Introduction to C Topics Covered n Creating a
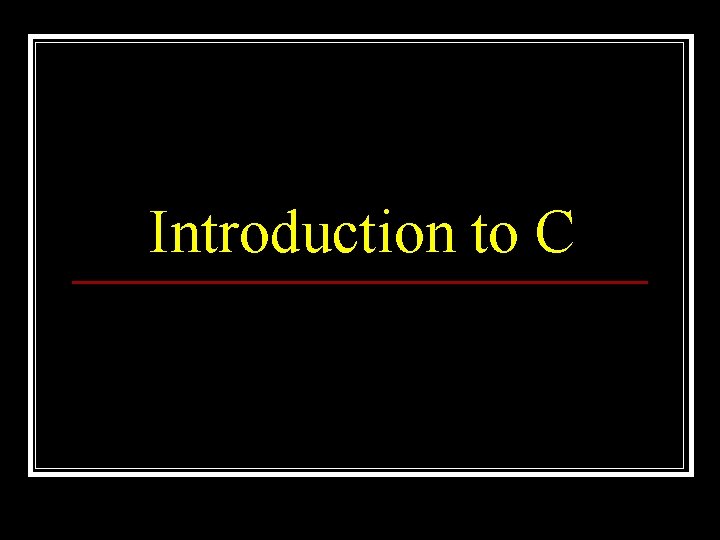
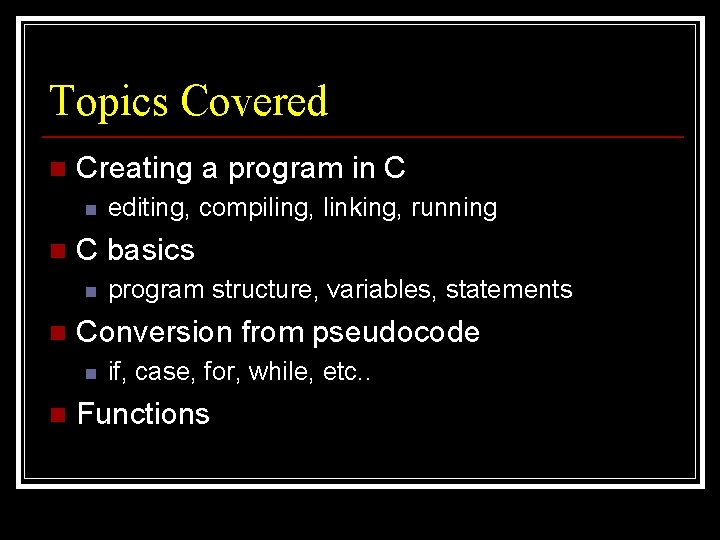
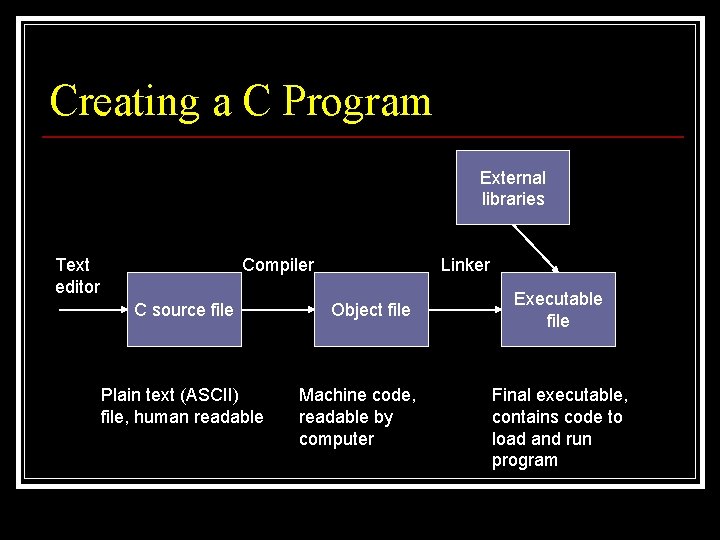
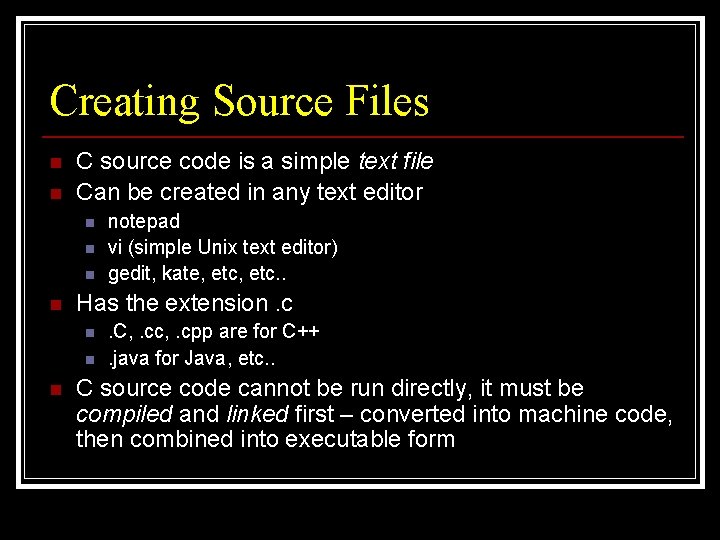
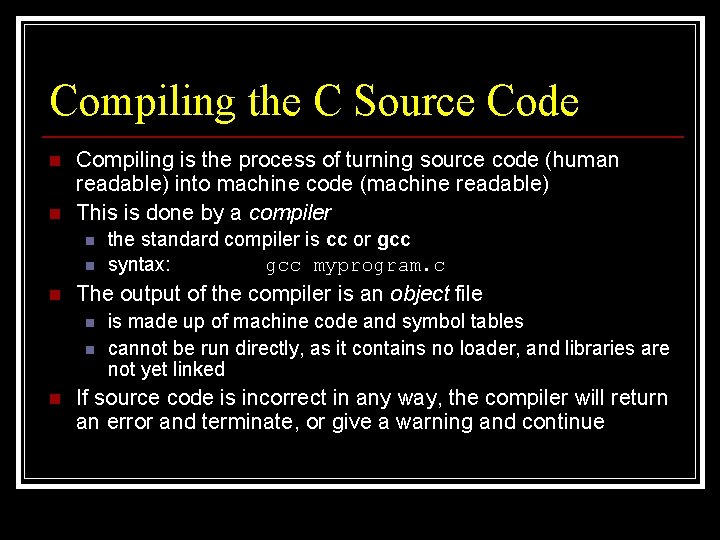
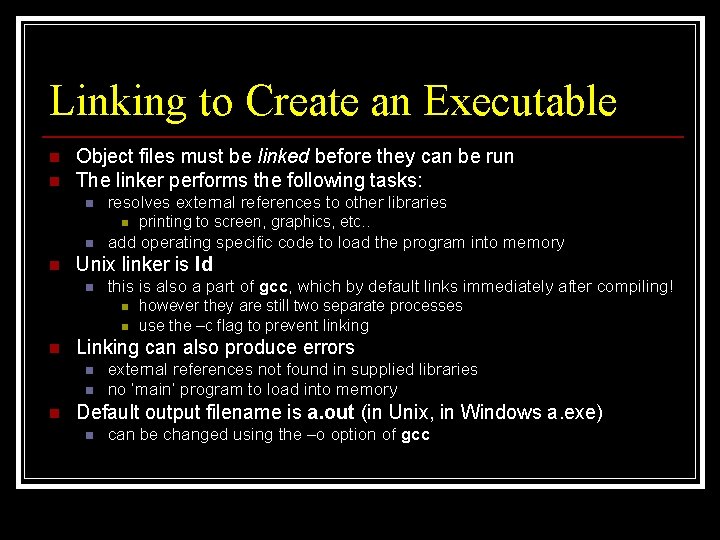
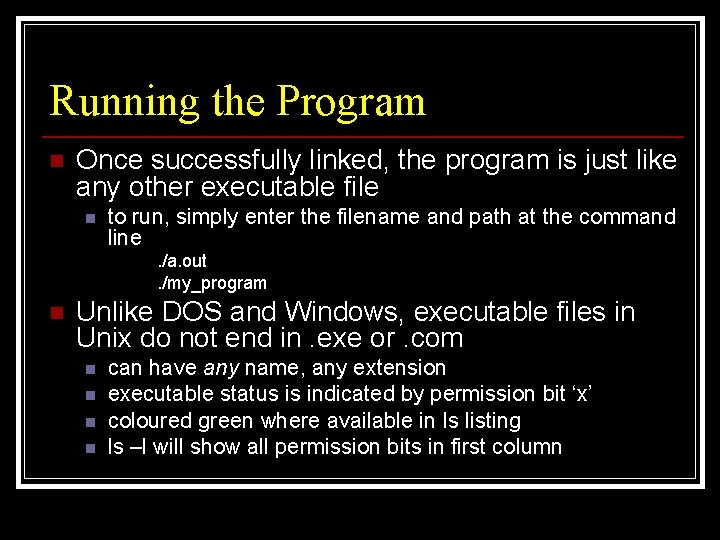
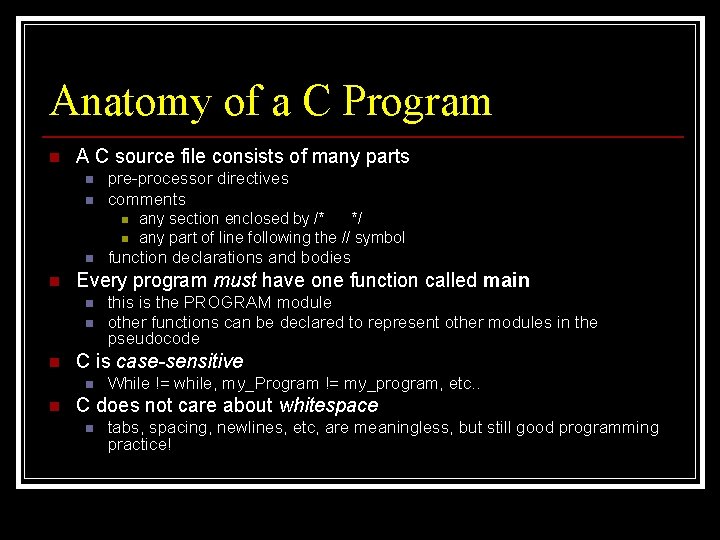
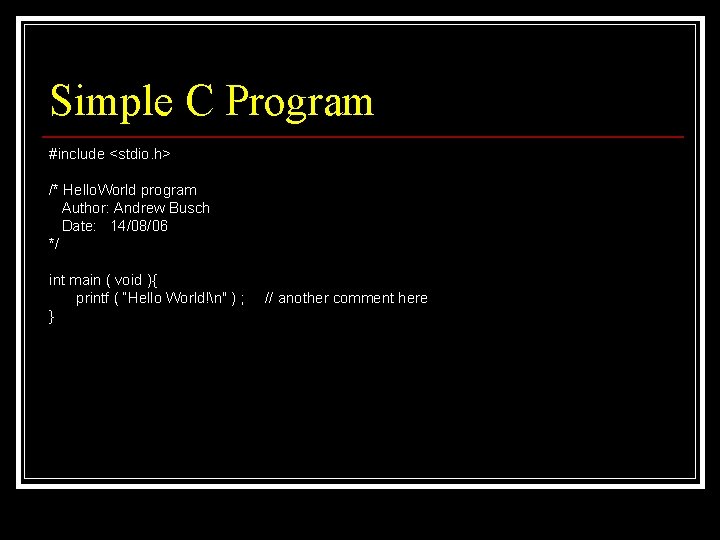
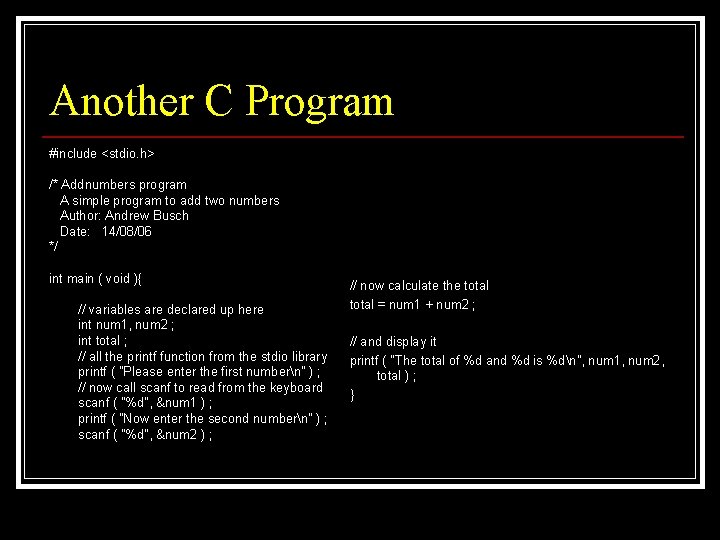
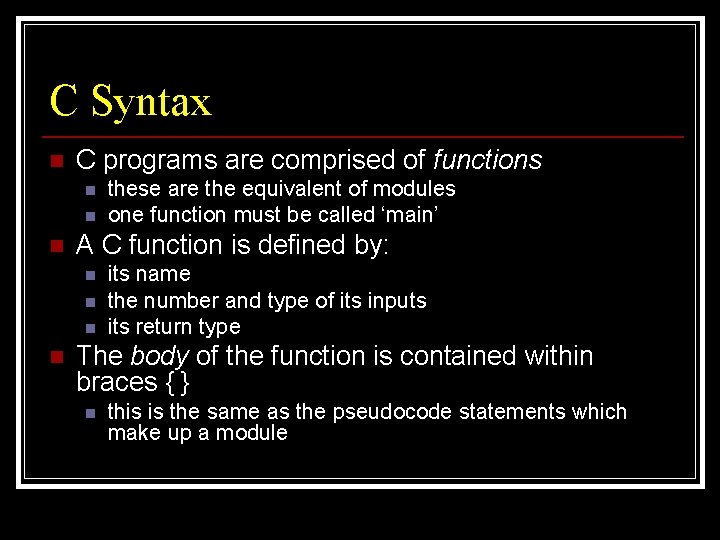
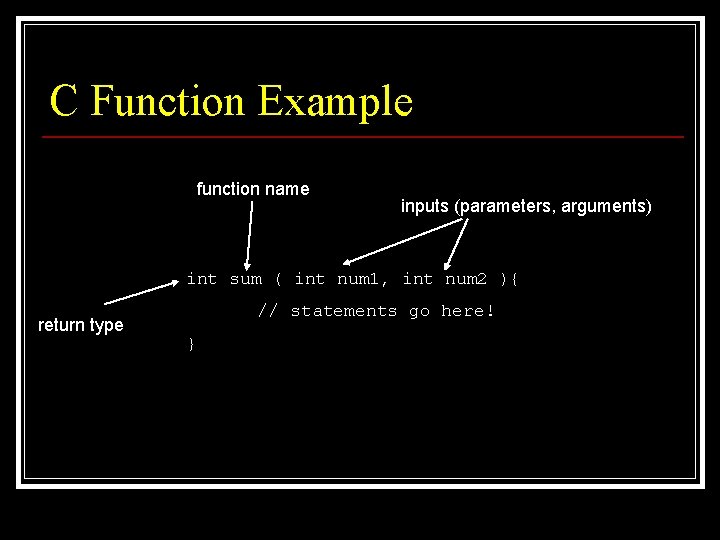
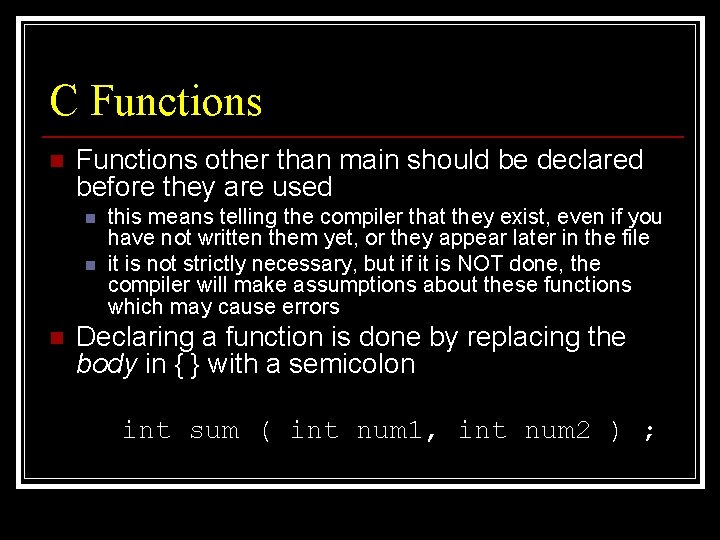
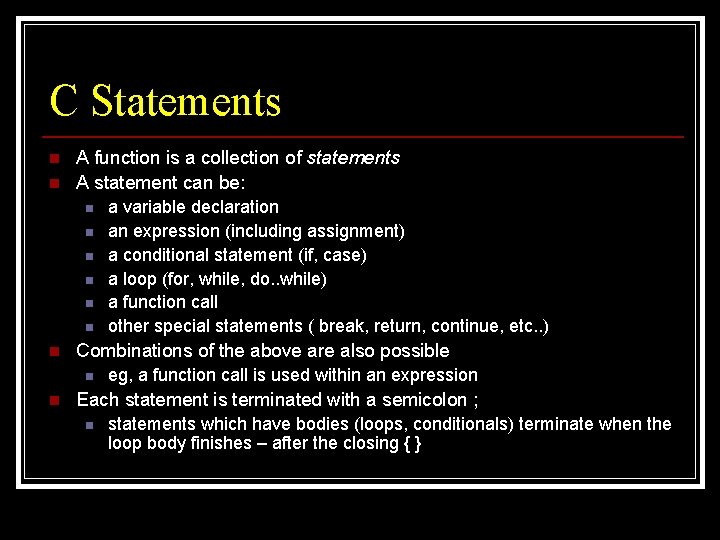
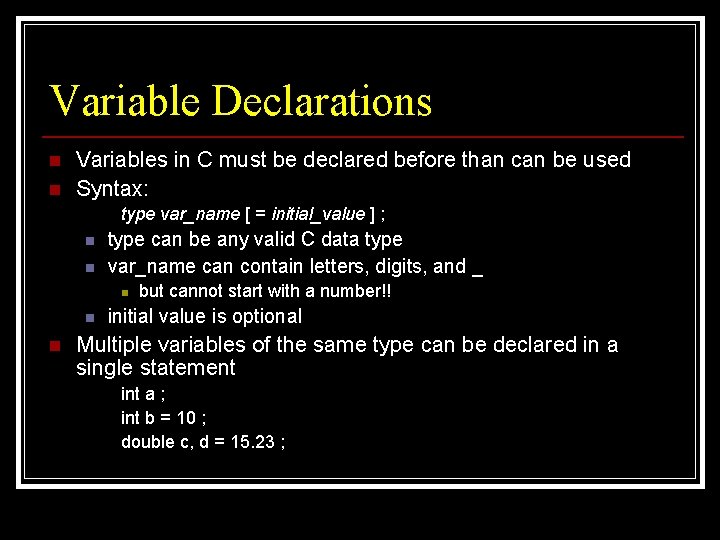
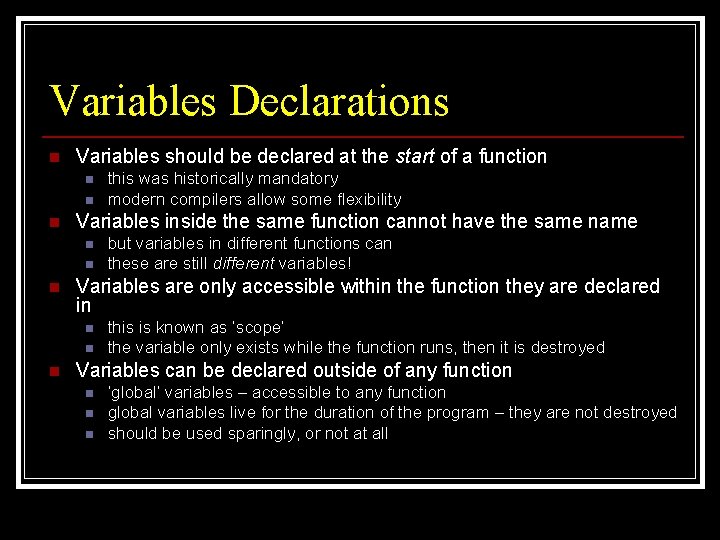
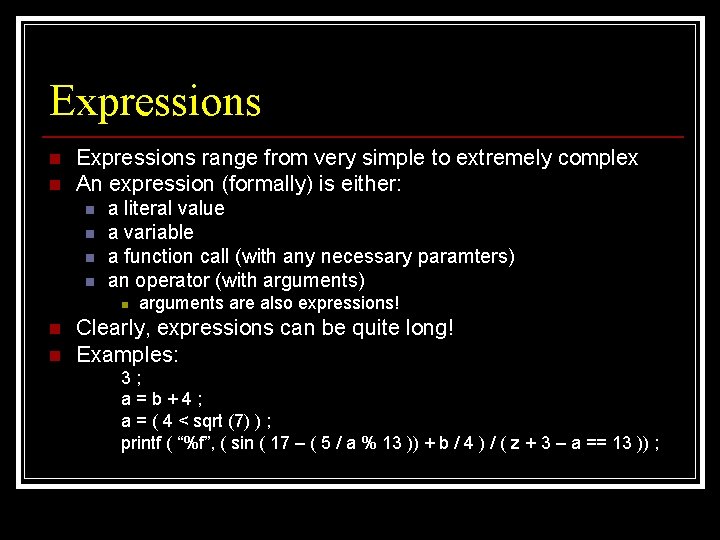
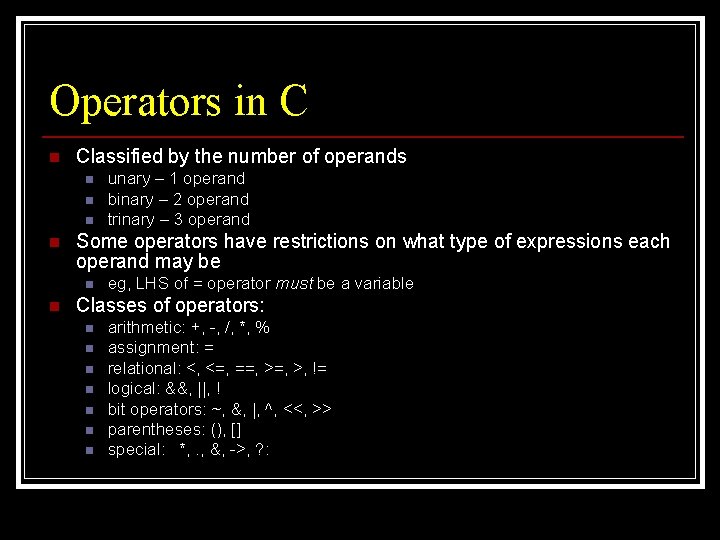
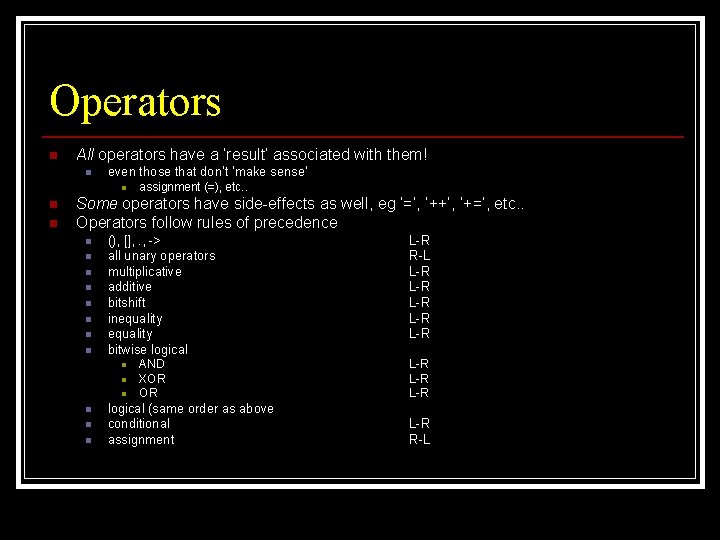
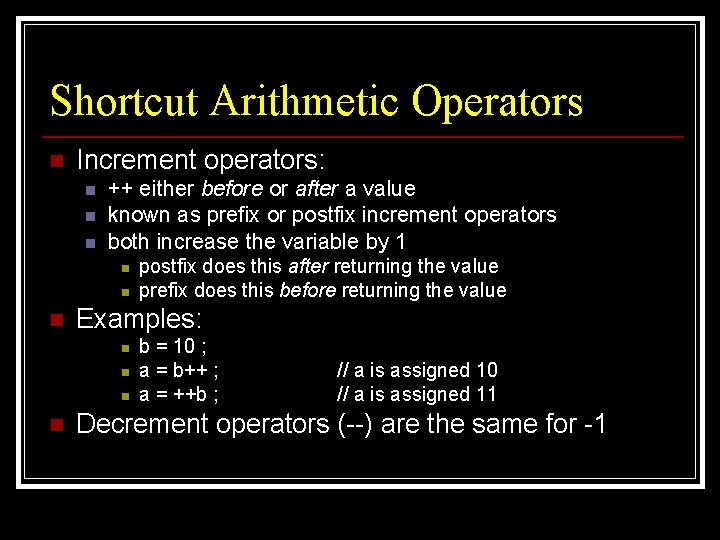
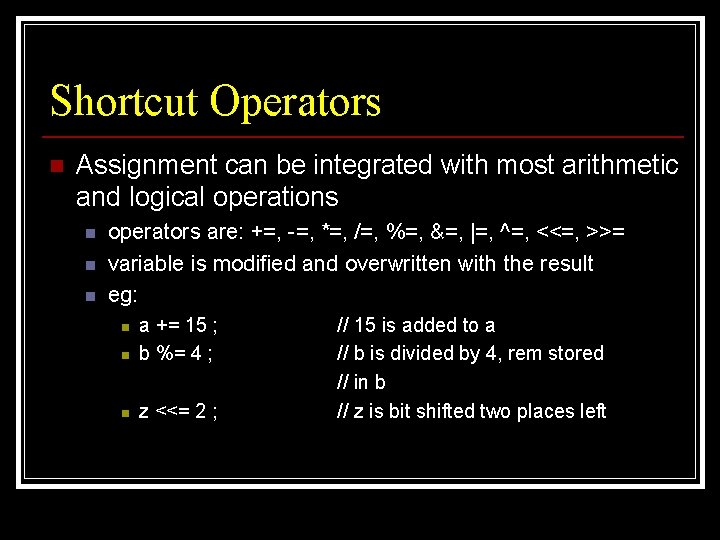
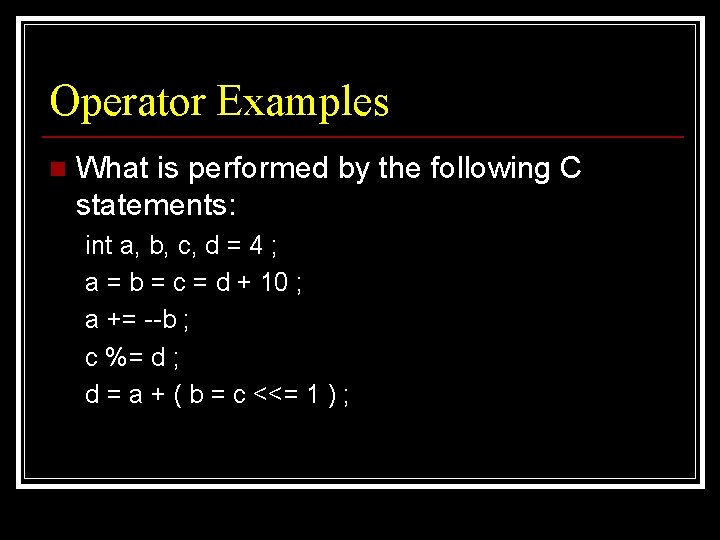
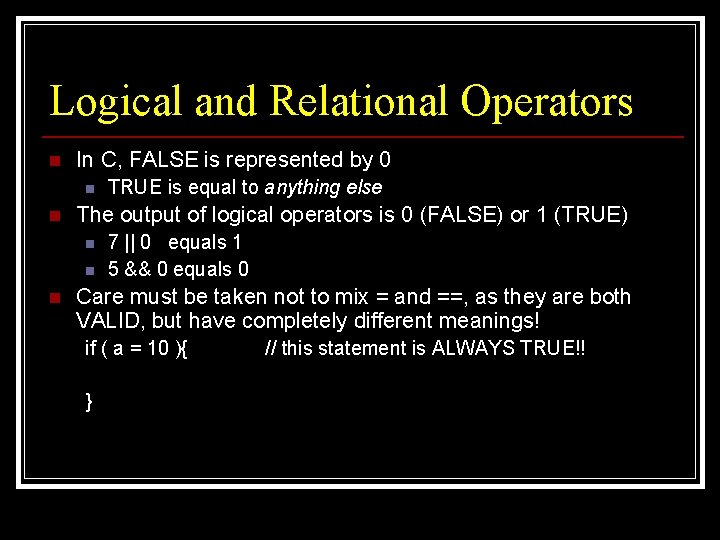
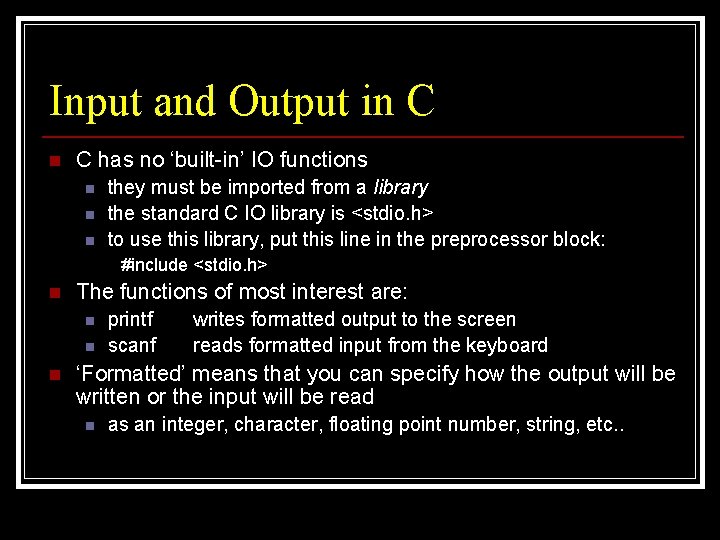
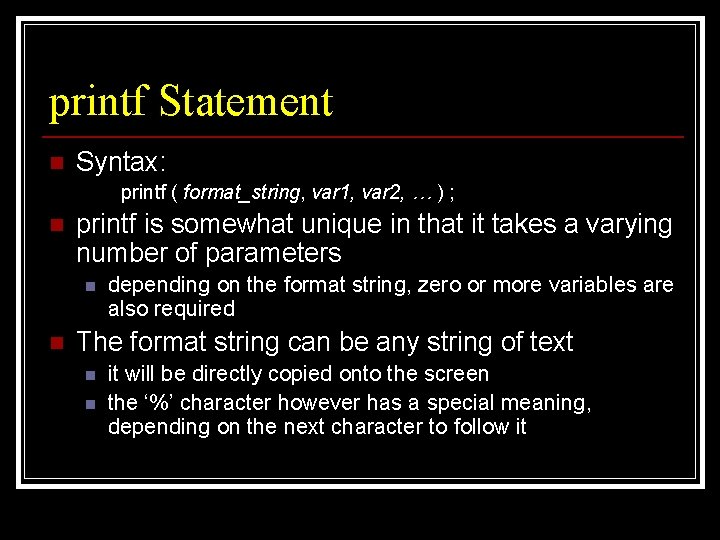
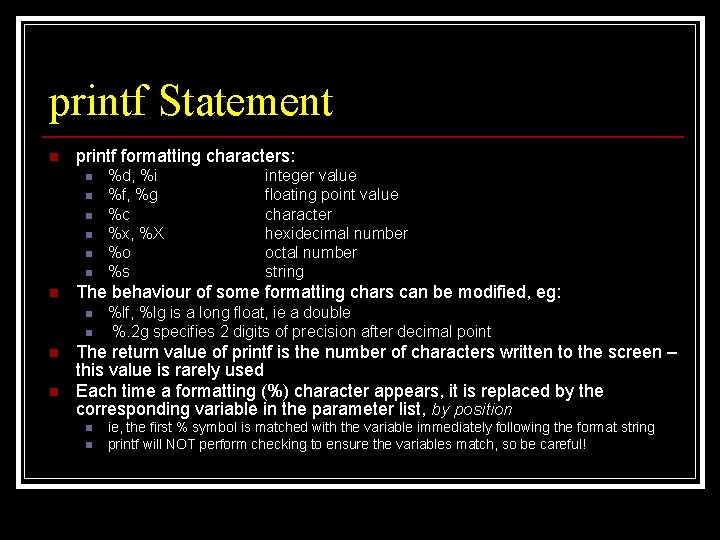
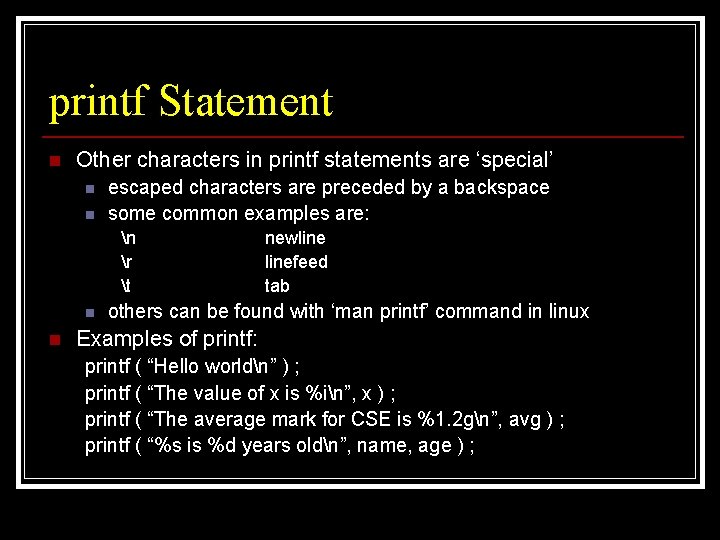
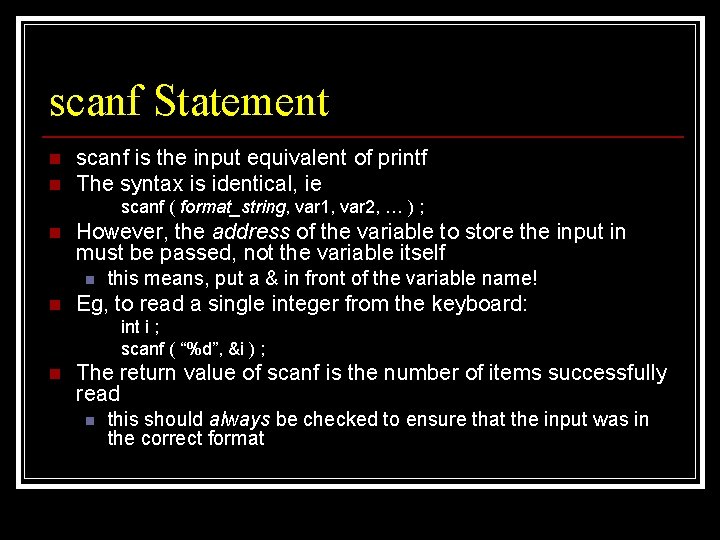
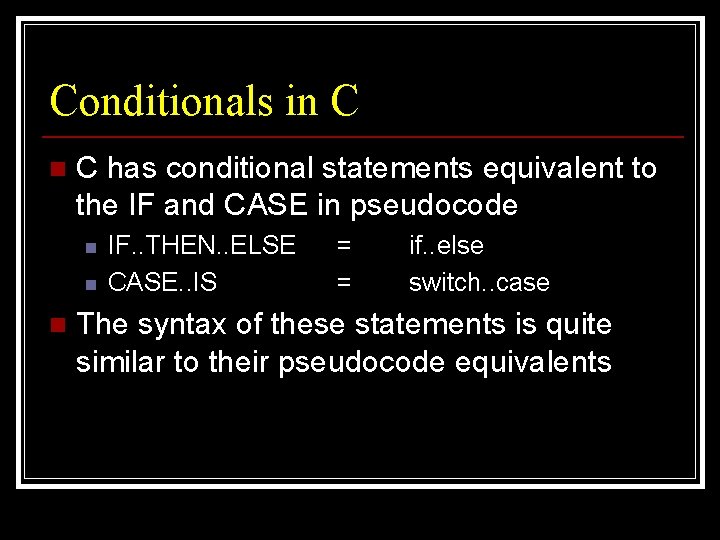
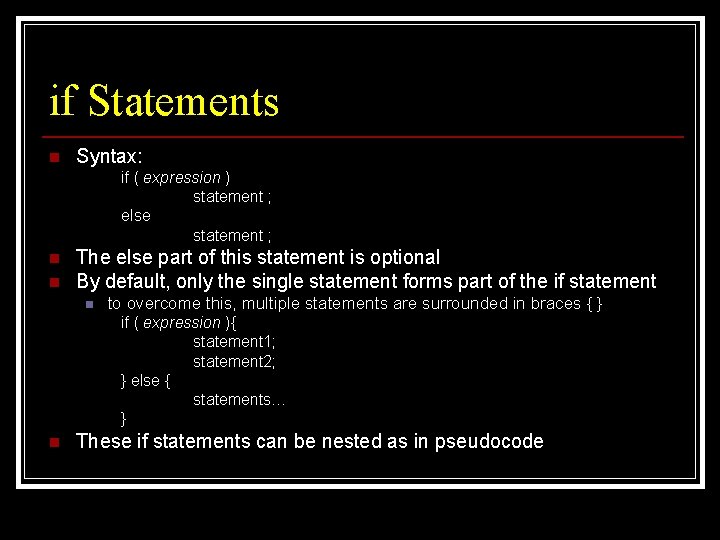
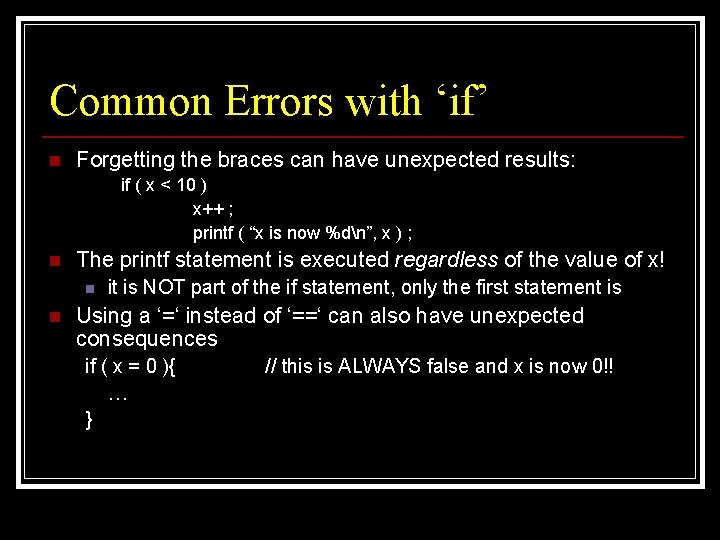
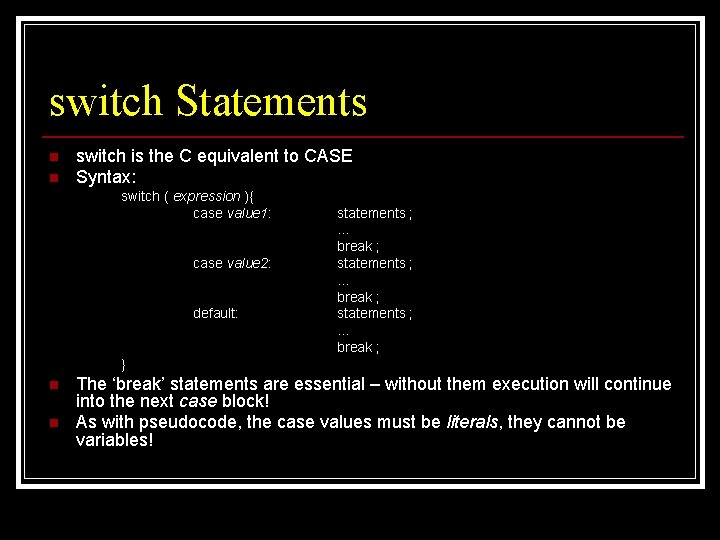
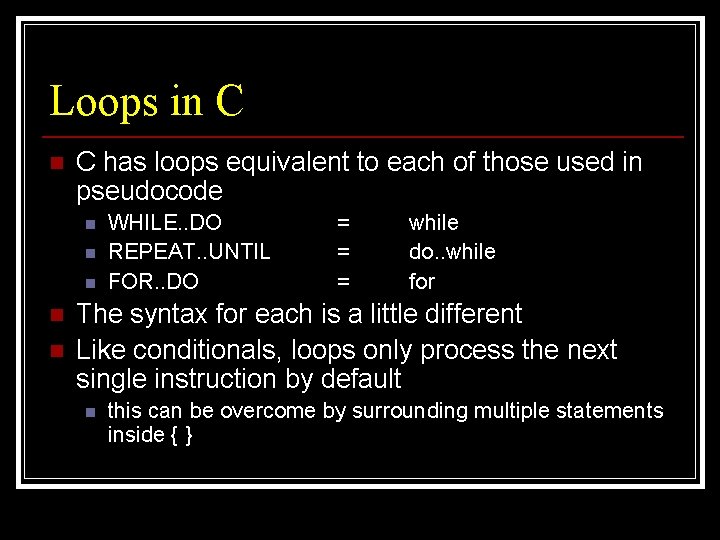
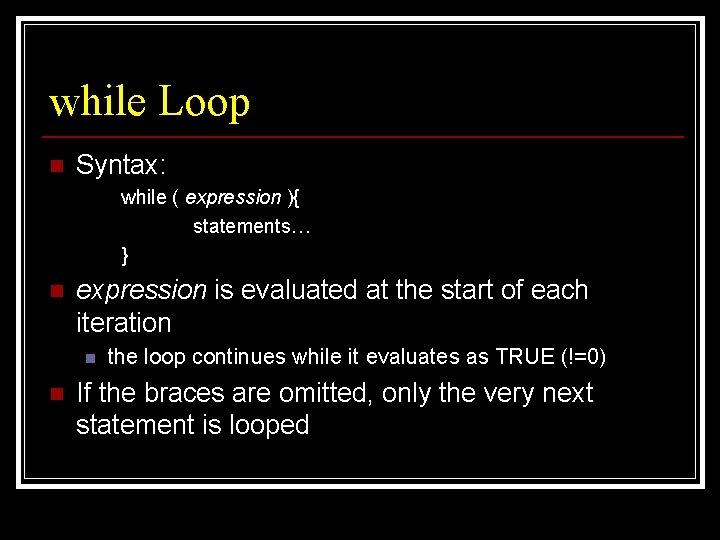
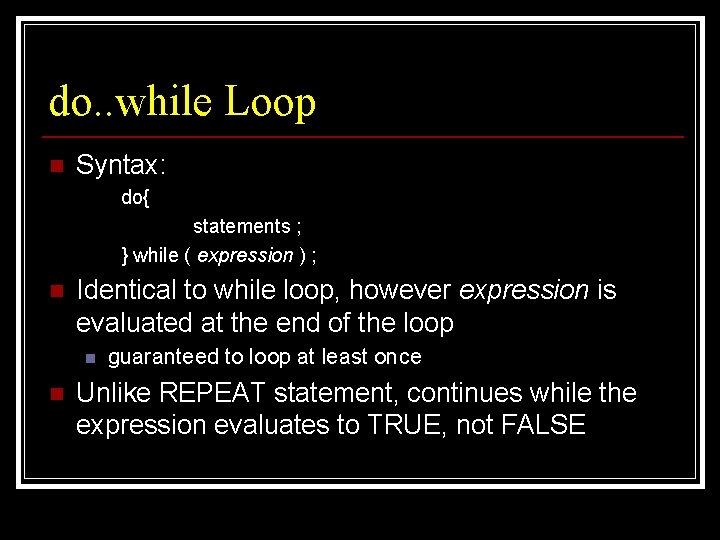
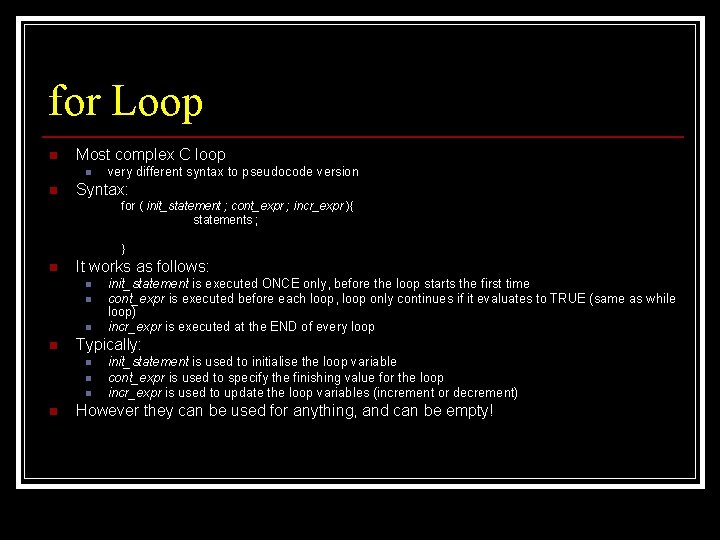
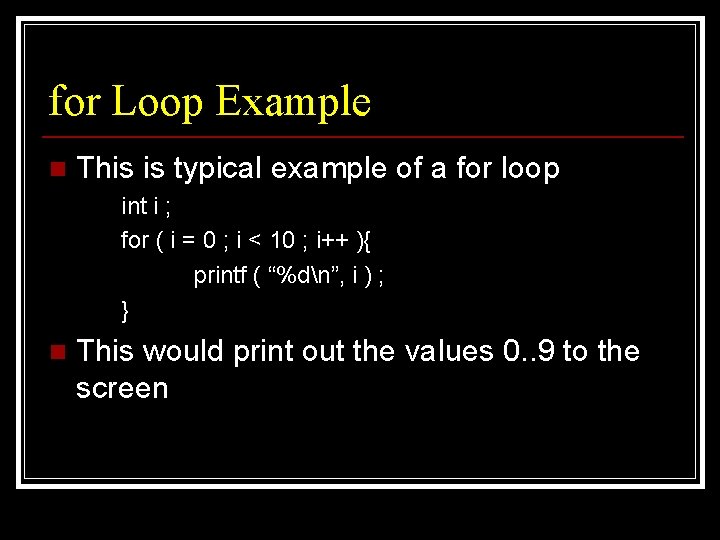
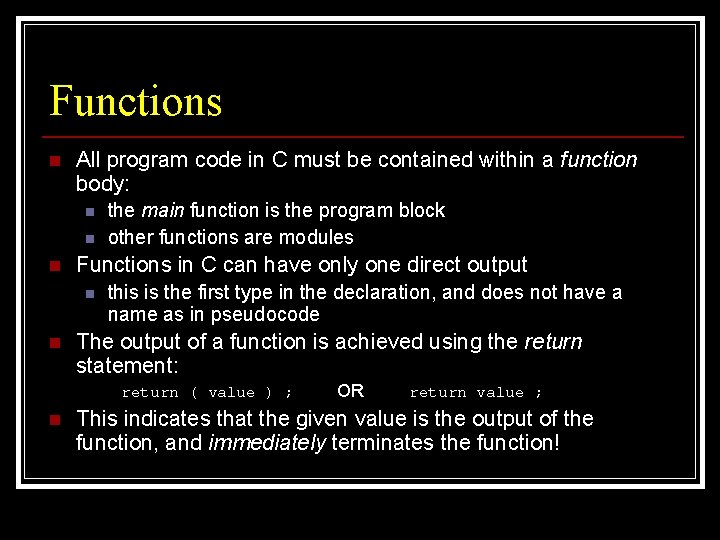
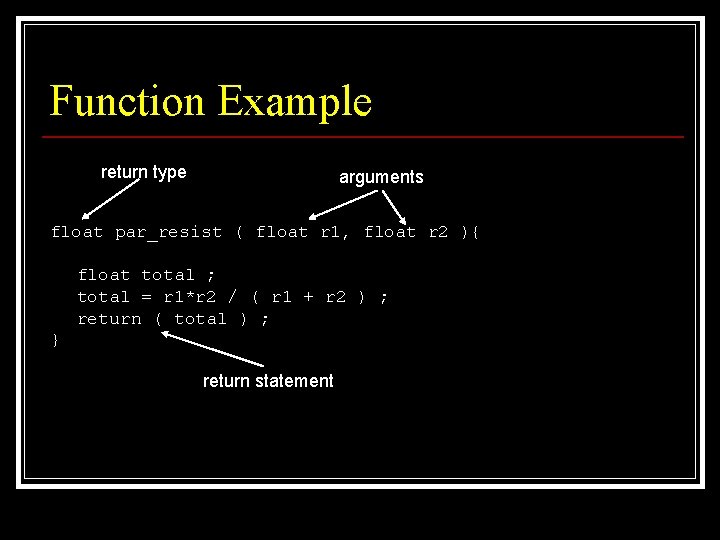
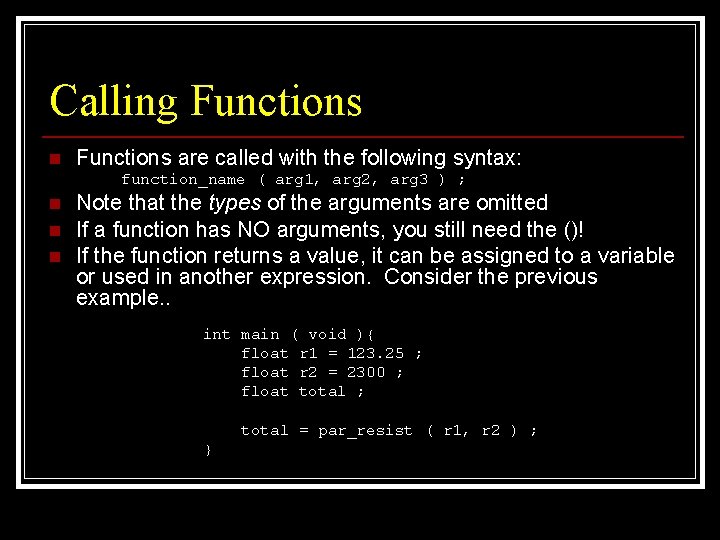
- Slides: 40
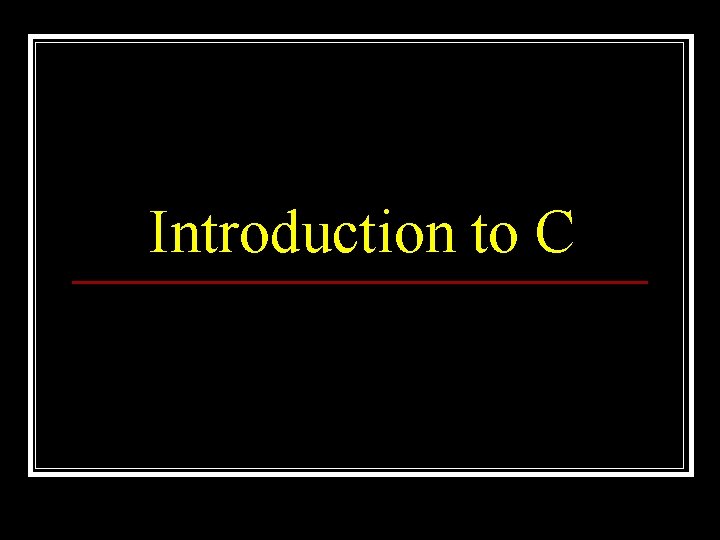
Introduction to C
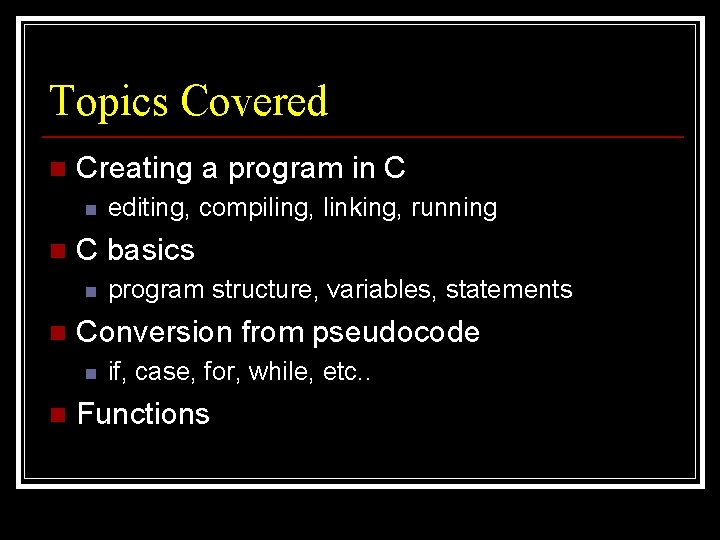
Topics Covered n Creating a program in C n n C basics n n program structure, variables, statements Conversion from pseudocode n n editing, compiling, linking, running if, case, for, while, etc. . Functions
