Introduction to Python Introduction to Computing Science and
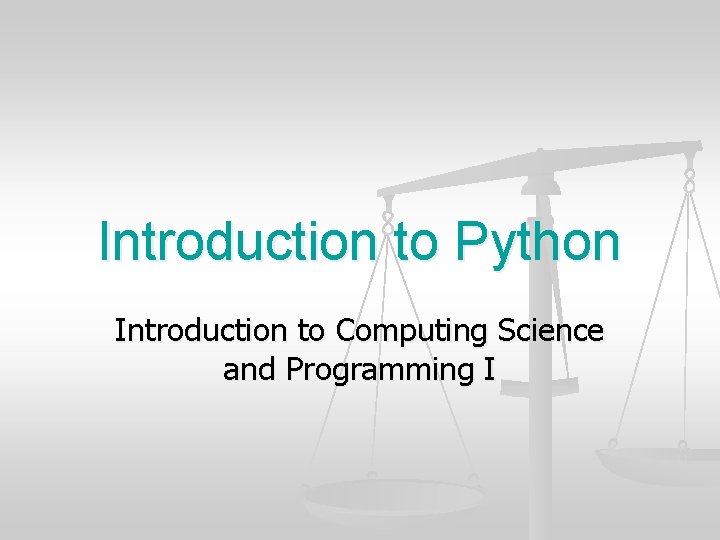
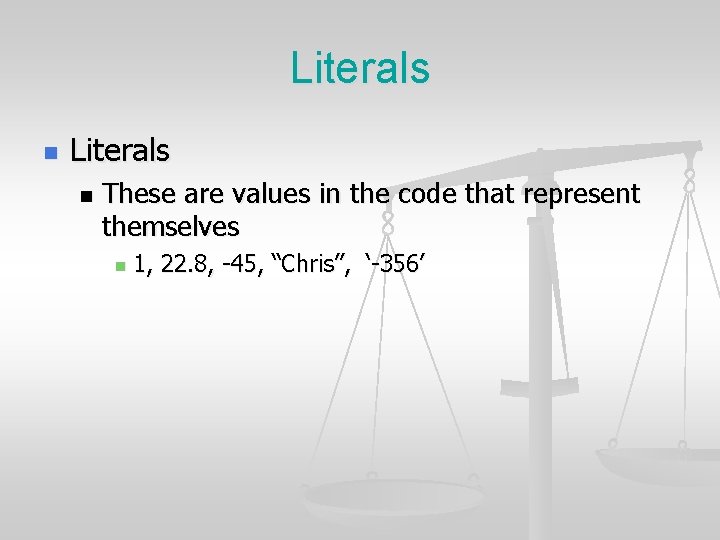
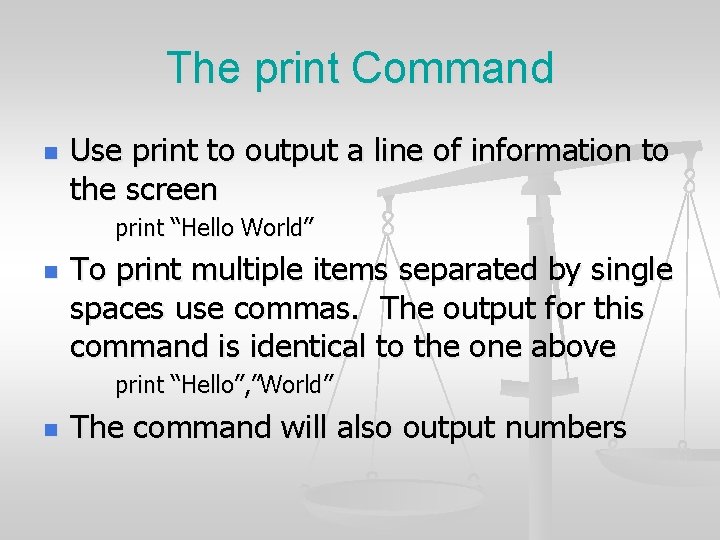
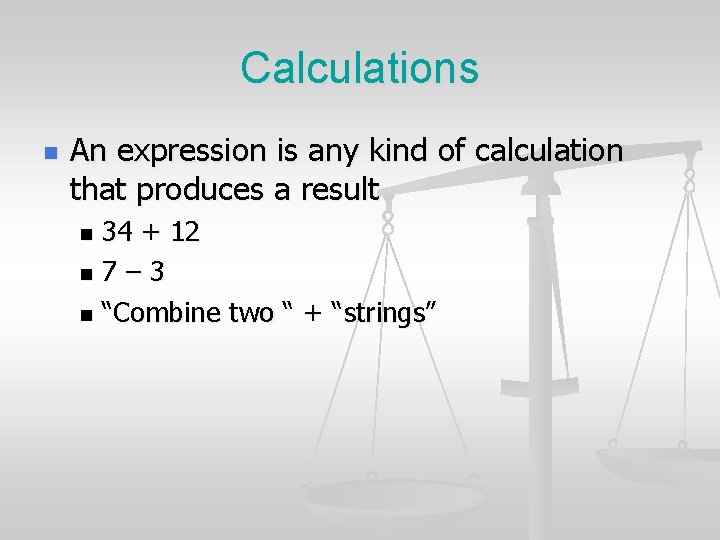
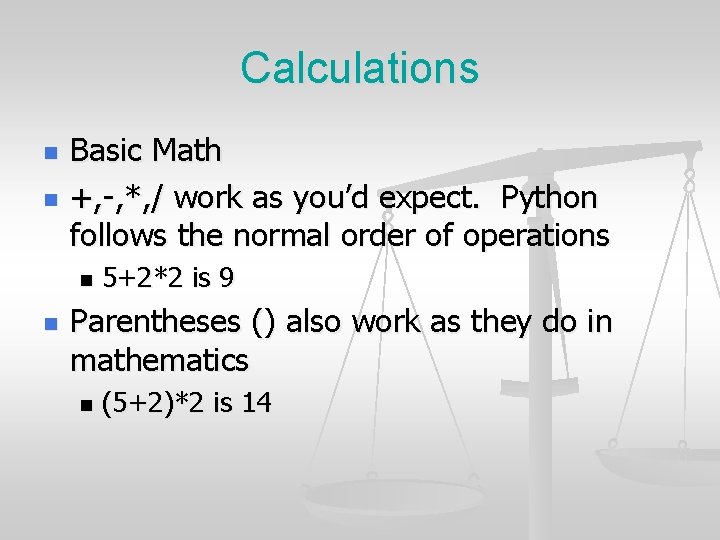
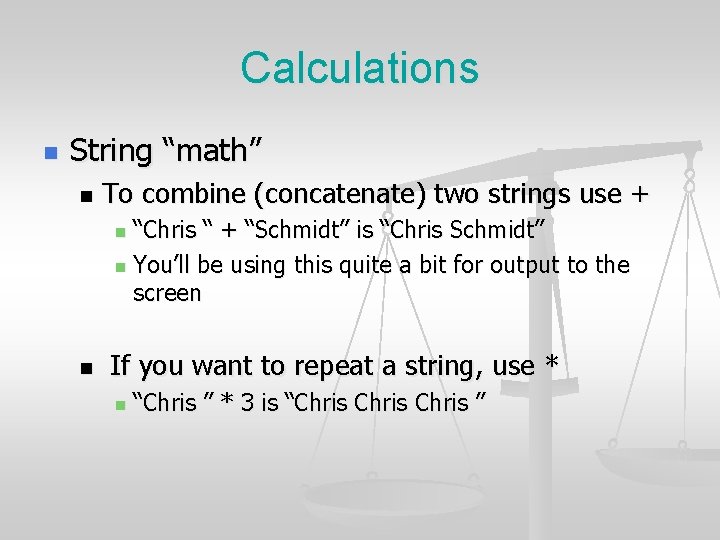
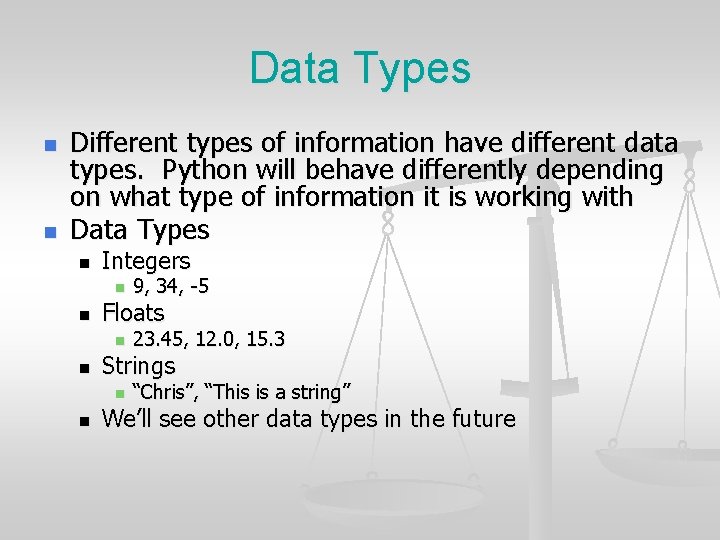
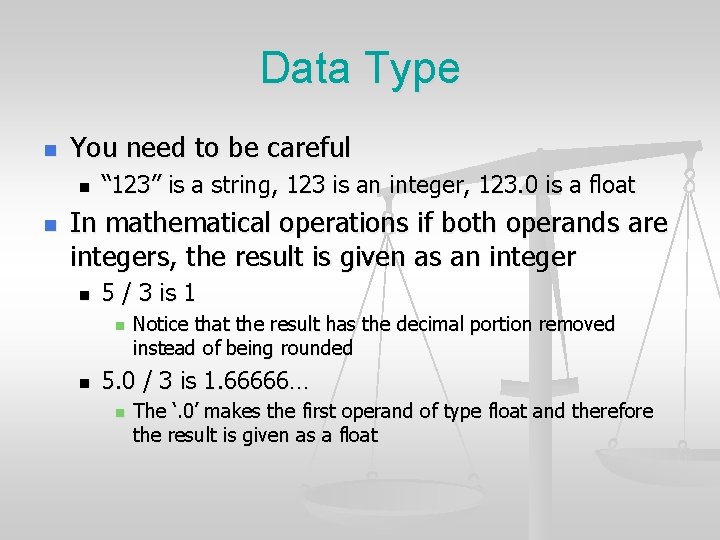
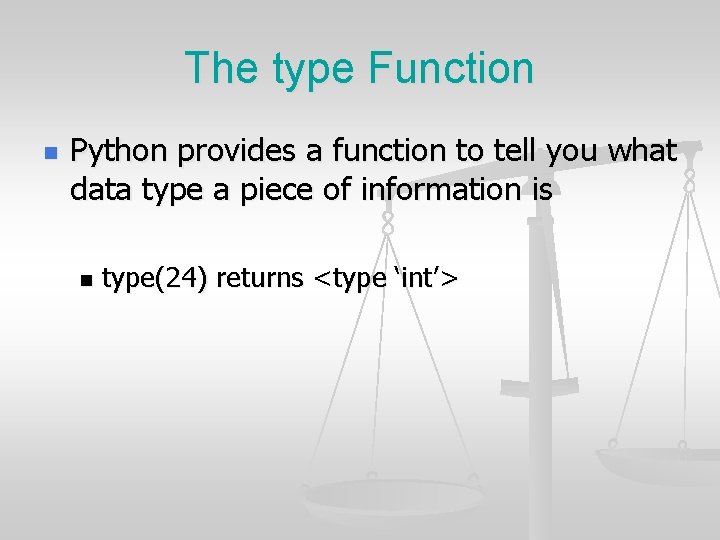
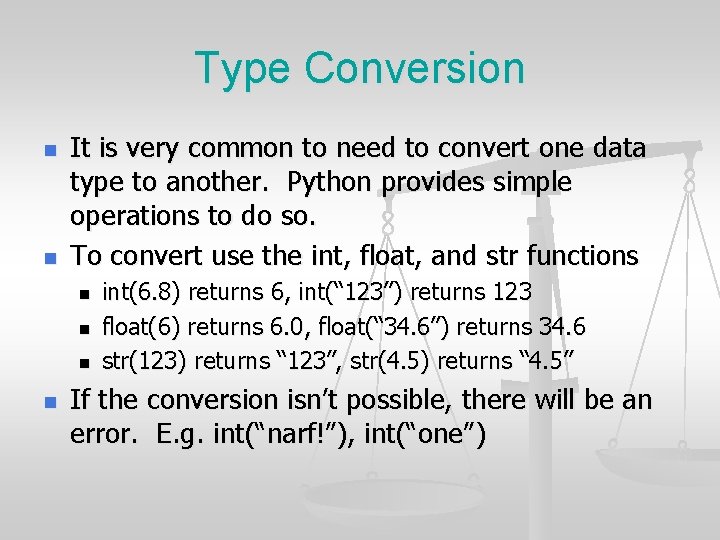
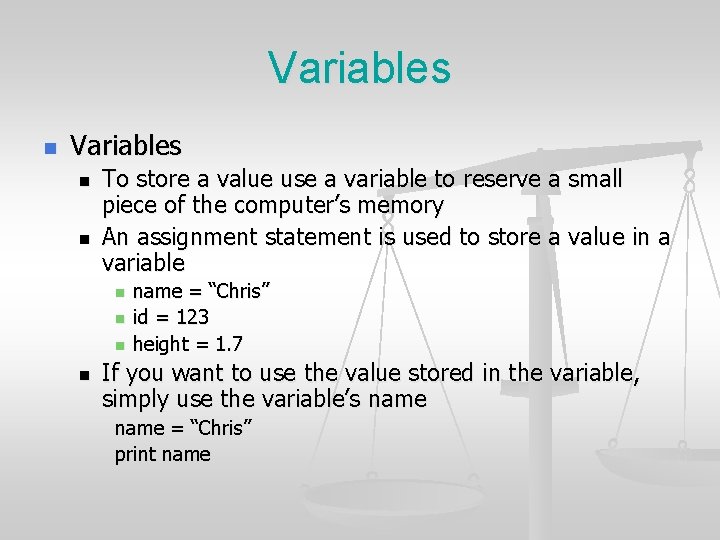
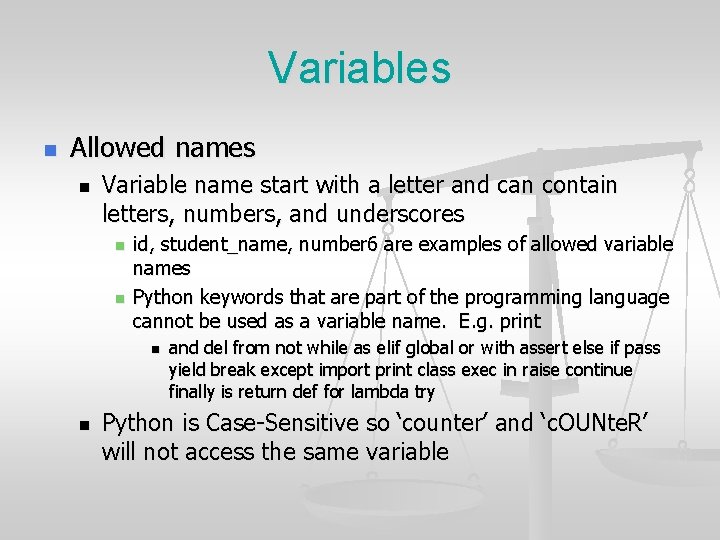
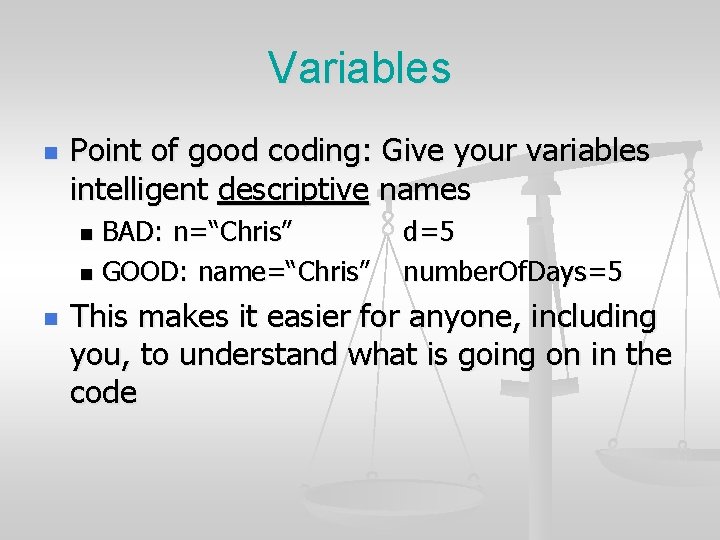
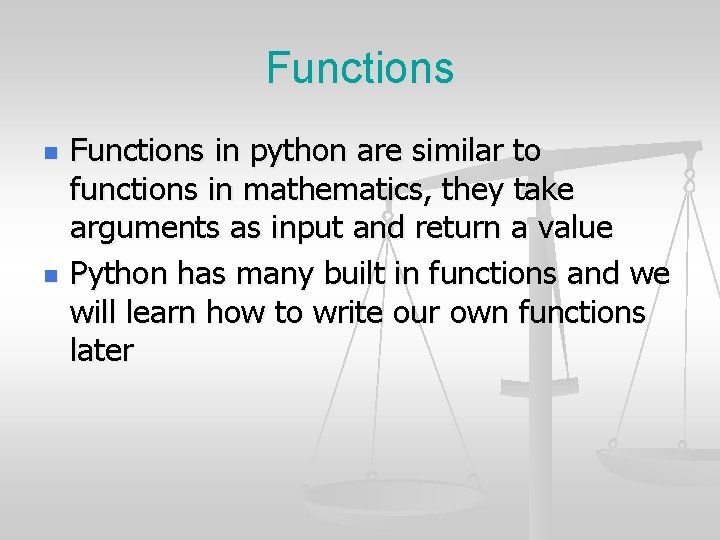
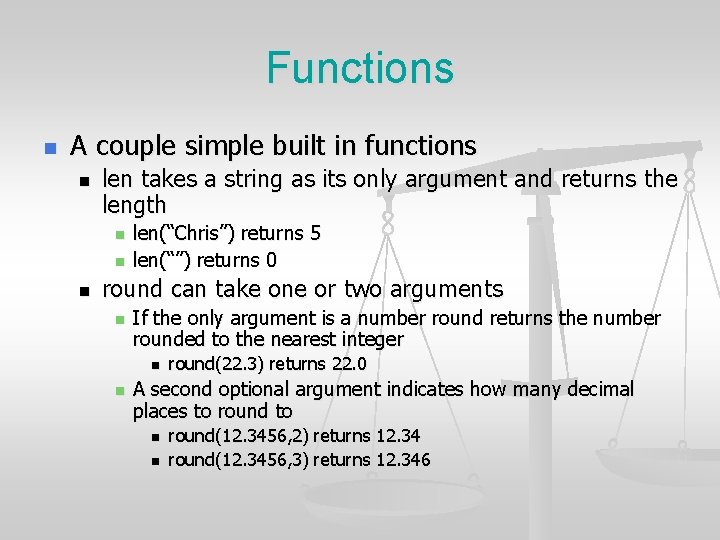
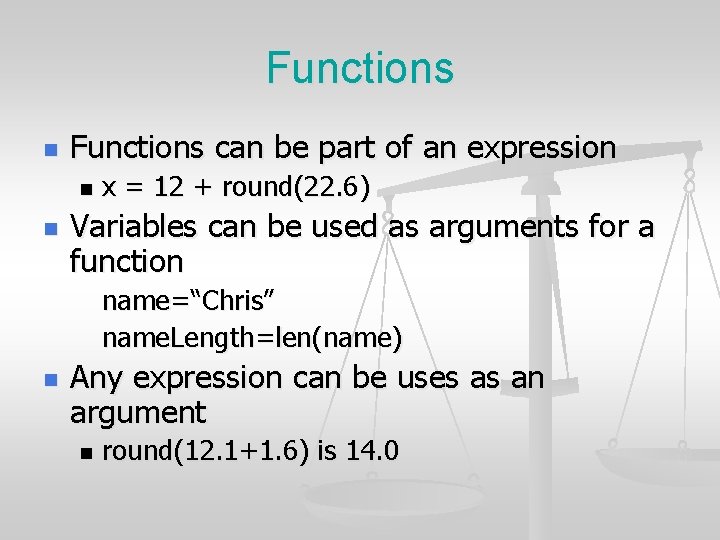
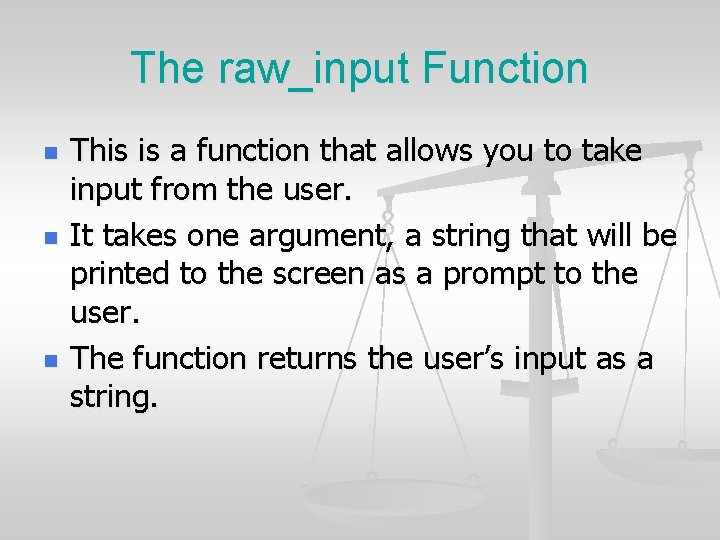
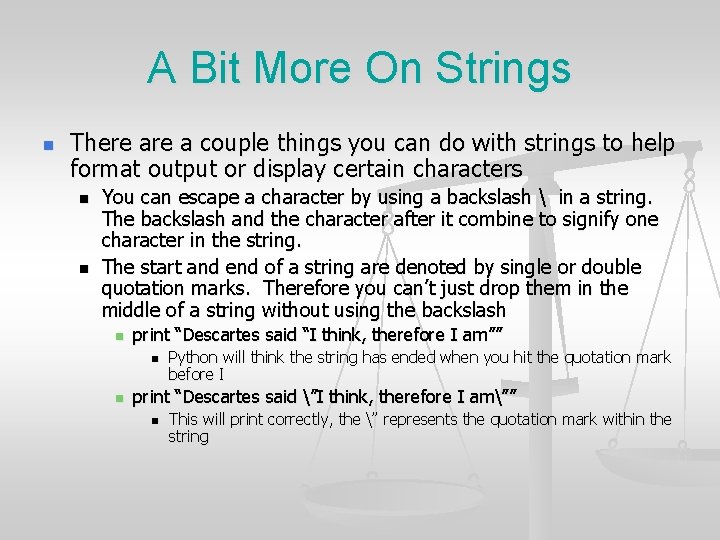
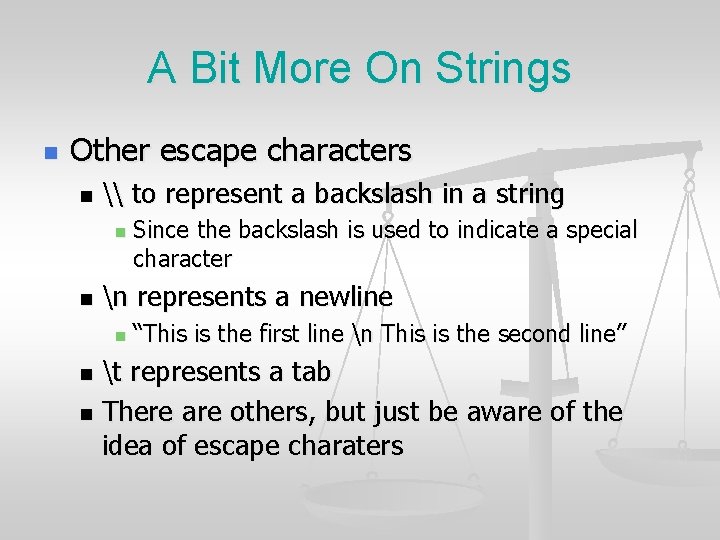
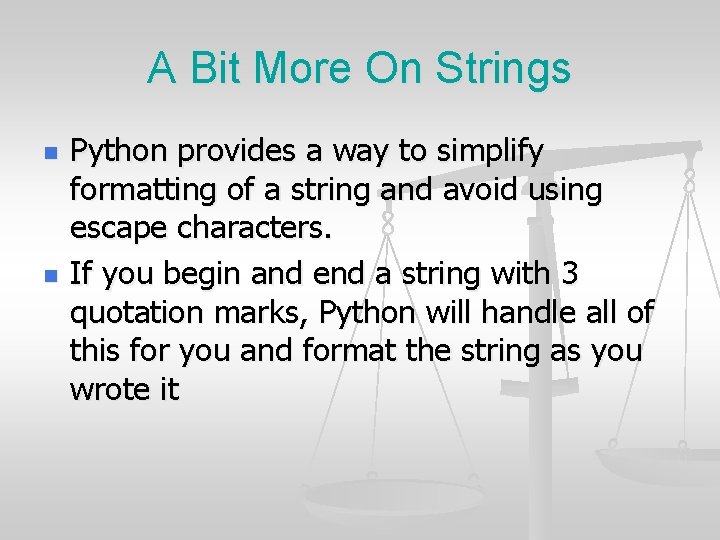
- Slides: 20
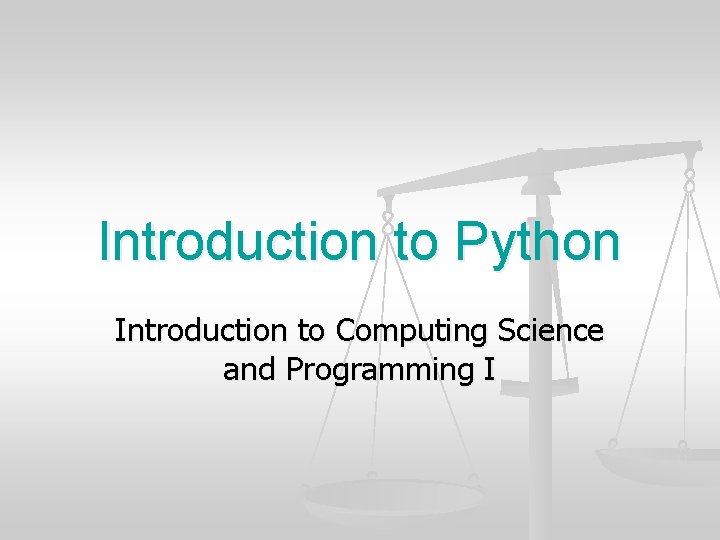
