Introduction to JAVA CMSC 331 Spring 1999 Introduction
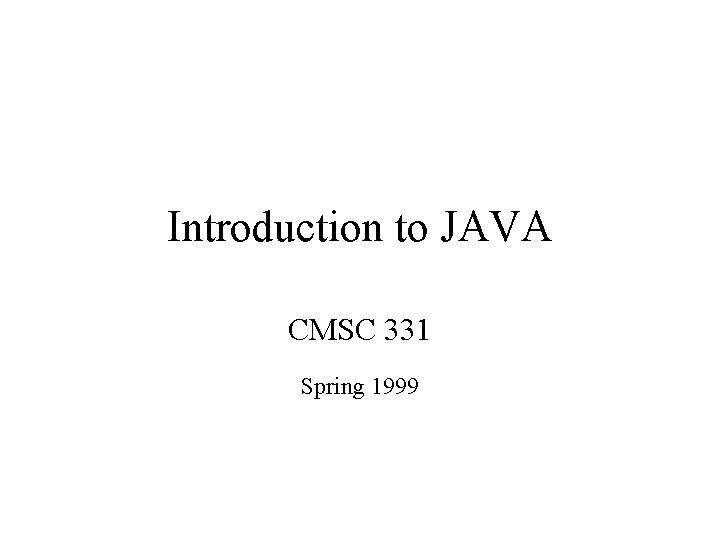
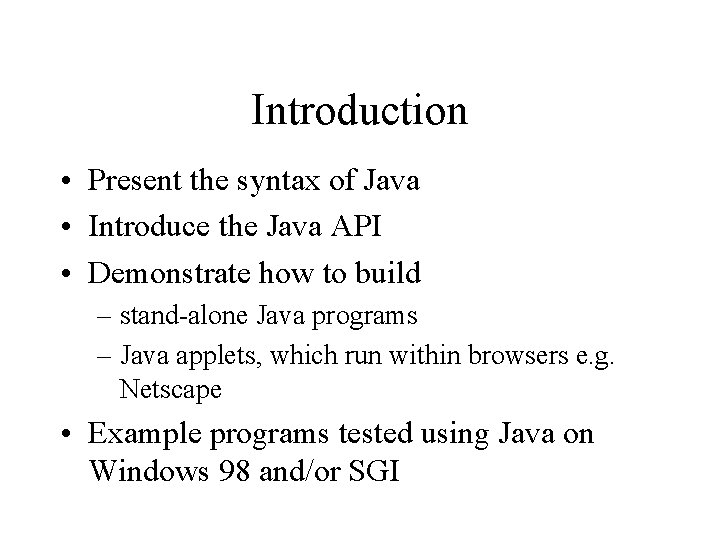
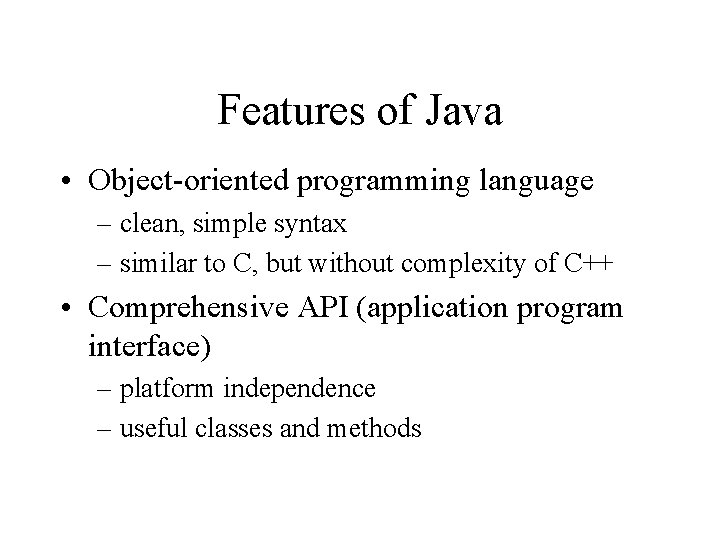
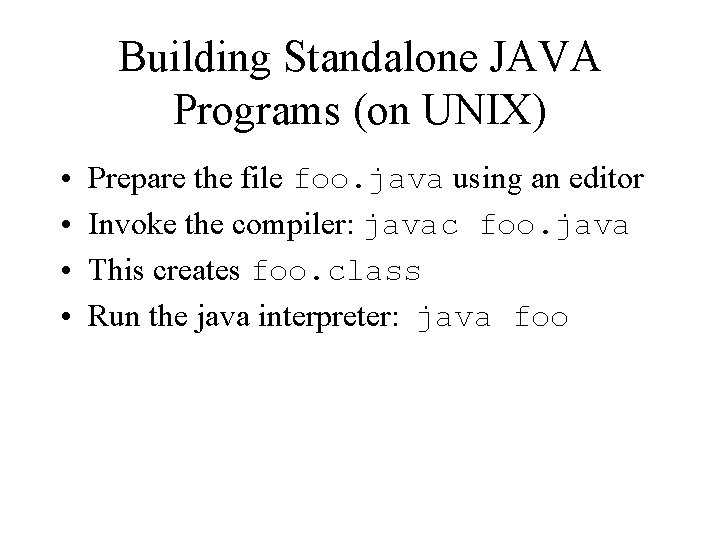
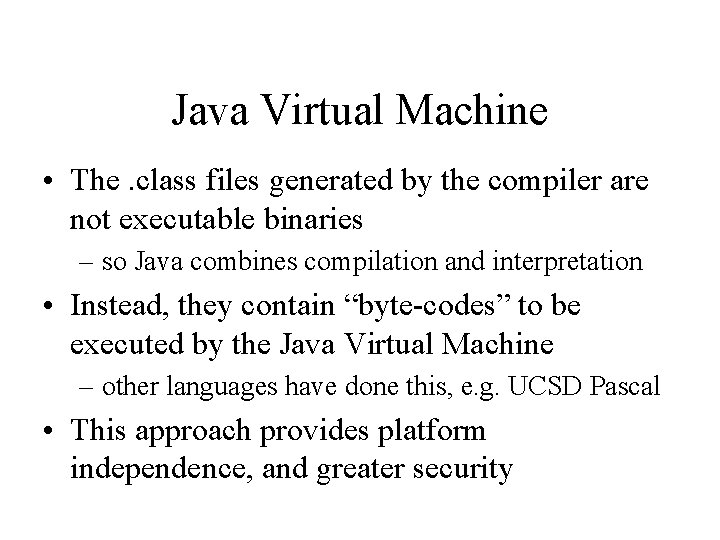
![Hello. World (standalone) public class Hello. World { public static void main(String[] args) { Hello. World (standalone) public class Hello. World { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/39b83049c2637973d5a68fd775a0e76b/image-6.jpg)
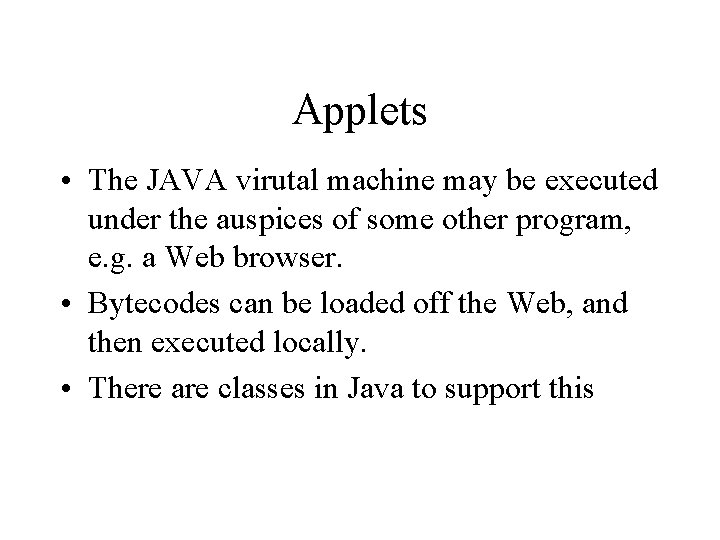
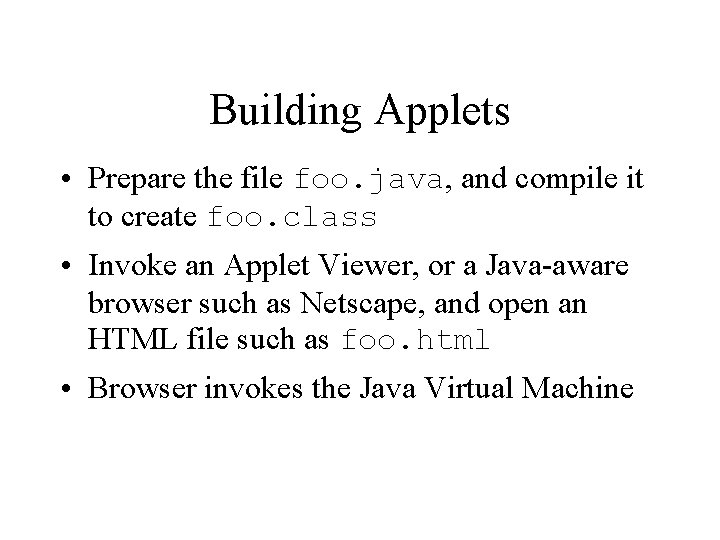
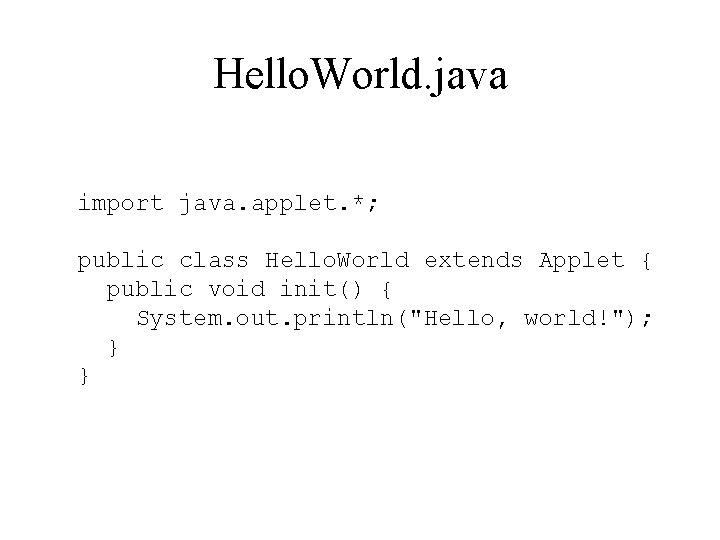
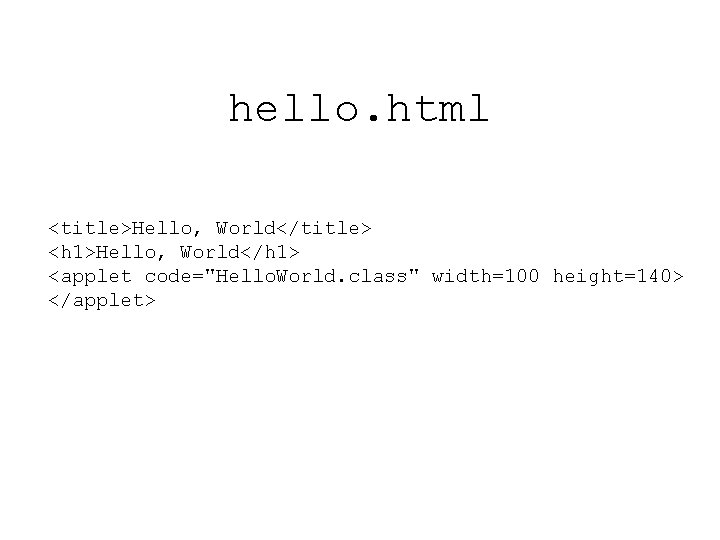
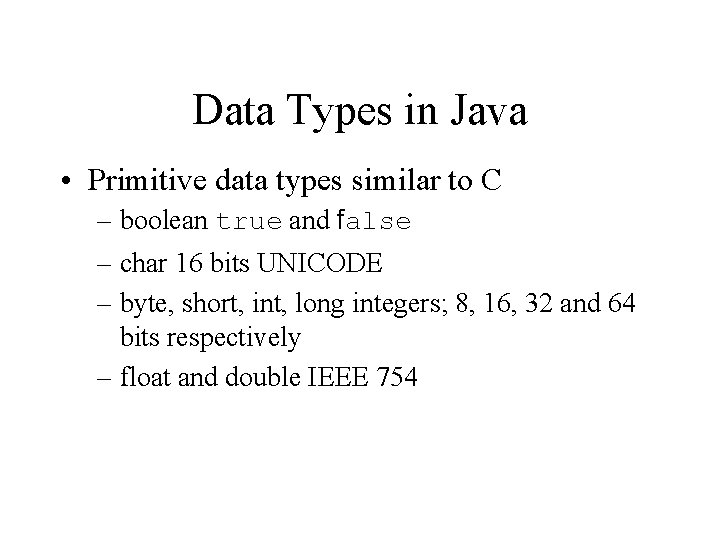
![Array Allocation • Declared in two ways: – float Vector 1[] = new float[500]; Array Allocation • Declared in two ways: – float Vector 1[] = new float[500];](https://slidetodoc.com/presentation_image/39b83049c2637973d5a68fd775a0e76b/image-12.jpg)
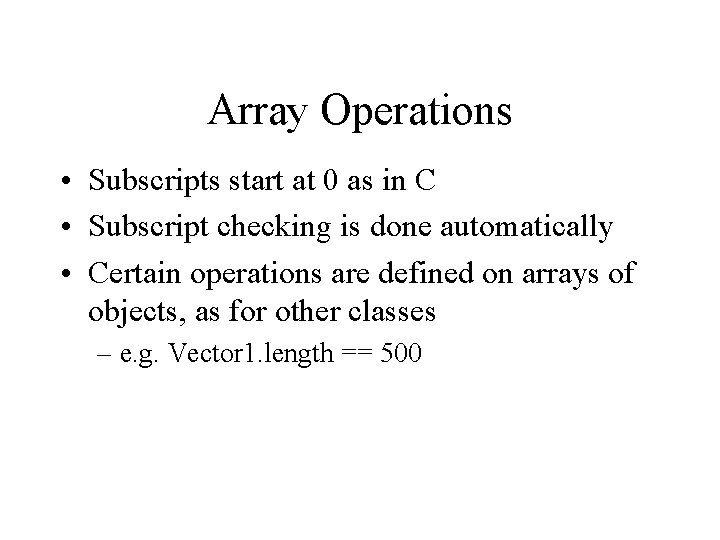
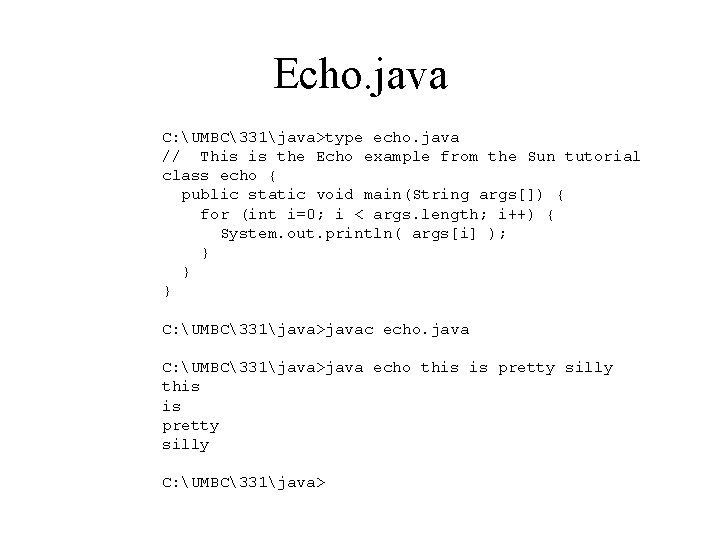
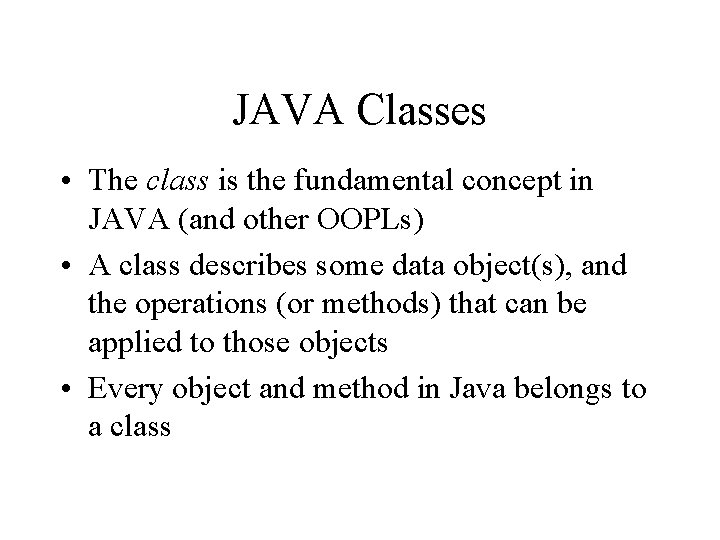
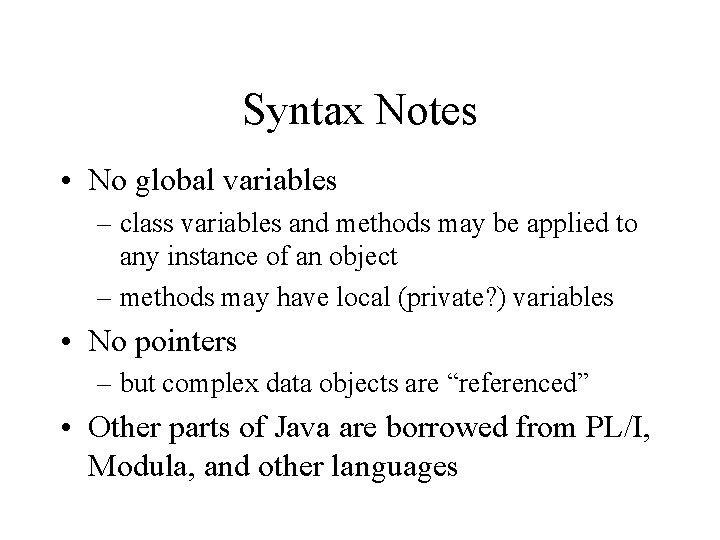
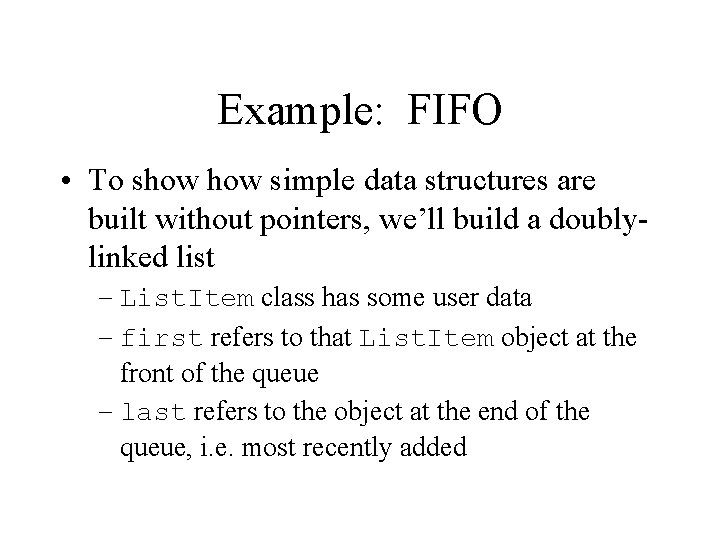
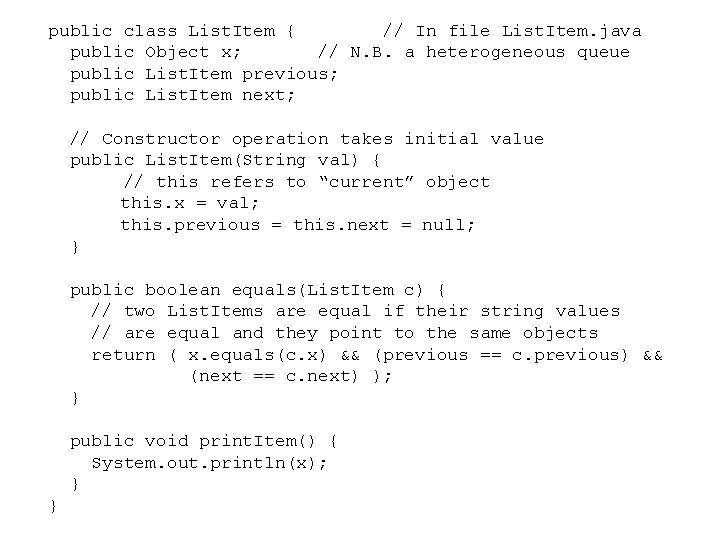
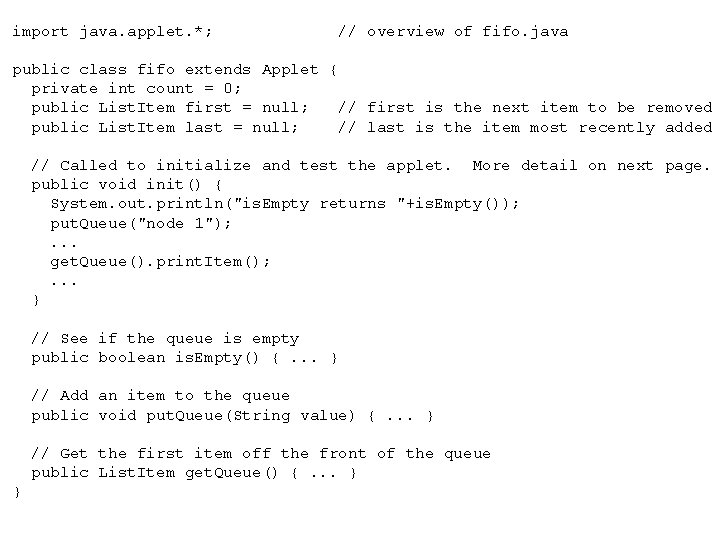
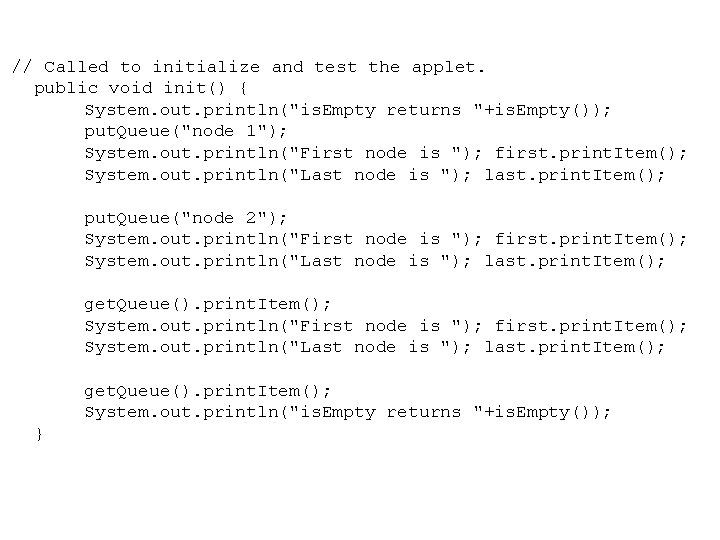
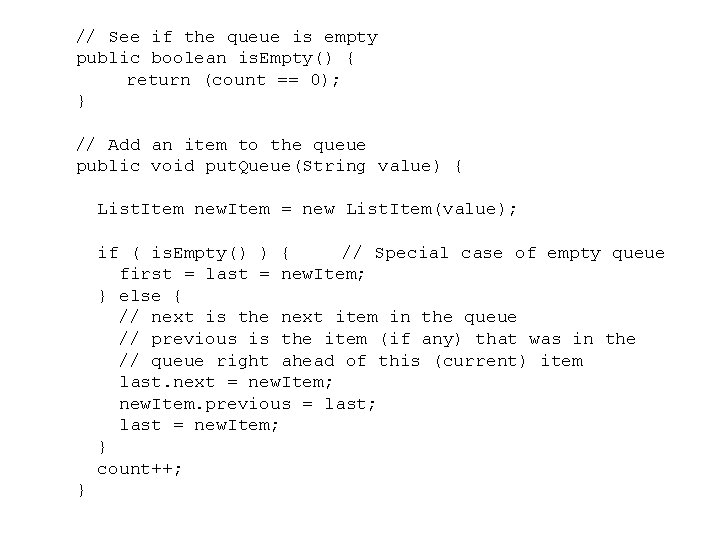
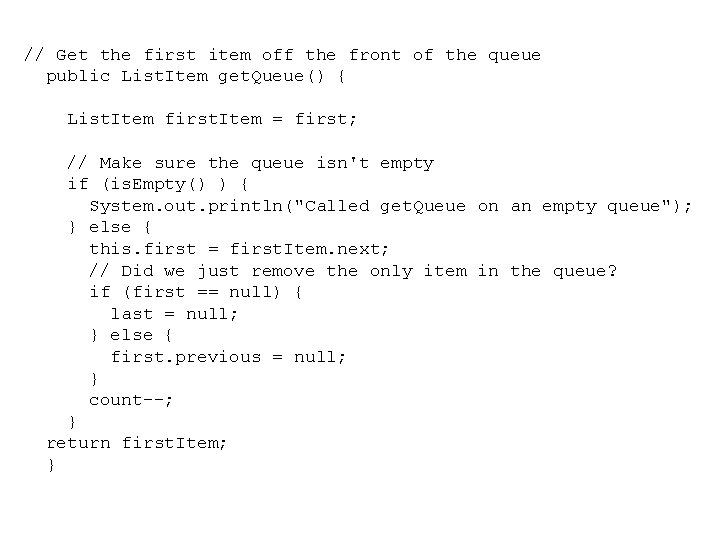
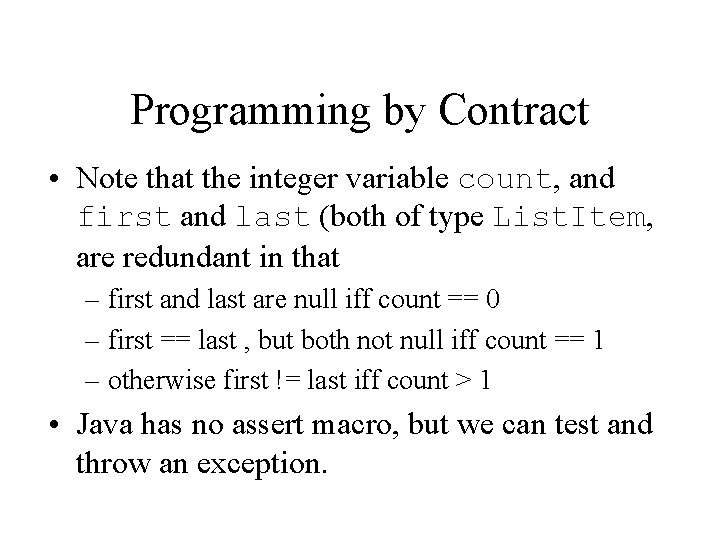
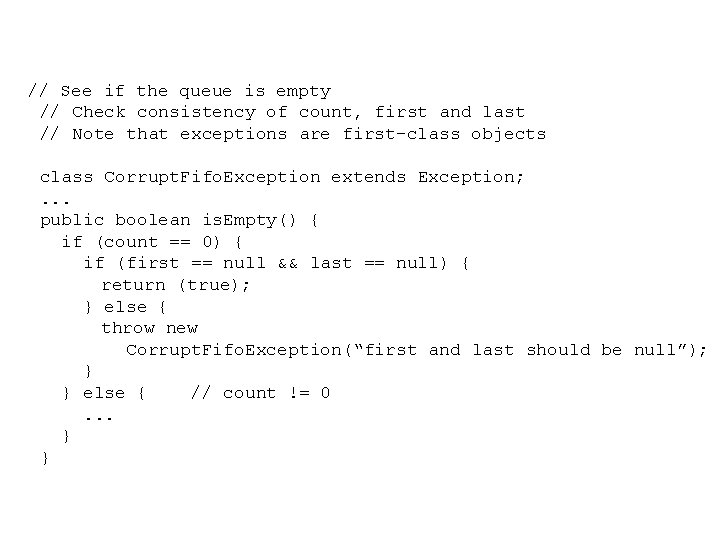
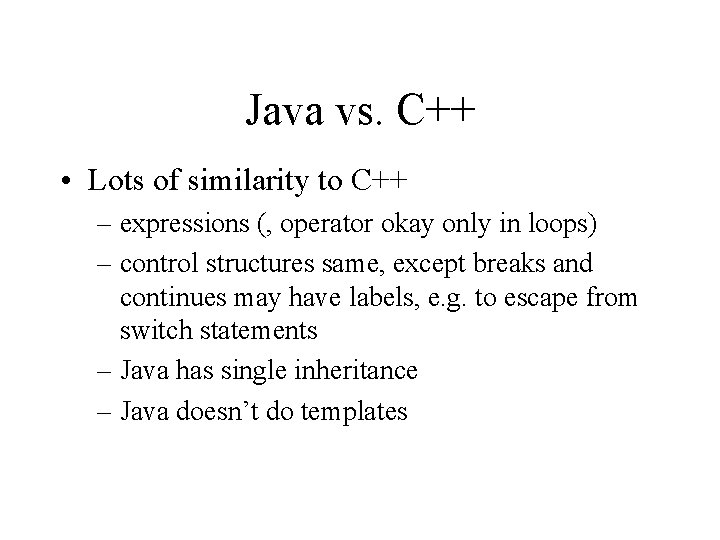
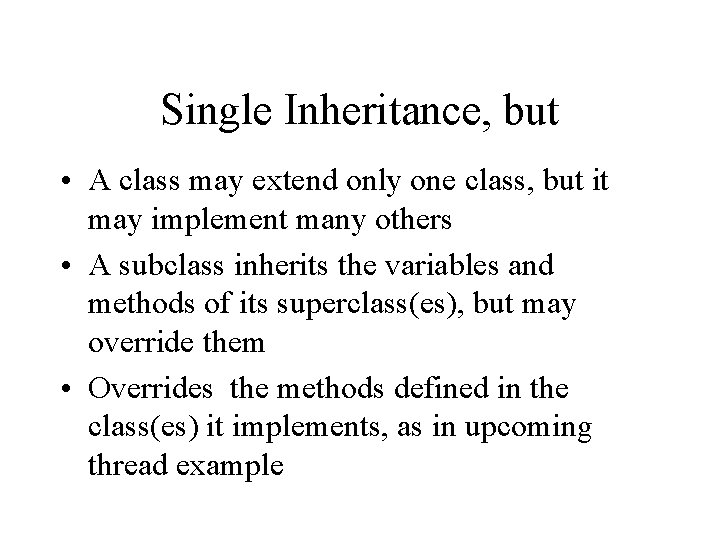
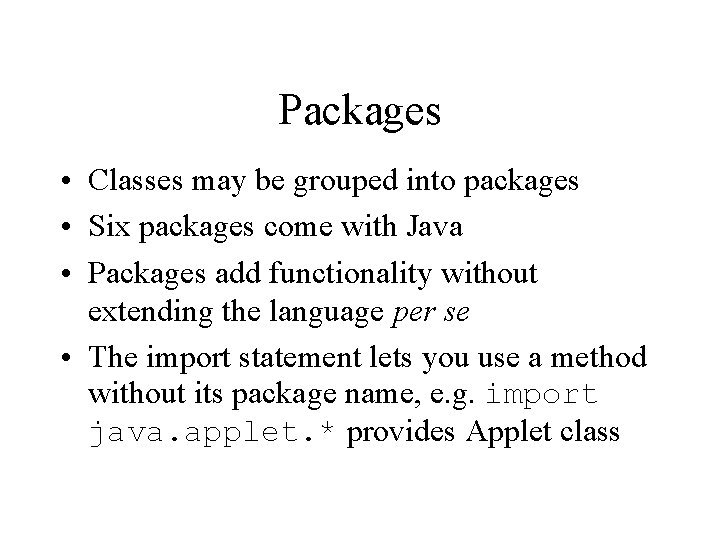
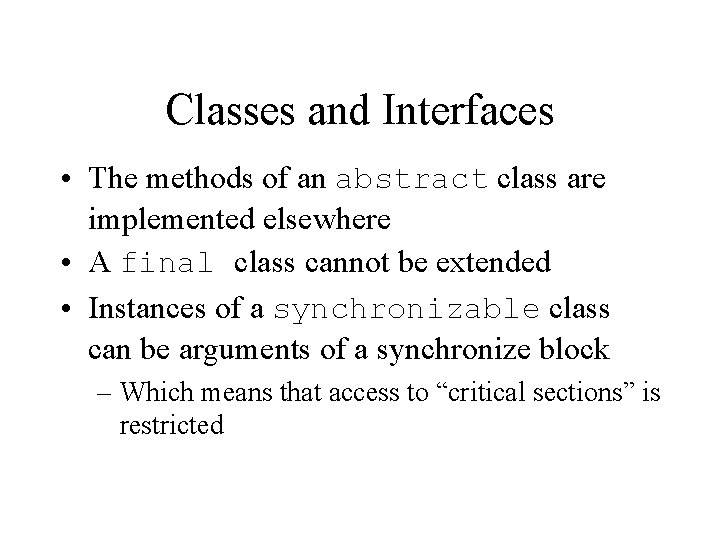
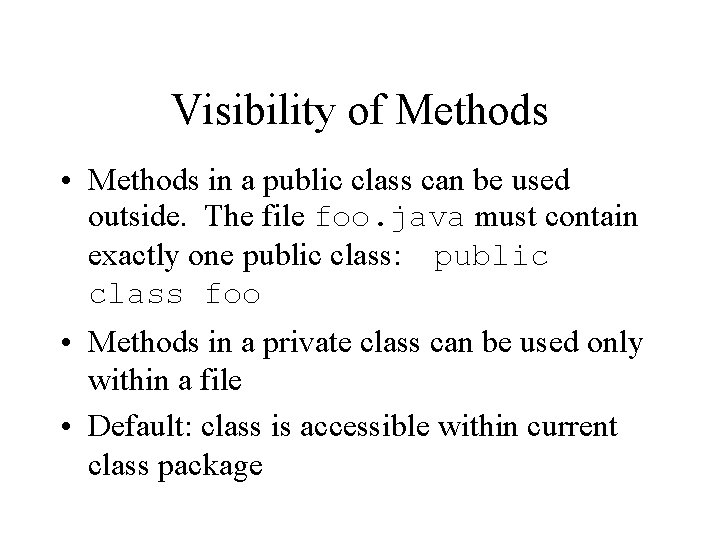
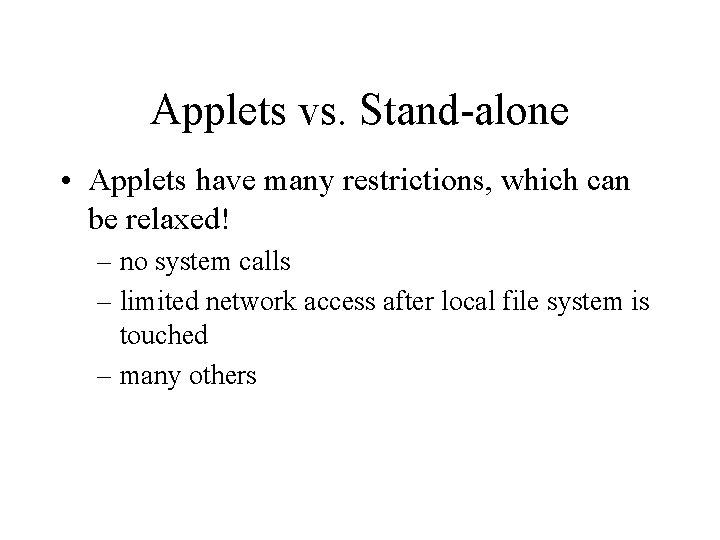
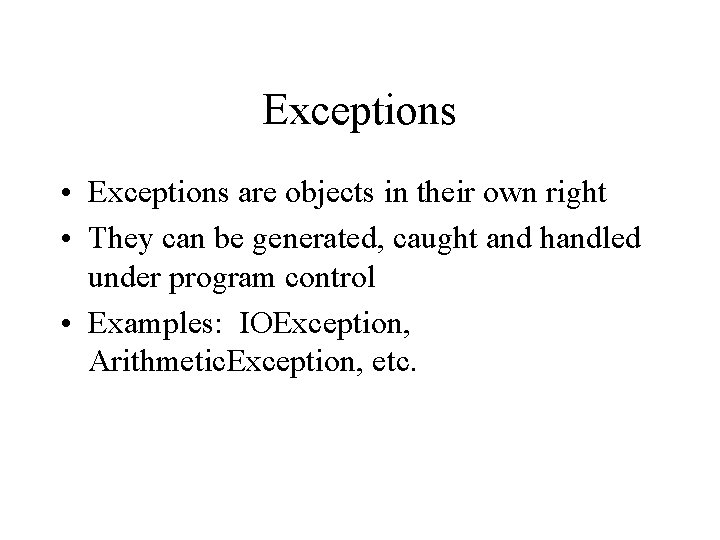
