INTRODUCTION TO PYTHON Rashid Ahmad Department of Physics
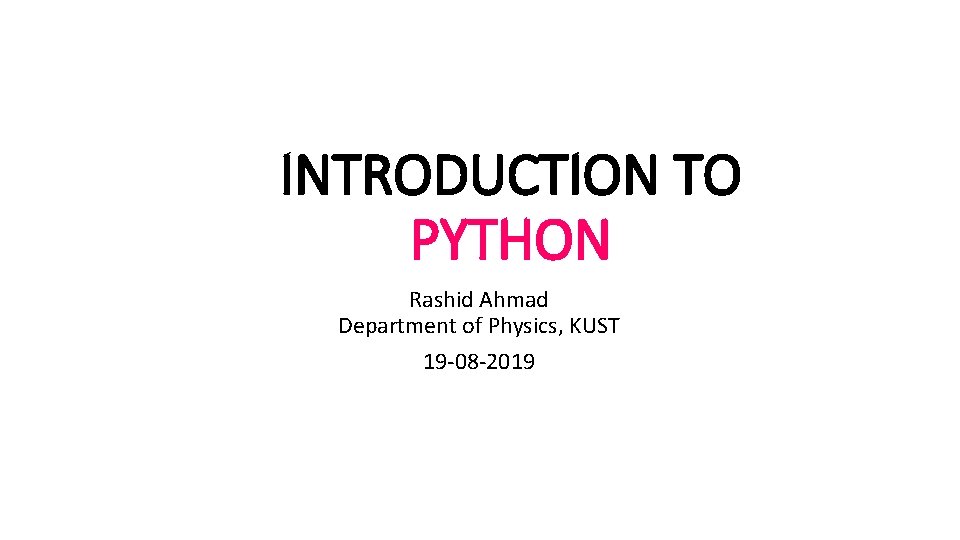
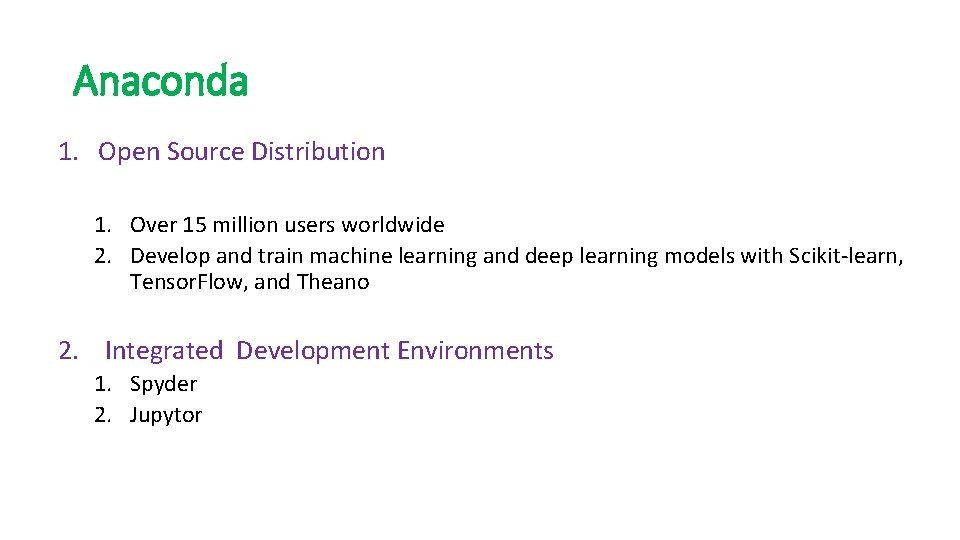
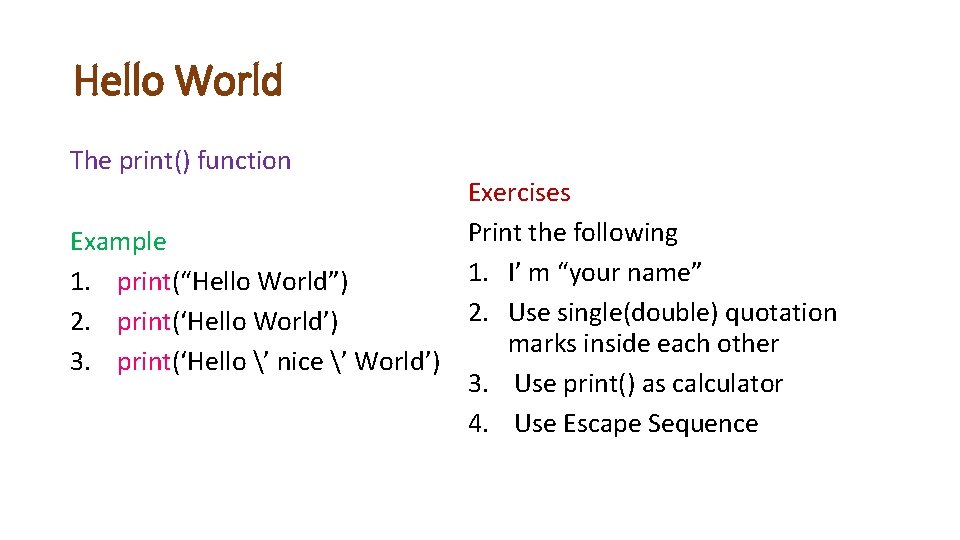
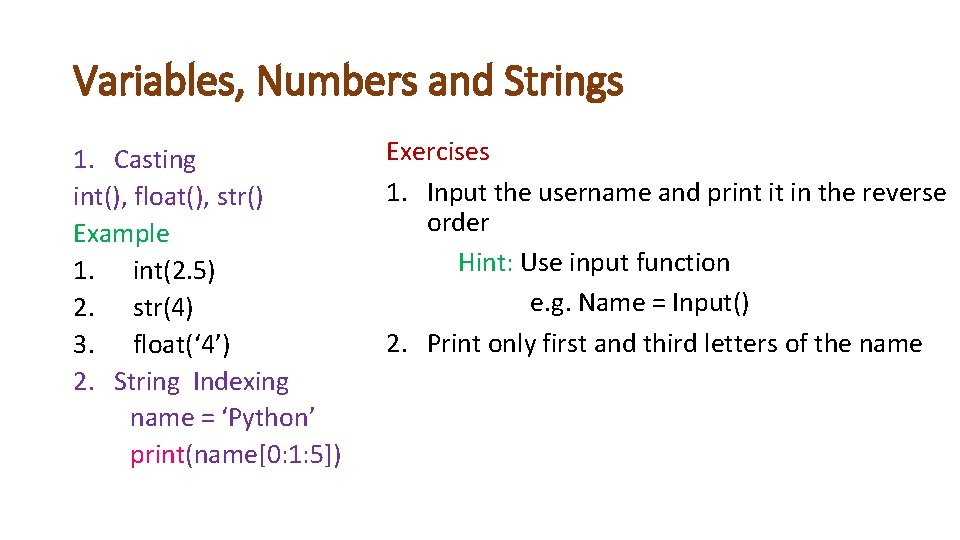
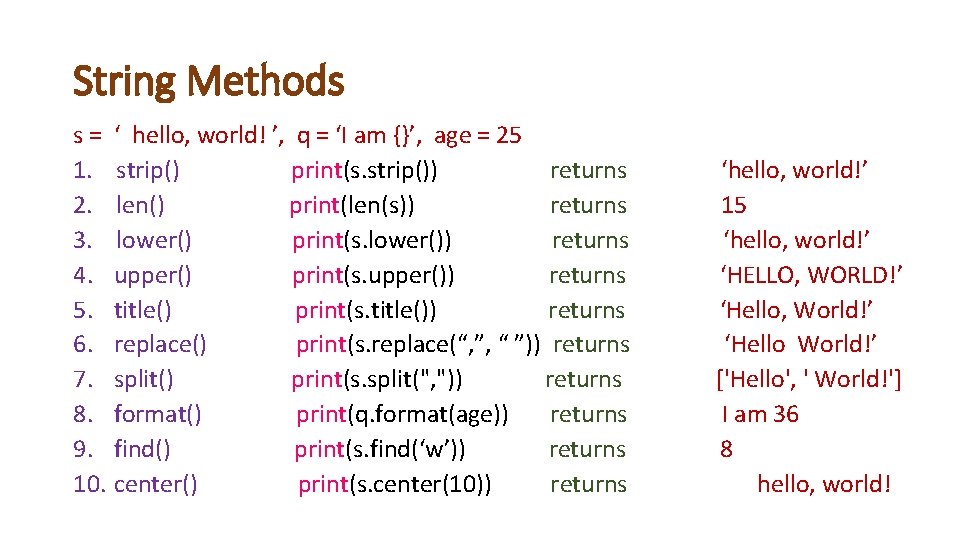
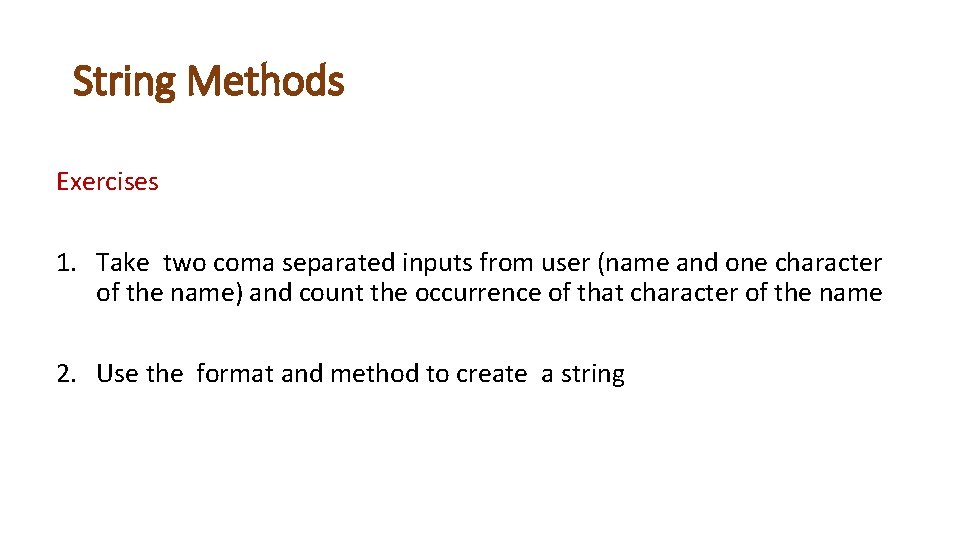
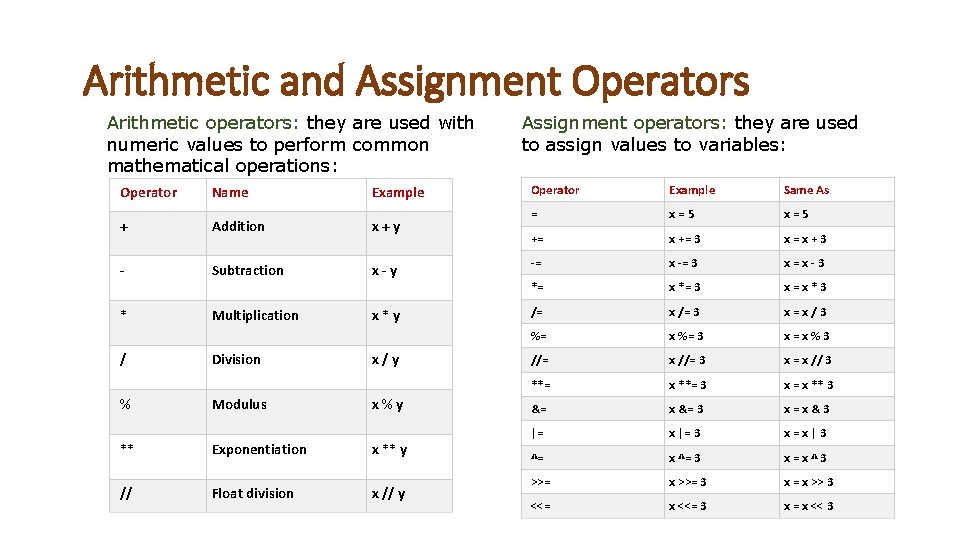
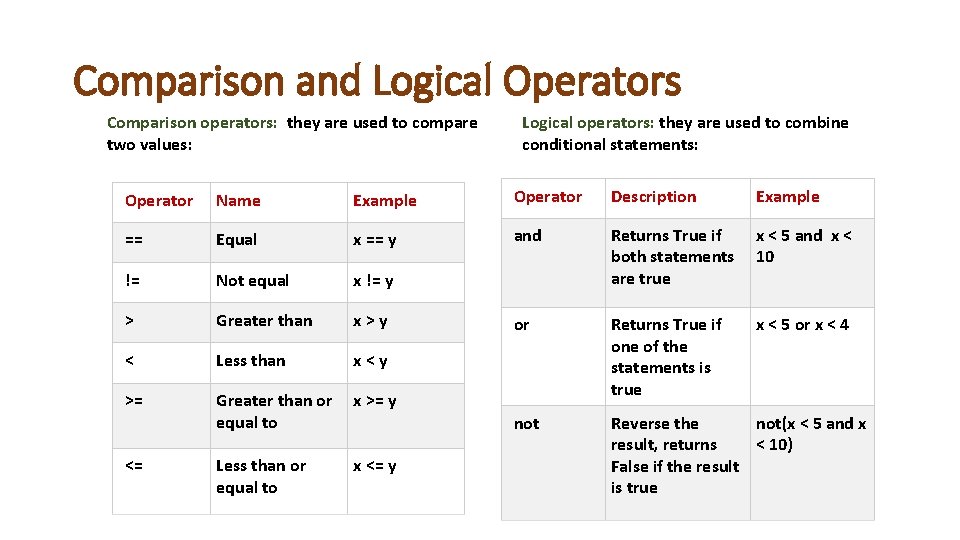
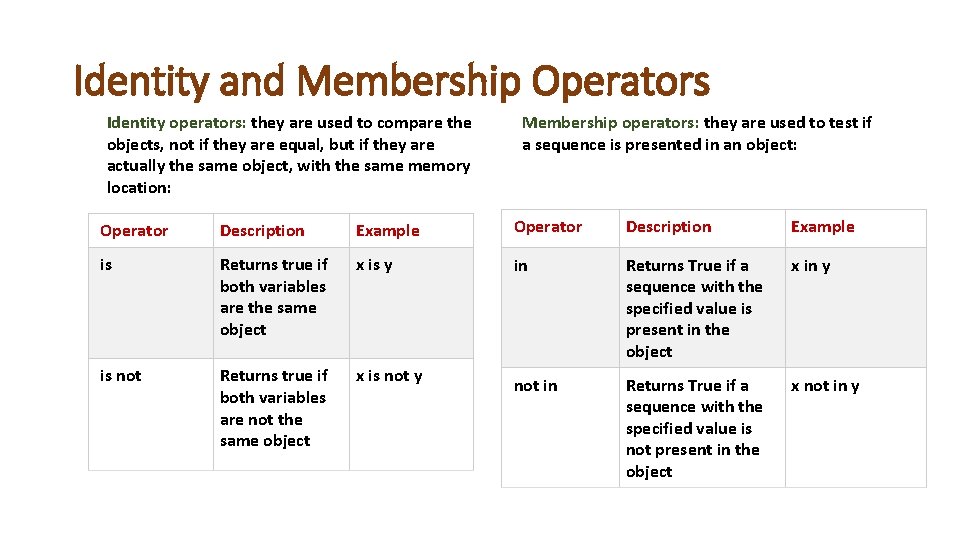
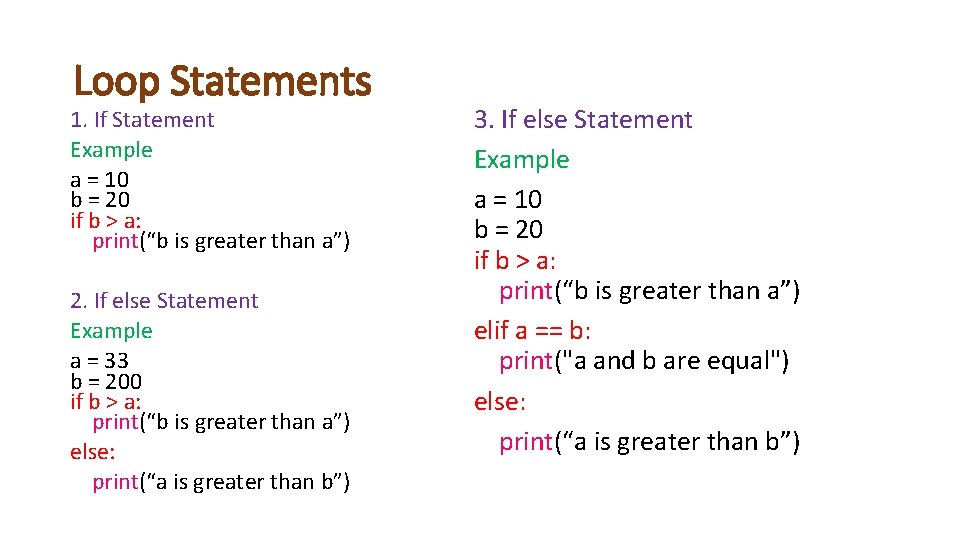
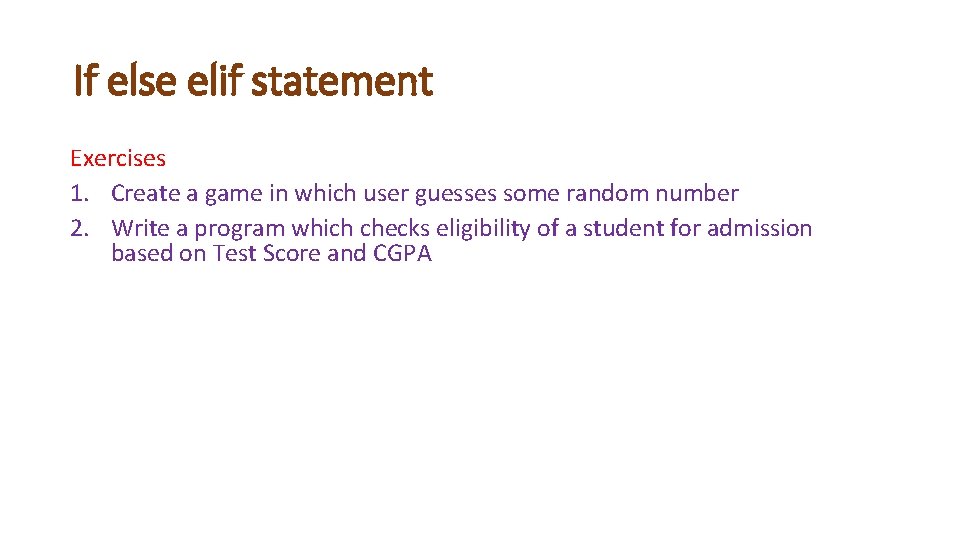
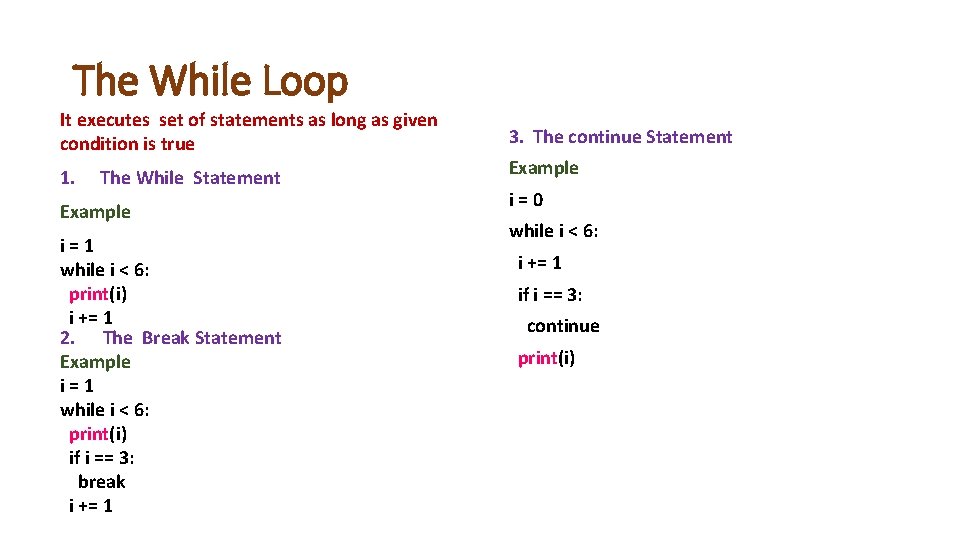
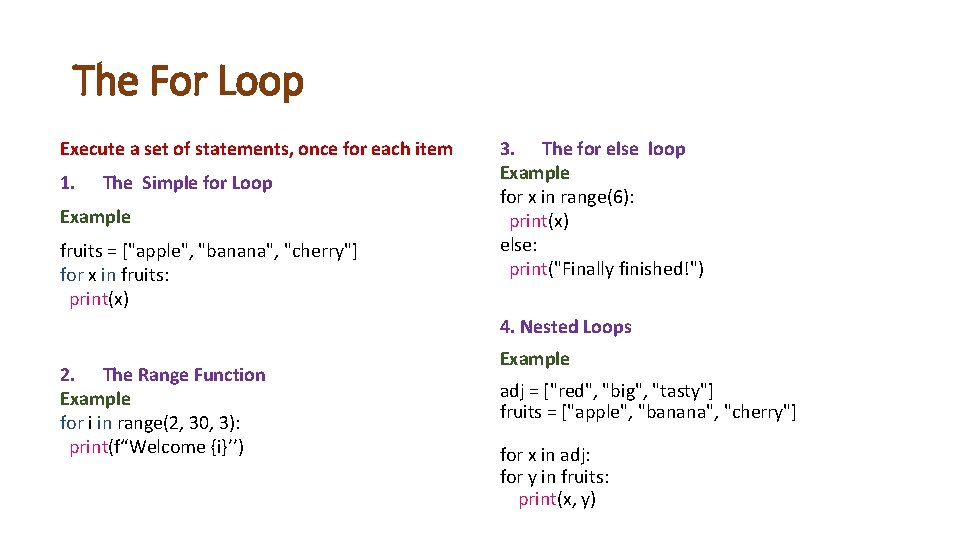
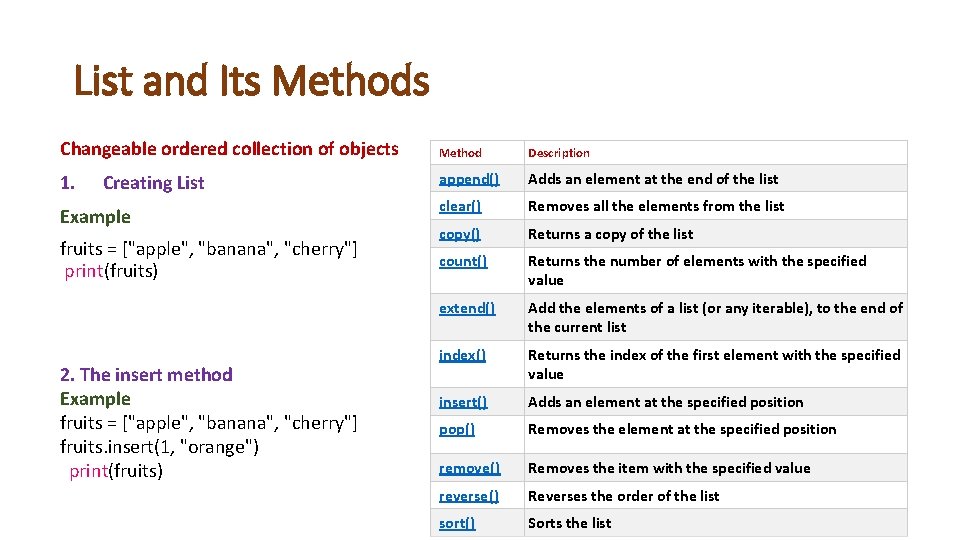
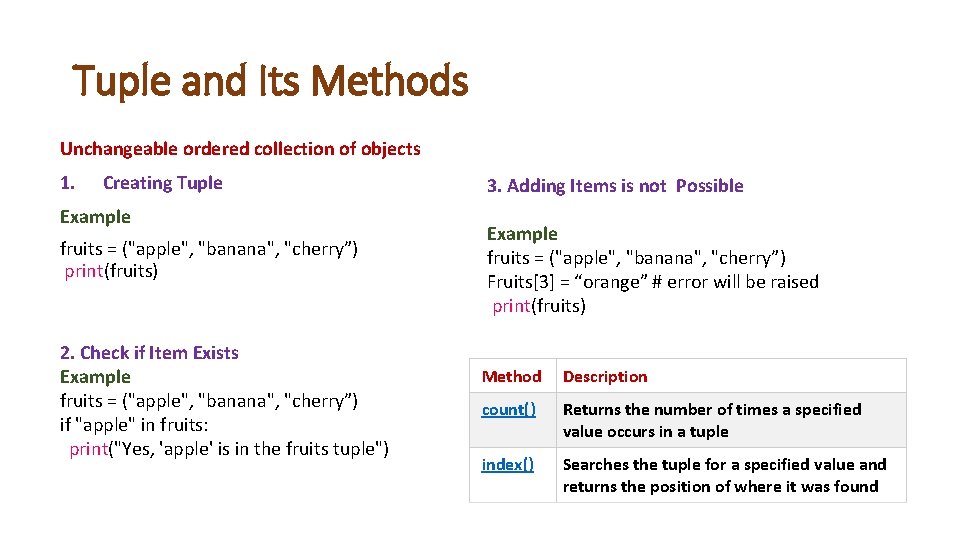
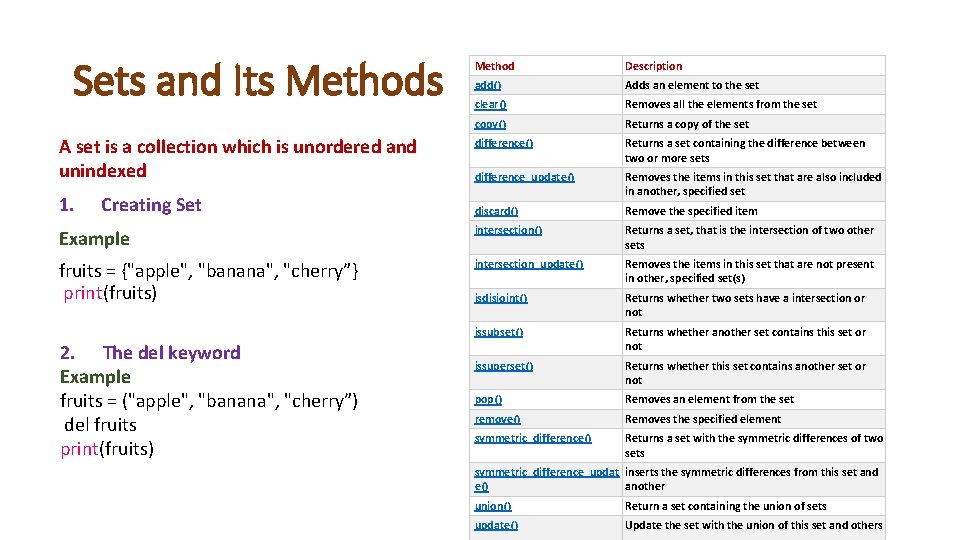
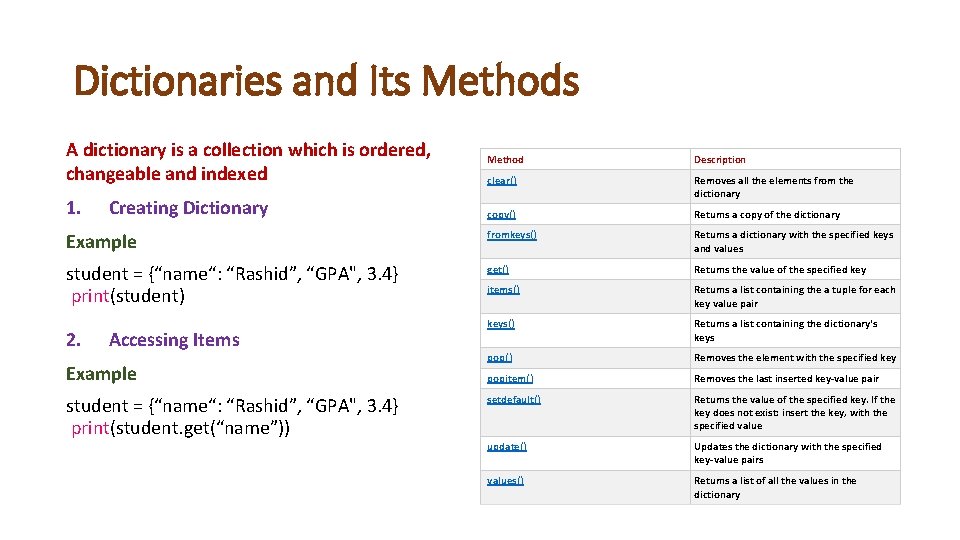
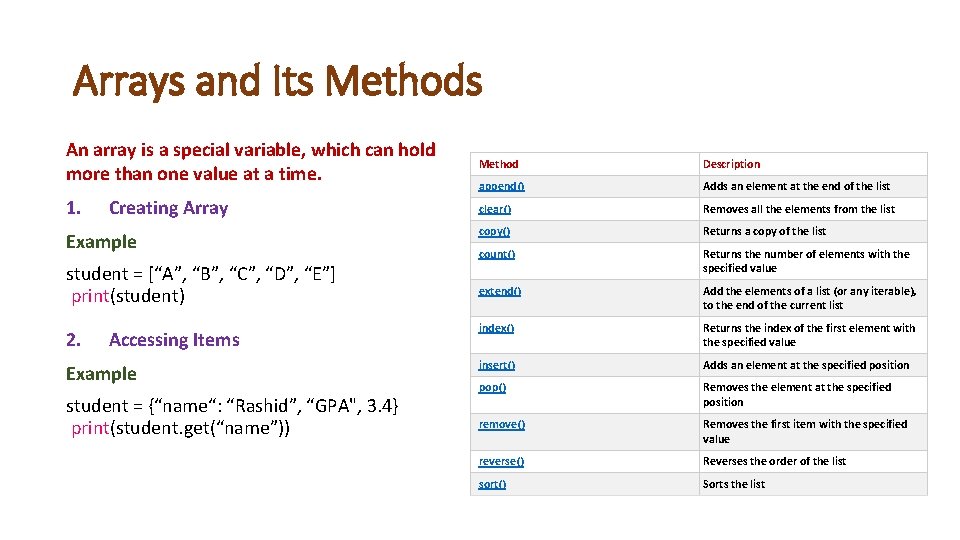
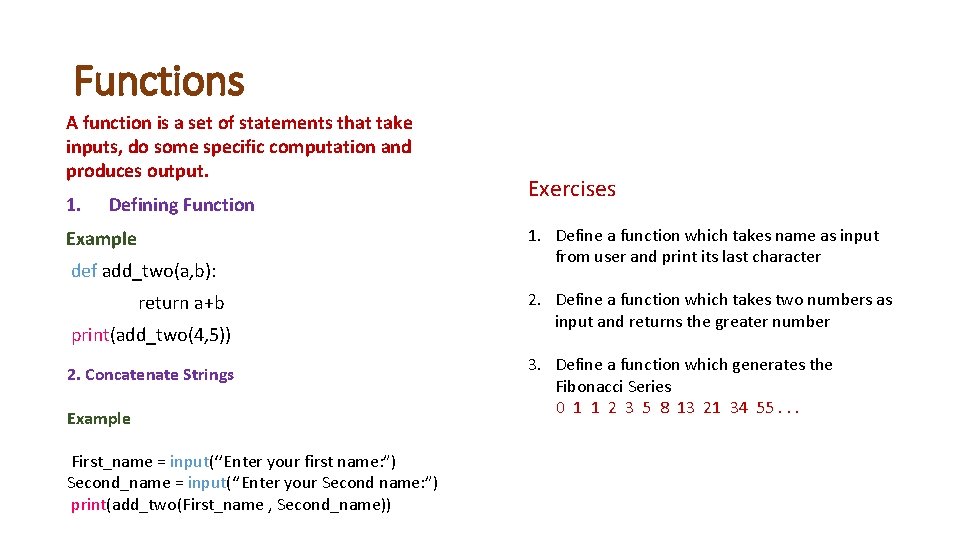
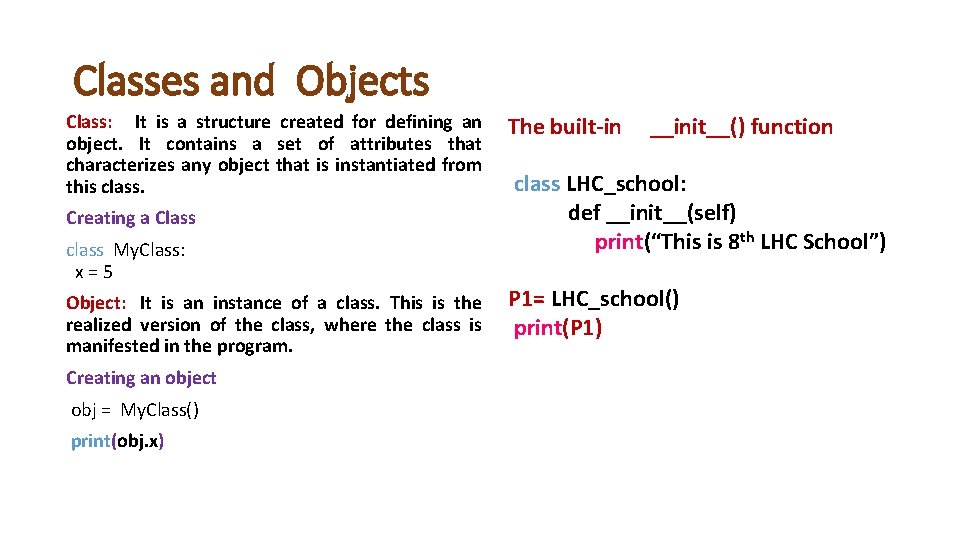
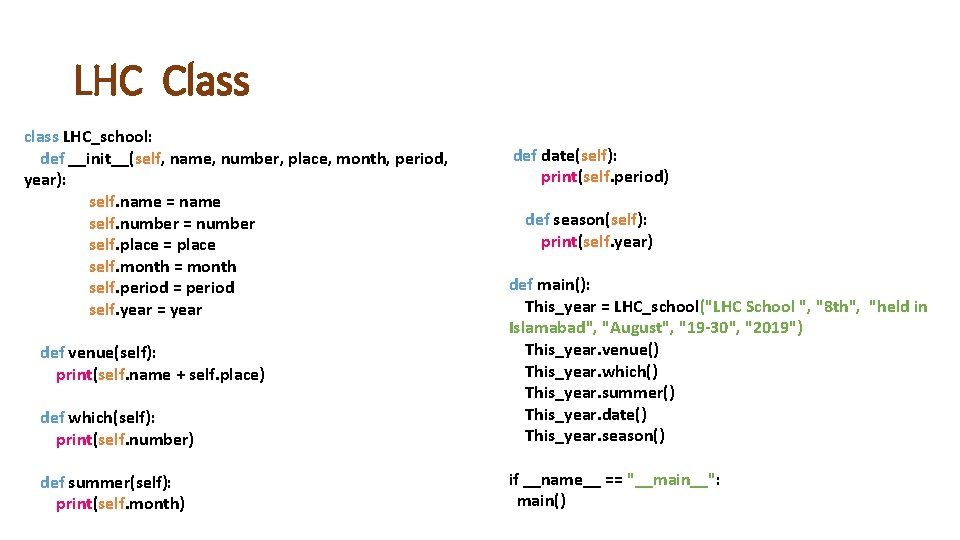
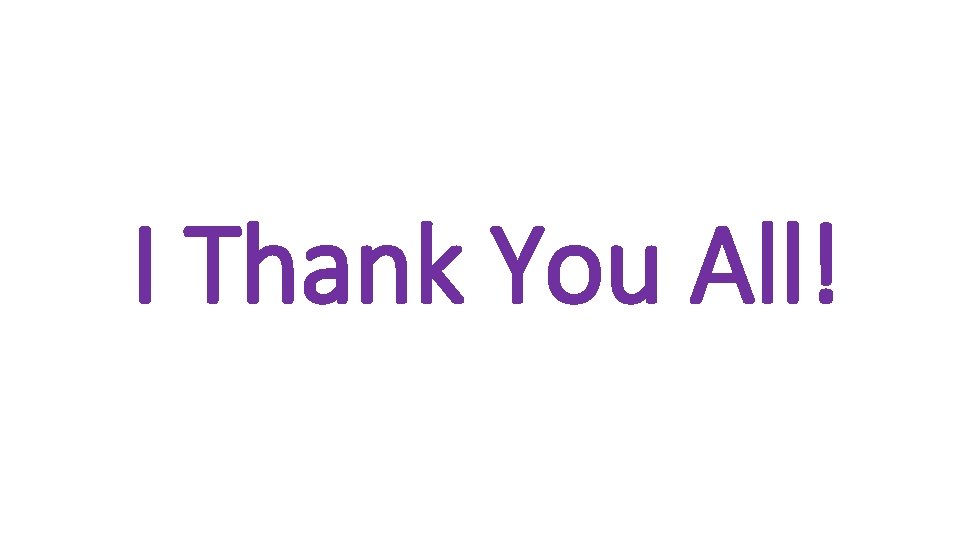
- Slides: 22
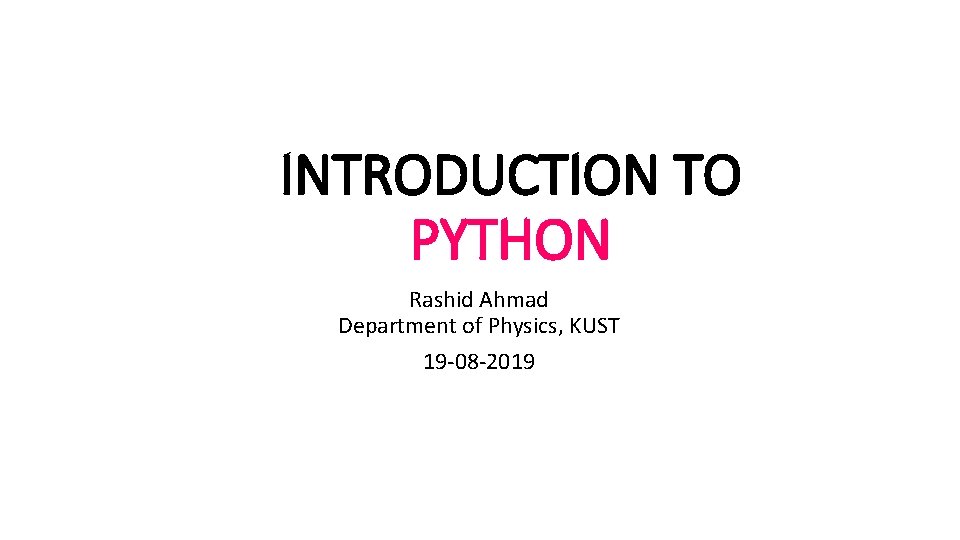
INTRODUCTION TO PYTHON Rashid Ahmad Department of Physics, KUST 19 -08 -2019
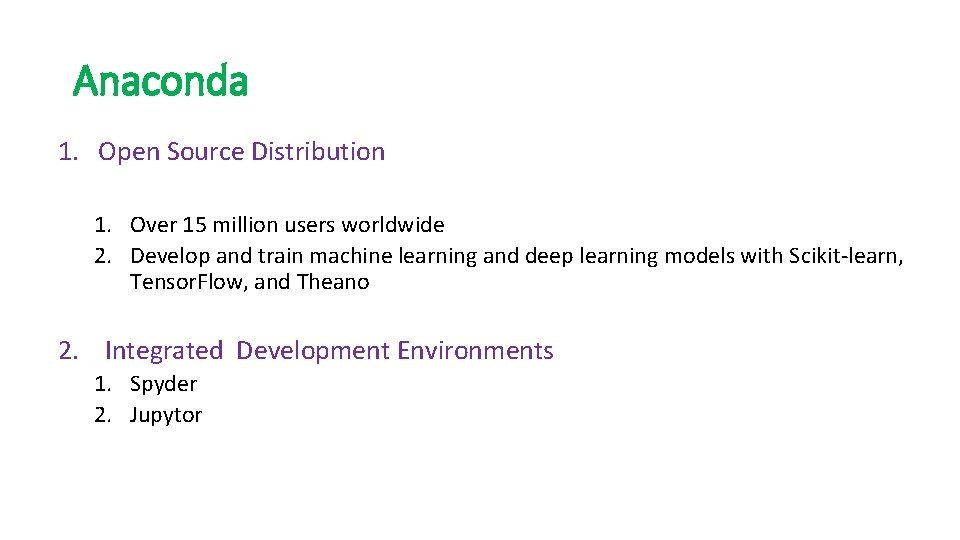
Anaconda 1. Open Source Distribution 1. Over 15 million users worldwide 2. Develop and train machine learning and deep learning models with Scikit-learn, Tensor. Flow, and Theano 2. Integrated Development Environments 1. Spyder 2. Jupytor
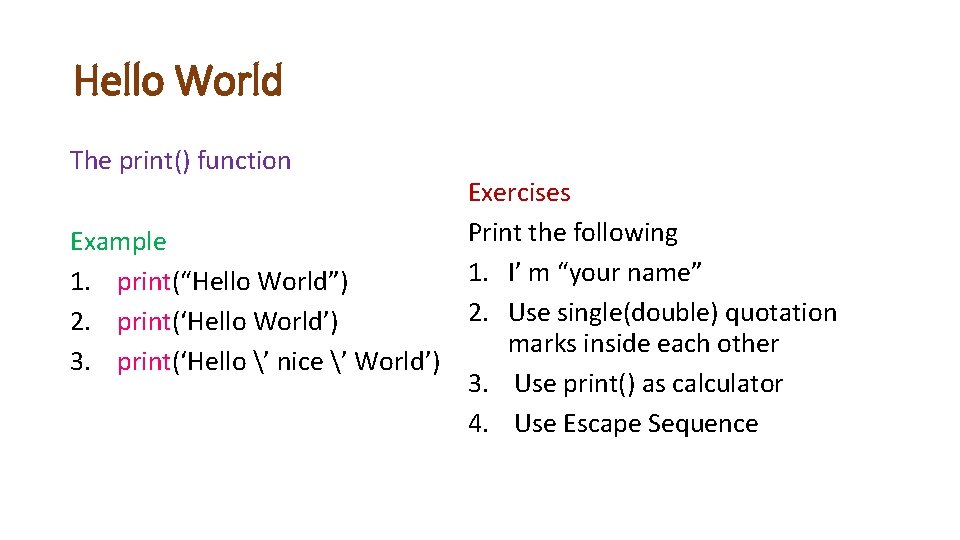
Hello World The print() function Exercises Print the following Example 1. I’ m “your name” 1. print(“Hello World”) 2. Use single(double) quotation 2. print(‘Hello World’) marks inside each other 3. print(‘Hello ’ nice ’ World’) 3. Use print() as calculator 4. Use Escape Sequence
