Instant Scala Programs and the REPL n A
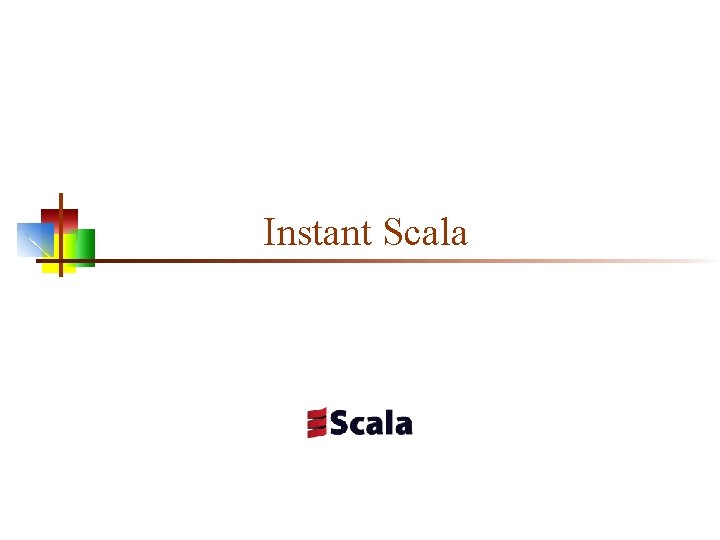
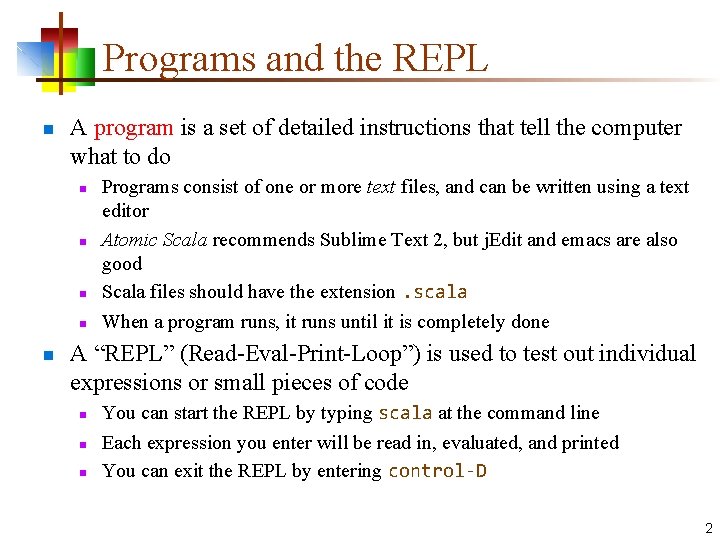
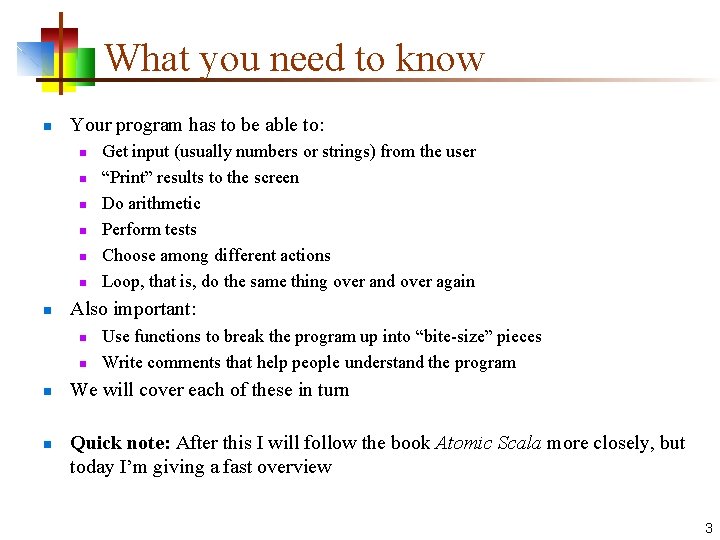
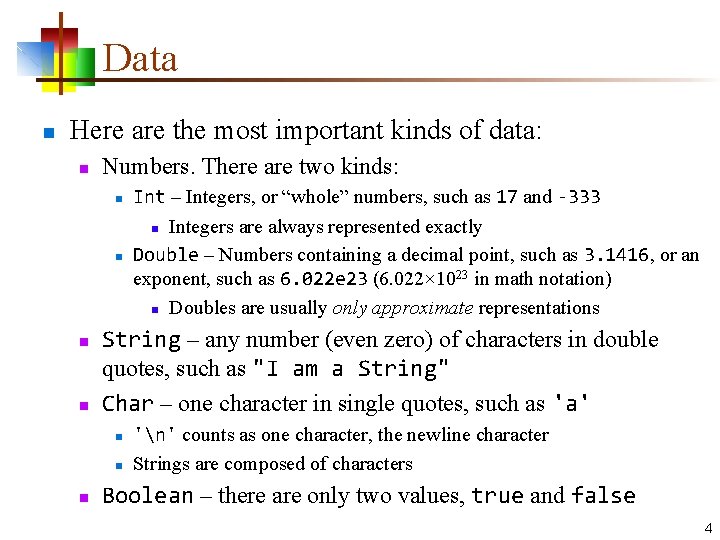
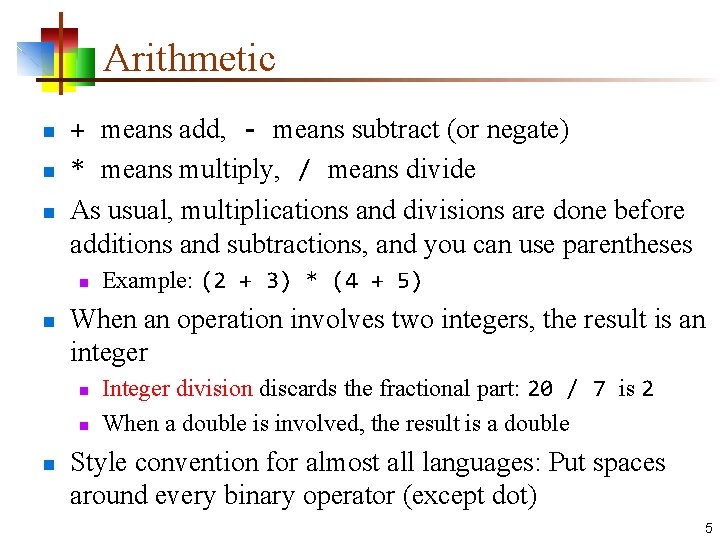
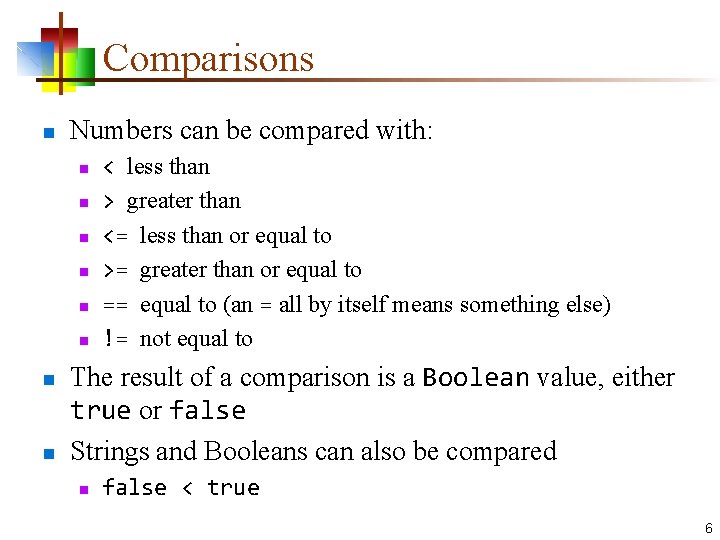
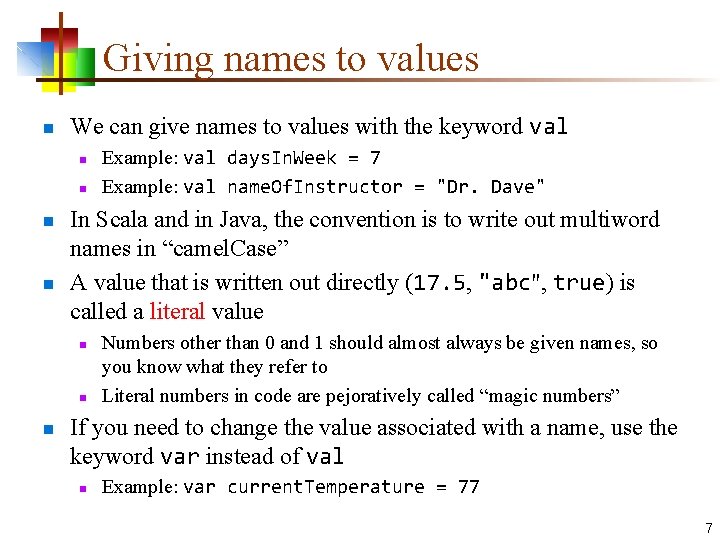
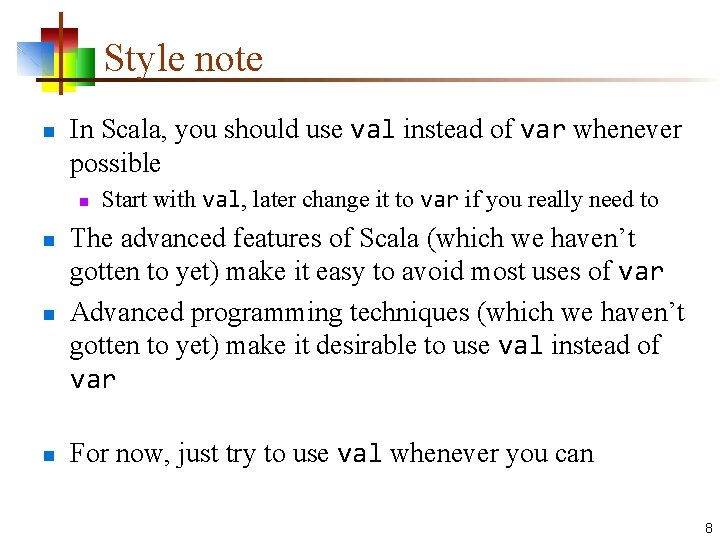
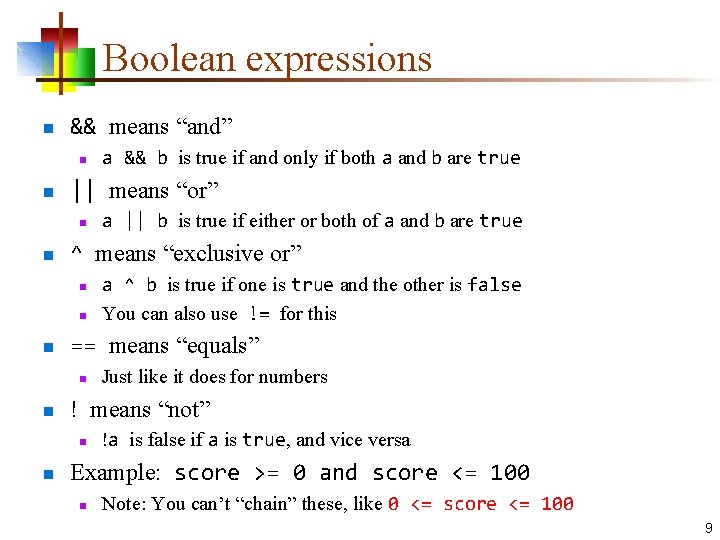
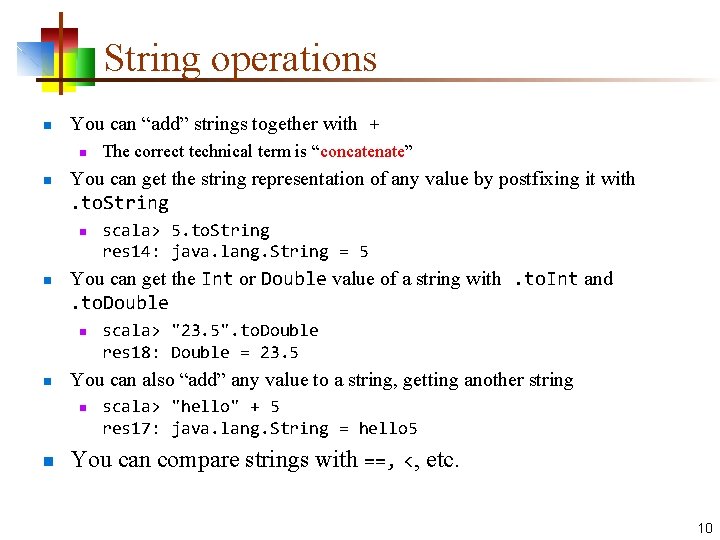
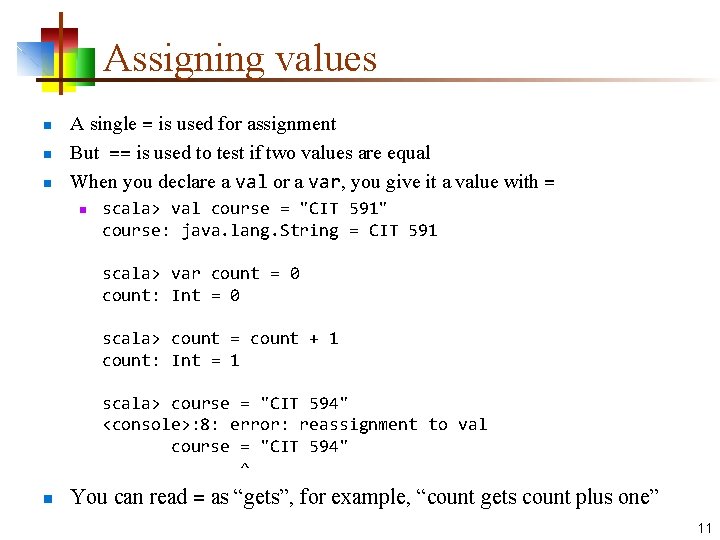
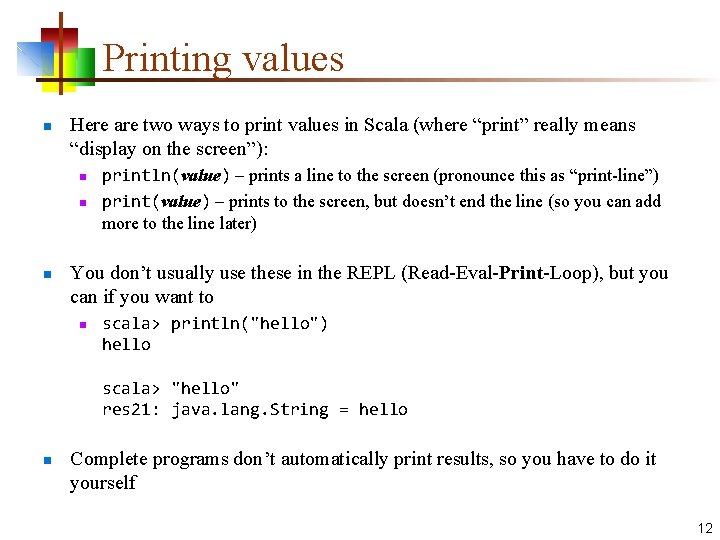
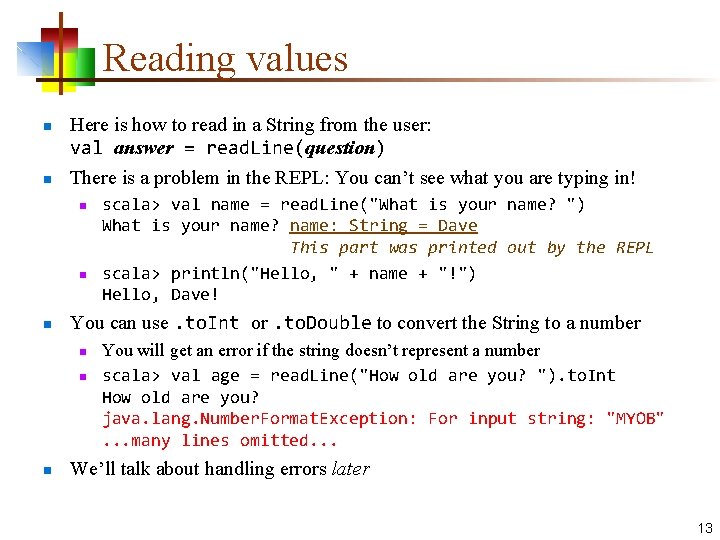
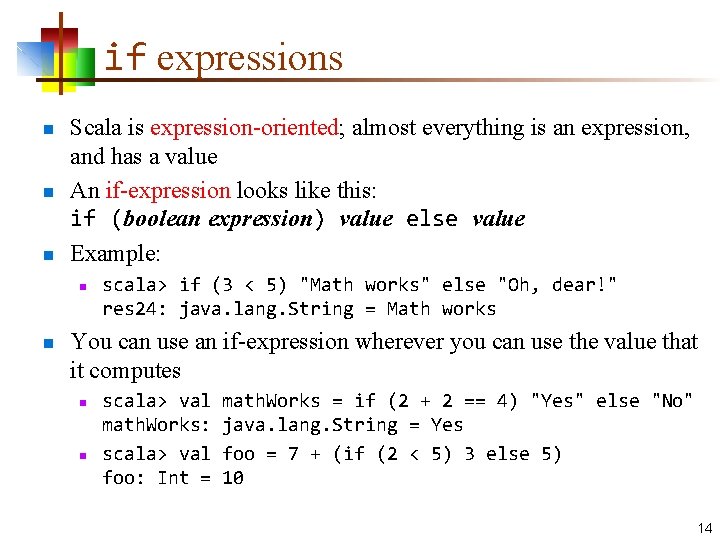
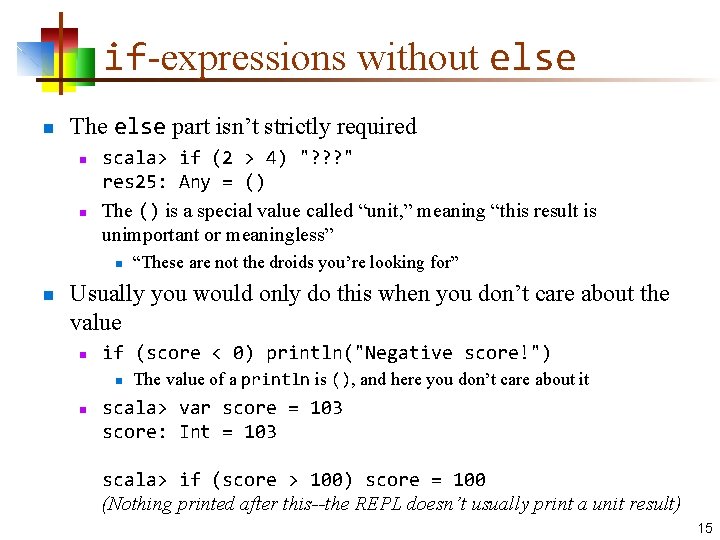
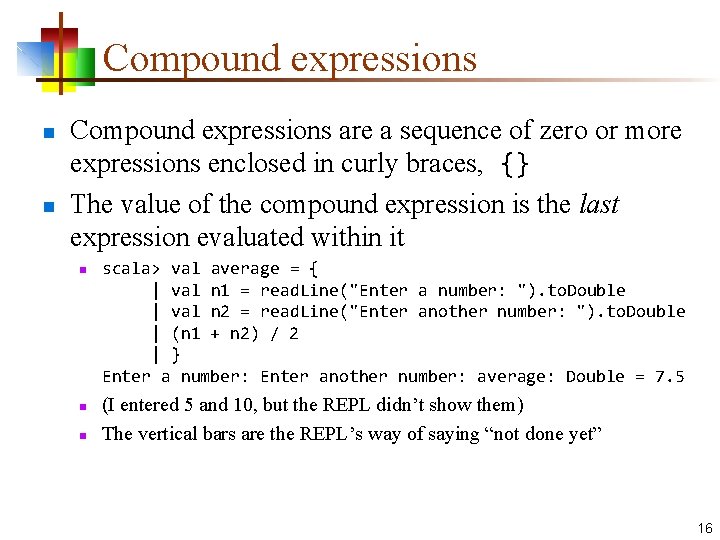
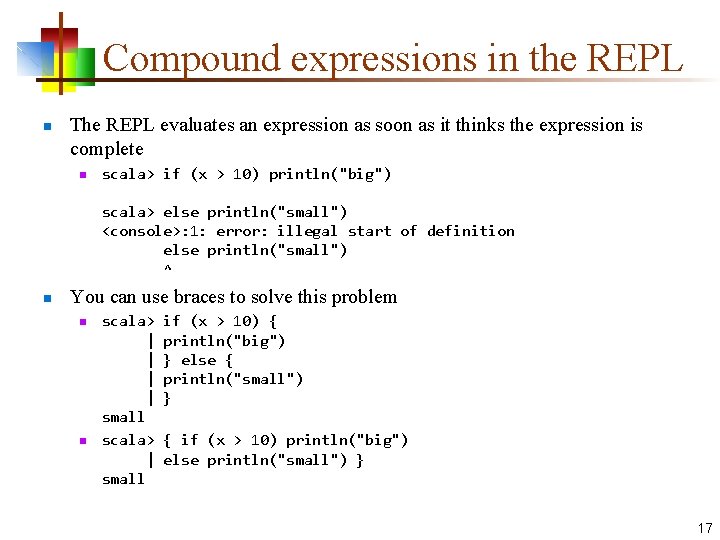
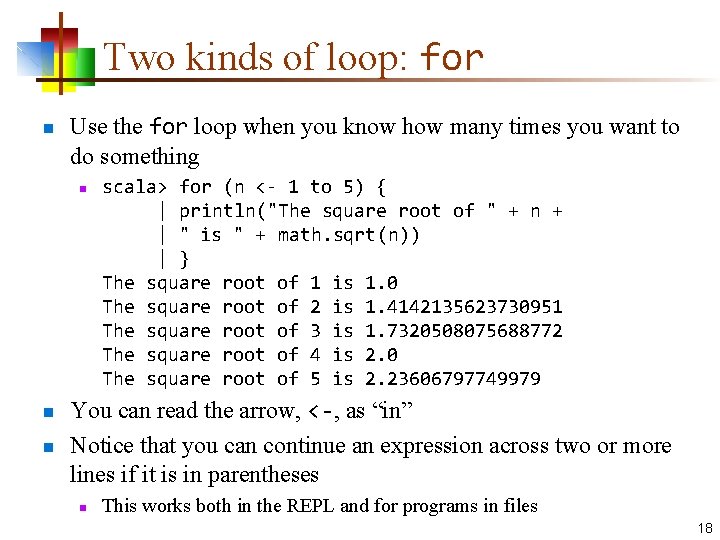
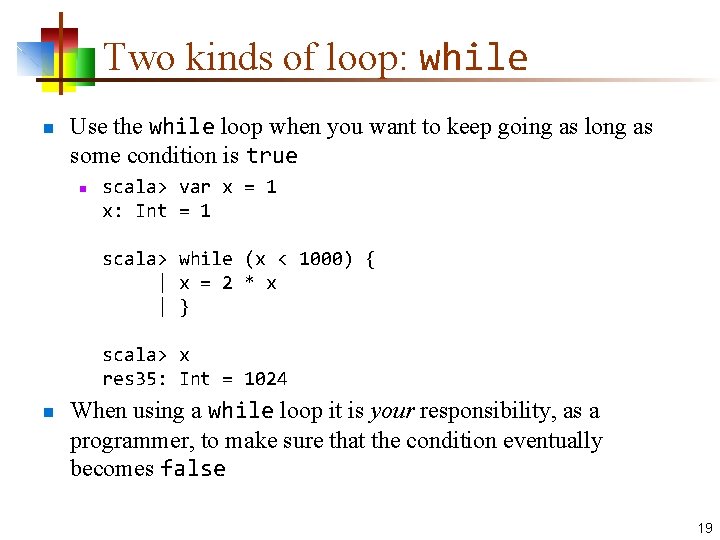
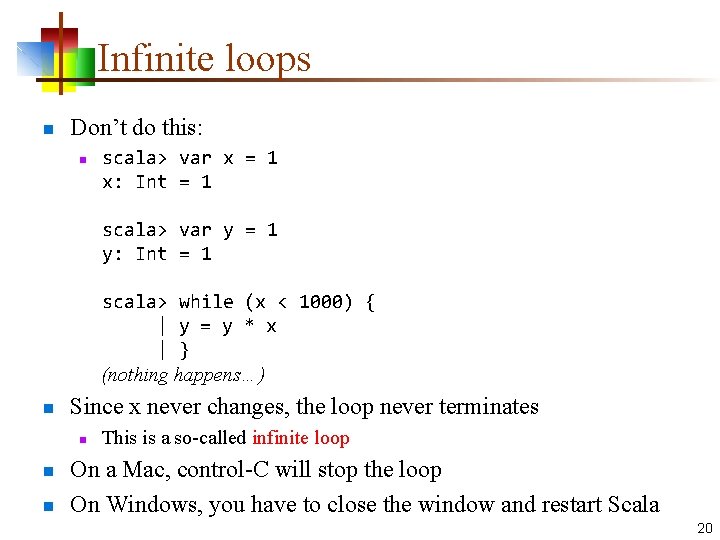
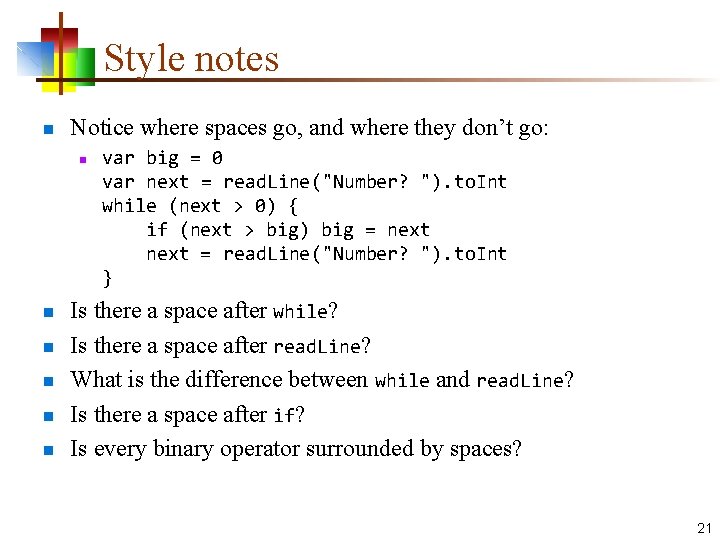
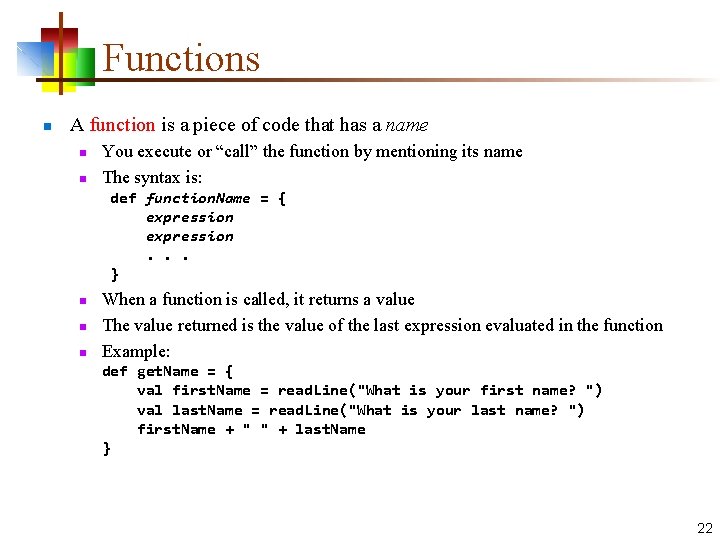
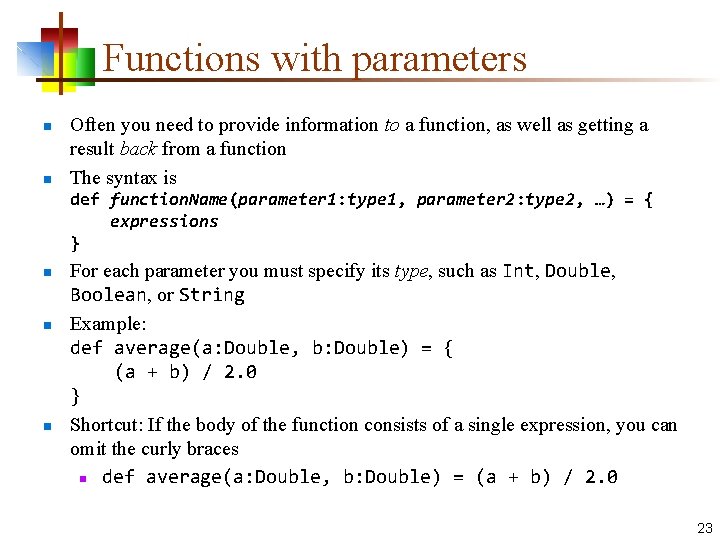
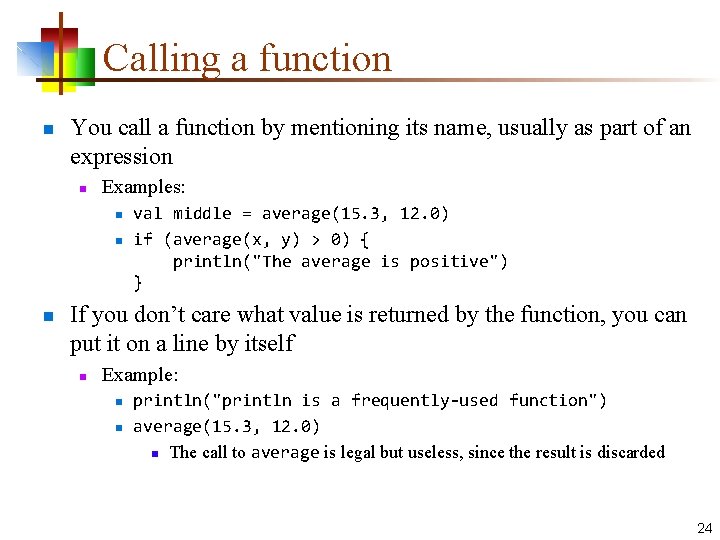
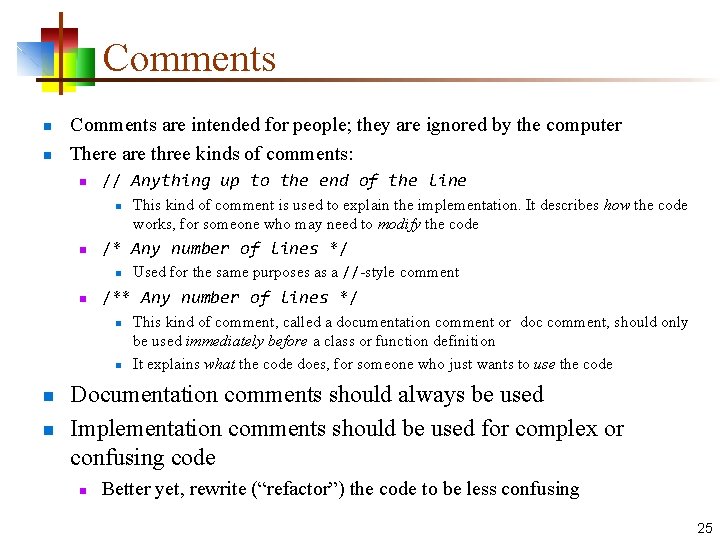
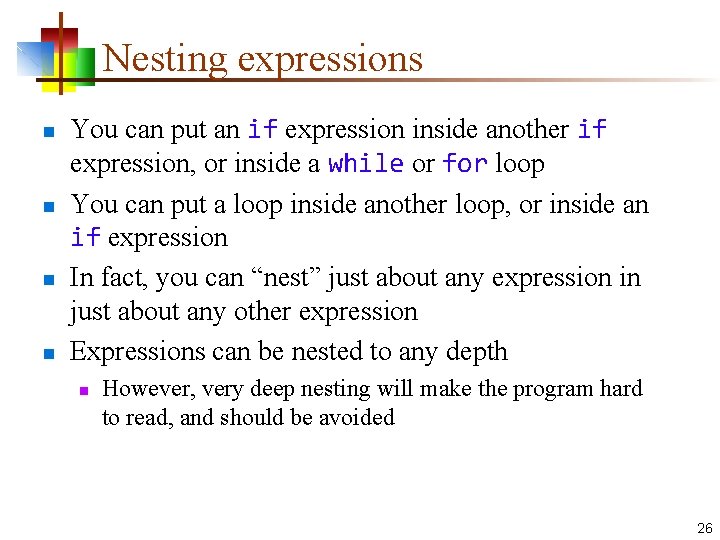
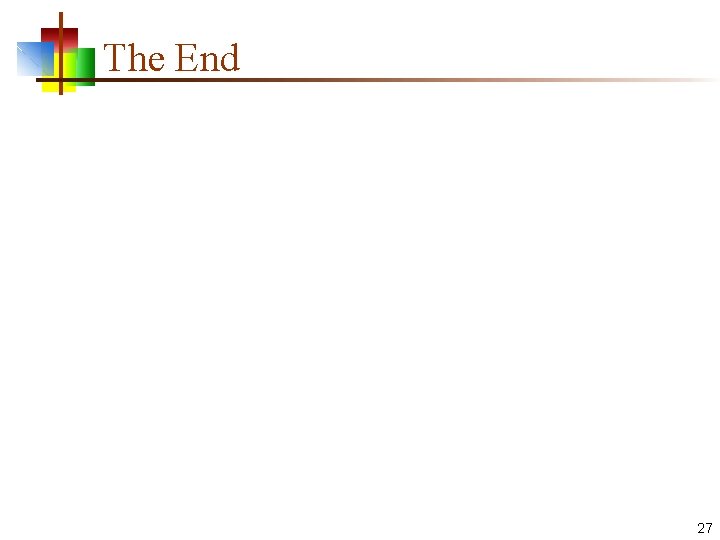
- Slides: 27
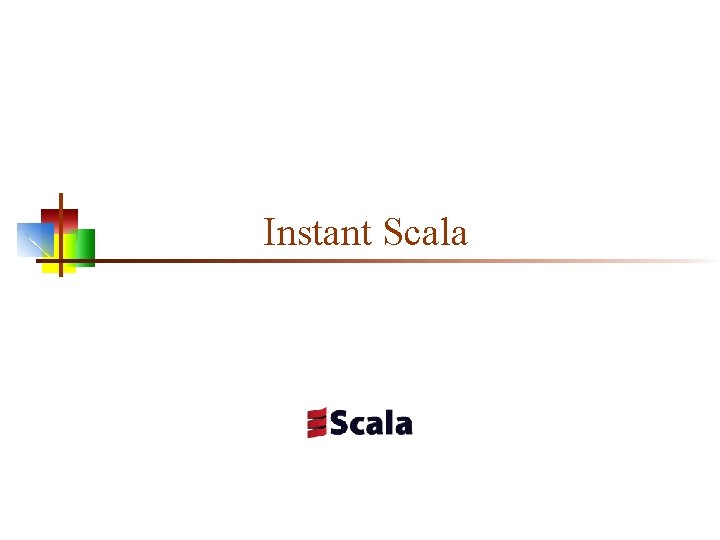
Instant Scala
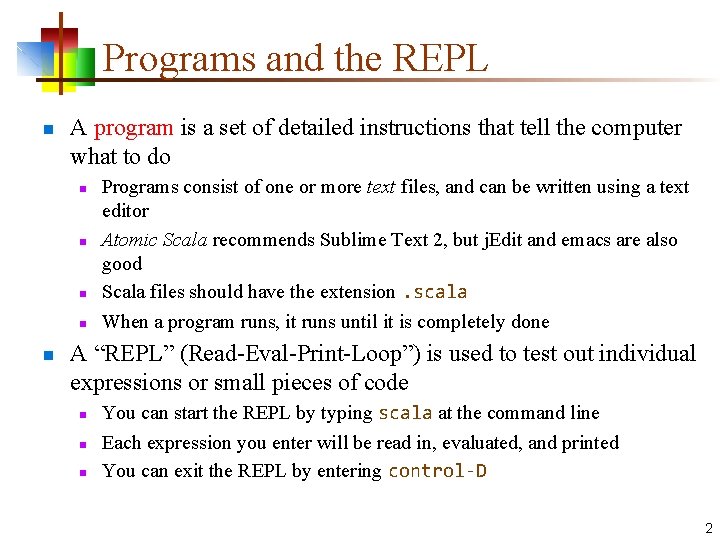
Programs and the REPL n A program is a set of detailed instructions that tell the computer what to do n n n Programs consist of one or more text files, and can be written using a text editor Atomic Scala recommends Sublime Text 2, but j. Edit and emacs are also good Scala files should have the extension. scala When a program runs, it runs until it is completely done A “REPL” (Read-Eval-Print-Loop”) is used to test out individual expressions or small pieces of code n n n You can start the REPL by typing scala at the command line Each expression you enter will be read in, evaluated, and printed You can exit the REPL by entering control-D 2
