Introduction to Programming Lecture 44 Class Matrix class
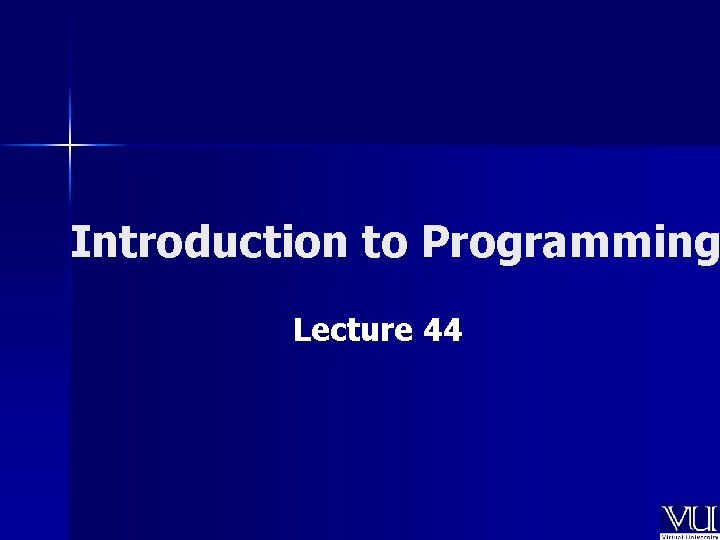
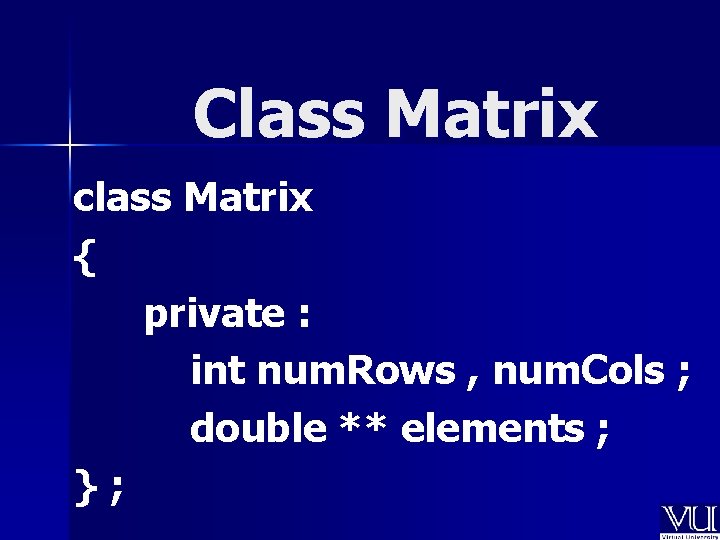
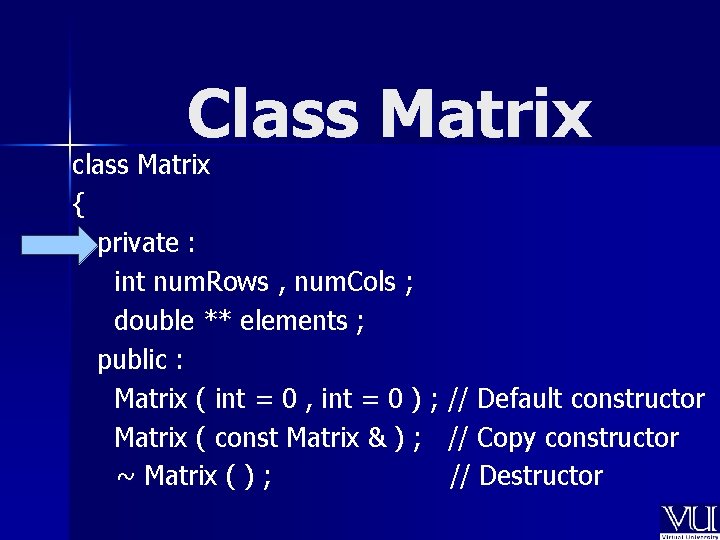
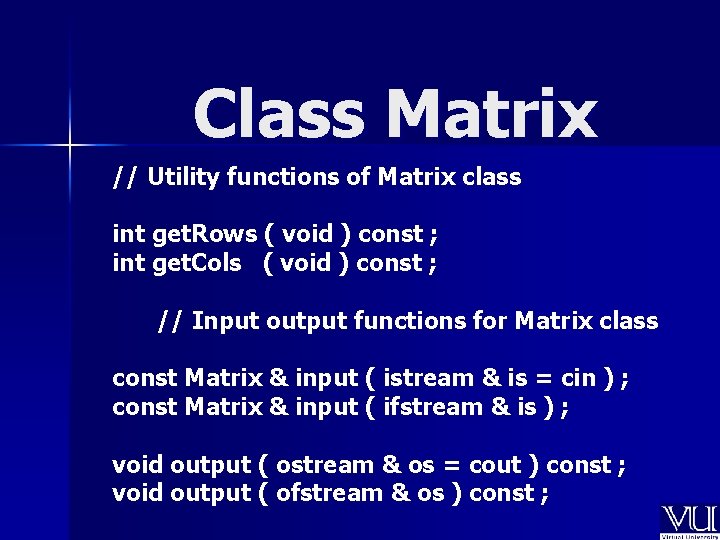
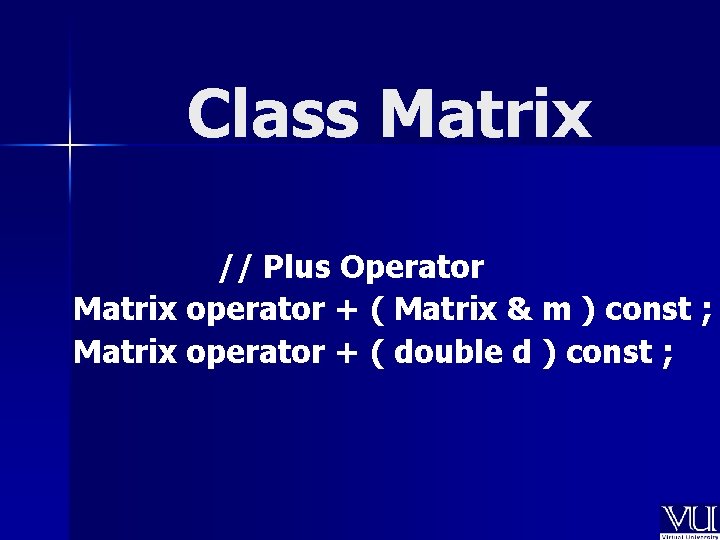
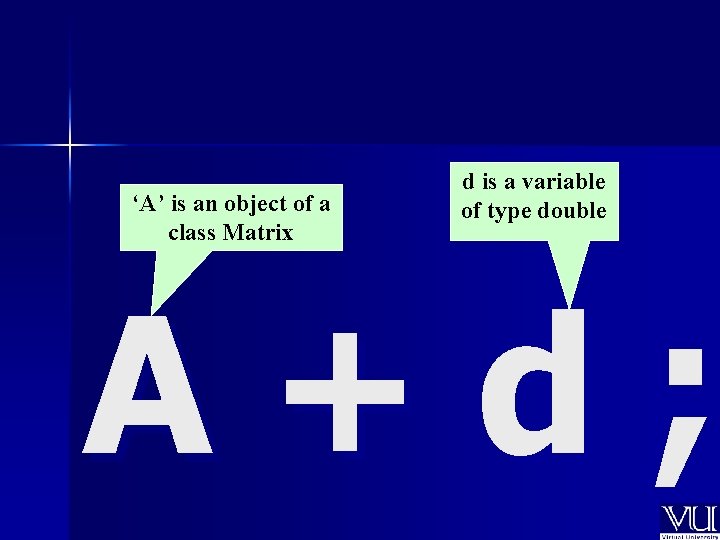
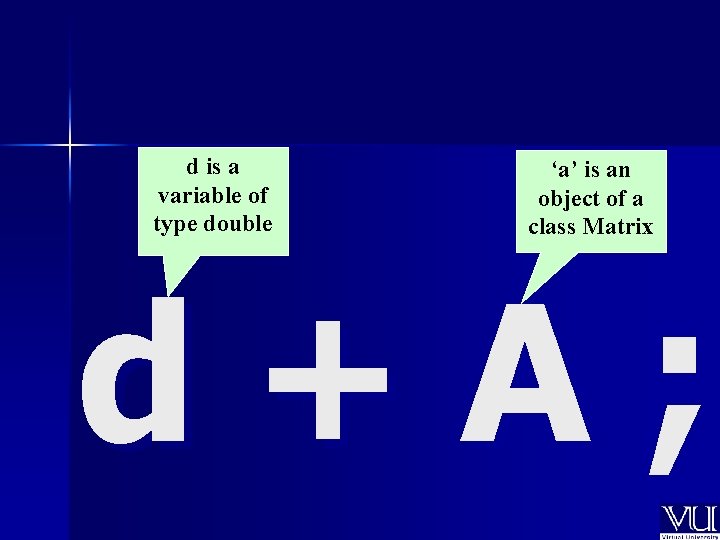
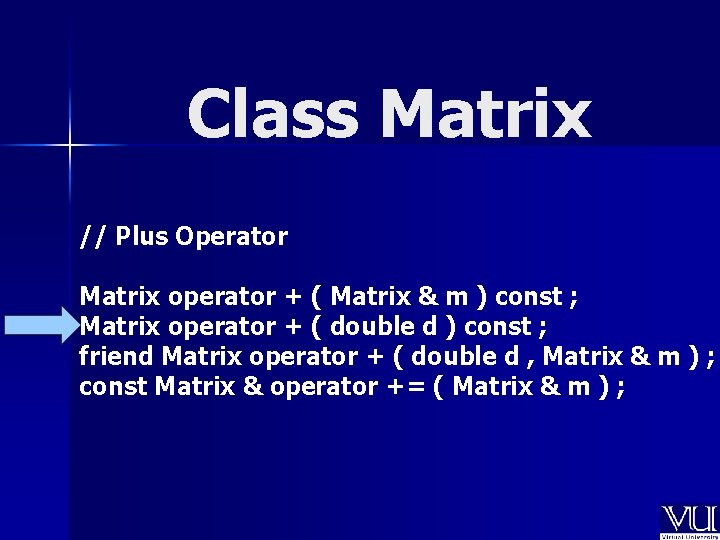
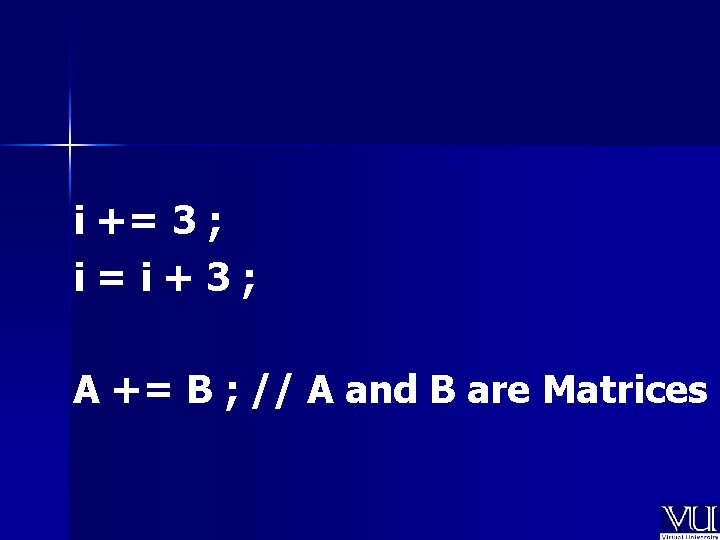
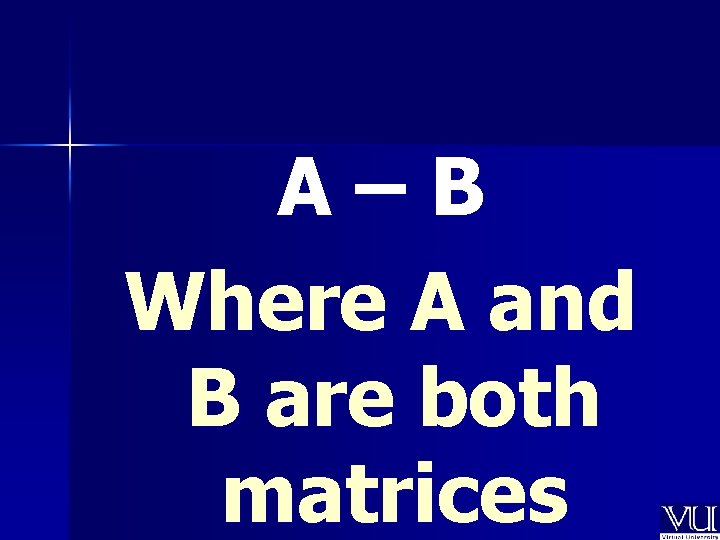
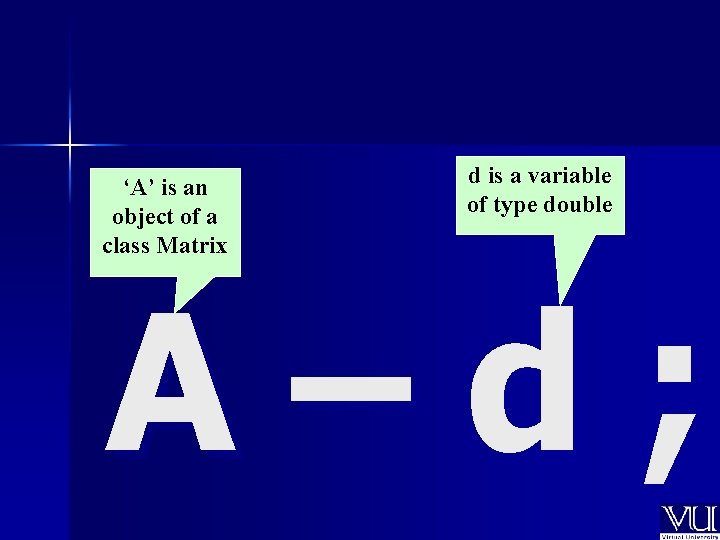
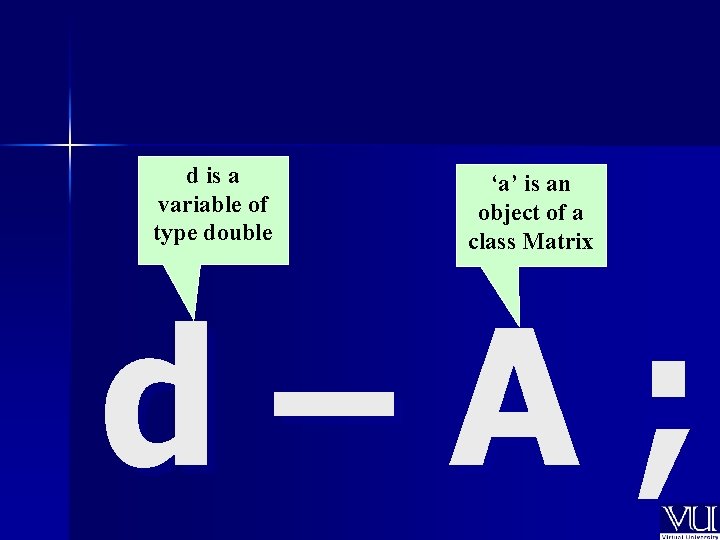
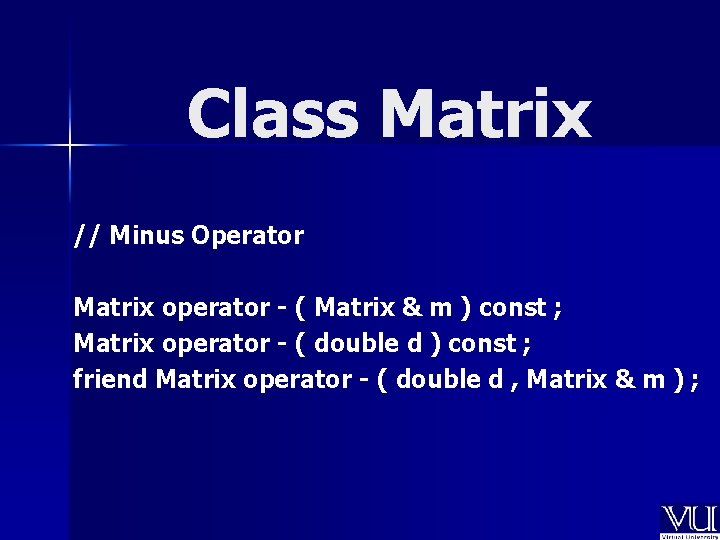
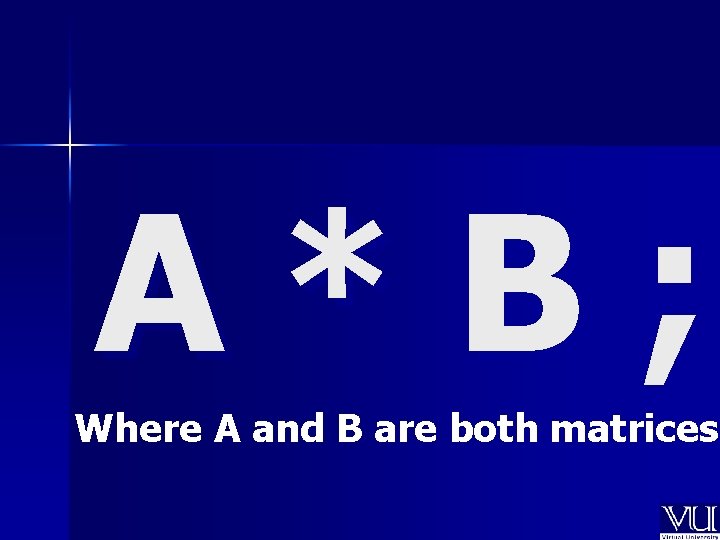
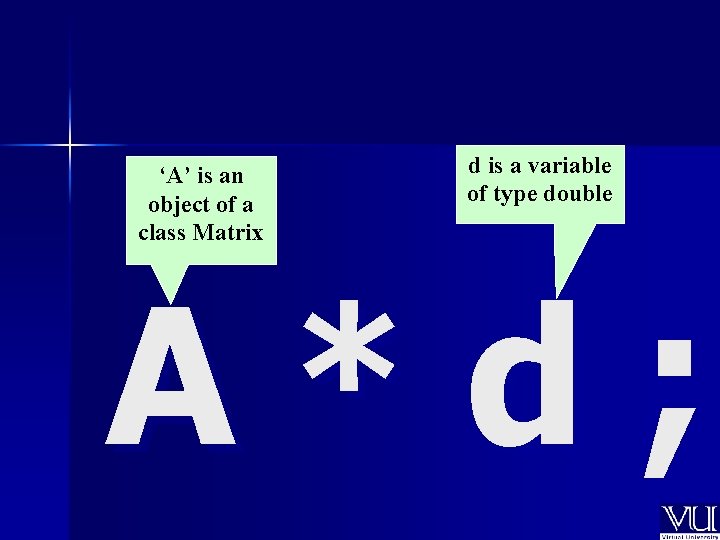
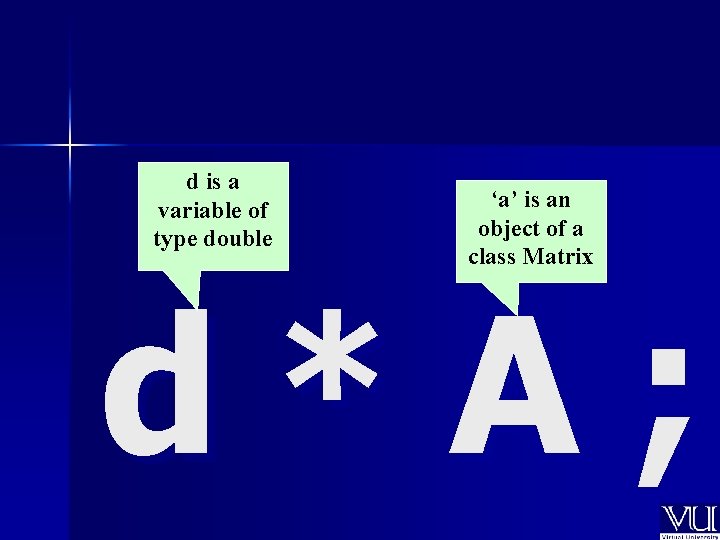
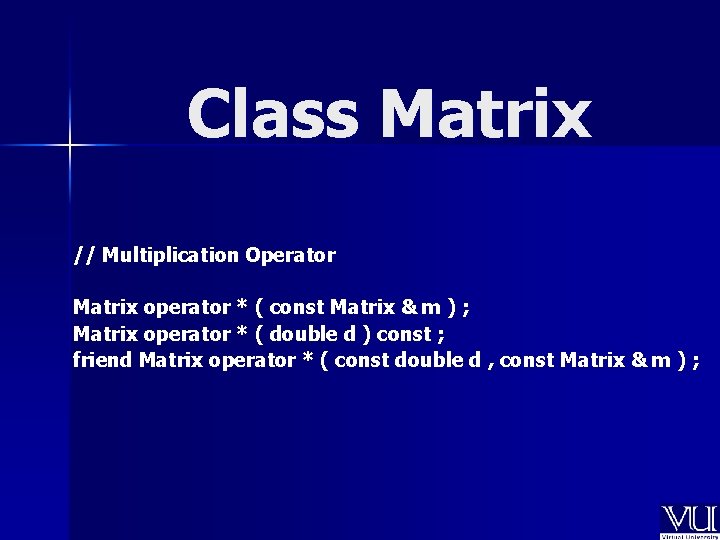
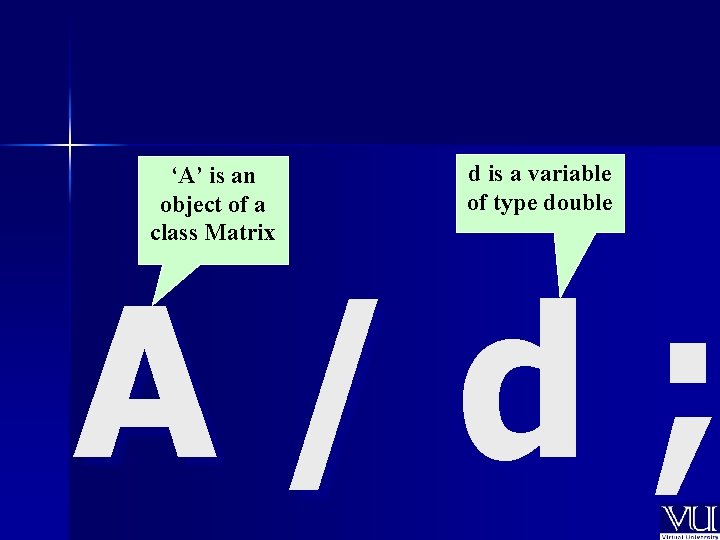
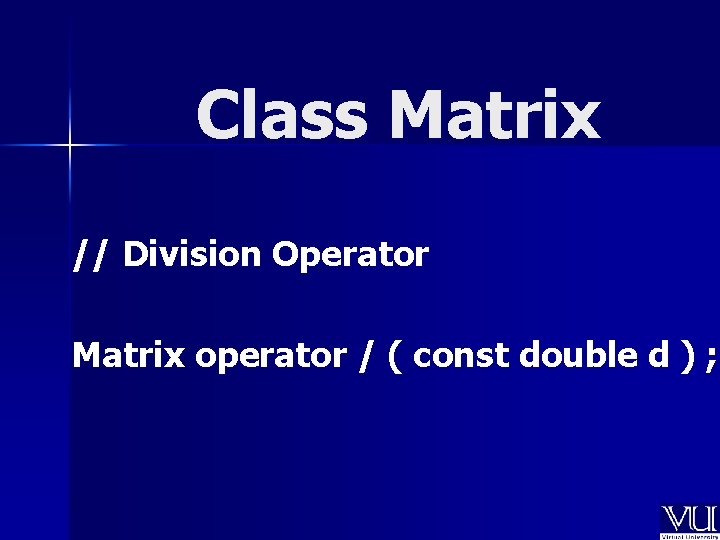
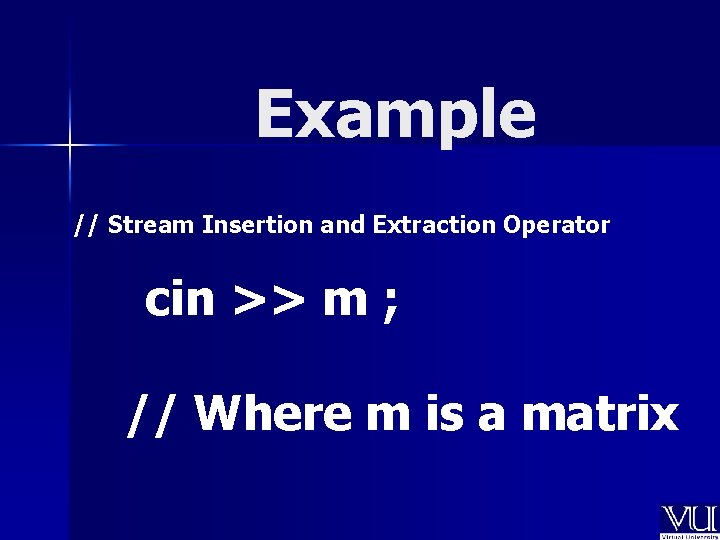
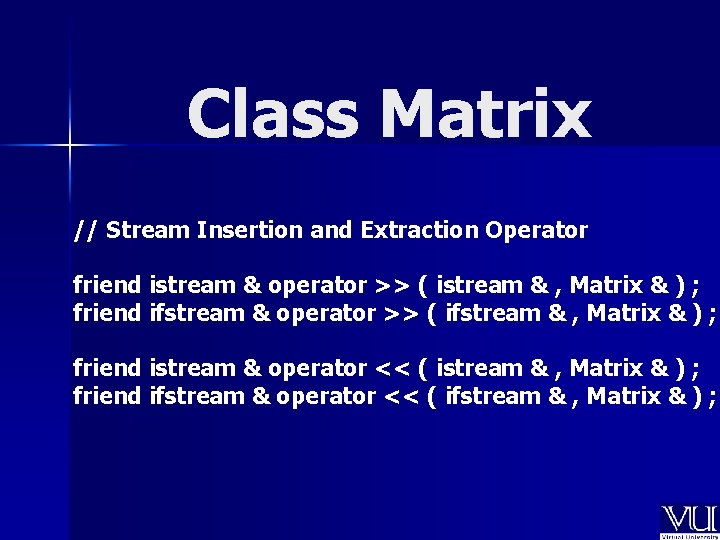
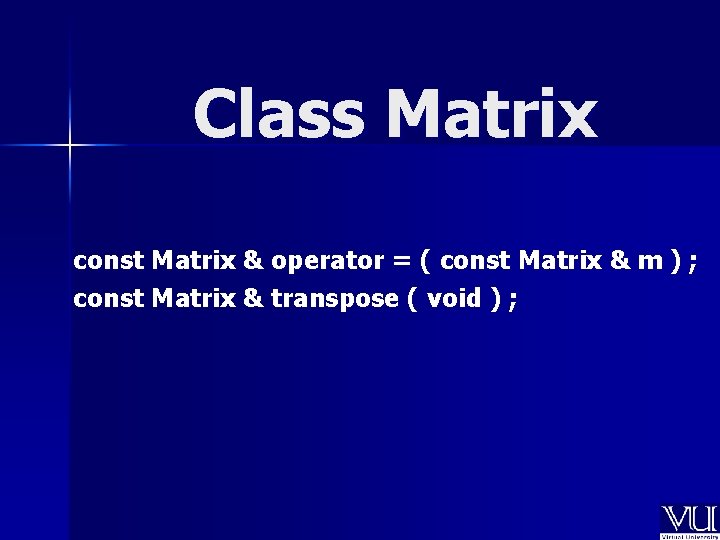
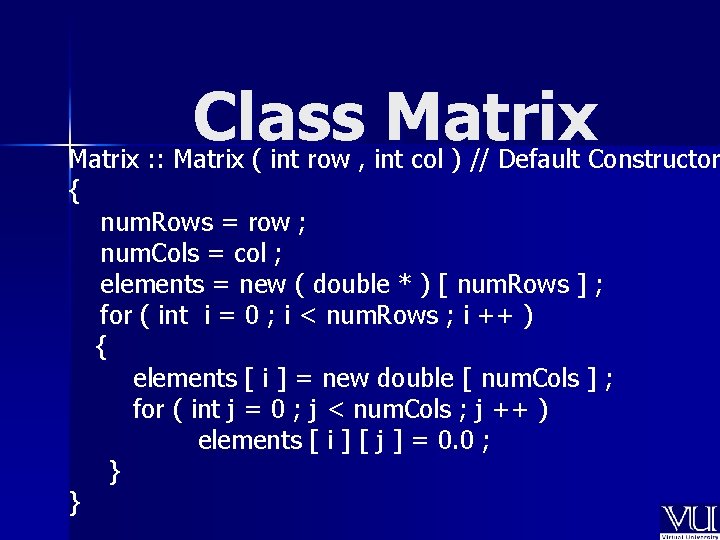
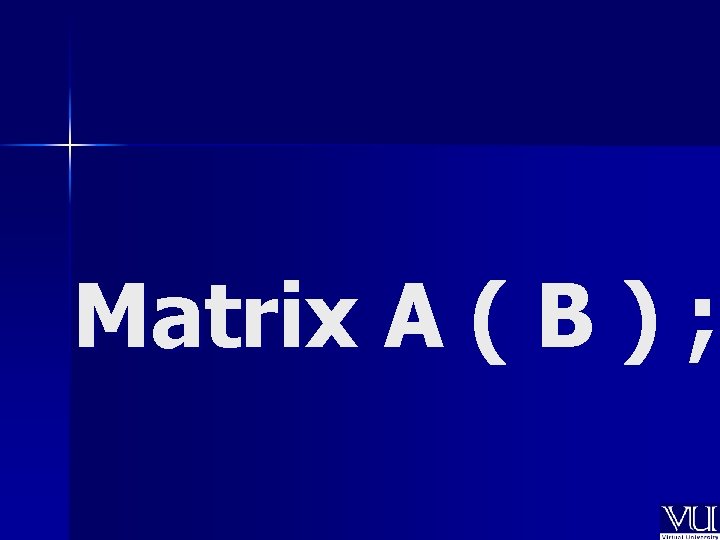
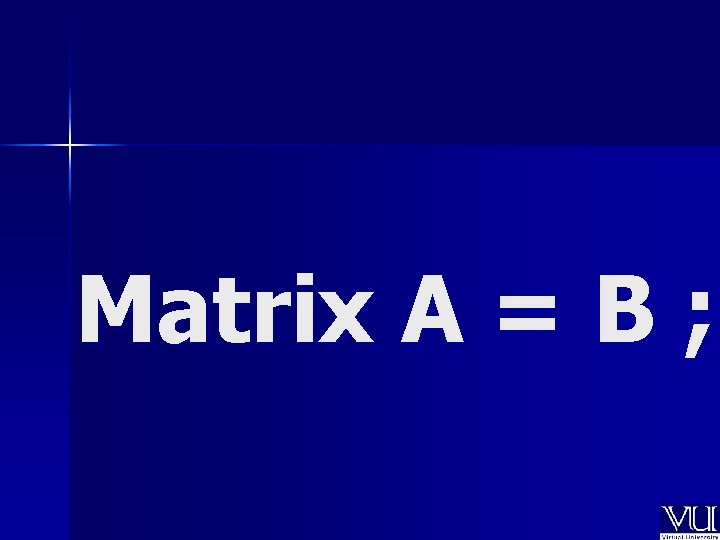
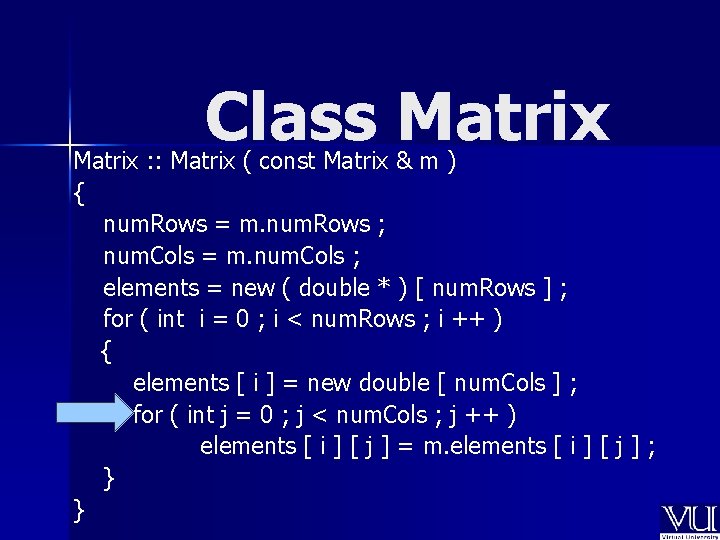