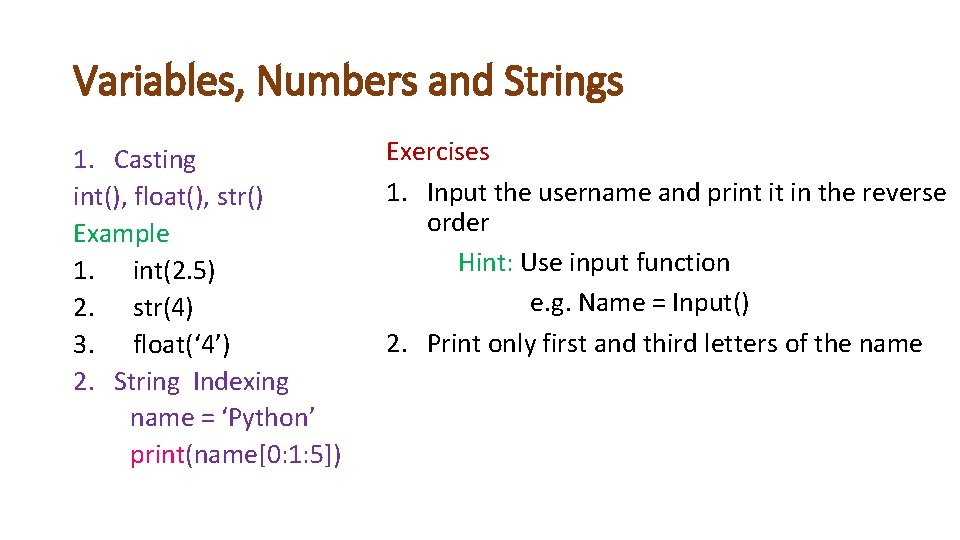
Variables, Numbers and Strings 1. Casting int(), float(), str() Example 1. int(2. 5) 2. str(4) 3. float(‘ 4’) 2. String Indexing name = ‘Python’ print(name[0: 1: 5]) Exercises 1. Input the username and print it in the reverse order Hint: Use input function e. g. Name = Input() 2. Print only first and third letters of the name
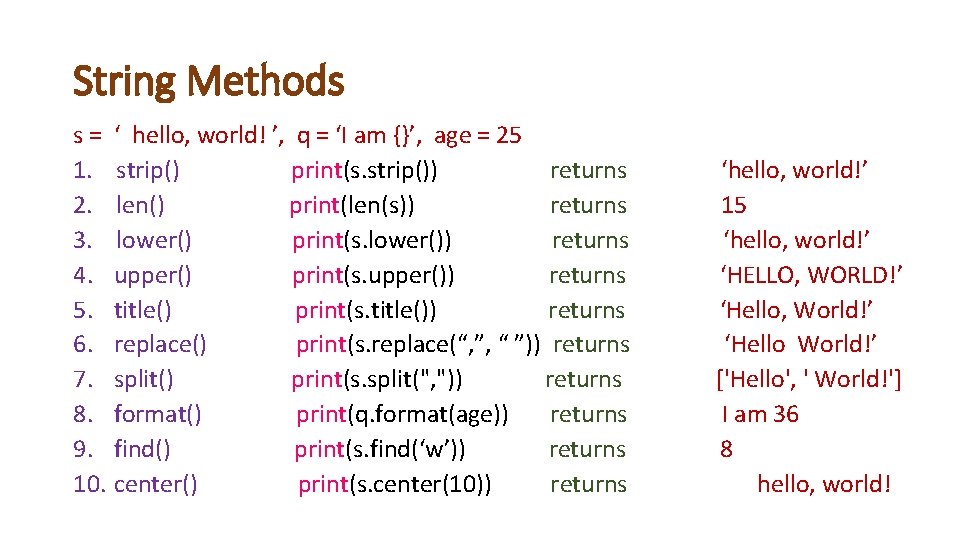
String Methods s = ‘ hello, world! ’, q = ‘I am {}’, age = 25 1. strip() print(s. strip()) returns 2. len() print(len(s)) returns 3. lower() print(s. lower()) returns 4. upper() print(s. upper()) returns 5. title() print(s. title()) returns 6. replace() print(s. replace(“, ”, “ ”)) returns 7. split() print(s. split(", ")) returns 8. format() print(q. format(age)) returns 9. find() print(s. find(‘w’)) returns 10. center() print(s. center(10)) returns ‘hello, world!’ 15 ‘hello, world!’ ‘HELLO, WORLD!’ ‘Hello, World!’ ‘Hello World!’ ['Hello', ' World!'] I am 36 8 hello, world!
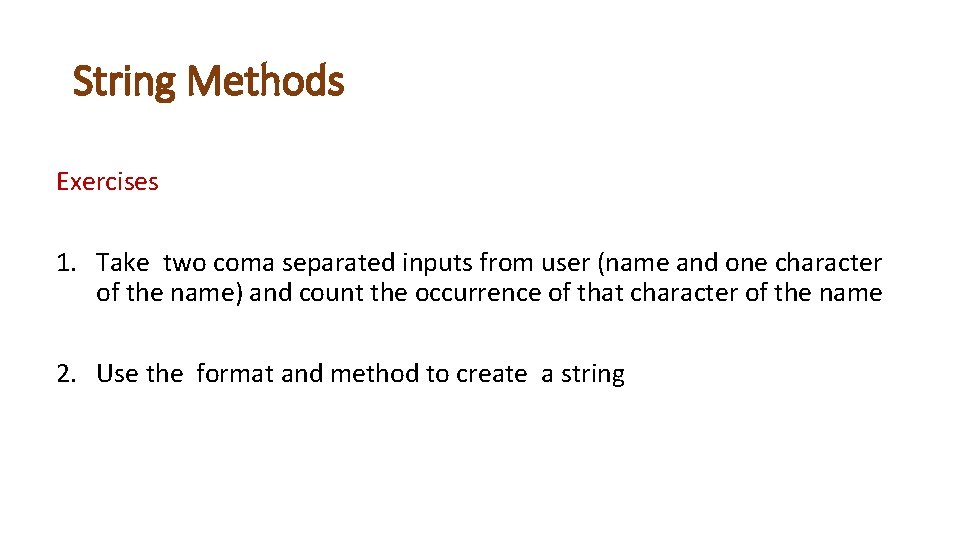
String Methods Exercises 1. Take two coma separated inputs from user (name and one character of the name) and count the occurrence of that character of the name 2. Use the format and method to create a string
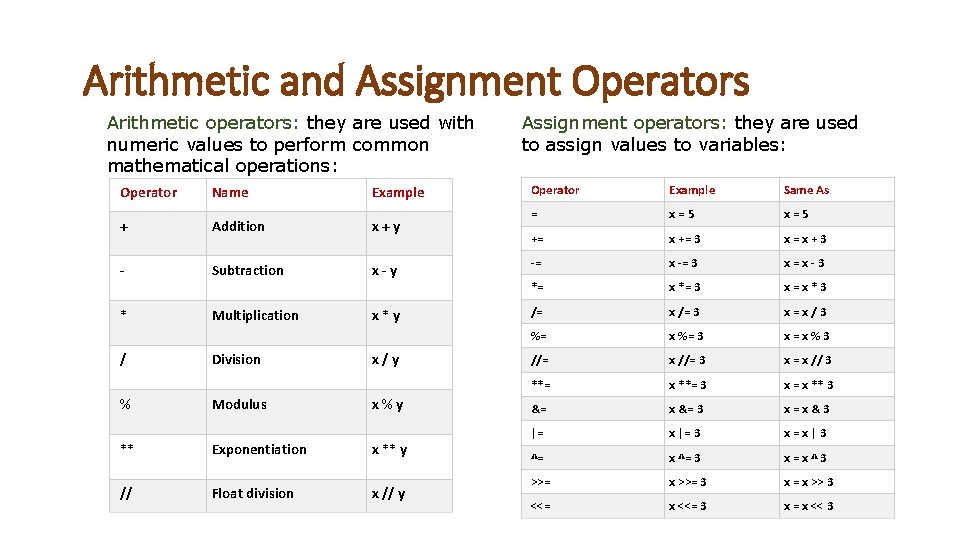
Arithmetic and Assignment Operators Arithmetic operators: they are used with numeric values to perform common mathematical operations: Operator Example Same As = x=5 += x += 3 x=x+3 x-y -= x -= 3 x=x-3 *= x *= 3 x=x*3 x*y /= x /= 3 x=x/3 %= x %= 3 x=x%3 //= x //= 3 x = x // 3 **= x **= 3 x = x ** 3 &= x &= 3 x=x&3 |= x |= 3 x=x|3 ^= x ^= 3 x=x^3 >>= x >>= 3 x = x >> 3 <<= x <<= 3 x = x << 3 Operator Name Example + Addition x+y - Subtraction * Multiplication / % Division Modulus Assignment operators: they are used to assign values to variables: x/y x%y ** Exponentiation x ** y // Float division x // y
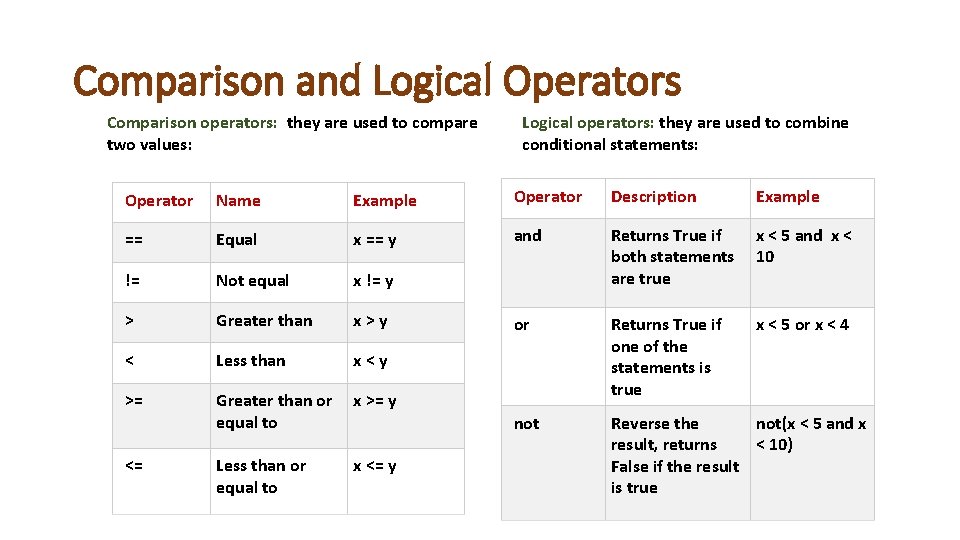
Comparison and Logical Operators Comparison operators: they are used to compare two values: Logical operators: they are used to combine conditional statements: Operator Name Example Operator Description Example == Equal x == y and x < 5 and x < 10 != Not equal x != y Returns True if both statements are true > Greater than x>y or x < 5 or x < 4 < Less than x<y >= Greater than or equal to x >= y Returns True if one of the statements is true not Less than or equal to x <= y Reverse the not(x < 5 and x result, returns < 10) False if the result is true <=
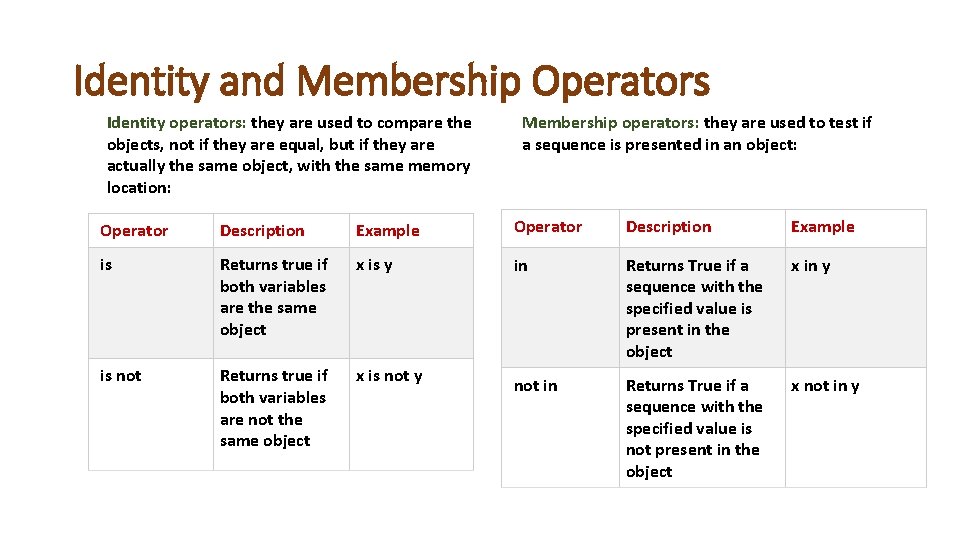
Identity and Membership Operators Identity operators: they are used to compare the objects, not if they are equal, but if they are actually the same object, with the same memory location: Membership operators: they are used to test if a sequence is presented in an object: Operator Description Example is Returns true if both variables are the same object x is y in Returns True if a sequence with the specified value is present in the object x in y is not Returns true if both variables are not the same object x is not y not in Returns True if a sequence with the specified value is not present in the object x not in y
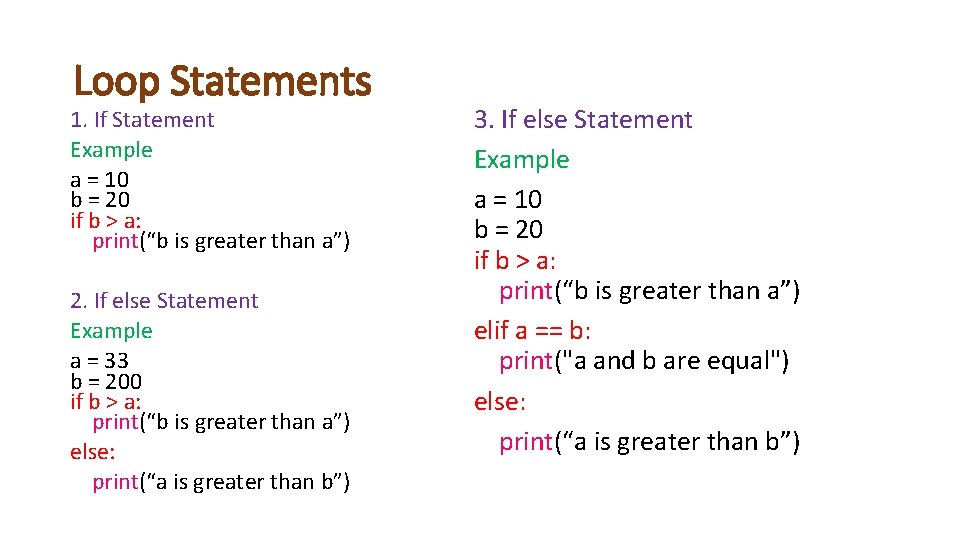
