Introduction to Collections Collections n A collection is
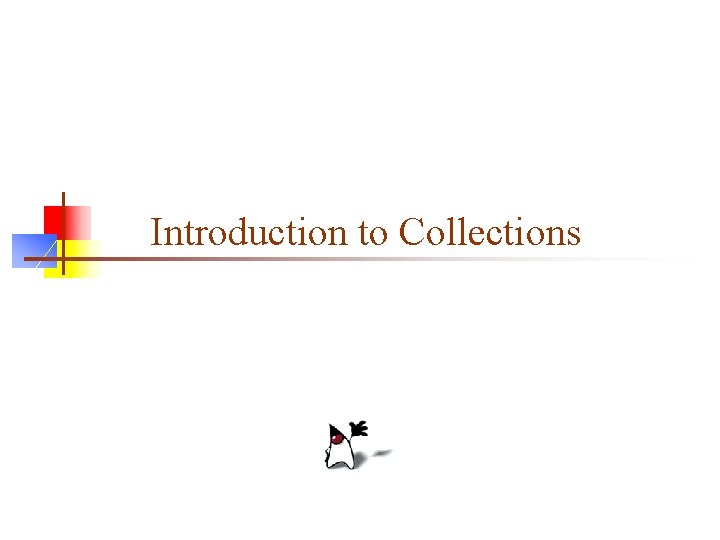
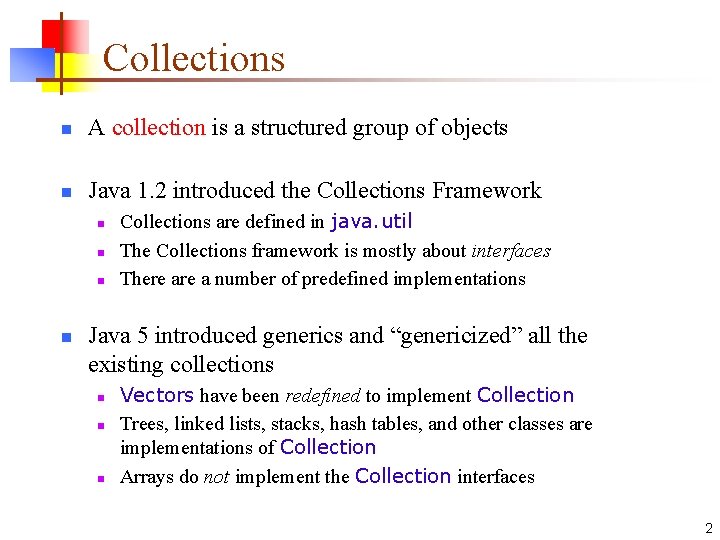
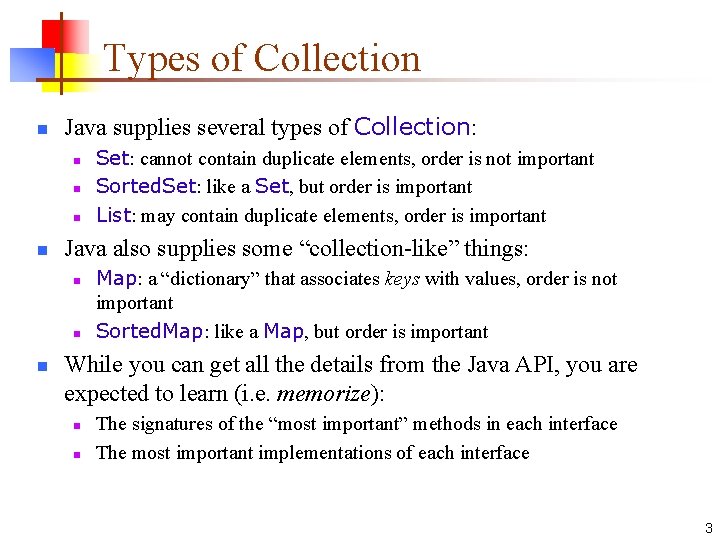
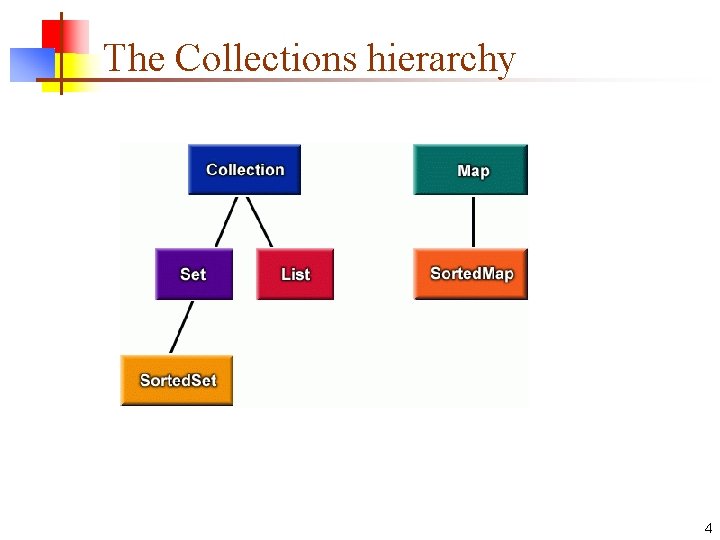
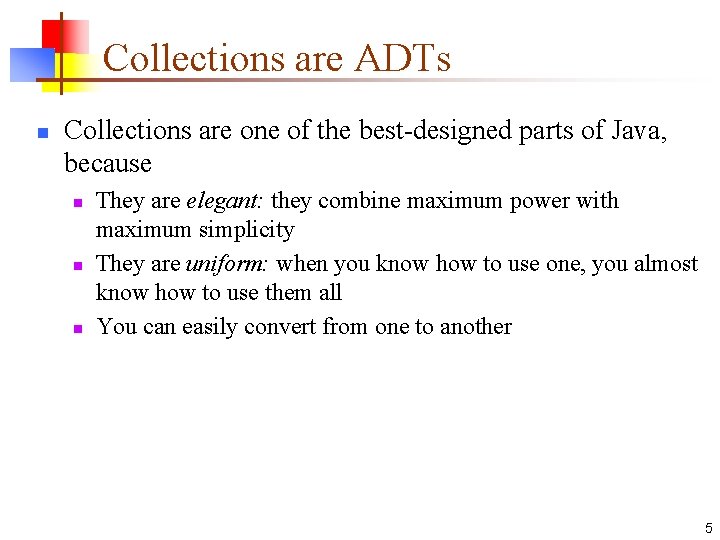
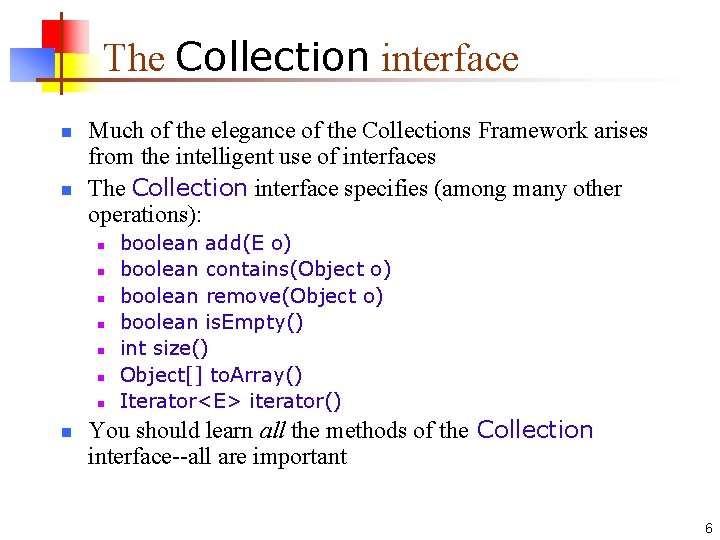
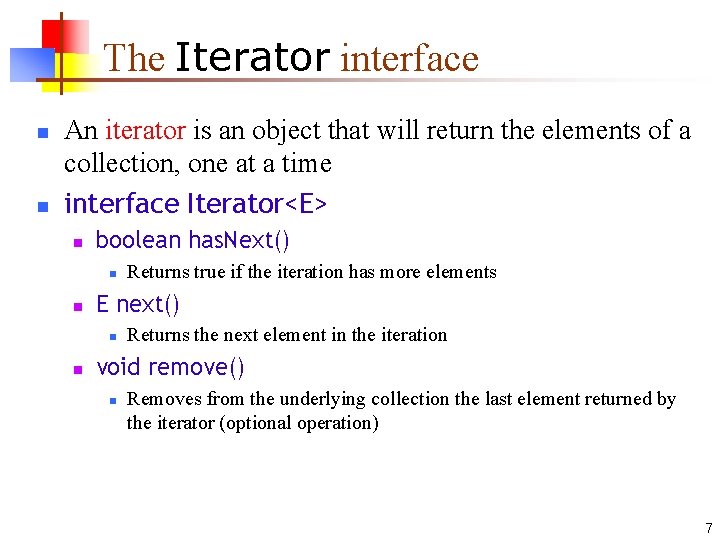
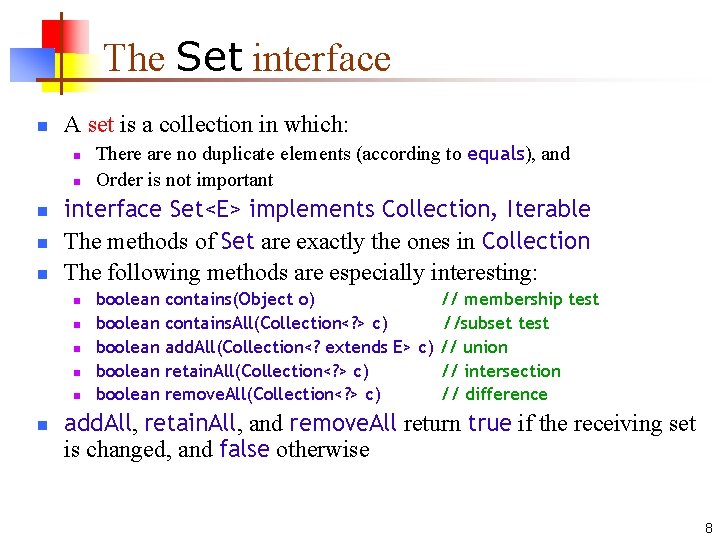
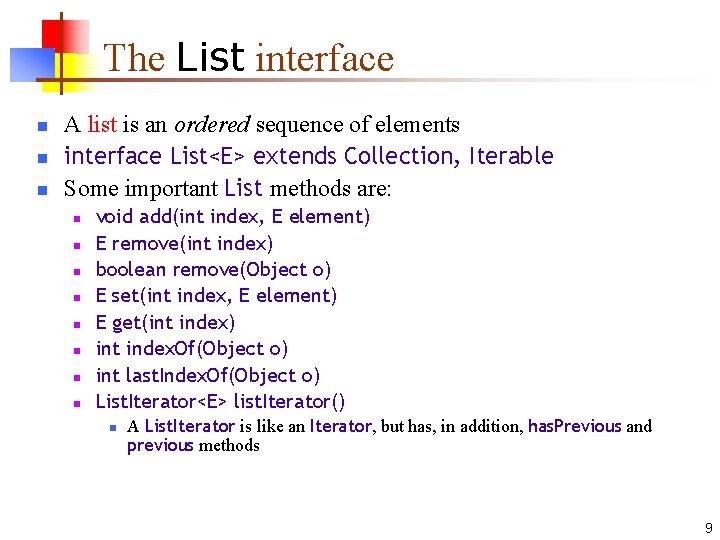
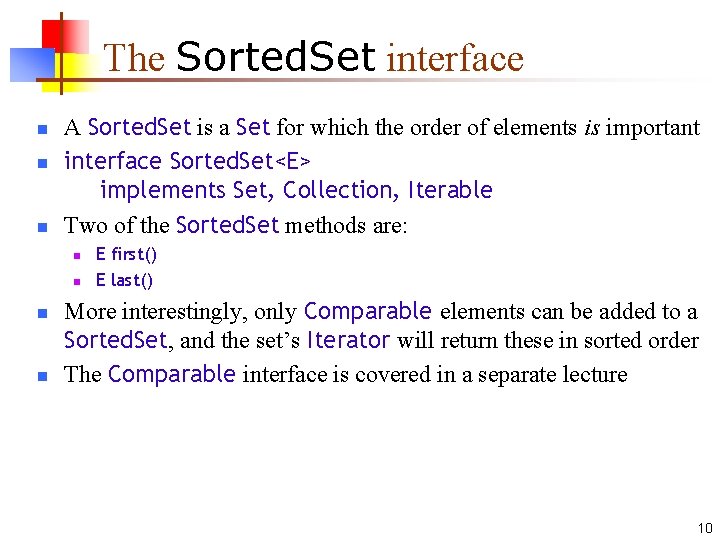
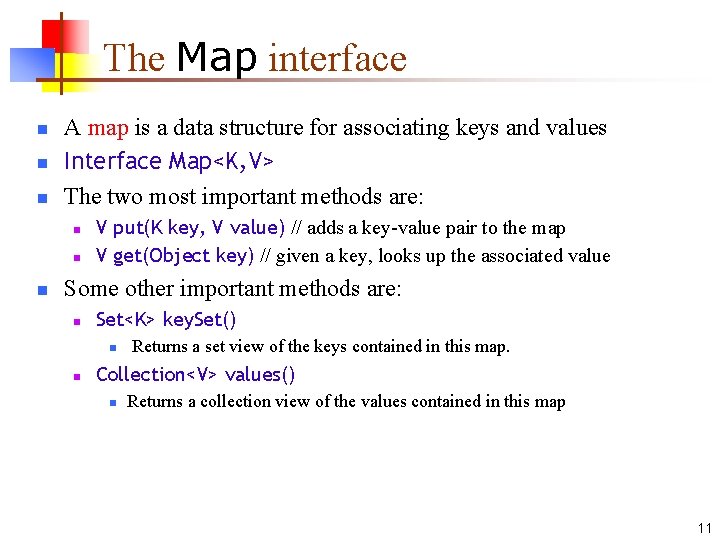
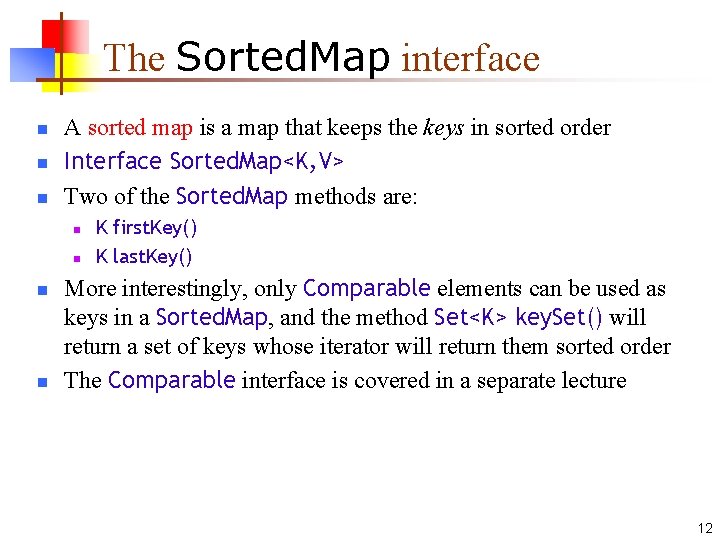
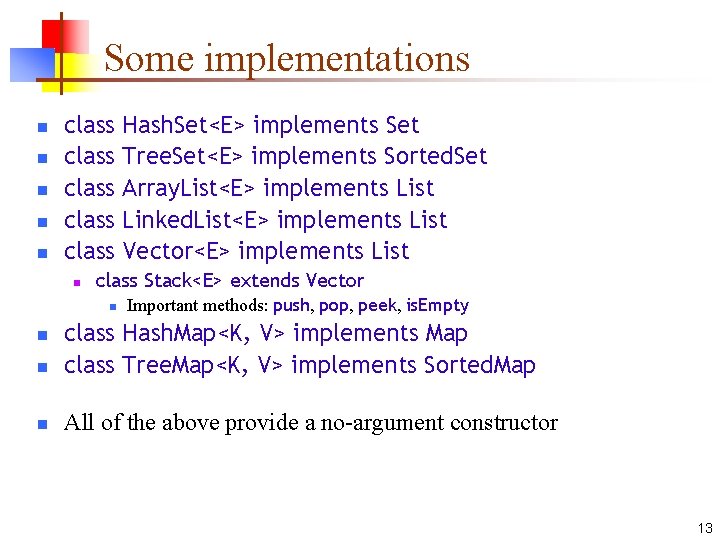
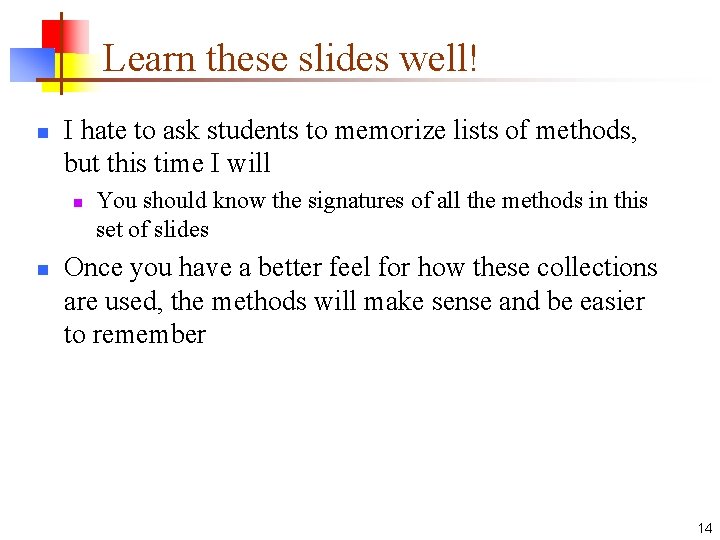
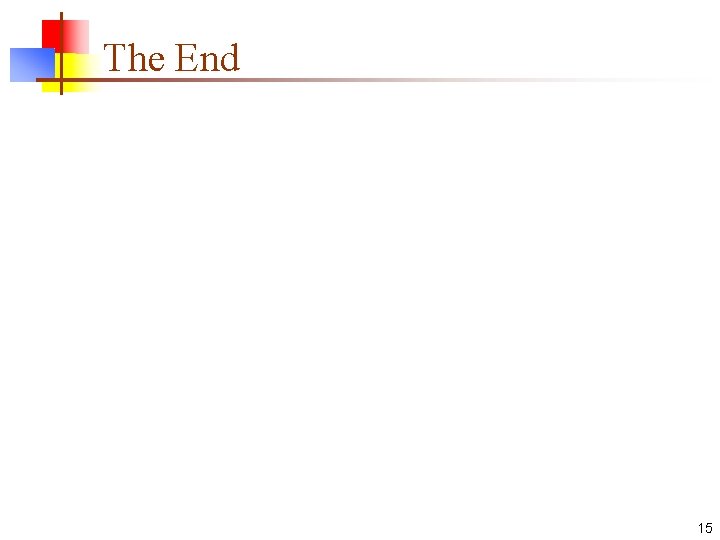
- Slides: 15
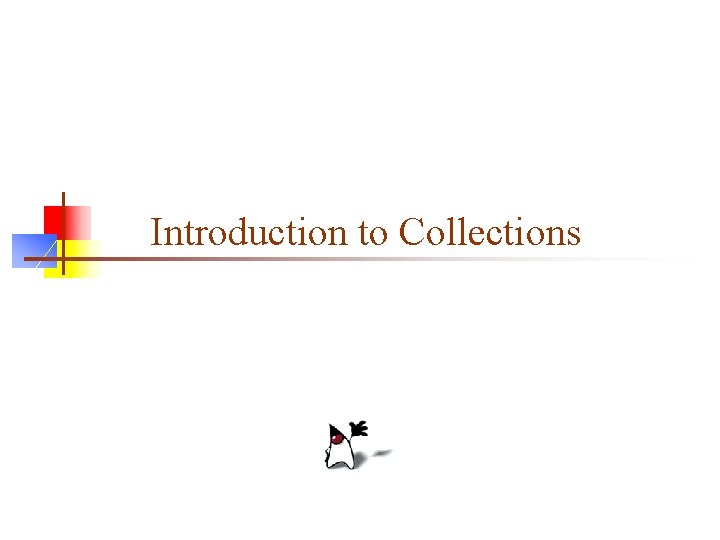
Introduction to Collections
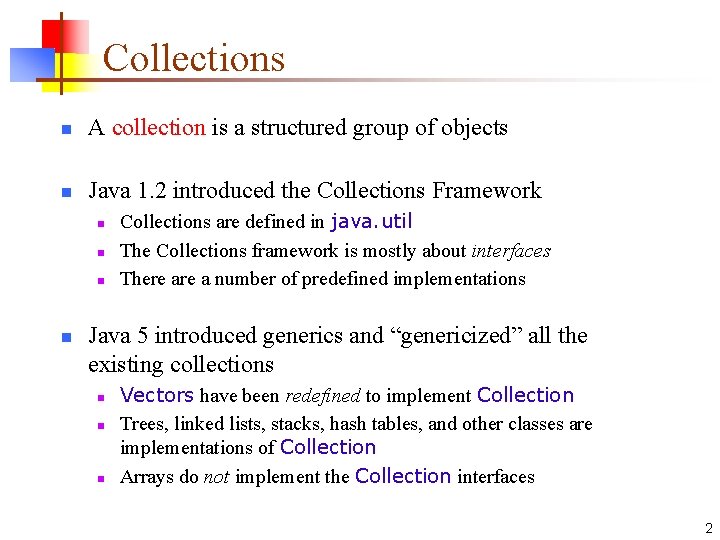