![Class Matrix : : ~ Matrix ( void ) { delete [ ] elements Class Matrix : : ~ Matrix ( void ) { delete [ ] elements](https://slidetodoc.com/presentation_image_h2/e4e19facb4518122c3f01f62f2ea1d0f/image-27.jpg)
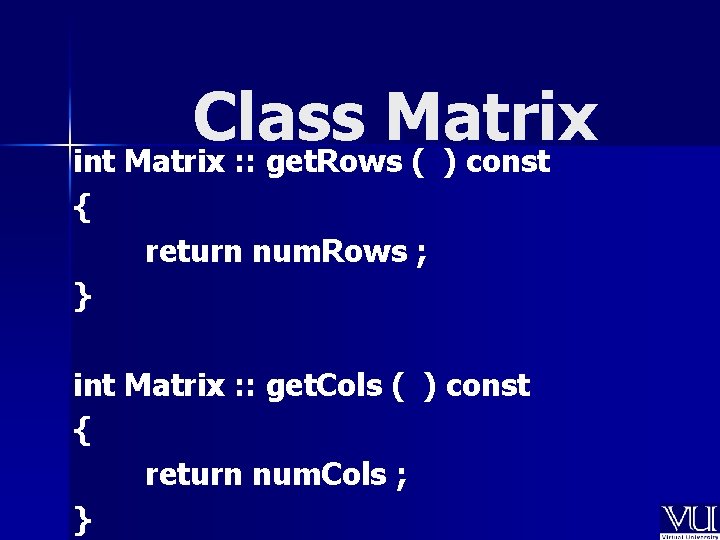
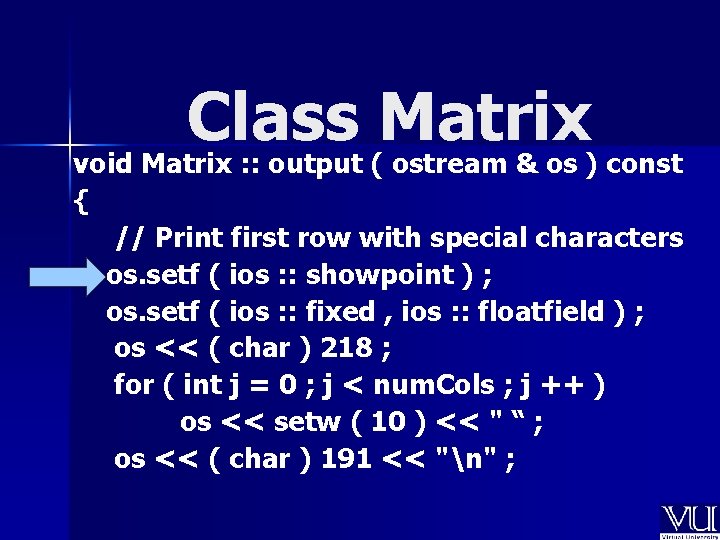
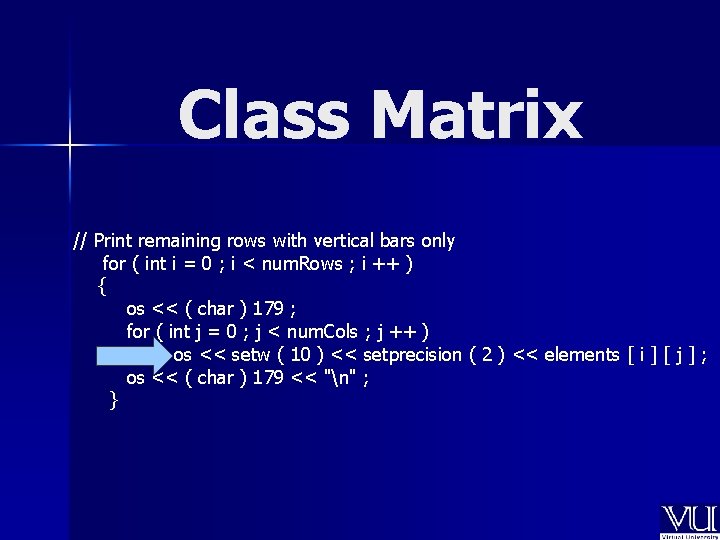
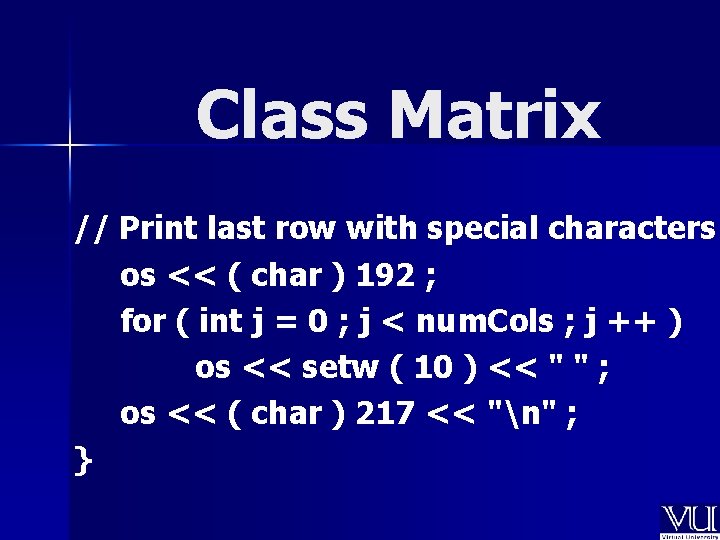
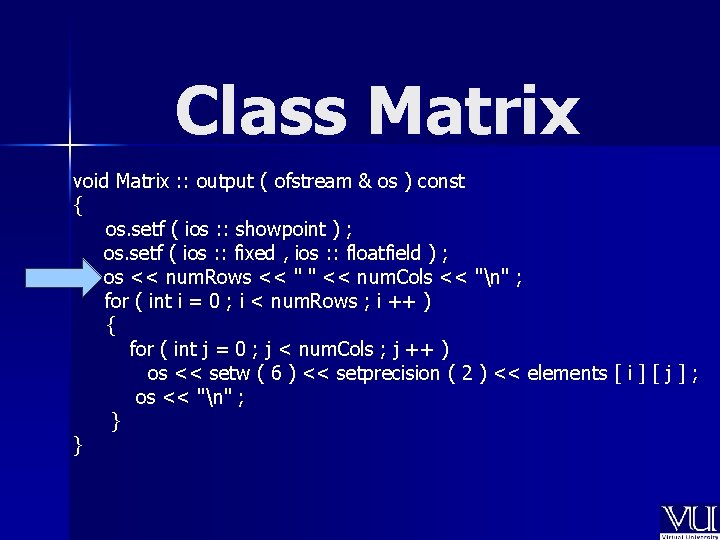
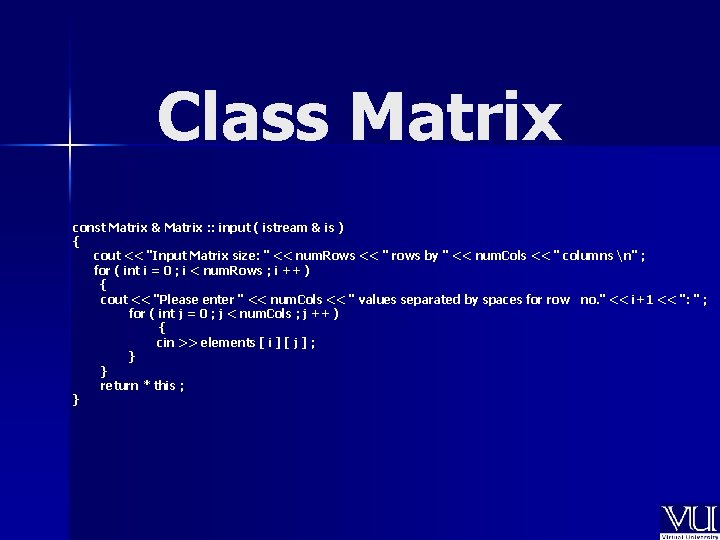
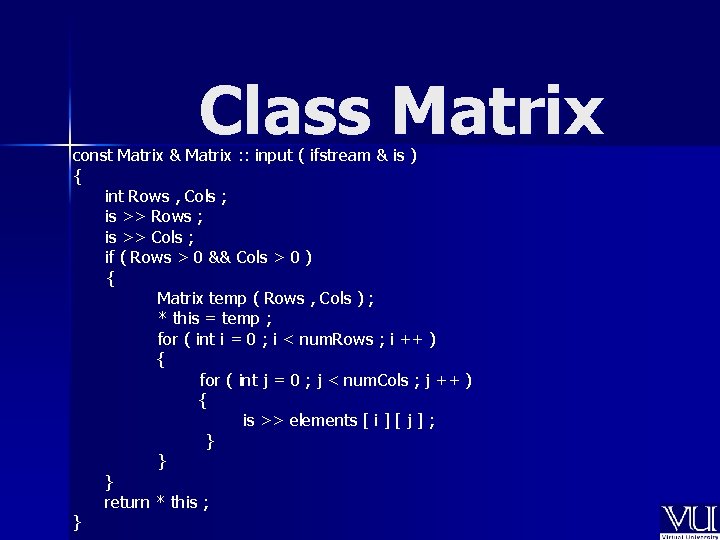
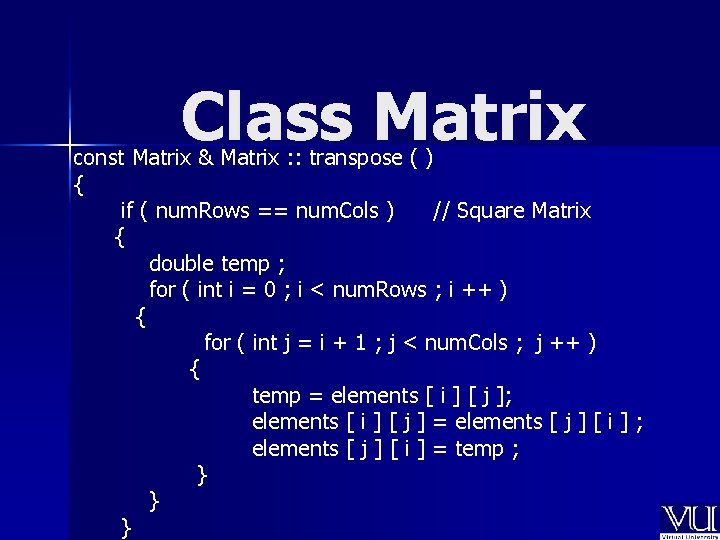
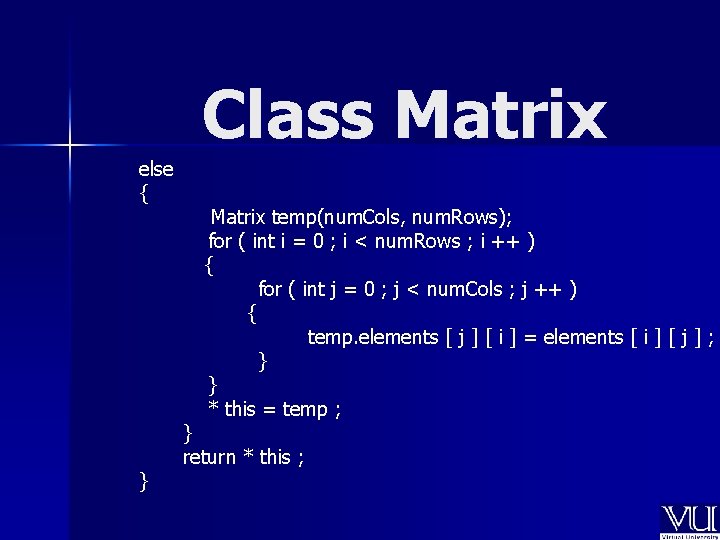
- Slides: 36
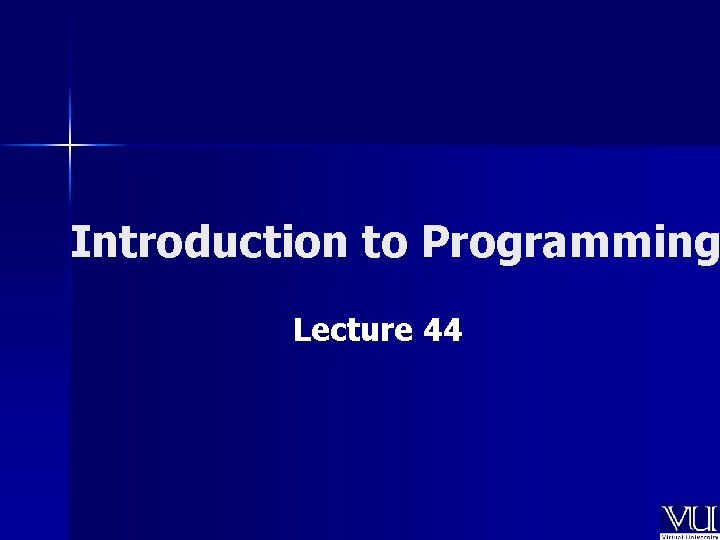
Introduction to Programming Lecture 44
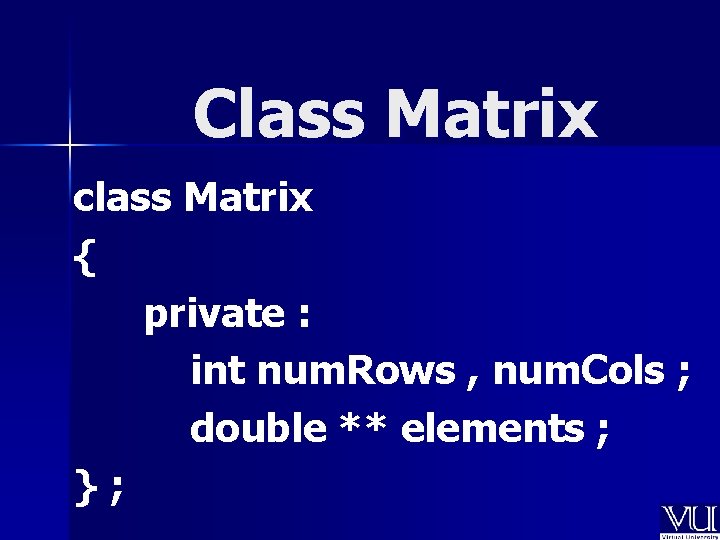
Class Matrix class Matrix { private : int num. Rows , num. Cols ; double ** elements ; };
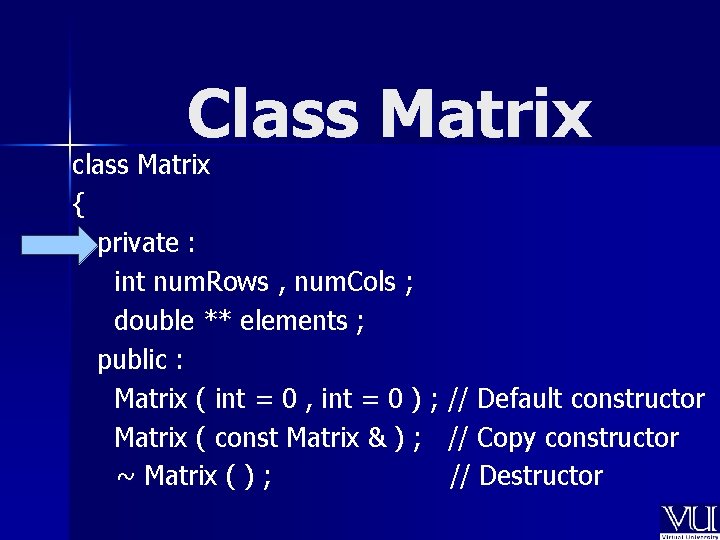
Class Matrix class Matrix { private : int num. Rows , num. Cols ; double ** elements ; public : Matrix ( int = 0 , int = 0 ) ; // Default constructor Matrix ( const Matrix & ) ; // Copy constructor ~ Matrix ( ) ; // Destructor
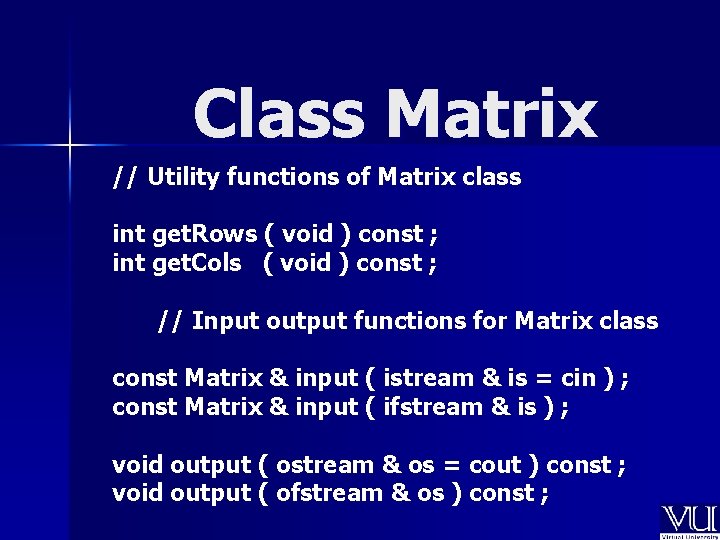
