Introduction to PHP and Postgre SQL CSC 436
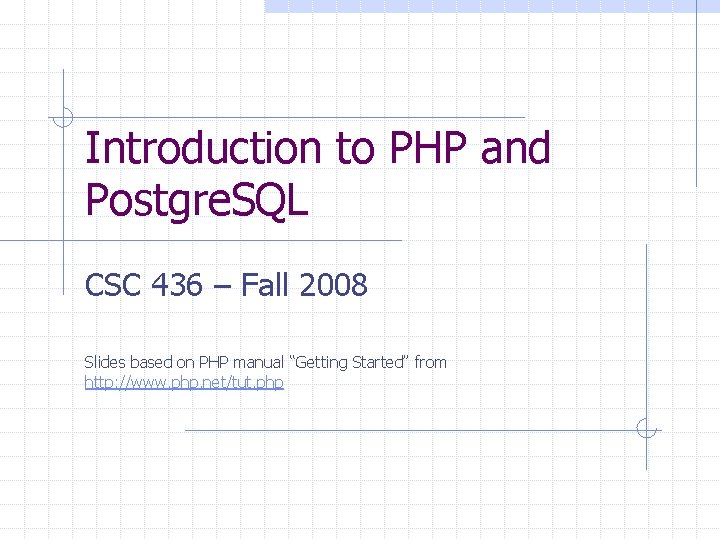
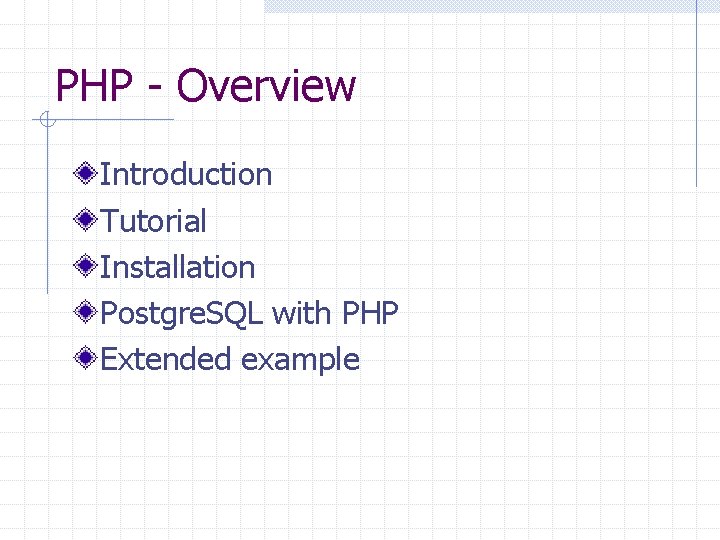
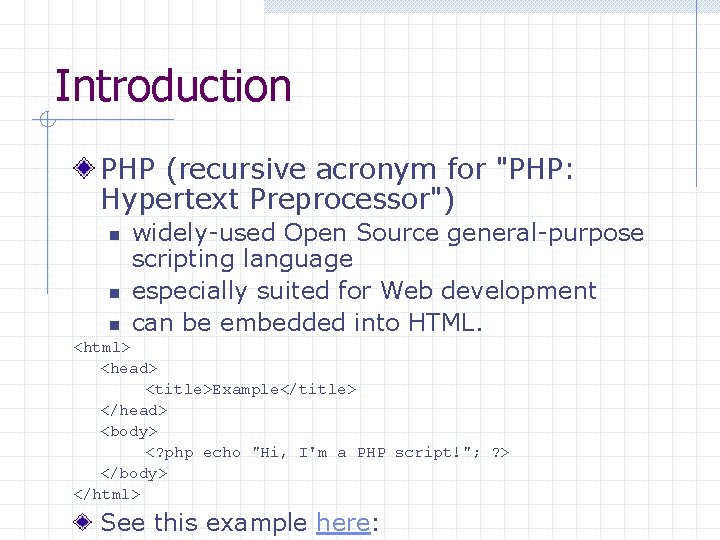
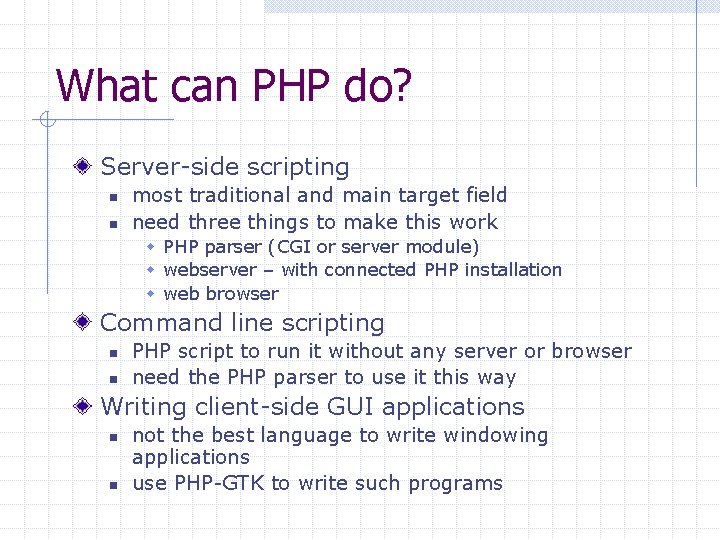
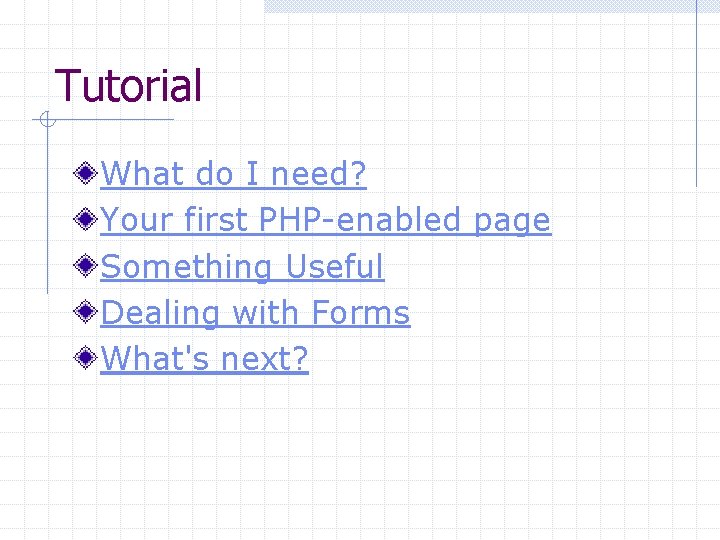
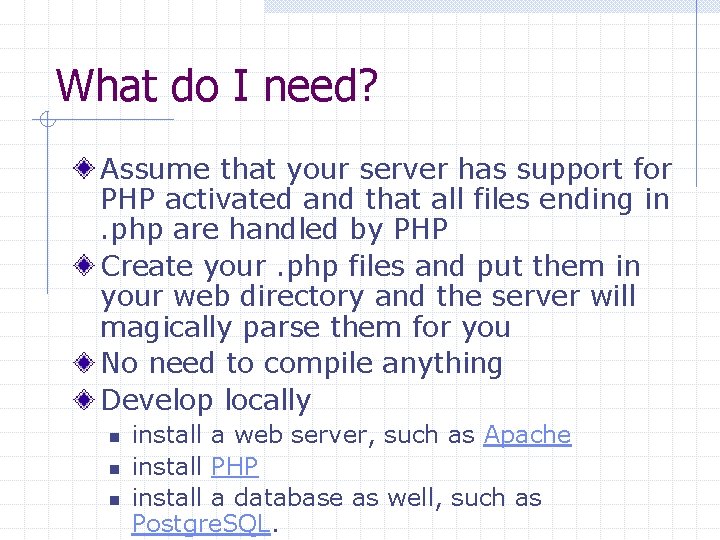
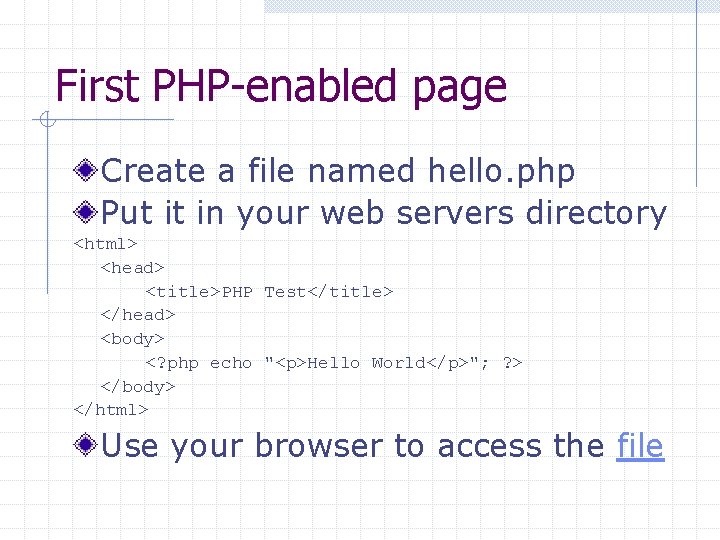
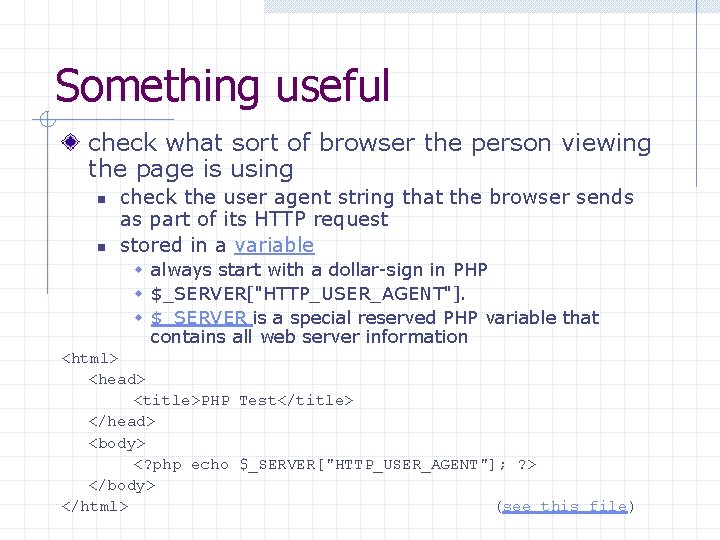
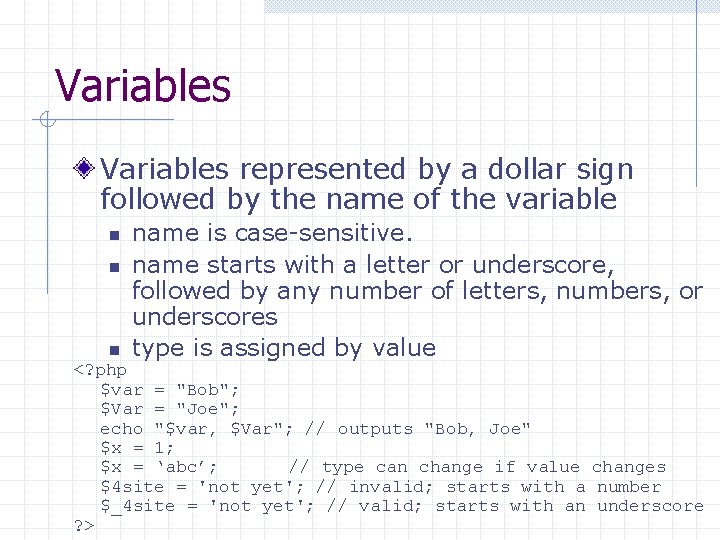
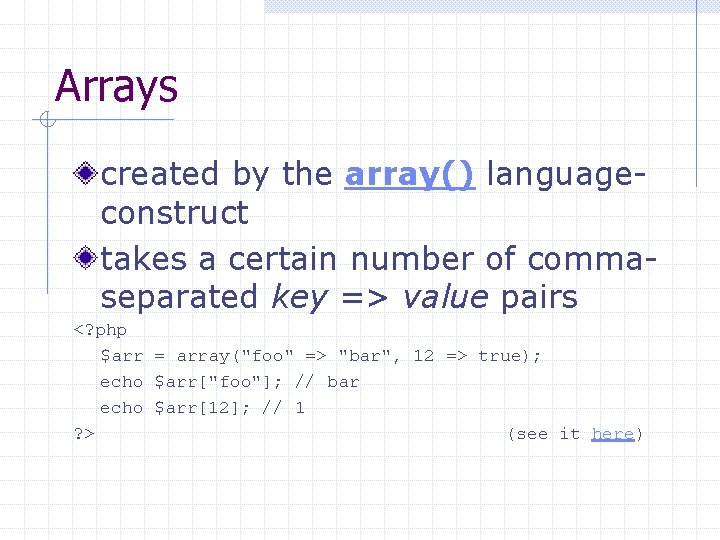
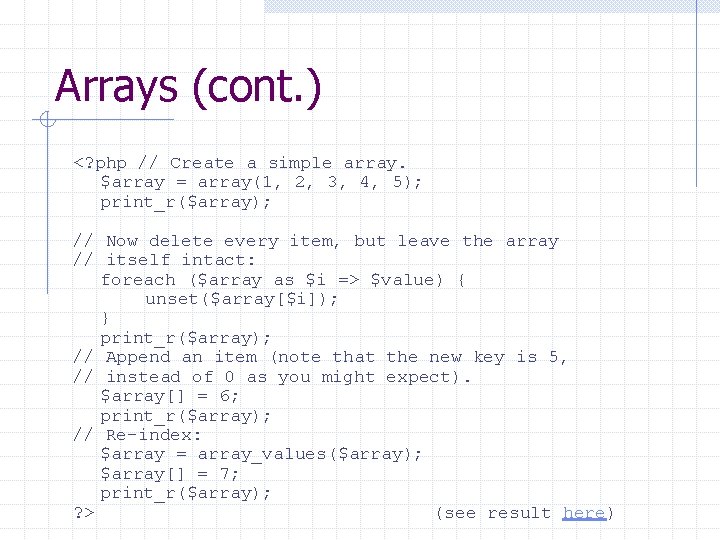
