Item Event and Item Listener You implement an
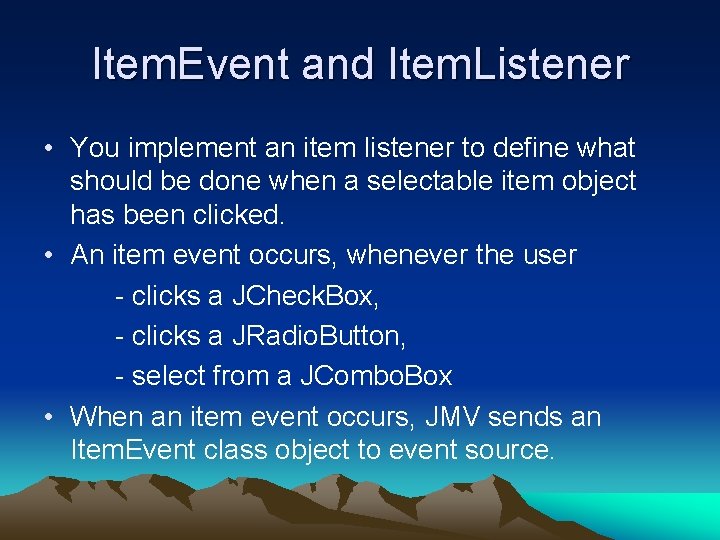
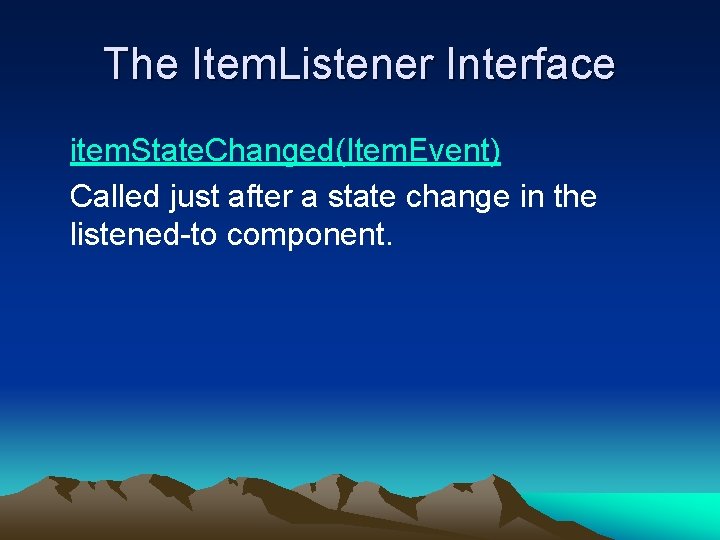
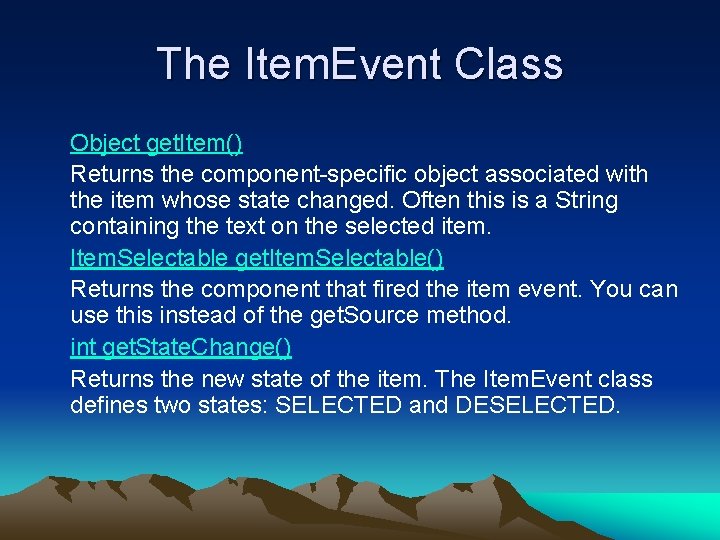
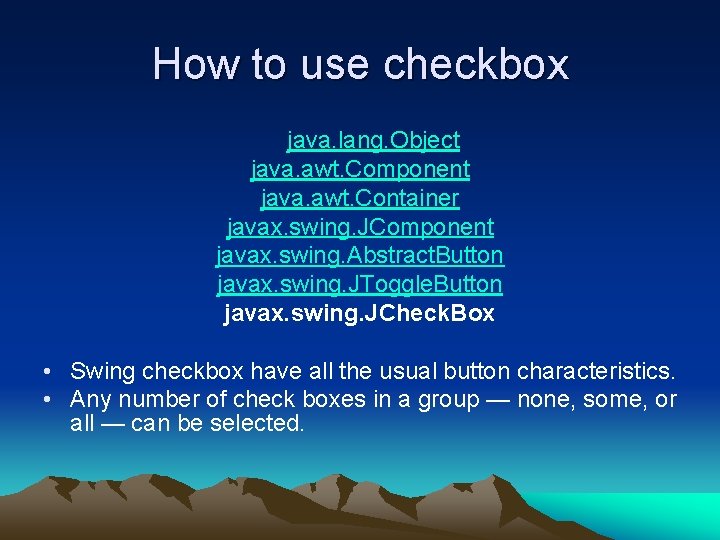
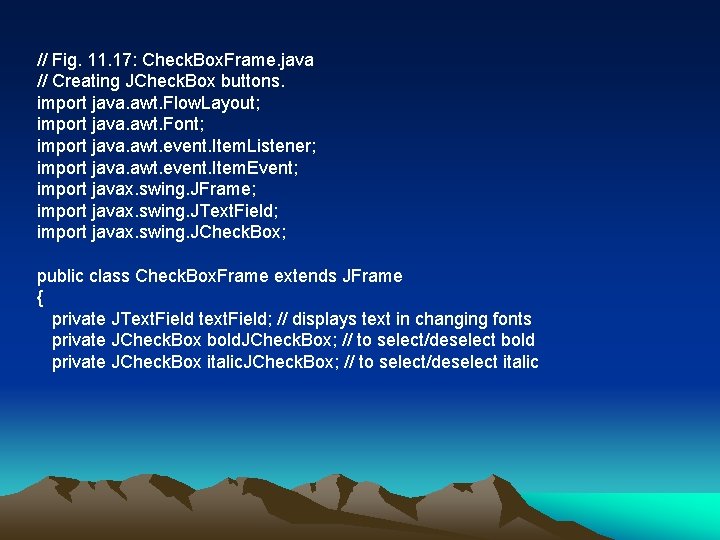
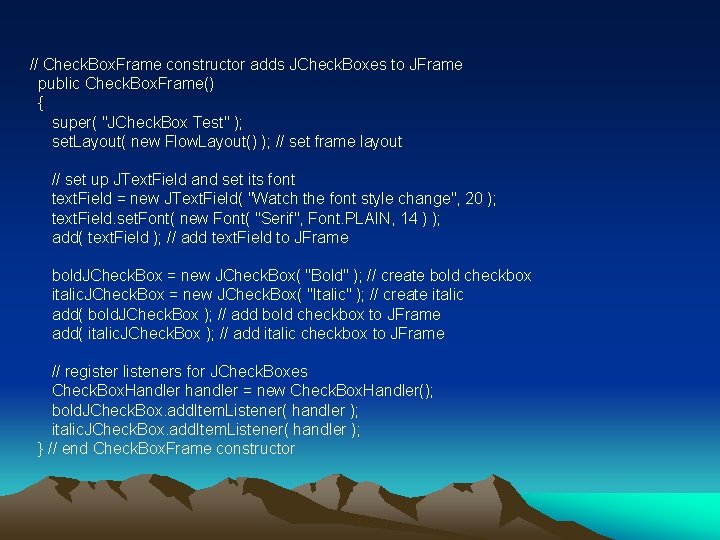
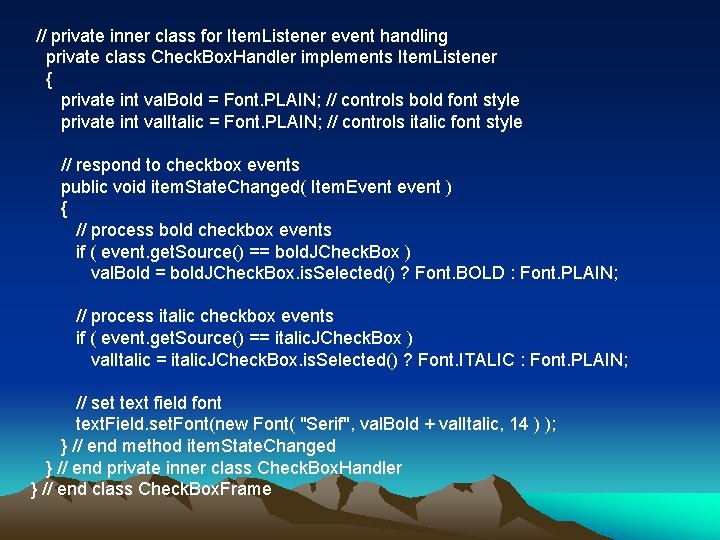
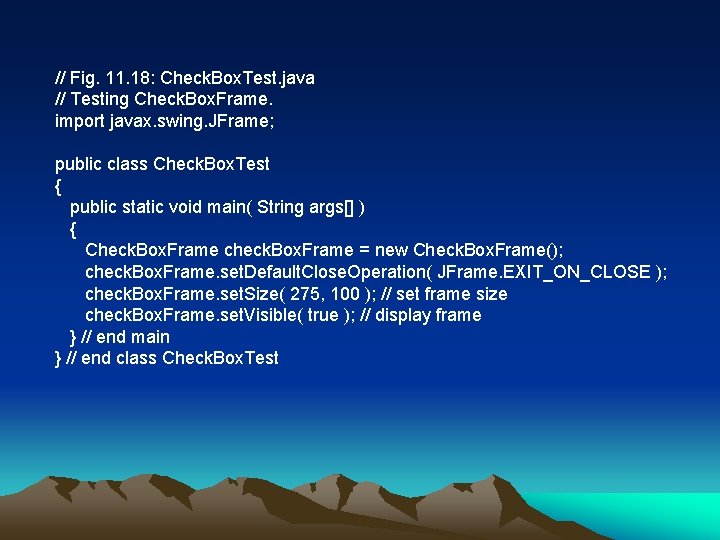
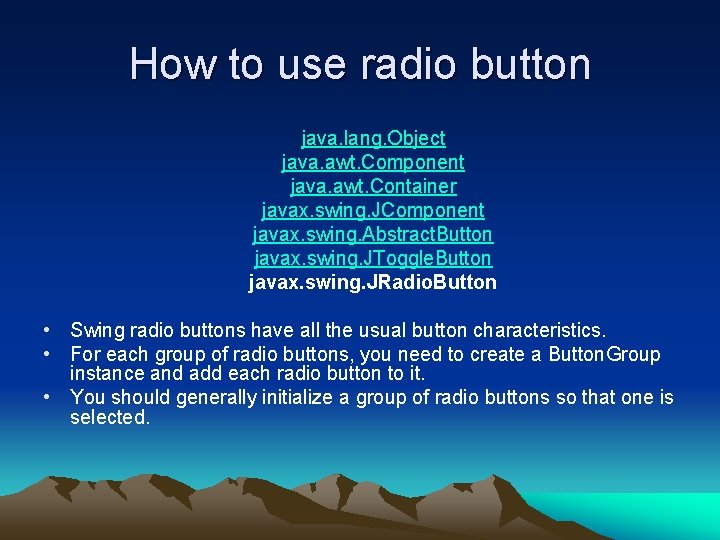
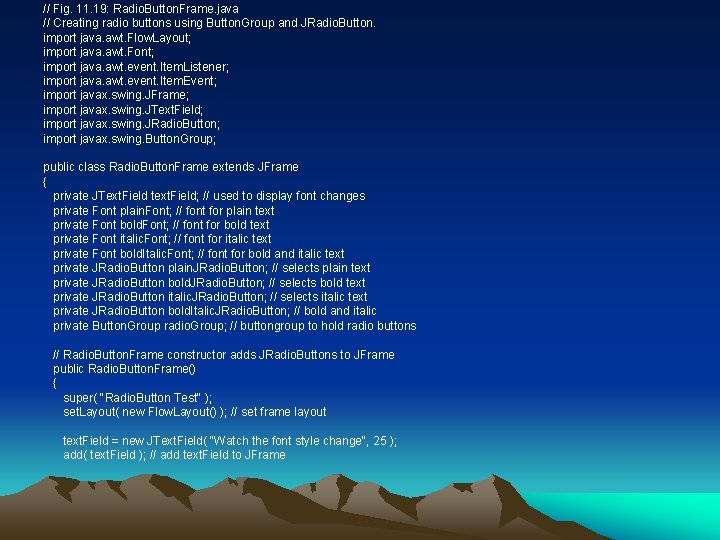
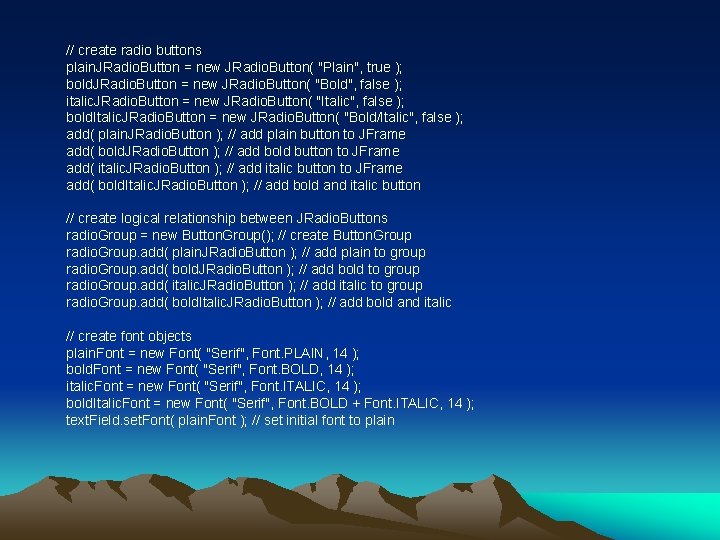
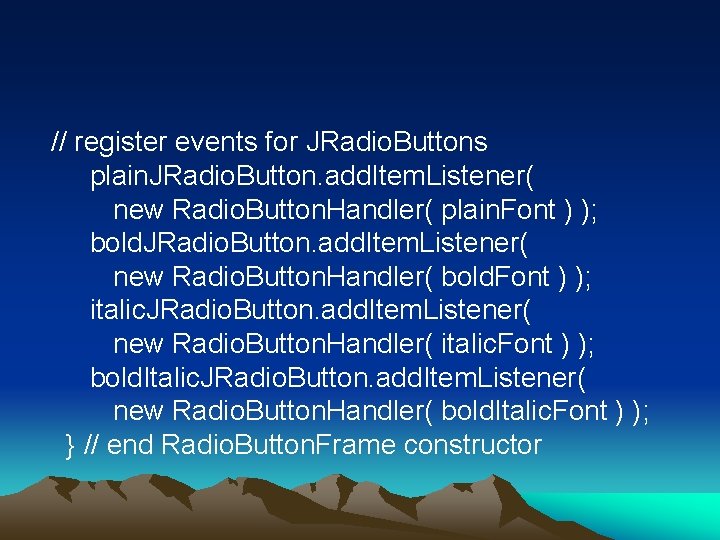
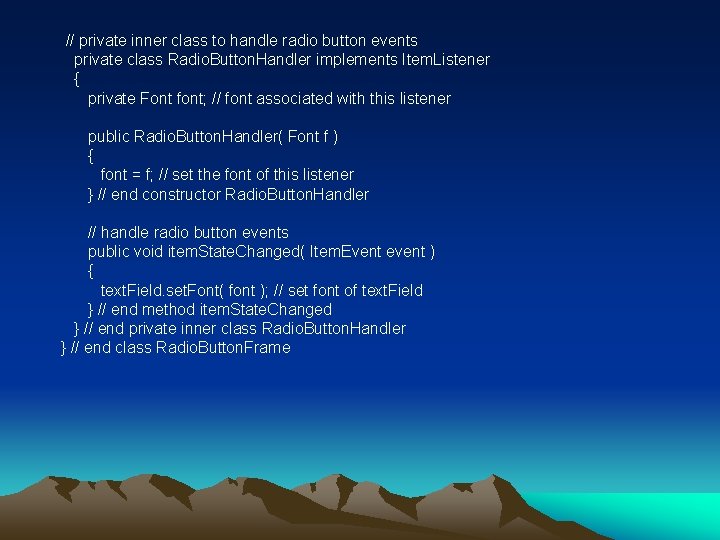
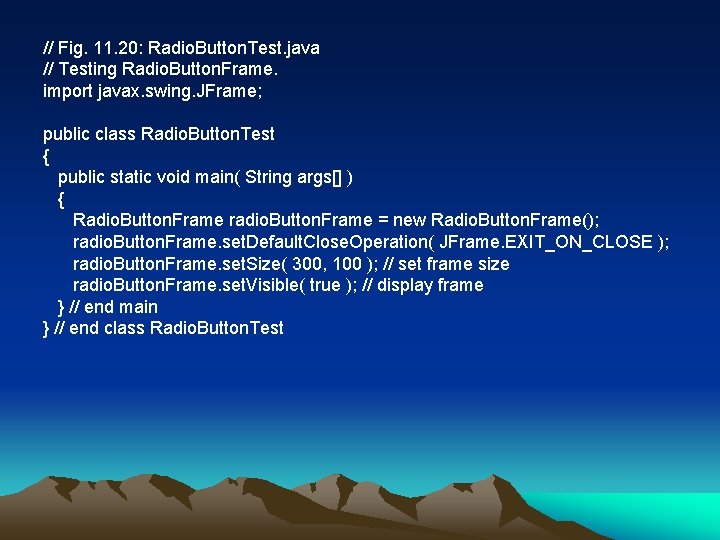
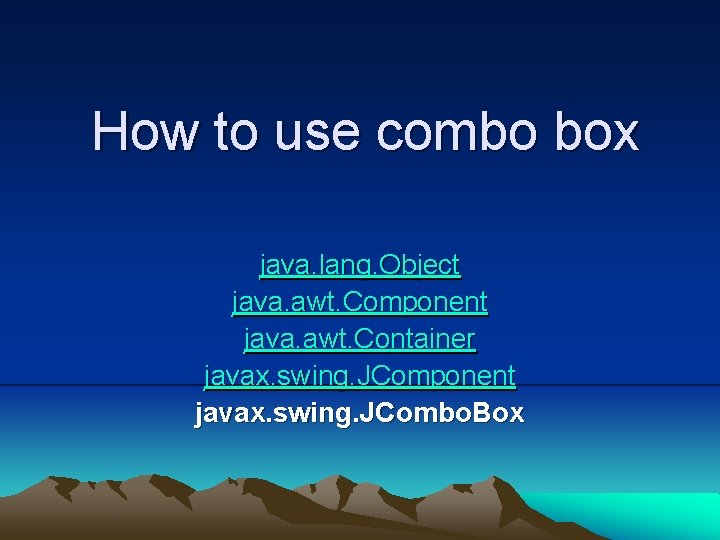
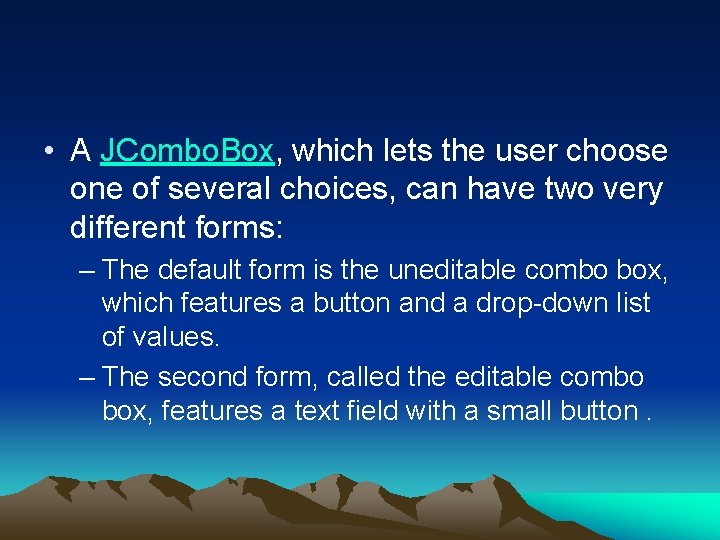
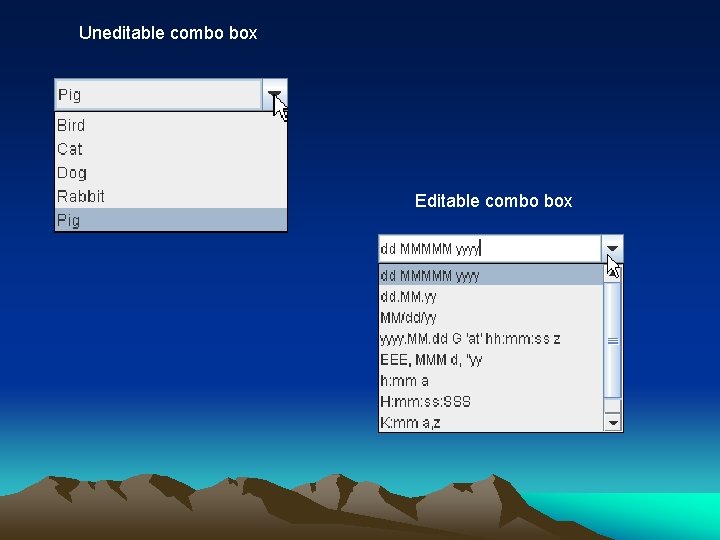
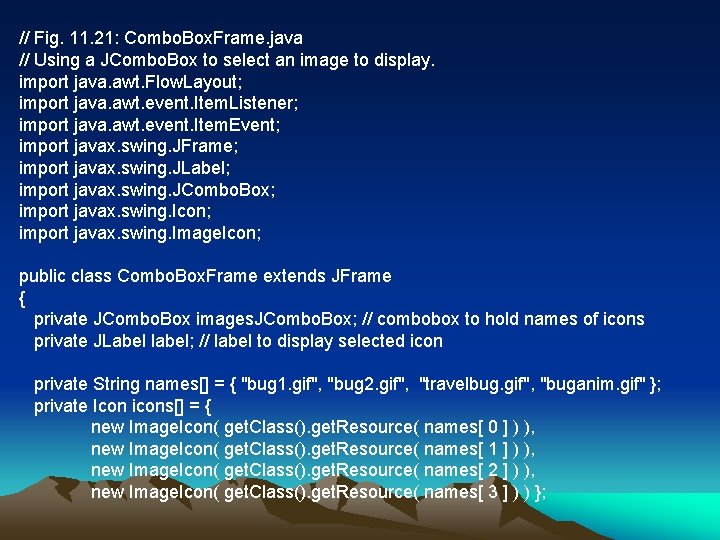
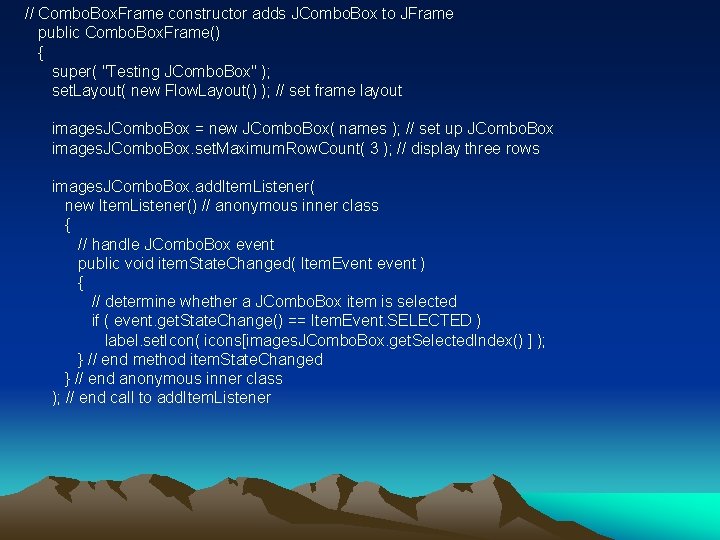
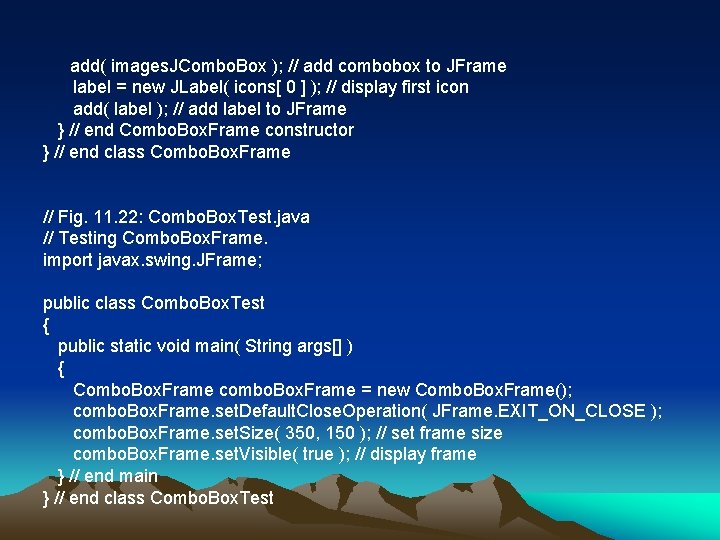
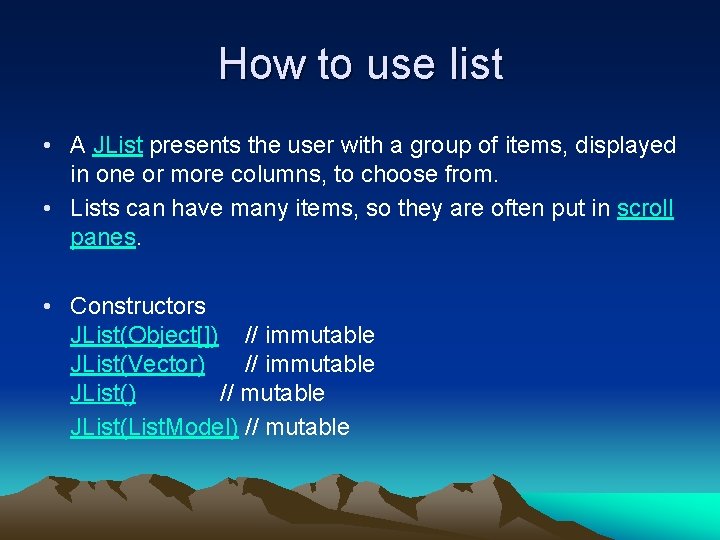
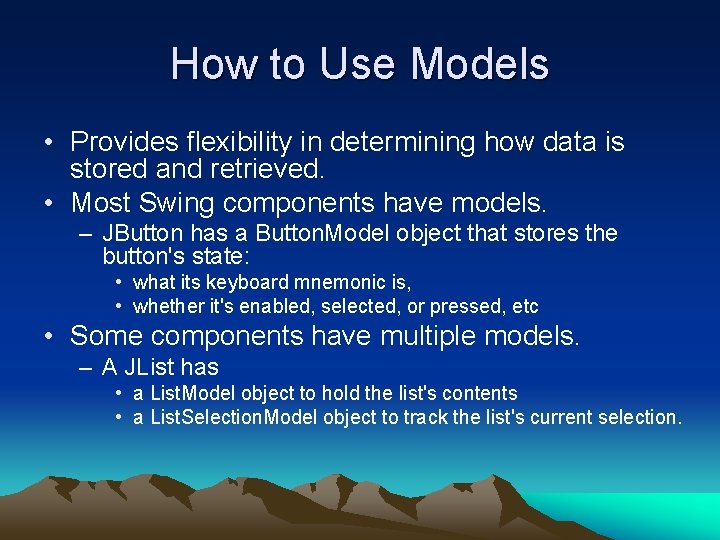
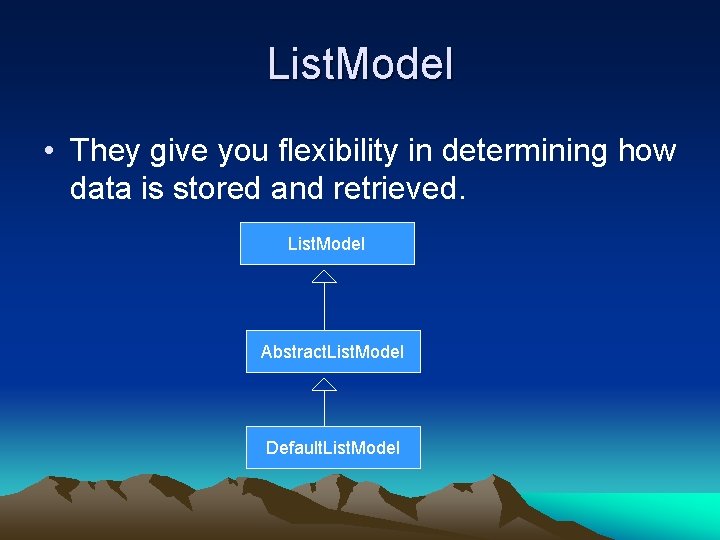
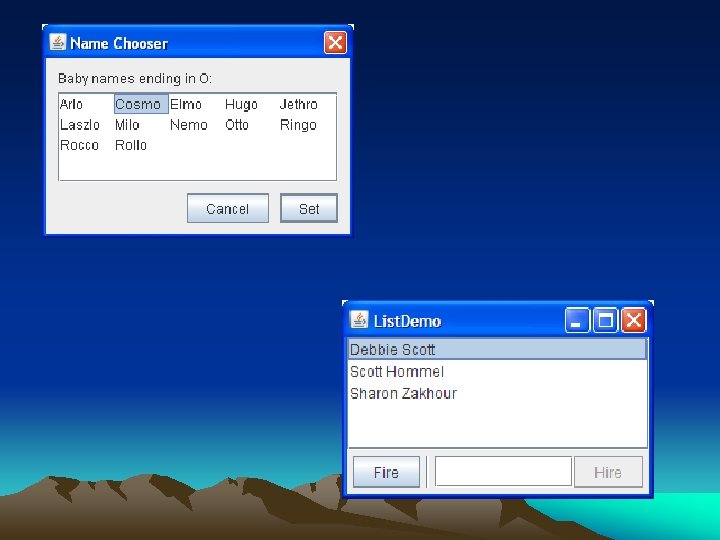
![String[] choices = {"A", "long", "array", "of", "strings"}; list = new JList(choice); list. set. String[] choices = {"A", "long", "array", "of", "strings"}; list = new JList(choice); list. set.](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-25.jpg)
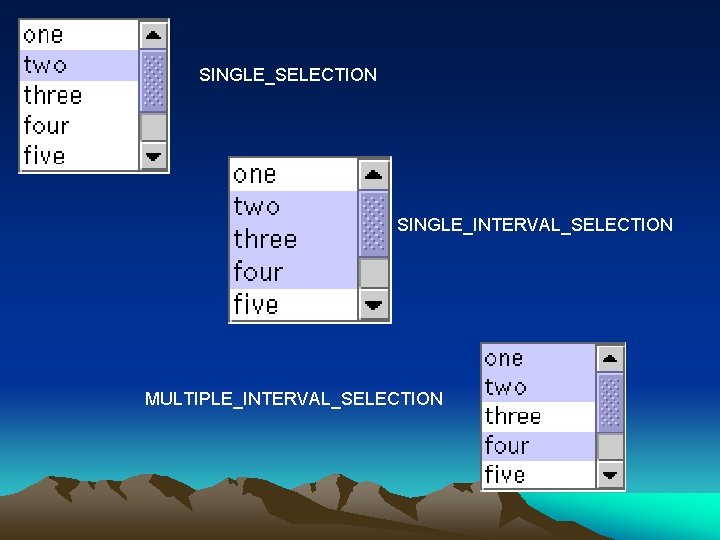
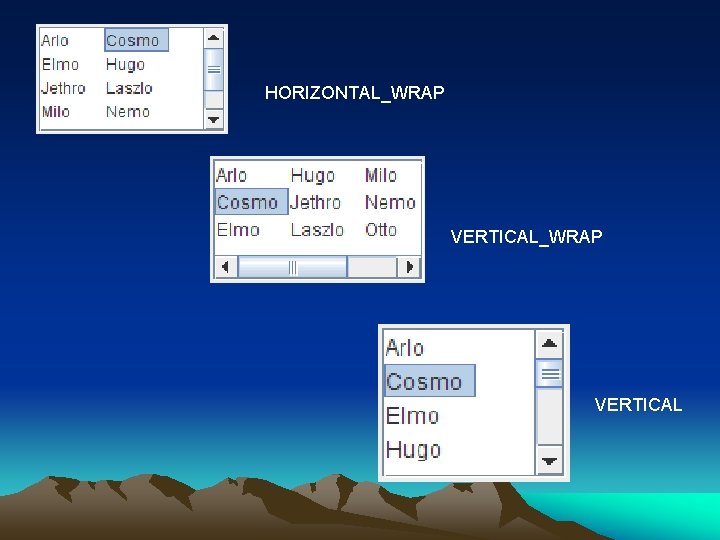
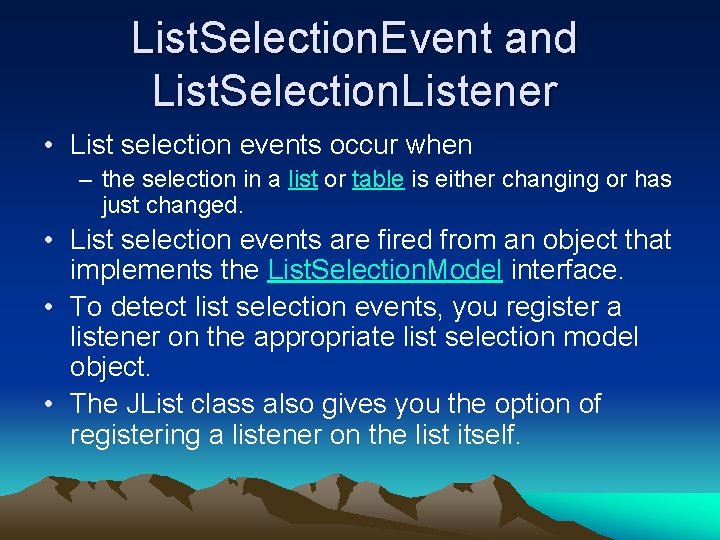
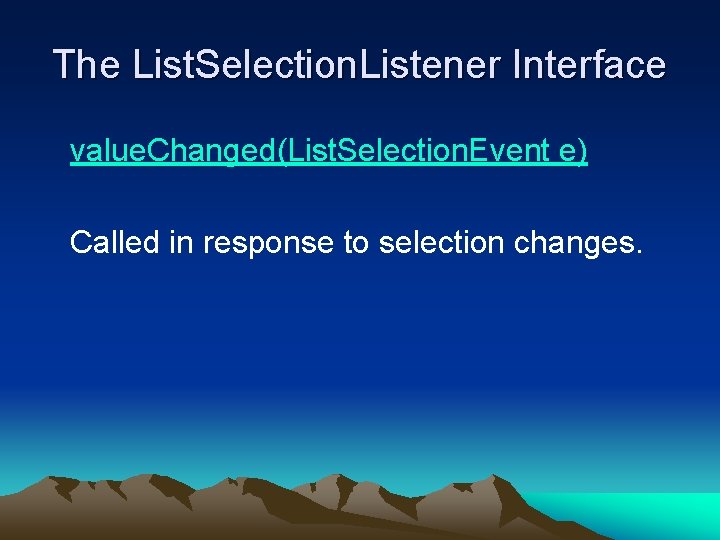
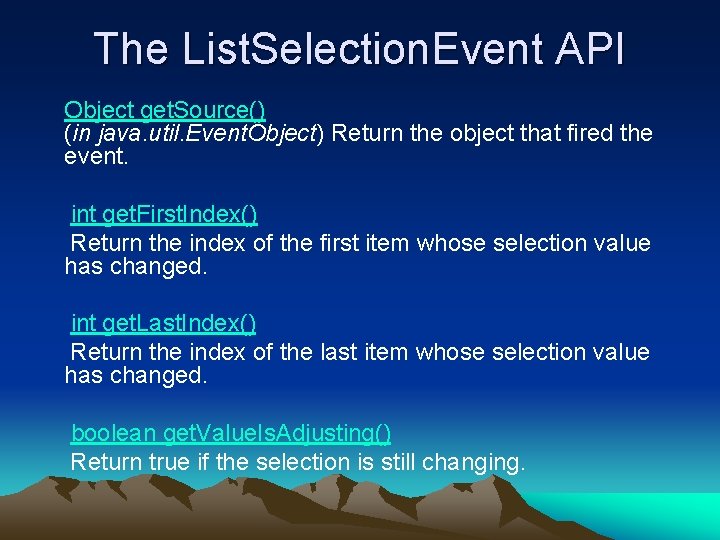
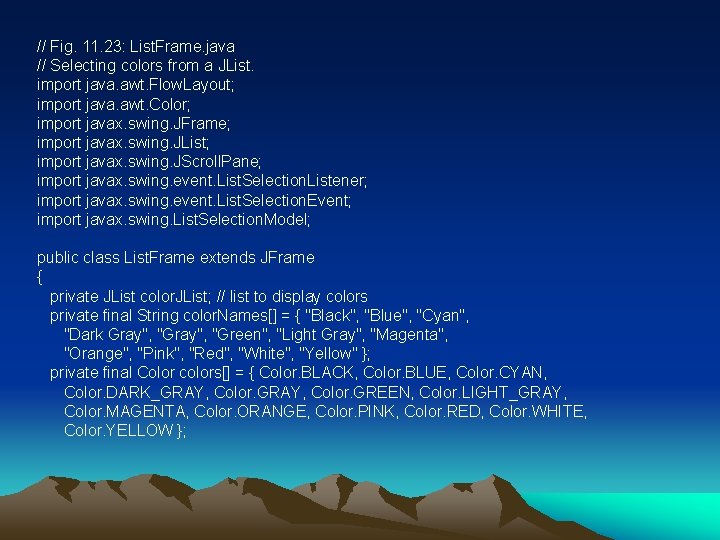
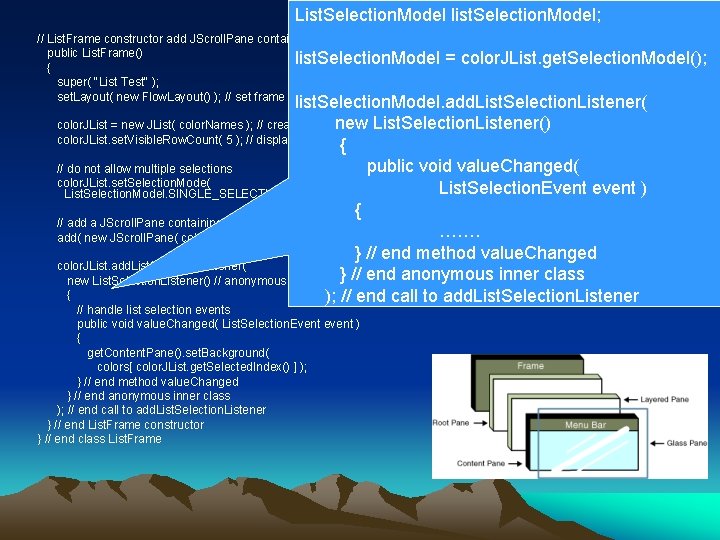
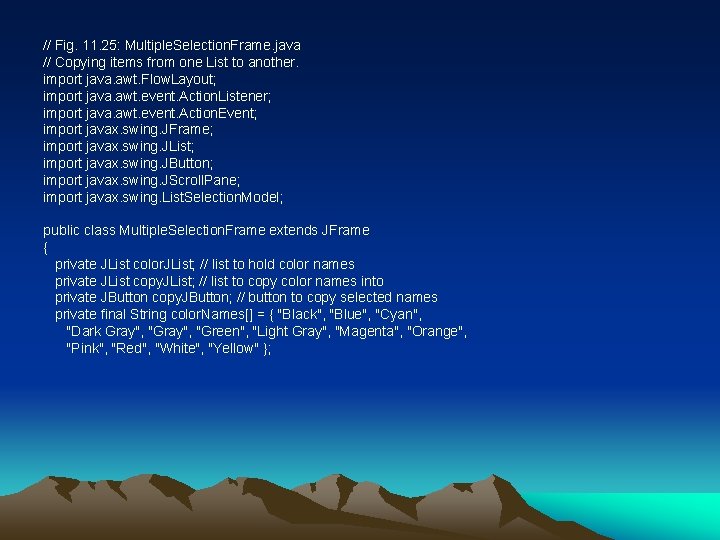
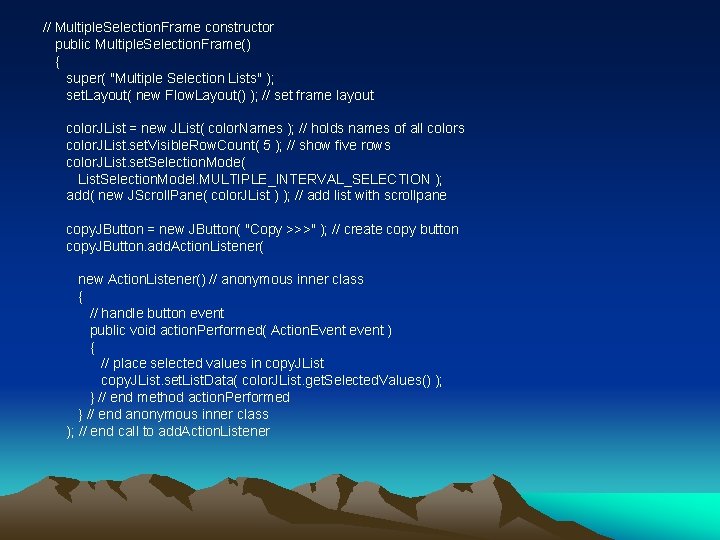
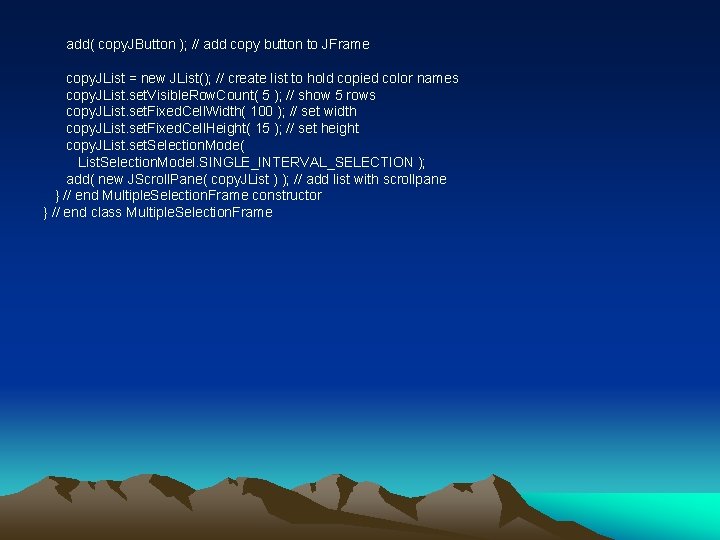
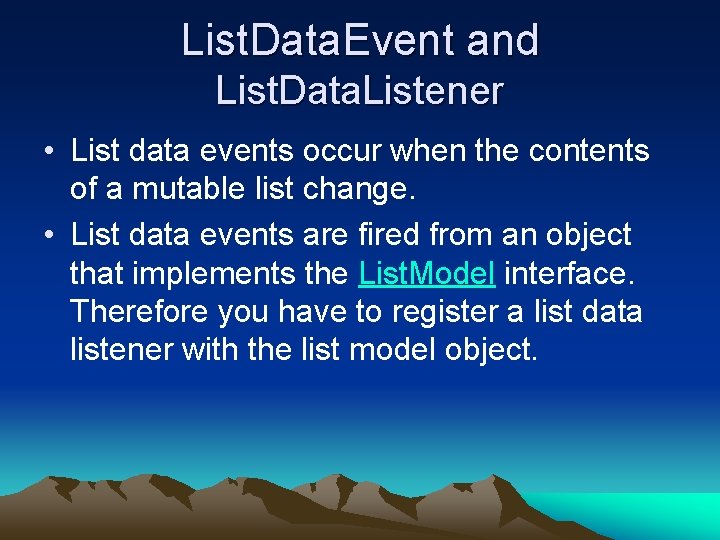
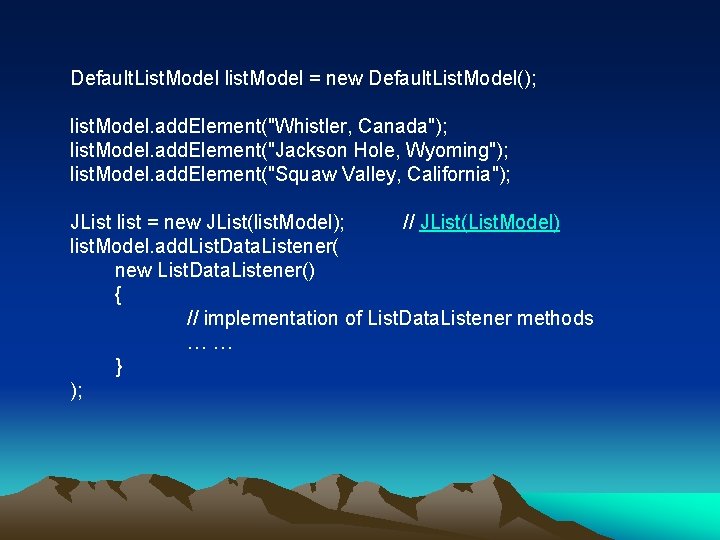
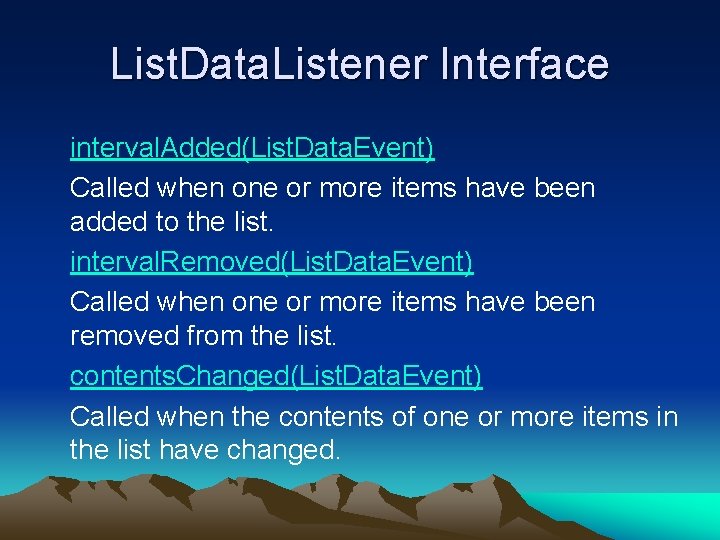
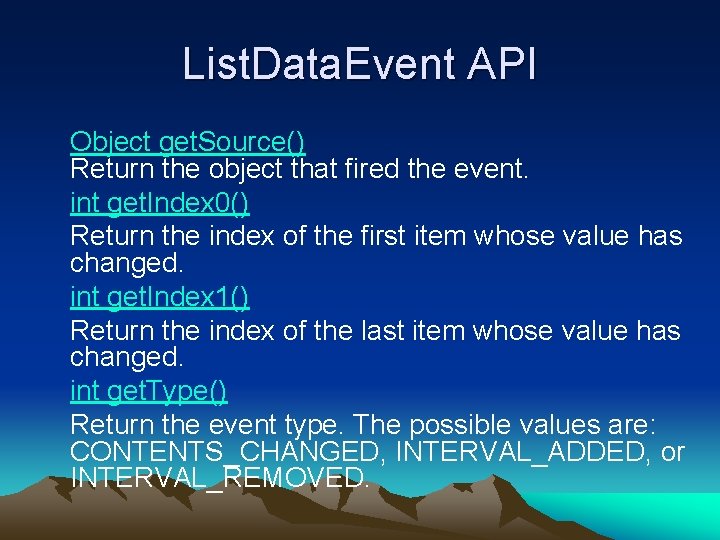
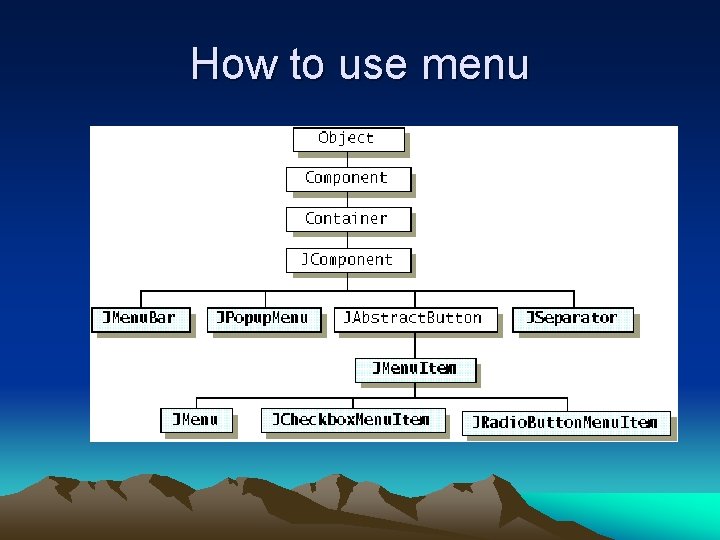
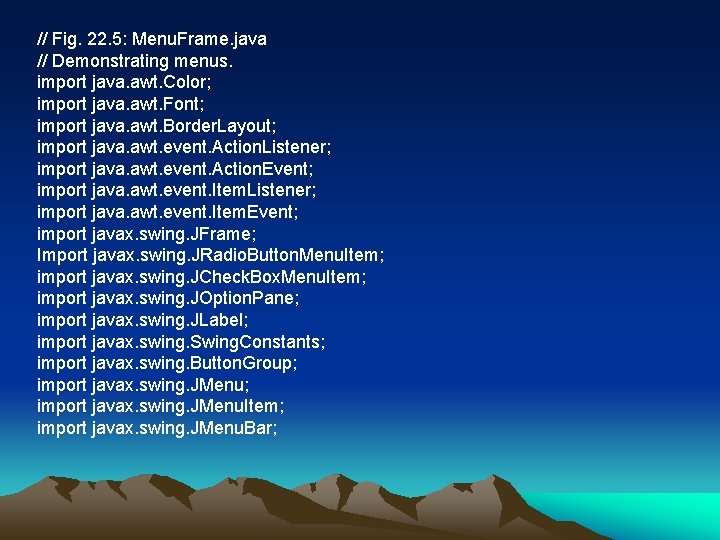