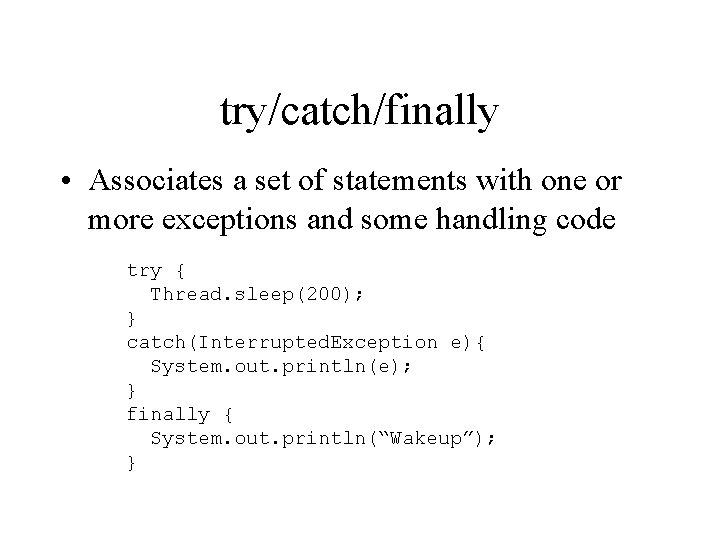
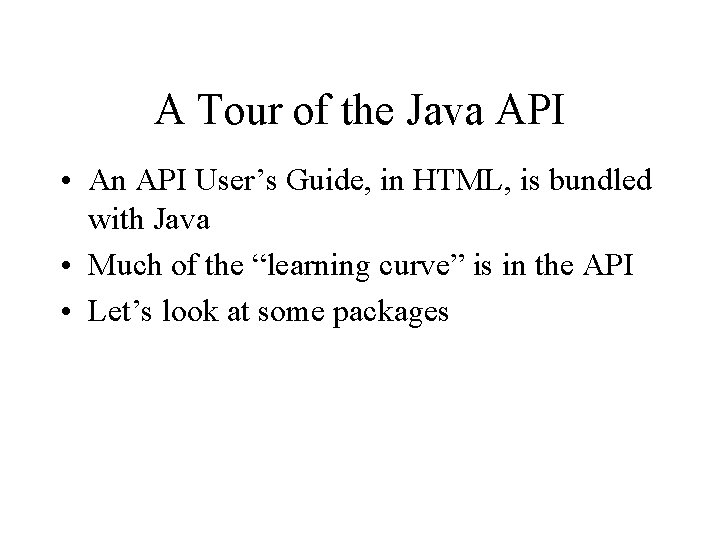
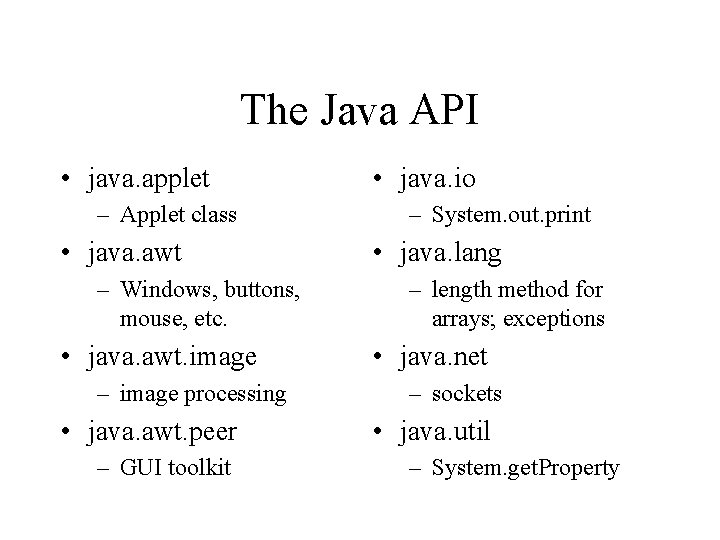
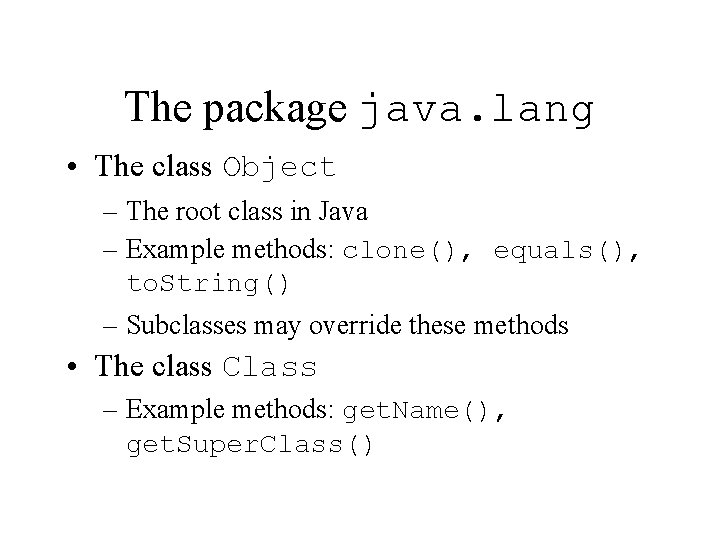
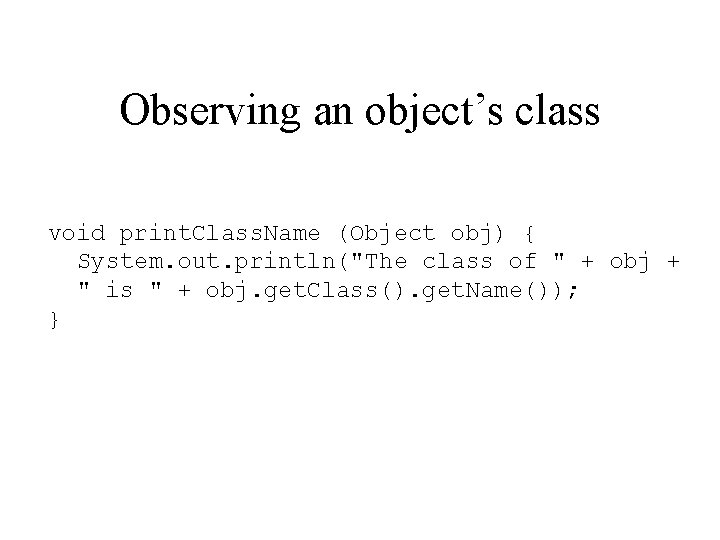
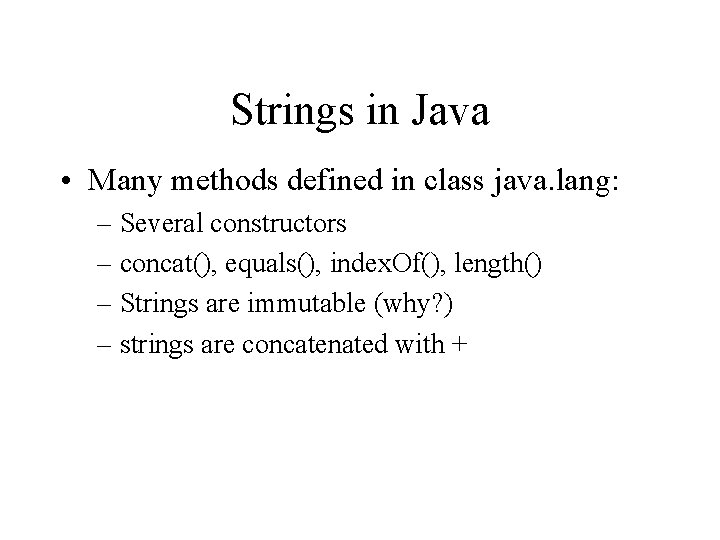
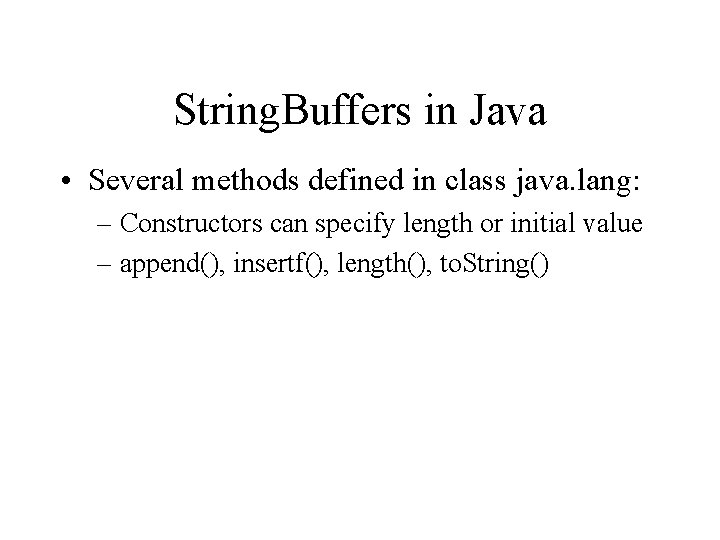
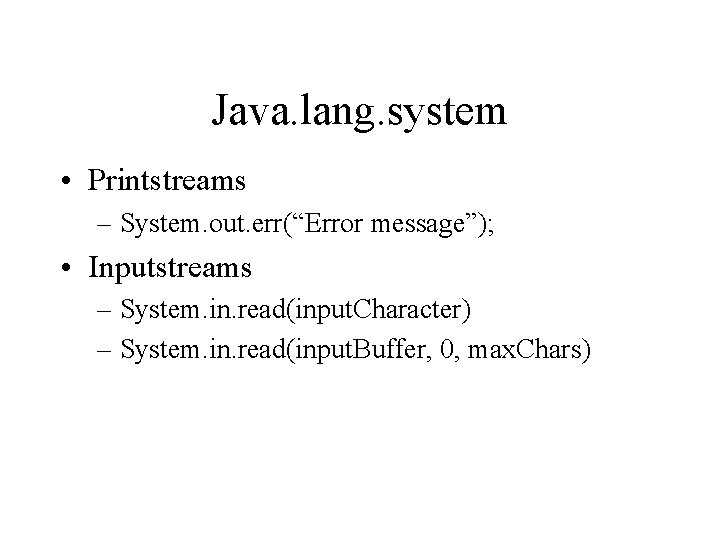
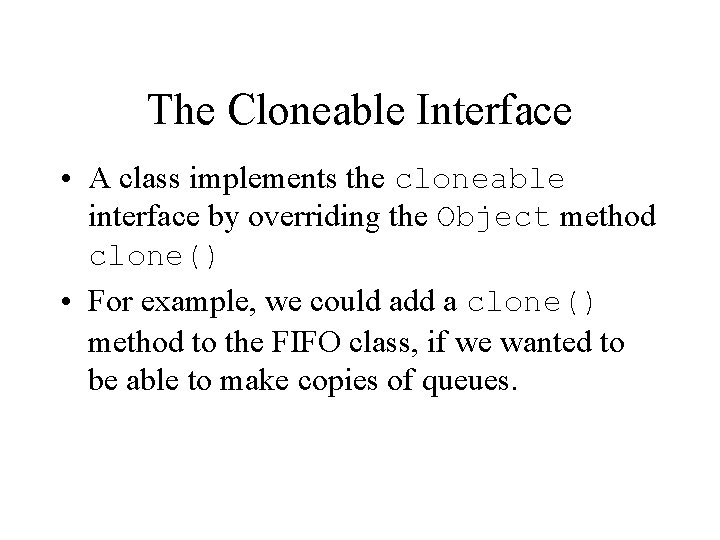
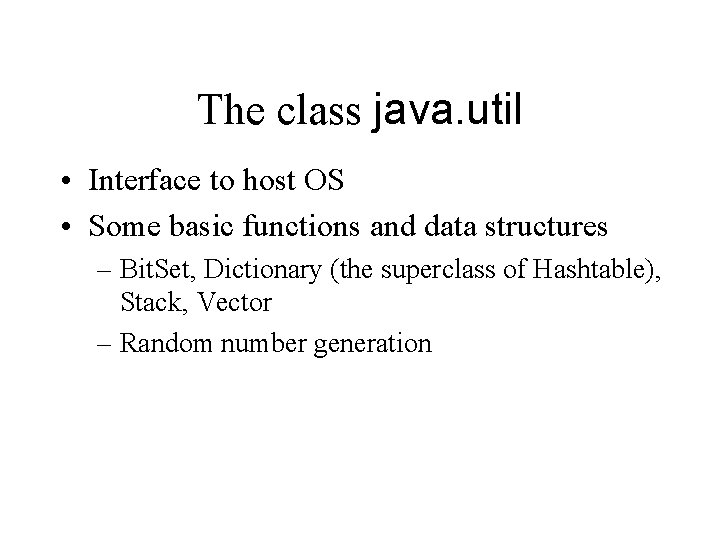
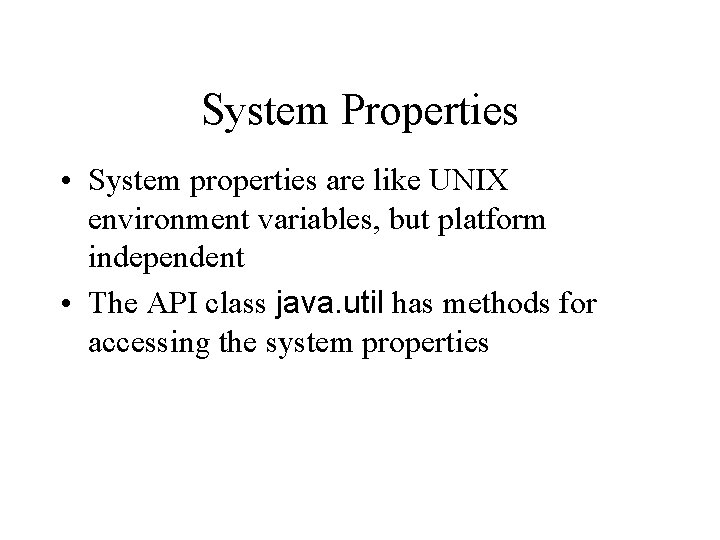
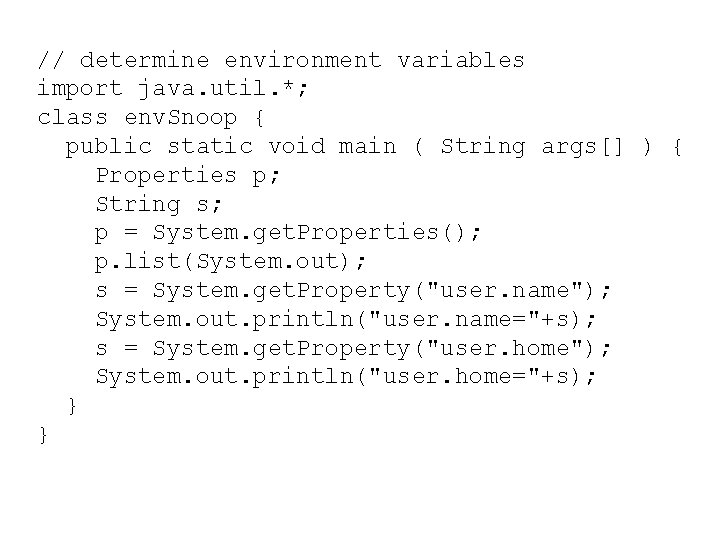
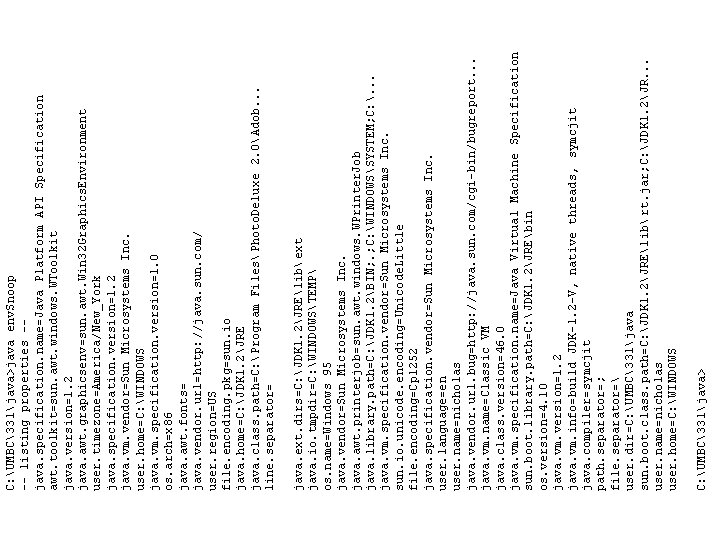
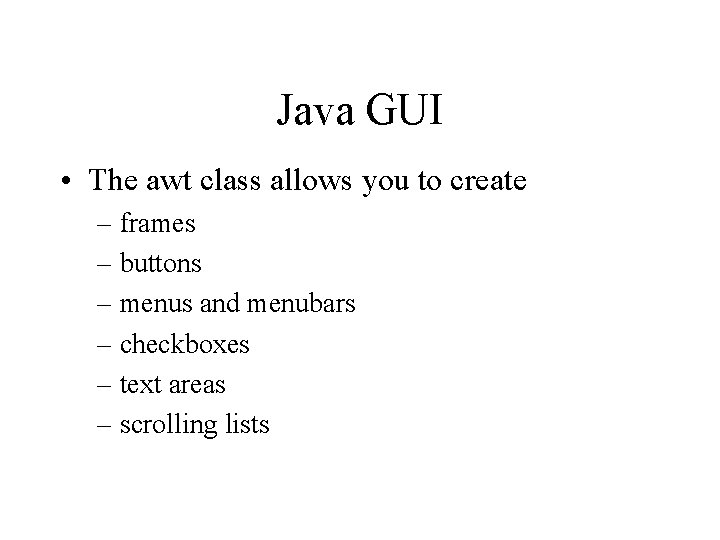
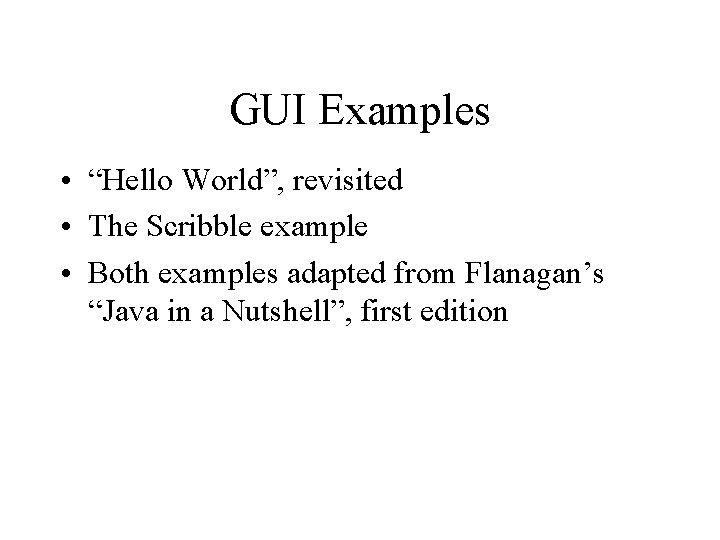
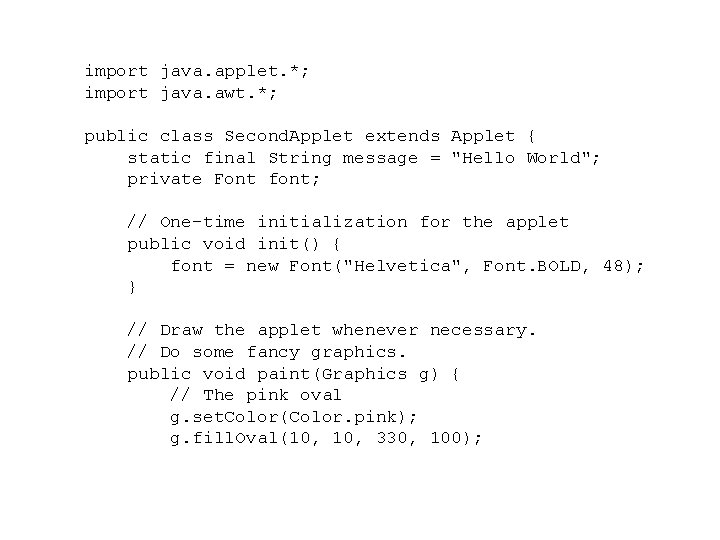
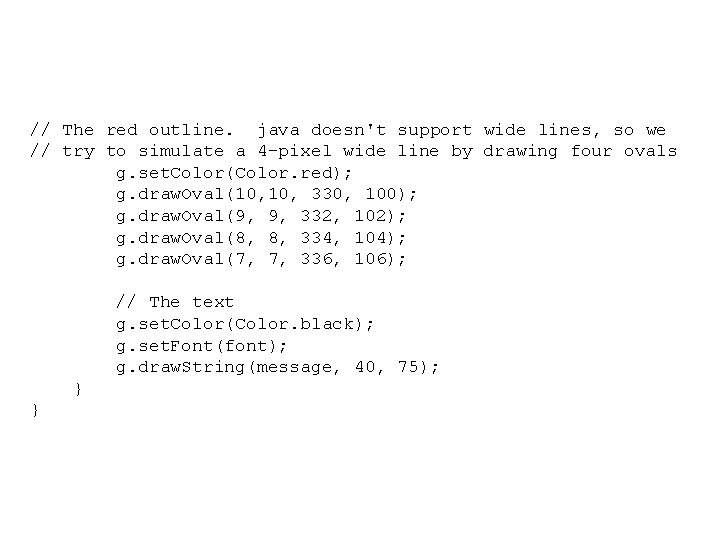
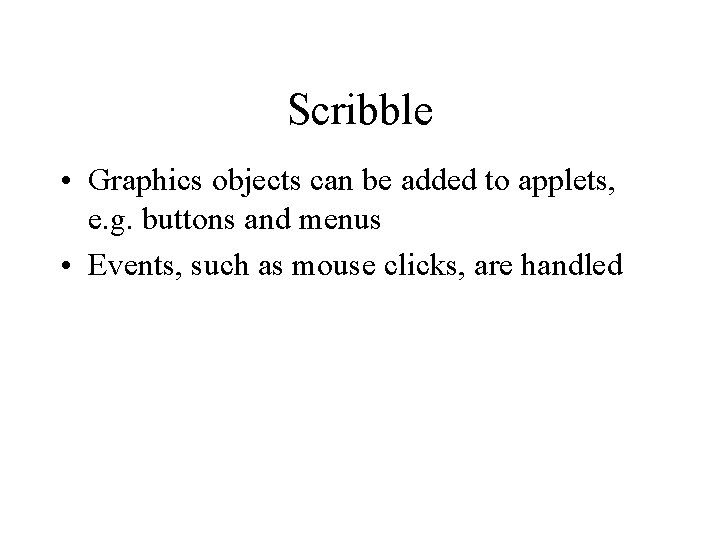
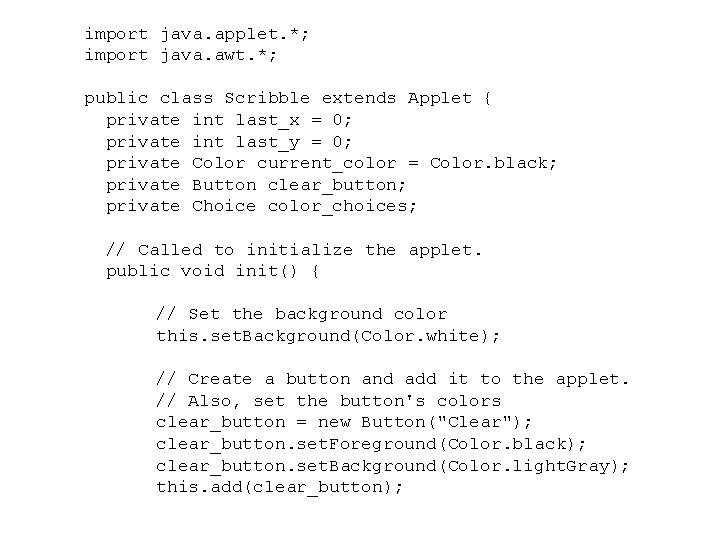
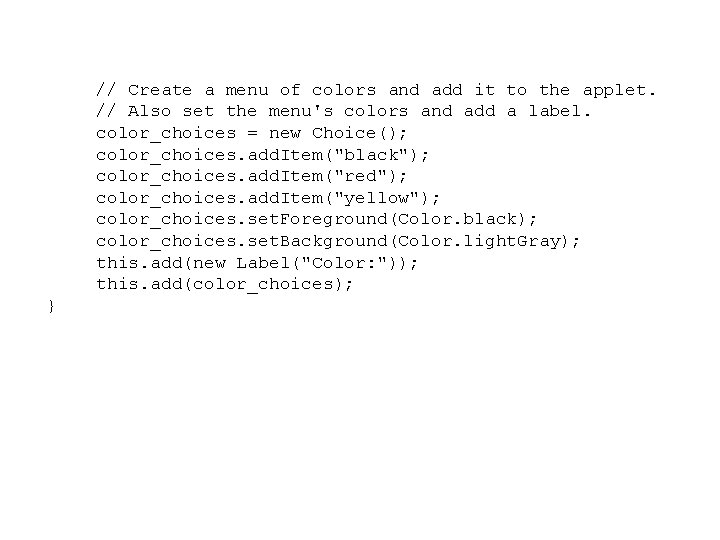
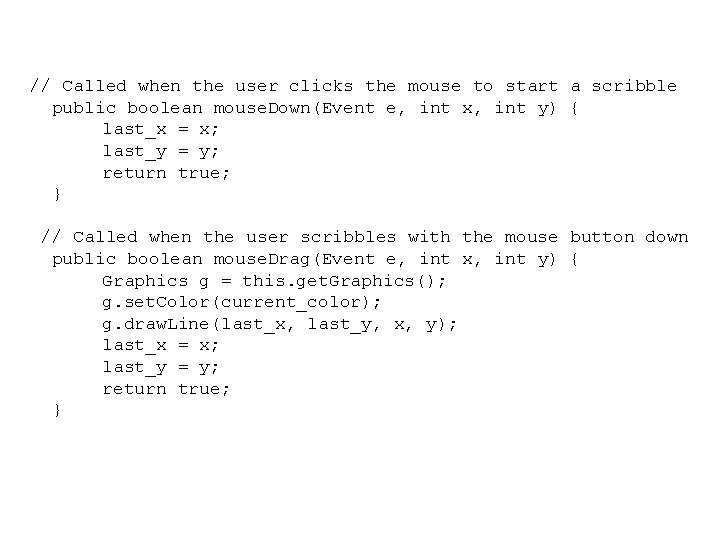
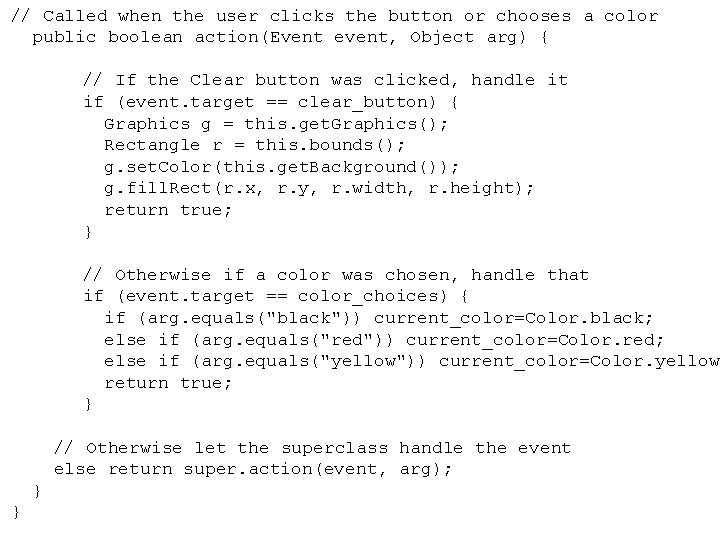
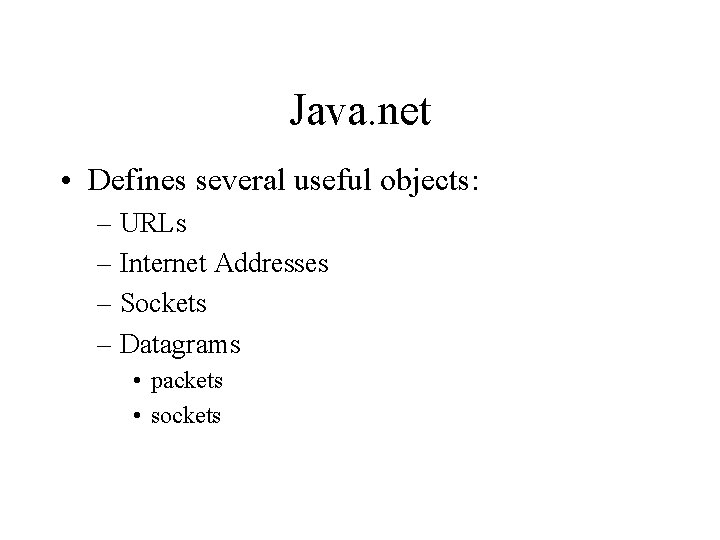
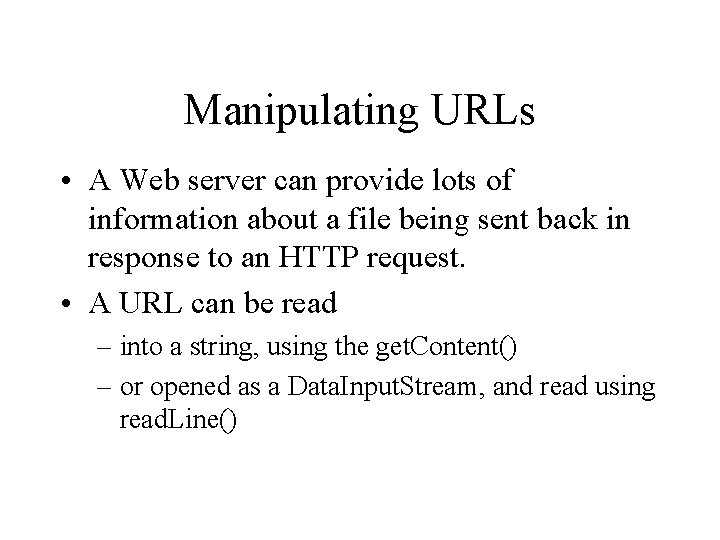
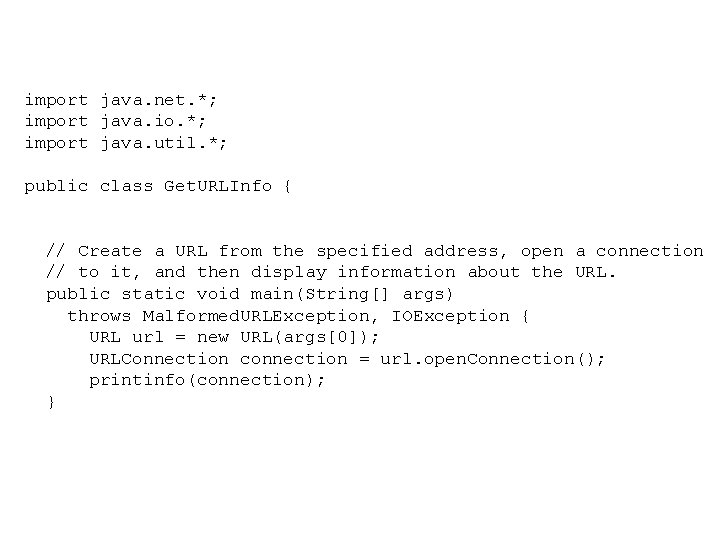
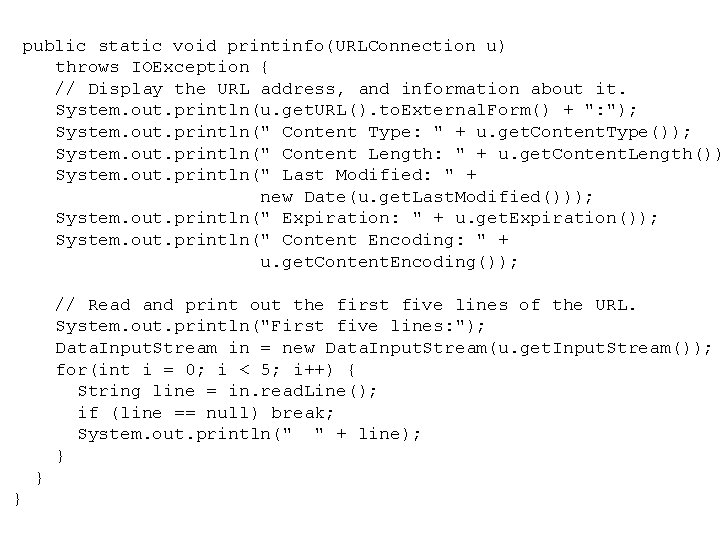
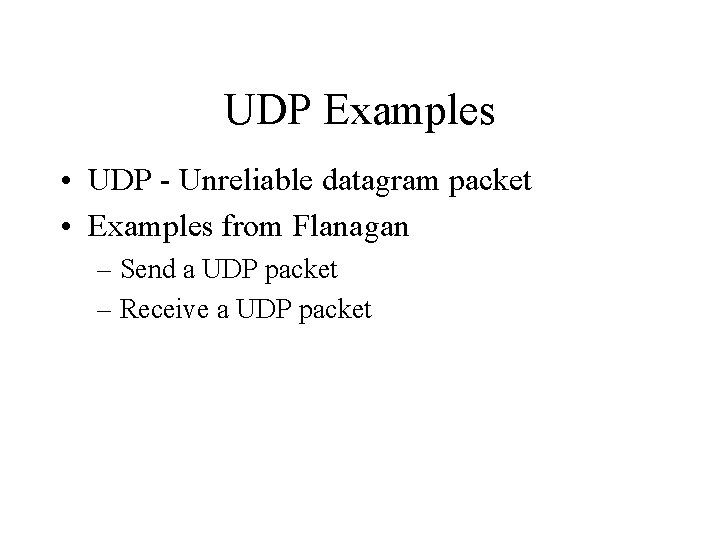
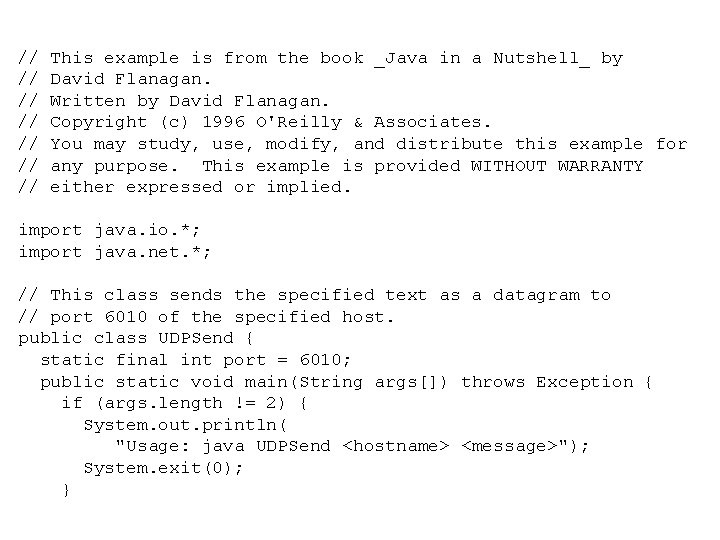
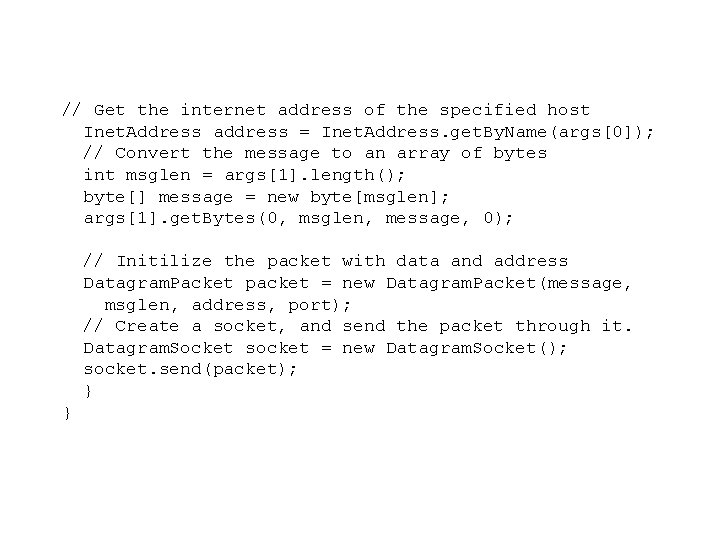
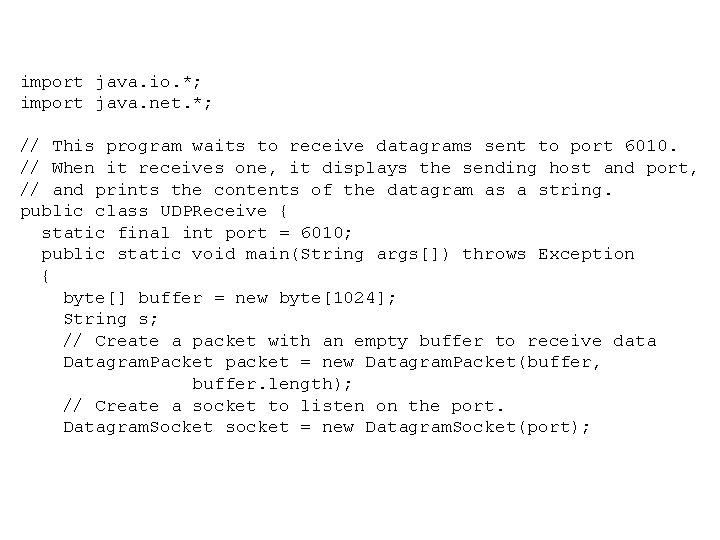
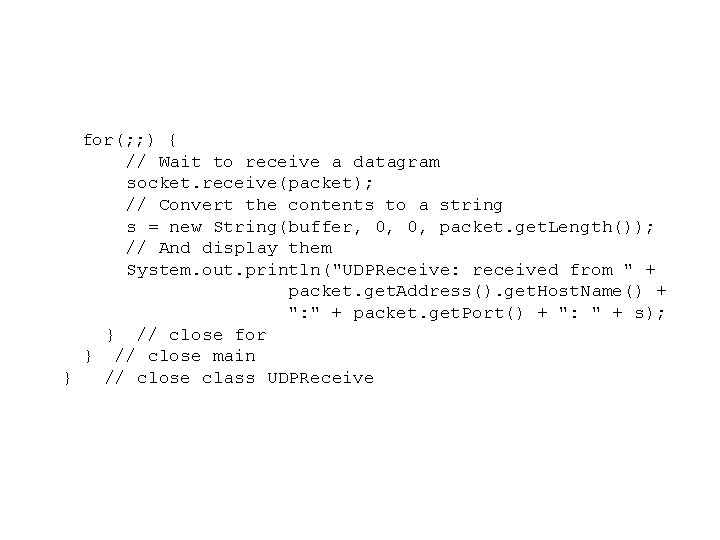
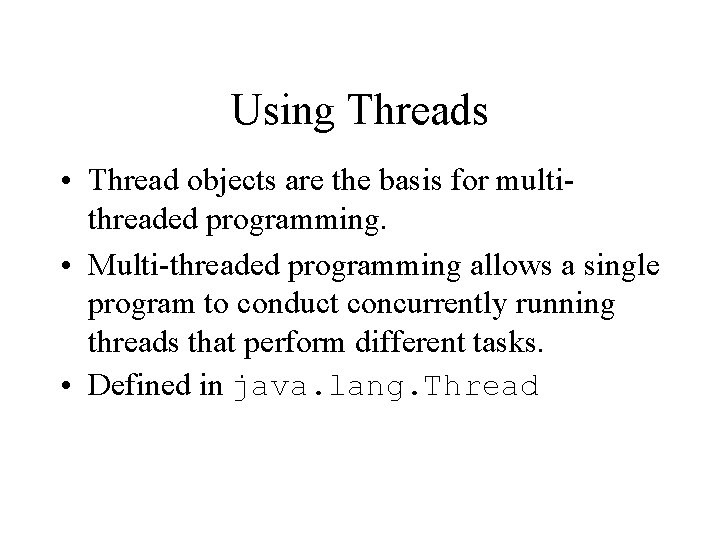
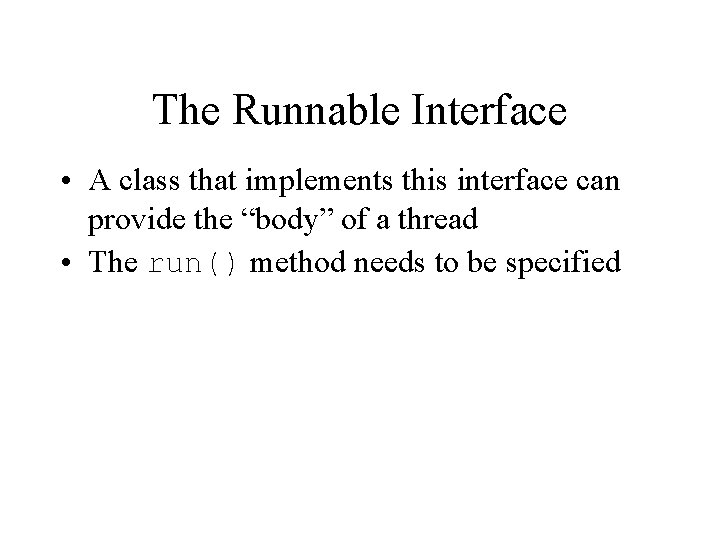
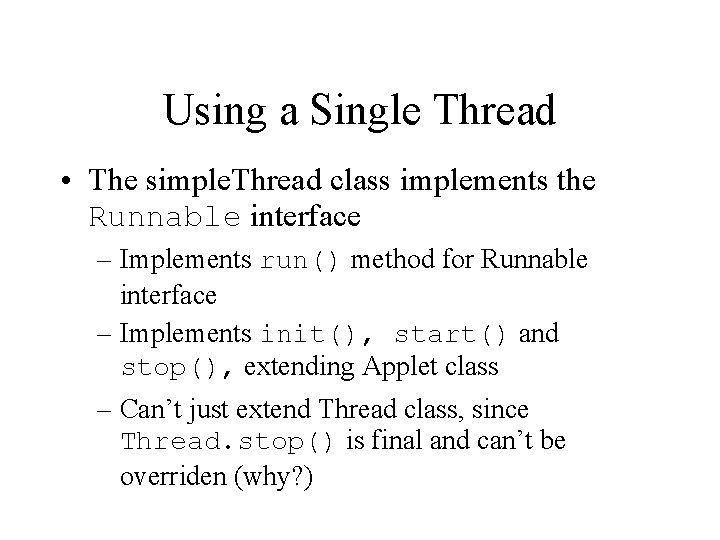
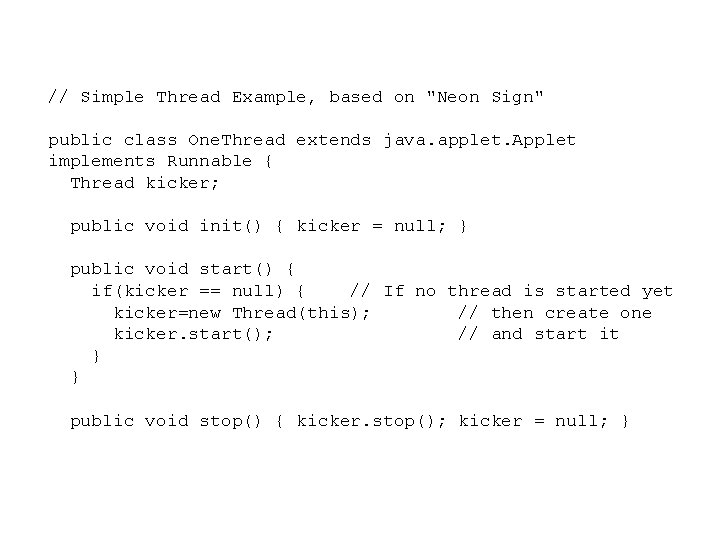
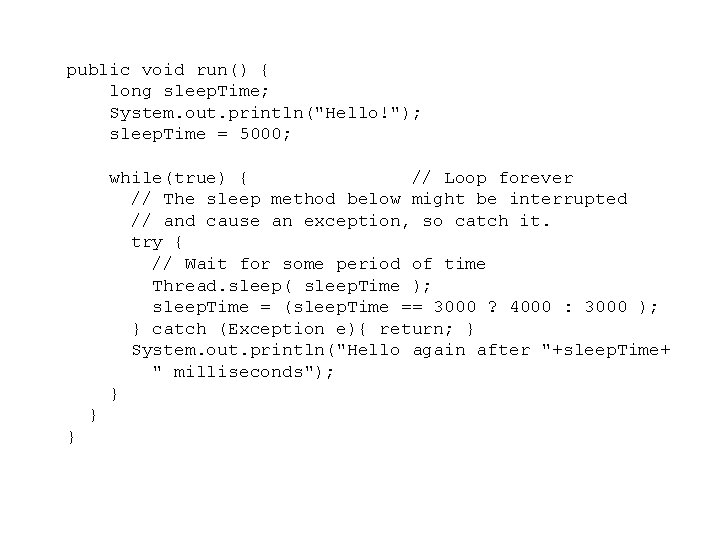
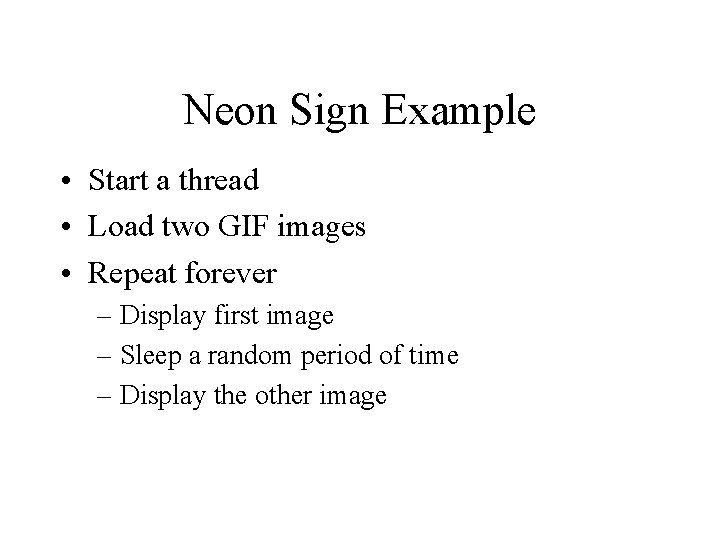
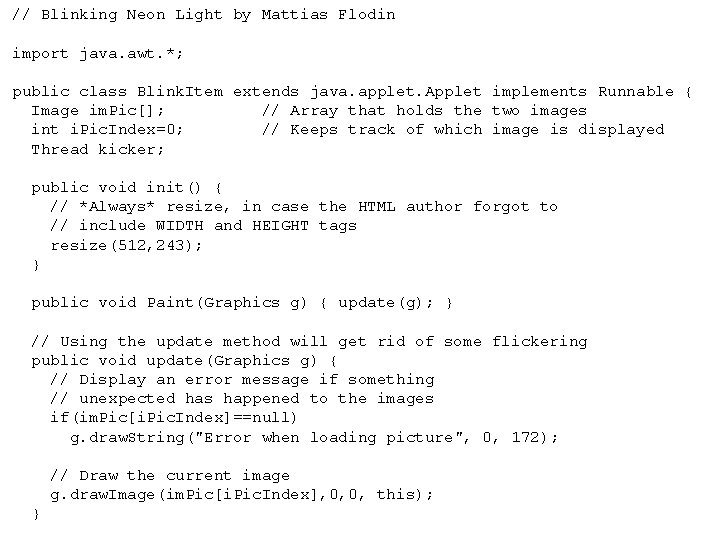
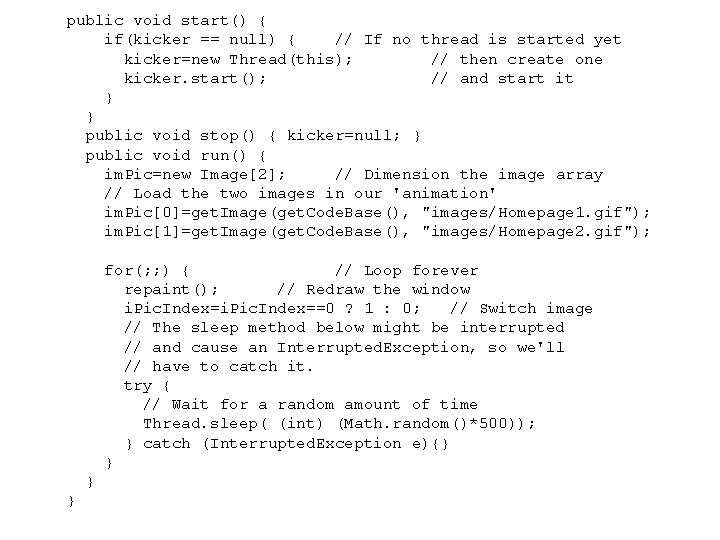
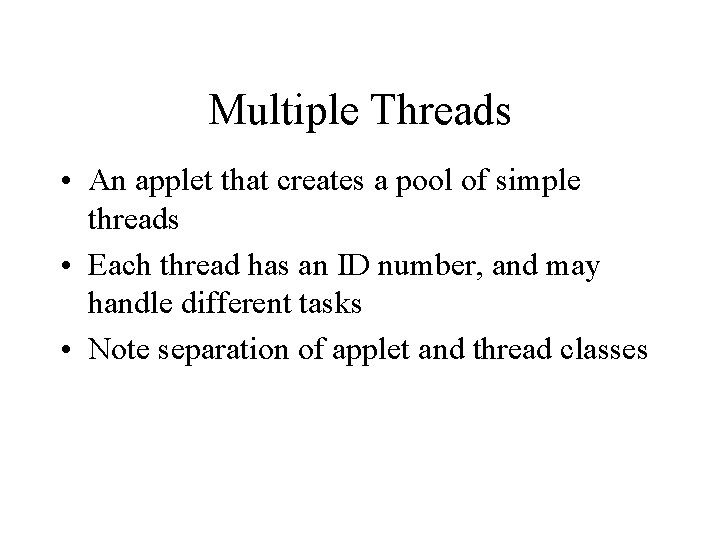
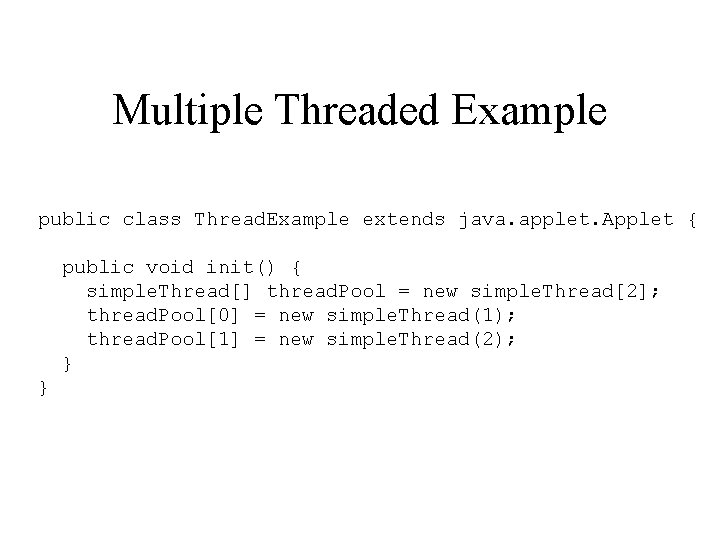
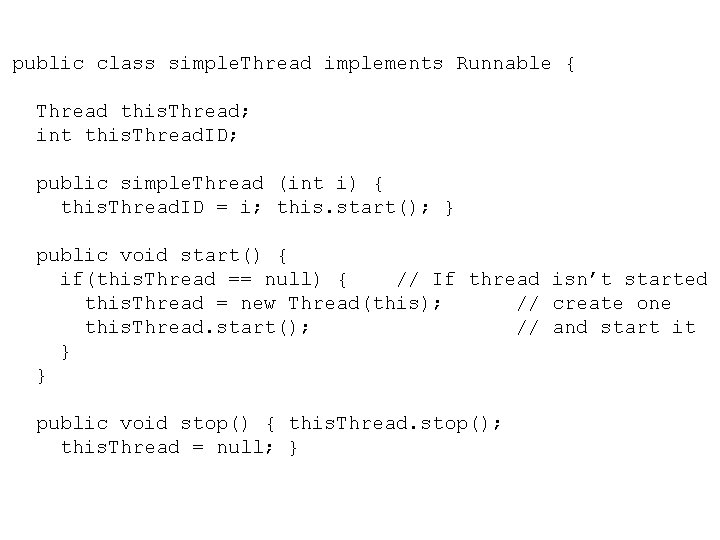
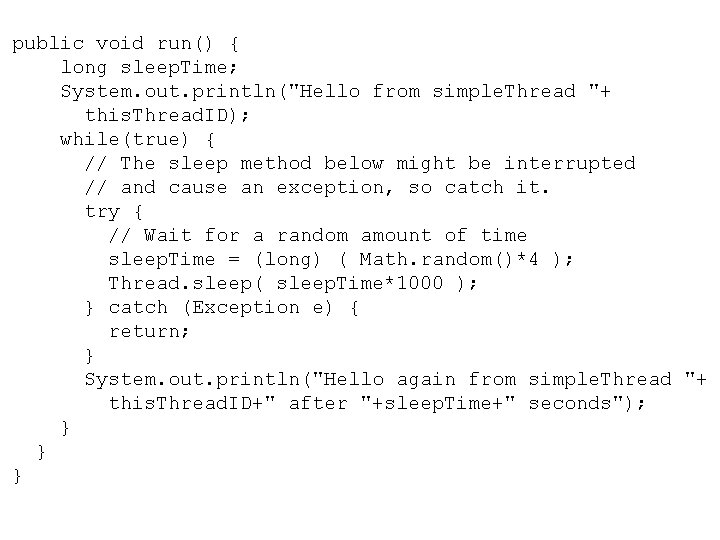