Loop Statements 1. If Statement Example a = 10 b = 20 if b > a: print(“b is greater than a”) 2. If else Statement Example a = 33 b = 200 if b > a: print(“b is greater than a”) else: print(“a is greater than b”) 3. If else Statement Example a = 10 b = 20 if b > a: print(“b is greater than a”) elif a == b: print("a and b are equal") else: print(“a is greater than b”)
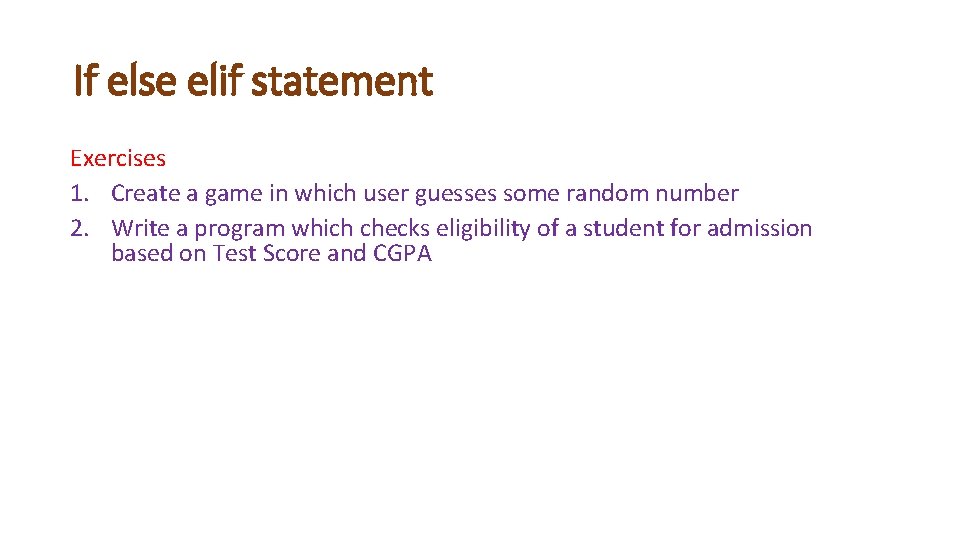
If else elif statement Exercises 1. Create a game in which user guesses some random number 2. Write a program which checks eligibility of a student for admission based on Test Score and CGPA
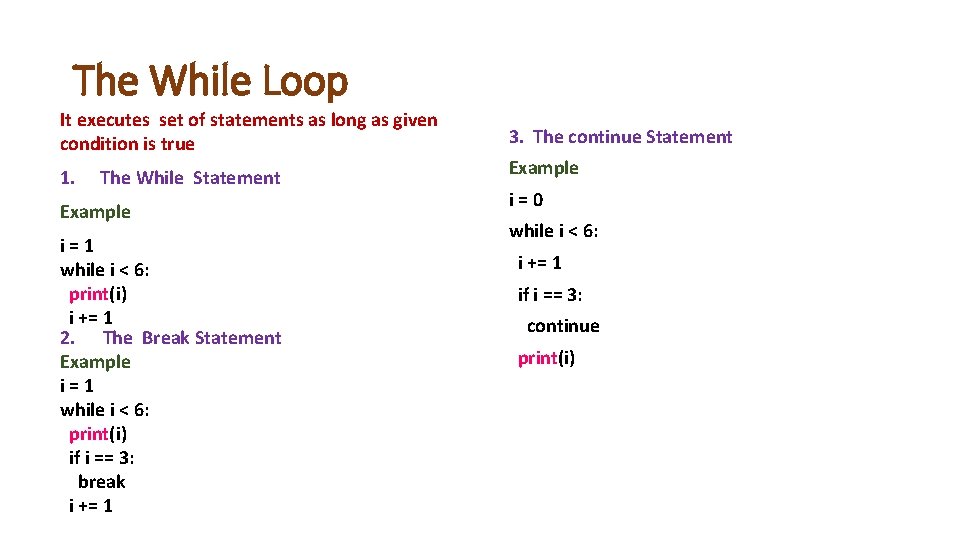
The While Loop It executes set of statements as long as given condition is true 1. The While Statement Example i=1 while i < 6: print(i) i += 1 2. The Break Statement Example i=1 while i < 6: print(i) if i == 3: break i += 1 3. The continue Statement Example i=0 while i < 6: i += 1 if i == 3: continue print(i)
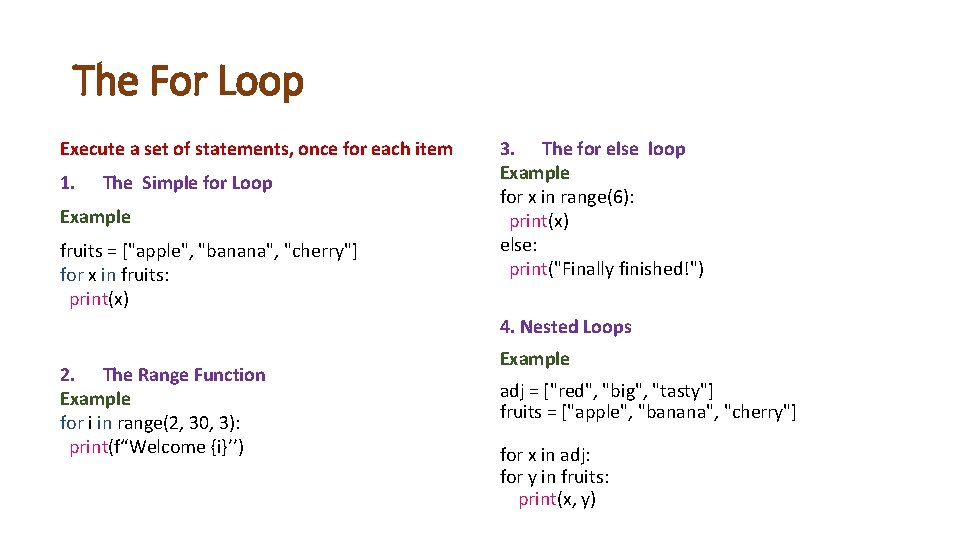
The For Loop Execute a set of statements, once for each item 1. The Simple for Loop Example fruits = ["apple", "banana", "cherry"] for x in fruits: print(x) 3. The for else loop Example for x in range(6): print(x) else: print("Finally finished!") 4. Nested Loops 2. The Range Function Example for i in range(2, 30, 3): print(f“Welcome {i}’’) Example adj = ["red", "big", "tasty"] fruits = ["apple", "banana", "cherry"] for x in adj: for y in fruits: print(x, y)
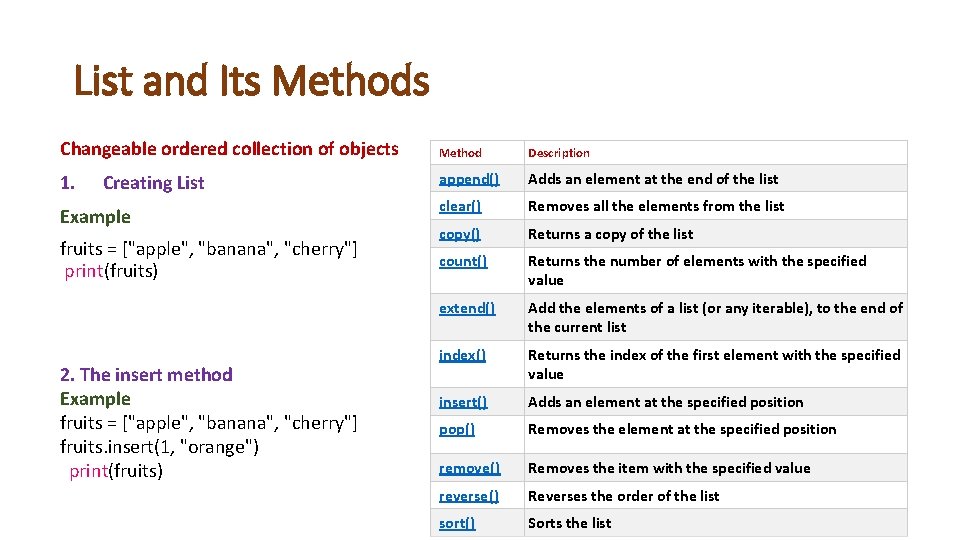
