Introduction to Java IST 311 MIS 307 Java
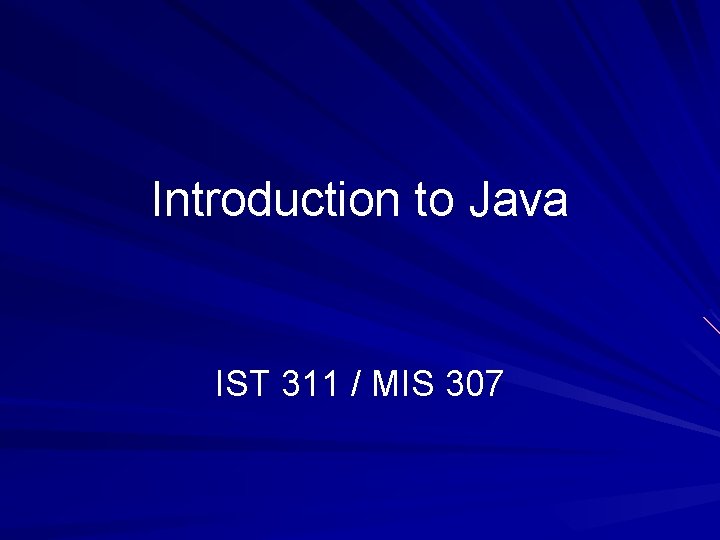
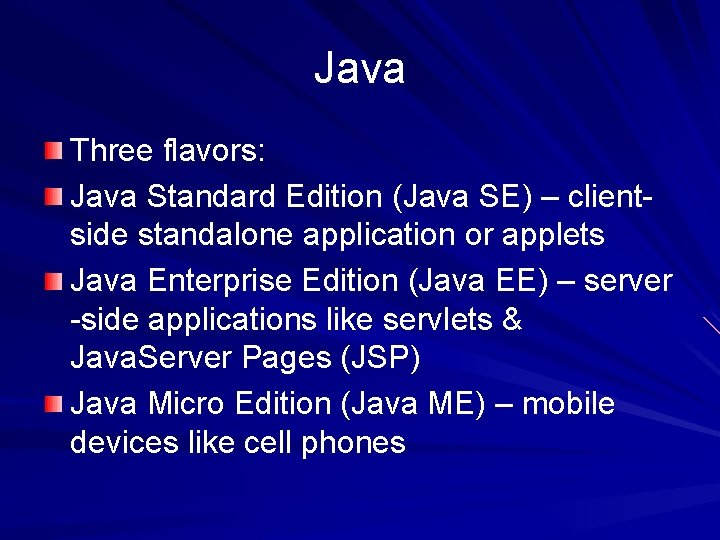
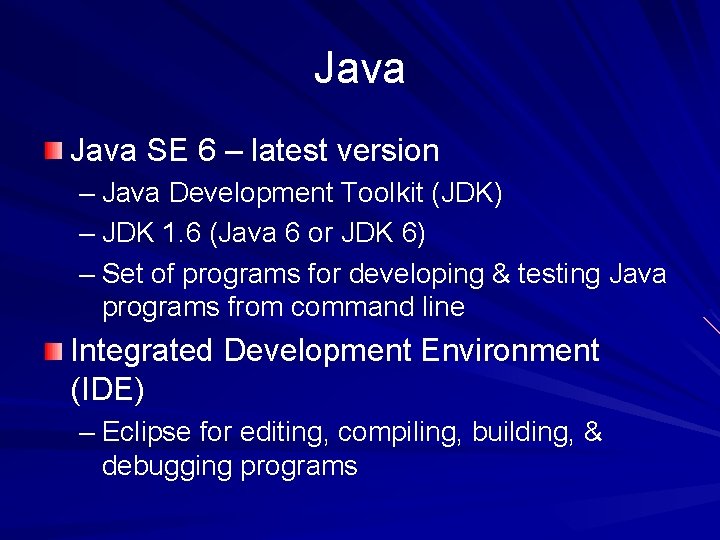
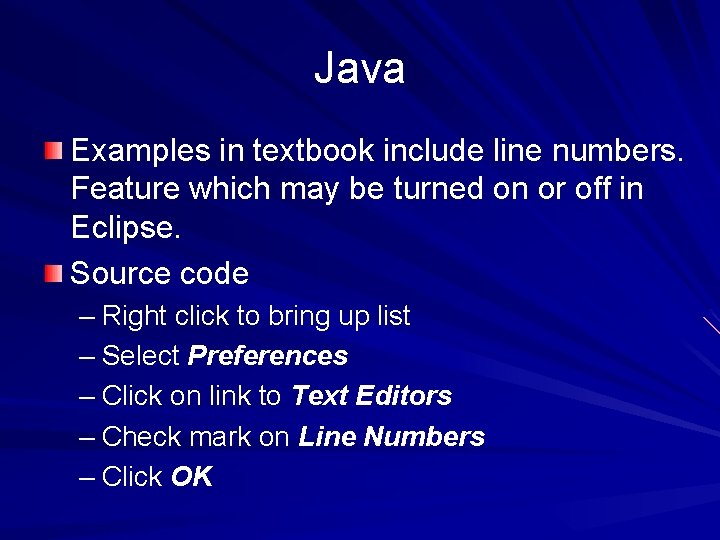
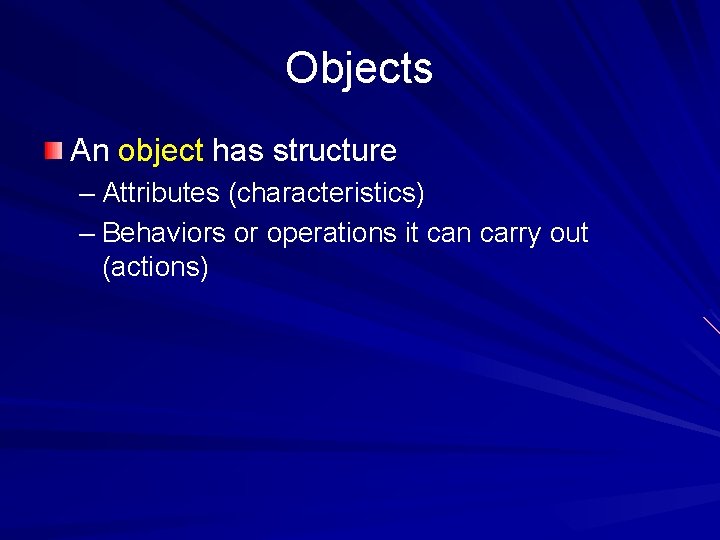
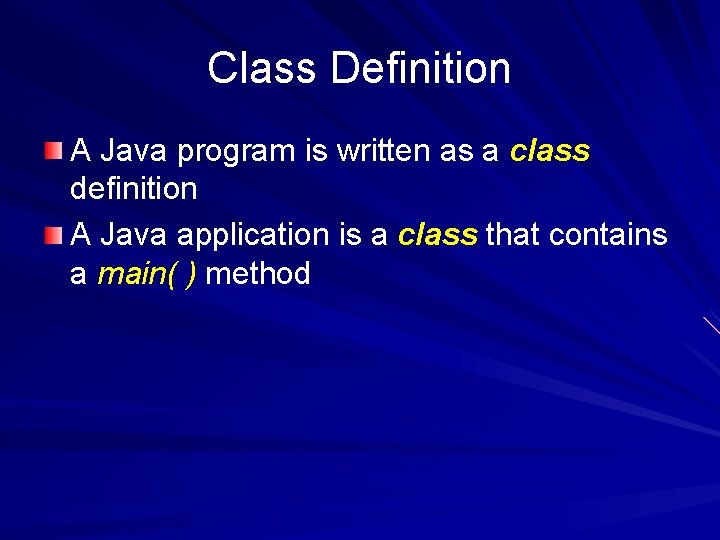
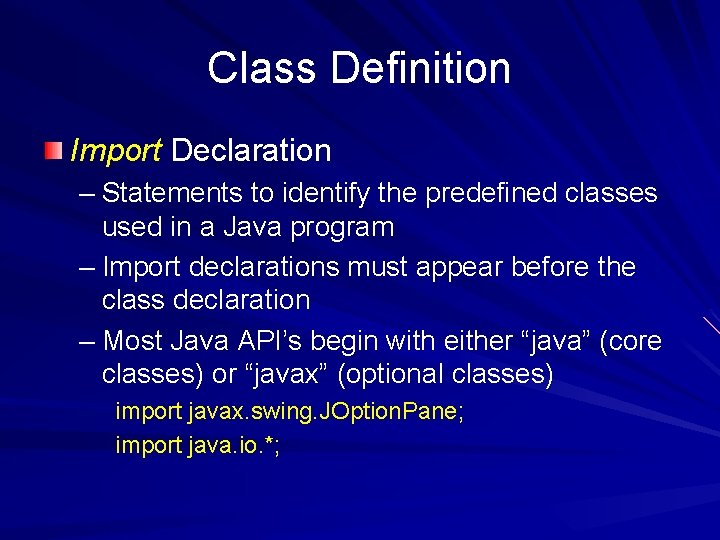
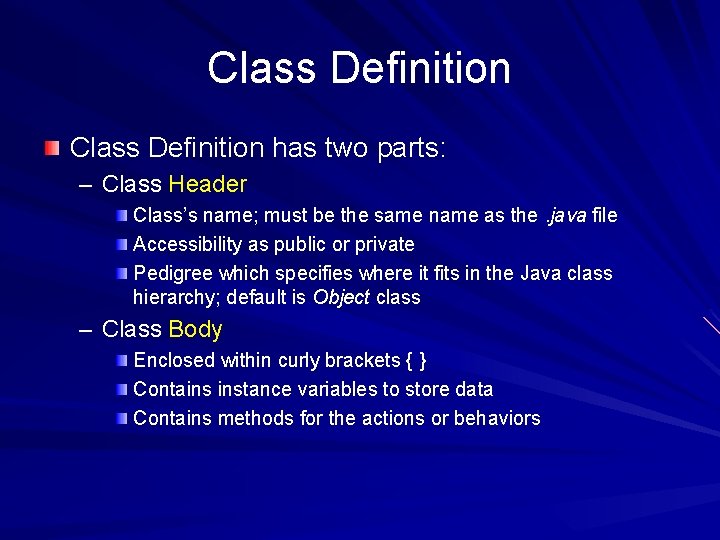
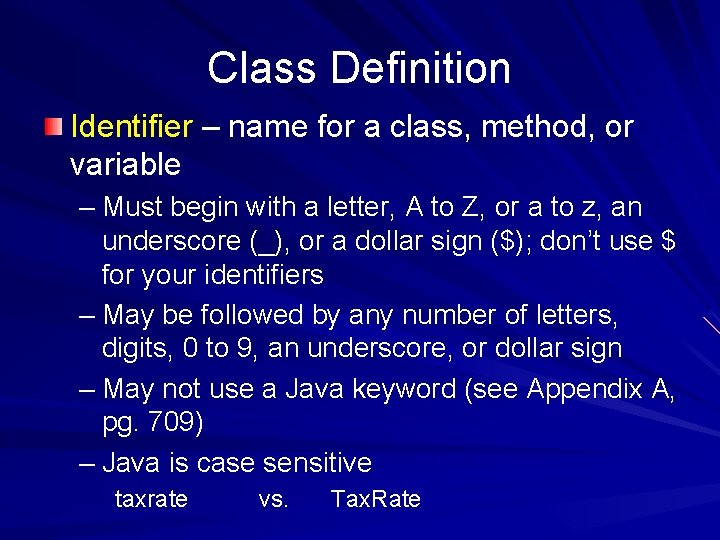
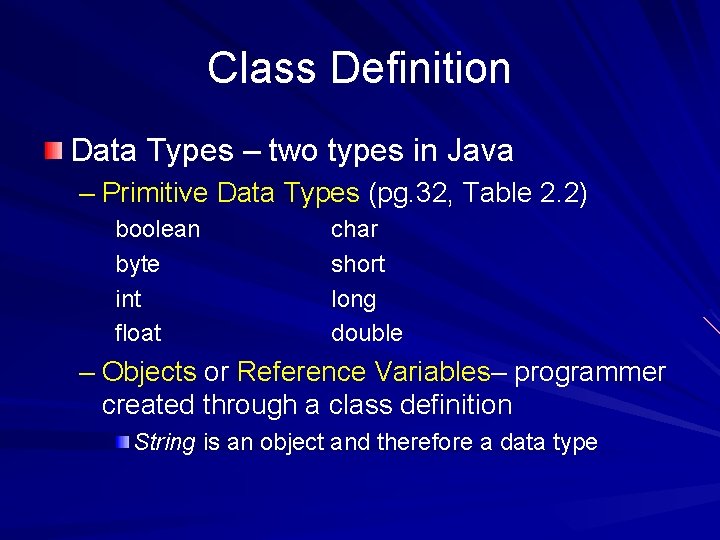
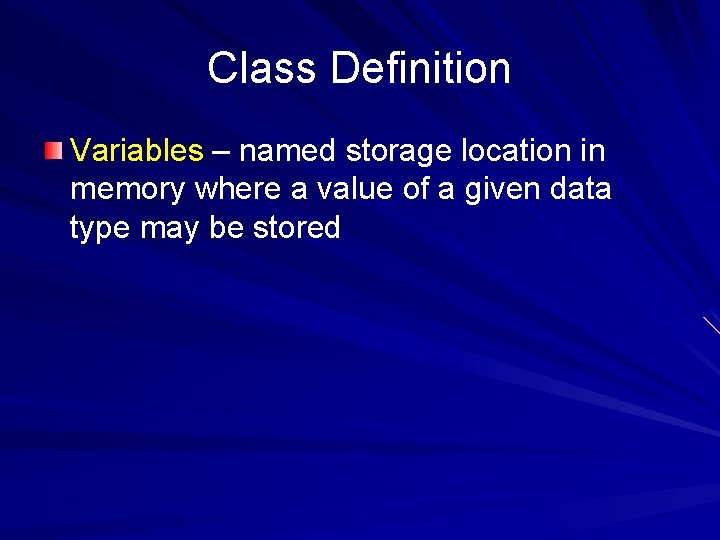
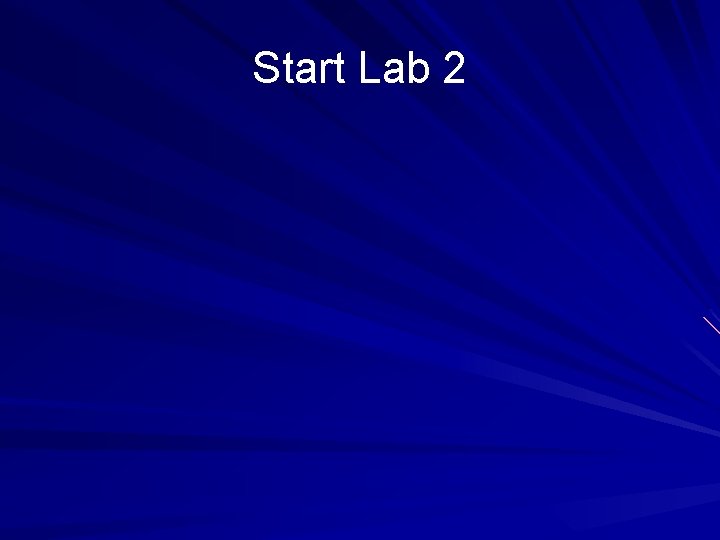
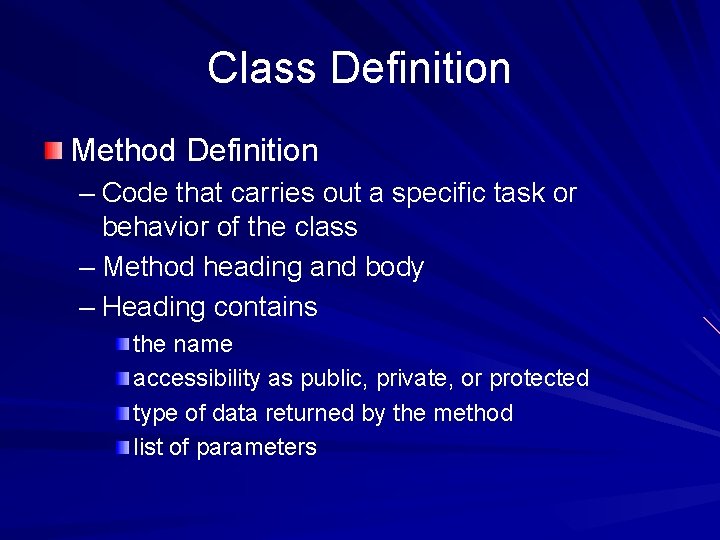
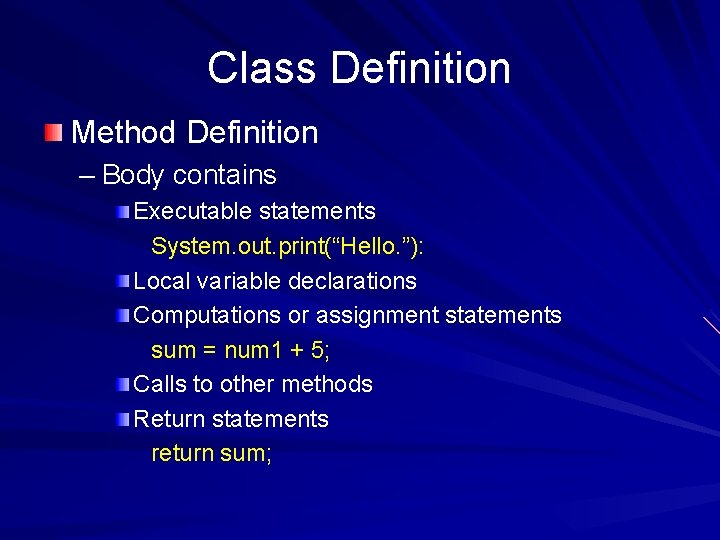
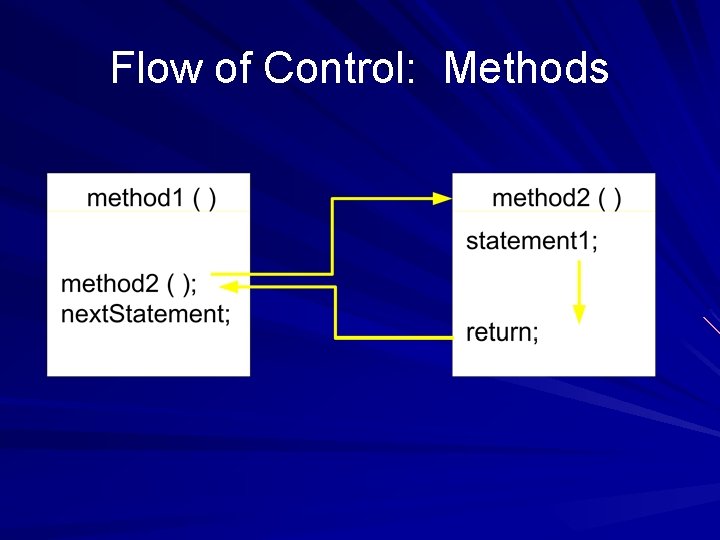
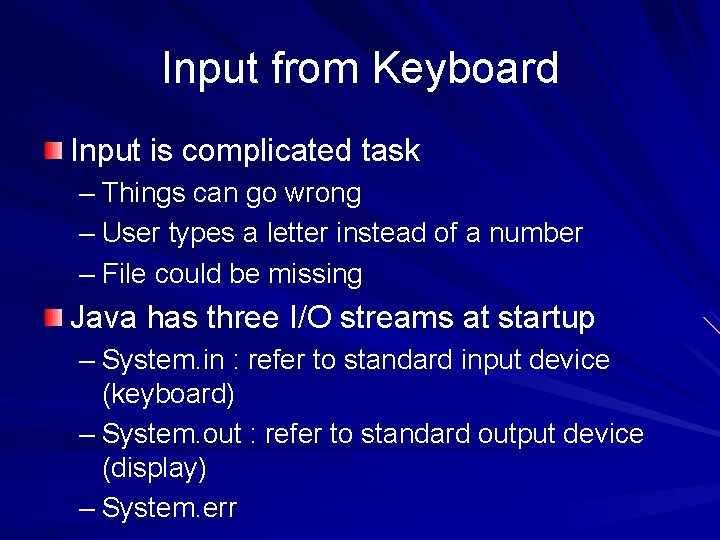
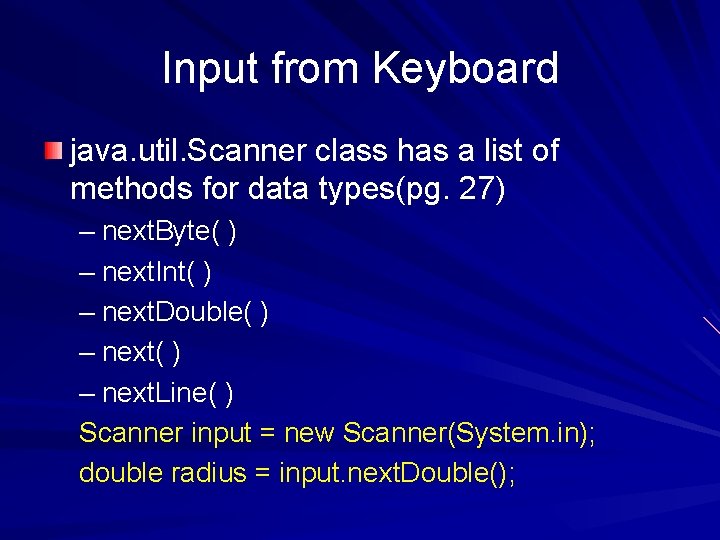
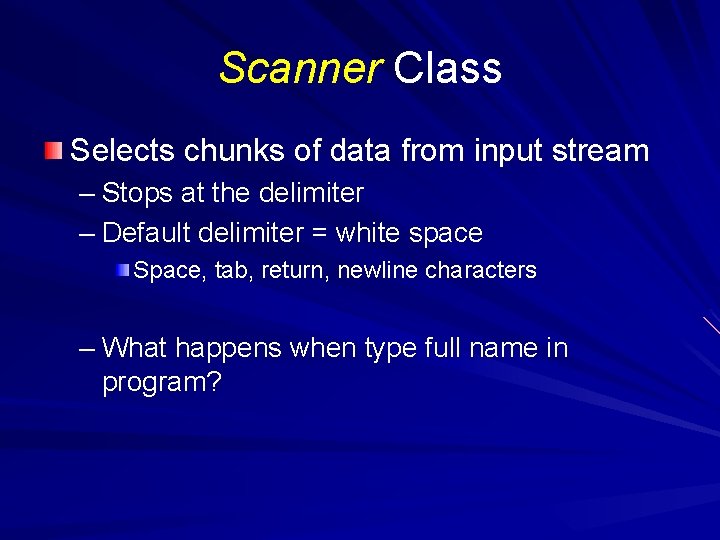
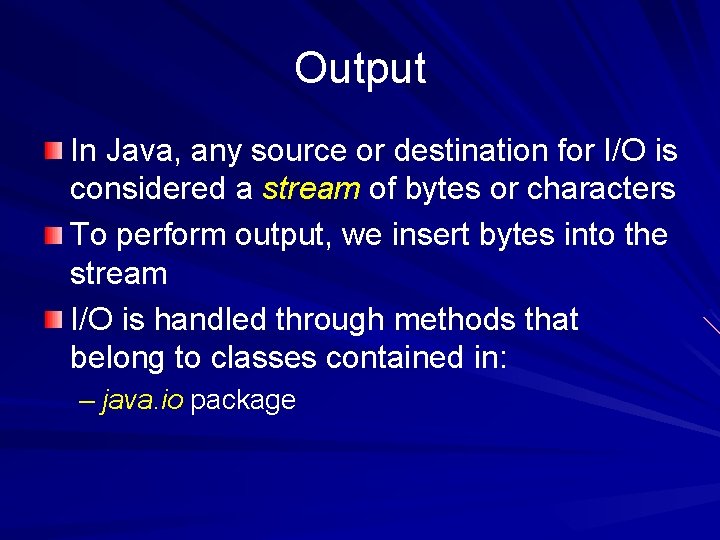
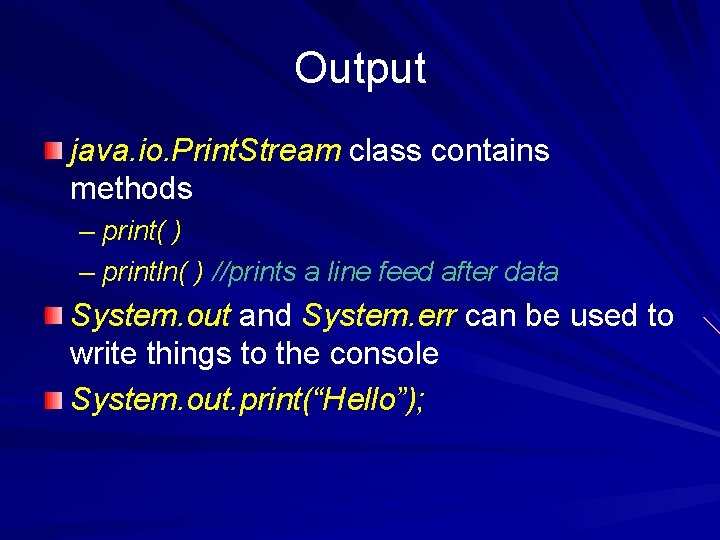
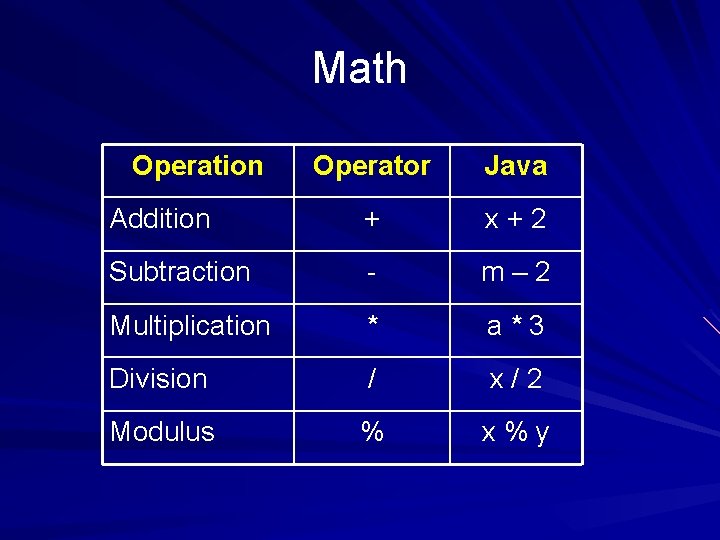
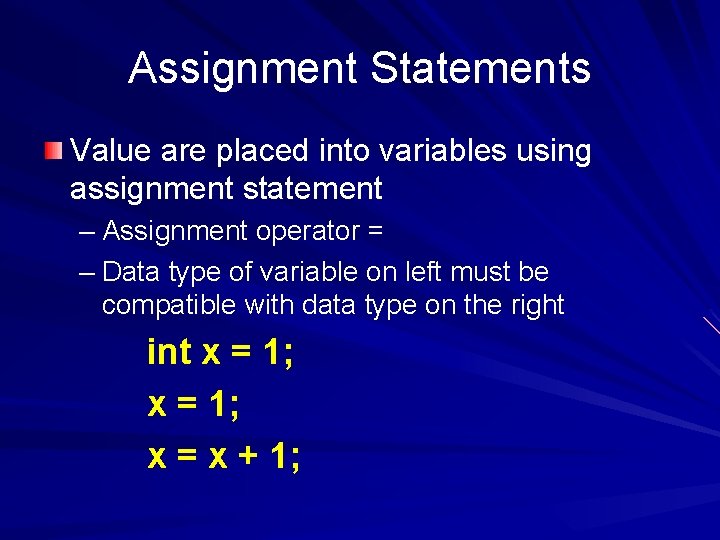
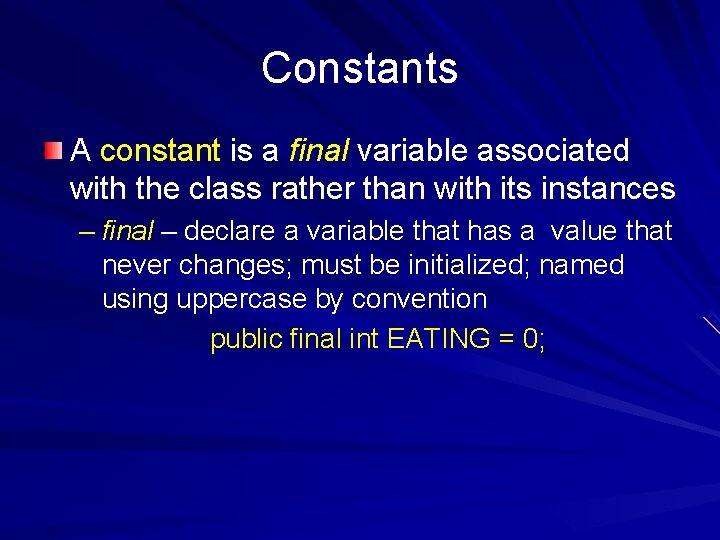
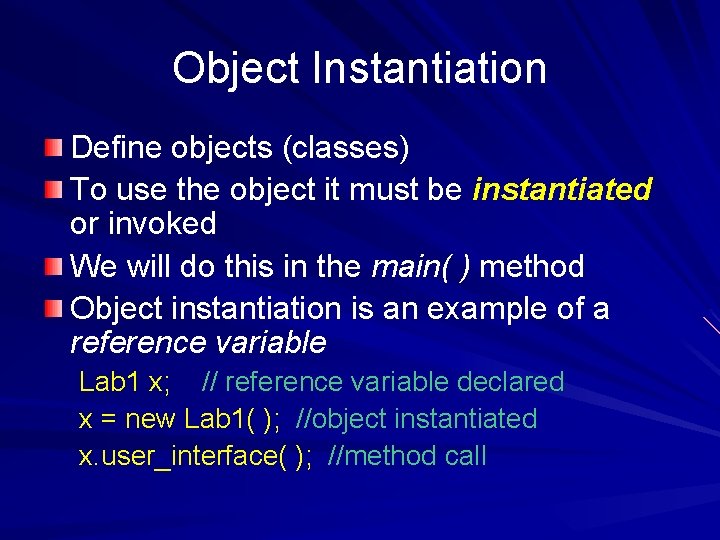
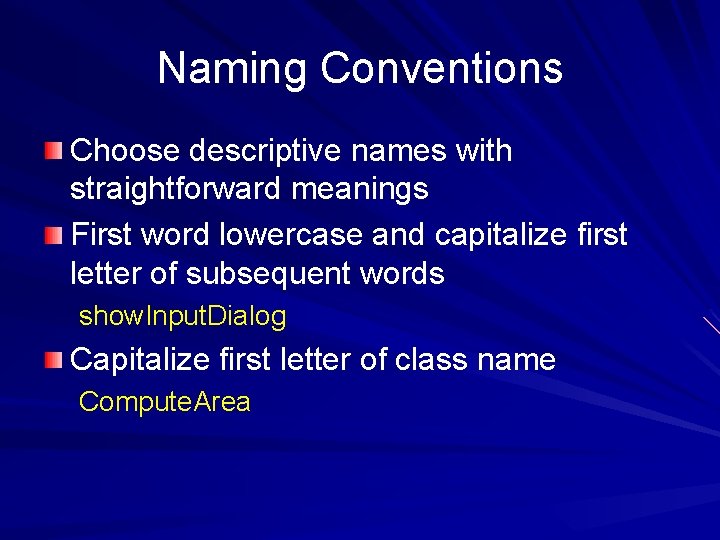
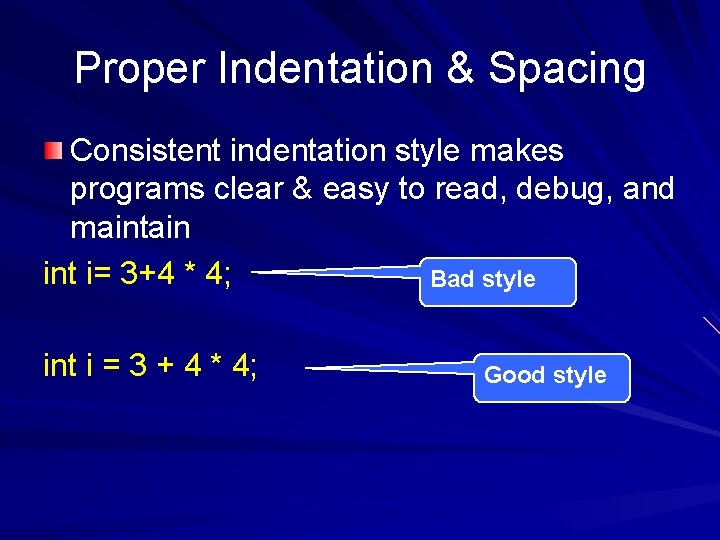
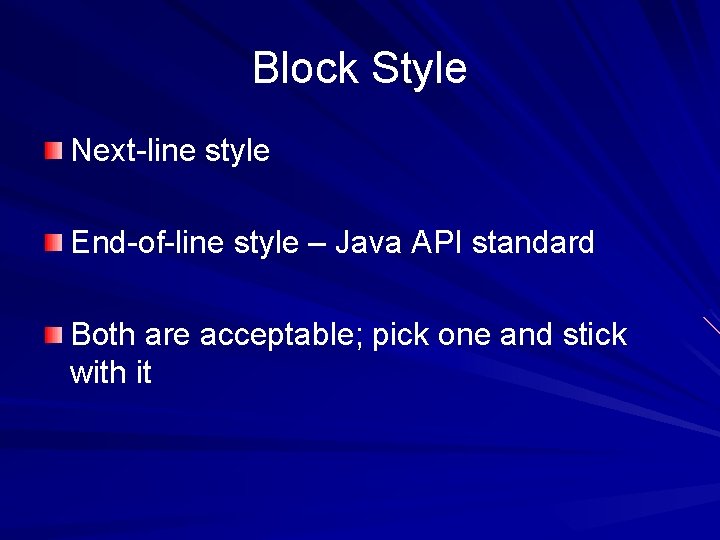
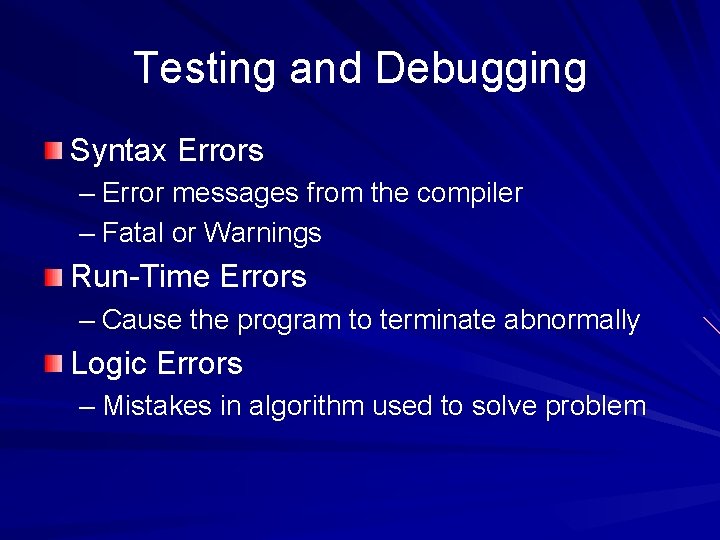
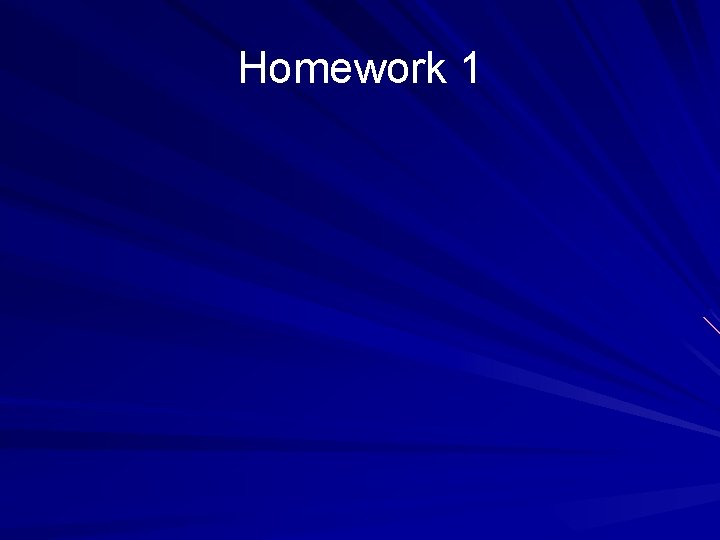
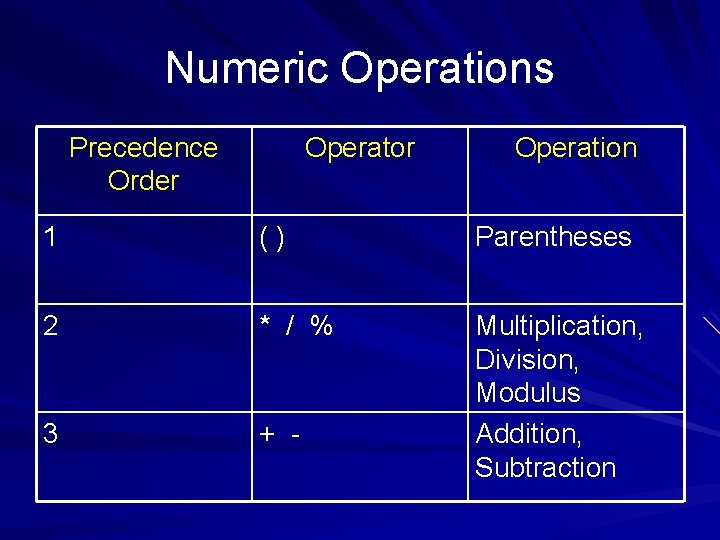
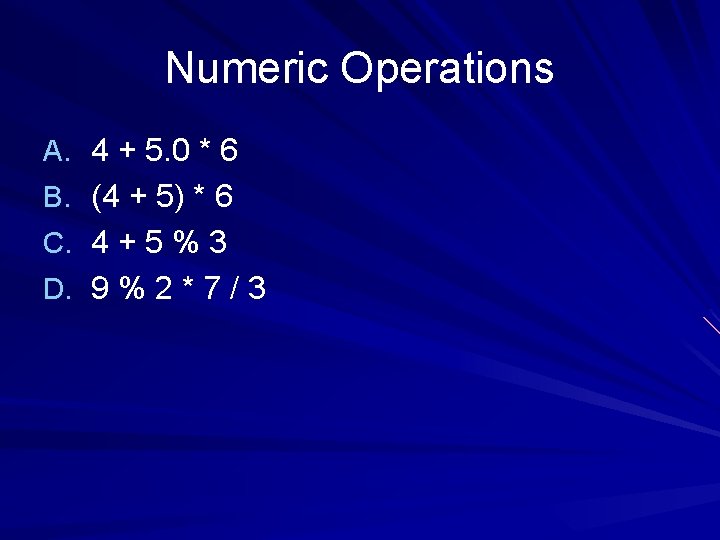
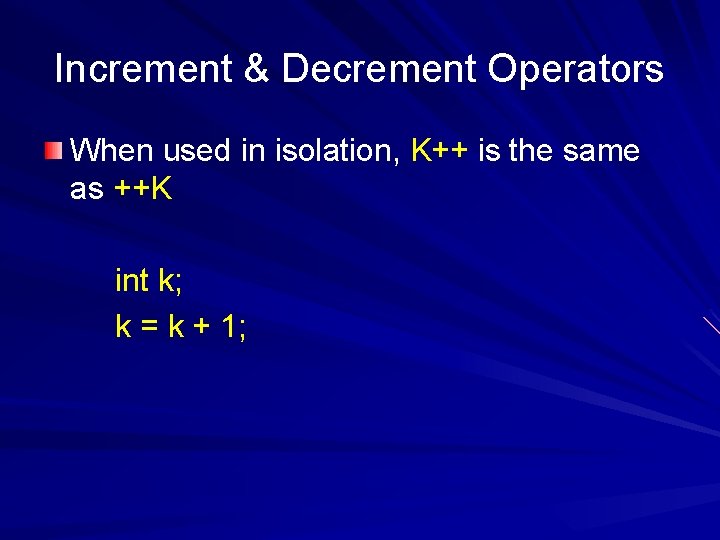
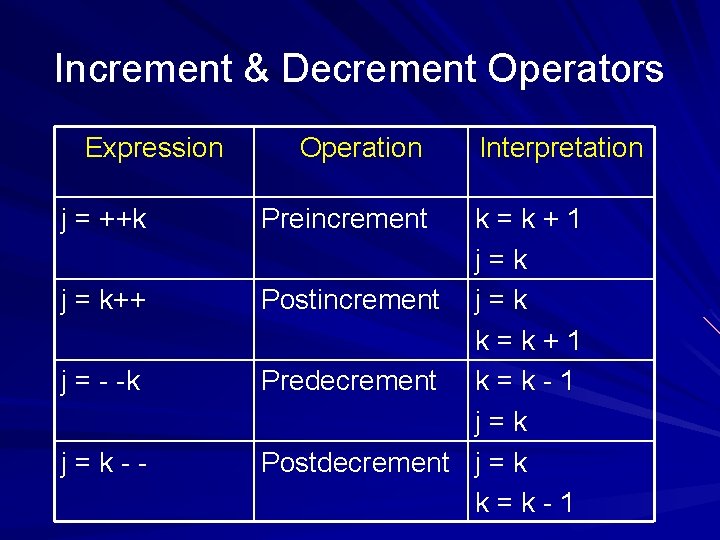
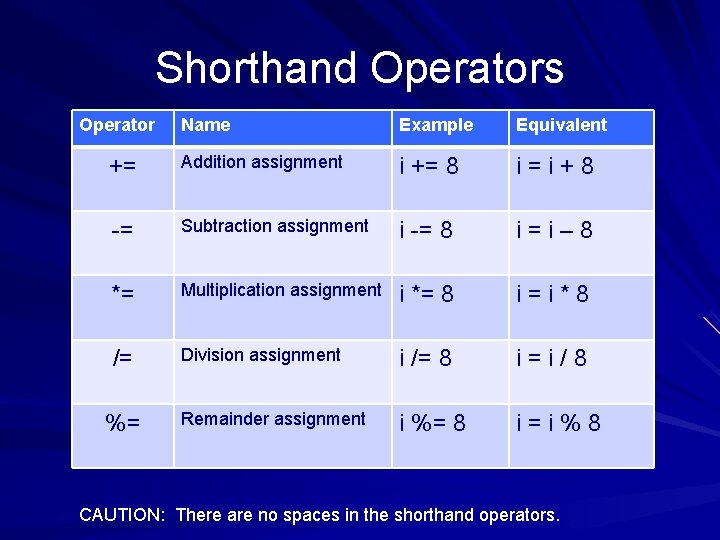
