Java GUI Structure of a GUI App A
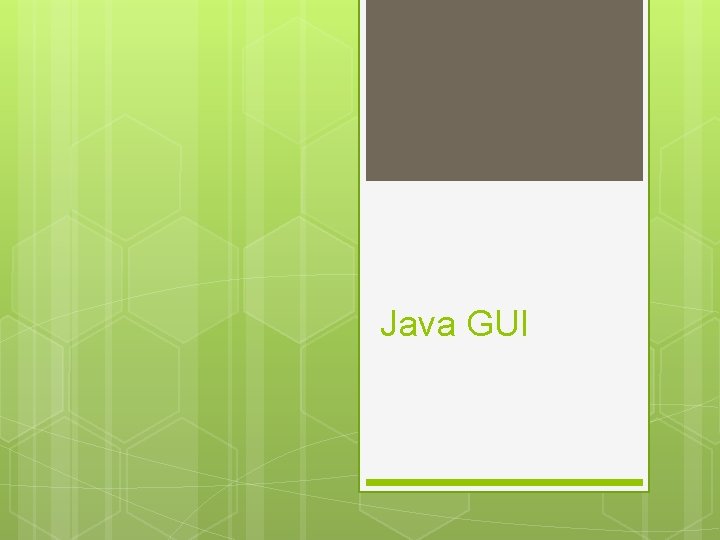
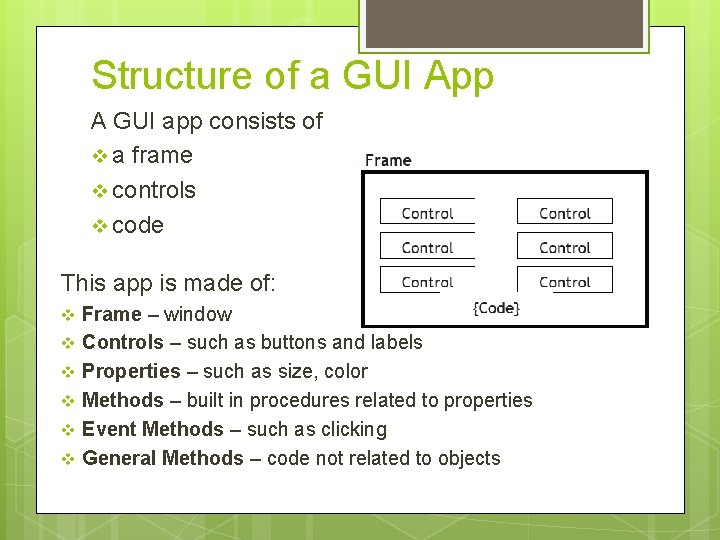
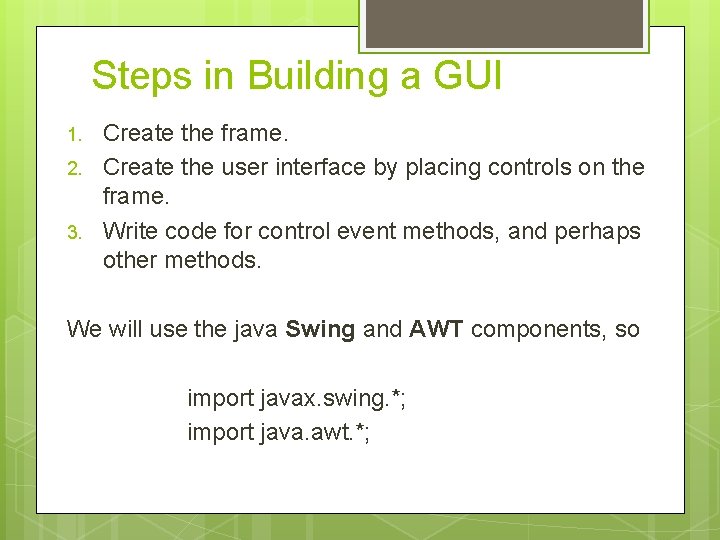
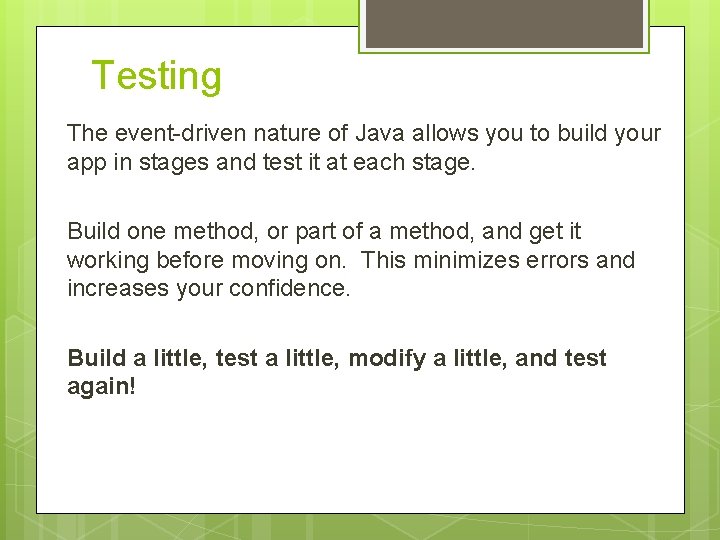
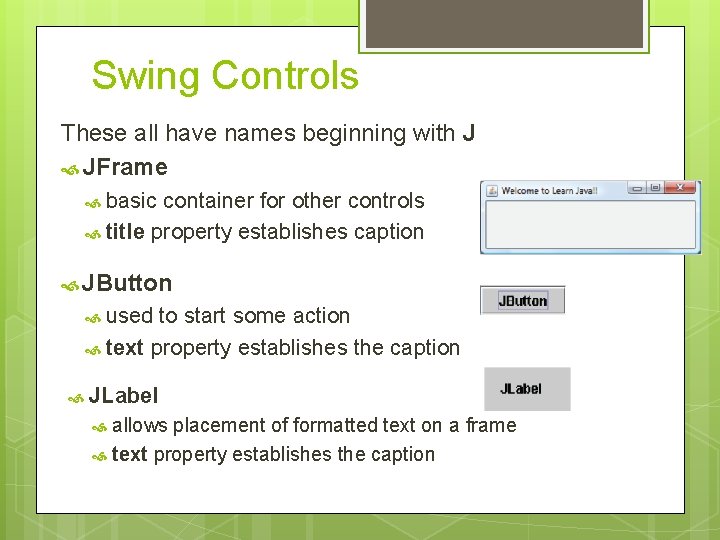
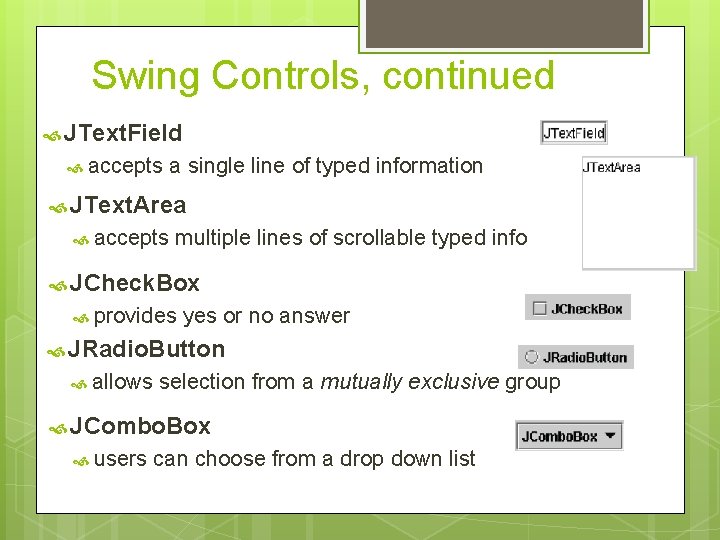
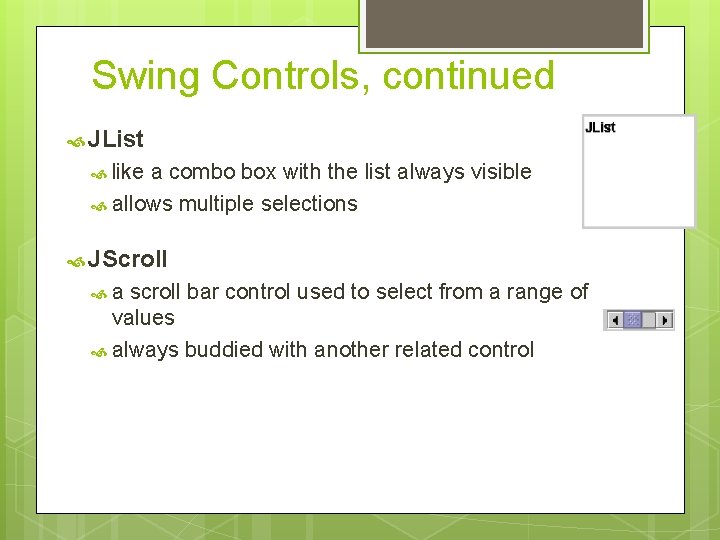
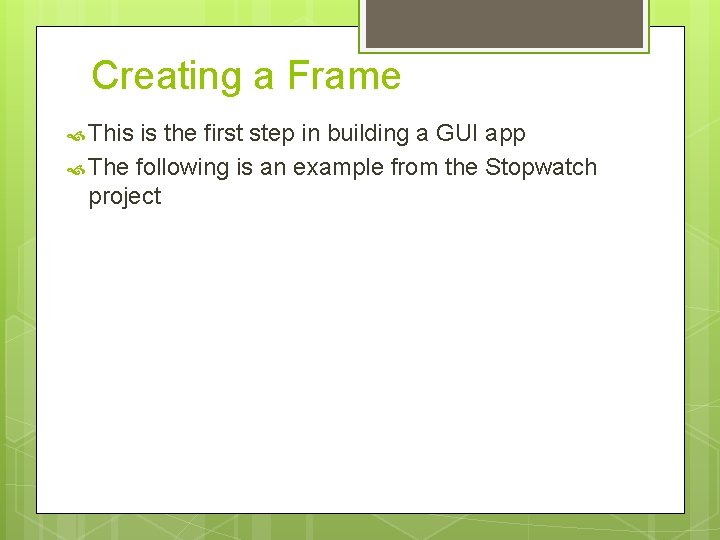
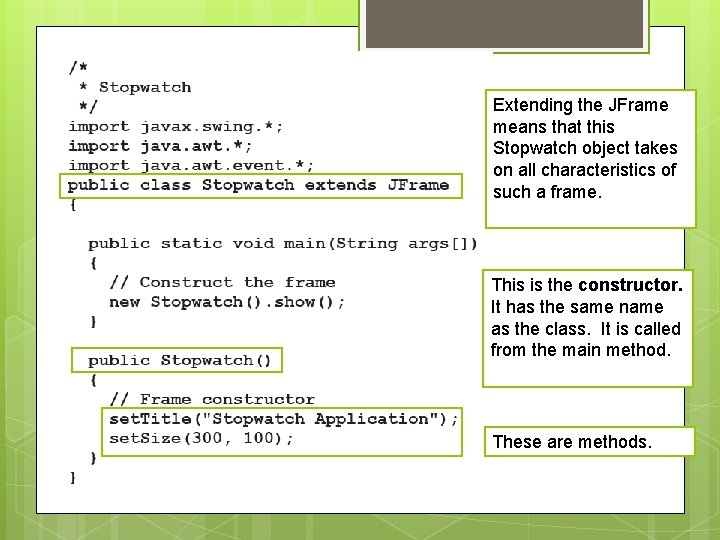
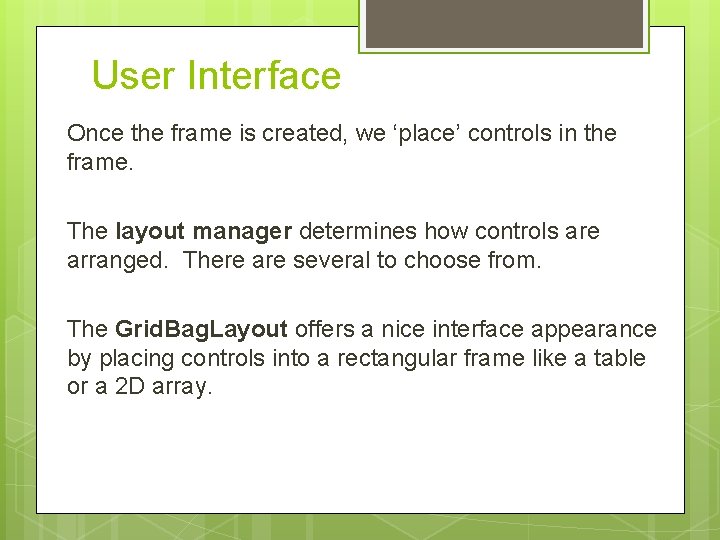
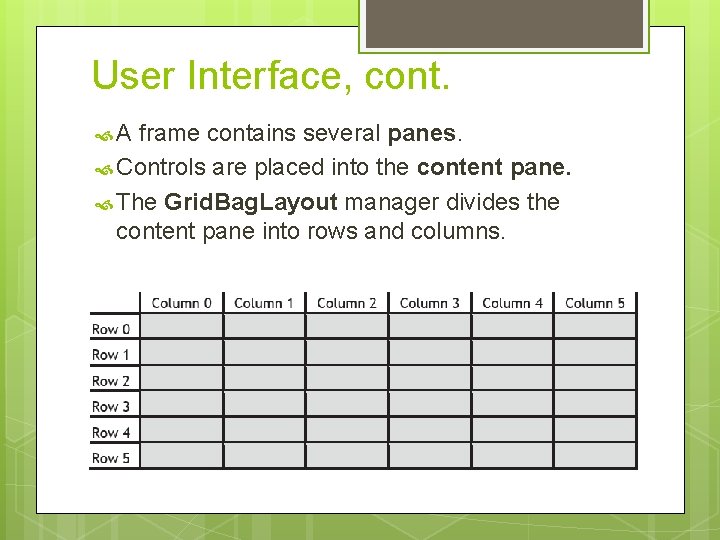
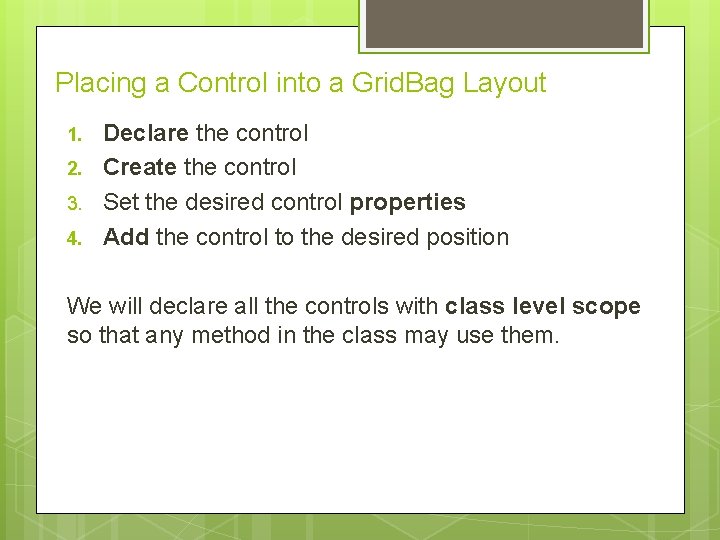
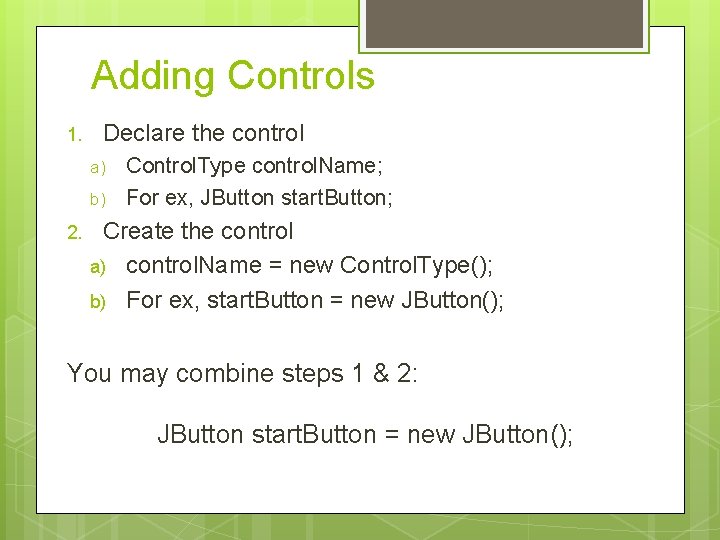
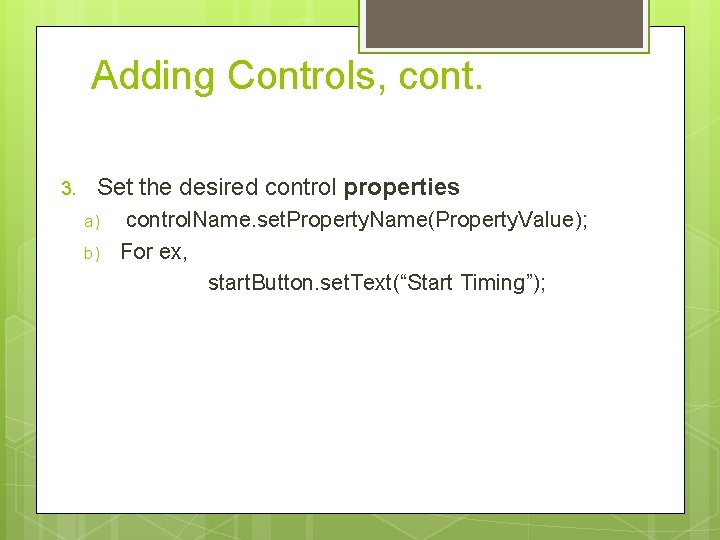
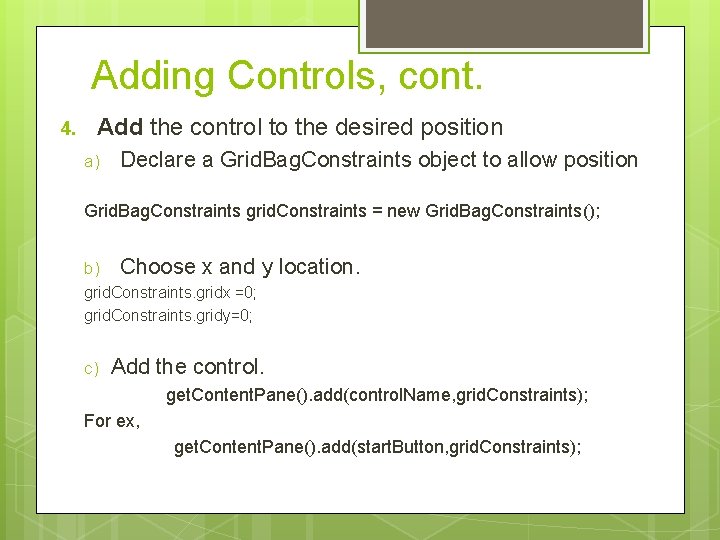
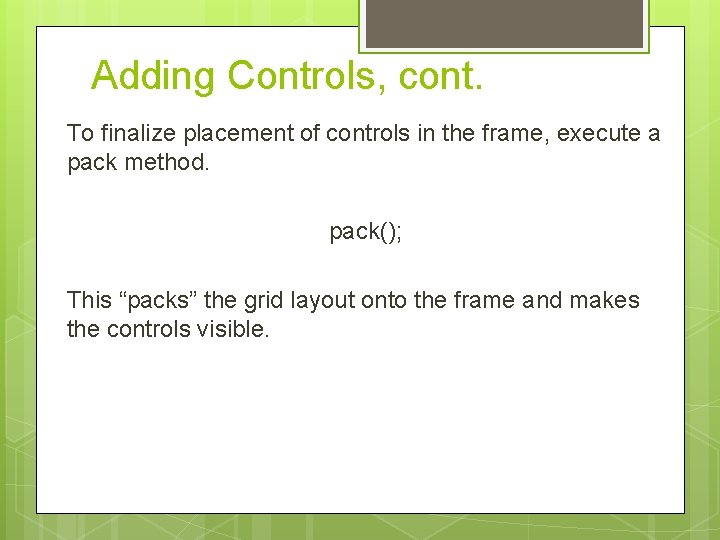
