Interfaces and Inner Classes What is an Interface
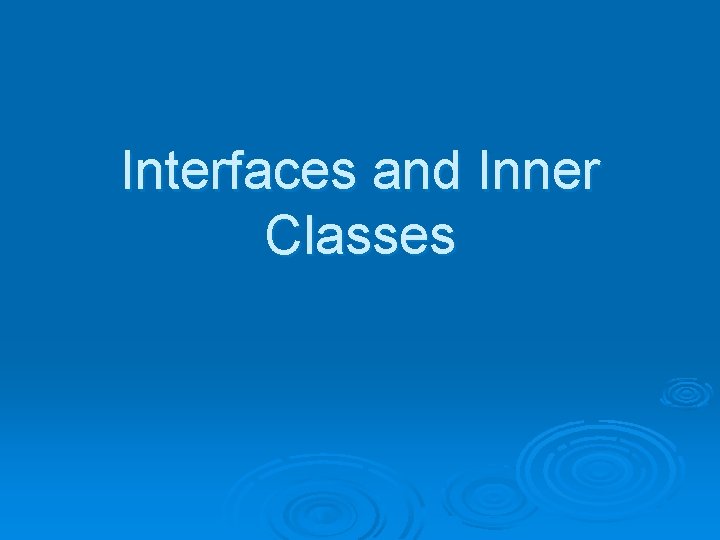
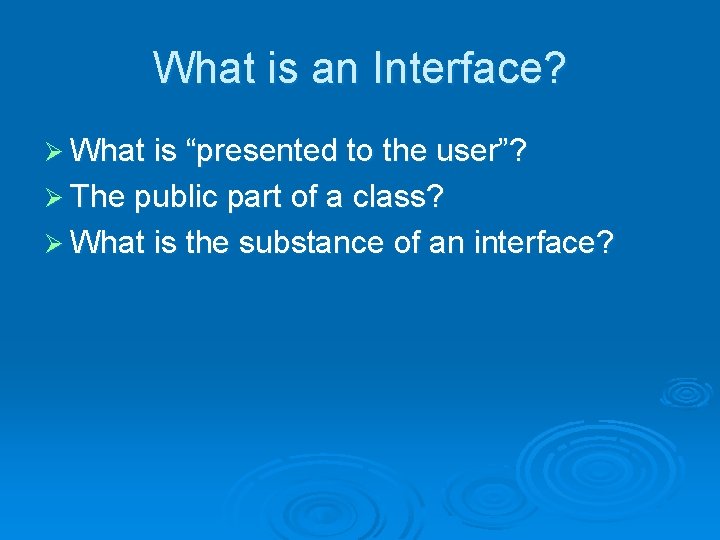
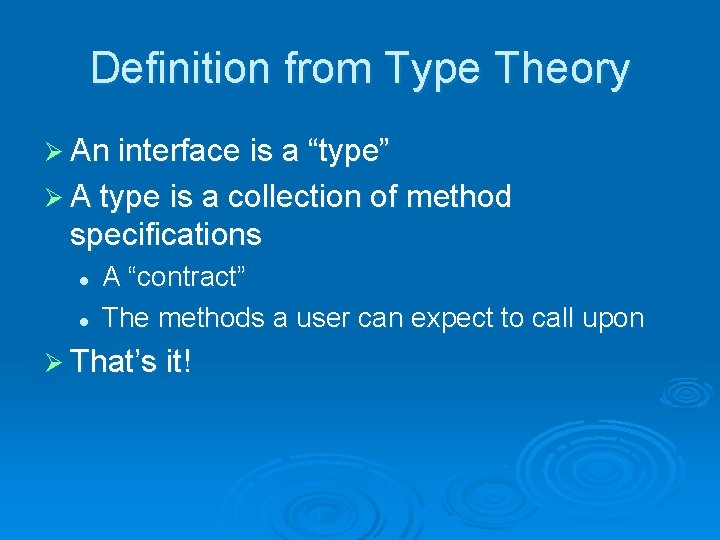
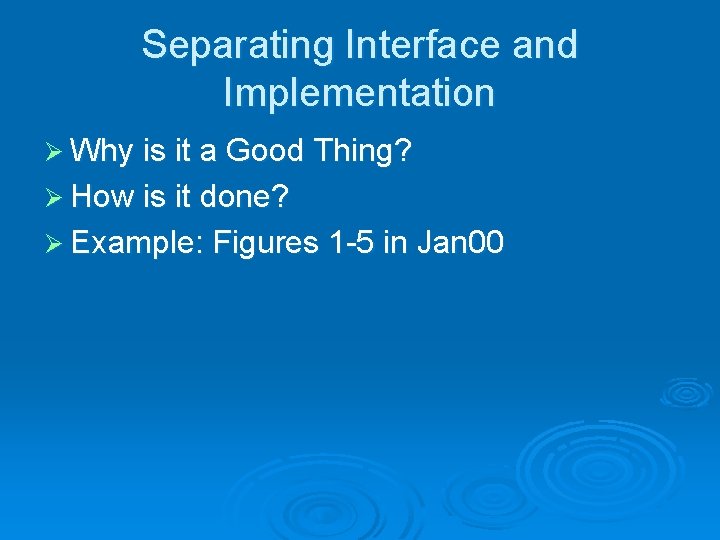
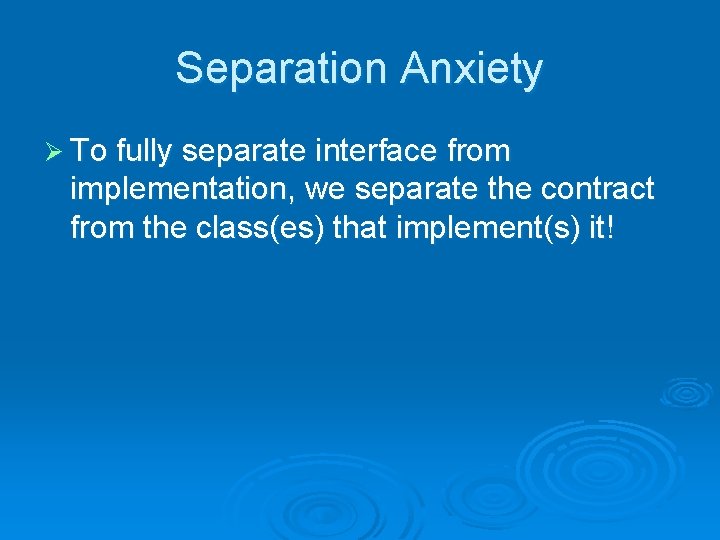
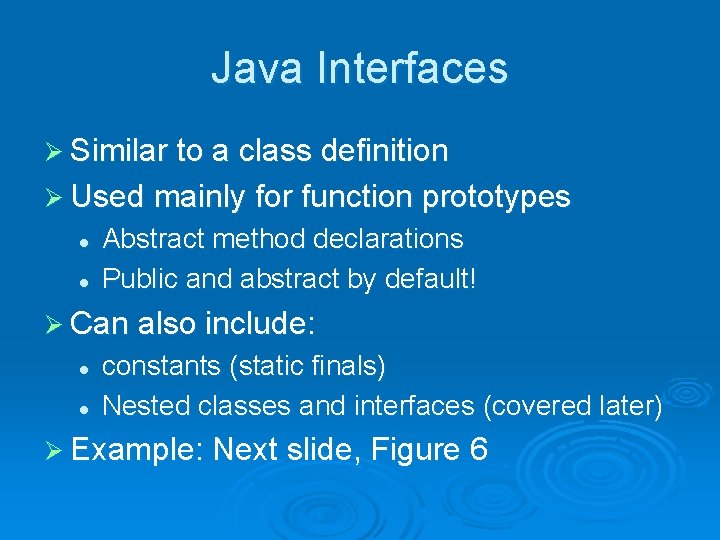
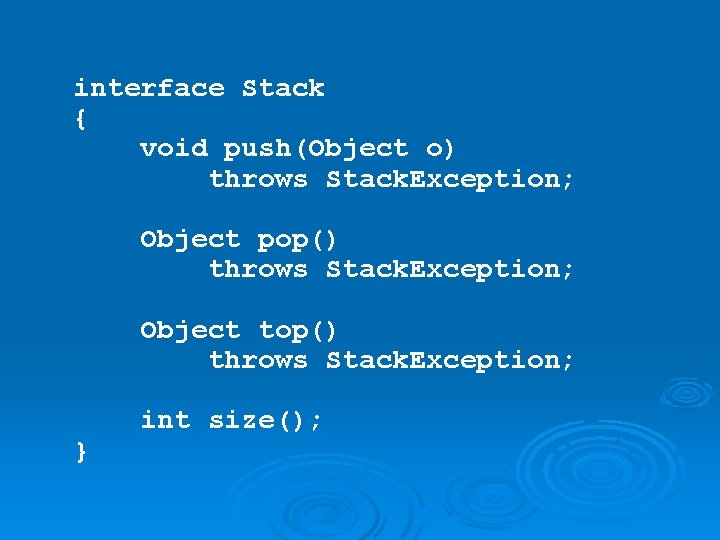
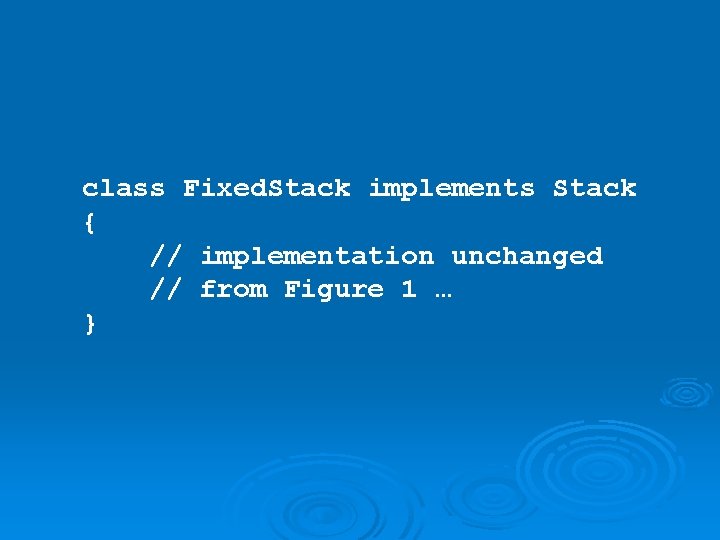
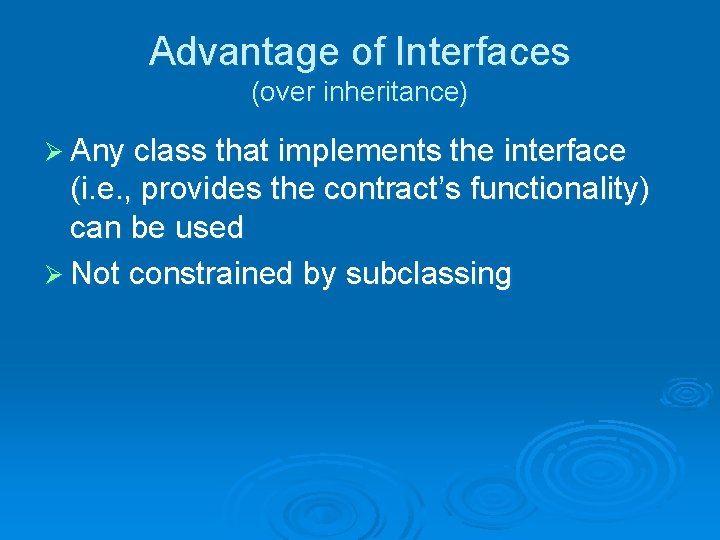