![public class Menu. Frame extends JFrame { private final Color color. Values[] = { public class Menu. Frame extends JFrame { private final Color color. Values[] = {](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-42.jpg)
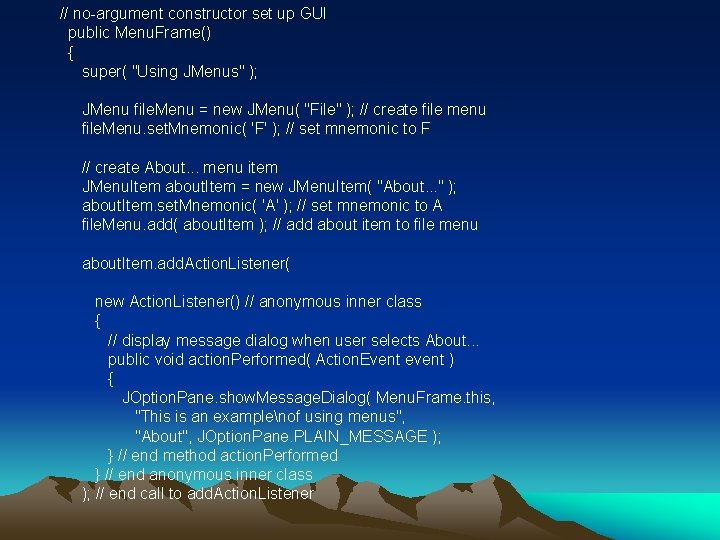
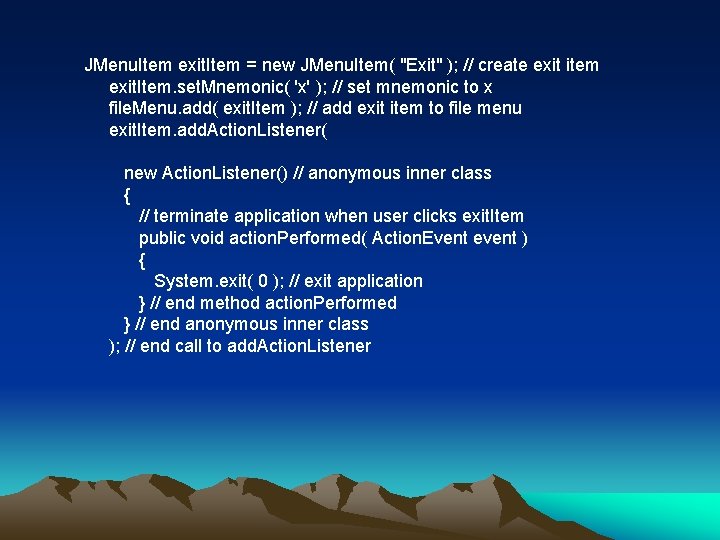
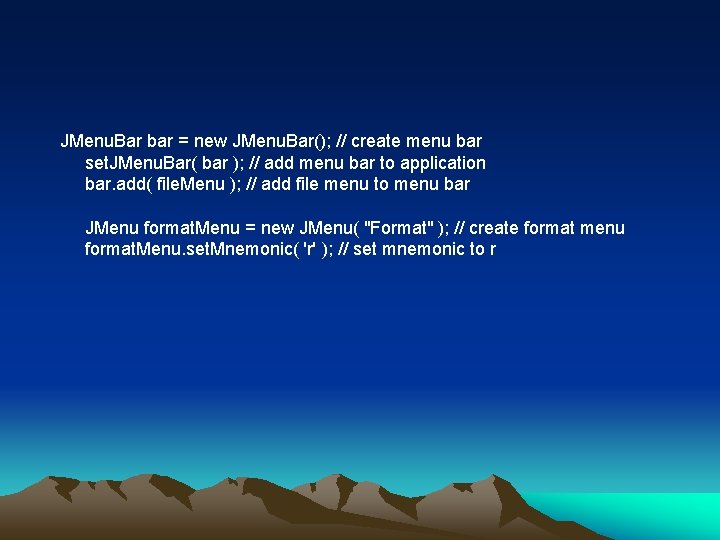
![// array listing string colors String colors[] = { "Black", "Blue", "Red", "Green" }; // array listing string colors String colors[] = { "Black", "Blue", "Red", "Green" };](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-46.jpg)
![// array listing font names String font. Names[] = { "Serif", "Monospaced", "Sans. Serif" // array listing font names String font. Names[] = { "Serif", "Monospaced", "Sans. Serif"](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-47.jpg)
![String style. Names[] = { "Bold", "Italic" }; // names of styles style. Items String style. Names[] = { "Bold", "Italic" }; // names of styles style. Items](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-48.jpg)
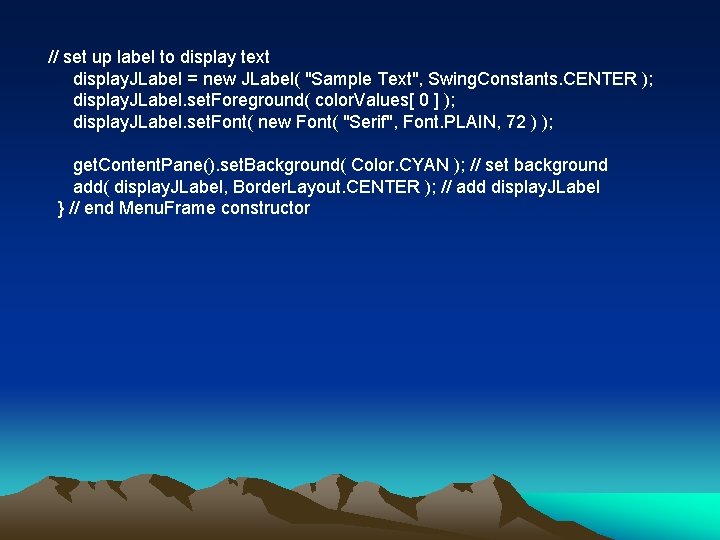
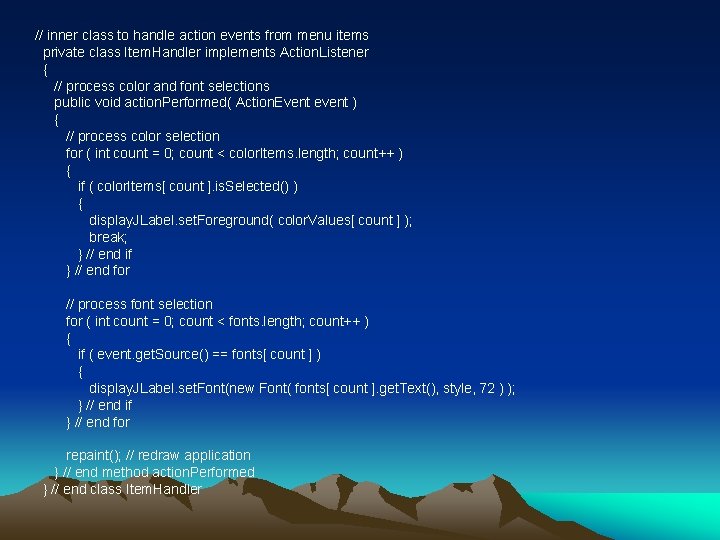
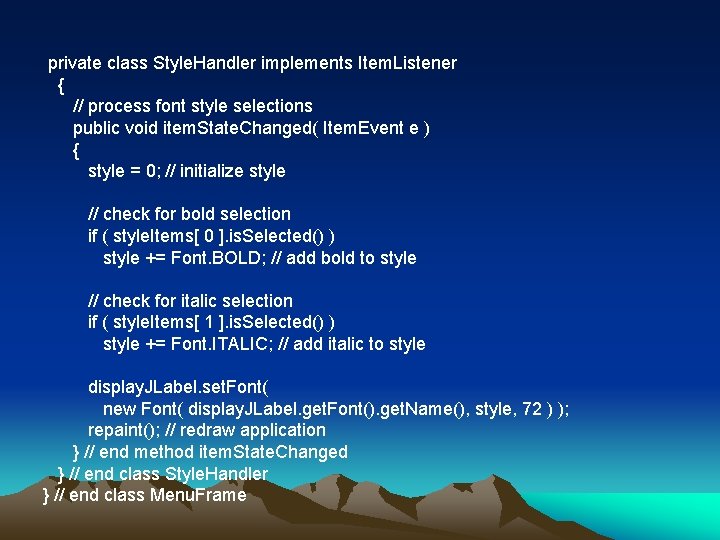
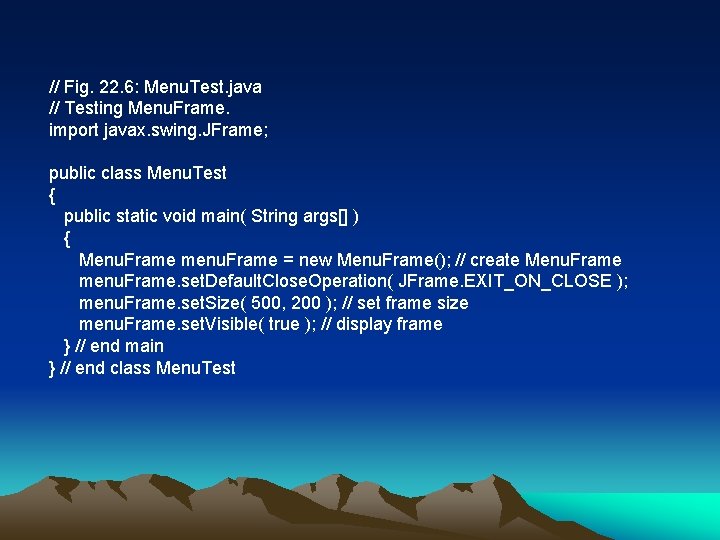
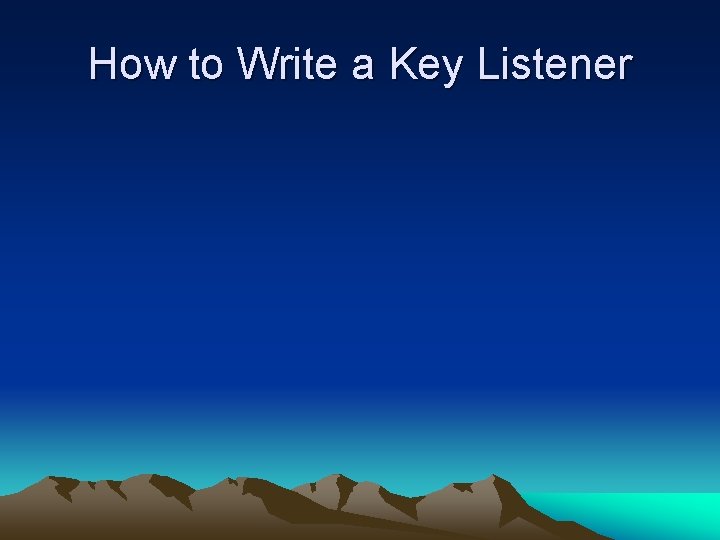
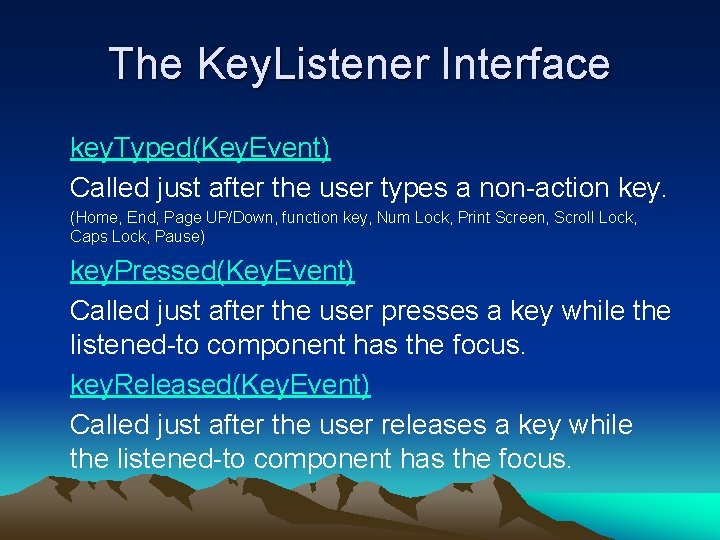
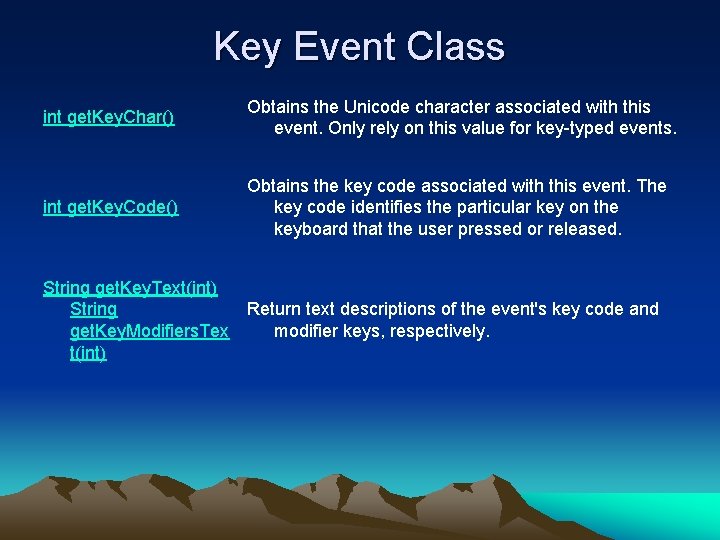
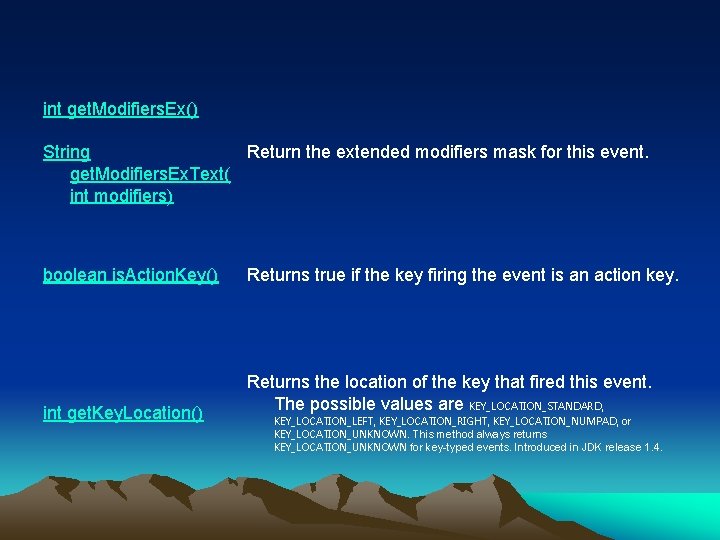
- Slides: 56
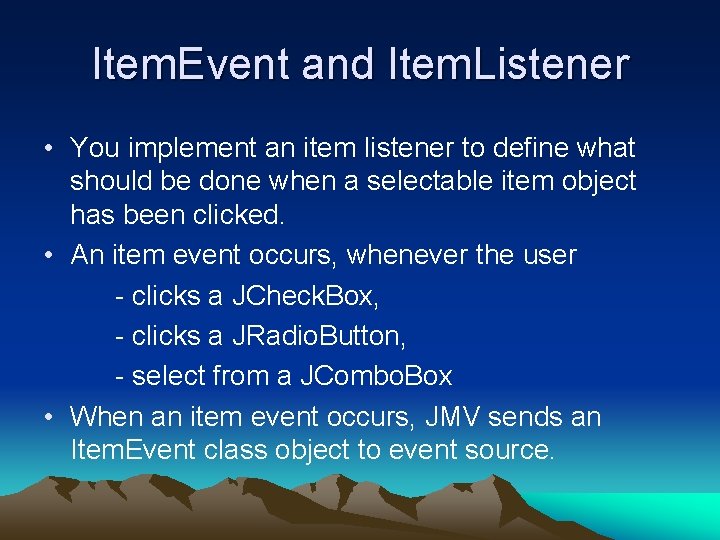
Item. Event and Item. Listener • You implement an item listener to define what should be done when a selectable item object has been clicked. • An item event occurs, whenever the user - clicks a JCheck. Box, - clicks a JRadio. Button, - select from a JCombo. Box • When an item event occurs, JMV sends an Item. Event class object to event source.
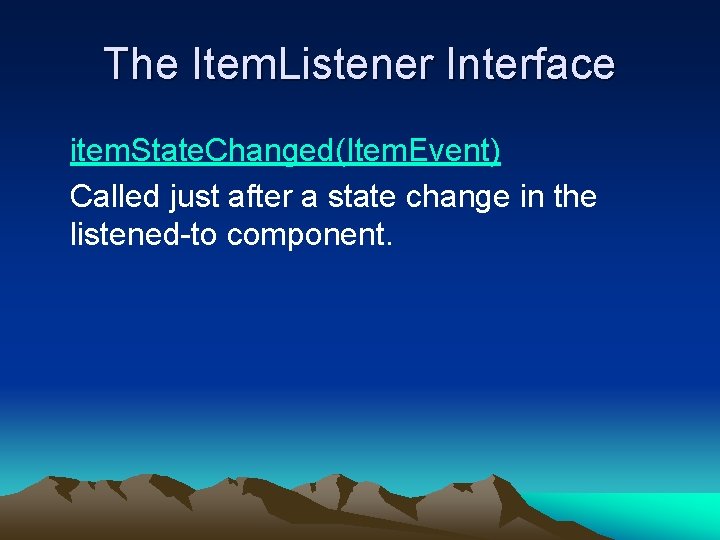
The Item. Listener Interface item. State. Changed(Item. Event) Called just after a state change in the listened-to component.
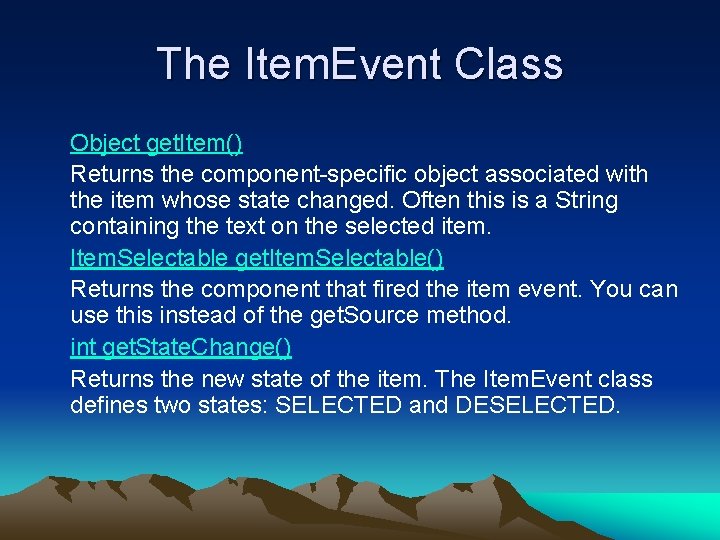
