Java 1 5 New Features Versions of Java
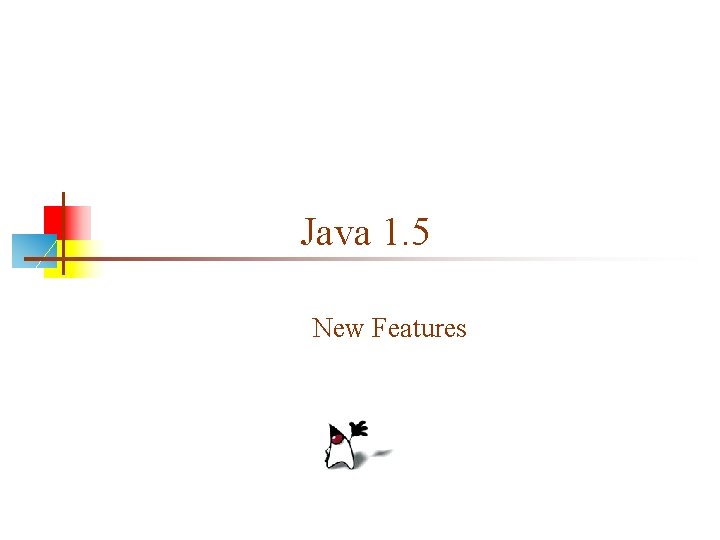
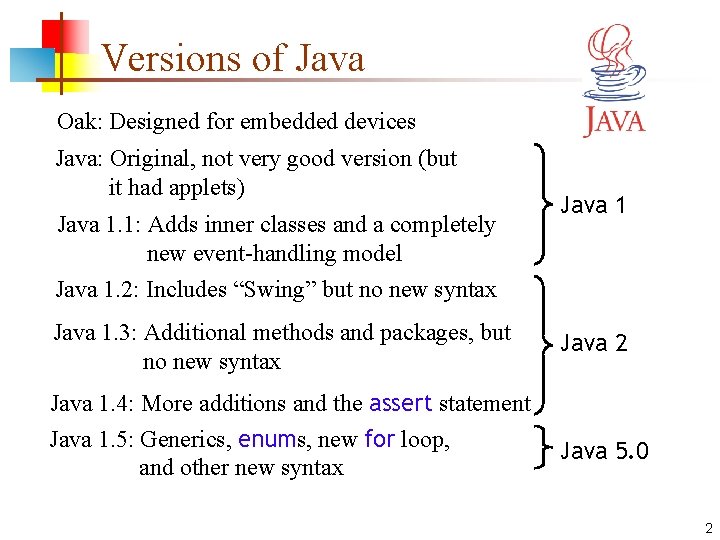
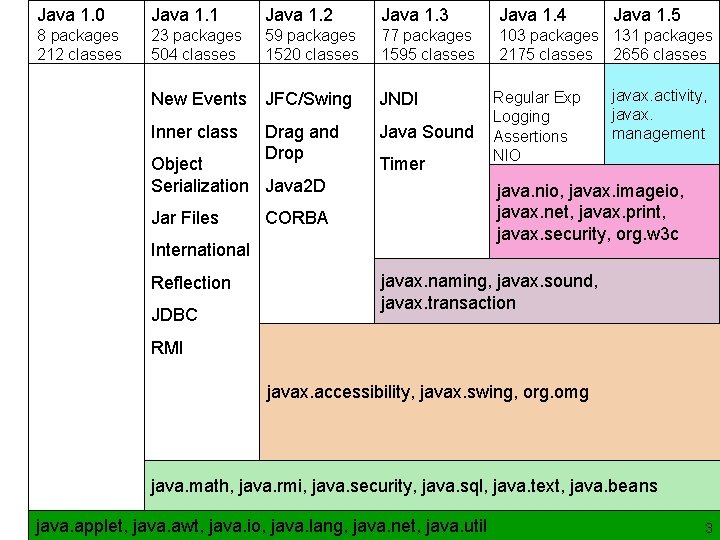
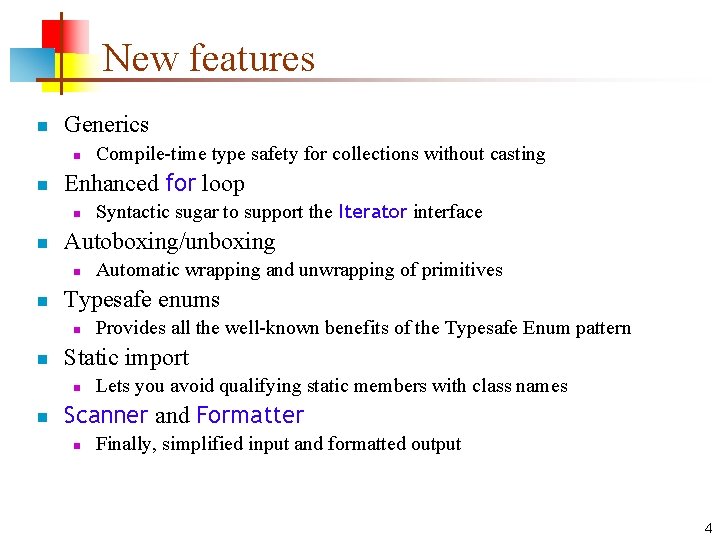
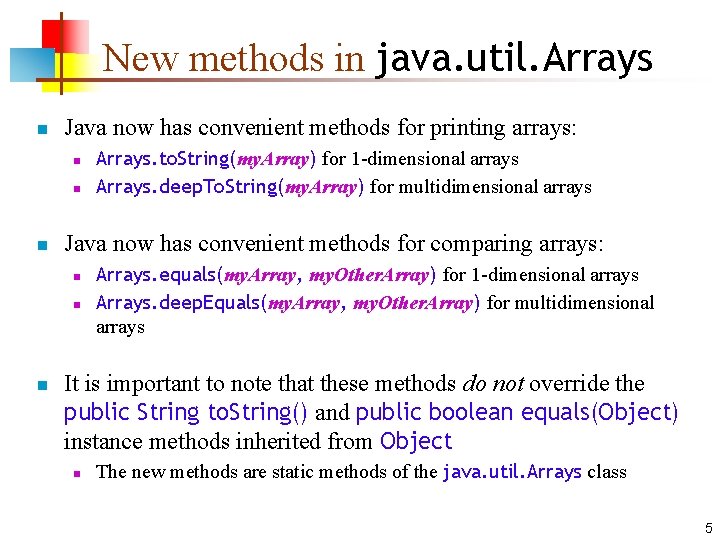
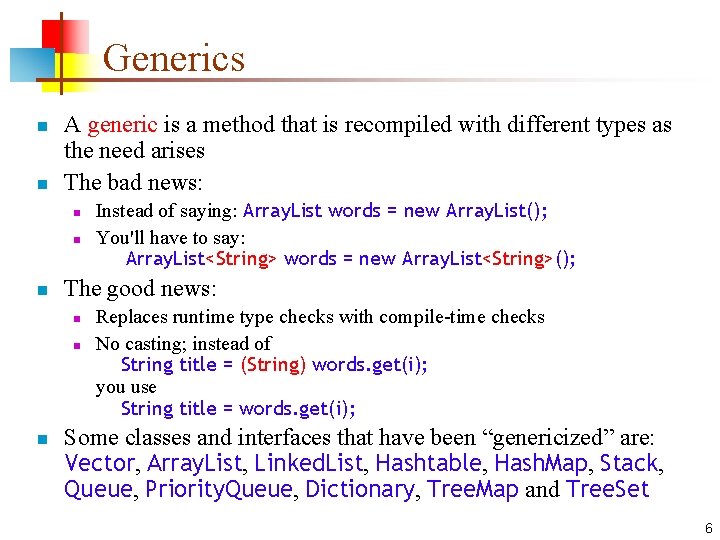
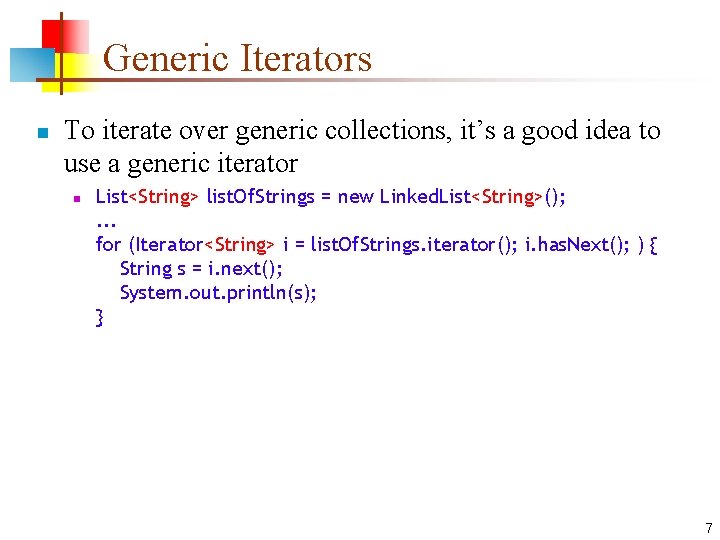
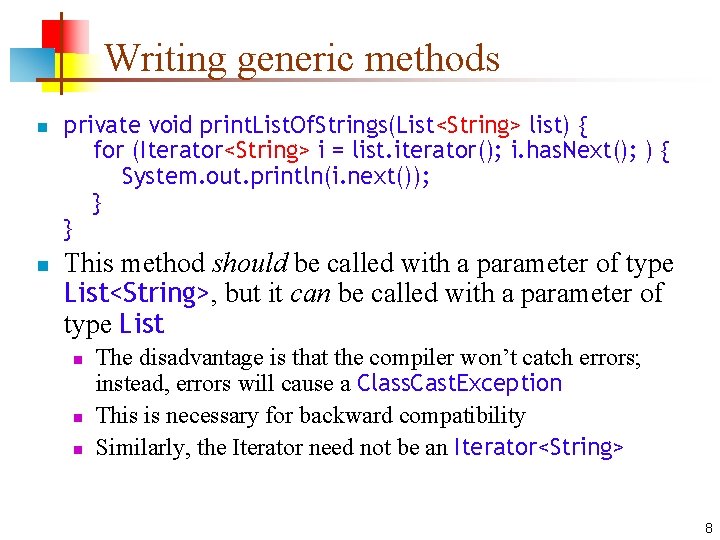
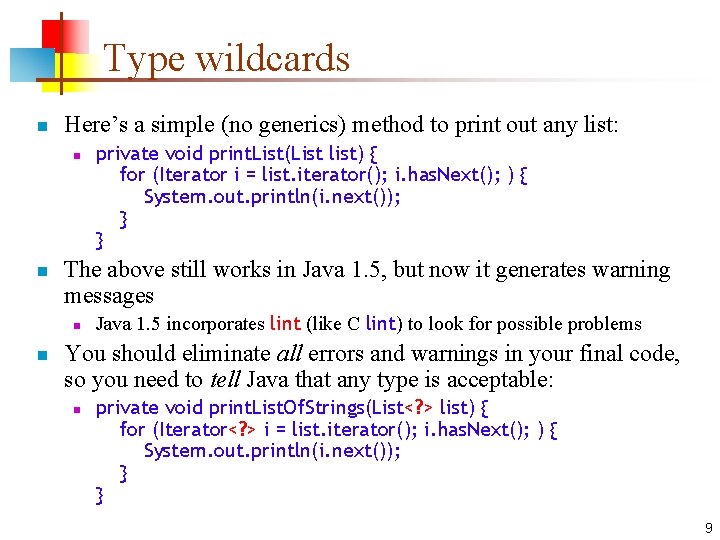
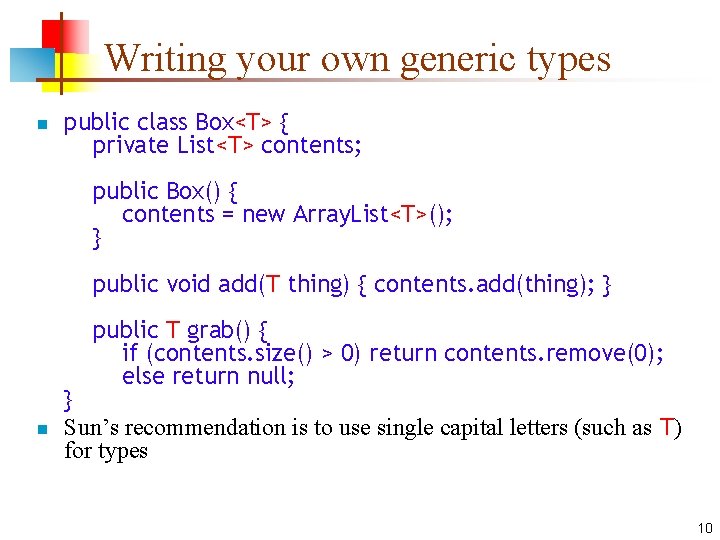
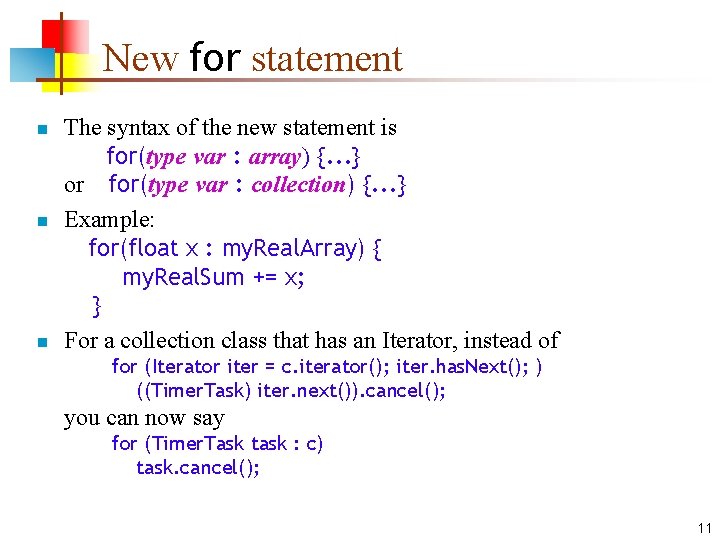
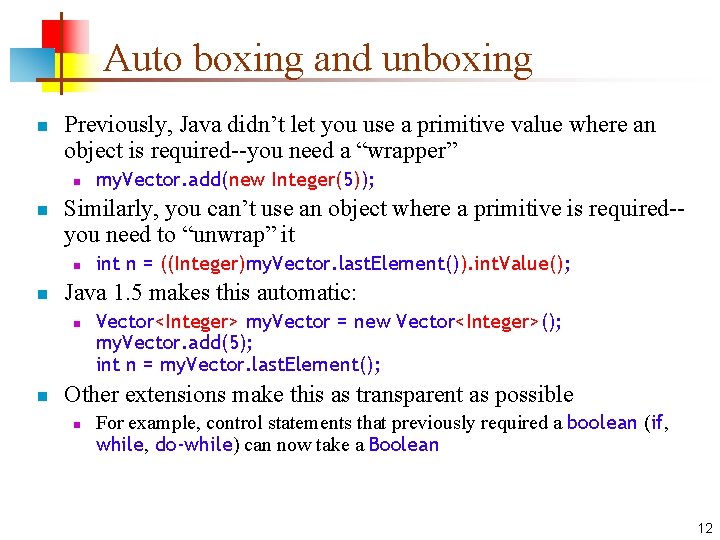
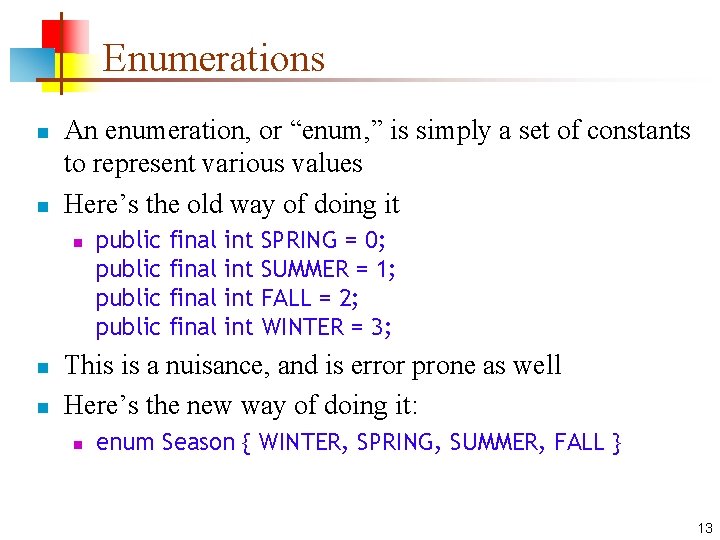
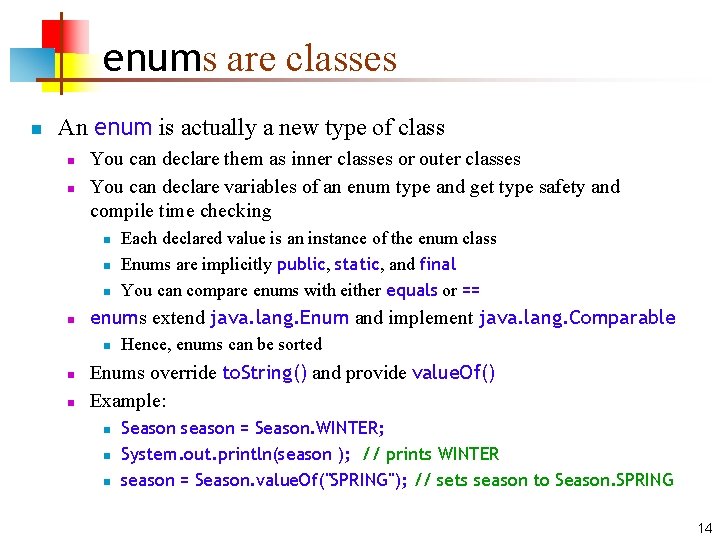
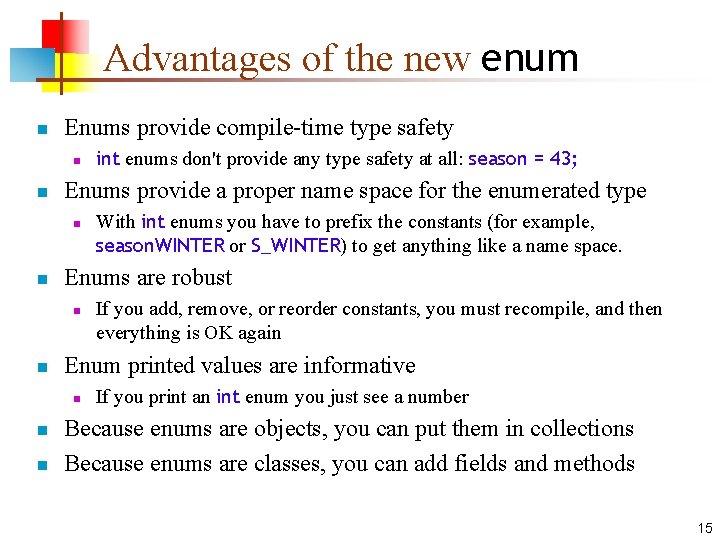
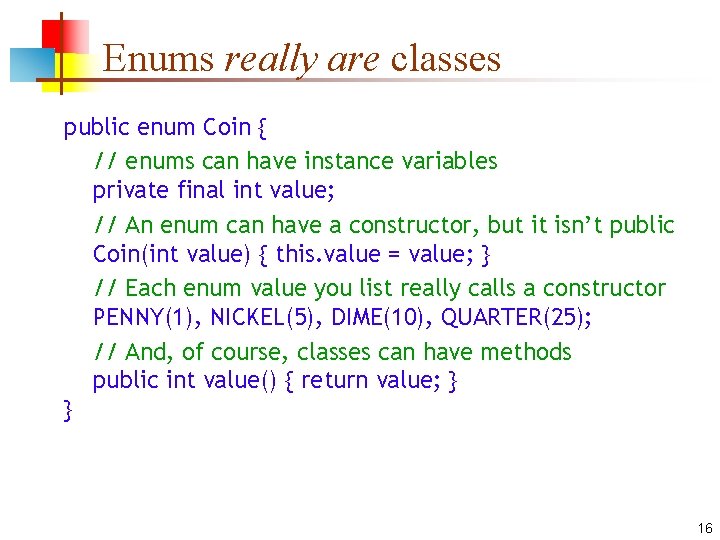
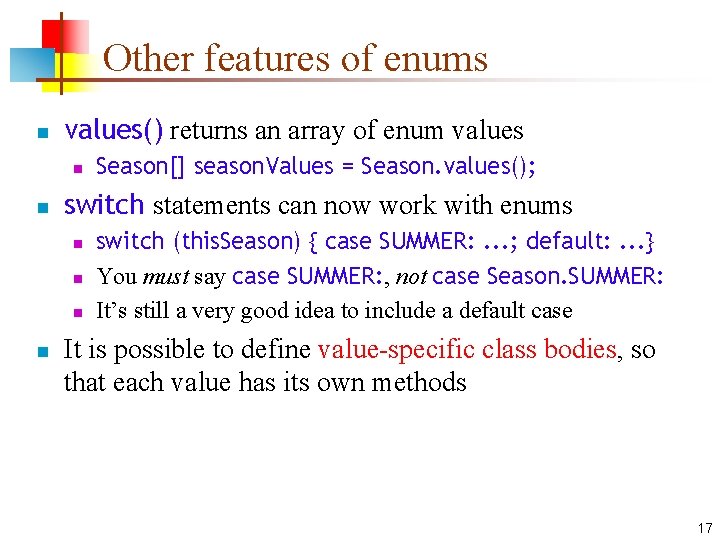
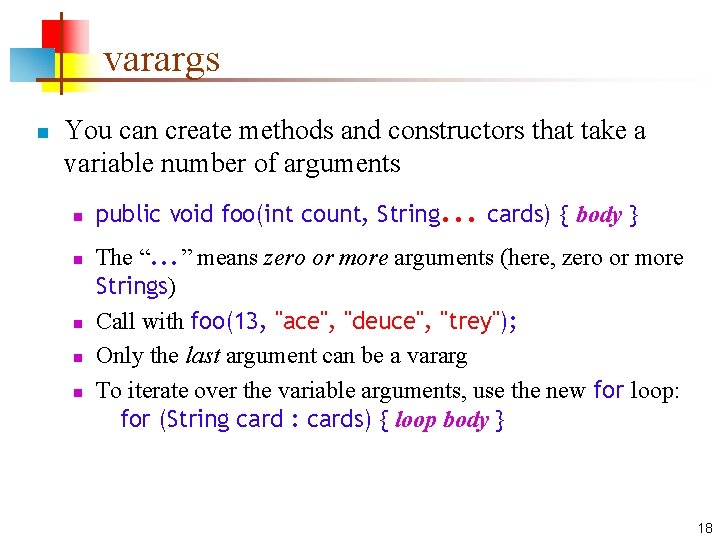
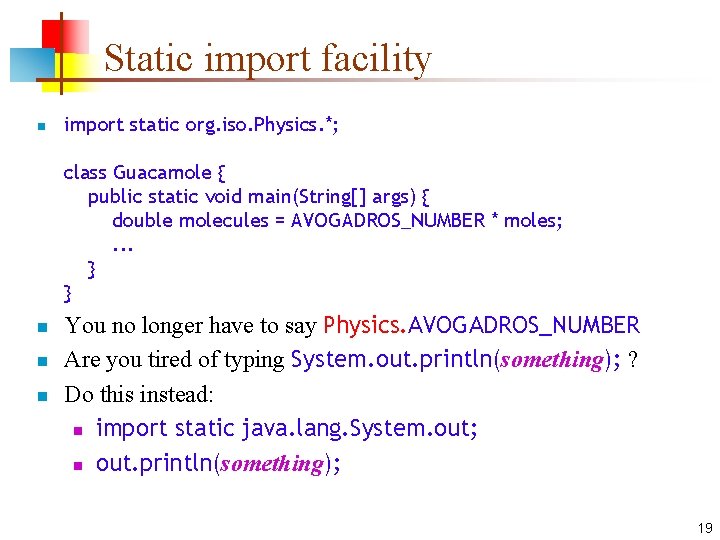
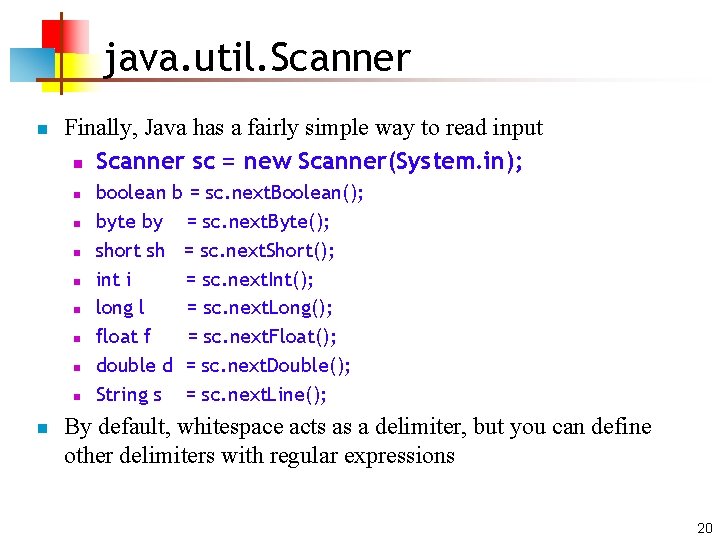
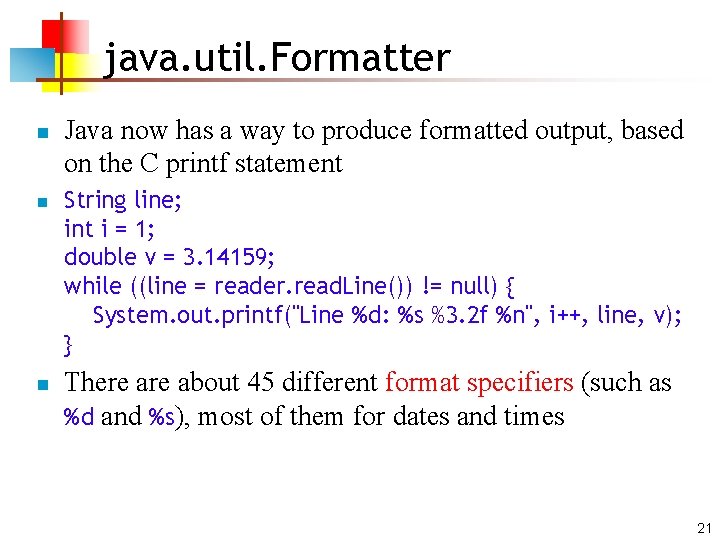
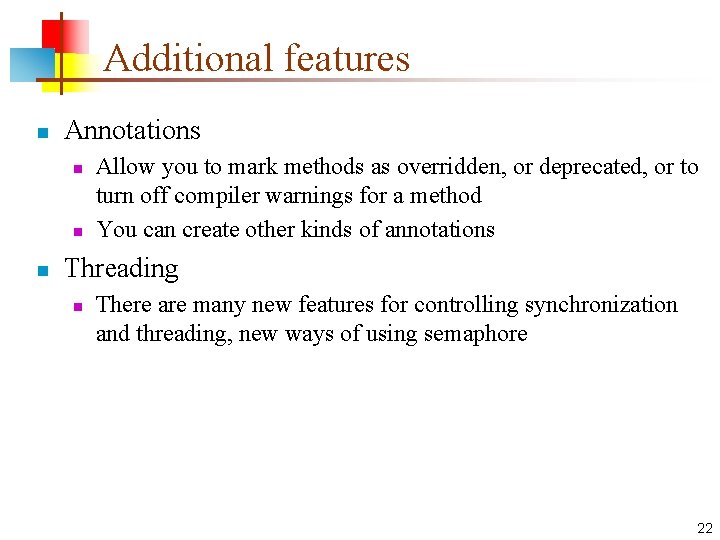
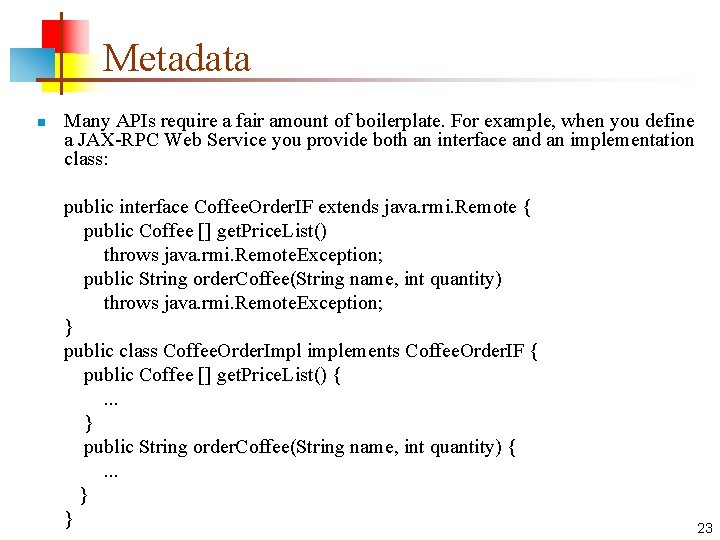
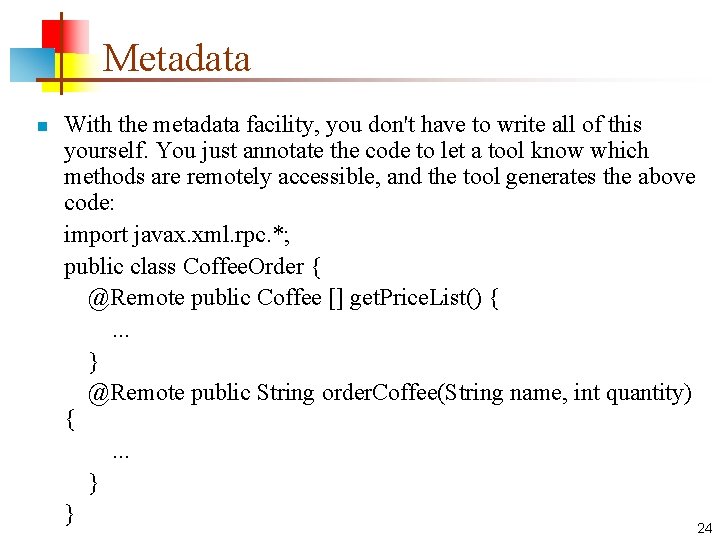
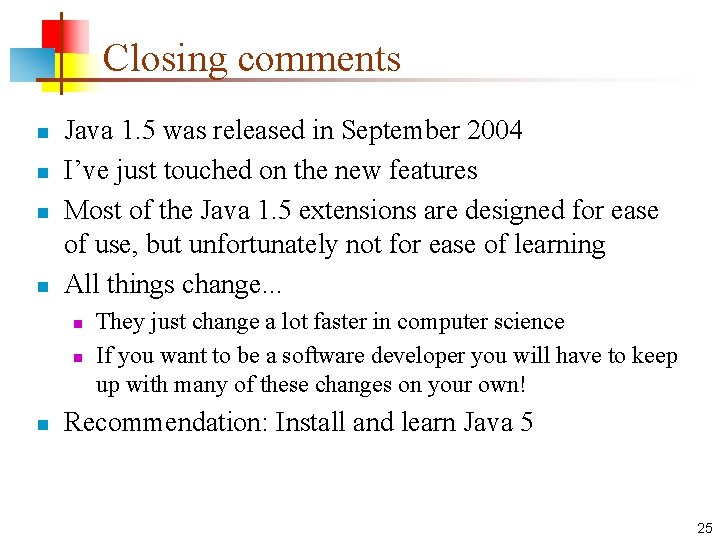
- Slides: 25
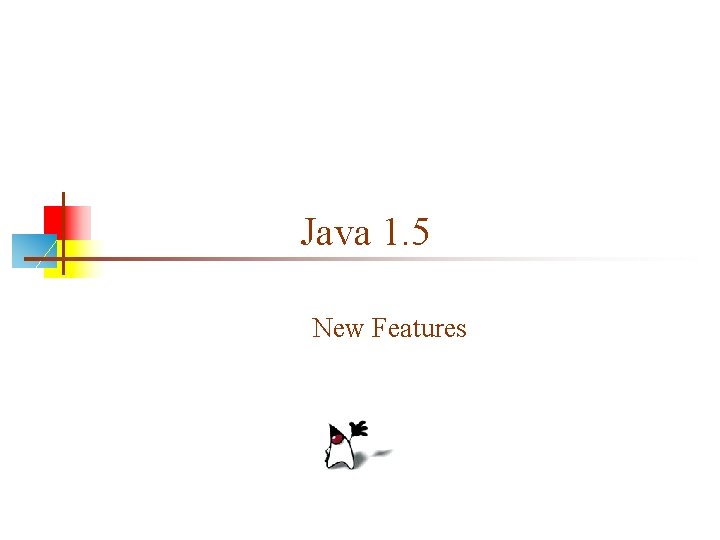
Java 1. 5 New Features
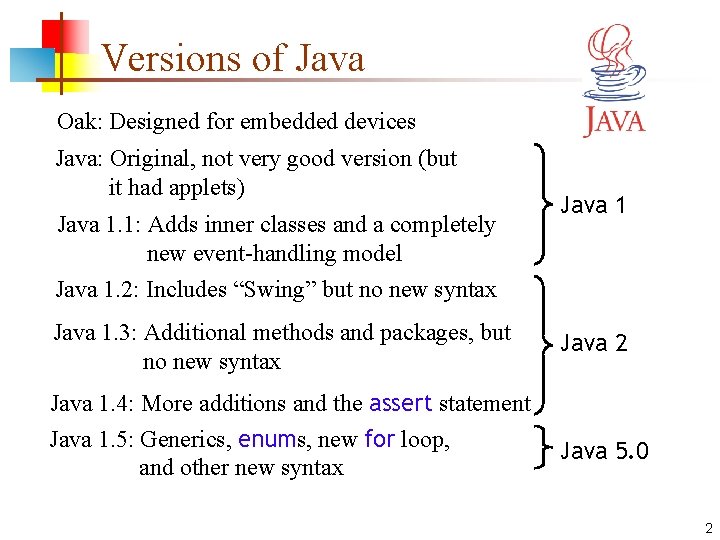
Versions of Java Oak: Designed for embedded devices Java: Original, not very good version (but it had applets) Java 1. 1: Adds inner classes and a completely new event-handling model Java 1. 2: Includes “Swing” but no new syntax Java 1. 3: Additional methods and packages, but no new syntax Java 1. 4: More additions and the assert statement Java 1. 5: Generics, enums, new for loop, and other new syntax Java 1 Java 2 Java 5. 0 2
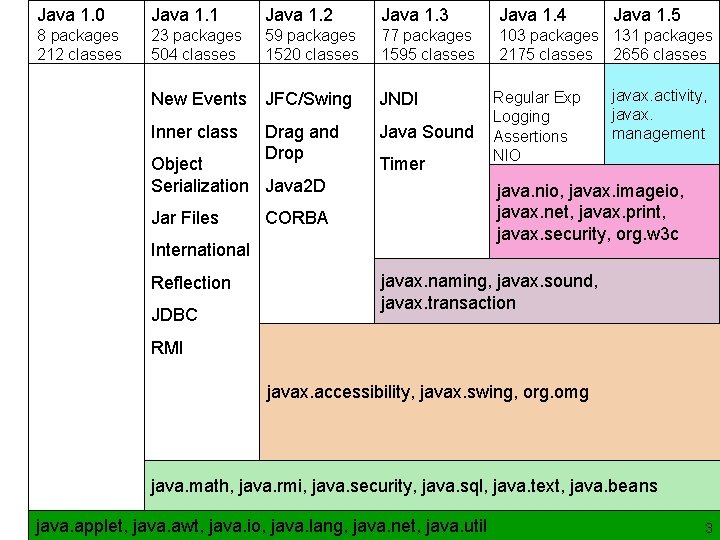