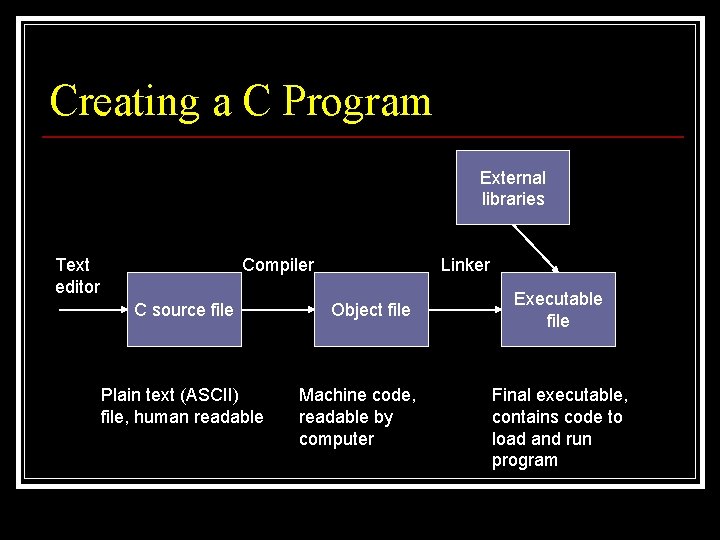
Creating a C Program External libraries Text editor Compiler C source file Plain text (ASCII) file, human readable Linker Object file Machine code, readable by computer Executable file Final executable, contains code to load and run program
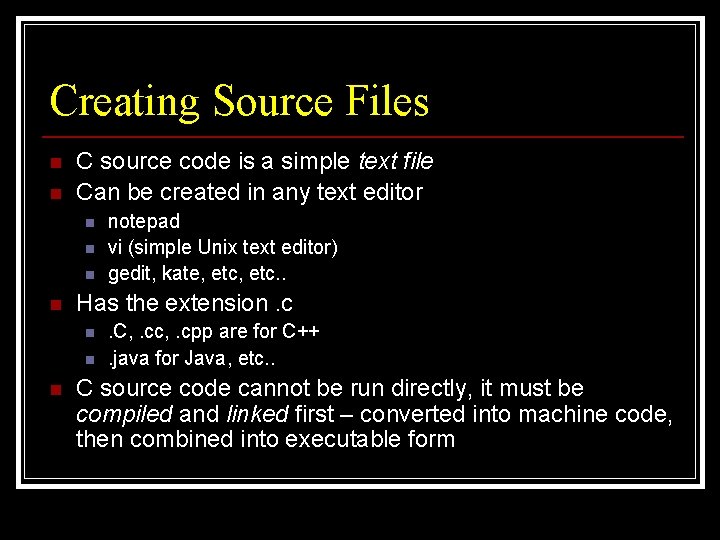
Creating Source Files n n C source code is a simple text file Can be created in any text editor n n Has the extension. c n notepad vi (simple Unix text editor) gedit, kate, etc. . . C, . cc, . cpp are for C++. java for Java, etc. . C source code cannot be run directly, it must be compiled and linked first – converted into machine code, then combined into executable form
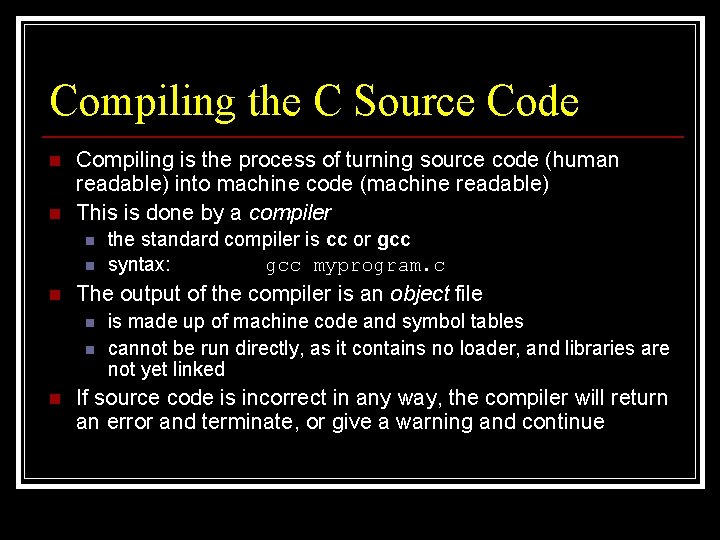
Compiling the C Source Code n n Compiling is the process of turning source code (human readable) into machine code (machine readable) This is done by a compiler n n n The output of the compiler is an object file n n n the standard compiler is cc or gcc syntax: gcc myprogram. c is made up of machine code and symbol tables cannot be run directly, as it contains no loader, and libraries are not yet linked If source code is incorrect in any way, the compiler will return an error and terminate, or give a warning and continue
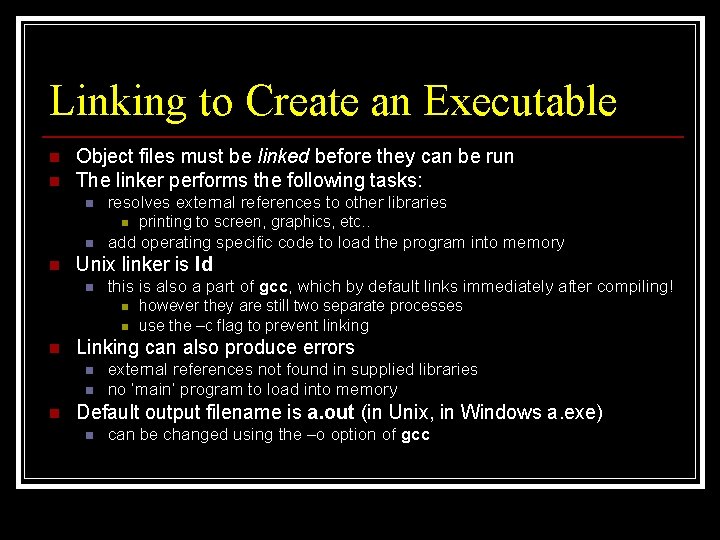
Linking to Create an Executable n n Object files must be linked before they can be run The linker performs the following tasks: n resolves external references to other libraries n n n add operating specific code to load the program into memory Unix linker is ld n this is also a part of gcc, which by default links immediately after compiling! n n n however they are still two separate processes use the –c flag to prevent linking Linking can also produce errors n n n printing to screen, graphics, etc. . external references not found in supplied libraries no ‘main’ program to load into memory Default output filename is a. out (in Unix, in Windows a. exe) n can be changed using the –o option of gcc