Collections n A collection is a structured group of objects n Java 1. 2 introduced the Collections Framework n n Collections are defined in java. util The Collections framework is mostly about interfaces There a number of predefined implementations Java 5 introduced generics and “genericized” all the existing collections n n n Vectors have been redefined to implement Collection Trees, linked lists, stacks, hash tables, and other classes are implementations of Collection Arrays do not implement the Collection interfaces 2
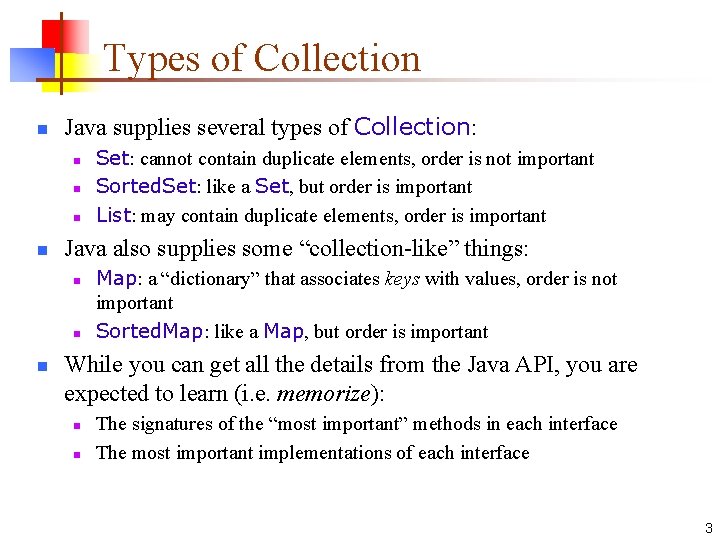
Types of Collection n Java supplies several types of Collection: n n Java also supplies some “collection-like” things: n n n Set: cannot contain duplicate elements, order is not important Sorted. Set: like a Set, but order is important List: may contain duplicate elements, order is important Map: a “dictionary” that associates