Inheritance Polymorphism and Virtual Functions What Is Inheritance
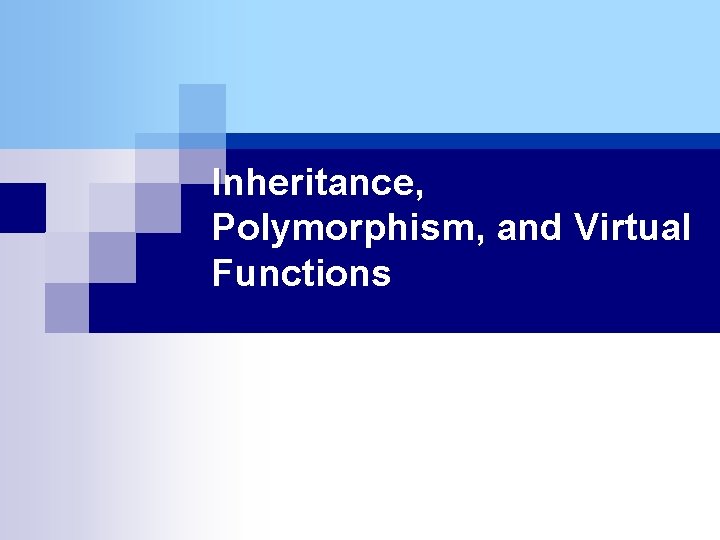
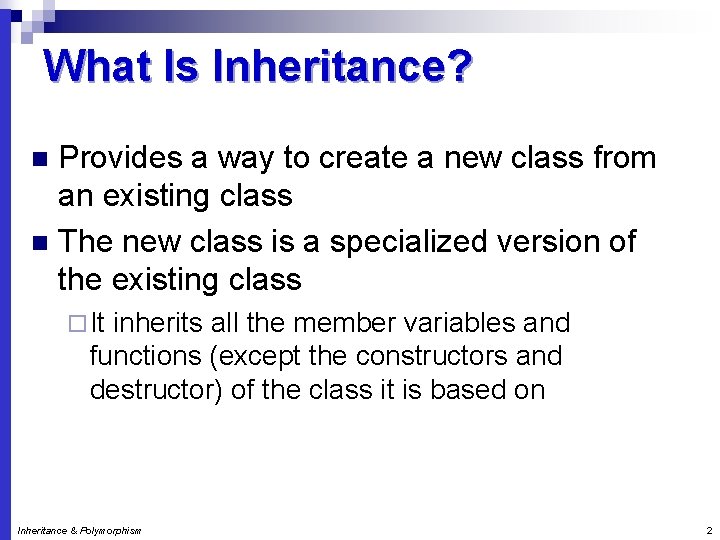
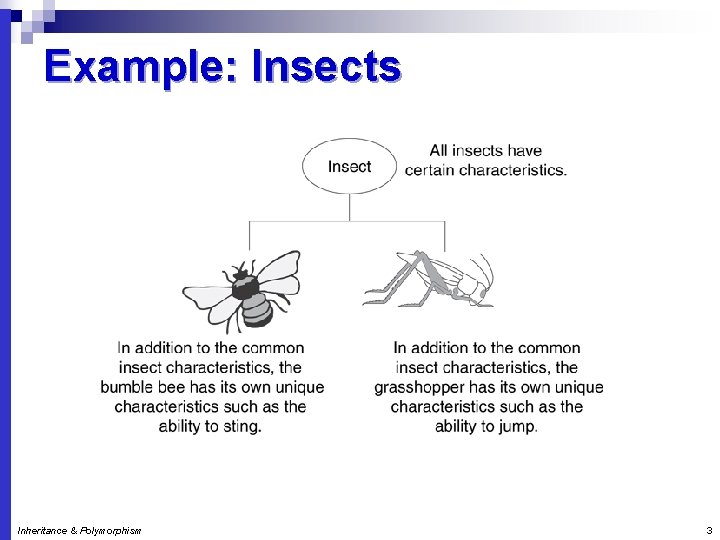
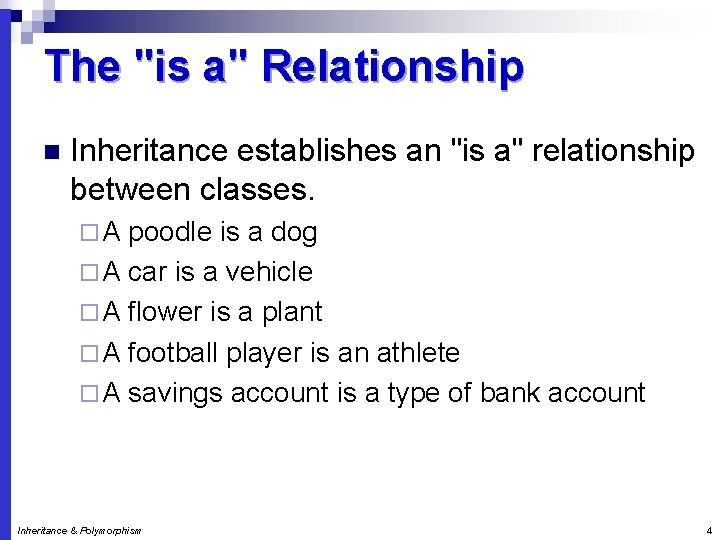
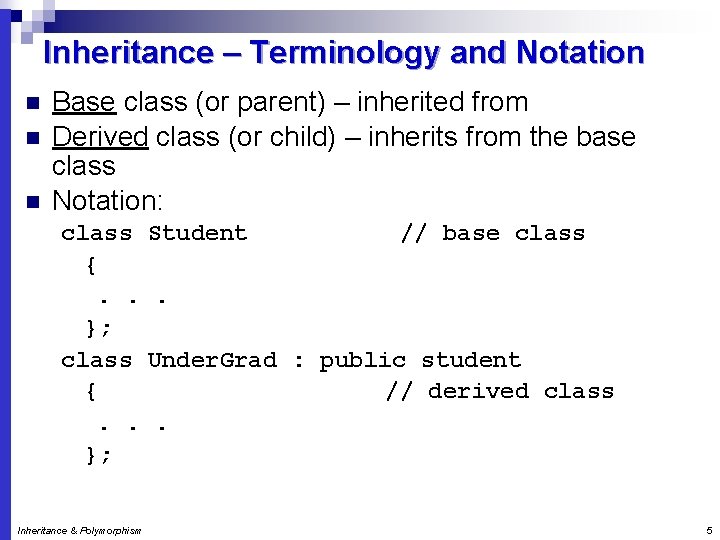
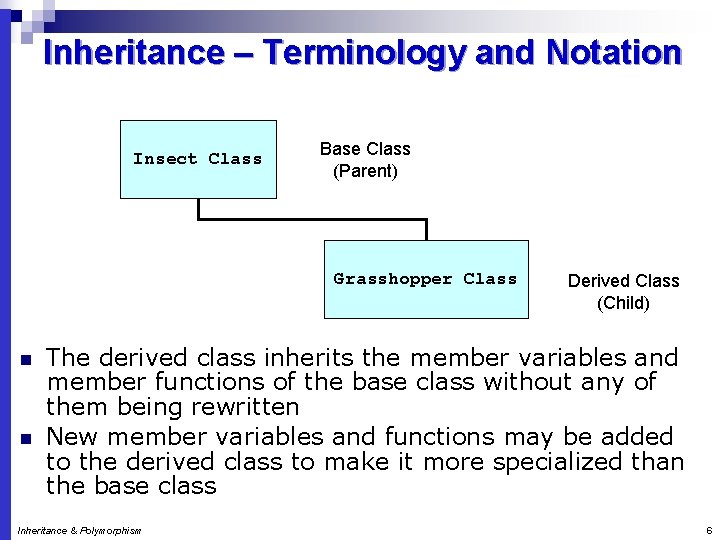
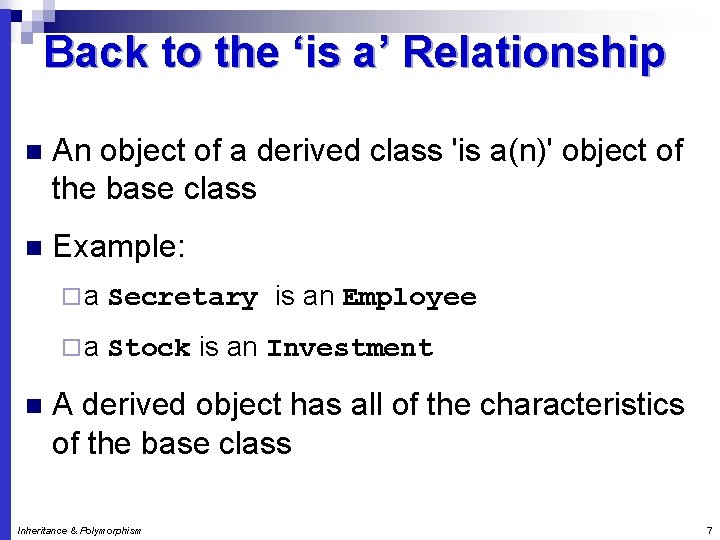