The Item. Event Class Object get. Item() Returns the component-specific object associated with the item whose state changed. Often this is a String containing the text on the selected item. Item. Selectable get. Item. Selectable() Returns the component that fired the item event. You can use this instead of the get. Source method. int get. State. Change() Returns the new state of the item. The Item. Event class defines two states: SELECTED and DESELECTED.
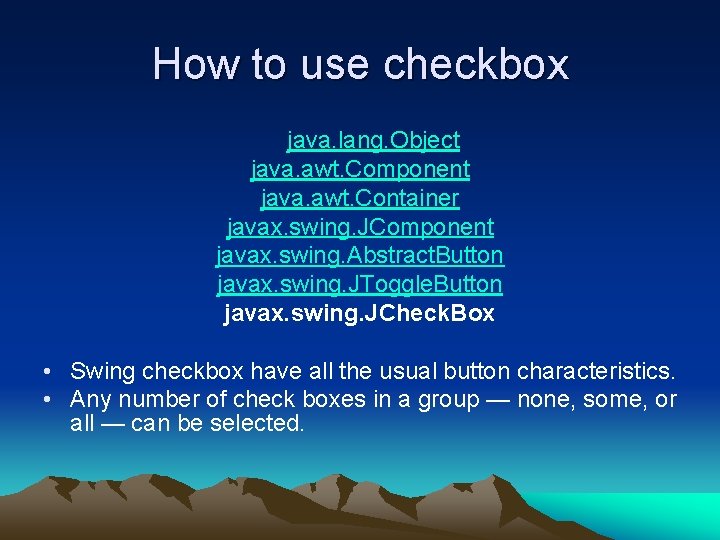
How to use checkbox java. lang. Object java. awt. Component java. awt. Container javax. swing. JComponent javax. swing. Abstract. Button javax. swing. JToggle. Button javax. swing. JCheck. Box • Swing checkbox have all the usual button characteristics. • Any number of check boxes in a group — none, some, or all — can be selected.
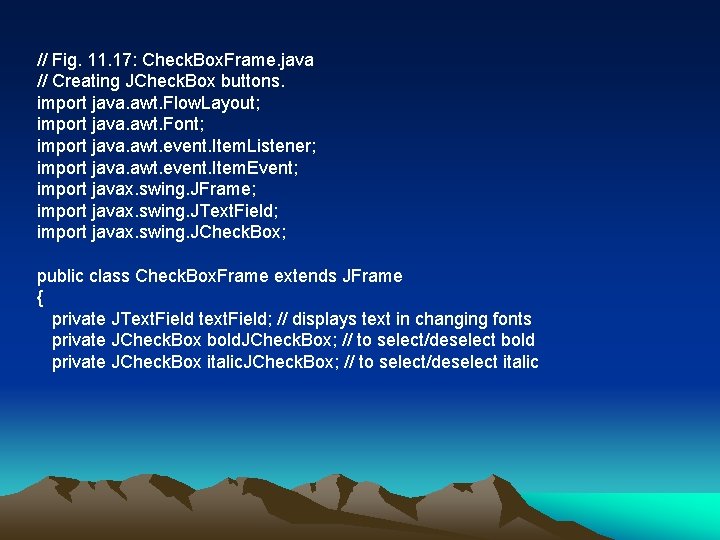
// Fig. 11. 17: Check. Box. Frame. java // Creating JCheck. Box buttons. import java. awt. Flow. Layout; import java. awt. Font; import java. awt. event. Item. Listener; import java. awt. event. Item. Event; import javax. swing. JFrame; import javax. swing. JText. Field; import javax. swing. JCheck. Box; public class Check. Box. Frame extends JFrame { private JText. Field text. Field; // displays text in changing fonts private JCheck. Box bold. JCheck. Box; // to select/deselect bold private JCheck. Box italic. JCheck. Box; // to select/deselect italic
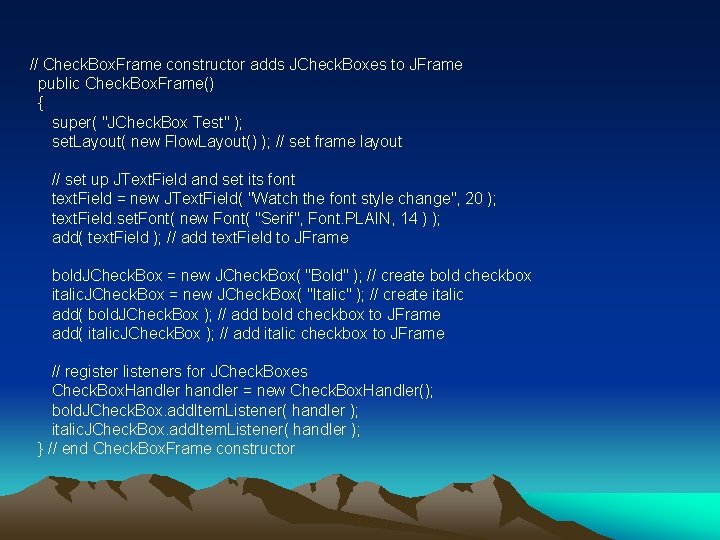
// Check. Box. Frame constructor adds JCheck. Boxes to JFrame public Check. Box. Frame() { super( "JCheck. Box Test" ); set. Layout( new Flow. Layout() ); // set frame layout // set up JText. Field and set its font text. Field = new JText. Field( "Watch the font style change", 20 ); text. Field. set. Font( new Font( "Serif", Font. PLAIN, 14 ) ); add( text. Field ); // add text. Field to JFrame bold. JCheck. Box = new JCheck. Box( "Bold" ); // create bold checkbox italic. JCheck. Box = new JCheck. Box( "Italic" ); // create italic add( bold. JCheck. Box ); // add bold checkbox to JFrame add( italic. JCheck. Box ); // add italic checkbox to JFrame // register listeners for JCheck. Boxes Check. Box. Handler handler = new Check. Box. Handler(); bold. JCheck. Box. add. Item. Listener( handler ); italic. JCheck. Box. add. Item. Listener( handler ); } // end Check. Box. Frame constructor
