Java Graphics Learn Java Applet and User Interface
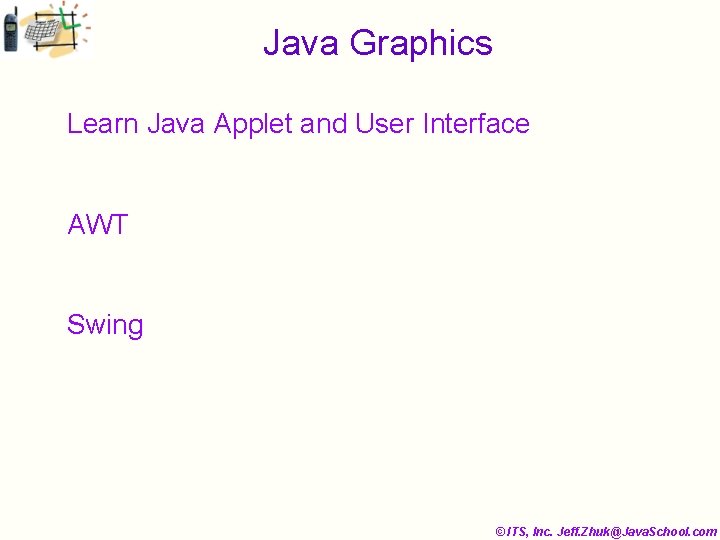
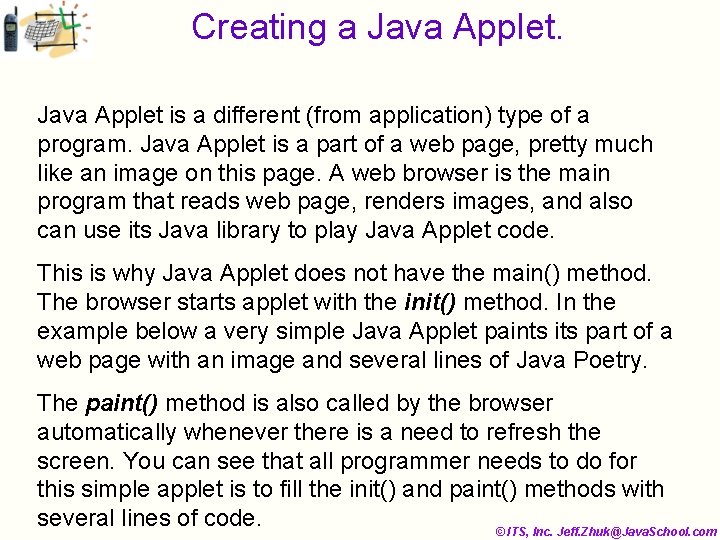
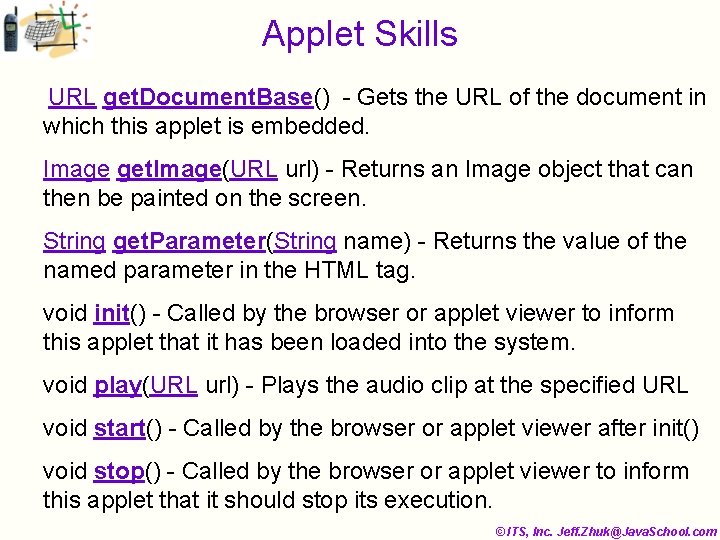
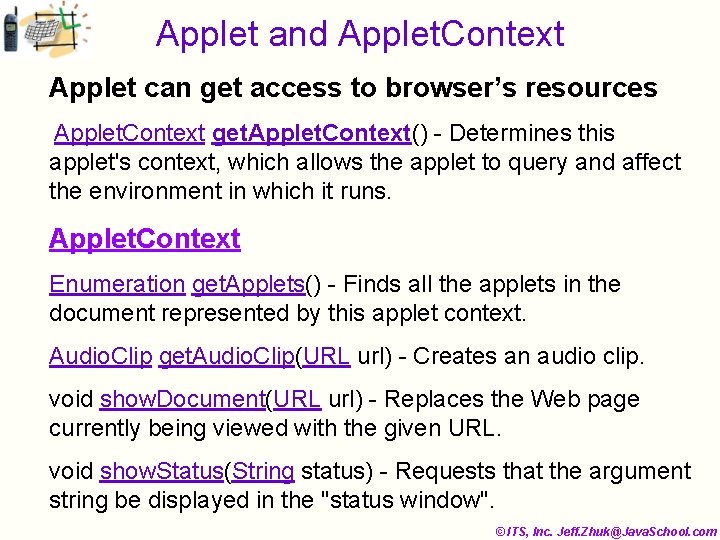
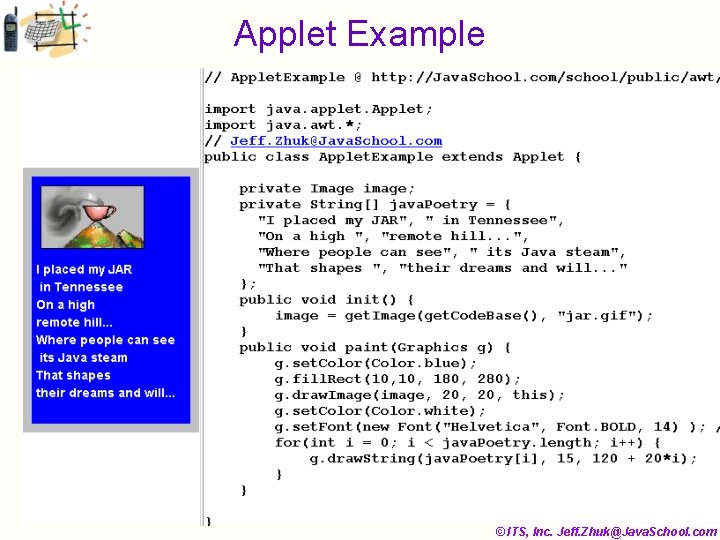
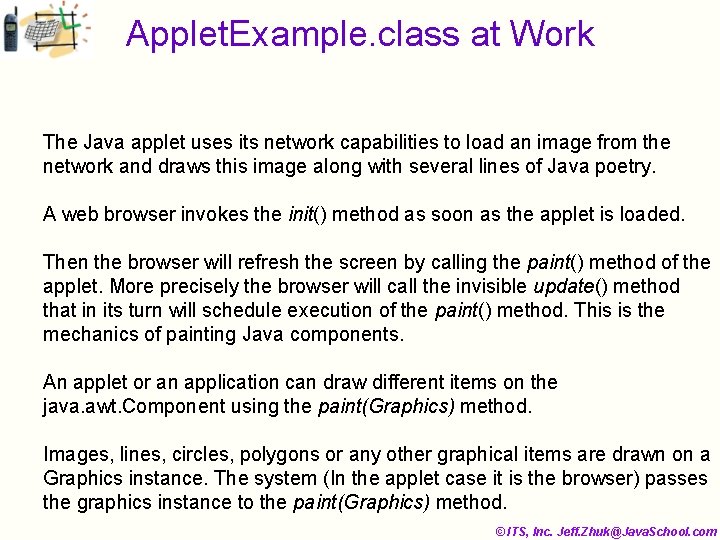
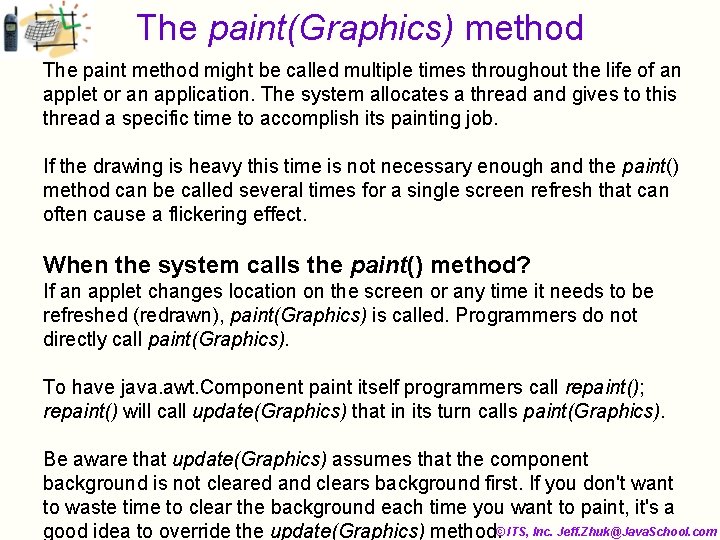
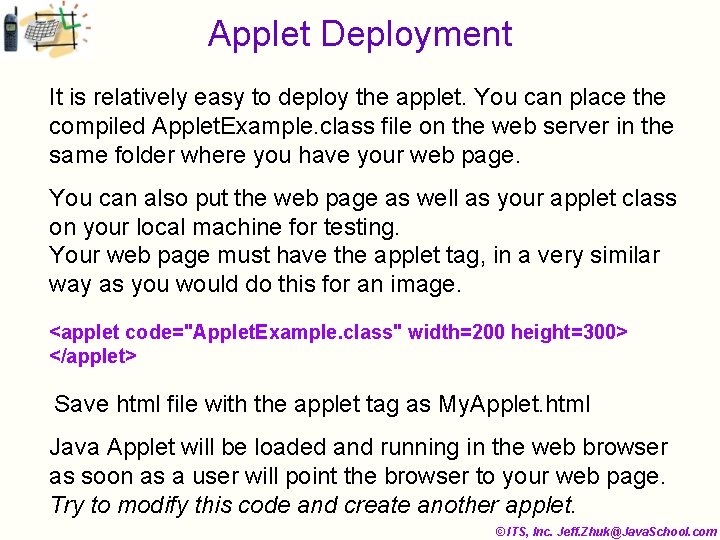
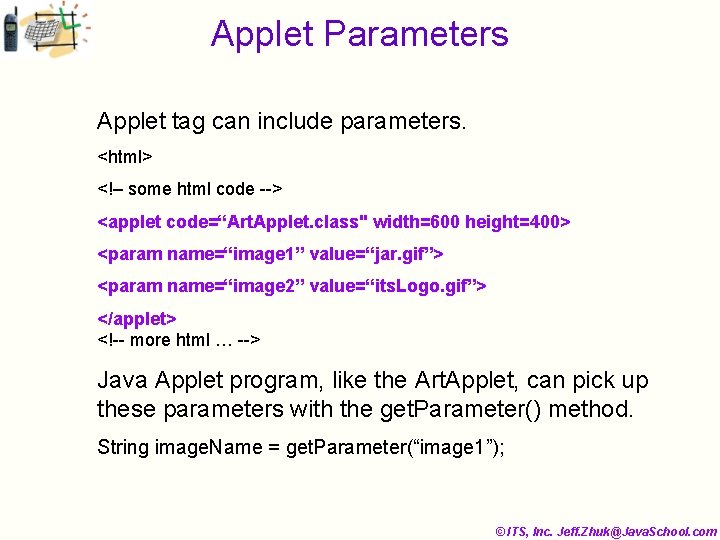
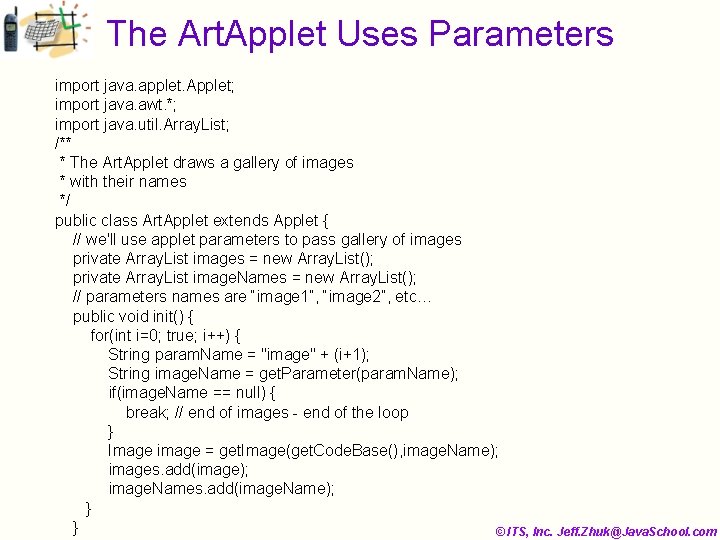
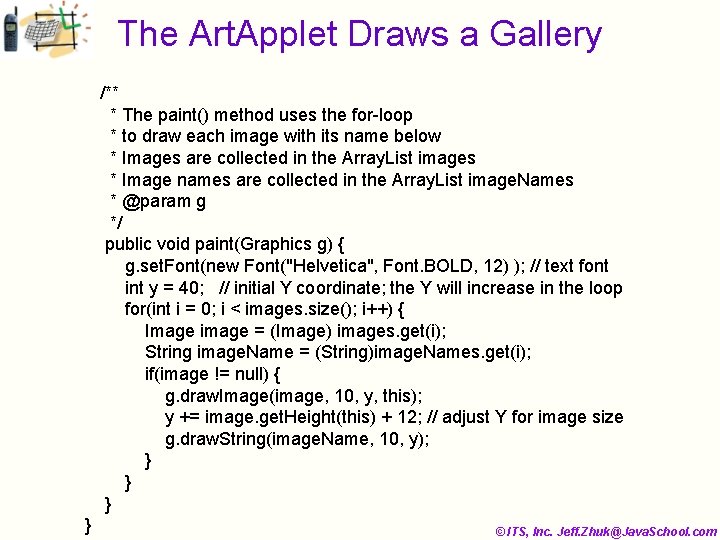
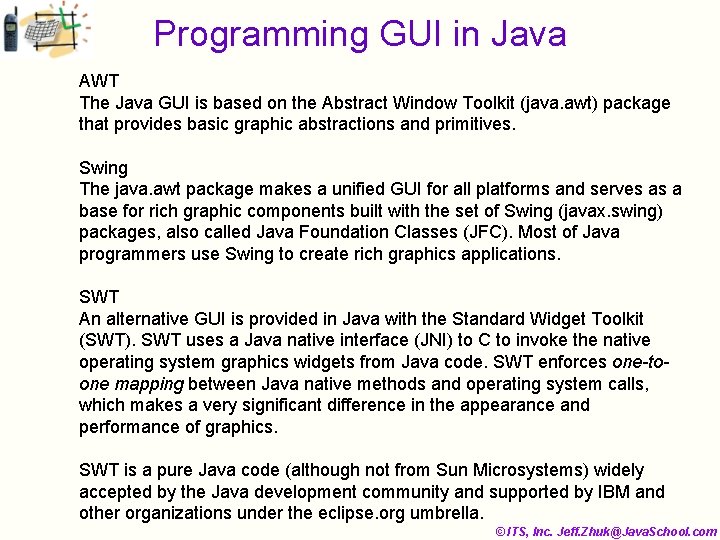
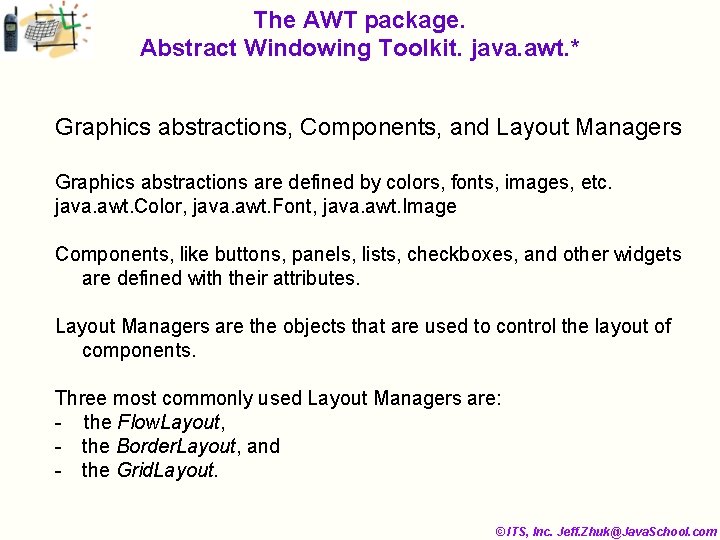
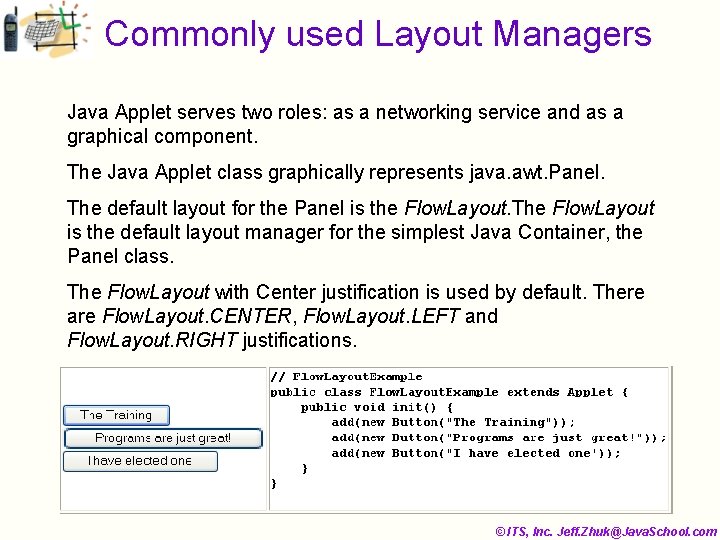
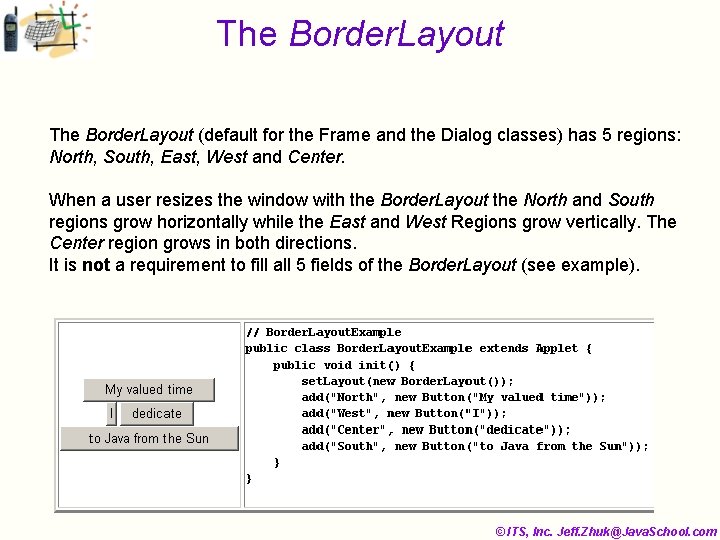
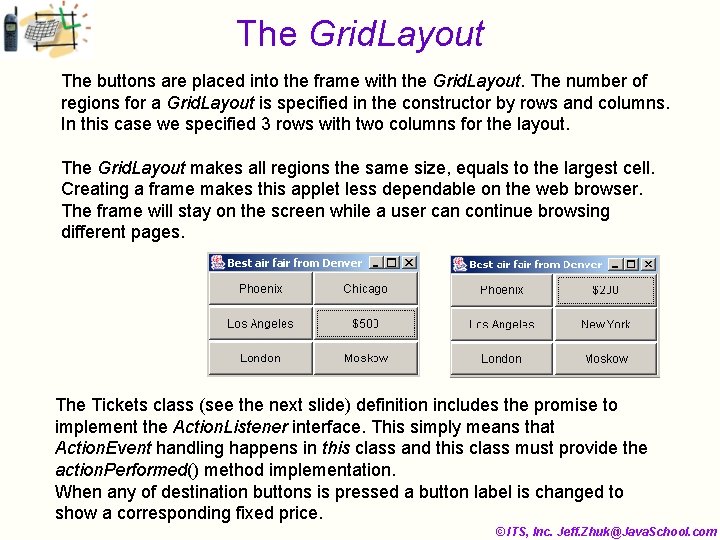
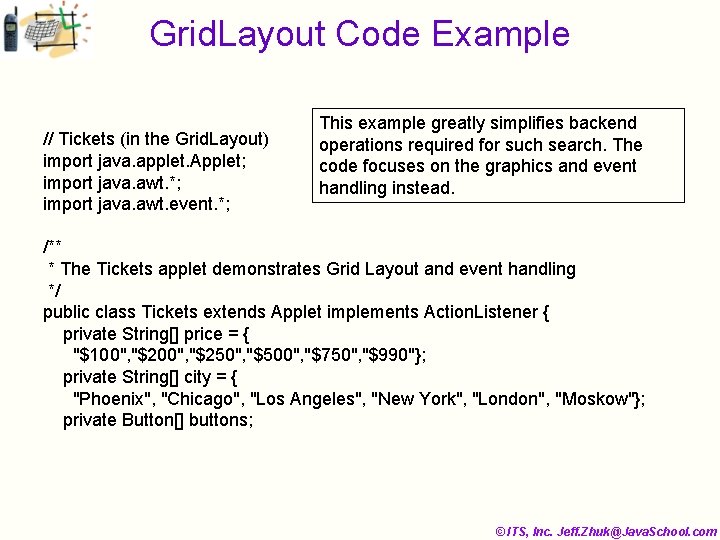
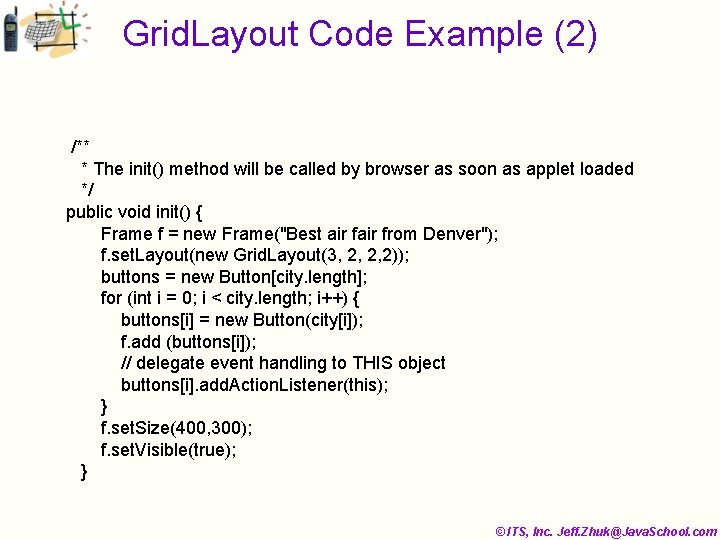
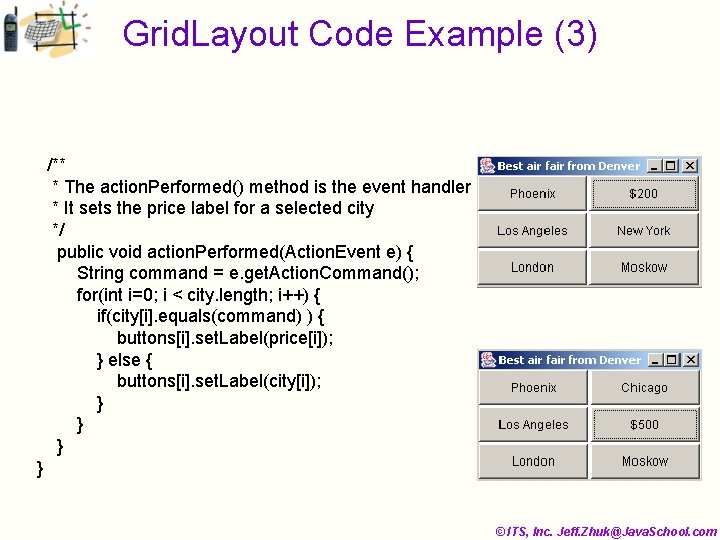
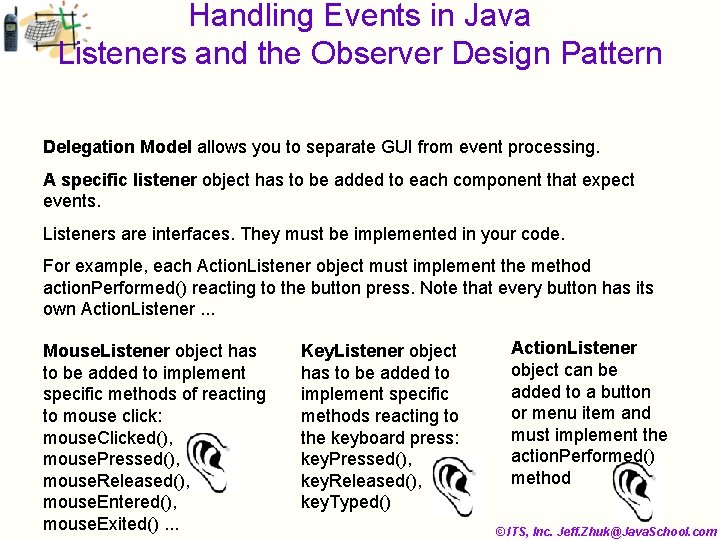
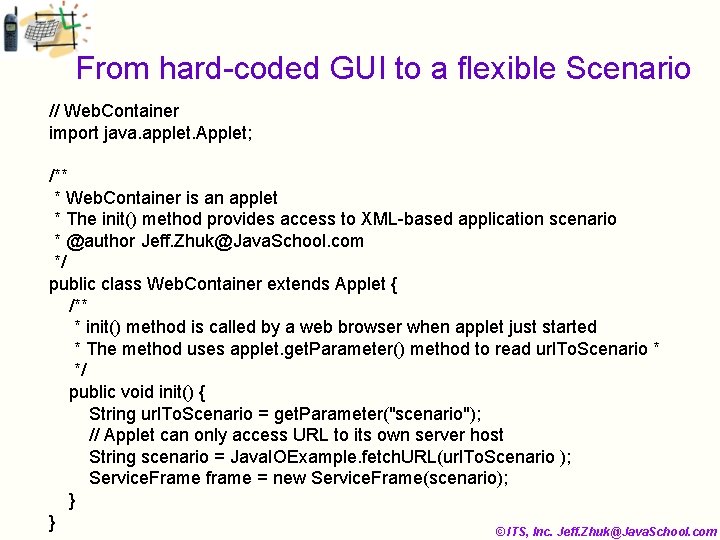
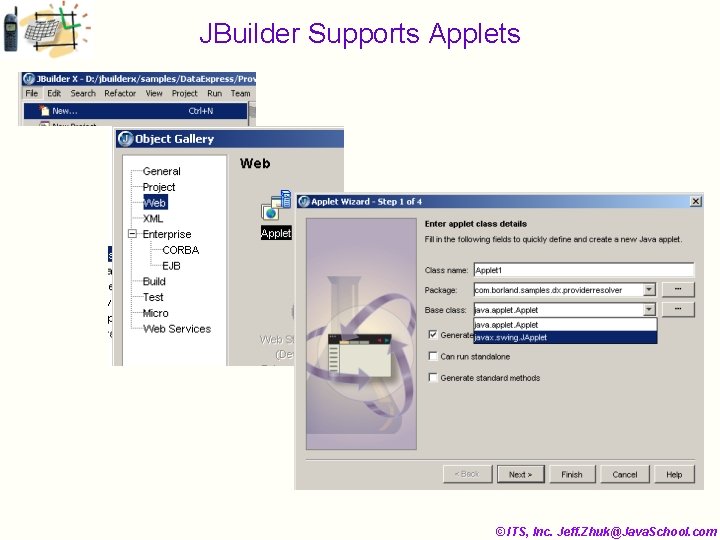
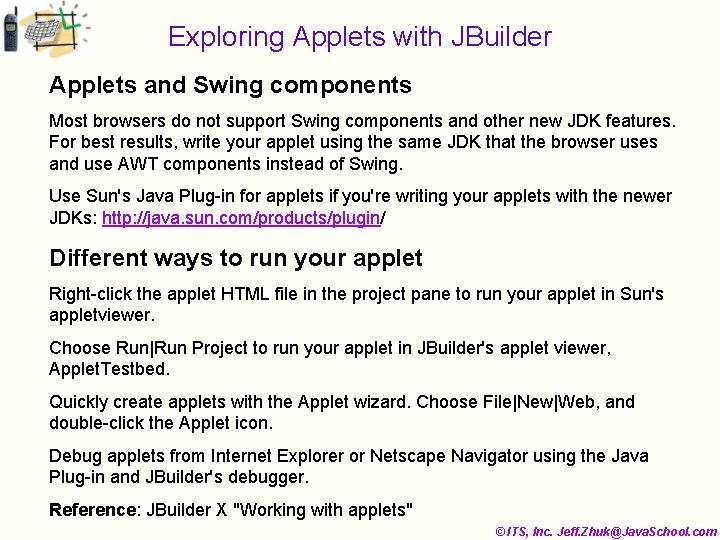
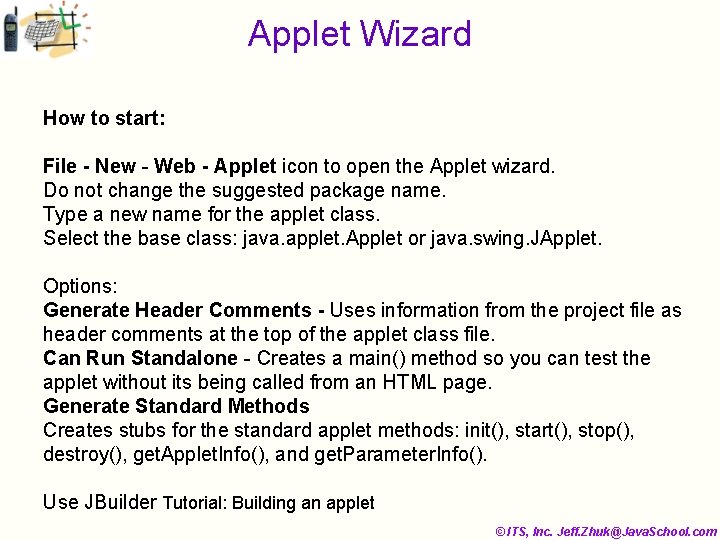
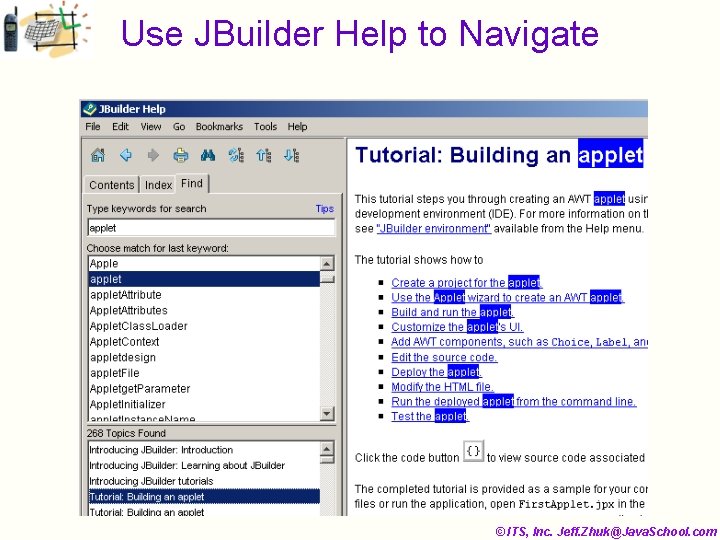
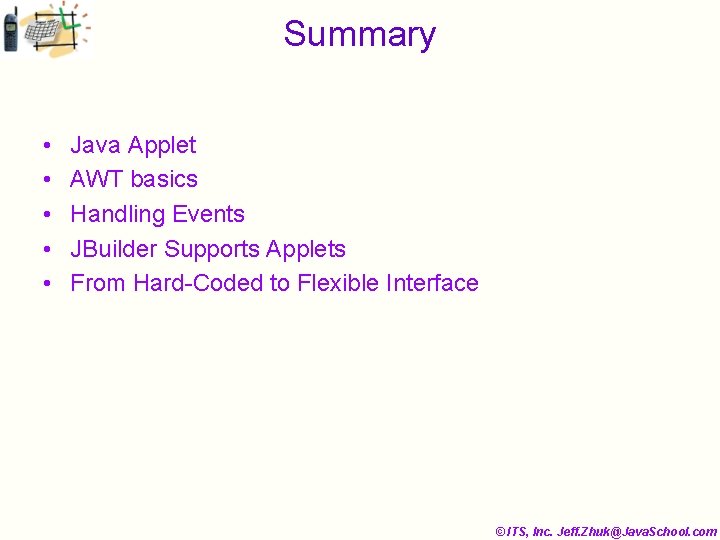
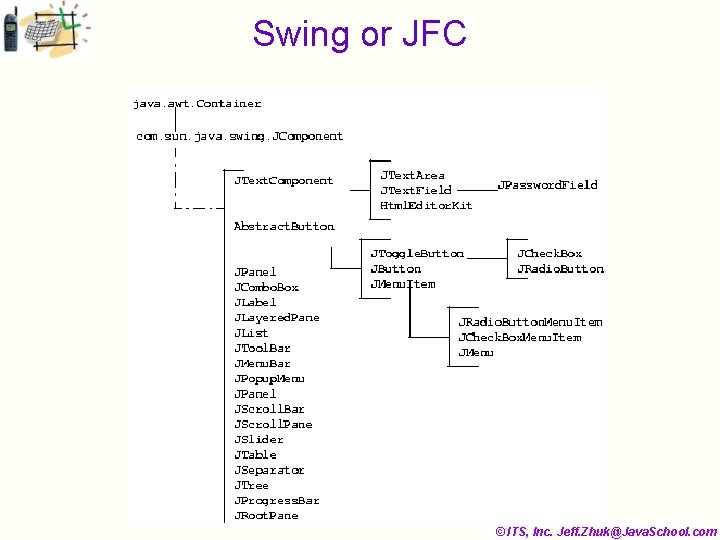
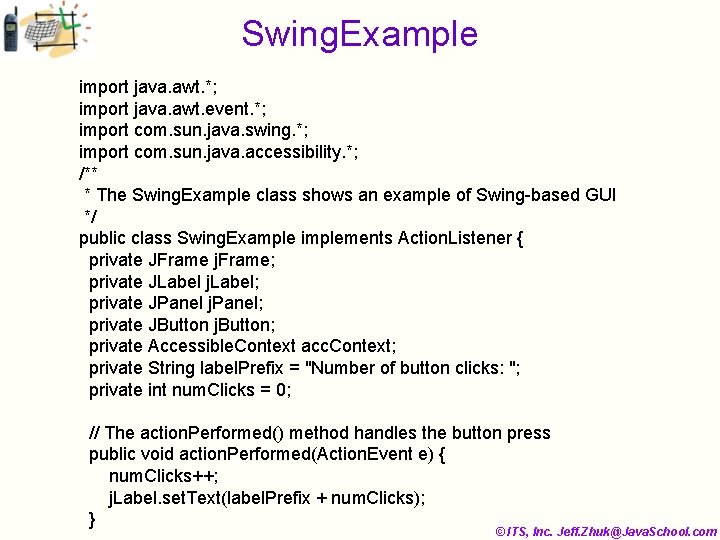
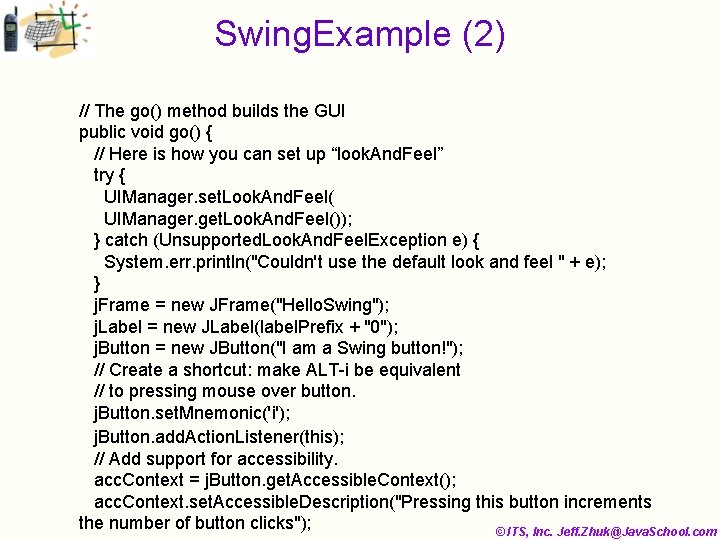
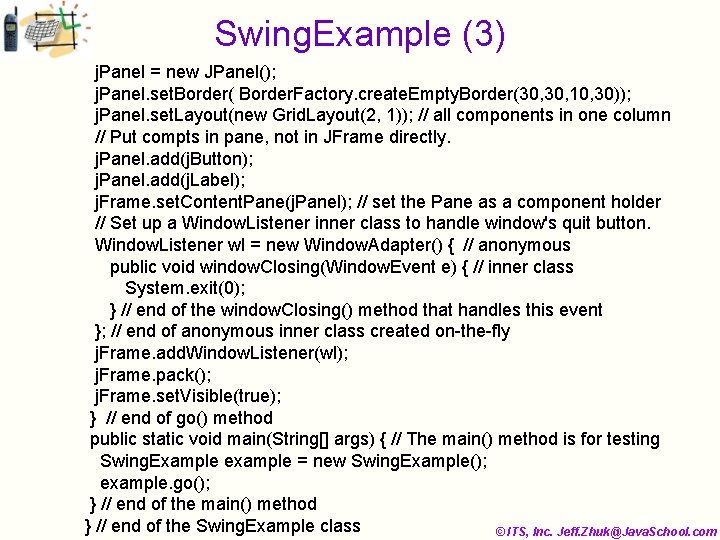
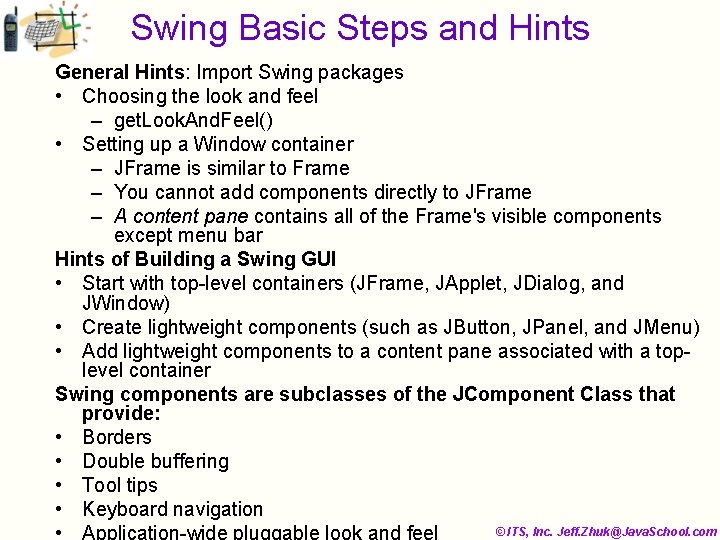
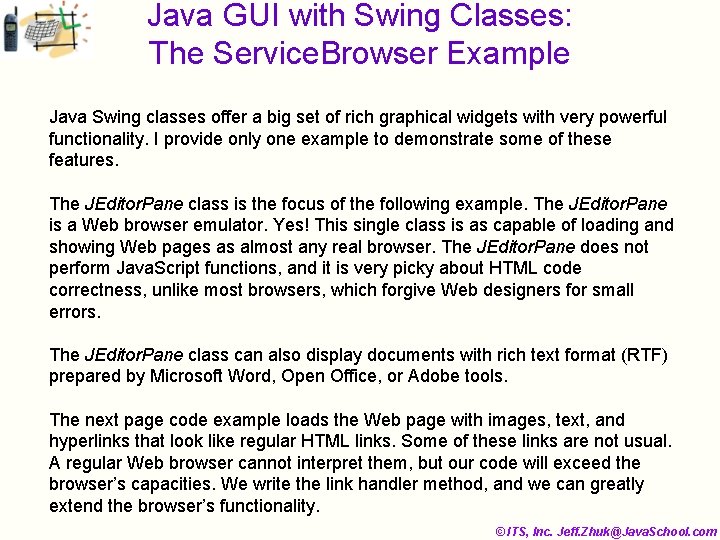
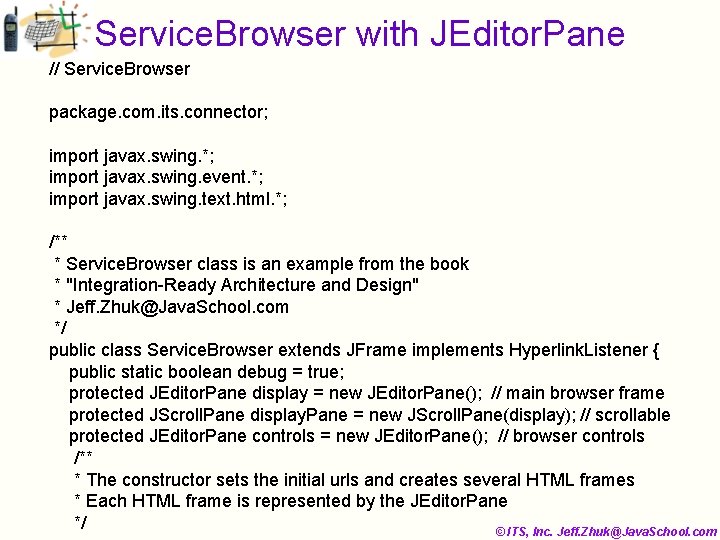
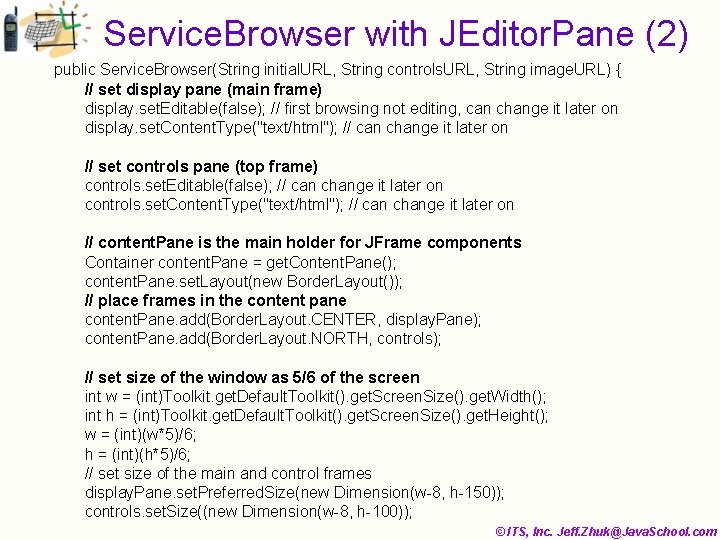
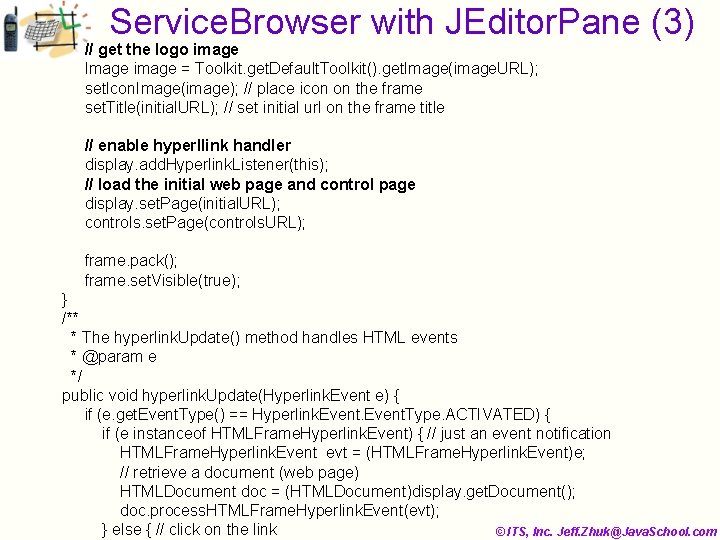
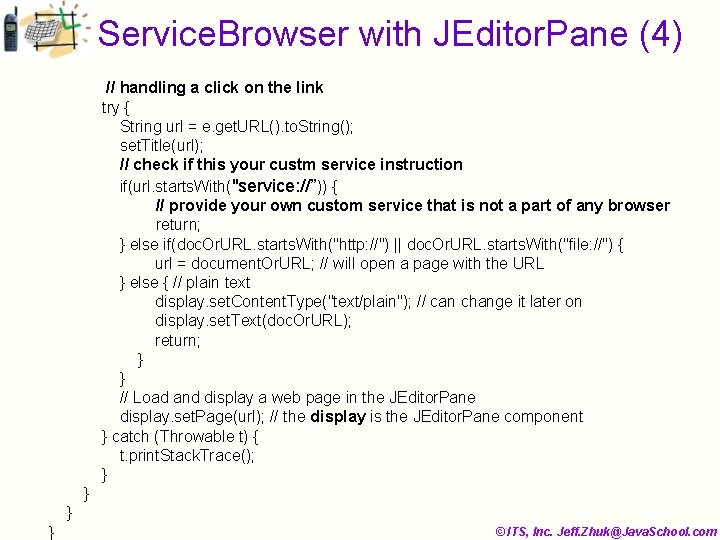
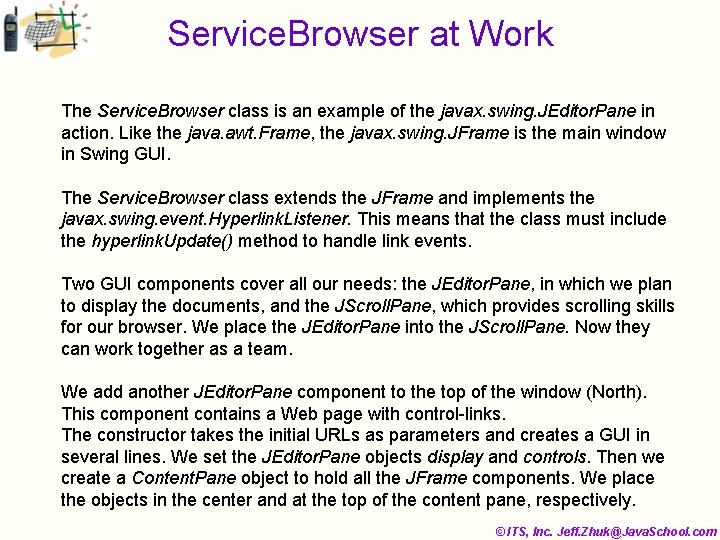