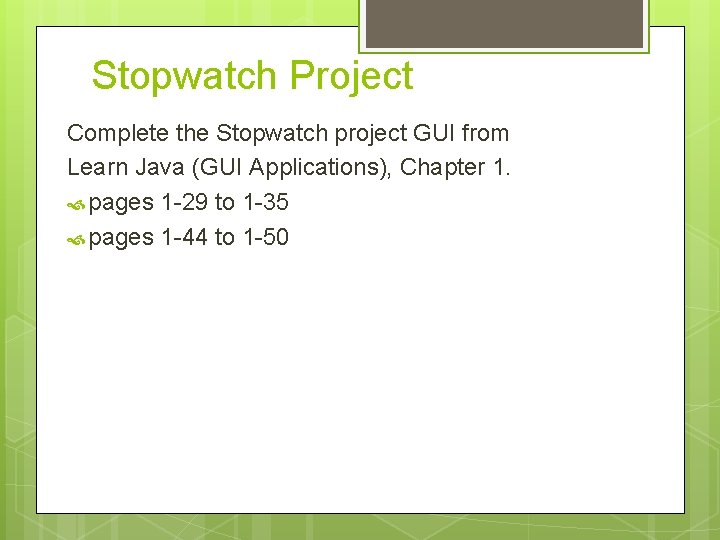
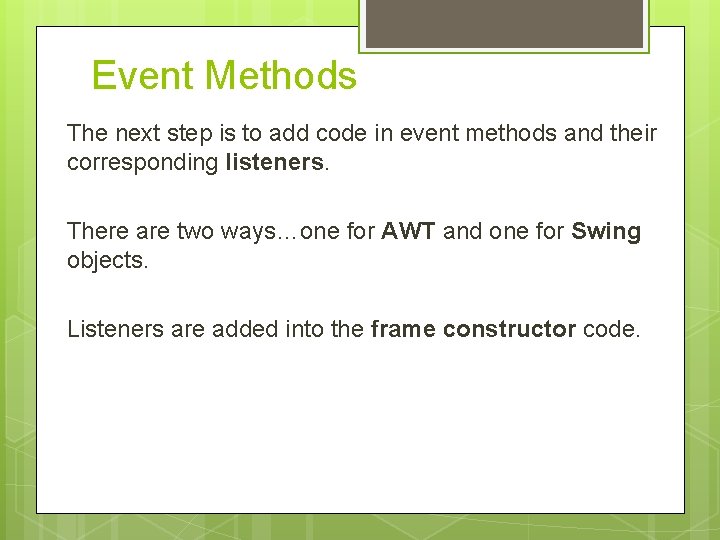
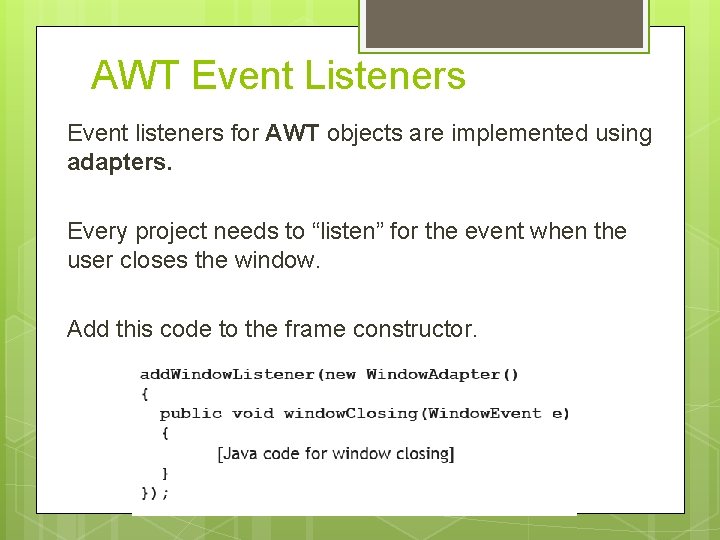
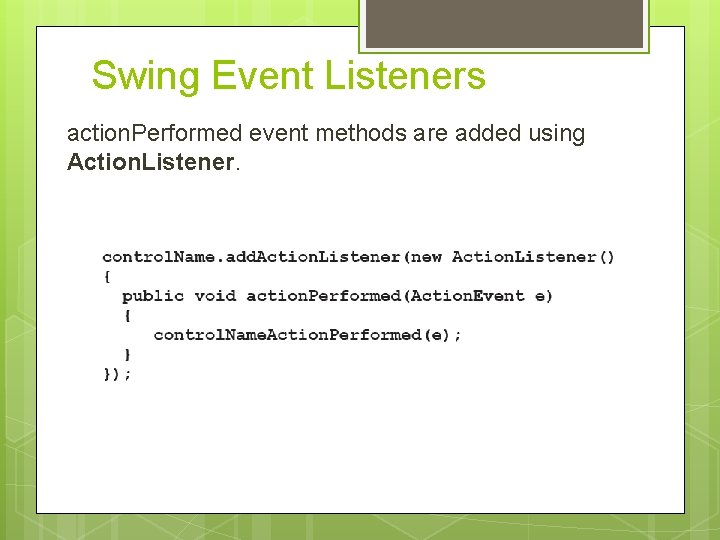
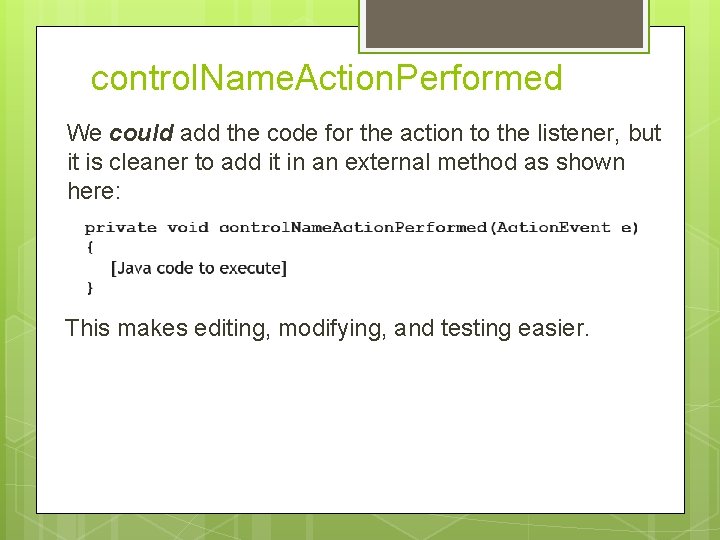
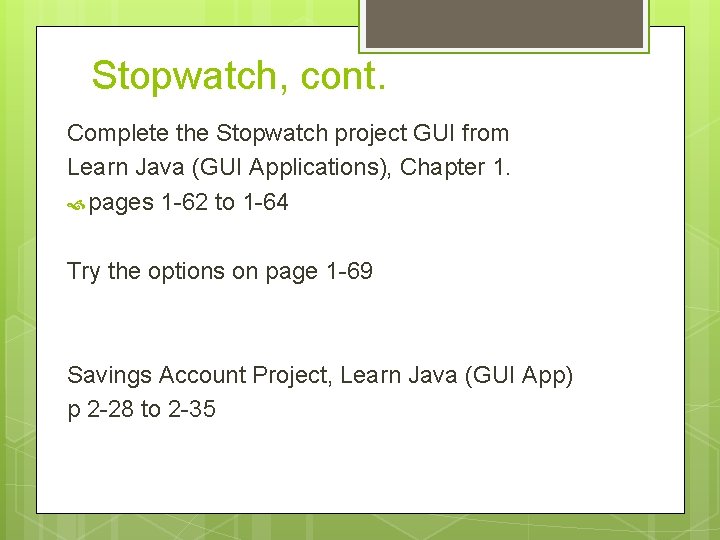
- Slides: 22
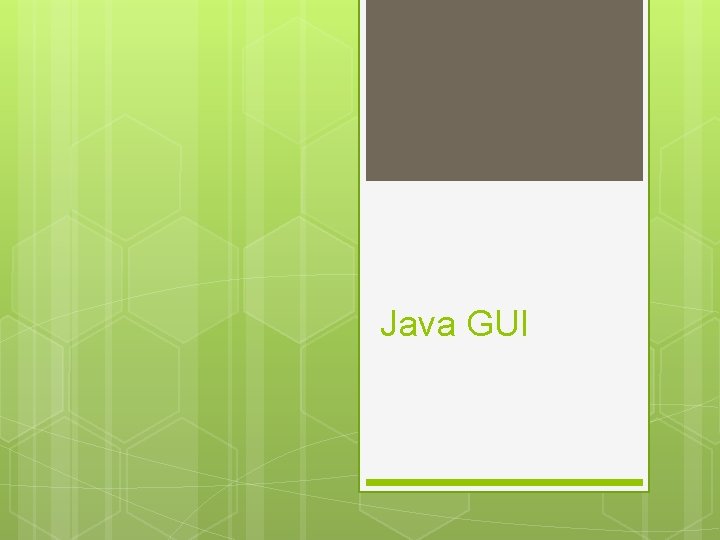
Java GUI
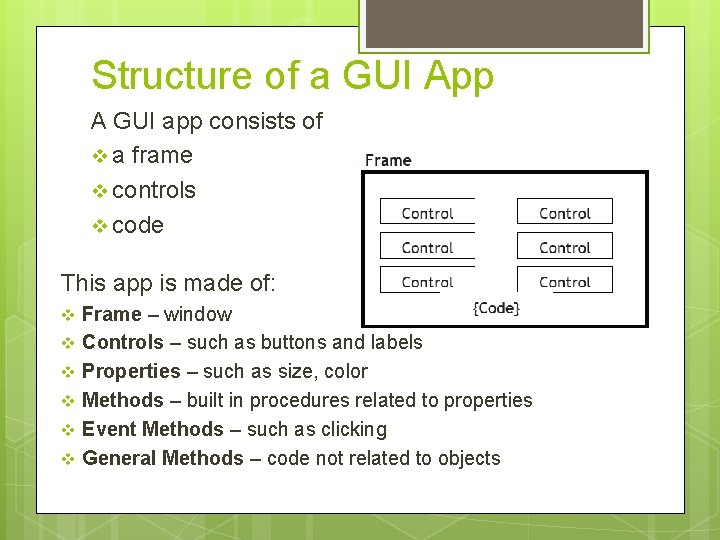
Structure of a GUI App A GUI app consists of v a frame v controls v code This app is made of: v v v Frame – window Controls – such as buttons and labels Properties – such as size, color Methods – built in procedures related to properties Event Methods – such as clicking General Methods – code not related to objects
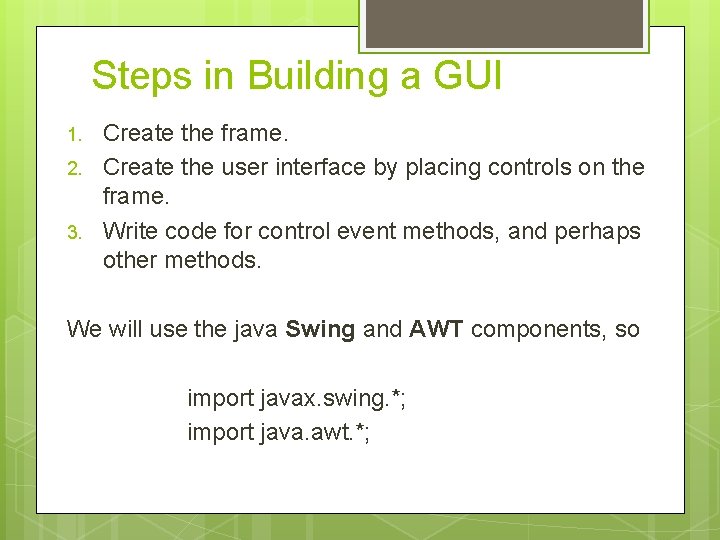