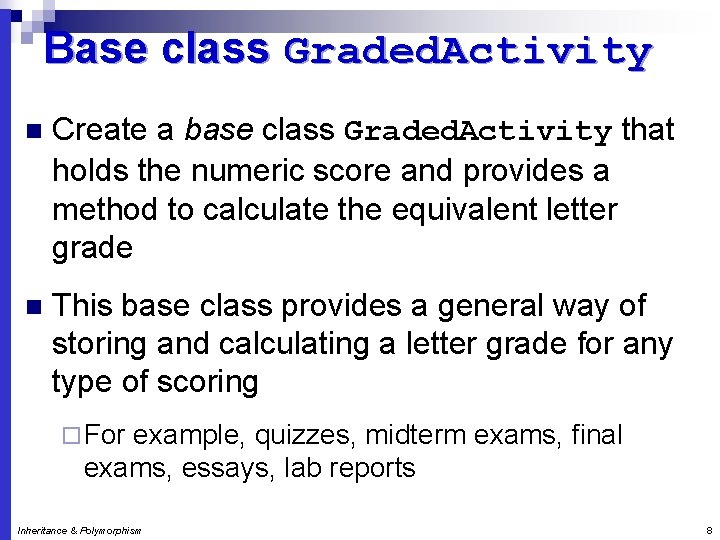
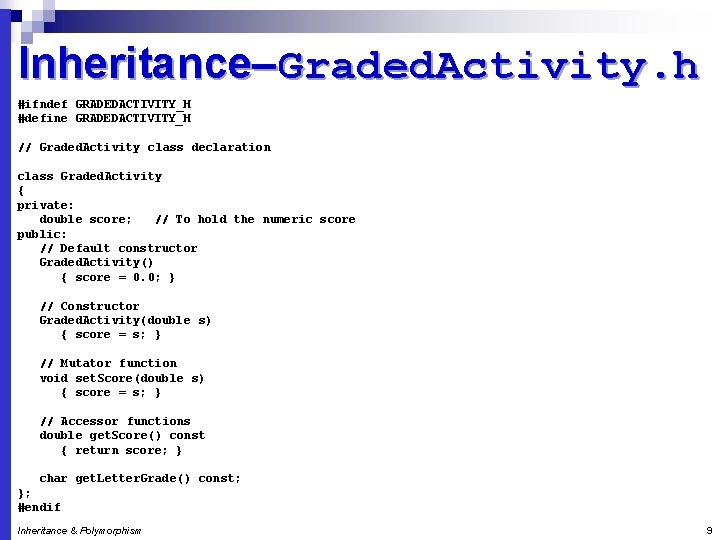
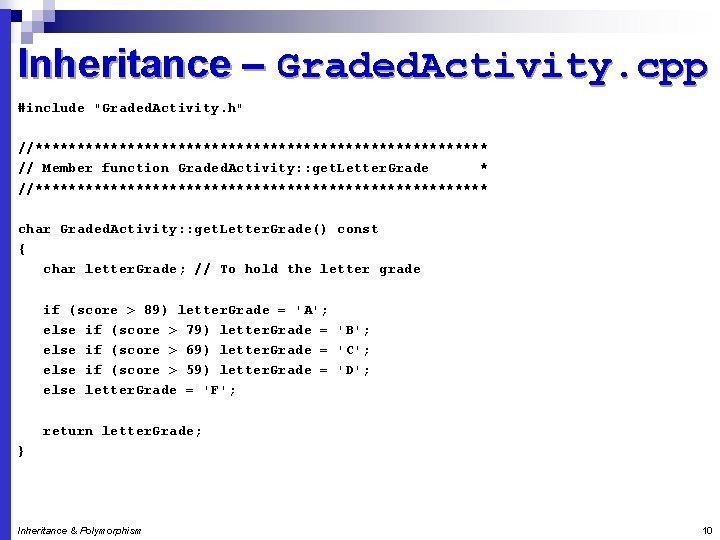
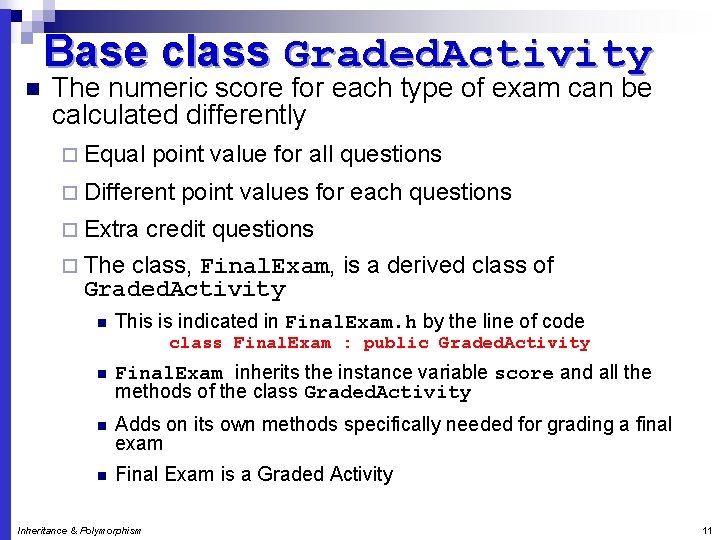
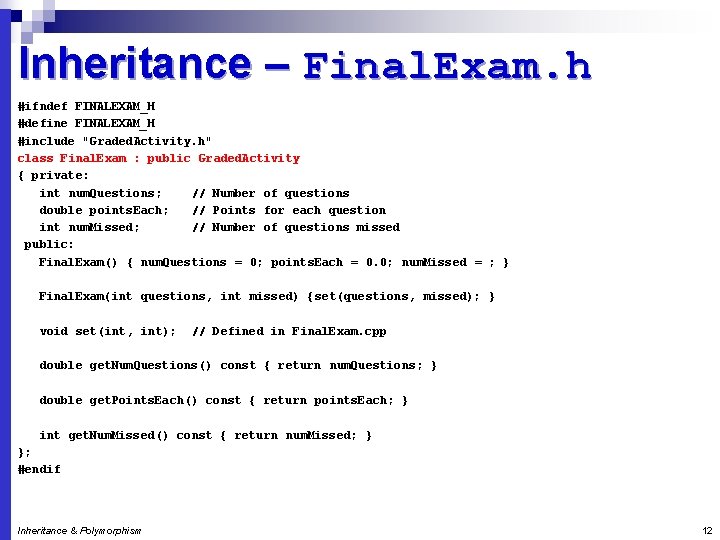
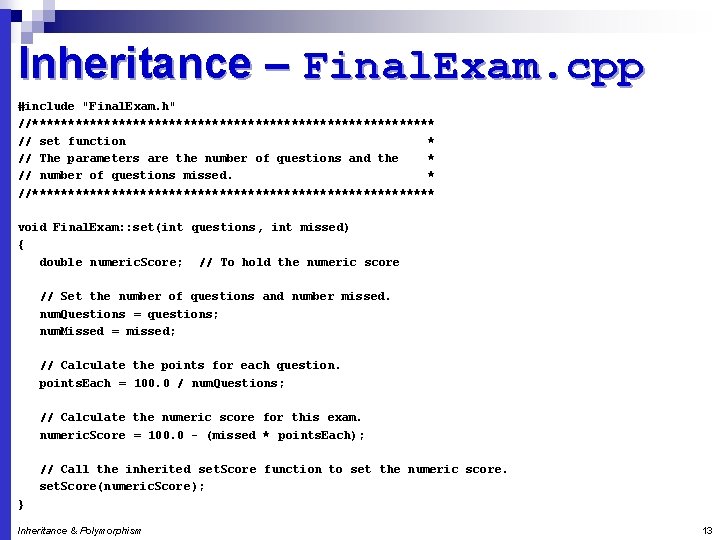
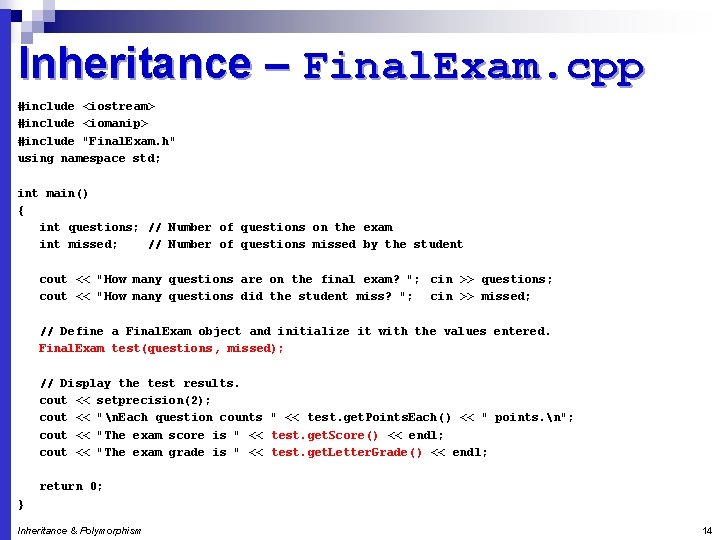
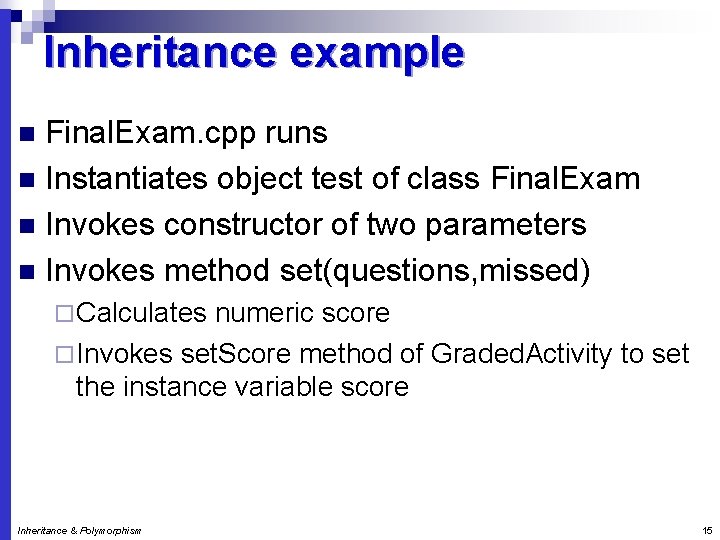