Introduction to Python Introduction to Computing Science and Programming I
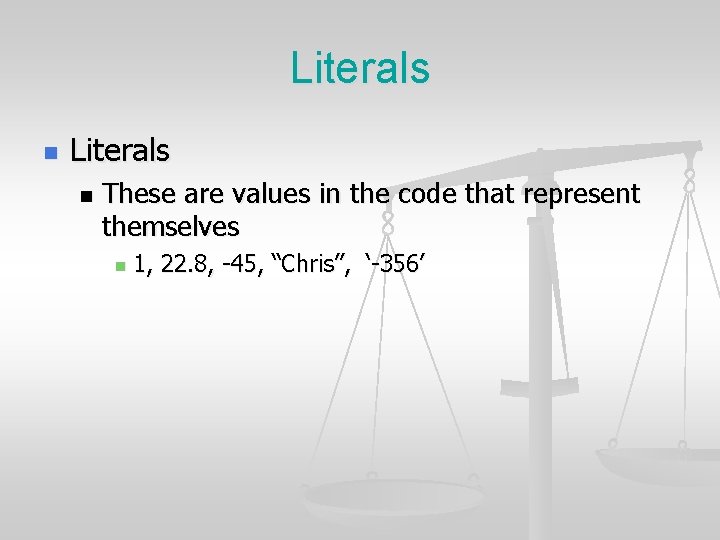
Literals n These are values in the code that represent themselves n 1, 22. 8, -45, “Chris”, ‘-356’
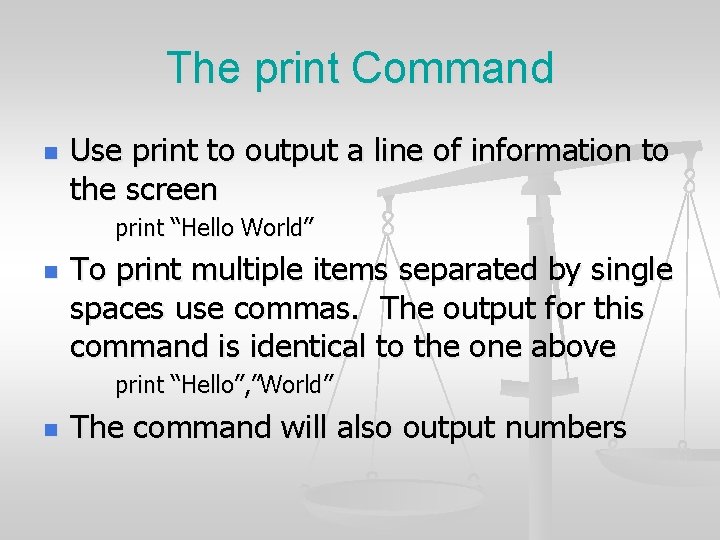
The print Command n Use print to output a line of information to the screen print “Hello World” n To print multiple items separated by single spaces use commas. The output for this command is identical to the one above print “Hello”, ”World” n The command will also output numbers
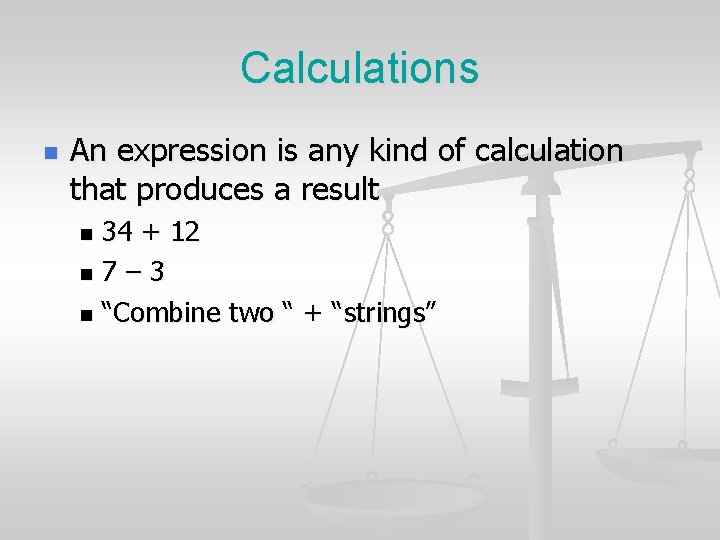
Calculations n An expression is any kind of calculation that produces a result 34 + 12 n 7 – 3 n “Combine two “ + “strings” n
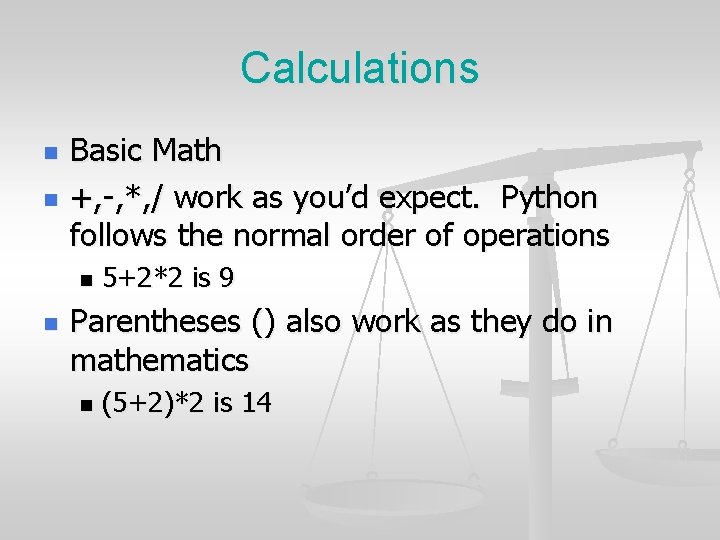
Calculations n n Basic Math +, -, *, / work as you’d expect. Python follows the normal order of operations n n 5+2*2 is 9 Parentheses () also work as they do in mathematics n (5+2)*2 is 14