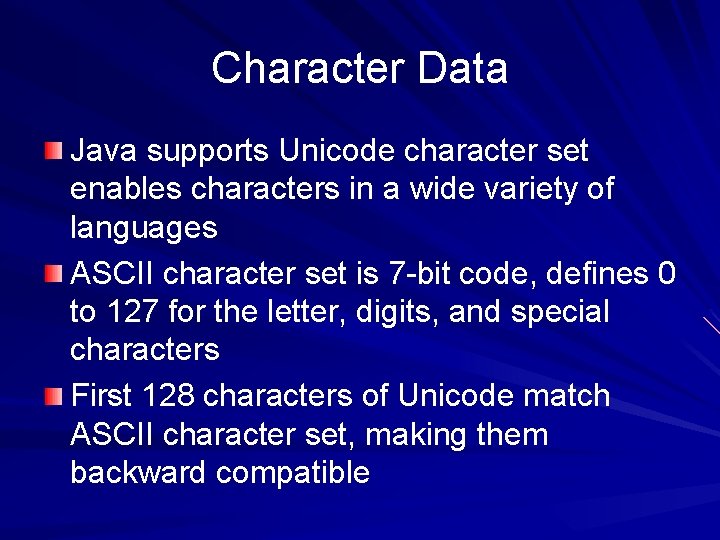
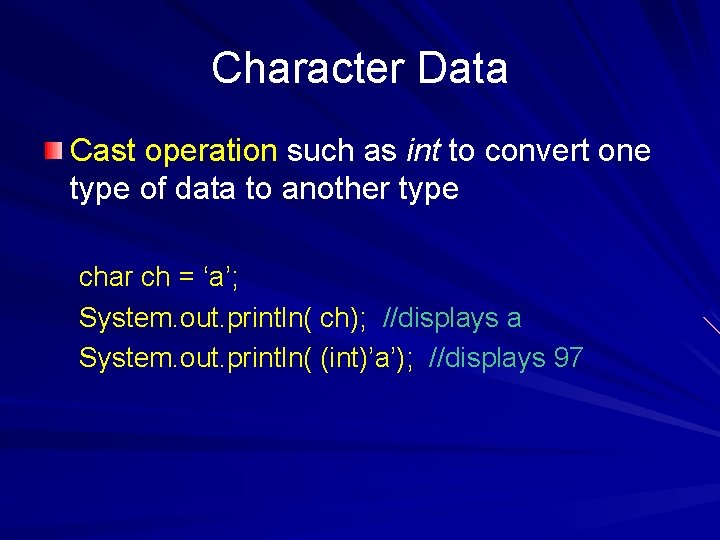
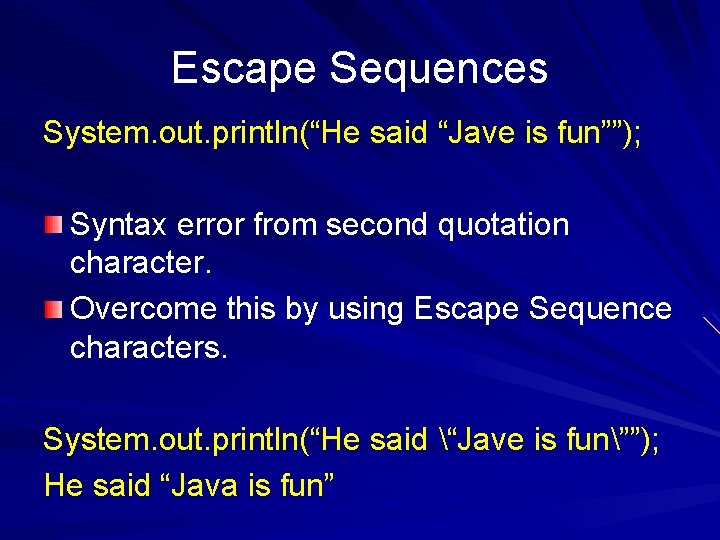
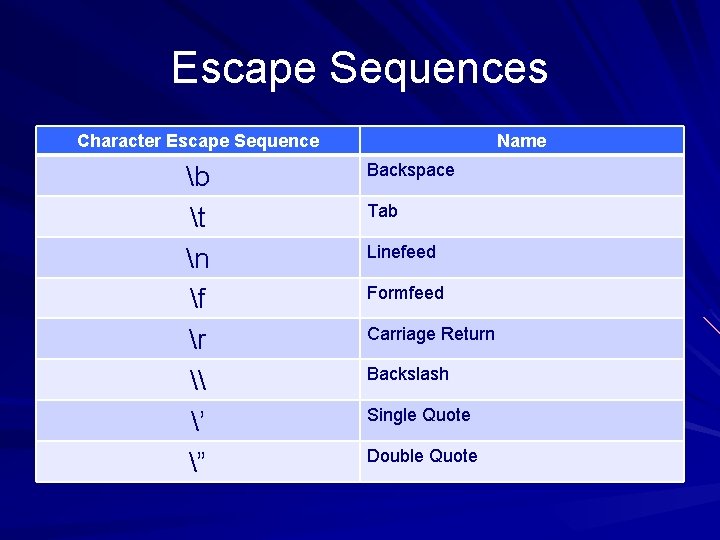
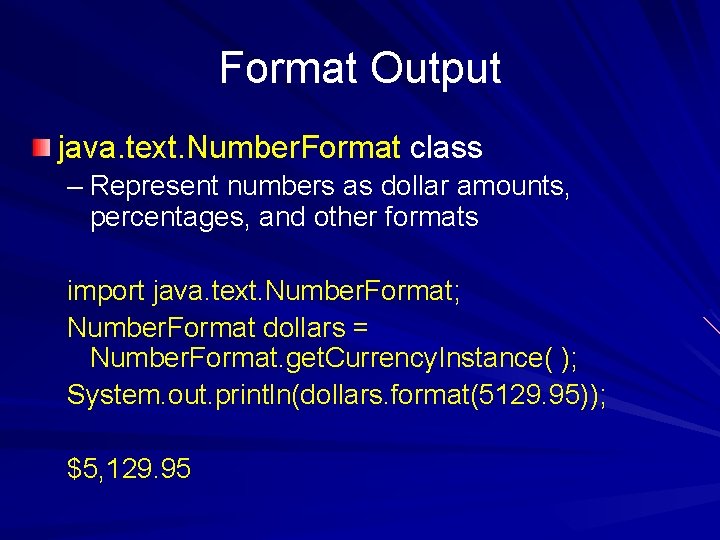
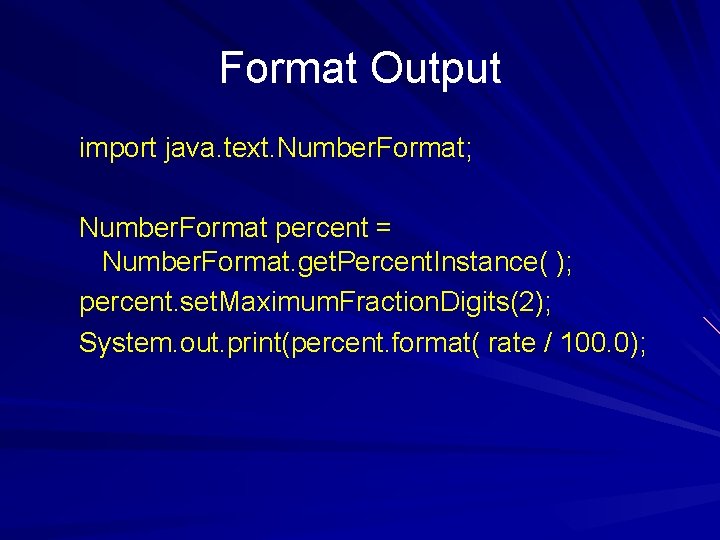
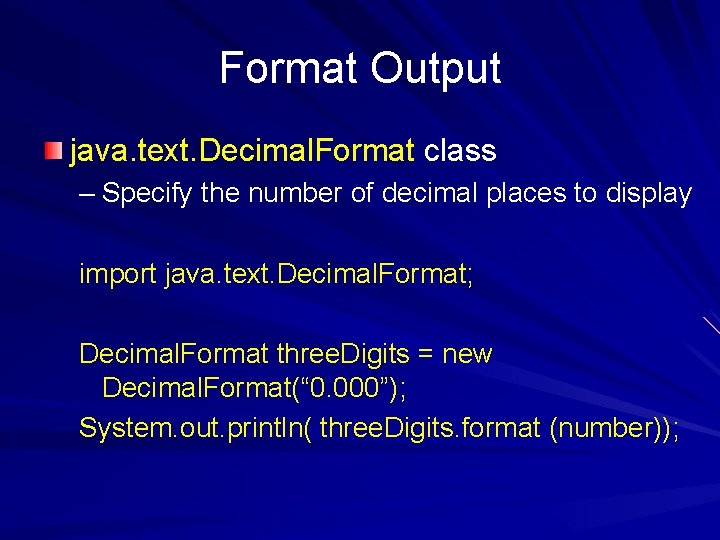
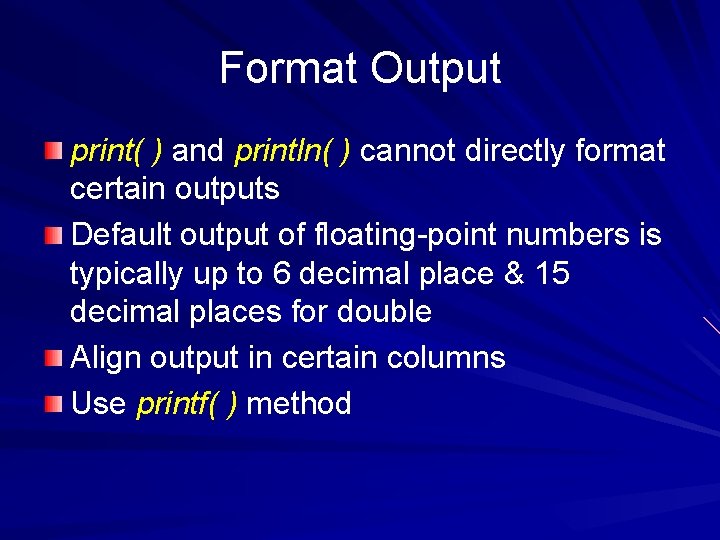
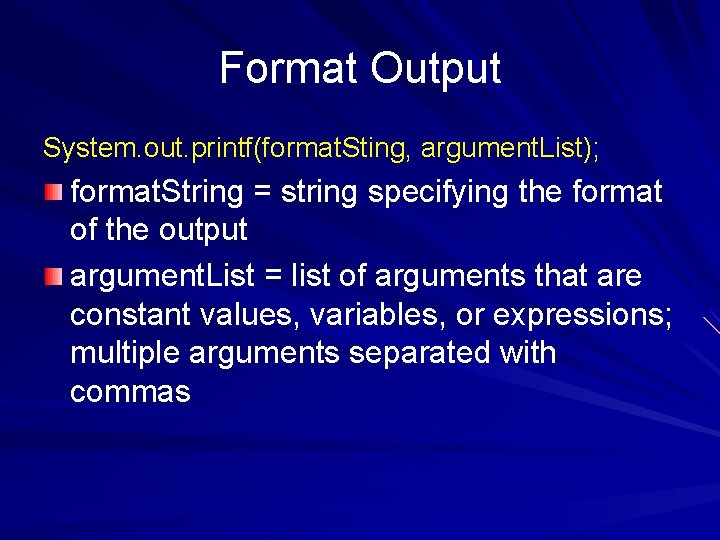
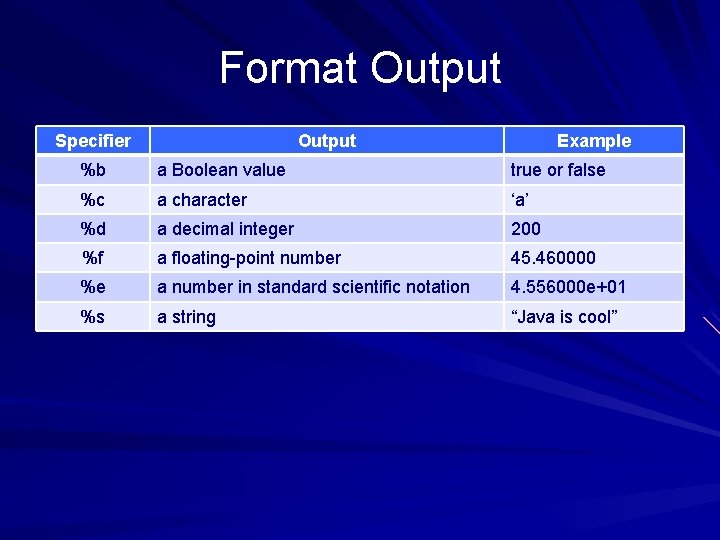
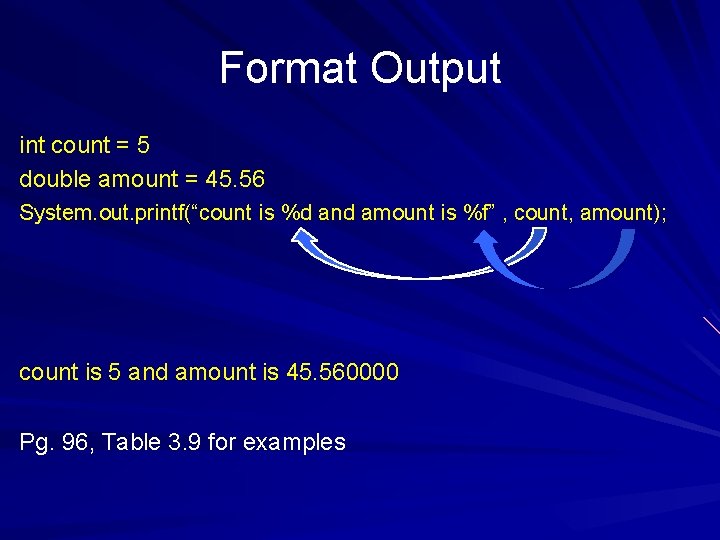
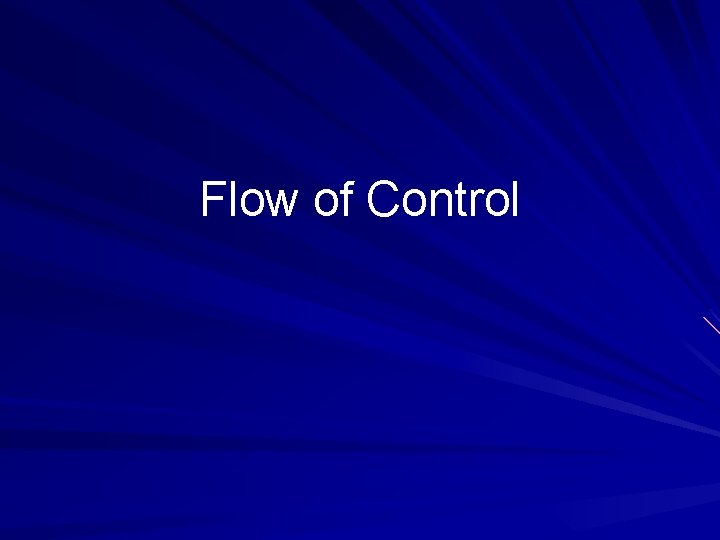
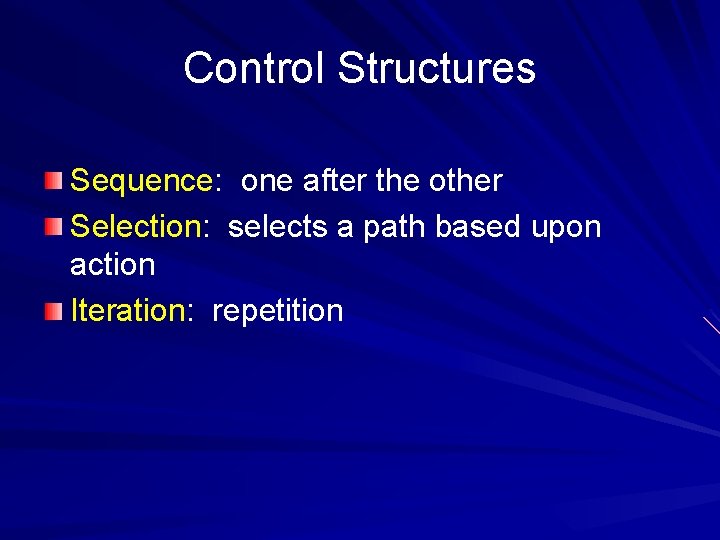
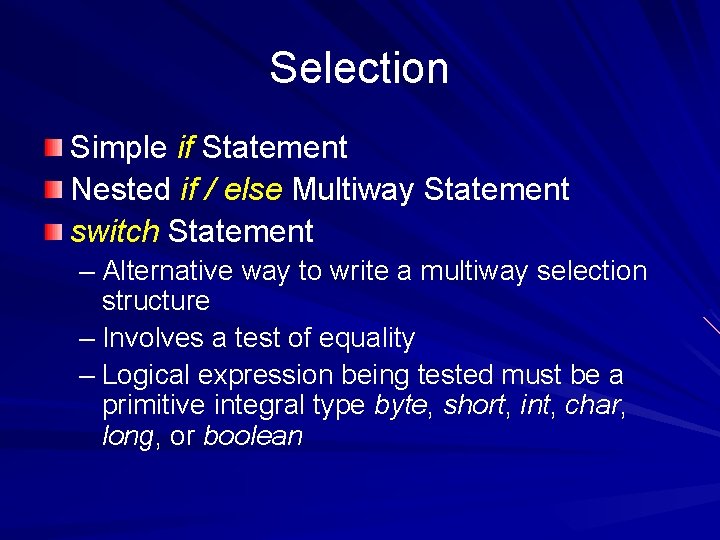
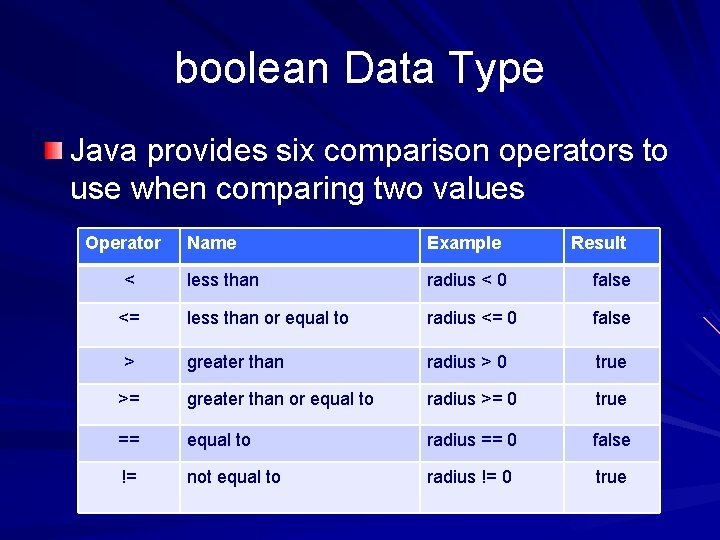
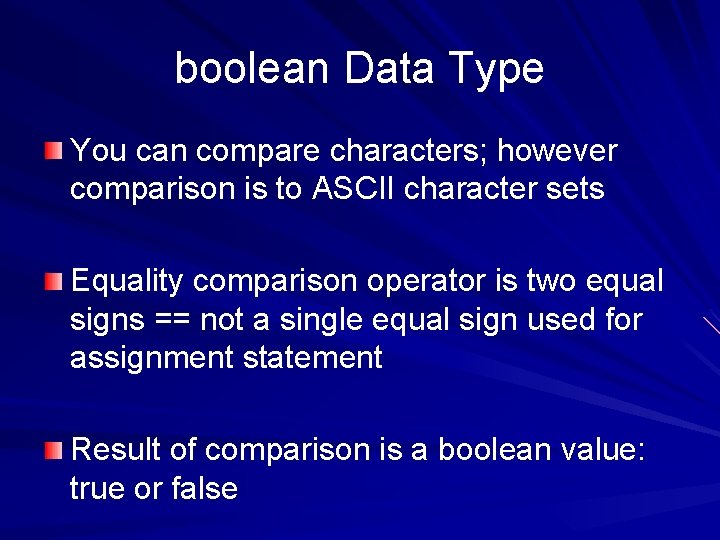
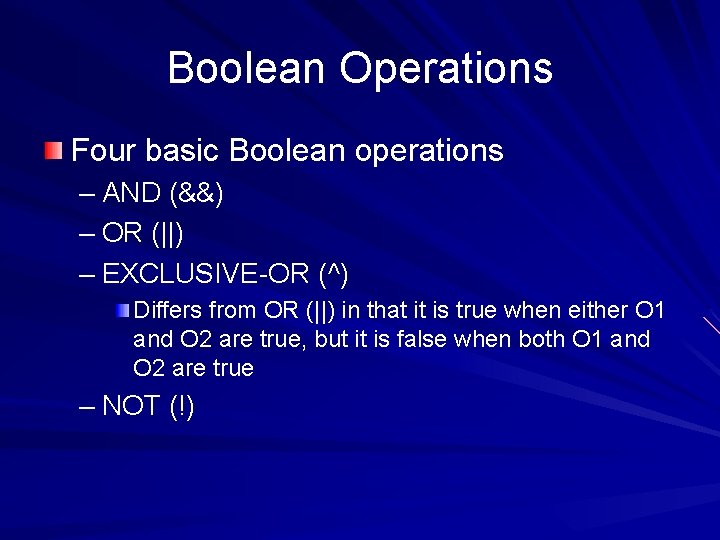
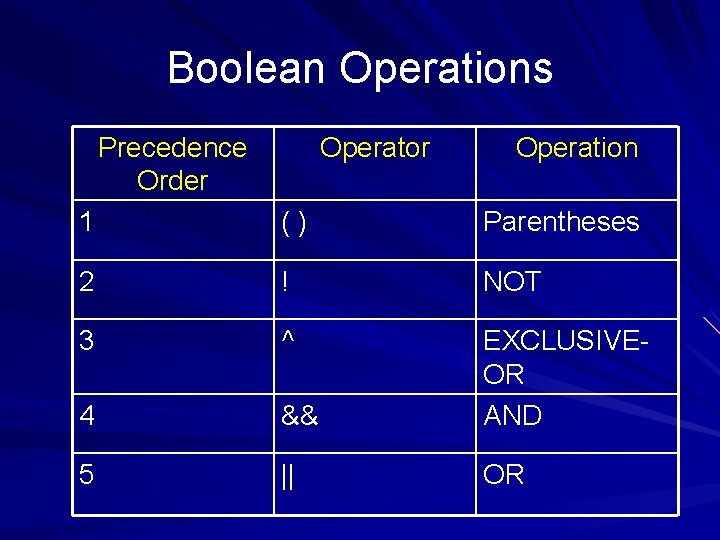
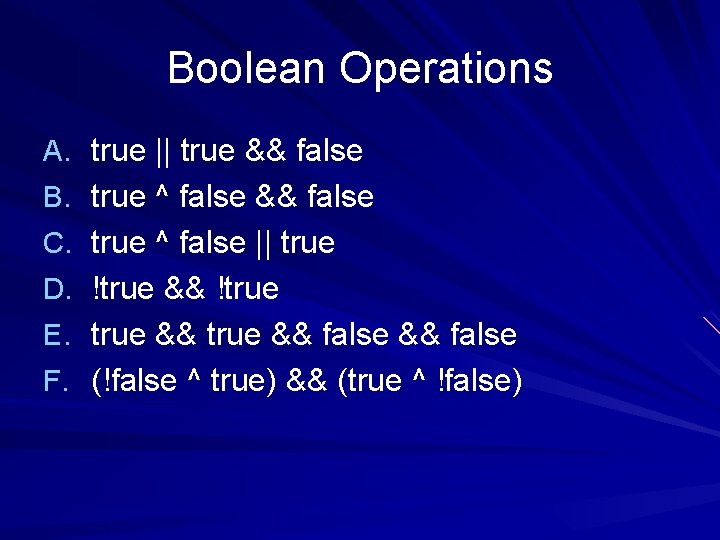
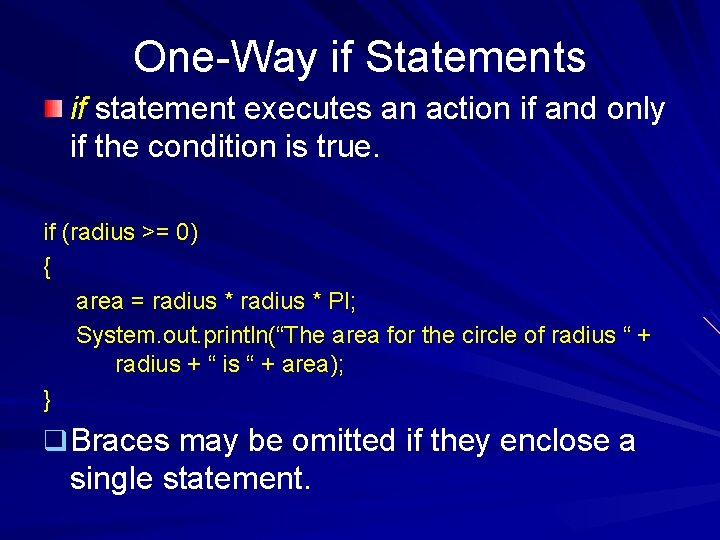
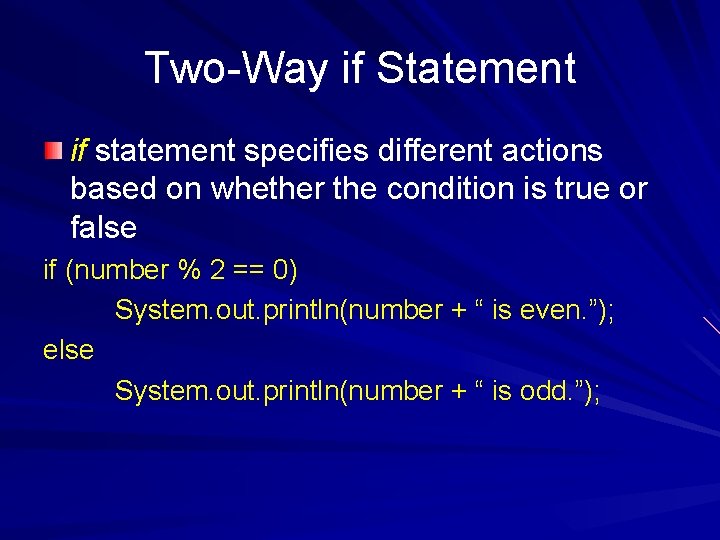
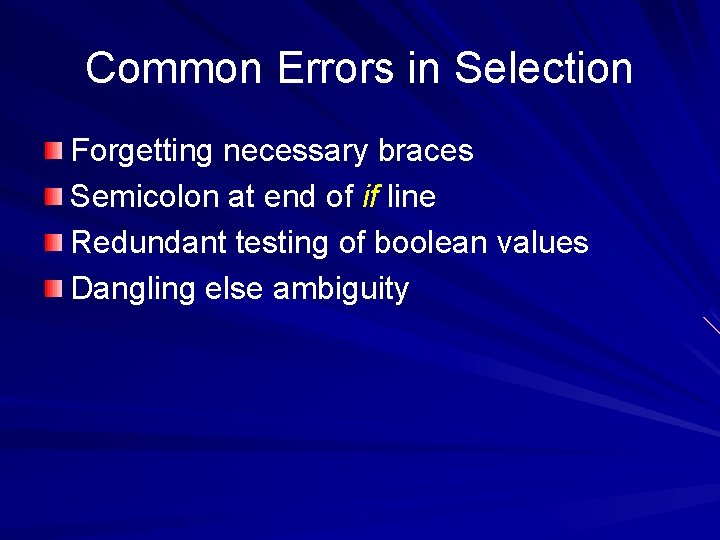
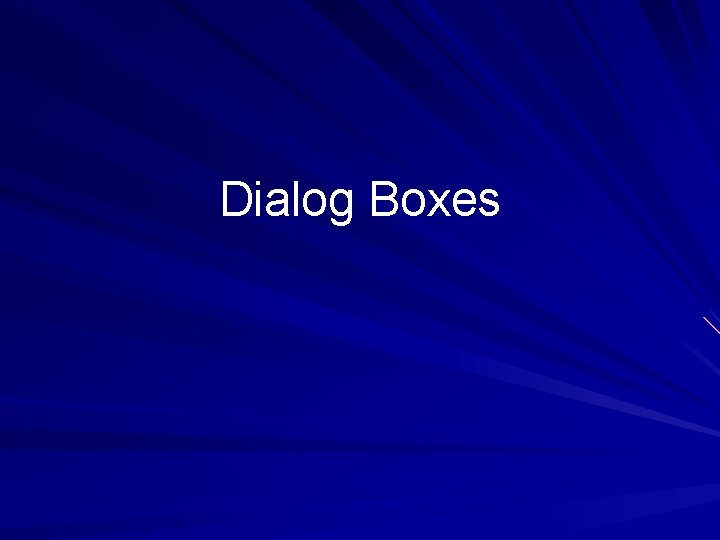
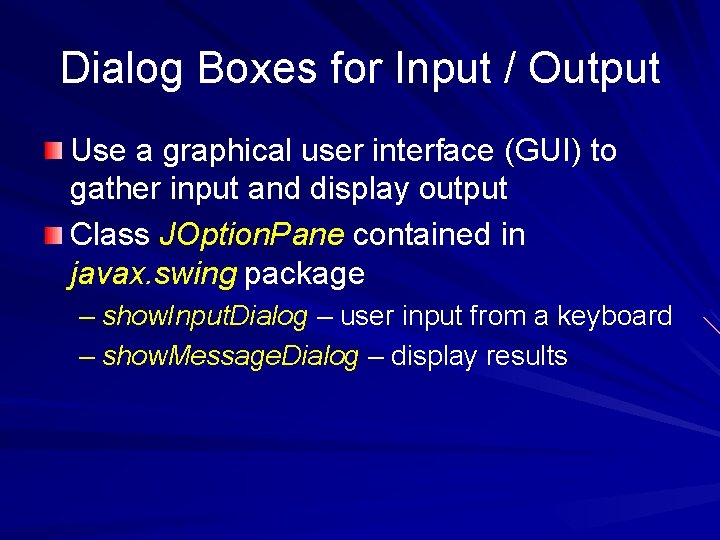
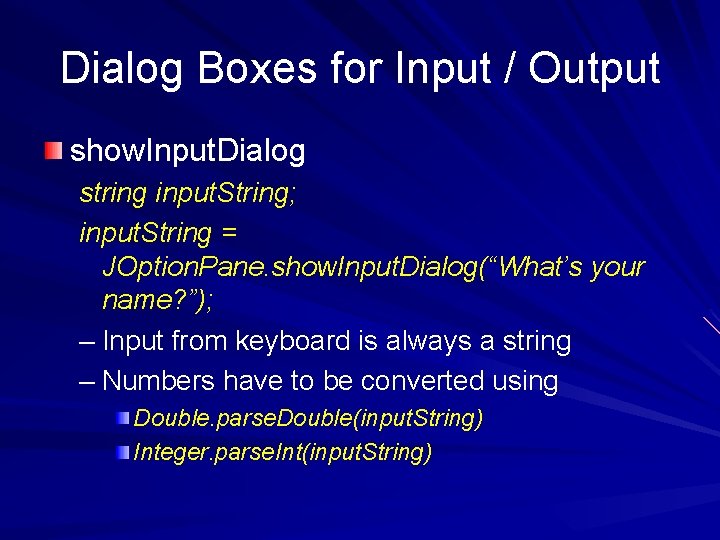
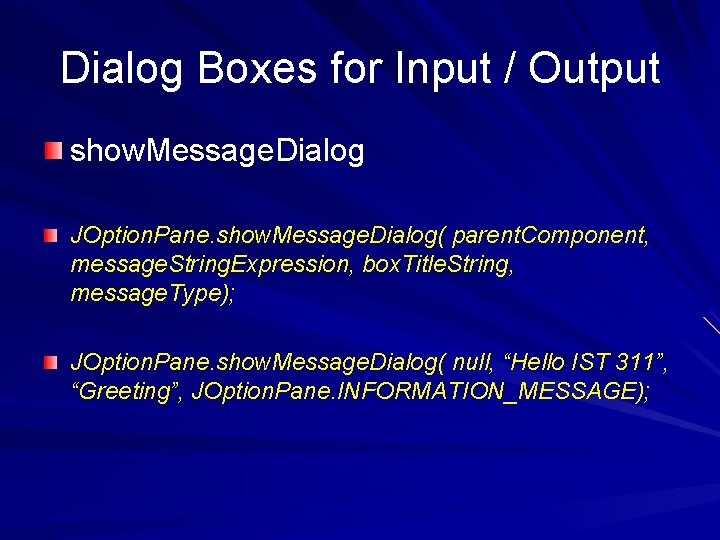
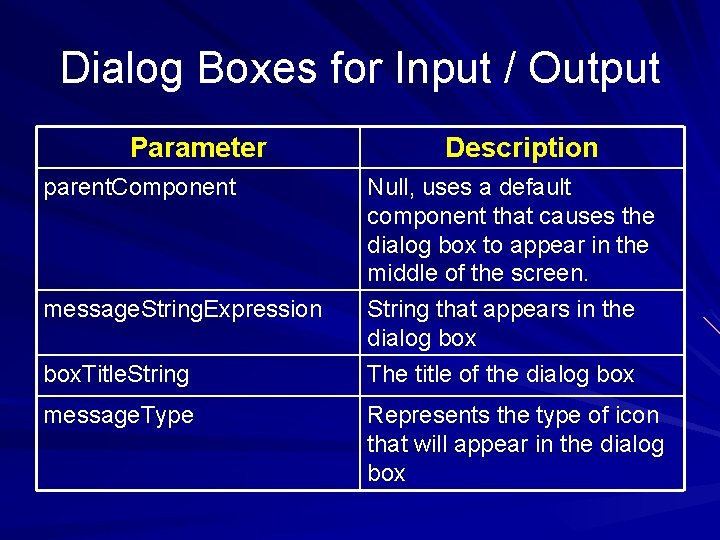
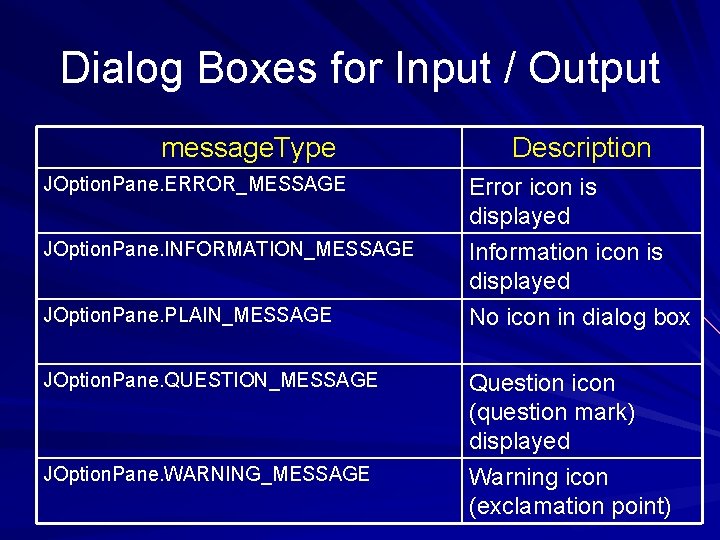
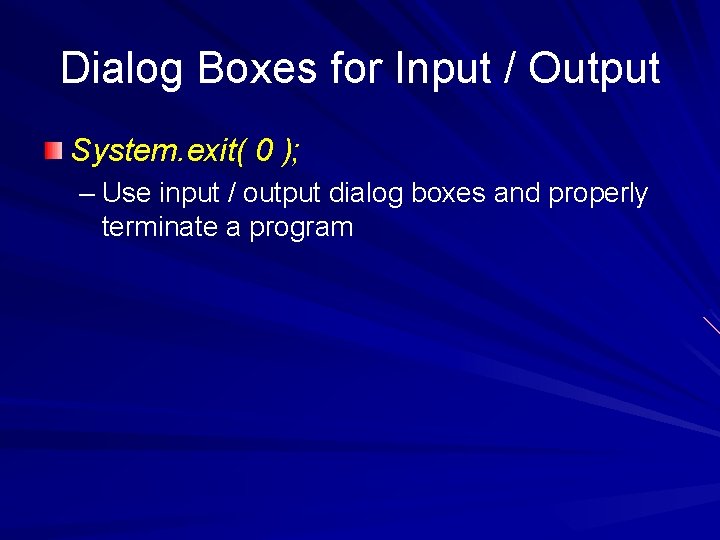
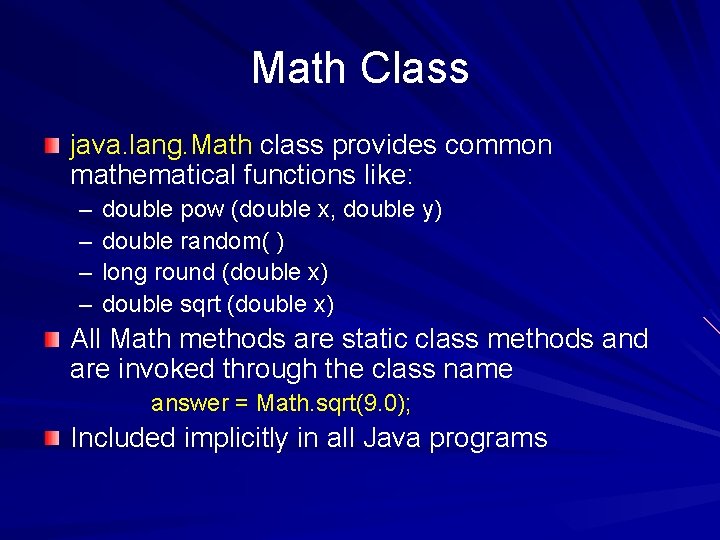
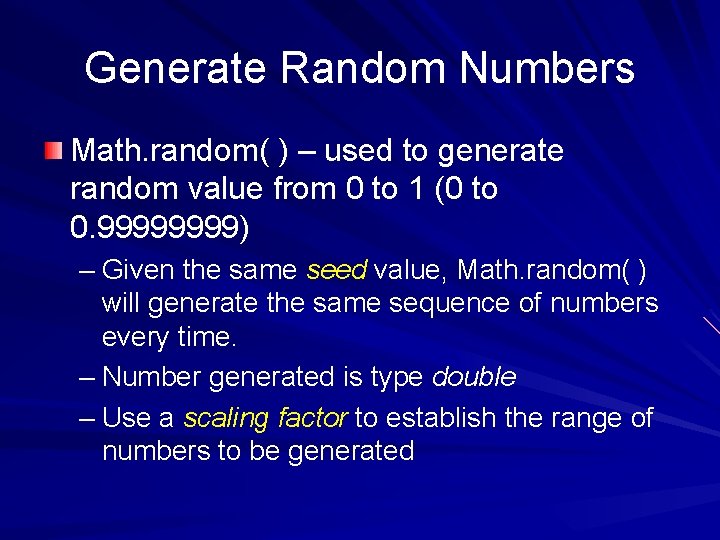
- Slides: 65
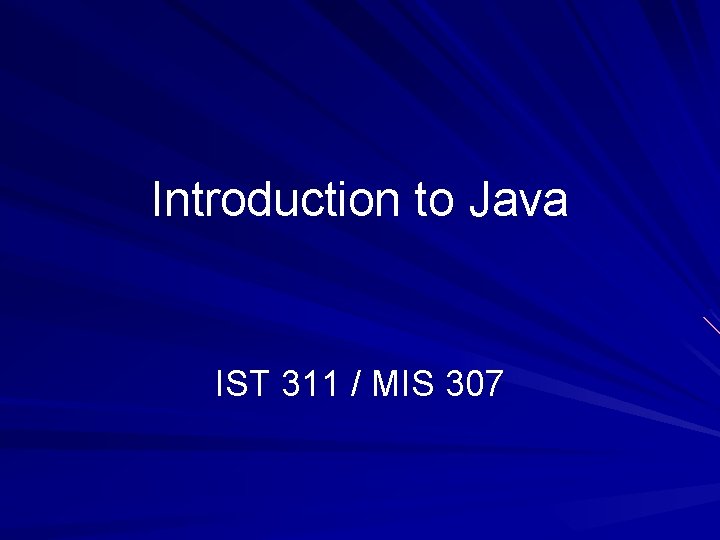
Introduction to Java IST 311 / MIS 307
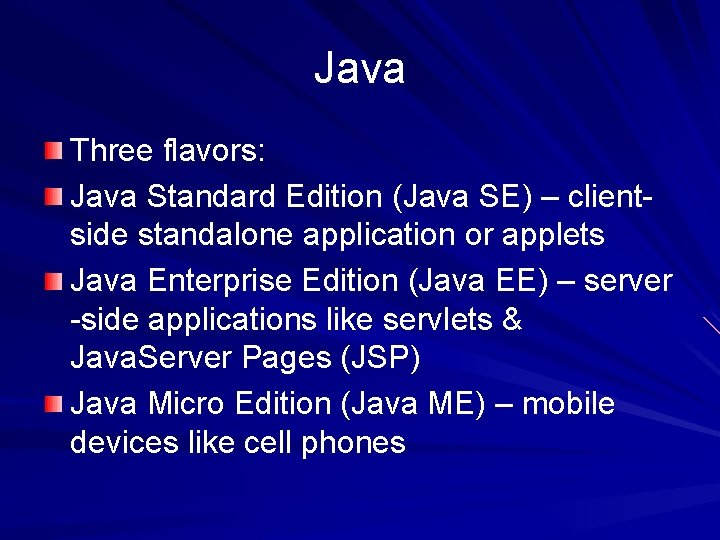