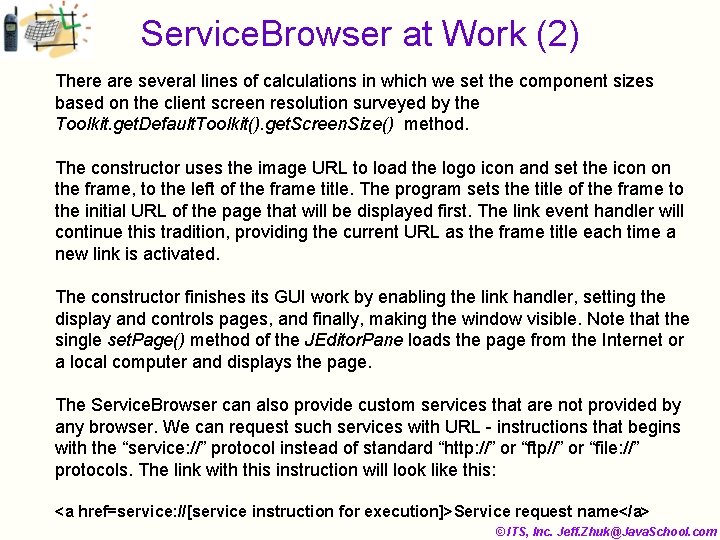
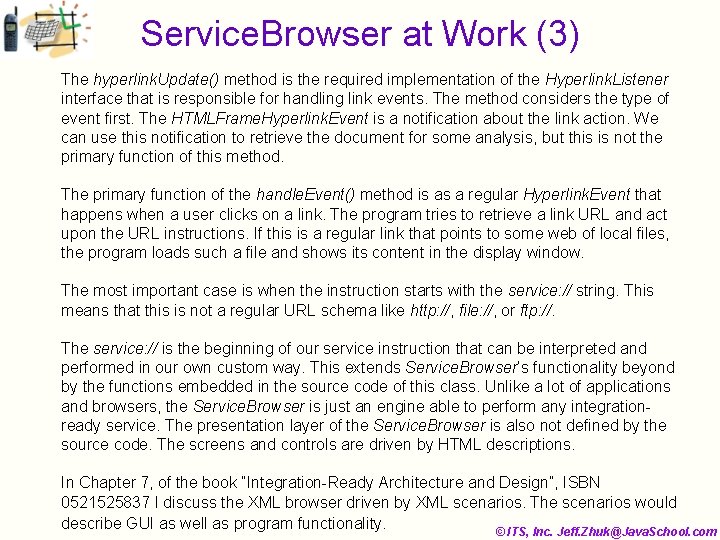
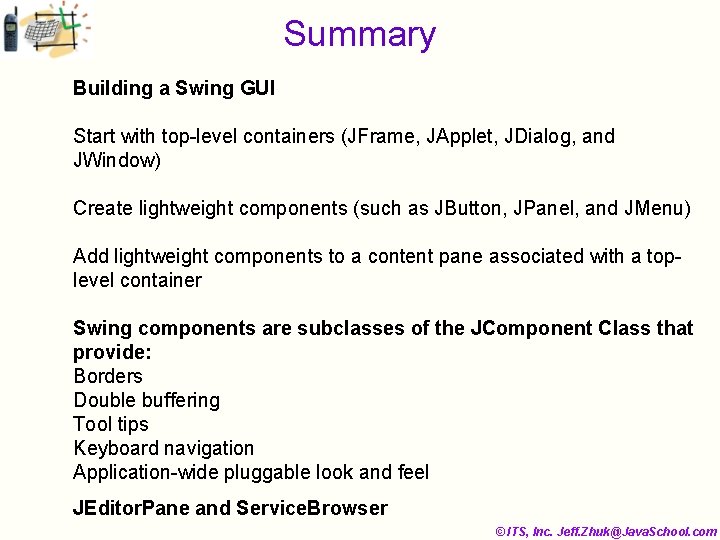
- Slides: 40
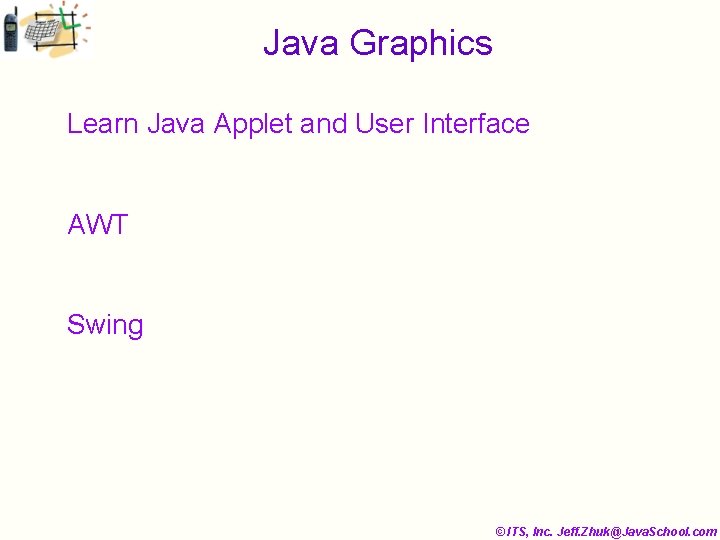
Java Graphics Learn Java Applet and User Interface AWT Swing © ITS, Inc. Jeff. Zhuk@Java. School. com
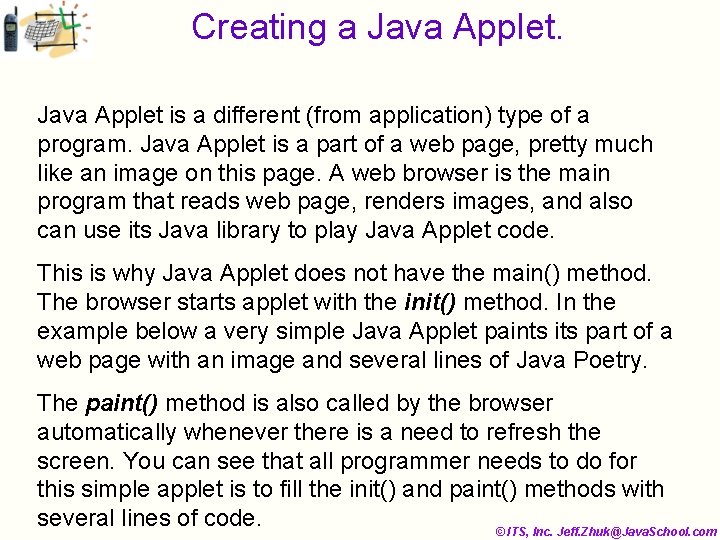
Creating a Java Applet is a different (from application) type of a program. Java Applet is a part of a web page, pretty much like an image on this page. A web browser is the main program that reads web page, renders images, and also can use its Java library to play Java Applet code. This is why Java Applet does not have the main() method. The browser starts applet with the init() method. In the example below a very simple Java Applet paints its part of a web page with an image and several lines of Java Poetry. The paint() method is also called by the browser automatically whenever there is a need to refresh the screen. You can see that all programmer needs to do for this simple applet is to fill the init() and paint() methods with several lines of code. © ITS, Inc. Jeff. Zhuk@Java. School. com
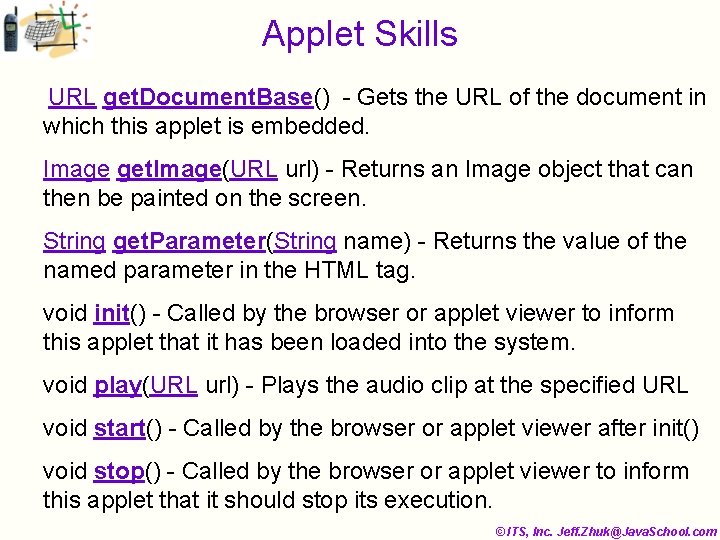
Applet Skills URL get. Document. Base() - Gets the URL of the document in which this applet is embedded. Image get. Image(URL url) - Returns an Image object that can then be painted on the screen. String get. Parameter(String name) - Returns the value of the named parameter in the HTML tag. void init() - Called by the browser or applet viewer to inform this applet that it has been