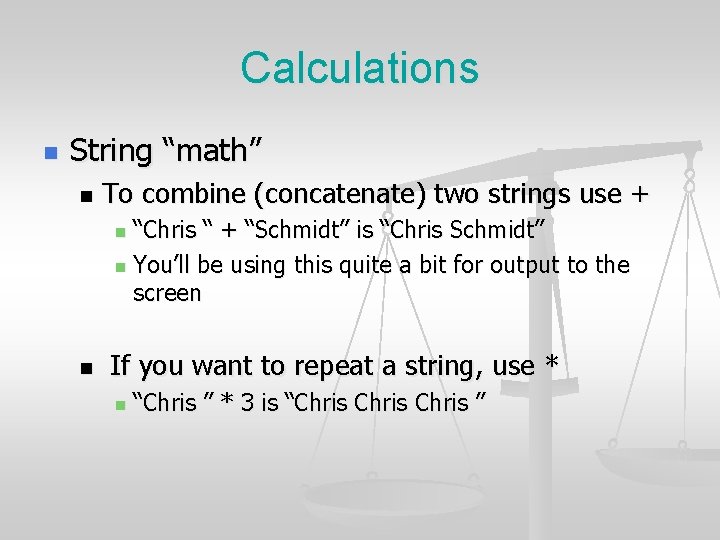
Calculations n String “math” n To combine (concatenate) two strings use + “Chris “ + “Schmidt” is “Chris Schmidt” n You’ll be using this quite a bit for output to the screen n n If you want to repeat a string, use * n “Chris ” * 3 is “Chris ”
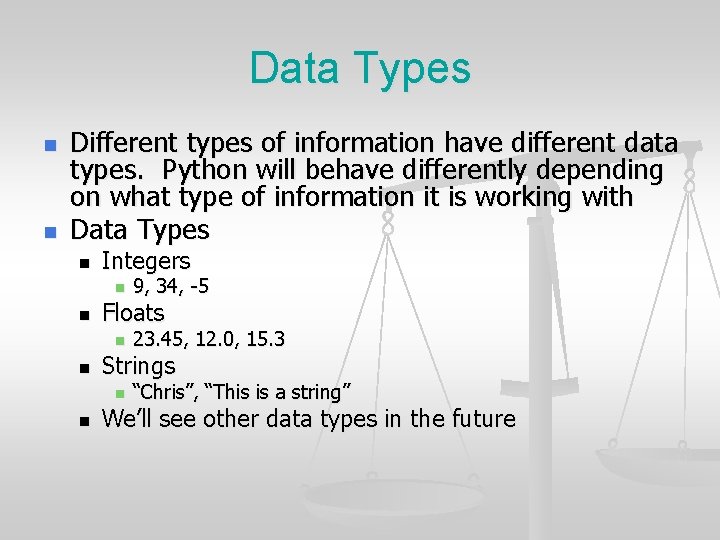
Data Types n n Different types of information have different data types. Python will behave differently depending on what type of information it is working with Data Types n Integers n n Floats n n 23. 45, 12. 0, 15. 3 Strings n n 9, 34, -5 “Chris”, “This is a string” We’ll see other data types in the future
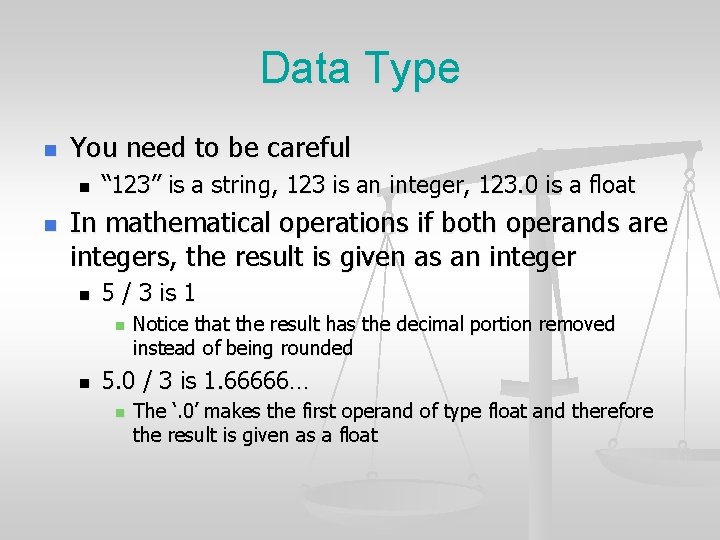
Data Type n You need to be careful n n “ 123” is a string, 123 is an integer, 123. 0 is a float In mathematical operations if both operands are integers, the result is given as an integer n 5 / 3 is 1 n n Notice that the result has the decimal portion removed instead of being rounded 5. 0 / 3 is 1. 66666… n The ‘. 0’ makes the first operand of type float and therefore the result is given as a float