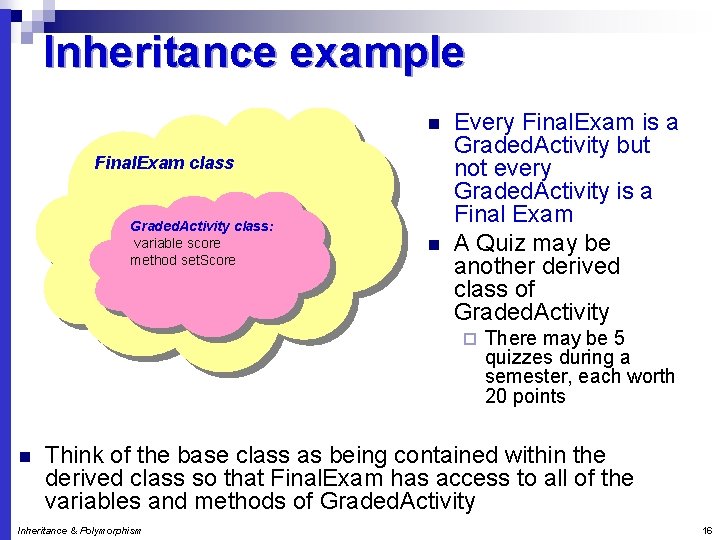
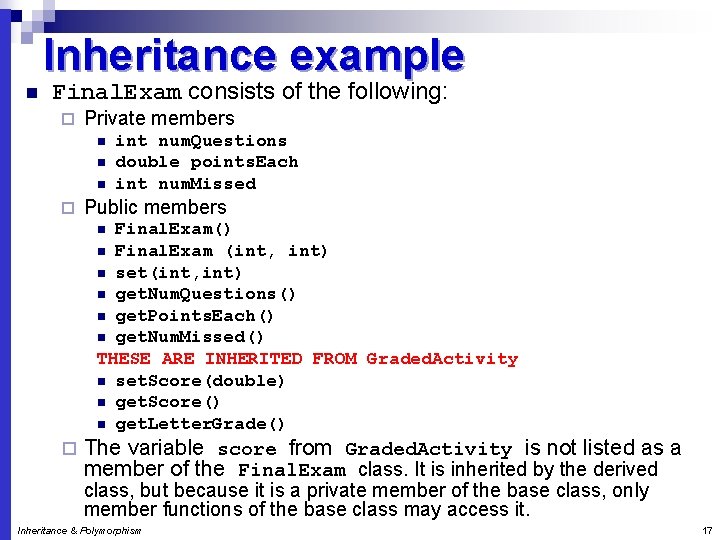
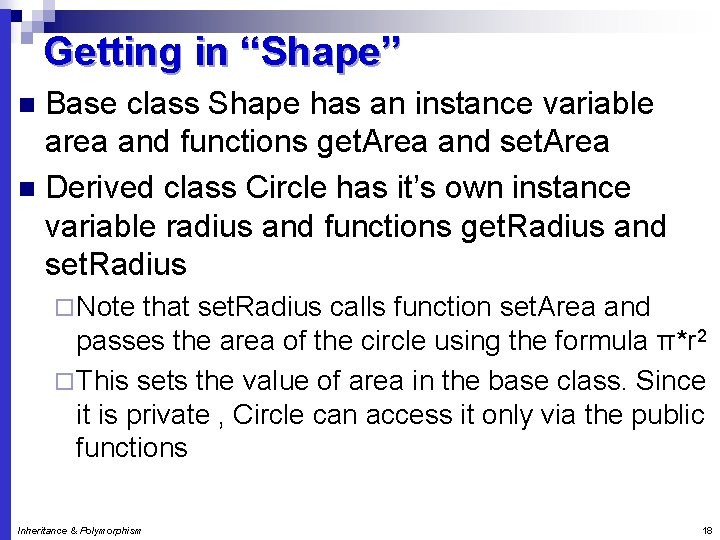
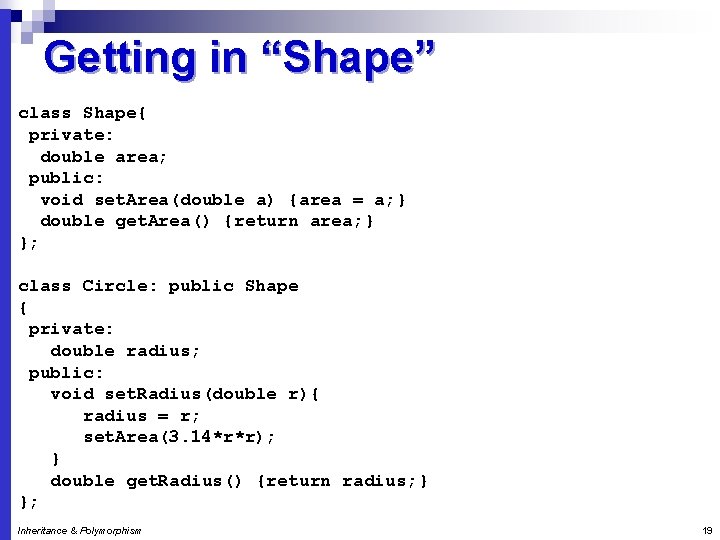
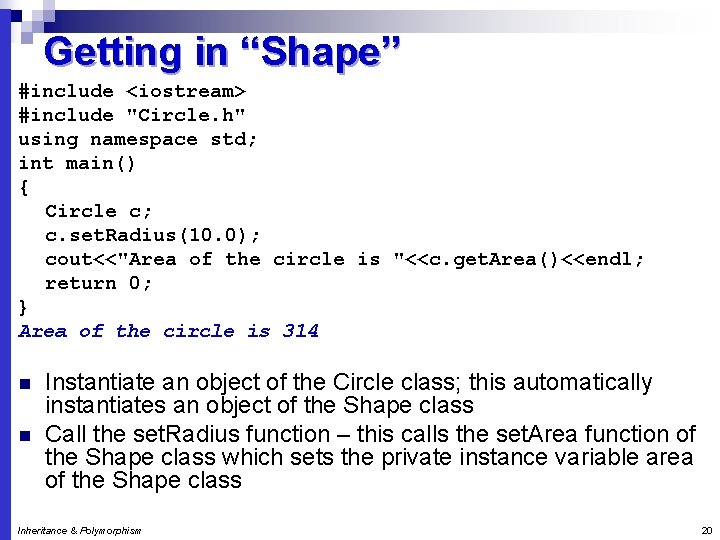
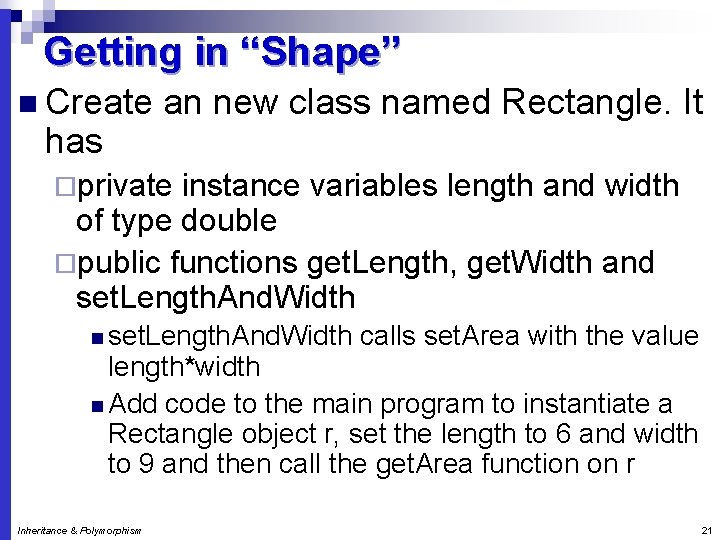
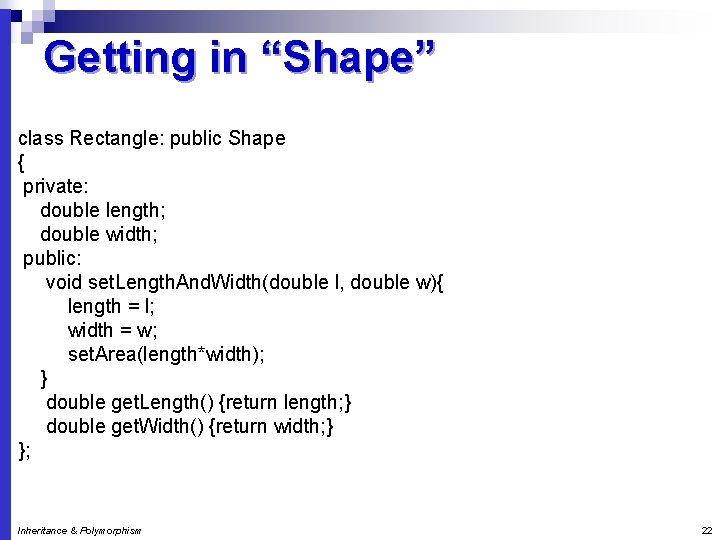
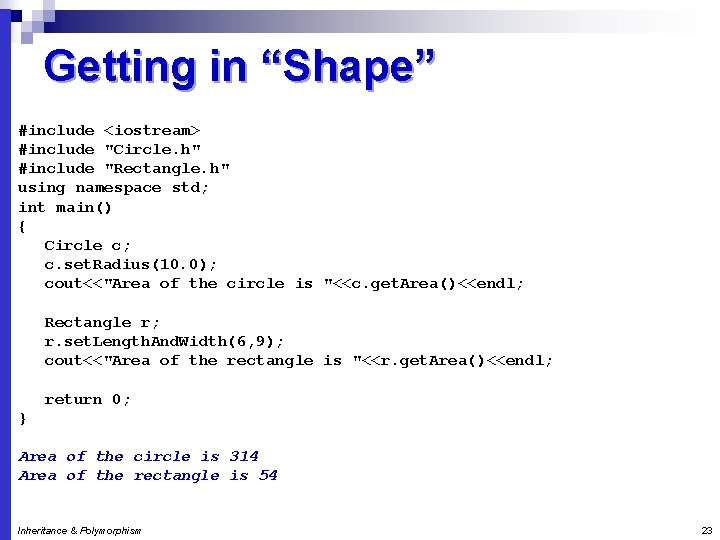