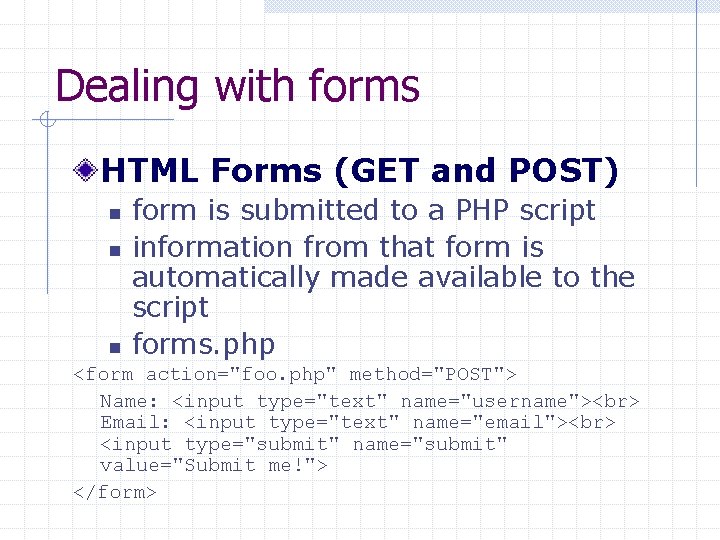
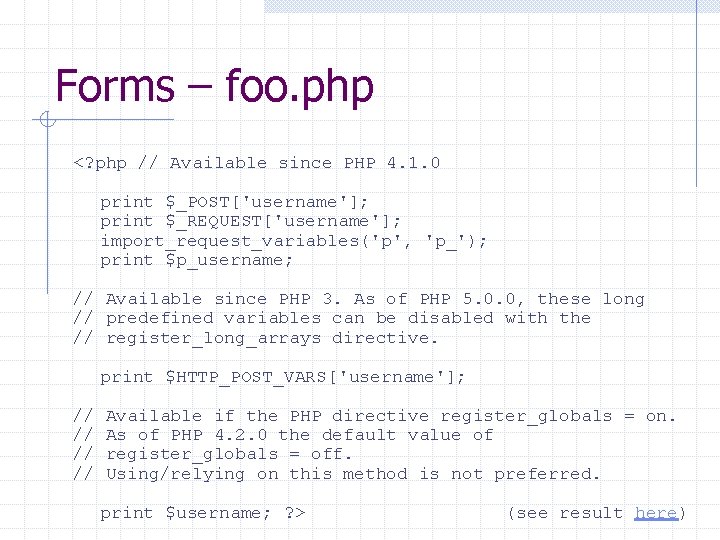
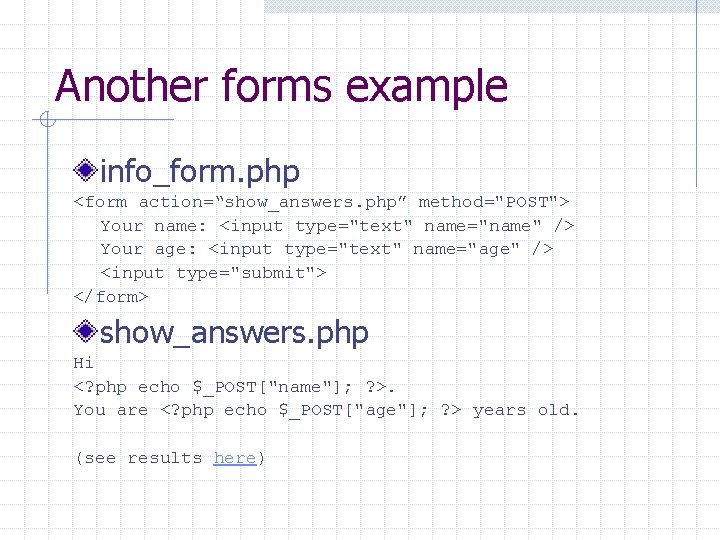
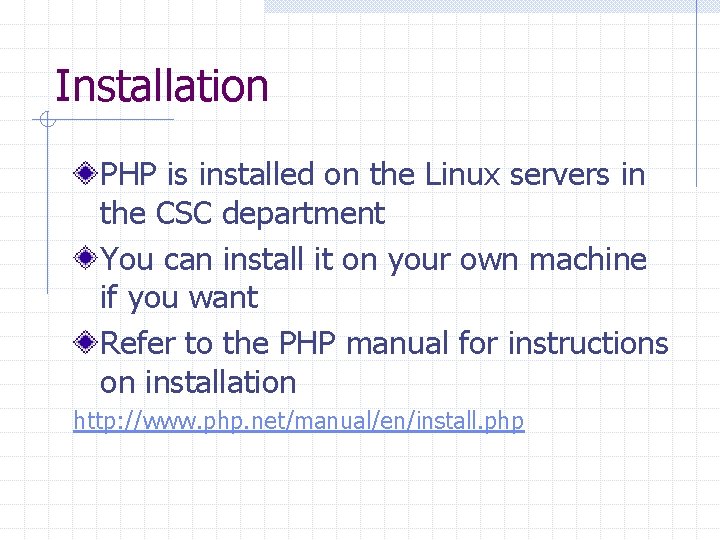
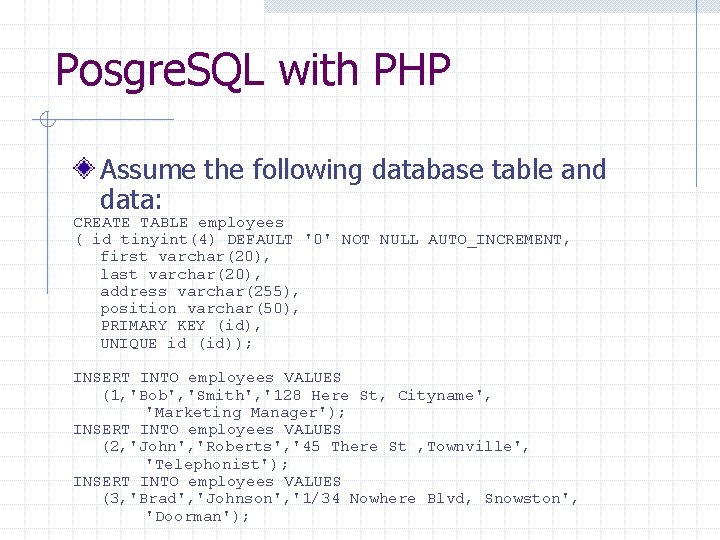
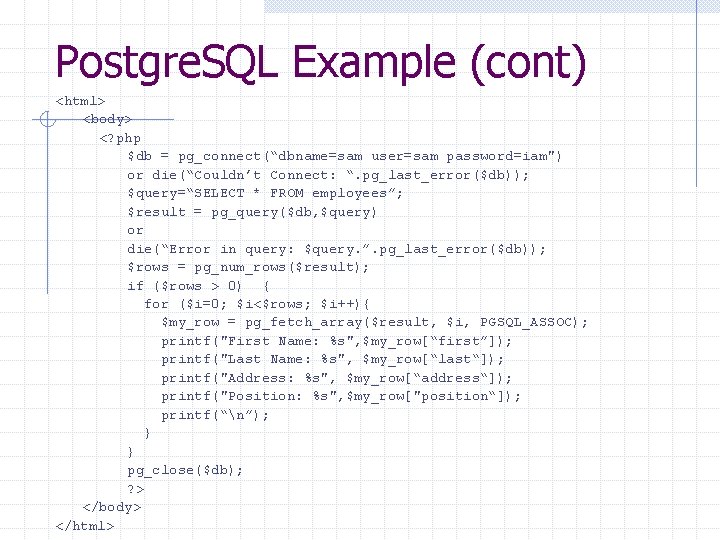
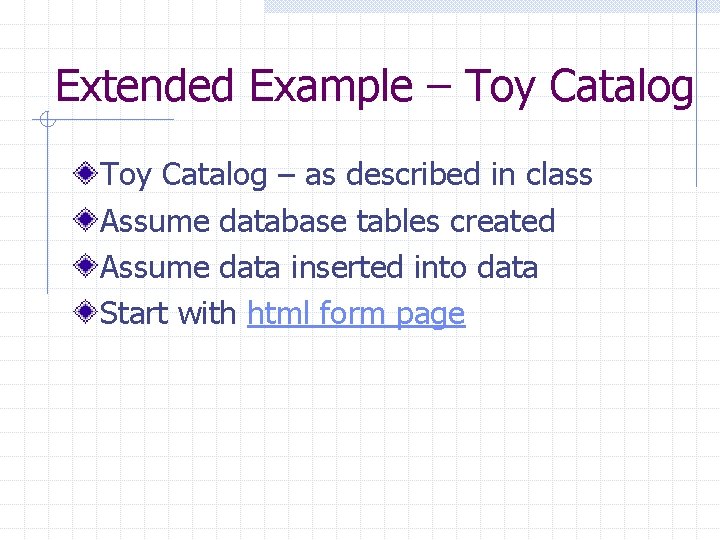
- Slides: 18
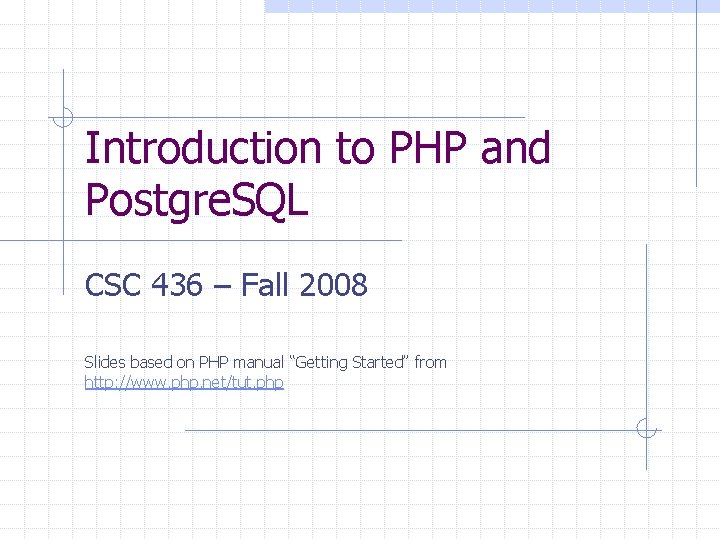
Introduction to PHP and Postgre. SQL CSC 436 – Fall 2008 Slides based on PHP manual “Getting Started” from http: //www. php. net/tut. php
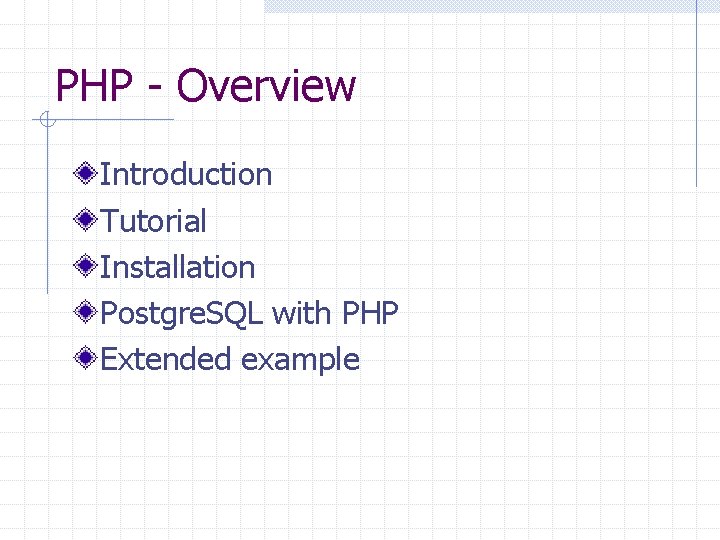