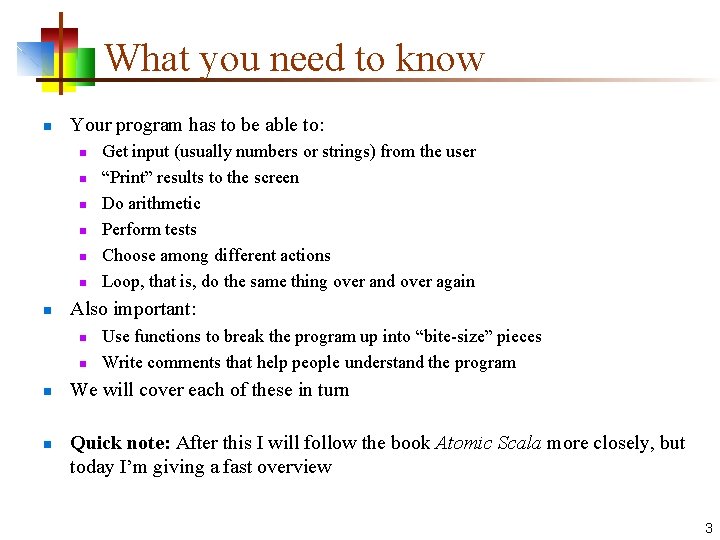
What you need to know n Your program has to be able to: n n n n Also important: n n Get input (usually numbers or strings) from the user “Print” results to the screen Do arithmetic Perform tests Choose among different actions Loop, that is, do the same thing over and over again Use functions to break the program up into “bite-size” pieces Write comments that help people understand the program We will cover each of these in turn Quick note: After this I will follow the book Atomic Scala more closely, but today I’m giving a fast overview 3
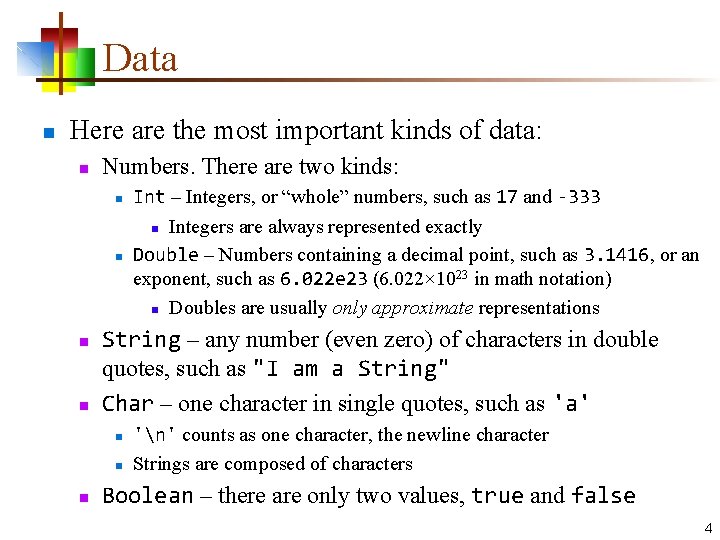
Data n Here are the most important kinds of data: n Numbers. There are two kinds: n n String – any number (even zero) of characters in double quotes, such as "I am a String" Char – one character in single quotes, such as 'a' n n n Int – Integers, or “whole” numbers, such as 17 and -333 n Integers are always represented exactly Double – Numbers containing a decimal point, such as 3. 1416, or an exponent, such as 6. 022 e 23 (6. 022× 1023 in math notation) n Doubles are usually only approximate representations 'n' counts as one character, the newline character Strings are composed of characters Boolean – there are only two values, true and false 4
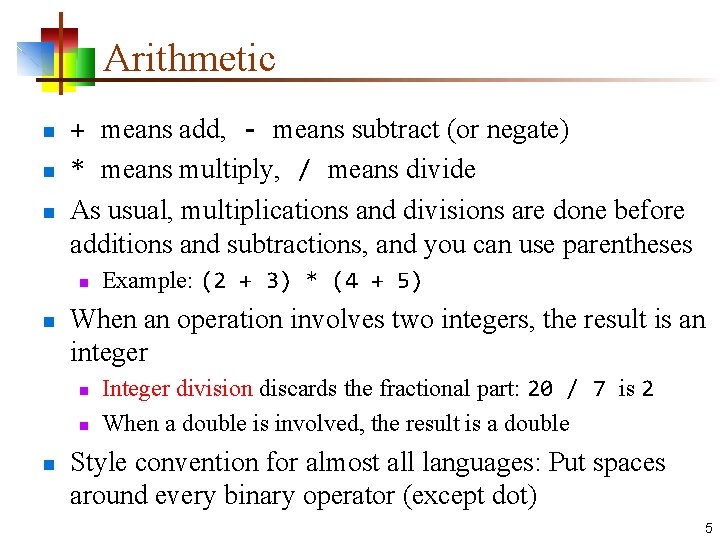
Arithmetic n n n + means add, - means subtract (or negate) * means multiply, / means divide As usual, multiplications and divisions are done before additions and subtractions, and you can use parentheses n n When an operation involves two integers, the result is an integer n n n Example: (2 + 3) * (4 + 5) Integer division discards the fractional part: 20 / 7 is 2 When a double is involved, the result is a double Style convention for almost all languages: Put spaces around every binary operator (except dot) 5
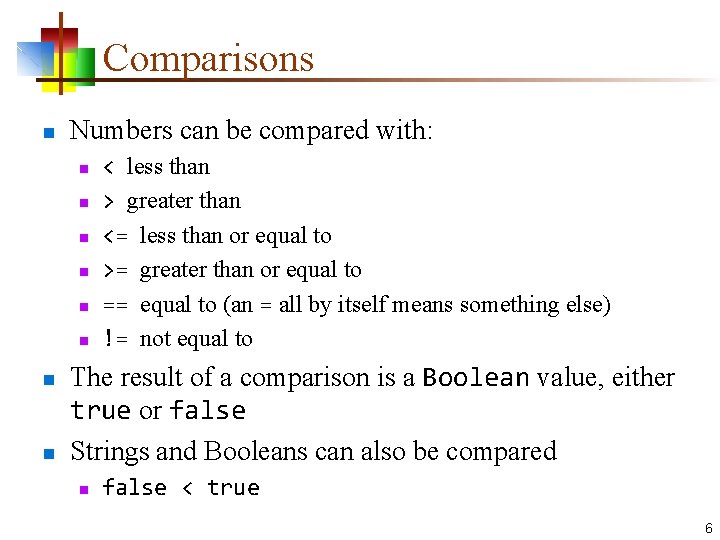