loaded into the system. void play(URL url) - Plays the audio clip at the specified URL void start() - Called by the browser or applet viewer after init() void stop() - Called by the browser or applet viewer to inform this applet that it should stop its execution. © ITS, Inc. Jeff. Zhuk@Java. School. com
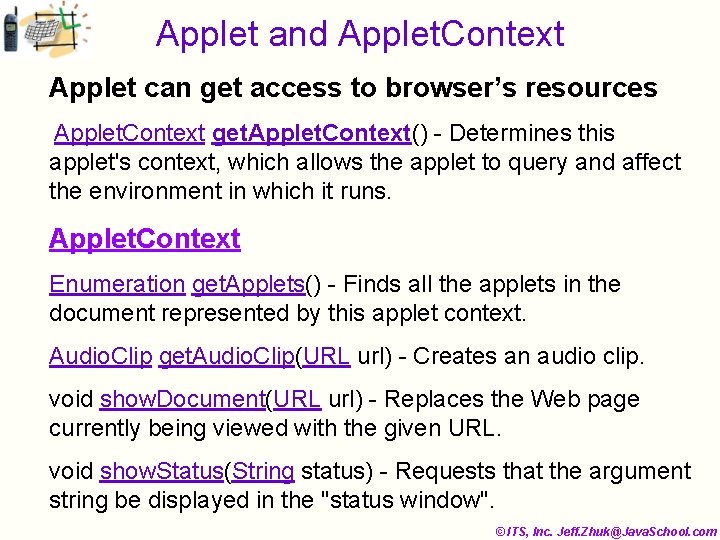
Applet and Applet. Context Applet can get access to browser’s resources Applet. Context get. Applet. Context() - Determines this applet's context, which allows the applet to query and affect the environment in which it runs. Applet. Context Enumeration get. Applets() - Finds all the applets in the document represented by this applet context. Audio. Clip get. Audio. Clip(URL url) - Creates an audio clip. void show. Document(URL url) - Replaces the Web page currently being viewed with the given URL. void show. Status(String status) - Requests that the argument string be displayed in the "status window". © ITS, Inc. Jeff. Zhuk@Java. School. com
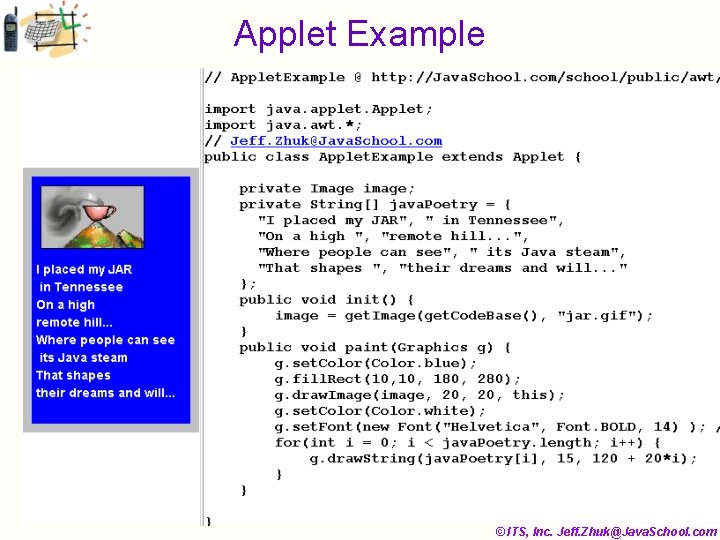
Applet Example © ITS, Inc. Jeff. Zhuk@Java. School. com
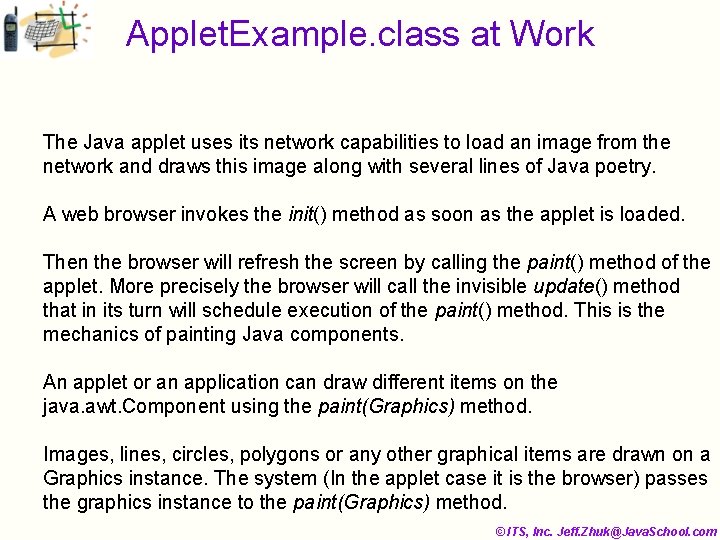
Applet. Example. class at Work The Java applet uses its network capabilities to load an image from the network and draws this image along with several lines of Java poetry. A web browser invokes the init() method as soon as the applet is loaded. Then the browser will refresh the screen by calling the paint() method of the applet. More precisely the browser will call the invisible update() method that in its turn will