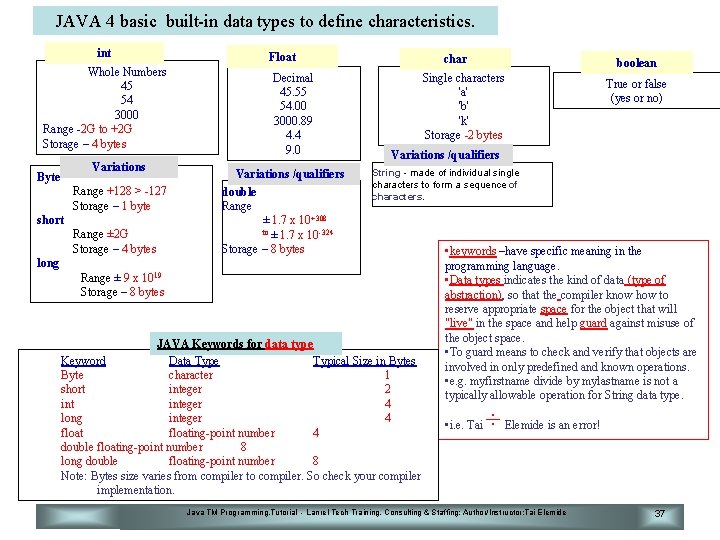
JAVA 4 basic built-in data types to define characteristics. int Whole Numbers 45 54 3000 Range -2 G to +2 G Storage – 4 bytes Variations Byte Range +128 > -127 Storage – 1 byte short Range ± 2 G Storage – 4 bytes Float Decimal 45. 55 54. 00 3000. 89 4. 4 9. 0 Variations /qualifiers double Range ± 1. 7 x 10+308 to ± 1. 7 x 10 -324 Storage – 8 bytes char boolean Single characters 'a' 'b' 'k' Storage -2 bytes True or false (yes or no) Variations /qualifiers String - made of individual single characters to form a sequence of characters. long Range ± 9 x 1019 Storage – 8 bytes JAVA Keywords for data type Keyword Data Type Typical Size in Bytes Byte character 1 short integer 2 integer 4 long integer 4 floating-point number 4 double floating-point number 8 long double floating-point number 8 Note: Bytes size varies from compiler to compiler. So check your compiler implementation. • keywords –have specific meaning in the programming language. • Data types indicates the kind of data (type of abstraction), so that the compiler know how to reserve appropriate space for the object that will "live" in the space and help guard against misuse of the object space. • To guard means to check and verify that objects are involved in only predefined and known operations. • e. g. myfirstname divide by mylastname is not a typically allowable operation for String data type. • i. e. Tai ÷ Elemide is an error! Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 37
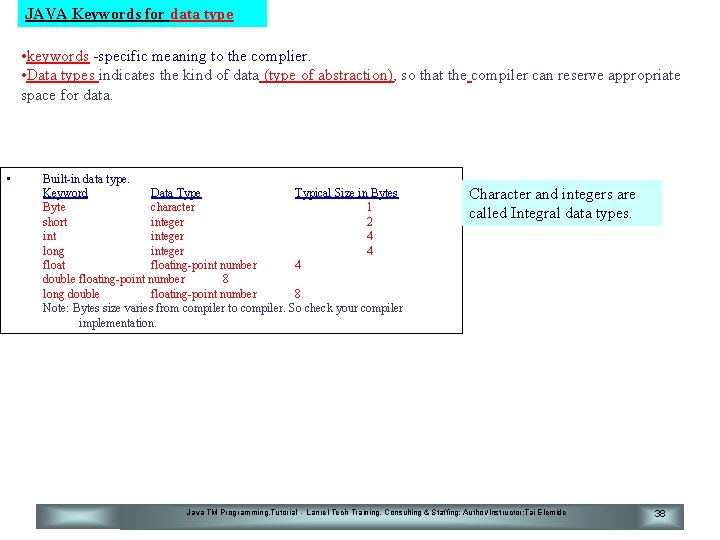
JAVA Keywords for data type • keywords -specific meaning to the complier. • Data types indicates the kind of data (type of abstraction), so that the compiler can reserve appropriate space for data. • Built-in data type. Keyword Data Type Typical Size in Bytes Byte character 1 short integer 2 integer 4 long integer 4 floating-point number 4 double floating-point number 8 long double floating-point number 8 Note: Bytes size varies from compiler to compiler. So check your compiler implementation. Character and integers are called Integral data types. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 38
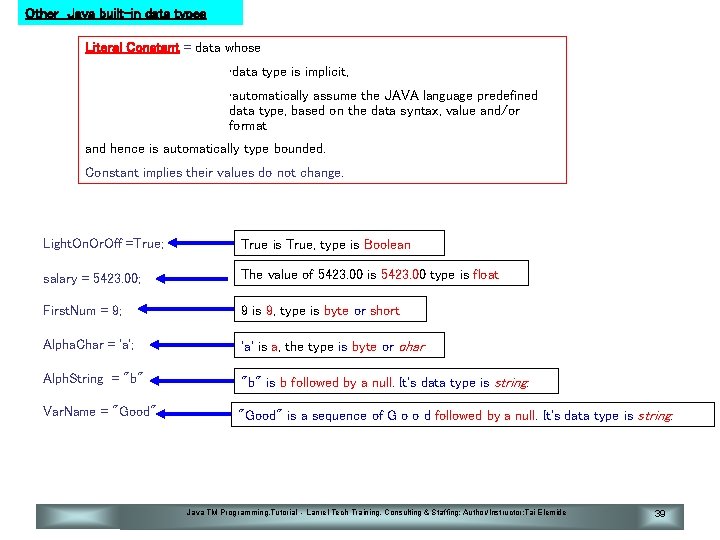
Other Java built-in data types Literal Constant = data whose • data type is implicit, • automatically assume the JAVA language predefined data type, based on the data syntax, value and/or format and hence is automatically type bounded. Constant implies their values do not change. Light. On. Or. Off =True; True is True, type is Boolean salary = 5423. 00; The value of 5423. 00 is 5423. 00 type is float First. Num = 9; 9 is 9, type is byte or short Alpha. Char = 'a'; 'a' is a, the type is byte or char Alph. String = "b" is b followed by a null. It's data type is string. Var. Name = "Good" is a sequence of G o o d followed by a null. It's data type is string. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 39
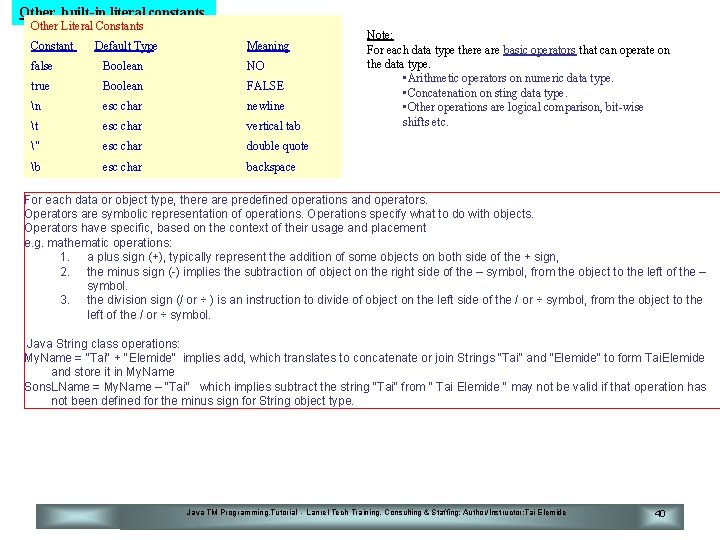
Other built-in literal constants Other Literal Constants Constant Default Type Meaning false Boolean NO true Boolean FALSE n esc char newline t esc char vertical tab " esc char double quote b esc char backspace Note: For each data type there are basic operators that can operate on the data type. • Arithmetic operators on numeric data type. • Concatenation on sting data type. • Other operations are logical comparison, bit-wise shifts etc. For each data or object type, there are predefined operations and operators. Operators are symbolic representation of operations. Operations specify what to do with objects. Operators have specific, based on the context of their usage and placement e. g. mathematic operations: 1. a plus sign (+), typically represent the addition of some objects on both side of the + sign, 2. the minus sign (-) implies the subtraction of object on the right side of the – symbol, from the object to the left of the – symbol. 3. the division sign (/ or ÷ ) is an instruction to divide of object on the left side of the / or ÷ symbol, from the object to the left of the / or ÷ symbol. Java String class operations: My. Name = "Tai" + "Elemide" implies add, which translates to concatenate or join Strings "Tai" and "Elemide" to form Tai. Elemide and store it in My. Name Sons. LName = My. Name – "Tai" which implies subtract the string "Tai" from " Tai Elemide " may not be valid if that operation has not been defined for the minus sign for String object type. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 40
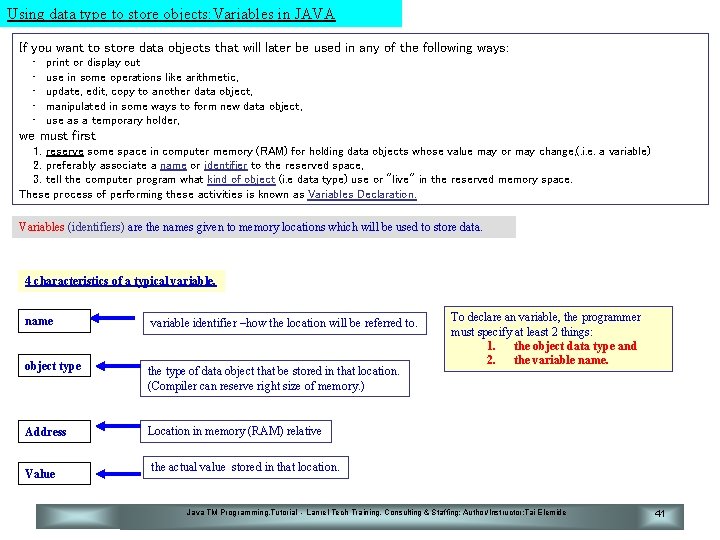
