Introduction to Java Java program example Introduction to
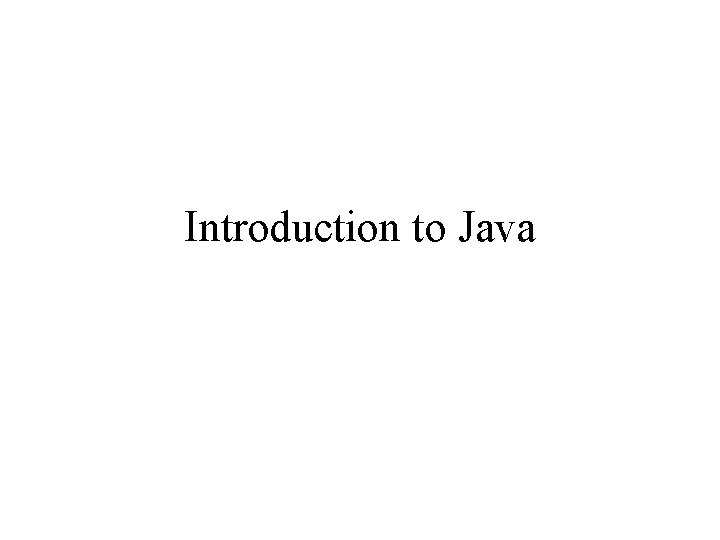
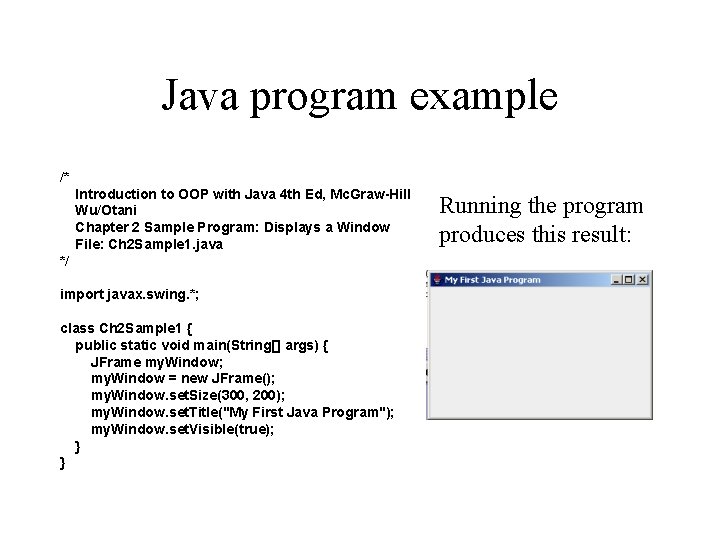
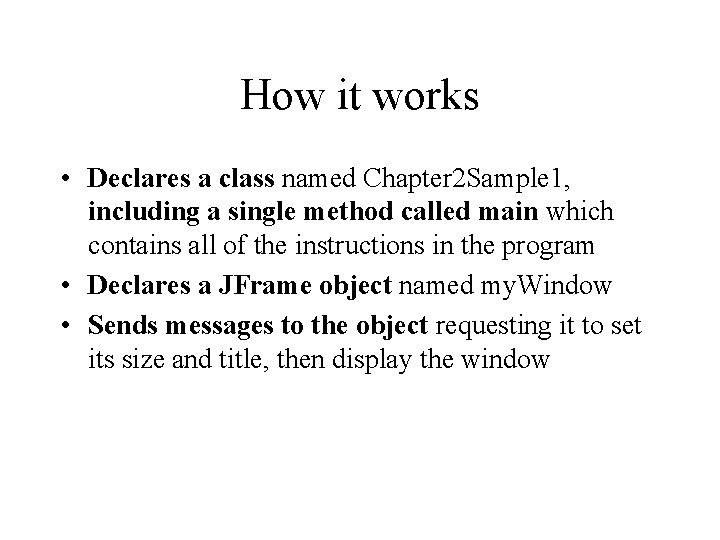
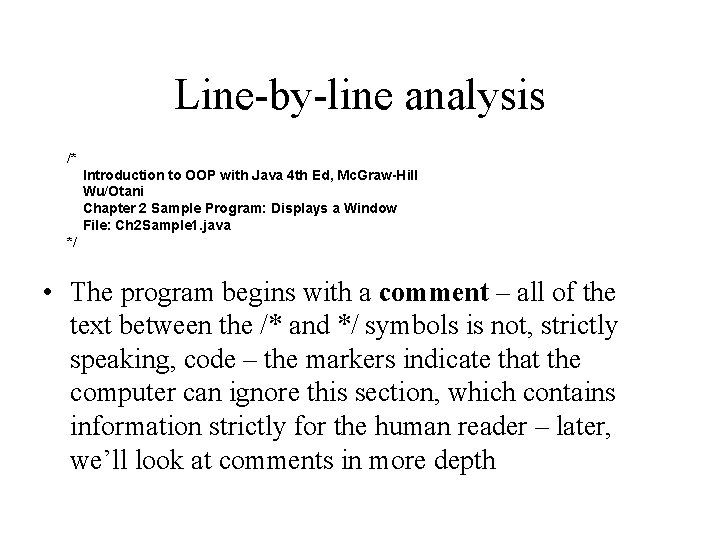
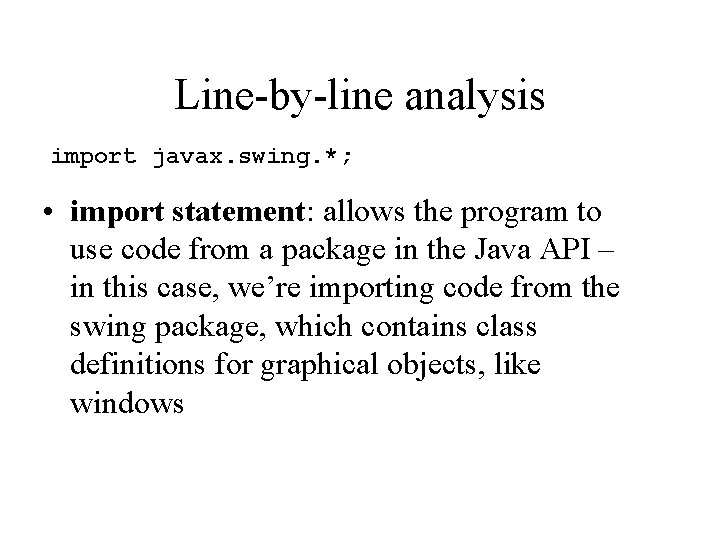
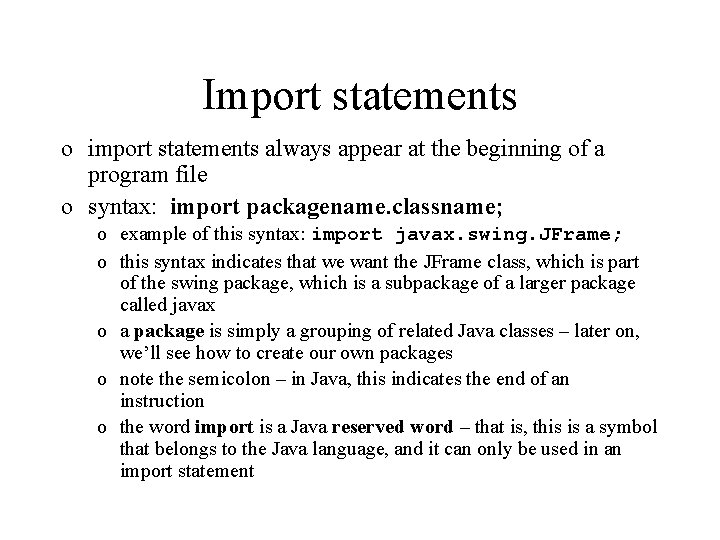
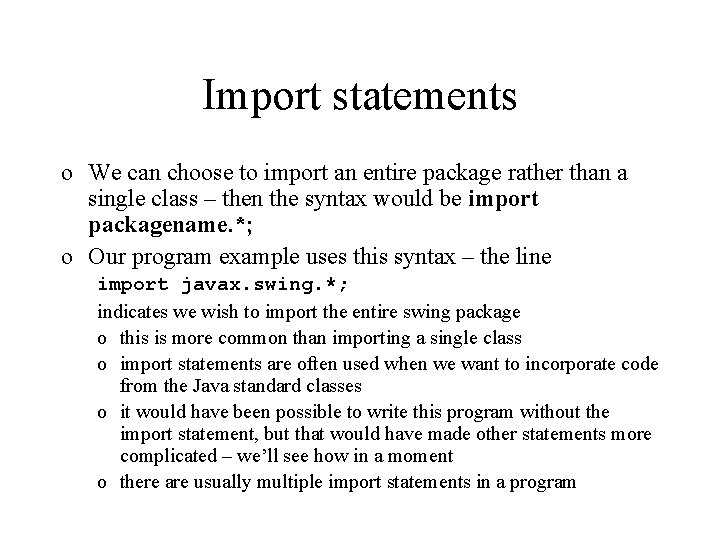
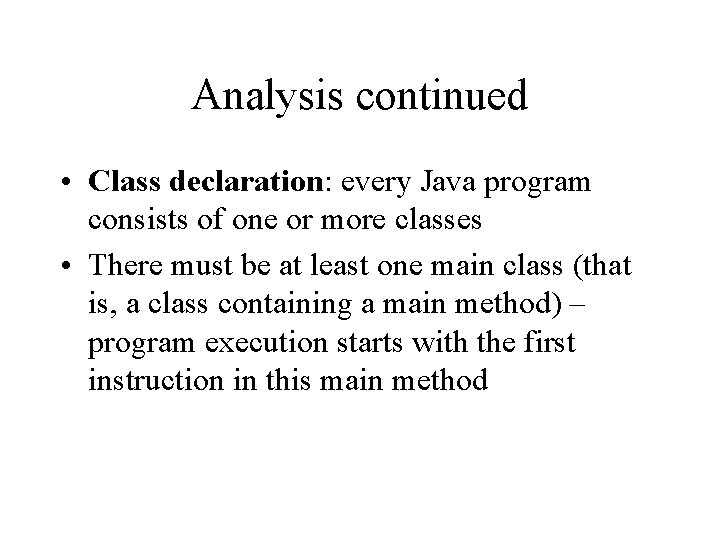
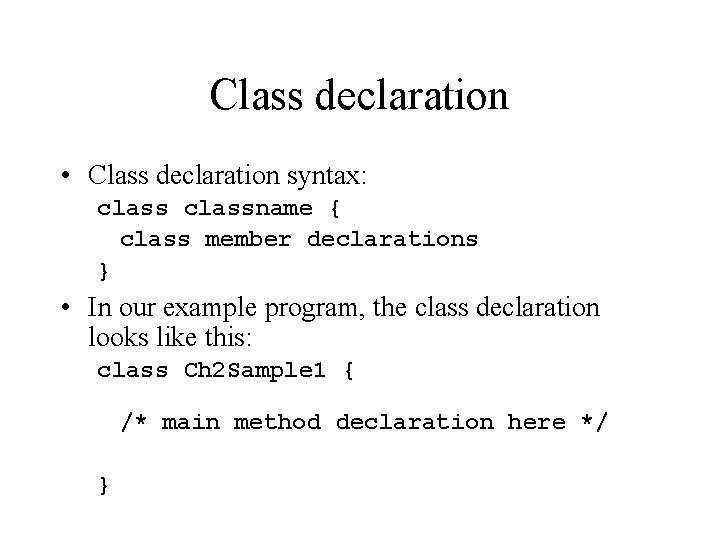
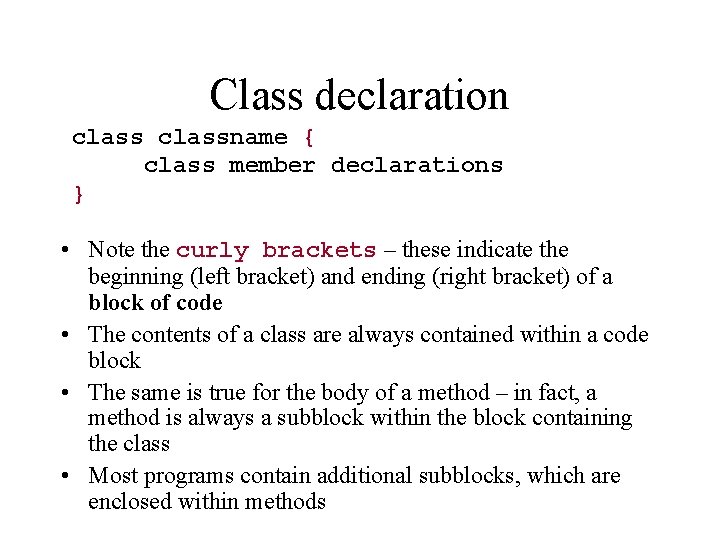
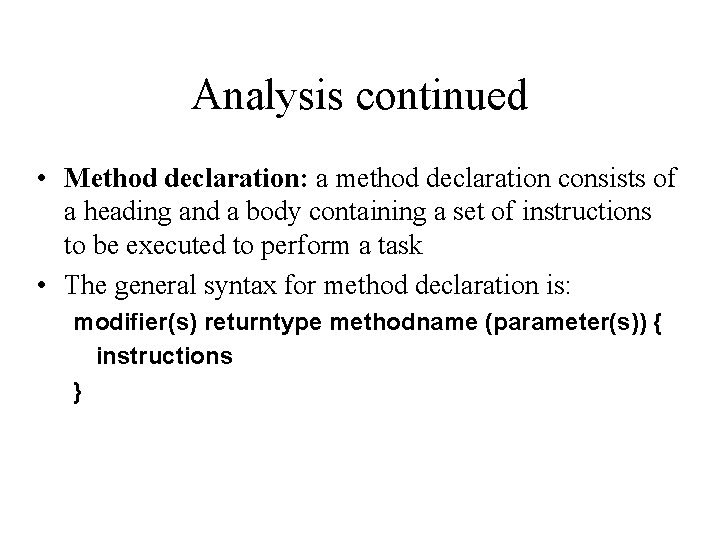
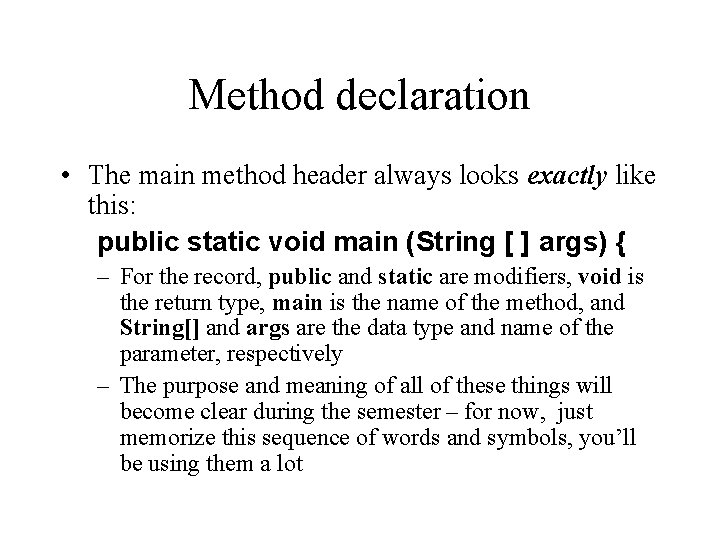
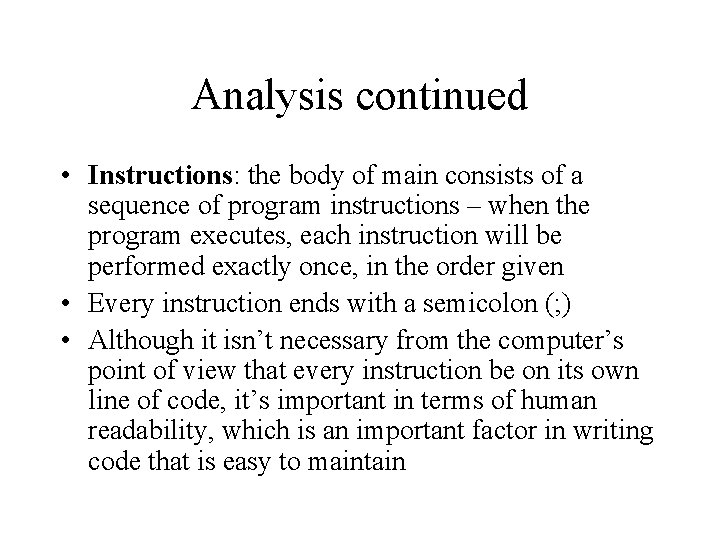
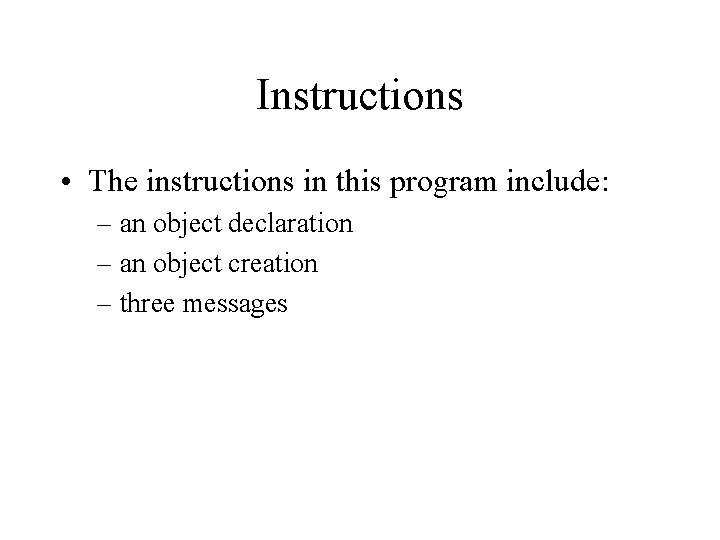
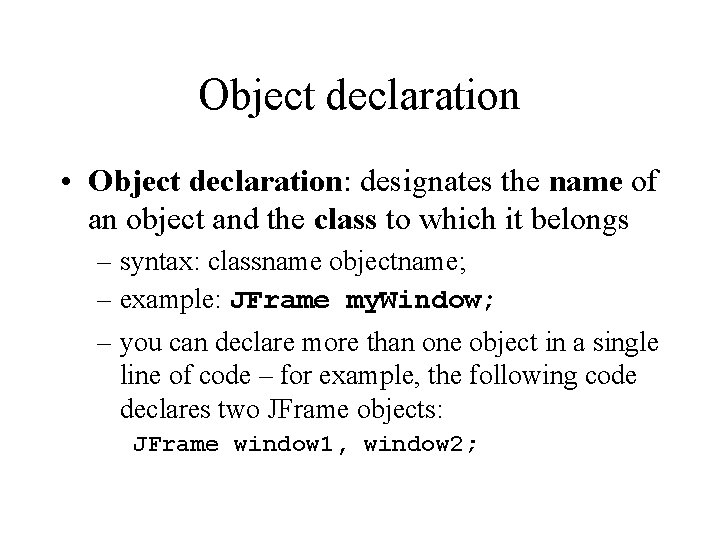
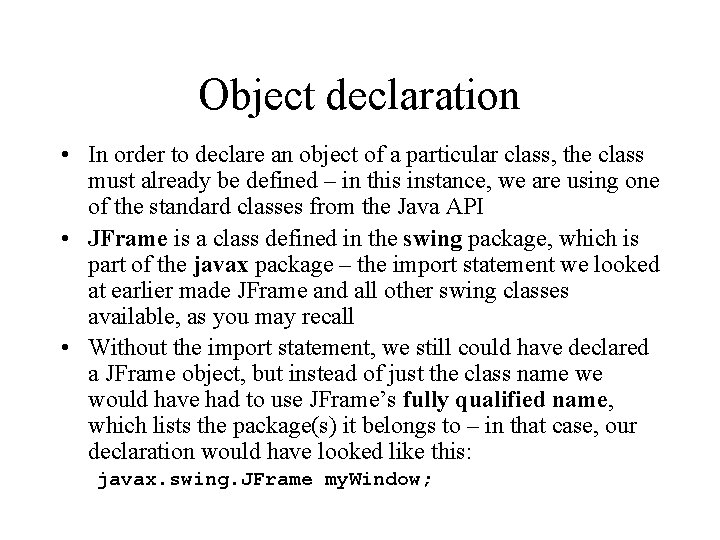
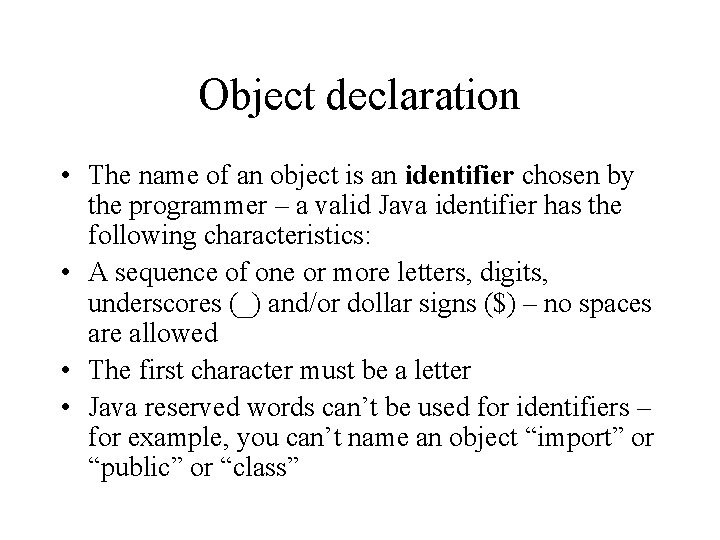
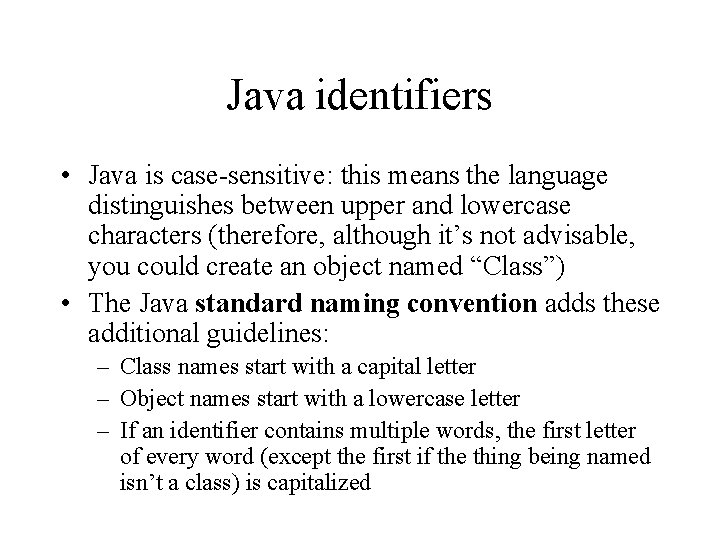
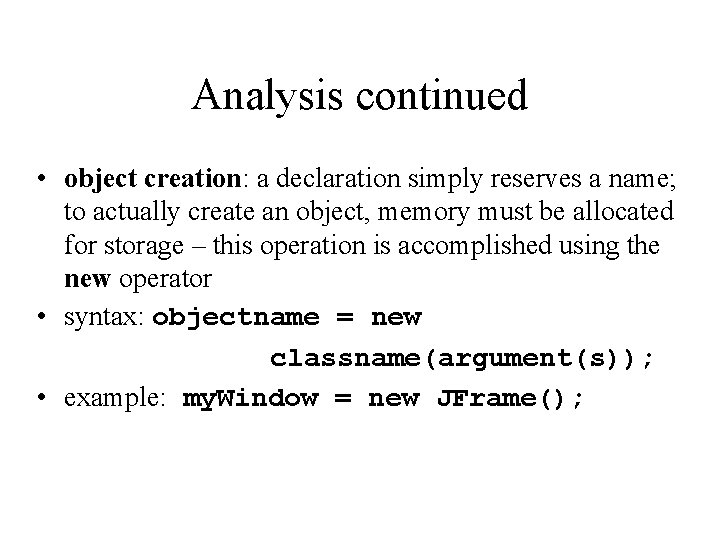
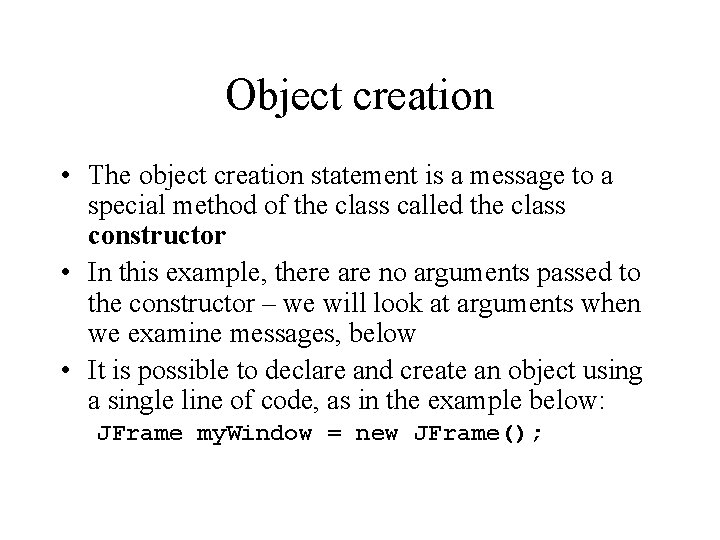
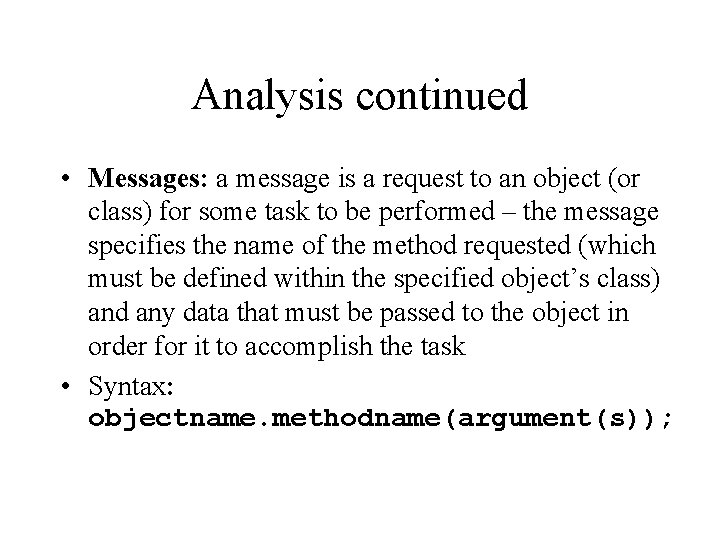
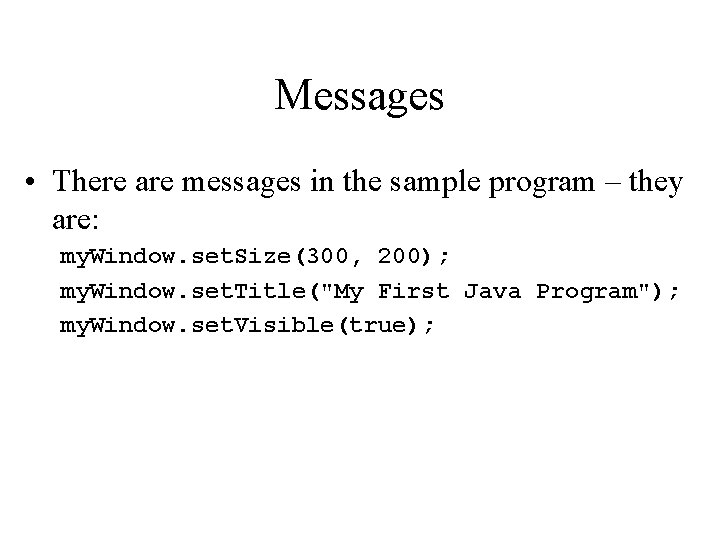
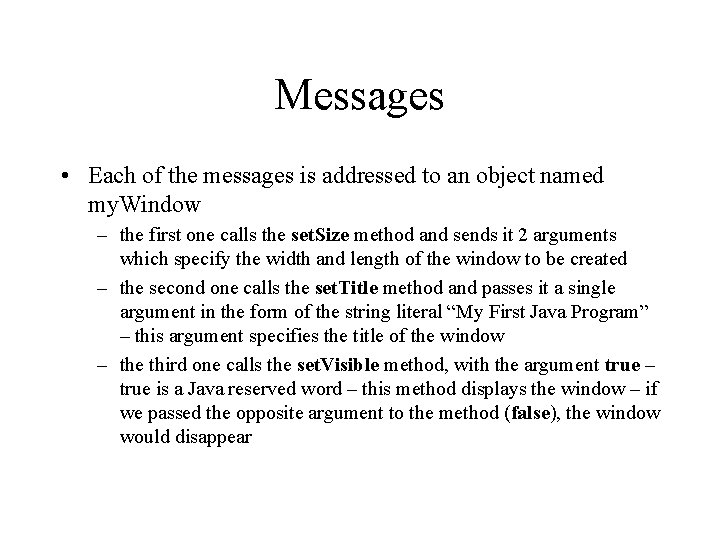
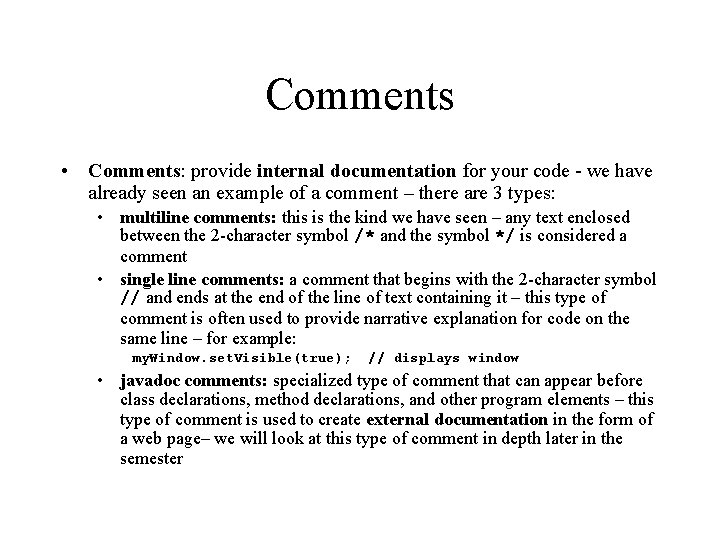
- Slides: 24
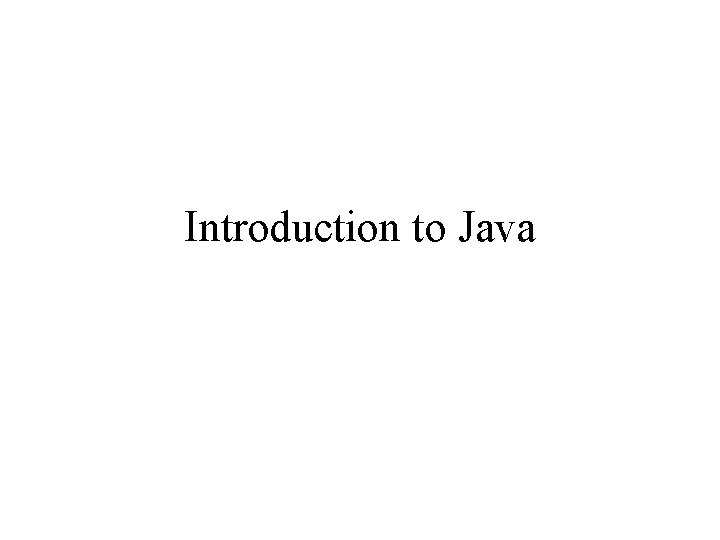
Introduction to Java
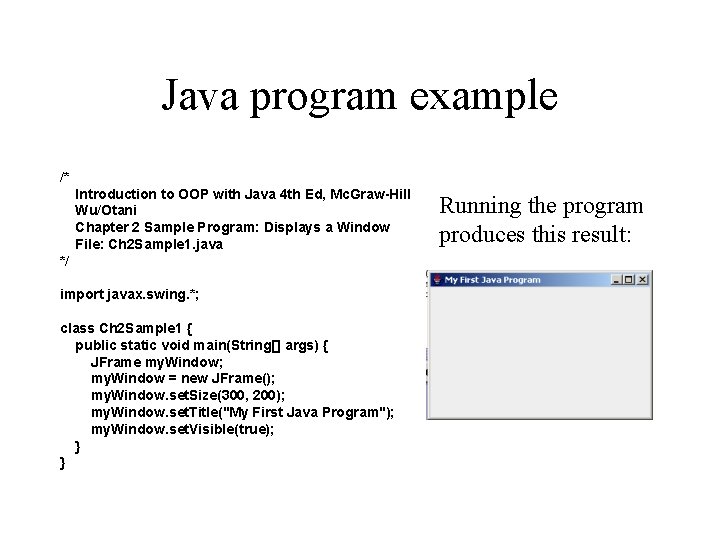
Java program example /* Introduction to OOP with Java 4 th Ed, Mc. Graw-Hill Wu/Otani Chapter 2 Sample Program: Displays a Window File: Ch 2 Sample 1. java */ import javax. swing. *; class Ch 2 Sample 1 { public static void main(String[] args) { JFrame my. Window; my. Window = new JFrame(); my. Window. set. Size(300, 200); my. Window. set. Title("My First Java Program"); my. Window. set. Visible(true); } } Running the program produces this result:
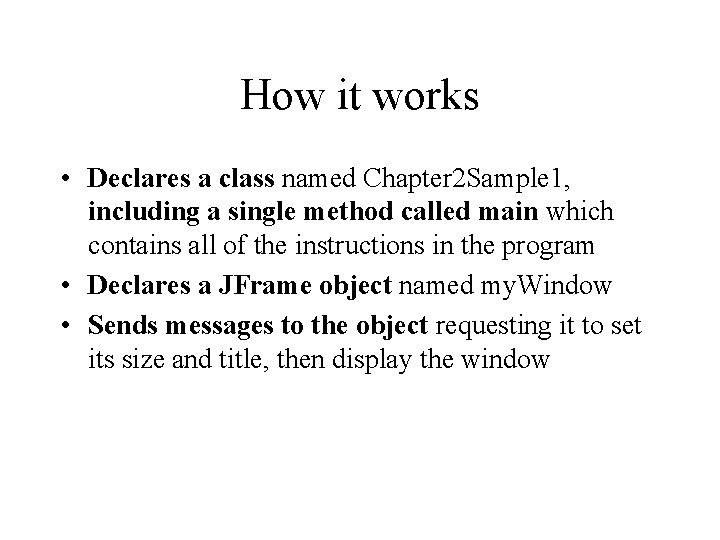
How it works • Declares a class named Chapter 2 Sample 1, including a single method called main which contains all of the instructions in the program • Declares a JFrame object named my. Window • Sends messages to the object requesting it to set its size and title, then display the window
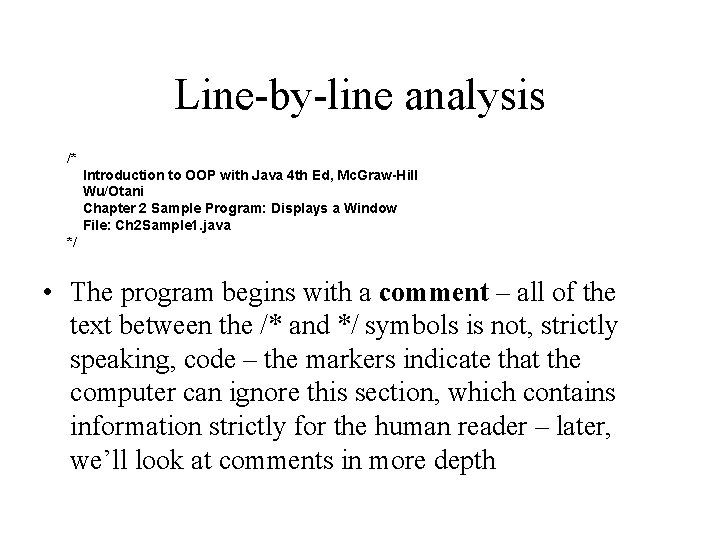
Line-by-line analysis /* Introduction to OOP with Java 4 th Ed, Mc. Graw-Hill Wu/Otani Chapter 2 Sample Program: Displays a Window File: Ch 2 Sample 1. java */ • The program begins with a comment – all of the text between the /* and */ symbols is not, strictly speaking, code – the markers indicate that the computer can ignore this section, which contains information strictly for the human reader – later, we’ll look at comments in more depth
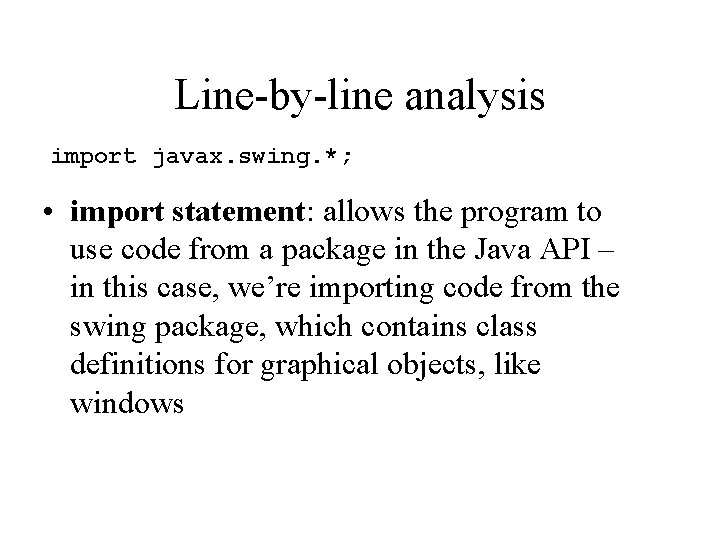
Line-by-line analysis import javax. swing. *; • import statement: allows the program to use code from a package in the Java API – in this case, we’re importing code from the swing package, which contains class definitions for graphical objects, like windows
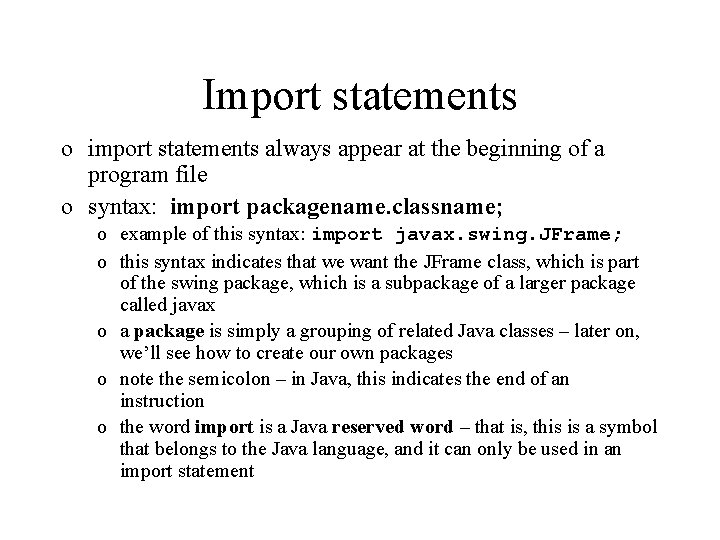