schedule execution of the paint() method. This is the mechanics of painting Java components. An applet or an application can draw different items on the java. awt. Component using the paint(Graphics) method. Images, lines, circles, polygons or any other graphical items are drawn on a Graphics instance. The system (In the applet case it is the browser) passes the graphics instance to the paint(Graphics) method. © ITS, Inc. Jeff. Zhuk@Java. School. com
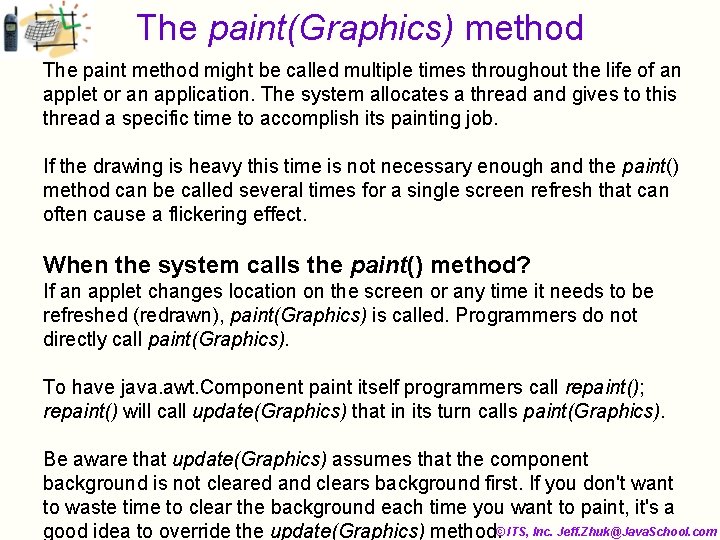
The paint(Graphics) method The paint method might be called multiple times throughout the life of an applet or an application. The system allocates a thread and gives to this thread a specific time to accomplish its painting job. If the drawing is heavy this time is not necessary enough and the paint() method can be called several times for a single screen refresh that can often cause a flickering effect. When the system calls the paint() method? If an applet changes location on the screen or any time it needs to be refreshed (redrawn), paint(Graphics) is called. Programmers do not directly call paint(Graphics). To have java. awt. Component paint itself programmers call repaint(); repaint() will call update(Graphics) that in its turn calls paint(Graphics). Be aware that update(Graphics) assumes that the component background is not cleared and clears background first. If you don't want to waste time to clear the background each time you want to paint, it's a © ITS, Inc. Jeff. Zhuk@Java. School. com good idea to override the update(Graphics) method.