Class Matrix // Utility functions of Matrix class int get. Rows ( void ) const ; int get. Cols ( void ) const ; // Input output functions for Matrix class const Matrix & input ( istream & is = cin ) ; const Matrix & input ( ifstream & is ) ; void output ( ostream & os = cout ) const ; void output ( ofstream & os ) const ;
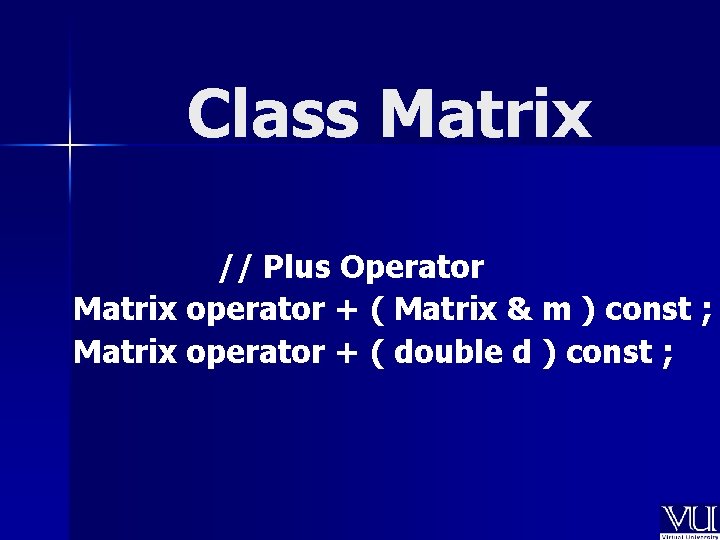
Class Matrix // Plus Operator Matrix operator + ( Matrix & m ) const ; Matrix operator + ( double d ) const ;
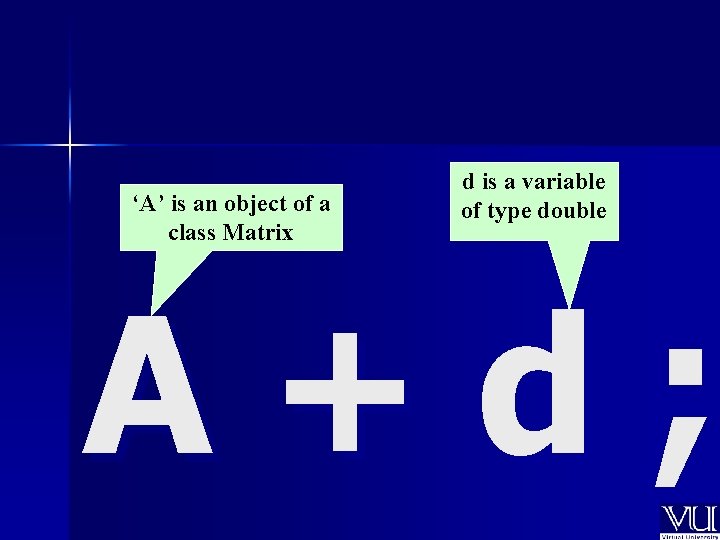
‘A’ is an object of a class Matrix d is a variable of type double A+d;
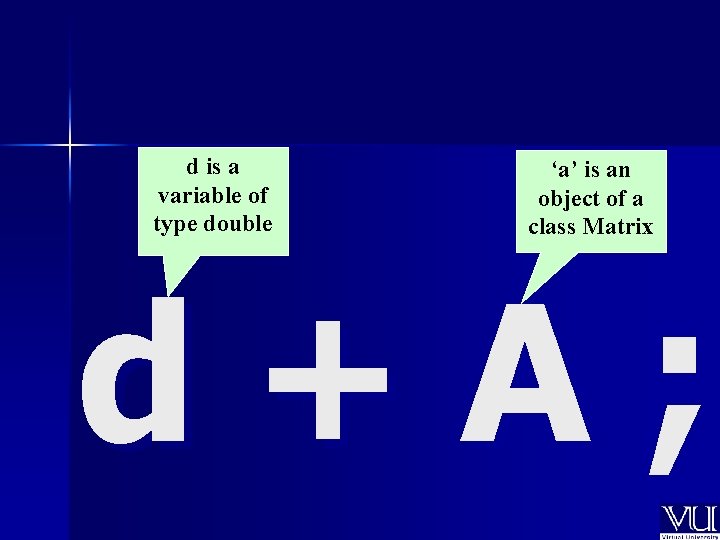
d is a variable of type double ‘a’ is an object of a class Matrix d+A;
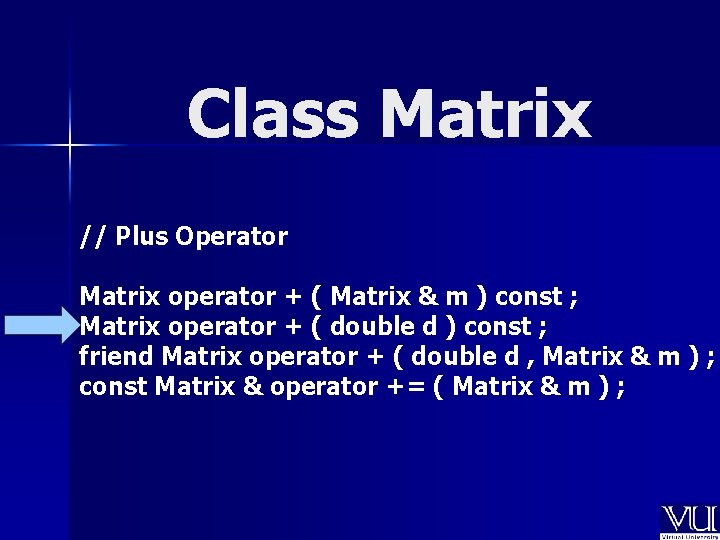
Class Matrix // Plus Operator Matrix operator + ( Matrix & m ) const ; Matrix operator + ( double d ) const ; friend Matrix operator + ( double d , Matrix & m ) ; const Matrix & operator += ( Matrix & m ) ;
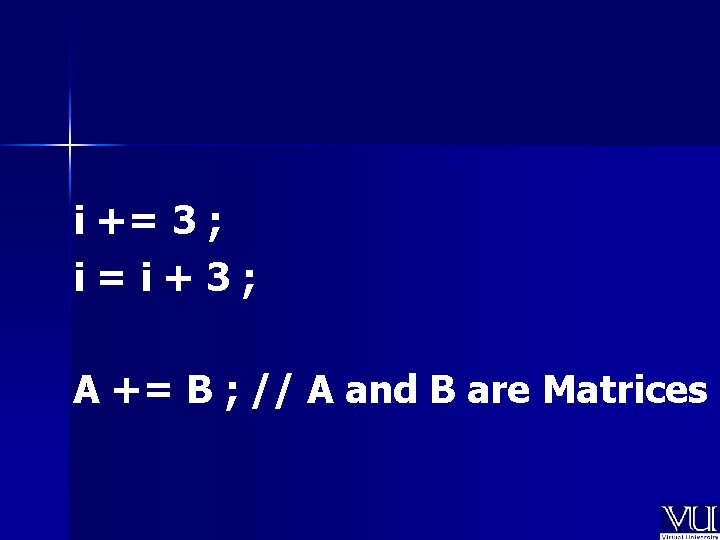
i += 3 ; i=i+3; A += B ; // A and B are Matrices
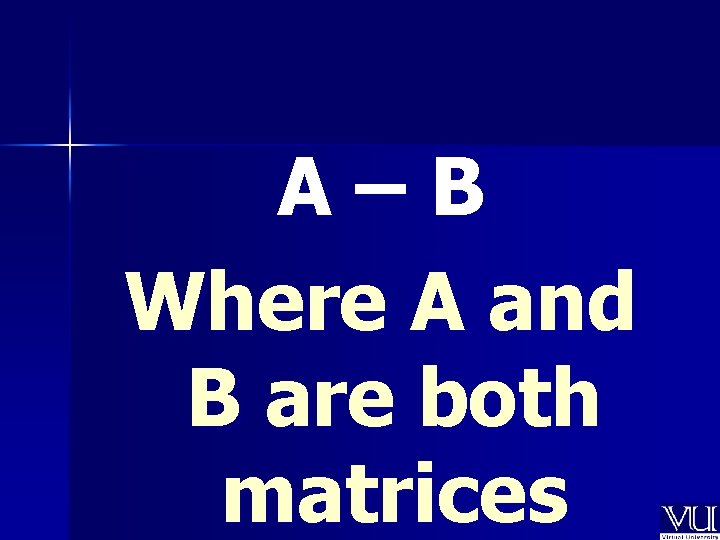
A–B Where A and B are both matrices
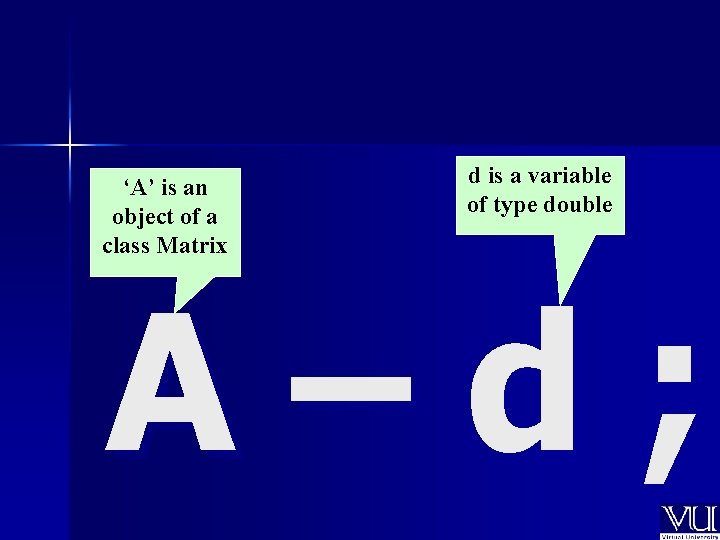