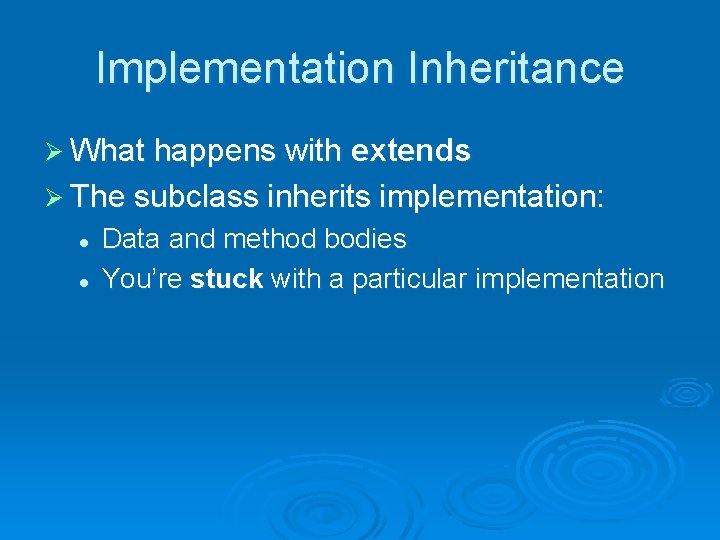
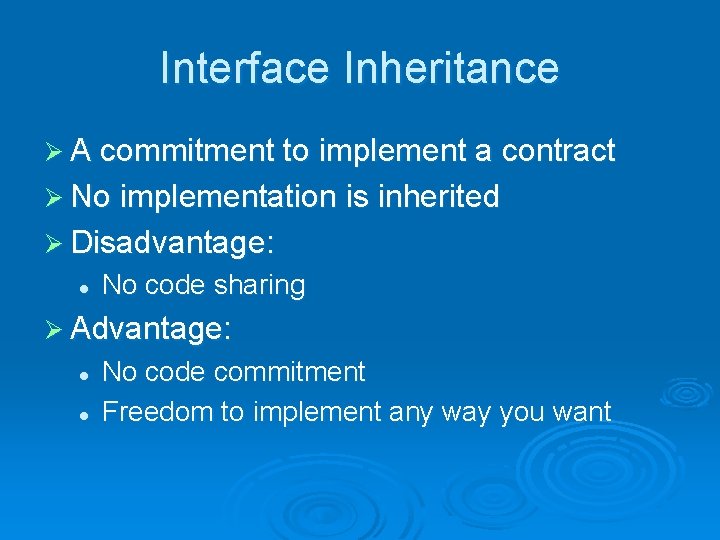
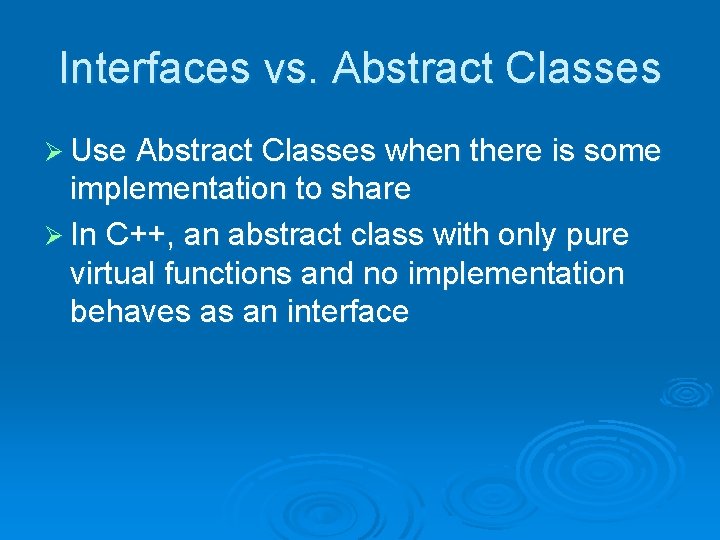
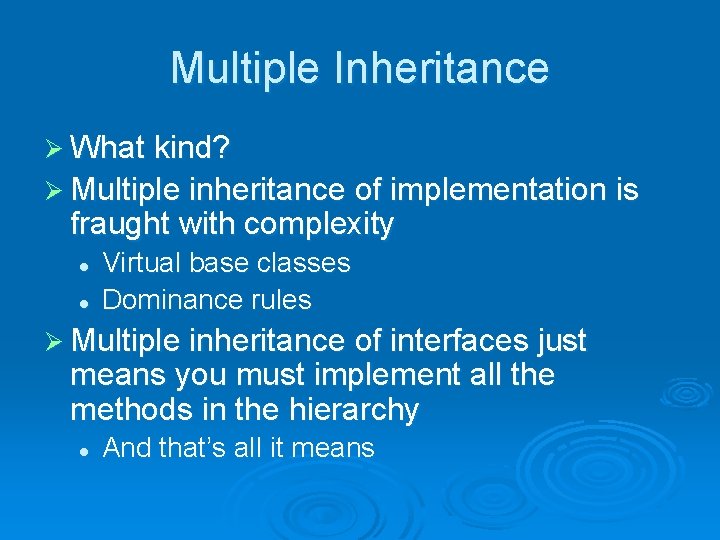
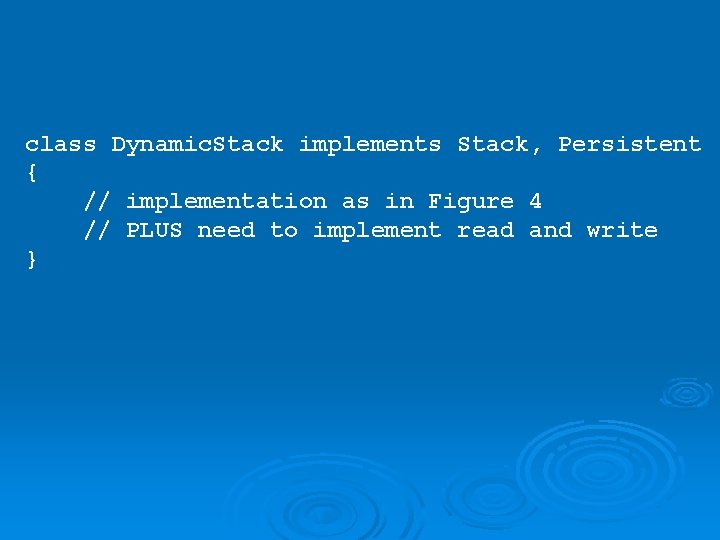
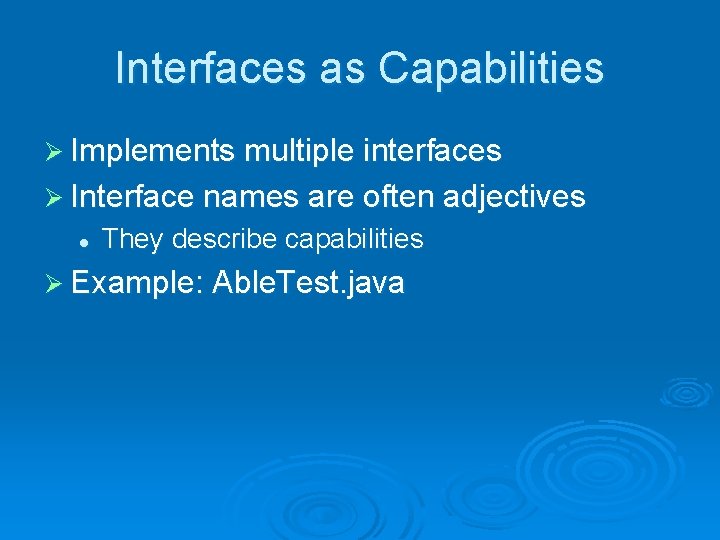
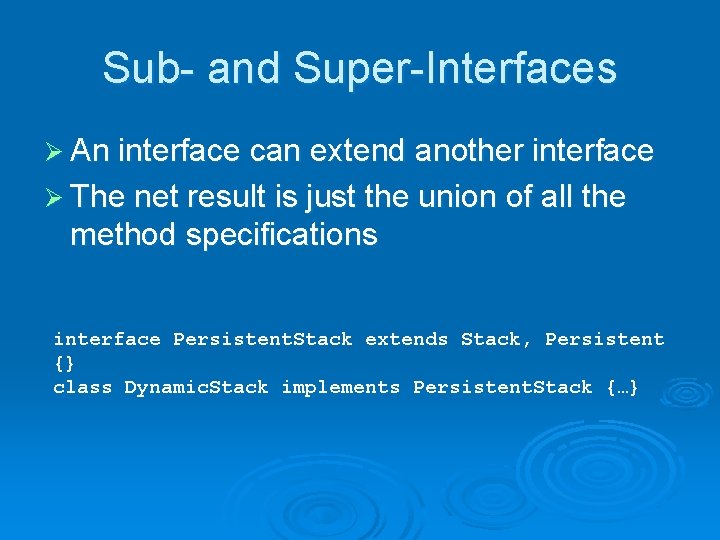
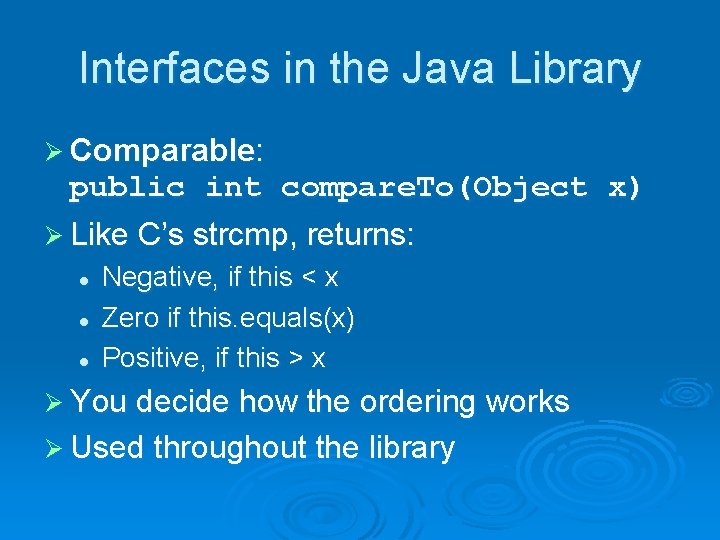
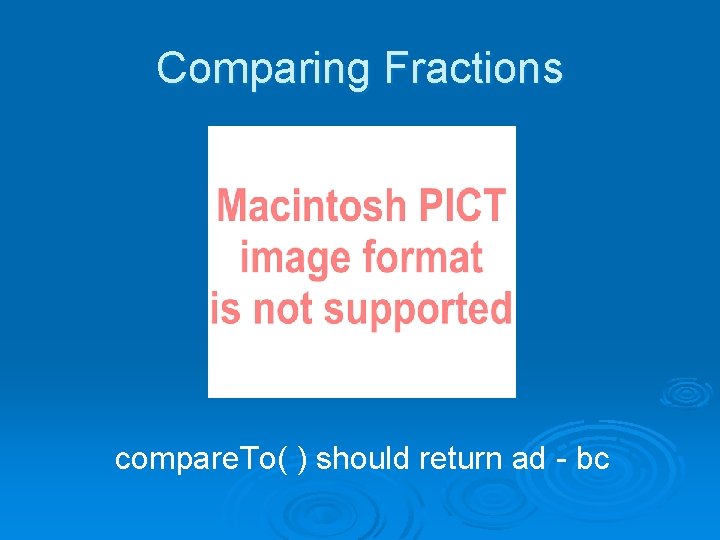