Java Three flavors: Java Standard Edition (Java SE) – clientside standalone application or applets Java Enterprise Edition (Java EE) – server -side applications like servlets & Java. Server Pages (JSP) Java Micro Edition (Java ME) – mobile devices like cell phones
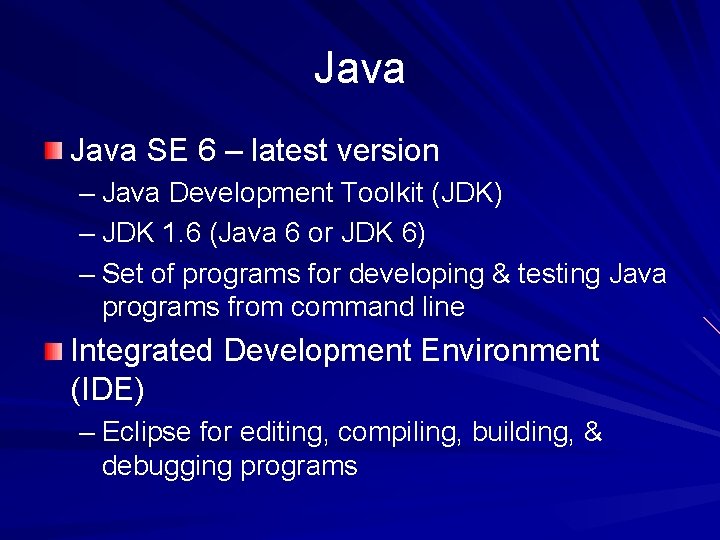
Java SE 6 – latest version – Java Development Toolkit (JDK) – JDK 1. 6 (Java 6 or JDK 6) – Set of programs for developing & testing Java programs from command line Integrated Development Environment (IDE) – Eclipse for editing, compiling, building, & debugging programs
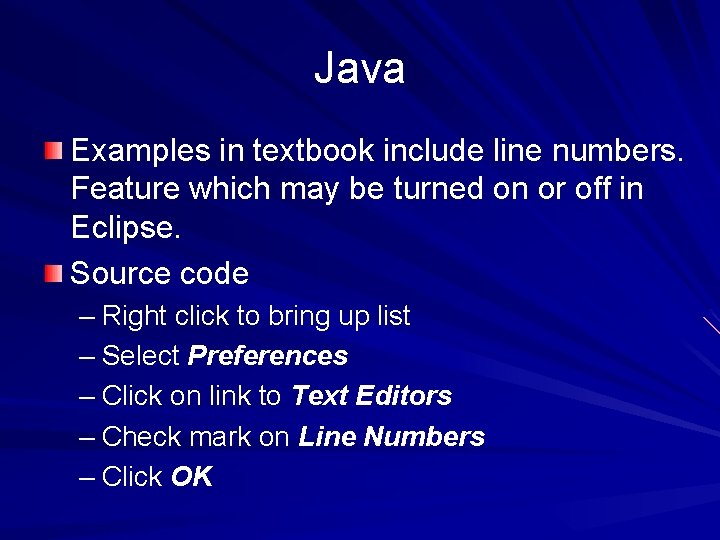
Java Examples in textbook include line numbers. Feature which may be turned on or off in Eclipse. Source code – Right click to bring up list – Select Preferences – Click on link to Text Editors – Check mark on Line Numbers – Click OK
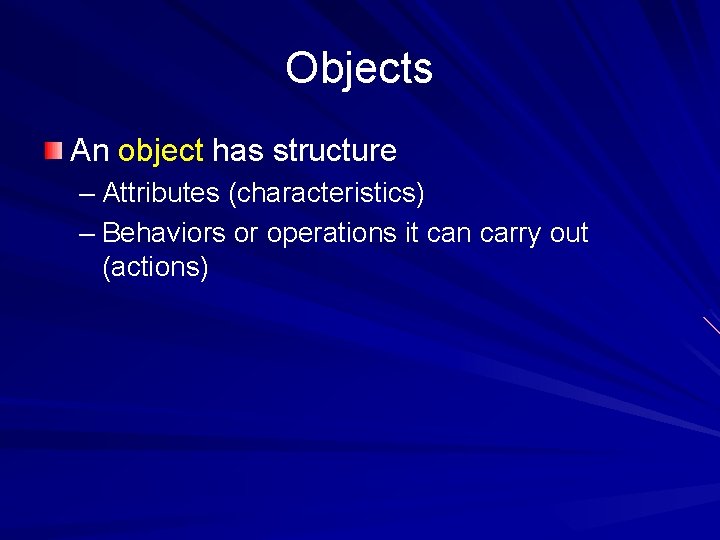
Objects An object has structure – Attributes (characteristics) – Behaviors or operations it can carry out (actions)
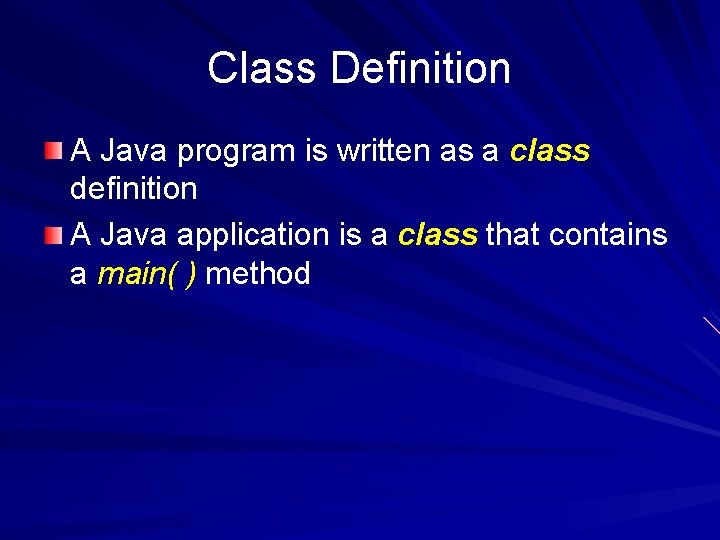
Class Definition A Java program is written as a class definition A Java application is a class that contains a main( ) method
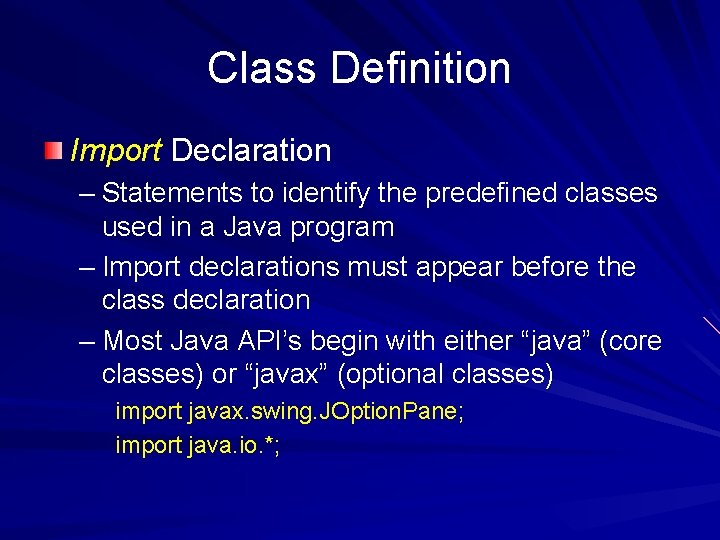
Class Definition Import Declaration – Statements to identify the predefined classes used in a Java program – Import declarations must appear before the class declaration – Most Java API’s begin with either “java” (core classes) or “javax” (optional classes) import javax. swing. JOption. Pane; import java. io. *;