‘A’ is an object of a class Matrix d is a variable of type double A–d;
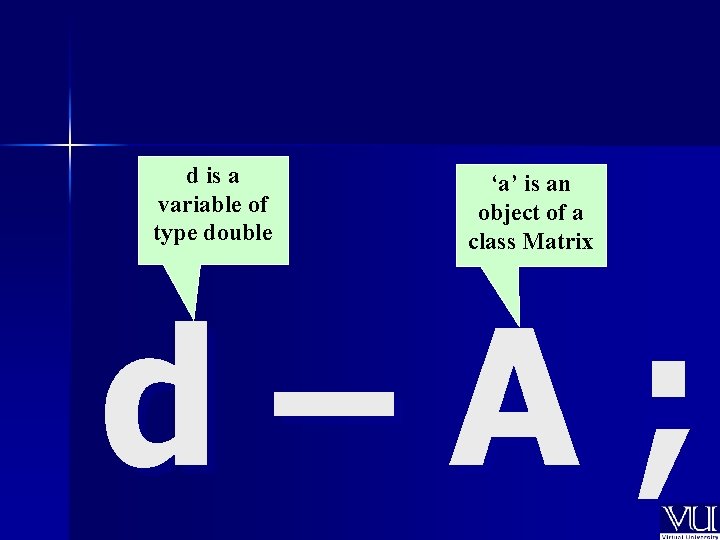
d is a variable of type double ‘a’ is an object of a class Matrix d–A;
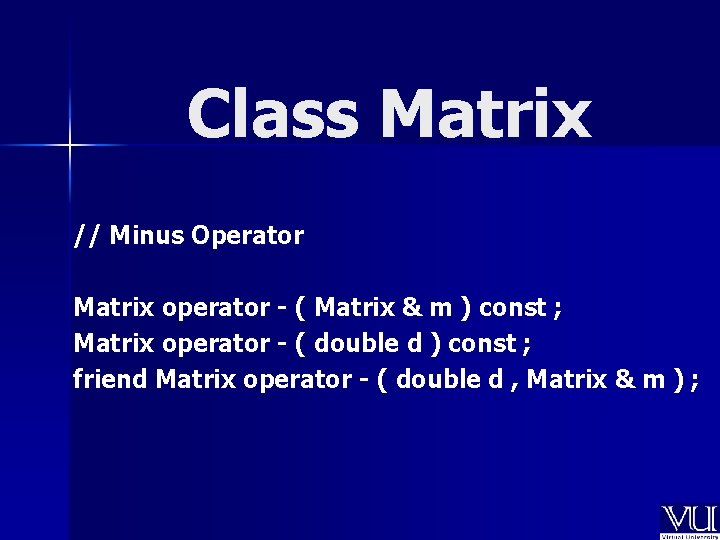
Class Matrix // Minus Operator Matrix operator - ( Matrix & m ) const ; Matrix operator - ( double d ) const ; friend Matrix operator - ( double d , Matrix & m ) ;
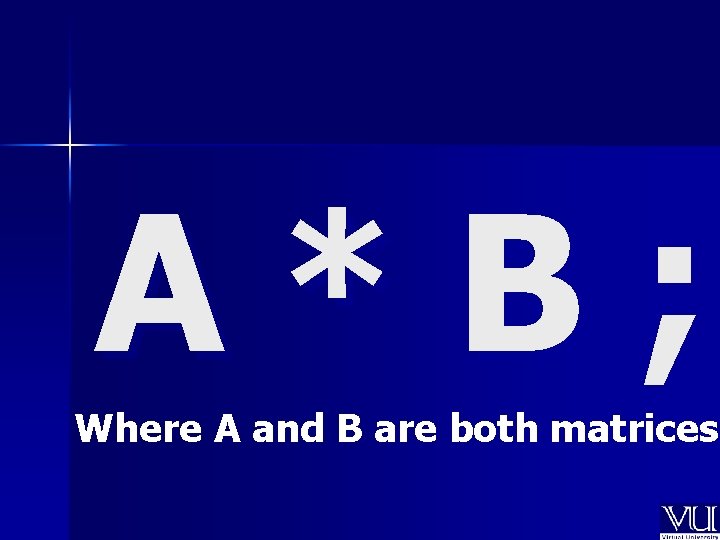
A*B; Where A and B are both matrices
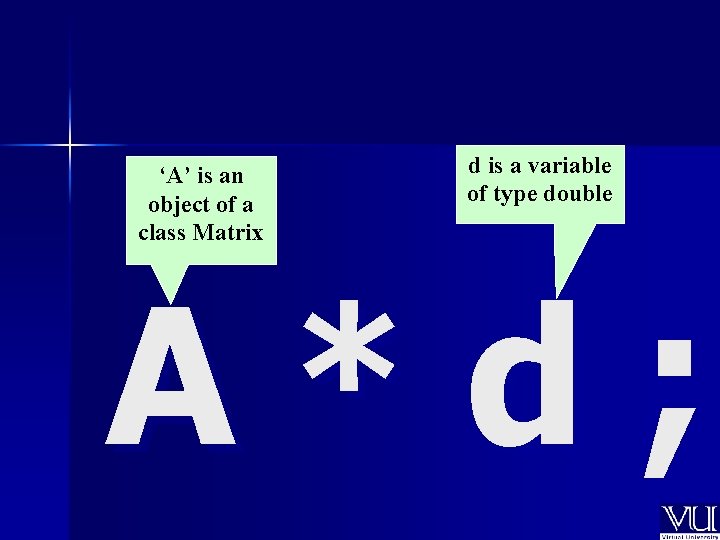
‘A’ is an object of a class Matrix d is a variable of type double A*d;
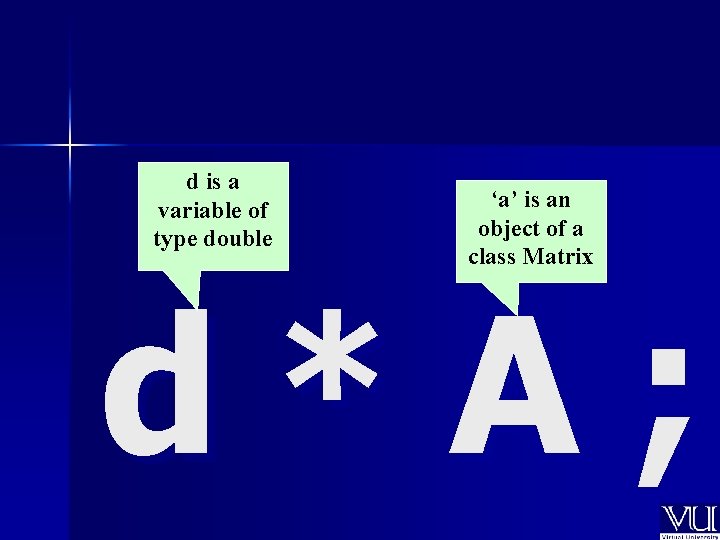
d is a variable of type double ‘a’ is an object of a class Matrix d*A;
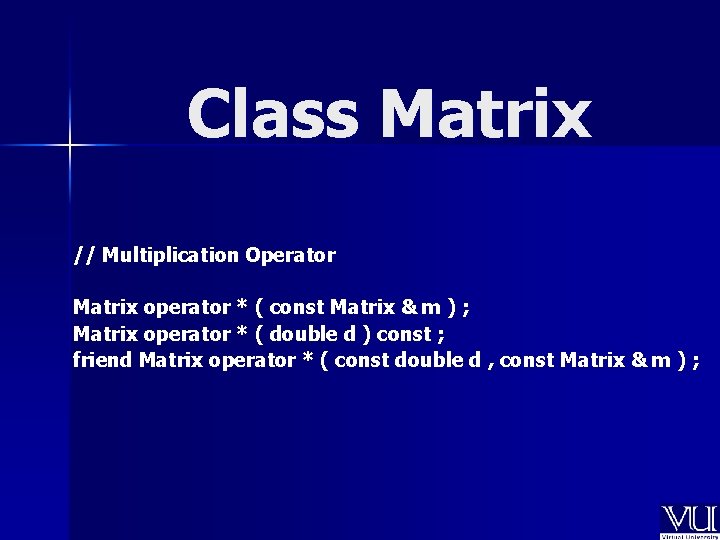
Class Matrix // Multiplication Operator Matrix operator * ( const Matrix & m ) ; Matrix operator * ( double d ) const ; friend Matrix operator * ( const double d , const Matrix & m ) ;
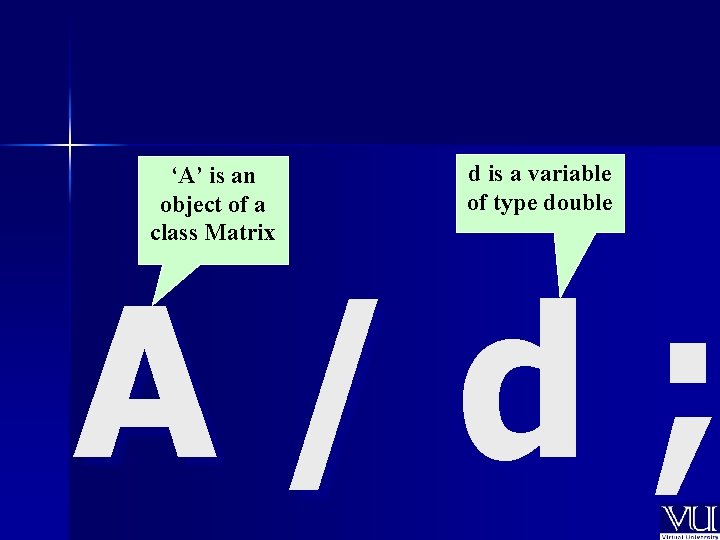
‘A’ is an object of a class Matrix d is a variable of type double A/d;
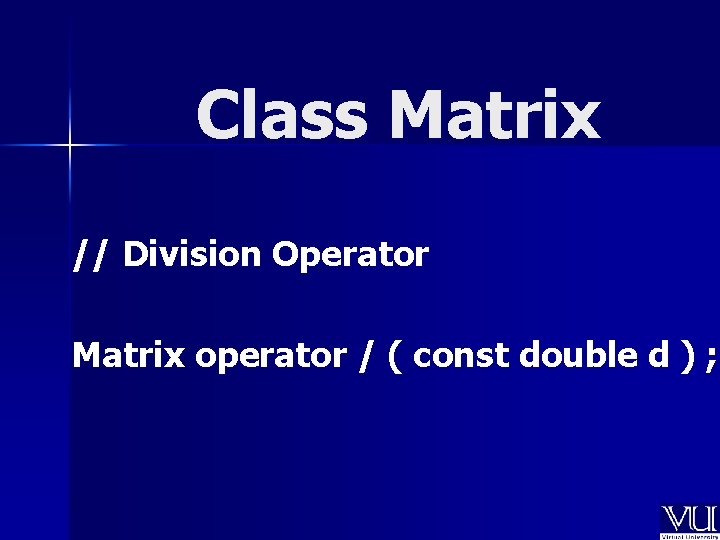
Class Matrix // Division Operator Matrix operator / ( const double d ) ;