PHP - Overview Introduction Tutorial Installation Postgre. SQL with PHP Extended example
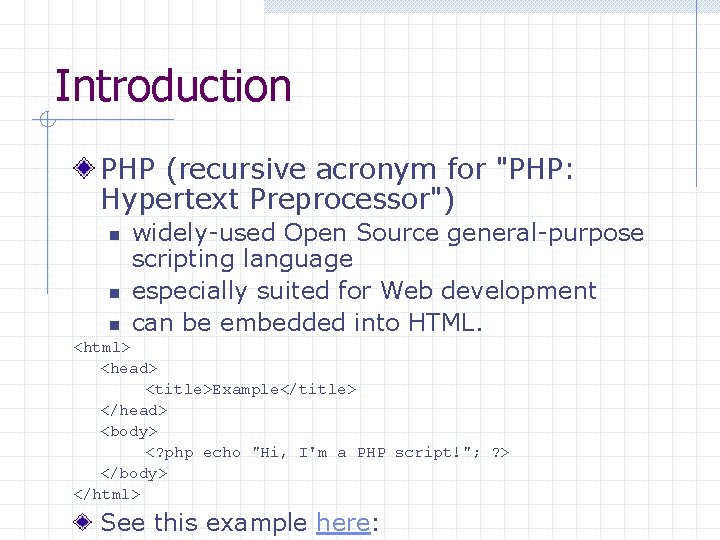
Introduction PHP (recursive acronym for "PHP: Hypertext Preprocessor") n n n widely-used Open Source general-purpose scripting language especially suited for Web development can be embedded into HTML. <html> <head> <title>Example</title> </head> <body> <? php echo "Hi, I'm a PHP script!"; ? > </body> </html> See this example here:
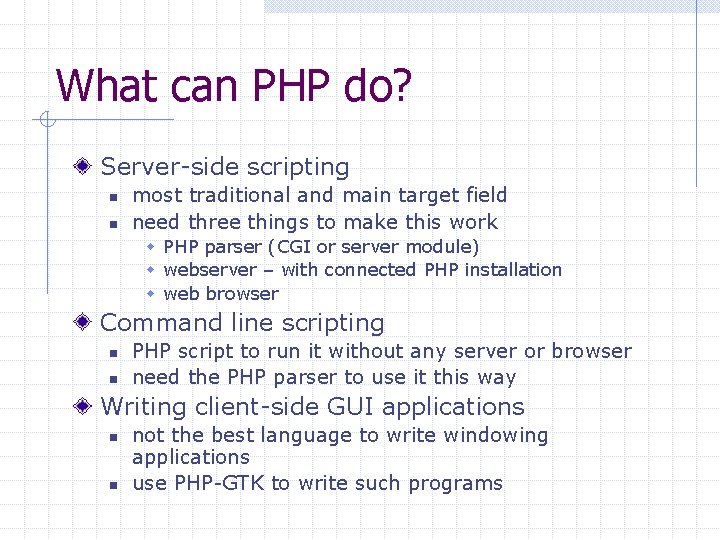