Java 1. 0 Java 1. 1 Java 1. 2 Java 1. 3 Java 1. 4 8 packages 212 classes 23 packages 504 classes 59 packages 1520 classes 77 packages 1595 classes 103 packages 131 packages 2175 classes 2656 classes New Events JFC/Swing JNDI Inner class Drag and Drop Java Sound Object Serialization Java 2 D Jar Files Timer JDBC javax. activity, javax. management java. nio, javax. imageio, javax. net, javax. print, javax. security, org. w 3 c CORBA International Reflection Regular Exp Logging Assertions NIO Java 1. 5 javax. naming, javax. sound, javax. transaction RMI javax. accessibility, javax. swing, org. omg java. math, java. rmi, java. security, java. sql, java. text, java. beans java. applet, java. awt, java. io, java. lang, java. net, java. util 3
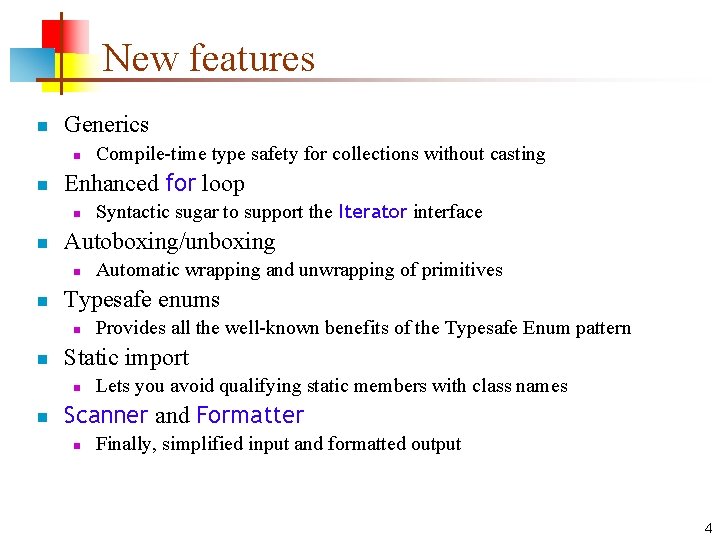
New features n Generics n n Enhanced for loop n n Provides all the well-known benefits of the Typesafe Enum pattern Static import n n Automatic wrapping and unwrapping of primitives Typesafe enums n n Syntactic sugar to support the Iterator interface Autoboxing/unboxing n n Compile-time type safety for collections without casting Lets you avoid qualifying static members with class names Scanner and Formatter n Finally, simplified input and formatted output 4
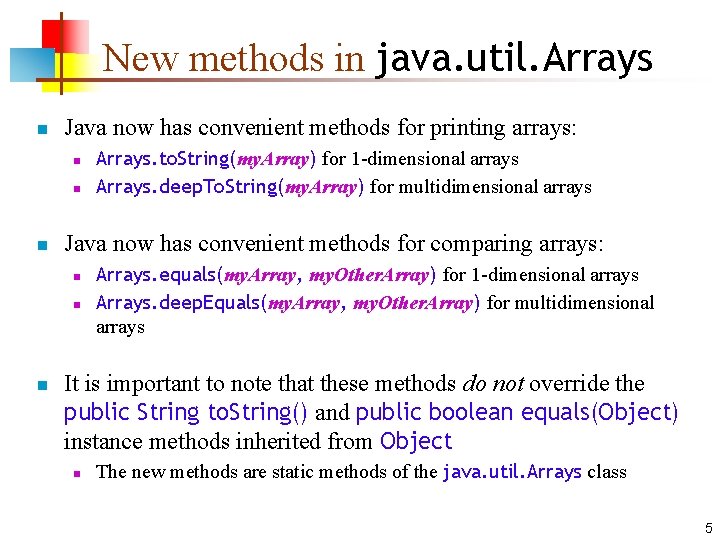
New methods in java. util. Arrays n Java now has convenient methods for printing arrays: n n n Java now has convenient methods for comparing arrays: n n n Arrays. to. String(my. Array) for 1 -dimensional arrays Arrays. deep. To. String(my. Array) for multidimensional arrays Arrays. equals(my. Array, my. Other. Array) for 1 -dimensional arrays Arrays. deep. Equals(my. Array, my. Other. Array) for multidimensional arrays It is important to note that these methods do not override the public String to. String() and public boolean equals(Object) instance methods inherited from Object n The new methods are static methods of the java. util. Arrays class 5