Import statements o import statements always appear at the beginning of a program file o syntax: import packagename. classname; o example of this syntax: import javax. swing. JFrame; o this syntax indicates that we want the JFrame class, which is part of the swing package, which is a subpackage of a larger package called javax o a package is simply a grouping of related Java classes – later on, we’ll see how to create our own packages o note the semicolon – in Java, this indicates the end of an instruction o the word import is a Java reserved word – that is, this is a symbol that belongs to the Java language, and it can only be used in an import statement
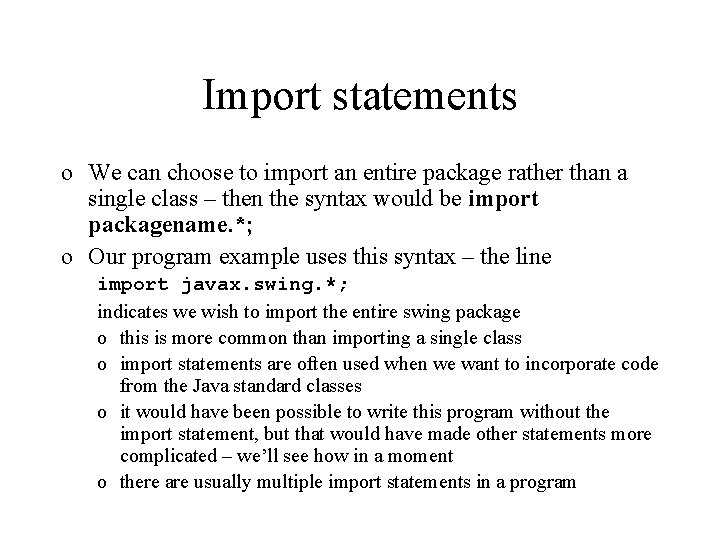
Import statements o We can choose to import an entire package rather than a single class – then the syntax would be import packagename. *; o Our program example uses this syntax – the line import javax. swing. *; indicates we wish to import the entire swing package o this is more common than importing a single class o import statements are often used when we want to incorporate code from the Java standard classes o it would have been possible to write this program without the import statement, but that would have made other statements more complicated – we’ll see how in a moment o there are usually multiple import statements in a program
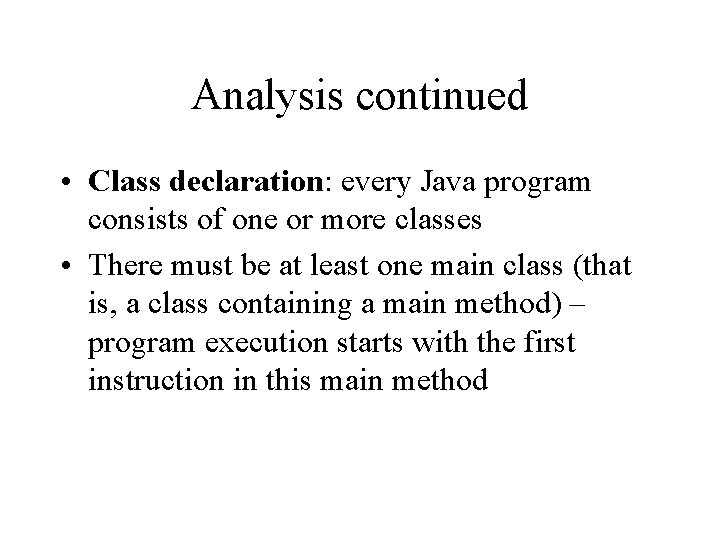
Analysis continued • Class declaration: every Java program consists of one or more classes • There must be at least one main class (that is, a class containing a main method) – program execution starts with the first instruction in this main method
