INPUT OUTPUT AND EXCEPTIONS TERMINAL OUTPUT Use the
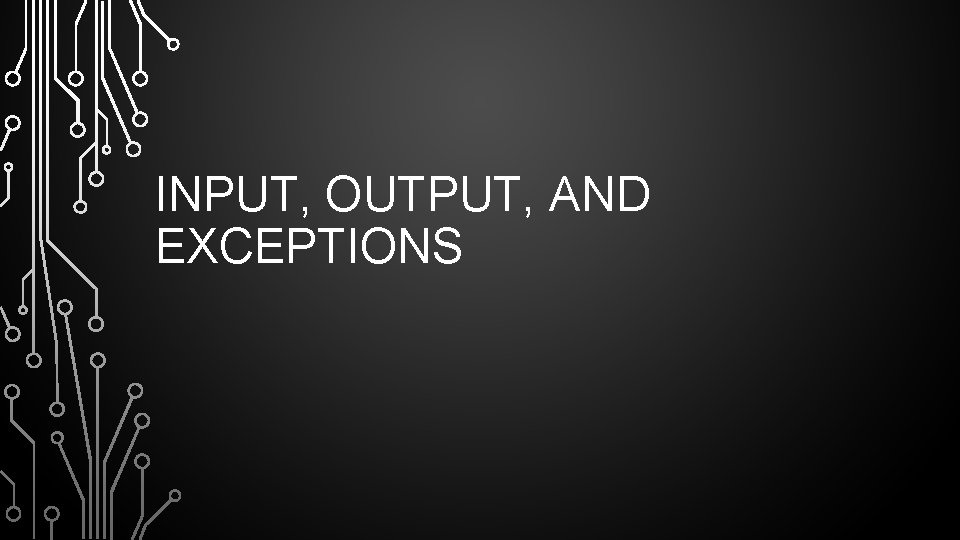
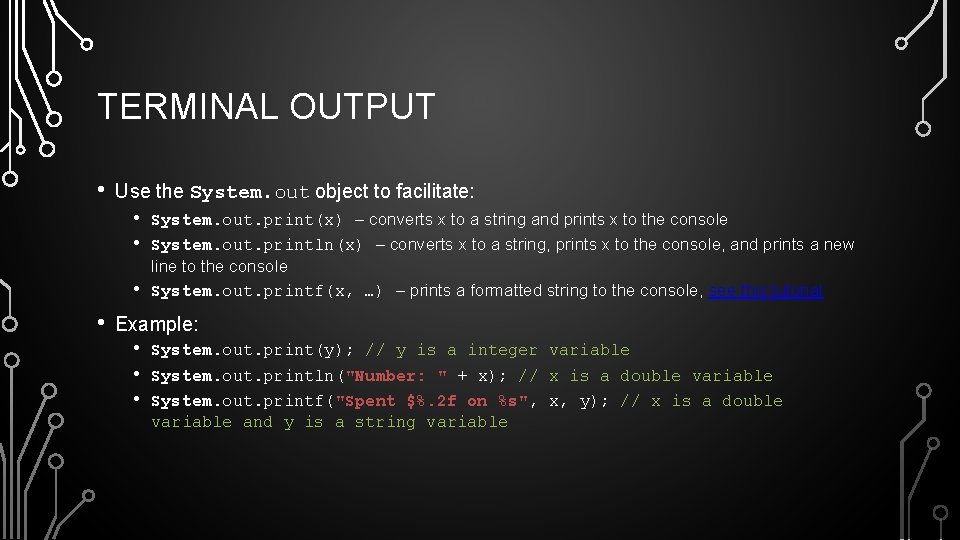
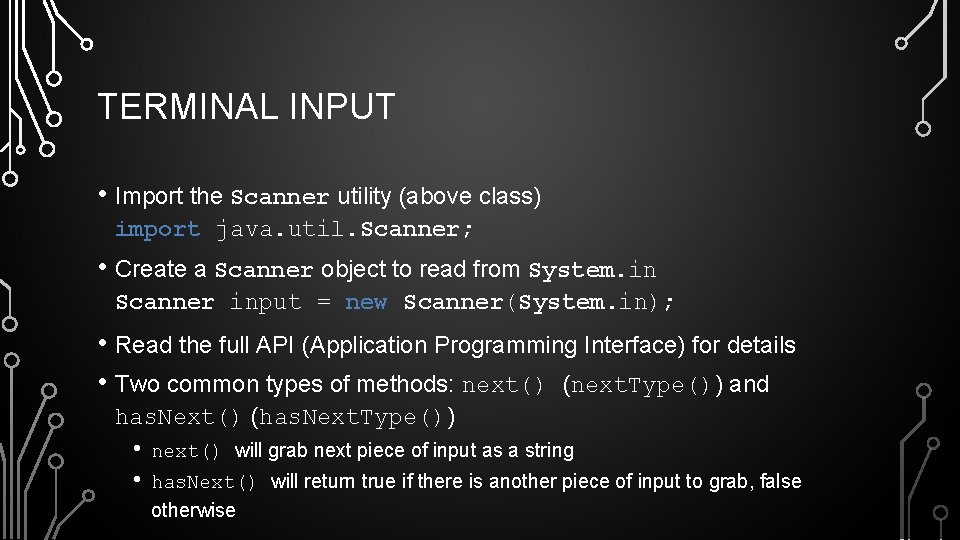
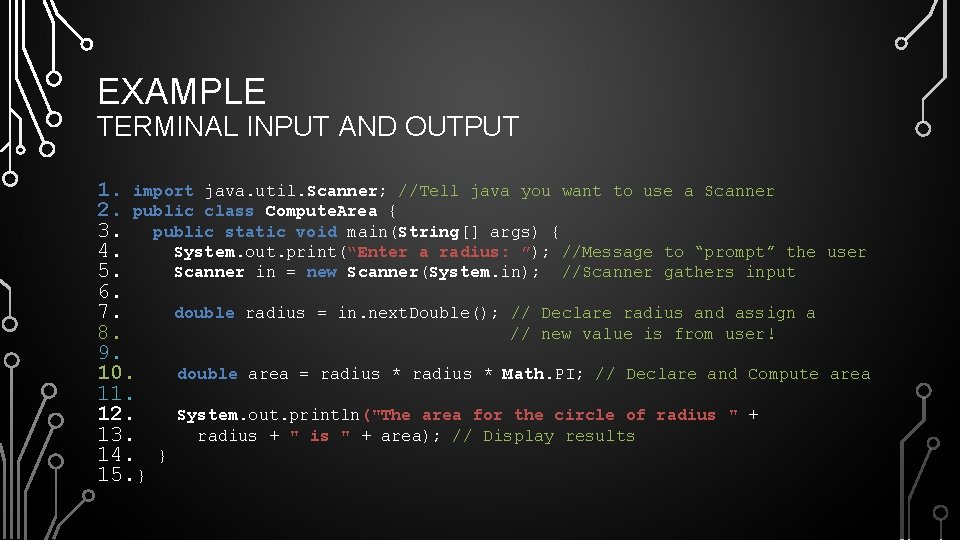
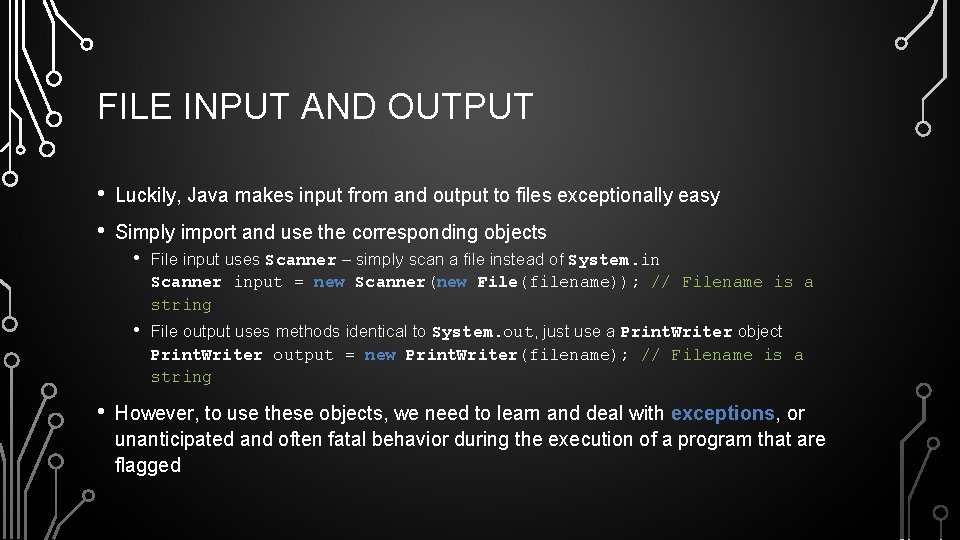
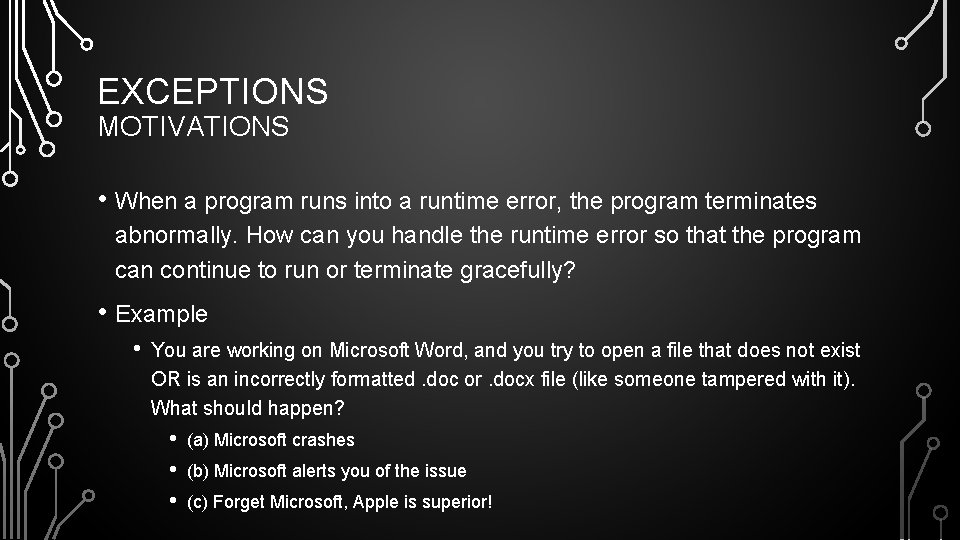
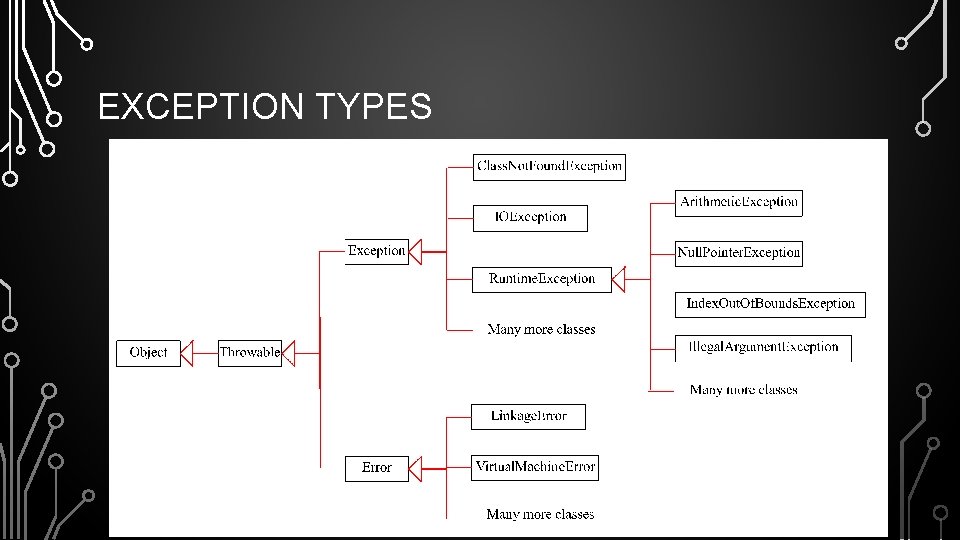
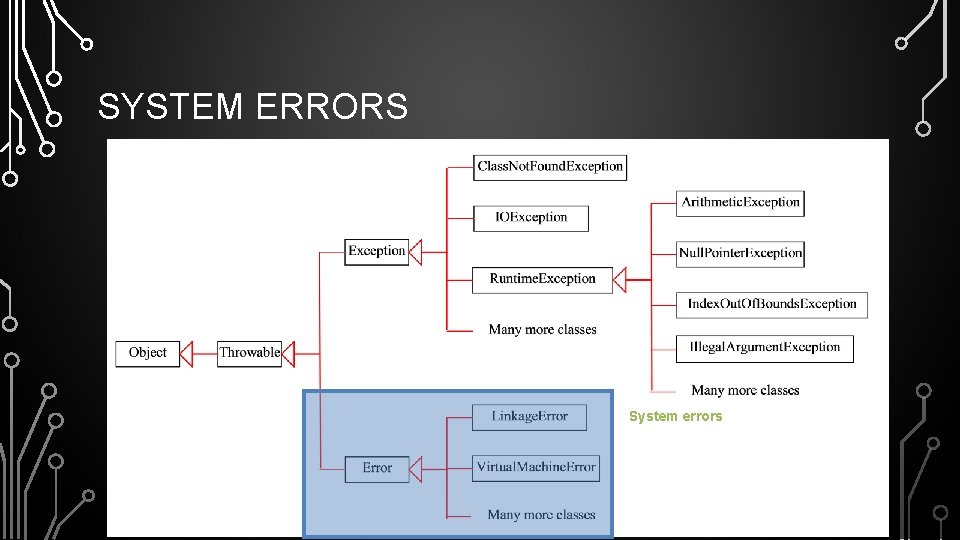
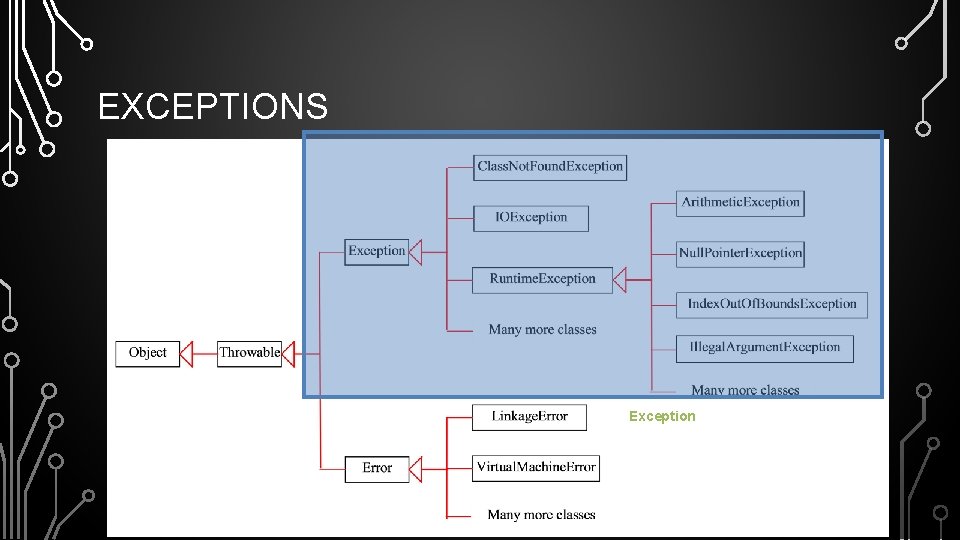
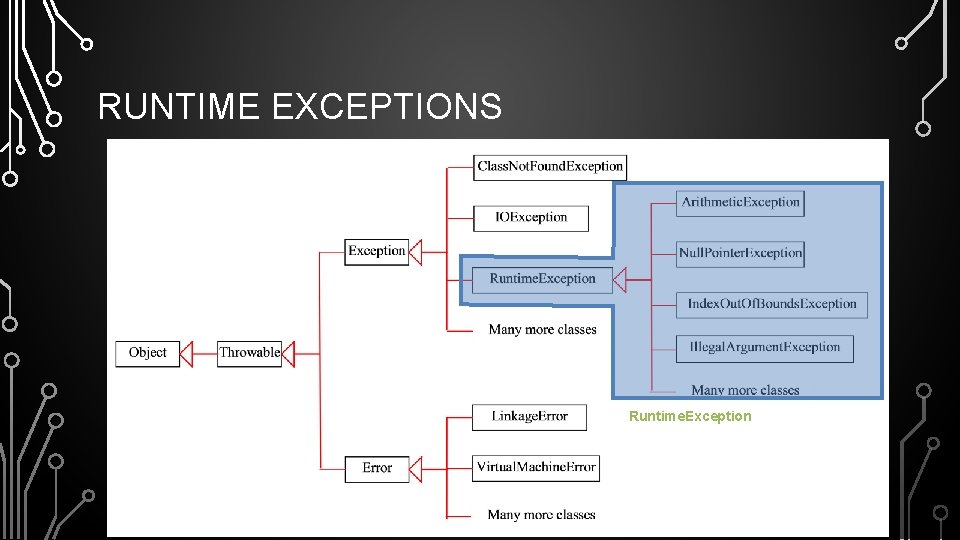
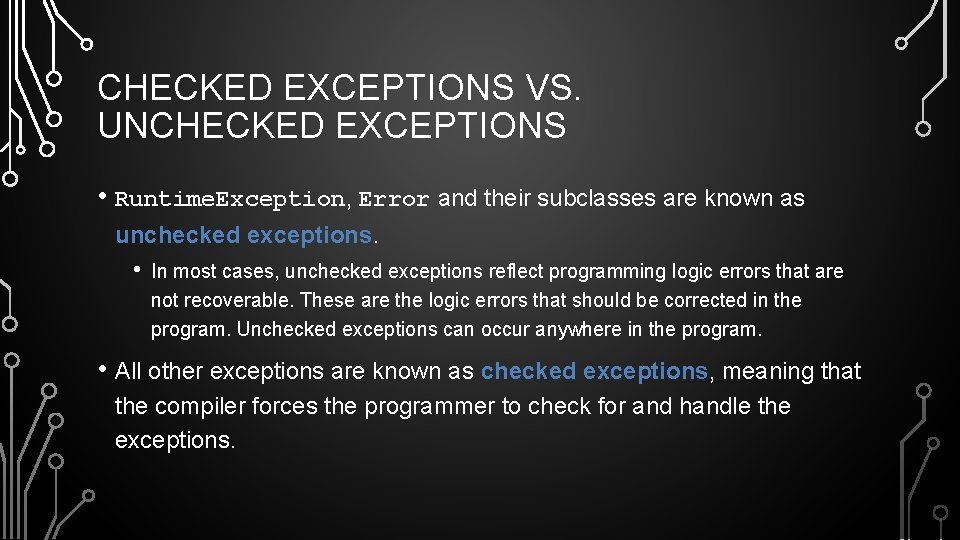
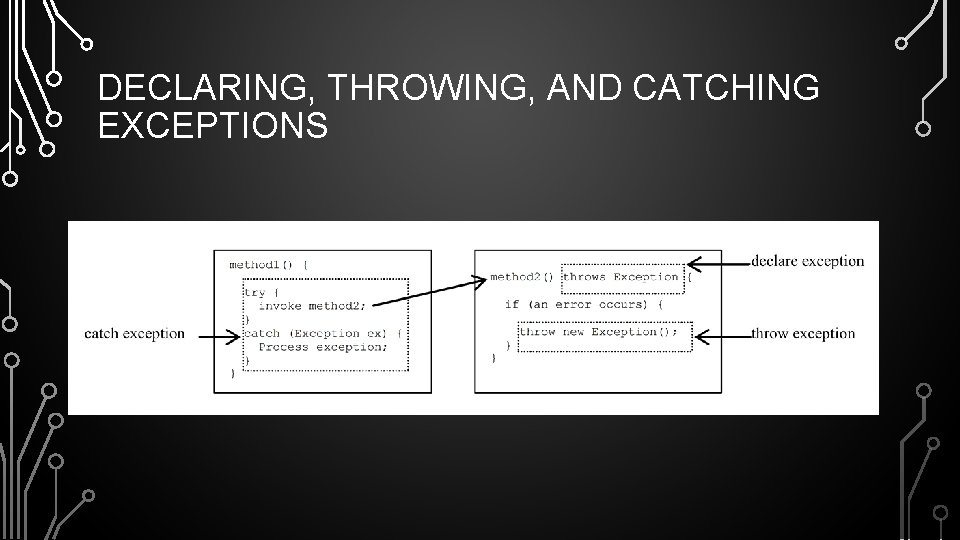
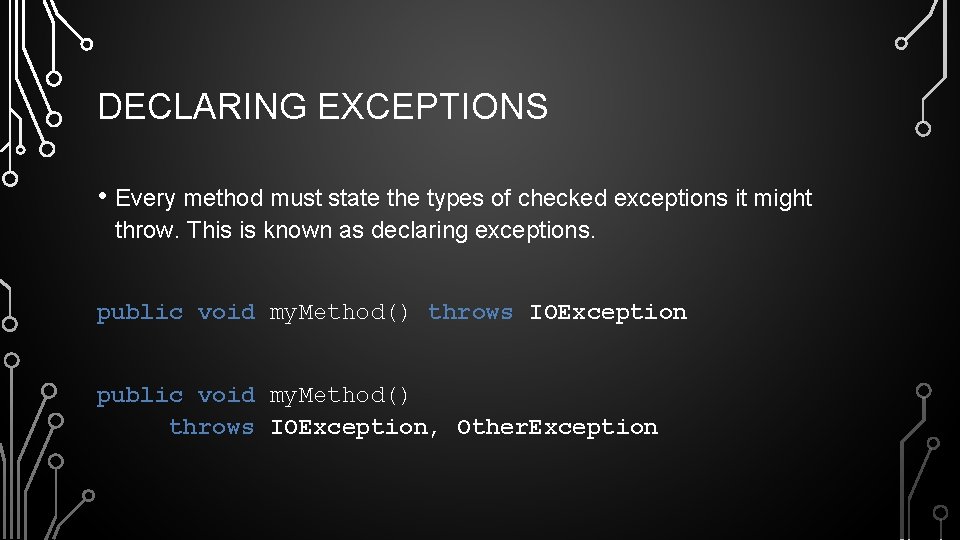
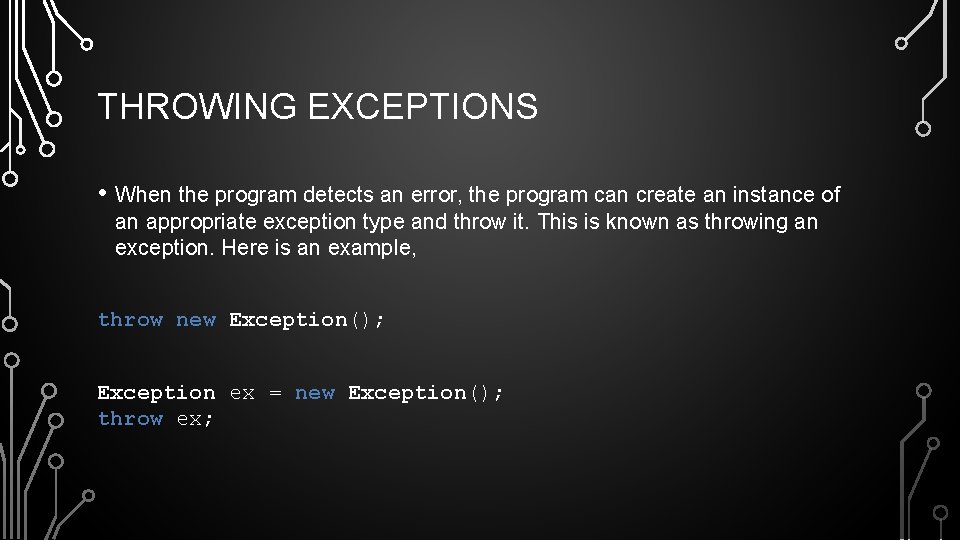
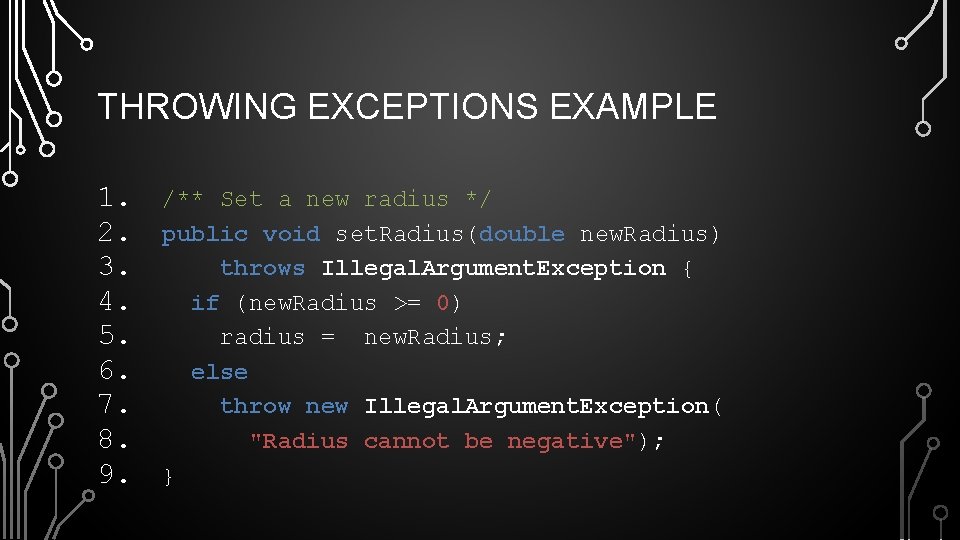
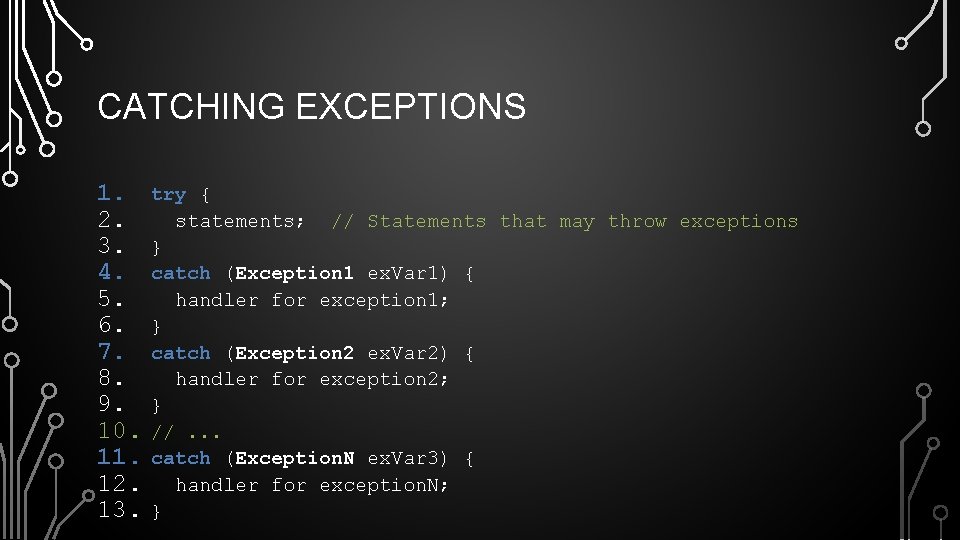
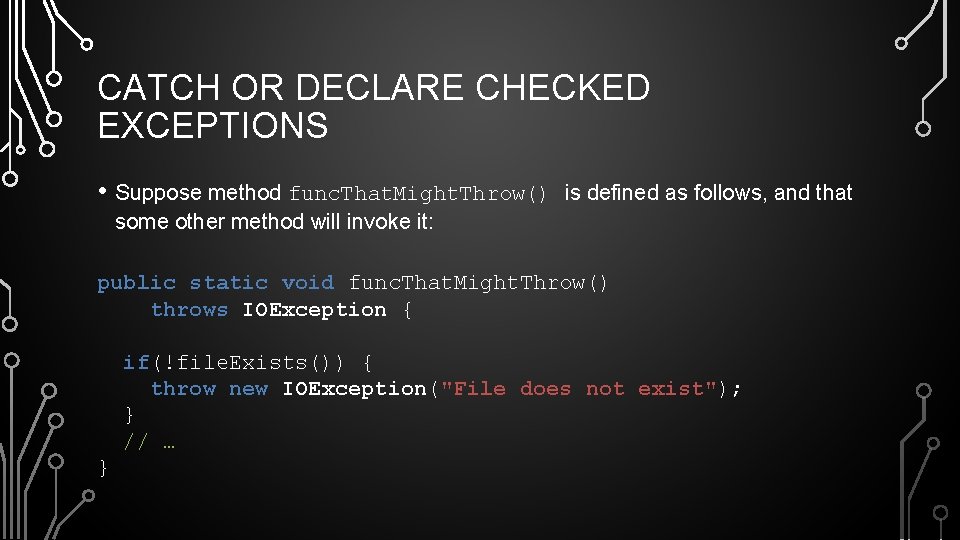
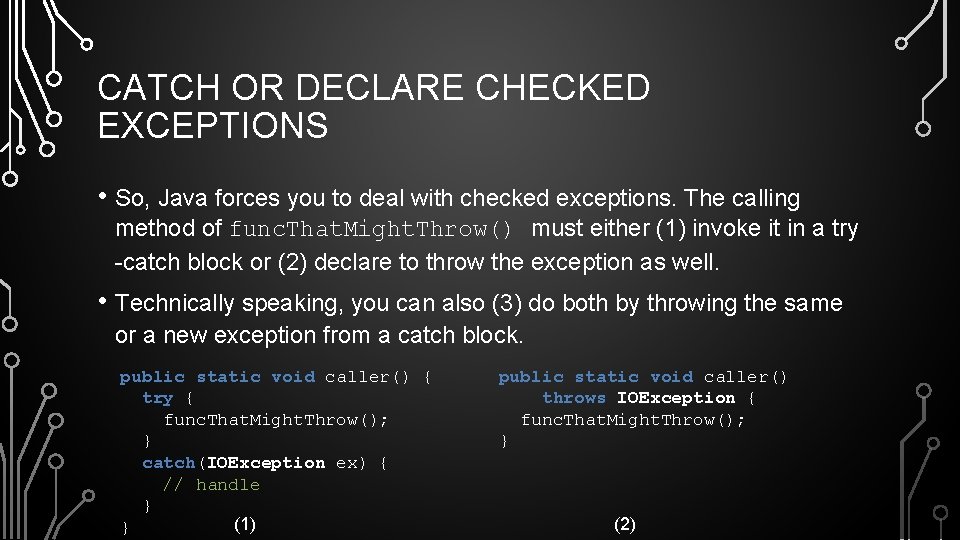
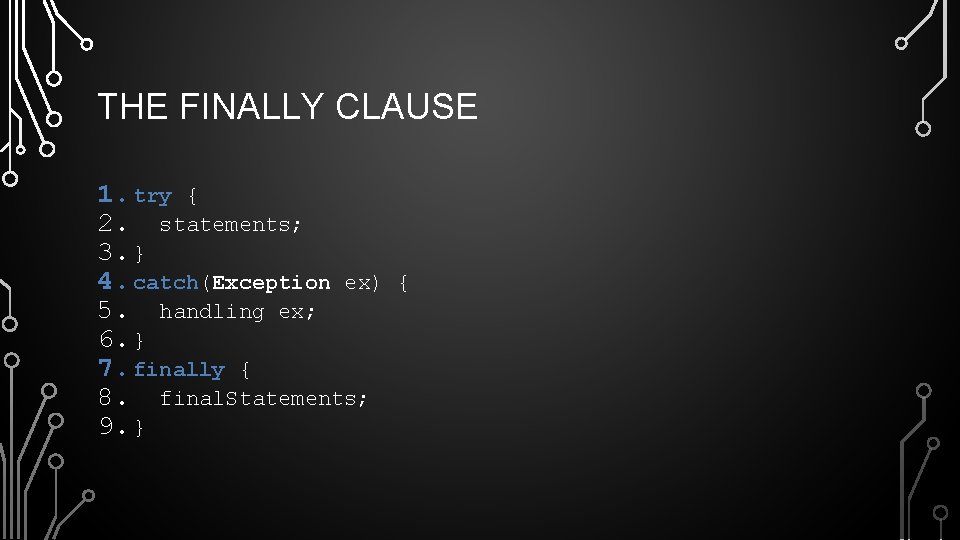
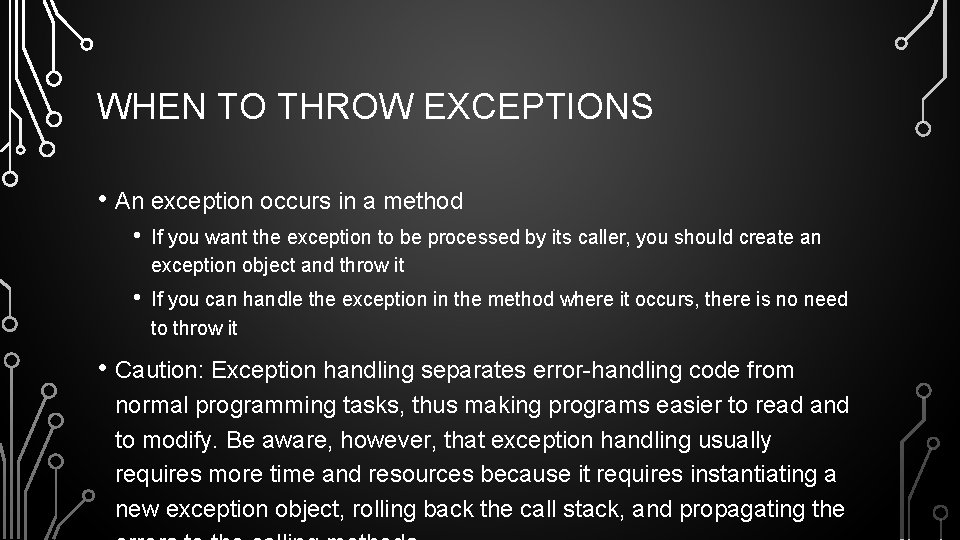
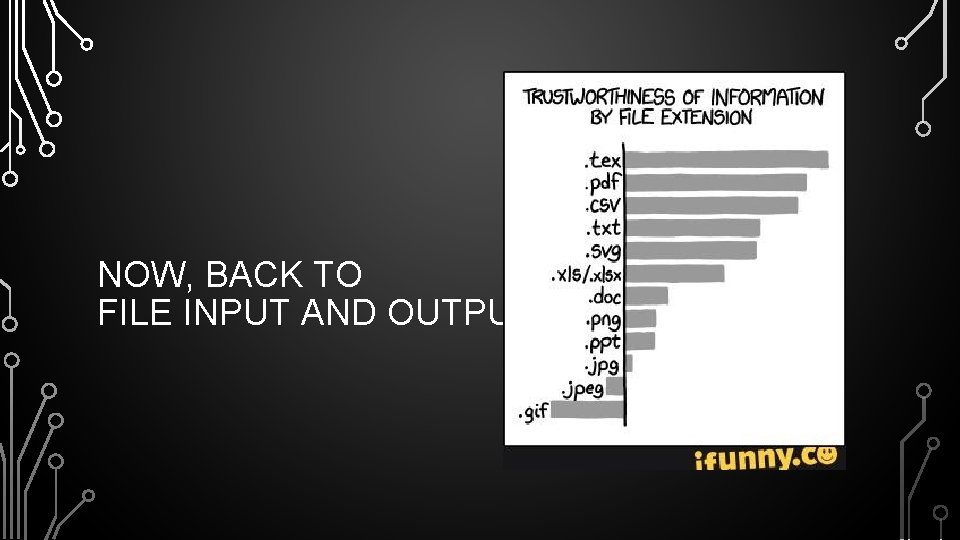