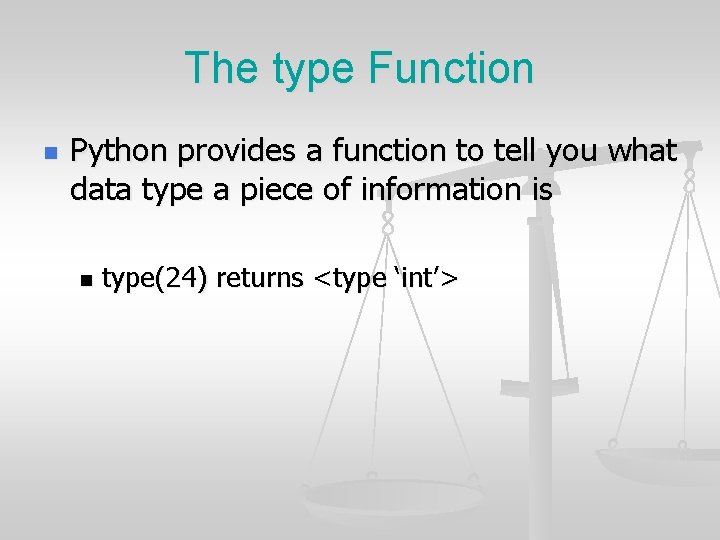
The type Function n Python provides a function to tell you what data type a piece of information is n type(24) returns <type ‘int’>
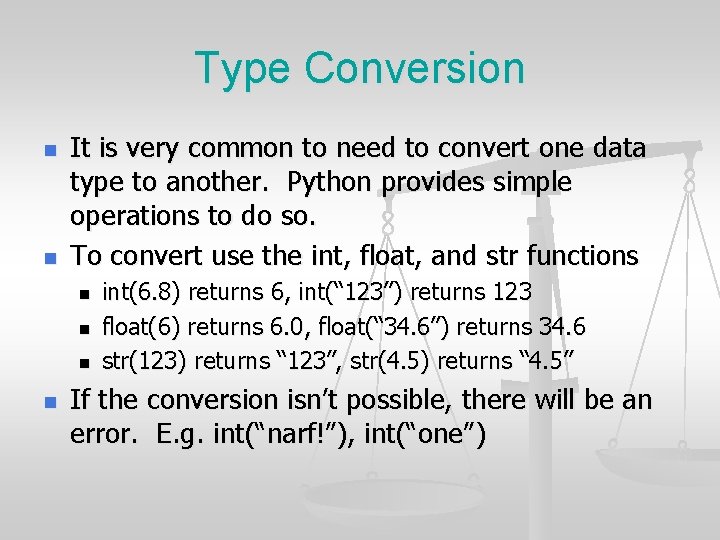
Type Conversion n n It is very common to need to convert one data type to another. Python provides simple operations to do so. To convert use the int, float, and str functions n n int(6. 8) returns 6, int(“ 123”) returns 123 float(6) returns 6. 0, float(“ 34. 6”) returns 34. 6 str(123) returns “ 123”, str(4. 5) returns “ 4. 5” If the conversion isn’t possible, there will be an error. E. g. int(“narf!”), int(“one”)
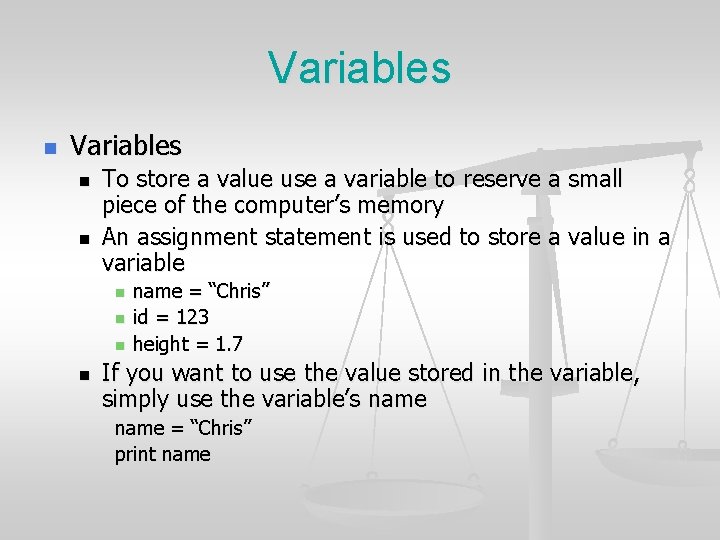