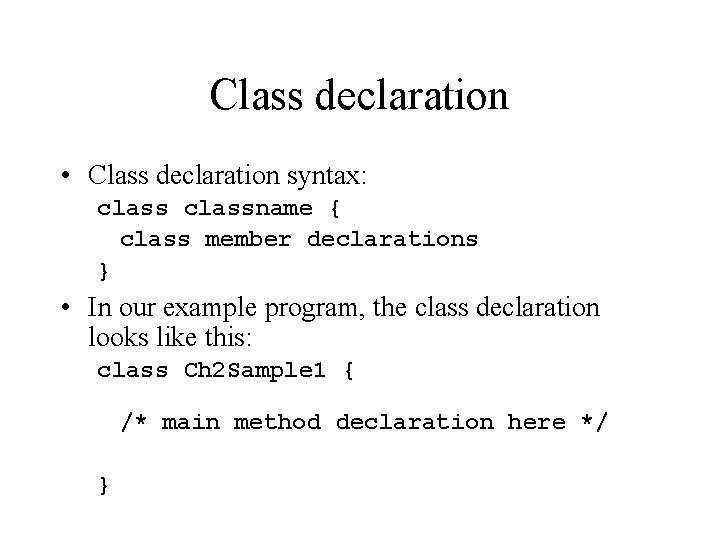
Class declaration • Class declaration syntax: classname { class member declarations } • In our example program, the class declaration looks like this: class Ch 2 Sample 1 { /* main method declaration here */ }
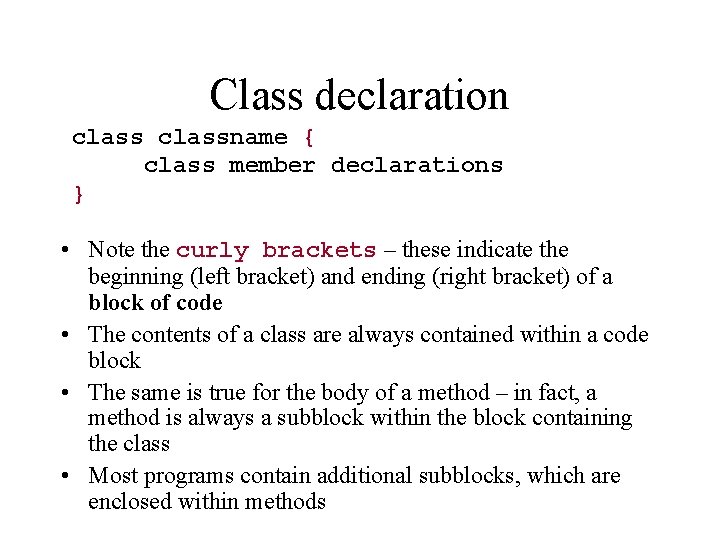
Class declaration classname { class member declarations } • Note the curly brackets – these indicate the beginning (left bracket) and ending (right bracket) of a block of code • The contents of a class are always contained within a code block • The same is true for the body of a method – in fact, a method is always a subblock within the block containing the class • Most programs contain additional subblocks, which are enclosed within methods
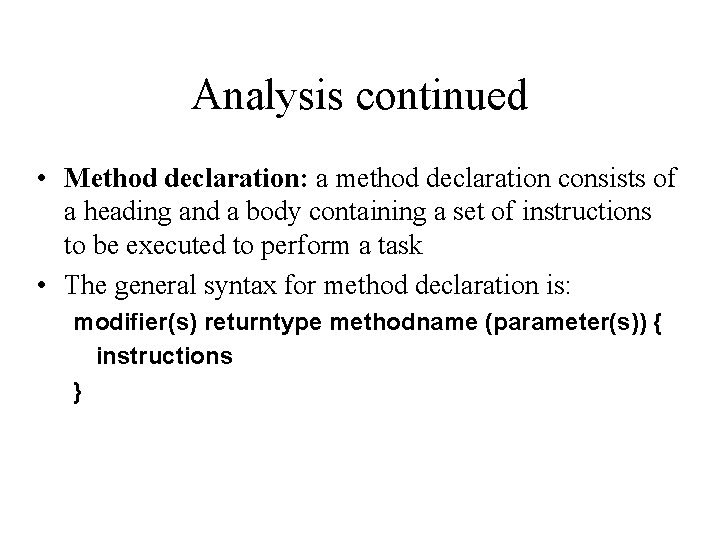
Analysis continued • Method declaration: a method declaration consists of a heading and a body containing a set of instructions to be executed to perform a task • The general syntax for method declaration is: modifier(s) returntype methodname (parameter(s)) { instructions }
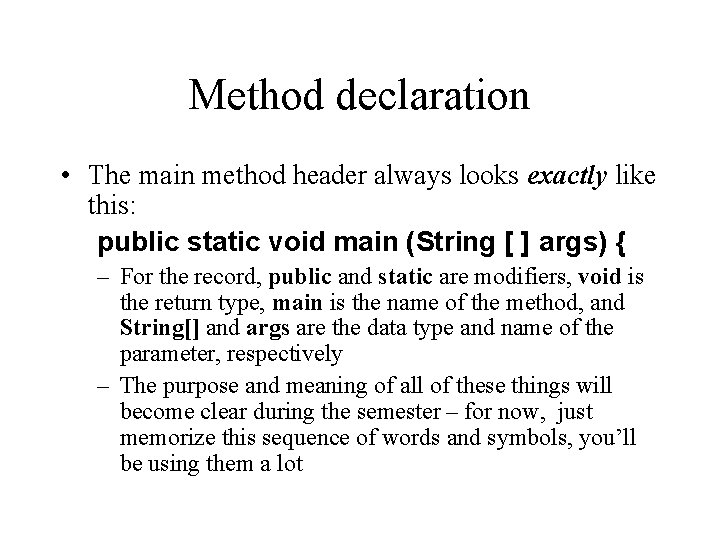
Method declaration • The main method header always looks exactly like this: public static void main (String [ ] args) { – For the record, public and static are modifiers, void is the return type, main is the name of the method, and String[] and args are the data type and name of the parameter, respectively – The purpose and meaning of all of these things will become clear during the semester – for now, just memorize this sequence of words and symbols, you’ll be using them a lot