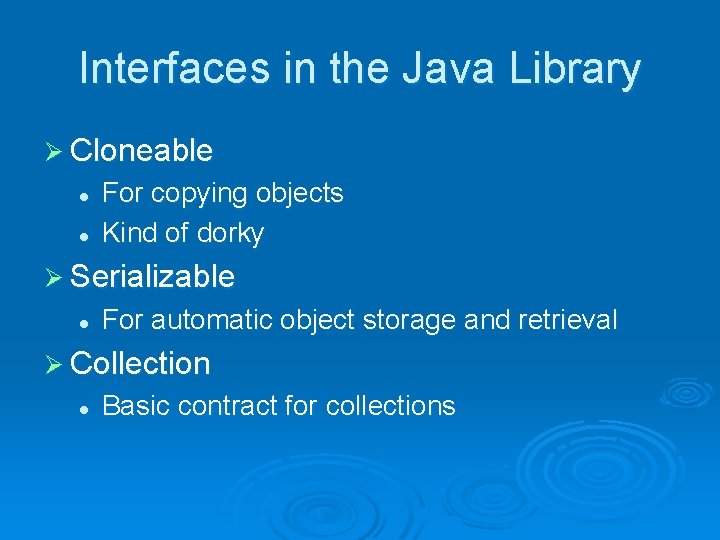
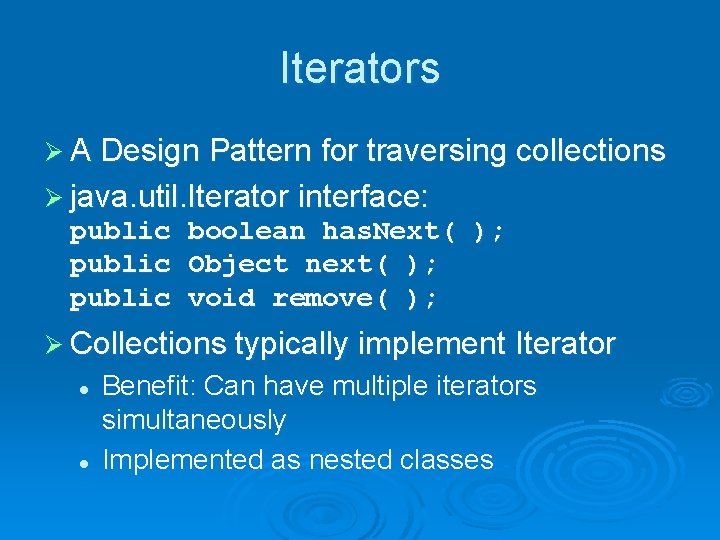
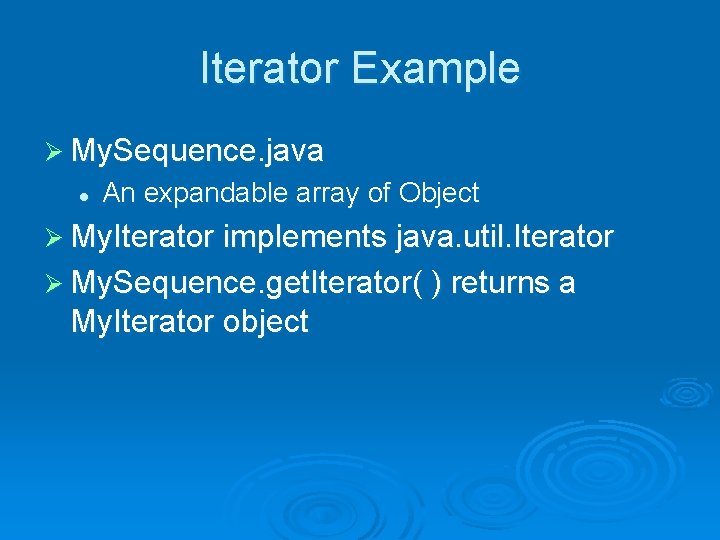
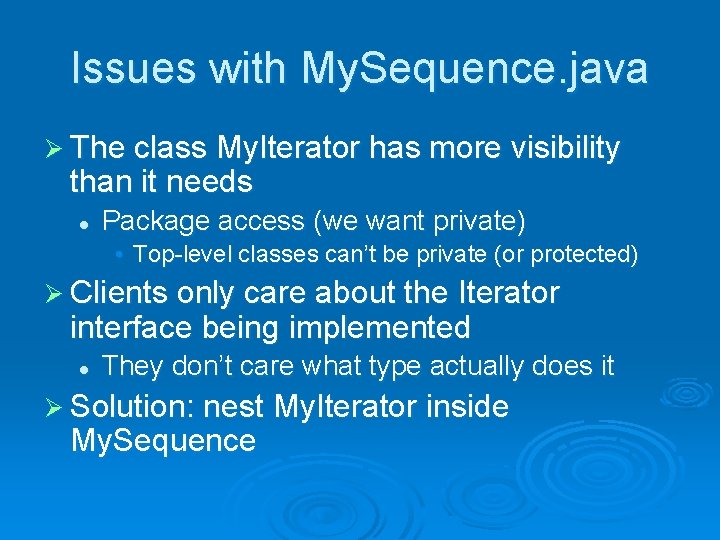
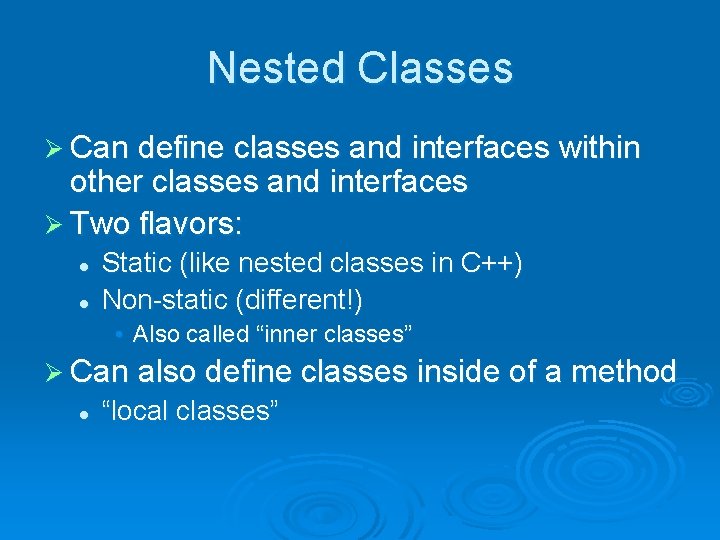
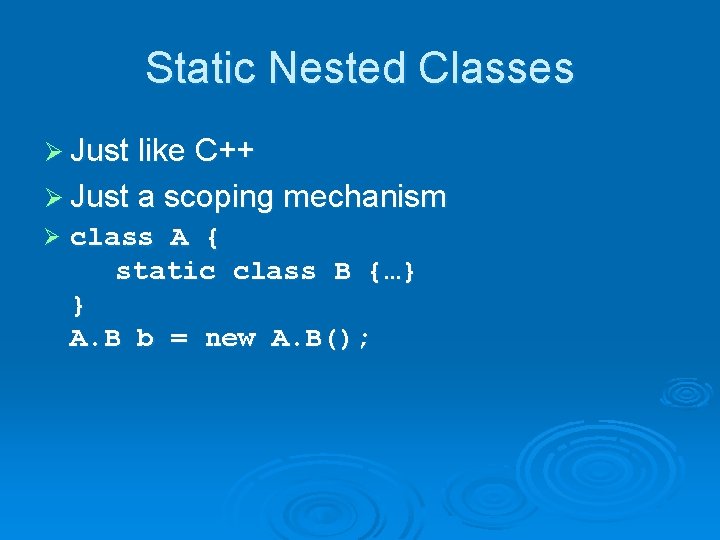
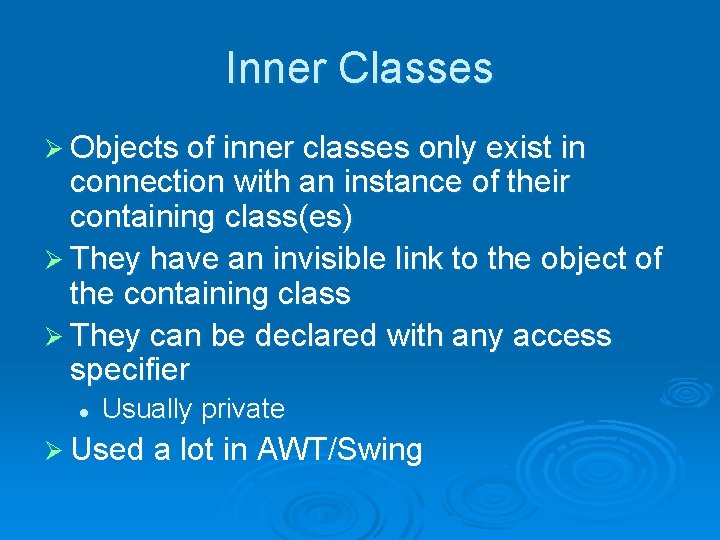
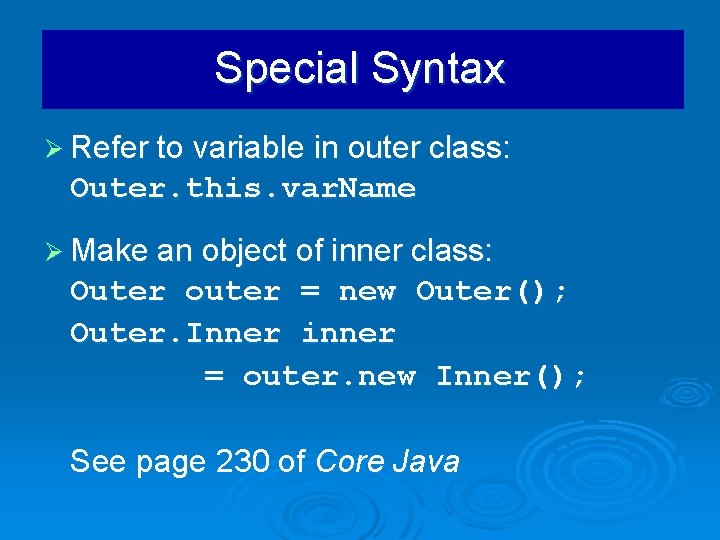
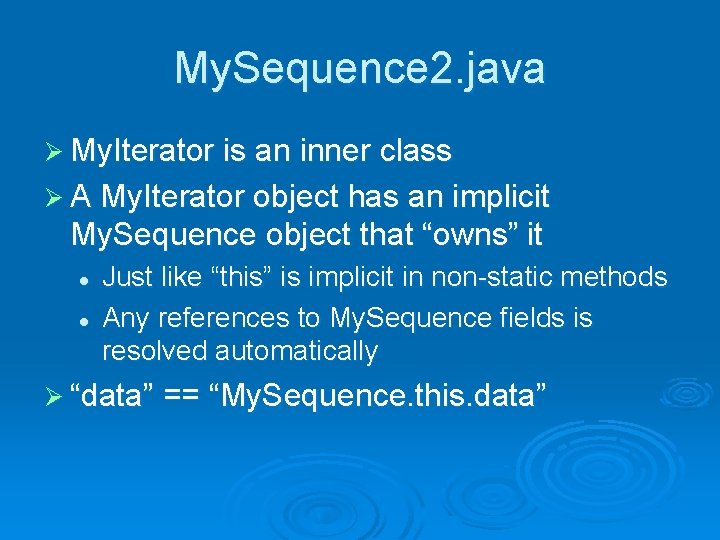
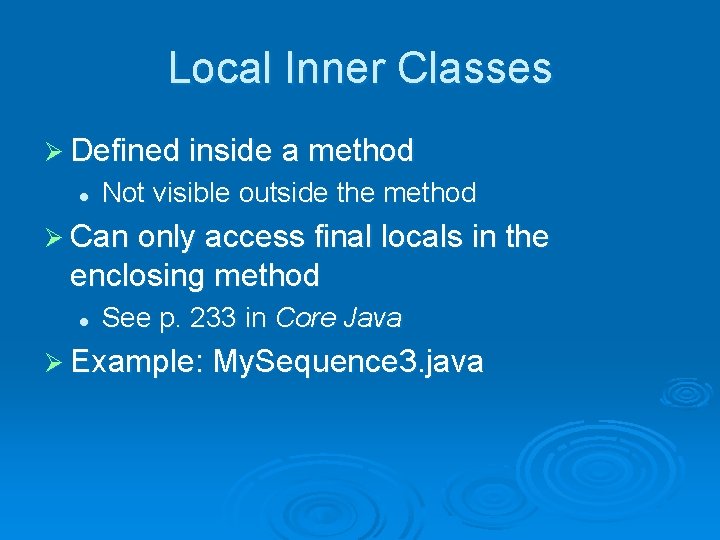