Variables n n To store a value use a variable to reserve a small piece of the computer’s memory An assignment statement is used to store a value in a variable n n name = “Chris” id = 123 height = 1. 7 If you want to use the value stored in the variable, simply use the variable’s name = “Chris” print name
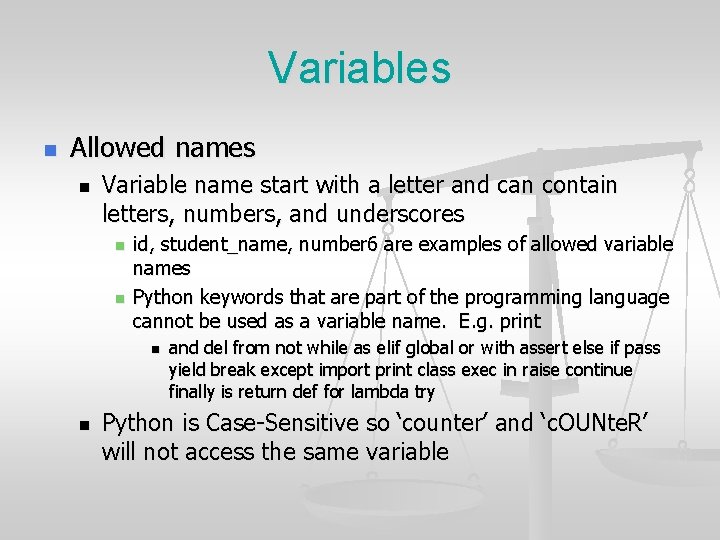
Variables n Allowed names n Variable name start with a letter and can contain letters, numbers, and underscores n n id, student_name, number 6 are examples of allowed variable names Python keywords that are part of the programming language cannot be used as a variable name. E. g. print n n and del from not while as elif global or with assert else if pass yield break except import print class exec in raise continue finally is return def for lambda try Python is Case-Sensitive so ‘counter’ and ‘c. OUNte. R’ will not access the same variable
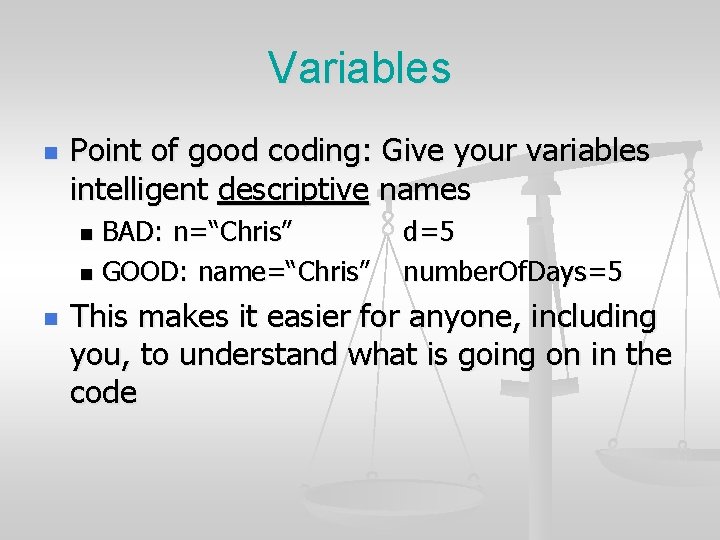
Variables n Point of good coding: Give your variables intelligent descriptive names BAD: n=“Chris” n GOOD: name=“Chris” n n d=5 number. Of. Days=5 This makes it easier for anyone, including you, to understand what is going on in the code