keys with values, order is not important Sorted. Map: like a Map, but order is important While you can get all the details from the Java API, you are expected to learn (i. e. memorize): n n The signatures of the “most important” methods in each interface The most important implementations of each interface 3
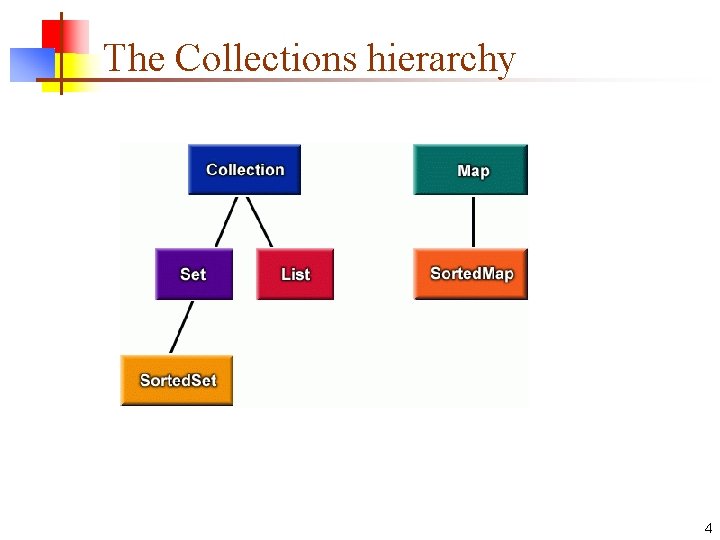
The Collections hierarchy 4
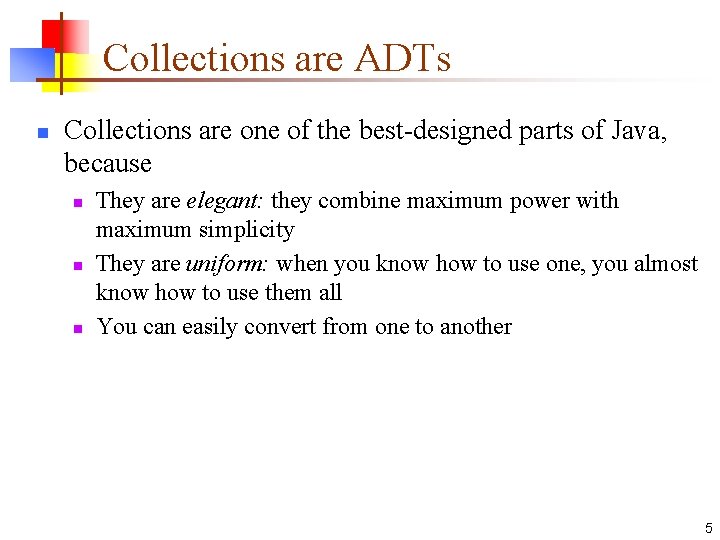
Collections are ADTs n Collections are one of the best-designed parts of Java, because n n n They are elegant: they combine maximum power with maximum simplicity They are uniform: when you know how to use one, you almost know how to use them all You can easily convert from one to another 5
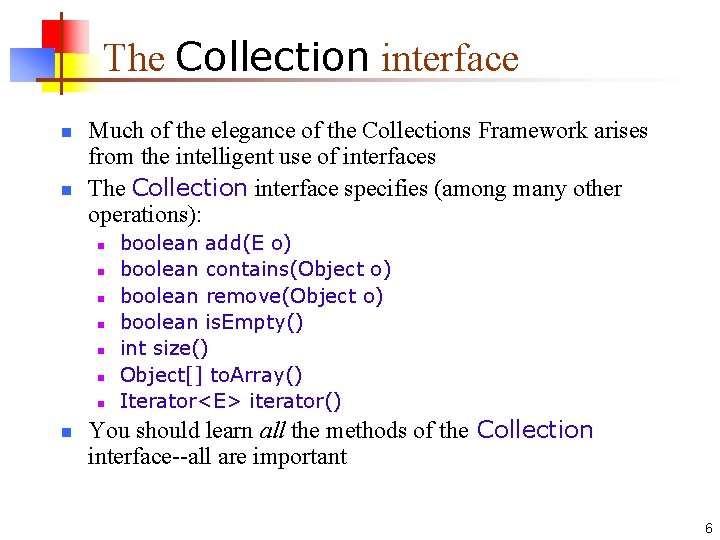