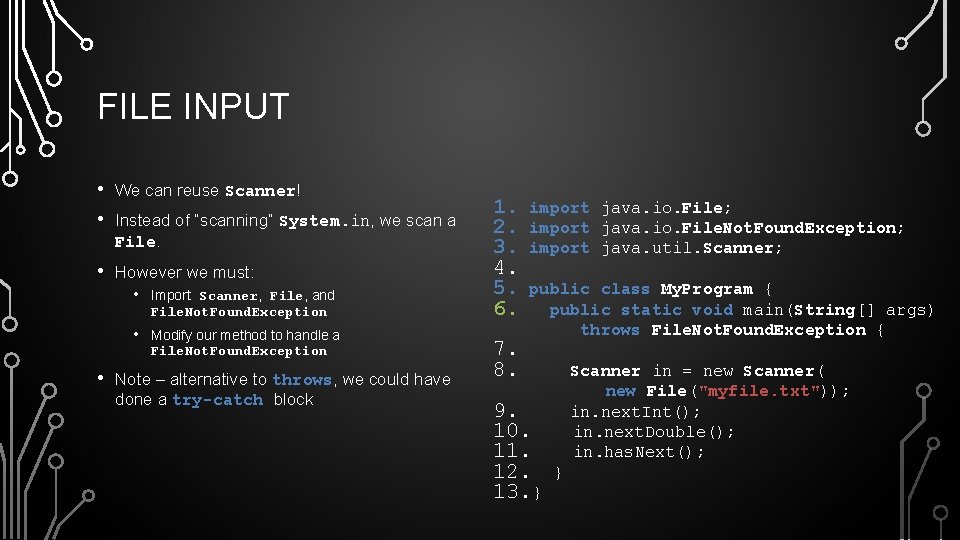
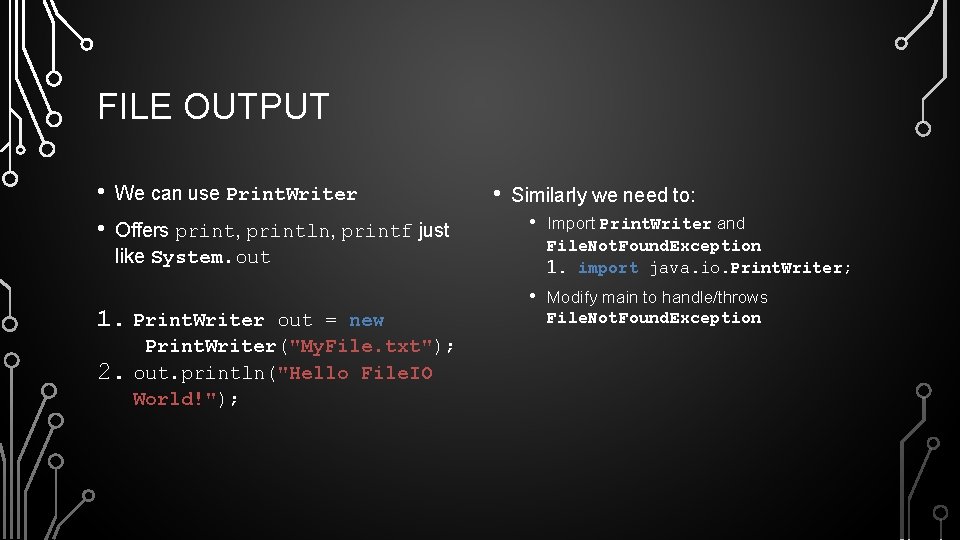
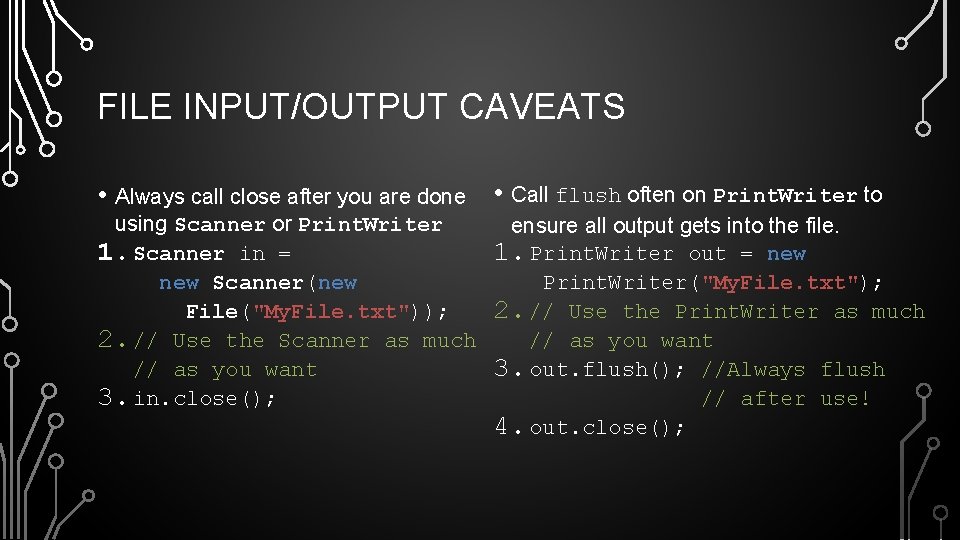
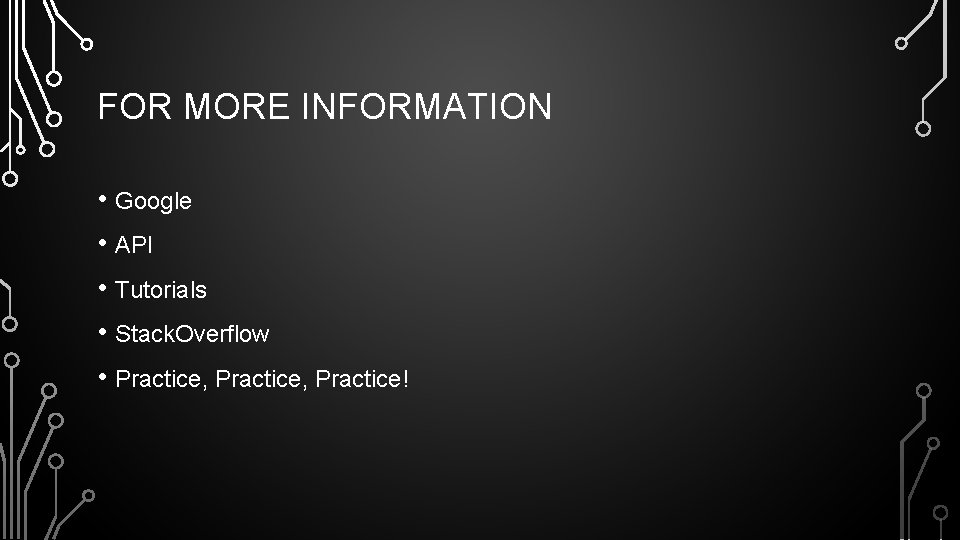
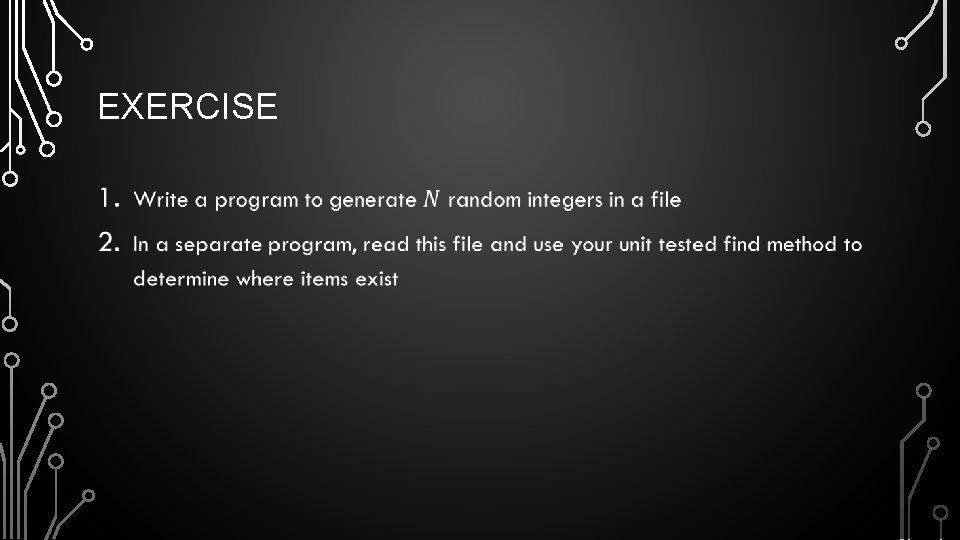
- Slides: 26
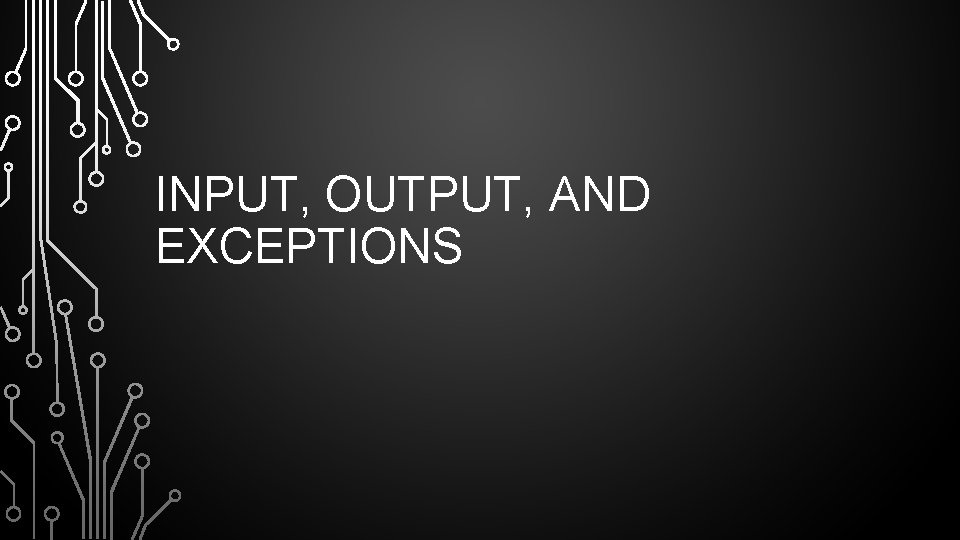
INPUT, OUTPUT, AND EXCEPTIONS
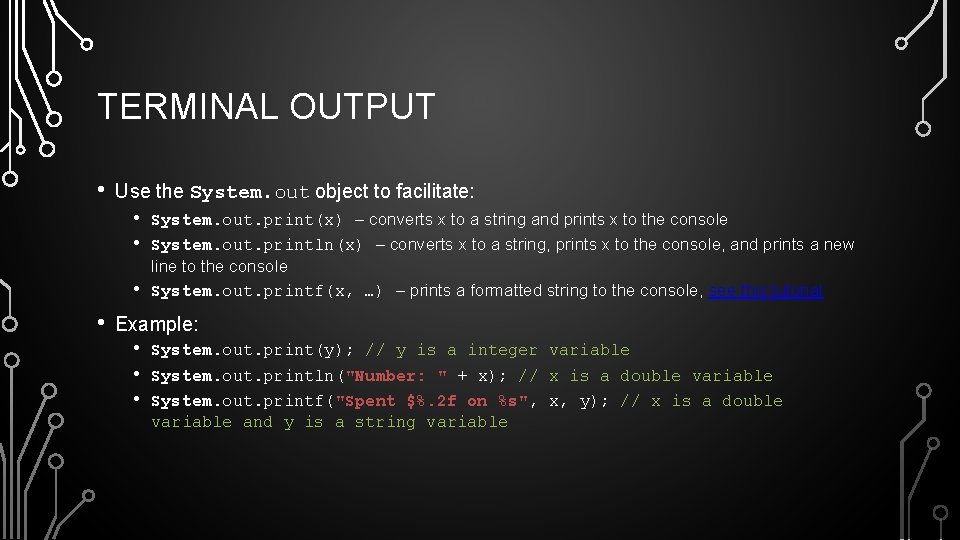
TERMINAL OUTPUT • Use the System. out object to facilitate: • • System. out. print(x) – converts x to a string and prints x to the console System. out. println(x) – converts x to a string, prints x to the console, and prints a new line to the console System. out. printf(x, …) – prints a formatted string to the console, see this tutorial Example: • • • System. out. print(y); // y is a integer variable System. out. println("Number: " + x); // x is a double variable System. out. printf("Spent $%. 2 f on %s", x, y); // x is a double variable and y is a string variable