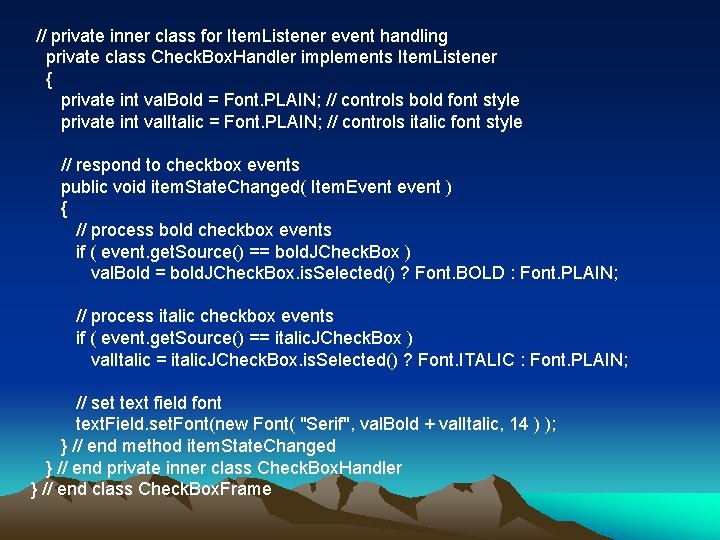
// private inner class for Item. Listener event handling private class Check. Box. Handler implements Item. Listener { private int val. Bold = Font. PLAIN; // controls bold font style private int val. Italic = Font. PLAIN; // controls italic font style // respond to checkbox events public void item. State. Changed( Item. Event event ) { // process bold checkbox events if ( event. get. Source() == bold. JCheck. Box ) val. Bold = bold. JCheck. Box. is. Selected() ? Font. BOLD : Font. PLAIN; // process italic checkbox events if ( event. get. Source() == italic. JCheck. Box ) val. Italic = italic. JCheck. Box. is. Selected() ? Font. ITALIC : Font. PLAIN; // set text field font text. Field. set. Font(new Font( "Serif", val. Bold + val. Italic, 14 ) ); } // end method item. State. Changed } // end private inner class Check. Box. Handler } // end class Check. Box. Frame
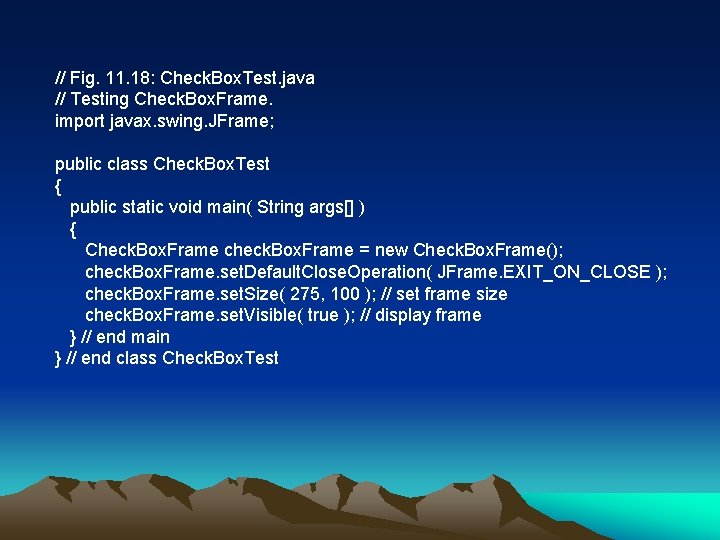
// Fig. 11. 18: Check. Box. Test. java // Testing Check. Box. Frame. import javax. swing. JFrame; public class Check. Box. Test { public static void main( String args[] ) { Check. Box. Frame check. Box. Frame = new Check. Box. Frame(); check. Box. Frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); check. Box. Frame. set. Size( 275, 100 ); // set frame size check. Box. Frame. set. Visible( true ); // display frame } // end main } // end class Check. Box. Test
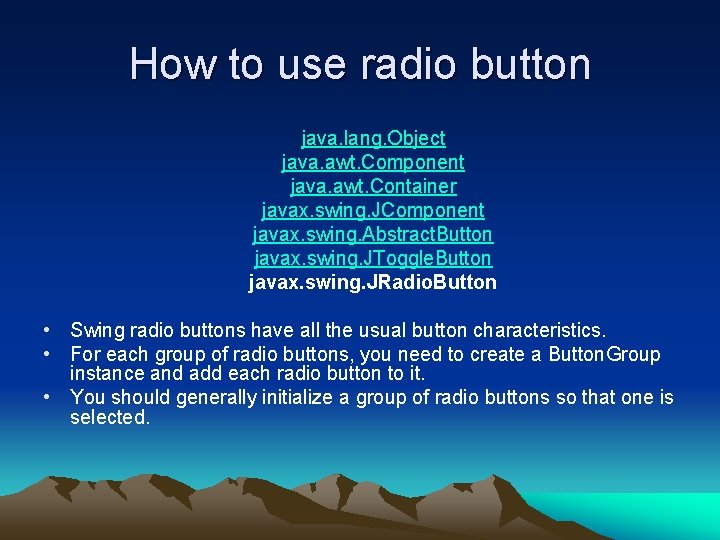
How to use radio button java. lang. Object java. awt. Component java. awt. Container javax. swing. JComponent javax. swing. Abstract. Button javax. swing. JToggle. Button javax. swing. JRadio. Button • Swing radio buttons have all the usual button characteristics. • For each group of radio buttons, you need to create a Button. Group instance and add each radio button to it. • You should generally initialize a group of radio buttons so that one is selected.