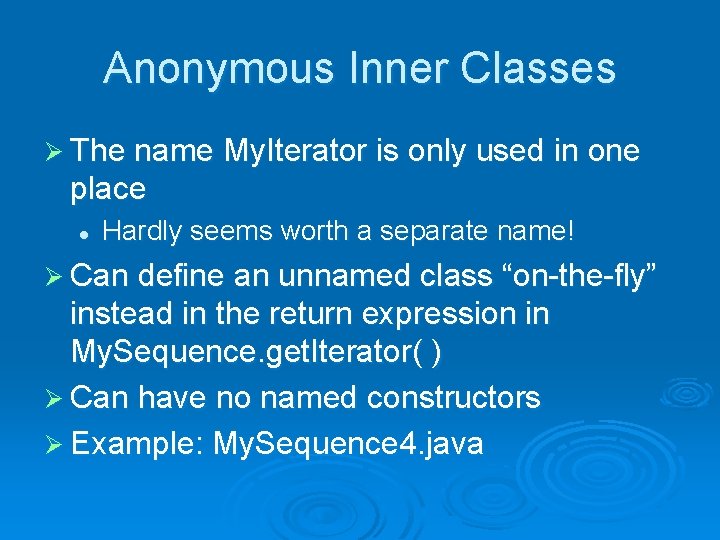
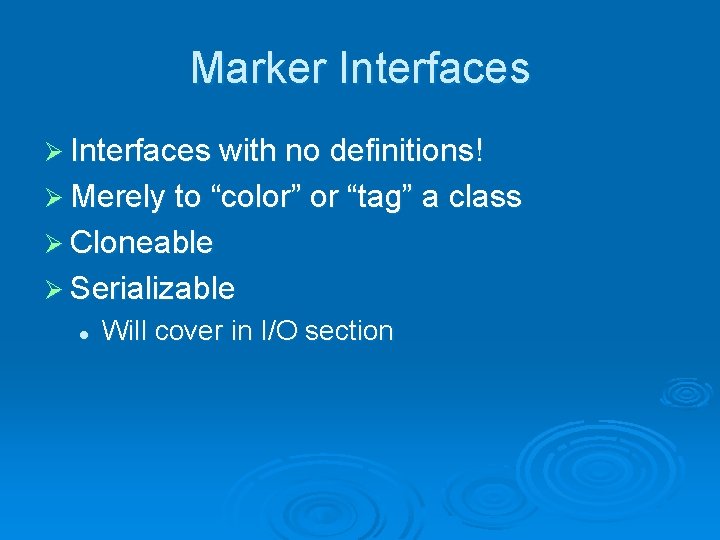
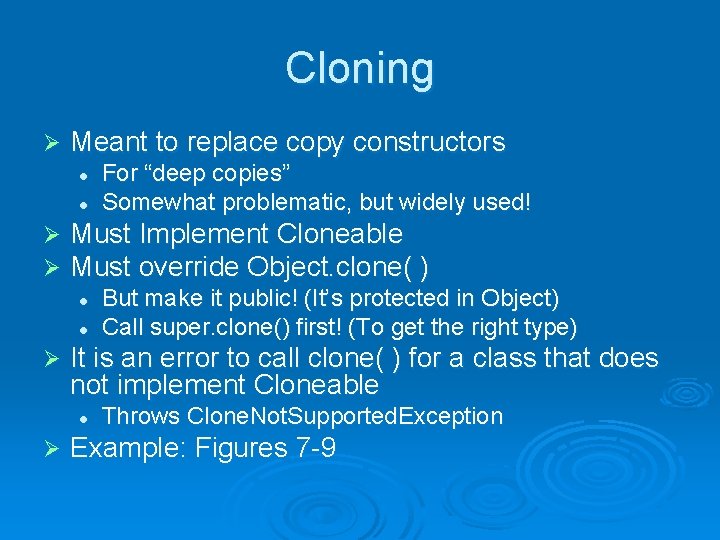
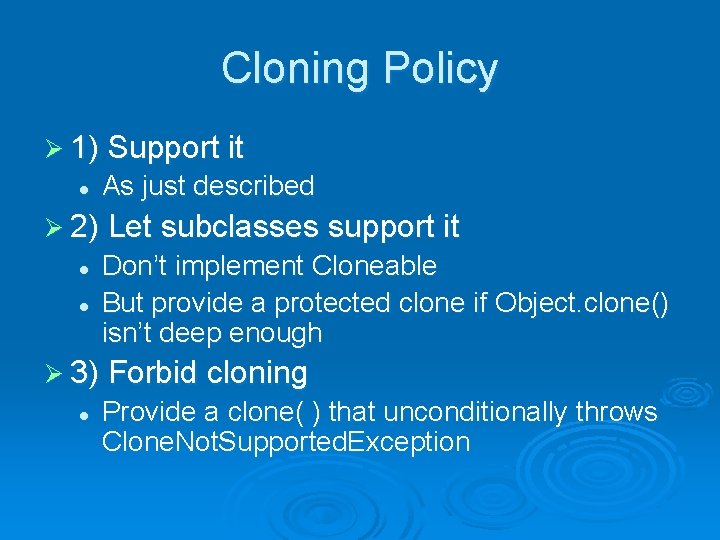
- Slides: 32
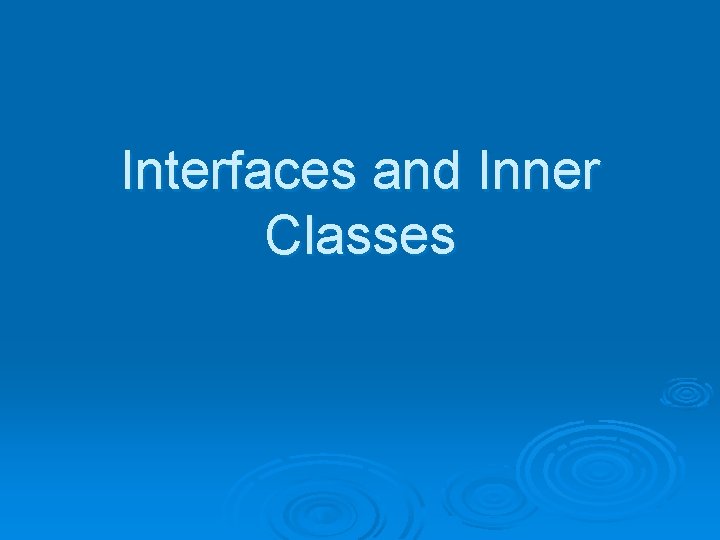
Interfaces and Inner Classes
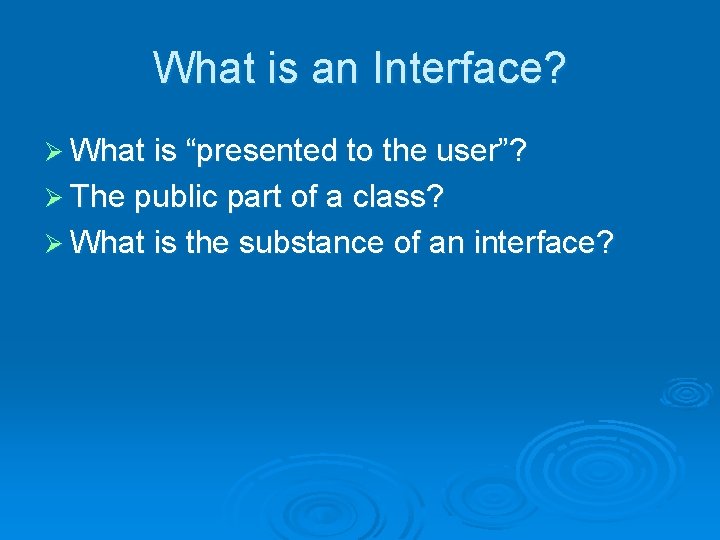
What is an Interface? Ø What is “presented to the user”? Ø The public part of a class? Ø What is the substance of an interface?
