Java InputOutput Java Inputoutput Input is any information
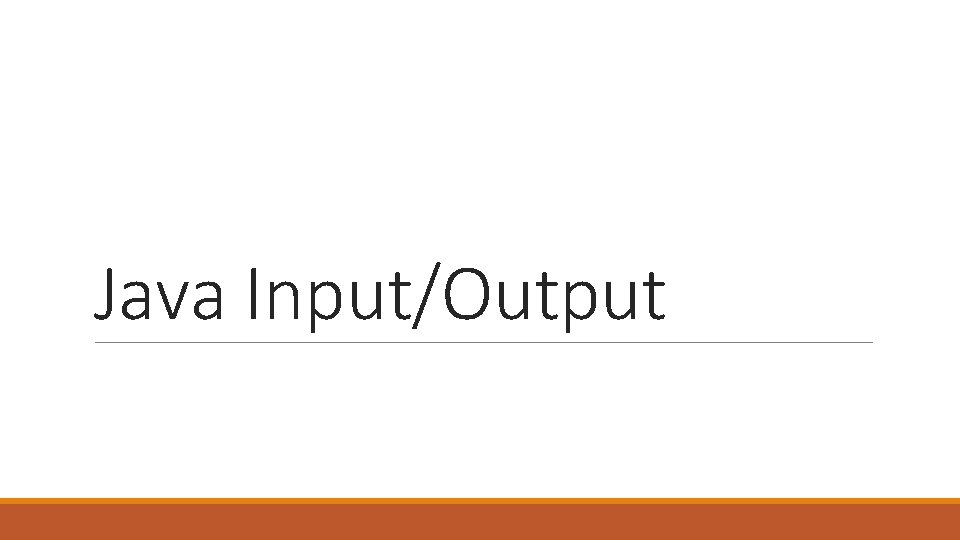
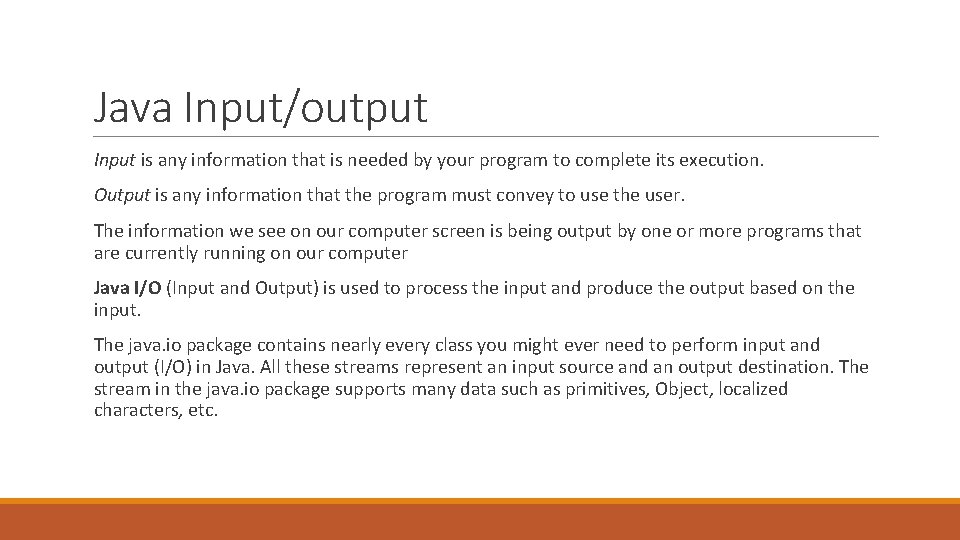
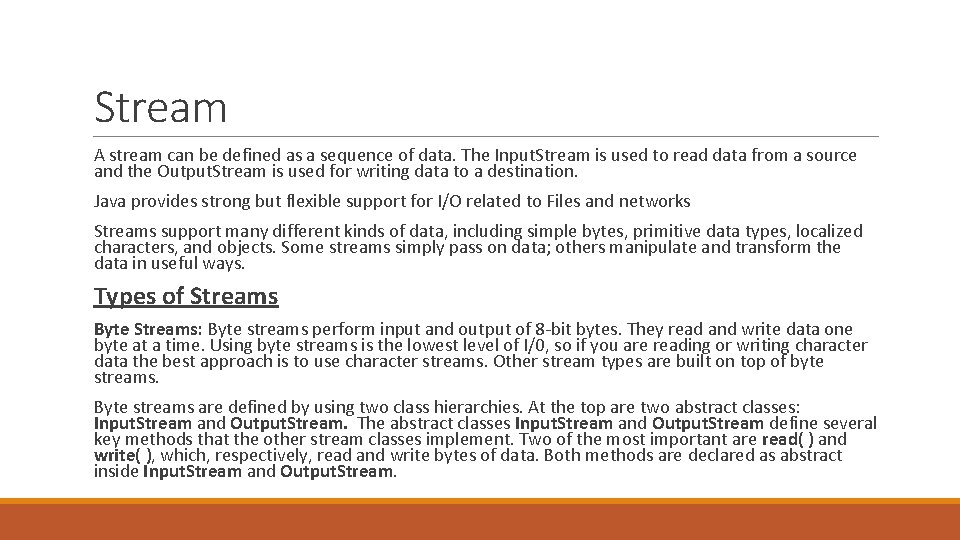
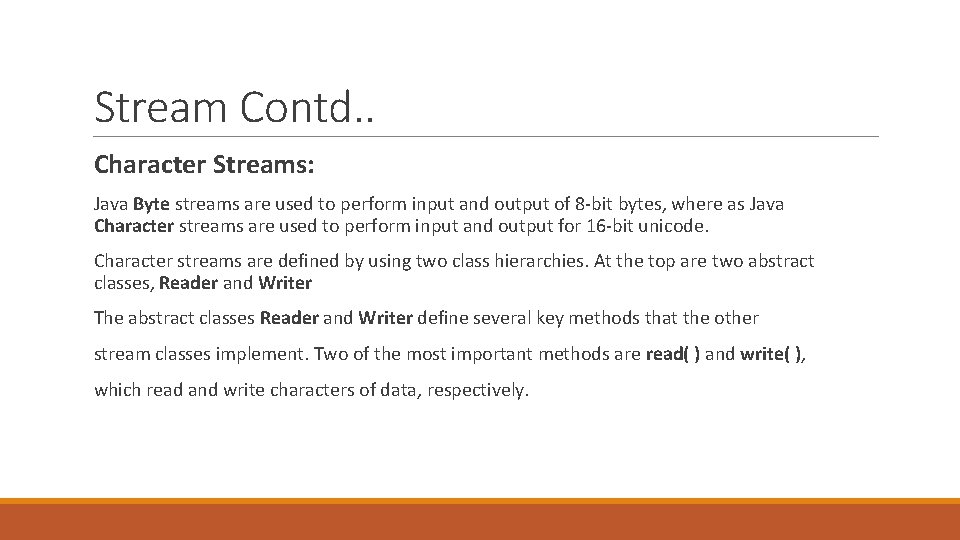
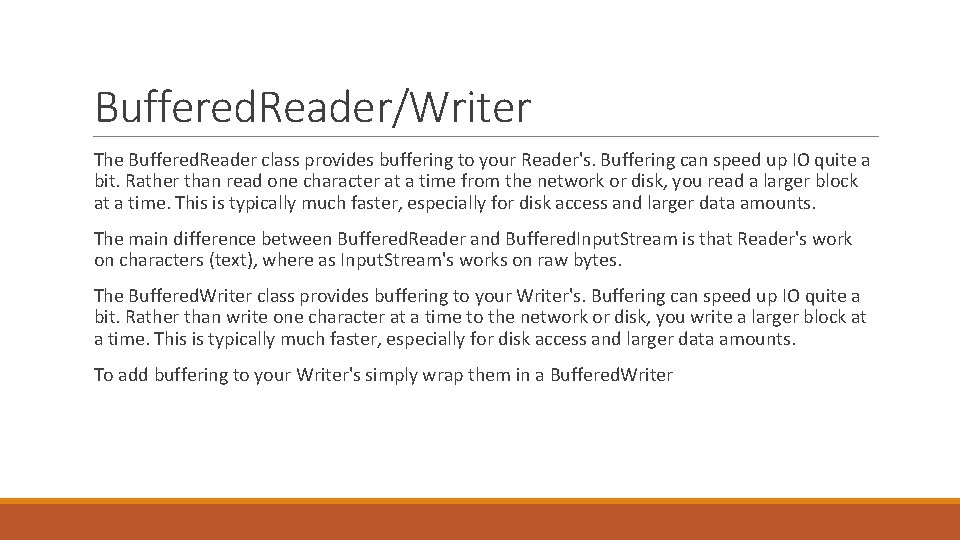
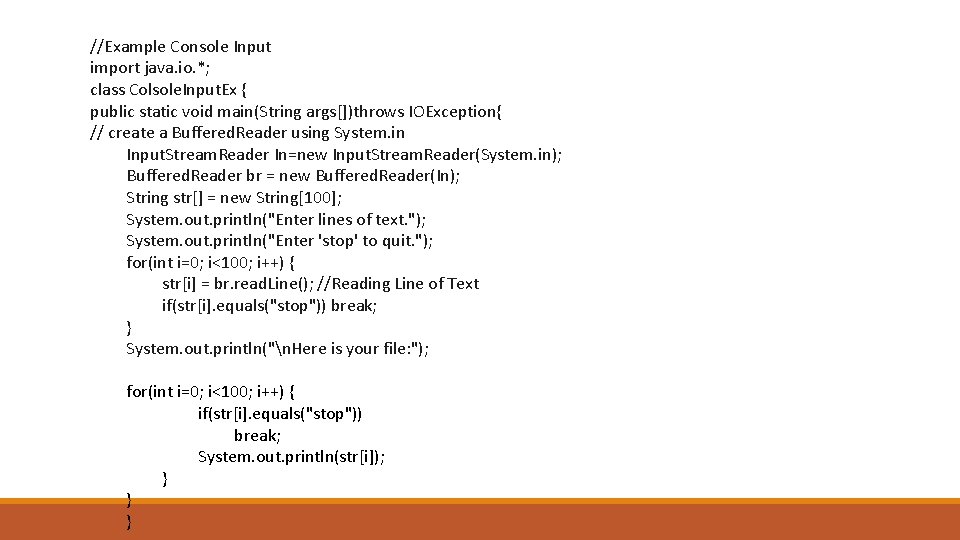
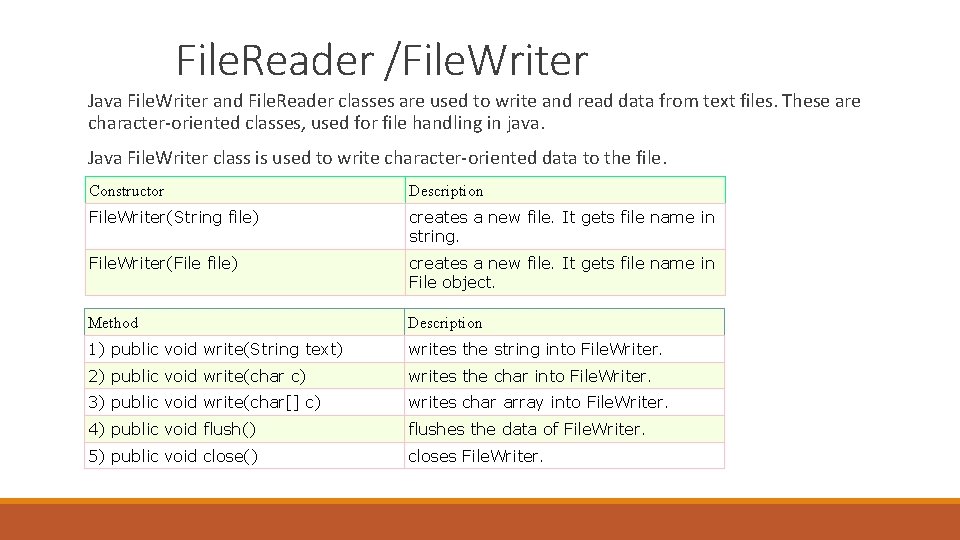
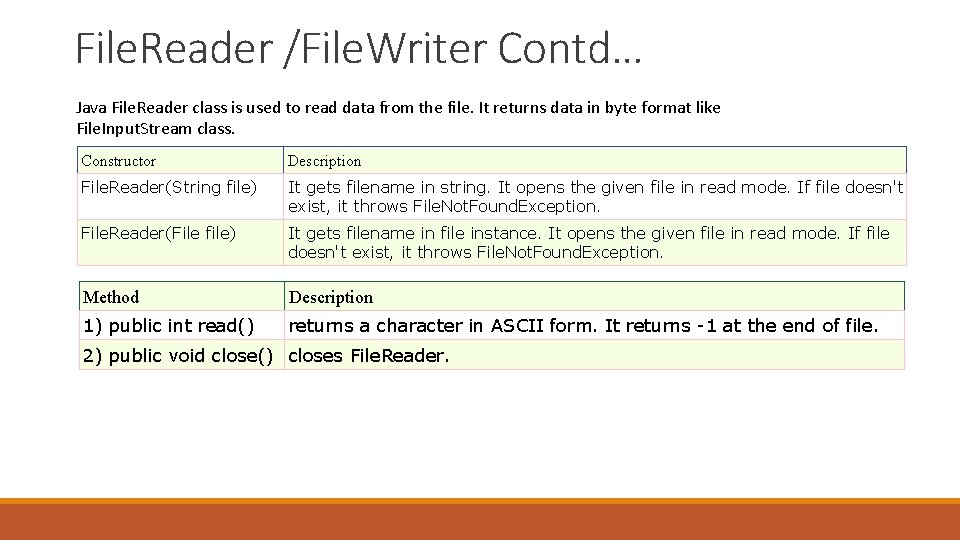
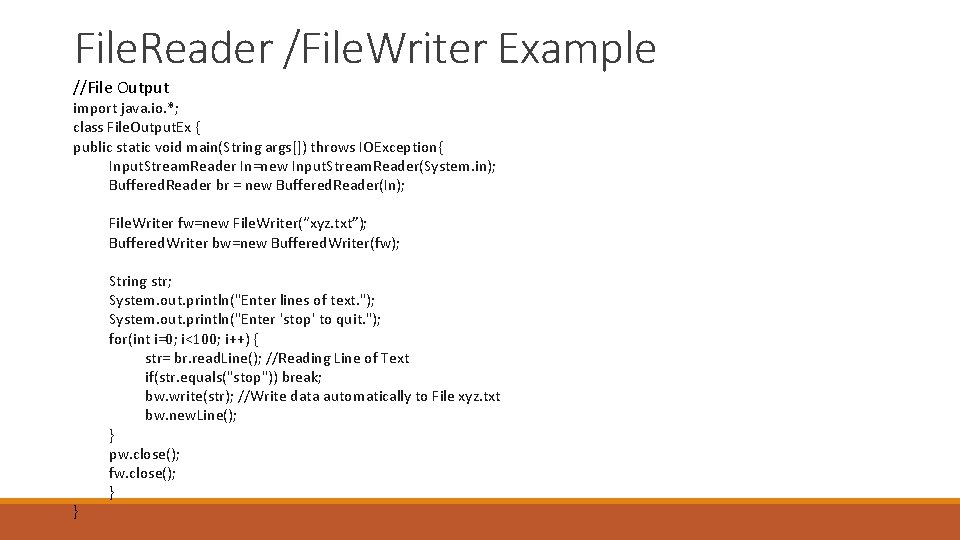