The Collection interface n n Much of the elegance of the Collections Framework arises from the intelligent use of interfaces The Collection interface specifies (among many other operations): n n n n boolean add(E o) boolean contains(Object o) boolean remove(Object o) boolean is. Empty() int size() Object[] to. Array() Iterator<E> iterator() You should learn all the methods of the Collection interface--all are important 6
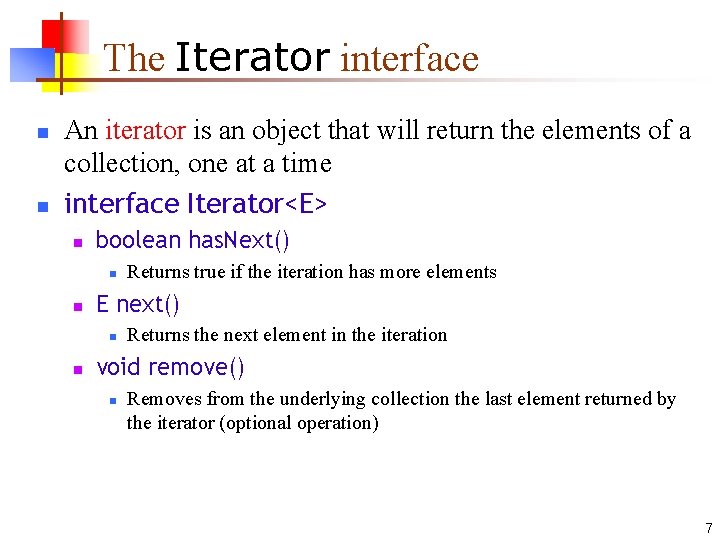
The Iterator interface n n An iterator is an object that will return the elements of a collection, one at a time interface Iterator<E> n boolean has. Next() n n E next() n n Returns true if the iteration has more elements Returns the next element in the iteration void remove() n Removes from the underlying collection the last element returned by the iterator (optional operation) 7
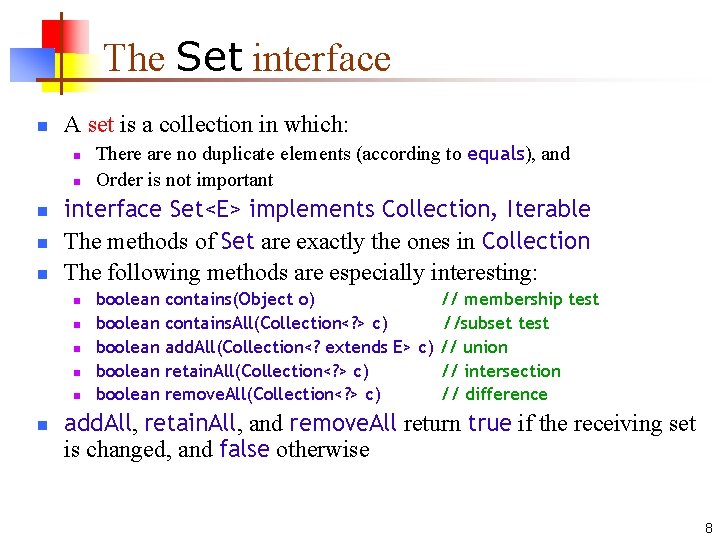