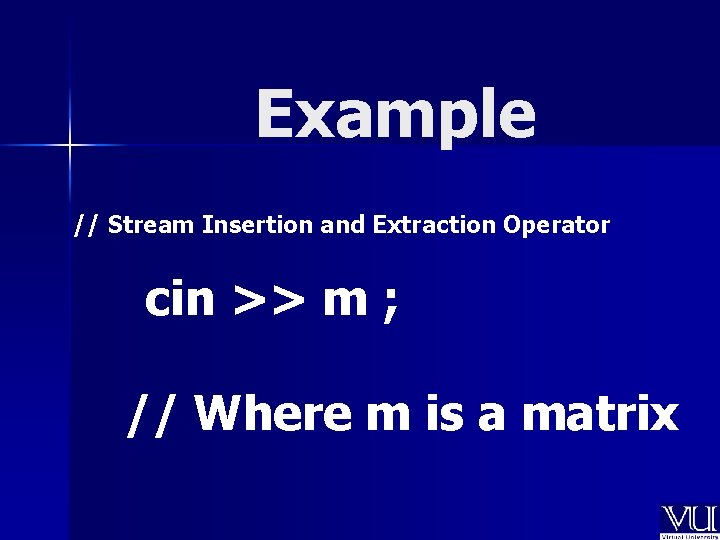
Example // Stream Insertion and Extraction Operator cin >> m ; // Where m is a matrix
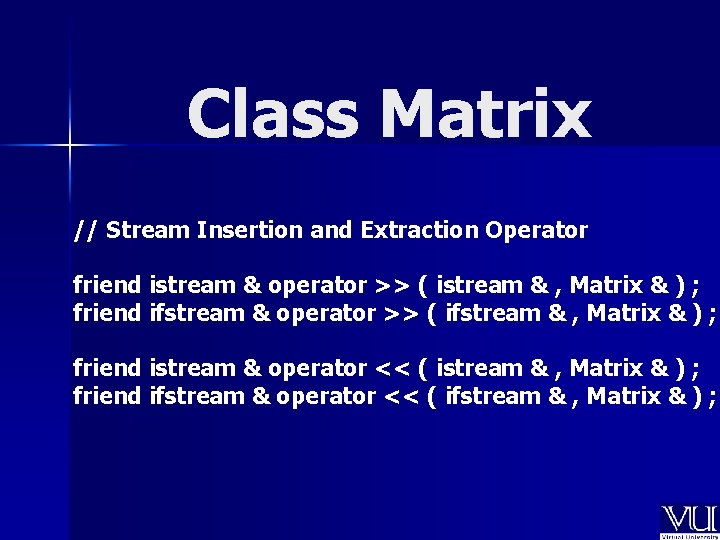
Class Matrix // Stream Insertion and Extraction Operator friend istream & operator >> ( istream & , Matrix & ) ; friend ifstream & operator >> ( ifstream & , Matrix & ) ; friend istream & operator << ( istream & , Matrix & ) ; friend ifstream & operator << ( ifstream & , Matrix & ) ;
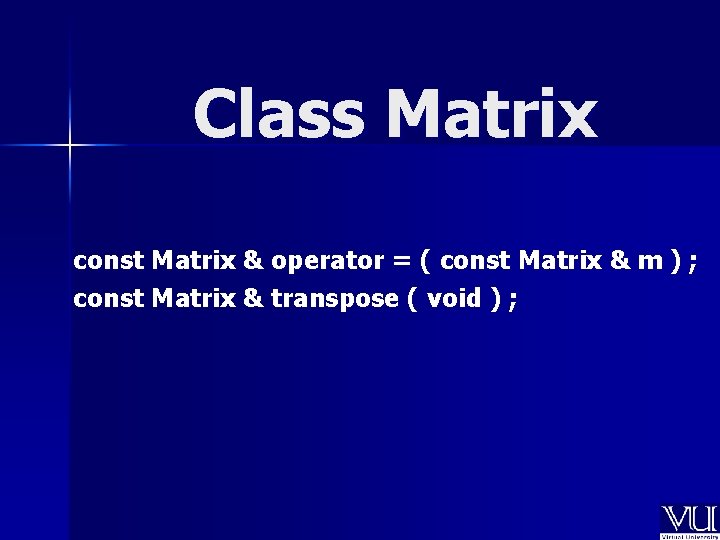
Class Matrix const Matrix & operator = ( const Matrix & m ) ; const Matrix & transpose ( void ) ;
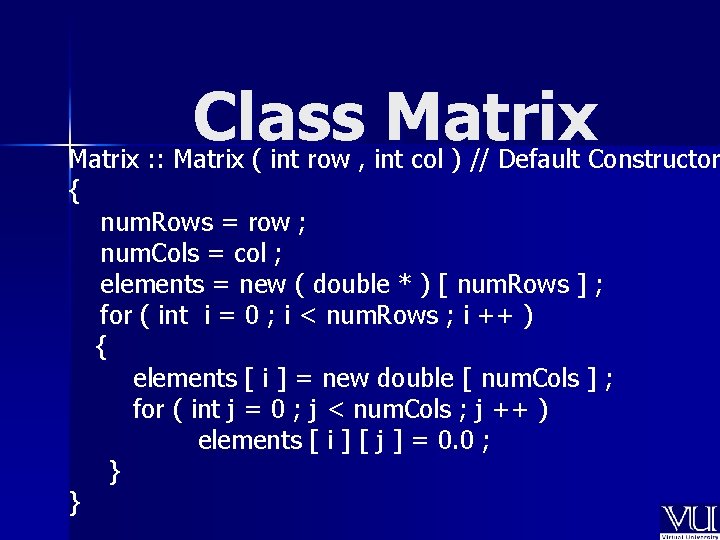
Class Matrix : : Matrix ( int row , int col ) // Default Constructor { num. Rows = row ; num. Cols = col ; elements = new ( double * ) [ num. Rows ] ; for ( int i = 0 ; i < num. Rows ; i ++ ) { elements [ i ] = new double [ num. Cols ] ; for ( int j = 0 ; j < num. Cols ; j ++ ) elements [ i ] [ j ] = 0. 0 ; } }
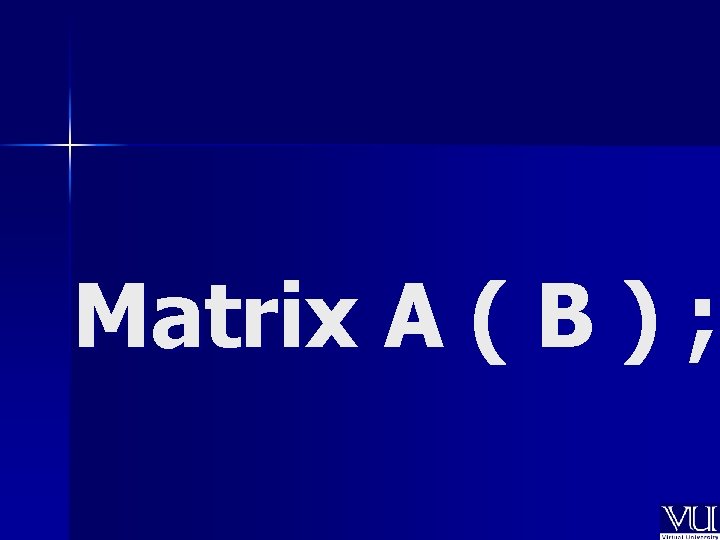
Matrix A ( B ) ;
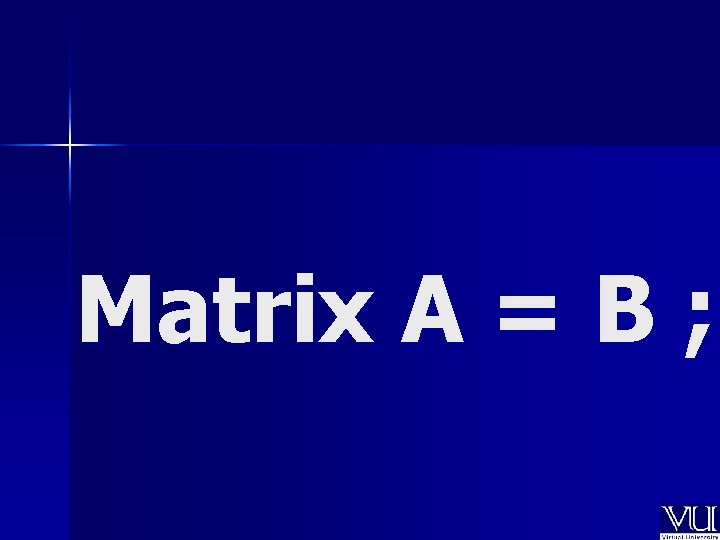
Matrix A = B ;
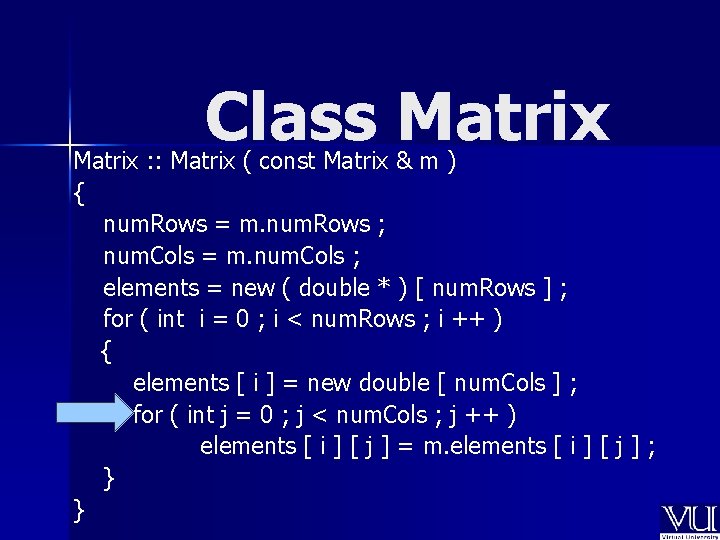