List and Its Methods Changeable ordered collection of objects Method Description 1. append() Adds an element at the end of the list clear() Removes all the elements from the list copy() Returns a copy of the list count() Returns the number of elements with the specified value extend() Add the elements of a list (or any iterable), to the end of the current list index() Returns the index of the first element with the specified value insert() Adds an element at the specified position pop() Removes the element at the specified position remove() Removes the item with the specified value reverse() Reverses the order of the list sort() Sorts the list Creating List Example fruits = ["apple", "banana", "cherry"] print(fruits) 2. The insert method Example fruits = ["apple", "banana", "cherry"] fruits. insert(1, "orange") print(fruits)
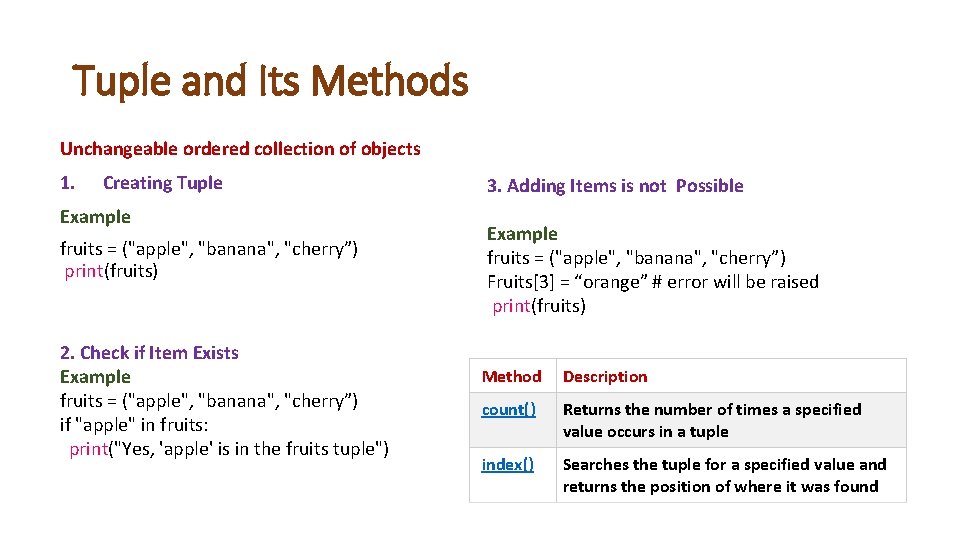
Tuple and Its Methods Unchangeable ordered collection of objects 1. Creating Tuple Example fruits = ("apple", "banana", "cherry”) print(fruits) 2. Check if Item Exists Example fruits = ("apple", "banana", "cherry”) if "apple" in fruits: print("Yes, 'apple' is in the fruits tuple") 3. Adding Items is not Possible Example fruits = ("apple", "banana", "cherry”) Fruits[3] = “orange” # error will be raised print(fruits) Method Description count() Returns the number of times a specified value occurs in a tuple index() Searches the tuple for a specified value and returns the position of where it was found
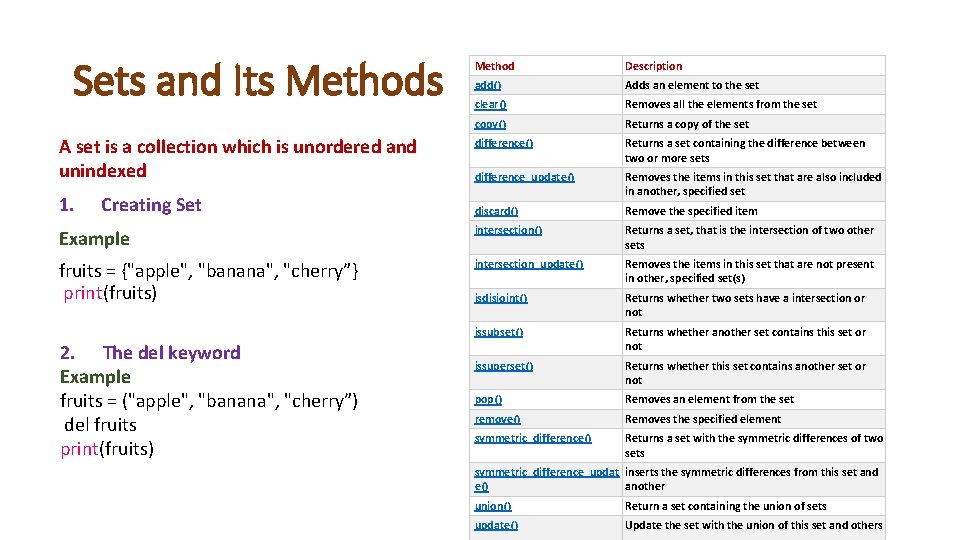
Sets and Its Method Description add() Adds an element to the set clear() Removes all the elements from the set copy() Returns a copy of the set difference() Returns a set containing the difference between two or more sets difference_update() Removes the items in this set that are also included