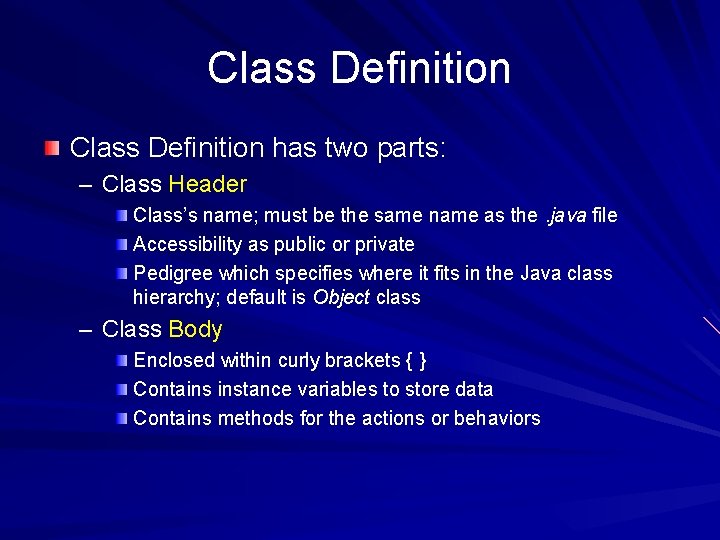
Class Definition has two parts: – Class Header Class’s name; must be the same name as the. java file Accessibility as public or private Pedigree which specifies where it fits in the Java class hierarchy; default is Object class – Class Body Enclosed within curly brackets { } Contains instance variables to store data Contains methods for the actions or behaviors
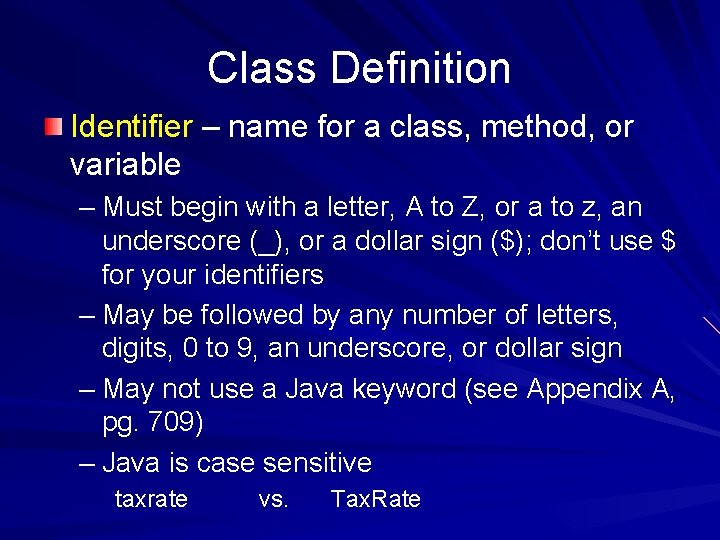
Class Definition Identifier – name for a class, method, or variable – Must begin with a letter, A to Z, or a to z, an underscore (_), or a dollar sign ($); don’t use $ for your identifiers – May be followed by any number of letters, digits, 0 to 9, an underscore, or dollar sign – May not use a Java keyword (see Appendix A, pg. 709) – Java is case sensitive taxrate vs. Tax. Rate
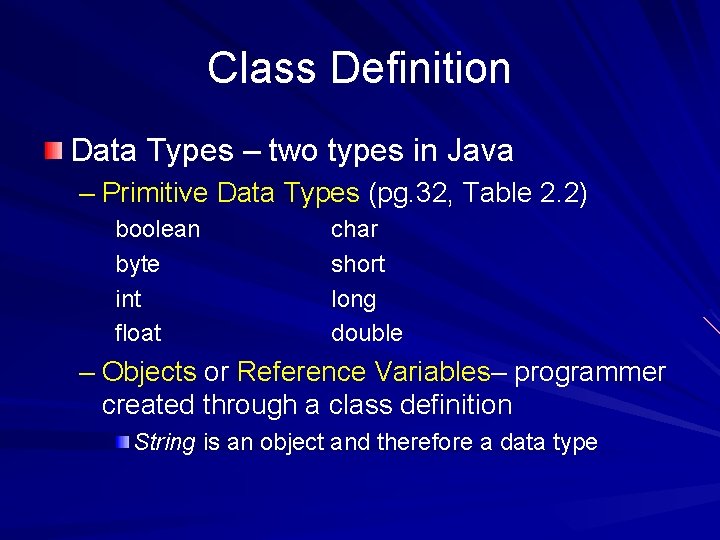
Class Definition Data Types – two types in Java – Primitive Data Types (pg. 32, Table 2. 2) boolean byte int float char short long double – Objects or Reference Variables– programmer created through a class definition String is an object and therefore a data type
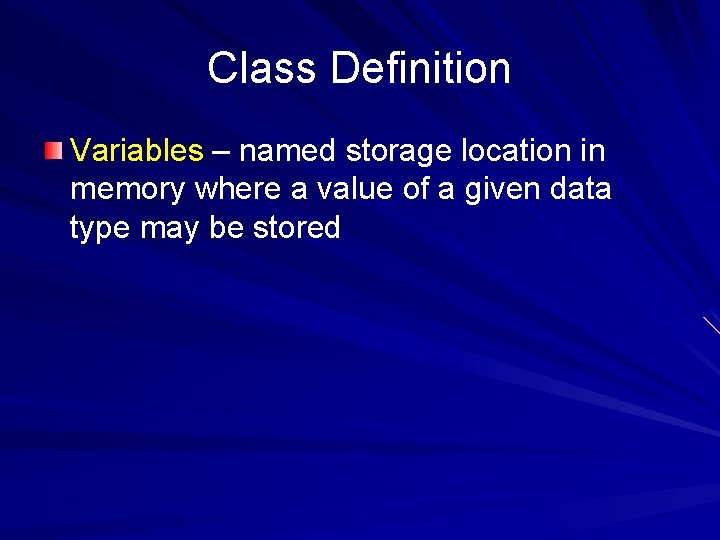
Class Definition Variables – named storage location in memory where a value of a given data type may be stored
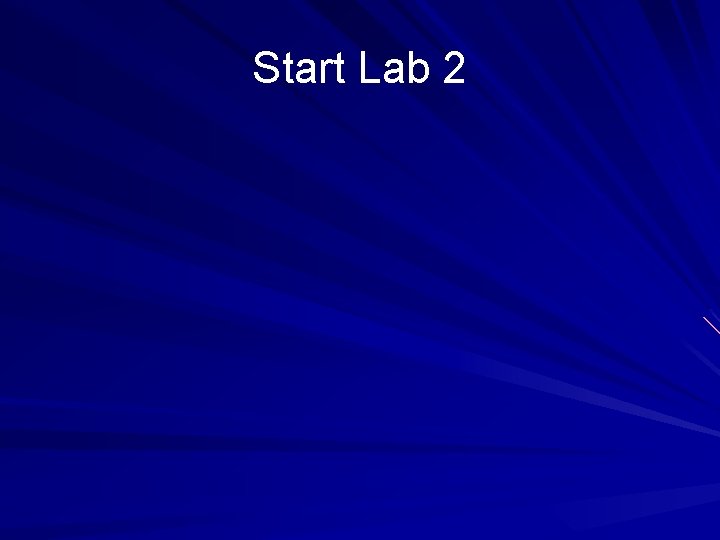
Start Lab 2
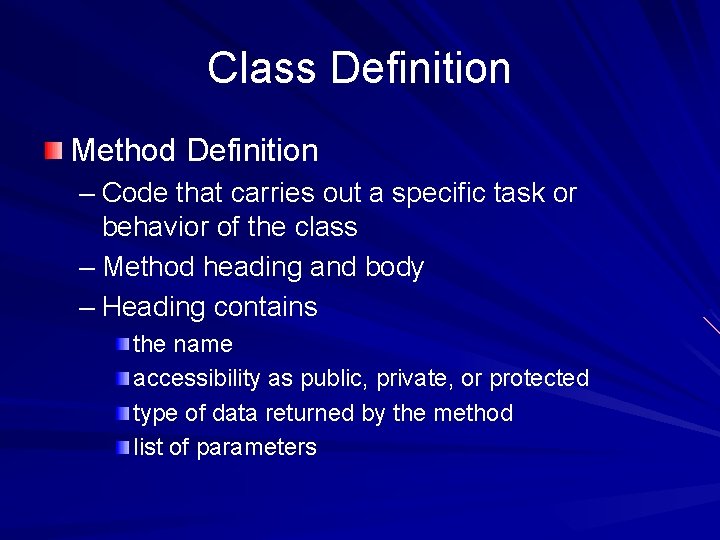