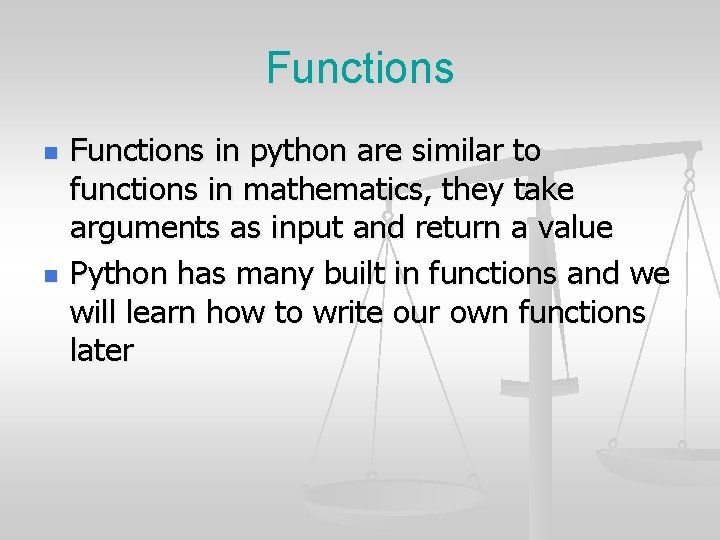
Functions n n Functions in python are similar to functions in mathematics, they take arguments as input and return a value Python has many built in functions and we will learn how to write our own functions later
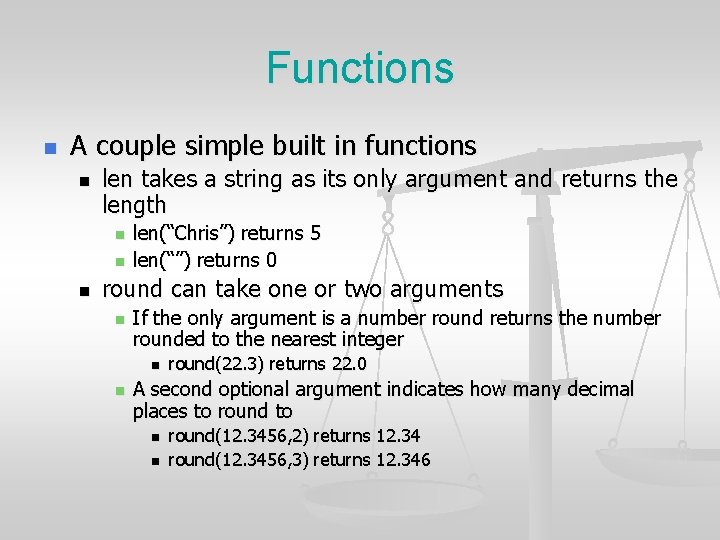
Functions n A couple simple built in functions n len takes a string as its only argument and returns the length n n n len(“Chris”) returns 5 len(“”) returns 0 round can take one or two arguments n If the only argument is a number round returns the number rounded to the nearest integer n n round(22. 3) returns 22. 0 A second optional argument indicates how many decimal places to round to n n round(12. 3456, 2) returns 12. 34 round(12. 3456, 3) returns 12. 346
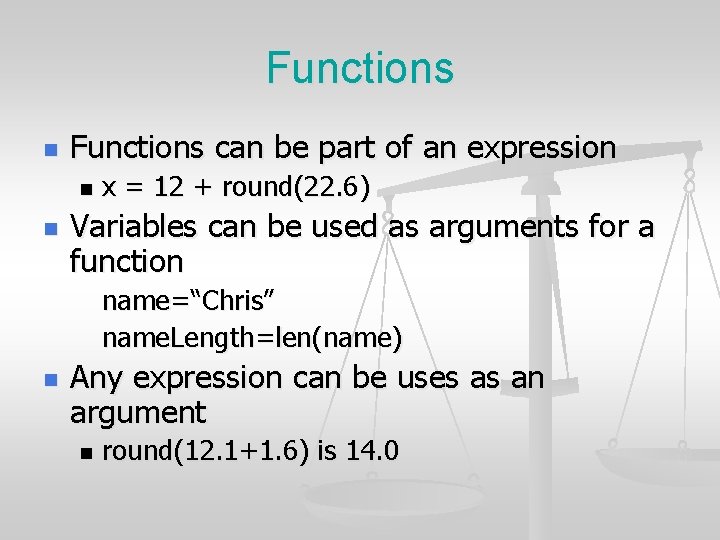