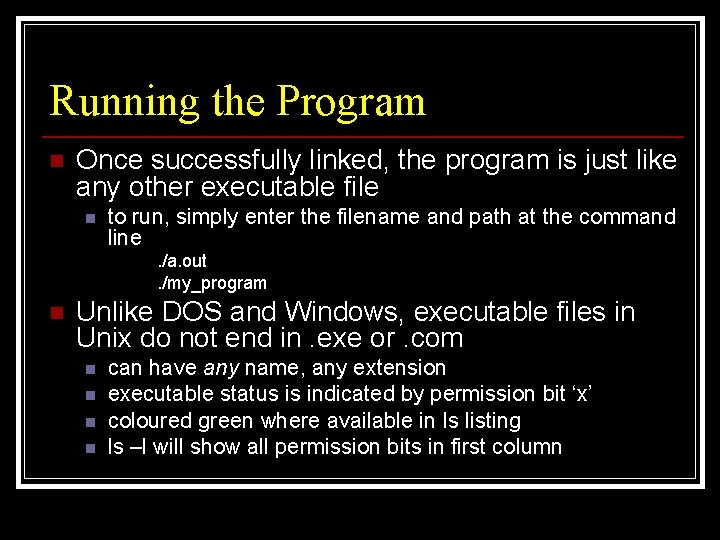
Running the Program n Once successfully linked, the program is just like any other executable file n to run, simply enter the filename and path at the command line. /a. out. /my_program n Unlike DOS and Windows, executable files in Unix do not end in. exe or. com n n can have any name, any extension executable status is indicated by permission bit ‘x’ coloured green where available in ls listing ls –l will show all permission bits in first column
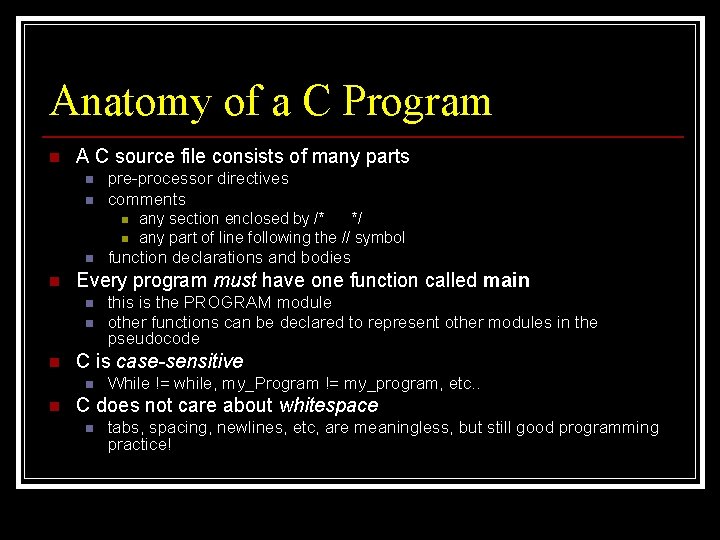
Anatomy of a C Program n A C source file consists of many parts n n pre-processor directives comments n n n this is the PROGRAM module other functions can be declared to represent other modules in the pseudocode C is case-sensitive n n function declarations and bodies Every program must have one function called main n n any section enclosed by /* */ any part of line following the // symbol While != while, my_Program != my_program, etc. . C does not care about whitespace n tabs, spacing, newlines, etc, are meaningless, but still good programming practice!
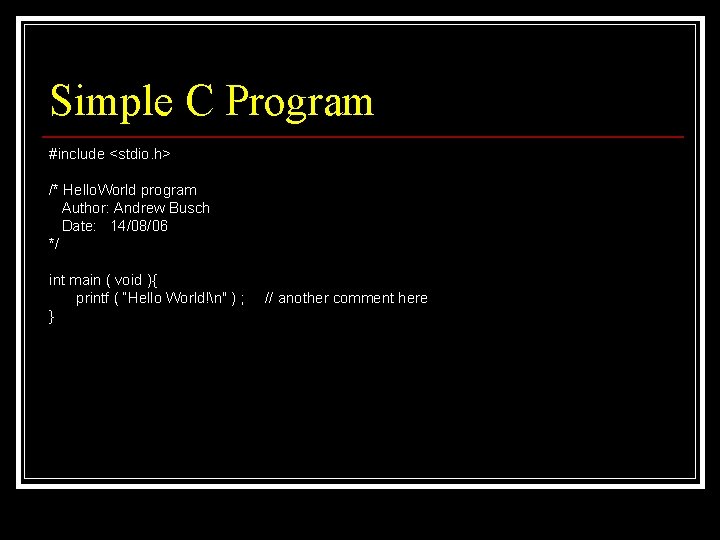
Simple C Program #include <stdio. h> /* Hello. World program Author: Andrew Busch Date: 14/08/06 */ int main ( void ){ printf ( “Hello World!n" ) ; } // another comment here
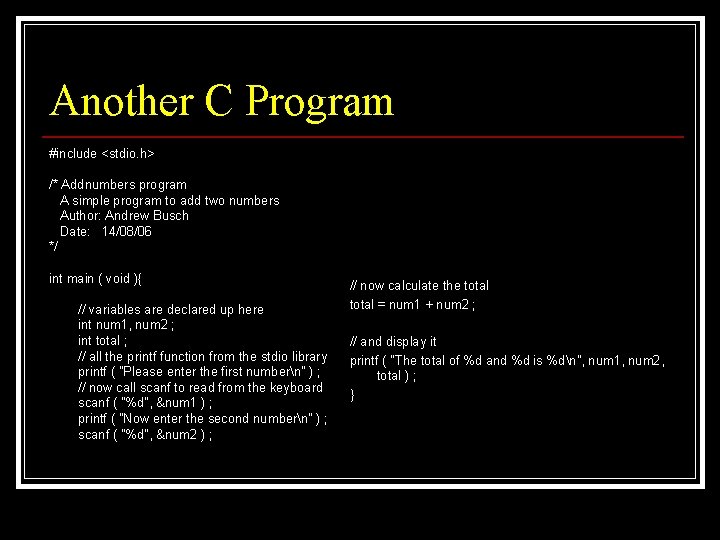
Another C Program #include <stdio. h> /* Addnumbers program A simple program to add two numbers Author: Andrew Busch Date: 14/08/06 */ int main ( void ){ // variables are declared up here int num 1, num 2 ; int total ; // all the printf function from the stdio library printf ( "Please enter the first numbern" ) ; // now call scanf to read from the keyboard scanf ( "%d", &num 1 ) ; printf ( "Now enter the second numbern" ) ; scanf ( "%d", &num 2 ) ; // now calculate the total = num 1 + num 2 ; // and display it printf ( "The total of %d and %d is %dn", num 1, num 2, total ) ; }