in another, specified set discard() Remove the specified item Example intersection() Returns a set, that is the intersection of two other sets fruits = {"apple", "banana", "cherry”} print(fruits) intersection_update() Removes the items in this set that are not present in other, specified set(s) isdisjoint() Returns whether two sets have a intersection or not issubset() Returns whether another set contains this set or not issuperset() Returns whether this set contains another set or not pop() Removes an element from the set remove() Removes the specified element symmetric_difference() Returns a set with the symmetric differences of two sets A set is a collection which is unordered and unindexed 1. Creating Set 2. The del keyword Example fruits = ("apple", "banana", "cherry”) del fruits print(fruits) symmetric_difference_updat inserts the symmetric differences from this set and e() another union() Return a set containing the union of sets update() Update the set with the union of this set and others
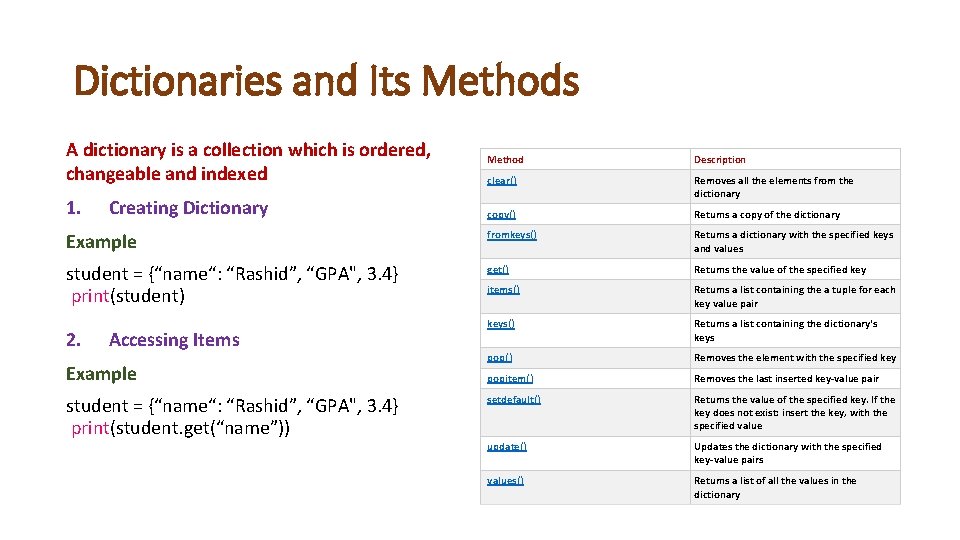
Dictionaries and Its Methods A dictionary is a collection which is ordered, changeable and indexed Method Description clear() Removes all the elements from the dictionary copy() Returns a copy of the dictionary Example fromkeys() Returns a dictionary with the specified keys and values student = {“name“: “Rashid”, “GPA", 3. 4} print(student) get() Returns the value of the specified key items() Returns a list containing the a tuple for each key value pair keys() Returns a list containing the dictionary's keys pop() Removes the element with the specified key popitem() Removes the