Class Definition Method Definition – Code that carries out a specific task or behavior of the class – Method heading and body – Heading contains the name accessibility as public, private, or protected type of data returned by the method list of parameters
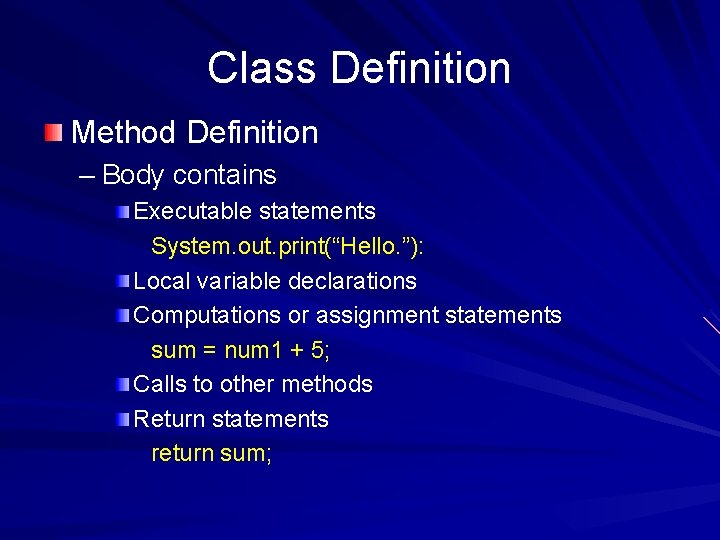
Class Definition Method Definition – Body contains Executable statements System. out. print(“Hello. ”): Local variable declarations Computations or assignment statements sum = num 1 + 5; Calls to other methods Return statements return sum;
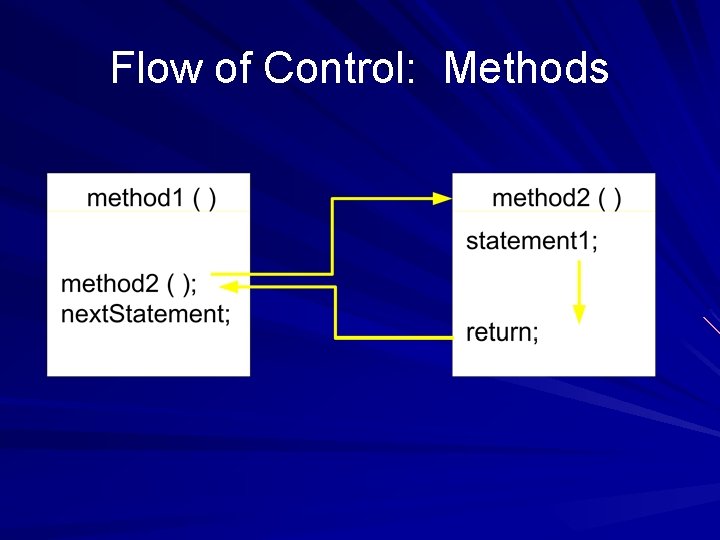
Flow of Control: Methods
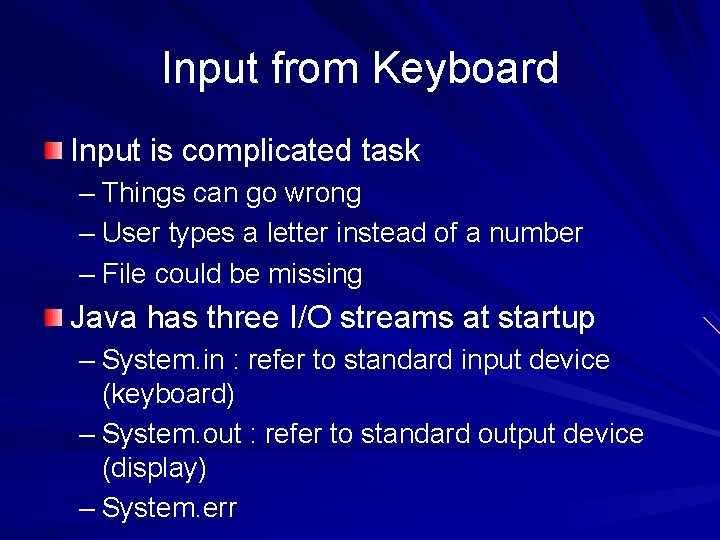
Input from Keyboard Input is complicated task – Things can go wrong – User types a letter instead of a number – File could be missing Java has three I/O streams at startup – System. in : refer to standard input device (keyboard) – System. out : refer to standard output device (display) – System. err
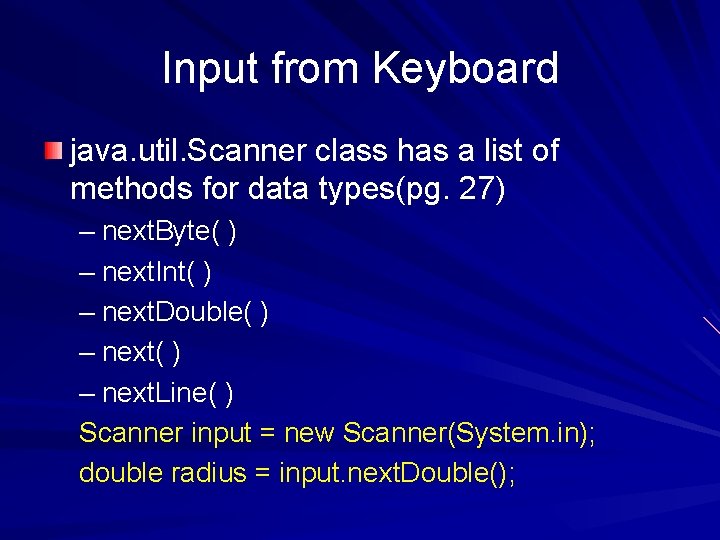
Input from Keyboard java. util. Scanner class has a list of methods for data types(pg. 27) – next. Byte( ) – next. Int( ) – next. Double( ) – next. Line( ) Scanner input = new Scanner(System. in); double radius = input. next. Double();
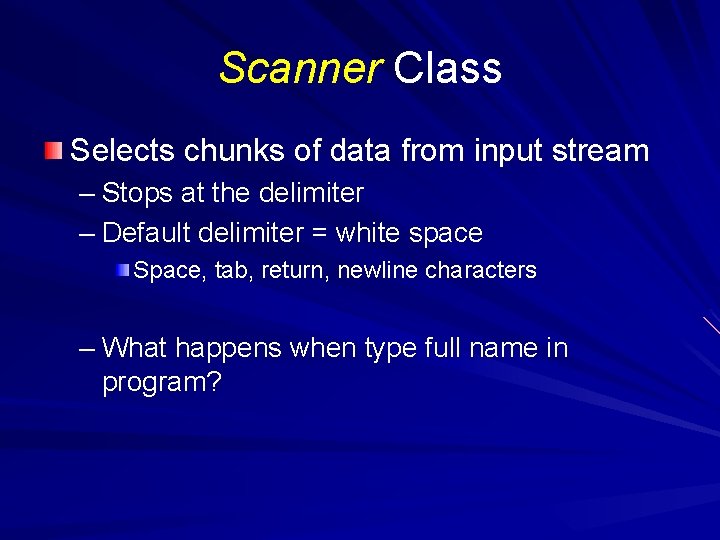
Scanner Class Selects chunks of data from input stream – Stops at the delimiter – Default delimiter = white space Space, tab, return, newline characters – What happens when type full name in program?