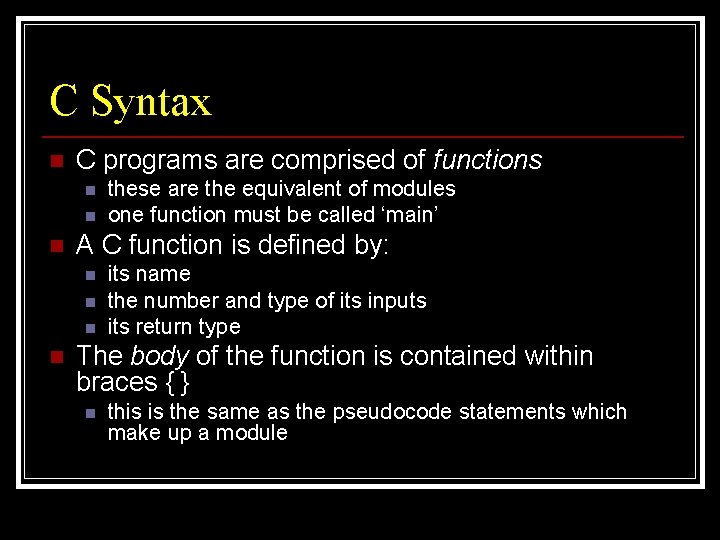
C Syntax n C programs are comprised of functions n n n A C function is defined by: n n these are the equivalent of modules one function must be called ‘main’ its name the number and type of its inputs its return type The body of the function is contained within braces { } n this is the same as the pseudocode statements which make up a module
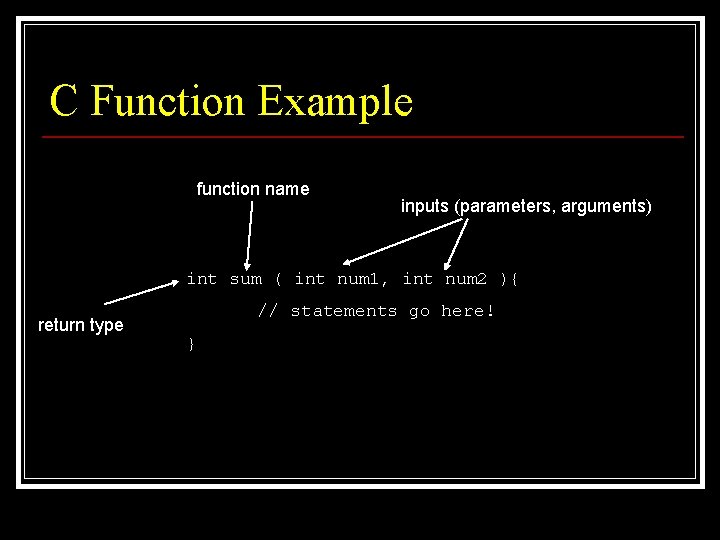
C Function Example function name inputs (parameters, arguments) int sum ( int num 1, int num 2 ){ return type // statements go here! }
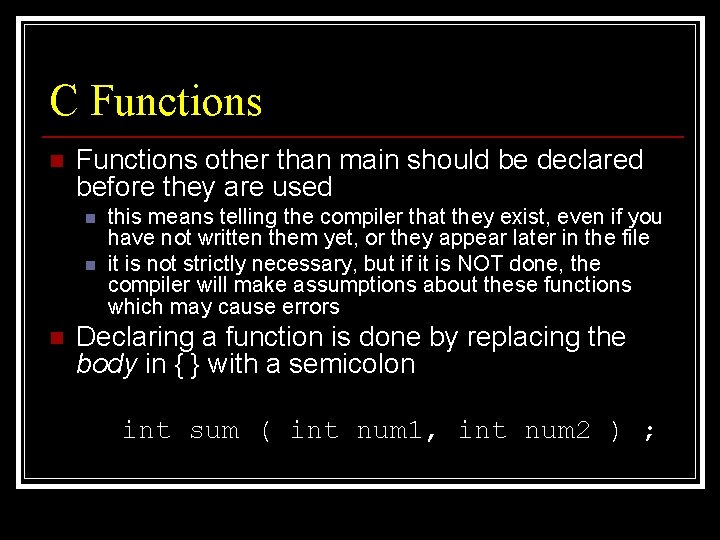
C Functions n Functions other than main should be declared before they are used n n n this means telling the compiler that they exist, even if you have not written them yet, or they appear later in the file it is not strictly necessary, but if it is NOT done, the compiler will make assumptions about these functions which may cause errors Declaring a function is done by replacing the body in { } with a semicolon int sum ( int num 1, int num 2 ) ;
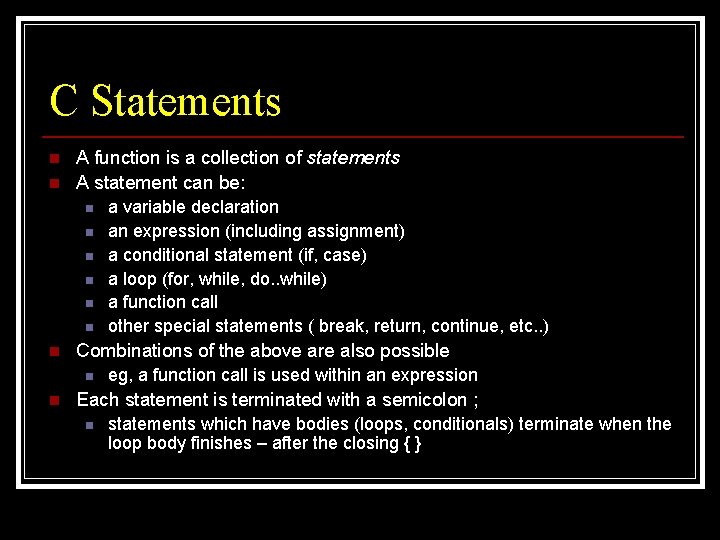
C Statements n n A function is a collection of statements A statement can be: n n n n Combinations of the above are also possible n n a variable declaration an expression (including assignment) a conditional statement (if, case) a loop (for, while, do. . while) a function call other special statements ( break, return, continue, etc. . ) eg, a function call is used within an expression Each statement is terminated with a semicolon ; n statements which have bodies (loops, conditionals) terminate when the loop body finishes – after the closing { }
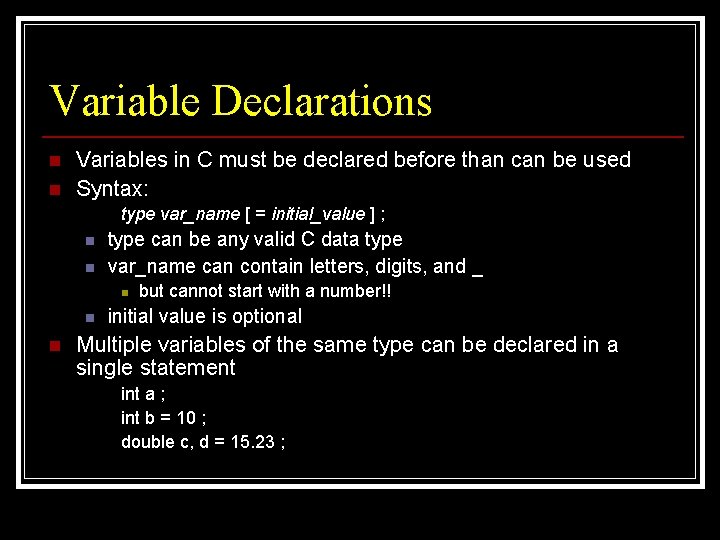