Steps in Building a GUI 1. 2. 3. Create the frame. Create the user interface by placing controls on the frame. Write code for control event methods, and perhaps other methods. We will use the java Swing and AWT components, so import javax. swing. *; import java. awt. *;
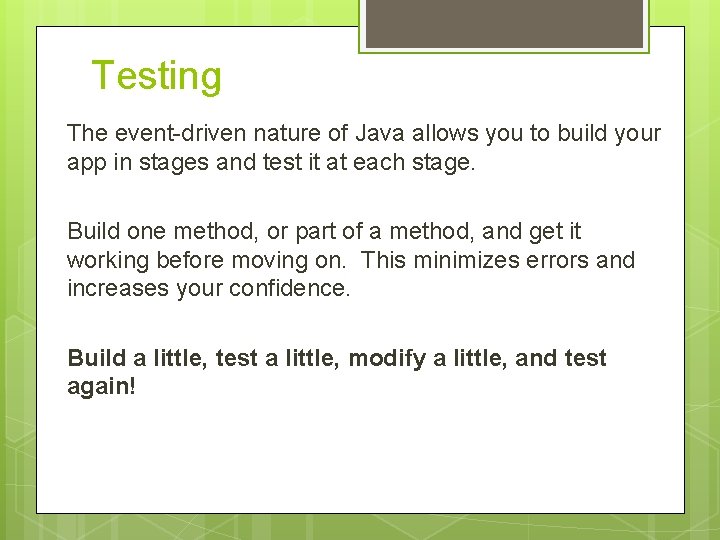
Testing The event-driven nature of Java allows you to build your app in stages and test it at each stage. Build one method, or part of a method, and get it working before moving on. This minimizes errors and increases your confidence. Build a little, test a little, modify a little, and test again!
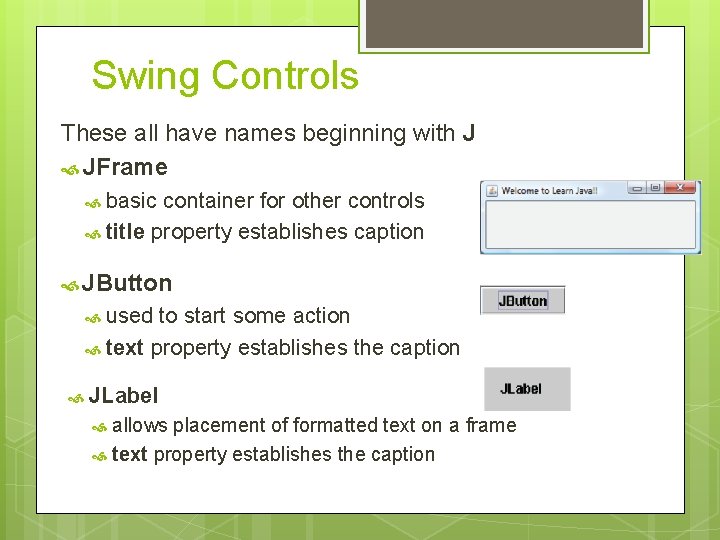