The Set interface n A set is a collection in which: n n n interface Set<E> implements Collection, Iterable The methods of Set are exactly the ones in Collection The following methods are especially interesting: n n n There are no duplicate elements (according to equals), and Order is not important boolean boolean contains(Object o) // membership test contains. All(Collection<? > c) //subset test add. All(Collection<? extends E> c) // union retain. All(Collection<? > c) // intersection remove. All(Collection<? > c) // difference add. All, retain. All, and remove. All return true if the receiving set is changed, and false otherwise 8
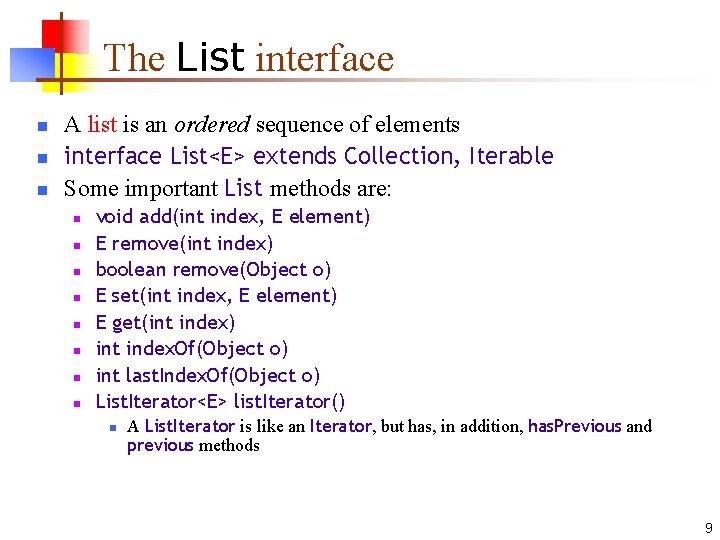
The List interface n n n A list is an ordered sequence of elements interface List<E> extends Collection, Iterable Some important List methods are: n n n n void add(int index, E element) E remove(int index) boolean