Comparisons n Numbers can be compared with: n n n n < less than > greater than <= less than or equal to >= greater than or equal to == equal to (an = all by itself means something else) != not equal to The result of a comparison is a Boolean value, either true or false Strings and Booleans can also be compared n false < true 6
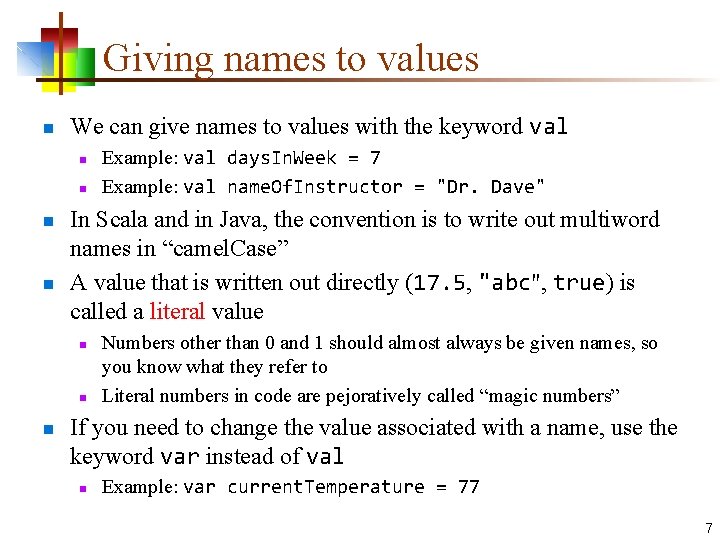
Giving names to values n We can give names to values with the keyword val n n In Scala and in Java, the convention is to write out multiword names in “camel. Case” A value that is written out directly (17. 5, "abc", true) is called a literal value n n n Example: val days. In. Week = 7 Example: val name. Of. Instructor = "Dr. Dave" Numbers other than 0 and 1 should almost always be given names, so you know what they refer to Literal numbers in code are pejoratively called “magic numbers” If you need to change the value associated with a name, use the keyword var instead of val n Example: var current. Temperature = 77 7
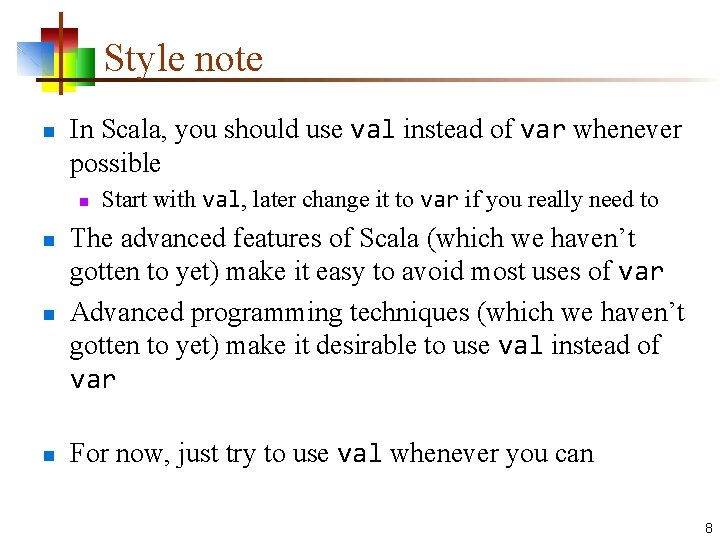
Style note n In Scala, you should use val instead of var whenever possible n Start with val, later change it to var if you really need to n The advanced features of Scala (which we haven’t gotten to yet) make it easy to avoid most uses of var Advanced programming techniques (which we haven’t gotten to yet) make it desirable to use val instead of var n For now, just try to use val whenever you can n 8
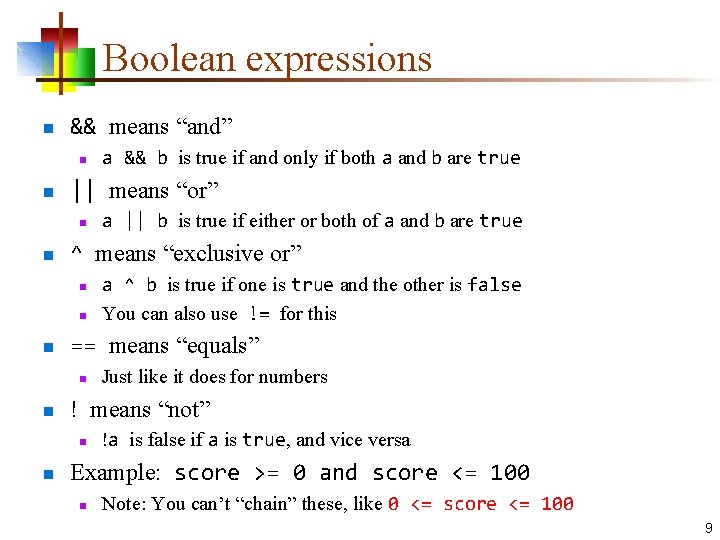
Boolean expressions n && means “and” n n || means “or” n n n Just like it does for numbers ! means “not” n n a ^ b is true if one is true and the other is false You can also use != for this == means “equals” n n a || b is true if either or both of a and b are true ^ means “exclusive or” n n a && b is true if and only if both a and b are true !a is false if a is true, and vice versa Example: score >= 0 and score <= 100 n Note: You can’t “chain” these, like 0 <= score <= 100 9