Swing Controls These all have names beginning with J JFrame basic container for other controls title property establishes caption JButton used to start some action text property establishes the caption JLabel allows placement of formatted text on a frame text property establishes the caption
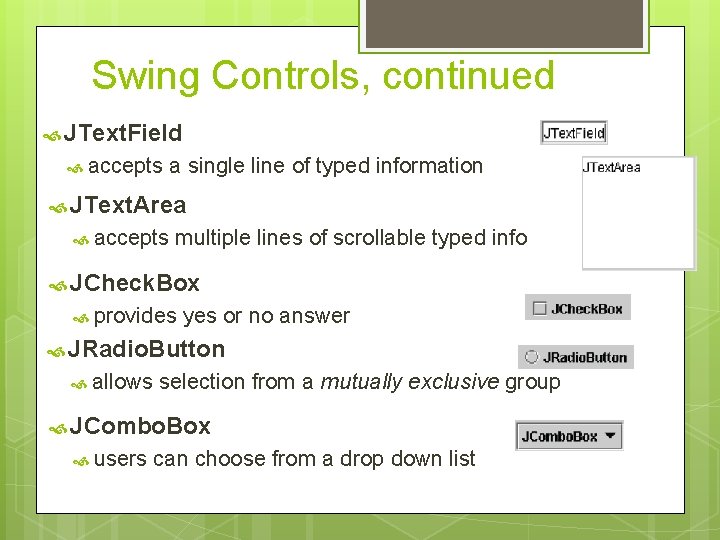
Swing Controls, continued JText. Field accepts a single line of typed information JText. Area accepts multiple lines of scrollable typed info JCheck. Box provides yes or no answer JRadio. Button allows selection from a mutually exclusive group JCombo. Box users can choose from a drop down list
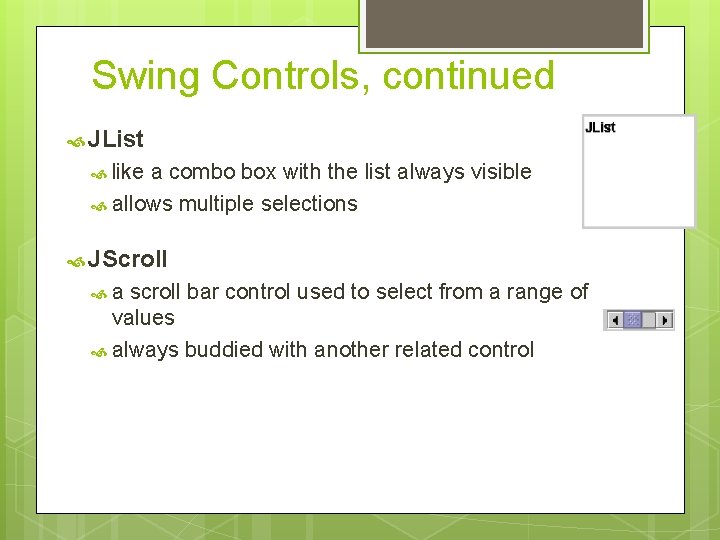