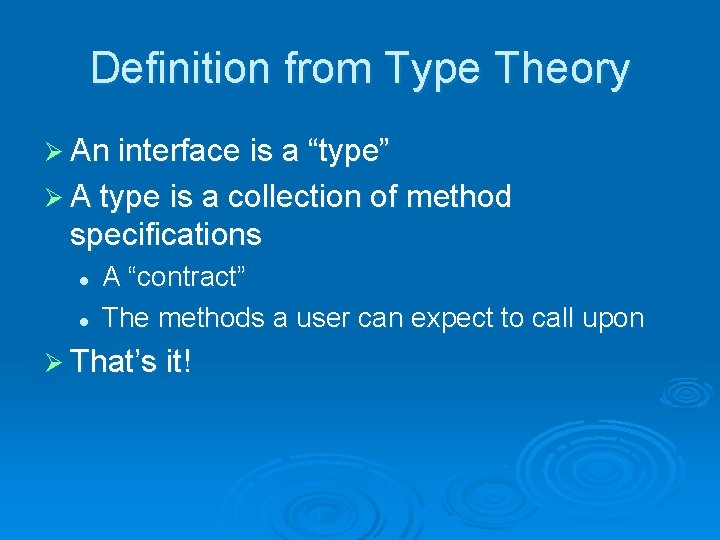
Definition from Type Theory Ø An interface is a “type” Ø A type is a collection of method specifications l l A “contract” The methods a user can expect to call upon Ø That’s it!
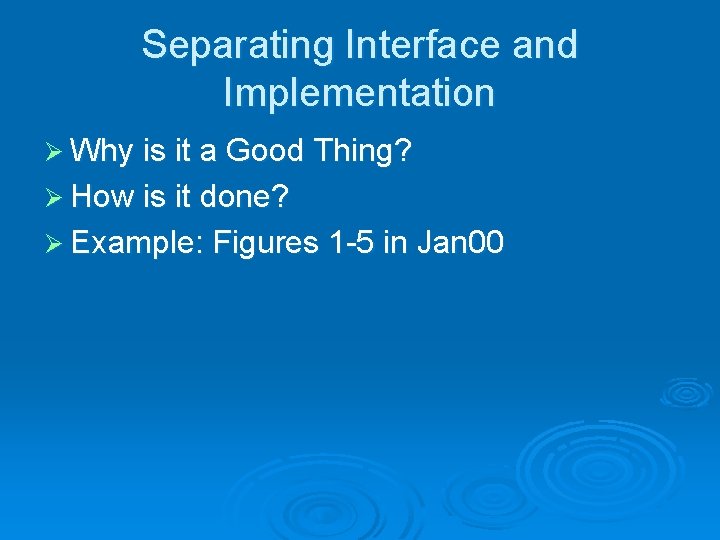
Separating Interface and Implementation Ø Why is it a Good Thing? Ø How is it done? Ø Example: Figures 1 -5 in Jan 00
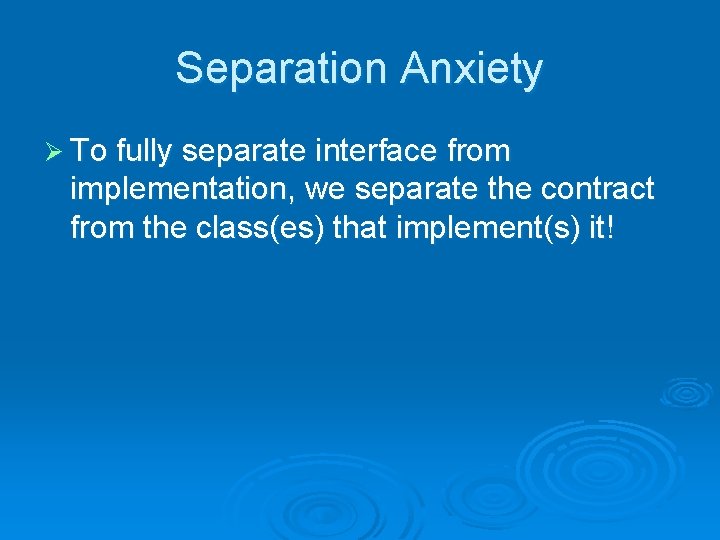
Separation Anxiety Ø To fully separate interface from implementation, we separate the contract from the class(es) that implement(s) it!
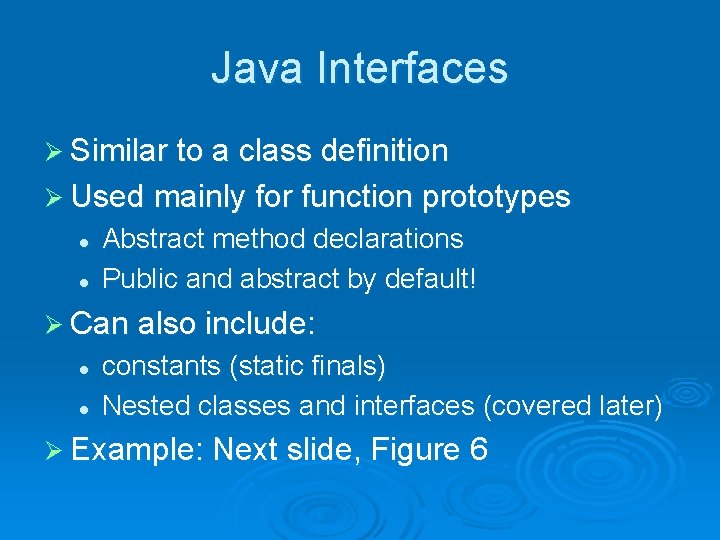
Java Interfaces Ø Similar to a class definition Ø Used mainly for function prototypes l l Abstract method declarations Public and abstract by default! Ø Can also include: l l constants (static finals) Nested classes and interfaces (covered later) Ø Example: Next slide, Figure 6
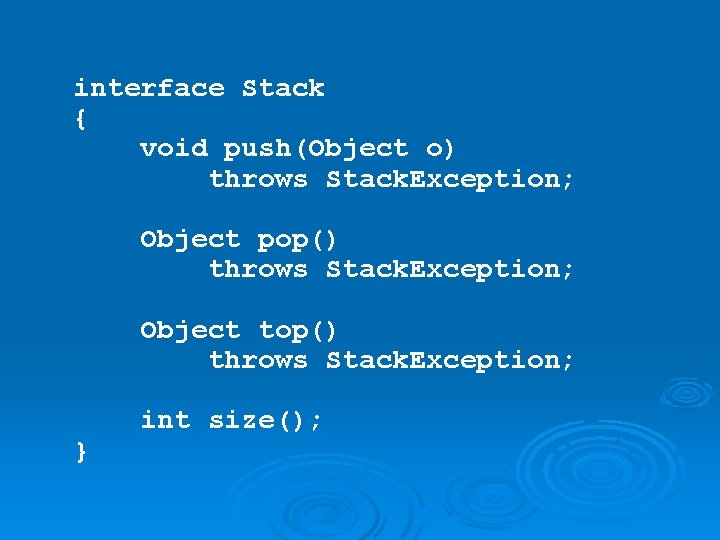
interface Stack { void push(Object o) throws Stack. Exception; Object pop() throws Stack. Exception; Object top() throws Stack. Exception; int size(); }
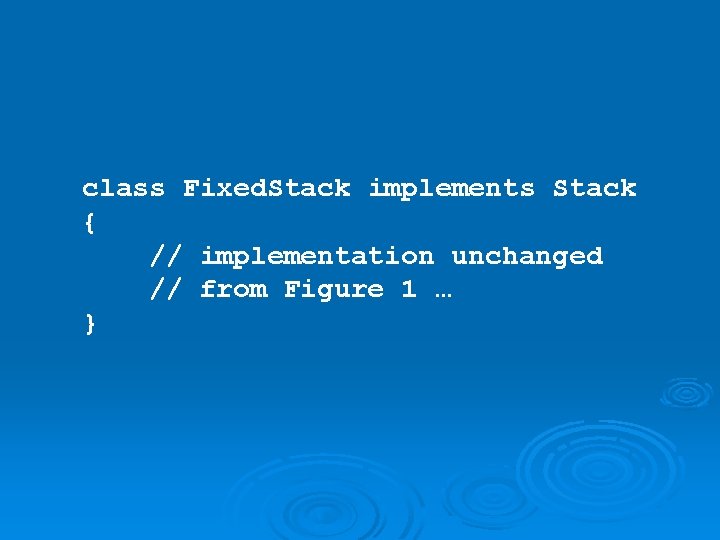
class Fixed. Stack implements Stack { // implementation unchanged // from Figure 1 … }
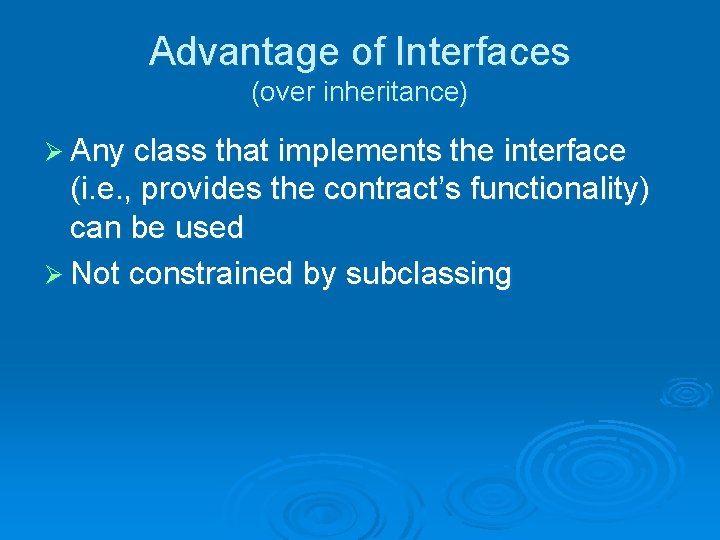
Advantage of Interfaces (over inheritance) Ø Any class that implements the interface (i. e. , provides the contract’s functionality) can be used Ø Not constrained by subclassing
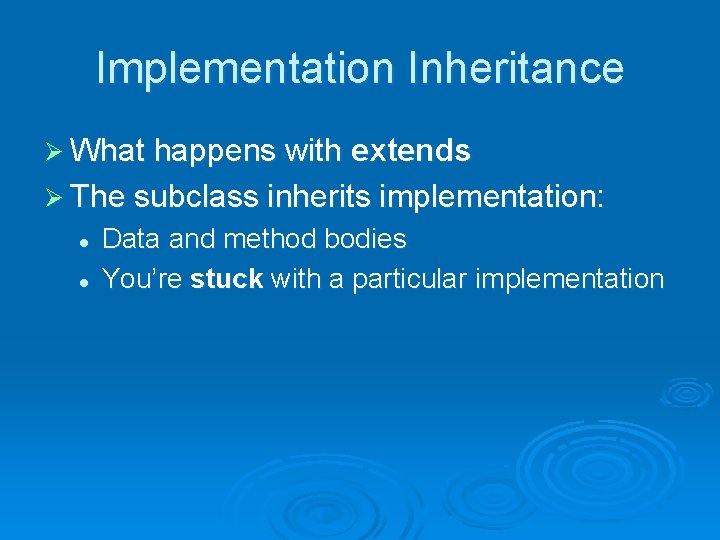
Implementation Inheritance Ø What happens with extends Ø The subclass inherits implementation: l l Data and method bodies You’re stuck with a particular implementation
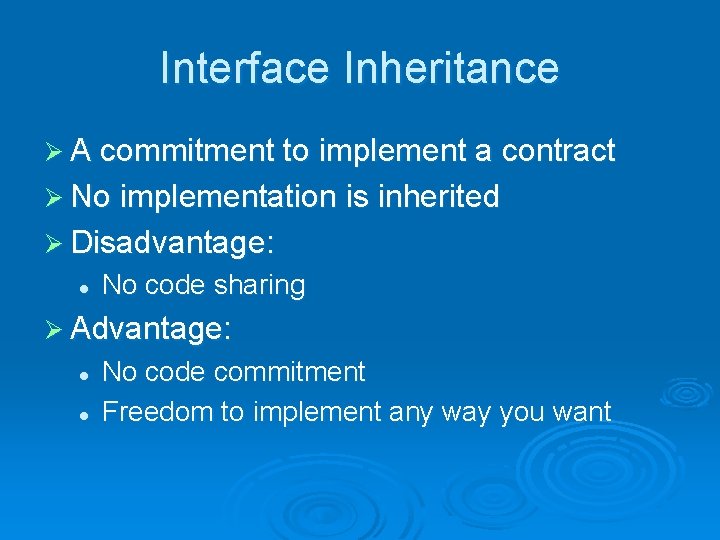
Interface Inheritance Ø A commitment to implement a contract Ø No implementation is inherited Ø Disadvantage: l No code sharing Ø Advantage: l l No code commitment Freedom to implement any way you want