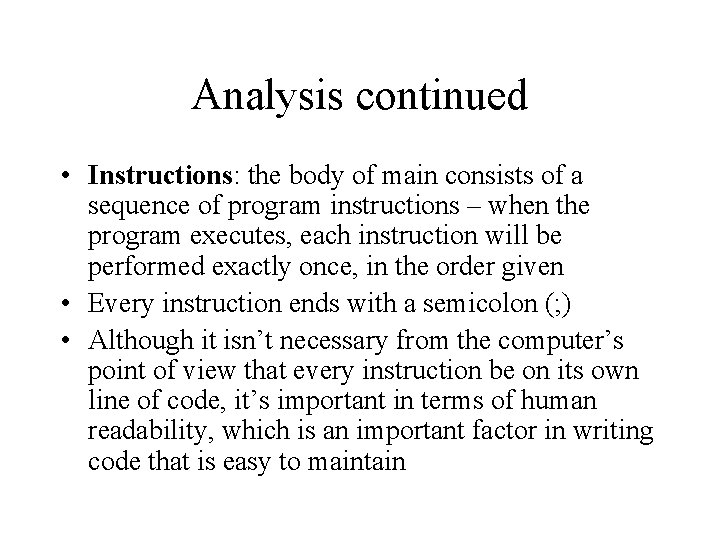
Analysis continued • Instructions: the body of main consists of a sequence of program instructions – when the program executes, each instruction will be performed exactly once, in the order given • Every instruction ends with a semicolon (; ) • Although it isn’t necessary from the computer’s point of view that every instruction be on its own line of code, it’s important in terms of human readability, which is an important factor in writing code that is easy to maintain
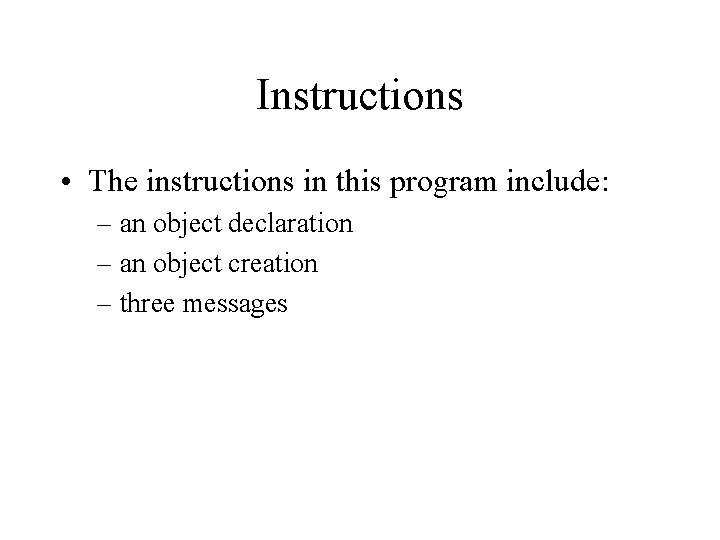
Instructions • The instructions in this program include: – an object declaration – an object creation – three messages
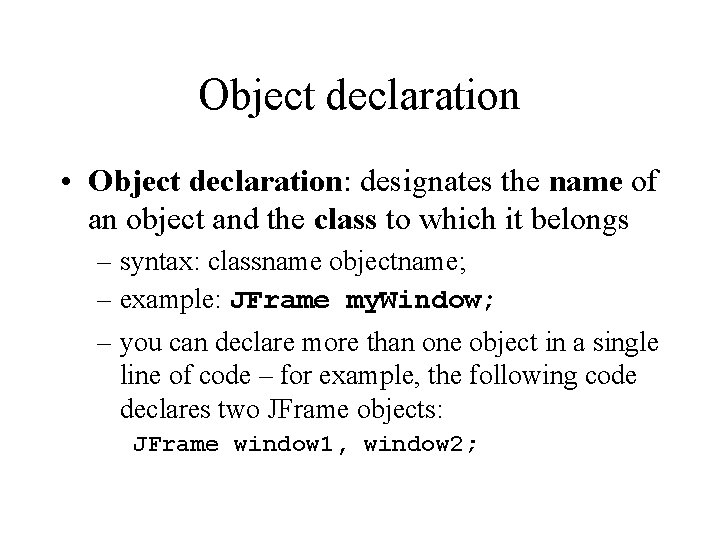
Object declaration • Object declaration: designates the name of an object and the class to which it belongs – syntax: classname objectname; – example: JFrame my. Window; – you can declare more than one object in a single line of code – for example, the following code declares two JFrame objects: JFrame window 1, window 2;
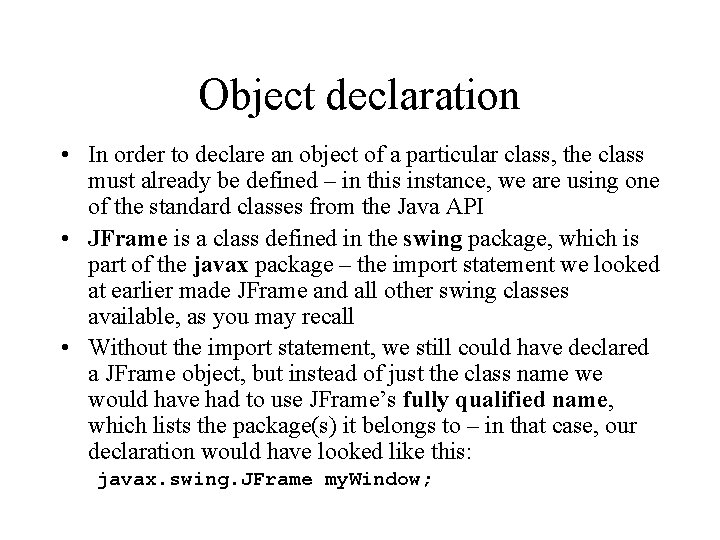
Object declaration • In order to declare an object of a particular class, the class must already be defined – in this instance, we are using one of the standard classes from the Java API • JFrame is a class defined in the swing package, which is part