Swing Controls, continued JList like a combo box with the list always visible allows multiple selections JScroll a scroll bar control used to select from a range of values always buddied with another related control
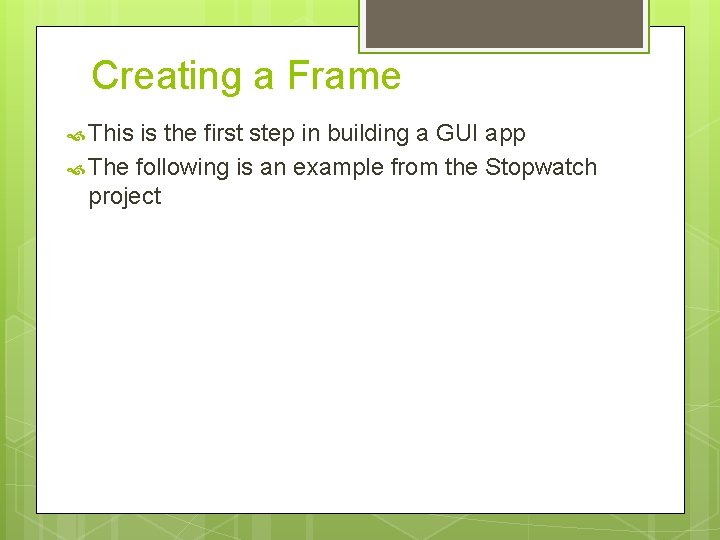
Creating a Frame This is the first step in building a GUI app The following is an example from the Stopwatch project
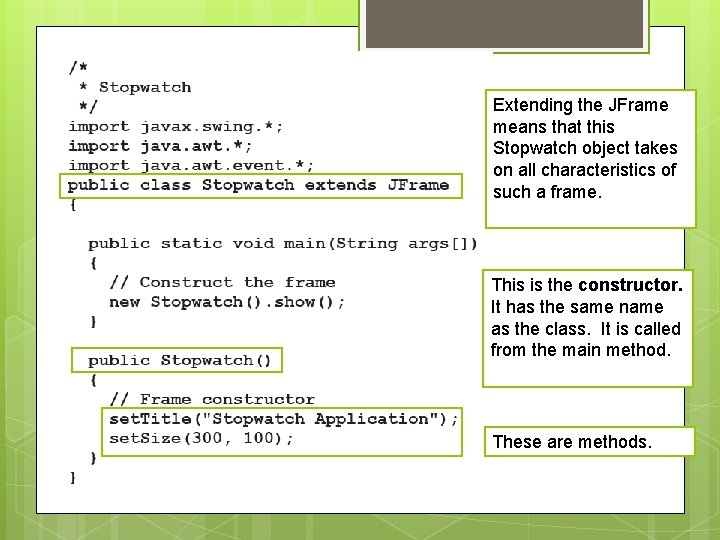
Extending the JFrame means that this Stopwatch object takes on all characteristics of such a frame. This is the constructor. It has the same name as the class. It is called from the main method. These are methods.
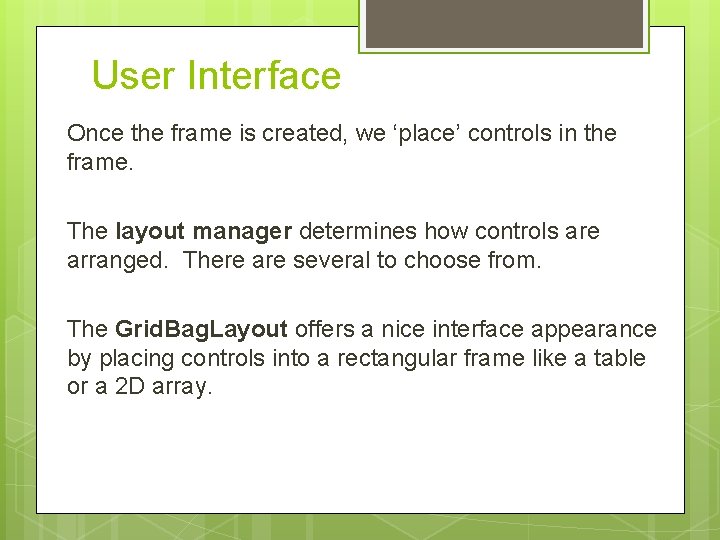
User Interface Once the frame is created, we ‘place’ controls in the frame. The layout manager determines how controls are