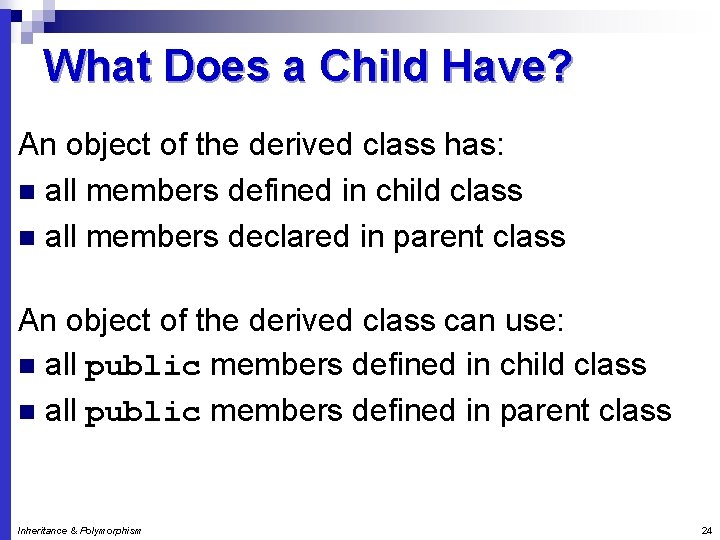
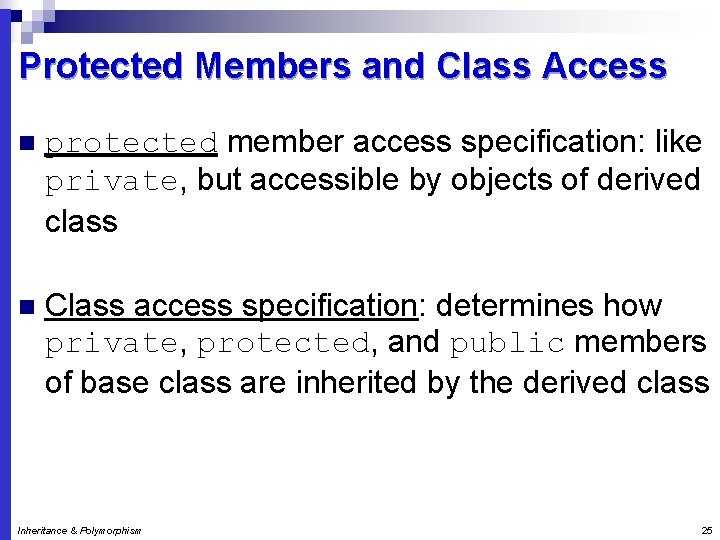
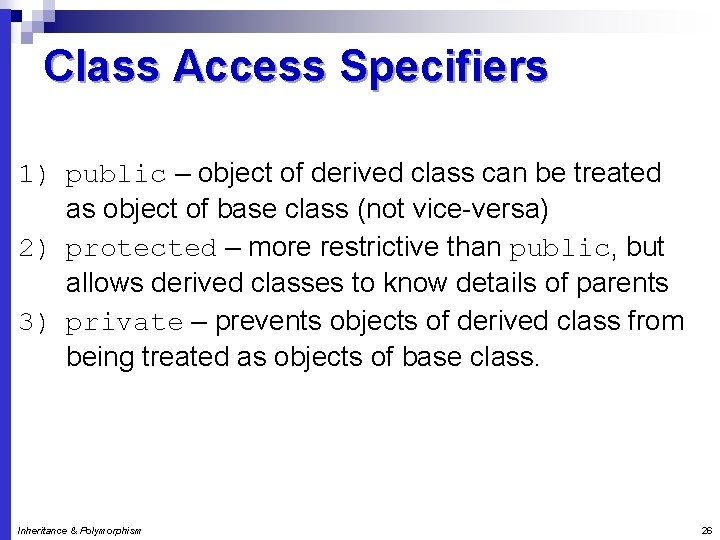
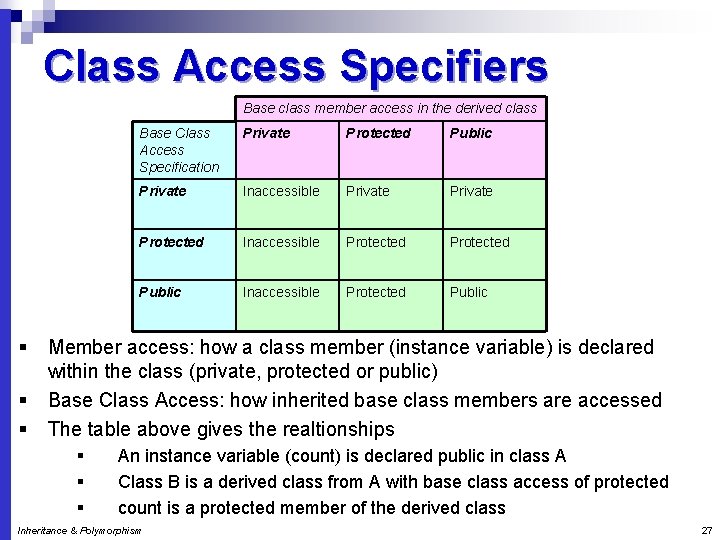
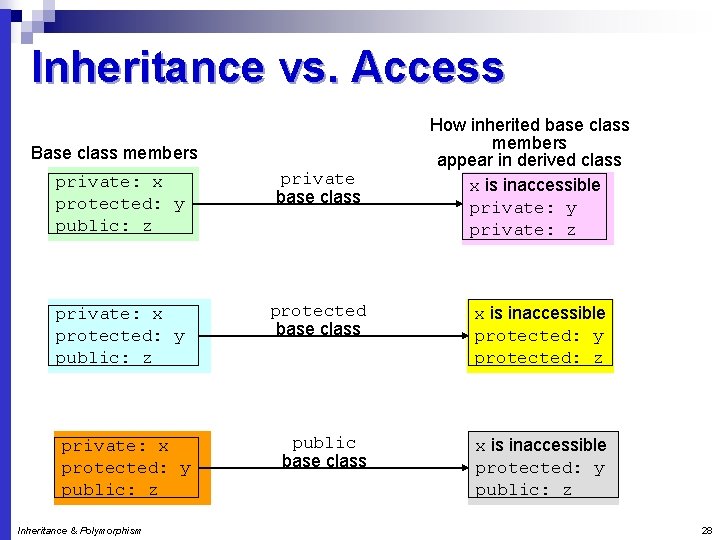
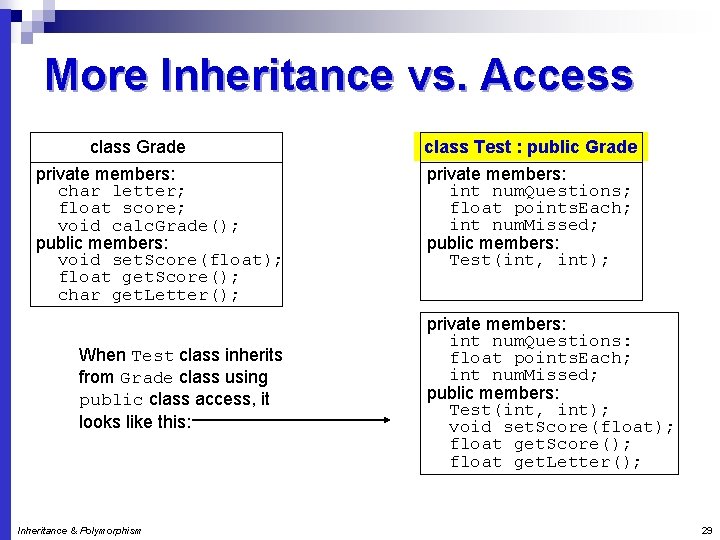
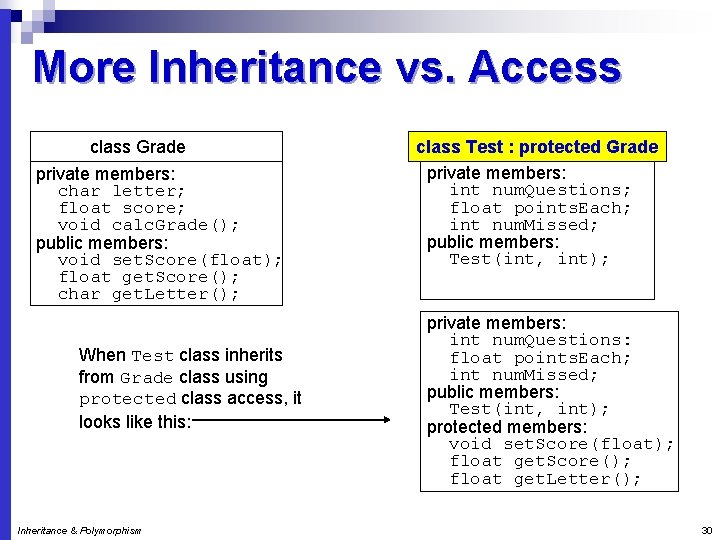
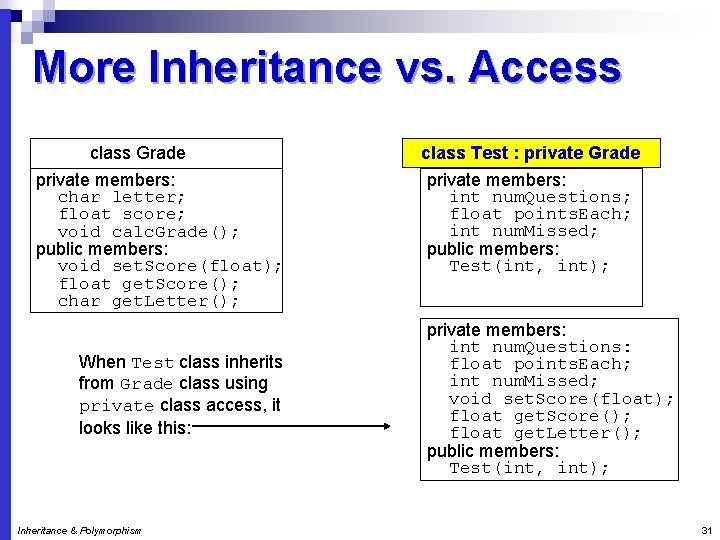