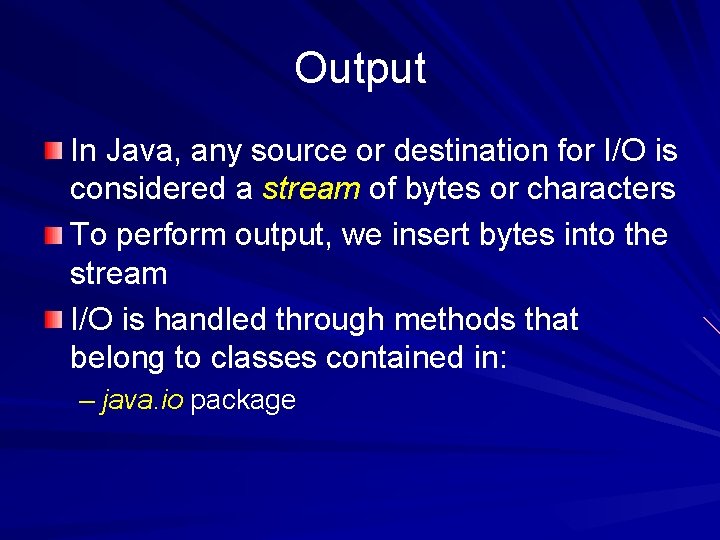
Output In Java, any source or destination for I/O is considered a stream of bytes or characters To perform output, we insert bytes into the stream I/O is handled through methods that belong to classes contained in: – java. io package
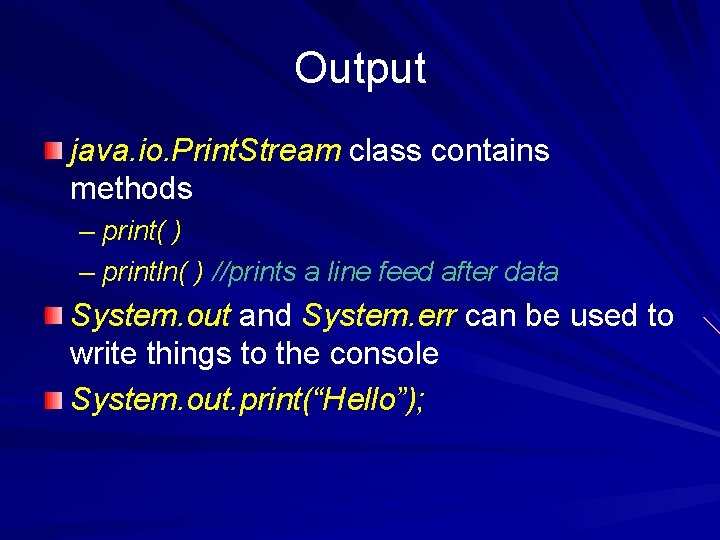
Output java. io. Print. Stream class contains methods – print( ) – println( ) //prints a line feed after data System. out and System. err can be used to write things to the console System. out. print(“Hello”);
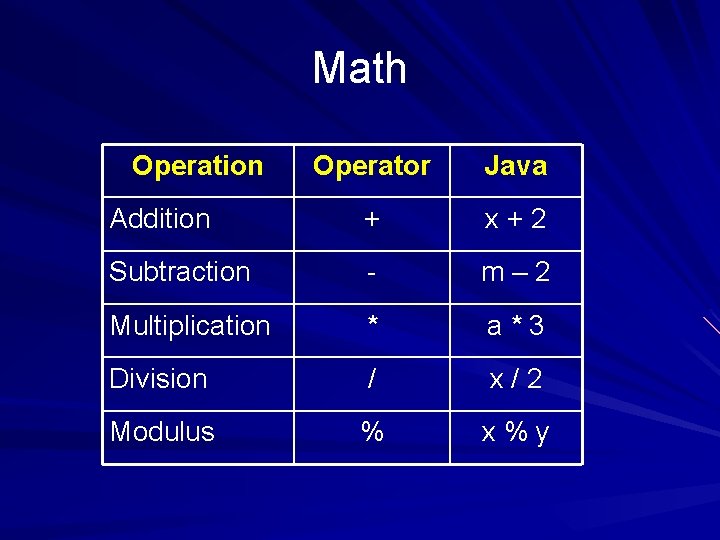
Math Operation Operator Java Addition + x+2 Subtraction - m– 2 Multiplication * a*3 Division / x/2 Modulus % x%y
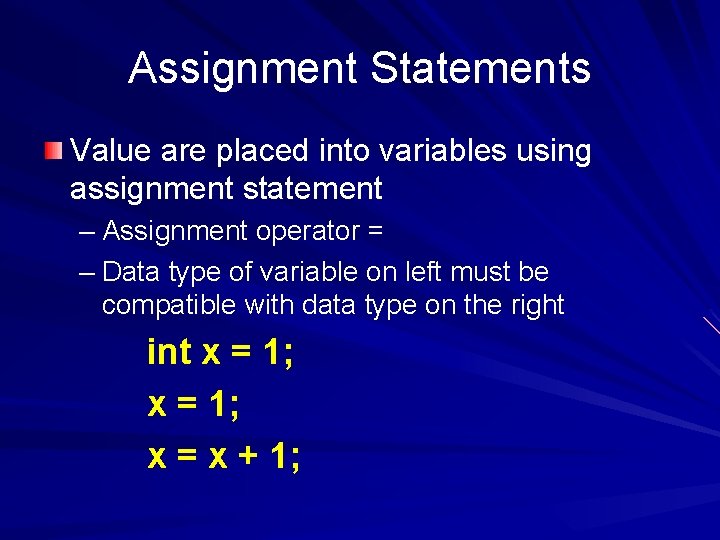
Assignment Statements Value are placed into variables using assignment statement – Assignment operator = – Data type of variable on left must be compatible with data type on the right int x = 1; x = x + 1;
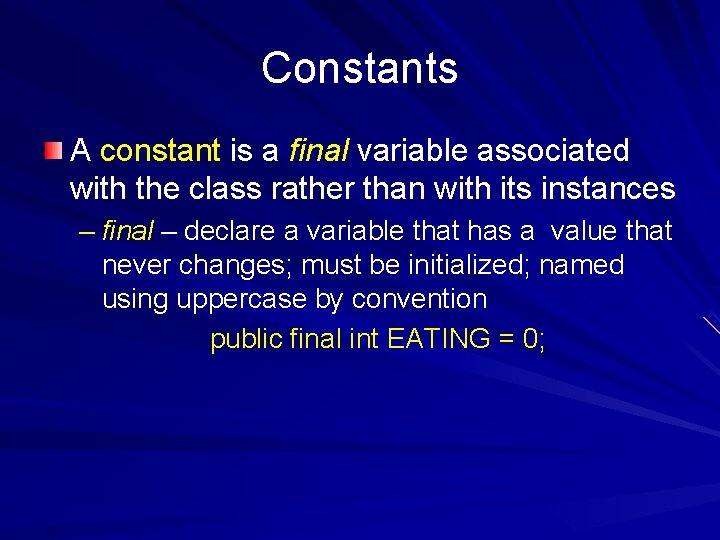
Constants A constant is a final variable associated with the class rather than with its instances – final – declare a variable that has a value that never changes; must be initialized; named using uppercase by convention public final int EATING = 0;
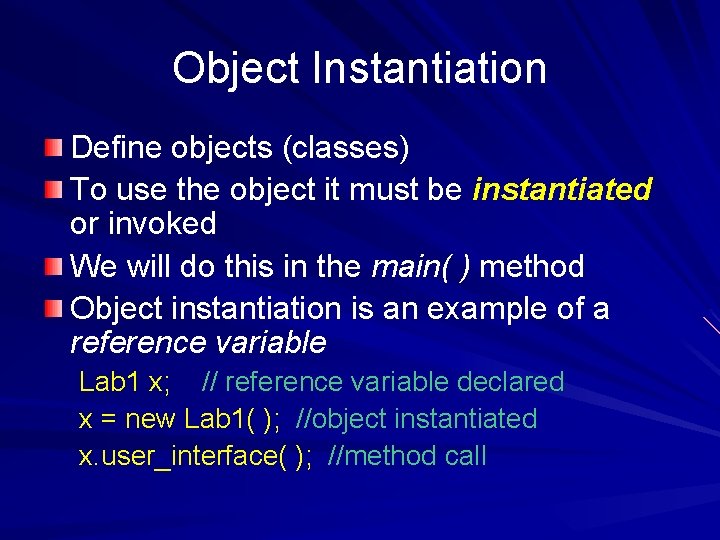
Object Instantiation Define objects (classes) To use the object it must be instantiated or invoked We will do this in the main( ) method Object instantiation is an example of a reference variable Lab 1 x; // reference variable declared x = new Lab 1( ); //object instantiated x. user_interface( ); //method call
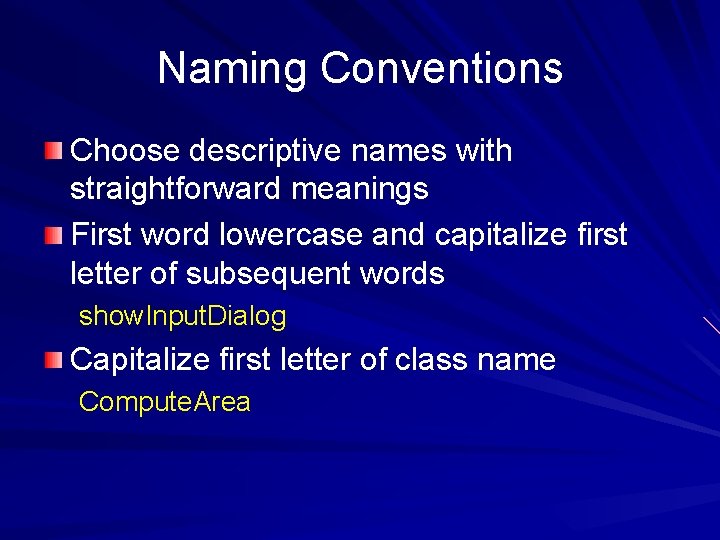
Naming Conventions Choose descriptive names with straightforward meanings First word lowercase and capitalize first letter of subsequent words show. Input. Dialog Capitalize first letter of class name Compute. Area
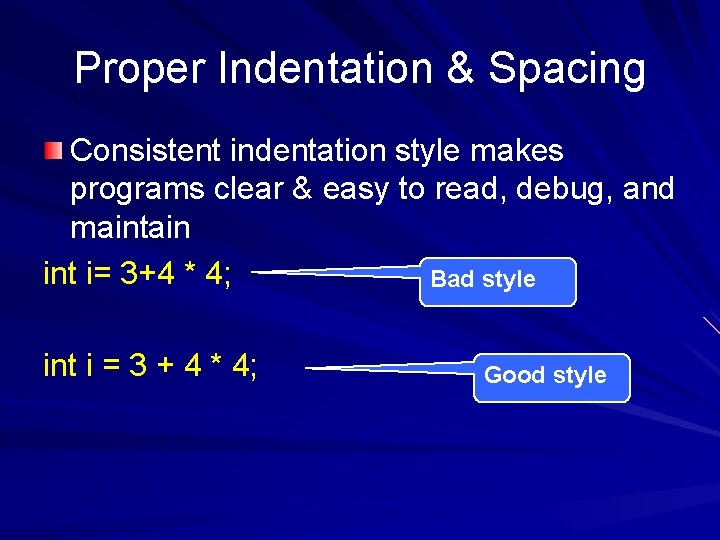
Proper Indentation & Spacing Consistent indentation style makes programs clear & easy to read, debug, and maintain int i= 3+4 * 4; Bad style int i = 3 + 4 * 4; Good style
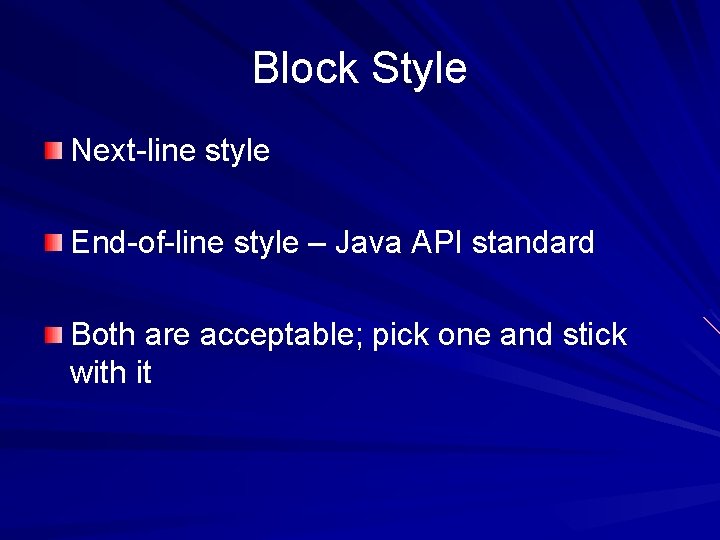
Block Style Next-line style End-of-line style – Java API standard Both are acceptable; pick one and stick with it
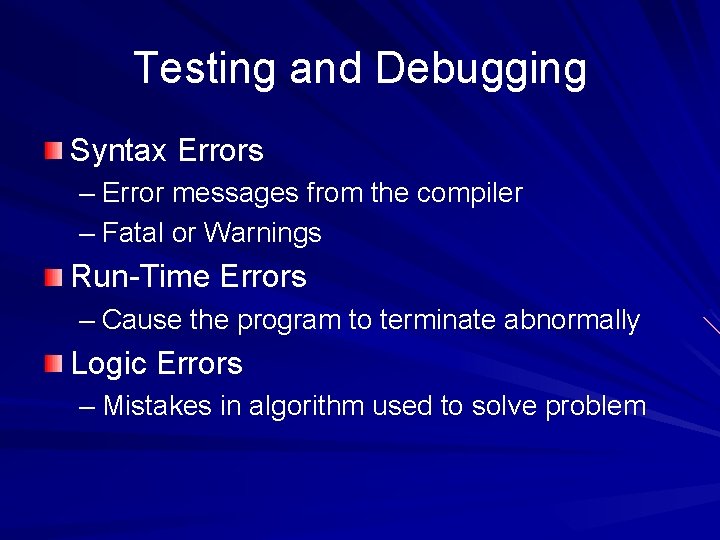
Testing and Debugging Syntax Errors – Error messages from the compiler – Fatal or Warnings Run-Time Errors – Cause the program to terminate abnormally Logic Errors – Mistakes in algorithm used to solve problem
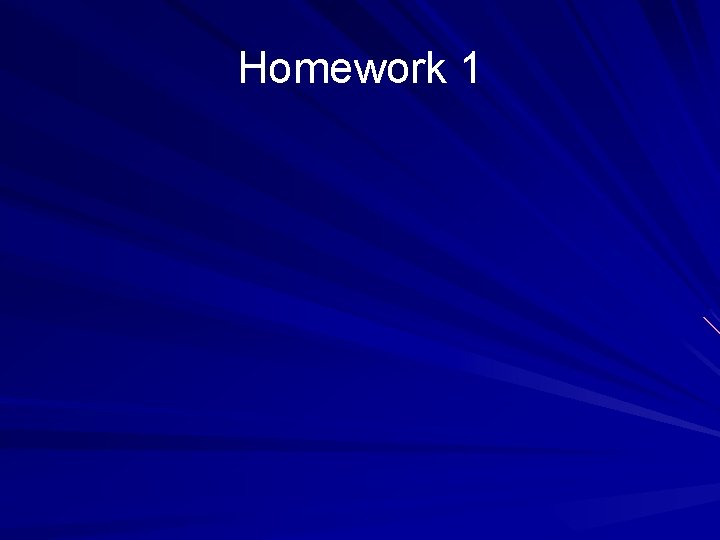
Homework 1
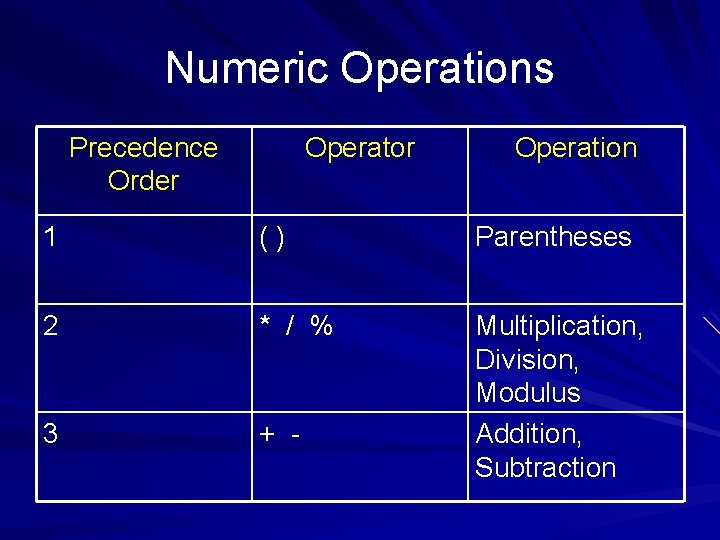
Numeric Operations Precedence Order Operator Operation 1 () Parentheses 2 * / % 3 + - Multiplication, Division, Modulus Addition, Subtraction
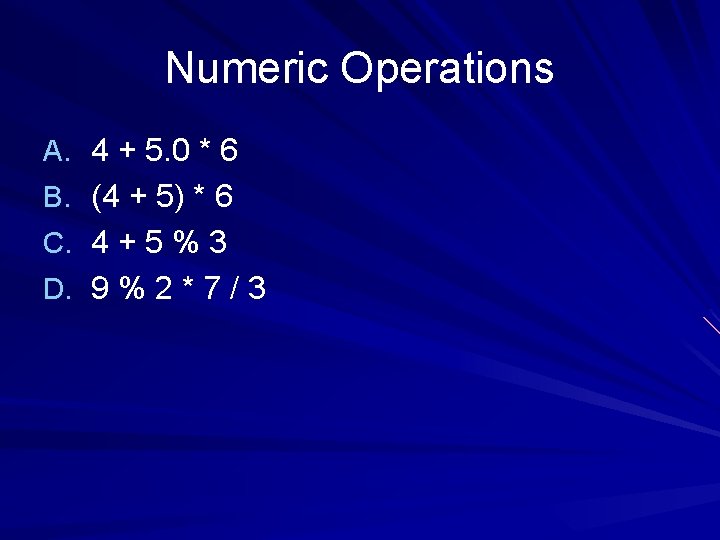
Numeric Operations A. 4 + 5. 0 * 6 B. (4 + 5) * 6 C. 4 + 5 % 3 D. 9 % 2 * 7 / 3
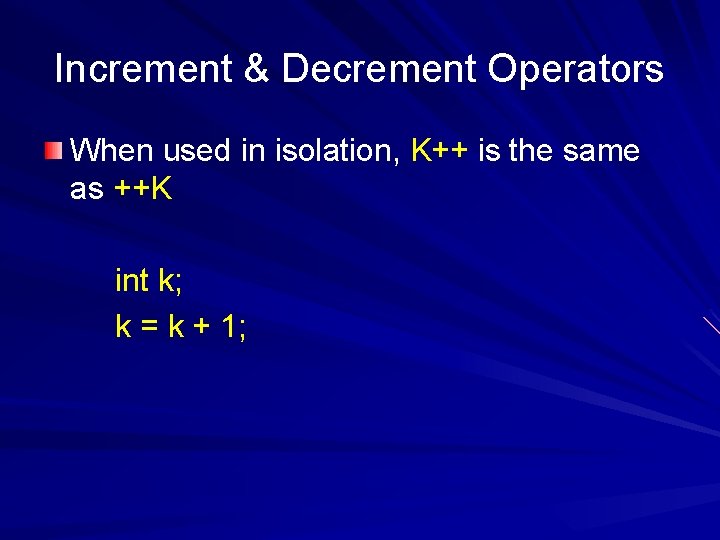
Increment & Decrement Operators When used in isolation, K++ is the same as ++K int k; k = k + 1;
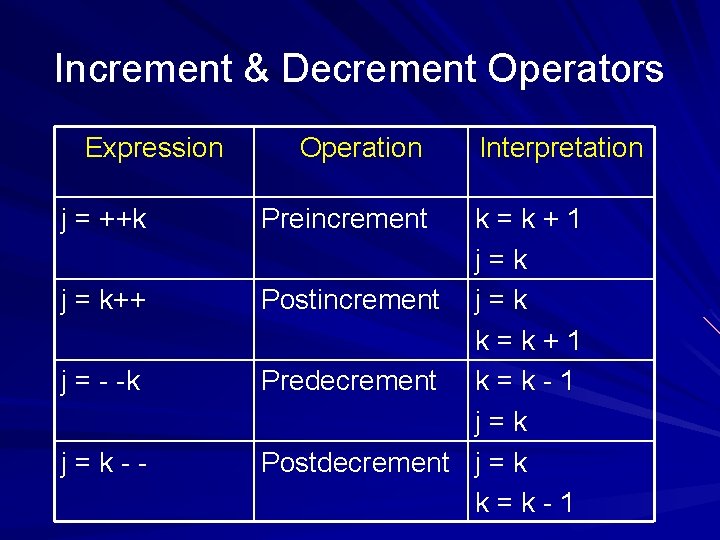
Increment & Decrement Operators Expression j = ++k j = k++ j = - -k j=k-- Operation Preincrement Interpretation k=k+1 j=k Postincrement j = k k=k+1 Predecrement k = k - 1 j=k Postdecrement j = k k=k-1
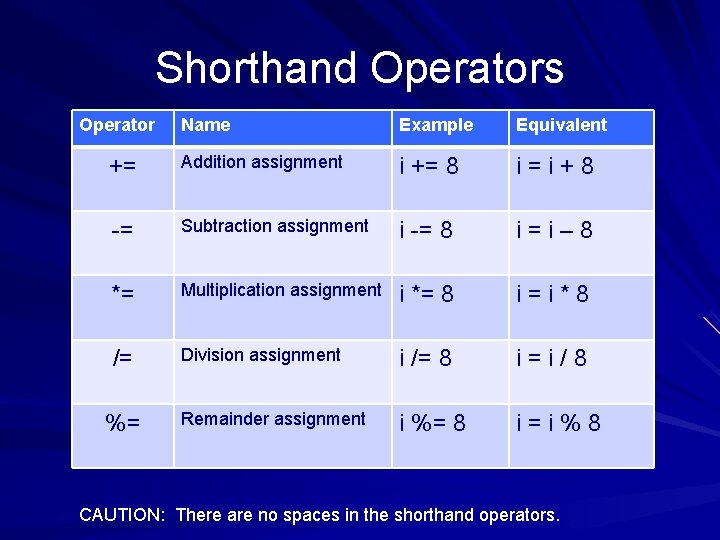
Shorthand Operators Operator Name Example Equivalent += Addition assignment i += 8 i=i+8 -= Subtraction assignment i -= 8 i=i– 8 *= Multiplication assignment i *= 8 i=i*8 /= Division assignment i /= 8 i=i/8 Remainder assignment i %= 8 i=i%8 %= CAUTION: There are no spaces in the shorthand operators.