of the javax package – the import statement we looked at earlier made JFrame and all other swing classes available, as you may recall • Without the import statement, we still could have declared a JFrame object, but instead of just the class name we would have had to use JFrame’s fully qualified name, which lists the package(s) it belongs to – in that case, our declaration would have looked like this: javax. swing. JFrame my. Window;
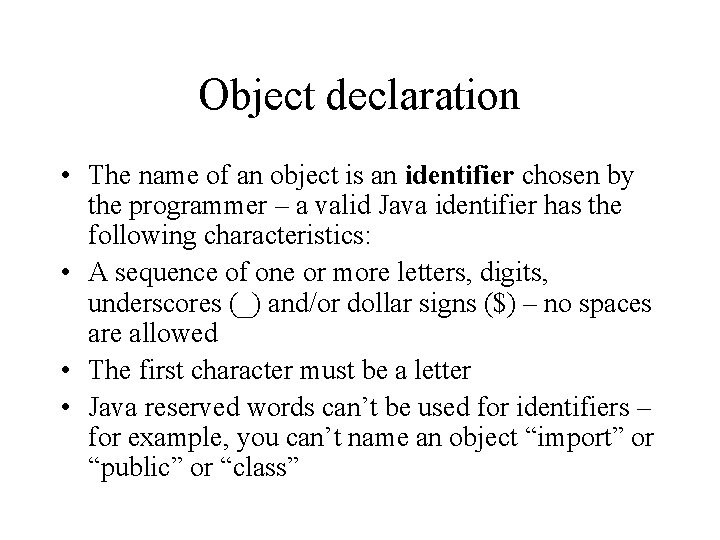
Object declaration • The name of an object is an identifier chosen by the programmer – a valid Java identifier has the following characteristics: • A sequence of one or more letters, digits, underscores (_) and/or dollar signs ($) – no spaces are allowed • The first character must be a letter • Java reserved words can’t be used for identifiers – for example, you can’t name an object “import” or “public” or “class”
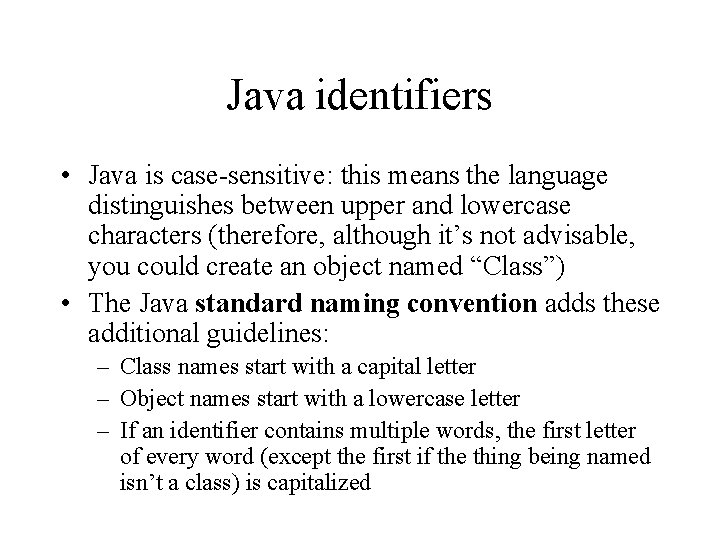
Java identifiers • Java is case-sensitive: this means the language distinguishes between upper and lowercase characters (therefore, although it’s not advisable, you could create an object named “Class”) • The Java standard naming convention adds these additional guidelines: – Class names start with a capital letter – Object names start with a lowercase letter – If an identifier contains multiple words, the first letter of every word (except the first if the thing being named isn’t a class) is capitalized