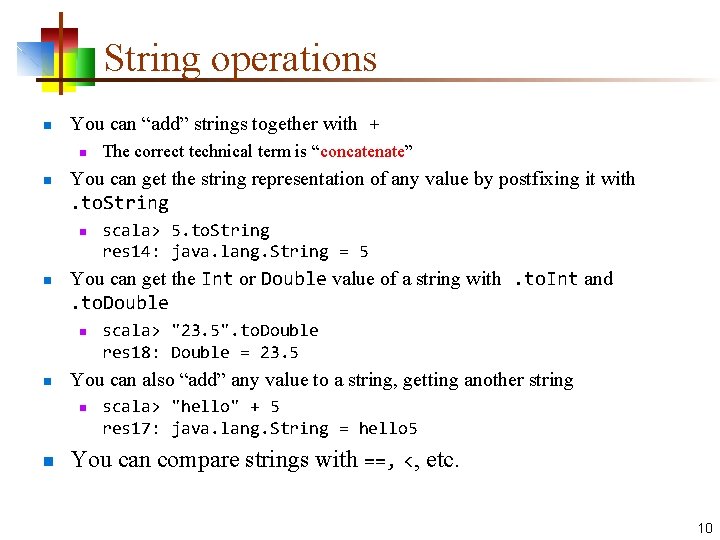
String operations n You can “add” strings together with + n n You can get the string representation of any value by postfixing it with. to. String n n scala> "23. 5". to. Double res 18: Double = 23. 5 You can also “add” any value to a string, getting another string n n scala> 5. to. String res 14: java. lang. String = 5 You can get the Int or Double value of a string with. to. Int and. to. Double n n The correct technical term is “concatenate” scala> "hello" + 5 res 17: java. lang. String = hello 5 You can compare strings with ==, <, etc. 10
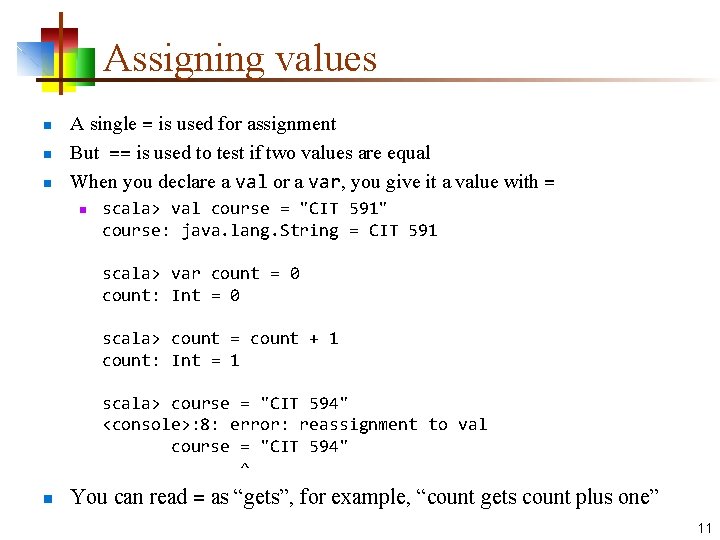
Assigning values n n n A single = is used for assignment But == is used to test if two values are equal When you declare a val or a var, you give it a value with = n scala> val course = "CIT 591" course: java. lang. String = CIT 591 scala> var count = 0 count: Int = 0 scala> count = count + 1 count: Int = 1 scala> course = "CIT 594" <console>: 8: error: reassignment to val course = "CIT 594" ^ n You can read = as “gets”, for example, “count gets count plus one” 11
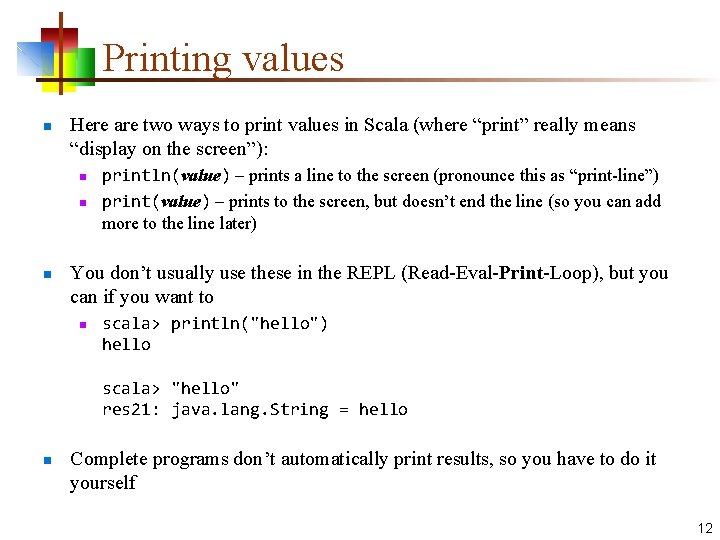
Printing values n Here are two ways to print values in Scala (where “print” really means “display on the screen”): n n n println(value) – prints a line to the screen (pronounce this as “print-line”) print(value) – prints to the screen, but doesn’t end the line (so you can add more to the line later) You don’t usually use these in the REPL (Read-Eval-Print-Loop), but you can if you want to n scala> println("hello") hello scala> "hello" res 21: java. lang. String = hello n Complete programs don’t automatically print results, so you have to do it yourself 12
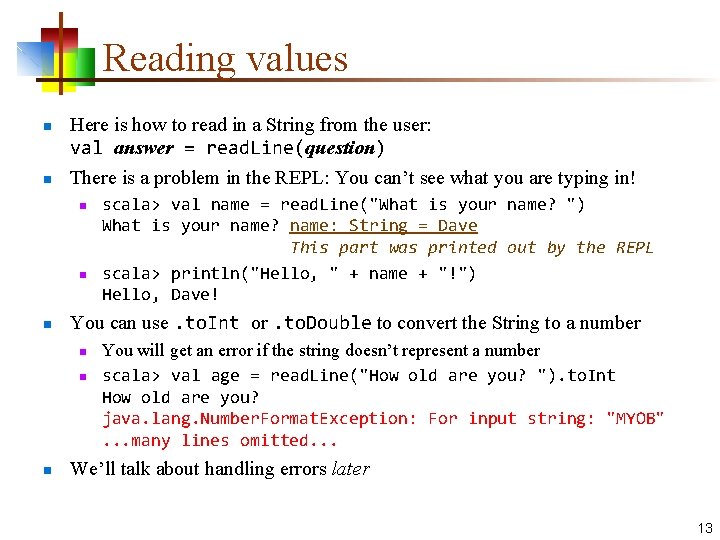