Using data type to store objects: Variables in JAVA If you want to store data objects that will later be used in any of the following ways: • • • print or display out use in some operations like arithmetic, update, edit, copy to another data object, manipulated in some ways to form new data object, use as a temporary holder, we must first 1. reserve some space in computer memory (RAM) for holding data objects whose value may or may change, (. i. e. a variable) 2. preferably associate a name or identifier to the reserved space, 3. tell the computer program what kind of object (i. e data type) use or "live" in the reserved memory space. These process of performing these activities is known as Variables Declaration. Variables (identifiers) are the names given to memory locations which will be used to store data. 4 characteristics of a typical variable. name variable identifier –how the location will be referred to. object type the type of data object that be stored in that location. (Compiler can reserve right size of memory. ) Address Location in memory (RAM) relative Value To declare an variable, the programmer must specify at least 2 things: 1. the object data type and 2. the variable name. the actual value stored in that location. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 41
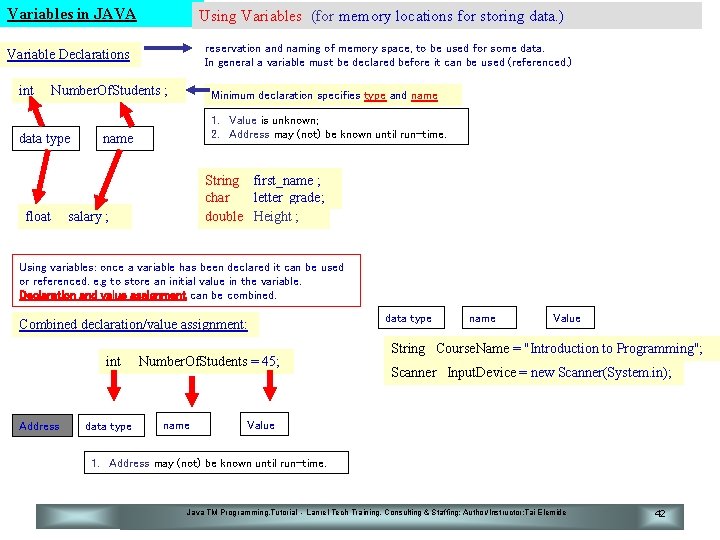
Variables in JAVA Using Variables (for memory locations for storing data. ) reservation and naming of memory space, to be used for some data. In general a variable must be declared before it can be used (referenced. ) Variable Declarations int Number. Of. Students ; data type float Minimum declaration specifies type and name 1. Value is unknown; 2. Address may (not) be known until run-time. name String first_name ; char letter_grade; double Height ; salary ; Using variables: once a variable has been declared it can be used or referenced. e. g to store an initial value in the variable. Declaration and value assignment can be combined. Combined declaration/value assignment: int Address data type Number. Of. Students = 45; name data type name Value String Course. Name = "Introduction to Programming"; Scanner Input. Device = new Scanner(System. in); Value 1. Address may (not) be known until run-time. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 42
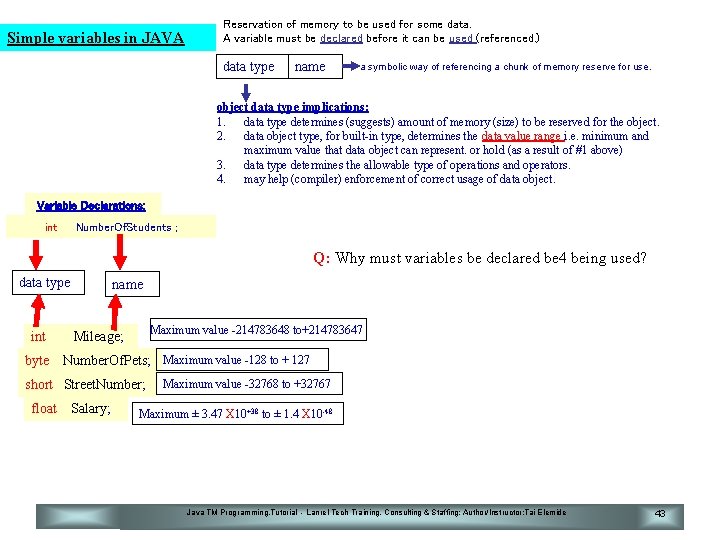
Simple variables in JAVA Reservation of memory to be used for some data. A variable must be declared before it can be used (referenced. ) data type name a symbolic way of referencing a chunk of memory reserve for use. object data type implications: 1. data type determines (suggests) amount of memory (size) to be reserved for the object. 2. data object type, for built-in type, determines the data value range i. e. minimum and maximum value that data object can represent. or hold (as a result of #1 above) 3. data type determines the allowable type of operations and operators. 4. may help (compiler) enforcement of correct usage of data object. Variable Declarations: int Number. Of. Students ; Q: Why must variables be declared be 4 being used? data type int byte name Maximum value -214783648 to+214783647 Mileage; Number. Of. Pets; Maximum value -128 to + 127 short Street. Number; float Salary; Maximum value -32768 to +32767 Maximum ± 3. 47 X 10+38 to ± 1. 4 X 10 -48 Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 43
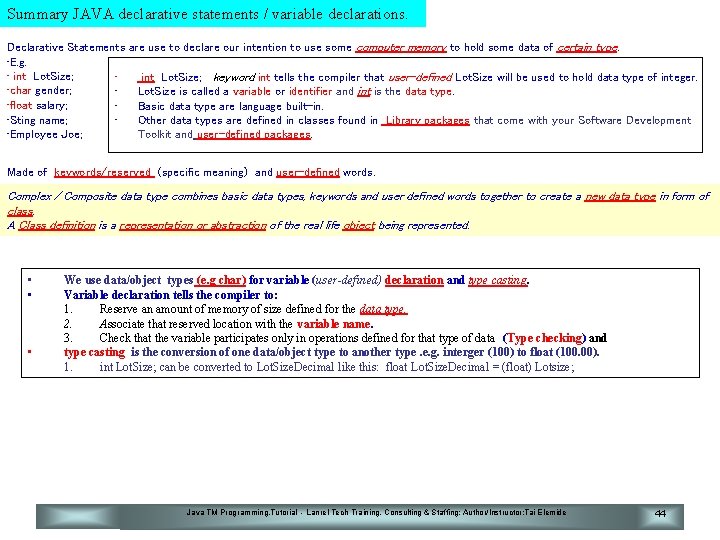
Summary JAVA declarative statements / variable declarations. Declarative Statements are use to declare our intention to use some computer memory to hold some data of certain type. • E. g. • int Lot. Size; keyword int tells the compiler that user-defined Lot. Size will be used to hold data type of integer. • char gender; • Lot. Size is called a variable or identifier and int is the data type. • float salary; • Basic data type are language built-in. • Other data types are defined in classes found in Library packages that come with your Software Development • Sting name; Toolkit and user-defined packages. • Employee Joe; Made of keywords/reserved (specific meaning) and user-defined words. Complex / Composite data type combines basic data types, keywords and user defined words together to create a new data type in form of class. A Class definition is a representation or abstraction of the real life object being represented. • • • We use data/object types (e. g char) for variable (user-defined) declaration and type casting. Variable declaration tells the compiler to: 1. Reserve an amount of memory of size defined for the data type. 2. Associate that reserved location with the variable name. 3. Check that the variable participates only in operations defined for that type of data (Type checking) and type casting is the conversion of one data/object type to another type. e. g. interger (100) to float (100. 00). 1. int Lot. Size; can be converted to Lot. Size. Decimal like this: float Lot. Size. Decimal = (float) Lotsize; Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 44