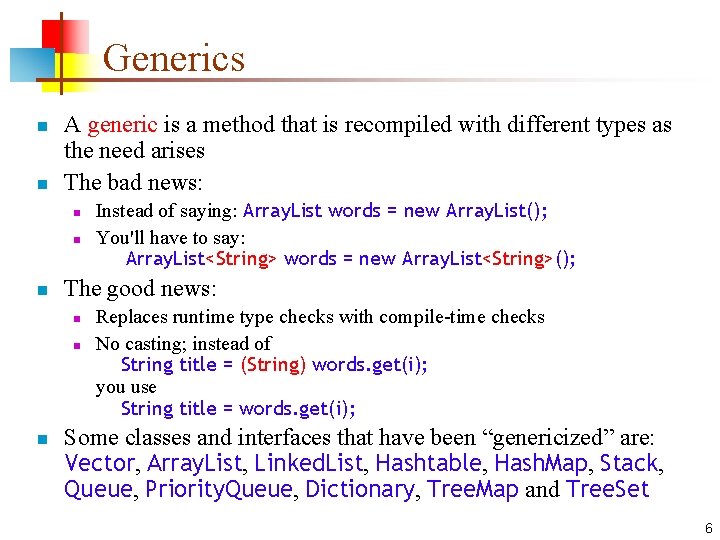
Generics n n A generic is a method that is recompiled with different types as the need arises The bad news: n n n The good news: n n n Instead of saying: Array. List words = new Array. List(); You'll have to say: Array. List<String> words = new Array. List<String>(); Replaces runtime type checks with compile-time checks No casting; instead of String title = (String) words. get(i); you use String title = words. get(i); Some classes and interfaces that have been “genericized” are: Vector, Array. List, Linked. List, Hashtable, Hash. Map, Stack, Queue, Priority. Queue, Dictionary, Tree. Map and Tree. Set 6
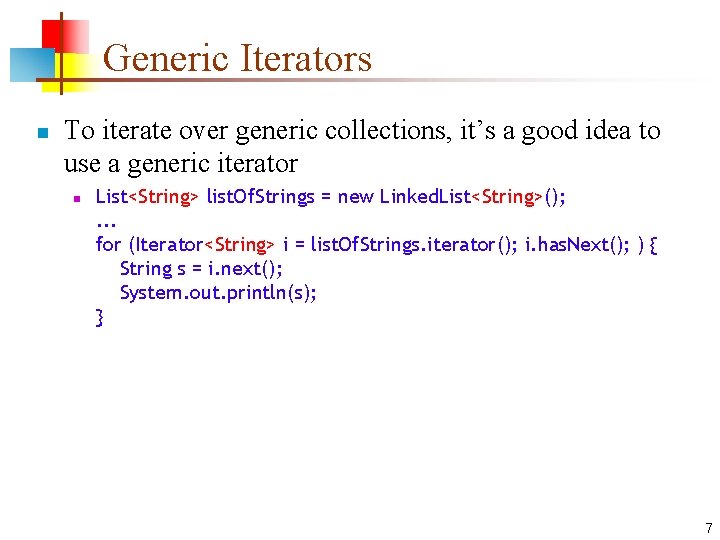
Generic Iterators n To iterate over generic collections, it’s a good idea to use a generic iterator n List<String> list. Of. Strings = new Linked. List<String>(); . . . for (Iterator<String> i = list. Of. Strings. iterator(); i. has. Next(); ) { String s = i. next(); System. out. println(s); } 7
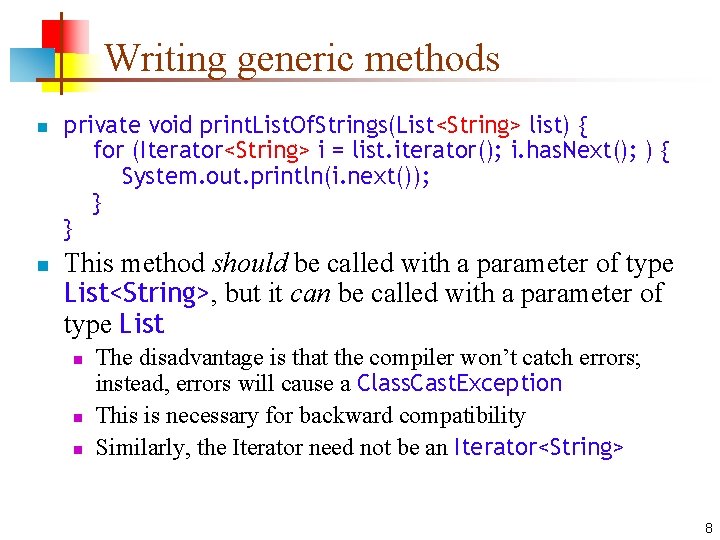
Writing generic methods n n private void print. List. Of. Strings(List<String> list) { for (Iterator<String> i = list. iterator(); i. has. Next(); ) { System. out. println(i. next()); } } This method should be called with a parameter of type List<String>, but it can be called with a parameter of type List n n n The disadvantage is that the compiler won’t catch errors; instead, errors will cause a Class. Cast. Exception This is necessary for backward compatibility Similarly, the Iterator need not be an Iterator<String> 8