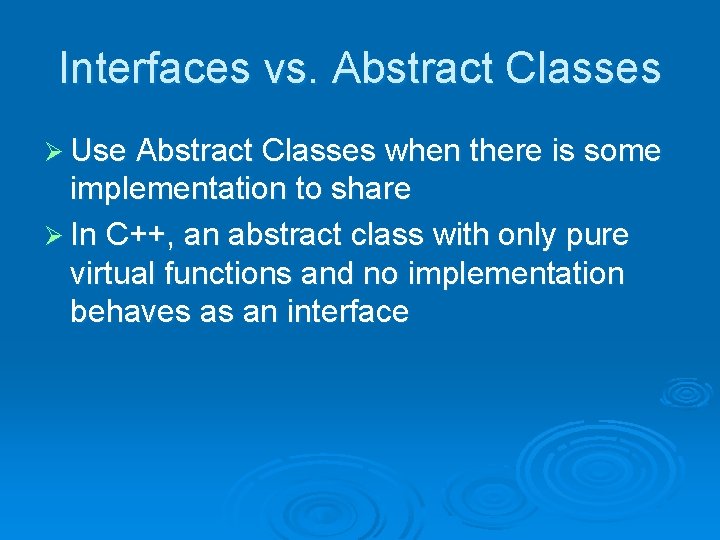
Interfaces vs. Abstract Classes Ø Use Abstract Classes when there is some implementation to share Ø In C++, an abstract class with only pure virtual functions and no implementation behaves as an interface
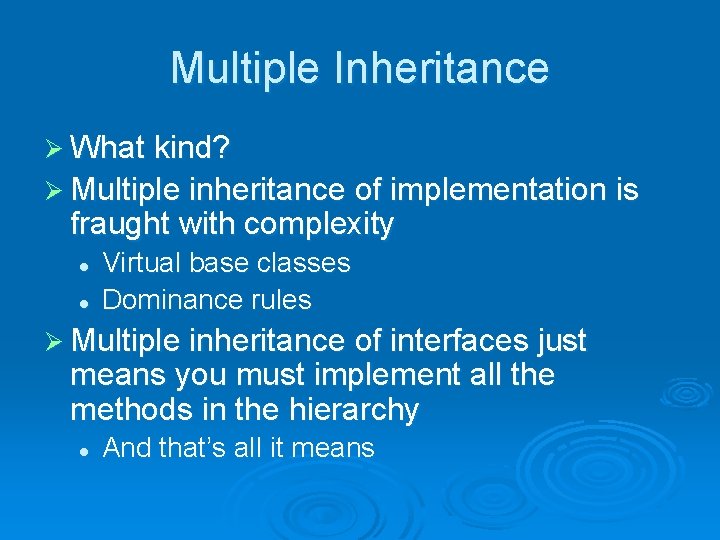
Multiple Inheritance Ø What kind? Ø Multiple inheritance of implementation is fraught with complexity l l Virtual base classes Dominance rules Ø Multiple inheritance of interfaces just means you must implement all the methods in the hierarchy l And that’s all it means
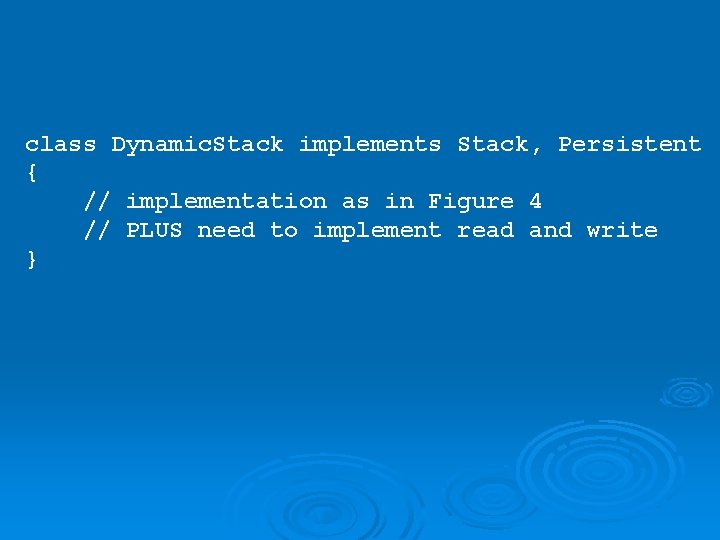
class Dynamic. Stack implements Stack, Persistent { // implementation as in Figure 4 // PLUS need to implement read and write }
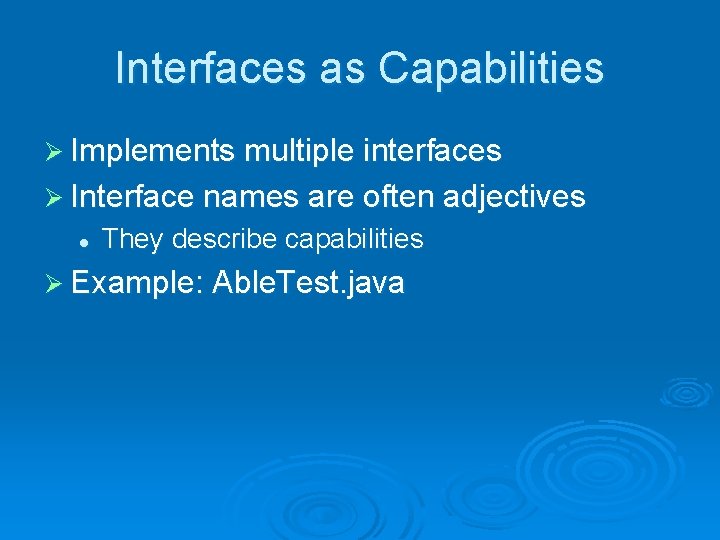
Interfaces as Capabilities Ø Implements multiple interfaces Ø Interface names are often adjectives l They describe capabilities Ø Example: Able. Test. java
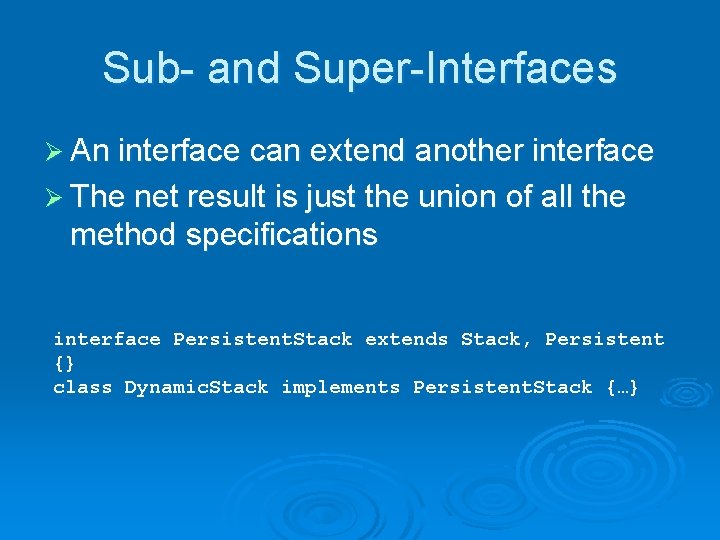
Sub- and Super-Interfaces Ø An interface can extend another interface Ø The net result is just the union of all the method specifications interface Persistent. Stack extends Stack, Persistent {} class Dynamic. Stack implements Persistent. Stack {…}
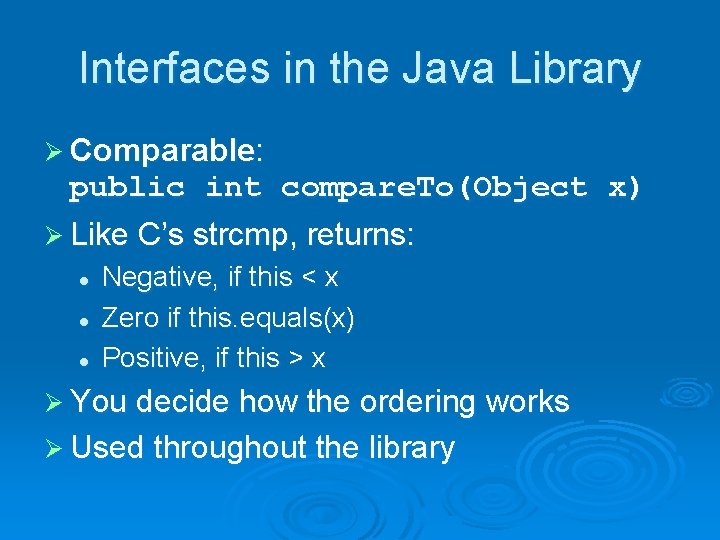
Interfaces in the Java Library Ø Comparable: public int compare. To(Object x) Ø Like C’s strcmp, returns: l l l Negative, if this < x Zero if this. equals(x) Positive, if this > x Ø You decide how the ordering works Ø Used throughout the library
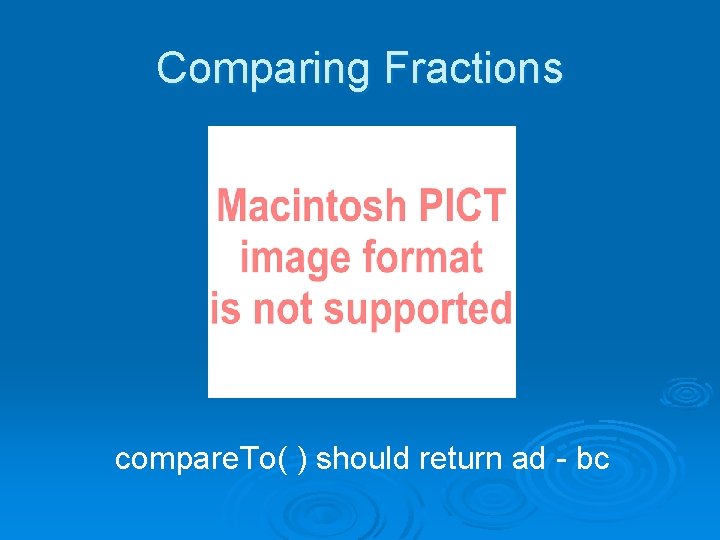
Comparing Fractions compare. To( ) should return ad - bc
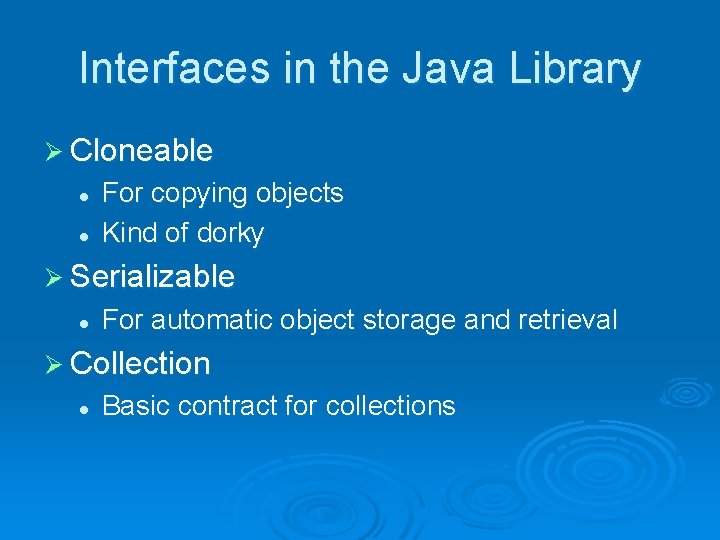
Interfaces in the Java Library Ø Cloneable l l For copying objects Kind of dorky Ø Serializable l For automatic object storage and retrieval Ø Collection l Basic contract for collections
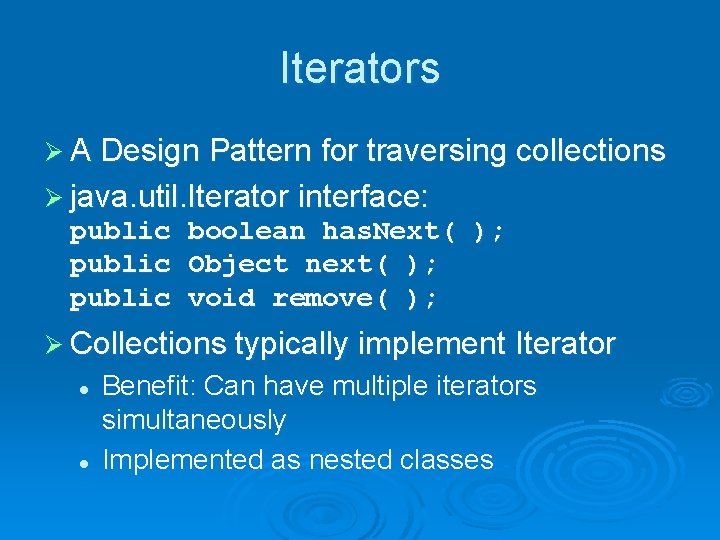