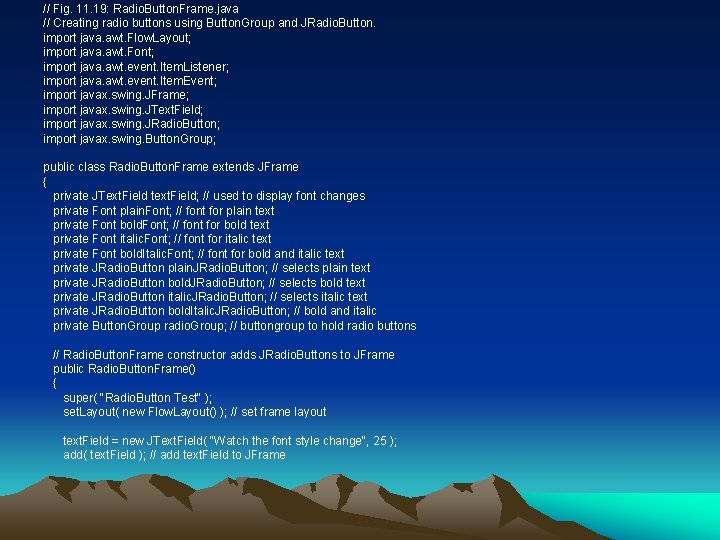
// Fig. 11. 19: Radio. Button. Frame. java // Creating radio buttons using Button. Group and JRadio. Button. import java. awt. Flow. Layout; import java. awt. Font; import java. awt. event. Item. Listener; import java. awt. event. Item. Event; import javax. swing. JFrame; import javax. swing. JText. Field; import javax. swing. JRadio. Button; import javax. swing. Button. Group; public class Radio. Button. Frame extends JFrame { private JText. Field text. Field; // used to display font changes private Font plain. Font; // font for plain text private Font bold. Font; // font for bold text private Font italic. Font; // font for italic text private Font bold. Italic. Font; // font for bold and italic text private JRadio. Button plain. JRadio. Button; // selects plain text private JRadio. Button bold. JRadio. Button; // selects bold text private JRadio. Button italic. JRadio. Button; // selects italic text private JRadio. Button bold. Italic. JRadio. Button; // bold and italic private Button. Group radio. Group; // buttongroup to hold radio buttons // Radio. Button. Frame constructor adds JRadio. Buttons to JFrame public Radio. Button. Frame() { super( "Radio. Button Test" ); set. Layout( new Flow. Layout() ); // set frame layout text. Field = new JText. Field( "Watch the font style change", 25 ); add( text. Field ); // add text. Field to JFrame
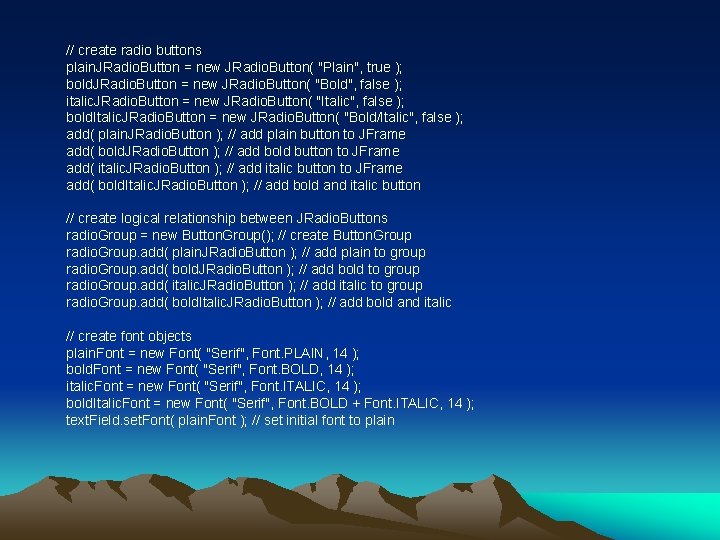
// create radio buttons plain. JRadio. Button = new JRadio. Button( "Plain", true ); bold. JRadio. Button = new JRadio. Button( "Bold", false ); italic. JRadio. Button = new JRadio. Button( "Italic", false ); bold. Italic. JRadio. Button = new JRadio. Button( "Bold/Italic", false ); add( plain. JRadio. Button ); // add plain button to JFrame add( bold. JRadio. Button ); // add bold button to JFrame add( italic. JRadio. Button ); // add italic button to JFrame add( bold. Italic. JRadio. Button ); // add bold and italic button // create logical relationship between JRadio. Buttons radio. Group = new Button. Group(); // create Button. Group radio. Group. add( plain. JRadio. Button ); // add plain to group radio. Group. add( bold. JRadio. Button ); // add bold to group radio. Group. add( italic. JRadio. Button ); // add italic to group radio. Group. add( bold. Italic. JRadio. Button ); // add bold and italic // create font objects plain. Font = new Font( "Serif", Font. PLAIN, 14 ); bold. Font = new Font( "Serif", Font. BOLD, 14 ); italic. Font = new Font( "Serif", Font. ITALIC, 14 ); bold. Italic. Font = new Font( "Serif", Font. BOLD + Font. ITALIC, 14 ); text. Field. set. Font( plain. Font ); // set initial font to plain
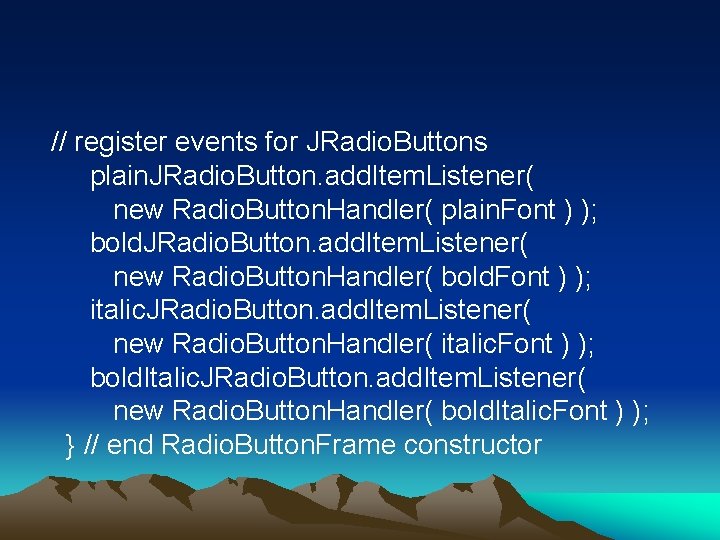
// register events for JRadio. Buttons plain. JRadio. Button. add. Item. Listener( new Radio. Button. Handler( plain. Font ) ); bold. JRadio. Button. add. Item. Listener( new Radio. Button. Handler( bold. Font ) ); italic. JRadio. Button. add. Item. Listener( new Radio. Button. Handler( italic. Font ) ); bold. Italic. JRadio. Button. add. Item. Listener( new Radio. Button. Handler( bold. Italic. Font ) ); } // end Radio. Button. Frame constructor
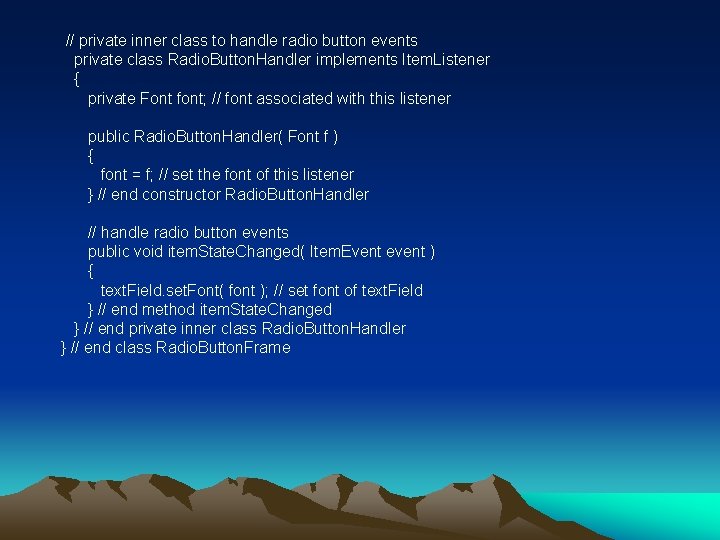
// private inner class to handle radio button events private class Radio. Button. Handler implements Item. Listener { private Font font; // font associated with this listener public Radio. Button. Handler( Font f ) { font = f; // set the font of this listener } // end constructor Radio. Button. Handler // handle radio button events public void item. State. Changed( Item. Event event ) { text. Field. set. Font( font ); // set font of text. Field } // end method item. State. Changed } // end private inner class Radio. Button. Handler } // end class Radio. Button. Frame
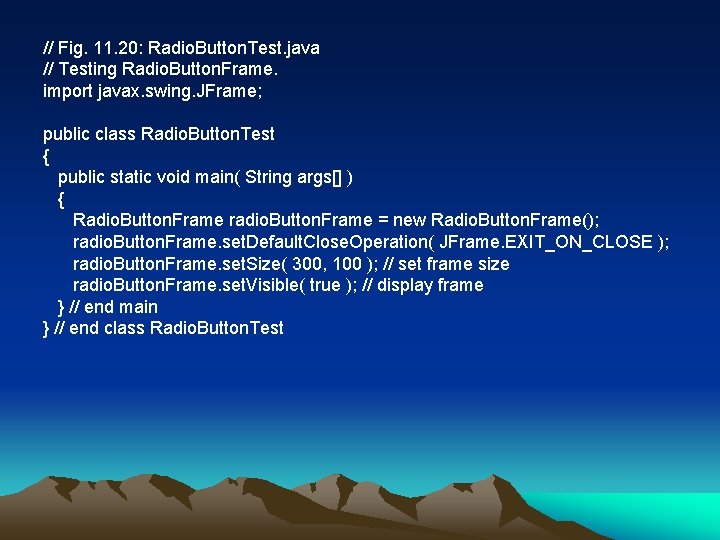
// Fig. 11. 20: Radio. Button. Test. java // Testing Radio. Button. Frame. import javax. swing. JFrame; public class Radio. Button. Test { public static void main( String args[] ) { Radio. Button. Frame radio. Button. Frame = new Radio. Button. Frame(); radio. Button. Frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); radio. Button. Frame. set. Size( 300, 100 ); // set frame size radio. Button. Frame. set. Visible( true ); // display frame } // end main } // end class Radio. Button. Test
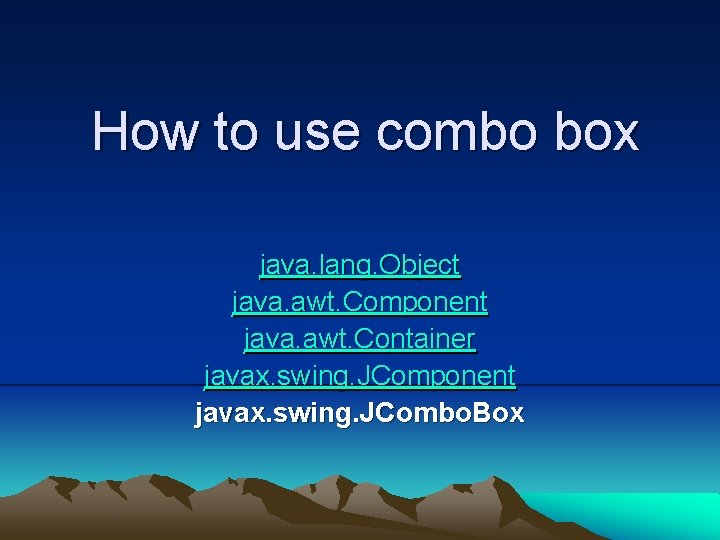
How to use combo box java. lang. Object java. awt. Component java. awt. Container javax. swing. JComponent javax. swing. JCombo. Box
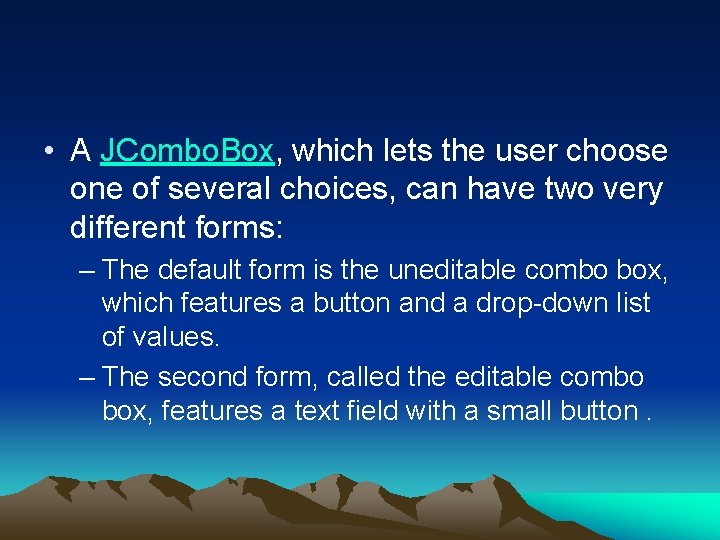
• A JCombo. Box, which lets the user choose one of several choices, can have two very different forms: – The default form is the uneditable combo box, which features a button and a drop-down list of values. – The second form, called the editable combo box, features a text field with a small button.