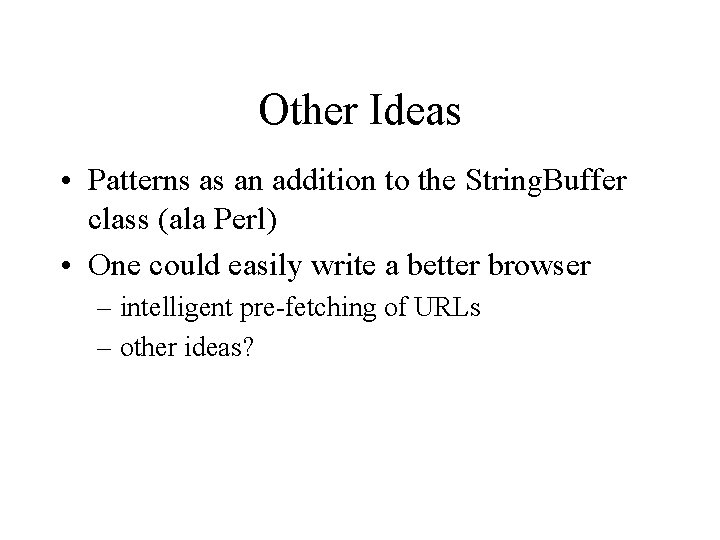
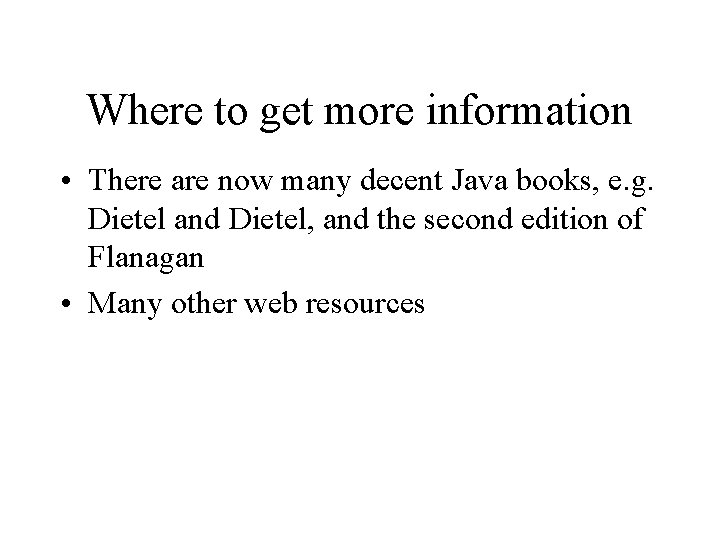
- Slides: 76
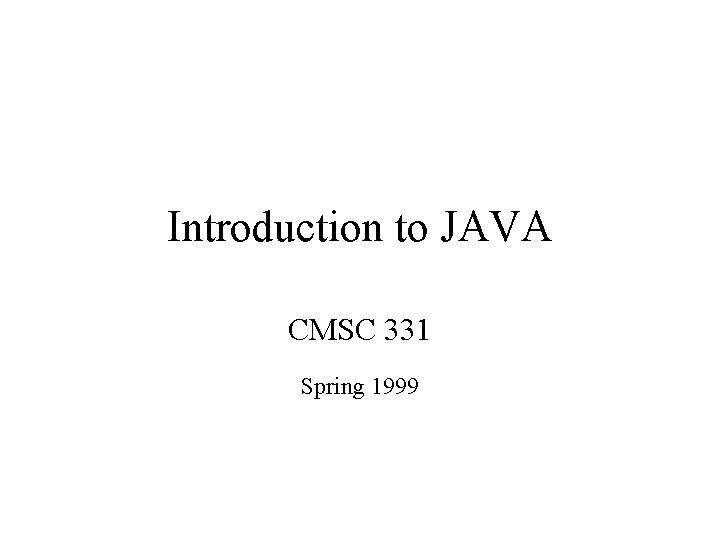
Introduction to JAVA CMSC 331 Spring 1999
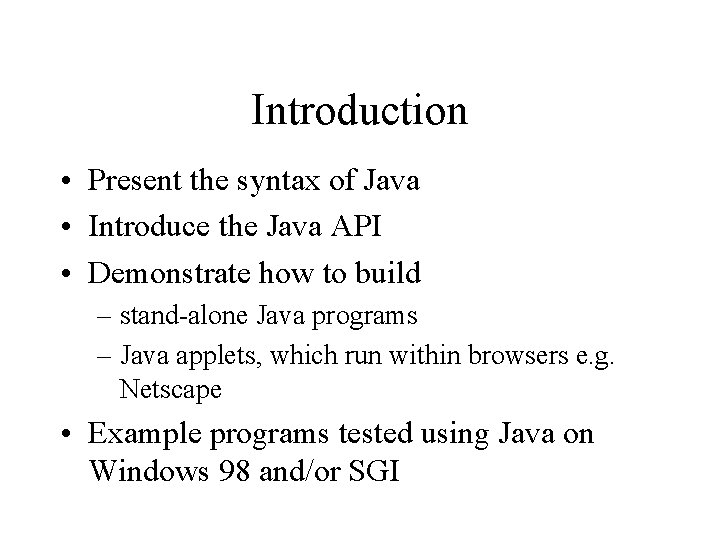
Introduction • Present the syntax of Java • Introduce the Java API • Demonstrate how to build – stand-alone Java programs – Java applets, which run within browsers e. g. Netscape • Example programs tested using Java on Windows 98 and/or SGI
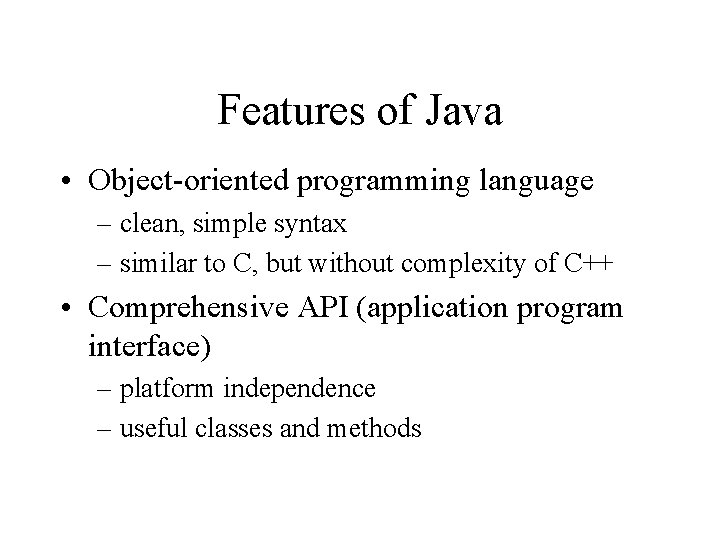
Features of Java • Object-oriented programming language – clean, simple syntax – similar to C, but without complexity of C++ • Comprehensive API (application program interface) – platform independence – useful classes and methods
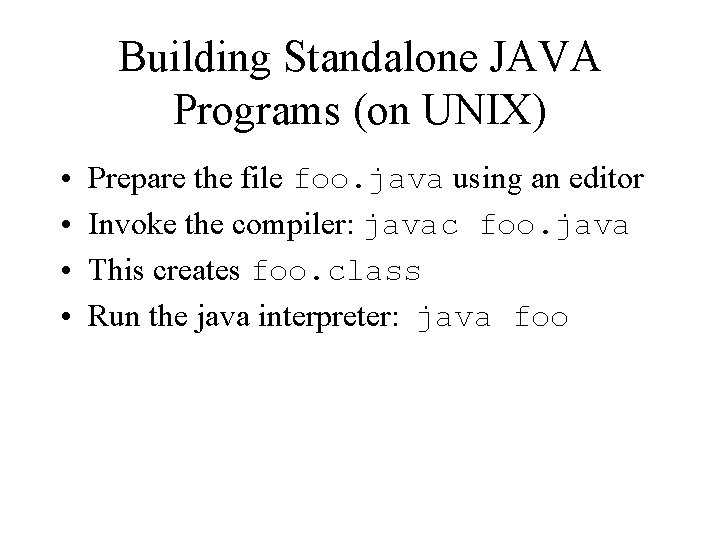
Building Standalone JAVA Programs (on UNIX) • • Prepare the file foo. java using an editor Invoke the compiler: javac foo. java This creates foo. class Run the java interpreter: java foo
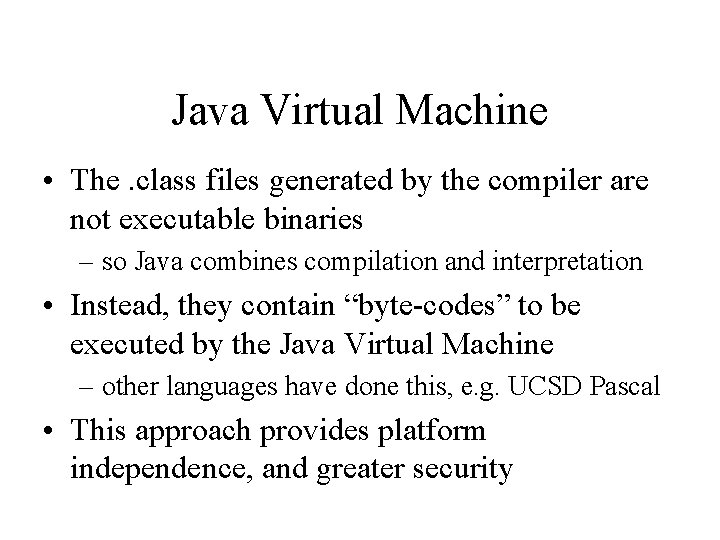
Java Virtual Machine • The. class files generated by the compiler are not executable binaries – so Java combines compilation and interpretation • Instead, they contain “byte-codes” to be executed by the Java Virtual Machine – other languages have done this, e. g. UCSD Pascal • This approach provides platform independence, and greater security
![Hello World standalone public class Hello World public static void mainString args Hello. World (standalone) public class Hello. World { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/39b83049c2637973d5a68fd775a0e76b/image-6.jpg)
Hello. World (standalone) public class Hello. World { public static void main(String[] args) { System. out. println("Hello World!"); } } • Note that String is built in • println is a member function for the System. out class