Class Matrix : : Matrix ( const Matrix & m ) { num. Rows = m. num. Rows ; num. Cols = m. num. Cols ; elements = new ( double * ) [ num. Rows ] ; for ( int i = 0 ; i < num. Rows ; i ++ ) { elements [ i ] = new double [ num. Cols ] ; for ( int j = 0 ; j < num. Cols ; j ++ ) elements [ i ] [ j ] = m. elements [ i ] [ j ] ; } }
![Class Matrix Matrix void delete elements Class Matrix : : ~ Matrix ( void ) { delete [ ] elements](https://slidetodoc.com/presentation_image_h2/e4e19facb4518122c3f01f62f2ea1d0f/image-27.jpg)
Class Matrix : : ~ Matrix ( void ) { delete [ ] elements ; }
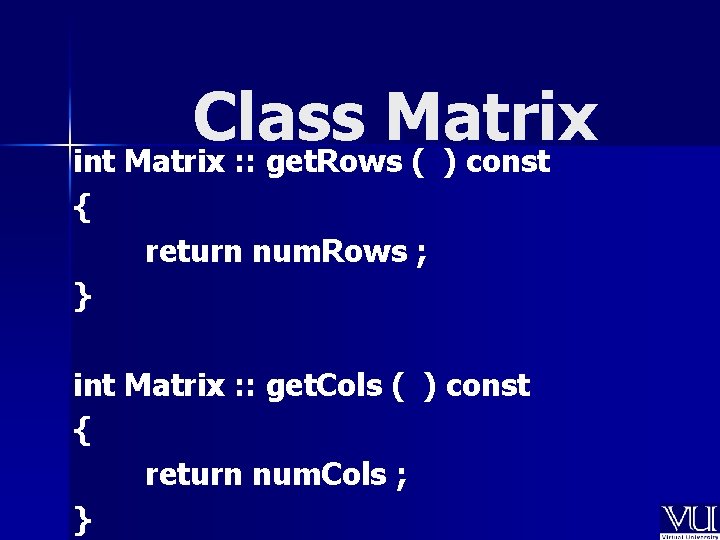
Class Matrix int Matrix : : get. Rows ( ) const { return num. Rows ; } int Matrix : : get. Cols ( ) const { return num. Cols ; }
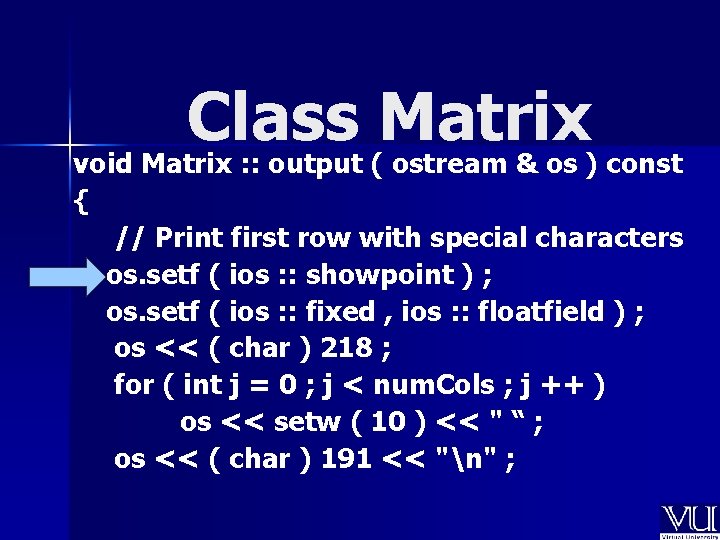
Class Matrix void Matrix : : output ( ostream & os ) const { // Print first row with special characters os. setf ( ios : : showpoint ) ; os. setf ( ios : : fixed , ios : : floatfield ) ; os << ( char ) 218 ; for ( int j = 0 ; j < num. Cols ; j ++ ) os << setw ( 10 ) << " “ ; os << ( char ) 191 << "n" ;
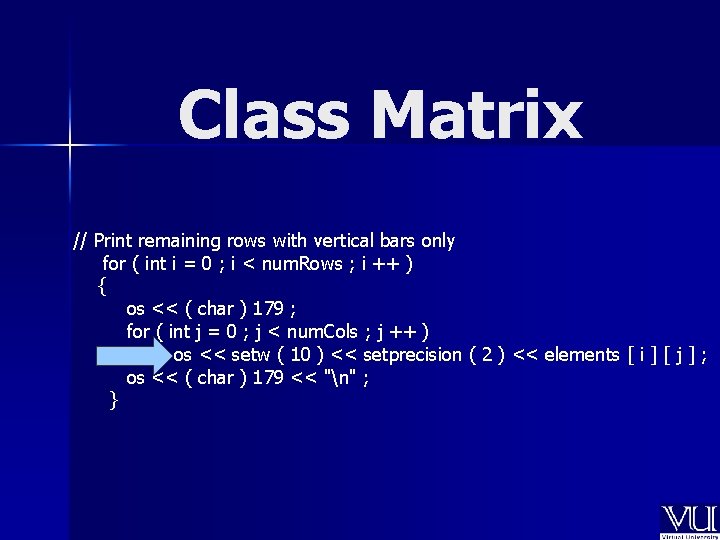