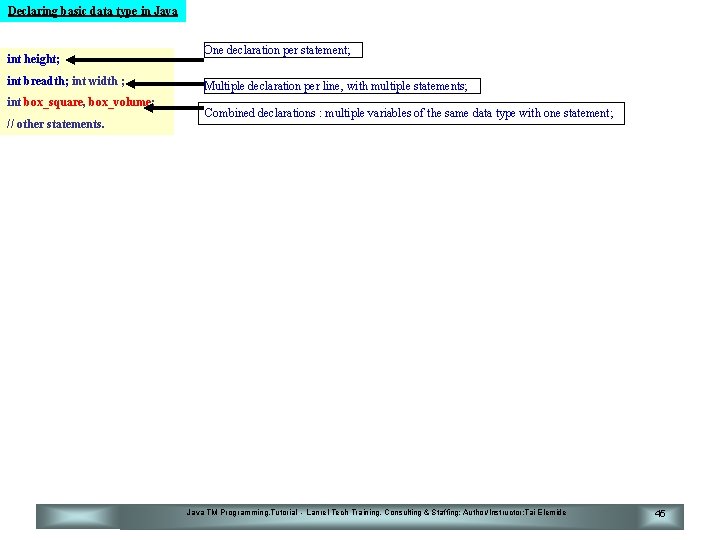
Declaring basic data type in Java int height; int breadth; int width ; int box_square, box_volume; // other statements. One declaration per statement; Multiple declaration per line, with multiple statements; Combined declarations : multiple variables of the same data type with one statement; Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 45
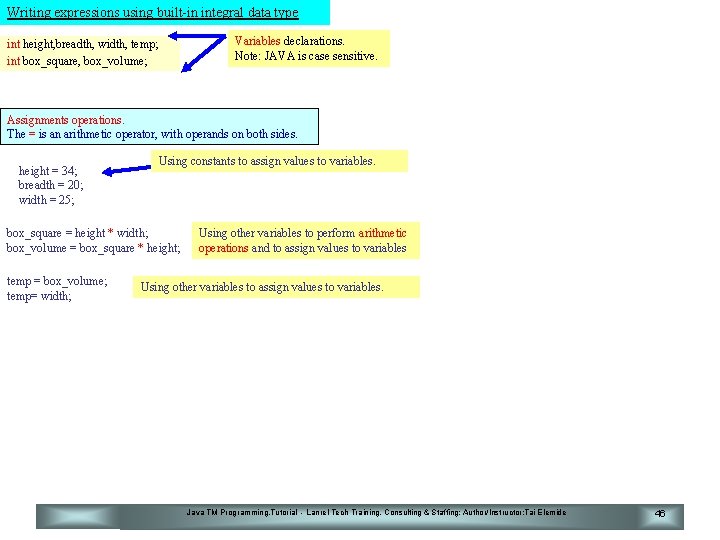
Writing expressions using built-in integral data type Variables declarations. Note: JAVA is case sensitive. int height, breadth, width, temp; int box_square, box_volume; Assignments operations. The = is an arithmetic operator, with operands on both sides. height = 34; breadth = 20; width = 25; Using constants to assign values to variables. box_square = height * width; box_volume = box_square * height; temp = box_volume; temp= width; Using other variables to perform arithmetic operations and to assign values to variables Using other variables to assign values to variables. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 46
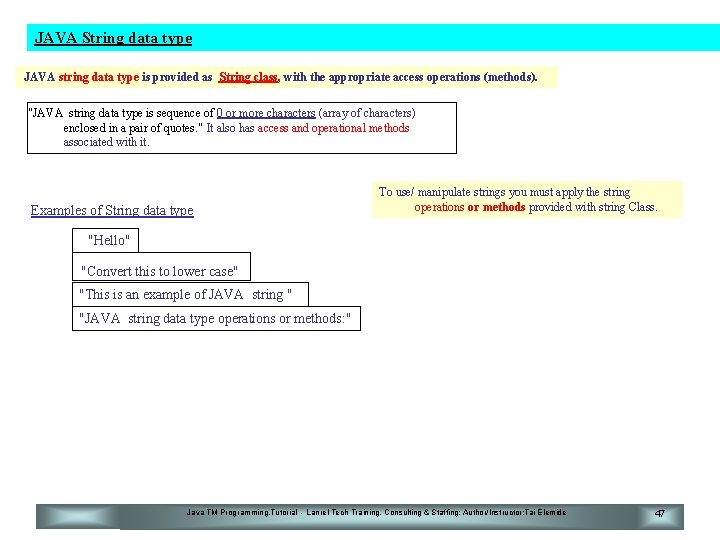
JAVA String data type JAVA string data type is provided as String class, with the appropriate access operations (methods). "JAVA string data type is sequence of 0 or more characters (array of characters) enclosed in a pair of quotes. " It also has access and operational methods associated with it. Examples of String data type To use/ manipulate strings you must apply the string operations or methods provided with string Class. "Hello" "Convert this to lower case" "This is an example of JAVA string " "JAVA string data type operations or methods: " Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 47
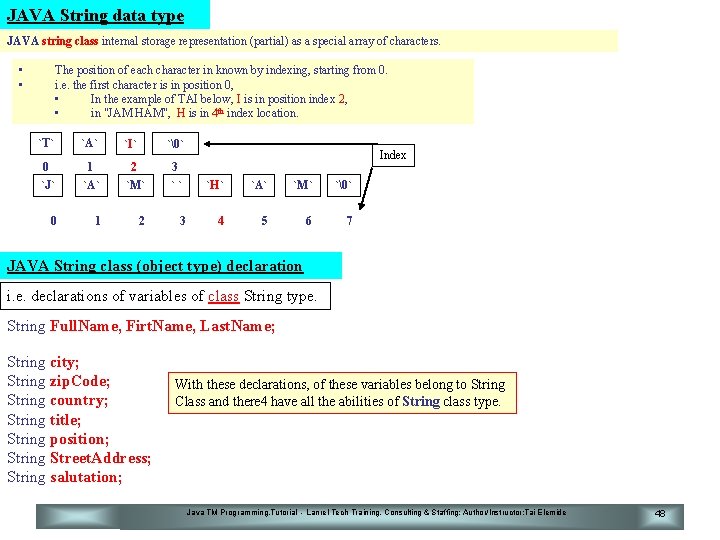
JAVA String data type JAVA string class internal storage representation (partial) as a special array of characters. • • The position of each character in known by indexing, starting from 0. i. e. the first character is in position 0, • In the example of TAI below, I is in position index 2, • in "JAM HAM", H is in 4 th index location. `T` `A` 0 1 2 3 `J` `A` `M` `` 0 1 2 `I` ` ` 3 Index `H` `A` `M` ` ` 4 5 6 7 JAVA String class (object type) declaration i. e. declarations of variables of class String type. String Full. Name, Firt. Name, Last. Name; String city; String zip. Code; String country; String title; String position; String Street. Address; String salutation; With these declarations, of these variables belong to String Class and there 4 have all the abilities of String class type. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 48
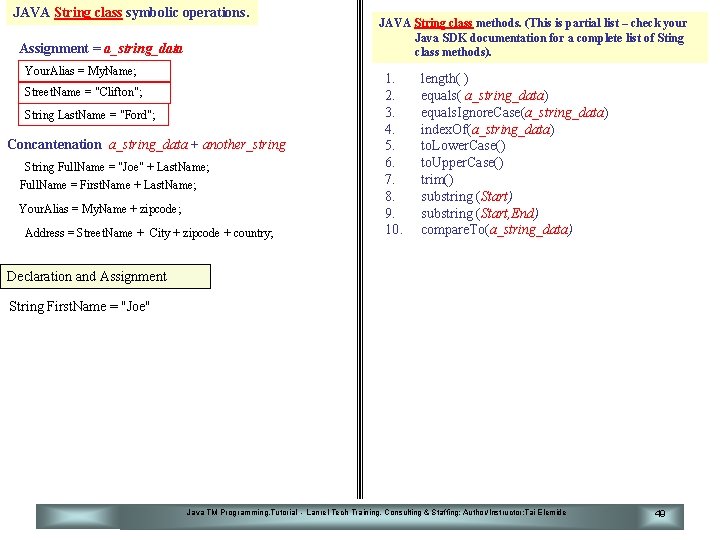
JAVA String class symbolic operations. Assignment = a_string_data Your. Alias = My. Name; Street. Name = "Clifton"; String Last. Name = "Ford"; Concantenation a_string_data + another_string String Full. Name = "Joe" + Last. Name; Full. Name = First. Name + Last. Name; Your. Alias = My. Name + zipcode; Address = Street. Name + City + zipcode + country; JAVA String class methods. (This is partial list – check your Java SDK documentation for a complete list of Sting class methods). 1. 2. 3. 4. 5. 6. 7. 8. 9. 10. length( ) equals( a_string_data) equals. Ignore. Case(a_string_data) index. Of(a_string_data) to. Lower. Case() to. Upper. Case() trim() substring (Start, End) compare. To(a_string_data) Declaration and Assignment String First. Name = "Joe" Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 49
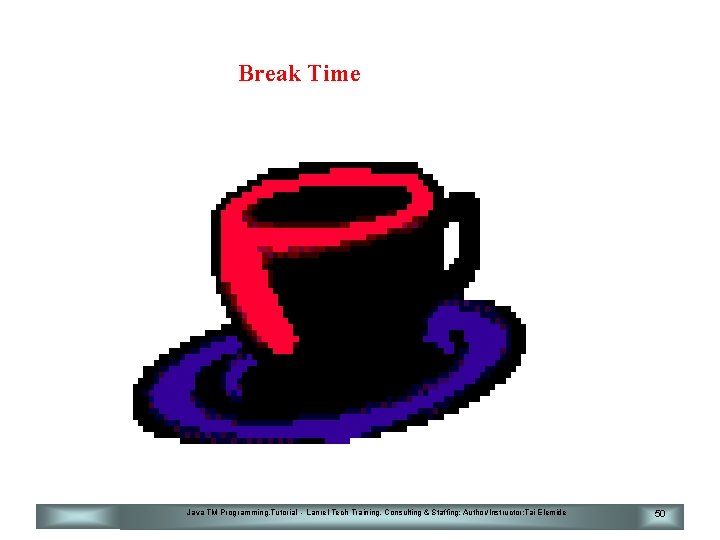
Break Time Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 50
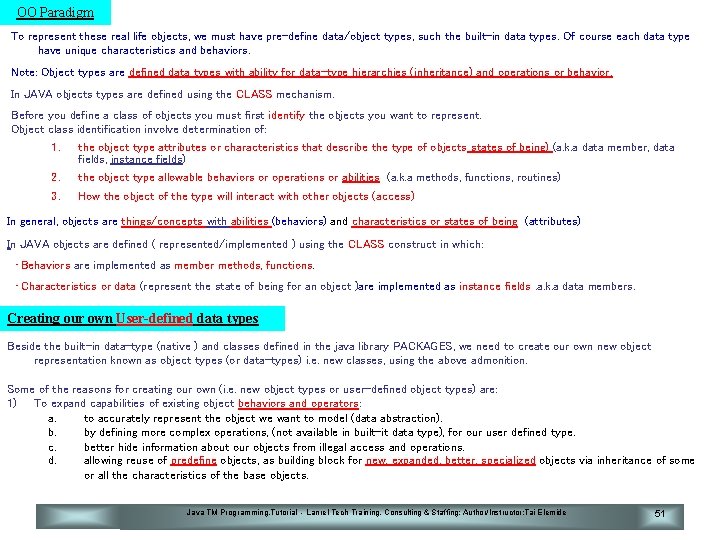
OO Paradigm To represent these real life objects, we must have pre-define data/object types, such the built-in data types. Of course each data type have unique characteristics and behaviors. Note: Object types are defined data types with ability for data-type hierarchies (inheritance) and operations or behavior. In JAVA objects types are defined using the CLASS mechanism. Before you define a class of objects you must first identify the objects you want to represent. Object class identification involve determination of: 1. the object type attributes or characteristics that describe the type of objects states of being) (a. k. a data member, data fields, instance fields) 2. the object type