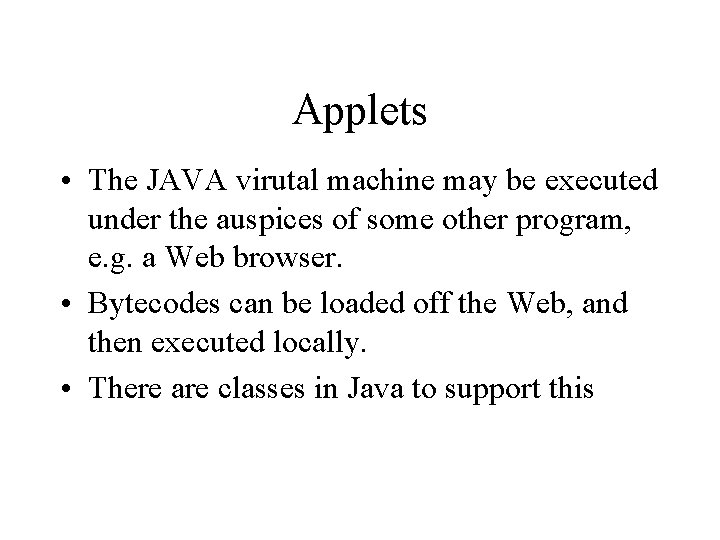
Applets • The JAVA virutal machine may be executed under the auspices of some other program, e. g. a Web browser. • Bytecodes can be loaded off the Web, and then executed locally. • There are classes in Java to support this
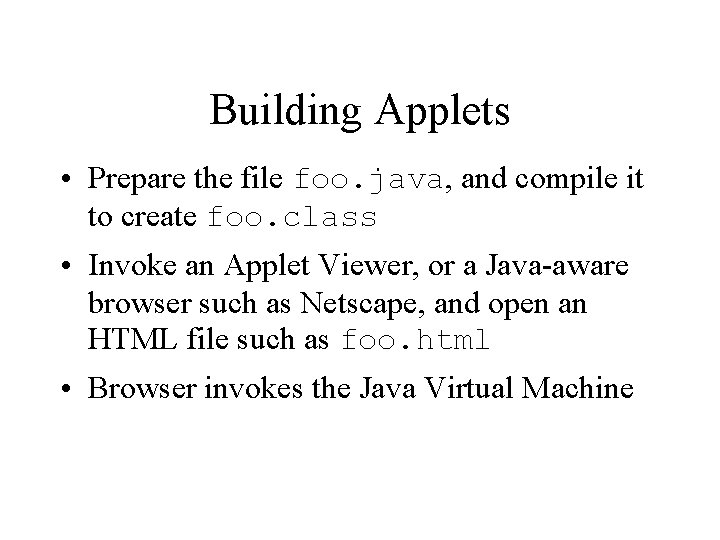
Building Applets • Prepare the file foo. java, and compile it to create foo. class • Invoke an Applet Viewer, or a Java-aware browser such as Netscape, and open an HTML file such as foo. html • Browser invokes the Java Virtual Machine
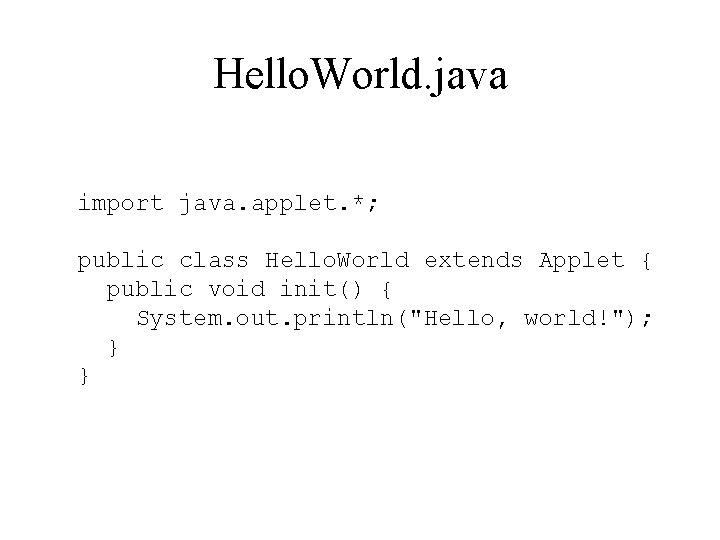
Hello. World. java import java. applet. *; public class Hello. World extends Applet { public void init() { System. out. println("Hello, world!"); } }
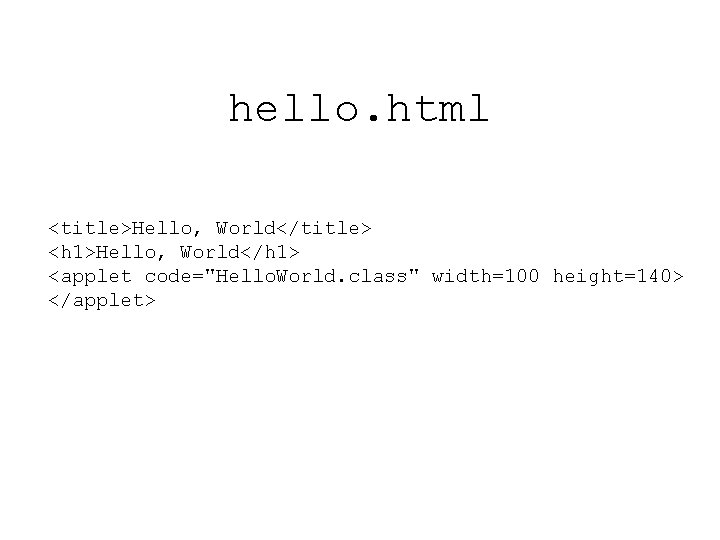
hello. html <title>Hello, World</title> <h 1>Hello, World</h 1> <applet code="Hello. World. class" width=100 height=140> </applet>
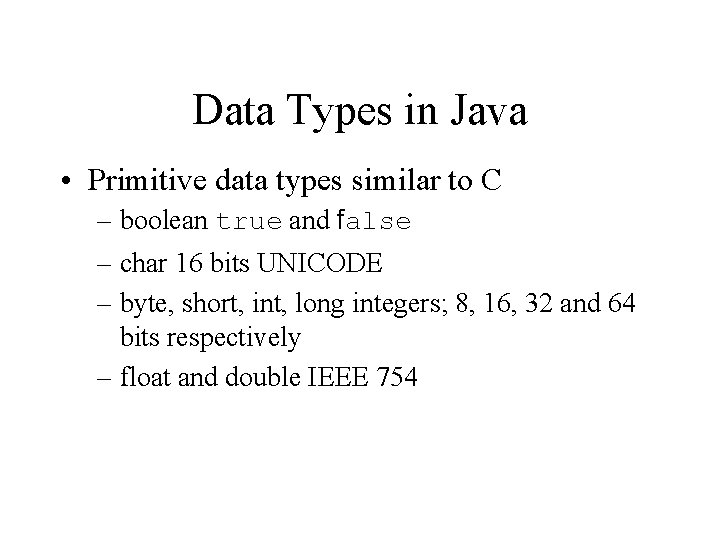
Data Types in Java • Primitive data types similar to C – boolean true and false – char 16 bits UNICODE – byte, short, int, long integers; 8, 16, 32 and 64 bits respectively – float and double IEEE 754
![Array Allocation Declared in two ways float Vector 1 new float500 Array Allocation • Declared in two ways: – float Vector 1[] = new float[500];](https://slidetodoc.com/presentation_image/39b83049c2637973d5a68fd775a0e76b/image-12.jpg)
Array Allocation • Declared in two ways: – float Vector 1[] = new float[500]; – int Vector 2[] = {10, 20, 30, 40}; • Not allocated on stack, but dynamically • Are subject to garbage collection when no more references remain – so fewer memory leaks – Java doesn’t have pointers!