last inserted key-value pair setdefault() Returns the value of the specified key. If the key does not exist: insert the key, with the specified value update() Updates the dictionary with the specified key-value pairs values() Returns a list of all the values in the dictionary 1. 2. Creating Dictionary Accessing Items Example student = {“name“: “Rashid”, “GPA", 3. 4} print(student. get(“name”))
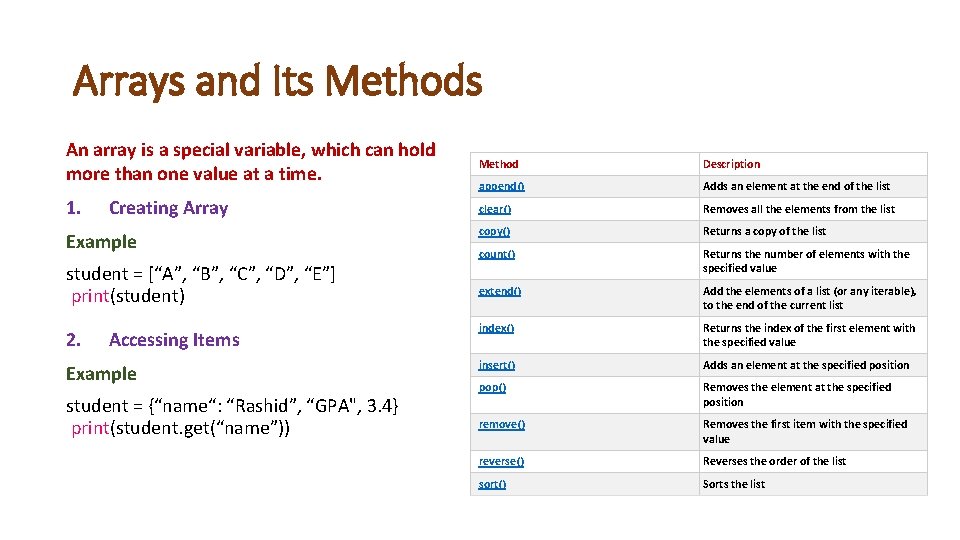
Arrays and Its Methods An array is a special variable, which can hold more than one value at a time. Method Description append() Adds an element at the end of the list 1. clear() Removes all the elements from the list copy() Returns a copy of the list count() Returns the number of elements with the specified value extend() Add the elements of a list (or any iterable), to the end of the current list index() Returns the index of the first element with the specified value insert() Adds an element at the specified position pop() Removes the element at the specified position remove() Removes the first item with the specified value reverse() Reverses the order of the list sort() Sorts the list Creating Array Example student = [“A”, “B”, “C”, “D”, “E”] print(student) 2. Accessing Items Example student = {“name“: “Rashid”, “GPA", 3. 4} print(student. get(“name”))
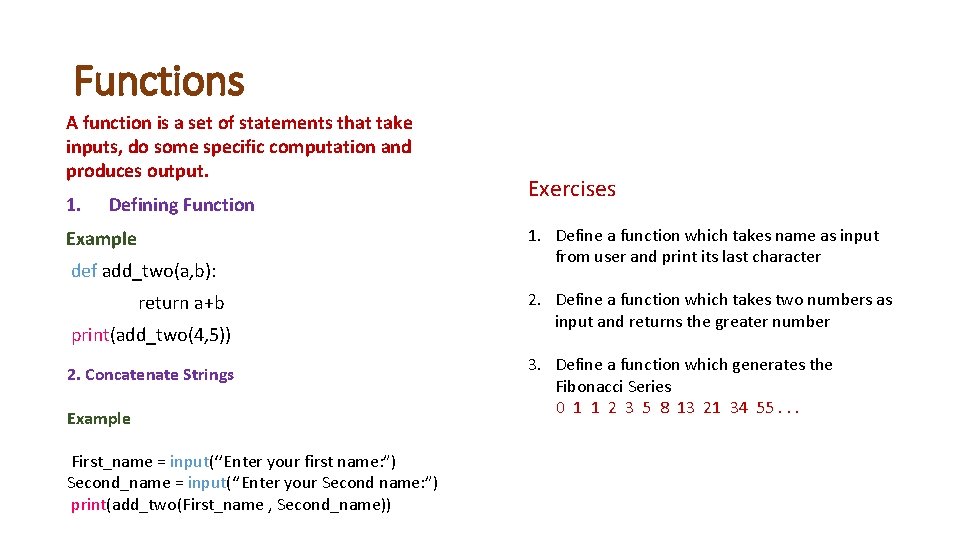
Functions A function is a set of statements that take inputs, do some specific computation and produces output. 