What can PHP do? Server-side scripting n n most traditional and main target field need three things to make this work w PHP parser (CGI or server module) w webserver – with connected PHP installation w web browser Command line scripting n n PHP script to run it without any server or browser need the PHP parser to use it this way Writing client-side GUI applications n n not the best language to write windowing applications use PHP-GTK to write such programs
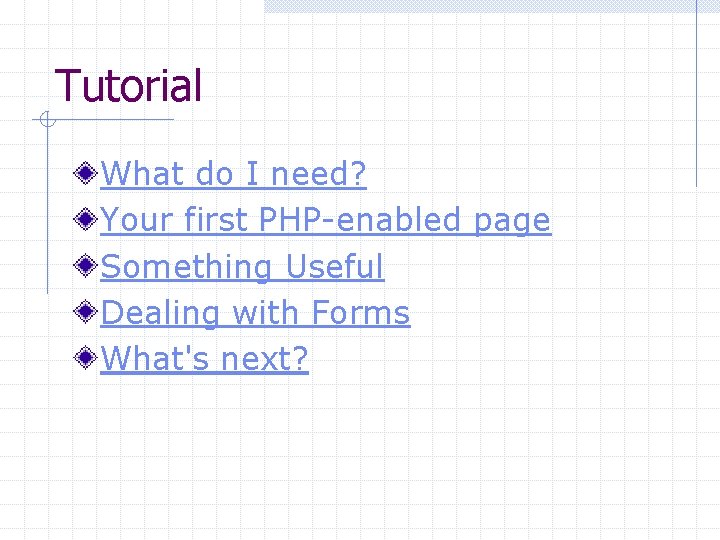