Variable Declarations n n Variables in C must be declared before than can be used Syntax: type var_name [ = initial_value ] ; n n type can be any valid C data type var_name can contain letters, digits, and _ n n n but cannot start with a number!! initial value is optional Multiple variables of the same type can be declared in a single statement int a ; int b = 10 ; double c, d = 15. 23 ;
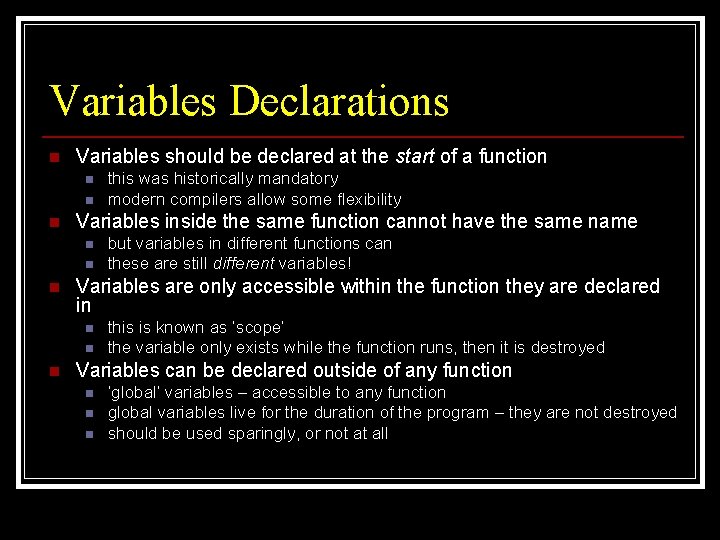
Variables Declarations n Variables should be declared at the start of a function n Variables inside the same function cannot have the same n n n but variables in different functions can these are still different variables! Variables are only accessible within the function they are declared in n this was historically mandatory modern compilers allow some flexibility this is known as ‘scope’ the variable only exists while the function runs, then it is destroyed Variables can be declared outside of any function n ‘global’ variables – accessible to any function global variables live for the duration of the program – they are not destroyed should be used sparingly, or not at all
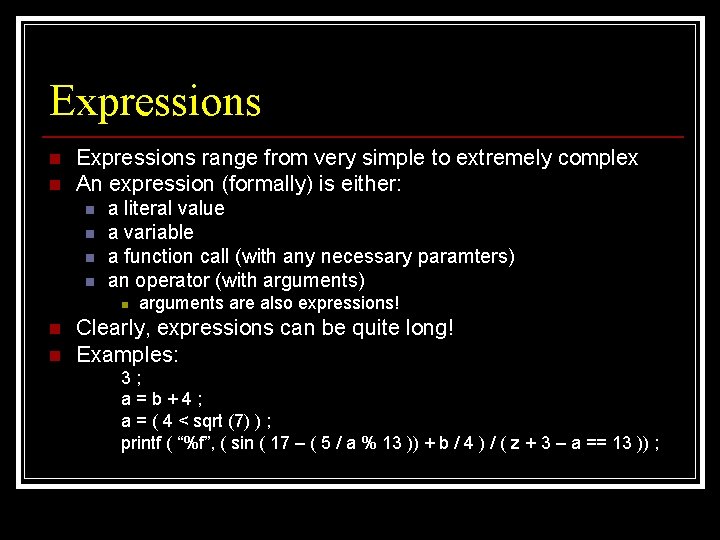
Expressions n n Expressions range from very simple to extremely complex An expression (formally) is either: n n a literal value a variable a function call (with any necessary paramters) an operator (with arguments) n n n arguments are also expressions! Clearly, expressions can be quite long! Examples: 3; a=b+4; a = ( 4 < sqrt (7) ) ; printf ( “%f”, ( sin ( 17 – ( 5 / a % 13 )) + b / 4 ) / ( z + 3 – a == 13 )) ;
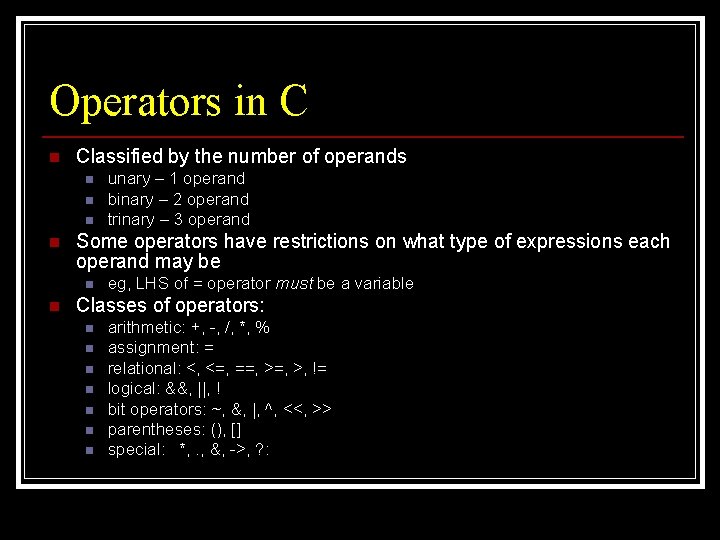
Operators in C n Classified by the number of operands n n Some operators have restrictions on what type of expressions each operand may be n n unary – 1 operand binary – 2 operand trinary – 3 operand eg, LHS of = operator must be a variable Classes of operators: n n n n arithmetic: +, -, /, *, % assignment: = relational: <, <=, ==, >, != logical: &&, ||, ! bit operators: ~, &, |, ^, <<, >> parentheses: (), [] special: *, . , &, ->, ? :