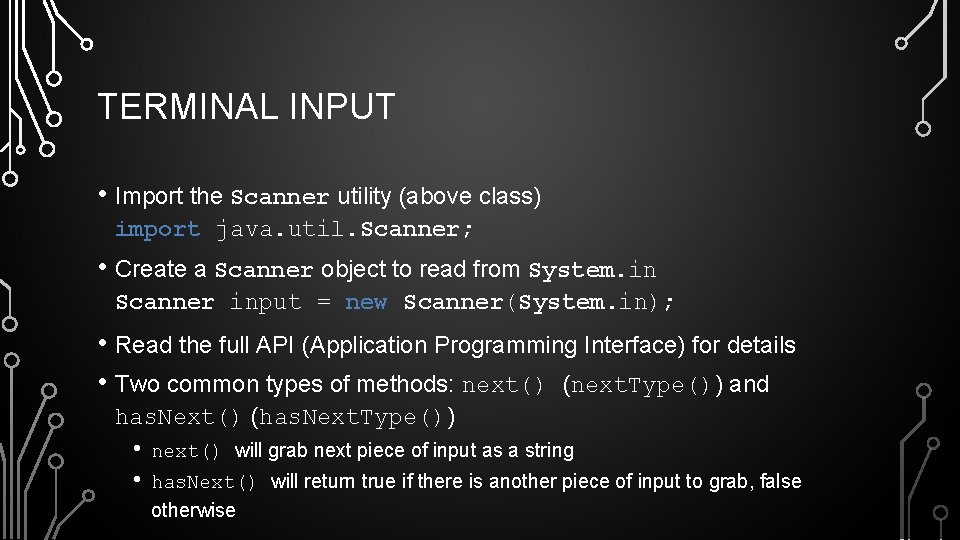
TERMINAL INPUT • Import the Scanner utility (above class) import java. util. Scanner; • Create a Scanner object to read from System. in Scanner input = new Scanner(System. in); • Read the full API (Application Programming Interface) for details • Two common types of methods: next() (next. Type()) and has. Next() (has. Next. Type()) • • next() will grab next piece of input as a string has. Next() will return true if there is another piece of input to grab, false otherwise
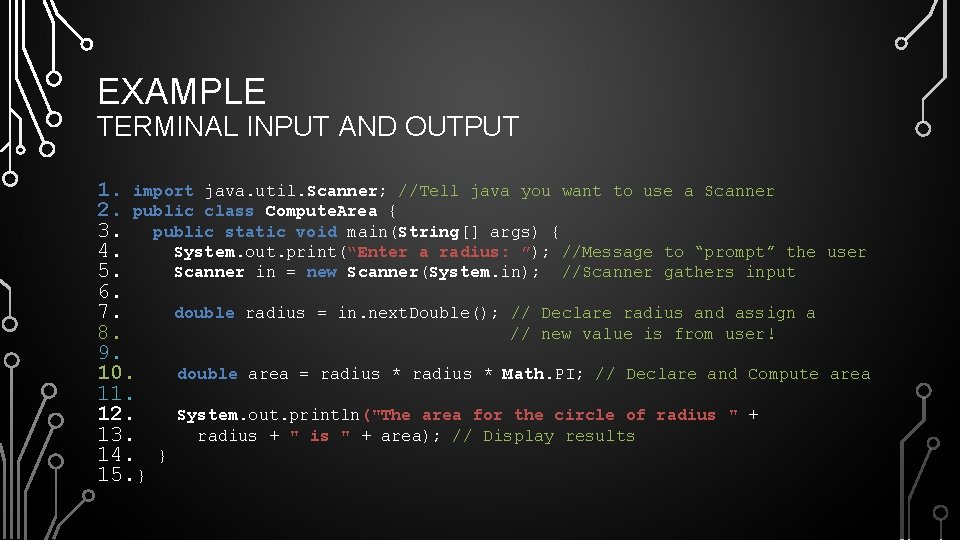
EXAMPLE TERMINAL INPUT AND OUTPUT 1. import java. util. Scanner; //Tell java you want to use a Scanner 2. public class Compute. Area { 3. public static void main(String[] args) { 4. System. out. print(“Enter a radius: ”); //Message to “prompt” the 5. Scanner in = new Scanner(System. in); //Scanner gathers input 6. 