Tutorial What do I need? Your first PHP-enabled page Something Useful Dealing with Forms What's next?
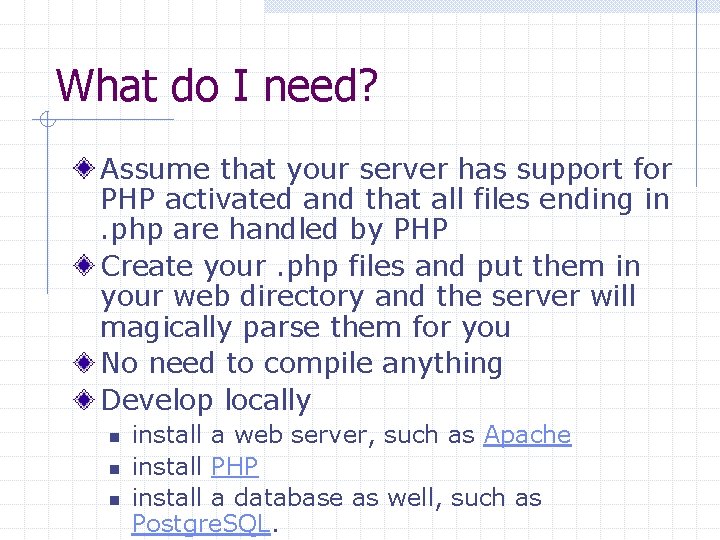
What do I need? Assume that your server has support for PHP activated and that all files ending in. php are handled by PHP Create your. php files and put them in your web directory and the server will magically parse them for you No need to compile anything Develop locally n n n install a web server, such as Apache install PHP install a database as well, such as Postgre. SQL.