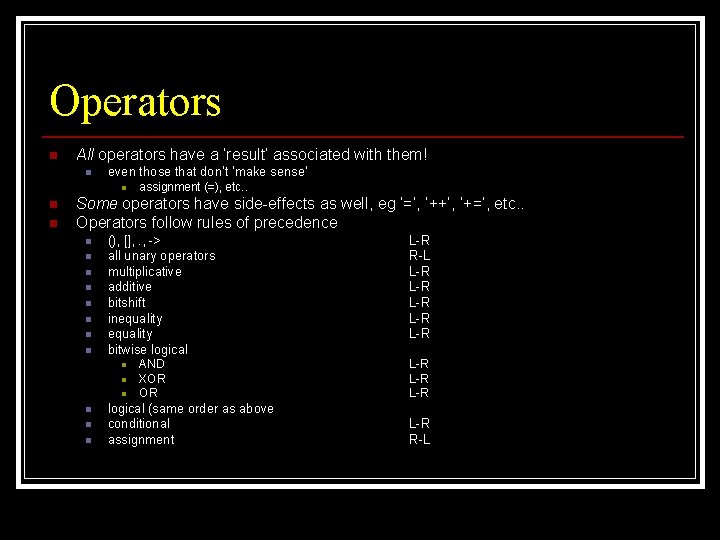
Operators n All operators have a ‘result’ associated with them! n even those that don’t ‘make sense’ n n n assignment (=), etc. . Some operators have side-effects as well, eg ‘=‘, ‘++’, ‘+=‘, etc. . Operators follow rules of precedence n n n n (), [], . , -> all unary operators multiplicative additive bitshift inequality bitwise logical n n n AND XOR OR logical (same order as above conditional assignment L-R R-L L-R L-R L-R R-L
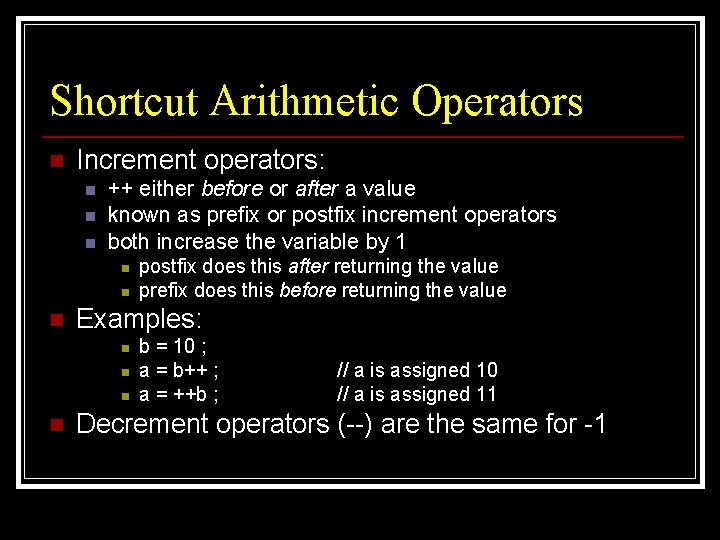
Shortcut Arithmetic Operators n Increment operators: n n n ++ either before or after a value known as prefix or postfix increment operators both increase the variable by 1 n n n Examples: n n postfix does this after returning the value prefix does this before returning the value b = 10 ; a = b++ ; a = ++b ; // a is assigned 10 // a is assigned 11 Decrement operators (--) are the same for -1
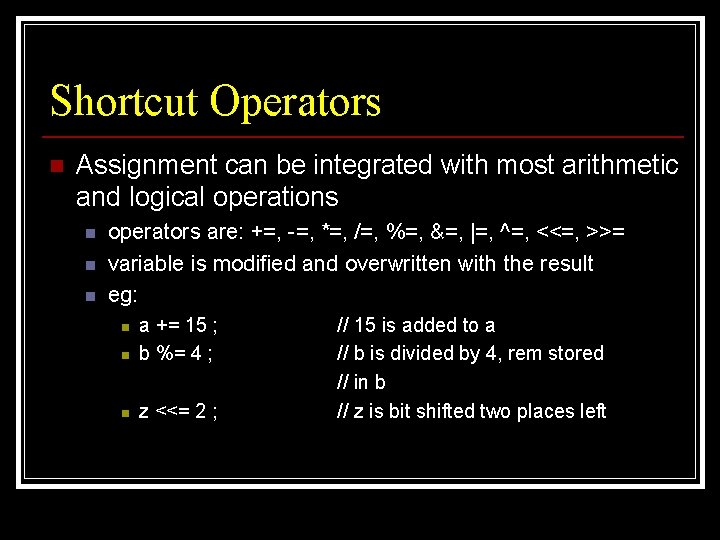
Shortcut Operators n Assignment can be integrated with most arithmetic and logical operations n n n operators are: +=, -=, *=, /=, %=, &=, |=, ^=, <<=, >>= variable is modified and overwritten with the result eg: n a += 15 ; b %= 4 ; n z <<= 2 ; n // 15 is added to a // b is divided by 4, rem stored // in b // z is bit shifted two places left
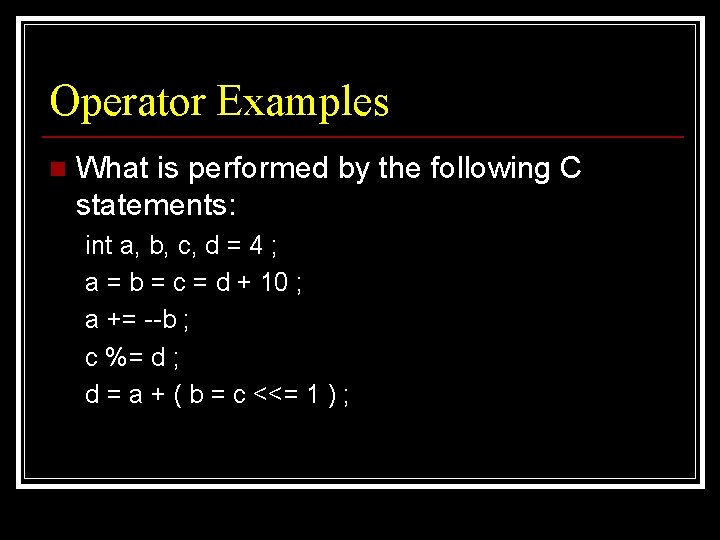
Operator Examples n What is performed by the following C statements: int a, b, c, d = 4 ; a = b = c = d + 10 ; a += --b ; c %= d ; d = a + ( b = c <<= 1 ) ;
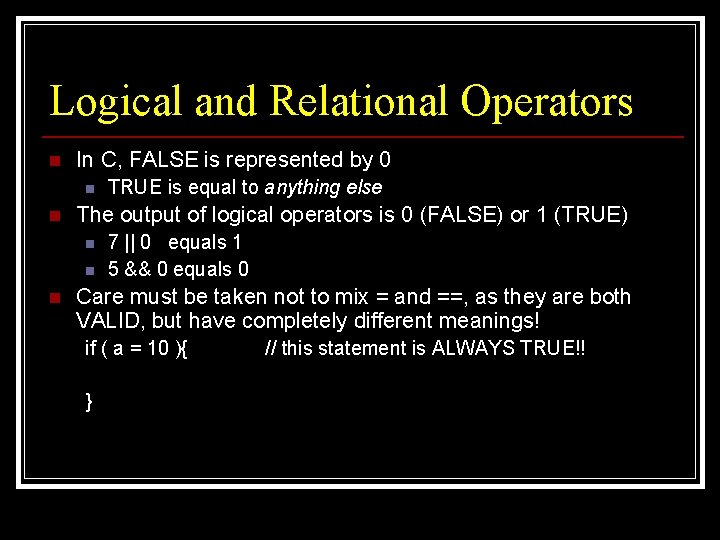
Logical and Relational Operators n In C, FALSE is represented by 0 n n The output of logical operators is 0 (FALSE) or 1 (TRUE) n n n TRUE is equal to anything else 7 || 0 equals 1 5 && 0 equals 0 Care must be taken not to mix = and ==, as they are both VALID, but have completely different meanings! if ( a = 10 ){ } // this statement is ALWAYS TRUE!!