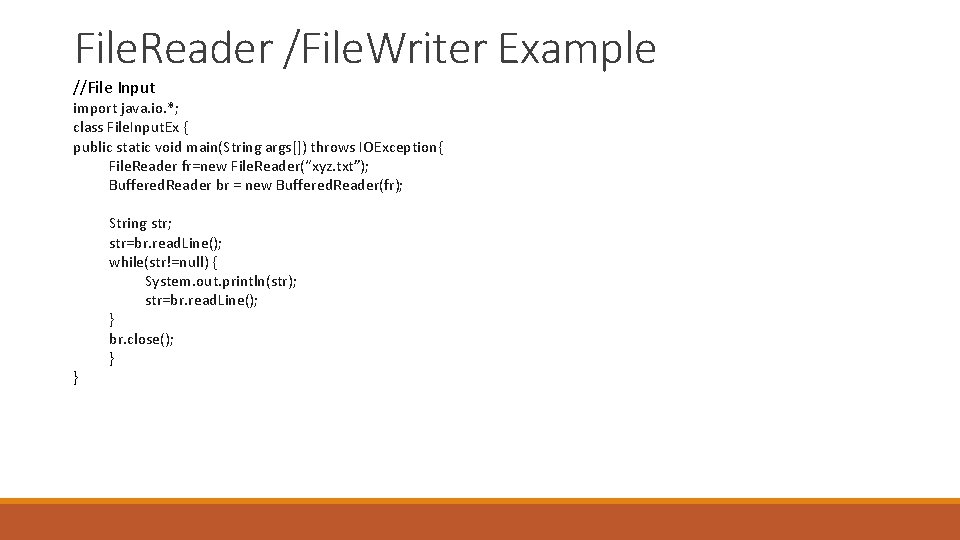
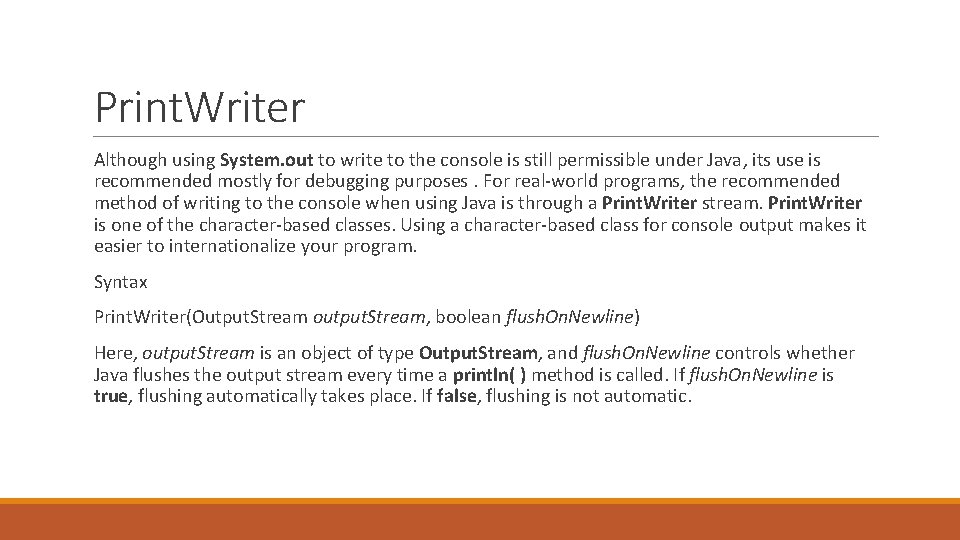
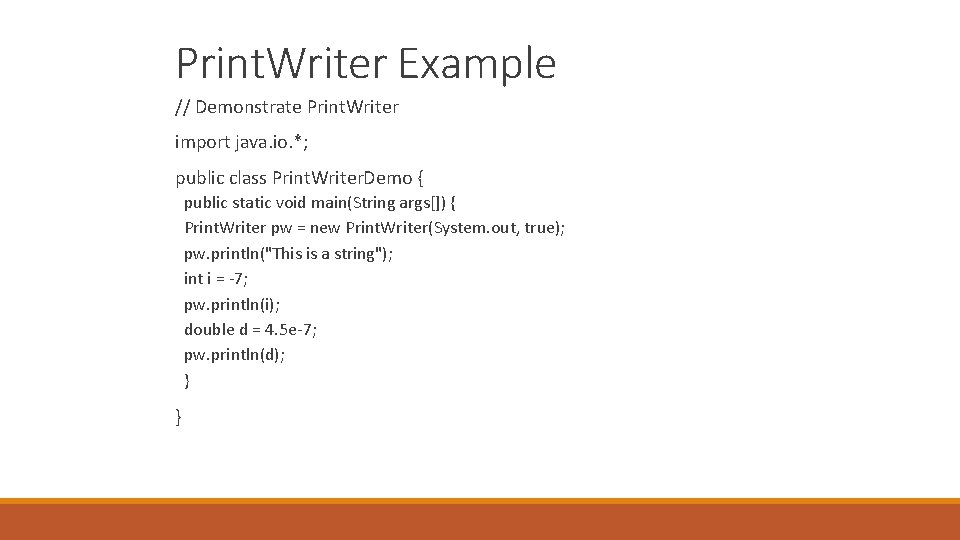
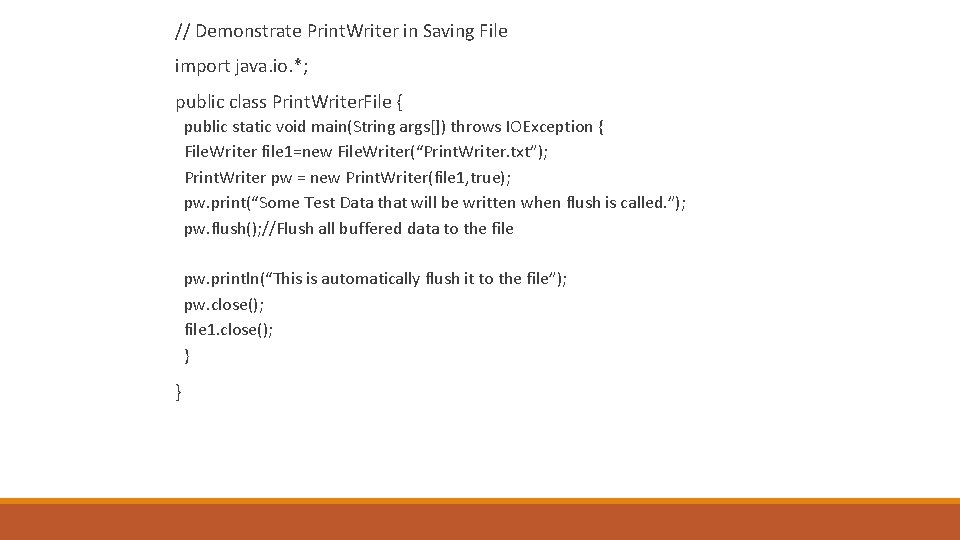
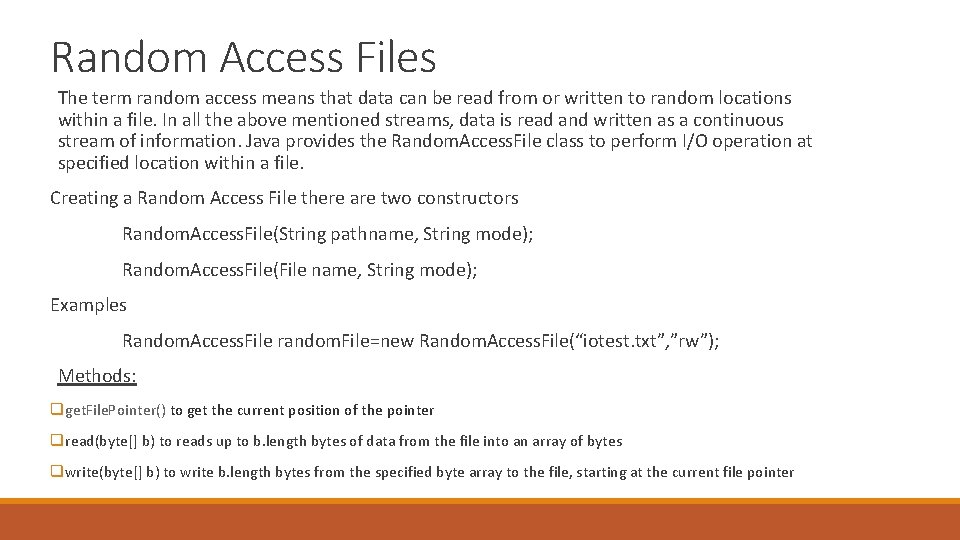
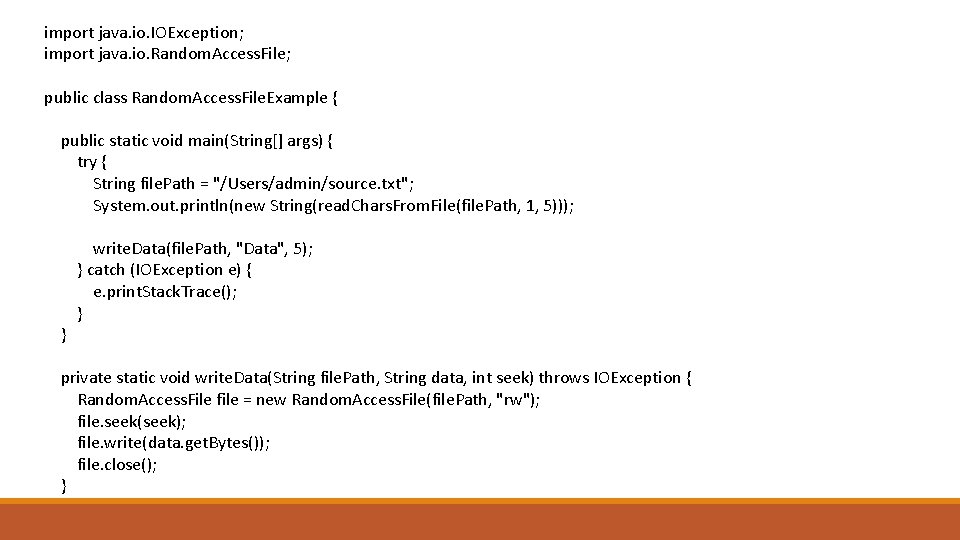
![private static byte[] read. Chars. From. File(String file. Path, int seek, int chars) private static byte[] read. Chars. From. File(String file. Path, int seek, int