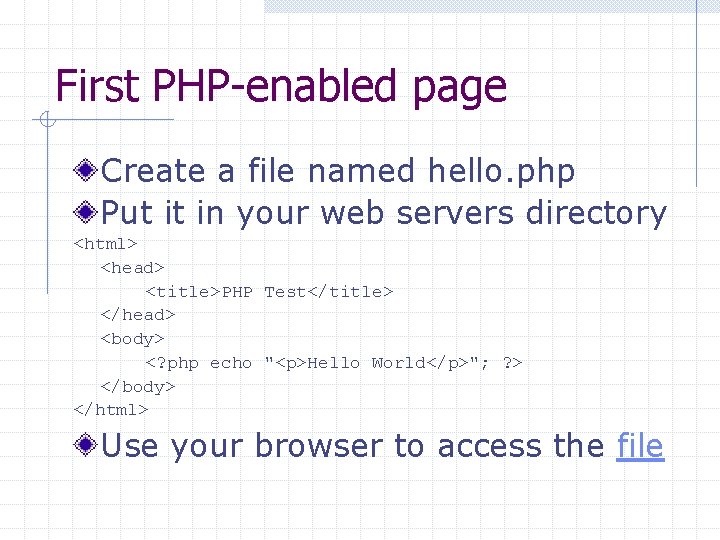
First PHP-enabled page Create a file named hello. php Put it in your web servers directory <html> <head> <title>PHP Test</title> </head> <body> <? php echo "<p>Hello World</p>"; ? > </body> </html> Use your browser to access the file
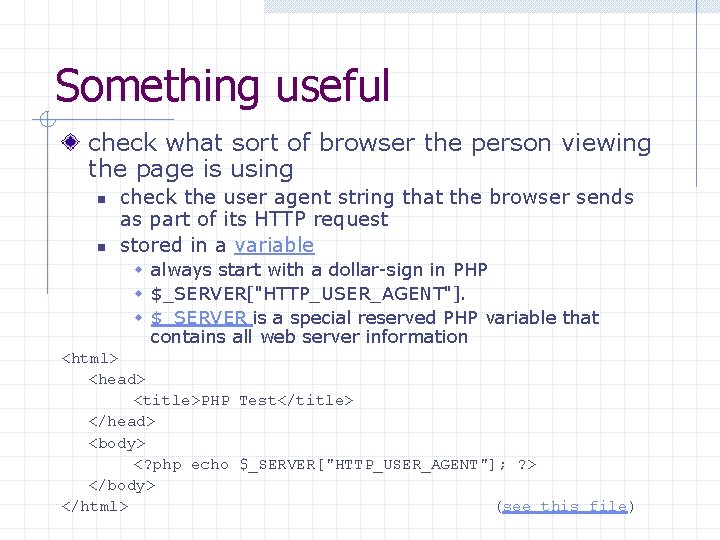
Something useful check what sort of browser the person viewing the page is using n n check the user agent string that the browser sends as part of its HTTP request stored in a variable w always start with a dollar-sign in PHP w $_SERVER["HTTP_USER_AGENT"]. w $_SERVER is a special reserved PHP variable that contains all web server information <html> <head> <title>PHP Test</title> </head> <body> <? php echo $_SERVER["HTTP_USER_AGENT"]; ? > </body> </html> (see this file)
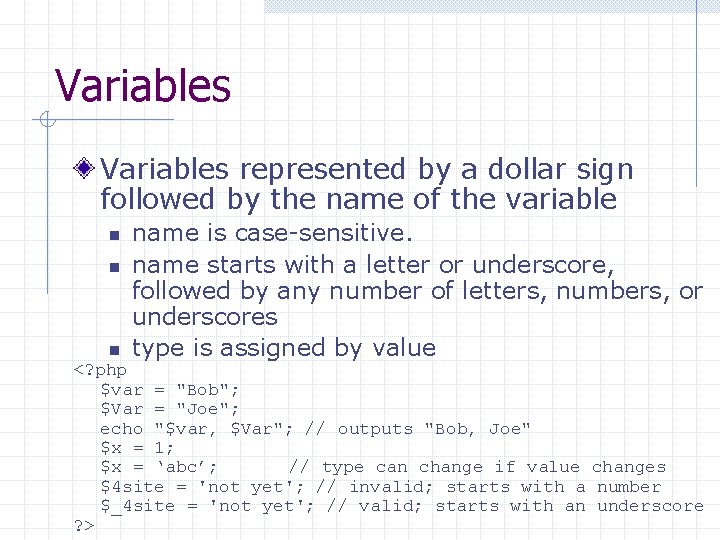
Variables represented by a dollar sign followed by the name of the variable n name is case-sensitive. name starts with a letter or underscore, followed by any number of letters, numbers, or underscores type is assigned by value <? php $var = "Bob"; $Var = "Joe"; echo "$var, $Var"; // outputs "Bob, Joe" $x = 1; $x = ‘abc’; // type can change if value changes $4 site = 'not yet'; // invalid; starts with a number $_4 site = 'not yet'; // valid; starts with an underscore ? >
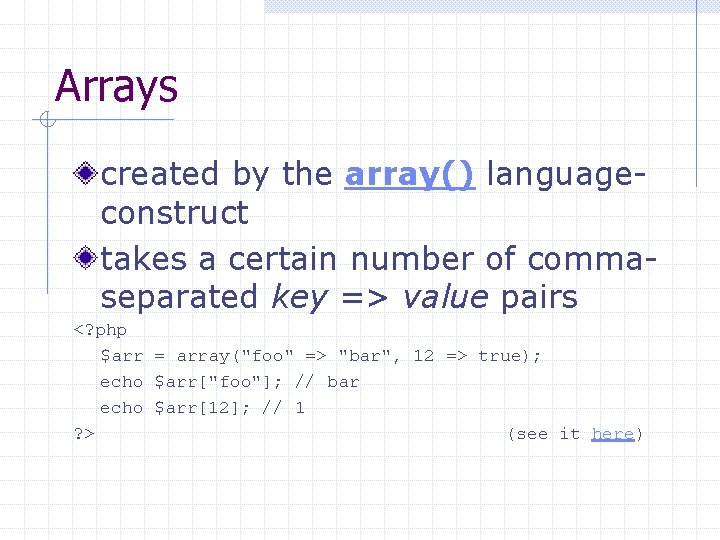
Arrays created by the array() languageconstruct takes a certain number of commaseparated key => value pairs <? php $arr = array("foo" => "bar", 12 => true); echo $arr["foo"]; // bar echo $arr[12]; // 1 ? > (see it here)
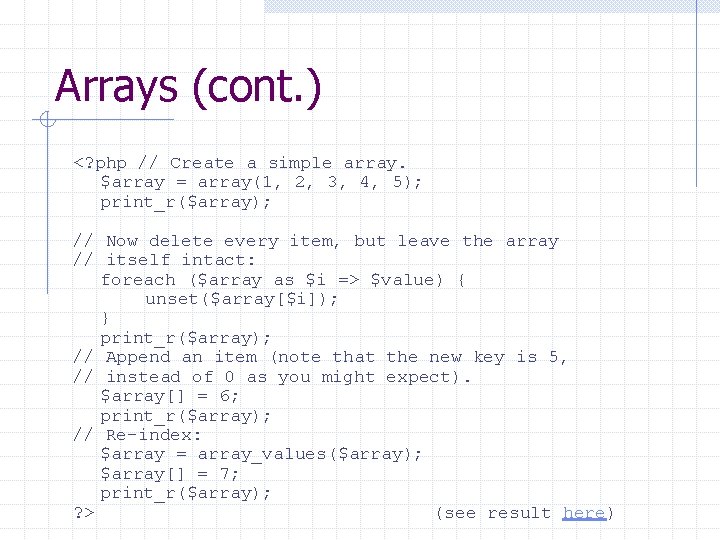