remove(Object o) E set(int index, E element) E get(int index) int index. Of(Object o) int last. Index. Of(Object o) List. Iterator<E> list. Iterator() n A List. Iterator is like an Iterator, but has, in addition, has. Previous and previous methods 9
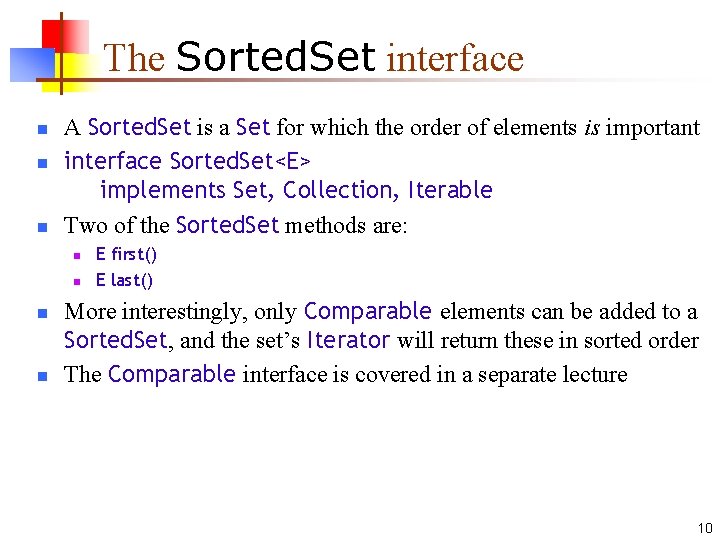
The Sorted. Set interface n n n A Sorted. Set is a Set for which the order of elements is important interface Sorted. Set<E> implements Set, Collection, Iterable Two of the Sorted. Set methods are: n n E first() E last() More interestingly, only Comparable elements can be added to a Sorted. Set, and the set’s Iterator will return these in sorted order The Comparable interface is covered in a separate lecture 10
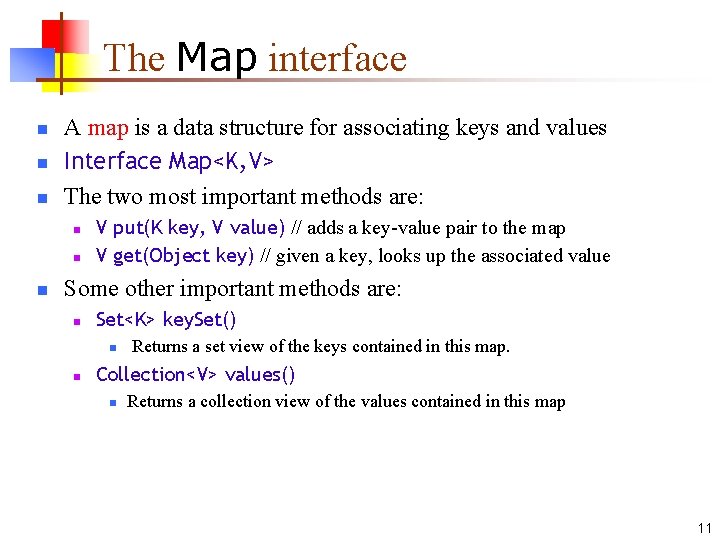