7. double radius = in. next. Double(); // Declare radius and assign a 8. // new value is from user! 9. 10. double area = radius * Math. PI; // Declare and Compute 11. 12. System. out. println("The area for the circle of radius " + 13. radius + " is " + area); // Display results 14. } 15. } user area
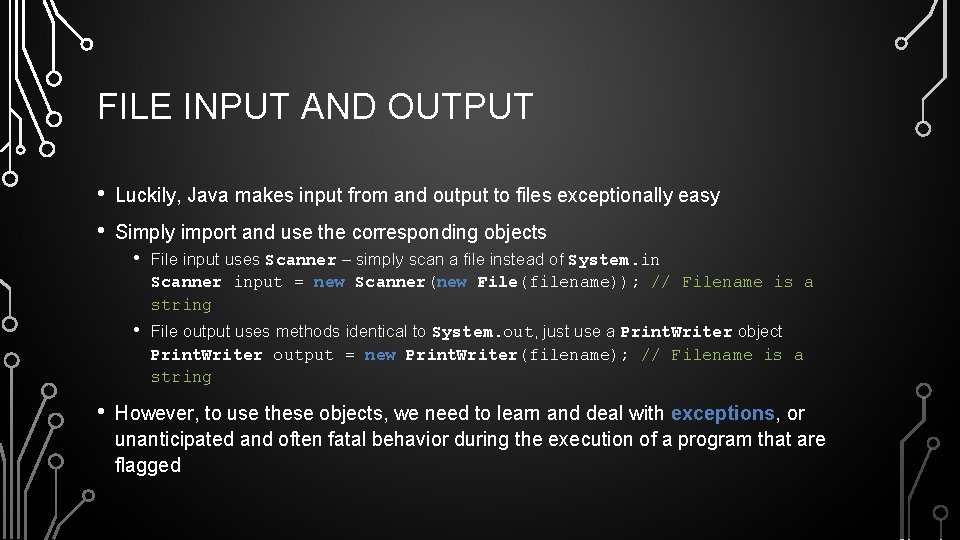
FILE INPUT AND OUTPUT • • • Luckily, Java makes input from and output to files exceptionally easy Simply import and use the corresponding objects • File input uses Scanner – simply scan a file instead of System. in Scanner input = new Scanner(new File(filename)); // Filename is a string • File output uses methods identical to System. out, just use a Print. Writer object Print. Writer output = new Print. Writer(filename); // Filename is a string However, to use these objects, we need to learn and deal with exceptions, or unanticipated and often fatal behavior during the execution of a program that are flagged