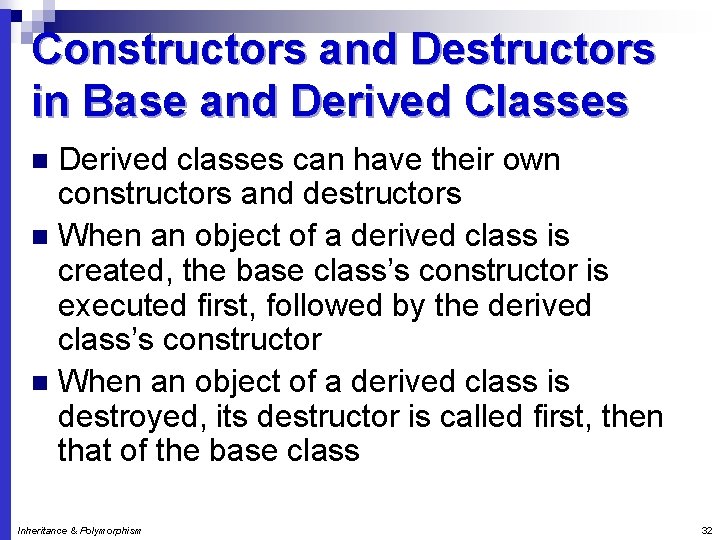
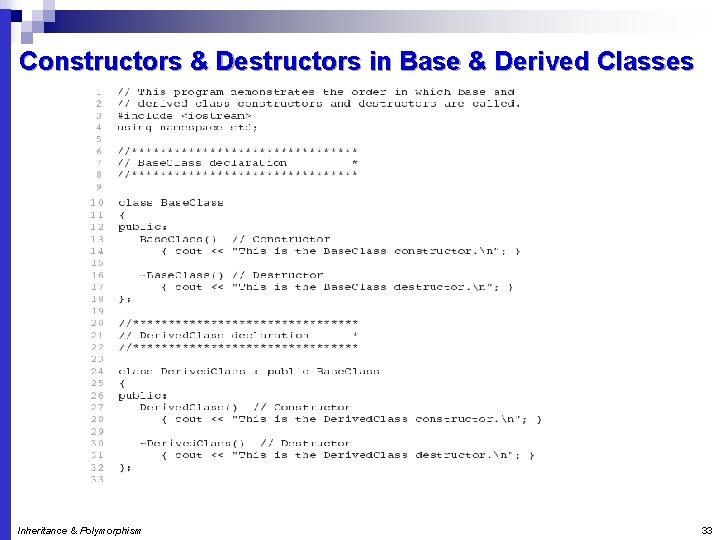
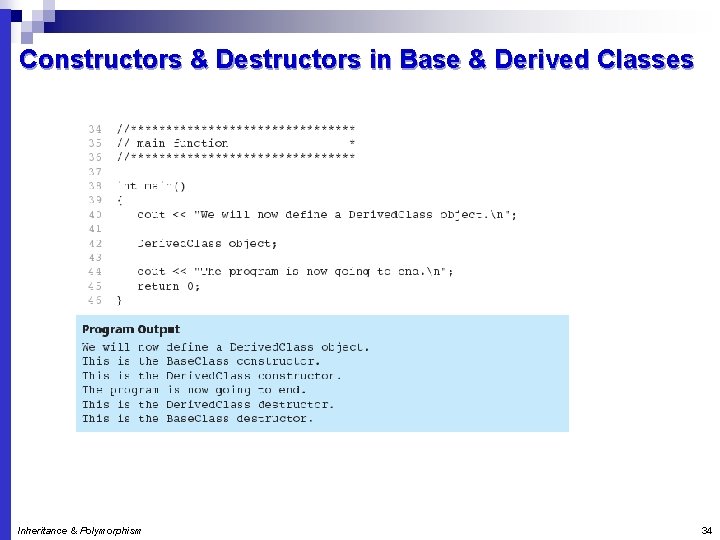
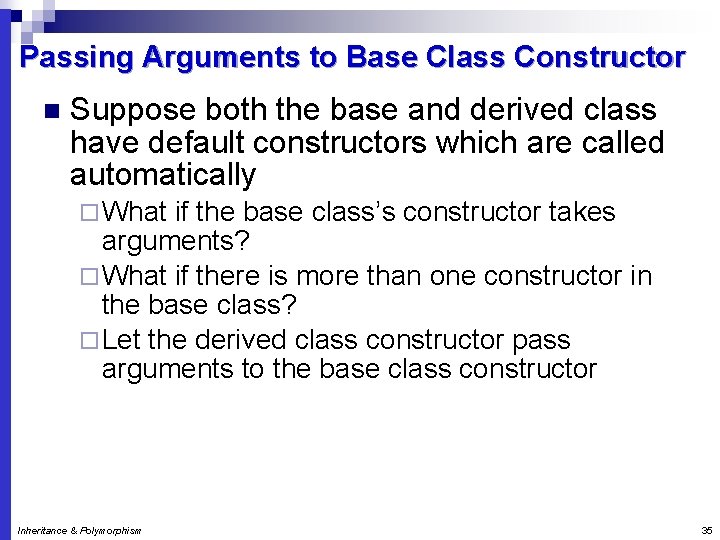
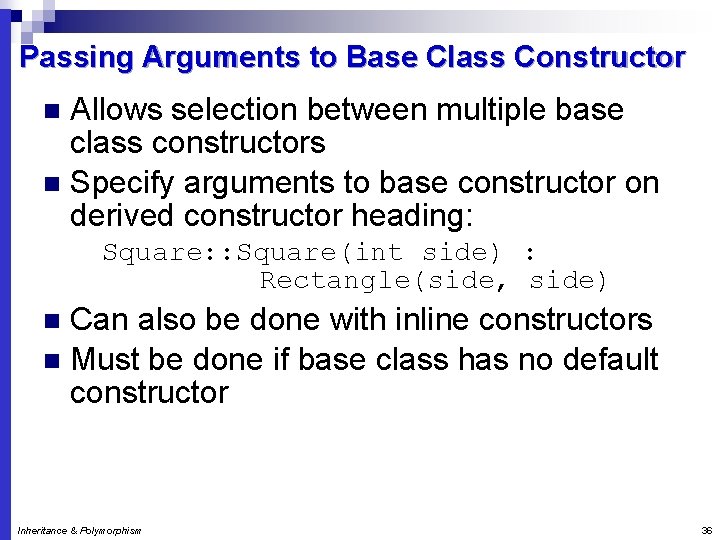
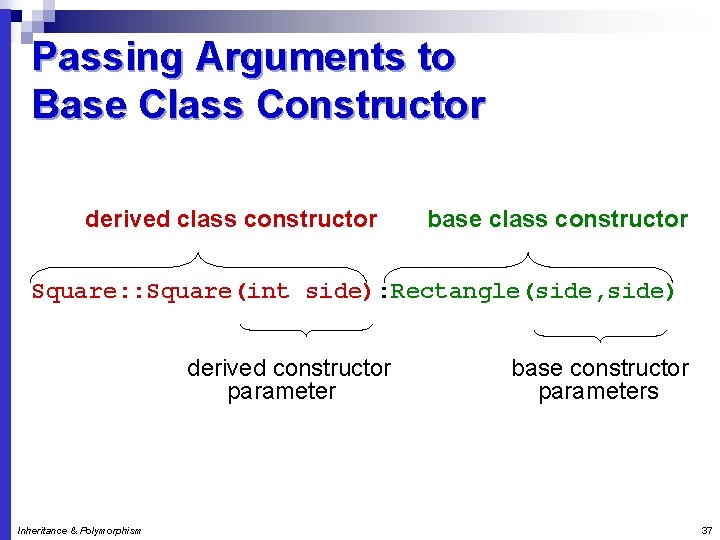
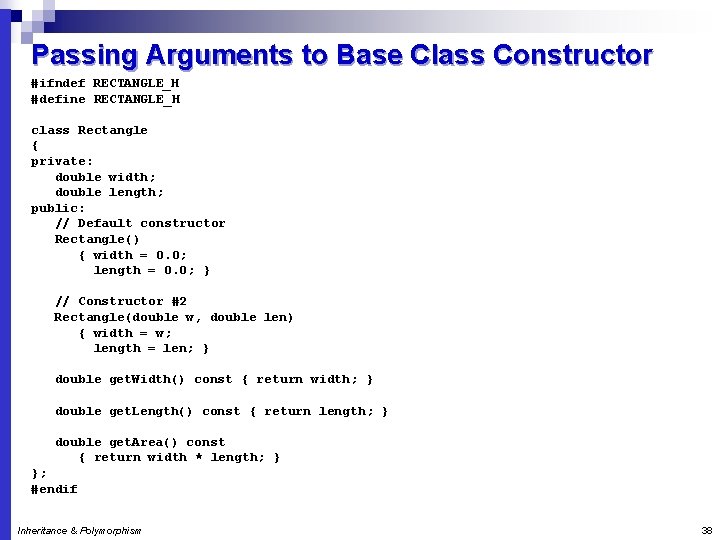
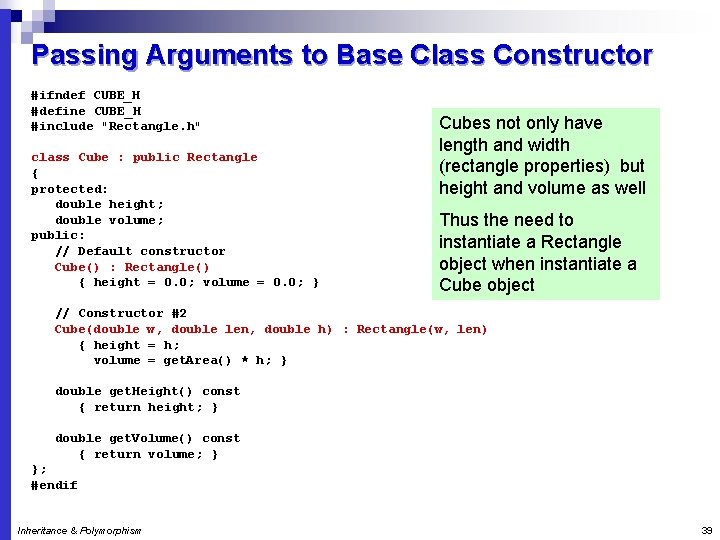