Class Matrix // Print remaining rows with vertical bars only for ( int i = 0 ; i < num. Rows ; i ++ ) { os << ( char ) 179 ; for ( int j = 0 ; j < num. Cols ; j ++ ) os << setw ( 10 ) << setprecision ( 2 ) << elements [ i ] [ j ] ; os << ( char ) 179 << "n" ; }
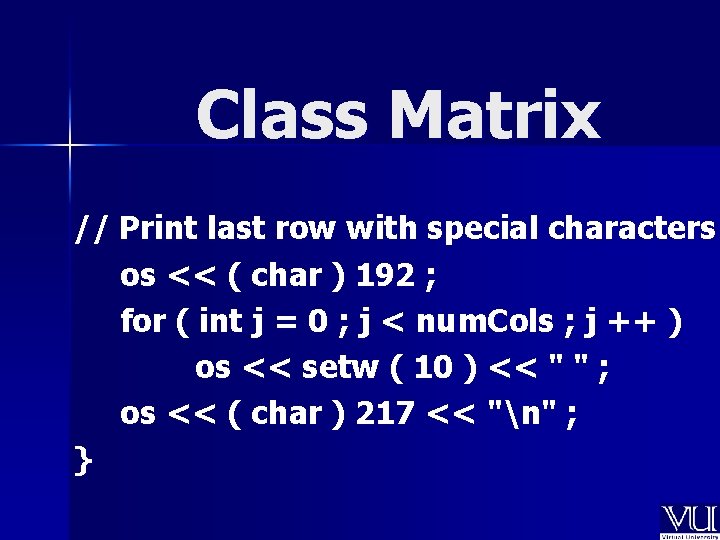
Class Matrix // Print last row with special characters os << ( char ) 192 ; for ( int j = 0 ; j < num. Cols ; j ++ ) os << setw ( 10 ) << " " ; os << ( char ) 217 << "n" ; }
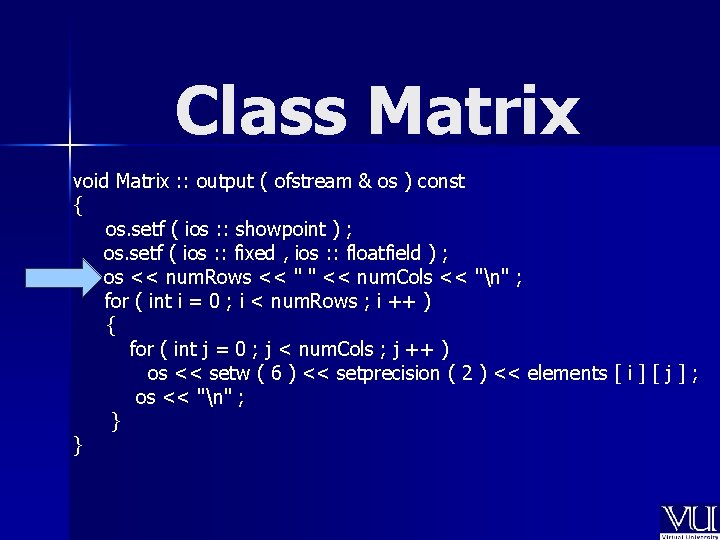
Class Matrix void Matrix : : output ( ofstream & os ) const { os. setf ( ios : : showpoint ) ; os. setf ( ios : : fixed , ios : : floatfield ) ; os << num. Rows << " " << num. Cols << "n" ; for ( int i = 0 ; i < num. Rows ; i ++ ) { for ( int j = 0 ; j < num. Cols ; j ++ ) os << setw ( 6 ) << setprecision ( 2 ) << elements [ i ] [ j ] ; os << "n" ; } }
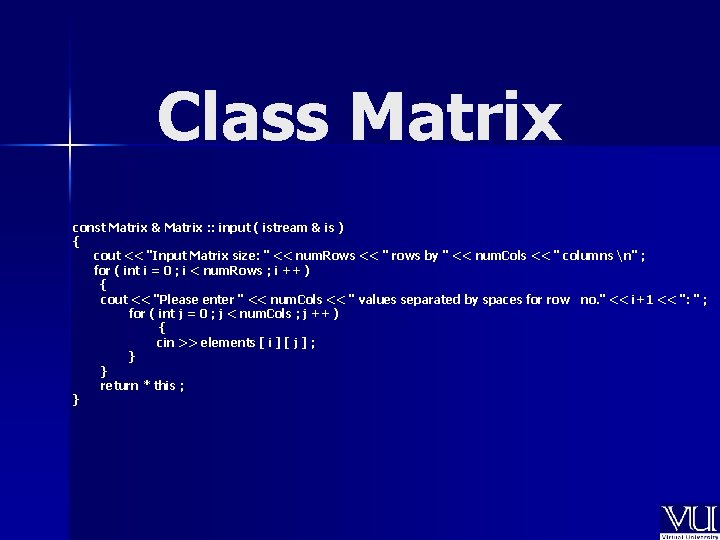
Class Matrix const Matrix & Matrix : : input ( istream & is ) { cout << "Input Matrix size: " << num. Rows << " rows by " << num. Cols << " columns n" ; for ( int i = 0 ; i < num. Rows ; i ++ ) { cout << "Please enter " << num. Cols << " values separated by spaces for row no. " << i+1 << ": " ; for ( int j = 0 ; j < num. Cols ; j ++ ) { cin >> elements [ i ] [ j ] ; } } return * this ; }