chars)](https://slidetodoc.com/presentation_image/aafe947d41f355bc9ad317a59ecc91f6/image-16.jpg)
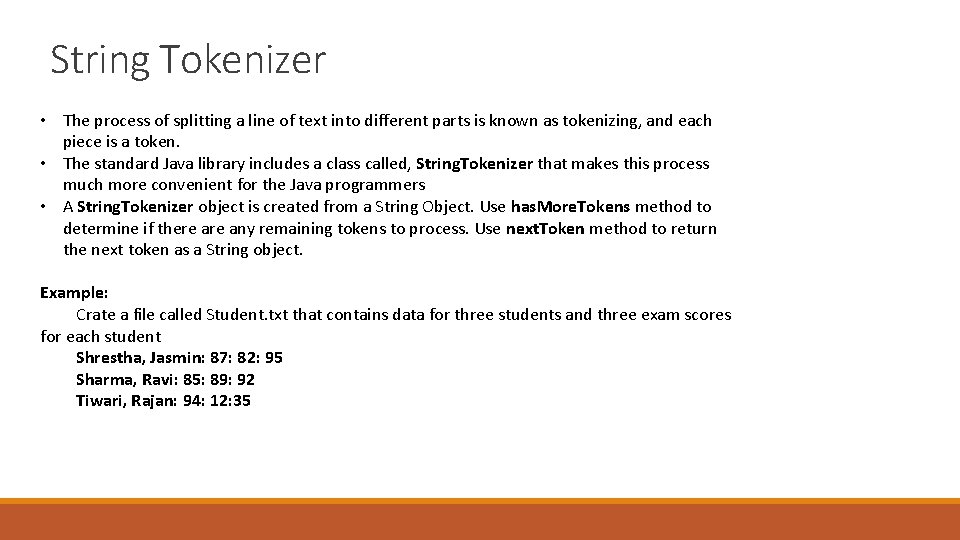
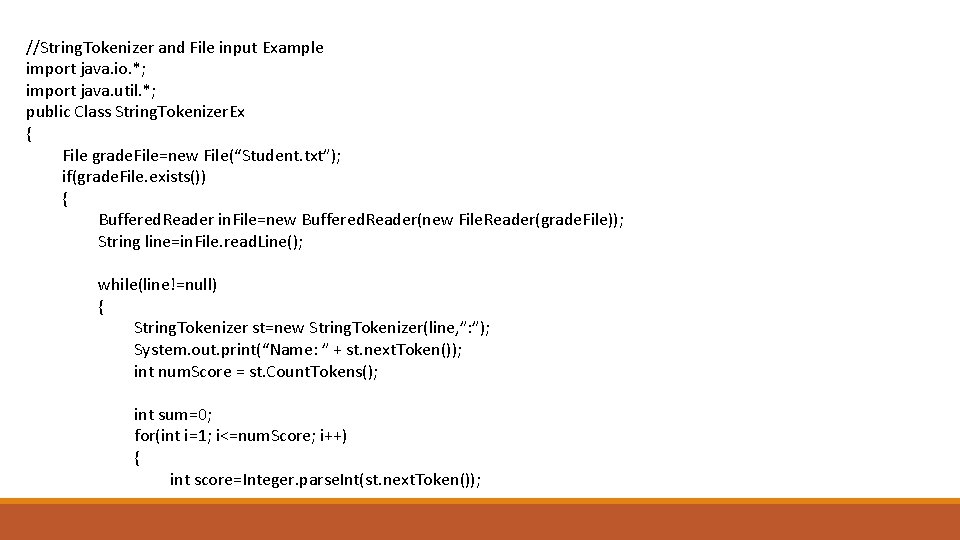
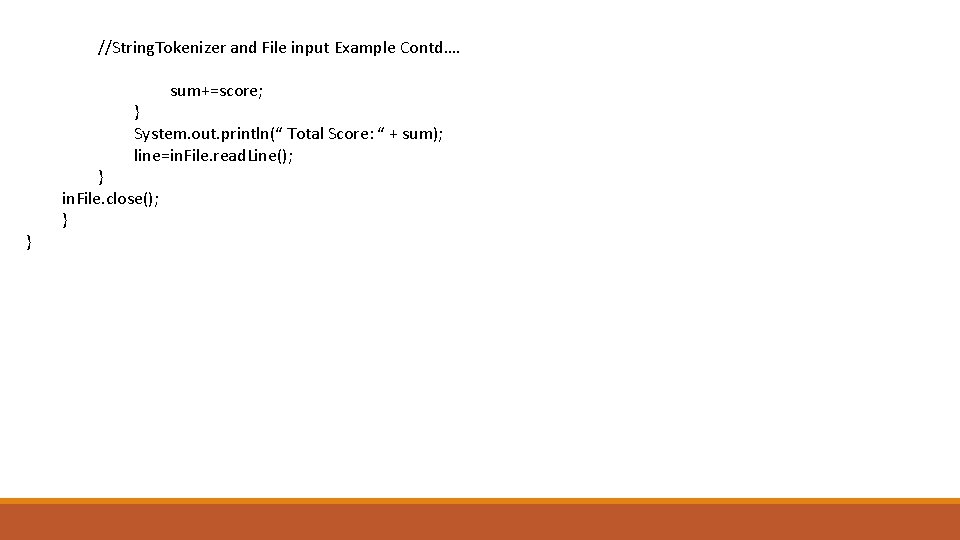
- Slides: 19
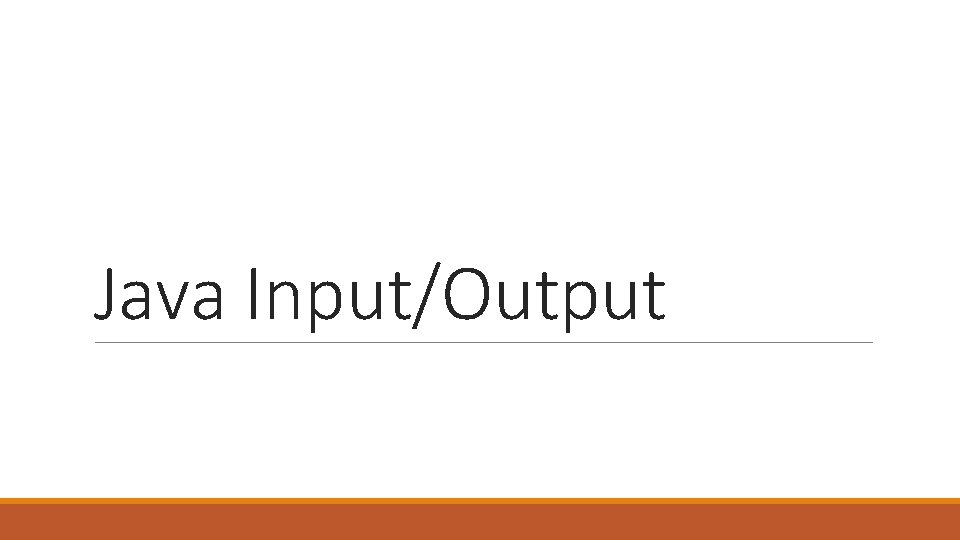
Java Input/Output
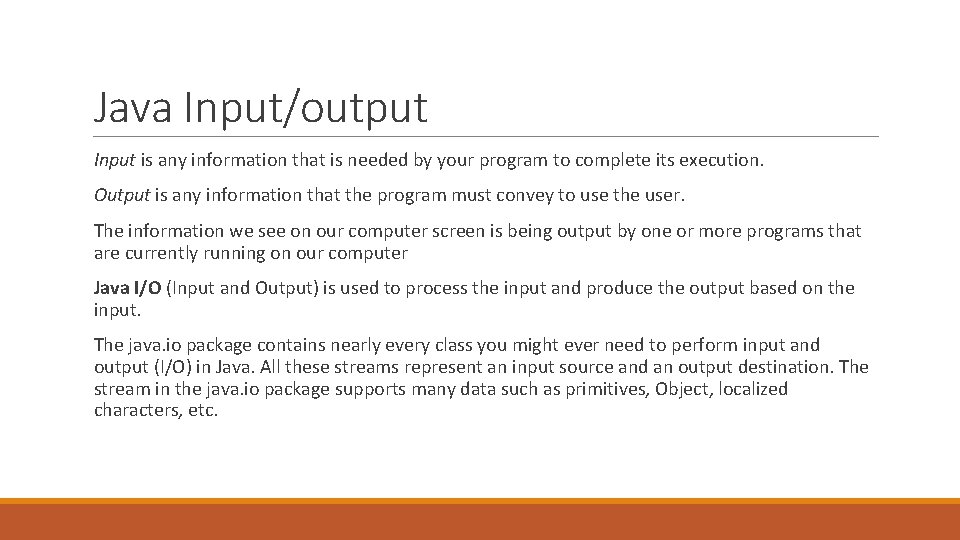
Java Input/output Input is any information that is needed by your program to complete its execution. Output is any information that the program must convey to use the user. The information we see on our computer screen is being output by one or more programs that are currently running on our computer Java I/O (Input and Output) is used to process the input and produce the output based on the input. The java. io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java. io package supports many data such as primitives, Object, localized characters, etc.