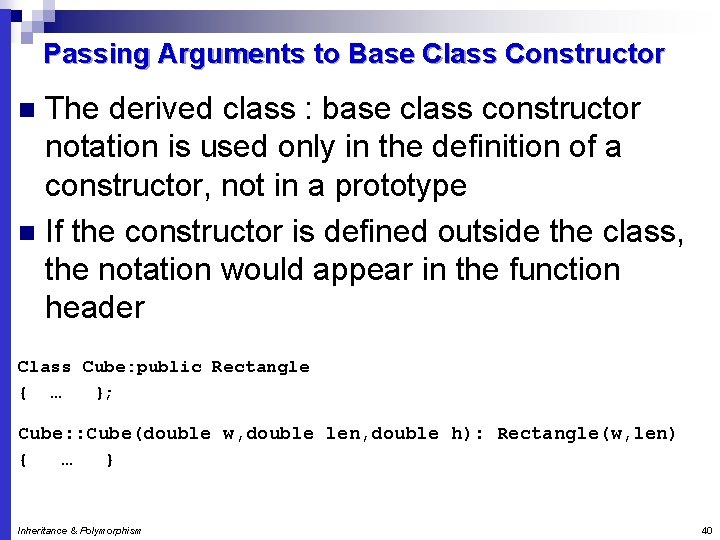
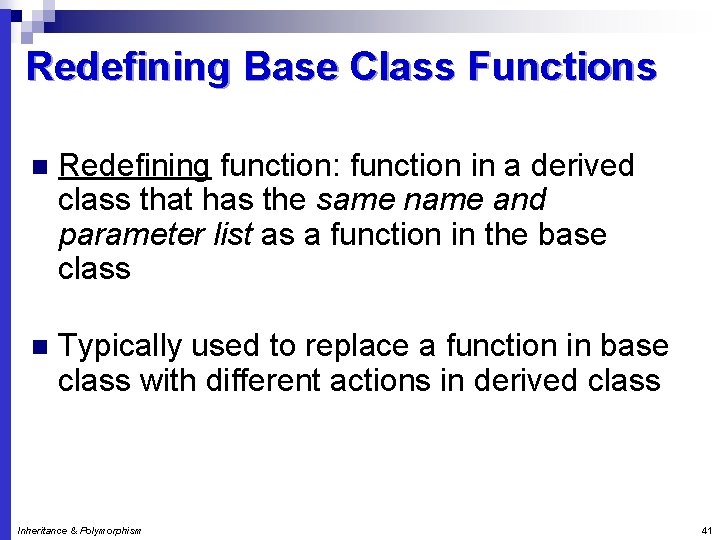
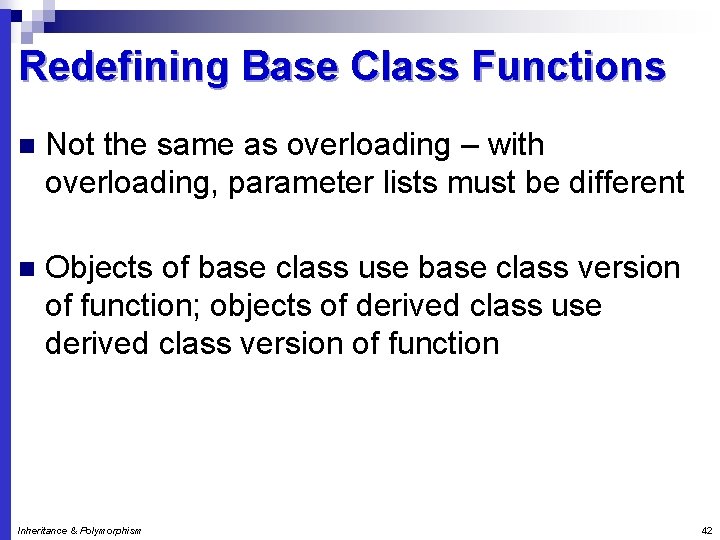
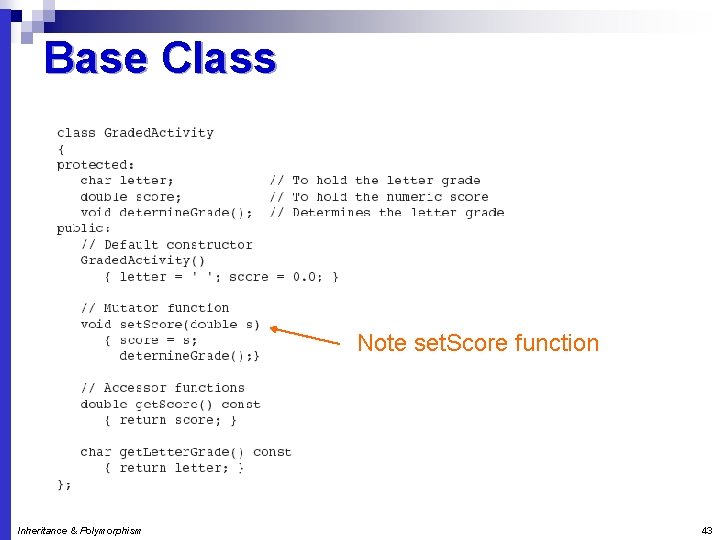
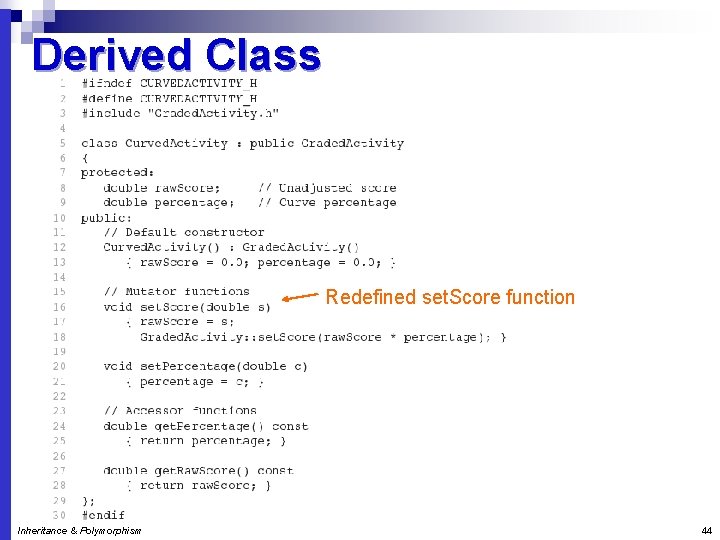
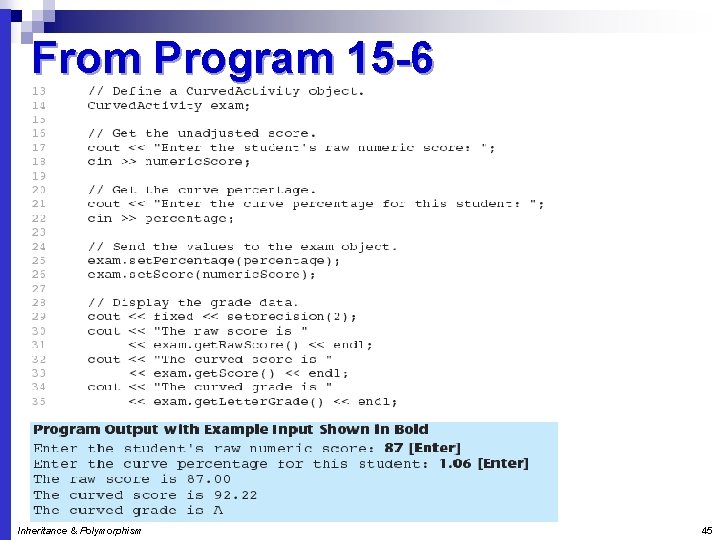
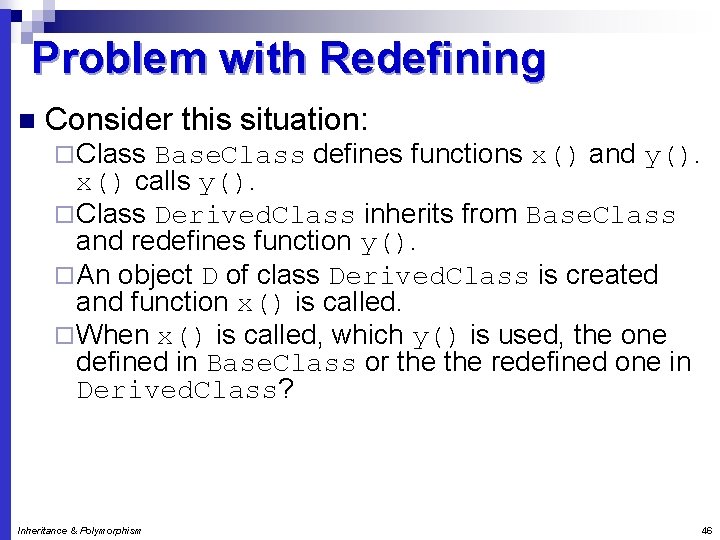
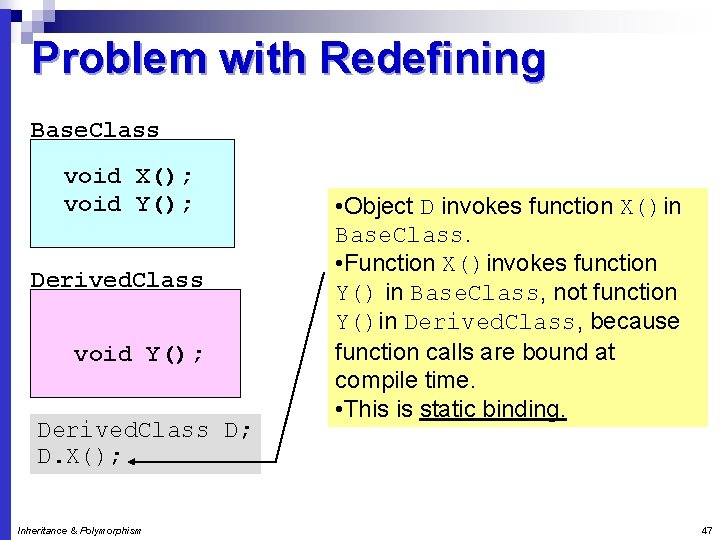
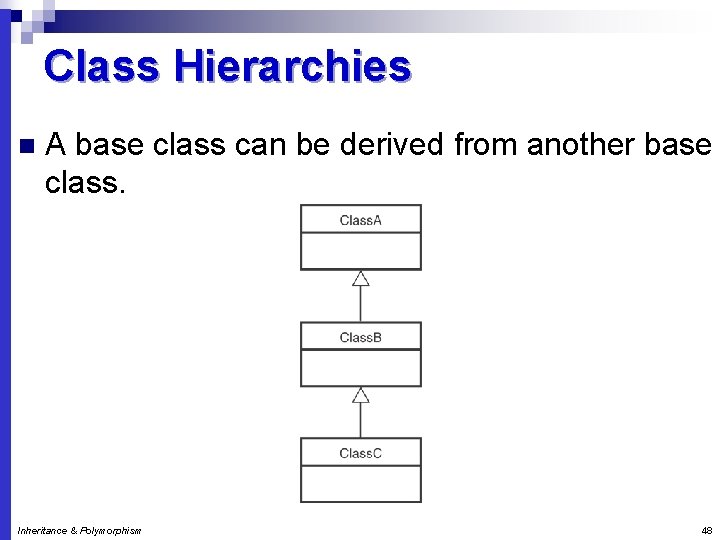