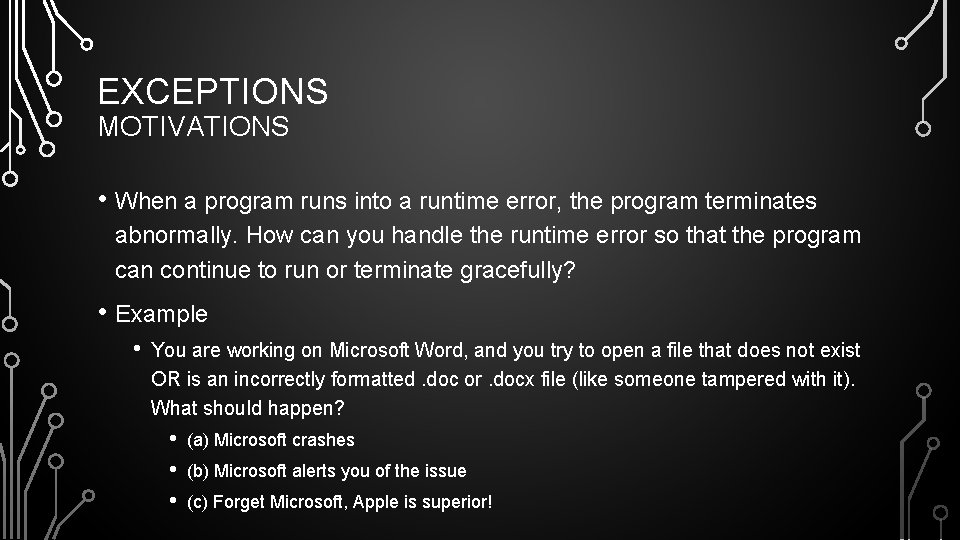
EXCEPTIONS MOTIVATIONS • When a program runs into a runtime error, the program terminates abnormally. How can you handle the runtime error so that the program can continue to run or terminate gracefully? • Example • You are working on Microsoft Word, and you try to open a file that does not exist OR is an incorrectly formatted. doc or. docx file (like someone tampered with it). What should happen? • • • (a) Microsoft crashes (b) Microsoft alerts you of the issue (c) Forget Microsoft, Apple is superior!
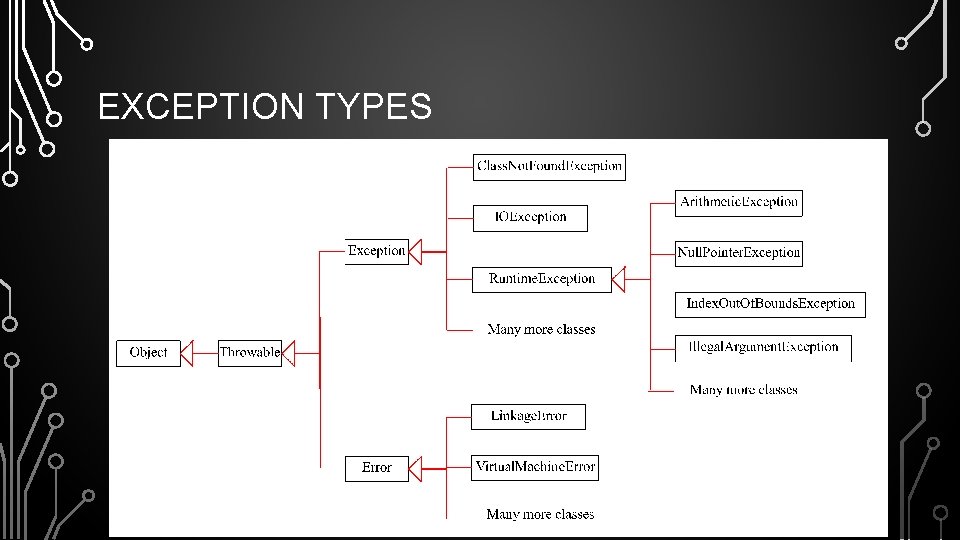
EXCEPTION TYPES
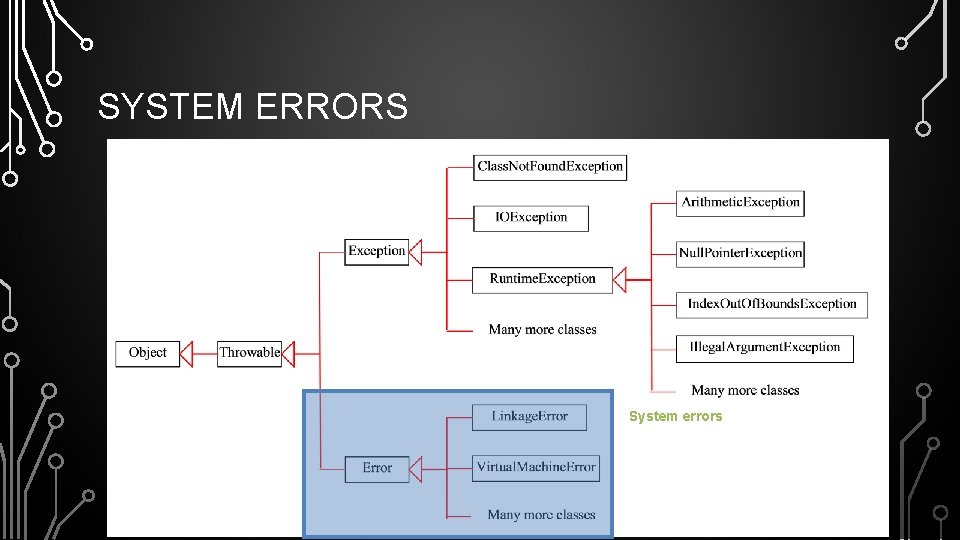
SYSTEM ERRORS System errors are thrown by JVM and represented in the Error class. The Error class describes internal system errors. Such errors rarely occur. If one does, there is little you can do beyond notifying the user and trying to terminate the program gracefully.