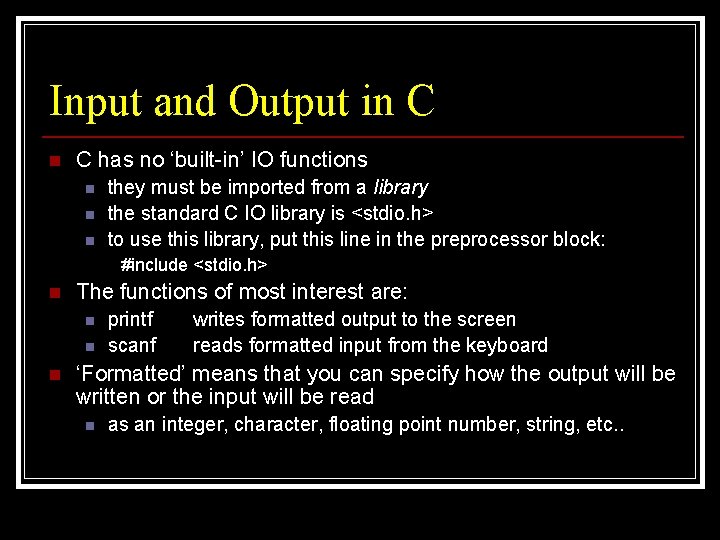
Input and Output in C has no ‘built-in’ IO functions n n n they must be imported from a library the standard C IO library is <stdio. h> to use this library, put this line in the preprocessor block: #include <stdio. h> n The functions of most interest are: n n n printf scanf writes formatted output to the screen reads formatted input from the keyboard ‘Formatted’ means that you can specify how the output will be written or the input will be read n as an integer, character, floating point number, string, etc. .
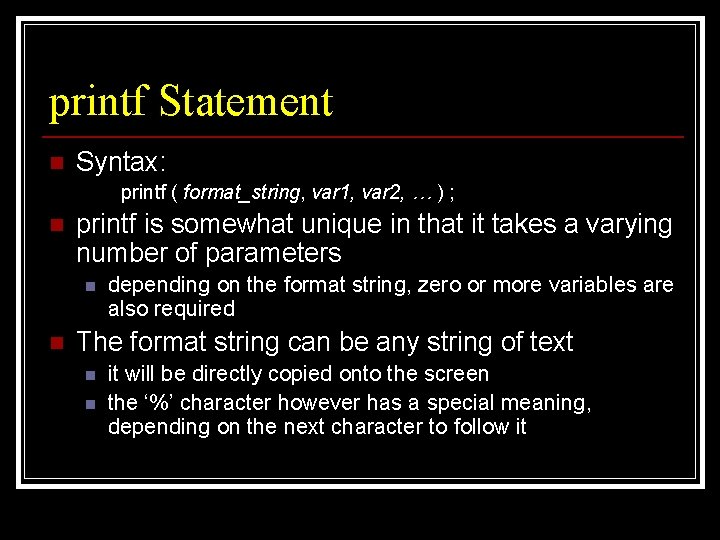
printf Statement n Syntax: printf ( format_string, var 1, var 2, … ) ; n printf is somewhat unique in that it takes a varying number of parameters n n depending on the format string, zero or more variables are also required The format string can be any string of text n n it will be directly copied onto the screen the ‘%’ character however has a special meaning, depending on the next character to follow it
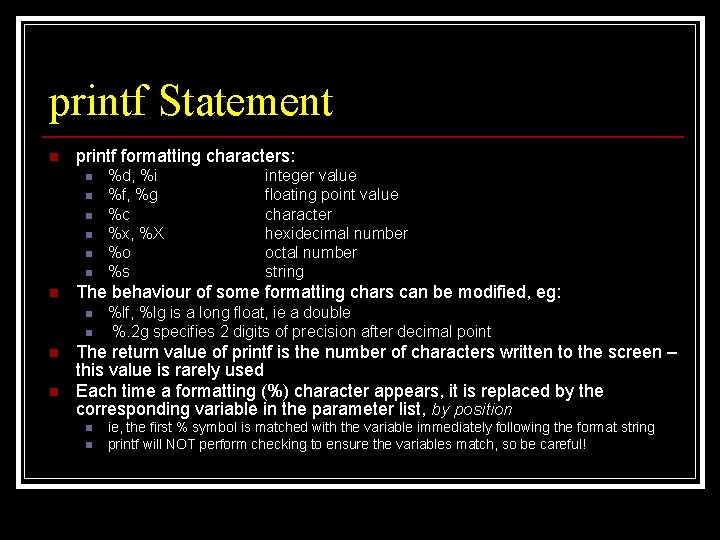
printf Statement n printf formatting characters: n n n n n integer value floating point value character hexidecimal number octal number string The behaviour of some formatting chars can be modified, eg: n n %d, %i %f, %g %c %x, %X %o %s %lf, %lg is a long float, ie a double %. 2 g specifies 2 digits of precision after decimal point The return value of printf is the number of characters written to the screen – this value is rarely used Each time a formatting (%) character appears, it is replaced by the corresponding variable in the parameter list, by position n n ie, the first % symbol is matched with the variable immediately following the format string printf will NOT perform checking to ensure the variables match, so be careful!
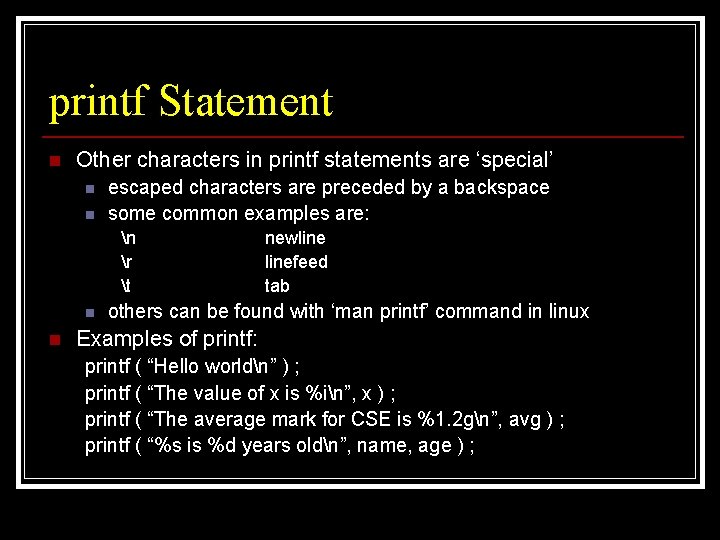
printf Statement n Other characters in printf statements are ‘special’ n n escaped characters are preceded by a backspace some common examples are: n r t n n newlinefeed tab others can be found with ‘man printf’ command in linux Examples of printf: printf ( “Hello worldn” ) ; printf ( “The value of x is %in”, x ) ; printf ( “The average mark for CSE is %1. 2 gn”, avg ) ; printf ( “%s is %d years oldn”, name, age ) ;
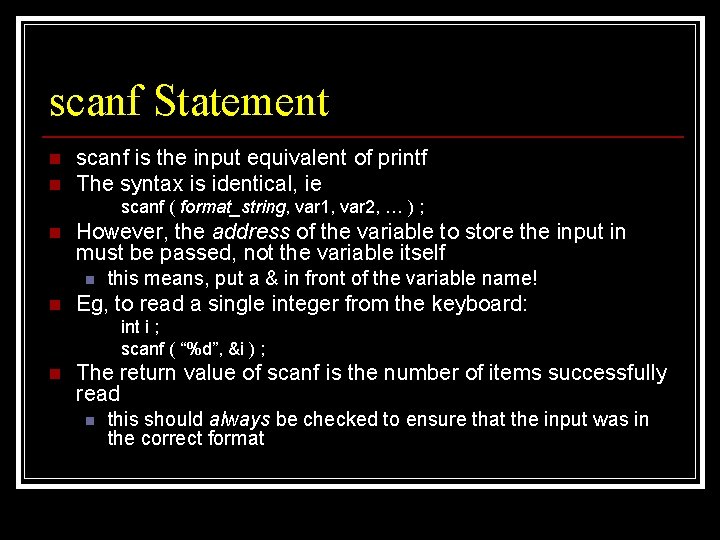
scanf Statement n n scanf is the input equivalent of printf The syntax is identical, ie scanf ( format_string, var 1, var 2, … ) ; n However, the address of the variable to store the input in must be passed, not the variable itself n n this means, put a & in front of the variable name! Eg, to read a single integer from the keyboard: int i ; scanf ( “%d”, &i ) ; n The return value of scanf is the number of items successfully read n this should always be checked to ensure that the input was in the correct format
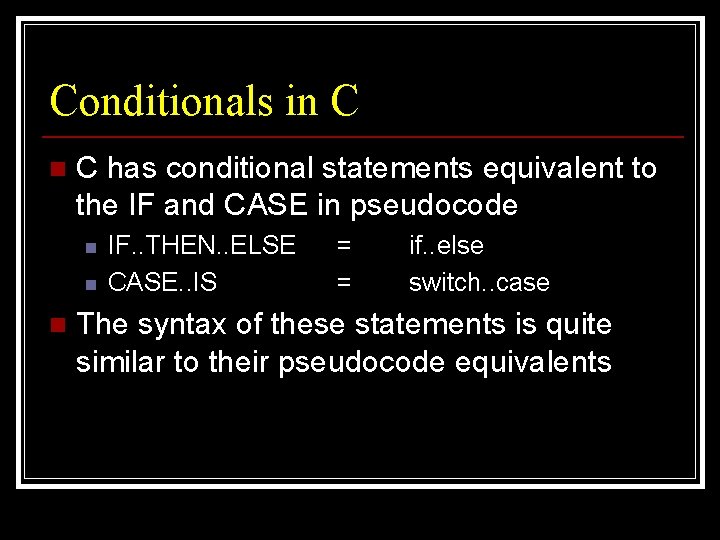
Conditionals in C has conditional statements equivalent to the IF and CASE in pseudocode n n n IF. . THEN. . ELSE CASE. . IS = = if. . else switch. . case The syntax of these statements is quite similar to their pseudocode equivalents
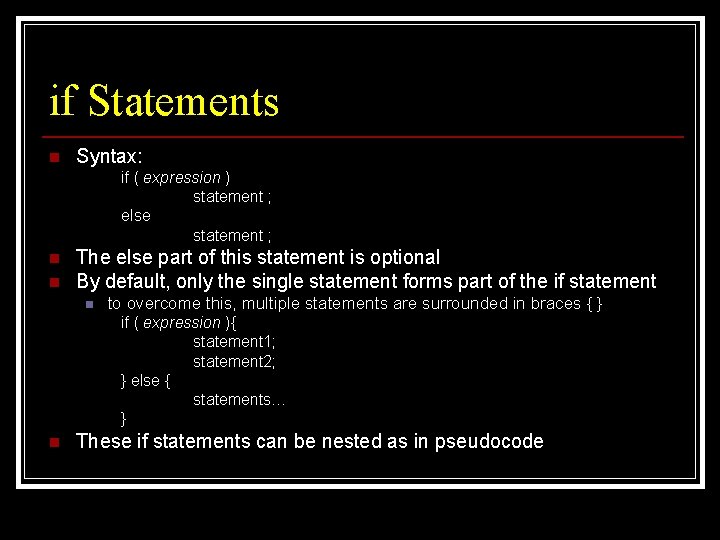
if Statements n Syntax: if ( expression ) statement ; else statement ; n n The else part of this statement is optional By default, only the single statement forms part of the if statement n to overcome this, multiple statements are surrounded in braces { } if ( expression ){ statement 1; statement 2; } else { statements… } n These if statements can be nested as in pseudocode
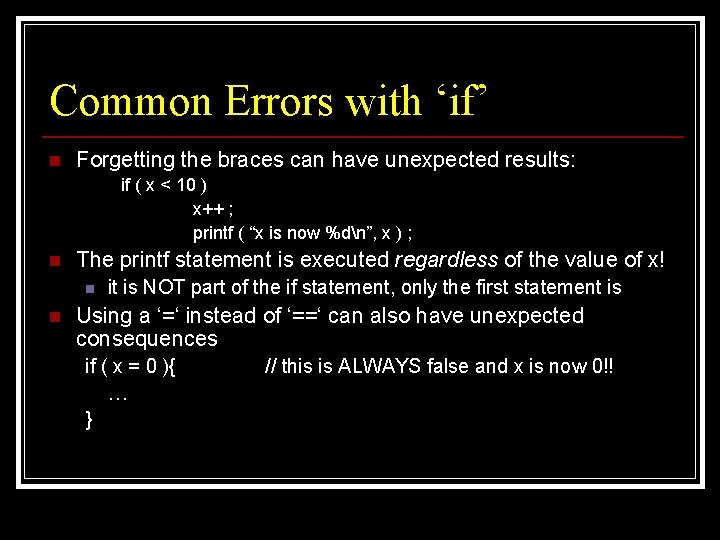
Common Errors with ‘if’ n Forgetting the braces can have unexpected results: if ( x < 10 ) x++ ; printf ( “x is now %dn”, x ) ; n The printf statement is executed regardless of the value of x! n n it is NOT part of the if statement, only the first statement is Using a ‘=‘ instead of ‘==‘ can also have unexpected consequences if ( x = 0 ){ … } // this is ALWAYS false and x is now 0!!