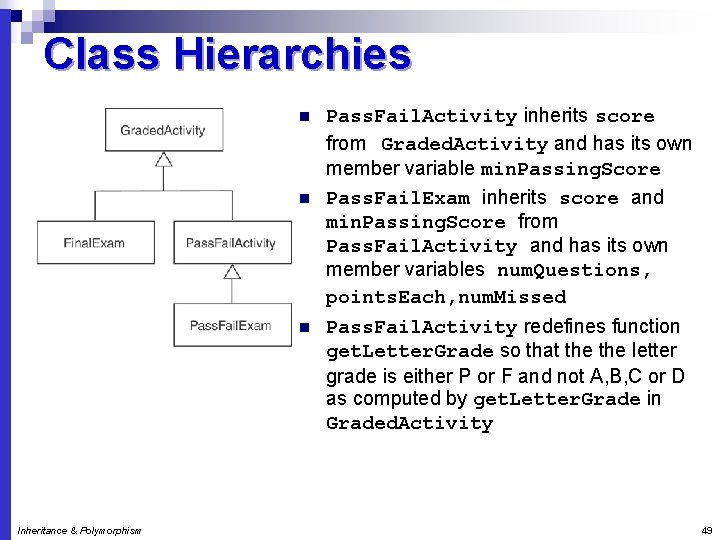
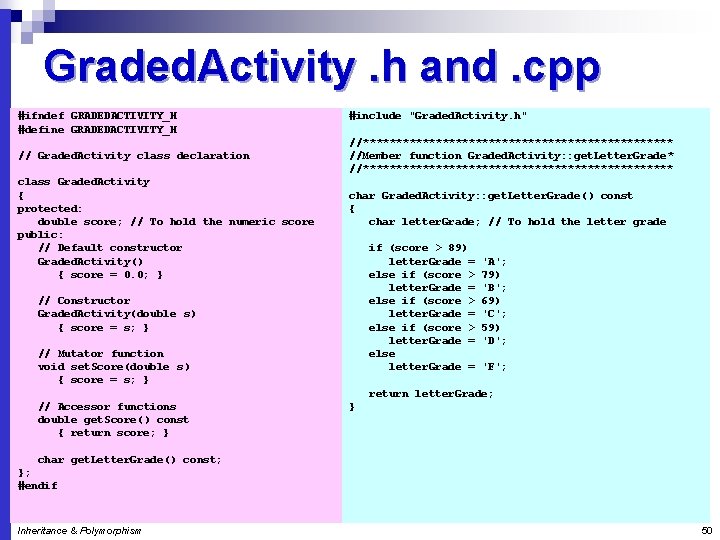
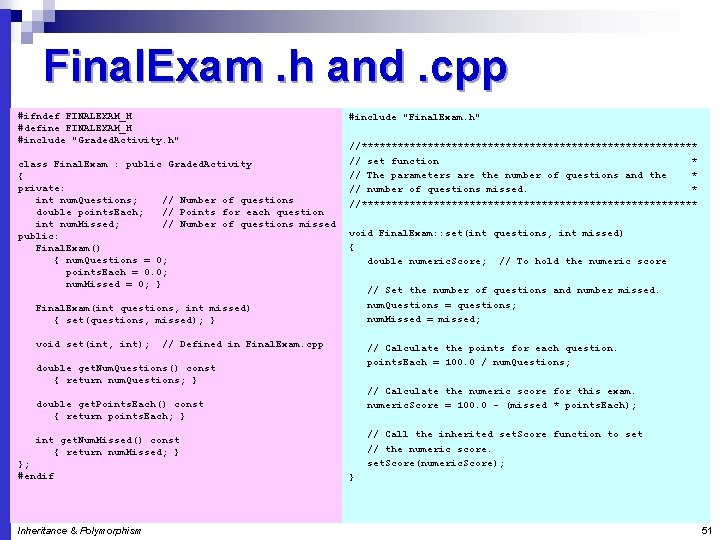
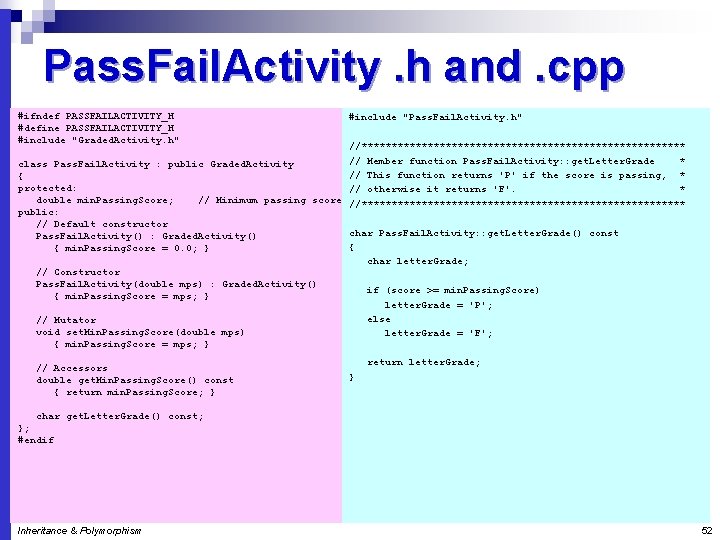
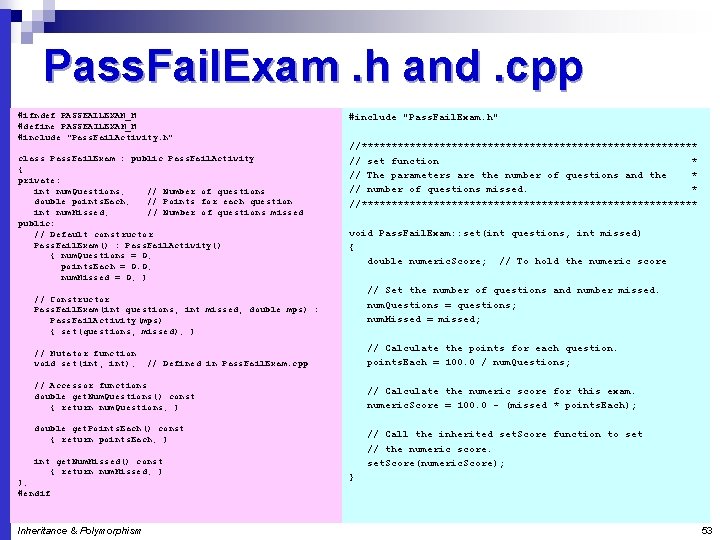
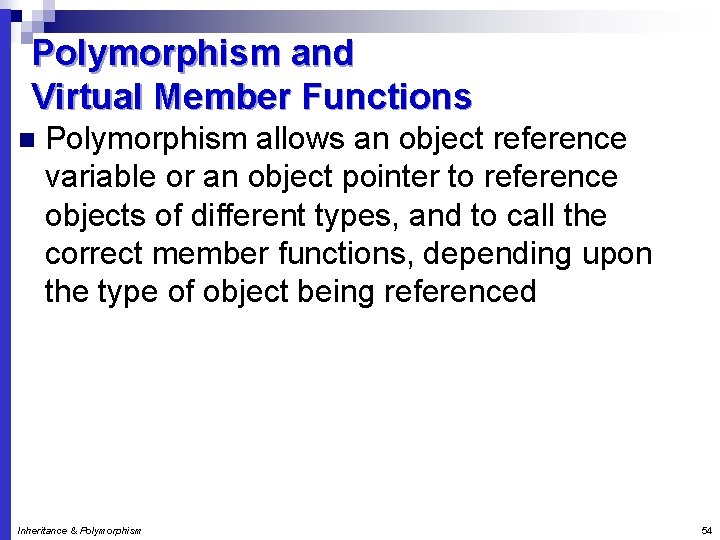
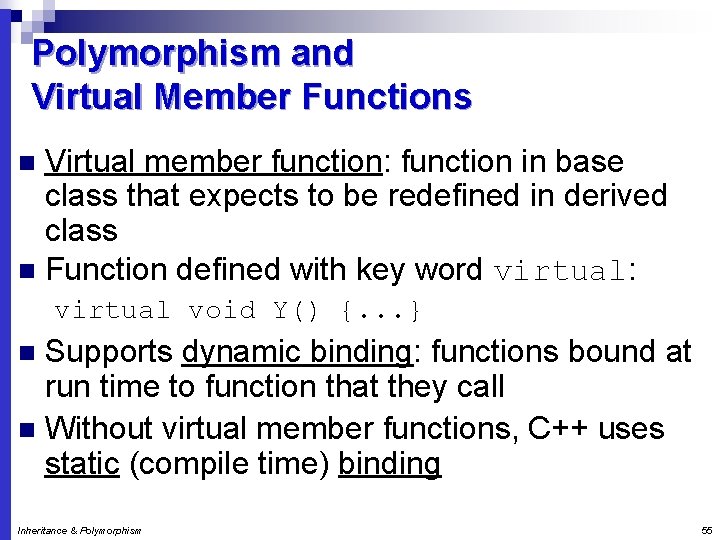
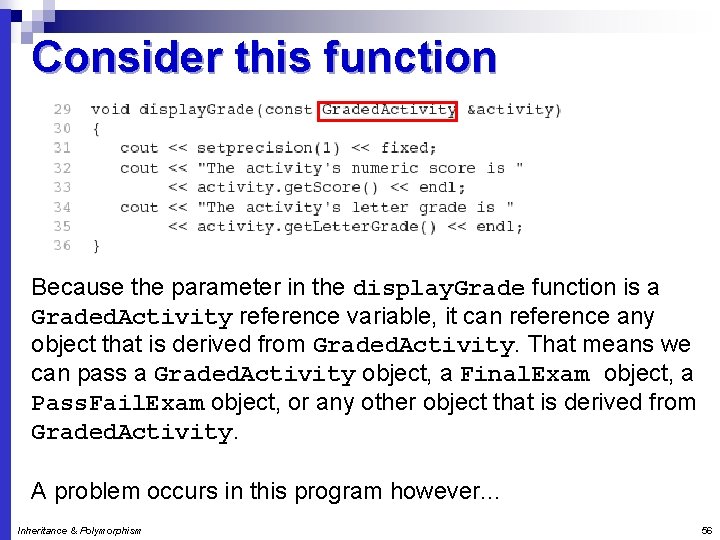