allowable behaviors or operations or abilities (a. k. a methods, functions, routines) 3. How the object of the type will interact with other objects (access) In general, objects are things/concepts with abilities (behaviors) and characteristics or states of being (attributes) In JAVA objects are defined ( represented/implemented ) using the CLASS construct in which: • Behaviors are implemented as member methods, functions. • Characteristics or data (represent the state of being for an object )are implemented as instance fields. a. k. a data members. Creating our own User-defined data types Beside the built-in data-type (native ) and classes defined in the java library PACKAGES, we need to create our own new object representation known as object types (or data-types) i. e. new classes, using the above admonition. Some of the reasons for creating our own (i. e. new object types or user-defined object types) are: 1) To expand capabilities of existing object behaviors and operators: a. to accurately represent the object we want to model (data abstraction). b. by defining more complex operations, (not available in built-it data type), for our user defined type. c. better hide information about our objects from illegal access and operations. d. allowing reuse of predefine objects, as building block for new, expanded, better, specialized objects via inheritance of some or all the characteristics of the base objects. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 51
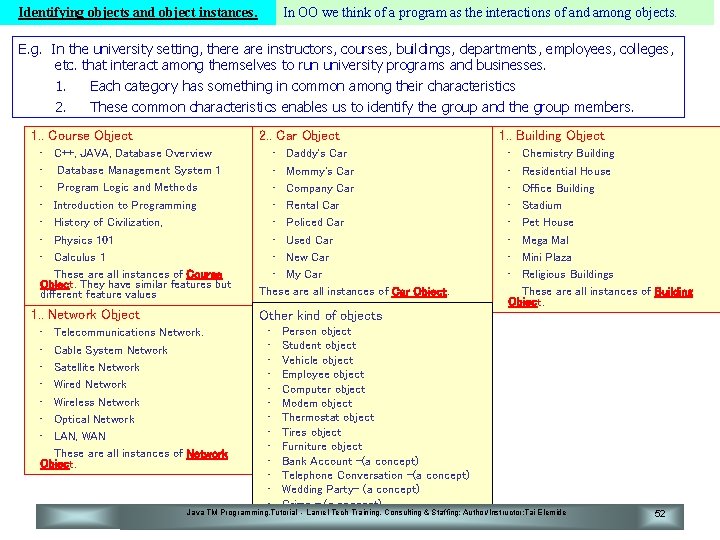
Identifying objects and object instances. In OO we think of a program as the interactions of and among objects. E. g. In the university setting, there are instructors, courses, buildings, departments, employees, colleges, etc. that interact among themselves to run university programs and businesses. 1. 2. Each category has something in common among their characteristics These common characteristics enables us to identify the group and the group members. 1. . Course Object 2. . Car Object • • C++, JAVA, Database Overview Database Management System 1 Program Logic and Methods Introduction to Programming History of Civilization, Physics 101 Calculus 1 These are all instances of Course Object. They have similar features but different feature values 1. . Network Object • • Daddy's Car • Mommy's Car • Company Car • Rental Car • Policed Car • Used Car • New Car • My Car These are all instances of Car Object. 1. . Building Object • • Chemistry Building Residential House Office Building Stadium Pet House Mega Mal Mini Plaza Religious Buildings These are all instances of Building Object. Other kind of objects • Person object • Student object • Vehicle object • Employee object • Computer object • Modem object • Thermostat object • Tires object • Furniture object • Bank Account –(a concept) • Telephone Conversation –(a concept) • Wedding Party– (a concept) • Crime – (a concept) Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide • Admission – (a concept) Telecommunications Network. Cable System Network Satellite Network Wired Network Wireless Network Optical Network LAN, WAN These are all instances of Network Object. 52
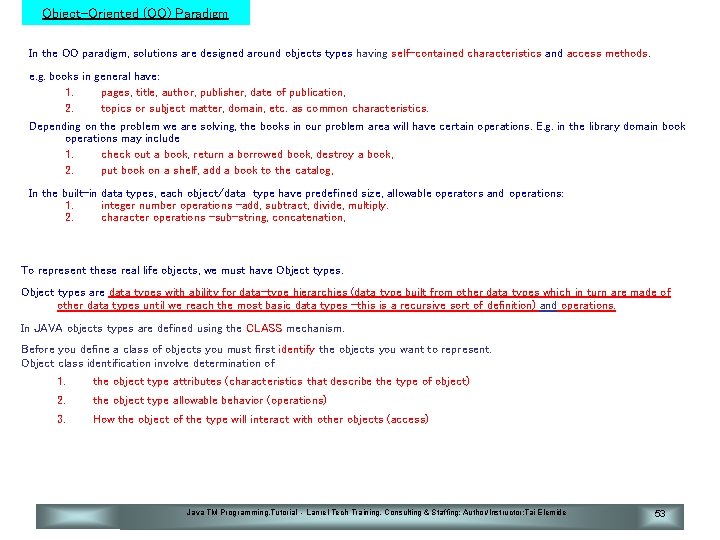
Object-Oriented (OO) Paradigm In the OO paradigm, solutions are designed around objects types having self-contained characteristics and access methods. e. g. books in general have: 1. pages, title, author, publisher, date of publication, 2. topics or subject matter, domain, etc. as common characteristics. Depending on the problem we are solving, the books in our problem area will have certain operations. E. g. in the library domain book operations may include 1. check out a book, return a borrowed book, destroy a book, 2. put book on a shelf, add a book to the catalog, In the built-in data types, each object/data type have predefined size, allowable operators and operations: 1. integer number operations -add, subtract, divide, multiply. 2. character operations –sub-string, concatenation, To represent these real life objects, we must have Object types are data types with ability for data-type hierarchies (data type built from other data types which in turn are made of other data types until we reach the most basic data types –this is a recursive sort of definition) and operations. In JAVA objects types are defined using the CLASS mechanism. Before you define a class of objects you must first identify the objects you want to represent. Object class identification involve determination of 1. the object type attributes (characteristics that describe the type of object) 2. 3. the object type allowable behavior (operations) How the object of the type will interact with other objects (access) Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 53