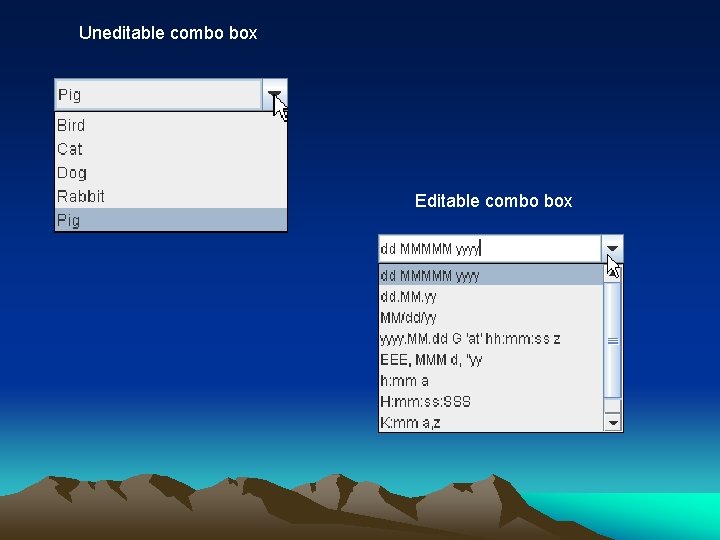
Uneditable combo box Editable combo box
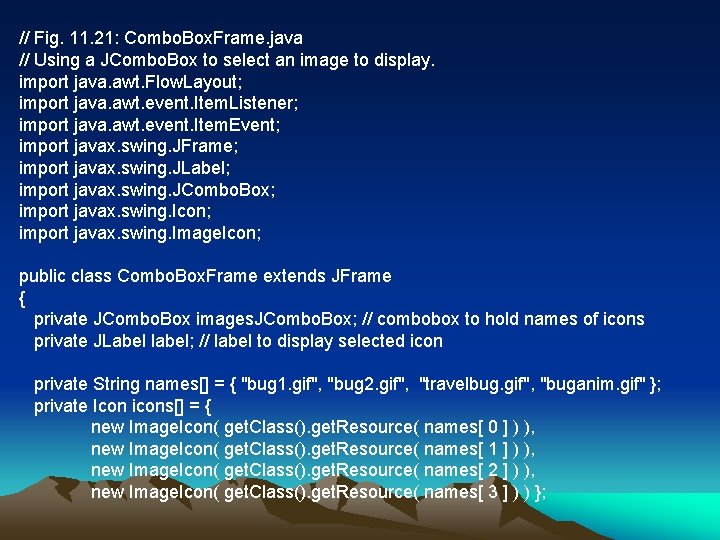
// Fig. 11. 21: Combo. Box. Frame. java // Using a JCombo. Box to select an image to display. import java. awt. Flow. Layout; import java. awt. event. Item. Listener; import java. awt. event. Item. Event; import javax. swing. JFrame; import javax. swing. JLabel; import javax. swing. JCombo. Box; import javax. swing. Icon; import javax. swing. Image. Icon; public class Combo. Box. Frame extends JFrame { private JCombo. Box images. JCombo. Box; // combobox to hold names of icons private JLabel label; // label to display selected icon private String names[] = { "bug 1. gif", "bug 2. gif", "travelbug. gif", "buganim. gif" }; private Icon icons[] = { new Image. Icon( get. Class(). get. Resource( names[ 0 ] ) ), new Image. Icon( get. Class(). get. Resource( names[ 1 ] ) ), new Image. Icon( get. Class(). get. Resource( names[ 2 ] ) ), new Image. Icon( get. Class(). get. Resource( names[ 3 ] ) ) };
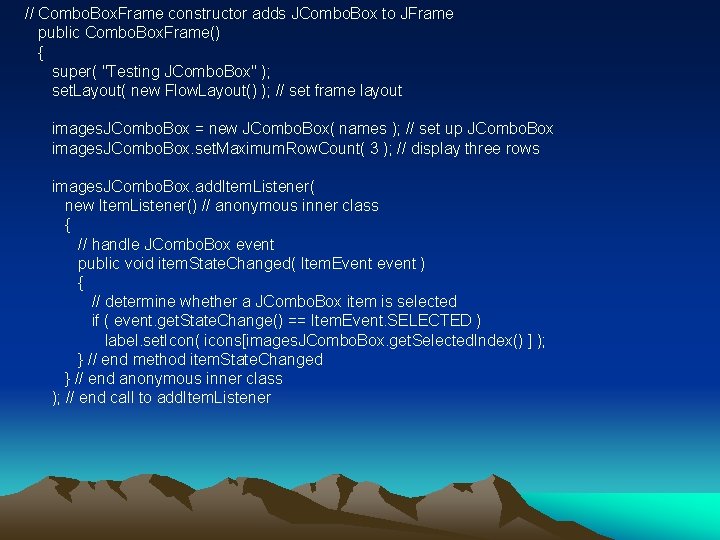
// Combo. Box. Frame constructor adds JCombo. Box to JFrame public Combo. Box. Frame() { super( "Testing JCombo. Box" ); set. Layout( new Flow. Layout() ); // set frame layout images. JCombo. Box = new JCombo. Box( names ); // set up JCombo. Box images. JCombo. Box. set. Maximum. Row. Count( 3 ); // display three rows images. JCombo. Box. add. Item. Listener( new Item. Listener() // anonymous inner class { // handle JCombo. Box event public void item. State. Changed( Item. Event event ) { // determine whether a JCombo. Box item is selected if ( event. get. State. Change() == Item. Event. SELECTED ) label. set. Icon( icons[images. JCombo. Box. get. Selected. Index() ] ); } // end method item. State. Changed } // end anonymous inner class ); // end call to add. Item. Listener
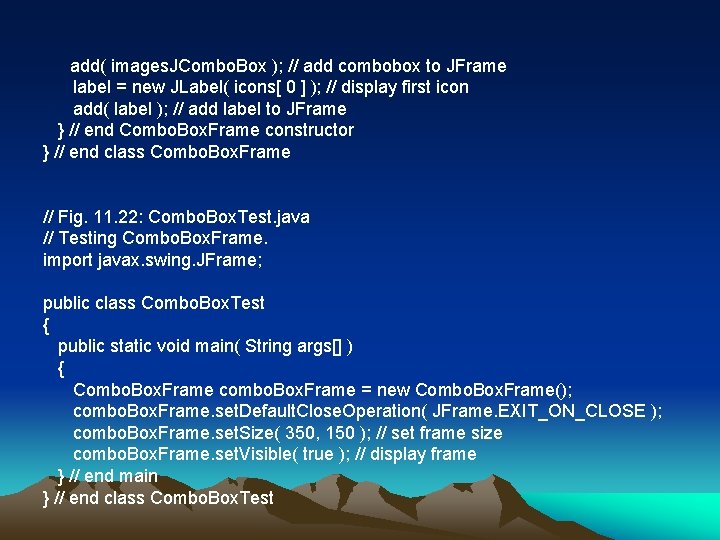
add( images. JCombo. Box ); // add combobox to JFrame label = new JLabel( icons[ 0 ] ); // display first icon add( label ); // add label to JFrame } // end Combo. Box. Frame constructor } // end class Combo. Box. Frame // Fig. 11. 22: Combo. Box. Test. java // Testing Combo. Box. Frame. import javax. swing. JFrame; public class Combo. Box. Test { public static void main( String args[] ) { Combo. Box. Frame combo. Box. Frame = new Combo. Box. Frame(); combo. Box. Frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); combo. Box. Frame. set. Size( 350, 150 ); // set frame size combo. Box. Frame. set. Visible( true ); // display frame } // end main } // end class Combo. Box. Test
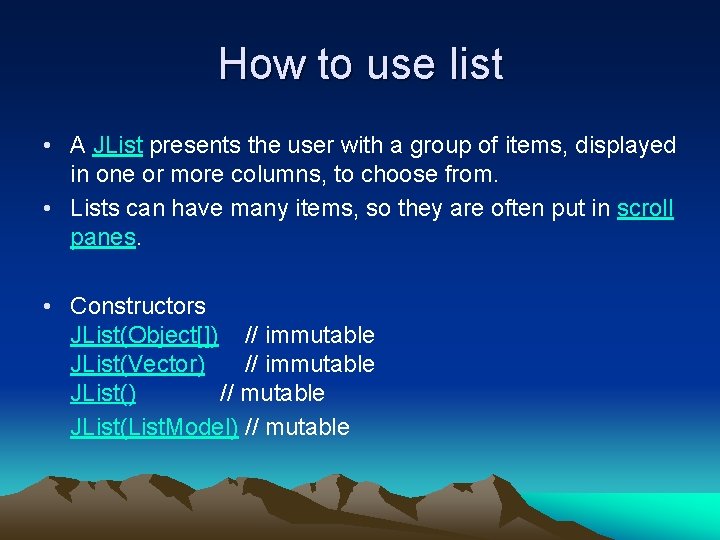
How to use list • A JList presents the user with a group of items, displayed in one or more columns, to choose from. • Lists can have many items, so they are often put in scroll panes. • Constructors JList(Object[]) // immutable JList(Vector) // immutable JList() // mutable JList(List. Model) // mutable
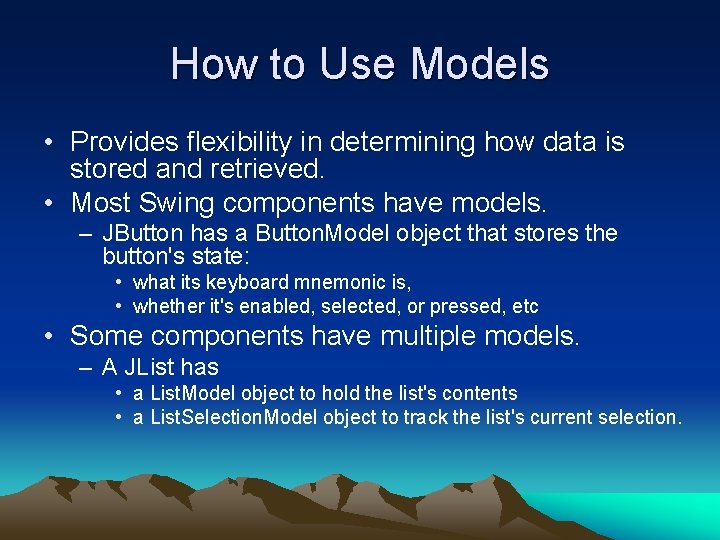
How to Use Models • Provides flexibility in determining how data is stored and retrieved. • Most Swing components have models. – JButton has a Button. Model object that stores the button's state: • what its keyboard mnemonic is, • whether it's enabled, selected, or pressed, etc • Some components have multiple models. – A JList has • a List. Model object to hold the list's contents • a List. Selection. Model object to track the list's current selection.
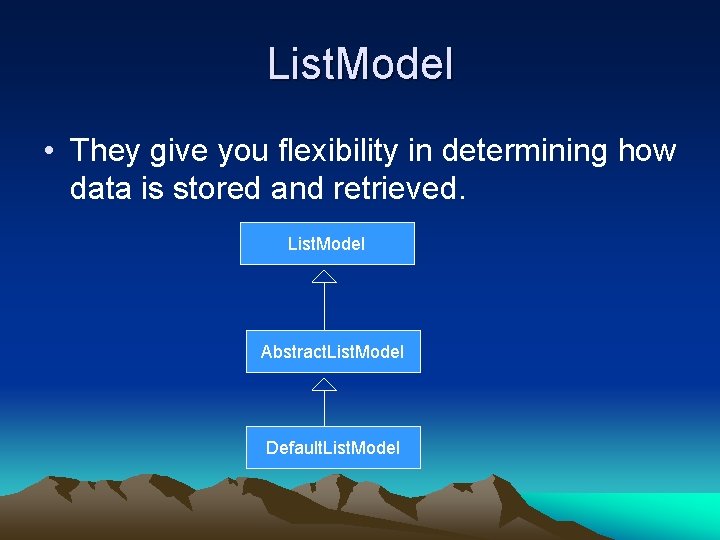
List. Model • They give you flexibility in determining how data is stored and retrieved. List. Model Abstract. List. Model Default. List. Model
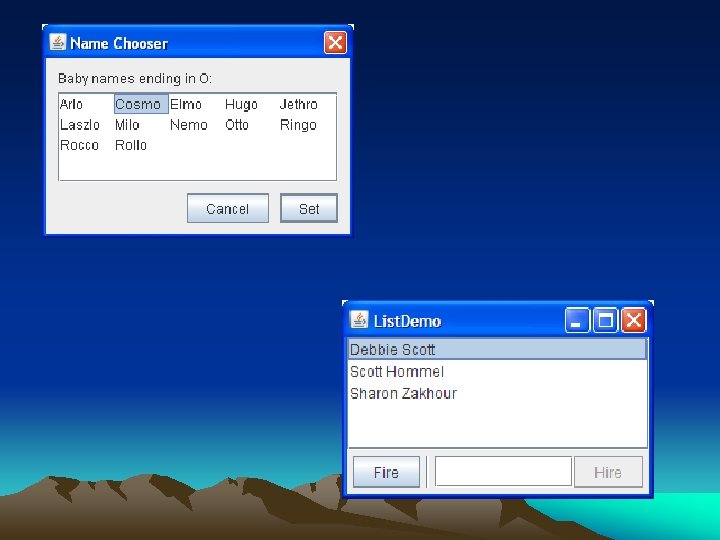
![String choices A long array of strings list new JListchoice list set String[] choices = {"A", "long", "array", "of", "strings"}; list = new JList(choice); list. set.](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-25.jpg)
String[] choices = {"A", "long", "array", "of", "strings"}; list = new JList(choice); list. set. Selection. Mode(List. Selection. Model. SINGLE_INTERVAL_SELECTION); list. set. Layout. Orientation(JList. HORIZONTAL_WRAP); JScroll. Pane list. Scroller = new JScroll. Pane(list); list. Scroller. set. Preferred. Size(new Dimension(250, 80));
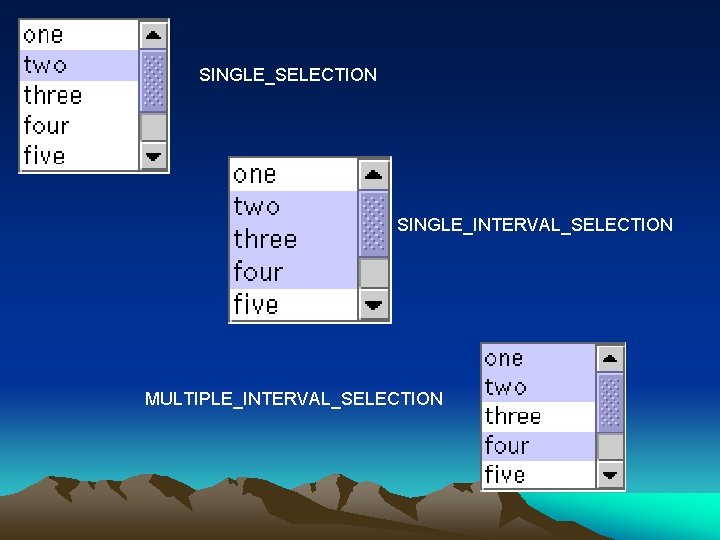
SINGLE_SELECTION SINGLE_INTERVAL_SELECTION MULTIPLE_INTERVAL_SELECTION
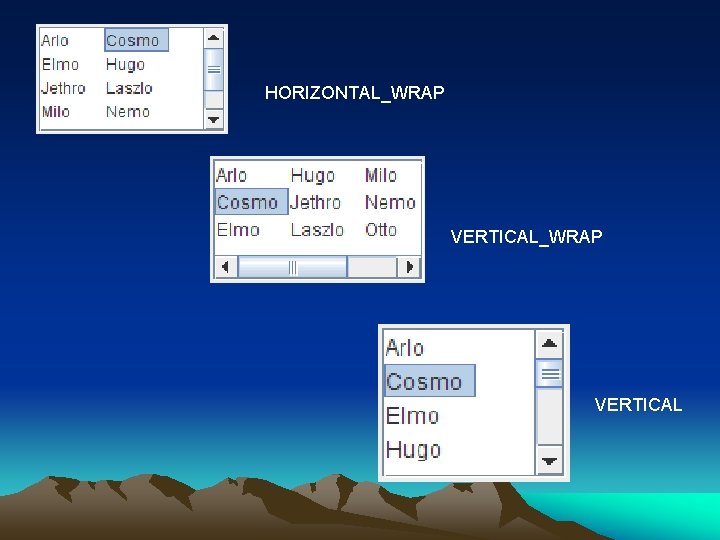
HORIZONTAL_WRAP VERTICAL
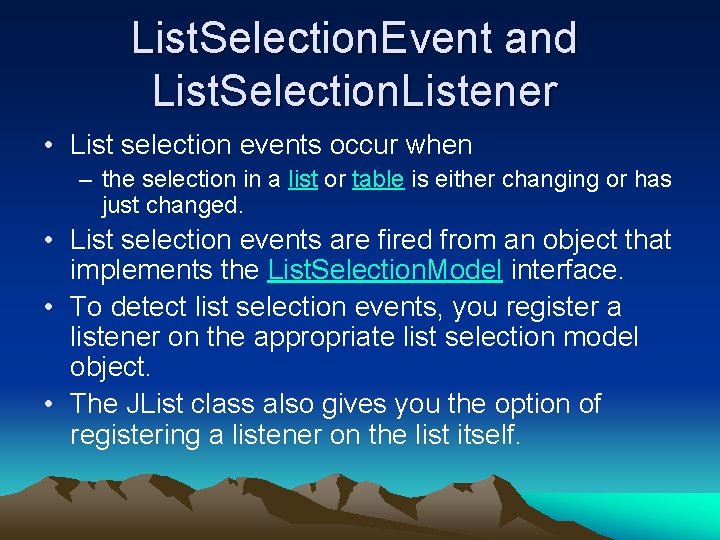
List. Selection. Event and List. Selection. Listener • List selection events occur when – the selection in a list or table is either changing or has just changed. • List selection events are fired from an object that implements the List. Selection. Model interface. • To detect list selection events, you register a listener on the appropriate list selection model object. • The JList class also gives you the option of registering a listener on the list itself.
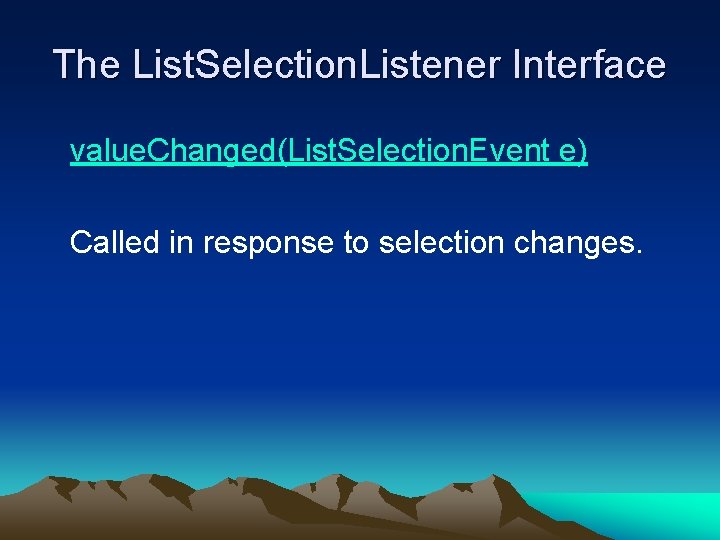
The List. Selection. Listener Interface value. Changed(List. Selection. Event e) Called in response to selection changes.
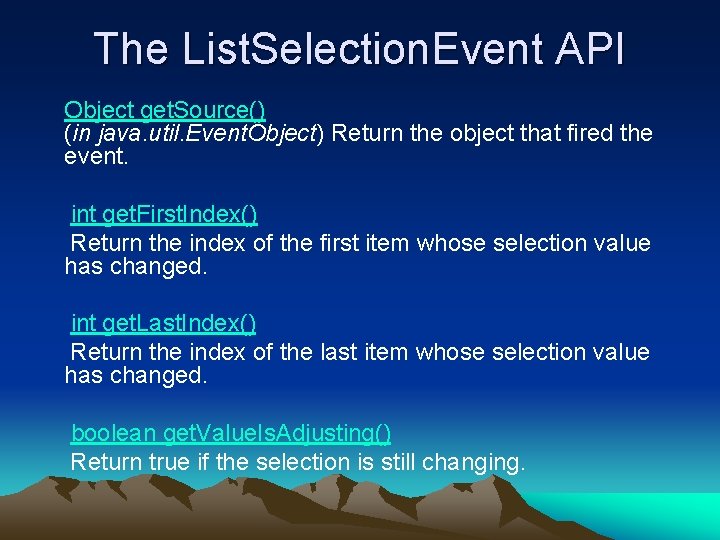
The List. Selection. Event API Object get. Source() (in java. util. Event. Object) Return the object that fired the event. int get. First. Index() Return the index of the first item whose selection value has changed. int get. Last. Index() Return the index of the last item whose selection value has changed. boolean get. Value. Is. Adjusting() Return true if the selection is still changing.