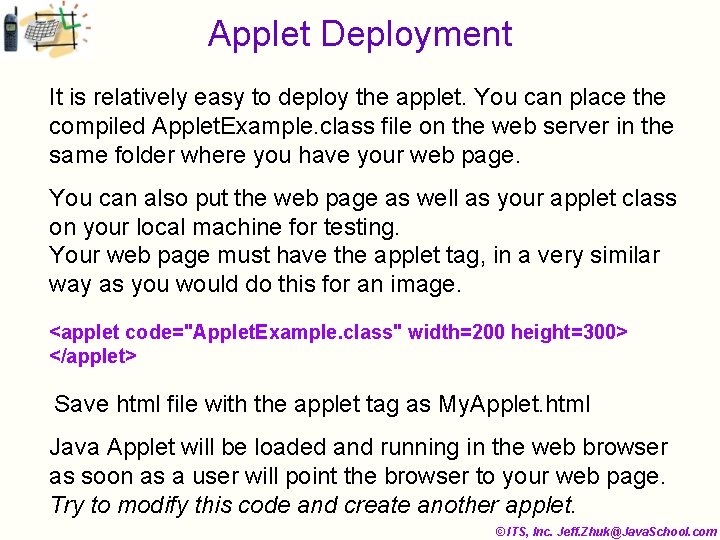
Applet Deployment It is relatively easy to deploy the applet. You can place the compiled Applet. Example. class file on the web server in the same folder where you have your web page. You can also put the web page as well as your applet class on your local machine for testing. Your web page must have the applet tag, in a very similar way as you would do this for an image. <applet code="Applet. Example. class" width=200 height=300> </applet> Save html file with the applet tag as My. Applet. html Java Applet will be loaded and running in the web browser as soon as a user will point the browser to your web page. Try to modify this code and create another applet. © ITS, Inc. Jeff. Zhuk@Java. School. com
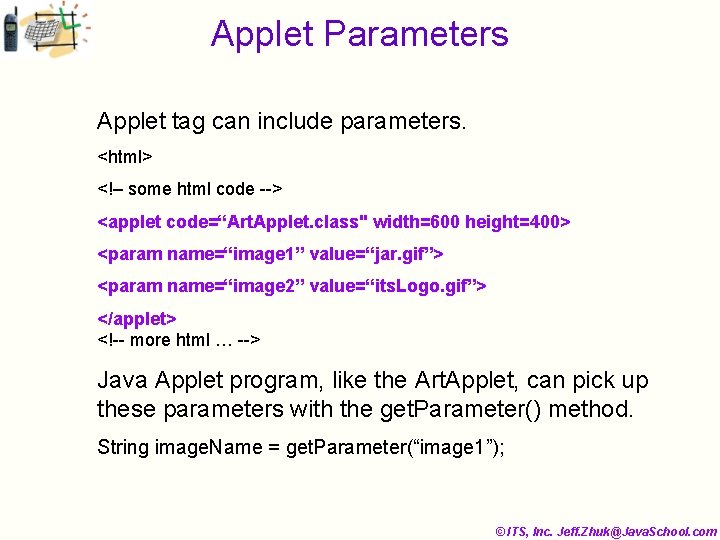
Applet Parameters Applet tag can include parameters. <html> <!– some html code --> <applet code=“Art. Applet. class" width=600 height=400> <param name=“image 1” value=“jar. gif”> <param name=“image 2” value=“its. Logo. gif”> </applet> <!-- more html … --> Java Applet program, like the Art. Applet, can pick up these parameters with the get. Parameter() method. String image. Name = get. Parameter(“image 1”); © ITS, Inc. Jeff. Zhuk@Java. School. com
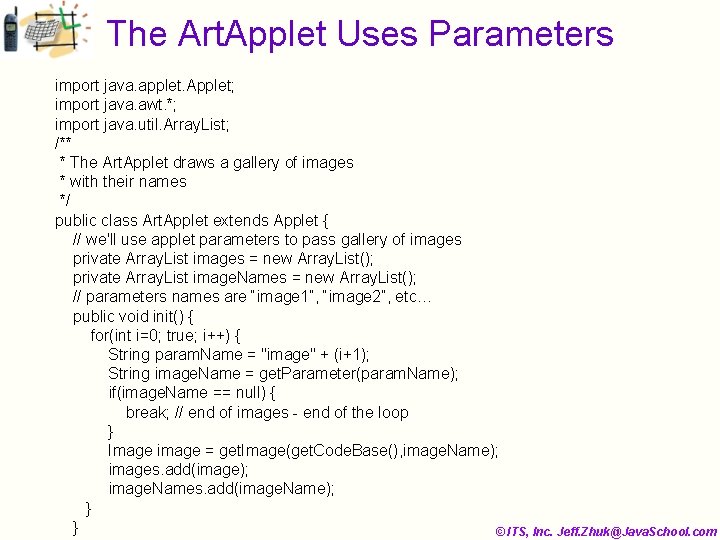
The Art. Applet Uses Parameters import java. applet. Applet; import java. awt. *; import java. util. Array. List; /** * The Art. Applet draws a gallery of images * with their names */ public class Art. Applet extends Applet { // we'll use applet parameters to pass gallery of images private Array. List images = new Array. List(); private Array. List image. Names = new Array. List(); // parameters names are “image 1”, “image 2”, etc… public void init() { for(int i=0; true; i++) { String param. Name = "image" + (i+1); String image. Name = get. Parameter(param. Name); if(image. Name == null) { break; // end of images - end of the loop } Image image = get. Image(get. Code. Base(), image. Name); images. add(image); image. Names. add(image. Name); } } © ITS, Inc. Jeff. Zhuk@Java. School. com
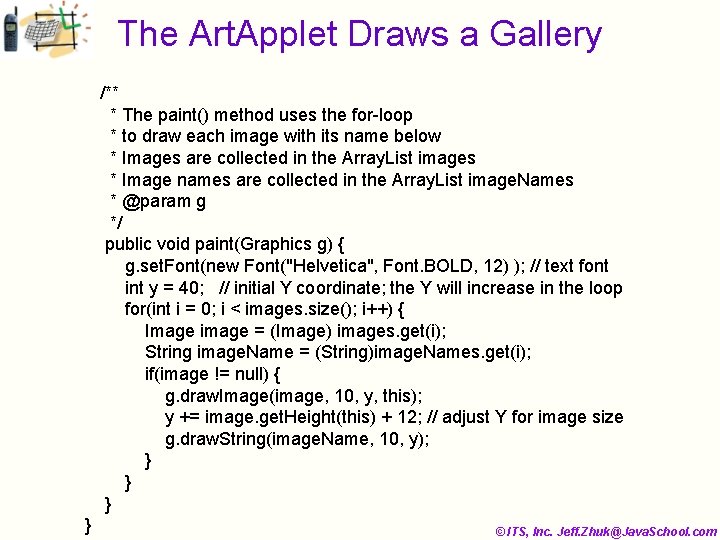
The Art. Applet Draws a Gallery /** * The paint() method uses the for-loop * to draw each image with its name below * Images are collected in the Array. List images * Image names are collected in the Array. List image. Names * @param g */ public void paint(Graphics g) { g. set. Font(new Font("Helvetica", Font. BOLD, 12) ); // text font int y = 40; // initial Y coordinate; the Y will increase in the loop for(int i = 0; i < images. size(); i++) { Image image = (Image) images. get(i); String image. Name = (String)image. Names. get(i); if(image != null) { g. draw. Image(image, 10, y, this); y += image. get. Height(this) + 12; // adjust Y for image size g. draw. String(image. Name, 10, y); } } } © ITS, Inc. Jeff. Zhuk@Java. School. com
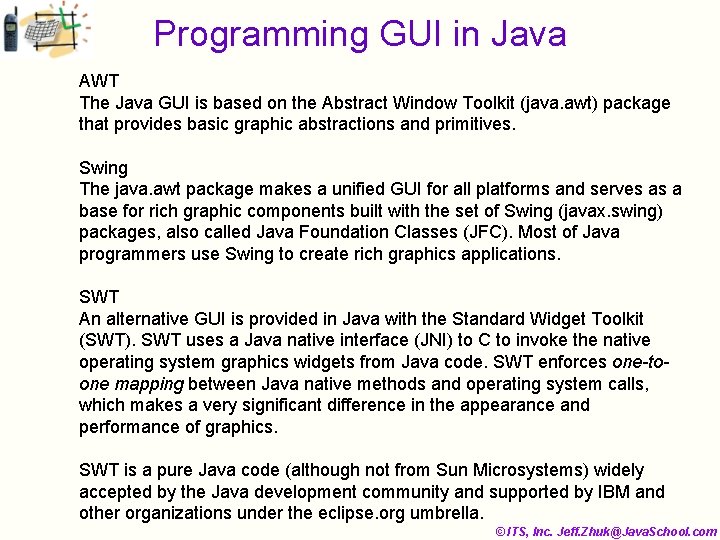
Programming GUI in Java AWT The Java GUI is based on the Abstract Window Toolkit (java. awt) package that provides basic graphic abstractions and primitives. Swing The java. awt package makes a unified GUI for all platforms and serves as a base for rich graphic components built with the set of Swing (javax. swing) packages, also called Java Foundation Classes (JFC). Most of Java programmers use Swing to create rich graphics applications. SWT An alternative GUI is provided in Java with the Standard Widget Toolkit (SWT). SWT uses a Java native interface (JNI) to C to invoke the native operating system graphics widgets from Java code. SWT enforces one-toone mapping between Java native methods and operating system calls, which makes a very significant difference in the appearance and performance of graphics. SWT is a pure Java code (although not from Sun Microsystems) widely accepted by the Java development community and supported by IBM and other organizations under the eclipse. org umbrella. © ITS, Inc. Jeff. Zhuk@Java. School. com
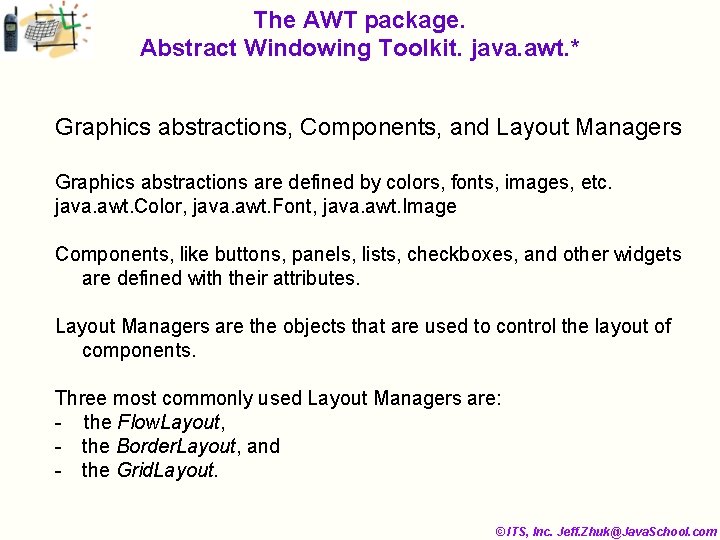
The AWT package. Abstract Windowing Toolkit. java. awt. * Graphics abstractions, Components, and Layout Managers Graphics abstractions are defined by colors, fonts, images, etc. java. awt. Color, java. awt. Font, java. awt. Image Components, like buttons, panels, lists, checkboxes, and other widgets are defined with their attributes. Layout Managers are the objects that are used to control the layout of components. Three most commonly used Layout Managers are: - the Flow. Layout, - the Border. Layout, and - the Grid. Layout. © ITS, Inc. Jeff. Zhuk@Java. School. com
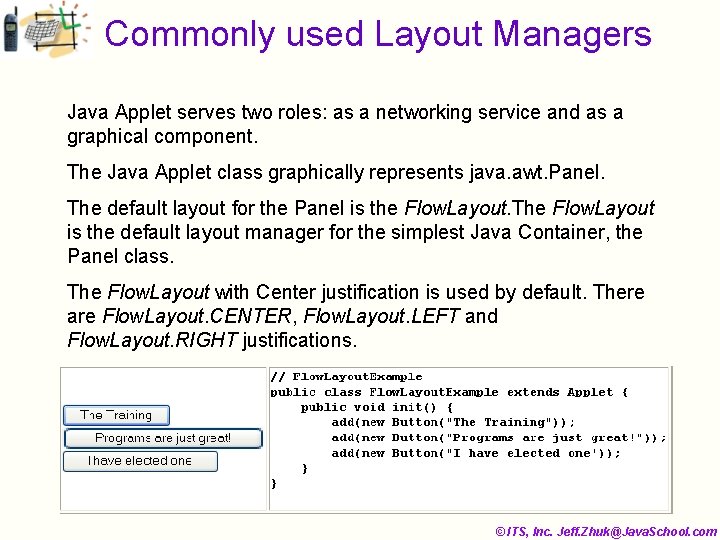
Commonly used Layout Managers Java Applet serves two roles: as a networking service and as a graphical component. The Java Applet class graphically represents java. awt. Panel. The default layout for the Panel is the Flow. Layout. The Flow. Layout is the default layout manager for the simplest Java Container, the Panel class. The Flow. Layout with Center justification is used by default. There are Flow. Layout. CENTER, Flow. Layout. LEFT and Flow. Layout. RIGHT justifications. © ITS, Inc. Jeff. Zhuk@Java. School. com
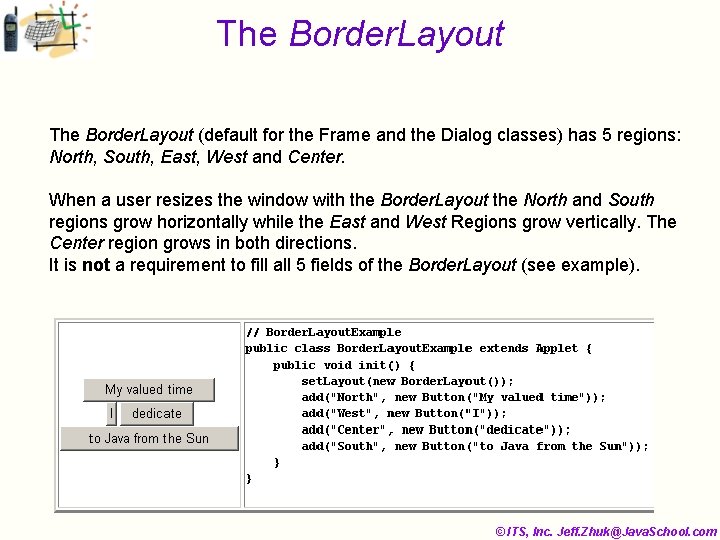
The Border. Layout (default for the Frame and the Dialog classes) has 5 regions: North, South, East, West and Center. When a user resizes the window with the Border. Layout the North and South regions grow horizontally while the East and West Regions grow vertically. The Center region grows in both directions. It is not a requirement to fill all 5 fields of the Border. Layout (see example). © ITS, Inc. Jeff. Zhuk@Java. School. com
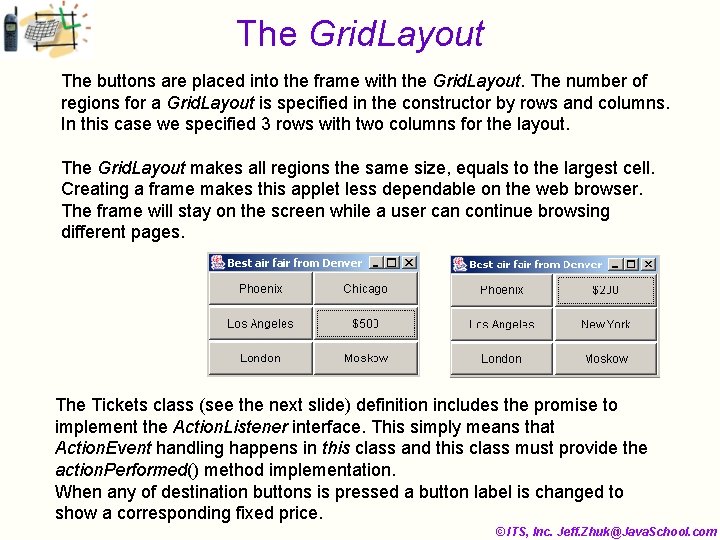
The Grid. Layout The buttons are placed into the frame with the Grid. Layout. The number of regions for a Grid. Layout is specified in the constructor by rows and columns. In this case we specified 3 rows with two columns for the layout. The Grid. Layout makes all regions the same size, equals to the largest cell. Creating a frame makes this applet less dependable on the web browser. The frame will stay on the screen while a user can continue browsing different pages. The Tickets class (see the next slide) definition includes the promise to implement the Action. Listener interface. This simply means that Action. Event handling happens in this class and this class must provide the action. Performed() method implementation. When any of destination buttons is pressed a button label is changed to show a corresponding fixed price. © ITS, Inc. Jeff. Zhuk@Java. School. com