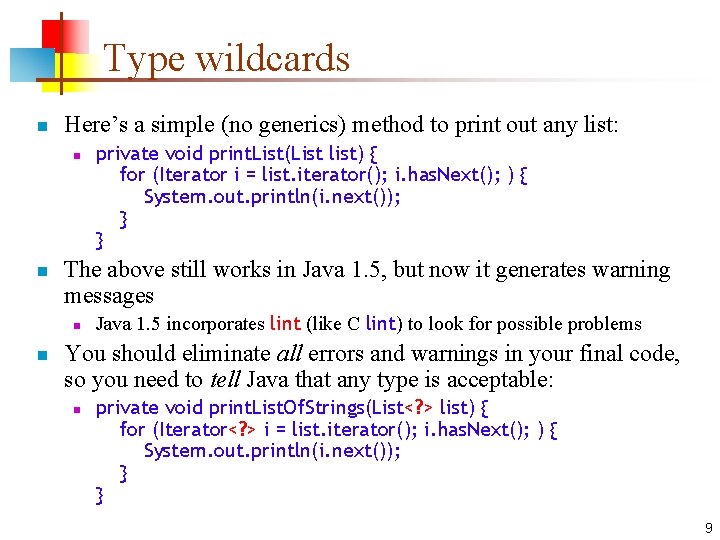
Type wildcards n Here’s a simple (no generics) method to print out any list: n n The above still works in Java 1. 5, but now it generates warning messages n n private void print. List(List list) { for (Iterator i = list. iterator(); i. has. Next(); ) { System. out. println(i. next()); } } Java 1. 5 incorporates lint (like C lint) to look for possible problems You should eliminate all errors and warnings in your final code, so you need to tell Java that any type is acceptable: n private void print. List. Of. Strings(List<? > list) { for (Iterator<? > i = list. iterator(); i. has. Next(); ) { System. out. println(i. next()); } } 9
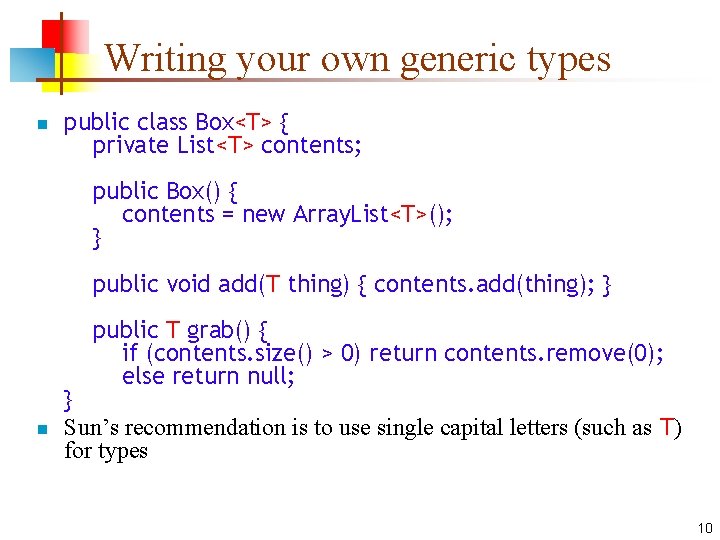
Writing your own generic types n public class Box<T> { private List<T> contents; public Box() { contents = new Array. List<T>(); } public void add(T thing) { contents. add(thing); } public T grab() { if (contents. size() > 0) return contents. remove(0); else return null; n } Sun’s recommendation is to use single capital letters (such as T) for types 10
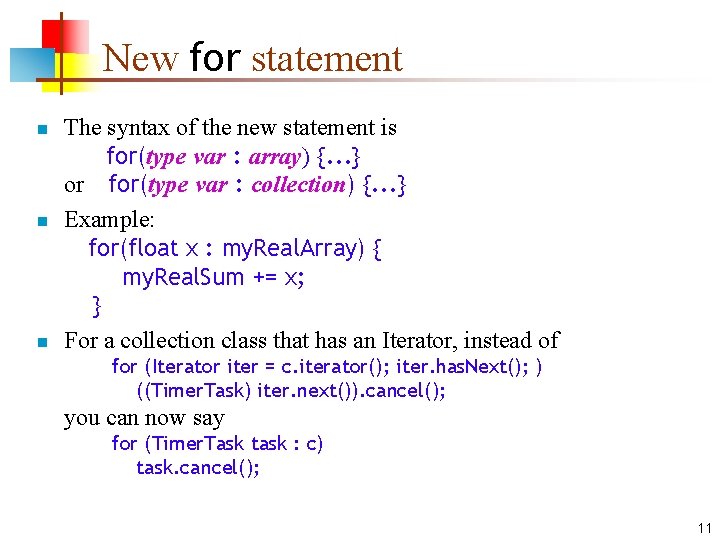
New for statement n n n The syntax of the new statement is for(type var : array) {. . . } or for(type var : collection) {. . . } Example: for(float x : my. Real. Array) { my. Real. Sum += x; } For a collection class that has an Iterator, instead of for (Iterator iter = c. iterator(); iter. has. Next(); ) ((Timer. Task) iter. next()). cancel(); you can now say for (Timer. Task task : c) task. cancel(); 11
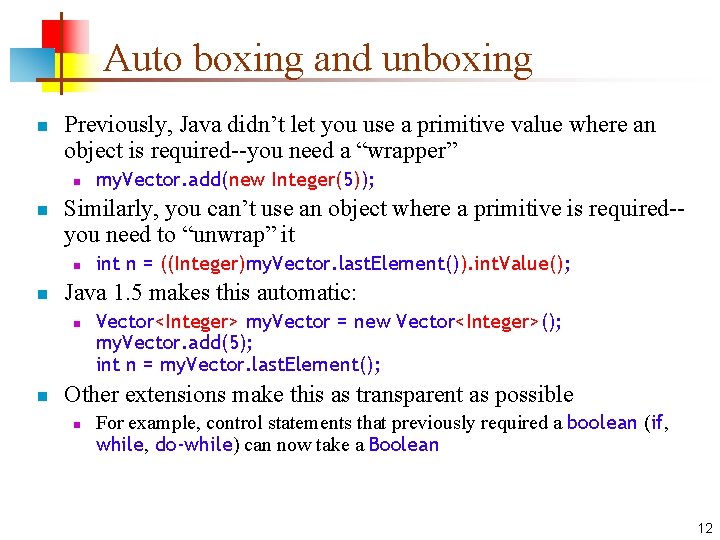