Reading values n n Here is how to read in a String from the user: val answer = read. Line(question) There is a problem in the REPL: You can’t see what you are typing in! n n n You can use. to. Int or. to. Double to convert the String to a number n n n scala> val name = read. Line("What is your name? ") What is your name? name: String = Dave This part was printed out by the REPL scala> println("Hello, " + name + "!") Hello, Dave! You will get an error if the string doesn’t represent a number scala> val age = read. Line("How old are you? "). to. Int How old are you? java. lang. Number. Format. Exception: For input string: "MYOB". . . many lines omitted. . . We’ll talk about handling errors later 13
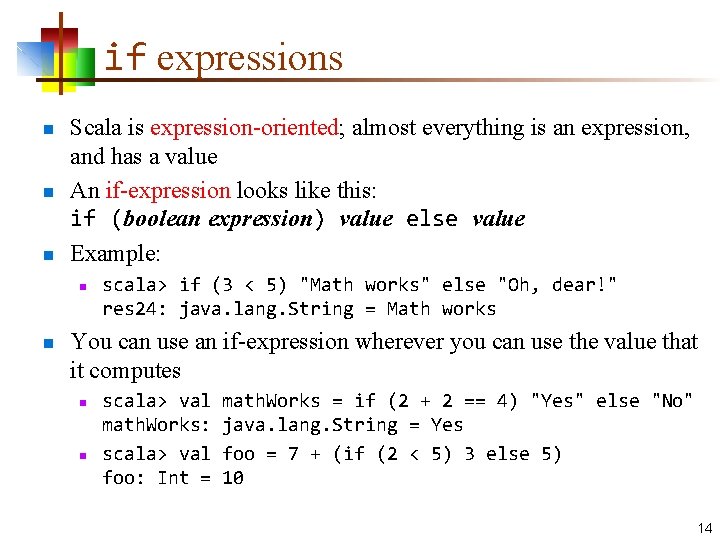
if expressions n n n Scala is expression-oriented; almost everything is an expression, and has a value An if-expression looks like this: if (boolean expression) value else value Example: n n scala> if (3 < 5) "Math works" else "Oh, dear!" res 24: java. lang. String = Math works You can use an if-expression wherever you can use the value that it computes n n scala> val math. Works: scala> val foo: Int = math. Works = if (2 + 2 == 4) "Yes" else "No" java. lang. String = Yes foo = 7 + (if (2 < 5) 3 else 5) 10 14
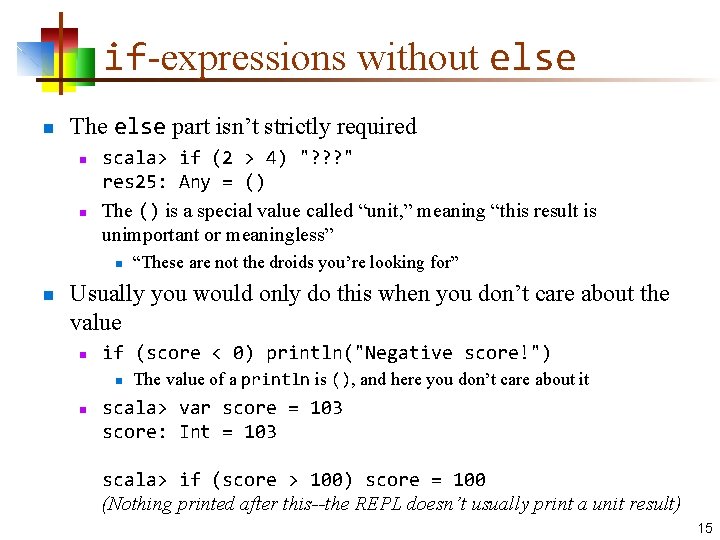
if-expressions without else n The else part isn’t strictly required n n scala> if (2 > 4) "? ? ? " res 25: Any = () The () is a special value called “unit, ” meaning “this result is unimportant or meaningless” n n “These are not the droids you’re looking for” Usually you would only do this when you don’t care about the value n if (score < 0) println("Negative score!") n n The value of a println is (), and here you don’t care about it scala> var score = 103 score: Int = 103 scala> if (score > 100) score = 100 (Nothing printed after this--the REPL doesn’t usually print a unit result) 15
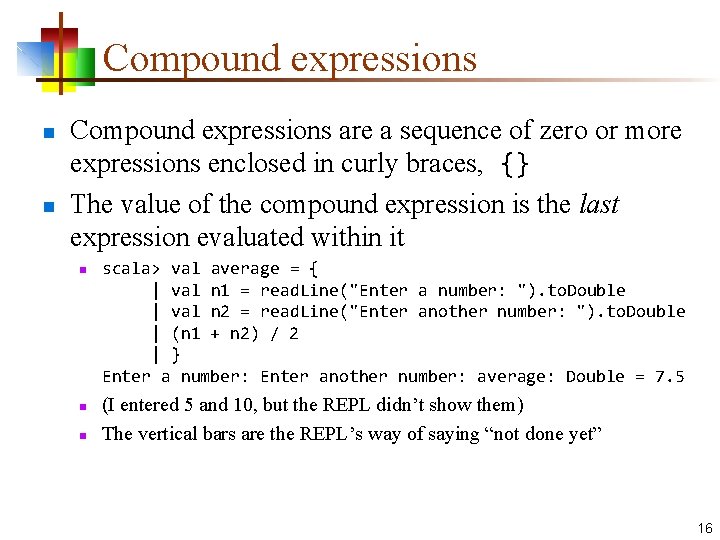