Arrays (cont. ) <? php // Create a simple array. $array = array(1, 2, 3, 4, 5); print_r($array); // Now delete every item, but leave the array // itself intact: foreach ($array as $i => $value) { unset($array[$i]); } print_r($array); // Append an item (note that the new key is 5, // instead of 0 as you might expect). $array[] = 6; print_r($array); // Re-index: $array = array_values($array); $array[] = 7; print_r($array); ? > (see result here)
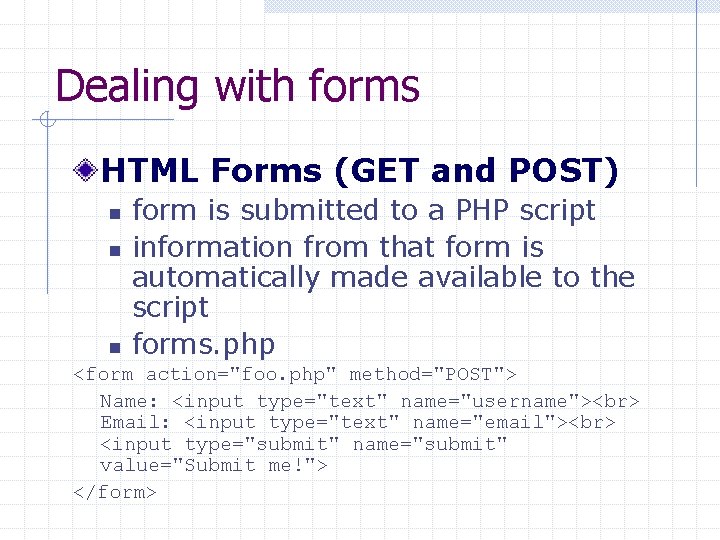
Dealing with forms HTML Forms (GET and POST) n n n form is submitted to a PHP script information from that form is automatically made available to the script forms. php <form action="foo. php" method="POST"> Name: <input type="text" name="username"> Email: <input type="text" name="email"> <input type="submit" name="submit" value="Submit me!"> </form>
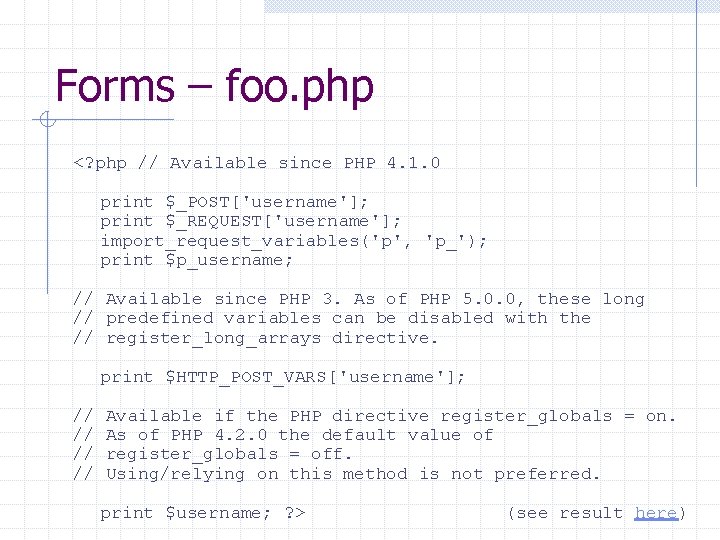
Forms – foo. php <? php // Available since PHP 4. 1. 0 print $_POST['username']; print $_REQUEST['username']; import_request_variables('p', 'p_'); print $p_username; // Available since PHP 3. As of PHP 5. 0. 0, these long // predefined variables can be disabled with the // register_long_arrays directive. print $HTTP_POST_VARS['username']; // // Available if the PHP directive register_globals = on. As of PHP 4. 2. 0 the default value of register_globals = off. Using/relying on this method is not preferred. print $username; ? > (see result here)
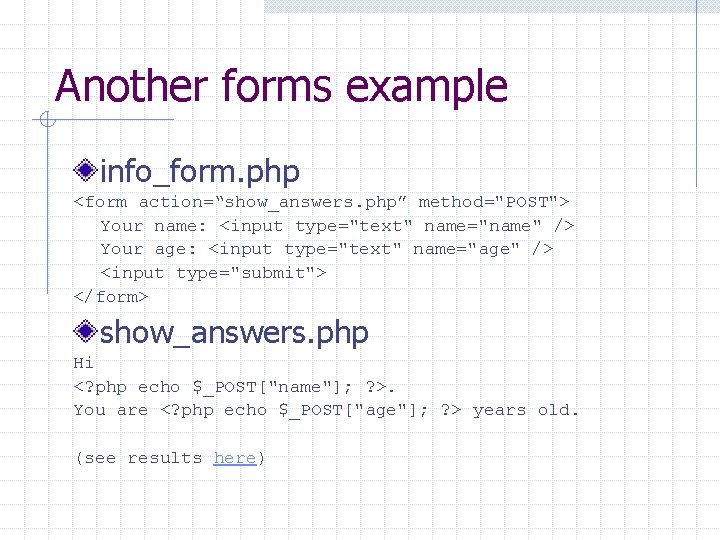
Another forms example info_form. php <form action=“show_answers. php” method="POST"> Your name: <input type="text" name="name" /> Your age: <input type="text" name="age" /> <input type="submit"> </form> show_answers. php Hi <? php echo $_POST["name"]; ? >. You are <? php echo $_POST["age"]; ? > years old. (see results here)