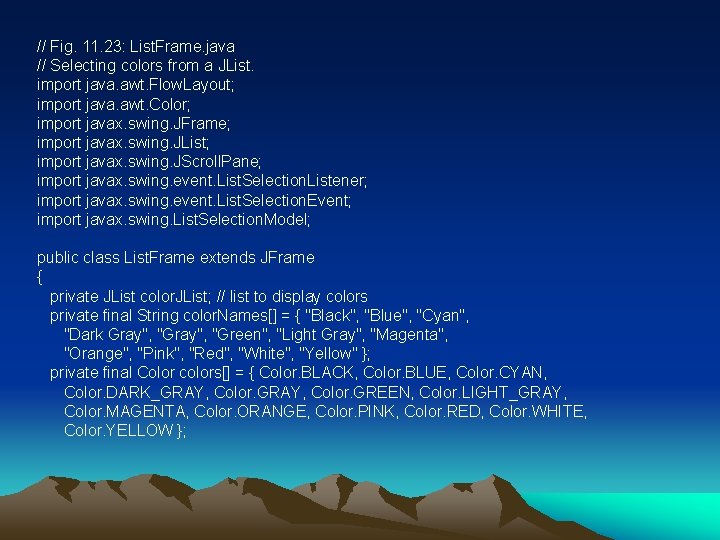
// Fig. 11. 23: List. Frame. java // Selecting colors from a JList. import java. awt. Flow. Layout; import java. awt. Color; import javax. swing. JFrame; import javax. swing. JList; import javax. swing. JScroll. Pane; import javax. swing. event. List. Selection. Listener; import javax. swing. event. List. Selection. Event; import javax. swing. List. Selection. Model; public class List. Frame extends JFrame { private JList color. JList; // list to display colors private final String color. Names[] = { "Black", "Blue", "Cyan", "Dark Gray", "Green", "Light Gray", "Magenta", "Orange", "Pink", "Red", "White", "Yellow" }; private final Color colors[] = { Color. BLACK, Color. BLUE, Color. CYAN, Color. DARK_GRAY, Color. GREEN, Color. LIGHT_GRAY, Color. MAGENTA, Color. ORANGE, Color. PINK, Color. RED, Color. WHITE, Color. YELLOW };
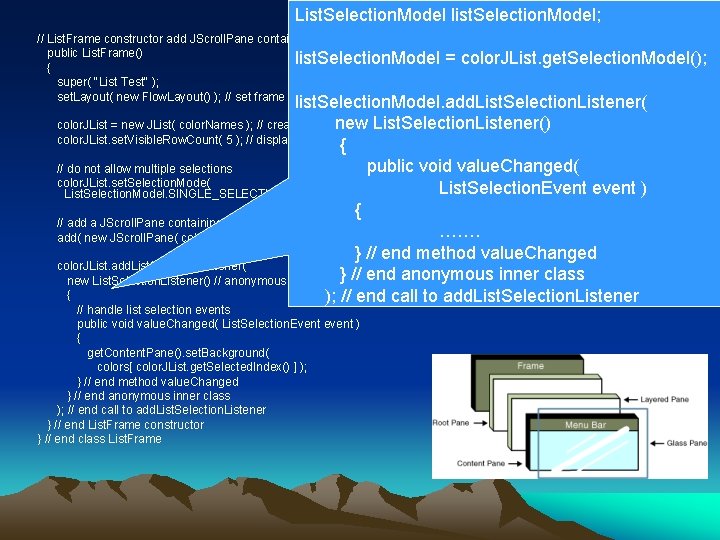
List. Selection. Model list. Selection. Model; // List. Frame constructor add JScroll. Pane containing JList to JFrame public List. Frame() list. Selection. Model { super( "List Test" ); set. Layout( new Flow. Layout() ); // set frame layout = color. JList. get. Selection. Model(); list. Selection. Model. add. List. Selection. Listener( new List. Selection. Listener() color. JList = new JList( color. Names ); // create with color. Names color. JList. set. Visible. Row. Count( 5 ); // display five rows at once { public void value. Changed( // do not allow multiple selections color. JList. set. Selection. Mode( List. Selection. Event event ) List. Selection. Model. SINGLE_SELECTION ); { // add a JScroll. Pane containing JList to frame ……. add( new JScroll. Pane( color. JList ) ); } // end method value. Changed color. JList. add. List. Selection. Listener( } // end anonymous inner class new List. Selection. Listener() // anonymous inner class { ); // end call to add. List. Selection. Listener // handle list selection events public void value. Changed( List. Selection. Event event ) { get. Content. Pane(). set. Background( colors[ color. JList. get. Selected. Index() ] ); } // end method value. Changed } // end anonymous inner class ); // end call to add. List. Selection. Listener } // end List. Frame constructor } // end class List. Frame
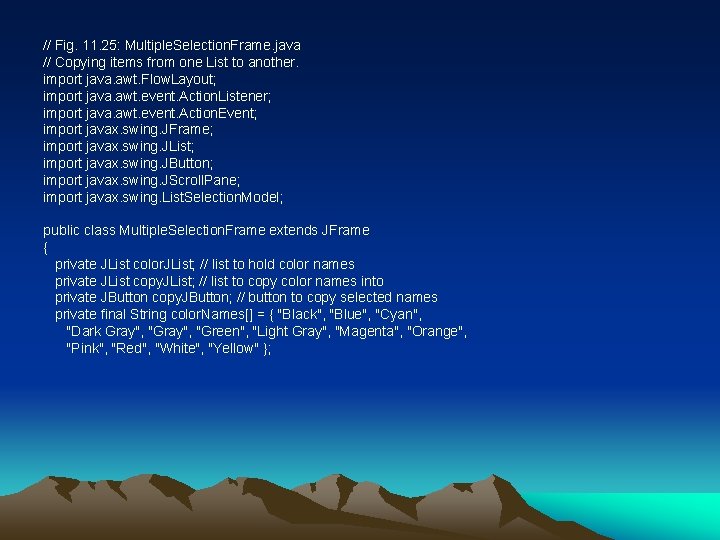
// Fig. 11. 25: Multiple. Selection. Frame. java // Copying items from one List to another. import java. awt. Flow. Layout; import java. awt. event. Action. Listener; import java. awt. event. Action. Event; import javax. swing. JFrame; import javax. swing. JList; import javax. swing. JButton; import javax. swing. JScroll. Pane; import javax. swing. List. Selection. Model; public class Multiple. Selection. Frame extends JFrame { private JList color. JList; // list to hold color names private JList copy. JList; // list to copy color names into private JButton copy. JButton; // button to copy selected names private final String color. Names[] = { "Black", "Blue", "Cyan", "Dark Gray", "Green", "Light Gray", "Magenta", "Orange", "Pink", "Red", "White", "Yellow" };
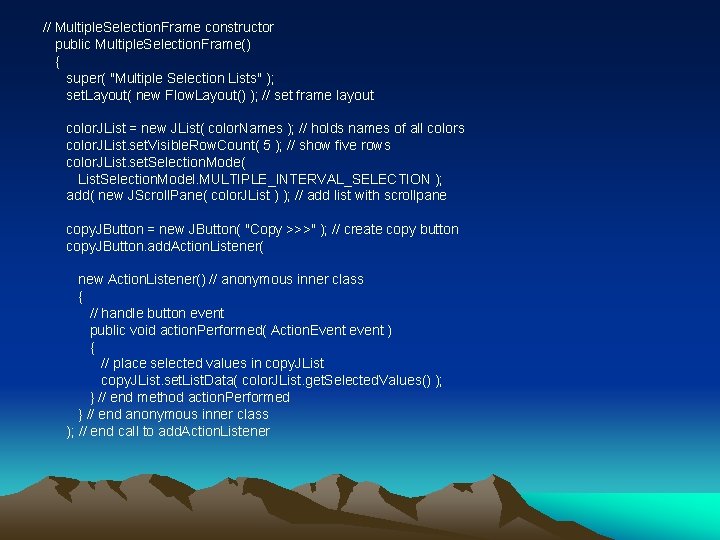
// Multiple. Selection. Frame constructor public Multiple. Selection. Frame() { super( "Multiple Selection Lists" ); set. Layout( new Flow. Layout() ); // set frame layout color. JList = new JList( color. Names ); // holds names of all colors color. JList. set. Visible. Row. Count( 5 ); // show five rows color. JList. set. Selection. Mode( List. Selection. Model. MULTIPLE_INTERVAL_SELECTION ); add( new JScroll. Pane( color. JList ) ); // add list with scrollpane copy. JButton = new JButton( "Copy >>>" ); // create copy button copy. JButton. add. Action. Listener( new Action. Listener() // anonymous inner class { // handle button event public void action. Performed( Action. Event event ) { // place selected values in copy. JList. set. List. Data( color. JList. get. Selected. Values() ); } // end method action. Performed } // end anonymous inner class ); // end call to add. Action. Listener
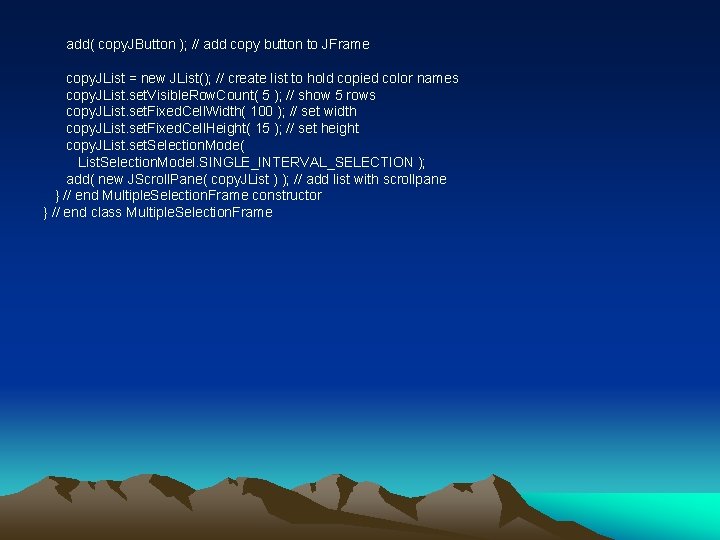
add( copy. JButton ); // add copy button to JFrame copy. JList = new JList(); // create list to hold copied color names copy. JList. set. Visible. Row. Count( 5 ); // show 5 rows copy. JList. set. Fixed. Cell. Width( 100 ); // set width copy. JList. set. Fixed. Cell. Height( 15 ); // set height copy. JList. set. Selection. Mode( List. Selection. Model. SINGLE_INTERVAL_SELECTION ); add( new JScroll. Pane( copy. JList ) ); // add list with scrollpane } // end Multiple. Selection. Frame constructor } // end class Multiple. Selection. Frame
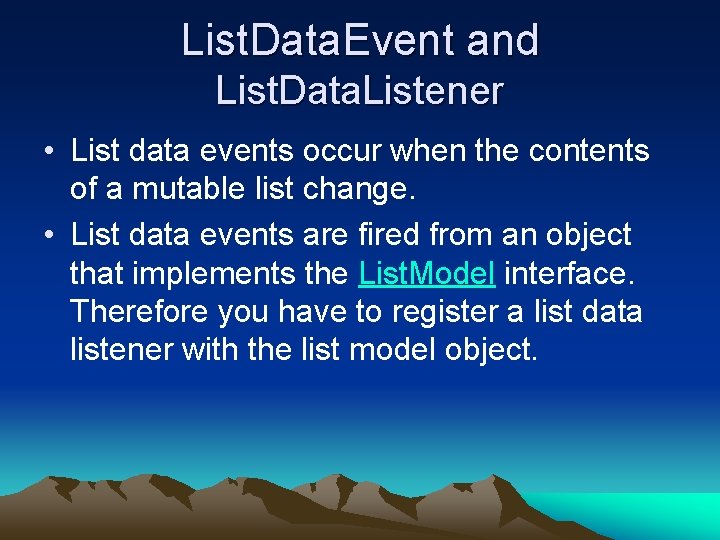
List. Data. Event and List. Data. Listener • List data events occur when the contents of a mutable list change. • List data events are fired from an object that implements the List. Model interface. Therefore you have to register a list data listener with the list model object.
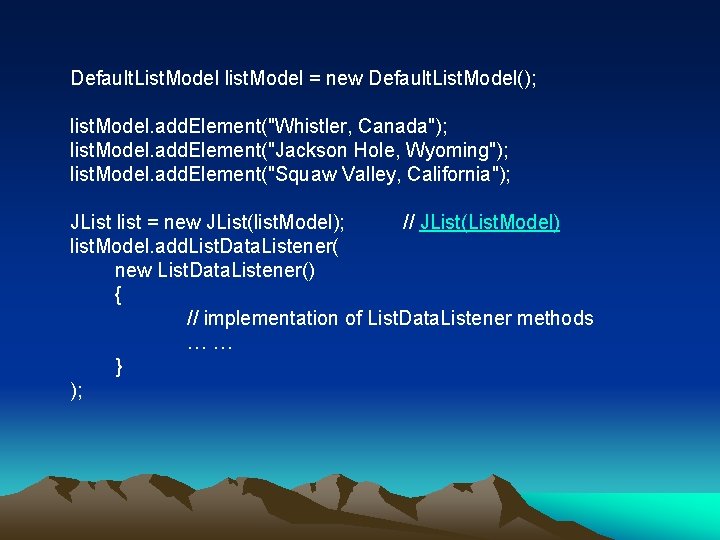
Default. List. Model list. Model = new Default. List. Model(); list. Model. add. Element("Whistler, Canada"); list. Model. add. Element("Jackson Hole, Wyoming"); list. Model. add. Element("Squaw Valley, California"); JList list = new JList(list. Model); // JList(List. Model) list. Model. add. List. Data. Listener( new List. Data. Listener() { // implementation of List. Data. Listener methods …… } );
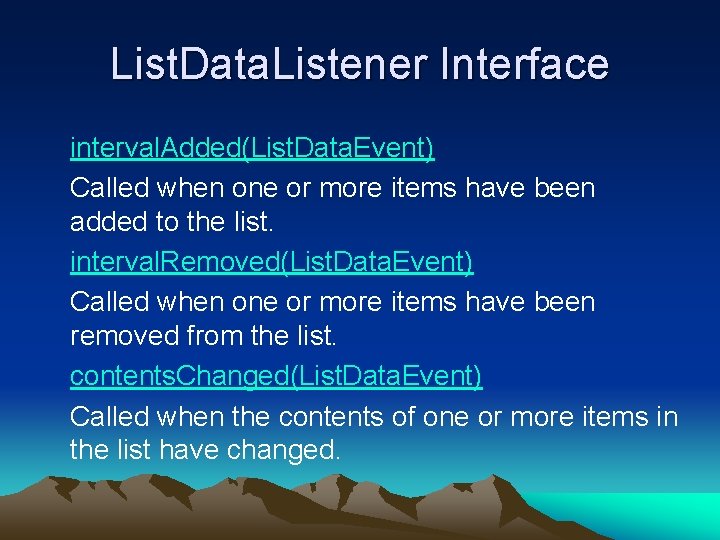
List. Data. Listener Interface interval. Added(List. Data. Event) Called when one or more items have been added to the list. interval. Removed(List. Data. Event) Called when one or more items have been removed from the list. contents. Changed(List. Data. Event) Called when the contents of one or more items in the list have changed.
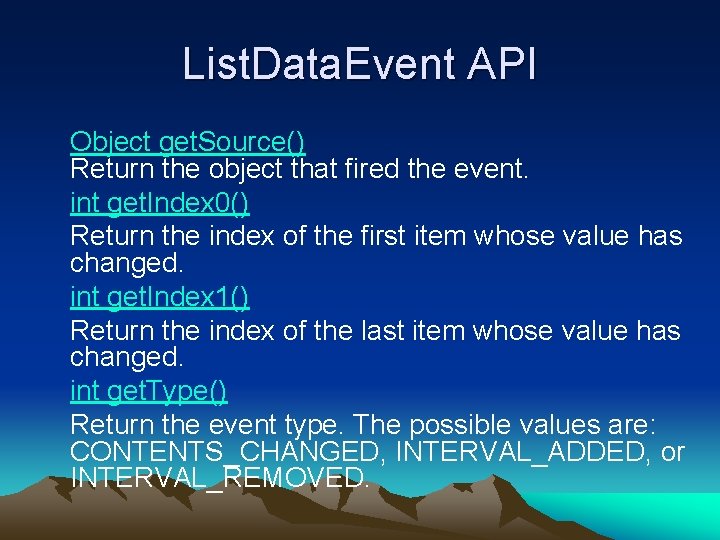
List. Data. Event API Object get. Source() Return the object that fired the event. int get. Index 0() Return the index of the first item whose value has changed. int get. Index 1() Return the index of the last item whose value has changed. int get. Type() Return the event type. The possible values are: CONTENTS_CHANGED, INTERVAL_ADDED, or INTERVAL_REMOVED.
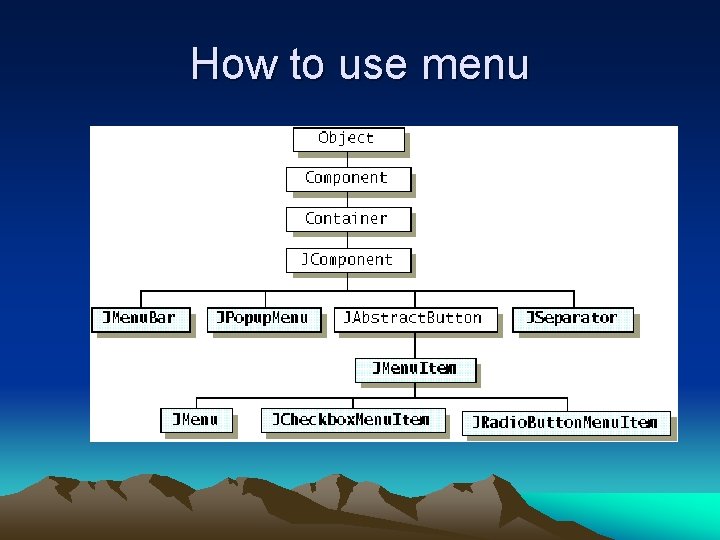
How to use menu
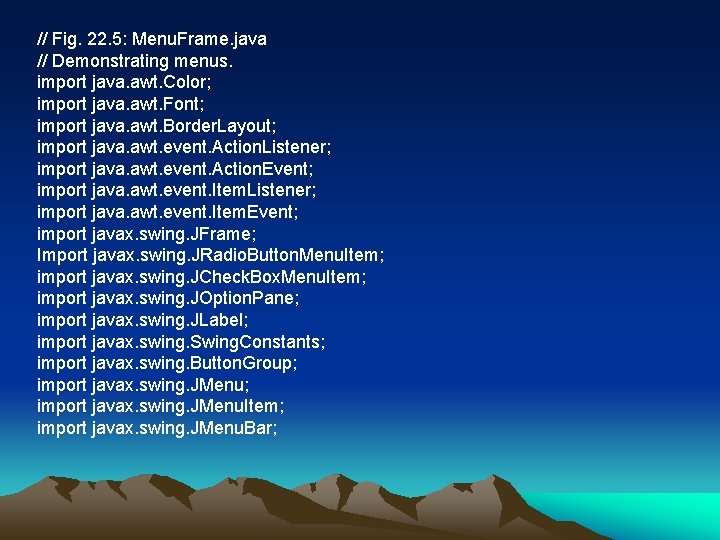
// Fig. 22. 5: Menu. Frame. java // Demonstrating menus. import java. awt. Color; import java. awt. Font; import java. awt. Border. Layout; import java. awt. event. Action. Listener; import java. awt. event. Action. Event; import java. awt. event. Item. Listener; import java. awt. event. Item. Event; import javax. swing. JFrame; Import javax. swing. JRadio. Button. Menu. Item; import javax. swing. JCheck. Box. Menu. Item; import javax. swing. JOption. Pane; import javax. swing. JLabel; import javax. swing. Swing. Constants; import javax. swing. Button. Group; import javax. swing. JMenu. Item; import javax. swing. JMenu. Bar;
![public class Menu Frame extends JFrame private final Color color Values public class Menu. Frame extends JFrame { private final Color color. Values[] = {](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-42.jpg)
public class Menu. Frame extends JFrame { private final Color color. Values[] = { Color. BLACK, Color. BLUE, Color. RED, Color. GREEN }; private JRadio. Button. Menu. Item color. Items[]; // color menu items private JRadio. Button. Menu. Item fonts[]; // font menu items private JCheck. Box. Menu. Item style. Items[]; // font style menu items private JLabel display. JLabel; // displays sample text private Button. Group font. Button. Group; // manages font menu items private Button. Group color. Button. Group; // manages color menu items private int style; // used to create style for font
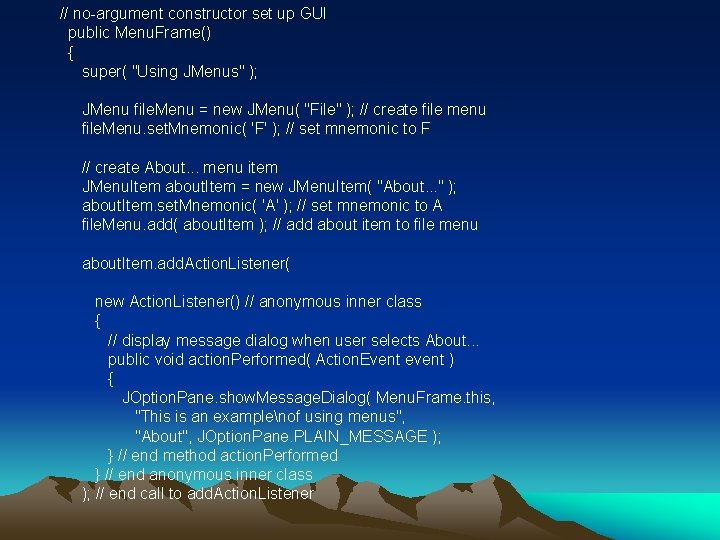
// no-argument constructor set up GUI public Menu. Frame() { super( "Using JMenus" ); JMenu file. Menu = new JMenu( "File" ); // create file menu file. Menu. set. Mnemonic( 'F' ); // set mnemonic to F // create About. . . menu item JMenu. Item about. Item = new JMenu. Item( "About. . . " ); about. Item. set. Mnemonic( 'A' ); // set mnemonic to A file. Menu. add( about. Item ); // add about item to file menu about. Item. add. Action. Listener( new Action. Listener() // anonymous inner class { // display message dialog when user selects About. . . public void action. Performed( Action. Event event ) { JOption. Pane. show. Message. Dialog( Menu. Frame. this, "This is an examplenof using menus", "About", JOption. Pane. PLAIN_MESSAGE ); } // end method action. Performed } // end anonymous inner class ); // end call to add. Action. Listener