Auto boxing and unboxing n Previously, Java didn’t let you use a primitive value where an object is required--you need a “wrapper” n n Similarly, you can’t use an object where a primitive is required-you need to “unwrap” it n n int n = ((Integer)my. Vector. last. Element()). int. Value(); Java 1. 5 makes this automatic: n n my. Vector. add(new Integer(5)); Vector<Integer> my. Vector = new Vector<Integer>(); my. Vector. add(5); int n = my. Vector. last. Element(); Other extensions make this as transparent as possible n For example, control statements that previously required a boolean (if, while, do-while) can now take a Boolean 12
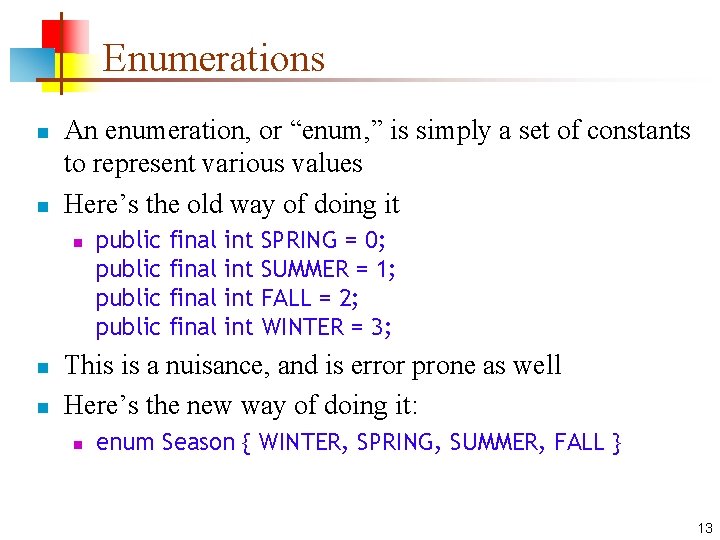
Enumerations n n An enumeration, or “enum, ” is simply a set of constants to represent various values Here’s the old way of doing it n n n public final int int SPRING = 0; SUMMER = 1; FALL = 2; WINTER = 3; This is a nuisance, and is error prone as well Here’s the new way of doing it: n enum Season { WINTER, SPRING, SUMMER, FALL } 13
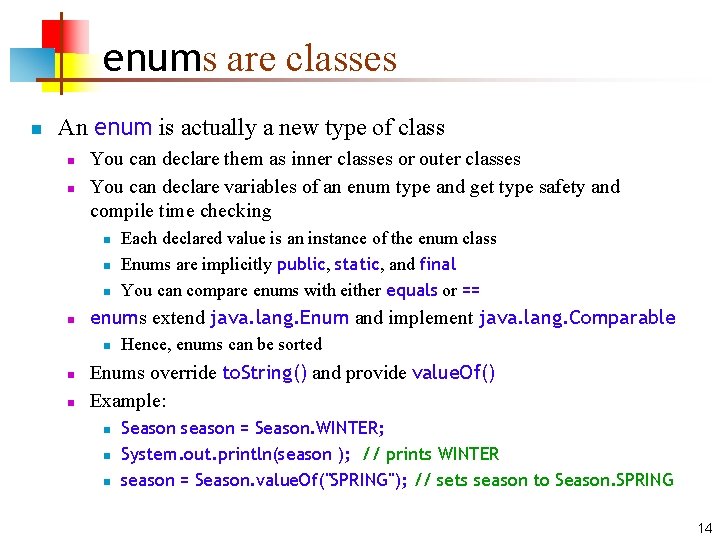
enums are classes n An enum is actually a new type of class n n You can declare them as inner classes or outer classes You can declare variables of an enum type and get type safety and compile time checking n n enums extend java. lang. Enum and implement java. lang. Comparable n n n Each declared value is an instance of the enum class Enums are implicitly public, static, and final You can compare enums with either equals or == Hence, enums can be sorted Enums override to. String() and provide value. Of() Example: n n n Season season = Season. WINTER; System. out. println(season ); // prints WINTER season = Season. value. Of("SPRING"); // sets season to Season. SPRING 14
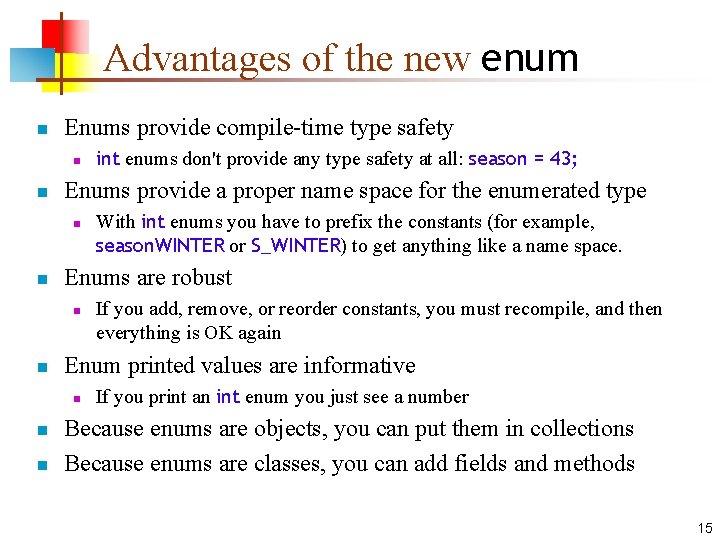