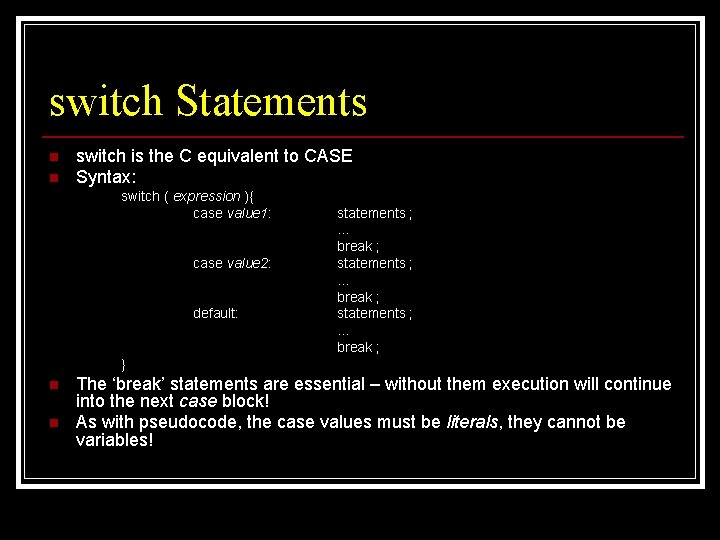
switch Statements n n switch is the C equivalent to CASE Syntax: switch ( expression ){ case value 1: case value 2: default: statements ; … break ; } n n The ‘break’ statements are essential – without them execution will continue into the next case block! As with pseudocode, the case values must be literals, they cannot be variables!
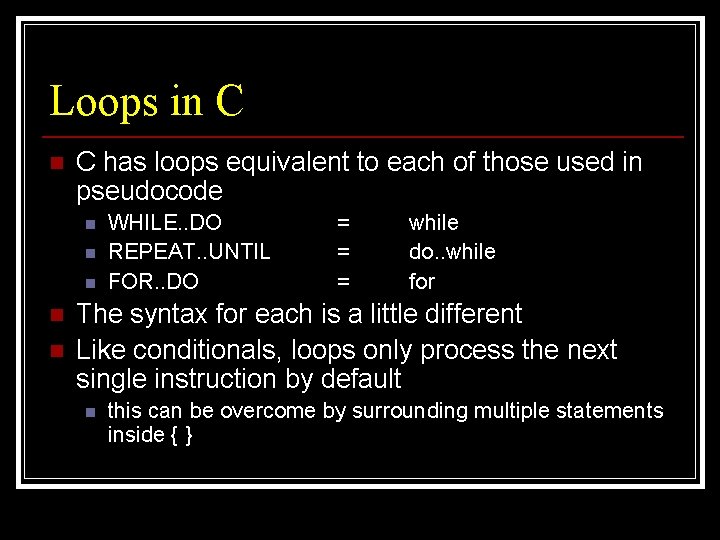
Loops in C has loops equivalent to each of those used in pseudocode n n n WHILE. . DO REPEAT. . UNTIL FOR. . DO = = = while do. . while for The syntax for each is a little different Like conditionals, loops only process the next single instruction by default n this can be overcome by surrounding multiple statements inside { }
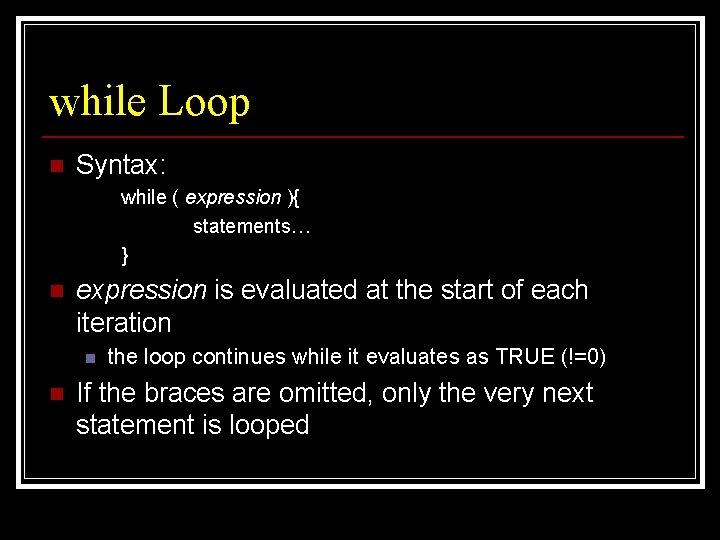
while Loop n Syntax: while ( expression ){ statements… } n expression is evaluated at the start of each iteration n n the loop continues while it evaluates as TRUE (!=0) If the braces are omitted, only the very next statement is looped
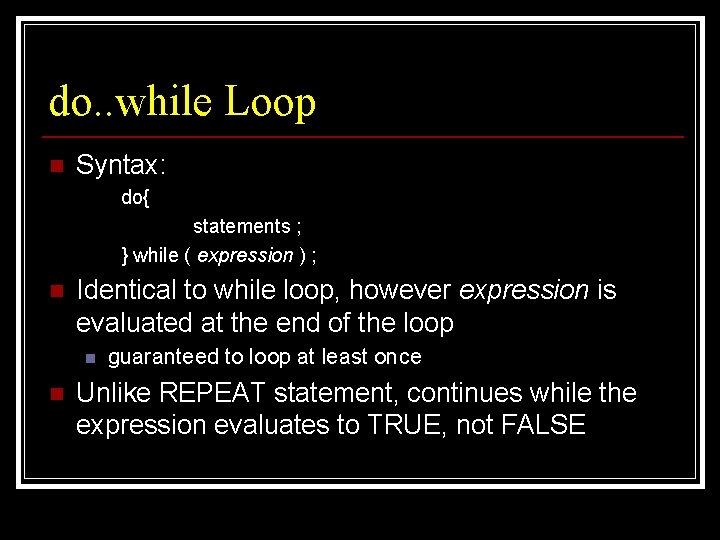
do. . while Loop n Syntax: do{ statements ; } while ( expression ) ; n Identical to while loop, however expression is evaluated at the end of the loop n n guaranteed to loop at least once Unlike REPEAT statement, continues while the expression evaluates to TRUE, not FALSE
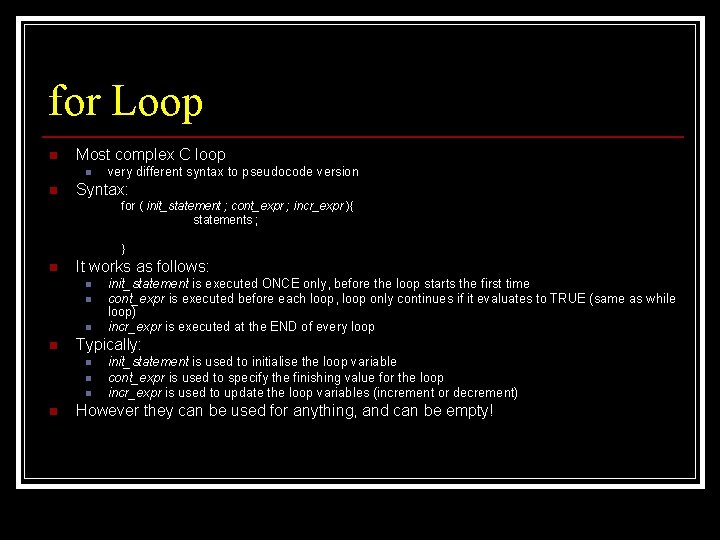
for Loop n Most complex C loop n n very different syntax to pseudocode version Syntax: for ( init_statement ; cont_expr ; incr_expr ){ statements ; } n It works as follows: n n Typically: n n init_statement is executed ONCE only, before the loop starts the first time cont_expr is executed before each loop, loop only continues if it evaluates to TRUE (same as while loop) incr_expr is executed at the END of every loop init_statement is used to initialise the loop variable cont_expr is used to specify the finishing value for the loop incr_expr is used to update the loop variables (increment or decrement) However they can be used for anything, and can be empty!
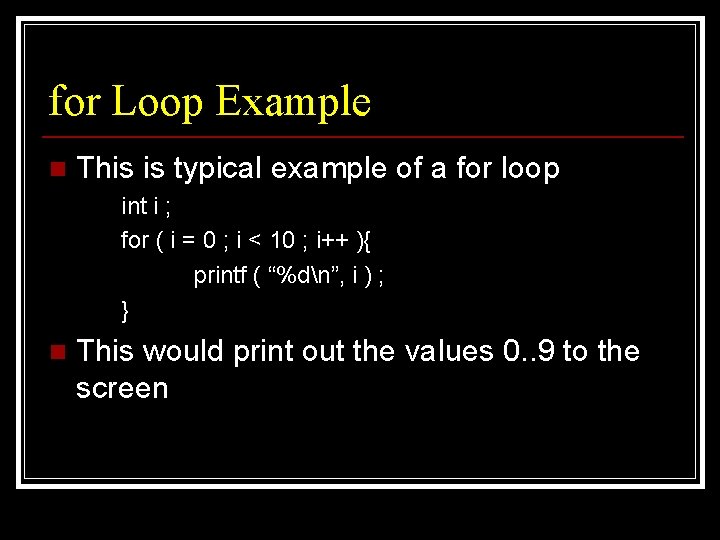
for Loop Example n This is typical example of a for loop int i ; for ( i = 0 ; i < 10 ; i++ ){ printf ( “%dn”, i ) ; } n This would print out the values 0. . 9 to the screen
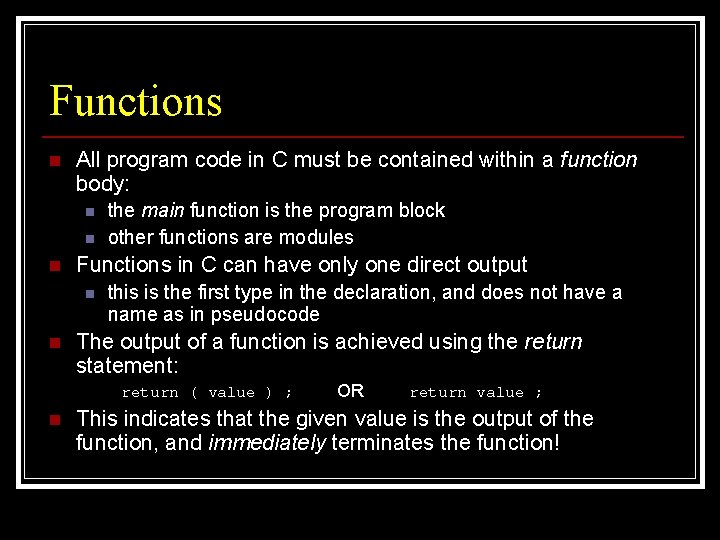
Functions n All program code in C must be contained within a function body: n n n Functions in C can have only one direct output n n the main function is the program block other functions are modules this is the first type in the declaration, and does not have a name as in pseudocode The output of a function is achieved using the return statement: return ( value ) ; n OR return value ; This indicates that the given value is the output of the function, and immediately terminates the function!
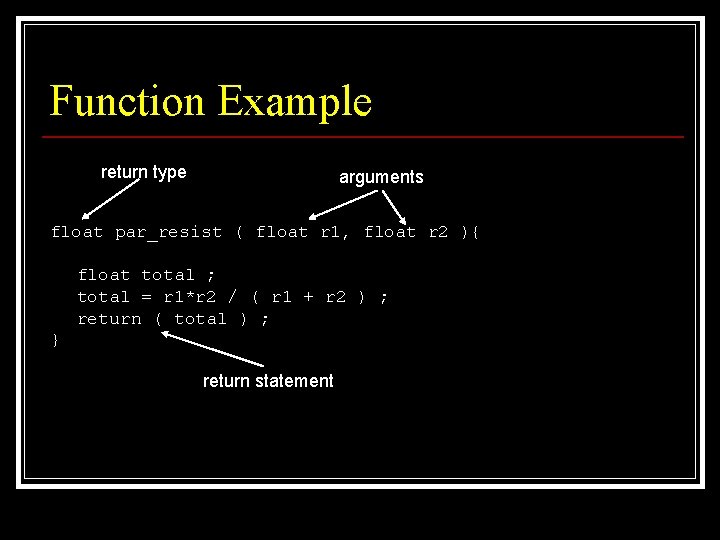
Function Example return type arguments float par_resist ( float r 1, float r 2 ){ float total ; total = r 1*r 2 / ( r 1 + r 2 ) ; return ( total ) ; } return statement
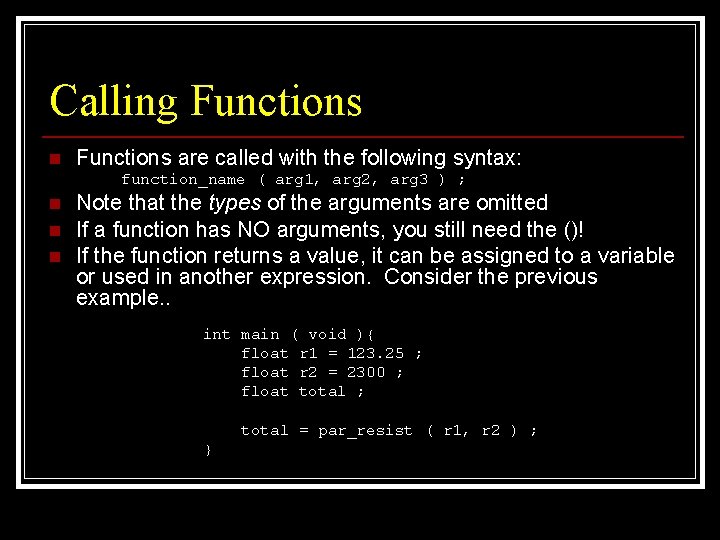
Calling Functions n Functions are called with the following syntax: function_name ( arg 1, arg 2, arg 3 ) ; n n n Note that the types of the arguments are omitted If a function has NO arguments, you still need the ()! If the function returns a value, it can be assigned to a variable or used in another expression. Consider the previous example. . int main ( void ){ float r 1 = 123. 25 ; float r 2 = 2300 ; float total ; total = par_resist ( r 1, r 2 ) ; }