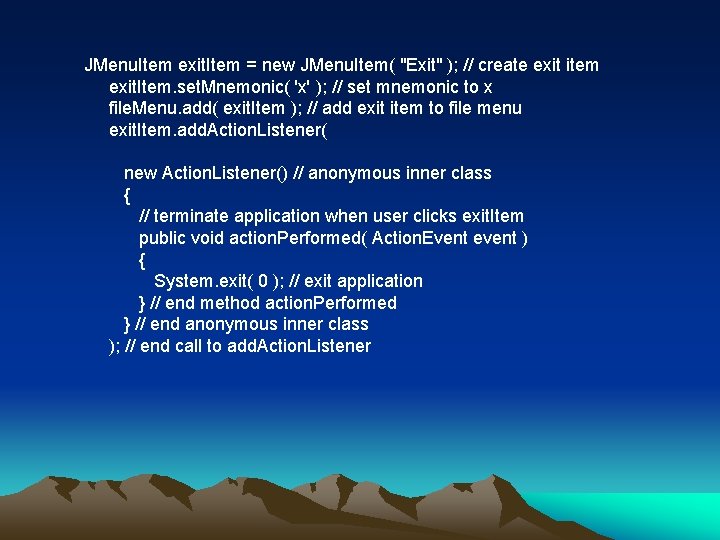
JMenu. Item exit. Item = new JMenu. Item( "Exit" ); // create exit item exit. Item. set. Mnemonic( 'x' ); // set mnemonic to x file. Menu. add( exit. Item ); // add exit item to file menu exit. Item. add. Action. Listener( new Action. Listener() // anonymous inner class { // terminate application when user clicks exit. Item public void action. Performed( Action. Event event ) { System. exit( 0 ); // exit application } // end method action. Performed } // end anonymous inner class ); // end call to add. Action. Listener
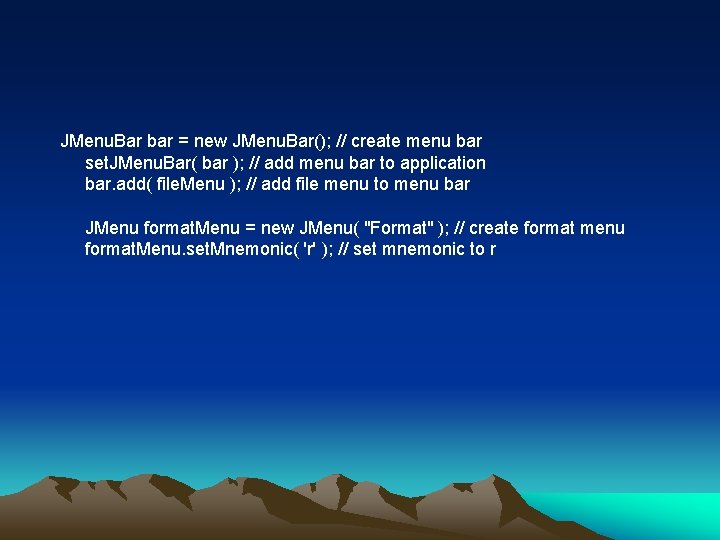
JMenu. Bar bar = new JMenu. Bar(); // create menu bar set. JMenu. Bar( bar ); // add menu bar to application bar. add( file. Menu ); // add file menu to menu bar JMenu format. Menu = new JMenu( "Format" ); // create format menu format. Menu. set. Mnemonic( 'r' ); // set mnemonic to r
![array listing string colors String colors Black Blue Red Green // array listing string colors String colors[] = { "Black", "Blue", "Red", "Green" };](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-46.jpg)
// array listing string colors String colors[] = { "Black", "Blue", "Red", "Green" }; JMenu color. Menu = new JMenu( "Color" ); // create color menu color. Menu. set. Mnemonic( 'C' ); // set mnemonic to C // create radiobutton menu items for colors color. Items = new JRadio. Button. Menu. Item[ colors. length ]; color. Button. Group = new Button. Group(); // manages colors Item. Handler item. Handler = new Item. Handler(); // handler for colors // create color radio button menu items for ( int count = 0; count < colors. length; count++ ) { color. Items[ count ] = new JRadio. Button. Menu. Item( colors[ count ] ); // create item color. Menu. add( color. Items[ count ] ); // add item to color menu color. Button. Group. add( color. Items[ count ] ); // add to group color. Items[ count ]. add. Action. Listener( item. Handler ); } // end for color. Items[ 0 ]. set. Selected( true ); // select first Color item format. Menu. add( color. Menu ); // add color menu to format menu format. Menu. add. Separator(); // add separator in menu
![array listing font names String font Names Serif Monospaced Sans Serif // array listing font names String font. Names[] = { "Serif", "Monospaced", "Sans. Serif"](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-47.jpg)
// array listing font names String font. Names[] = { "Serif", "Monospaced", "Sans. Serif" }; JMenu font. Menu = new JMenu( "Font" ); // create font menu font. Menu. set. Mnemonic( 'n' ); // set mnemonic to n // create radiobutton menu items for font names fonts = new JRadio. Button. Menu. Item[ font. Names. length ]; font. Button. Group = new Button. Group(); // manages font names // create Font radio button menu items for ( int count = 0; count < fonts. length; count++ ) { fonts[ count ] = new JRadio. Button. Menu. Item( font. Names[ count ] ); font. Menu. add( fonts[ count ] ); // add font to font menu font. Button. Group. add( fonts[ count ] ); // add to button group fonts[ count ]. add. Action. Listener( item. Handler ); // add handler } // end for fonts[ 0 ]. set. Selected( true ); // select first Font menu item font. Menu. add. Separator(); // add separator bar to font menu
![String style Names Bold Italic names of styles style Items String style. Names[] = { "Bold", "Italic" }; // names of styles style. Items](https://slidetodoc.com/presentation_image_h2/84ae8c2d5787fa11143ba07acac00971/image-48.jpg)
String style. Names[] = { "Bold", "Italic" }; // names of styles style. Items = new JCheck. Box. Menu. Item[ style. Names. length ]; Style. Handler style. Handler = new Style. Handler(); // style handler // create style checkbox menu items for ( int count = 0; count < style. Names. length; count++ ) { style. Items[ count ] = new JCheck. Box. Menu. Item( style. Names[ count ] ); // for style font. Menu. add( style. Items[ count ] ); // add to font menu style. Items[ count ]. add. Item. Listener( style. Handler ); // handler } // end format. Menu. add( font. Menu ); // add Font menu to Format menu bar. add( format. Menu ); // add Format menu to menu bar
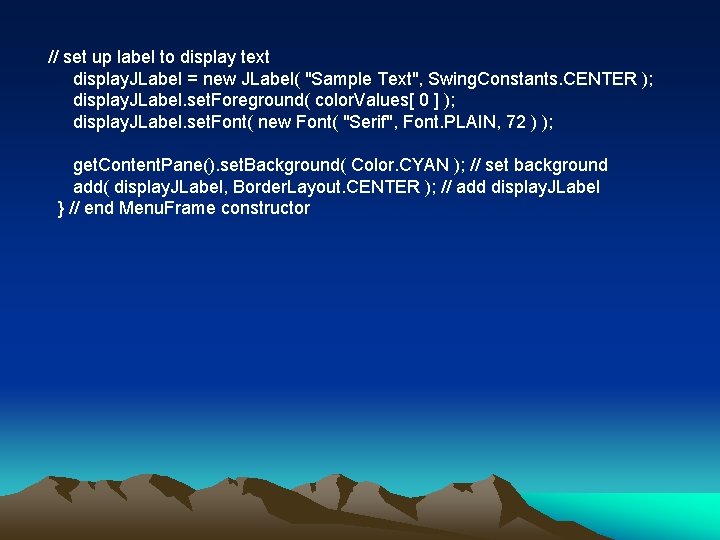
// set up label to display text display. JLabel = new JLabel( "Sample Text", Swing. Constants. CENTER ); display. JLabel. set. Foreground( color. Values[ 0 ] ); display. JLabel. set. Font( new Font( "Serif", Font. PLAIN, 72 ) ); get. Content. Pane(). set. Background( Color. CYAN ); // set background add( display. JLabel, Border. Layout. CENTER ); // add display. JLabel } // end Menu. Frame constructor
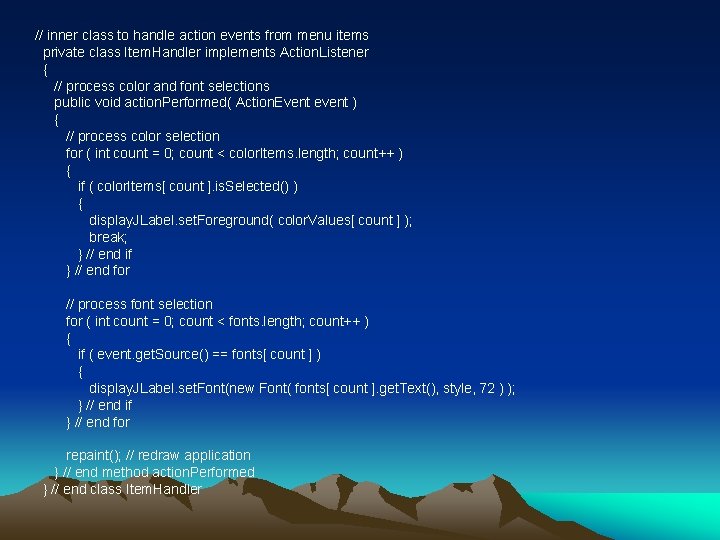
// inner class to handle action events from menu items private class Item. Handler implements Action. Listener { // process color and font selections public void action. Performed( Action. Event event ) { // process color selection for ( int count = 0; count < color. Items. length; count++ ) { if ( color. Items[ count ]. is. Selected() ) { display. JLabel. set. Foreground( color. Values[ count ] ); break; } // end if } // end for // process font selection for ( int count = 0; count < fonts. length; count++ ) { if ( event. get. Source() == fonts[ count ] ) { display. JLabel. set. Font(new Font( fonts[ count ]. get. Text(), style, 72 ) ); } // end if } // end for repaint(); // redraw application } // end method action. Performed } // end class Item. Handler
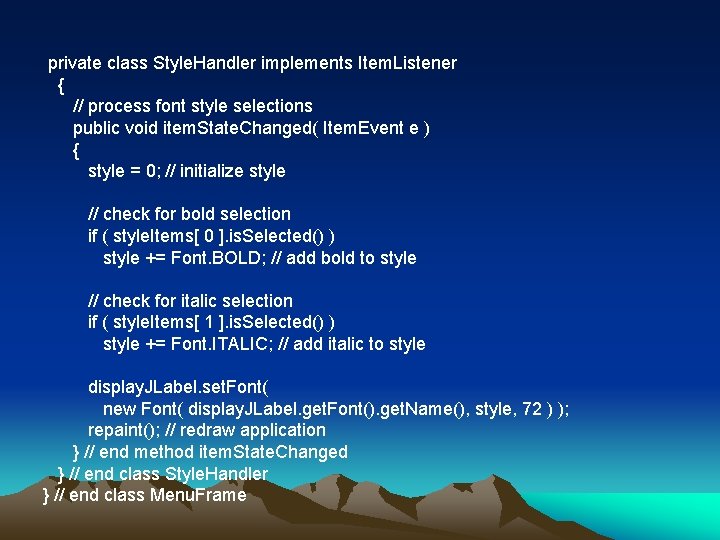
private class Style. Handler implements Item. Listener { // process font style selections public void item. State. Changed( Item. Event e ) { style = 0; // initialize style // check for bold selection if ( style. Items[ 0 ]. is. Selected() ) style += Font. BOLD; // add bold to style // check for italic selection if ( style. Items[ 1 ]. is. Selected() ) style += Font. ITALIC; // add italic to style display. JLabel. set. Font( new Font( display. JLabel. get. Font(). get. Name(), style, 72 ) ); repaint(); // redraw application } // end method item. State. Changed } // end class Style. Handler } // end class Menu. Frame
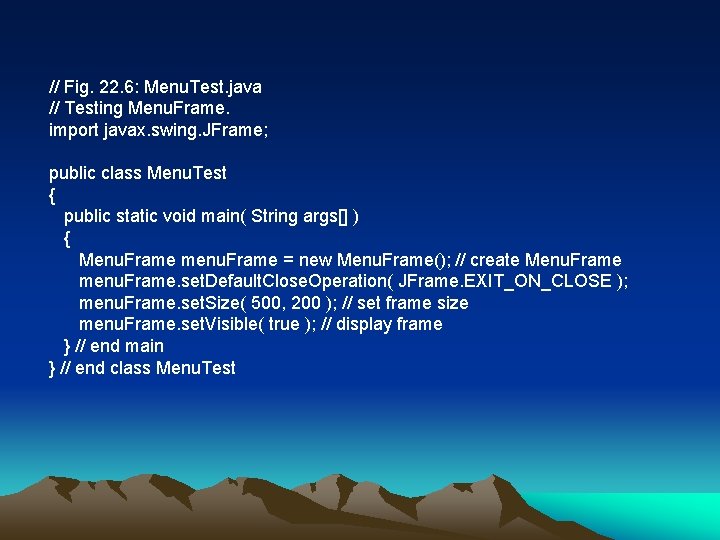
// Fig. 22. 6: Menu. Test. java // Testing Menu. Frame. import javax. swing. JFrame; public class Menu. Test { public static void main( String args[] ) { Menu. Frame menu. Frame = new Menu. Frame(); // create Menu. Frame menu. Frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); menu. Frame. set. Size( 500, 200 ); // set frame size menu. Frame. set. Visible( true ); // display frame } // end main } // end class Menu. Test
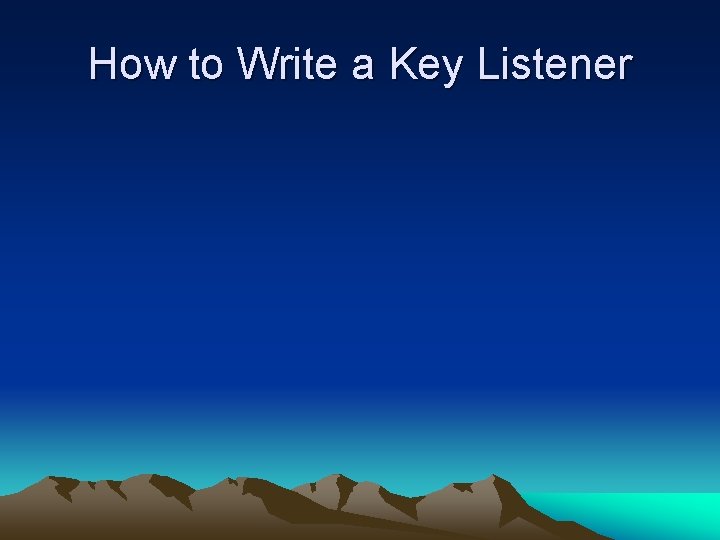
How to Write a Key Listener
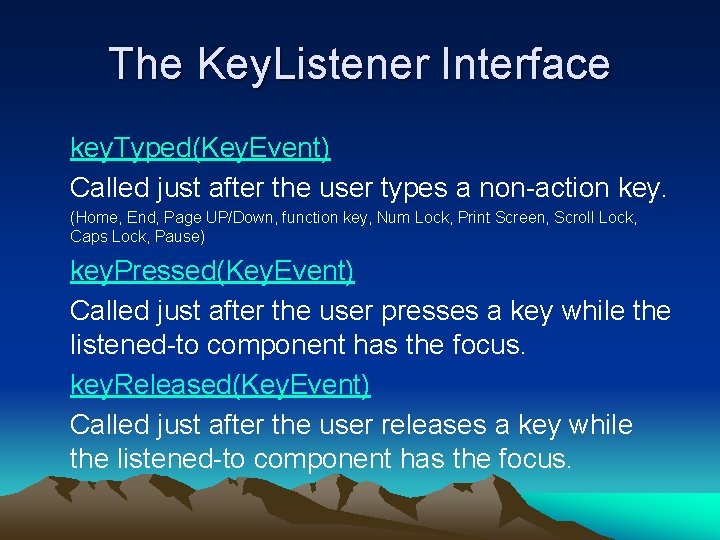
The Key. Listener Interface key. Typed(Key. Event) Called just after the user types a non-action key. (Home, End, Page UP/Down, function key, Num Lock, Print Screen, Scroll Lock, Caps Lock, Pause) key. Pressed(Key. Event) Called just after the user presses a key while the listened-to component has the focus. key. Released(Key. Event) Called just after the user releases a key while the listened-to component has the focus.
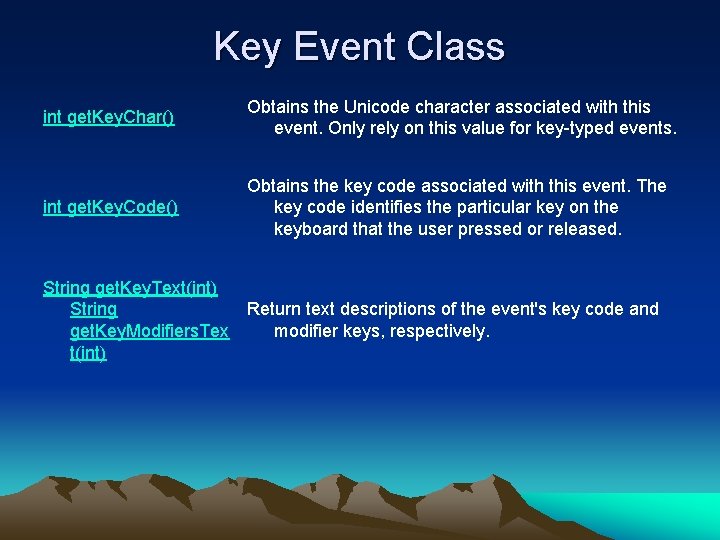
Key Event Class int get. Key. Char() Obtains the Unicode character associated with this event. Only rely on this value for key-typed events. int get. Key. Code() Obtains the key code associated with this event. The key code identifies the particular key on the keyboard that the user pressed or released. String get. Key. Text(int) String Return text descriptions of the event's key code and get. Key. Modifiers. Tex modifier keys, respectively. t(int)
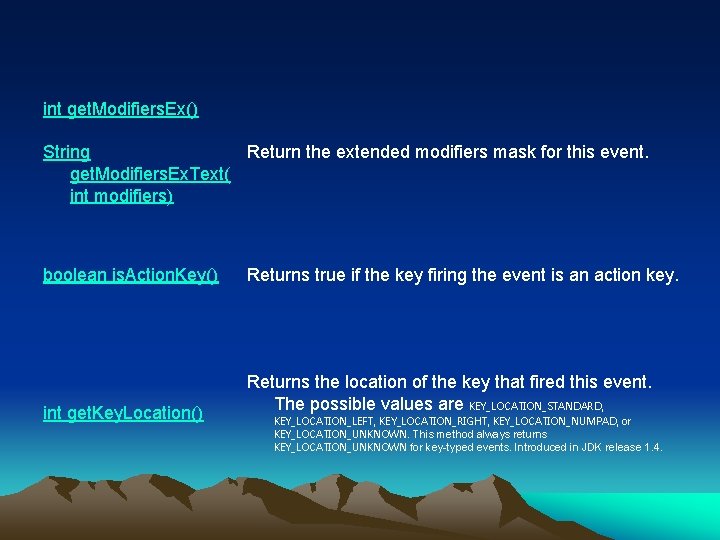
int get. Modifiers. Ex() String Return the extended modifiers mask for this event. get. Modifiers. Ex. Text( int modifiers) boolean is. Action. Key() Returns true if the key firing the event is an action key. int get. Key. Location() Returns the location of the key that fired this event. The possible values are KEY_LOCATION_STANDARD, KEY_LOCATION_LEFT, KEY_LOCATION_RIGHT, KEY_LOCATION_NUMPAD, or KEY_LOCATION_UNKNOWN. This method always returns KEY_LOCATION_UNKNOWN for key-typed events. Introduced in JDK release 1. 4.