arranged. There are several to choose from. The Grid. Bag. Layout offers a nice interface appearance by placing controls into a rectangular frame like a table or a 2 D array.
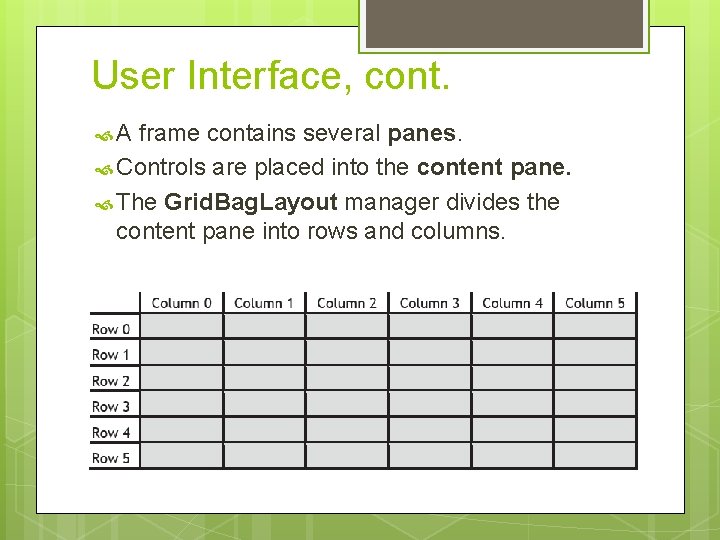
User Interface, cont. A frame contains several panes. Controls are placed into the content pane. The Grid. Bag. Layout manager divides the content pane into rows and columns.
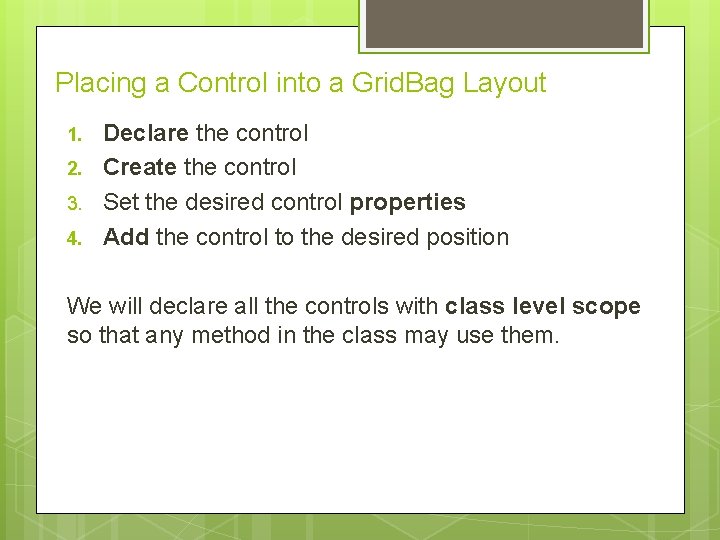
Placing a Control into a Grid. Bag Layout 1. 2. 3. 4. Declare the control Create the control Set the desired control properties Add the control to the desired position We will declare all the controls with class level scope so that any method in the class may use them.