Compound expressions n n Compound expressions are a sequence of zero or more expressions enclosed in curly braces, {} The value of the compound expression is the last expression evaluated within it n n n scala> val average = { | val n 1 = read. Line("Enter a number: "). to. Double | val n 2 = read. Line("Enter another number: "). to. Double | (n 1 + n 2) / 2 | } Enter a number: Enter another number: average: Double = 7. 5 (I entered 5 and 10, but the REPL didn’t show them) The vertical bars are the REPL’s way of saying “not done yet” 16
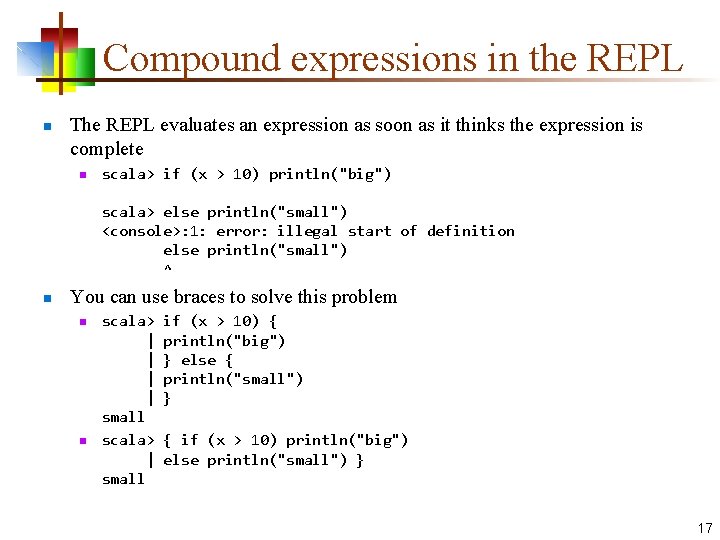
Compound expressions in the REPL n The REPL evaluates an expression as soon as it thinks the expression is complete n scala> if (x > 10) println("big") scala> else println("small") <console>: 1: error: illegal start of definition else println("small") ^ n You can use braces to solve this problem n n scala> | | small scala> | small if (x > 10) { println("big") } else { println("small") } { if (x > 10) println("big") else println("small") } 17
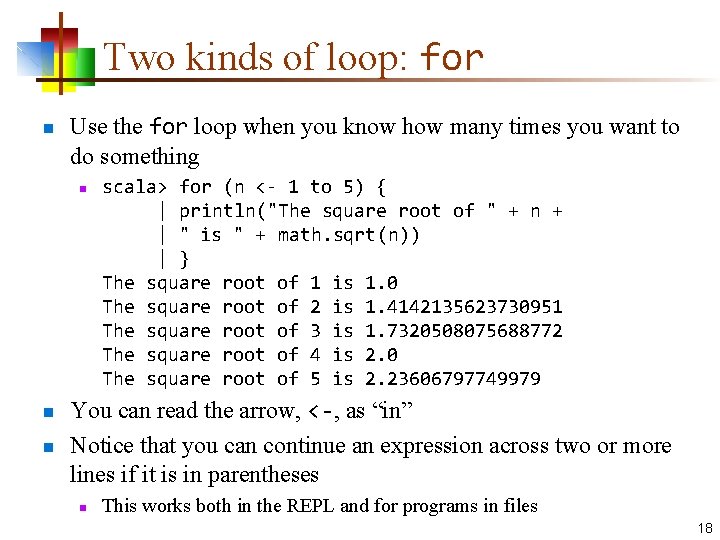
Two kinds of loop: for n Use the for loop when you know how many times you want to do something n n n scala> for (n <- 1 to 5) { | println("The square root of " + n + | " is " + math. sqrt(n)) | } The square root of 1 is 1. 0 The square root of 2 is 1. 4142135623730951 The square root of 3 is 1. 7320508075688772 The square root of 4 is 2. 0 The square root of 5 is 2. 23606797749979 You can read the arrow, <-, as “in” Notice that you can continue an expression across two or more lines if it is in parentheses n This works both in the REPL and for programs in files 18
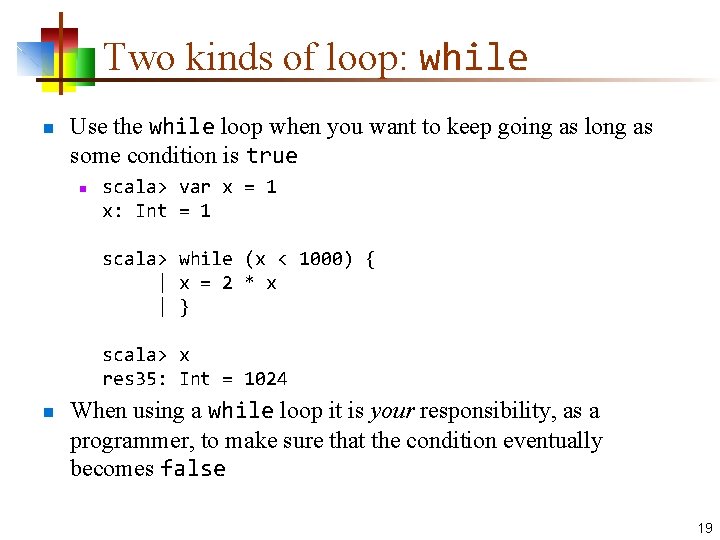
Two kinds of loop: while n Use the while loop when you want to keep going as long as some condition is true n scala> var x = 1 x: Int = 1 scala> while (x < 1000) { | x = 2 * x | } scala> x res 35: Int = 1024 n When using a while loop it is your responsibility, as a programmer, to make sure that the condition eventually becomes false 19