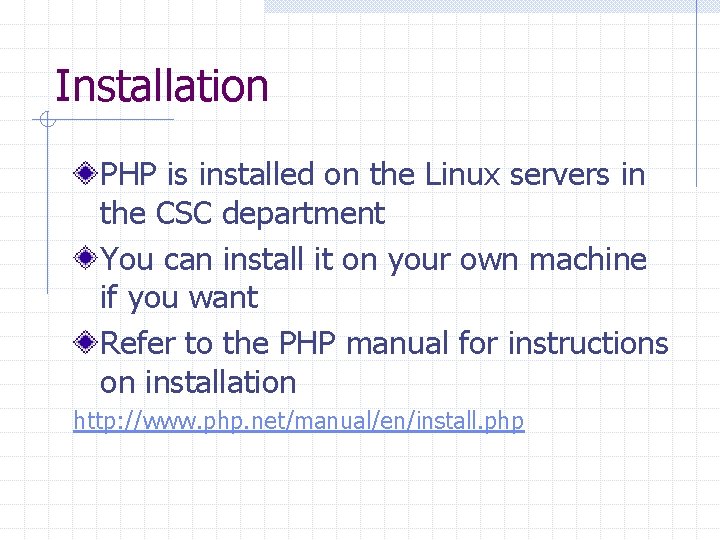
Installation PHP is installed on the Linux servers in the CSC department You can install it on your own machine if you want Refer to the PHP manual for instructions on installation http: //www. php. net/manual/en/install. php
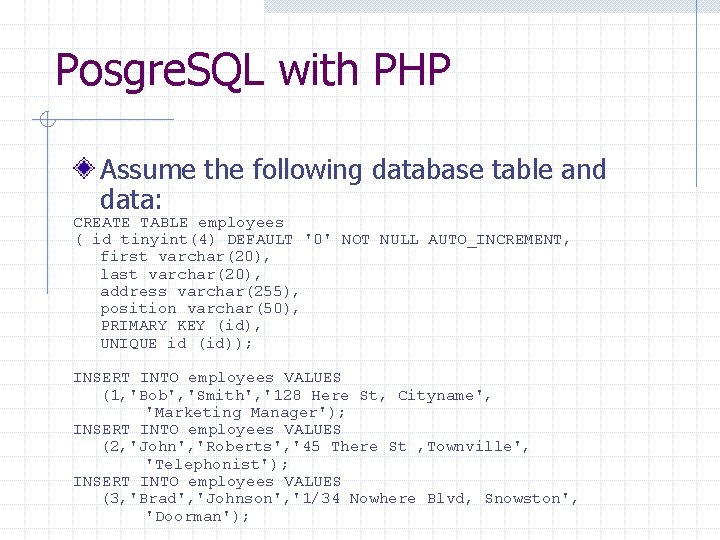
Posgre. SQL with PHP Assume the following database table and data: CREATE TABLE employees ( id tinyint(4) DEFAULT '0' NOT NULL AUTO_INCREMENT, first varchar(20), last varchar(20), address varchar(255), position varchar(50), PRIMARY KEY (id), UNIQUE id (id)); INSERT INTO employees VALUES (1, 'Bob', 'Smith', '128 Here St, Cityname', 'Marketing Manager'); INSERT INTO employees VALUES (2, 'John', 'Roberts', '45 There St , Townville', 'Telephonist'); INSERT INTO employees VALUES (3, 'Brad', 'Johnson', '1/34 Nowhere Blvd, Snowston', 'Doorman');
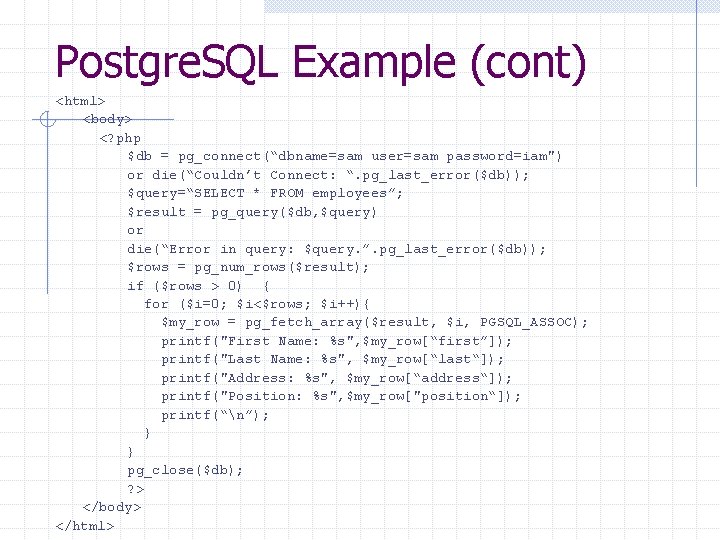
Postgre. SQL Example (cont) <html> <body> <? php $db = pg_connect(“dbname=sam user=sam password=iam") or die(“Couldn’t Connect: “. pg_last_error($db)); $query=“SELECT * FROM employees”; $result = pg_query($db, $query) or die(“Error in query: $query. ”. pg_last_error($db)); $rows = pg_num_rows($result); if ($rows > 0) { for ($i=0; $i<$rows; $i++){ $my_row = pg_fetch_array($result, $i, PGSQL_ASSOC); printf("First Name: %s", $my_row[“first”]); printf("Last Name: %s", $my_row[“last“]); printf("Address: %s", $my_row[“address“]); printf("Position: %s", $my_row["position“]); printf(“n”); } } pg_close($db); ? > </body> </html>
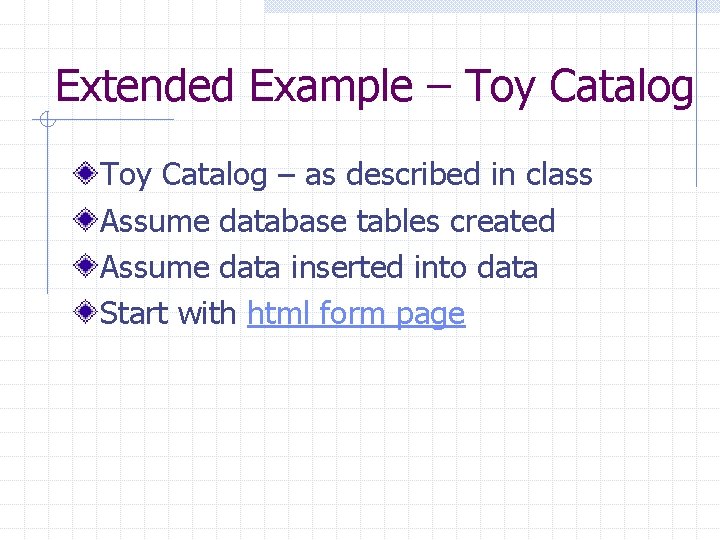
Extended Example – Toy Catalog – as described in class Assume database tables created Assume data inserted into data Start with html form page