Iterators Ø A Design Pattern for traversing collections Ø java. util. Iterator interface: public boolean has. Next( ); public Object next( ); public void remove( ); Ø Collections typically implement Iterator l l Benefit: Can have multiple iterators simultaneously Implemented as nested classes
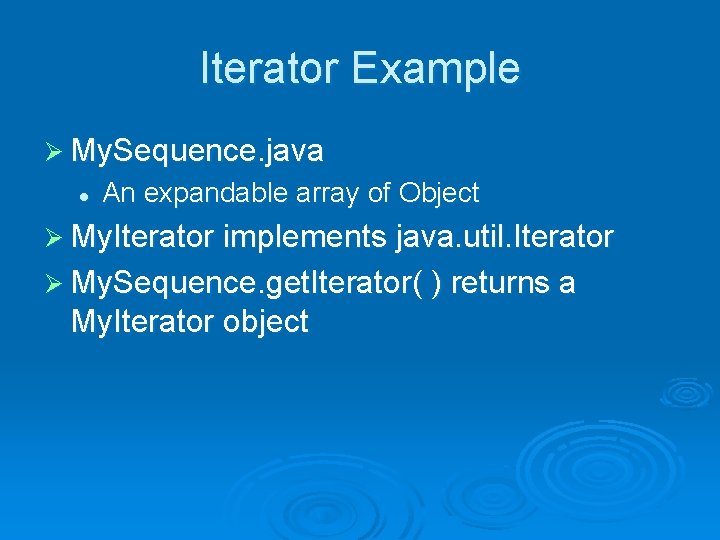
Iterator Example Ø My. Sequence. java l An expandable array of Object Ø My. Iterator implements java. util. Iterator Ø My. Sequence. get. Iterator( ) returns a My. Iterator object
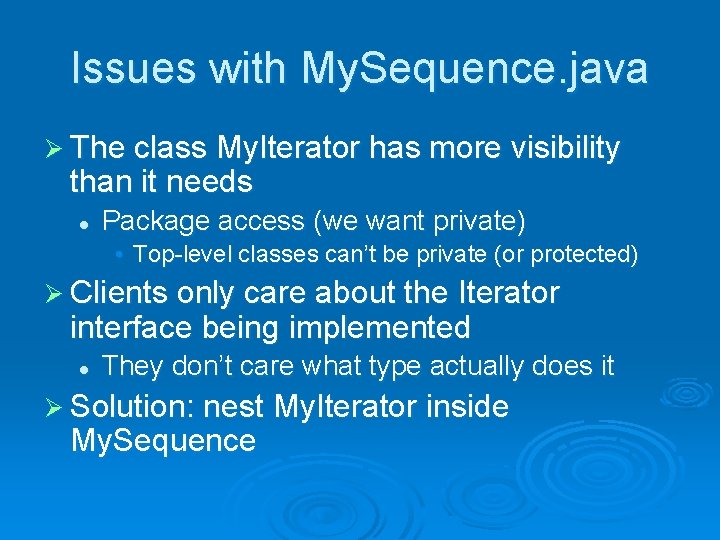
Issues with My. Sequence. java Ø The class My. Iterator has more visibility than it needs l Package access (we want private) • Top-level classes can’t be private (or protected) Ø Clients only care about the Iterator interface being implemented l They don’t care what type actually does it Ø Solution: nest My. Iterator inside My. Sequence
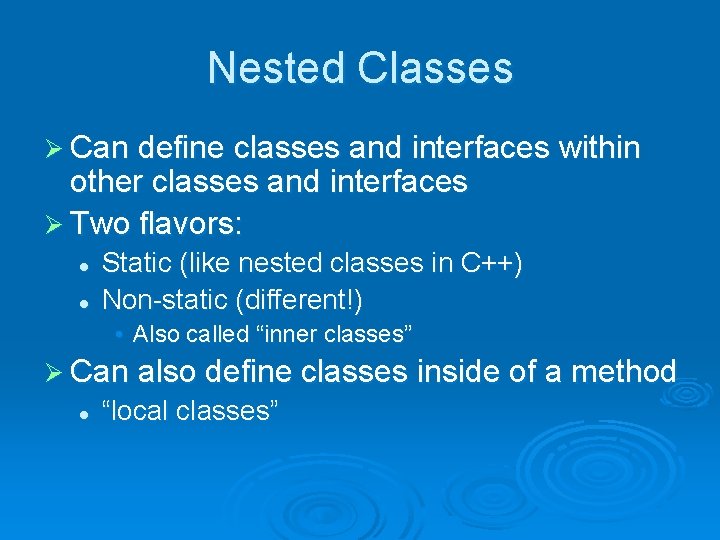
Nested Classes Ø Can define classes and interfaces within other classes and interfaces Ø Two flavors: l l Static (like nested classes in C++) Non-static (different!) • Also called “inner classes” Ø Can also define classes inside of a method l “local classes”
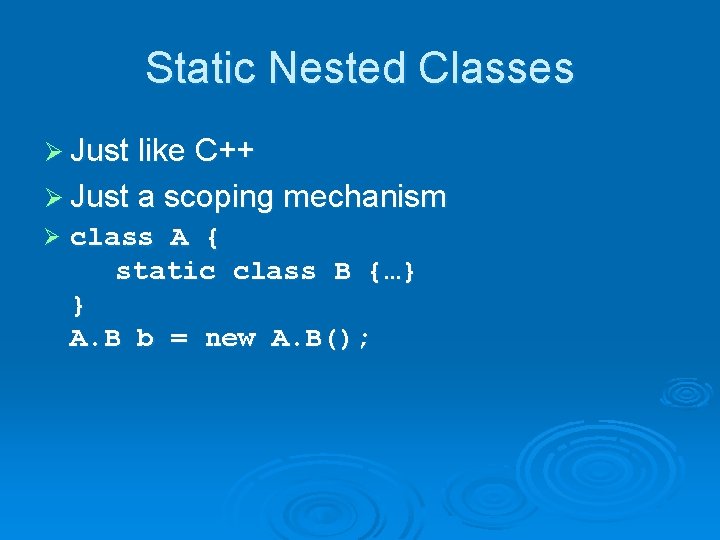
Static Nested Classes Ø Just like C++ Ø Just a scoping mechanism Ø class A { static class B {…} } A. B b = new A. B();
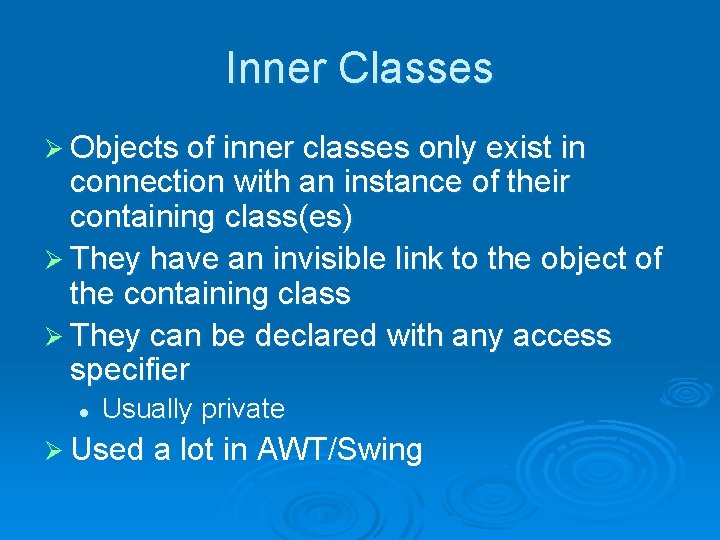
Inner Classes Ø Objects of inner classes only exist in connection with an instance of their containing class(es) Ø They have an invisible link to the object of the containing class Ø They can be declared with any access specifier l Usually private Ø Used a lot in AWT/Swing
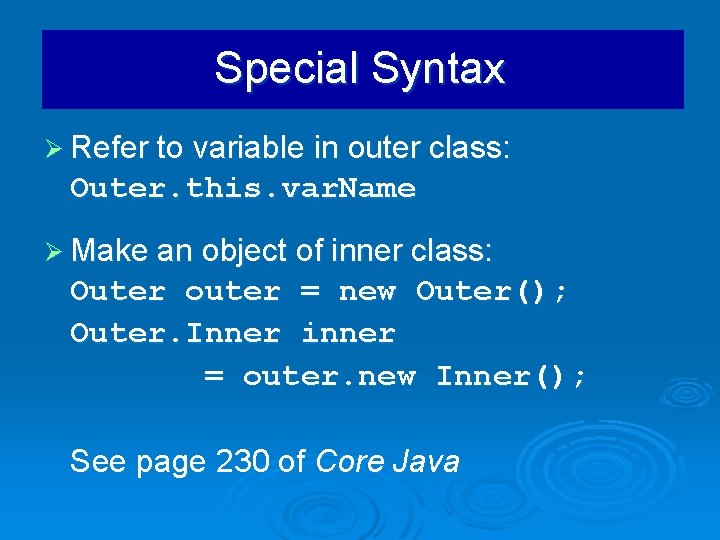
Special Syntax Ø Refer to variable in outer class: Outer. this. var. Name Ø Make an object of inner class: Outer outer = new Outer(); Outer. Inner inner = outer. new Inner(); See page 230 of Core Java