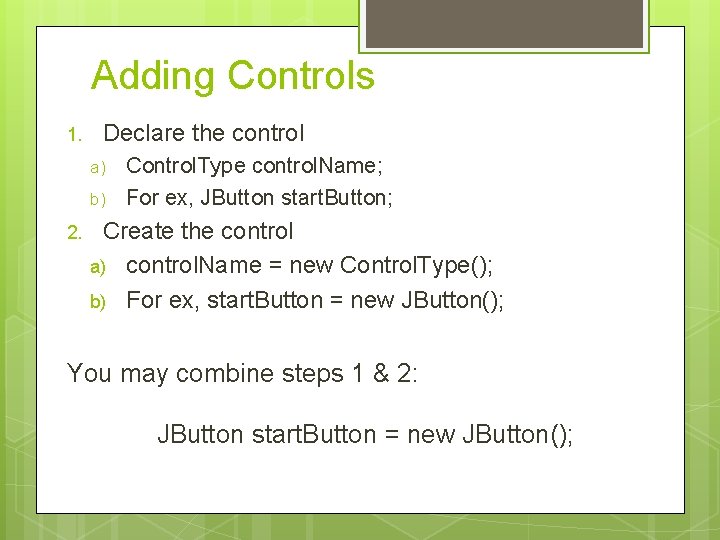
Adding Controls 1. Declare the control a) b) 2. Control. Type control. Name; For ex, JButton start. Button; Create the control a) control. Name = new Control. Type(); b) For ex, start. Button = new JButton(); You may combine steps 1 & 2: JButton start. Button = new JButton();
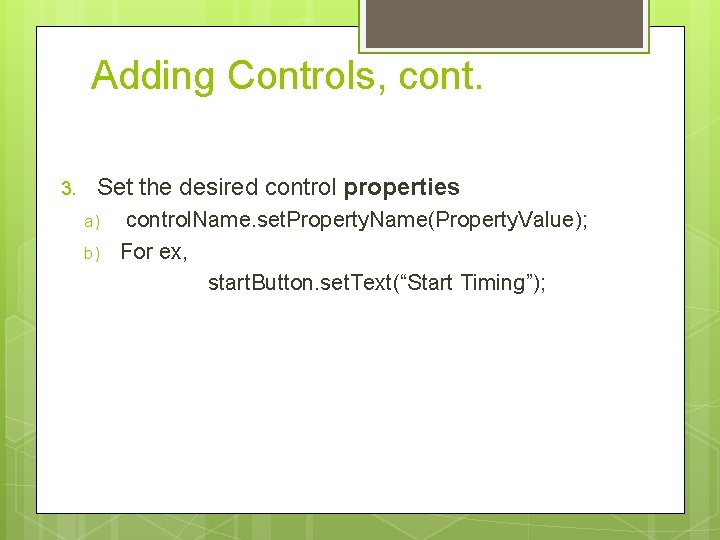
Adding Controls, cont. 3. Set the desired control properties a) b) control. Name. set. Property. Name(Property. Value); For ex, start. Button. set. Text(“Start Timing”);
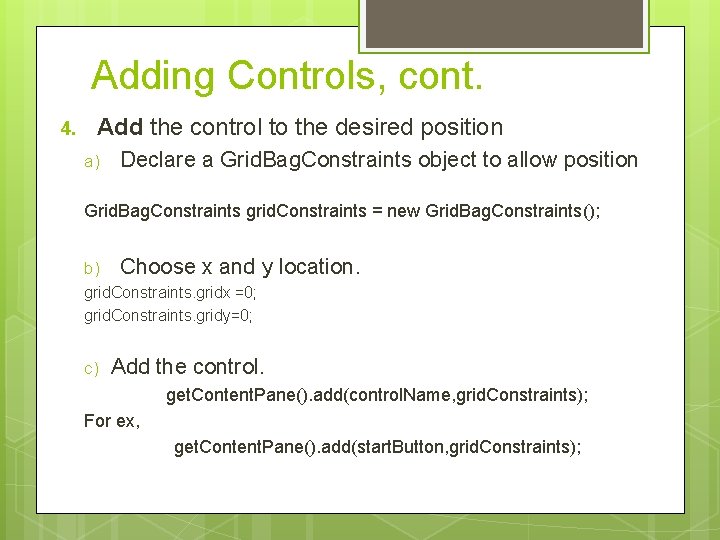
Adding Controls, cont. 4. Add the control to the desired position a) Declare a Grid. Bag. Constraints object to allow position Grid. Bag. Constraints grid. Constraints = new Grid. Bag. Constraints(); b) Choose x and y location. grid. Constraints. gridx =0; grid. Constraints. gridy=0; c) Add the control. get. Content. Pane(). add(control. Name, grid. Constraints); For ex, get. Content. Pane(). add(start. Button, grid. Constraints);
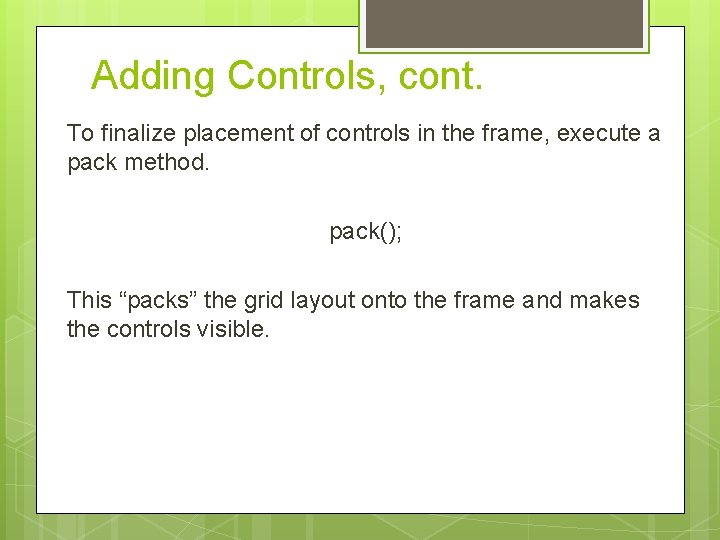
Adding Controls, cont. To finalize placement of controls in the frame, execute a pack method. pack(); This “packs” the grid layout onto the frame and makes the controls visible.
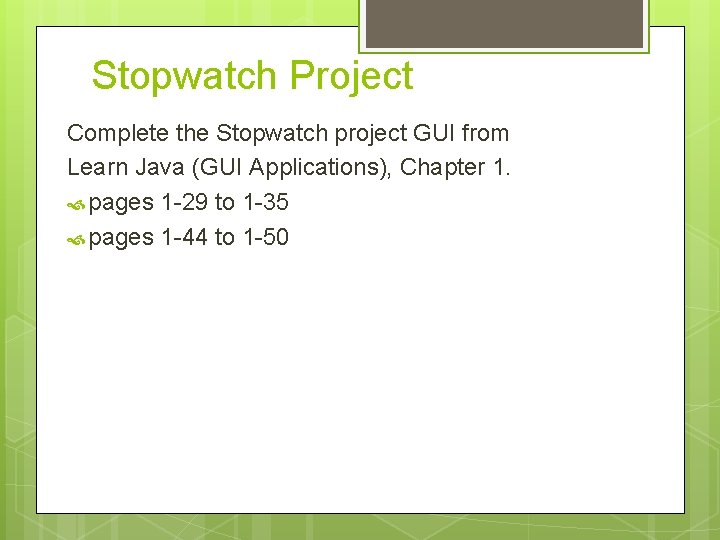
Stopwatch Project Complete the Stopwatch project GUI from Learn Java (GUI Applications), Chapter 1. pages 1 -29 to 1 -35 pages 1 -44 to 1 -50
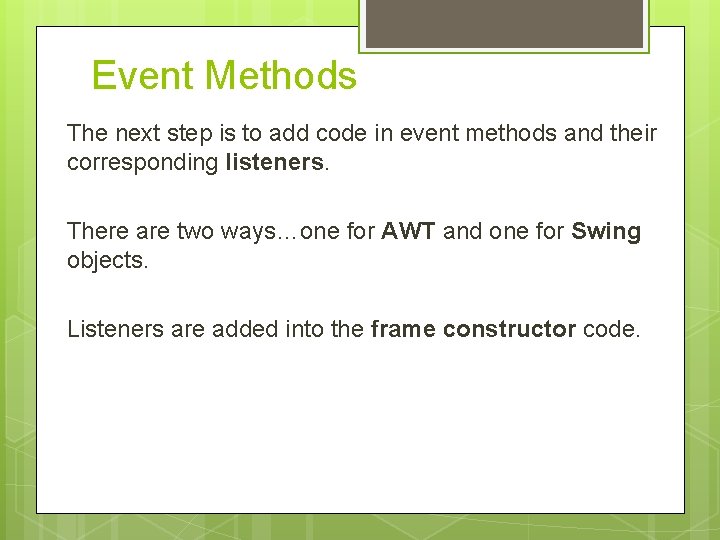
Event Methods The next step is to add code in event methods and their corresponding listeners. There are two ways…one for AWT and one for Swing objects. Listeners are added into the frame constructor code.