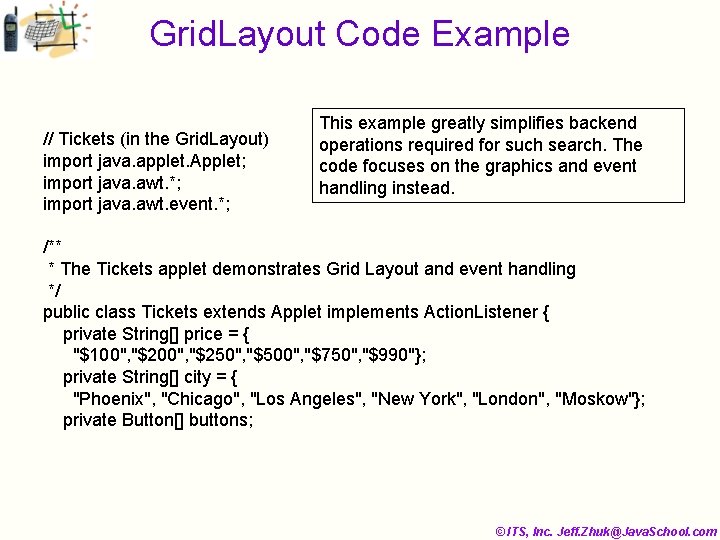
Grid. Layout Code Example // Tickets (in the Grid. Layout) import java. applet. Applet; import java. awt. *; import java. awt. event. *; This example greatly simplifies backend operations required for such search. The code focuses on the graphics and event handling instead. /** * The Tickets applet demonstrates Grid Layout and event handling */ public class Tickets extends Applet implements Action. Listener { private String[] price = { "$100", "$250", "$500", "$750", "$990"}; private String[] city = { "Phoenix", "Chicago", "Los Angeles", "New York", "London", "Moskow"}; private Button[] buttons; © ITS, Inc. Jeff. Zhuk@Java. School. com
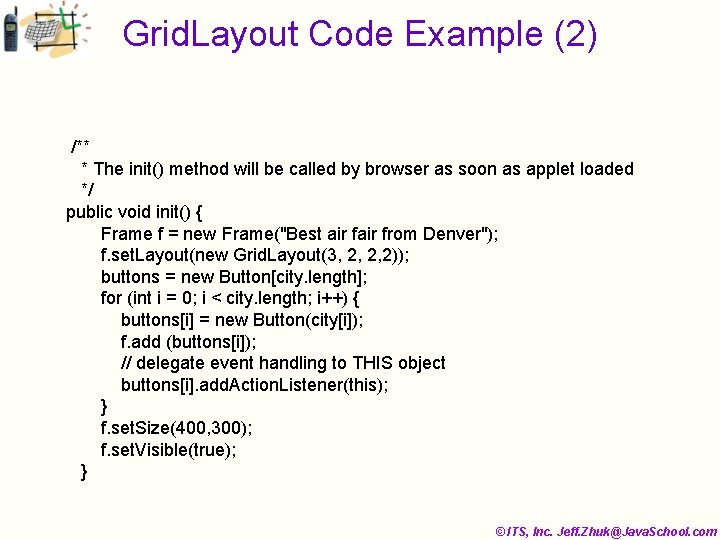
Grid. Layout Code Example (2) /** * The init() method will be called by browser as soon as applet loaded */ public void init() { Frame f = new Frame("Best air from Denver"); f. set. Layout(new Grid. Layout(3, 2, 2, 2)); buttons = new Button[city. length]; for (int i = 0; i < city. length; i++) { buttons[i] = new Button(city[i]); f. add (buttons[i]); // delegate event handling to THIS object buttons[i]. add. Action. Listener(this); } f. set. Size(400, 300); f. set. Visible(true); } © ITS, Inc. Jeff. Zhuk@Java. School. com
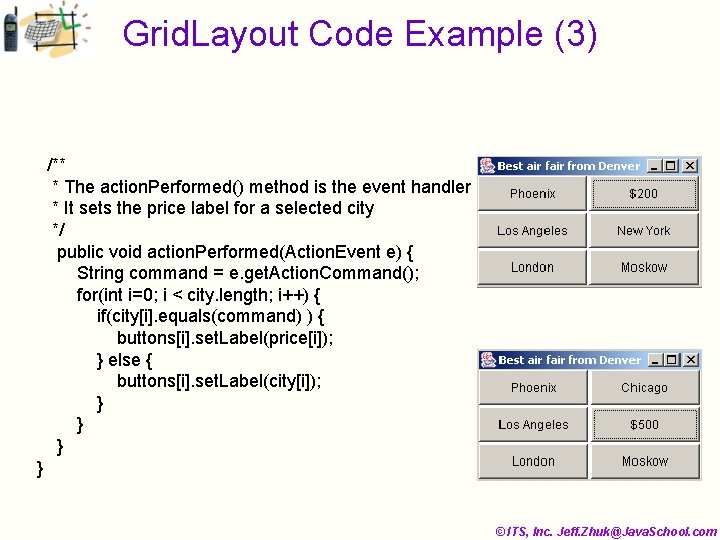
Grid. Layout Code Example (3) /** * The action. Performed() method is the event handler * It sets the price label for a selected city */ public void action. Performed(Action. Event e) { String command = e. get. Action. Command(); for(int i=0; i < city. length; i++) { if(city[i]. equals(command) ) { buttons[i]. set. Label(price[i]); } else { buttons[i]. set. Label(city[i]); } } } © ITS, Inc. Jeff. Zhuk@Java. School. com
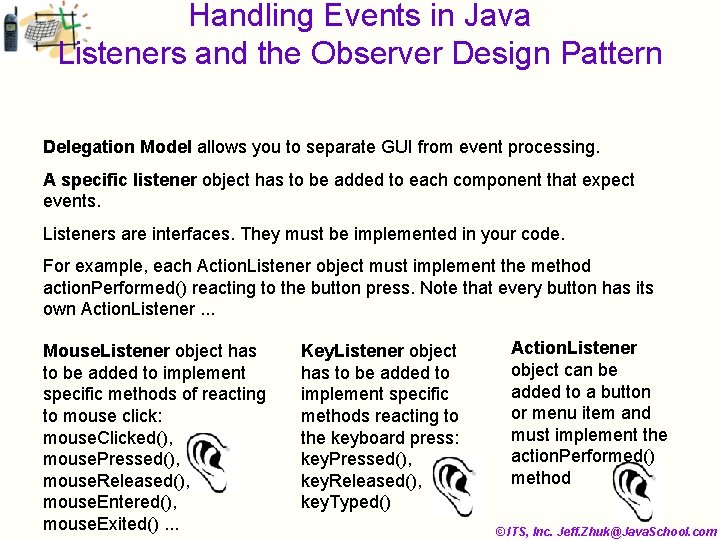
Handling Events in Java Listeners and the Observer Design Pattern Delegation Model allows you to separate GUI from event processing. A specific listener object has to be added to each component that expect events. Listeners are interfaces. They must be implemented in your code. For example, each Action. Listener object must implement the method action. Performed() reacting to the button press. Note that every button has its own Action. Listener. . . Mouse. Listener object has to be added to implement specific methods of reacting to mouse click: mouse. Clicked(), mouse. Pressed(), mouse. Released(), mouse. Entered(), mouse. Exited(). . . Key. Listener object has to be added to implement specific methods reacting to the keyboard press: key. Pressed(), key. Released(), key. Typed() Action. Listener object can be added to a button or menu item and must implement the action. Performed() method © ITS, Inc. Jeff. Zhuk@Java. School. com
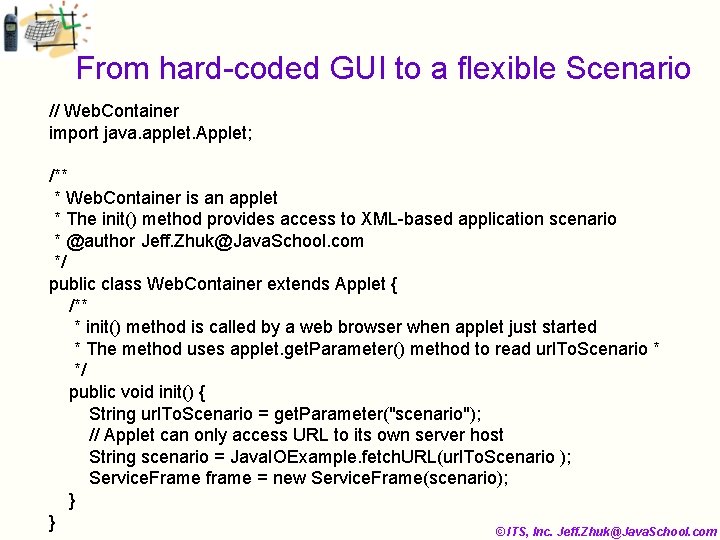
From hard-coded GUI to a flexible Scenario // Web. Container import java. applet. Applet; /** * Web. Container is an applet * The init() method provides access to XML-based application scenario * @author Jeff. Zhuk@Java. School. com */ public class Web. Container extends Applet { /** * init() method is called by a web browser when applet just started * The method uses applet. get. Parameter() method to read url. To. Scenario * */ public void init() { String url. To. Scenario = get. Parameter("scenario"); // Applet can only access URL to its own server host String scenario = Java. IOExample. fetch. URL(url. To. Scenario ); Service. Frame frame = new Service. Frame(scenario); } } © ITS, Inc. Jeff. Zhuk@Java. School. com
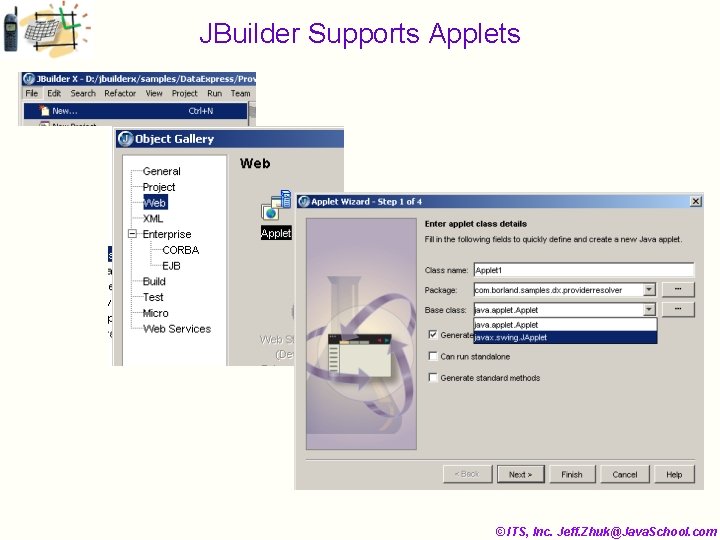
JBuilder Supports Applets © ITS, Inc. Jeff. Zhuk@Java. School. com
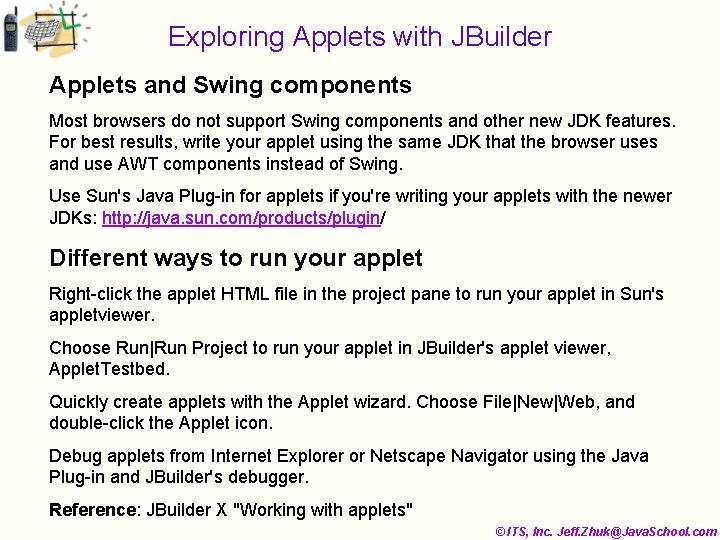
Exploring Applets with JBuilder Applets and Swing components Most browsers do not support Swing components and other new JDK features. For best results, write your applet using the same JDK that the browser uses and use AWT components instead of Swing. Use Sun's Java Plug-in for applets if you're writing your applets with the newer JDKs: http: //java. sun. com/products/plugin/ Different ways to run your applet Right-click the applet HTML file in the project pane to run your applet in Sun's appletviewer. Choose Run|Run Project to run your applet in JBuilder's applet viewer, Applet. Testbed. Quickly create applets with the Applet wizard. Choose File|New|Web, and double-click the Applet icon. Debug applets from Internet Explorer or Netscape Navigator using the Java Plug-in and JBuilder's debugger. Reference: JBuilder X "Working with applets" © ITS, Inc. Jeff. Zhuk@Java. School. com
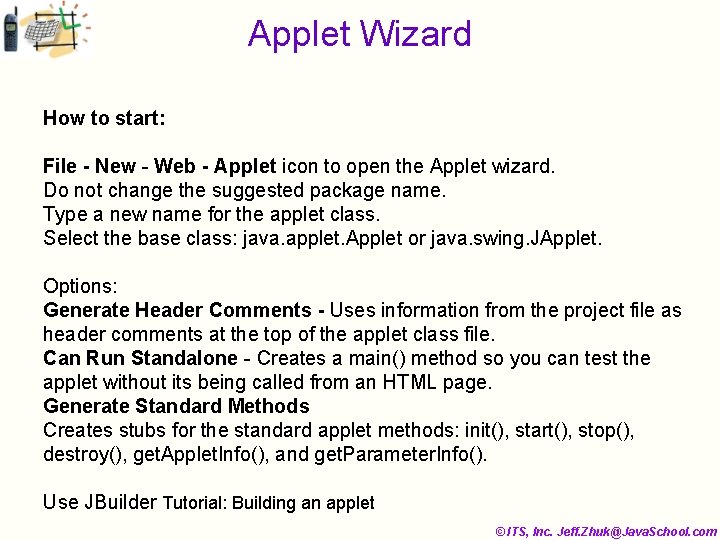
Applet Wizard How to start: File - New - Web - Applet icon to open the Applet wizard. Do not change the suggested package name. Type a new name for the applet class. Select the base class: java. applet. Applet or java. swing. JApplet. Options: Generate Header Comments - Uses information from the project file as header comments at the top of the applet class file. Can Run Standalone - Creates a main() method so you can test the applet without its being called from an HTML page. Generate Standard Methods Creates stubs for the standard applet methods: init(), start(), stop(), destroy(), get. Applet. Info(), and get. Parameter. Info(). Use JBuilder Tutorial: Building an applet © ITS, Inc. Jeff. Zhuk@Java. School. com
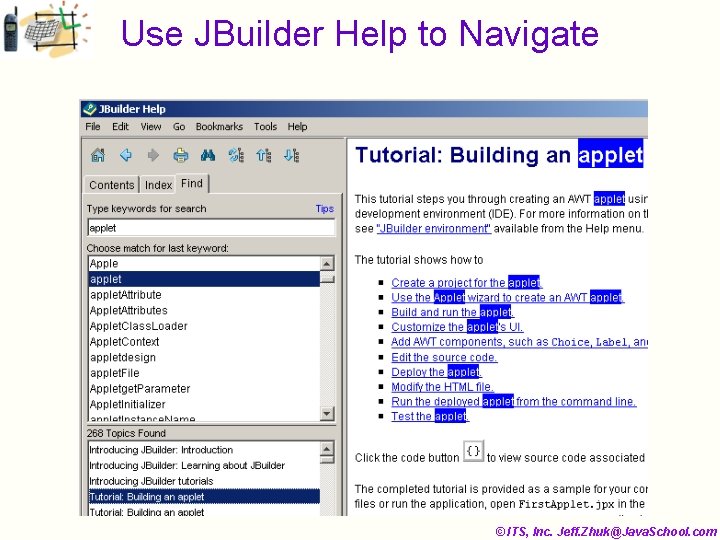
Use JBuilder Help to Navigate © ITS, Inc. Jeff. Zhuk@Java. School. com
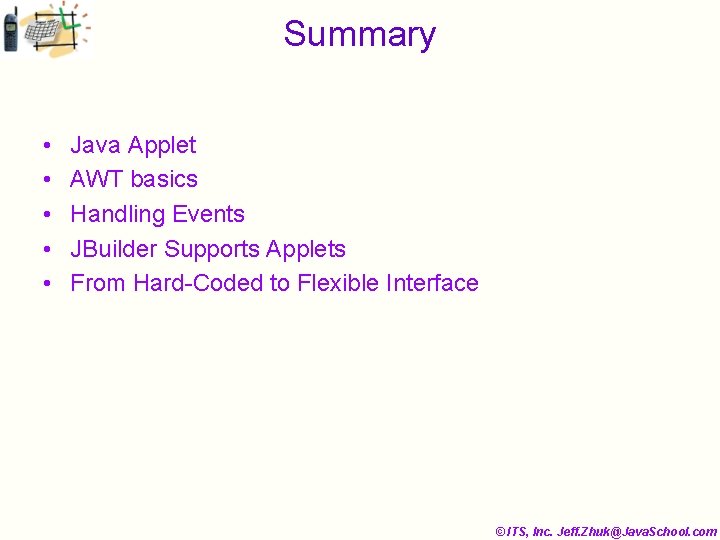
Summary • • • Java Applet AWT basics Handling Events JBuilder Supports Applets From Hard-Coded to Flexible Interface © ITS, Inc. Jeff. Zhuk@Java. School. com
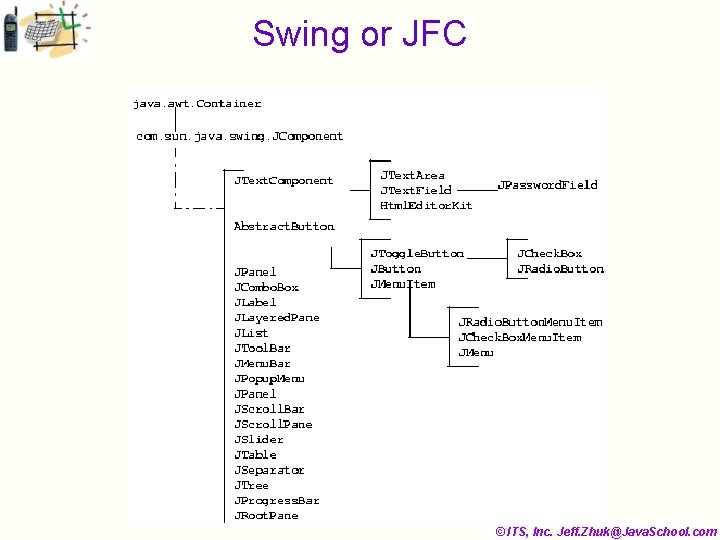
Swing or JFC © ITS, Inc. Jeff. Zhuk@Java. School. com
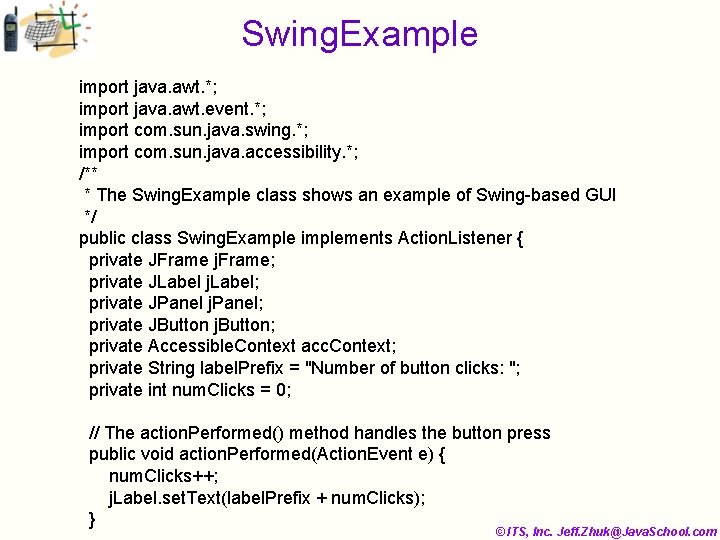
Swing. Example import java. awt. *; import java. awt. event. *; import com. sun. java. swing. *; import com. sun. java. accessibility. *; /** * The Swing. Example class shows an example of Swing-based GUI */ public class Swing. Example implements Action. Listener { private JFrame j. Frame; private JLabel j. Label; private JPanel j. Panel; private JButton j. Button; private Accessible. Context acc. Context; private String label. Prefix = "Number of button clicks: "; private int num. Clicks = 0; // The action. Performed() method handles the button press public void action. Performed(Action. Event e) { num. Clicks++; j. Label. set. Text(label. Prefix + num. Clicks); } © ITS, Inc. Jeff. Zhuk@Java. School. com