Advantages of the new enum n Enums provide compile-time type safety n n Enums provide a proper name space for the enumerated type n n n If you add, remove, or reorder constants, you must recompile, and then everything is OK again Enum printed values are informative n n With int enums you have to prefix the constants (for example, season. WINTER or S_WINTER) to get anything like a name space. Enums are robust n n int enums don't provide any type safety at all: season = 43; If you print an int enum you just see a number Because enums are objects, you can put them in collections Because enums are classes, you can add fields and methods 15
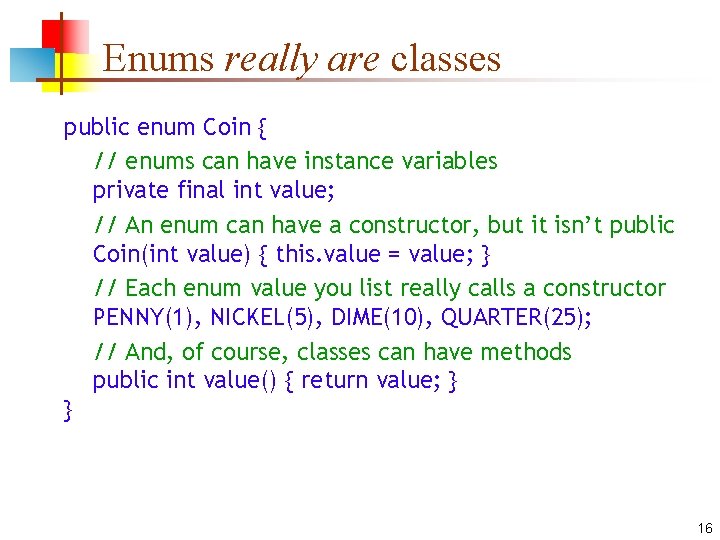
Enums really are classes public enum Coin { // enums can have instance variables private final int value; // An enum can have a constructor, but it isn’t public Coin(int value) { this. value = value; } // Each enum value you list really calls a constructor PENNY(1), NICKEL(5), DIME(10), QUARTER(25); // And, of course, classes can have methods public int value() { return value; } } 16
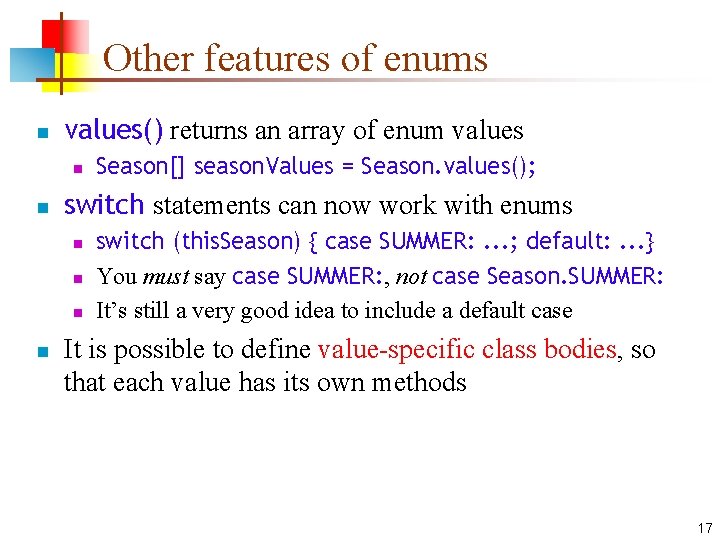
Other features of enums n values() returns an array of enum values n n switch statements can now work with enums n n Season[] season. Values = Season. values(); switch (this. Season) { case SUMMER: . . . ; default: . . . } You must say case SUMMER: , not case Season. SUMMER: It’s still a very good idea to include a default case It is possible to define value-specific class bodies, so that each value has its own methods 17
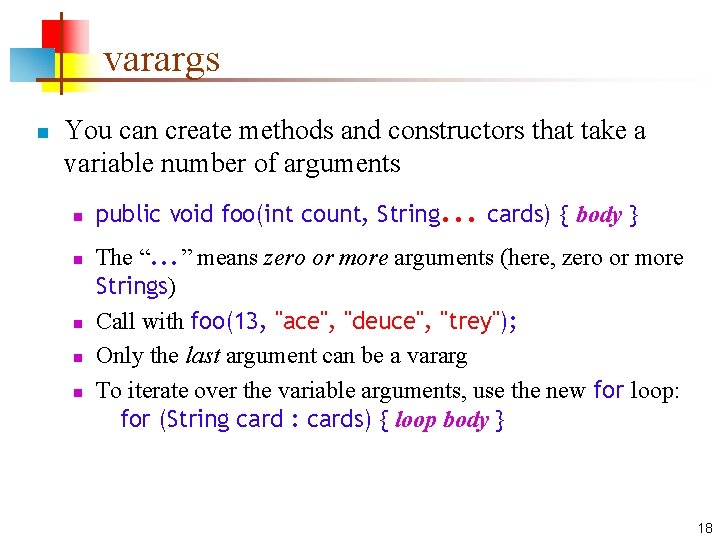