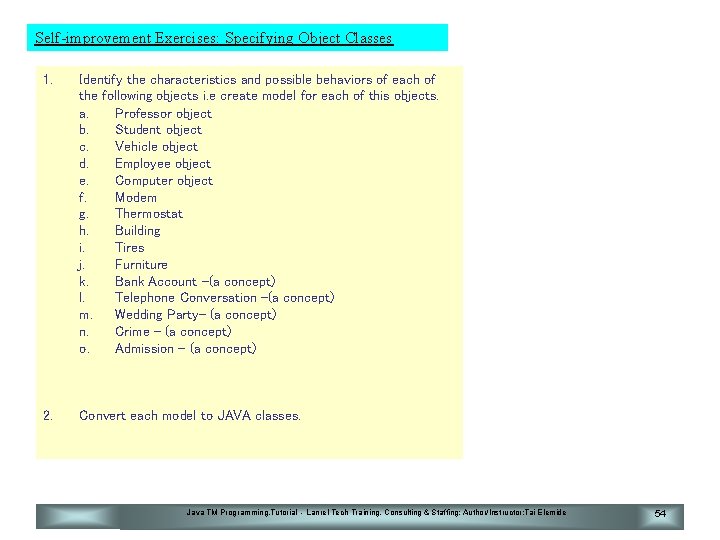
Self-improvement Exercises: Specifying Object Classes 1. Identify the characteristics and possible behaviors of each of the following objects i. e create model for each of this objects. a. Professor object b. Student object c. Vehicle object d. Employee object e. Computer object f. Modem g. Thermostat h. Building i. Tires j. Furniture k. Bank Account –(a concept) l. Telephone Conversation –(a concept) m. Wedding Party– (a concept) n. Crime – (a concept) o. Admission – (a concept) 2. Convert each model to JAVA classes. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 54
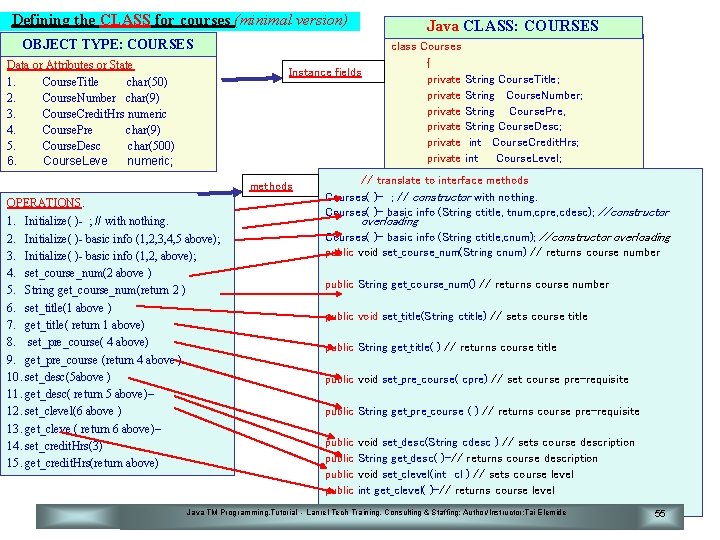
Defining the CLASS for courses (minimal version) Java CLASS: COURSES OBJECT TYPE: COURSES Data or Attributes or State 1. Course. Title char(50) 2. Course. Number char(9) 3. Course. Credit. Hrs numeric 4. Course. Pre char(9) 5. Course. Desc char(500) 6. Course. Leve numeric; Instance fields methods OPERATIONS. 1. Initialize( )- ; // with nothing. 2. Initialize( )- basic info (1, 2, 3, 4, 5 above); 3. Initialize( )- basic info (1, 2, above); 4. set_course_num(2 above ) 5. String get_course_num(return 2 ) 6. set_title(1 above ) 7. get_title( return 1 above) 8. set_pre_course( 4 above) 9. get_pre_course (return 4 above ) 10. set_desc(5 above ) 11. get_desc( return 5 above)– 12. set_clevel(6 above ) 13. get_cleve ( return 6 above)– 14. set_credit. Hrs(3) 15. get_credit. Hrs(return above) class Courses { private private String Course. Title; String Course. Number; String Course. Pre, String Course. Desc; int Course. Credit. Hrs; int Course. Level; // translate to interface methods Courses( )- ; // constructor with nothing. Courses( )- basic info (String ctitle, tnum, cpre, cdesc); //constructor overloading Courses( )- basic info (String ctitle, cnum); //constructor overloading public void set_course_num(String cnum) // returns course number public String get_course_num() // returns course number public void set_title(String ctitle) // sets course title public String get_title( ) // returns course title public void set_pre_course( cpre) // set course pre-requisite public String get_pre_course ( ) // returns course pre-requisite public void set_desc(String cdesc ) // sets course description String get_desc( )–// returns course description void set_clevel(int cl ) // sets course level int get_clevel( )–// returns course level Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 55
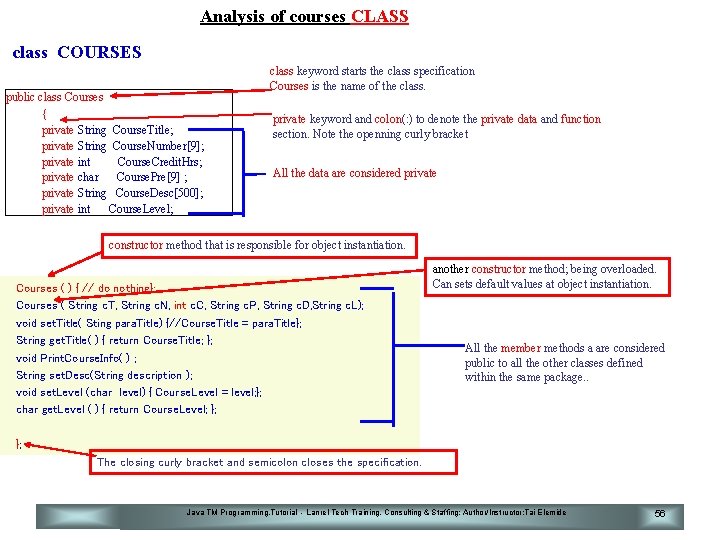
Analysis of courses CLASS class COURSES public class Courses { private String Course. Title; private String Course. Number[9]; private int Course. Credit. Hrs; private char Course. Pre[9] ; private String Course. Desc[500]; private int Course. Level; class keyword starts the class specification Courses is the name of the class. private keyword and colon(: ) to denote the private data and function section. Note the openning curly bracket All the data are considered private constructor method that is responsible for object instantiation. Courses ( ) { // do nothing}; Courses ( String c. T, String c. N, int c. C, String c. P, String c. D, String c. L); void set. Title( Sting para. Title) {//Course. Title = para. Title}; String get. Title( ) { return Course. Title; }; void Print. Course. Info( ) ; String set. Desc(String description ); void set. Level (char level) { Course. Level = level; }; char get. Level ( ) { return Course. Level; }; another constructor method; being overloaded. Can sets default values at object instantiation. All the member methods a are considered public to all the other classes defined within the same package. . }; The closing curly bracket and semicolon closes the specification. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 56
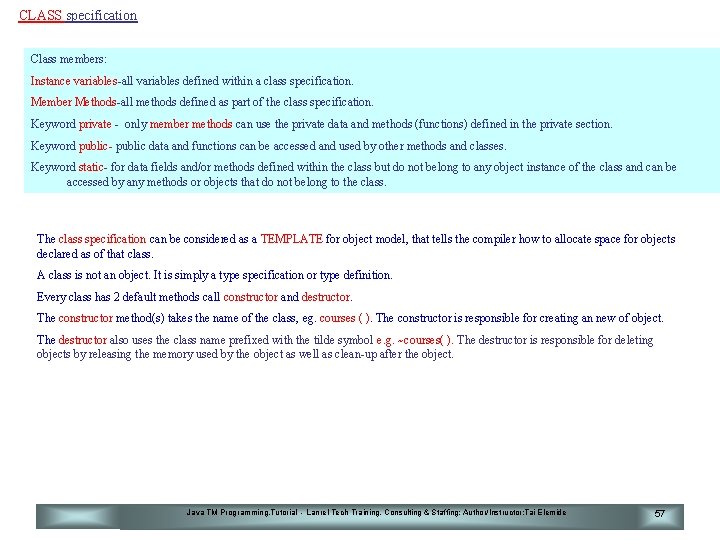