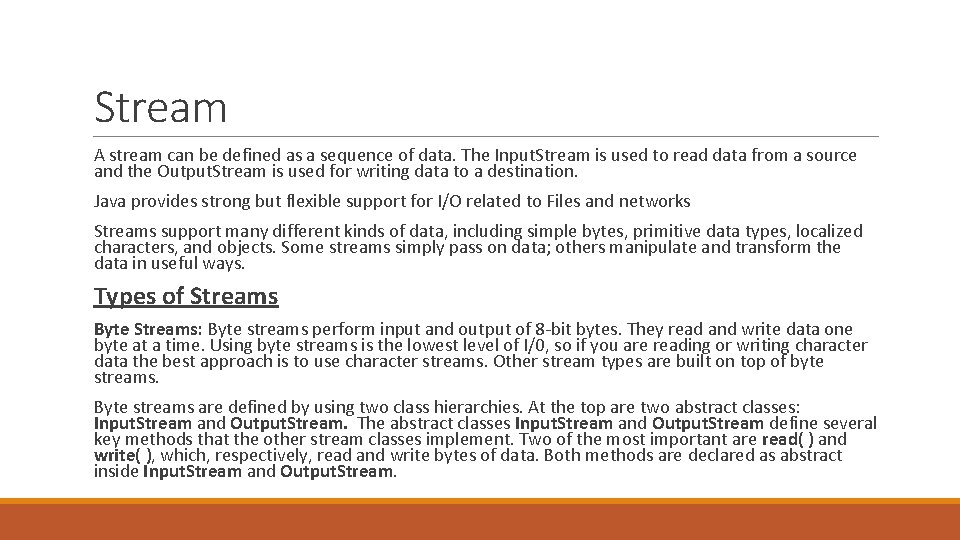
Stream A stream can be defined as a sequence of data. The Input. Stream is used to read data from a source and the Output. Stream is used for writing data to a destination. Java provides strong but flexible support for I/O related to Files and networks Streams support many different kinds of data, including simple bytes, primitive data types, localized characters, and objects. Some streams simply pass on data; others manipulate and transform the data in useful ways. Types of Streams Byte Streams: Byte streams perform input and output of 8 -bit bytes. They read and write data one byte at a time. Using byte streams is the lowest level of I/0, so if you are reading or writing character data the best approach is to use character streams. Other stream types are built on top of byte streams. Byte streams are defined by using two class hierarchies. At the top are two abstract classes: Input. Stream and Output. Stream. The abstract classes Input. Stream and Output. Stream define several key methods that the other stream classes implement. Two of the most important are read( ) and write( ), which, respectively, read and write bytes of data. Both methods are declared as abstract inside Input. Stream and Output. Stream.
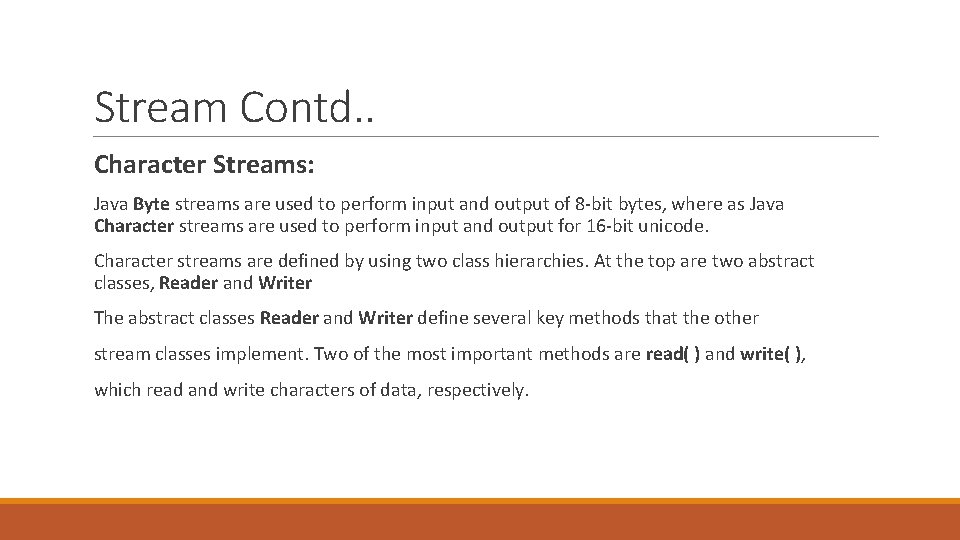
Stream Contd. . Character Streams: Java Byte streams are used to perform input and output of 8 -bit bytes, where as Java Character streams are used to perform input and output for 16 -bit unicode. Character streams are defined by using two class hierarchies. At the top are two abstract classes, Reader and Writer The abstract classes Reader and Writer define several key methods that the other stream classes implement. Two of the most important methods are read( ) and write( ), which read and write characters of data, respectively.
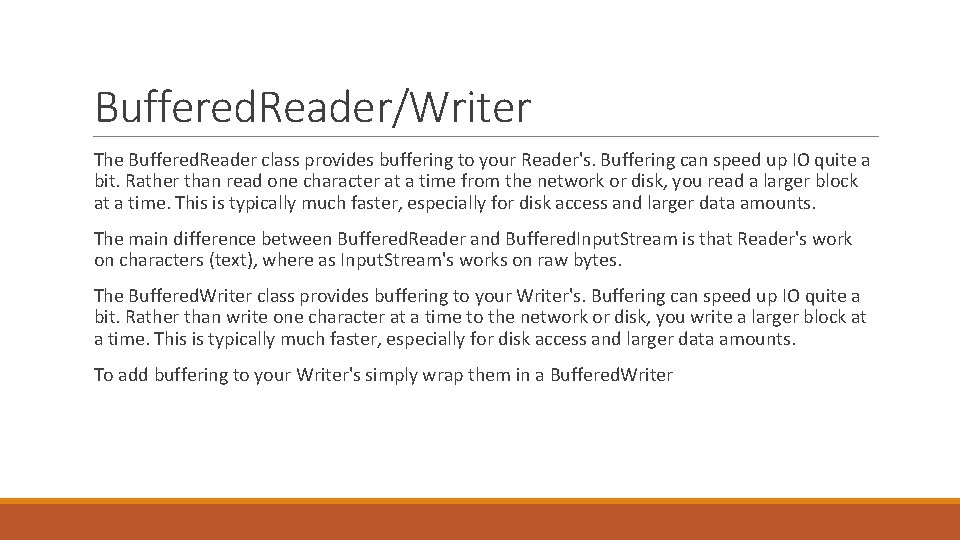
Buffered. Reader/Writer The Buffered. Reader class provides buffering to your Reader's. Buffering can speed up IO quite a bit. Rather than read one character at a time from the network or disk, you read a larger block at a time. This is typically much faster, especially for disk access and larger data amounts. The main difference between Buffered. Reader and Buffered. Input. Stream is that Reader's work on characters (text), where as Input. Stream's works on raw bytes. The Buffered. Writer class provides buffering to your Writer's. Buffering can speed up IO quite a bit. Rather than write one character at a time to the network or disk, you write a larger block at a time. This is typically much faster, especially for disk access and larger data amounts. To add buffering to your Writer's simply wrap them in a Buffered. Writer
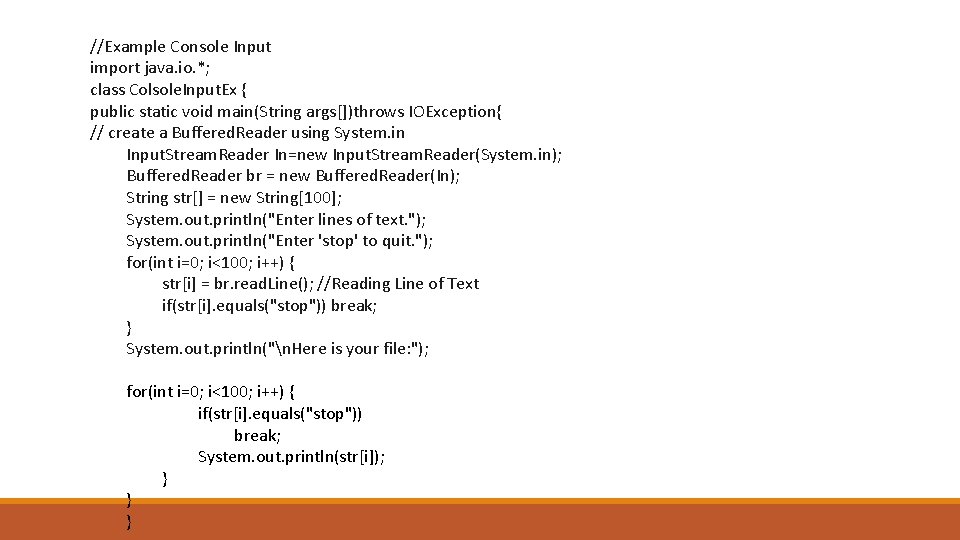
//Example Console Input import java. io. *; class Colsole. Input. Ex { public static void main(String args[])throws IOException{ // create a Buffered. Reader using System. in Input. Stream. Reader In=new Input. Stream. Reader(System. in); Buffered. Reader br = new Buffered. Reader(In); String str[] = new String[100]; System. out. println("Enter lines of text. "); System. out. println("Enter 'stop' to quit. "); for(int i=0; i<100; i++) { str[i] = br. read. Line(); //Reading Line of Text if(str[i]. equals("stop")) break; } System. out. println("n. Here is your file: "); for(int i=0; i<100; i++) { if(str[i]. equals("stop")) break; System. out. println(str[i]); } } }
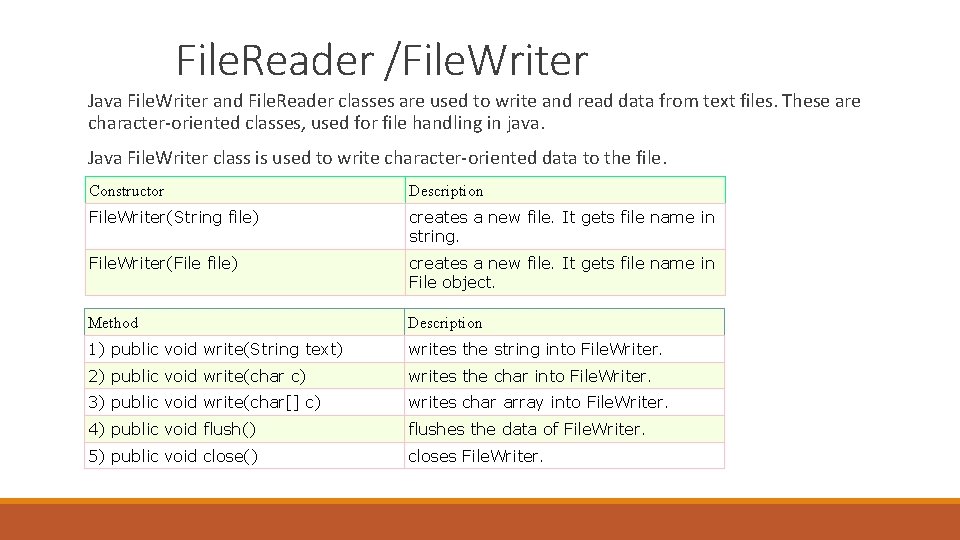
File. Reader /File. Writer Java File. Writer and File. Reader classes are used to write and read data from text files. These are character-oriented classes, used for file handling in java. Java File. Writer class is used to write character-oriented data to the file. Constructor Description File. Writer(String file) creates a new file. It gets file name in string. File. Writer(File file) creates a new file. It gets file name in File object. Method Description 1) public void write(String text) writes the string into File. Writer. 2) public void write(char c) writes the char into File. Writer. 3) public void write(char[] c) writes char array into File. Writer. 4) public void flush() flushes the data of File. Writer. 5) public void close() closes File. Writer.