varargs n You can create methods and constructors that take a variable number of arguments n n n public void foo(int count, String. . . cards) { body } The “. . . ” means zero or more arguments (here, zero or more Strings) Call with foo(13, "ace", "deuce", "trey"); Only the last argument can be a vararg To iterate over the variable arguments, use the new for loop: for (String card : cards) { loop body } 18
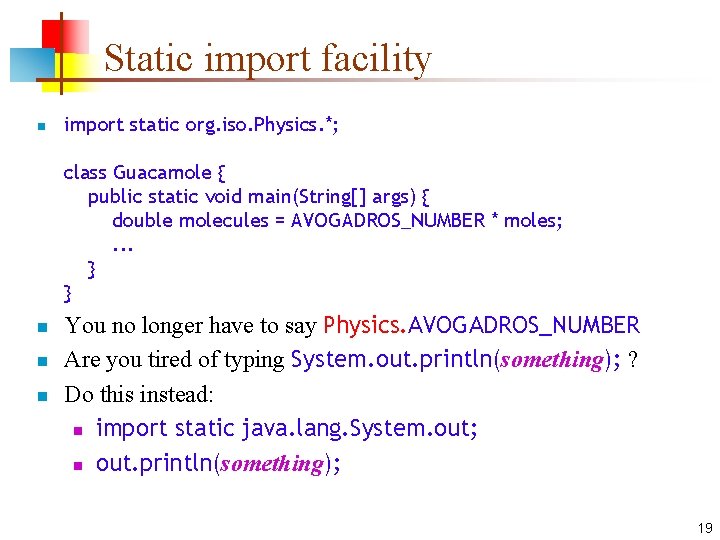
Static import facility n import static org. iso. Physics. *; class Guacamole { public static void main(String[] args) { double molecules = AVOGADROS_NUMBER * moles; . . . } } n n n You no longer have to say Physics. AVOGADROS_NUMBER Are you tired of typing System. out. println(something); ? Do this instead: n import static java. lang. System. out; n out. println(something); 19
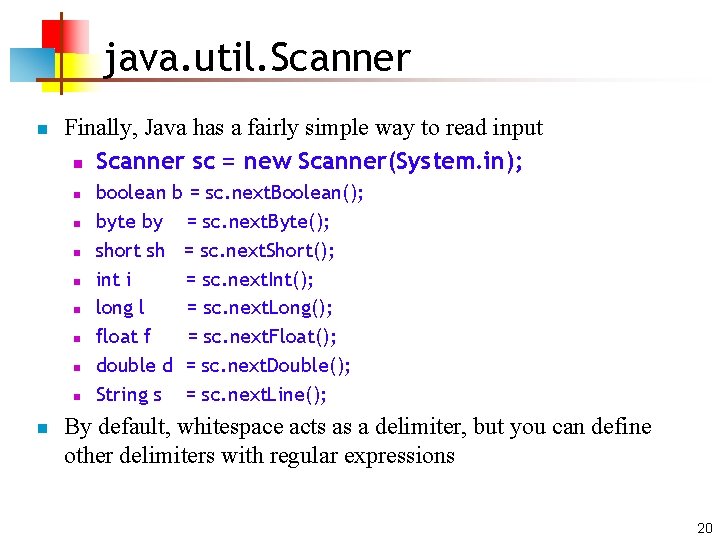
java. util. Scanner n Finally, Java has a fairly simple way to read input n Scanner sc = new Scanner(System. in); n n n n n boolean b = sc. next. Boolean(); byte by = sc. next. Byte(); short sh = sc. next. Short(); int i = sc. next. Int(); long l = sc. next. Long(); float f = sc. next. Float(); double d = sc. next. Double(); String s = sc. next. Line(); By default, whitespace acts as a delimiter, but you can define other delimiters with regular expressions 20
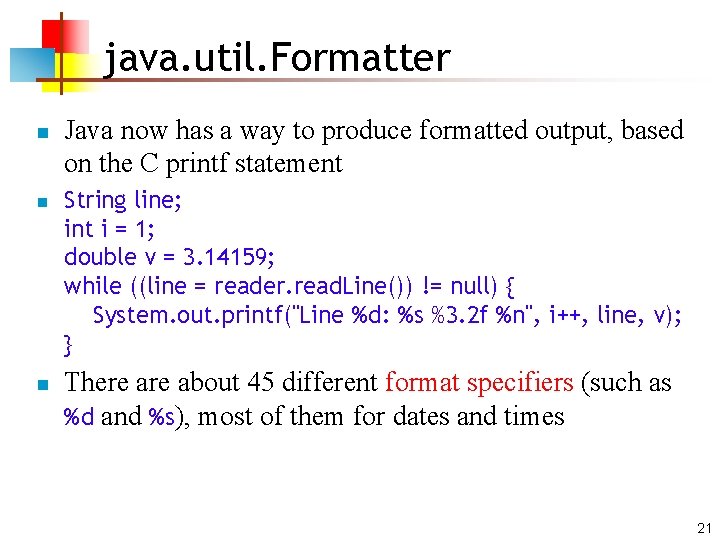
java. util. Formatter n n n Java now has a way to produce formatted output, based on the C printf statement String line; int i = 1; double v = 3. 14159; while ((line = reader. read. Line()) != null) { System. out. printf("Line %d: %s %3. 2 f %n", i++, line, v); } There about 45 different format specifiers (such as %d and %s), most of them for dates and times 21
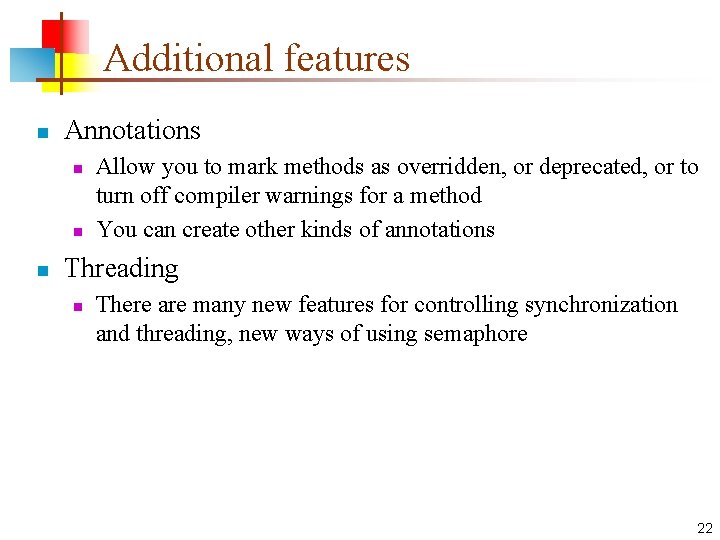