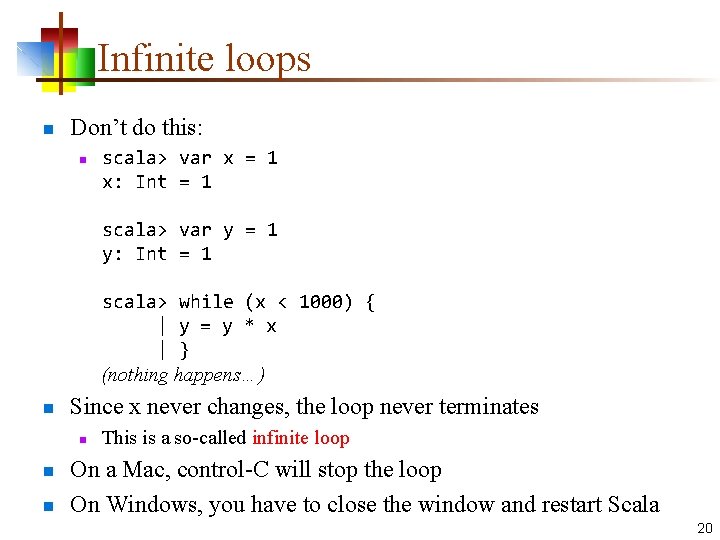
Infinite loops n Don’t do this: n scala> var x = 1 x: Int = 1 scala> var y = 1 y: Int = 1 scala> while (x < 1000) { | y = y * x | } (nothing happens…) n Since x never changes, the loop never terminates n n n This is a so-called infinite loop On a Mac, control-C will stop the loop On Windows, you have to close the window and restart Scala 20
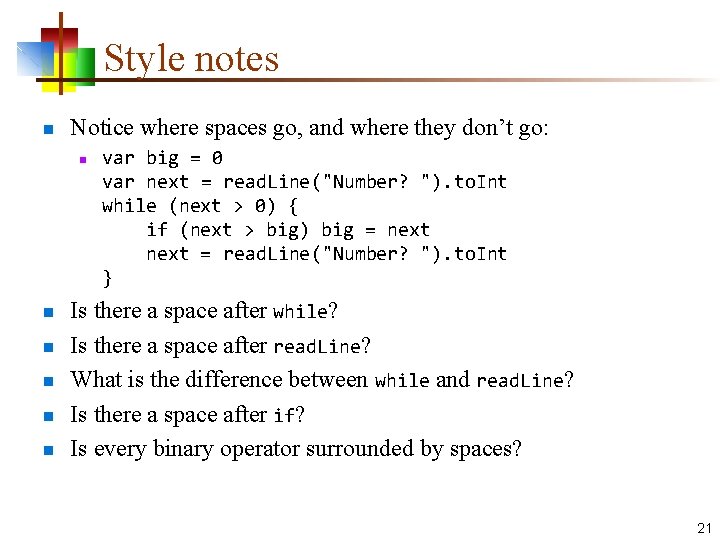
Style notes n Notice where spaces go, and where they don’t go: n n n var big = 0 var next = read. Line("Number? "). to. Int while (next > 0) { if (next > big) big = next = read. Line("Number? "). to. Int } Is there a space after while? Is there a space after read. Line? What is the difference between while and read. Line? Is there a space after if? Is every binary operator surrounded by spaces? 21
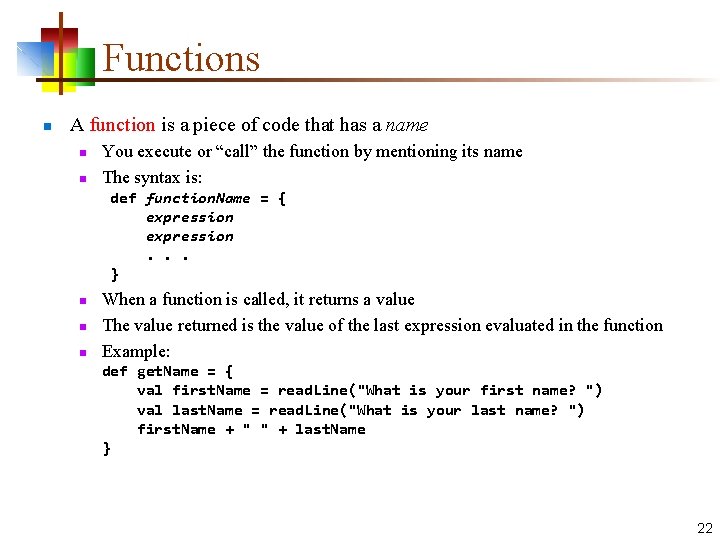
Functions n A function is a piece of code that has a name n n You execute or “call” the function by mentioning its name The syntax is: def function. Name = { expression. . . } n n n When a function is called, it returns a value The value returned is the value of the last expression evaluated in the function Example: def get. Name = { val first. Name = read. Line("What is your first name? ") val last. Name = read. Line("What is your last name? ") first. Name + " " + last. Name } 22
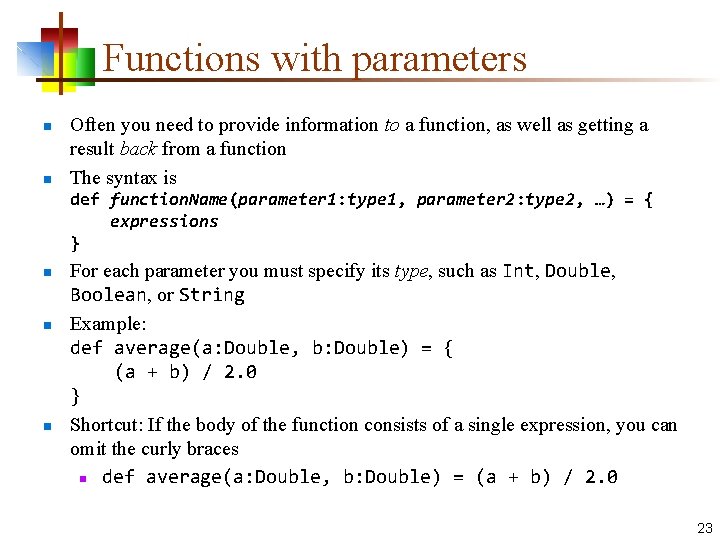
Functions with parameters n n Often you need to provide information to a function, as well as getting a result back from a function The syntax is def function. Name(parameter 1: type 1, parameter 2: type 2, …) = { expressions } n n n For each parameter you must specify its type, such as Int, Double, Boolean, or String Example: def average(a: Double, b: Double) = { (a + b) / 2. 0 } Shortcut: If the body of the function consists of a single expression, you can omit the curly braces n def average(a: Double, b: Double) = (a + b) / 2. 0 23