CLASS specification Class members: Instance variables-all variables defined within a class specification. Member Methods-all methods defined as part of the class specification. Keyword private - only member methods can use the private data and methods (functions) defined in the private section. Keyword public- public data and functions can be accessed and used by other methods and classes. Keyword static- for data fields and/or methods defined within the class but do not belong to any object instance of the class and can be accessed by any methods or objects that do not belong to the class. The class specification can be considered as a TEMPLATE for object model, that tells the compiler how to allocate space for objects declared as of that class. A class is not an object. It is simply a type specification or type definition. Every class has 2 default methods call constructor and destructor. The constructor method(s) takes the name of the class, eg. courses ( ). The constructor is responsible for creating an new of object. The destructor also uses the class name prefixed with the tilde symbol e. g. ~courses( ). The destructor is responsible for deleting objects by releasing the memory used by the object as well as clean-up after the object. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 57
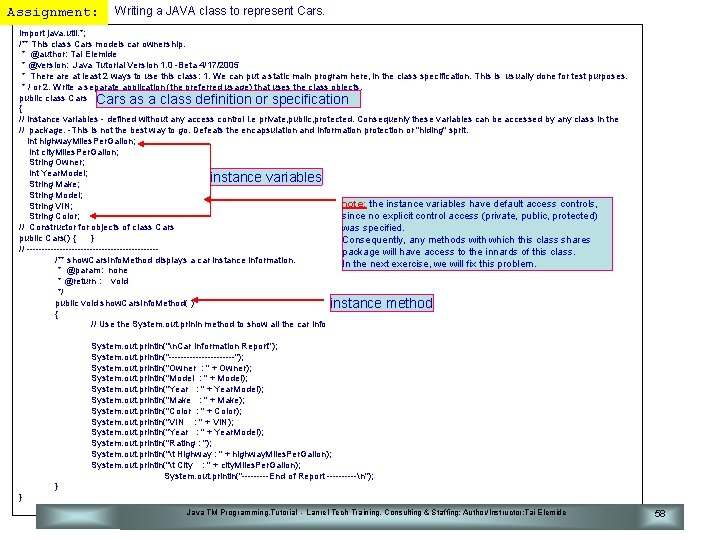
Assignment: Writing a JAVA class to represent Cars. import java. util. *; /** This class Cars models car ownership. * @author: Tai Elemide * @version: Java Tutorial Version 1. 0 -Beta 4/17/2005 * There at least 2 ways to use this class: 1. We can put a static main program here, in the class specification. This is usually done for test purposes. * / or 2. Write a separate application (the preferred usage) that uses the class objects. public class Cars as a class definition or specification { // instance variables - defined without any access control i. e private, public, protected. Consequenly these variables can be accessed by any class in the // package. -This is not the best way to go. Defeats the encapsulation and information protection or "hiding" sprit. int highway. Miles. Per. Gallon; int city. Miles. Per. Gallon; String Owner; int Year. Model; instance variables String Make; String Model; note: the instance variables have default access controls, String VIN; since no explicit control access (private, public, protected) String Color; // Constructor for objects of class Cars was specified. public Cars() { } Consequently, any methods with which this class shares // ----------------------package will have access to the innards of this class. /** show. Cars. Info. Method displays a car instance information. In the next exercise, we will fix this problem. * @param: none * @return : void */ public void show. Cars. Info. Method( ) instance method { // Use the System. out. prinln method to show all the car info System. out. println("n. Car Information Report"); System. out. println("-----------"); System. out. println("Owner : " + Owner); System. out. println("Model : " + Model); System. out. println("Year : " + Year. Model); System. out. println("Make : " + Make); System. out. println("Color : " + Color); System. out. println("VIN : " + VIN); System. out. println("Year : " + Year. Model); System. out. println("Rating : "); System. out. println("t Highway : " + highway. Miles. Per. Gallon); System. out. println("t City : " + city. Miles. Per. Gallon); System. out. println("-----End of Report -----n"); } } Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 58
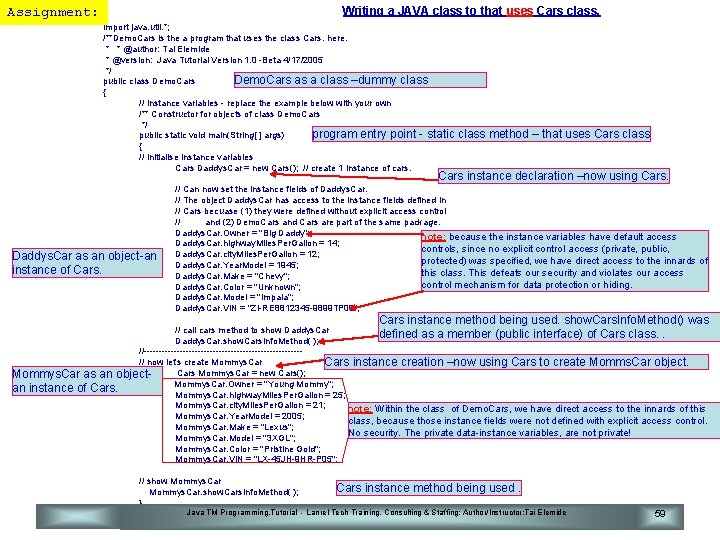
Assignment: Writing a JAVA class to that uses Cars class. import java. util. *; /**Demo. Cars is the a program that uses the class Cars. here. * * @author: Tai Elemide * @version: Java Tutorial Version 1. 0 -Beta 4/17/2005 */ Demo. Cars as a class –dummy class public class Demo. Cars { // instance variables - replace the example below with your own /** Constructor for objects of class Demo. Cars */ program entry point - static public static void main(String[ ] args) { // initialise instance variables Cars Daddys. Car = new Cars(); // create 1 instance of cars. class method – that uses Cars class Cars instance declaration –now using Cars. Daddys. Car as an object-an instance of Cars. // Can now set the instance fields of Daddys. Car. // The object Daddys. Car has access to the instance fields defined in // Cars becuase (1) they were defined without explicit access control // and (2) Demo. Cars and Cars are part of the same package. Daddys. Car. Owner = "Big Daddy"; note: because the instance variables have default access Daddys. Car. highway. Miles. Per. Gallon = 14; controls, since no explicit control access (private, public, Daddys. Car. city. Miles. Per. Gallon = 12; protected) was specified, we have direct access to the innards of Daddys. Car. Year. Model = 1945; this class. This defeats our security and violates our access Daddys. Car. Make = "Chevy"; control mechanism for data protection or hiding. Daddys. Car. Color = "Unknown"; Daddys. Car. Model = "Impala"; Daddys. Car. VIN = "ZI-RE 8812345 -9899 TP 09"; Cars instance method being used. show. Cars. Info. Method() was // call cars method to show Daddys. Car defined as a member (public interface) of