Functions n Functions can be part of an expression n n x = 12 + round(22. 6) Variables can be used as arguments for a function name=“Chris” name. Length=len(name) n Any expression can be uses as an argument n round(12. 1+1. 6) is 14. 0
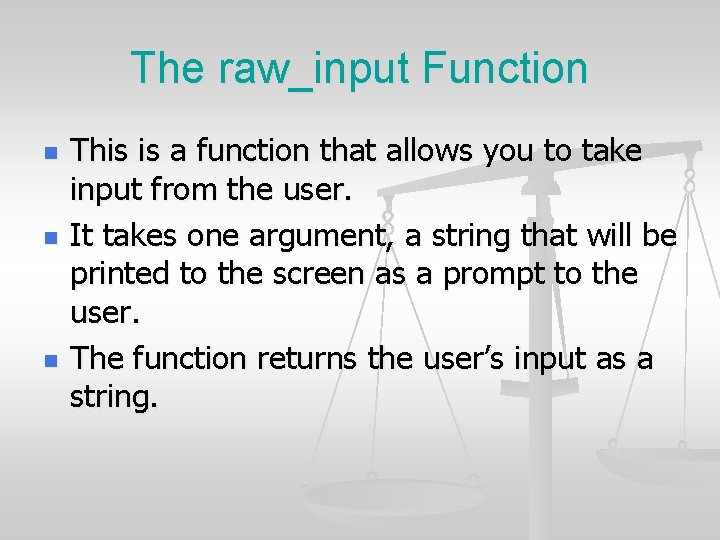
The raw_input Function n This is a function that allows you to take input from the user. It takes one argument, a string that will be printed to the screen as a prompt to the user. The function returns the user’s input as a string.
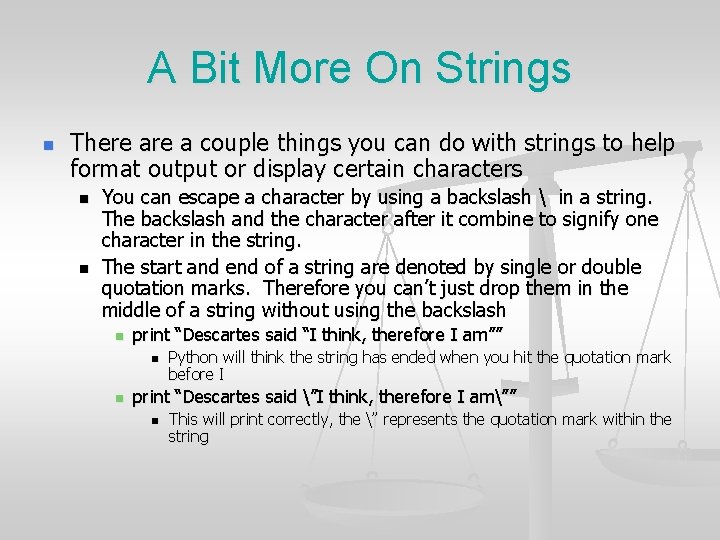
A Bit More On Strings n There a couple things you can do with strings to help format output or display certain characters n n You can escape a character by using a backslash in a string. The backslash and the character after it combine to signify one character in the string. The start and end of a string are denoted by single or double quotation marks. Therefore you can’t just drop them in the middle of a string without