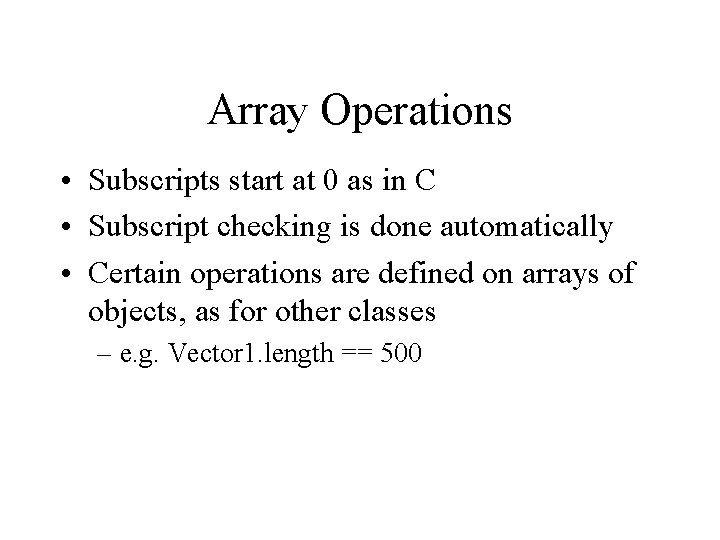
Array Operations • Subscripts start at 0 as in C • Subscript checking is done automatically • Certain operations are defined on arrays of objects, as for other classes – e. g. Vector 1. length == 500
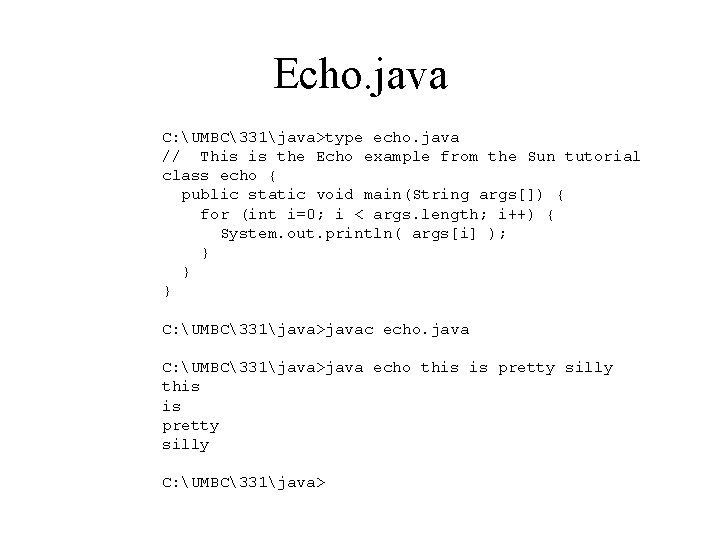
Echo. java C: UMBC331java>type echo. java // This is the Echo example from the Sun tutorial class echo { public static void main(String args[]) { for (int i=0; i < args. length; i++) { System. out. println( args[i] ); } } } C: UMBC331java>javac echo. java C: UMBC331java>java echo this is pretty silly C: UMBC331java>
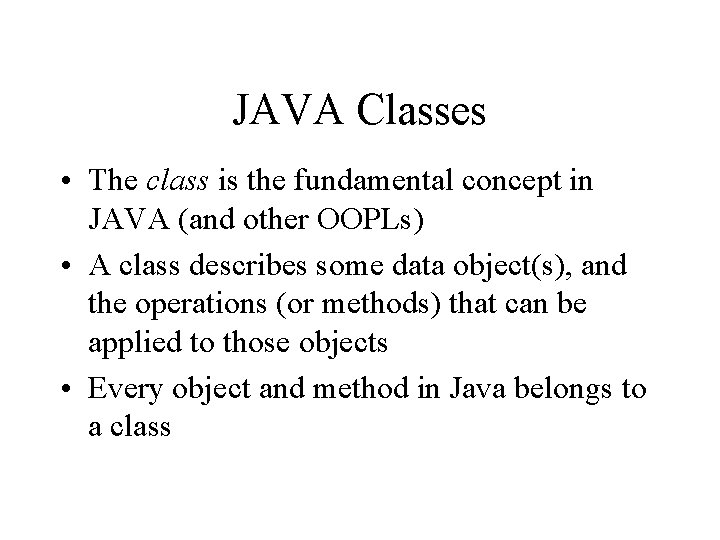
JAVA Classes • The class is the fundamental concept in JAVA (and other OOPLs) • A class describes some data object(s), and the operations (or methods) that can be applied to those objects • Every object and method in Java belongs to a class
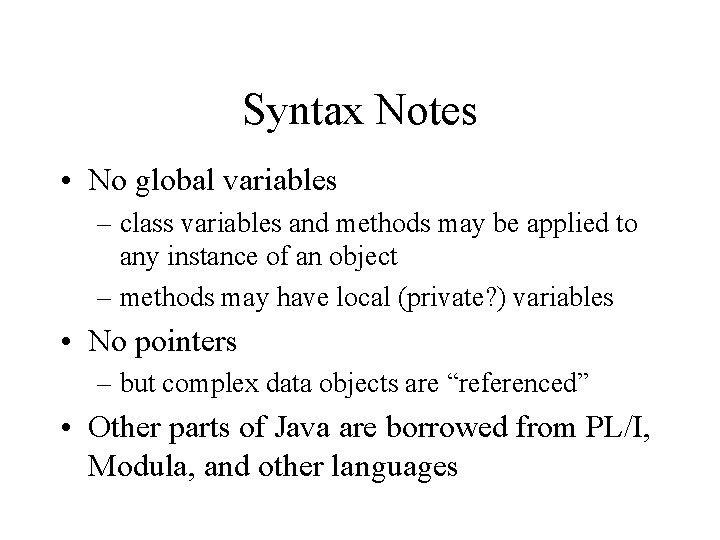
Syntax Notes • No global variables – class variables and methods may be applied to any instance of an object – methods may have local (private? ) variables • No pointers – but complex data objects are “referenced” • Other parts of Java are borrowed from PL/I, Modula, and other languages
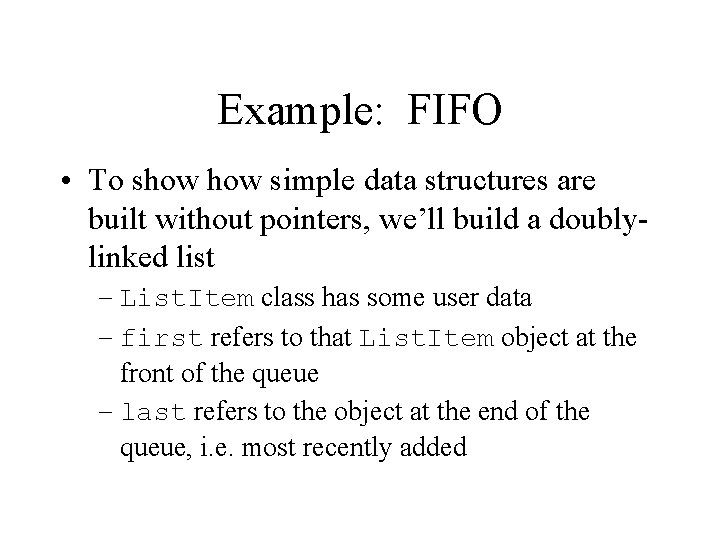
Example: FIFO • To show simple data structures are built without pointers, we’ll build a doublylinked list – List. Item class has some user data – first refers to that List. Item object at the front of the queue – last refers to the object at the end of the queue, i. e. most recently added
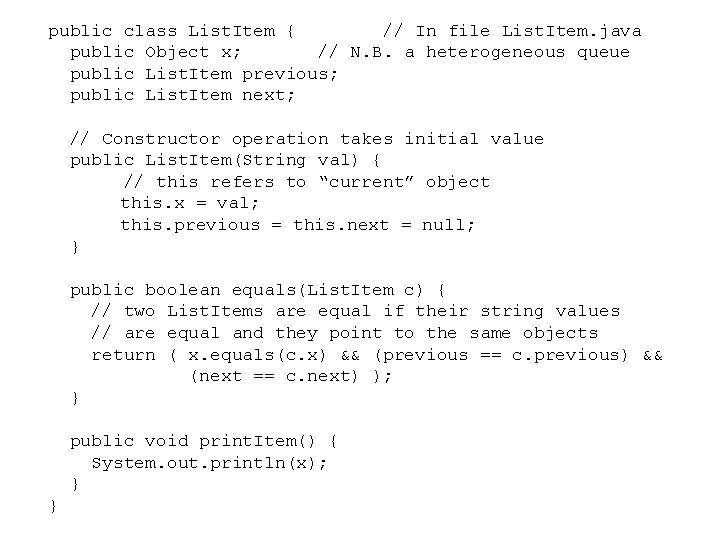
public class List. Item { // In file List. Item. java public Object x; // N. B. a heterogeneous queue public List. Item previous; public List. Item next; // Constructor operation takes initial value public List. Item(String val) { // this refers to “current” object this. x = val; this. previous = this. next = null; } public boolean equals(List. Item c) { // two List. Items are equal if their string values // are equal and they point to the same objects return ( x. equals(c. x) && (previous == c. previous) && (next == c. next) ); } public void print. Item() { System. out. println(x); } }
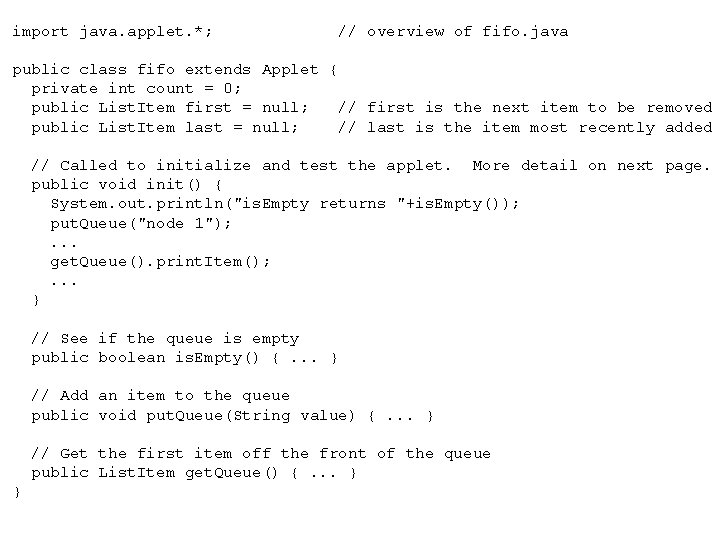
import java. applet. *; // overview of fifo. java public class fifo extends Applet { private int count = 0; public List. Item first = null; // first is the next item to be removed public List. Item last = null; // last is the item most recently added // Called to initialize and test the applet. More detail on next page. public void init() { System. out. println("is. Empty returns "+is. Empty()); put. Queue("node 1"); . . . get. Queue(). print. Item(); . . . } // See if the queue is empty public boolean is. Empty() {. . . } // Add an item to the queue public void put. Queue(String value) {. . . } // Get the first item off the front of the queue public List. Item get. Queue() {. . . } }
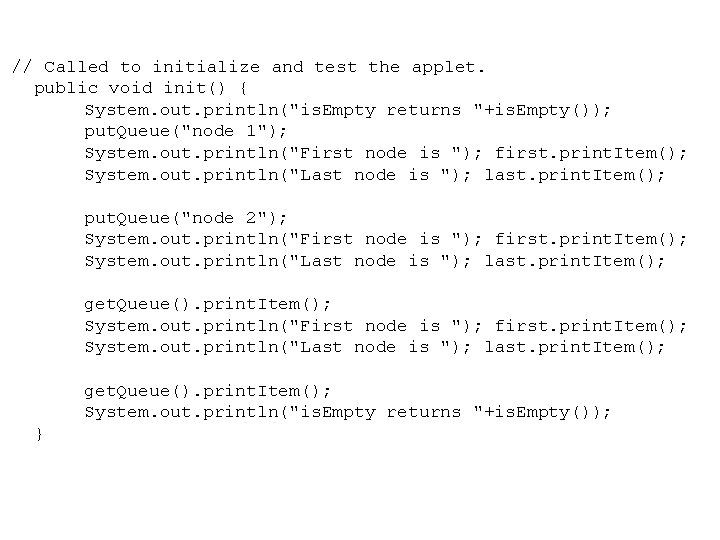
// Called to initialize and test the applet. public void init() { System. out. println("is. Empty returns "+is. Empty()); put. Queue("node 1"); System. out. println("First node is "); first. print. Item(); System. out. println("Last node is "); last. print. Item(); put. Queue("node 2"); System. out. println("First node is "); first. print. Item(); System. out. println("Last node is "); last. print. Item(); get. Queue(). print. Item(); System. out. println("is. Empty returns "+is. Empty()); }
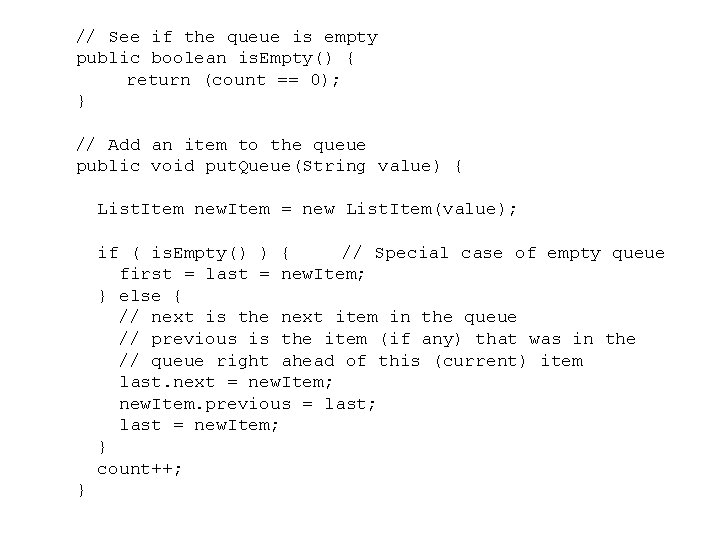
// See if the queue is empty public boolean is. Empty() { return (count == 0); } // Add an item to the queue public void put. Queue(String value) { List. Item new. Item = new List. Item(value); if ( is. Empty() ) { // Special case of empty queue first = last = new. Item; } else { // next is the next item in the queue // previous is the item (if any) that was in the // queue right ahead of this (current) item last. next = new. Item; new. Item. previous = last; last = new. Item; } count++; }
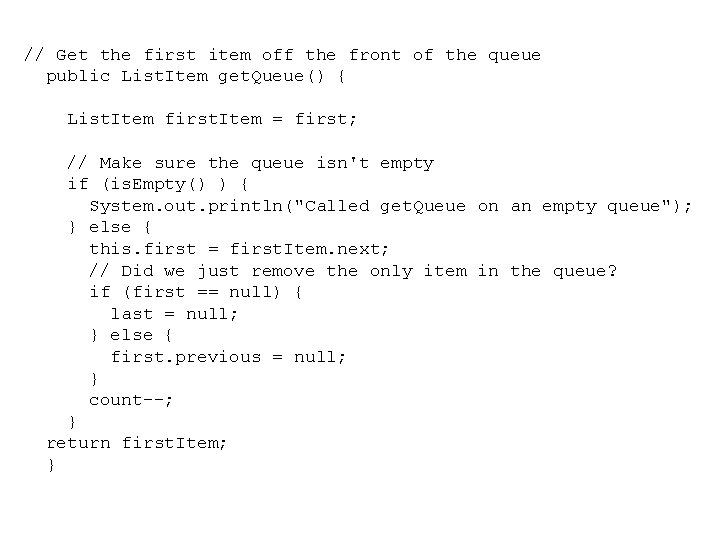
// Get the first item off the front of the queue public List. Item get. Queue() { List. Item first. Item = first; // Make sure the queue isn't empty if (is. Empty() ) { System. out. println("Called get. Queue on an empty queue"); } else { this. first = first. Item. next; // Did we just remove the only item in the queue? if (first == null) { last = null; } else { first. previous = null; } count--; } return first. Item; }
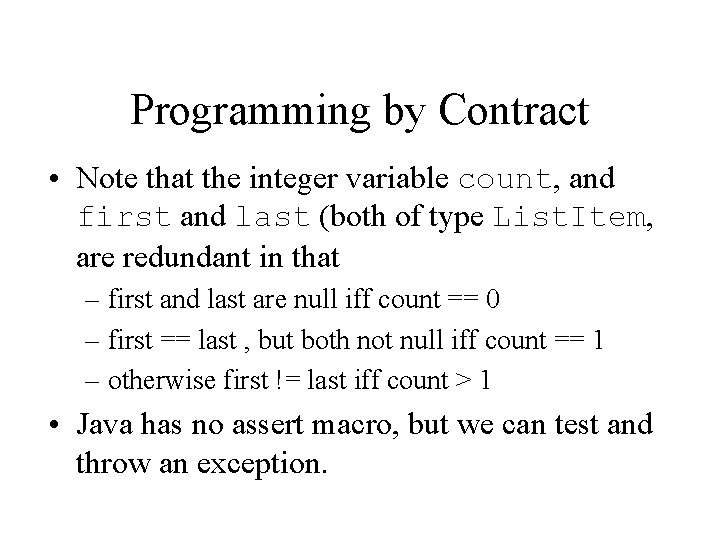
Programming by Contract • Note that the integer variable count, and first and last (both of type List. Item, are redundant in that – first and last are null iff count == 0 – first == last , but both not null iff count == 1 – otherwise first != last iff count > 1 • Java has no assert macro, but we can test and throw an exception.