1. Defining Function Example def add_two(a, b): return a+b print(add_two(4, 5)) 2. Concatenate Strings Example First_name = input(‘’Enter your first name: ”) Second_name = input(‘’Enter your Second name: ”) print(add_two(First_name , Second_name)) Exercises 1. Define a function which takes name as input from user and print its last character 2. Define a function which takes two numbers as input and returns the greater number 3. Define a function which generates the Fibonacci Series 0 1 1 2 3 5 8 13 21 34 55. . .
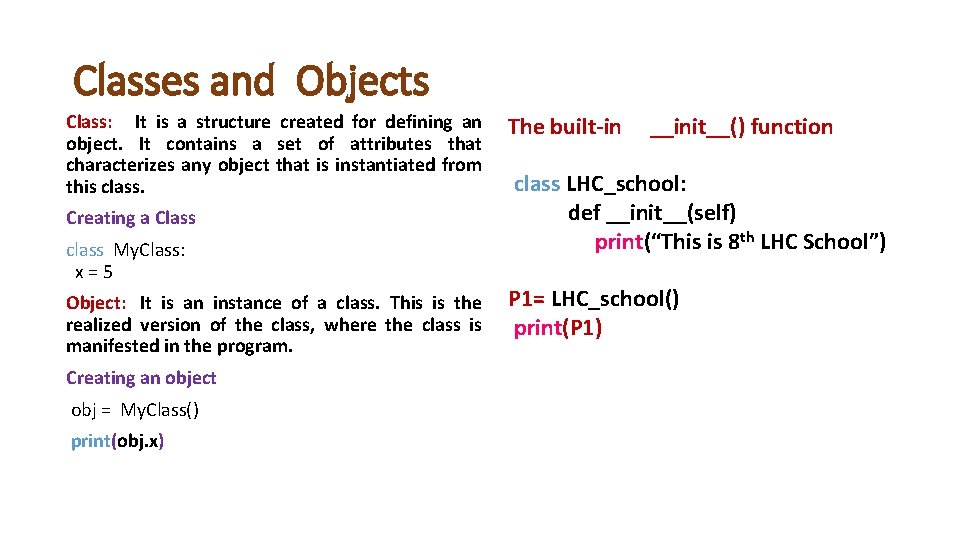
Classes and Objects Class: It is a structure created for defining an object. It contains a set of attributes that characterizes any object that is instantiated from this class. Creating a Class class My. Class: x=5 Object: It is an instance of a class. This is the realized version of the class, where the class is manifested in the program. Creating an object obj = My. Class() print(obj. x) The built-in __init__() function class LHC_school: def __init__(self) print(“This is 8 th LHC School”) P 1= LHC_school() print(P 1)
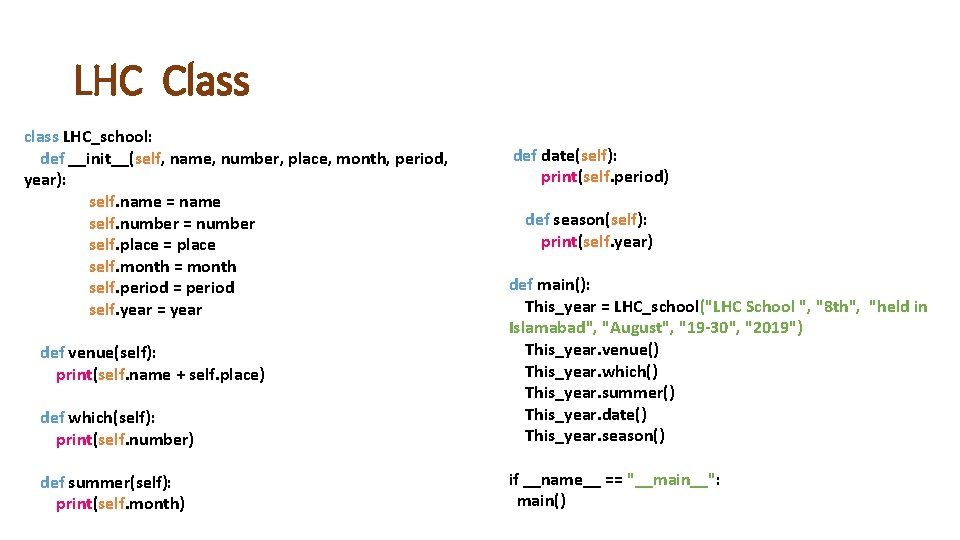
LHC Class class LHC_school: def __init__(self, name, number, place, month, period, year): self. name = name self. number = number self. place = place self. month = month self. period = period self. year = year def date(self): print(self. period) def season(self): print(self. year) def which(self): print(self. number) def main(): This_year = LHC_school("LHC School ", "8 th", "held in Islamabad", "August", "19 -30", "2019") This_year. venue() This_year. which() This_year. summer() This_year. date() This_year. season() def summer(self): print(self. month) if __name__ == "__main__": main() def venue(self): print(self. name + self. place)
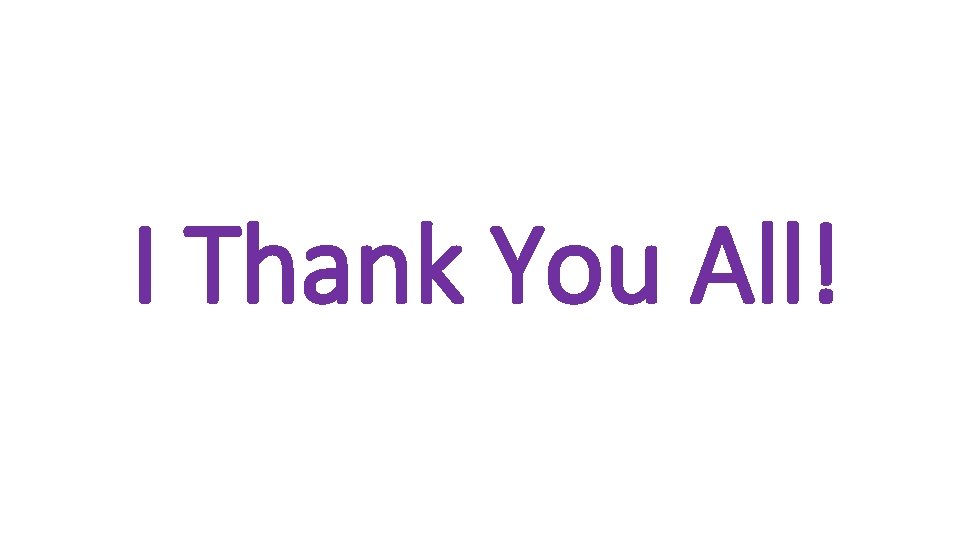
I Thank You All!