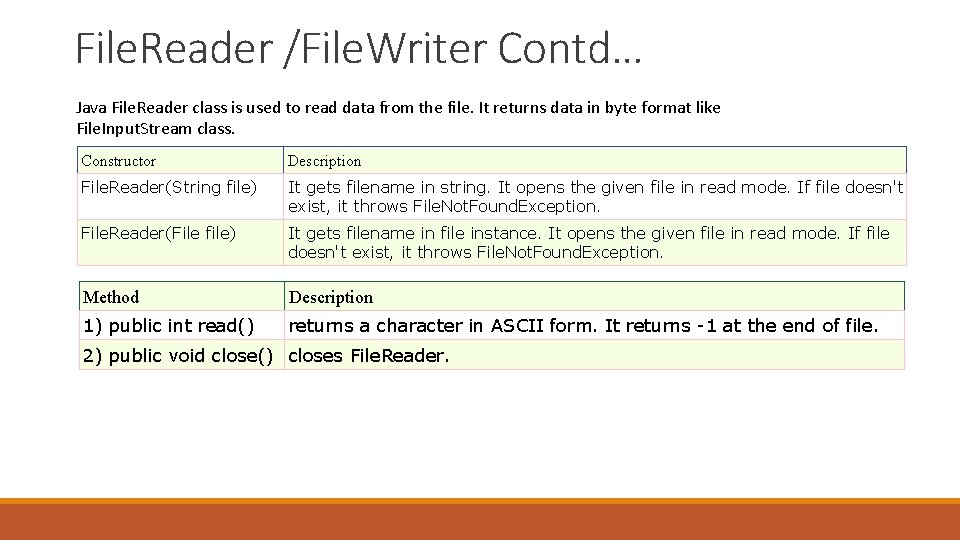
File. Reader /File. Writer Contd… Java File. Reader class is used to read data from the file. It returns data in byte format like File. Input. Stream class. Constructor Description File. Reader(String file) It gets filename in string. It opens the given file in read mode. If file doesn't exist, it throws File. Not. Found. Exception. File. Reader(File file) It gets filename in file instance. It opens the given file in read mode. If file doesn't exist, it throws File. Not. Found. Exception. Method Description 1) public int read() returns a character in ASCII form. It returns -1 at the end of file. 2) public void close() closes File. Reader.
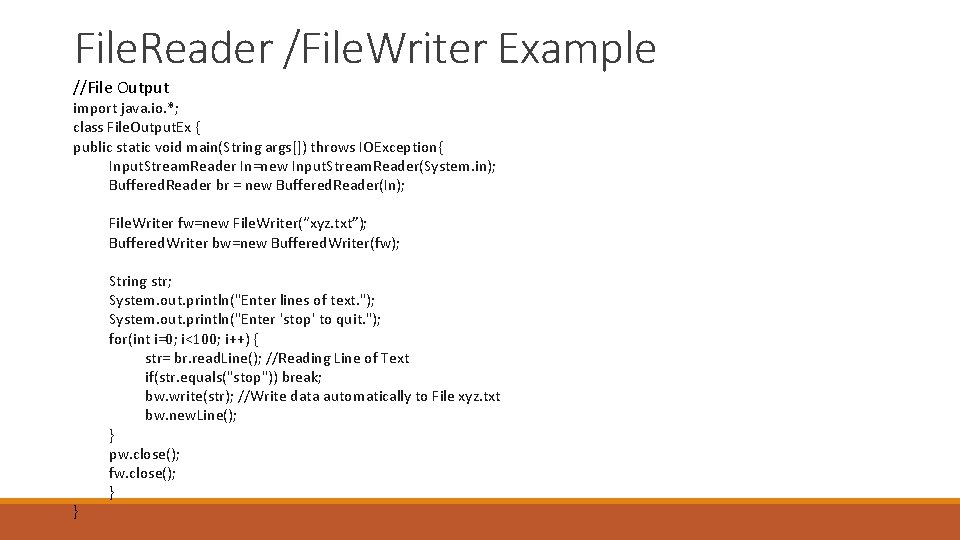
File. Reader /File. Writer Example //File Output import java. io. *; class File. Output. Ex { public static void main(String args[]) throws IOException{ Input. Stream. Reader In=new Input. Stream. Reader(System. in); Buffered. Reader br = new Buffered. Reader(In); } File. Writer fw=new File. Writer(“xyz. txt”); Buffered. Writer bw=new Buffered. Writer(fw); String str; System. out. println("Enter lines of text. "); System. out. println("Enter 'stop' to quit. "); for(int i=0; i<100; i++) { str= br. read. Line(); //Reading Line of Text if(str. equals("stop")) break; bw. write(str); //Write data automatically to File xyz. txt bw. new. Line(); } pw. close(); fw. close(); }
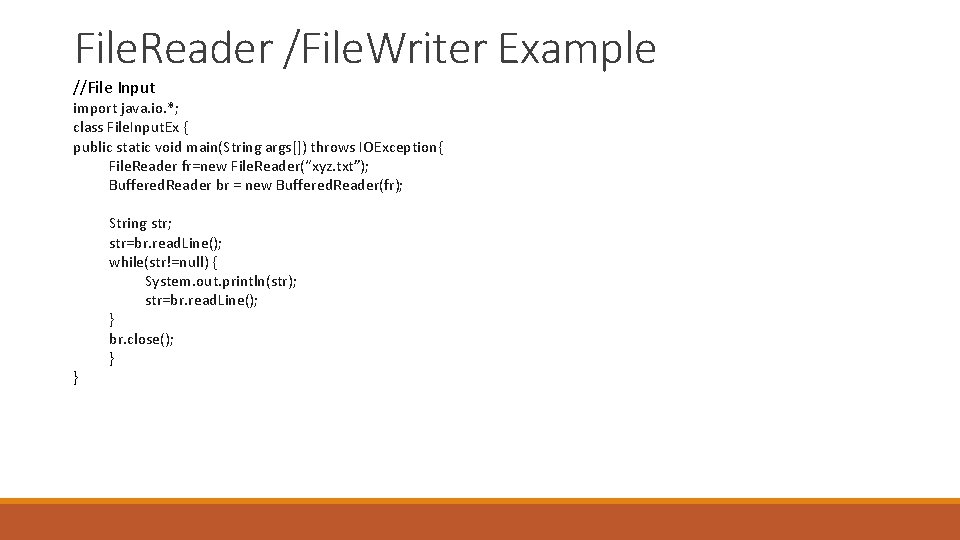
File. Reader /File. Writer Example //File Input import java. io. *; class File. Input. Ex { public static void main(String args[]) throws IOException{ File. Reader fr=new File. Reader(“xyz. txt”); Buffered. Reader br = new Buffered. Reader(fr); String str; str=br. read. Line(); while(str!=null) { System. out. println(str); str=br. read. Line(); } br. close(); } }
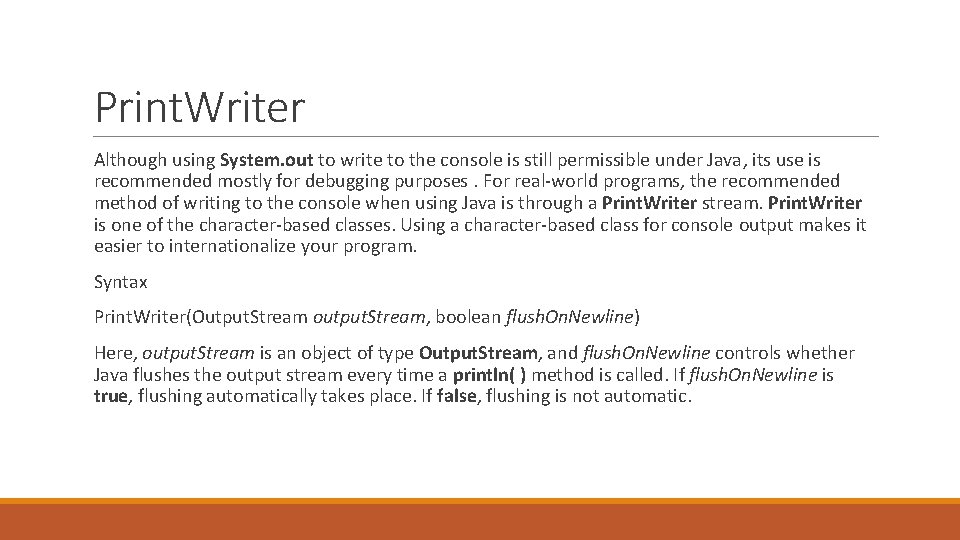
Print. Writer Although using System. out to write to the console is still permissible under Java, its use is recommended mostly for debugging purposes. For real-world programs, the recommended method of writing to the console when using Java is through a Print. Writer stream. Print. Writer is one of the character-based classes. Using a character-based class for console output makes it easier to internationalize your program. Syntax Print. Writer(Output. Stream output. Stream, boolean flush. On. Newline) Here, output. Stream is an object of type Output. Stream, and flush. On. Newline controls whether Java flushes the output stream every time a println( ) method is called. If flush. On. Newline is true, flushing automatically takes place. If false, flushing is not automatic.