Additional features n Annotations n n n Allow you to mark methods as overridden, or deprecated, or to turn off compiler warnings for a method You can create other kinds of annotations Threading n There are many new features for controlling synchronization and threading, new ways of using semaphore 22
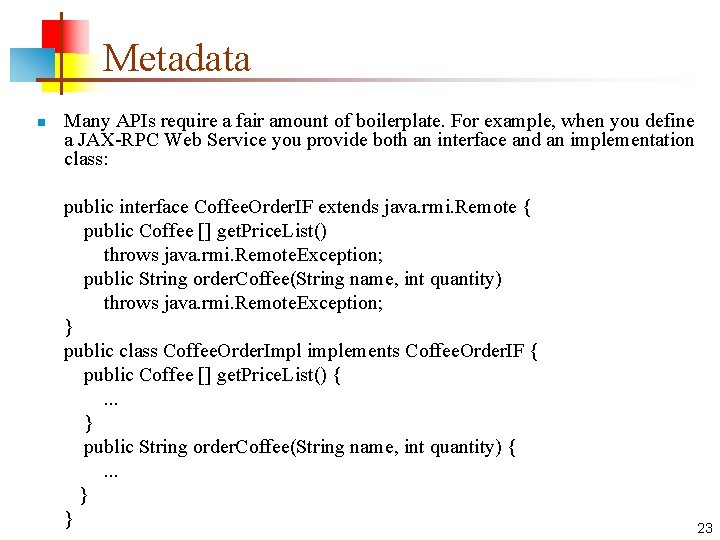
Metadata n Many APIs require a fair amount of boilerplate. For example, when you define a JAX-RPC Web Service you provide both an interface and an implementation class: public interface Coffee. Order. IF extends java. rmi. Remote { public Coffee [] get. Price. List() throws java. rmi. Remote. Exception; public String order. Coffee(String name, int quantity) throws java. rmi. Remote. Exception; } public class Coffee. Order. Impl implements Coffee. Order. IF { public Coffee [] get. Price. List() {. . . } public String order. Coffee(String name, int quantity) {. . . } } 23
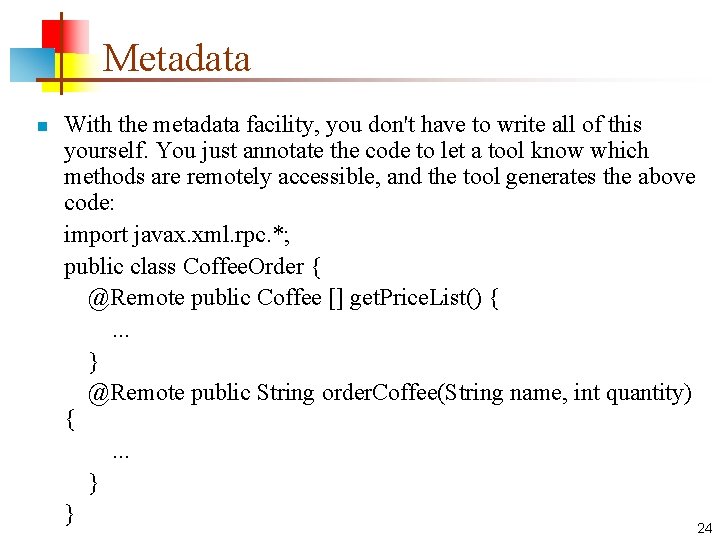
Metadata n With the metadata facility, you don't have to write all of this yourself. You just annotate the code to let a tool know which methods are remotely accessible, and the tool generates the above code: import javax. xml. rpc. *; public class Coffee. Order { @Remote public Coffee [] get. Price. List() {. . . } @Remote public String order. Coffee(String name, int quantity) {. . . } } 24
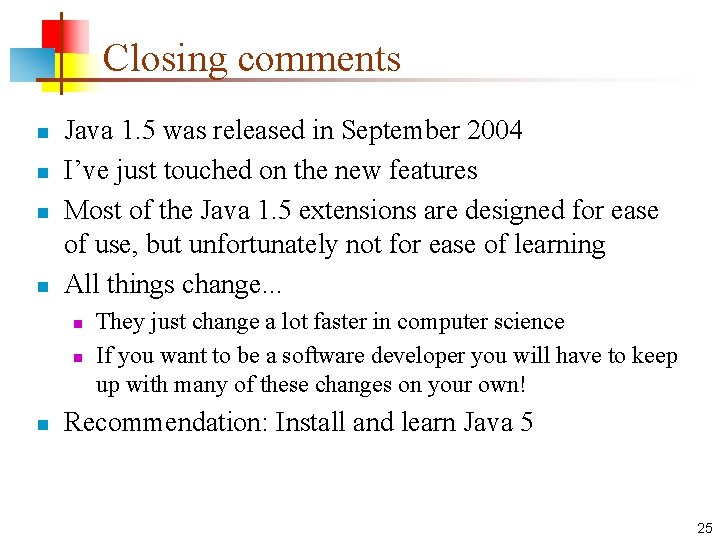
Closing comments n n Java 1. 5 was released in September 2004 I’ve just touched on the new features Most of the Java 1. 5 extensions are designed for ease of use, but unfortunately not for ease of learning All things change. . . n n n They just change a lot faster in computer science If you want to be a software developer you will have to keep up with many of these changes on your own! Recommendation: Install and learn Java 5 25