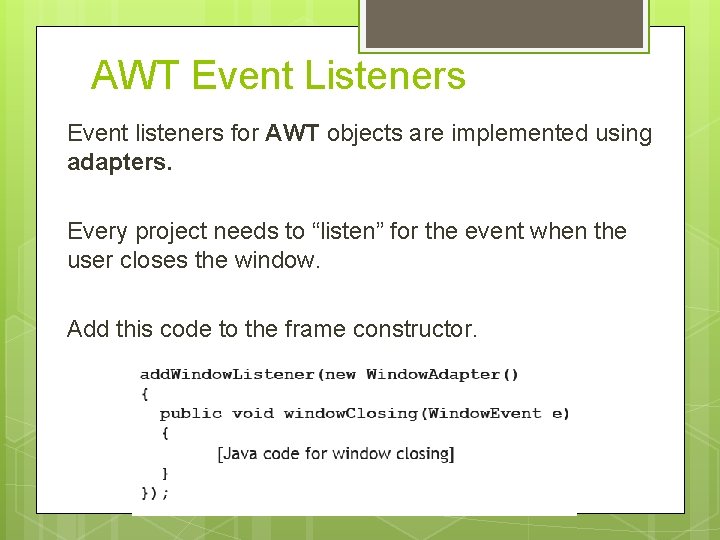
AWT Event Listeners Event listeners for AWT objects are implemented using adapters. Every project needs to “listen” for the event when the user closes the window. Add this code to the frame constructor.
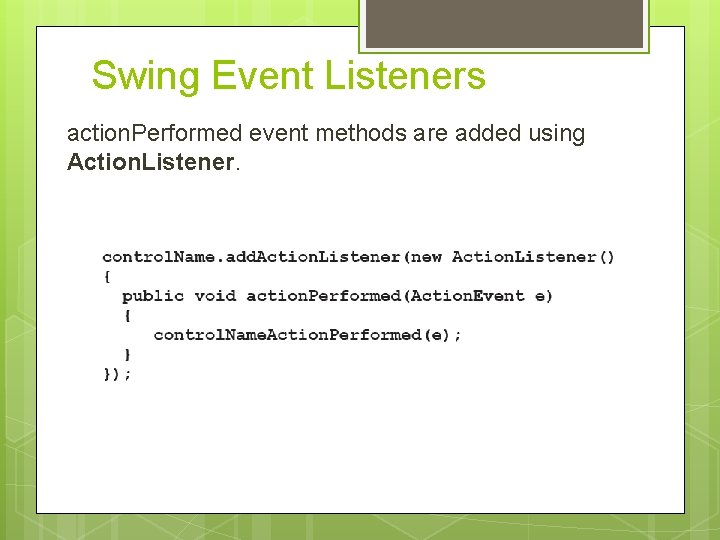
Swing Event Listeners action. Performed event methods are added using Action. Listener.
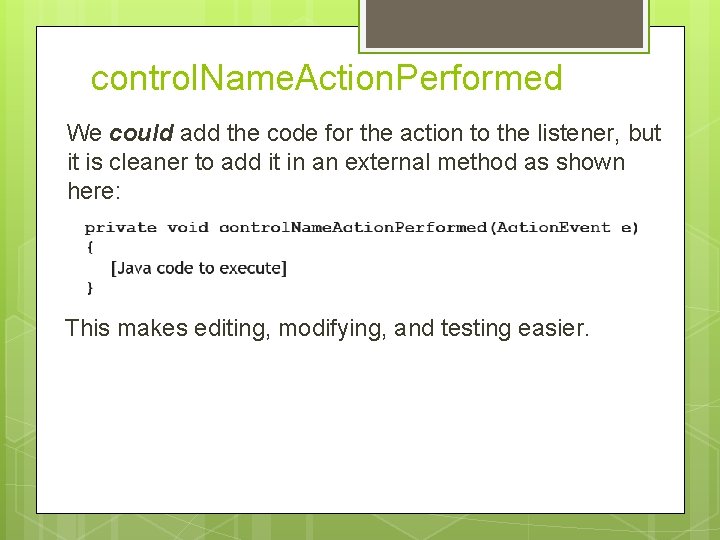
control. Name. Action. Performed We could add the code for the action to the listener, but it is cleaner to add it in an external method as shown here: This makes editing, modifying, and testing easier.
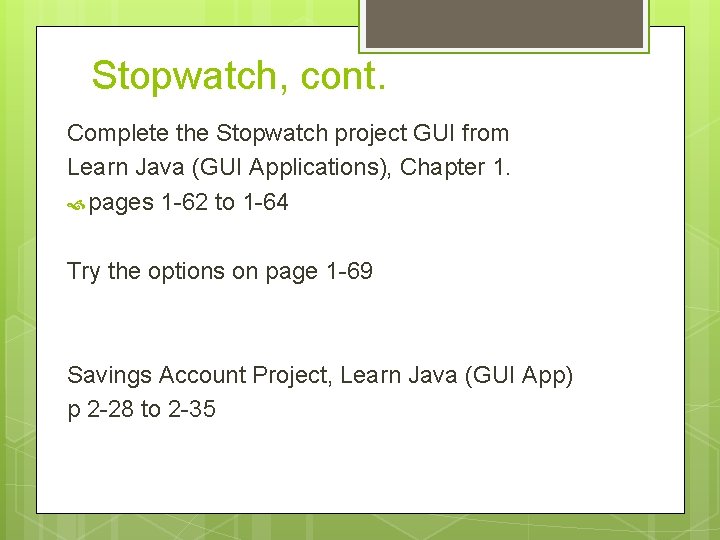
Stopwatch, cont. Complete the Stopwatch project GUI from Learn Java (GUI Applications), Chapter 1. pages 1 -62 to 1 -64 Try the options on page 1 -69 Savings Account Project, Learn Java (GUI App) p 2 -28 to 2 -35
Ge gi gue gui güe güi
Chrome river tutorial
Intune mdm
Gui programmierung java
Java gui awt
Java gui basics
Common gui event types and listener interfaces in java
Gui programmierung java
Swing containers
Java gui thread
Gui event handling
Gui event handling
Java gui toolkit
Java gui for r
Java gui
Java gui design
Modern java gui
Jframe animation
History of the gui
Java gui fx
Java gui basics
Java gui programmierung
자바 스윙