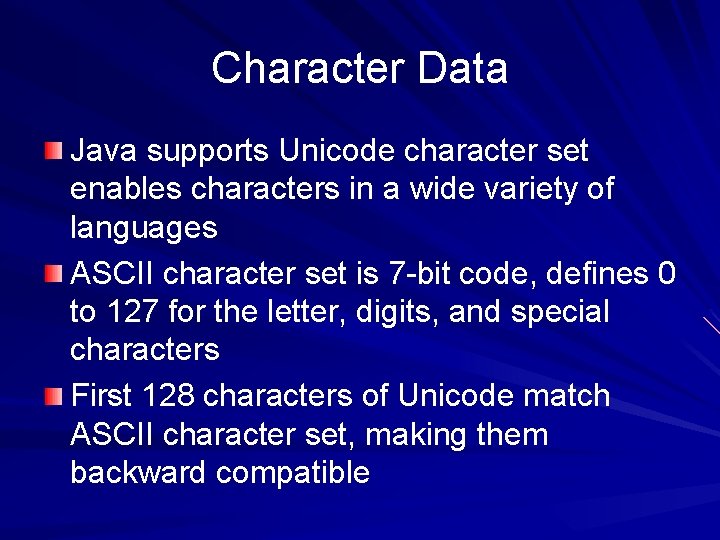
Character Data Java supports Unicode character set enables characters in a wide variety of languages ASCII character set is 7 -bit code, defines 0 to 127 for the letter, digits, and special characters First 128 characters of Unicode match ASCII character set, making them backward compatible
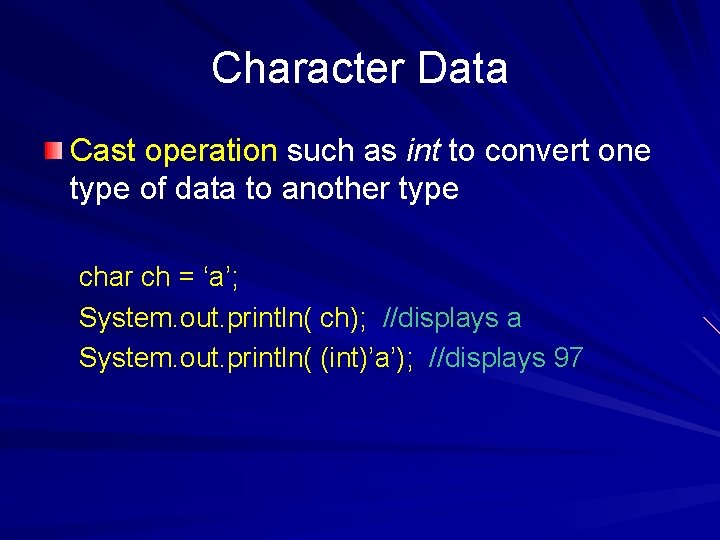
Character Data Cast operation such as int to convert one type of data to another type char ch = ‘a’; System. out. println( ch); //displays a System. out. println( (int)’a’); //displays 97
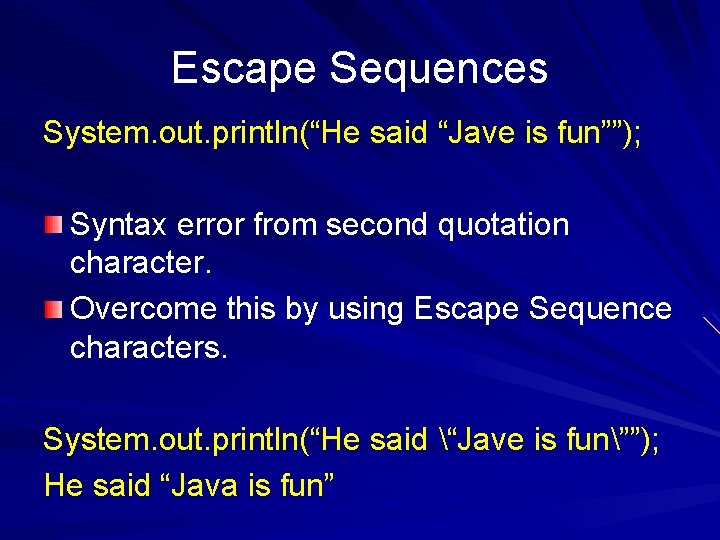
Escape Sequences System. out. println(“He said “Jave is fun””); Syntax error from second quotation character. Overcome this by using Escape Sequence characters. System. out. println(“He said “Jave is fun””); He said “Java is fun”
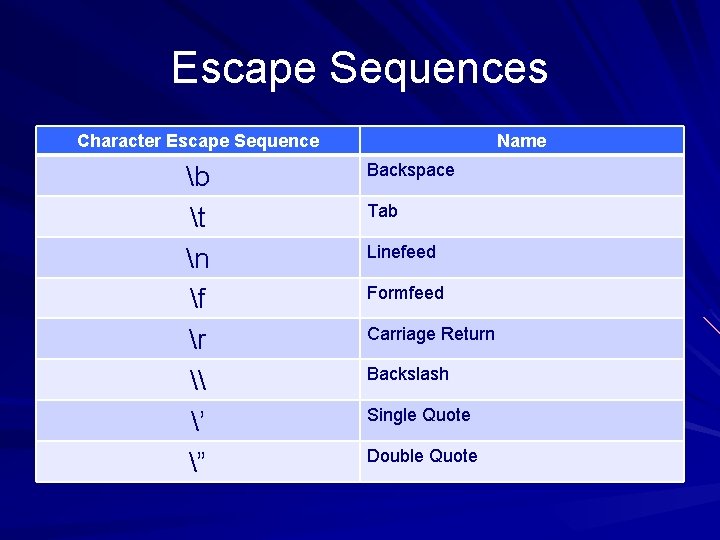
Escape Sequences Character Escape Sequence b t n f r \ ’ ” Name Backspace Tab Linefeed Formfeed Carriage Return Backslash Single Quote Double Quote
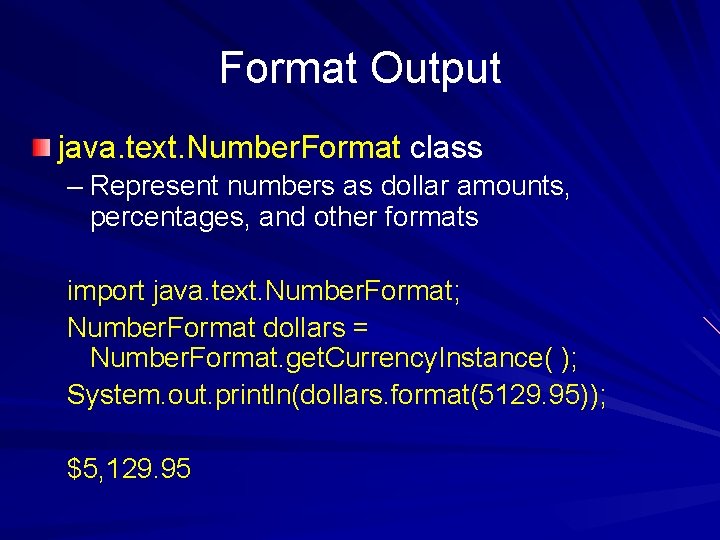
Format Output java. text. Number. Format class – Represent numbers as dollar amounts, percentages, and other formats import java. text. Number. Format; Number. Format dollars = Number. Format. get. Currency. Instance( ); System. out. println(dollars. format(5129. 95)); $5, 129. 95
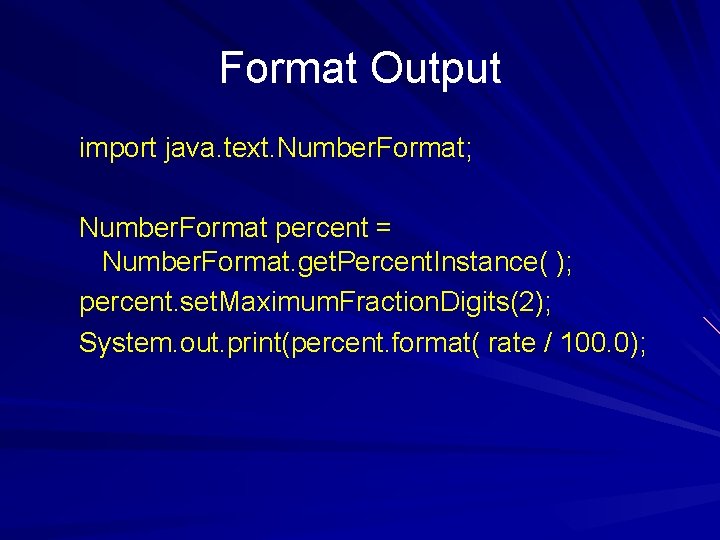
Format Output import java. text. Number. Format; Number. Format percent = Number. Format. get. Percent. Instance( ); percent. set. Maximum. Fraction. Digits(2); System. out. print(percent. format( rate / 100. 0);
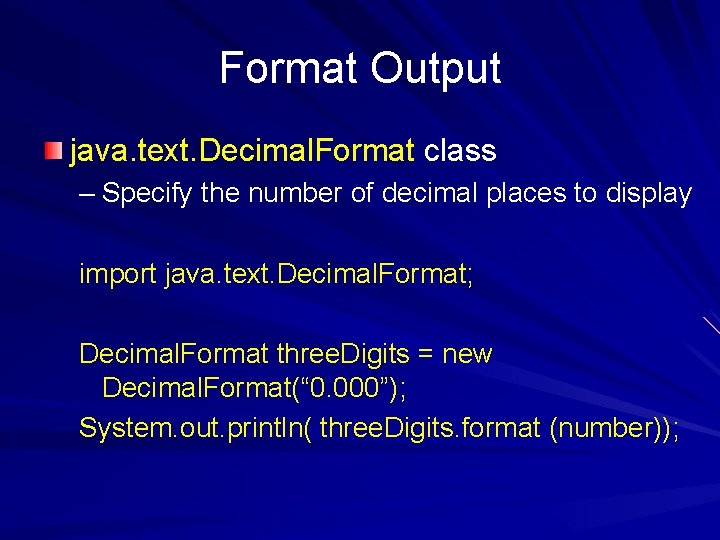
Format Output java. text. Decimal. Format class – Specify the number of decimal places to display import java. text. Decimal. Format; Decimal. Format three. Digits = new Decimal. Format(“ 0. 000”); System. out. println( three. Digits. format (number));
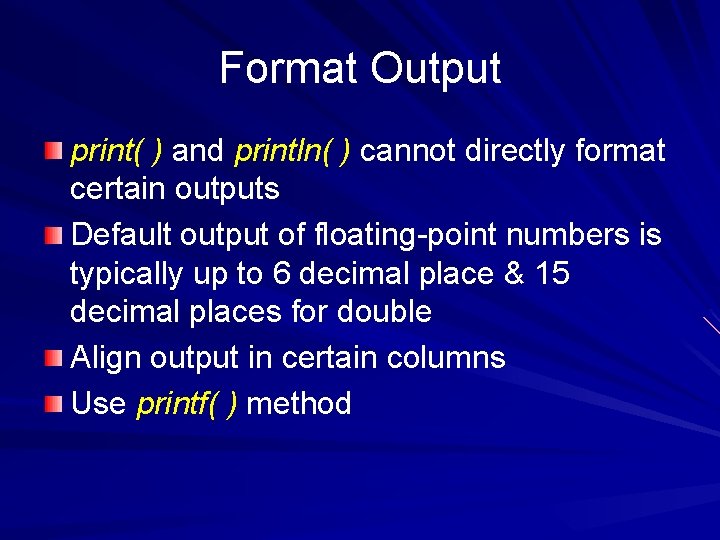
Format Output print( ) and println( ) cannot directly format certain outputs Default output of floating-point numbers is typically up to 6 decimal place & 15 decimal places for double Align output in certain columns Use printf( ) method
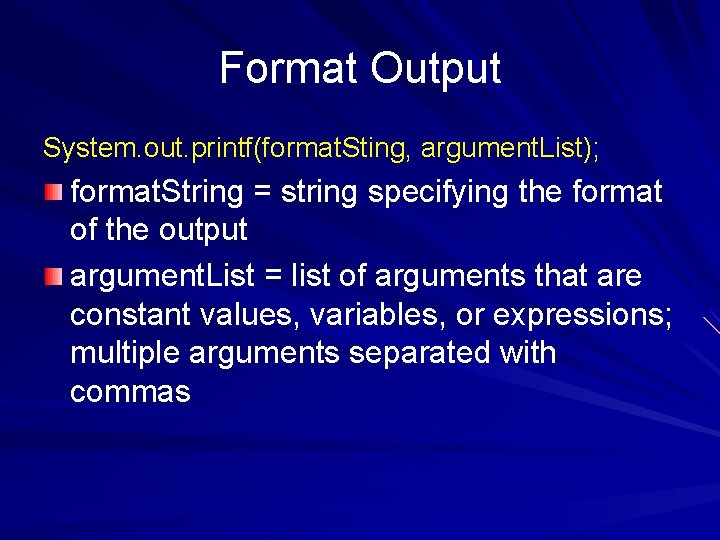
Format Output System. out. printf(format. Sting, argument. List); format. String = string specifying the format of the output argument. List = list of arguments that are constant values, variables, or expressions; multiple arguments separated with commas
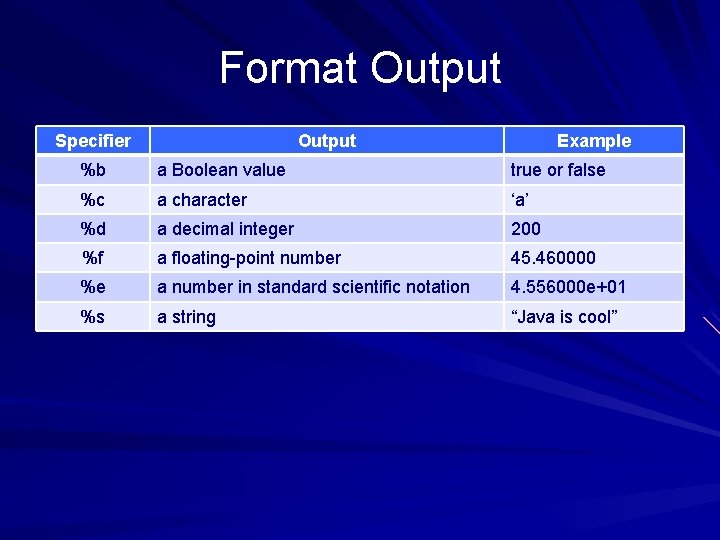
Format Output Specifier Output Example %b a Boolean value true or false %c a character ‘a’ %d a decimal integer 200 %f a floating-point number 45. 460000 %e a number in standard scientific notation 4. 556000 e+01 %s a string “Java is cool”
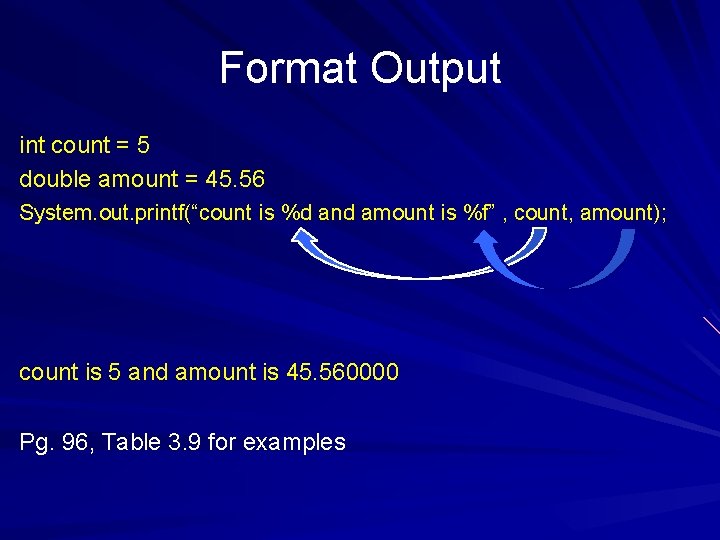
Format Output int count = 5 double amount = 45. 56 System. out. printf(“count is %d and amount is %f” , count, amount); count is 5 and amount is 45. 560000 Pg. 96, Table 3. 9 for examples
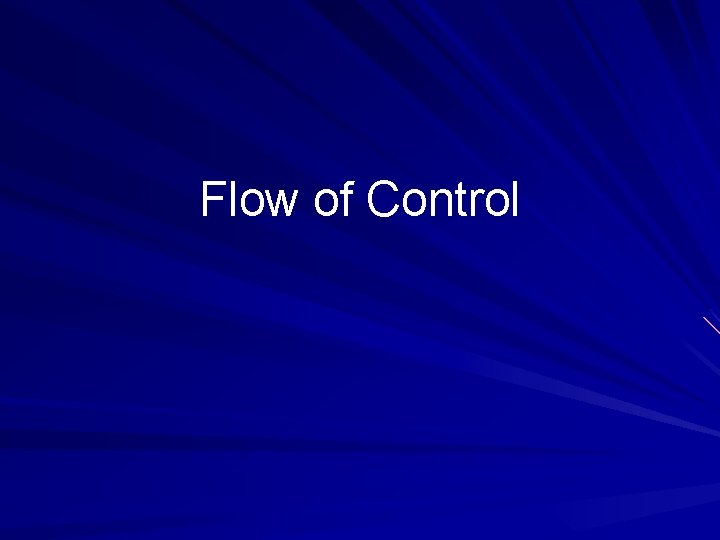
Flow of Control
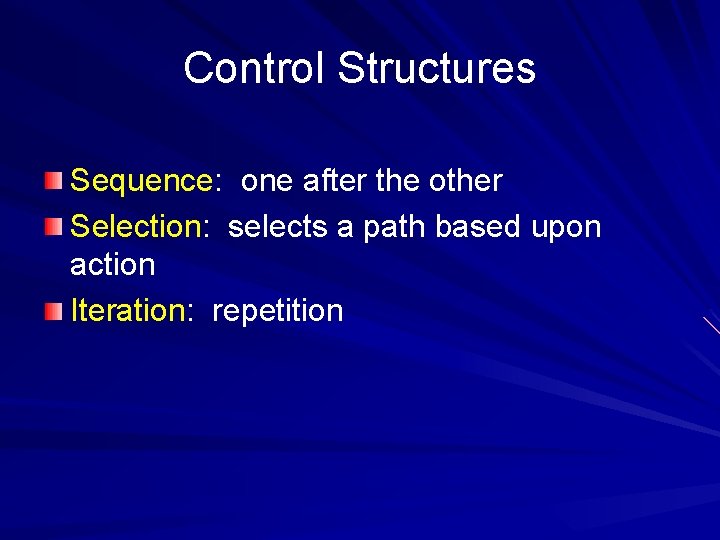
Control Structures Sequence: one after the other Selection: selects a path based upon action Iteration: repetition
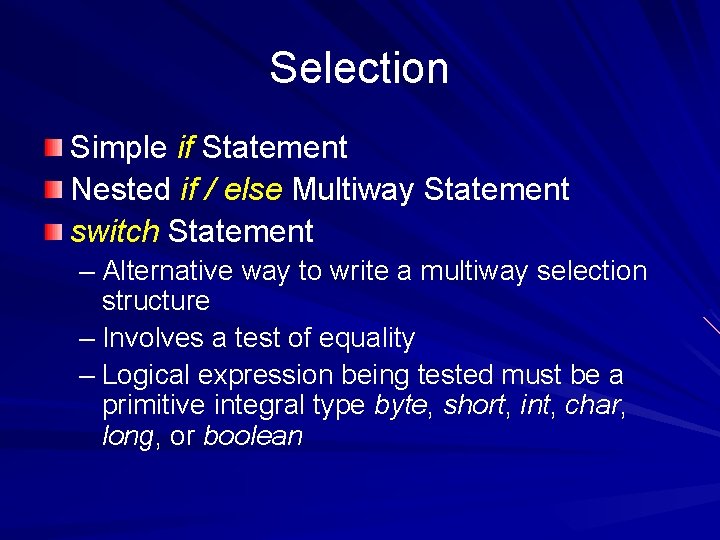
Selection Simple if Statement Nested if / else Multiway Statement switch Statement – Alternative way to write a multiway selection structure – Involves a test of equality – Logical expression being tested must be a primitive integral type byte, short, int, char, long, or boolean
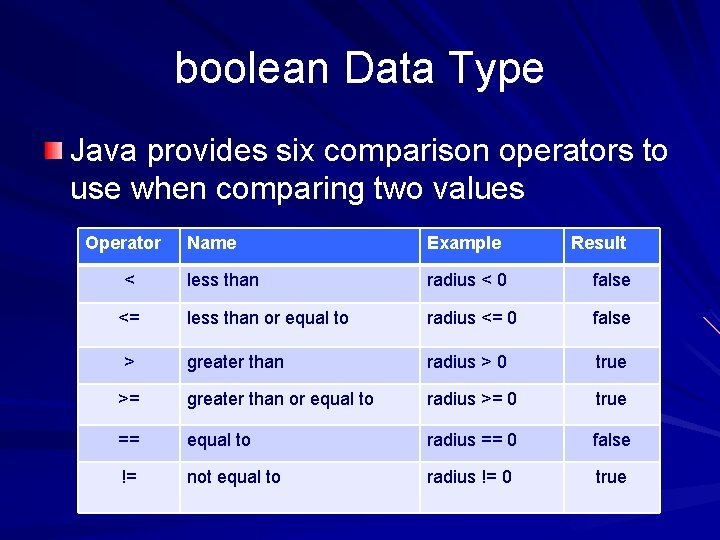
boolean Data Type Java provides six comparison operators to use when comparing two values Operator Name Example Result < less than radius < 0 false <= less than or equal to radius <= 0 false > greater than radius > 0 true >= greater than or equal to radius >= 0 true == equal to radius == 0 false != not equal to radius != 0 true
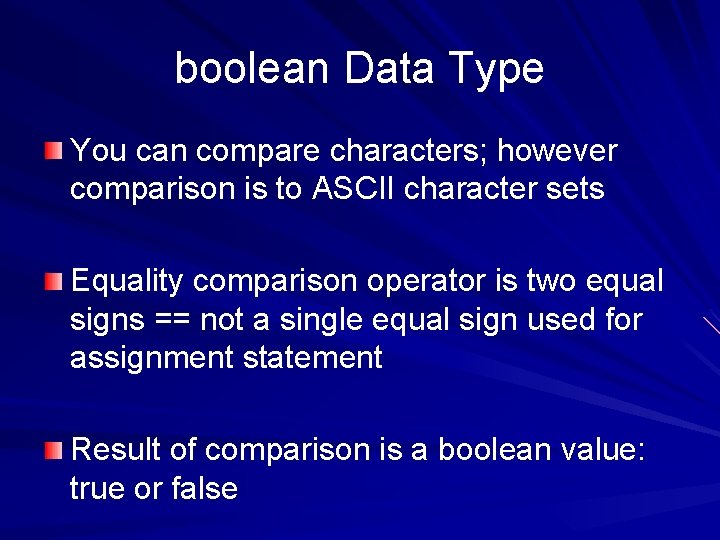
boolean Data Type You can compare characters; however comparison is to ASCII character sets Equality comparison operator is two equal signs == not a single equal sign used for assignment statement Result of comparison is a boolean value: true or false
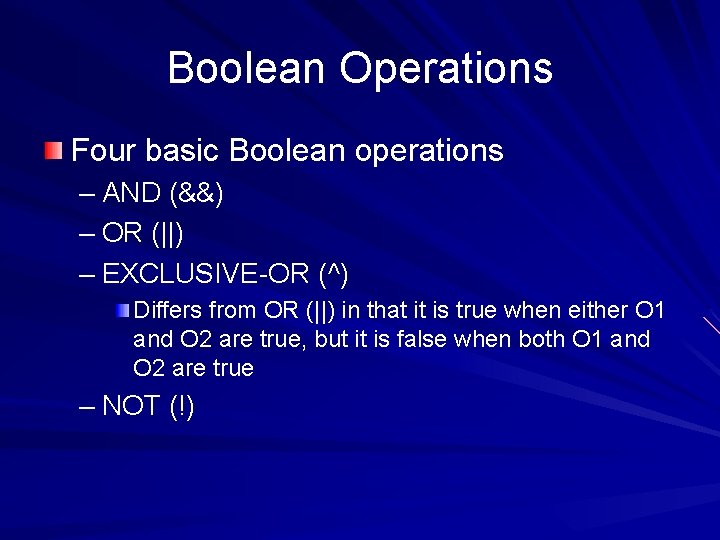
Boolean Operations Four basic Boolean operations – AND (&&) – OR (||) – EXCLUSIVE-OR (^) Differs from OR (||) in that it is true when either O 1 and O 2 are true, but it is false when both O 1 and O 2 are true – NOT (!)