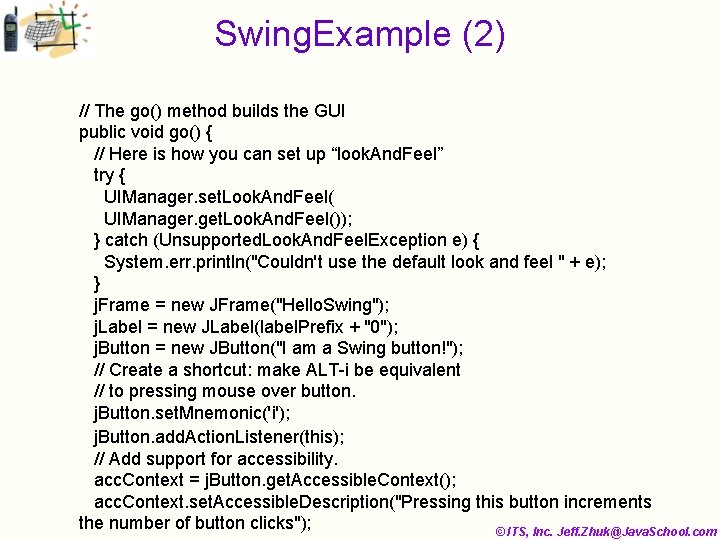
Swing. Example (2) // The go() method builds the GUI public void go() { // Here is how you can set up “look. And. Feel” try { UIManager. set. Look. And. Feel( UIManager. get. Look. And. Feel()); } catch (Unsupported. Look. And. Feel. Exception e) { System. err. println("Couldn't use the default look and feel " + e); } j. Frame = new JFrame("Hello. Swing"); j. Label = new JLabel(label. Prefix + "0"); j. Button = new JButton("I am a Swing button!"); // Create a shortcut: make ALT-i be equivalent // to pressing mouse over button. j. Button. set. Mnemonic('i'); j. Button. add. Action. Listener(this); // Add support for accessibility. acc. Context = j. Button. get. Accessible. Context(); acc. Context. set. Accessible. Description("Pressing this button increments the number of button clicks"); © ITS, Inc. Jeff. Zhuk@Java. School. com
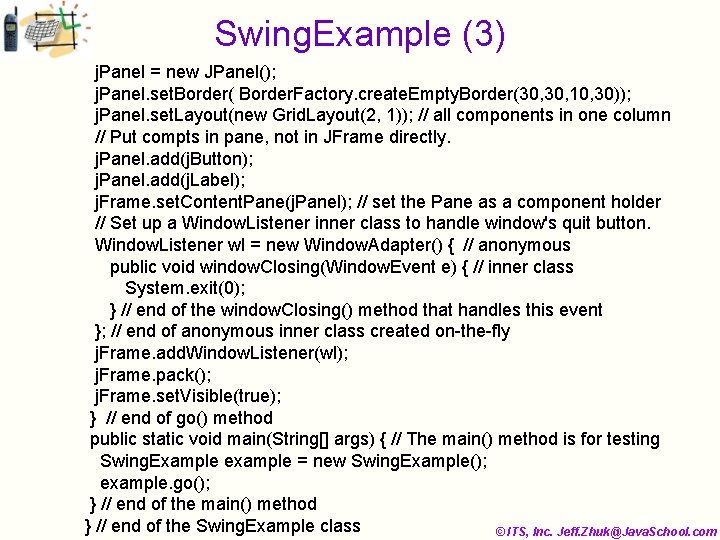
Swing. Example (3) j. Panel = new JPanel(); j. Panel. set. Border( Border. Factory. create. Empty. Border(30, 10, 30)); j. Panel. set. Layout(new Grid. Layout(2, 1)); // all components in one column // Put compts in pane, not in JFrame directly. j. Panel. add(j. Button); j. Panel. add(j. Label); j. Frame. set. Content. Pane(j. Panel); // set the Pane as a component holder // Set up a Window. Listener inner class to handle window's quit button. Window. Listener wl = new Window. Adapter() { // anonymous public void window. Closing(Window. Event e) { // inner class System. exit(0); } // end of the window. Closing() method that handles this event }; // end of anonymous inner class created on-the-fly j. Frame. add. Window. Listener(wl); j. Frame. pack(); j. Frame. set. Visible(true); } // end of go() method public static void main(String[] args) { // The main() method is for testing Swing. Example example = new Swing. Example(); example. go(); } // end of the main() method } // end of the Swing. Example class © ITS, Inc. Jeff. Zhuk@Java. School. com
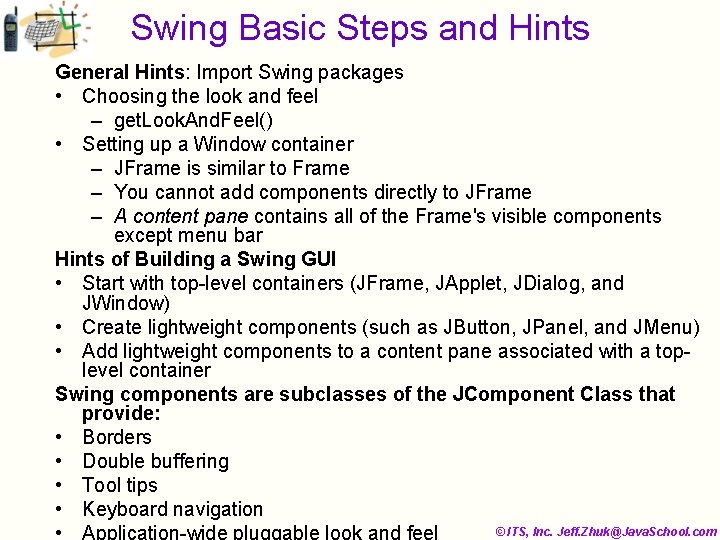
Swing Basic Steps and Hints General Hints: Import Swing packages • Choosing the look and feel – get. Look. And. Feel() • Setting up a Window container – JFrame is similar to Frame – You cannot add components directly to JFrame – A content pane contains all of the Frame's visible components except menu bar Hints of Building a Swing GUI • Start with top-level containers (JFrame, JApplet, JDialog, and JWindow) • Create lightweight components (such as JButton, JPanel, and JMenu) • Add lightweight components to a content pane associated with a toplevel container Swing components are subclasses of the JComponent Class that provide: • Borders • Double buffering • Tool tips • Keyboard navigation © ITS, Inc. Jeff. Zhuk@Java. School. com • Application-wide pluggable look and feel
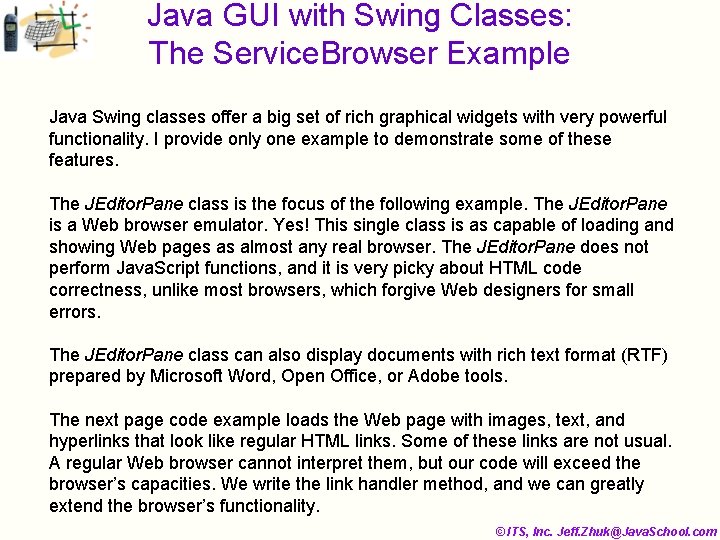
Java GUI with Swing Classes: The Service. Browser Example Java Swing classes offer a big set of rich graphical widgets with very powerful functionality. I provide only one example to demonstrate some of these features. The JEditor. Pane class is the focus of the following example. The JEditor. Pane is a Web browser emulator. Yes! This single class is as capable of loading and showing Web pages as almost any real browser. The JEditor. Pane does not perform Java. Script functions, and it is very picky about HTML code correctness, unlike most browsers, which forgive Web designers for small errors. The JEditor. Pane class can also display documents with rich text format (RTF) prepared by Microsoft Word, Open Office, or Adobe tools. The next page code example loads the Web page with images, text, and hyperlinks that look like regular HTML links. Some of these links are not usual. A regular Web browser cannot interpret them, but our code will exceed the browser’s capacities. We write the link handler method, and we can greatly extend the browser’s functionality. © ITS, Inc. Jeff. Zhuk@Java. School. com
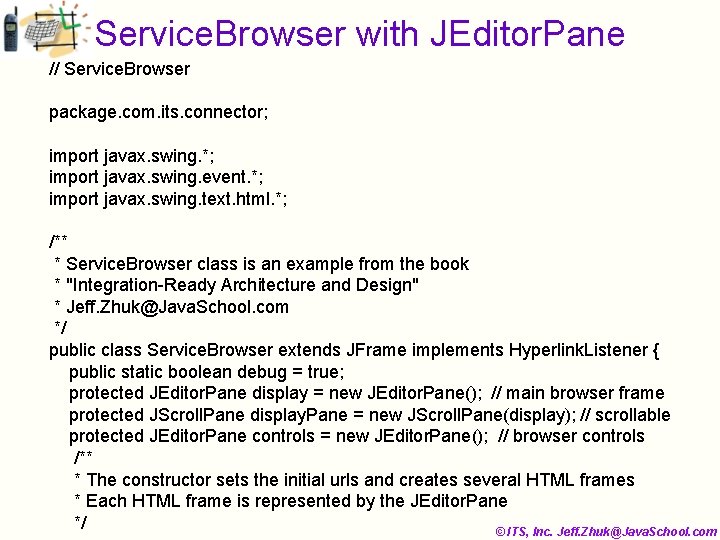
Service. Browser with JEditor. Pane // Service. Browser package. com. its. connector; import javax. swing. *; import javax. swing. event. *; import javax. swing. text. html. *; /** * Service. Browser class is an example from the book * "Integration-Ready Architecture and Design" * Jeff. Zhuk@Java. School. com */ public class Service. Browser extends JFrame implements Hyperlink. Listener { public static boolean debug = true; protected JEditor. Pane display = new JEditor. Pane(); // main browser frame protected JScroll. Pane display. Pane = new JScroll. Pane(display); // scrollable protected JEditor. Pane controls = new JEditor. Pane(); // browser controls /** * The constructor sets the initial urls and creates several HTML frames * Each HTML frame is represented by the JEditor. Pane */ © ITS, Inc. Jeff. Zhuk@Java. School. com
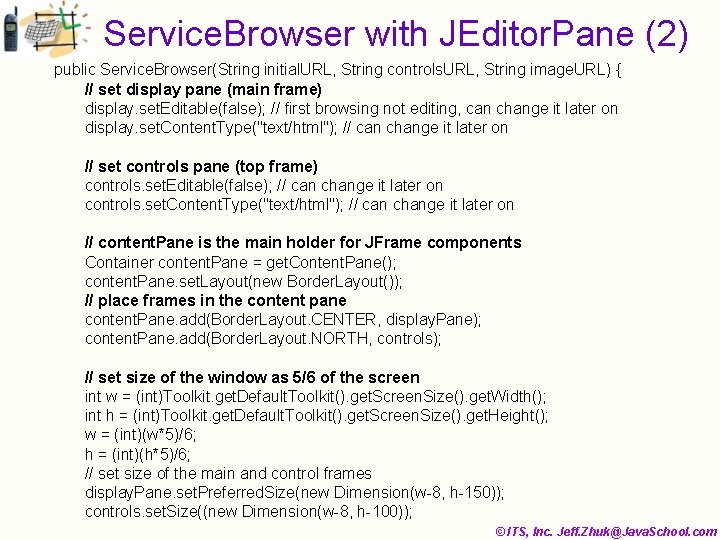
Service. Browser with JEditor. Pane (2) public Service. Browser(String initial. URL, String controls. URL, String image. URL) { // set display pane (main frame) display. set. Editable(false); // first browsing not editing, can change it later on display. set. Content. Type("text/html"); // can change it later on // set controls pane (top frame) controls. set. Editable(false); // can change it later on controls. set. Content. Type("text/html"); // can change it later on // content. Pane is the main holder for JFrame components Container content. Pane = get. Content. Pane(); content. Pane. set. Layout(new Border. Layout()); // place frames in the content pane content. Pane. add(Border. Layout. CENTER, display. Pane); content. Pane. add(Border. Layout. NORTH, controls); // set size of the window as 5/6 of the screen int w = (int)Toolkit. get. Default. Toolkit(). get. Screen. Size(). get. Width(); int h = (int)Toolkit. get. Default. Toolkit(). get. Screen. Size(). get. Height(); w = (int)(w*5)/6; h = (int)(h*5)/6; // set size of the main and control frames display. Pane. set. Preferred. Size(new Dimension(w-8, h-150)); controls. set. Size((new Dimension(w-8, h-100)); © ITS, Inc. Jeff. Zhuk@Java. School. com
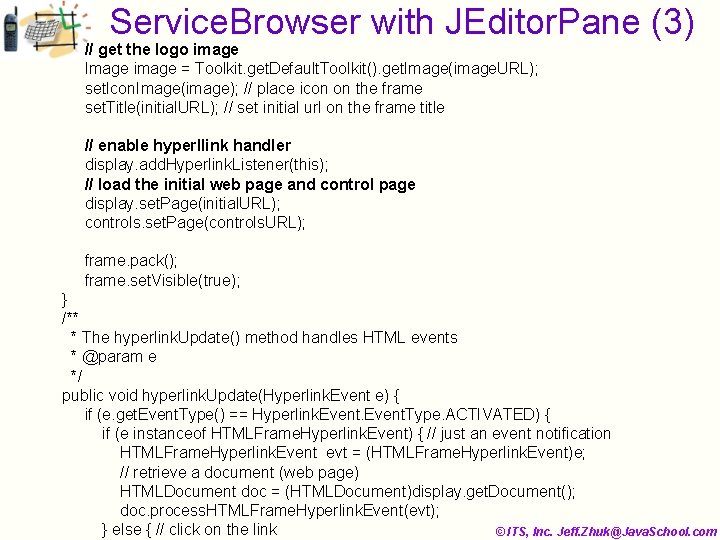