Cars class. . Daddys. Car. show. Cars. Info. Method( ); //--------------------------// now let's create Mommys. Cars instance creation –now using Cars to create Momms. Car object. Cars Mommys. Car = new Cars(); Mommys. Car as an object. Mommys. Car. Owner = "Young Mommy"; an instance of Cars. Mommys. Car. highway. Miles. Per. Gallon = 25; Mommys. Car. city. Miles. Per. Gallon = 21; note: Within the class of Demo. Cars, we have direct access to the innards of this Mommys. Car. Year. Model = 2005; class, because those instance fields were not defined with explicit access control. Mommys. Car. Make = "Lexus"; No security. The private data-instance variables, are not private! Mommys. Car. Model = "3 XGL"; Mommys. Car. Color = "Pristine Gold"; Mommys. Car. VIN = "LX-45 JH-9 HR-P 05"; // show Mommys. Car. show. Cars. Info. Method( ); } } Cars instance method being used. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 59
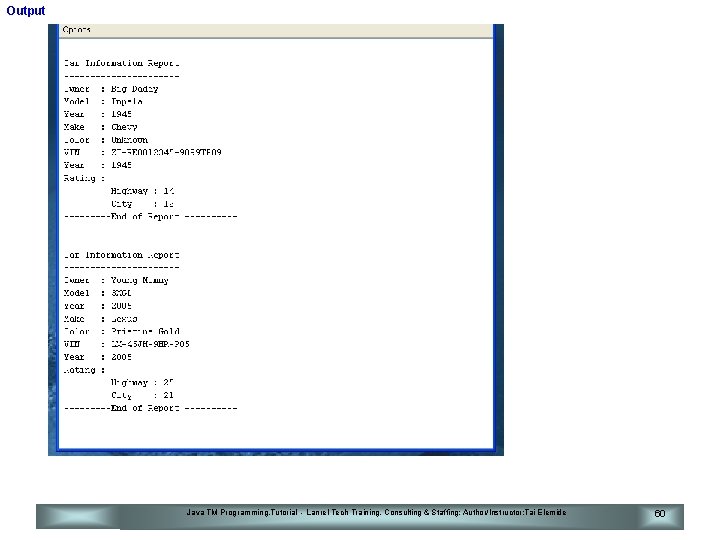
Output Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 60
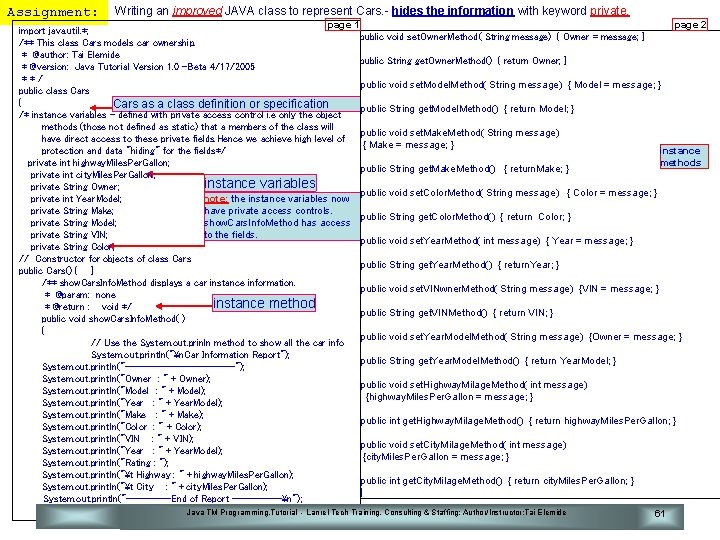
Assignment: Writing an improved JAVA class to represent Cars. - hides the information with keyword private. page 1 page 2 import java. util. *; public void set. Owner. Method( String message) { Owner = message; } /** This class Cars models car ownership. * @author: Tai Elemide public String get. Owner. Method() { return Owner; } * @version: Java Tutorial Version 1. 0 -Beta 4/17/2005 **/ public void set. Model. Method( String message) { Model = message; } public class Cars { Cars as a class definition or specification public String get. Model. Method() { return Model; } /* instance variables - defined with private access control i. e only the object methods (those not defined as static) that a members of the class will public void set. Make. Method( String message) have direct access to these private fields. Hence we achieve high level of { Make = message; } instance protection and data "hiding" for the fields*/ methods private int highway. Miles. Per. Gallon; public String get. Make. Method() { return. Make; } private int city. Miles. Per. Gallon; instance variables private String Owner; public void set. Color. Method( String message) { Color = message; } note: the instance variables now private int Year. Model; have private access controls. private String Make; public String get. Color. Method() { return Color; } show. Cars. Info. Method has access private String Model; to the fields. private String VIN; public void set. Year. Method( int message) { Year = message; } private String Color; // Constructor for objects of class Cars public String get. Year. Method() { return. Year; } public Cars() { } /** show. Cars. Info. Method displays a car instance information. public void set. VINwner. Method( String message) {VIN = message; } * @param: none instance method * @return : void */ public String get. VINMethod() { return VIN; } public void show. Cars. Info. Method( ) { public void set. Year. Model. Method( String message) {Owner = message; } // Use the System. out. prinln method to show all the car info System. out. println("n. Car Information Report"); public String get. Year. Model. Method() { return Year. Model; } System. out. println("-----------"); System. out. println("Owner : " + Owner); public void set. Highway. Milage. Method( int message) System. out. println("Model : " + Model); {highway. Miles. Per. Gallon = message; } System. out. println("Year : " + Year. Model); System. out. println("Make : " + Make); public int get. Highway. Milage. Method() { return highway. Miles. Per. Gallon; } System. out. println("Color : " + Color); System. out. println("VIN : " + VIN); public void set. City. Milage. Method( int message) System. out. println("Year : " + Year. Model); {city. Miles. Per. Gallon = message; } System. out. println("Rating : "); System. out. println("t Highway : " + highway. Miles. Per. Gallon); public int get. City. Milage. Method() { return city. Miles. Per. Gallon; } System. out. println("t City : " + city. Miles. Per. Gallon); } System. out. println("-----End of Report -----n"); } Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 61
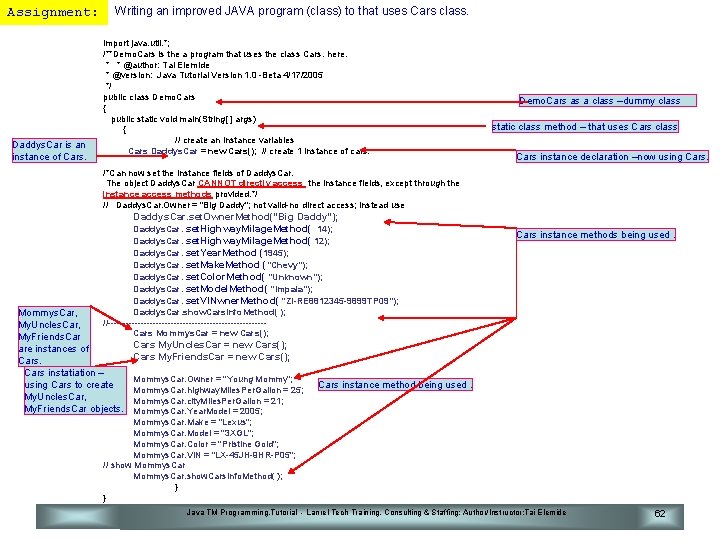