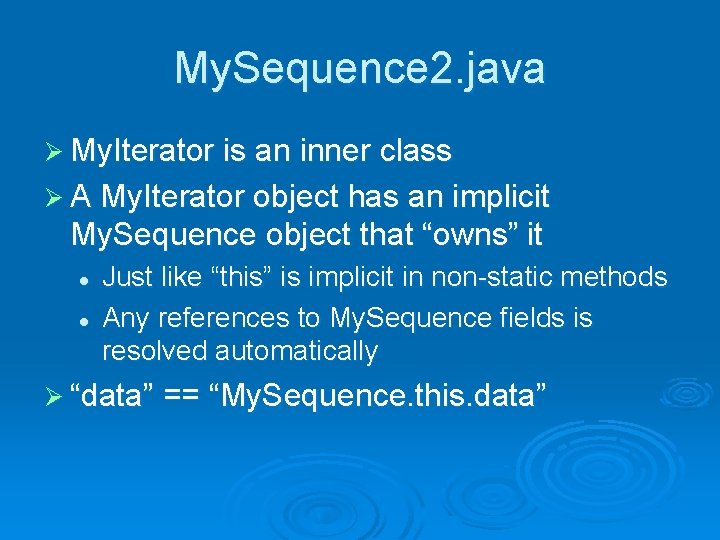
My. Sequence 2. java Ø My. Iterator is an inner class Ø A My. Iterator object has an implicit My. Sequence object that “owns” it l l Just like “this” is implicit in non-static methods Any references to My. Sequence fields is resolved automatically Ø “data” == “My. Sequence. this. data”
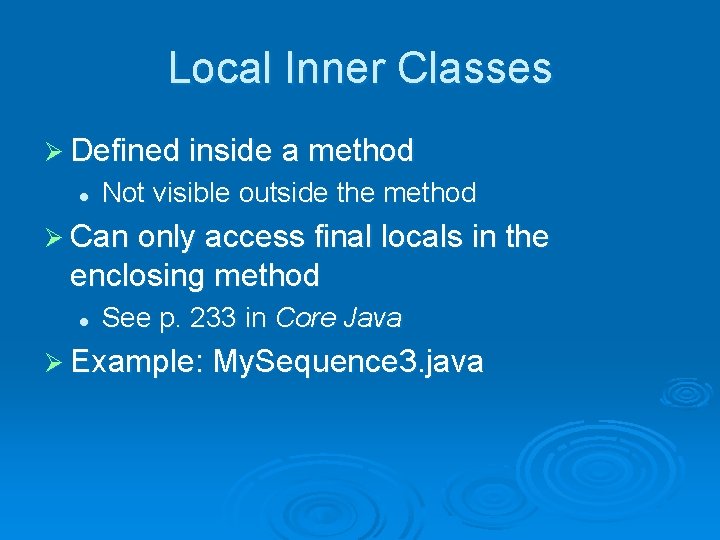
Local Inner Classes Ø Defined inside a method l Not visible outside the method Ø Can only access final locals in the enclosing method l See p. 233 in Core Java Ø Example: My. Sequence 3. java
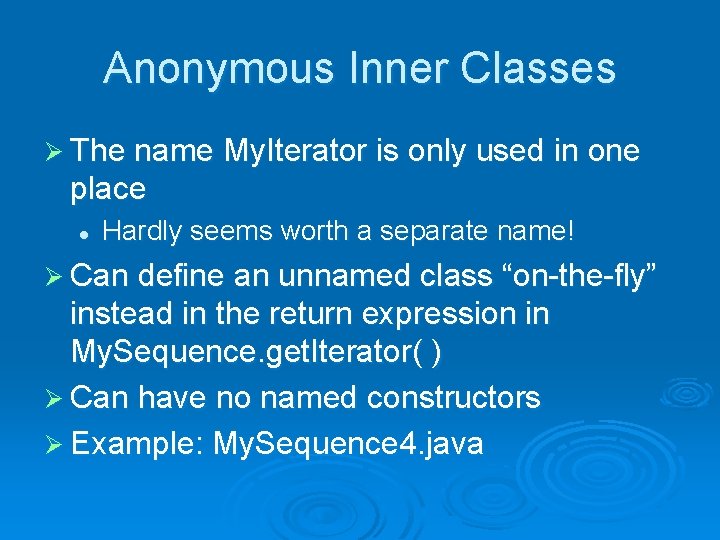
Anonymous Inner Classes Ø The name My. Iterator is only used in one place l Hardly seems worth a separate name! Ø Can define an unnamed class “on-the-fly” instead in the return expression in My. Sequence. get. Iterator( ) Ø Can have no named constructors Ø Example: My. Sequence 4. java
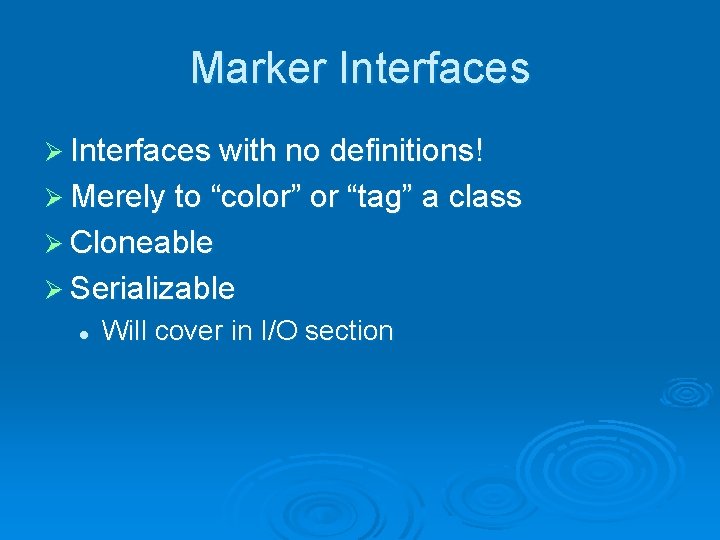
Marker Interfaces Ø Interfaces with no definitions! Ø Merely to “color” or “tag” a class Ø Cloneable Ø Serializable l Will cover in I/O section
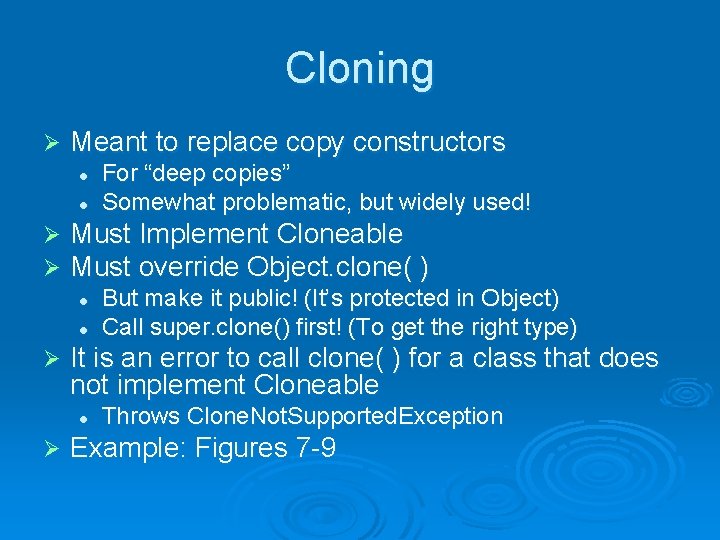
Cloning Ø Meant to replace copy constructors l l Ø Ø Must Implement Cloneable Must override Object. clone( ) l l Ø But make it public! (It’s protected in Object) Call super. clone() first! (To get the right type) It is an error to call clone( ) for a class that does not implement Cloneable l Ø For “deep copies” Somewhat problematic, but widely used! Throws Clone. Not. Supported. Exception Example: Figures 7 -9
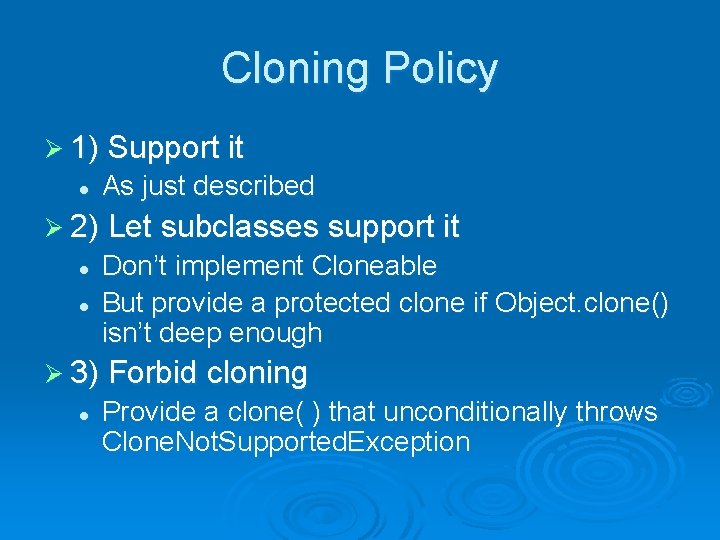
Cloning Policy Ø 1) Support it l As just described Ø 2) Let subclasses support it l l Don’t implement Cloneable But provide a protected clone if Object. clone() isn’t deep enough Ø 3) Forbid cloning l Provide a clone( ) that unconditionally throws Clone. Not. Supported. Exception