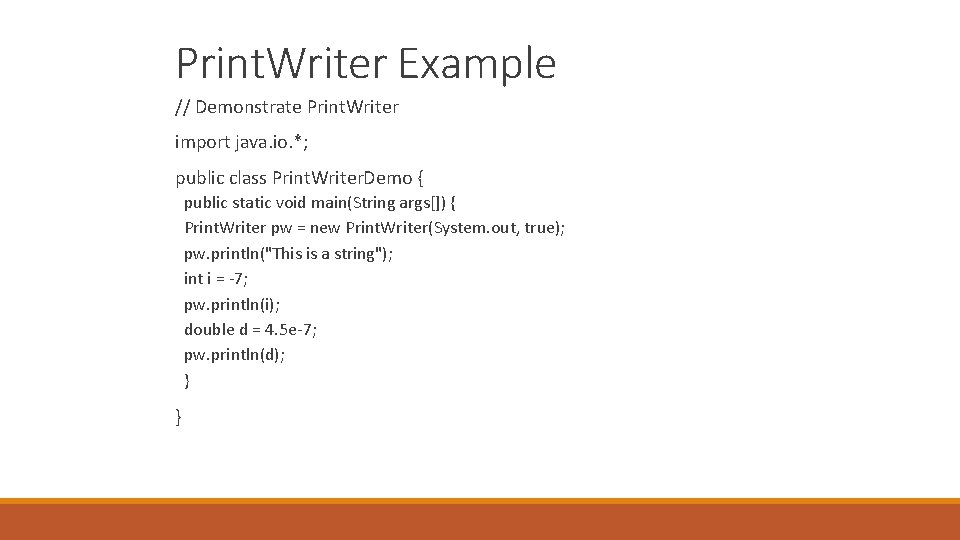
Print. Writer Example // Demonstrate Print. Writer import java. io. *; public class Print. Writer. Demo { public static void main(String args[]) { Print. Writer pw = new Print. Writer(System. out, true); pw. println("This is a string"); int i = -7; pw. println(i); double d = 4. 5 e-7; pw. println(d); } }
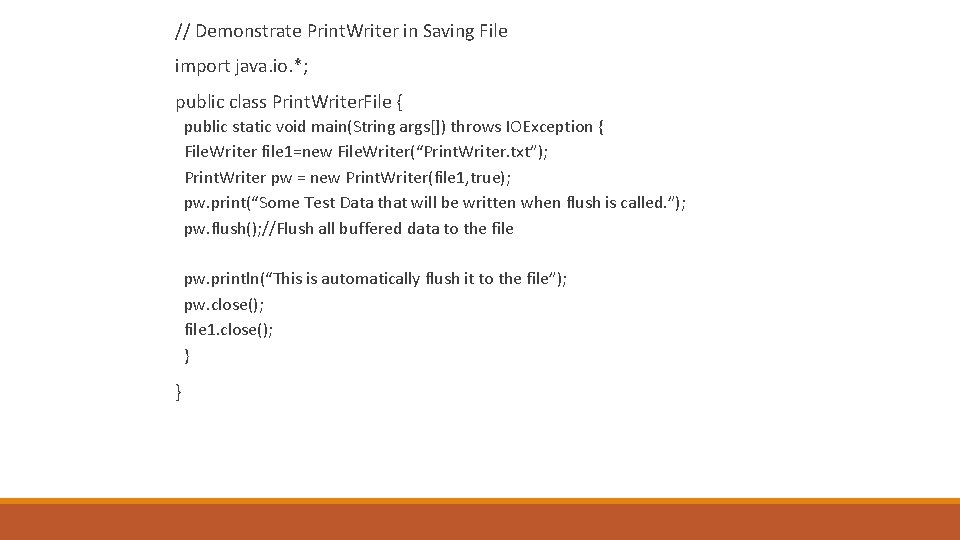
// Demonstrate Print. Writer in Saving File import java. io. *; public class Print. Writer. File { public static void main(String args[]) throws IOException { File. Writer file 1=new File. Writer(“Print. Writer. txt”); Print. Writer pw = new Print. Writer(file 1, true); pw. print(“Some Test Data that will be written when flush is called. ”); pw. flush(); //Flush all buffered data to the file pw. println(“This is automatically flush it to the file”); pw. close(); file 1. close(); } }
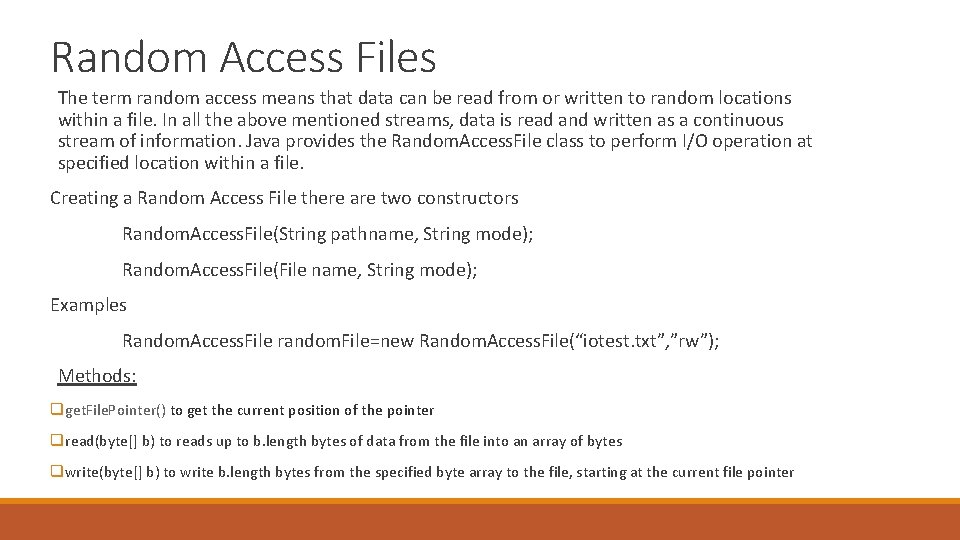
Random Access Files The term random access means that data can be read from or written to random locations within a file. In all the above mentioned streams, data is read and written as a continuous stream of information. Java provides the Random. Access. File class to perform I/O operation at specified location within a file. Creating a Random Access File there are two constructors Random. Access. File(String pathname, String mode); Random. Access. File(File name, String mode); Examples Random. Access. File random. File=new Random. Access. File(“iotest. txt”, ”rw”); Methods: qget. File. Pointer() to get the current position of the pointer qread(byte[] b) to reads up to b. length bytes of data from the file into an array of bytes qwrite(byte[] b) to write b. length bytes from the specified byte array to the file, starting at the current file pointer
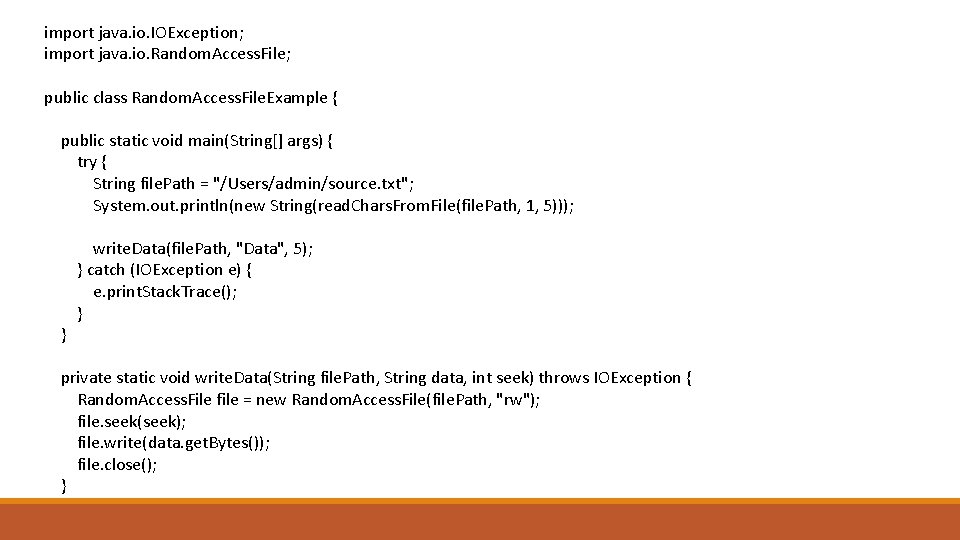
import java. io. IOException; import java. io. Random. Access. File; public class Random. Access. File. Example { public static void main(String[] args) { try { String file. Path = "/Users/admin/source. txt"; System. out. println(new String(read. Chars. From. File(file. Path, 1, 5))); write. Data(file. Path, "Data", 5); } catch (IOException e) { e. print. Stack. Trace(); } } private static void write. Data(String file. Path, String data, int seek) throws IOException { Random. Access. File file = new Random. Access. File(file. Path, "rw"); file. seek(seek); file. write(data. get. Bytes()); file. close(); }