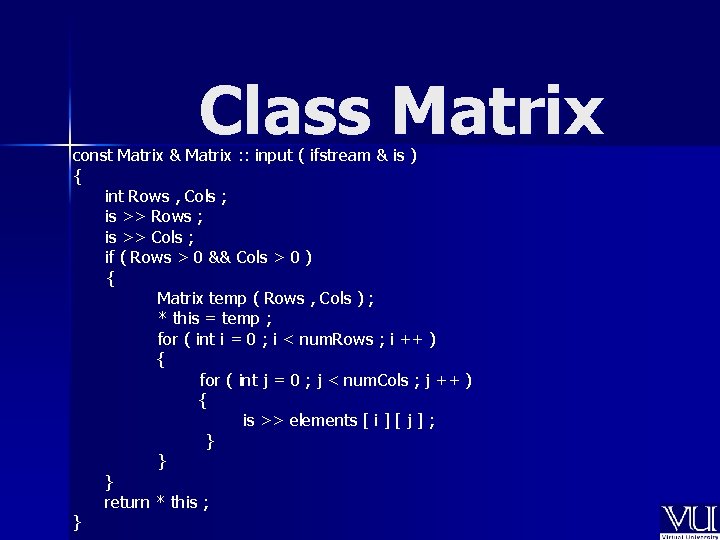
Class Matrix const Matrix & Matrix : : input ( ifstream & is ) { int Rows , Cols ; is >> Rows ; is >> Cols ; if ( Rows > 0 && Cols > 0 ) { Matrix temp ( Rows , Cols ) ; * this = temp ; for ( int i = 0 ; i < num. Rows ; i ++ ) { for ( int j = 0 ; j < num. Cols ; j ++ ) { is >> elements [ i ] [ j ] ; } } } return * this ; }
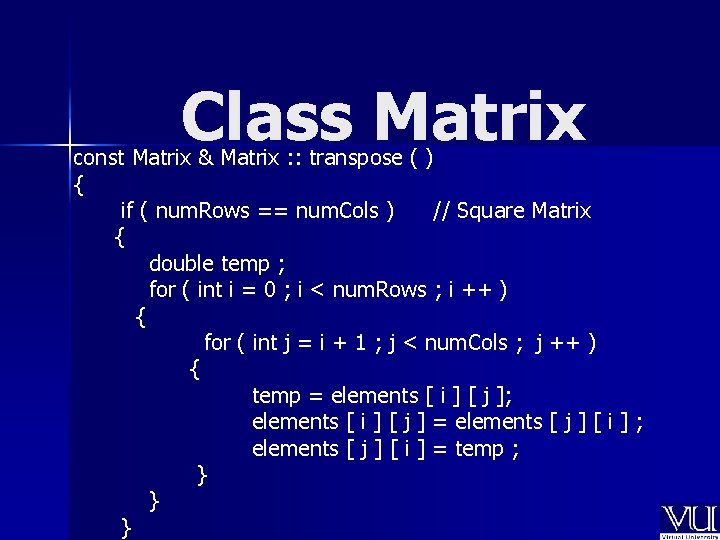
Class Matrix const Matrix & Matrix : : transpose ( ) { if ( num. Rows == num. Cols ) // Square Matrix { double temp ; for ( int i = 0 ; i < num. Rows ; i ++ ) { for ( int j = i + 1 ; j < num. Cols ; j ++ ) { temp = elements [ i ] [ j ]; elements [ i ] [ j ] = elements [ j ] [ i ] ; elements [ j ] [ i ] = temp ; } } }
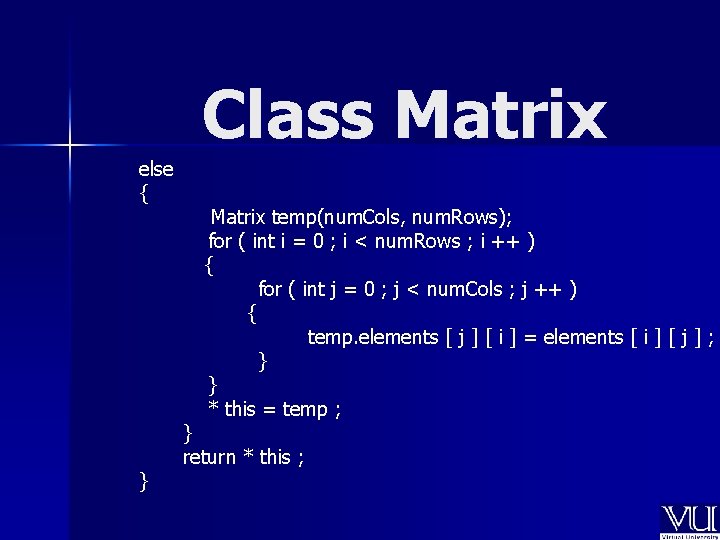
Class Matrix else { } Matrix temp(num. Cols, num. Rows); for ( int i = 0 ; i < num. Rows ; i ++ ) { for ( int j = 0 ; j < num. Cols ; j ++ ) { temp. elements [ j ] [ i ] = elements [ i ] [ j ] ; } } * this = temp ; } return * this ;