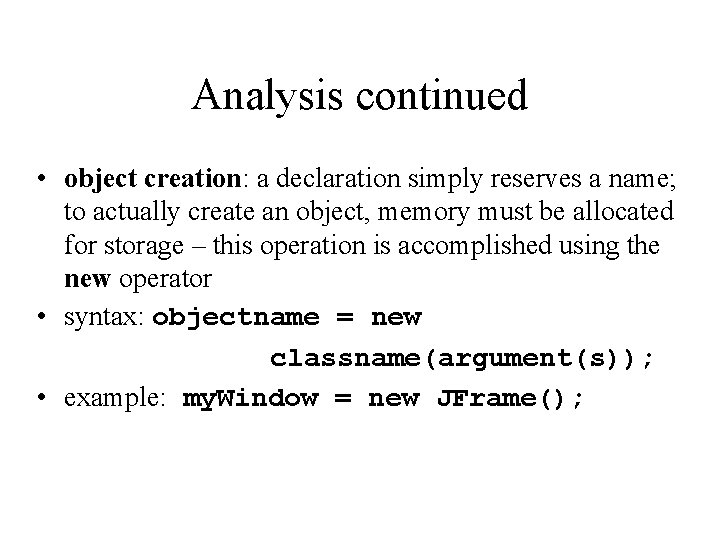
Analysis continued • object creation: a declaration simply reserves a name; to actually create an object, memory must be allocated for storage – this operation is accomplished using the new operator • syntax: objectname = new classname(argument(s)); • example: my. Window = new JFrame();
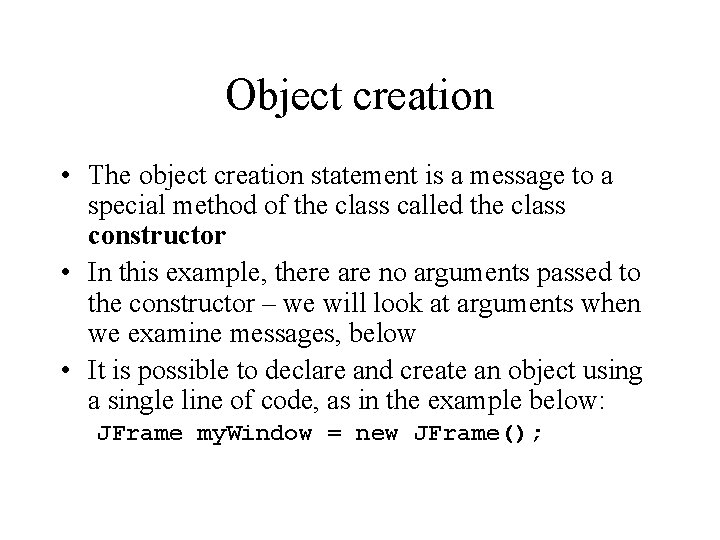
Object creation • The object creation statement is a message to a special method of the class called the class constructor • In this example, there are no arguments passed to the constructor – we will look at arguments when we examine messages, below • It is possible to declare and create an object using a single line of code, as in the example below: JFrame my. Window = new JFrame();
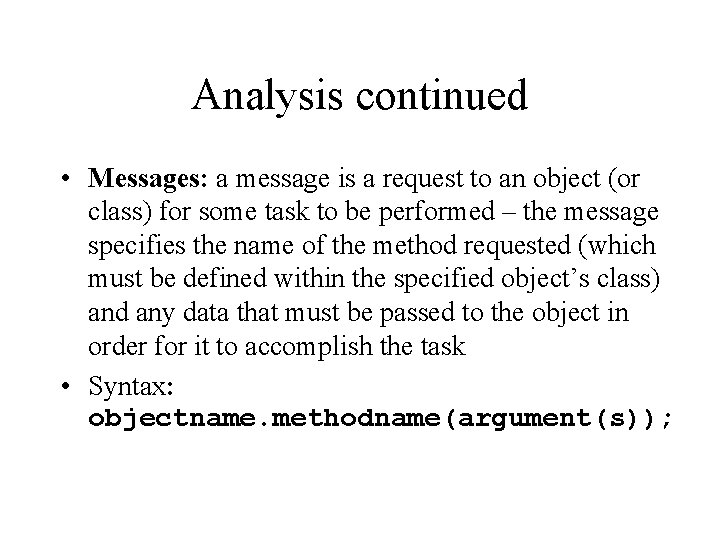
Analysis continued • Messages: a message is a request to an object (or class) for some task to be performed – the message specifies the name of the method requested (which must be defined within the specified object’s class) and any data that must be passed to the object in order for it to accomplish the task • Syntax: objectname. methodname(argument(s));
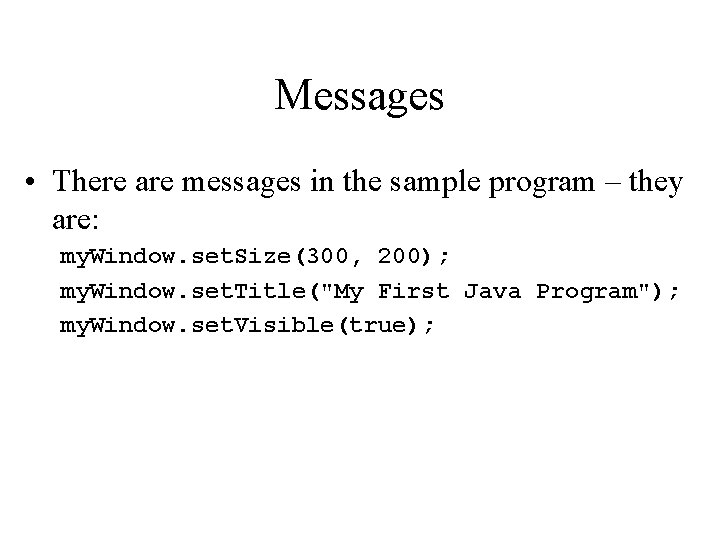
Messages • There are messages in the sample program – they are: my. Window. set. Size(300, 200); my. Window. set. Title("My First Java Program"); my. Window. set. Visible(true);
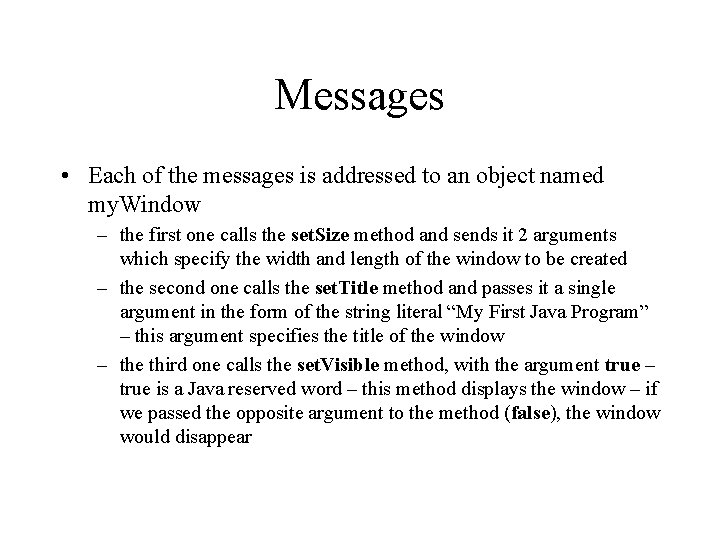