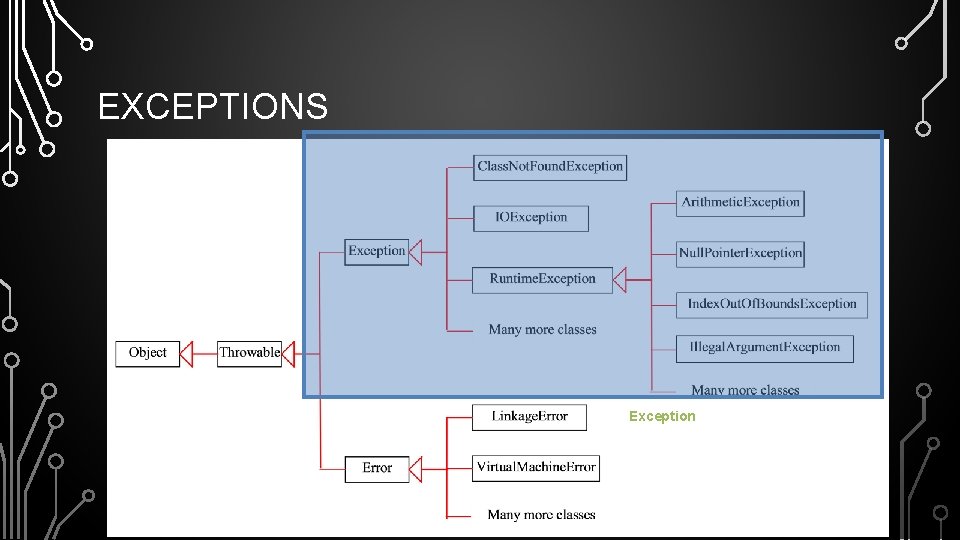
EXCEPTIONS Exception describes errors caused by your program and external circumstances. These errors can be caught and handled by your program.
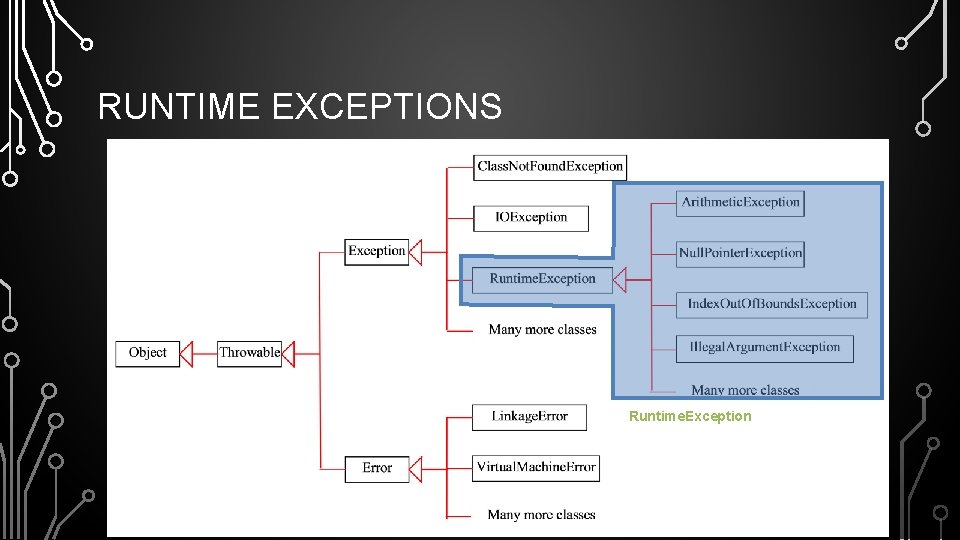
RUNTIME EXCEPTIONS Runtime. Exception is caused by programming errors, such as bad casting, accessing an out-of-bounds array, and numeric errors.
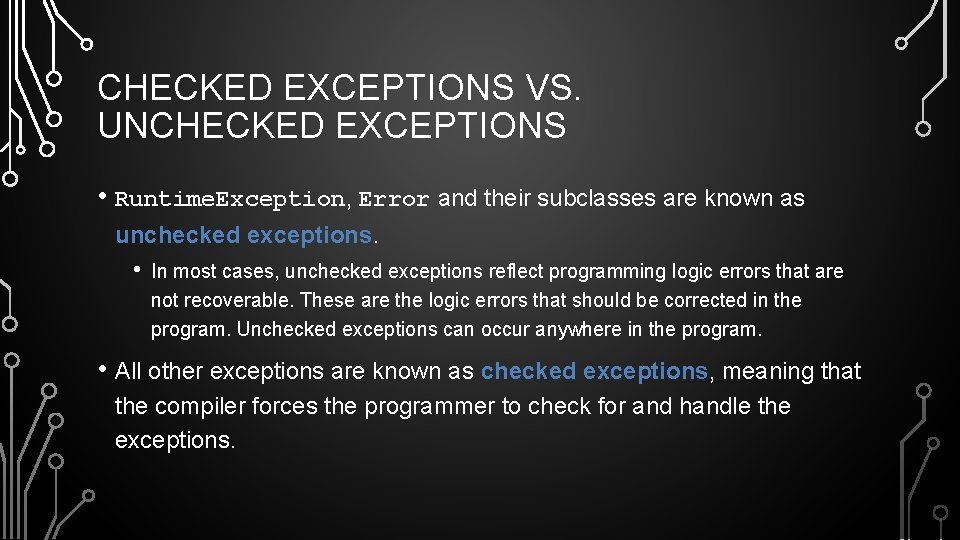
CHECKED EXCEPTIONS VS. UNCHECKED EXCEPTIONS • Runtime. Exception, Error and their subclasses are known as unchecked exceptions. • In most cases, unchecked exceptions reflect programming logic errors that are not recoverable. These are the logic errors that should be corrected in the program. Unchecked exceptions can occur anywhere in the program. • All other exceptions are known as checked exceptions, meaning that the compiler forces the programmer to check for and handle the exceptions.
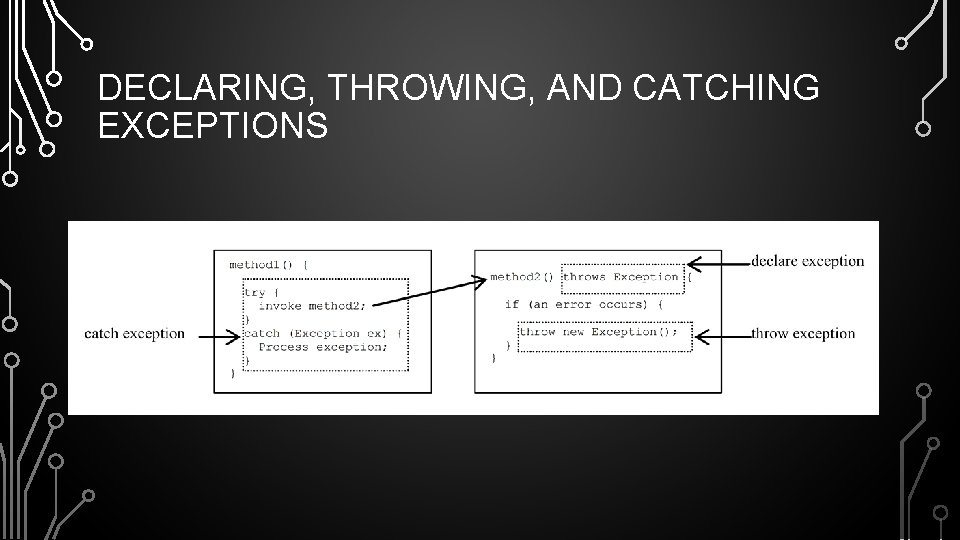
DECLARING, THROWING, AND CATCHING EXCEPTIONS
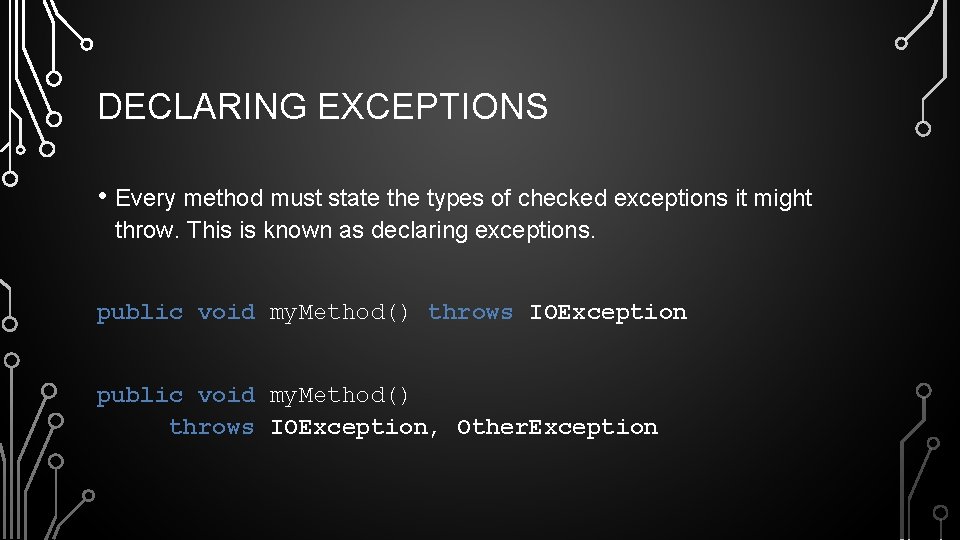
DECLARING EXCEPTIONS • Every method must state the types of checked exceptions it might throw. This is known as declaring exceptions. public void my. Method() throws IOException, Other. Exception
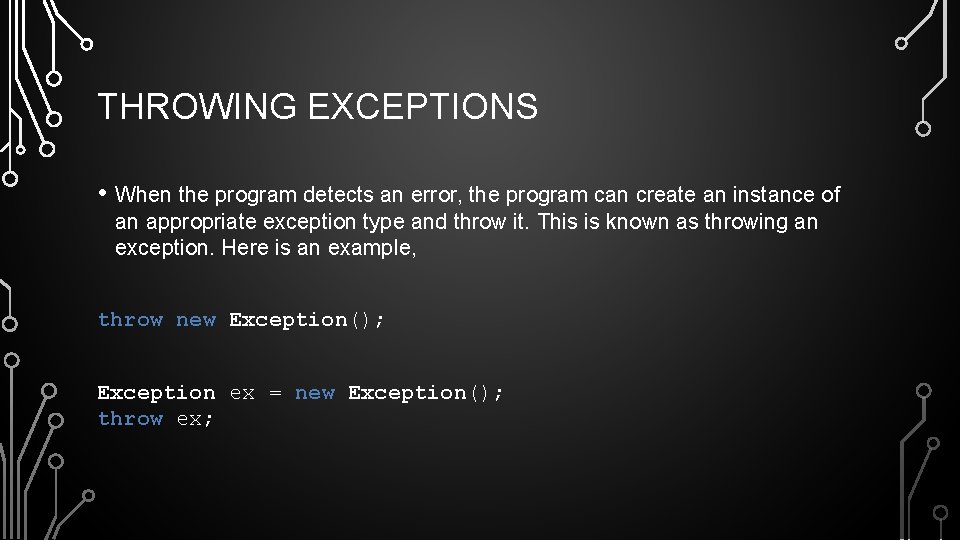
THROWING EXCEPTIONS • When the program detects an error, the program can create an instance of an appropriate exception type and throw it. This is known as throwing an exception. Here is an example, throw new Exception(); Exception ex = new Exception(); throw ex;
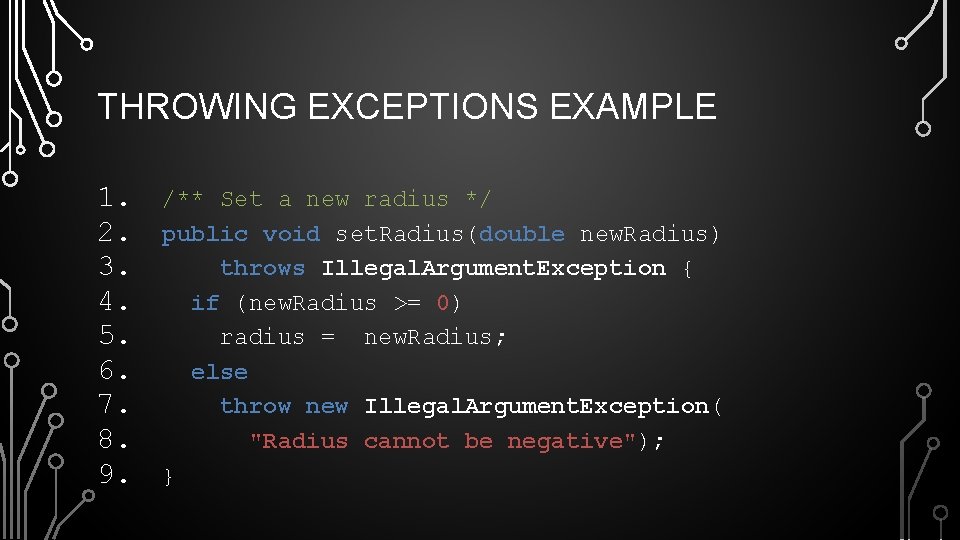
THROWING EXCEPTIONS EXAMPLE 1. 2. 3. 4. 5. 6. 7. 8. 9. /** Set a new radius */ public void set. Radius(double new. Radius) throws Illegal. Argument. Exception { if (new. Radius >= 0) radius = new. Radius; else throw new Illegal. Argument. Exception( "Radius cannot be negative"); }
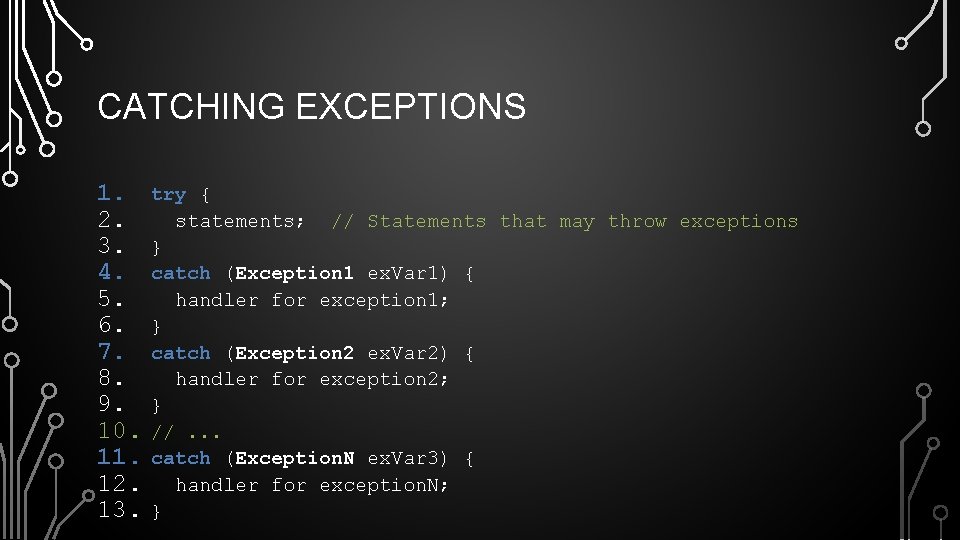