using the backslash n print “Descartes said “I think, therefore I am”” n n Python will think the string has ended when you hit the quotation mark before I print “Descartes said ”I think, therefore I am”” n This will print correctly, the ” represents the quotation mark within the string
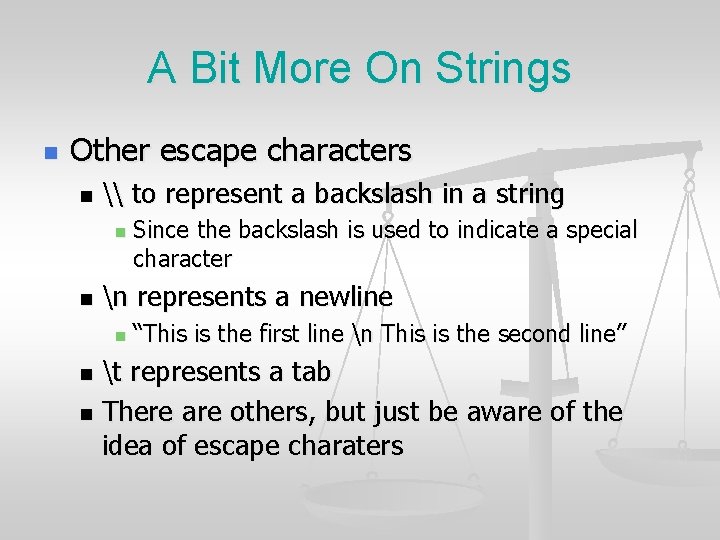
A Bit More On Strings n Other escape characters n \ to represent a backslash in a string n n Since the backslash is used to indicate a special character n represents a newline n “This is the first line n This is the second line” t represents a tab n There are others, but just be aware of the idea of escape charaters n
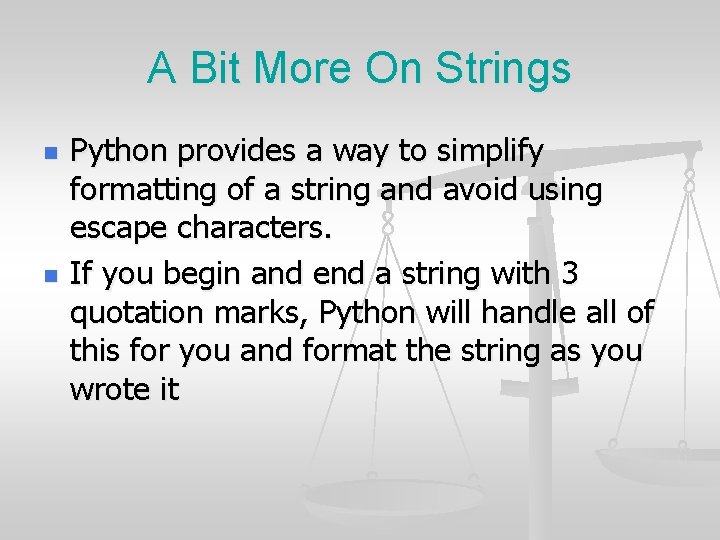
A Bit More On Strings n n Python provides a way to simplify formatting of a string and avoid using escape characters. If you begin and end a string with 3 quotation marks, Python will handle all of this for you and format the string as you wrote it