The Map interface n n n A map is a data structure for associating keys and values Interface Map<K, V> The two most important methods are: n n n V put(K key, V value) // adds a key-value pair to the map V get(Object key) // given a key, looks up the associated value Some other important methods are: n Set<K> key. Set() n n Returns a set view of the keys contained in this map. Collection<V> values() n Returns a collection view of the values contained in this map 11
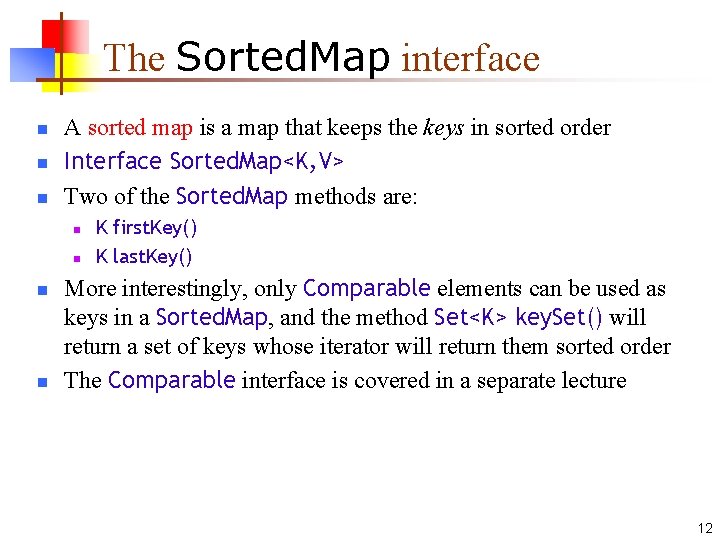
The Sorted. Map interface n n n A sorted map is a map that keeps the keys in sorted order Interface Sorted. Map<K, V> Two of the Sorted. Map methods are: n n K first. Key() K last. Key() More interestingly, only Comparable elements can be used as keys in a Sorted. Map, and the method Set<K> key. Set() will return a set of keys whose iterator will return them sorted order The Comparable interface is covered in a separate lecture 12