CATCHING EXCEPTIONS 1. 2. 3. 4. 5. 6. 7. 8. 9. 10. 11. 12. 13. try { statements; // Statements that may throw exceptions } catch (Exception 1 ex. Var 1) { handler for exception 1; } catch (Exception 2 ex. Var 2) { handler for exception 2; } //. . . catch (Exception. N ex. Var 3) { handler for exception. N; }
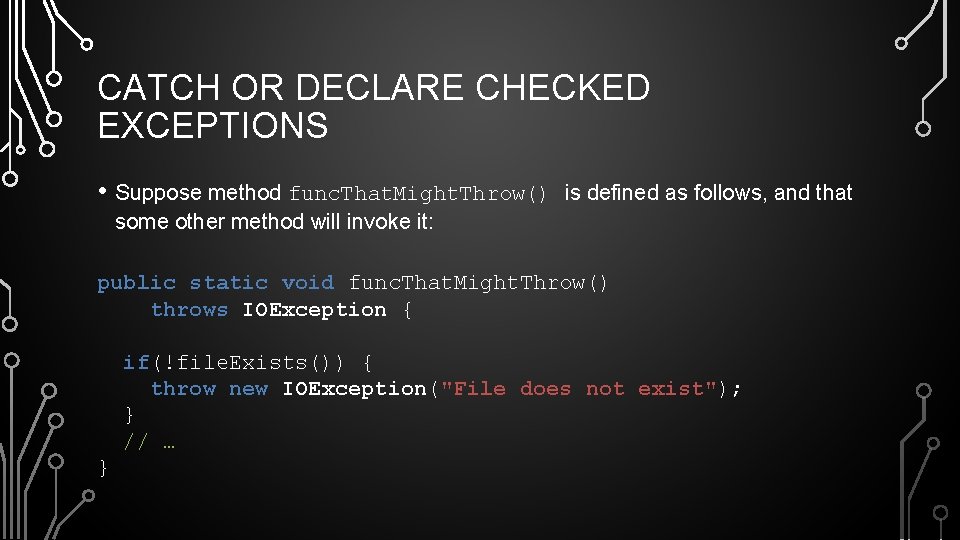
CATCH OR DECLARE CHECKED EXCEPTIONS • Suppose method func. That. Might. Throw() is defined as follows, and that some other method will invoke it: public static void func. That. Might. Throw() throws IOException { if(!file. Exists()) { throw new IOException("File does not exist"); } // … }
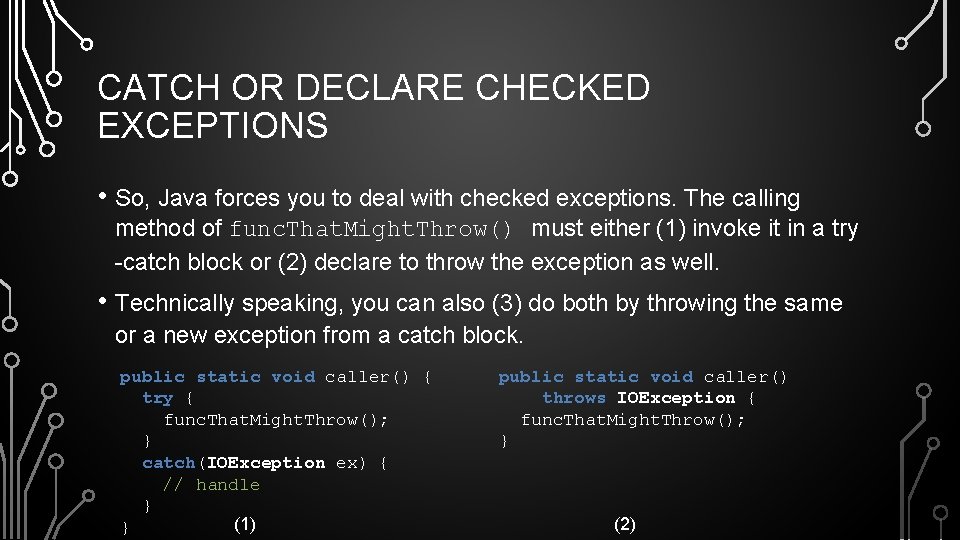
CATCH OR DECLARE CHECKED EXCEPTIONS • So, Java forces you to deal with checked exceptions. The calling method of func. That. Might. Throw() must either (1) invoke it in a try -catch block or (2) declare to throw the exception as well. • Technically speaking, you can also (3) do both by throwing the same or a new exception from a catch block. public static void caller() { try { func. That. Might. Throw(); } catch(IOException ex) { // handle } (1) } public static void caller() throws IOException { func. That. Might. Throw(); } (2)
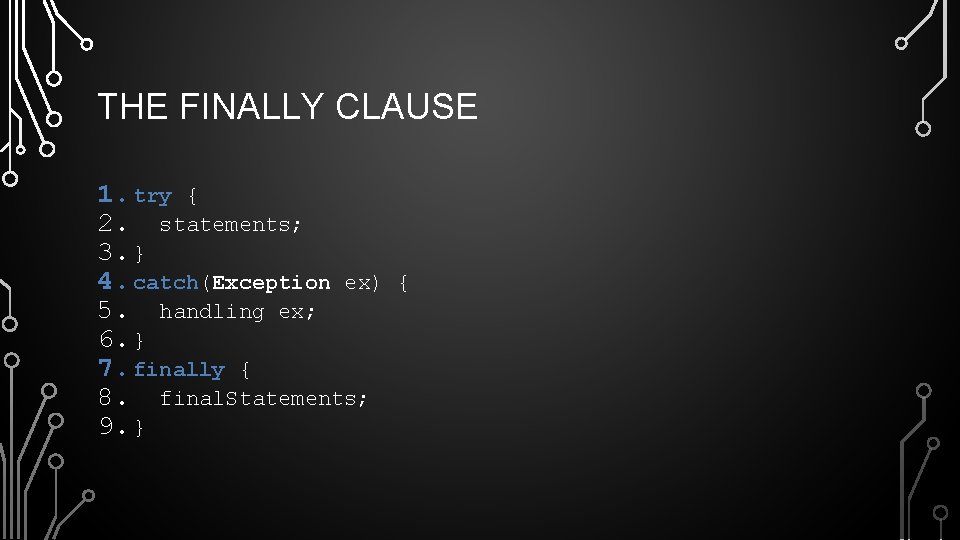
THE FINALLY CLAUSE 1. try { 2. statements; 3. } 4. catch(Exception ex) 5. handling ex; 6. } 7. finally { 8. final. Statements; 9. } {
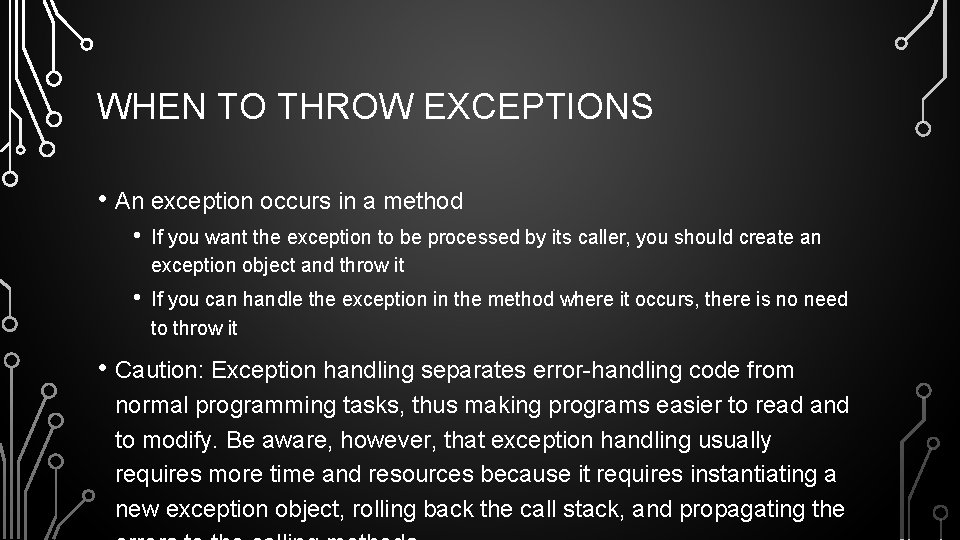
WHEN TO THROW EXCEPTIONS • An exception occurs in a method • If you want the exception to be processed by its caller, you should create an exception object and throw it • If you can handle the exception in the method where it occurs, there is no need to throw it • Caution: Exception handling separates error-handling code from normal programming tasks, thus making programs easier to read and to modify. Be aware, however, that exception handling usually requires more time and resources because it requires instantiating a new exception object, rolling back the call stack, and propagating the