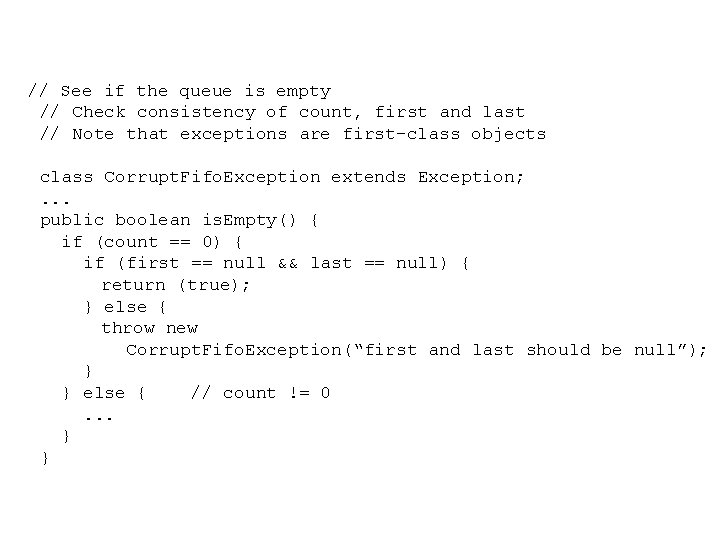
// See if the queue is empty // Check consistency of count, first and last // Note that exceptions are first-class objects class Corrupt. Fifo. Exception extends Exception; . . . public boolean is. Empty() { if (count == 0) { if (first == null && last == null) { return (true); } else { throw new Corrupt. Fifo. Exception(“first and last should be null”); } } else { // count != 0. . . } }
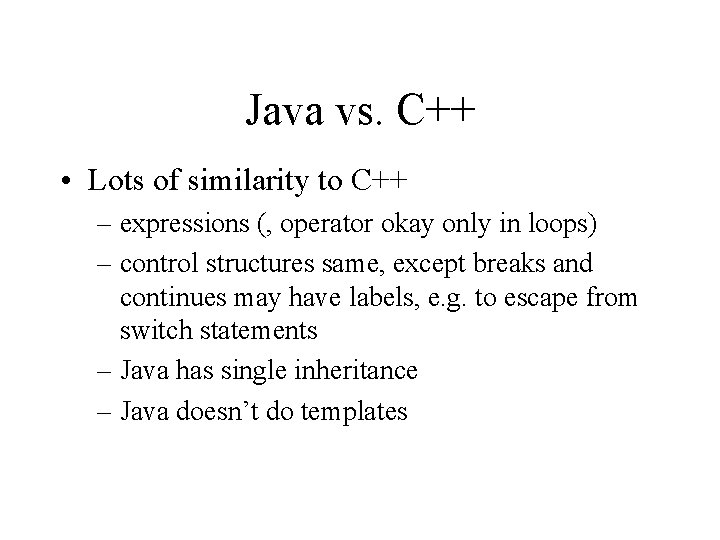
Java vs. C++ • Lots of similarity to C++ – expressions (, operator okay only in loops) – control structures same, except breaks and continues may have labels, e. g. to escape from switch statements – Java has single inheritance – Java doesn’t do templates
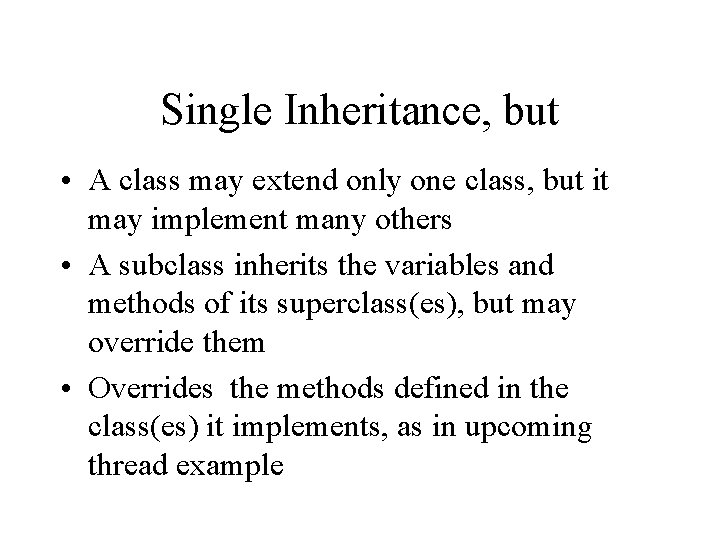
Single Inheritance, but • A class may extend only one class, but it may implement many others • A subclass inherits the variables and methods of its superclass(es), but may override them • Overrides the methods defined in the class(es) it implements, as in upcoming thread example
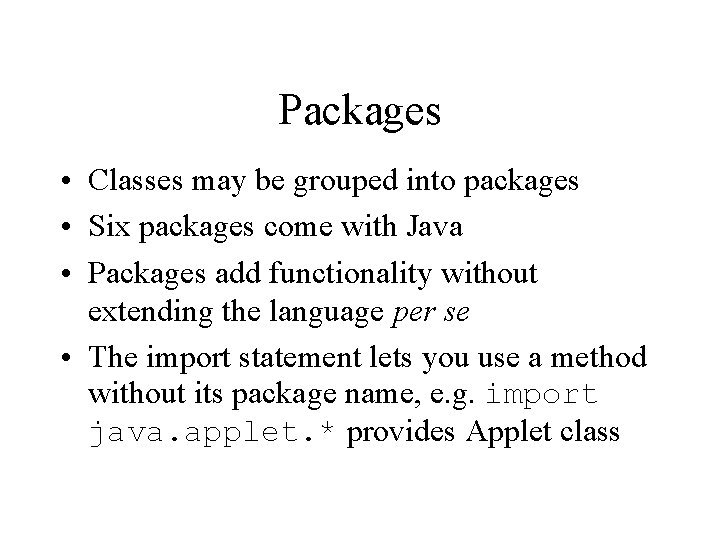
Packages • Classes may be grouped into packages • Six packages come with Java • Packages add functionality without extending the language per se • The import statement lets you use a method without its package name, e. g. import java. applet. * provides Applet class
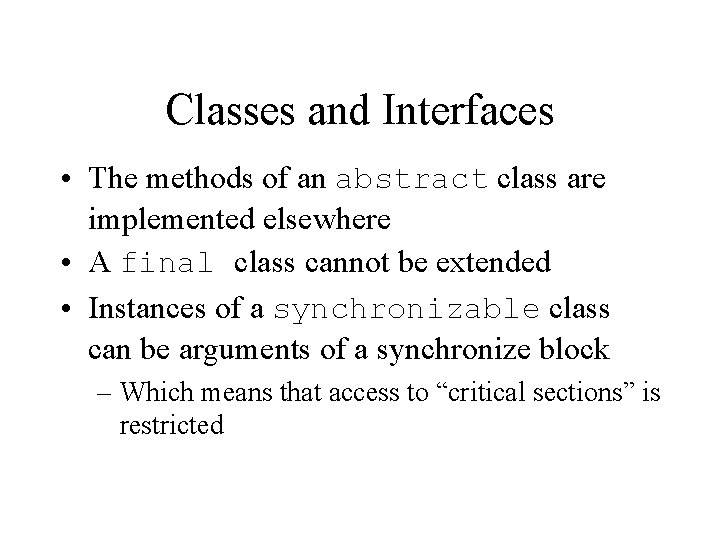
Classes and Interfaces • The methods of an abstract class are implemented elsewhere • A final class cannot be extended • Instances of a synchronizable class can be arguments of a synchronize block – Which means that access to “critical sections” is restricted
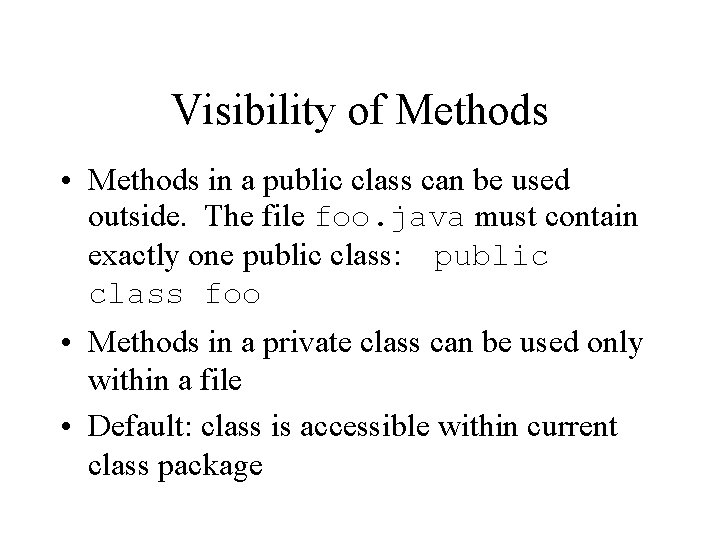
Visibility of Methods • Methods in a public class can be used outside. The file foo. java must contain exactly one public class: public class foo • Methods in a private class can be used only within a file • Default: class is accessible within current class package
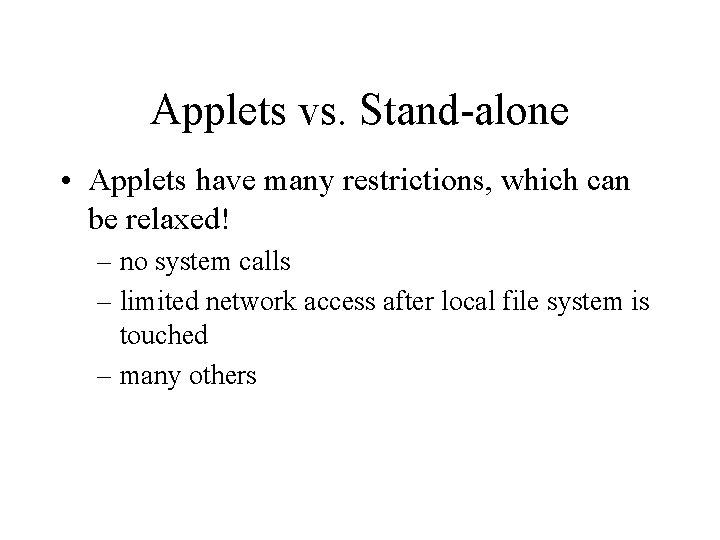
Applets vs. Stand-alone • Applets have many restrictions, which can be relaxed! – no system calls – limited network access after local file system is touched – many others
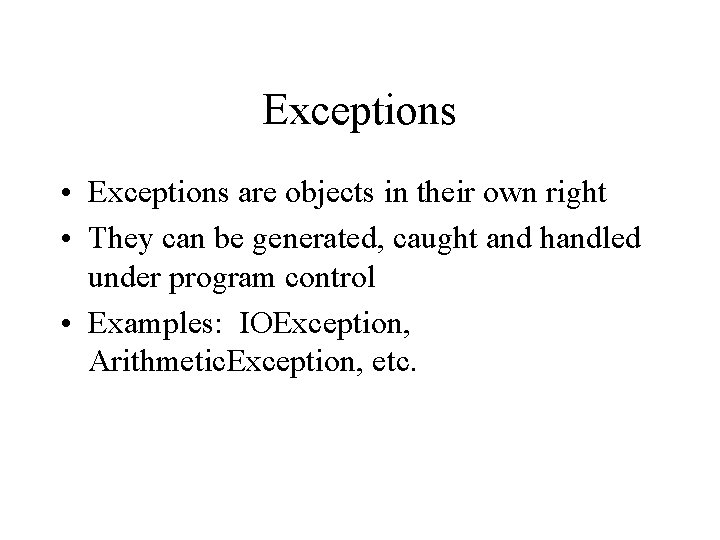
Exceptions • Exceptions are objects in their own right • They can be generated, caught and handled under program control • Examples: IOException, Arithmetic. Exception, etc.
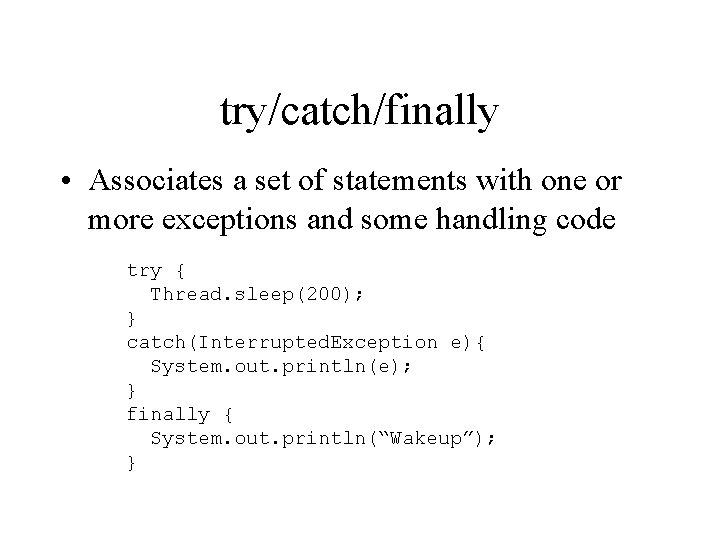
try/catch/finally • Associates a set of statements with one or more exceptions and some handling code try { Thread. sleep(200); } catch(Interrupted. Exception e){ System. out. println(e); } finally { System. out. println(“Wakeup”); }
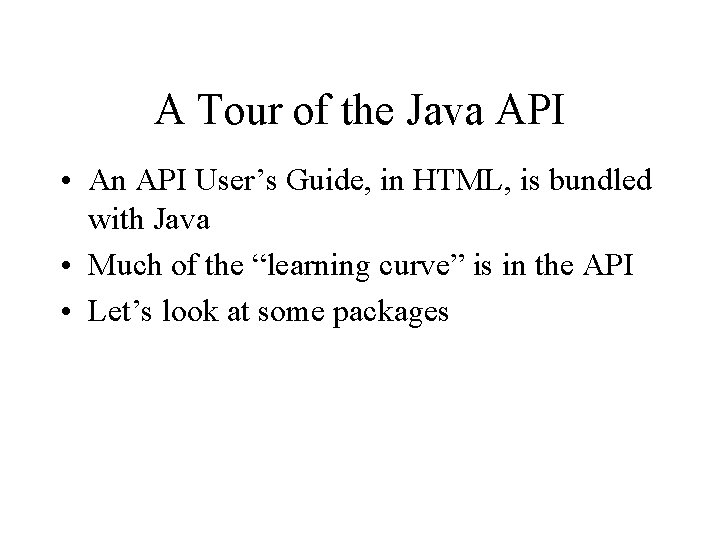
A Tour of the Java API • An API User’s Guide, in HTML, is bundled with Java • Much of the “learning curve” is in the API • Let’s look at some packages
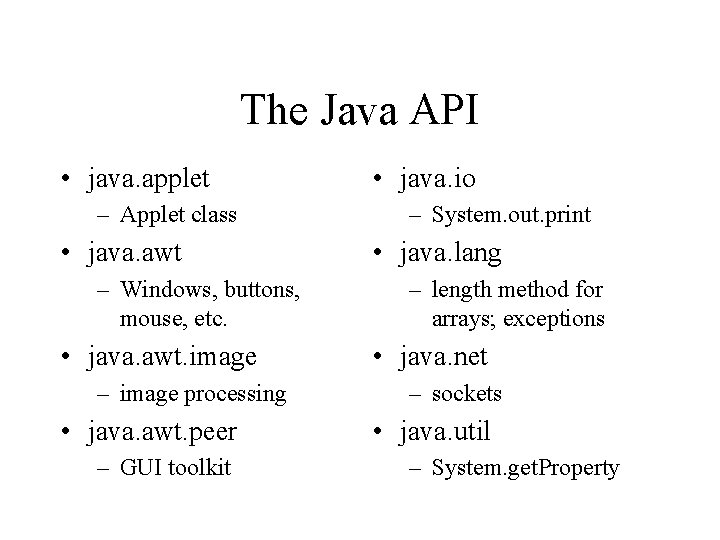
The Java API • java. applet – Applet class • java. awt – Windows, buttons, mouse, etc. • java. awt. image – image processing • java. awt. peer – GUI toolkit • java. io – System. out. print • java. lang – length method for arrays; exceptions • java. net – sockets • java. util – System. get. Property
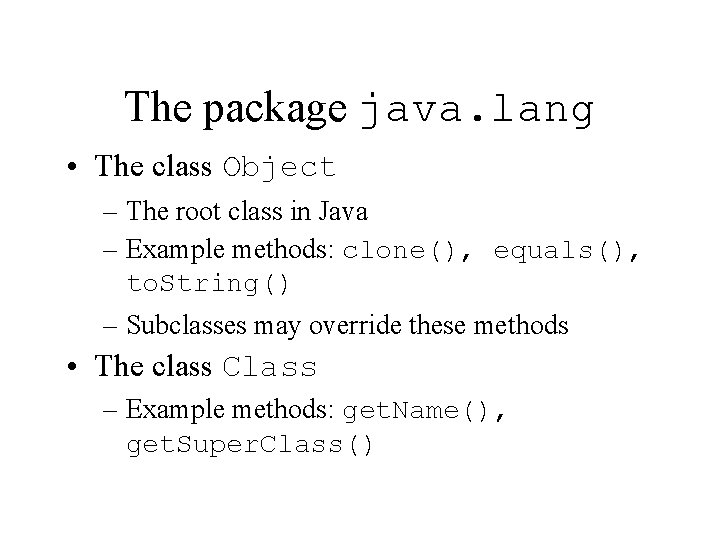
The package java. lang • The class Object – The root class in Java – Example methods: clone(), equals(), to. String() – Subclasses may override these methods • The class Class – Example methods: get. Name(), get. Super. Class()
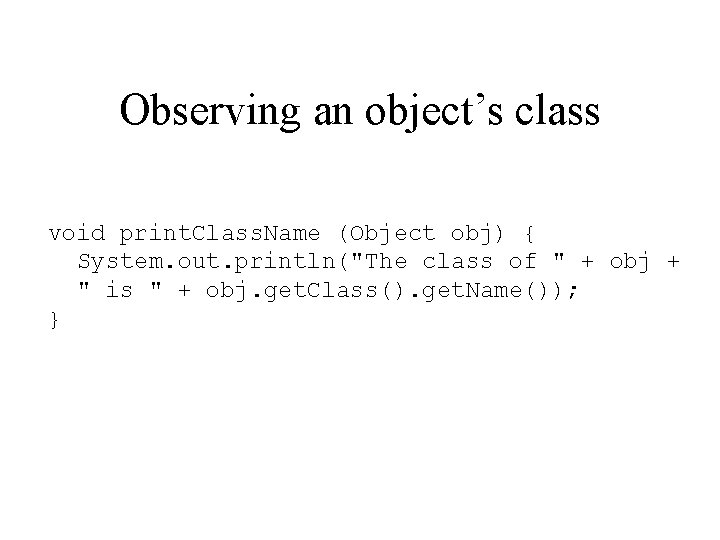
Observing an object’s class void print. Class. Name (Object obj) { System. out. println("The class of " + obj + " is " + obj. get. Class(). get. Name()); }
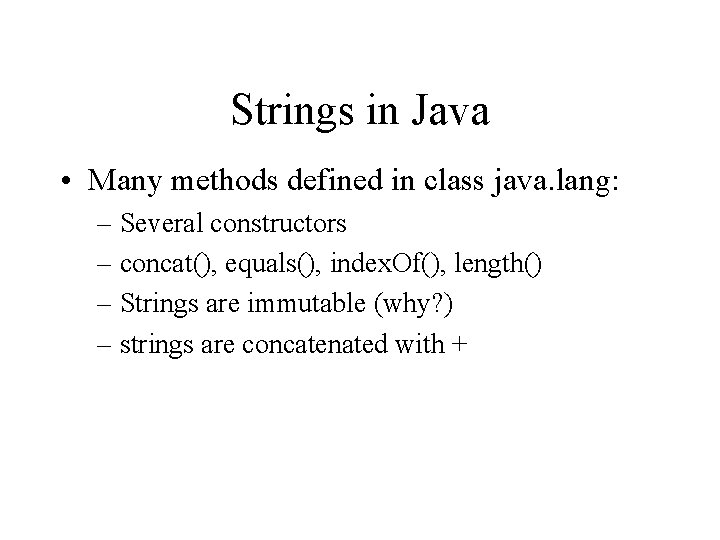
Strings in Java • Many methods defined in class java. lang: – Several constructors – concat(), equals(), index. Of(), length() – Strings are immutable (why? ) – strings are concatenated with +
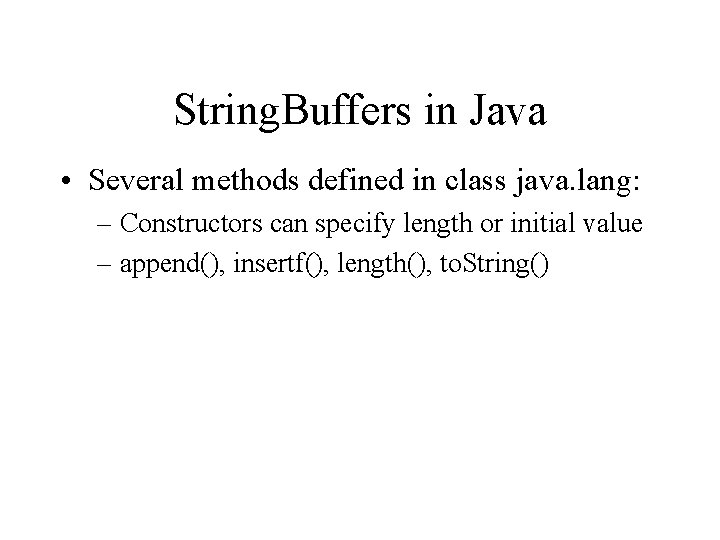
String. Buffers in Java • Several methods defined in class java. lang: – Constructors can specify length or initial value – append(), insertf(), length(), to. String()
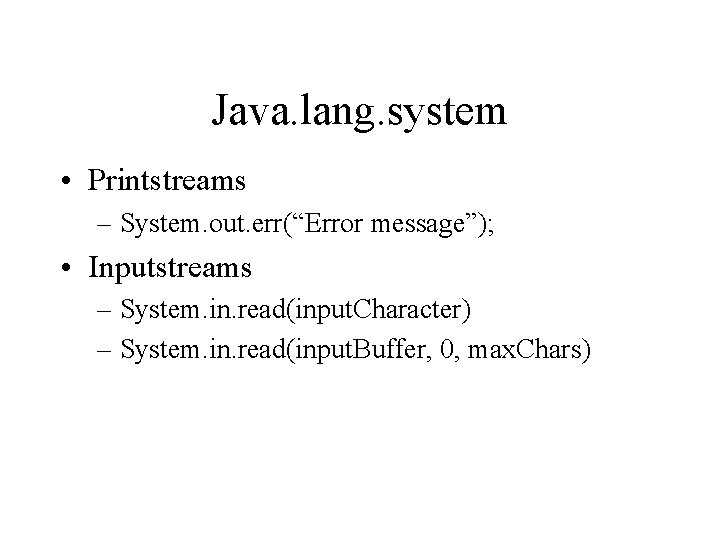
Java. lang. system • Printstreams – System. out. err(“Error message”); • Inputstreams – System. in. read(input. Character) – System. in. read(input. Buffer, 0, max. Chars)
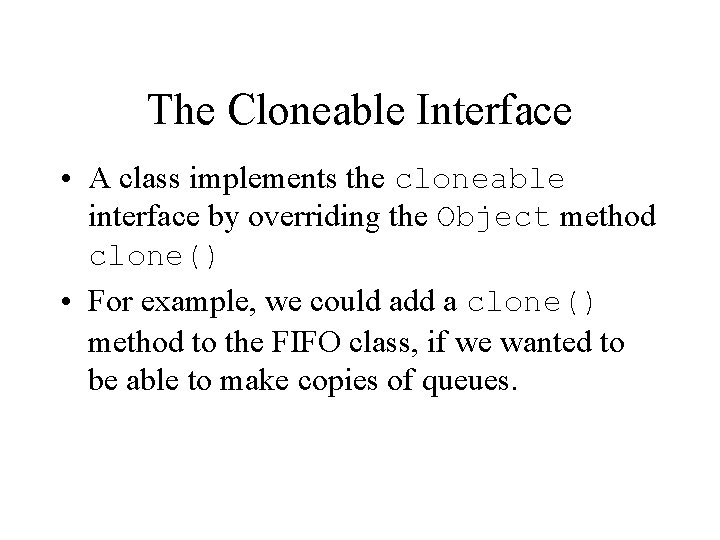
The Cloneable Interface • A class implements the cloneable interface by overriding the Object method clone() • For example, we could add a clone() method to the FIFO class, if we wanted to be able to make copies of queues.