Service. Browser with JEditor. Pane (3) // get the logo image Image image = Toolkit. get. Default. Toolkit(). get. Image(image. URL); set. Icon. Image(image); // place icon on the frame set. Title(initial. URL); // set initial url on the frame title // enable hyperllink handler display. add. Hyperlink. Listener(this); // load the initial web page and control page display. set. Page(initial. URL); controls. set. Page(controls. URL); frame. pack(); frame. set. Visible(true); } /** * The hyperlink. Update() method handles HTML events * @param e */ public void hyperlink. Update(Hyperlink. Event e) { if (e. get. Event. Type() == Hyperlink. Event. Type. ACTIVATED) { if (e instanceof HTMLFrame. Hyperlink. Event) { // just an event notification HTMLFrame. Hyperlink. Event evt = (HTMLFrame. Hyperlink. Event)e; // retrieve a document (web page) HTMLDocument doc = (HTMLDocument)display. get. Document(); doc. process. HTMLFrame. Hyperlink. Event(evt); } else { // click on the link © ITS, Inc. Jeff. Zhuk@Java. School. com
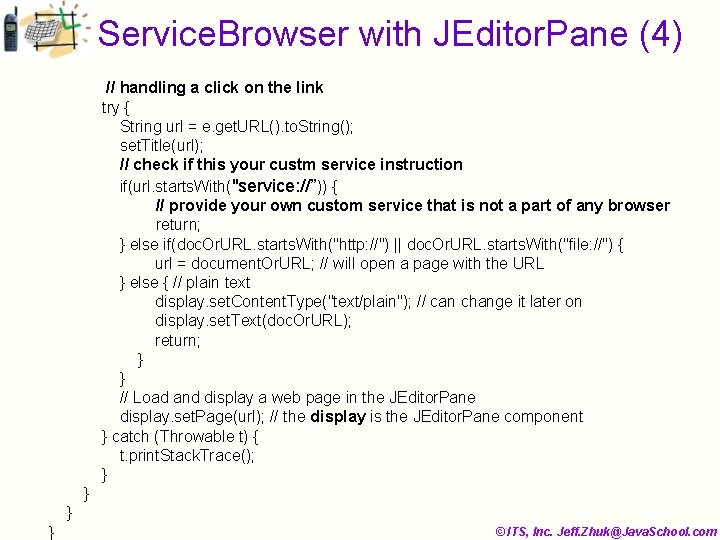
Service. Browser with JEditor. Pane (4) // handling a click on the link try { String url = e. get. URL(). to. String(); set. Title(url); // check if this your custm service instruction if(url. starts. With("service: //”)) { // provide your own custom service that is not a part of any browser return; } else if(doc. Or. URL. starts. With("http: //") || doc. Or. URL. starts. With("file: //") { url = document. Or. URL; // will open a page with the URL } else { // plain text display. set. Content. Type("text/plain"); // can change it later on display. set. Text(doc. Or. URL); return; } // Load and display a web page in the JEditor. Pane display. set. Page(url); // the display is the JEditor. Pane component } catch (Throwable t) { t. print. Stack. Trace(); } } © ITS, Inc. Jeff. Zhuk@Java. School. com }
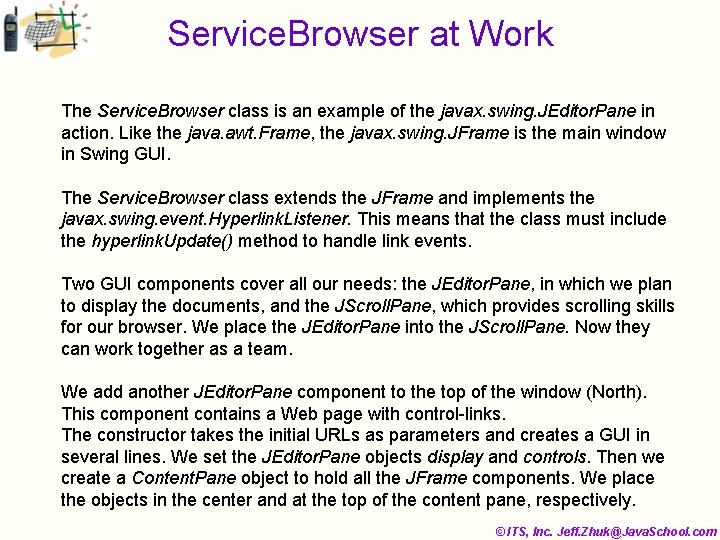
Service. Browser at Work The Service. Browser class is an example of the javax. swing. JEditor. Pane in action. Like the java. awt. Frame, the javax. swing. JFrame is the main window in Swing GUI. The Service. Browser class extends the JFrame and implements the javax. swing. event. Hyperlink. Listener. This means that the class must include the hyperlink. Update() method to handle link events. Two GUI components cover all our needs: the JEditor. Pane, in which we plan to display the documents, and the JScroll. Pane, which provides scrolling skills for our browser. We place the JEditor. Pane into the JScroll. Pane. Now they can work together as a team. We add another JEditor. Pane component to the top of the window (North). This component contains a Web page with control-links. The constructor takes the initial URLs as parameters and creates a GUI in several lines. We set the JEditor. Pane objects display and controls. Then we create a Content. Pane object to hold all the JFrame components. We place the objects in the center and at the top of the content pane, respectively. © ITS, Inc. Jeff. Zhuk@Java. School. com
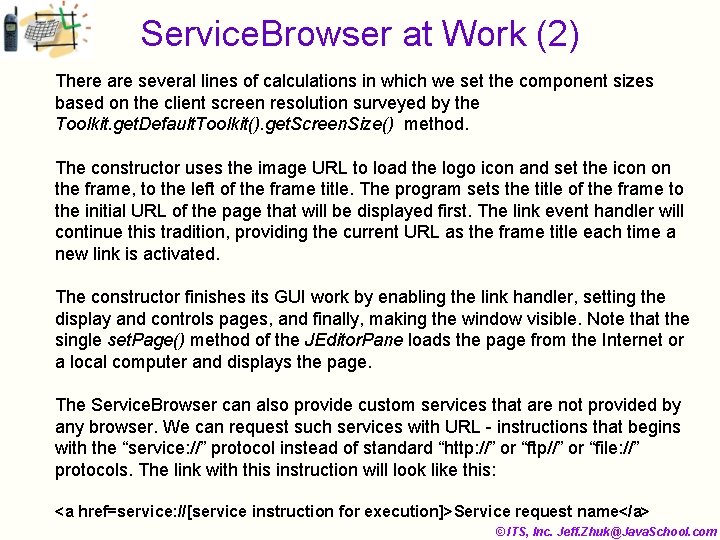
Service. Browser at Work (2) There are several lines of calculations in which we set the component sizes based on the client screen resolution surveyed by the Toolkit. get. Default. Toolkit(). get. Screen. Size() method. The constructor uses the image URL to load the logo icon and set the icon on the frame, to the left of the frame title. The program sets the title of the frame to the initial URL of the page that will be displayed first. The link event handler will continue this tradition, providing the current URL as the frame title each time a new link is activated. The constructor finishes its GUI work by enabling the link handler, setting the display and controls pages, and finally, making the window visible. Note that the single set. Page() method of the JEditor. Pane loads the page from the Internet or a local computer and displays the page. The Service. Browser can also provide custom services that are not provided by any browser. We can request such services with URL - instructions that begins with the “service: //” protocol instead of standard “http: //” or “ftp//” or “file: //” protocols. The link with this instruction will look like this: <a href=service: //[service instruction for execution]>Service request name</a> © ITS, Inc. Jeff. Zhuk@Java. School. com
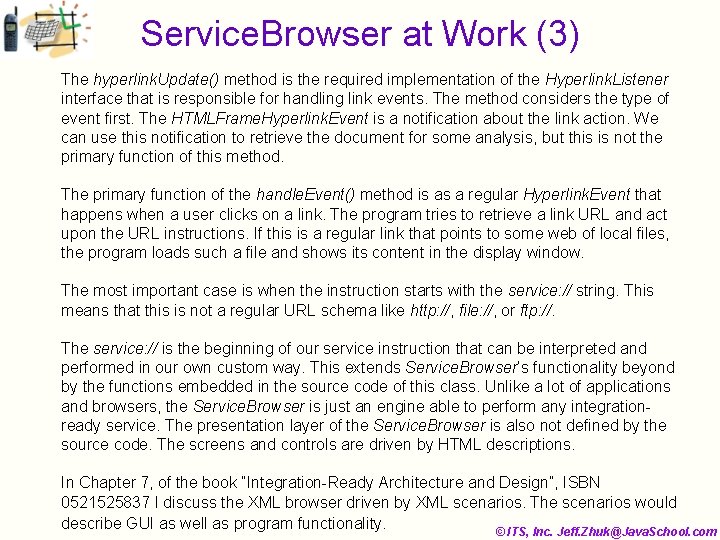
Service. Browser at Work (3) The hyperlink. Update() method is the required implementation of the Hyperlink. Listener interface that is responsible for handling link events. The method considers the type of event first. The HTMLFrame. Hyperlink. Event is a notification about the link action. We can use this notification to retrieve the document for some analysis, but this is not the primary function of this method. The primary function of the handle. Event() method is as a regular Hyperlink. Event that happens when a user clicks on a link. The program tries to retrieve a link URL and act upon the URL instructions. If this is a regular link that points to some web of local files, the program loads such a file and shows its content in the display window. The most important case is when the instruction starts with the service: // string. This means that this is not a regular URL schema like http: //, file: //, or ftp: //. The service: // is the beginning of our service instruction that can be interpreted and performed in our own custom way. This extends Service. Browser’s functionality beyond by the functions embedded in the source code of this class. Unlike a lot of applications and browsers, the Service. Browser is just an engine able to perform any integrationready service. The presentation layer of the Service. Browser is also not defined by the source code. The screens and controls are driven by HTML descriptions. In Chapter 7, of the book “Integration-Ready Architecture and Design”, ISBN 0521525837 I discuss the XML browser driven by XML scenarios. The scenarios would describe GUI as well as program functionality. © ITS, Inc. Jeff. Zhuk@Java. School. com
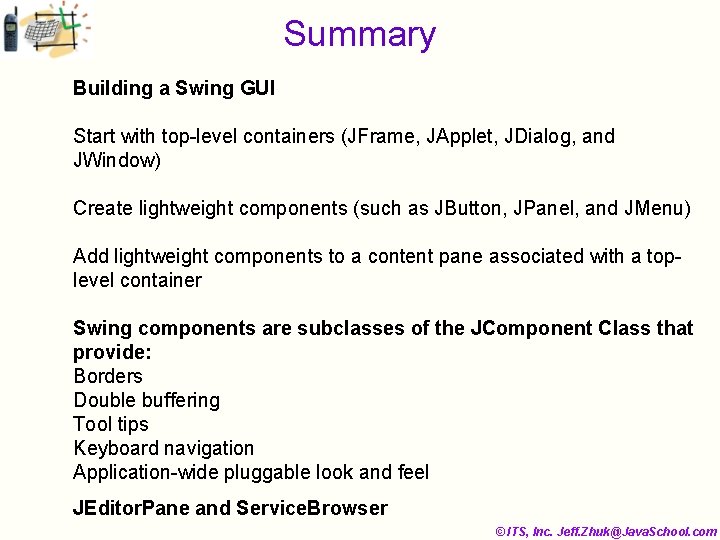
Summary Building a Swing GUI Start with top-level containers (JFrame, JApplet, JDialog, and JWindow) Create lightweight components (such as JButton, JPanel, and JMenu) Add lightweight components to a content pane associated with a toplevel container Swing components are subclasses of the JComponent Class that provide: Borders Double buffering Tool tips Keyboard navigation Application-wide pluggable look and feel JEditor. Pane and Service. Browser © ITS, Inc. Jeff. Zhuk@Java. School. com