![private static byte read Chars From FileString file Path int seek int chars private static byte[] read. Chars. From. File(String file. Path, int seek, int chars)](https://slidetodoc.com/presentation_image/aafe947d41f355bc9ad317a59ecc91f6/image-16.jpg)
private static byte[] read. Chars. From. File(String file. Path, int seek, int chars) throws IOException { Random. Access. File file = new Random. Access. File(file. Path, "r"); file. seek(seek); byte[] bytes = new byte[chars]; file. read(bytes); file. close(); return bytes; } }
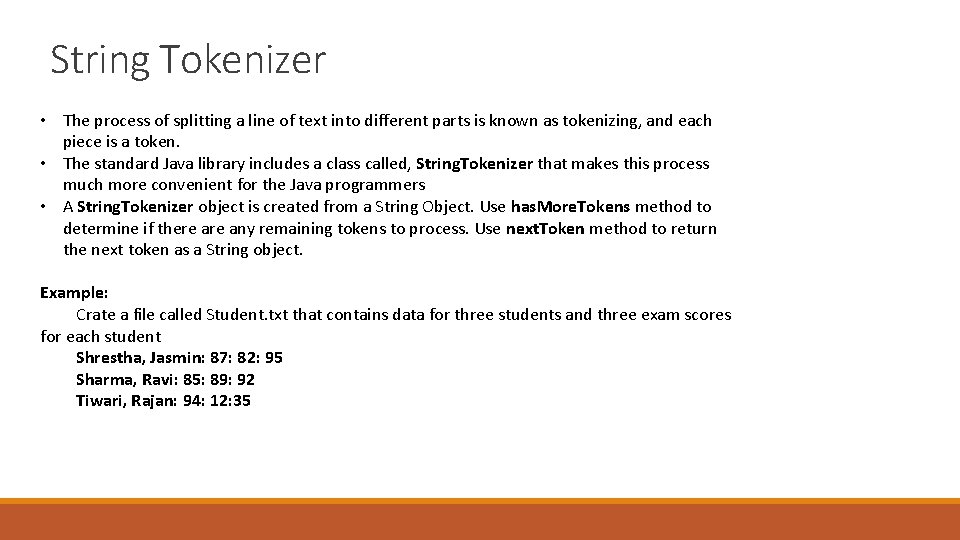
String Tokenizer • The process of splitting a line of text into different parts is known as tokenizing, and each piece is a token. • The standard Java library includes a class called, String. Tokenizer that makes this process much more convenient for the Java programmers • A String. Tokenizer object is created from a String Object. Use has. More. Tokens method to determine if there any remaining tokens to process. Use next. Token method to return the next token as a String object. Example: Crate a file called Student. txt that contains data for three students and three exam scores for each student Shrestha, Jasmin: 87: 82: 95 Sharma, Ravi: 85: 89: 92 Tiwari, Rajan: 94: 12: 35
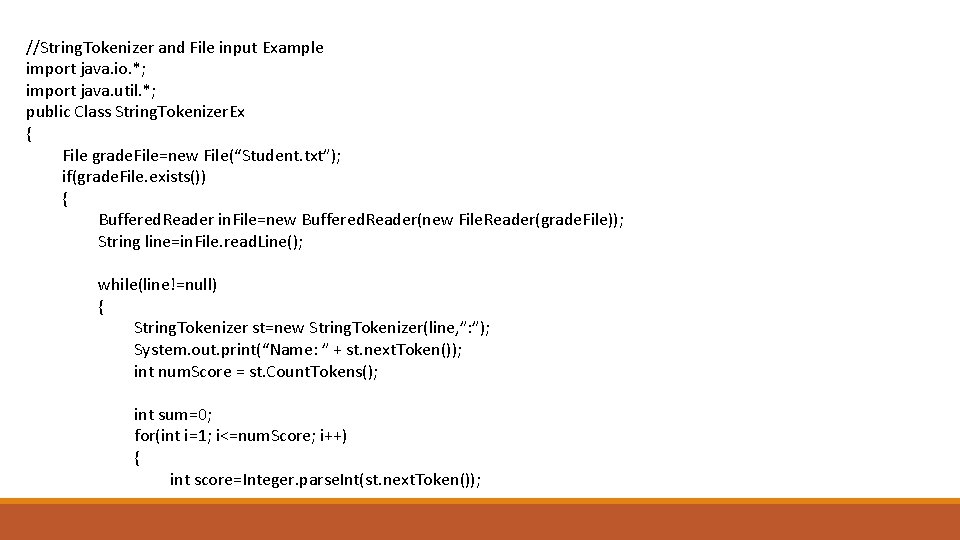
//String. Tokenizer and File input Example import java. io. *; import java. util. *; public Class String. Tokenizer. Ex { File grade. File=new File(“Student. txt”); if(grade. File. exists()) { Buffered. Reader in. File=new Buffered. Reader(new File. Reader(grade. File)); String line=in. File. read. Line(); while(line!=null) { String. Tokenizer st=new String. Tokenizer(line, ”: ”); System. out. print(“Name: ” + st. next. Token()); int num. Score = st. Count. Tokens(); int sum=0; for(int i=1; i<=num. Score; i++) { int score=Integer. parse. Int(st. next. Token());
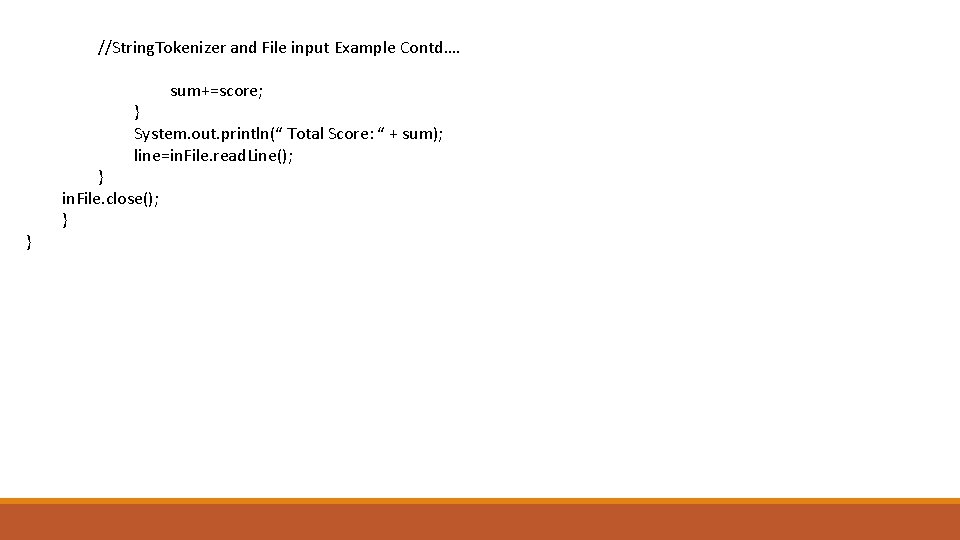
//String. Tokenizer and File input Example Contd…. sum+=score; } System. out. println(“ Total Score: “ + sum); line=in. File. read. Line(); } } in. File. close(); }