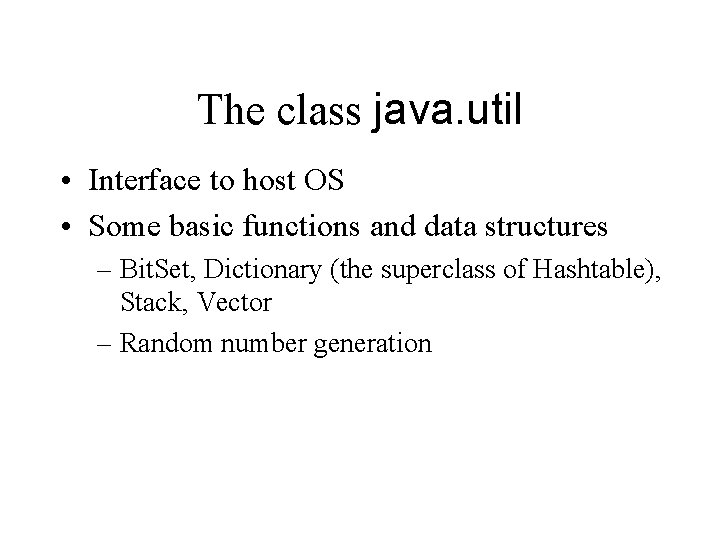
The class java. util • Interface to host OS • Some basic functions and data structures – Bit. Set, Dictionary (the superclass of Hashtable), Stack, Vector – Random number generation
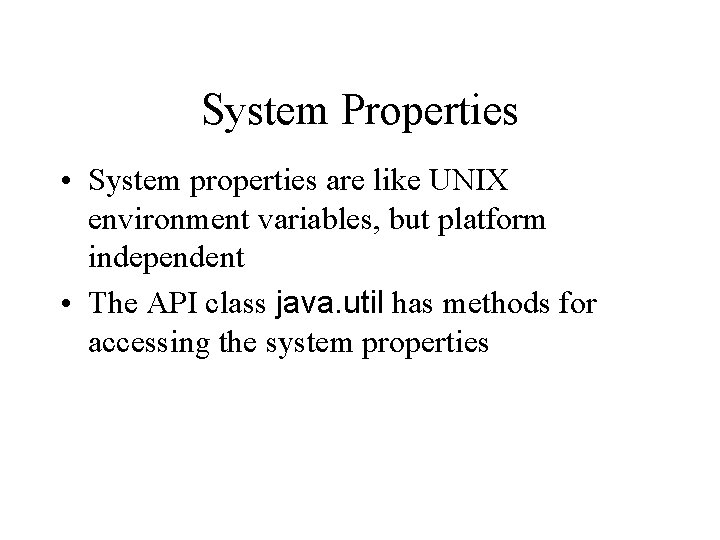
System Properties • System properties are like UNIX environment variables, but platform independent • The API class java. util has methods for accessing the system properties
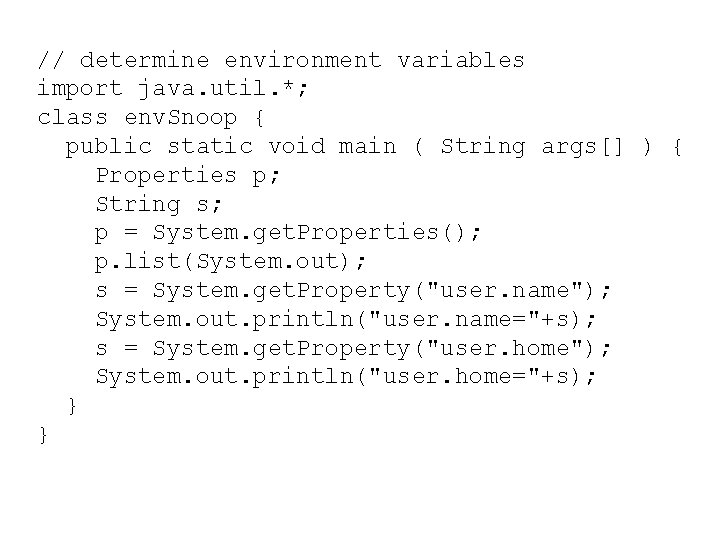
// determine environment variables import java. util. *; class env. Snoop { public static void main ( String args[] ) { Properties p; String s; p = System. get. Properties(); p. list(System. out); s = System. get. Property("user. name"); System. out. println("user. name="+s); s = System. get. Property("user. home"); System. out. println("user. home="+s); } }
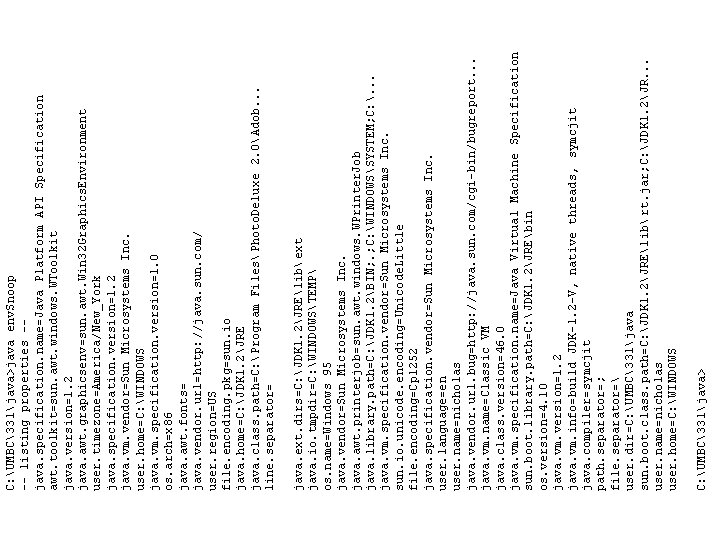
C: UMBC331java> java. ext. dirs=C: JDK 1. 2JRElibext java. io. tmpdir=C: WINDOWSTEMP os. name=Windows 95 java. vendor=Sun Microsystems Inc. java. awt. printerjob=sun. awt. windows. WPrinter. Job java. library. path=C: JDK 1. 2BIN; . ; C: WINDOWSSYSTEM; C: . . . java. vm. specification. vendor=Sun Microsystems Inc. sun. io. unicode. encoding=Unicode. Little file. encoding=Cp 1252 java. specification. vendor=Sun Microsystems Inc. user. language=en user. name=nicholas java. vendor. url. bug=http: //java. sun. com/cgi-bin/bugreport. . . java. vm. name=Classic VM java. class. version=46. 0 java. vm. specification. name=Java Virtual Machine Specification sun. boot. library. path=C: JDK 1. 2JREbin os. version=4. 10 java. vm. version=1. 2 java. vm. info=build JDK-1. 2 -V, native threads, symcjit java. compiler=symcjit path. separator=; file. separator= user. dir=C: UMBC331java sun. boot. class. path=C: JDK 1. 2JRElib rt. jar; C: JDK 1. 2JR. . . user. name=nicholas user. home=C: WINDOWS C: UMBC331java>java env. Snoop -- listing properties -java. specification. name=Java Platform API Specification awt. toolkit=sun. awt. windows. WToolkit java. version=1. 2 java. awt. graphicsenv=sun. awt. Win 32 Graphics. Environment user. timezone=America/New_York java. specification. version=1. 2 java. vm. vendor=Sun Microsystems Inc. user. home=C: WINDOWS java. vm. specification. version=1. 0 os. arch=x 86 java. awt. fonts= java. vendor. url=http: //java. sun. com/ user. region=US file. encoding. pkg=sun. io java. home=C: JDK 1. 2JRE java. class. path=C: Program Files Photo. Deluxe 2. 0Adob. . . line. separator=
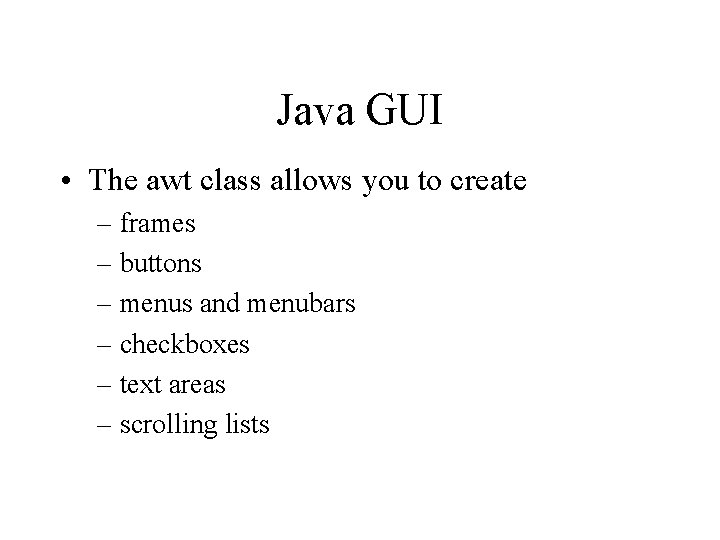
Java GUI • The awt class allows you to create – frames – buttons – menus and menubars – checkboxes – text areas – scrolling lists
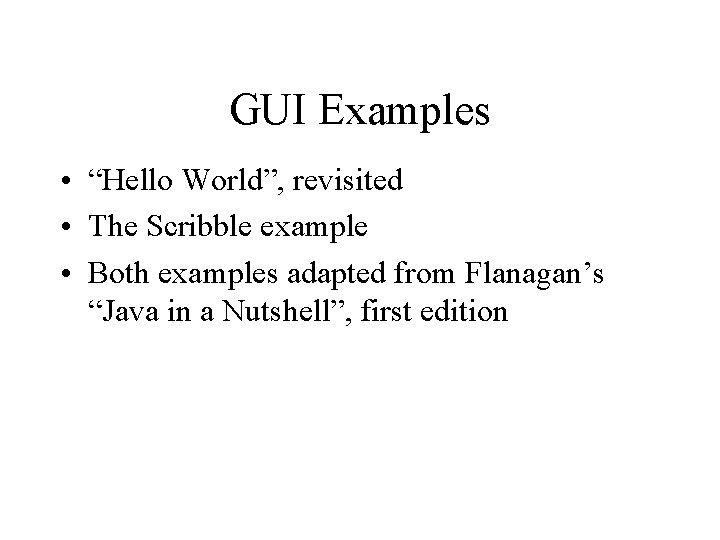
GUI Examples • “Hello World”, revisited • The Scribble example • Both examples adapted from Flanagan’s “Java in a Nutshell”, first edition
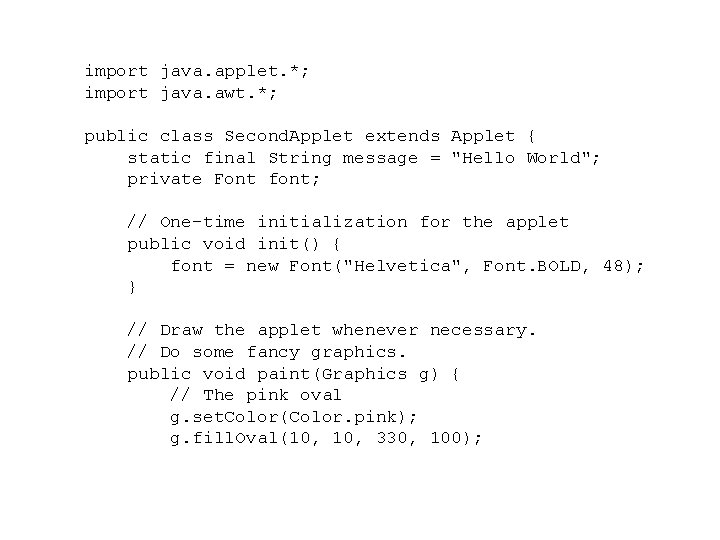
import java. applet. *; import java. awt. *; public class Second. Applet extends Applet { static final String message = "Hello World"; private Font font; // One-time initialization for the applet public void init() { font = new Font("Helvetica", Font. BOLD, 48); } // Draw the applet whenever necessary. // Do some fancy graphics. public void paint(Graphics g) { // The pink oval g. set. Color(Color. pink); g. fill. Oval(10, 330, 100);
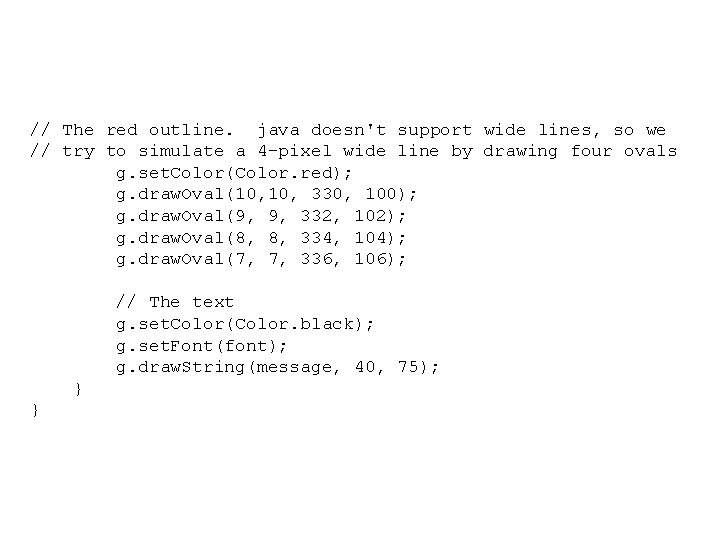
// The red outline. java doesn't support wide lines, so we // try to simulate a 4 -pixel wide line by drawing four ovals g. set. Color(Color. red); g. draw. Oval(10, 330, 100); g. draw. Oval(9, 9, 332, 102); g. draw. Oval(8, 8, 334, 104); g. draw. Oval(7, 7, 336, 106); // The text g. set. Color(Color. black); g. set. Font(font); g. draw. String(message, 40, 75); } }
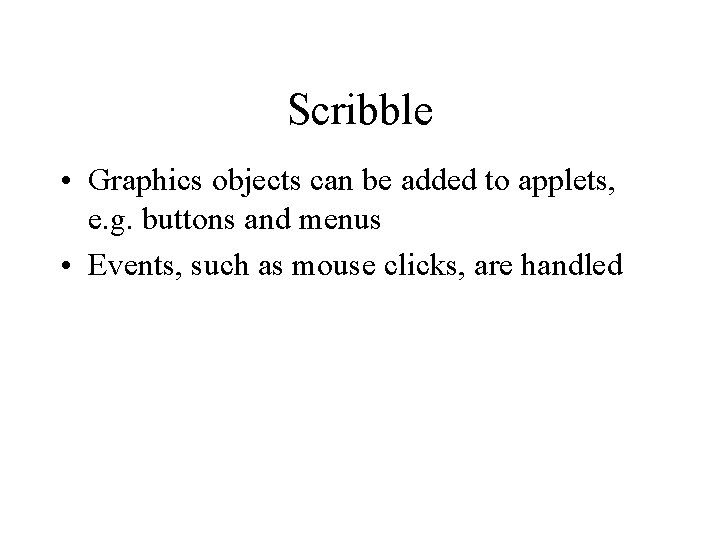
Scribble • Graphics objects can be added to applets, e. g. buttons and menus • Events, such as mouse clicks, are handled
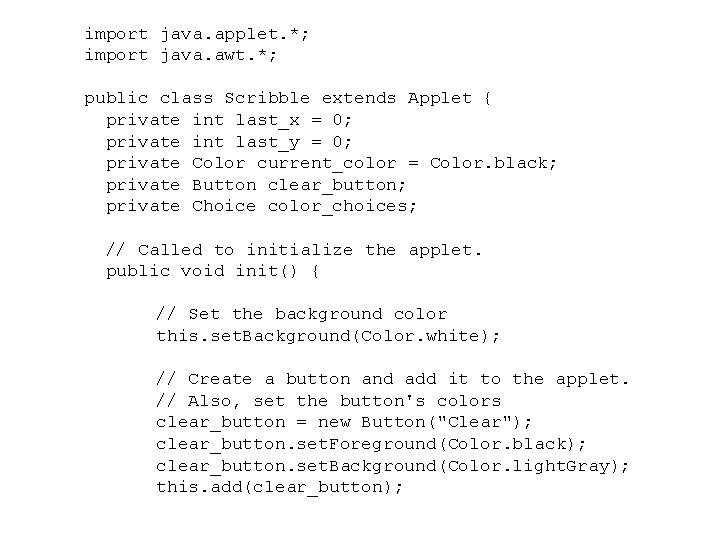
import java. applet. *; import java. awt. *; public class Scribble extends Applet { private int last_x = 0; private int last_y = 0; private Color current_color = Color. black; private Button clear_button; private Choice color_choices; // Called to initialize the applet. public void init() { // Set the background color this. set. Background(Color. white); // Create a button and add it to the applet. // Also, set the button's colors clear_button = new Button("Clear"); clear_button. set. Foreground(Color. black); clear_button. set. Background(Color. light. Gray); this. add(clear_button);
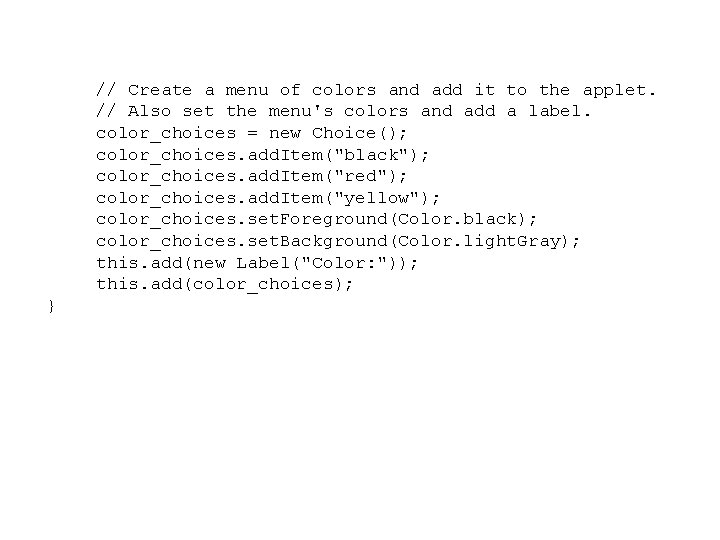
// Create a menu of colors and add it to the applet. // Also set the menu's colors and add a label. color_choices = new Choice(); color_choices. add. Item("black"); color_choices. add. Item("red"); color_choices. add. Item("yellow"); color_choices. set. Foreground(Color. black); color_choices. set. Background(Color. light. Gray); this. add(new Label("Color: ")); this. add(color_choices); }
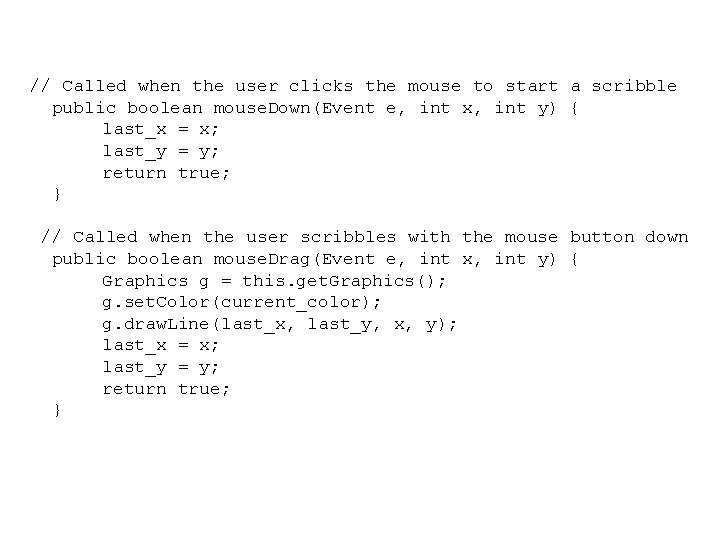
// Called when the user clicks the mouse to start a scribble public boolean mouse. Down(Event e, int x, int y) { last_x = x; last_y = y; return true; } // Called when the user scribbles with the mouse button down public boolean mouse. Drag(Event e, int x, int y) { Graphics g = this. get. Graphics(); g. set. Color(current_color); g. draw. Line(last_x, last_y, x, y); last_x = x; last_y = y; return true; }
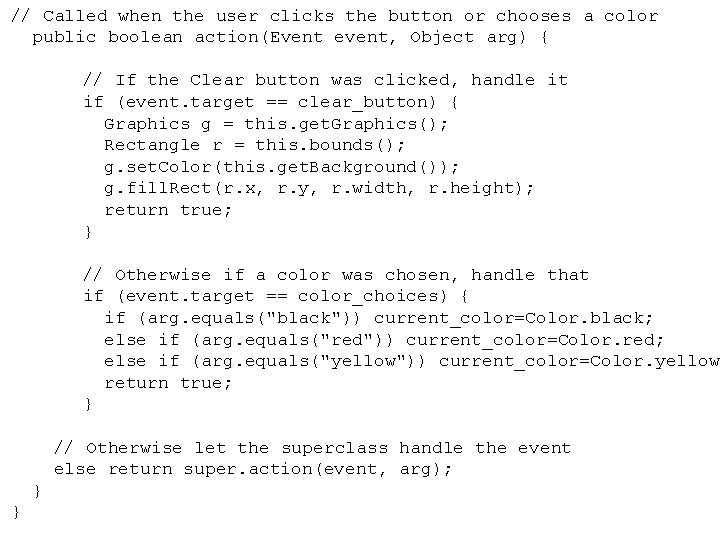
// Called when the user clicks the button or chooses a color public boolean action(Event event, Object arg) { // If the Clear button was clicked, handle it if (event. target == clear_button) { Graphics g = this. get. Graphics(); Rectangle r = this. bounds(); g. set. Color(this. get. Background()); g. fill. Rect(r. x, r. y, r. width, r. height); return true; } // Otherwise if a color was chosen, handle that if (event. target == color_choices) { if (arg. equals("black")) current_color=Color. black; else if (arg. equals("red")) current_color=Color. red; else if (arg. equals("yellow")) current_color=Color. yellow; return true; } // Otherwise let the superclass handle the event else return super. action(event, arg); } }
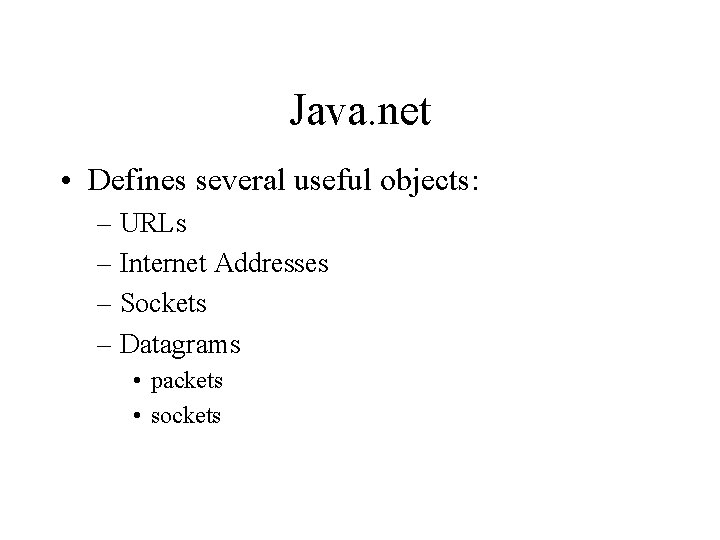
Java. net • Defines several useful objects: – URLs – Internet Addresses – Sockets – Datagrams • packets • sockets
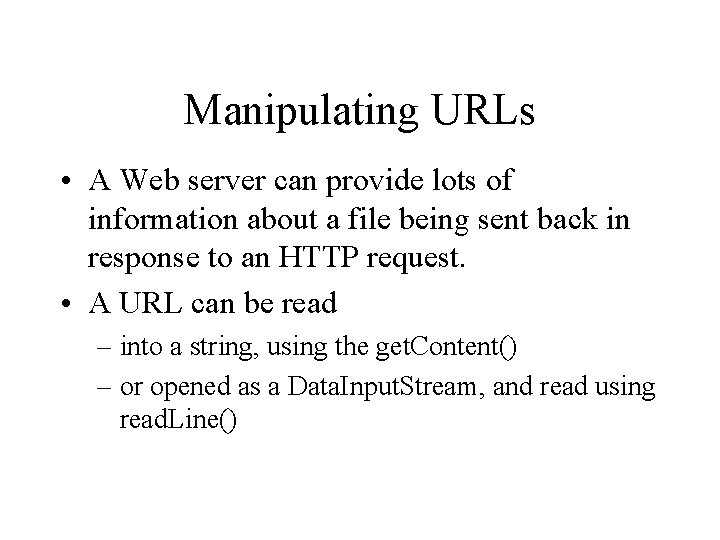
Manipulating URLs • A Web server can provide lots of information about a file being sent back in response to an HTTP request. • A URL can be read – into a string, using the get. Content() – or opened as a Data. Input. Stream, and read using read. Line()
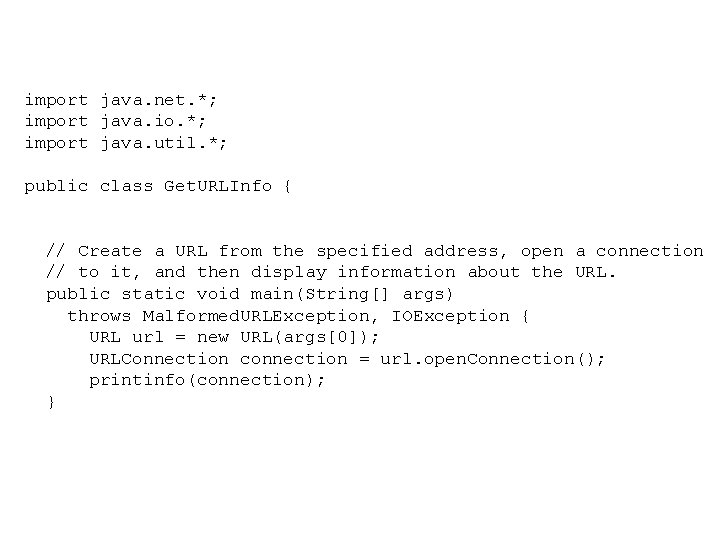
import java. net. *; import java. io. *; import java. util. *; public class Get. URLInfo { // Create a URL from the specified address, open a connection // to it, and then display information about the URL. public static void main(String[] args) throws Malformed. URLException, IOException { URL url = new URL(args[0]); URLConnection connection = url. open. Connection(); printinfo(connection); }
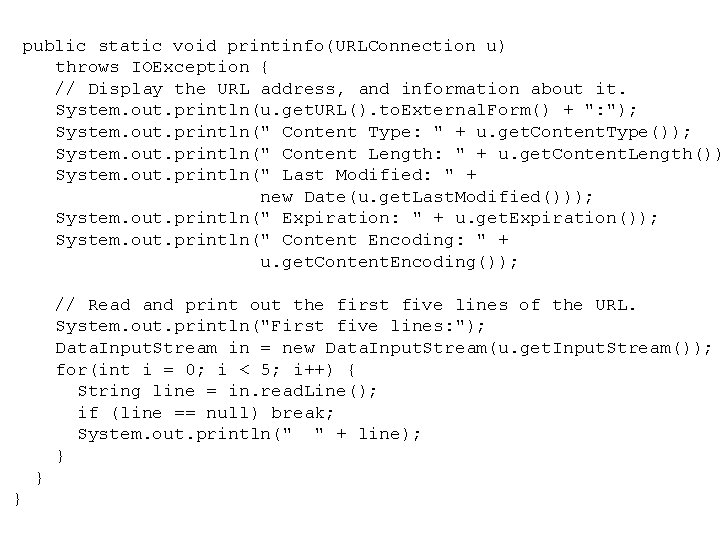
public static void printinfo(URLConnection u) throws IOException { // Display the URL address, and information about it. System. out. println(u. get. URL(). to. External. Form() + ": "); System. out. println(" Content Type: " + u. get. Content. Type()); System. out. println(" Content Length: " + u. get. Content. Length()) System. out. println(" Last Modified: " + new Date(u. get. Last. Modified())); System. out. println(" Expiration: " + u. get. Expiration()); System. out. println(" Content Encoding: " + u. get. Content. Encoding()); // Read and print out the first five lines of the URL. System. out. println("First five lines: "); Data. Input. Stream in = new Data. Input. Stream(u. get. Input. Stream()); for(int i = 0; i < 5; i++) { String line = in. read. Line(); if (line == null) break; System. out. println(" " + line); } } }