Assignment: Daddys. Car is an instance of Cars. Writing an improved JAVA program (class) to that uses Cars class. import java. util. *; /**Demo. Cars is the a program that uses the class Cars. here. * * @author: Tai Elemide * @version: Java Tutorial Version 1. 0 -Beta 4/17/2005 */ public class Demo. Cars { public static void main(String[ ] args) { // create an instance variables Cars Daddys. Car = new Cars(); // create 1 instance of cars. Demo. Cars as a class –dummy class static class method – that uses Cars class Cars instance declaration –now using Cars. /*Can now set the instance fields of Daddys. Car. The object Daddys. Car CANNOT directly access the instance fields, except through the instance access methods provided. */ // Daddys. Car. Owner = "Big Daddy"; not valid-no direct access; instead use Daddys. Car. set. Owner. Method("Big Daddy"); Daddys. Car. set. Highway. Milage. Method( 14); Daddys. Car. set. Highway. Milage. Method( 12); Daddys. Car. set. Year. Method (1945); Daddys. Car. set. Make. Method ("Chevy"); Daddys. Car. set. Color. Method( "Unknown"); Daddys. Car. set. Model. Method( "Impala"); Daddys. Car. set. VINwner. Method( "ZI-RE 8812345 -9899 TP 09"); Daddys. Car. show. Cars. Info. Method( ); //--------------------------Cars Mommys. Car = new Cars(); Mommys. Car, My. Uncles. Car, My. Friends. Car are instances of Cars instatiation – using Cars to create My. Uncles. Car, My. Friends. Car objects. Cars instance methods being used. Cars My. Uncles. Car = new Cars(); Cars My. Friends. Car = new Cars(); Mommys. Car. Owner = "Young Mommy"; Mommys. Car. highway. Miles. Per. Gallon = 25; Mommys. Car. city. Miles. Per. Gallon = 21; Mommys. Car. Year. Model = 2005; Mommys. Car. Make = "Lexus"; Mommys. Car. Model = "3 XGL"; Mommys. Car. Color = "Pristine Gold"; Mommys. Car. VIN = "LX-45 JH-9 HR-P 05"; // show Mommys. Car. show. Cars. Info. Method( ); } } Cars instance method being used. Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 62
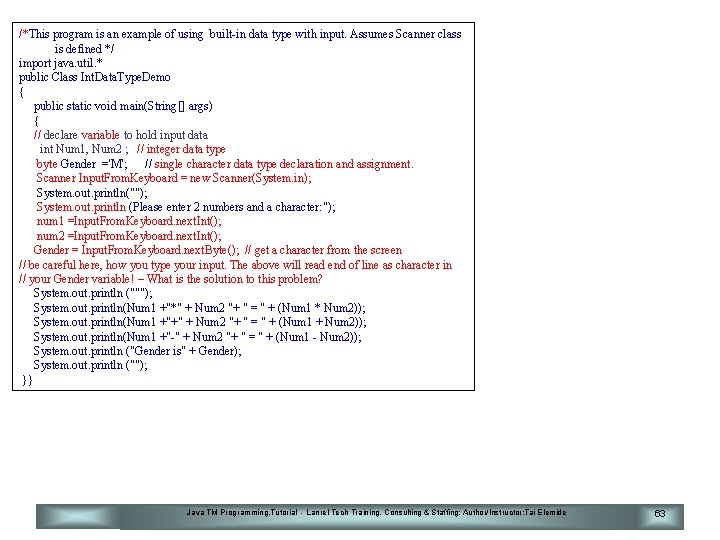
/*This program is an example of using built-in data type with input. Assumes Scanner class is defined */ import java. util. * public Class Int. Data. Type. Demo { public static void main(String[] args) { // declare variable to hold input data int Num 1, Num 2 ; // integer data type byte Gender ='M'; // single character data type declaration and assignment. Scanner Input. From. Keyboard = new Scanner(System. in); System. out. println(""); System. out. println (Please enter 2 numbers and a character: "); num 1 =Input. From. Keyboard. next. Int(); num 2 =Input. From. Keyboard. next. Int(); Gender = Input. From. Keyboard. next. Byte(); // get a character from the screen // be careful here, how you type your input. The above will read end of line as character in // your Gender variable! – What is the solution to this problem? System. out. println ("""); System. out. println(Num 1 +"*" + Num 2 "+ " = " + (Num 1 * Num 2)); System. out. println(Num 1 +"+" + Num 2 "+ " = " + (Num 1 + Num 2)); System. out. println(Num 1 +"-" + Num 2 "+ " = " + (Num 1 - Num 2)); System. out. println ("Gender is" + Gender); System. out. println (""); }} Java TM Programming. Tutorial - Lanrel Tech Training, Consulting & Staffing; Author/Instructor: Tai Elemide 63
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
System programming vs application programming
Linear vs integer programming
Definisi integer
Lc3 examples
Dsp programming tutorial
Lc3 programming tutorial
Logic programming tutorial
Microprocessor programming tutorial
Constraint programming tutorial
Microprocessor programming tutorial
Microprocessor programming tutorial
Machine learning octave
Microstation mdl programming tutorial pdf
Assembly mips tutorial
Flash mx tutorial
Structured programming tutorial
Android udp client
What is parallel programming in java
Java advanced exercises
Problem solving
Event driven programming in java
Daniel liang introduction to java programming
Java asynchronous programming
Java structured programming
Importance of java programming
Khan academy object oriented programming
Event driven programming in java
Defensive programming java
Java refresher course
Java games programming
Java programming symbols
Java introduction to problem solving and programming
Programming c
Java database programming
Java asynchronous programming
Conclusion of java
Java programming
Elementary programming in java
Programming in java
Advanced programming in java
Java programming language
Java enterprise architecture
Dangling else java
Java introduction to problem solving and programming
Introduction to java programming 10th edition quizzes
Xml dom tutorial
What is jsp file
Tutorial java swing
Java jade tutorial
Gui programmierung java
Corba java tutorial
Tutorial jsp
Java agent development framework tutorial
Common object request broker architecture
Mpi express
Java swing tutorial
Tdd tutorial java
Rhino java
Java agent development framework
Import java.lang.*
Java import java.util.*
Import java.applet.*