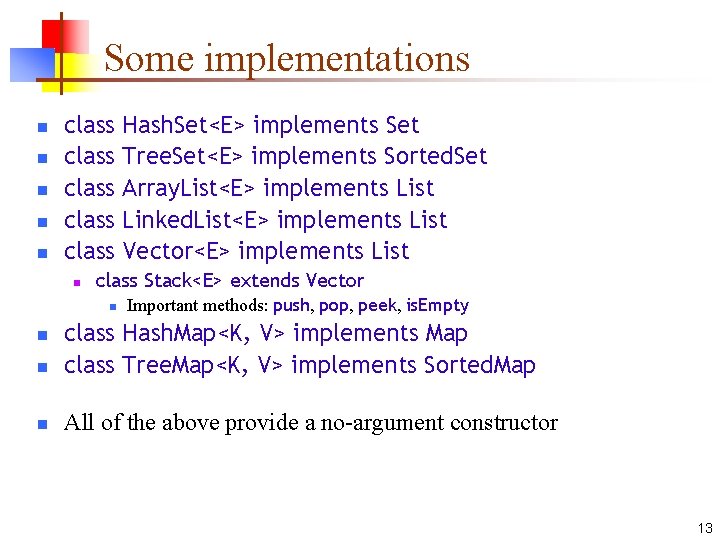
Some implementations n n n class class n Hash. Set<E> implements Set Tree. Set<E> implements Sorted. Set Array. List<E> implements List Linked. List<E> implements List Vector<E> implements List class Stack<E> extends Vector n Important methods: push, pop, peek, is. Empty n class Hash. Map<K, V> implements Map class Tree. Map<K, V> implements Sorted. Map n All of the above provide a no-argument constructor n 13
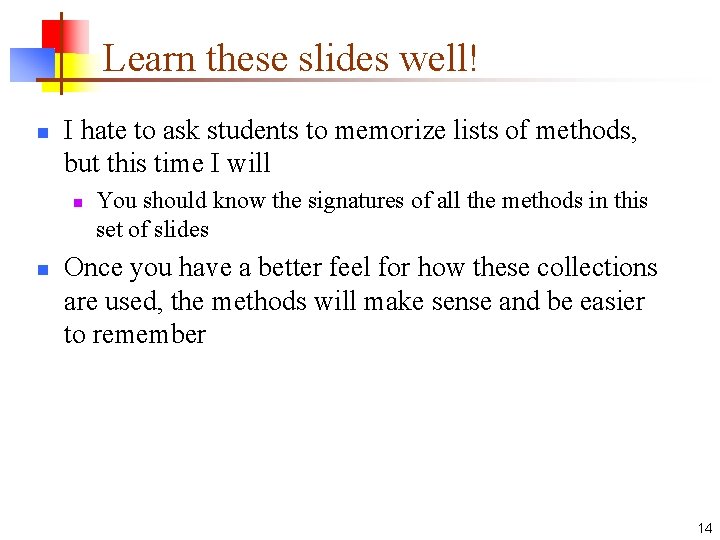
Learn these slides well! n I hate to ask students to memorize lists of methods, but this time I will n n You should know the signatures of all the methods in this set of slides Once you have a better feel for how these collections are used, the methods will make sense and be easier to remember 14
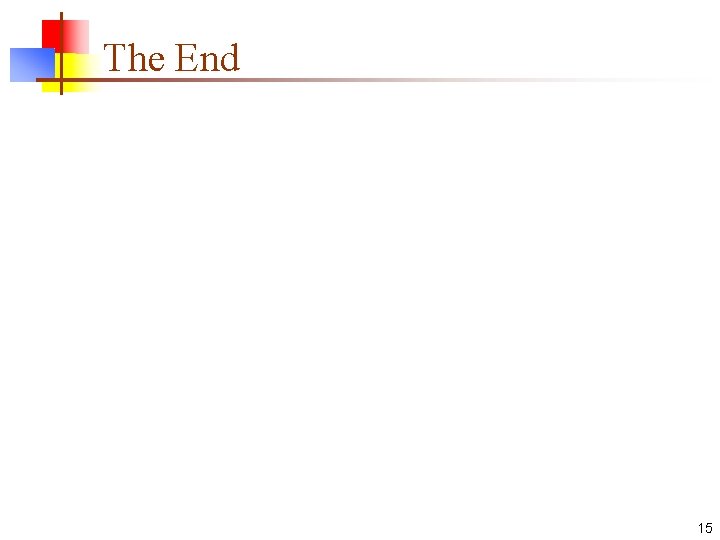
The End 15