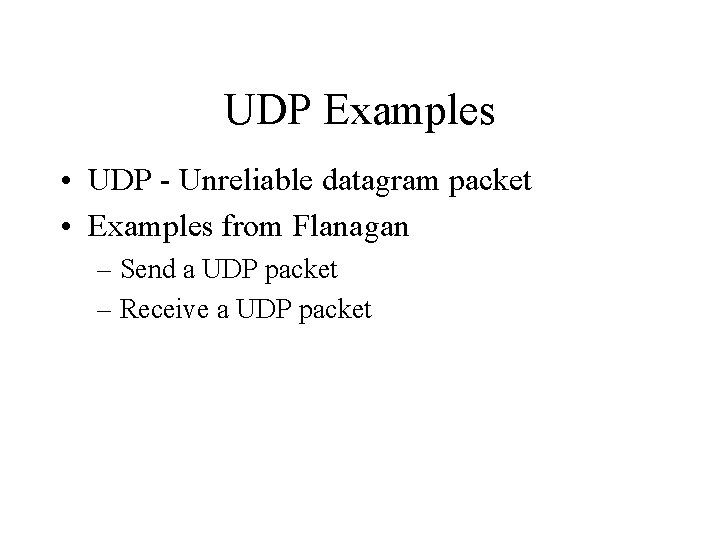
UDP Examples • UDP - Unreliable datagram packet • Examples from Flanagan – Send a UDP packet – Receive a UDP packet
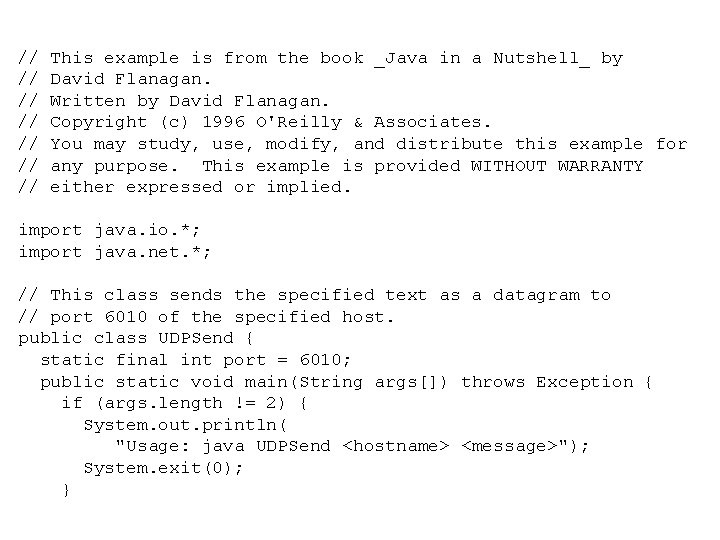
// // This example is from the book _Java in a Nutshell_ by David Flanagan. Written by David Flanagan. Copyright (c) 1996 O'Reilly & Associates. You may study, use, modify, and distribute this example for any purpose. This example is provided WITHOUT WARRANTY either expressed or implied. import java. io. *; import java. net. *; // This class sends the specified text as a datagram to // port 6010 of the specified host. public class UDPSend { static final int port = 6010; public static void main(String args[]) throws Exception { if (args. length != 2) { System. out. println( "Usage: java UDPSend <hostname> <message>"); System. exit(0); }
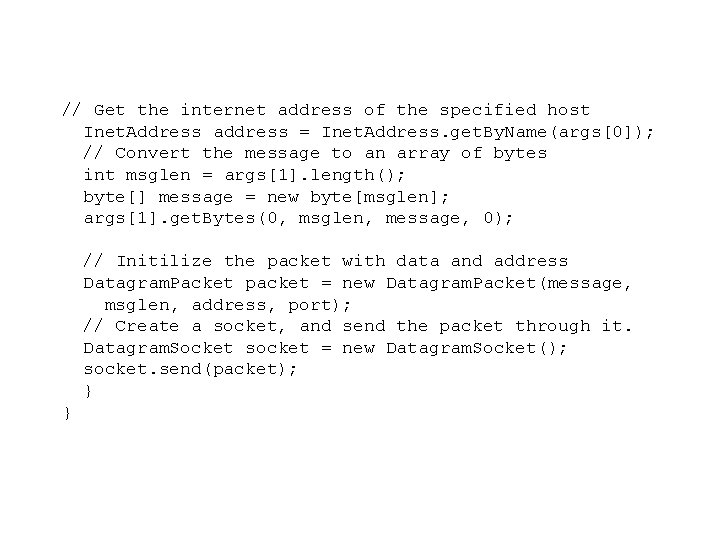
// Get the internet address of the specified host Inet. Address address = Inet. Address. get. By. Name(args[0]); // Convert the message to an array of bytes int msglen = args[1]. length(); byte[] message = new byte[msglen]; args[1]. get. Bytes(0, msglen, message, 0); // Initilize the packet with data and address Datagram. Packet packet = new Datagram. Packet(message, msglen, address, port); // Create a socket, and send the packet through it. Datagram. Socket socket = new Datagram. Socket(); socket. send(packet); } }
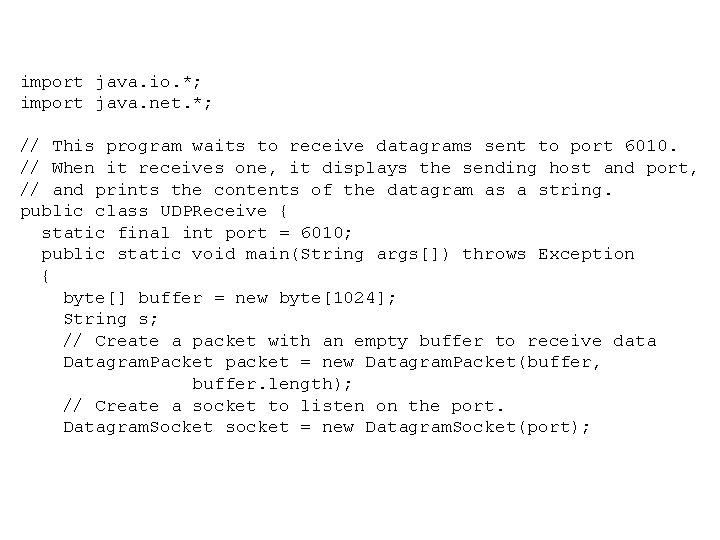
import java. io. *; import java. net. *; // This program waits to receive datagrams sent to port 6010. // When it receives one, it displays the sending host and port, // and prints the contents of the datagram as a string. public class UDPReceive { static final int port = 6010; public static void main(String args[]) throws Exception { byte[] buffer = new byte[1024]; String s; // Create a packet with an empty buffer to receive data Datagram. Packet packet = new Datagram. Packet(buffer, buffer. length); // Create a socket to listen on the port. Datagram. Socket socket = new Datagram. Socket(port);
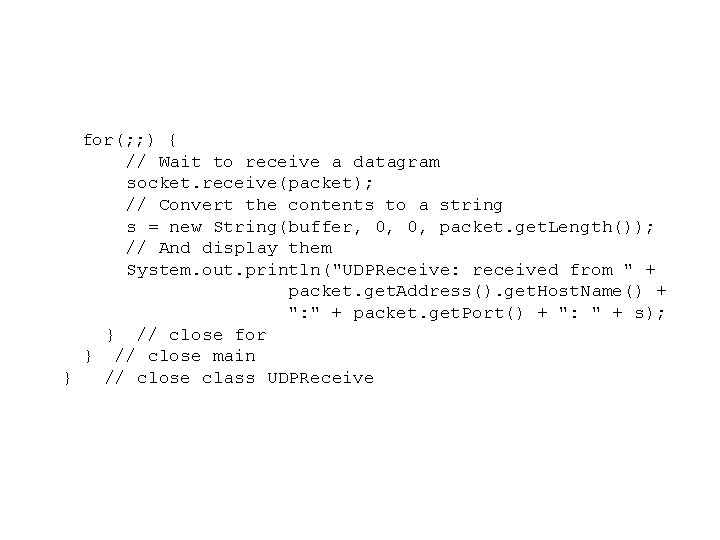
for(; ; ) { // Wait to receive a datagram socket. receive(packet); // Convert the contents to a string s = new String(buffer, 0, 0, packet. get. Length()); // And display them System. out. println("UDPReceive: received from " + packet. get. Address(). get. Host. Name() + ": " + packet. get. Port() + ": " + s); } // close for } // close main } // close class UDPReceive
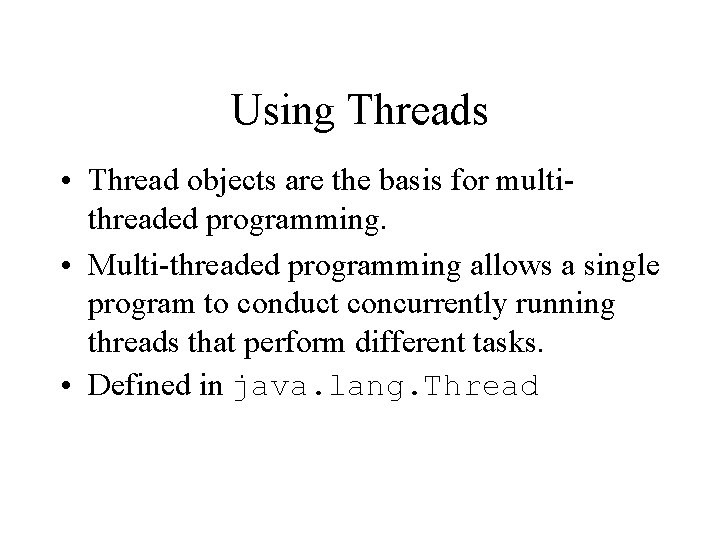
Using Threads • Thread objects are the basis for multithreaded programming. • Multi-threaded programming allows a single program to conduct concurrently running threads that perform different tasks. • Defined in java. lang. Thread
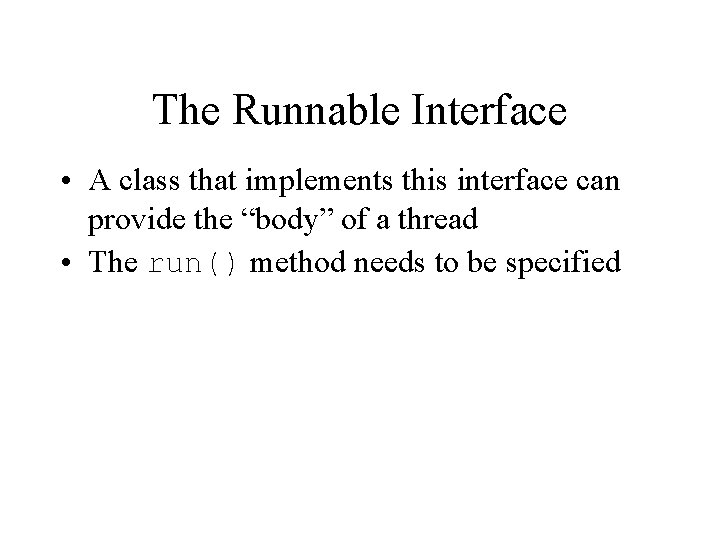
The Runnable Interface • A class that implements this interface can provide the “body” of a thread • The run() method needs to be specified
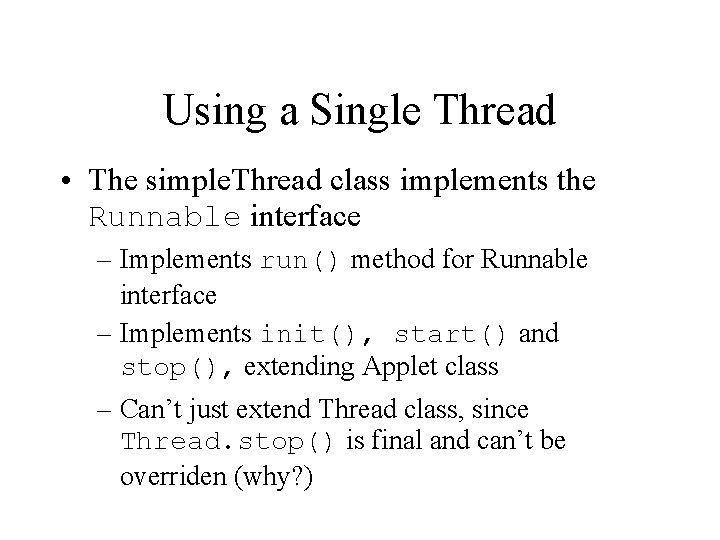
Using a Single Thread • The simple. Thread class implements the Runnable interface – Implements run() method for Runnable interface – Implements init(), start() and stop(), extending Applet class – Can’t just extend Thread class, since Thread. stop() is final and can’t be overriden (why? )
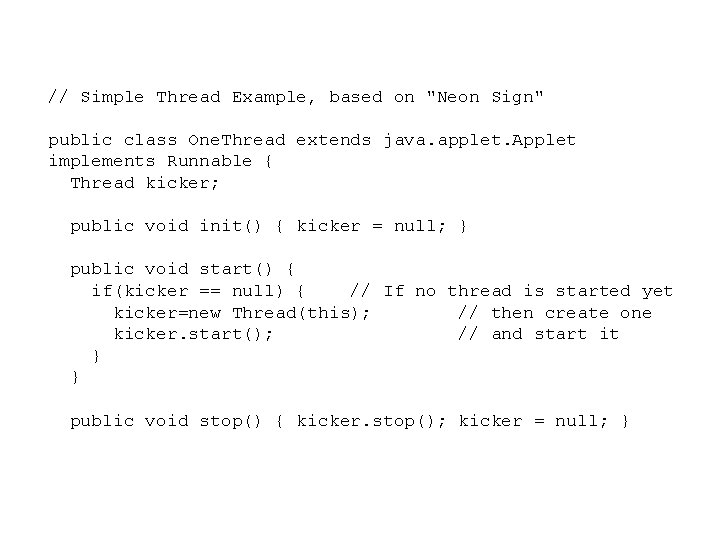
// Simple Thread Example, based on "Neon Sign" public class One. Thread extends java. applet. Applet implements Runnable { Thread kicker; public void init() { kicker = null; } public void start() { if(kicker == null) { // If no thread is started yet kicker=new Thread(this); // then create one kicker. start(); // and start it } } public void stop() { kicker. stop(); kicker = null; }
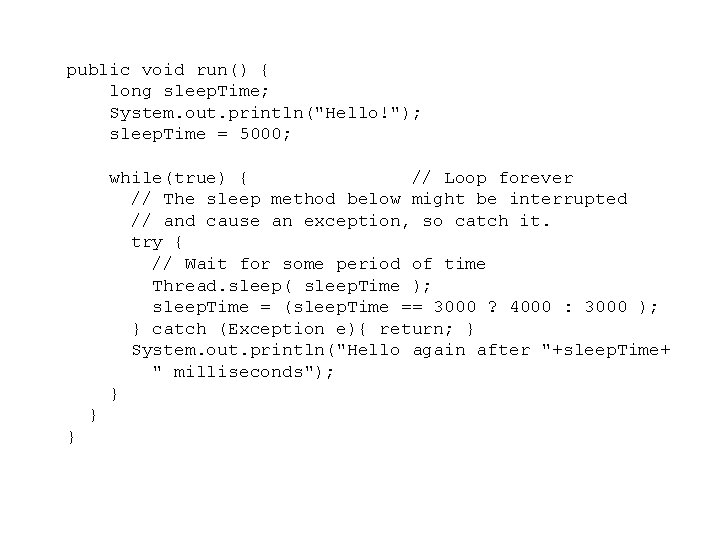
public void run() { long sleep. Time; System. out. println("Hello!"); sleep. Time = 5000; while(true) { // Loop forever // The sleep method below might be interrupted // and cause an exception, so catch it. try { // Wait for some period of time Thread. sleep( sleep. Time ); sleep. Time = (sleep. Time == 3000 ? 4000 : 3000 ); } catch (Exception e){ return; } System. out. println("Hello again after "+sleep. Time+ " milliseconds"); } } }
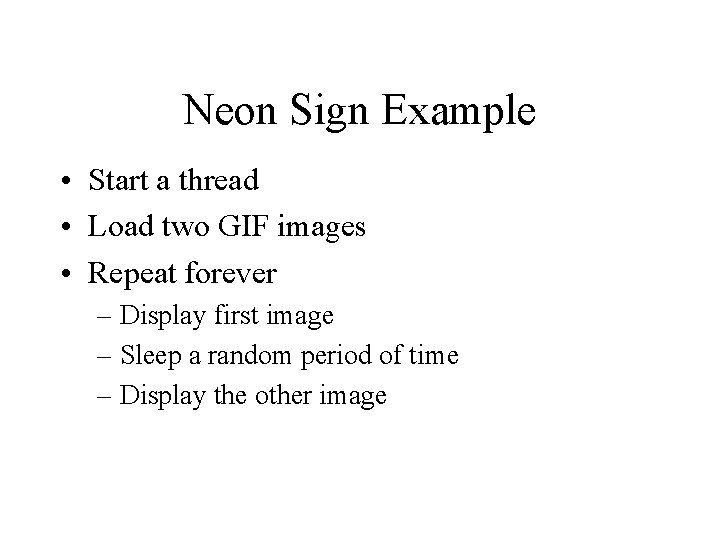
Neon Sign Example • Start a thread • Load two GIF images • Repeat forever – Display first image – Sleep a random period of time – Display the other image
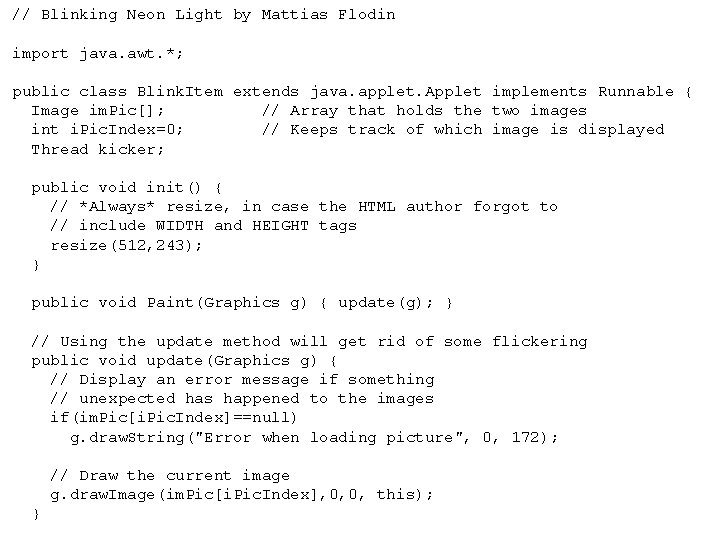
// Blinking Neon Light by Mattias Flodin import java. awt. *; public class Blink. Item extends java. applet. Applet implements Runnable { Image im. Pic[]; // Array that holds the two images int i. Pic. Index=0; // Keeps track of which image is displayed Thread kicker; public void init() { // *Always* resize, in case the HTML author forgot to // include WIDTH and HEIGHT tags resize(512, 243); } public void Paint(Graphics g) { update(g); } // Using the update method will get rid of some flickering public void update(Graphics g) { // Display an error message if something // unexpected has happened to the images if(im. Pic[i. Pic. Index]==null) g. draw. String("Error when loading picture", 0, 172); // Draw the current image g. draw. Image(im. Pic[i. Pic. Index], 0, 0, this); }
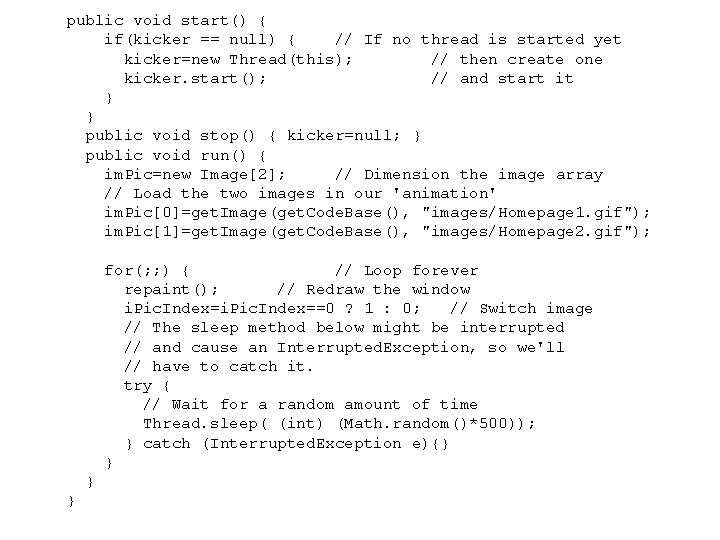
public void start() { if(kicker == null) { // If no thread is started yet kicker=new Thread(this); // then create one kicker. start(); // and start it } } public void stop() { kicker=null; } public void run() { im. Pic=new Image[2]; // Dimension the image array // Load the two images in our 'animation' im. Pic[0]=get. Image(get. Code. Base(), "images/Homepage 1. gif"); im. Pic[1]=get. Image(get. Code. Base(), "images/Homepage 2. gif"); for(; ; ) { // Loop forever repaint(); // Redraw the window i. Pic. Index==0 ? 1 : 0; // Switch image // The sleep method below might be interrupted // and cause an Interrupted. Exception, so we'll // have to catch it. try { // Wait for a random amount of time Thread. sleep( (int) (Math. random()*500)); } catch (Interrupted. Exception e){} }
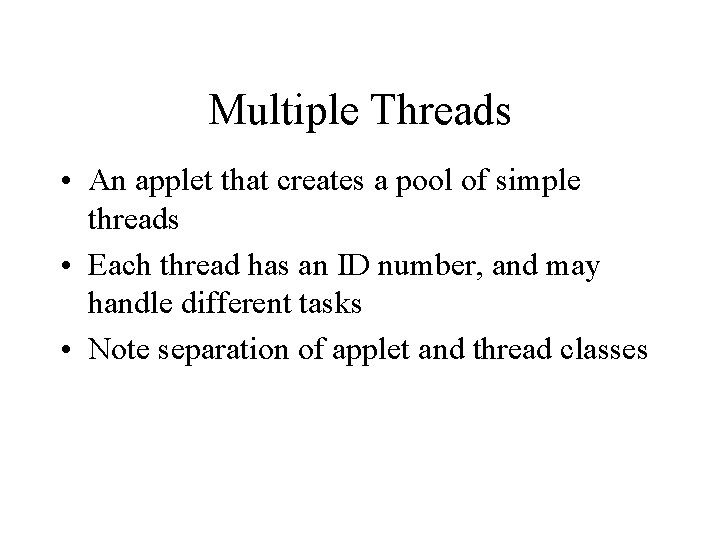
Multiple Threads • An applet that creates a pool of simple threads • Each thread has an ID number, and may handle different tasks • Note separation of applet and thread classes
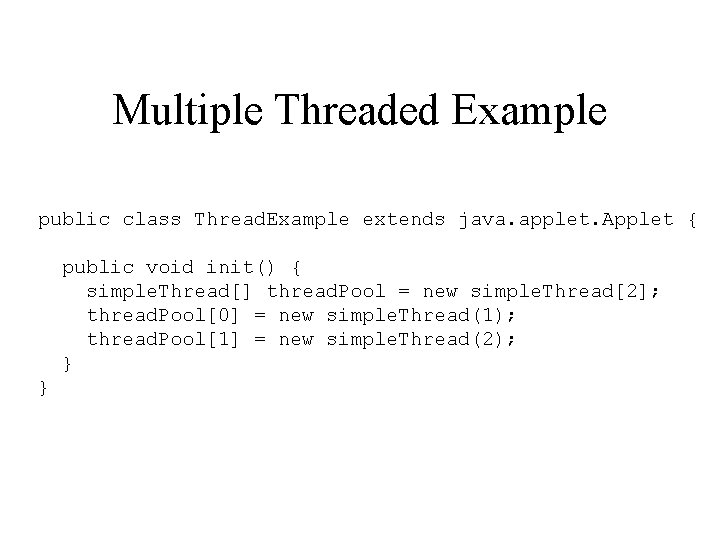
Multiple Threaded Example public class Thread. Example extends java. applet. Applet { public void init() { simple. Thread[] thread. Pool = new simple. Thread[2]; thread. Pool[0] = new simple. Thread(1); thread. Pool[1] = new simple. Thread(2); } }
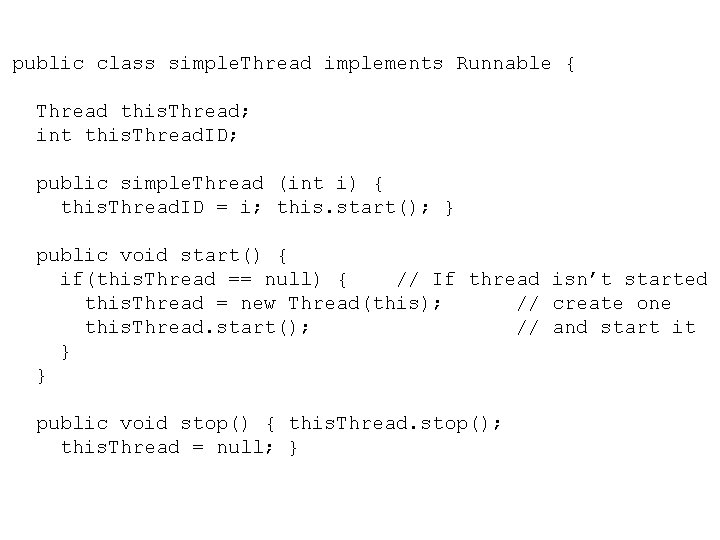
public class simple. Thread implements Runnable { Thread this. Thread; int this. Thread. ID; public simple. Thread (int i) { this. Thread. ID = i; this. start(); } public void start() { if(this. Thread == null) { // If thread isn’t started this. Thread = new Thread(this); // create one this. Thread. start(); // and start it } } public void stop() { this. Thread. stop(); this. Thread = null; }
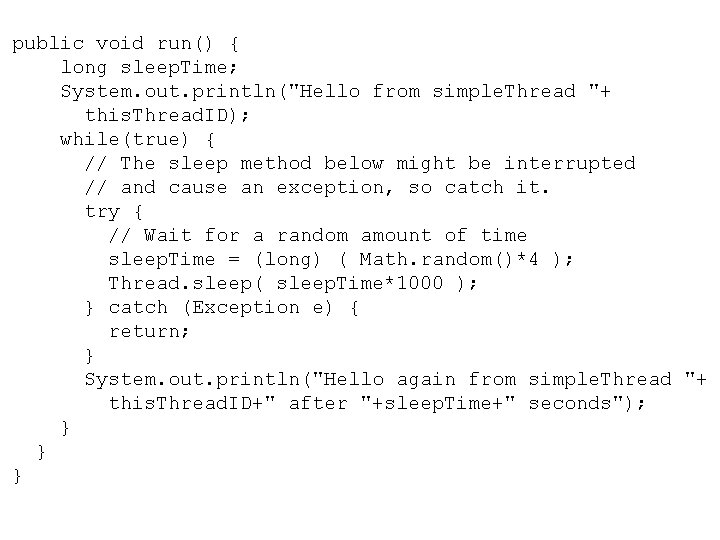
public void run() { long sleep. Time; System. out. println("Hello from simple. Thread "+ this. Thread. ID); while(true) { // The sleep method below might be interrupted // and cause an exception, so catch it. try { // Wait for a random amount of time sleep. Time = (long) ( Math. random()*4 ); Thread. sleep( sleep. Time*1000 ); } catch (Exception e) { return; } System. out. println("Hello again from simple. Thread "+ this. Thread. ID+" after "+sleep. Time+" seconds"); } } }
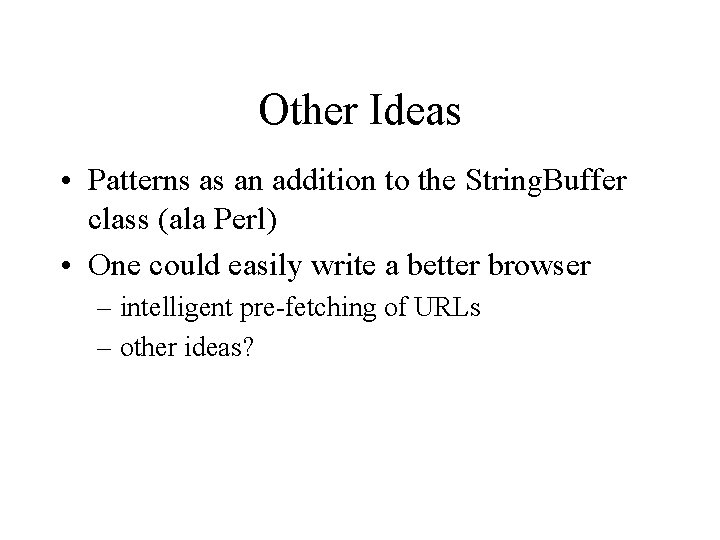
Other Ideas • Patterns as an addition to the String. Buffer class (ala Perl) • One could easily write a better browser – intelligent pre-fetching of URLs – other ideas?
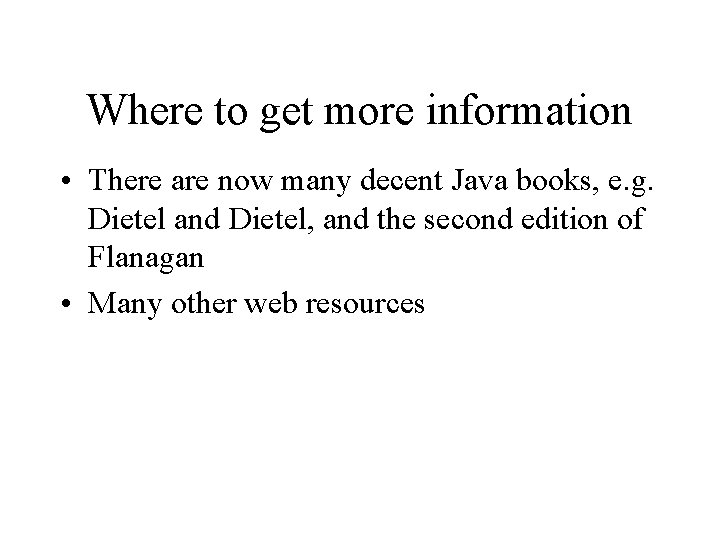
Where to get more information • There are now many decent Java books, e. g. Dietel and Dietel, and the second edition of Flanagan • Many other web resources