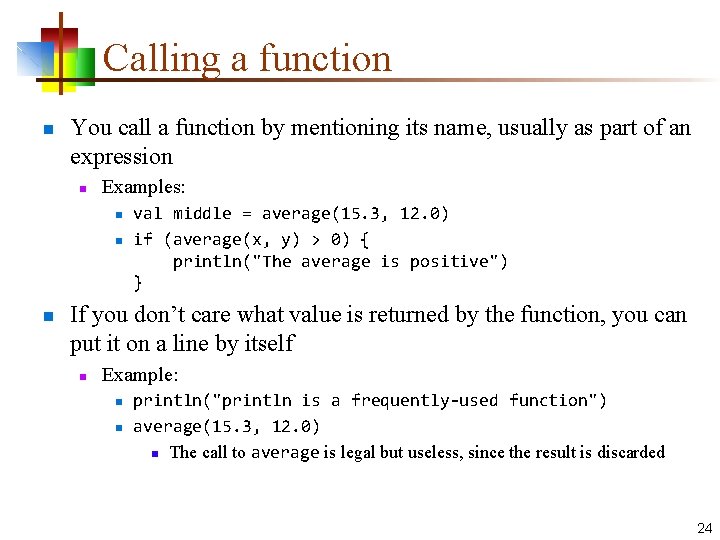
Calling a function n You call a function by mentioning its name, usually as part of an expression n Examples: n n n val middle = average(15. 3, 12. 0) if (average(x, y) > 0) { println("The average is positive") } If you don’t care what value is returned by the function, you can put it on a line by itself n Example: n n println("println is a frequently-used function") average(15. 3, 12. 0) n The call to average is legal but useless, since the result is discarded 24
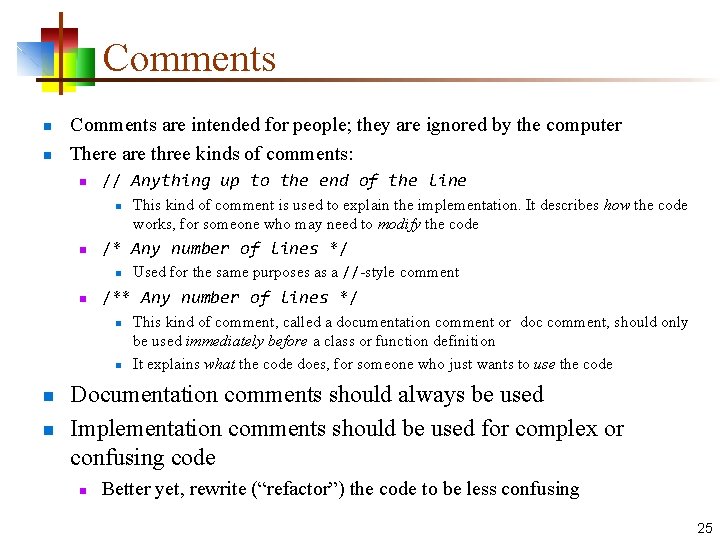
Comments n n Comments are intended for people; they are ignored by the computer There are three kinds of comments: n // Anything up to the end of the line n n /* Any number of lines */ n n Used for the same purposes as a //-style comment /** Any number of lines */ n n This kind of comment is used to explain the implementation. It describes how the code works, for someone who may need to modify the code This kind of comment, called a documentation comment or doc comment, should only be used immediately before a class or function definition It explains what the code does, for someone who just wants to use the code Documentation comments should always be used Implementation comments should be used for complex or confusing code n Better yet, rewrite (“refactor”) the code to be less confusing 25
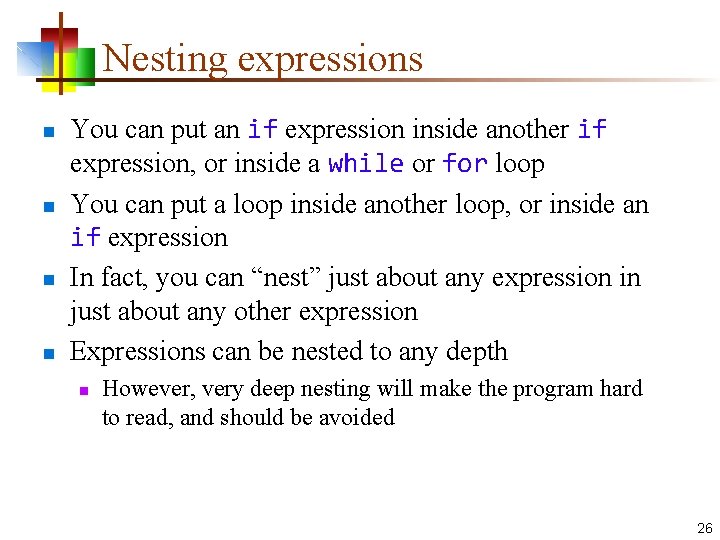
Nesting expressions n n You can put an if expression inside another if expression, or inside a while or for loop You can put a loop inside another loop, or inside an if expression In fact, you can “nest” just about any expression in just about any other expression Expressions can be nested to any depth n However, very deep nesting will make the program hard to read, and should be avoided 26
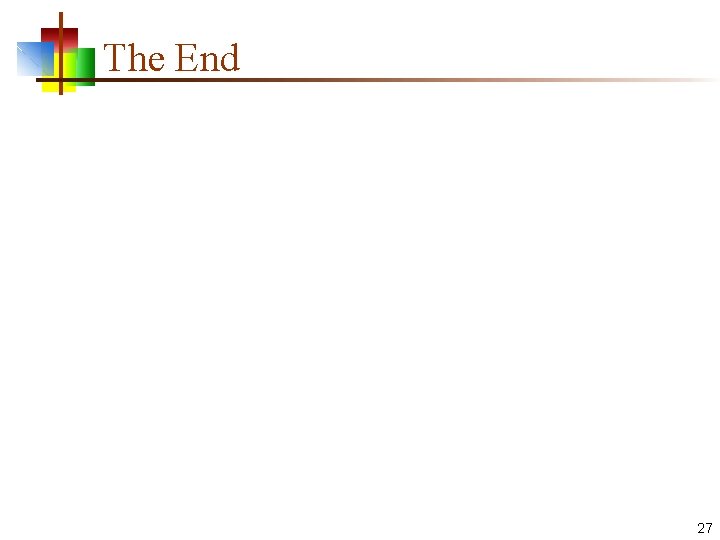
The End 27