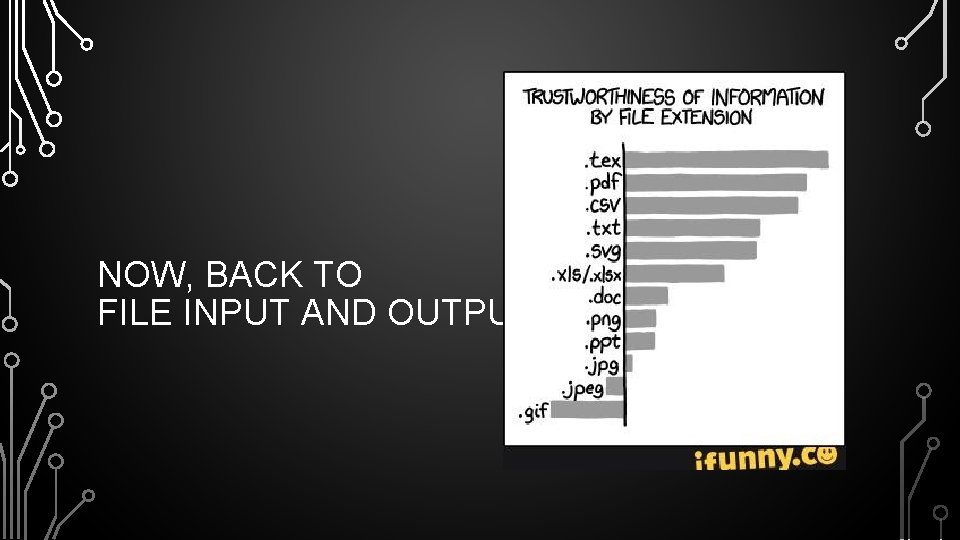
NOW, BACK TO FILE INPUT AND OUTPUT
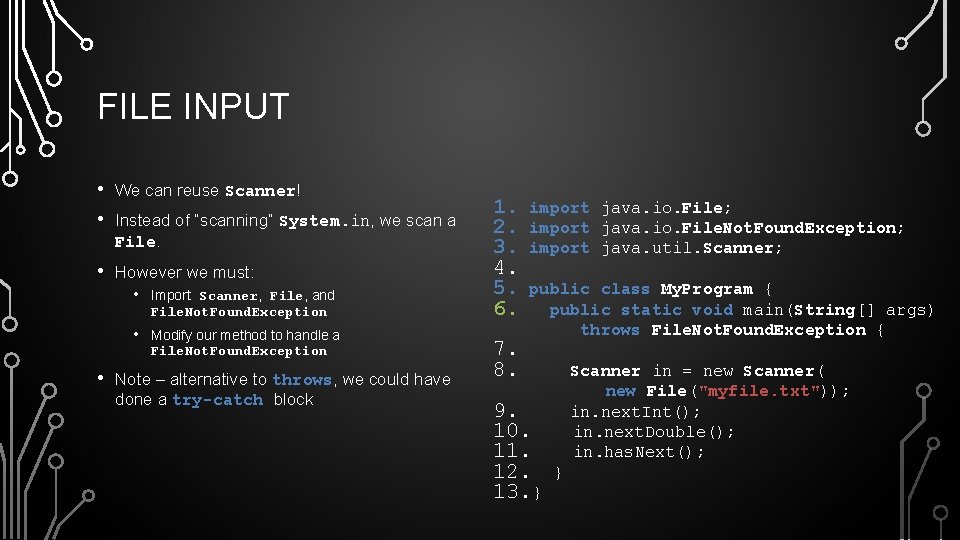
FILE INPUT • • We can reuse Scanner! • However we must: • Instead of “scanning” System. in, we scan a File. • Import Scanner, File, and File. Not. Found. Exception • Modify our method to handle a File. Not. Found. Exception Note – alternative to throws, we could have done a try-catch block 1. 2. 3. 4. 5. 6. 7. 8. import java. io. File; import java. io. File. Not. Found. Exception; import java. util. Scanner; public class My. Program { public static void main(String[] args) throws File. Not. Found. Exception { 9. 10. 11. 12. 13. } Scanner in = new Scanner( new File("myfile. txt")); in. next. Int(); in. next. Double(); in. has. Next(); }
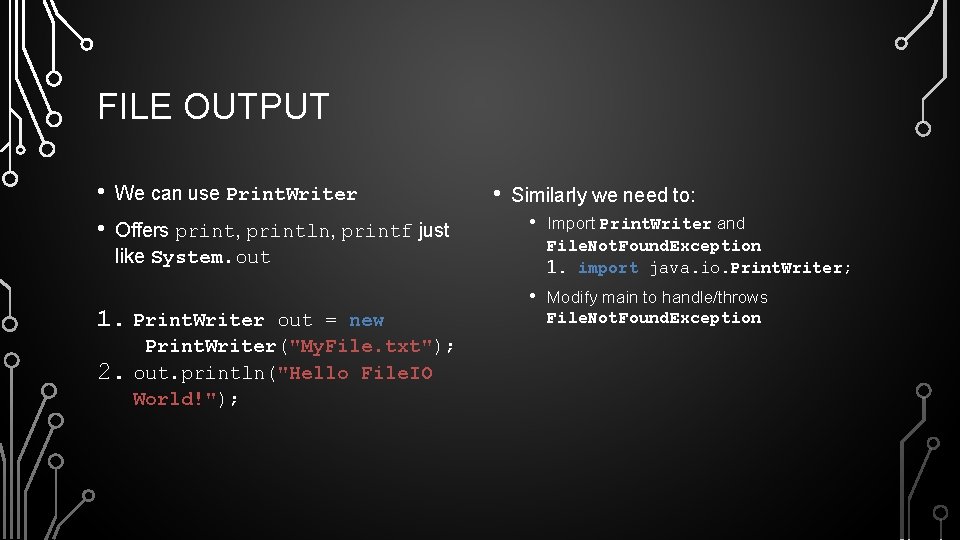
FILE OUTPUT • • We can use Print. Writer Offers print, println, printf just like System. out 1. Print. Writer out = new Print. Writer("My. File. txt"); 2. out. println("Hello File. IO World!"); • Similarly we need to: • Import Print. Writer and File. Not. Found. Exception 1. import java. io. Print. Writer; • Modify main to handle/throws File. Not. Found. Exception
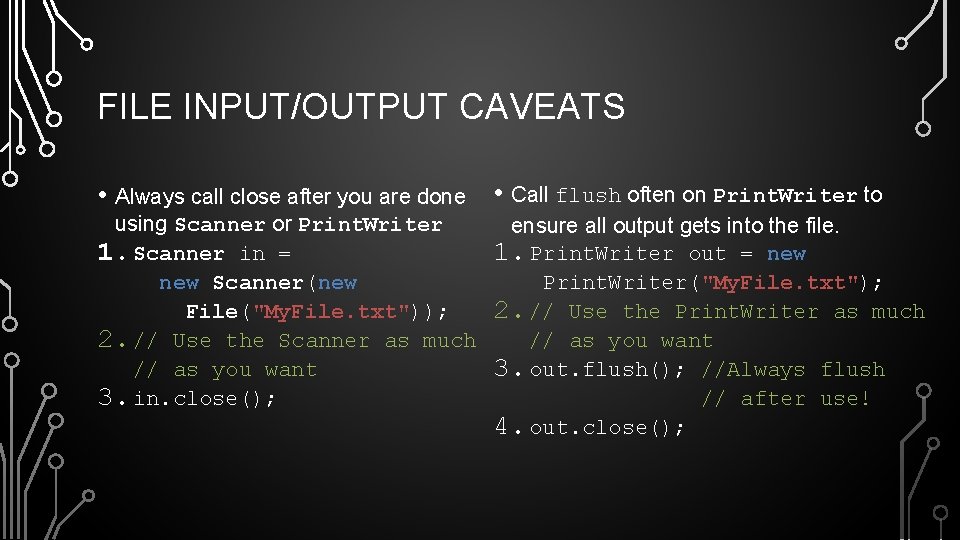
FILE INPUT/OUTPUT CAVEATS • Always call close after you are done • Call flush often on Print. Writer to using Scanner or Print. Writer 1. Scanner in = new Scanner(new File("My. File. txt")); 2. // Use the Scanner as much // as you want 3. in. close(); ensure all output gets into the file. 1. Print. Writer out = new Print. Writer("My. File. txt"); 2. // Use the Print. Writer as much // as you want 3. out. flush(); //Always flush // after use! 4. out. close();
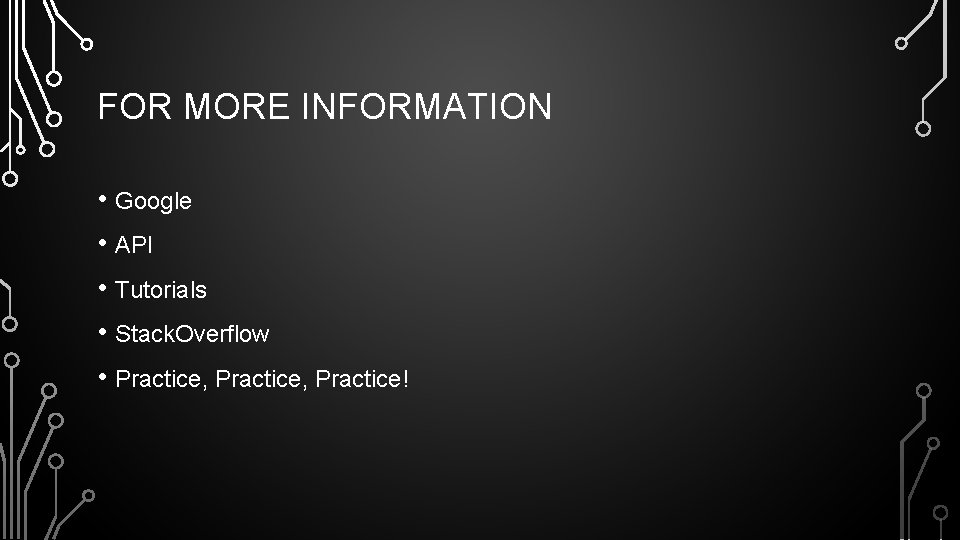
FOR MORE INFORMATION • Google • API • Tutorials • Stack. Overflow • Practice, Practice!
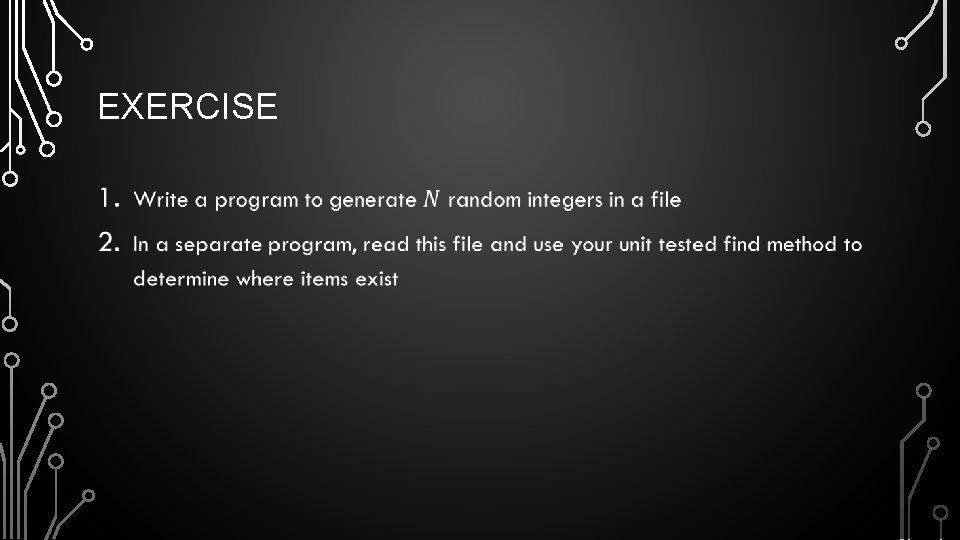
EXERCISE •