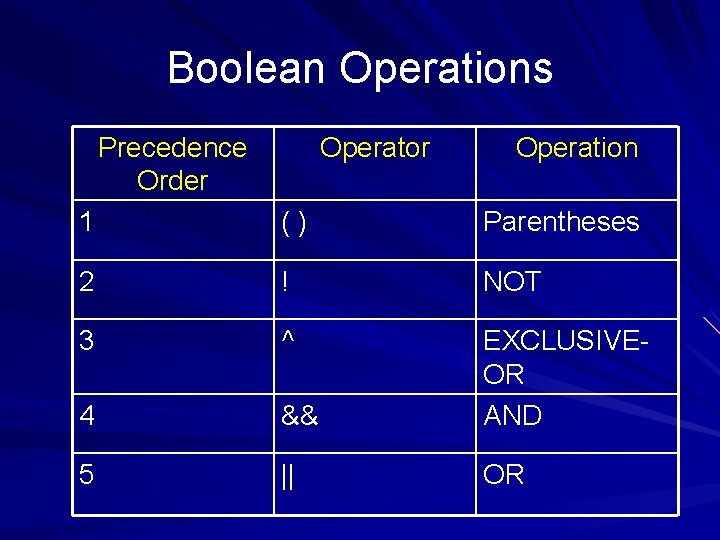
Boolean Operations Precedence Order Operator Operation 1 () Parentheses 2 ! NOT 3 ^ 4 && EXCLUSIVEOR AND 5 || OR
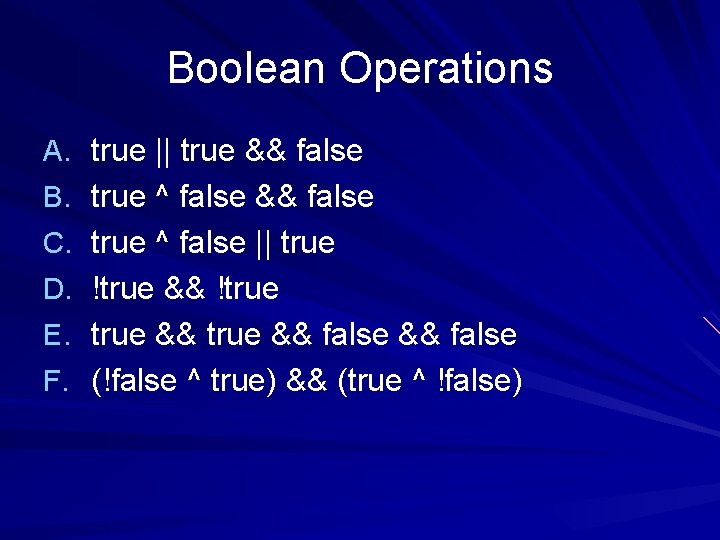
Boolean Operations A. true || true && false B. true ^ false && false C. true ^ false || true D. !true && !true E. true && false F. (!false ^ true) && (true ^ !false)
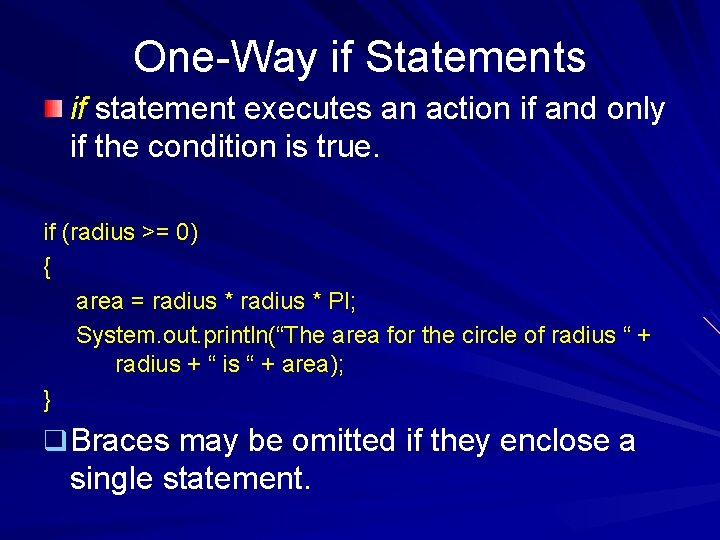
One-Way if Statements if statement executes an action if and only if the condition is true. if (radius >= 0) { area = radius * PI; System. out. println(“The area for the circle of radius “ + radius + “ is “ + area); } q Braces may be omitted if they enclose a single statement.
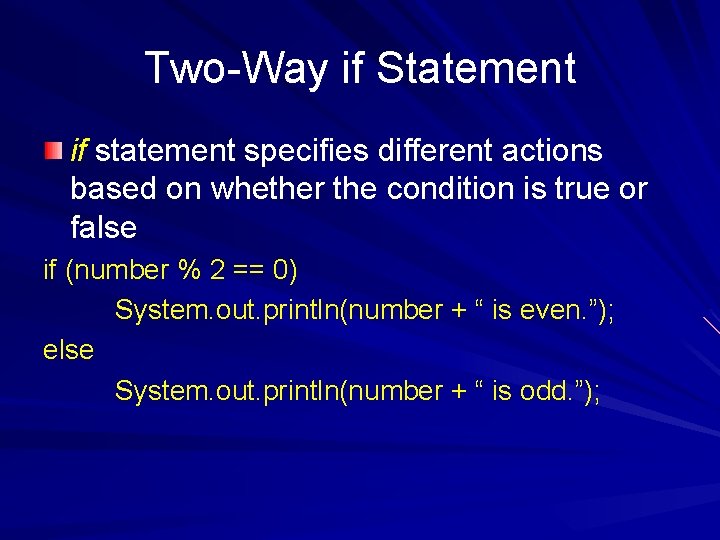
Two-Way if Statement if statement specifies different actions based on whether the condition is true or false if (number % 2 == 0) System. out. println(number + “ is even. ”); else System. out. println(number + “ is odd. ”);
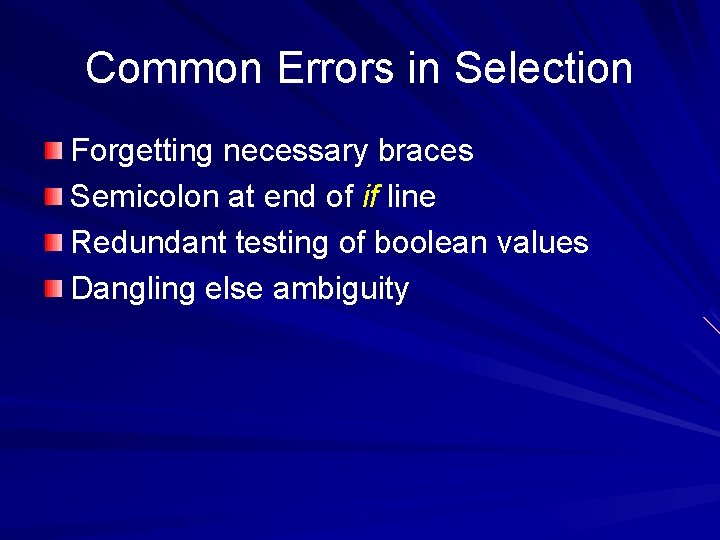
Common Errors in Selection Forgetting necessary braces Semicolon at end of if line Redundant testing of boolean values Dangling else ambiguity
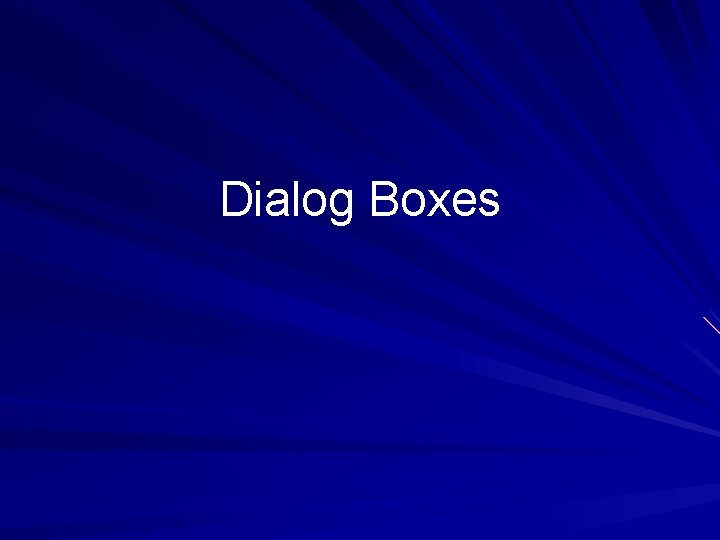
Dialog Boxes
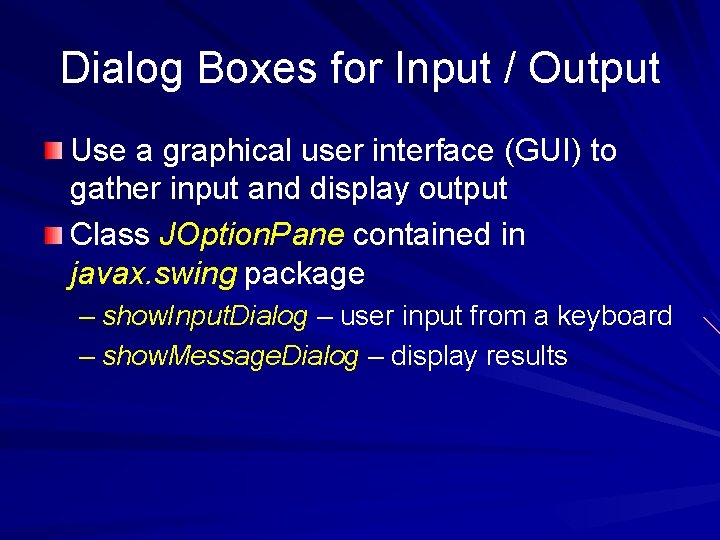
Dialog Boxes for Input / Output Use a graphical user interface (GUI) to gather input and display output Class JOption. Pane contained in javax. swing package – show. Input. Dialog – user input from a keyboard – show. Message. Dialog – display results
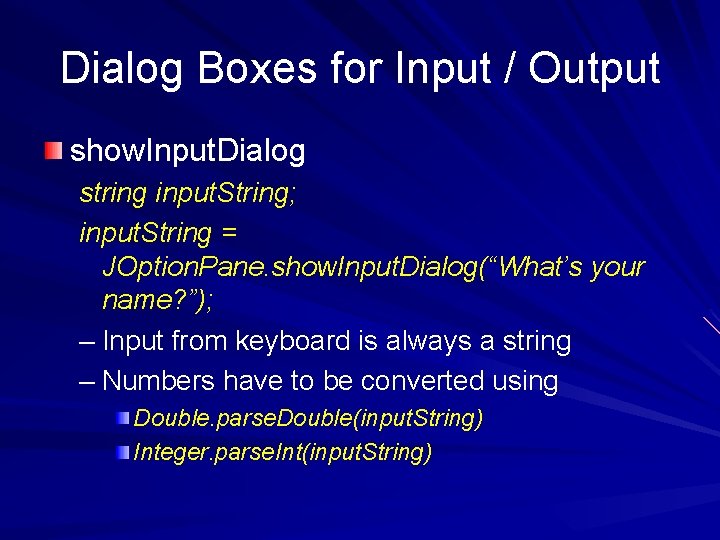
Dialog Boxes for Input / Output show. Input. Dialog string input. String; input. String = JOption. Pane. show. Input. Dialog(“What’s your name? ”); – Input from keyboard is always a string – Numbers have to be converted using Double. parse. Double(input. String) Integer. parse. Int(input. String)
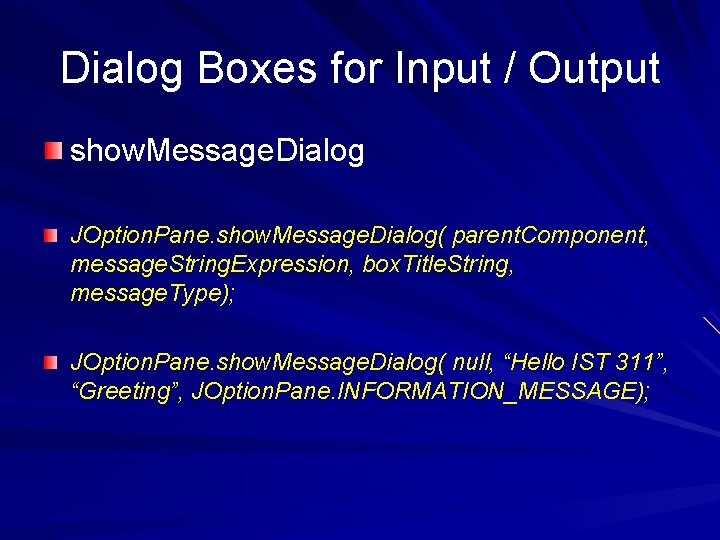
Dialog Boxes for Input / Output show. Message. Dialog JOption. Pane. show. Message. Dialog( parent. Component, message. String. Expression, box. Title. String, message. Type); JOption. Pane. show. Message. Dialog( null, “Hello IST 311”, “Greeting”, JOption. Pane. INFORMATION_MESSAGE);
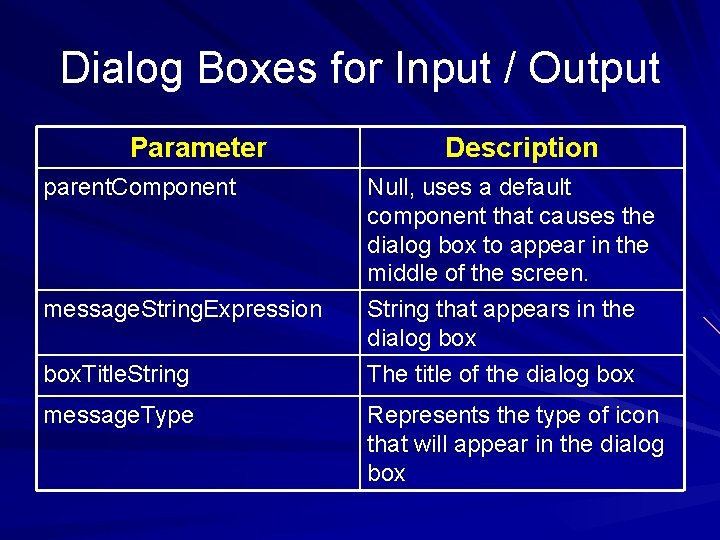
Dialog Boxes for Input / Output Parameter parent. Component message. String. Expression box. Title. String message. Type Description Null, uses a default component that causes the dialog box to appear in the middle of the screen. String that appears in the dialog box The title of the dialog box Represents the type of icon that will appear in the dialog box
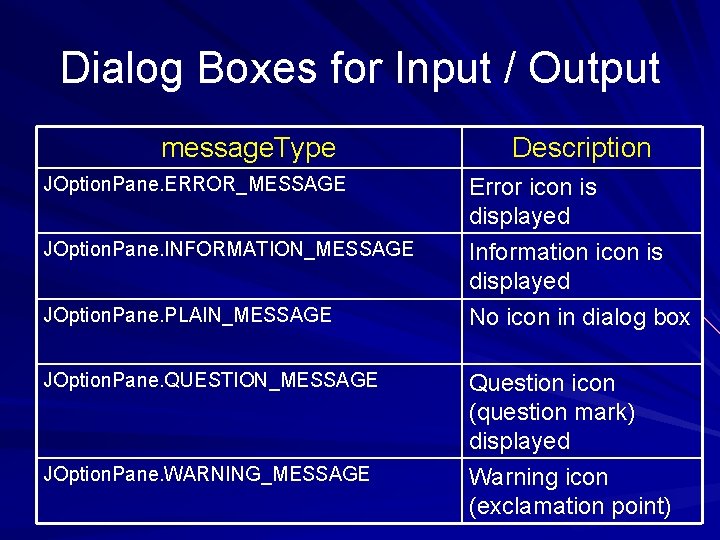
Dialog Boxes for Input / Output message. Type JOption. Pane. ERROR_MESSAGE JOption. Pane. INFORMATION_MESSAGE JOption. Pane. PLAIN_MESSAGE JOption. Pane. QUESTION_MESSAGE JOption. Pane. WARNING_MESSAGE Description Error icon is displayed Information icon is displayed No icon in dialog box Question icon (question mark) displayed Warning icon (exclamation point)
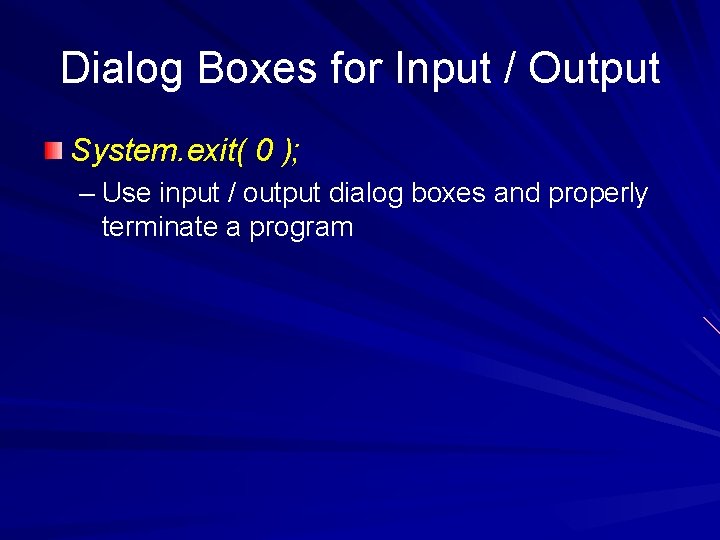
Dialog Boxes for Input / Output System. exit( 0 ); – Use input / output dialog boxes and properly terminate a program
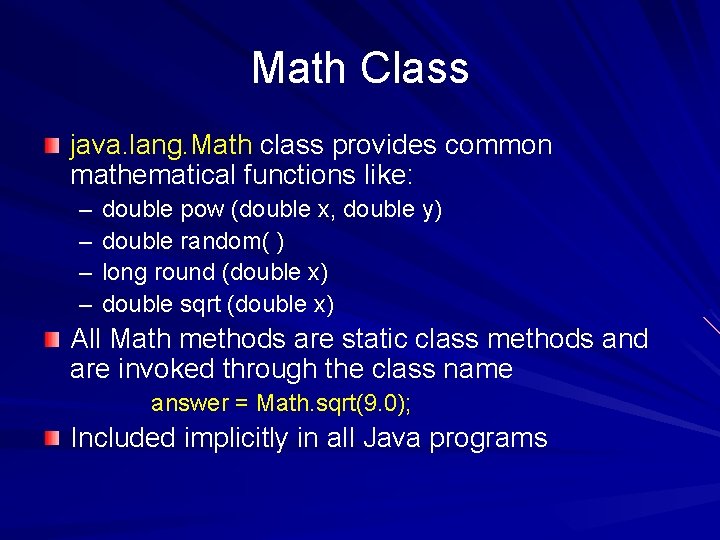
Math Class java. lang. Math class provides common mathematical functions like: – – double pow (double x, double y) double random( ) long round (double x) double sqrt (double x) All Math methods are static class methods and are invoked through the class name answer = Math. sqrt(9. 0); Included implicitly in all Java programs
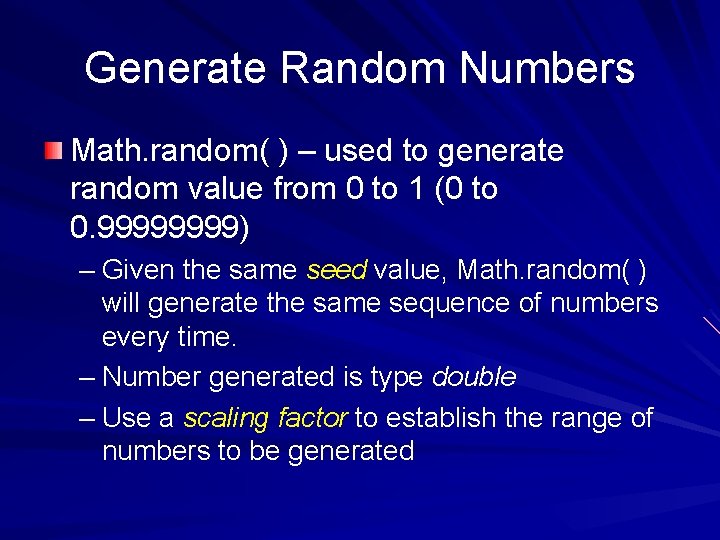
Generate Random Numbers Math. random( ) – used to generate random value from 0 to 1 (0 to 0. 9999) – Given the same seed value, Math. random( ) will generate the same sequence of numbers every time. – Number generated is type double – Use a scaling factor to establish the range of numbers to be generated