Messages • Each of the messages is addressed to an object named my. Window – the first one calls the set. Size method and sends it 2 arguments which specify the width and length of the window to be created – the second one calls the set. Title method and passes it a single argument in the form of the string literal “My First Java Program” – this argument specifies the title of the window – the third one calls the set. Visible method, with the argument true – true is a Java reserved word – this method displays the window – if we passed the opposite argument to the method (false), the window would disappear
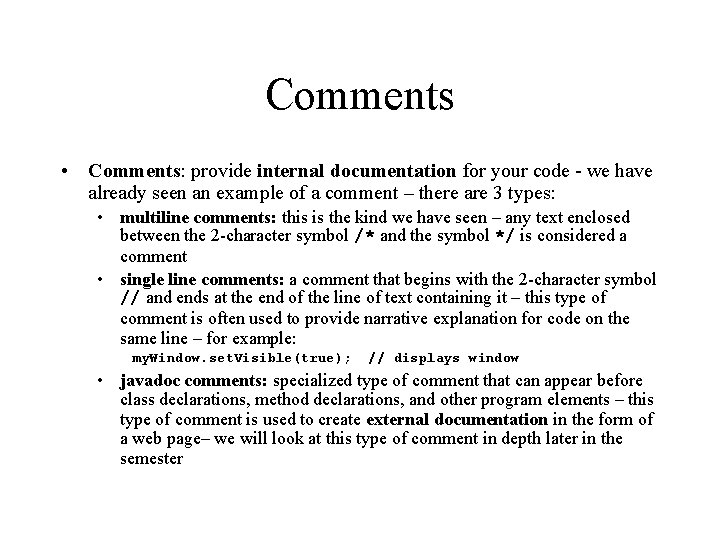
Comments • Comments: provide internal documentation for your code - we have already seen an example of a comment – there are 3 types: • multiline comments: this is the kind we have seen – any text enclosed between the 2 -character symbol /* and the symbol */ is considered a comment • single line comments: a comment that begins with the 2 -character symbol // and ends at the end of the line of text containing it – this type of comment is often used to provide narrative explanation for code on the same line – for example: my. Window. set. Visible(true); // displays window • javadoc comments: specialized type of comment that can appear before class declarations, method declarations, and other program elements – this type of comment is used to create external documentation in the form of a web page– we will look at this type of comment in depth later in the semester