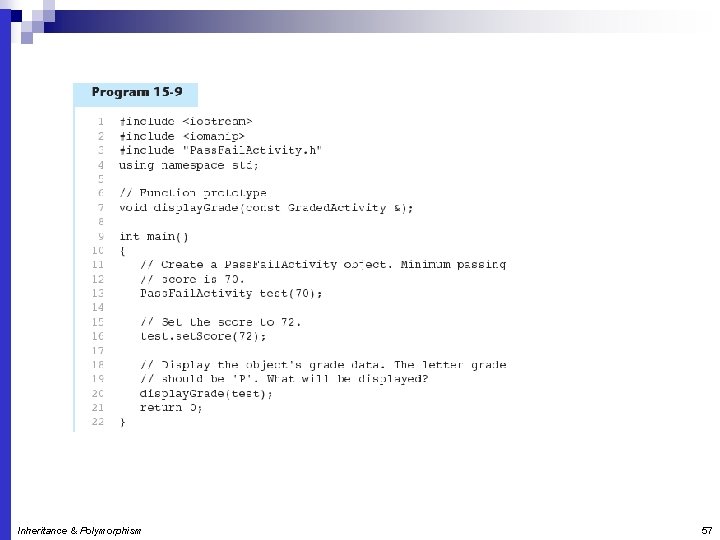
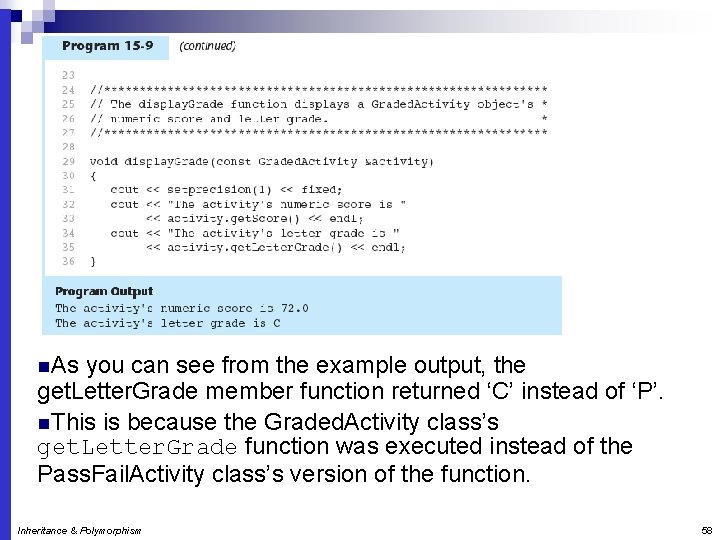
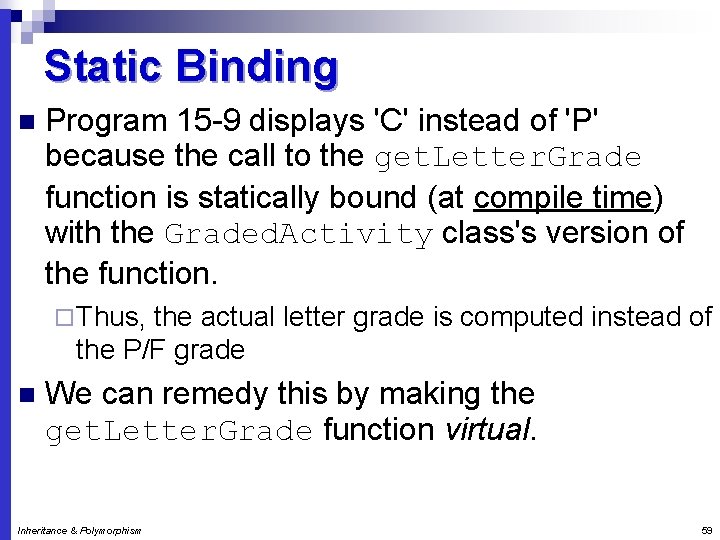
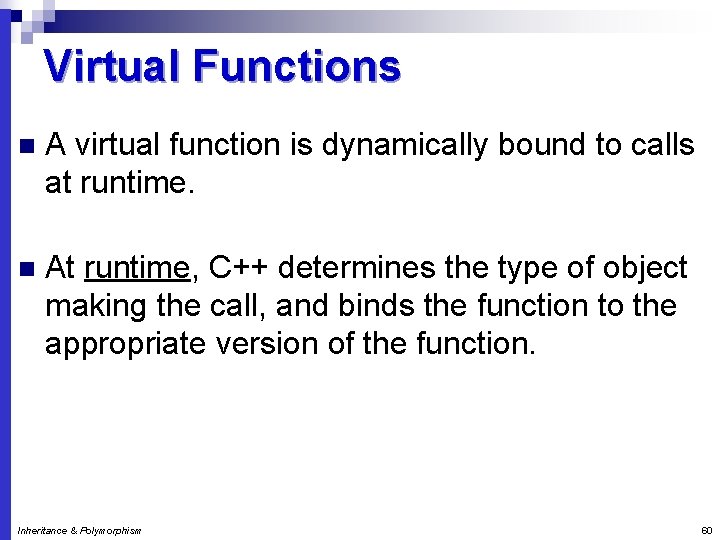
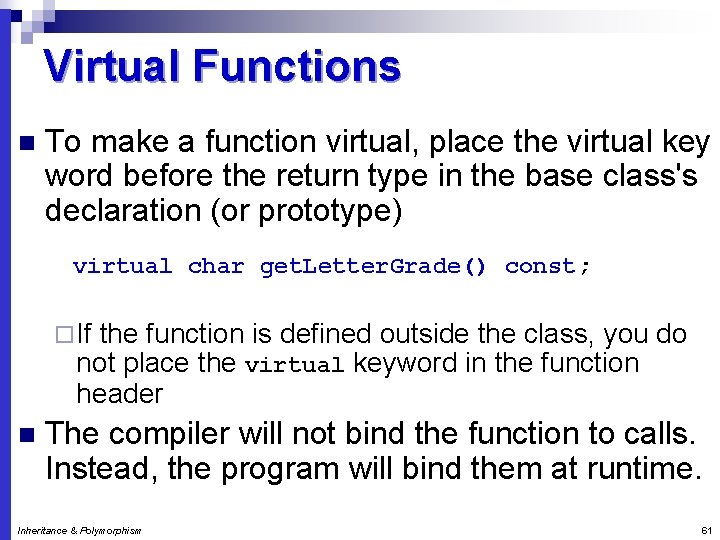
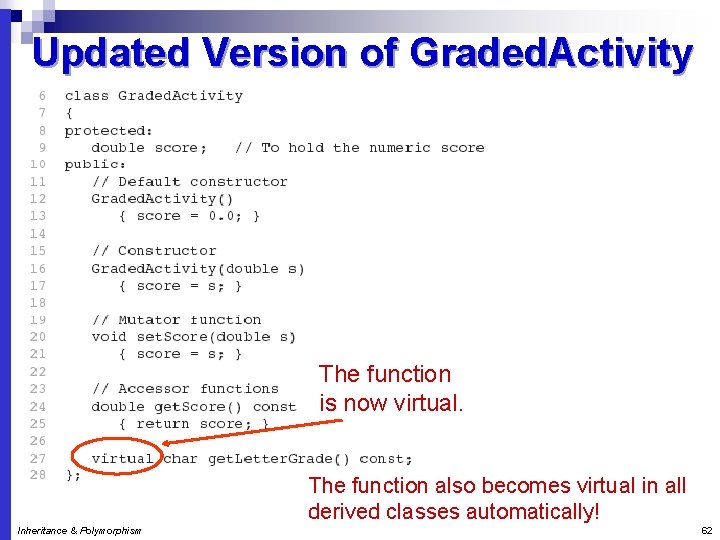
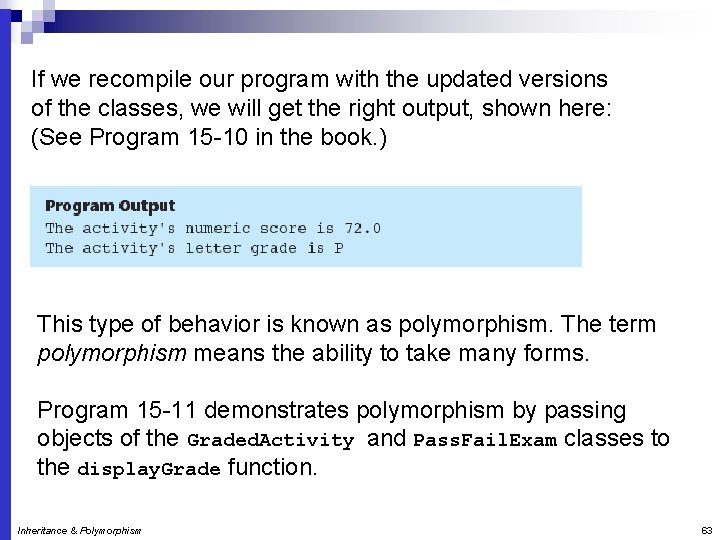
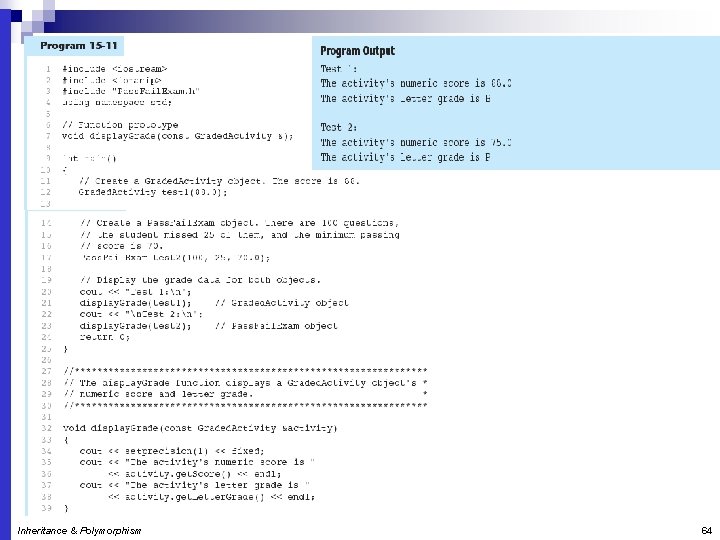
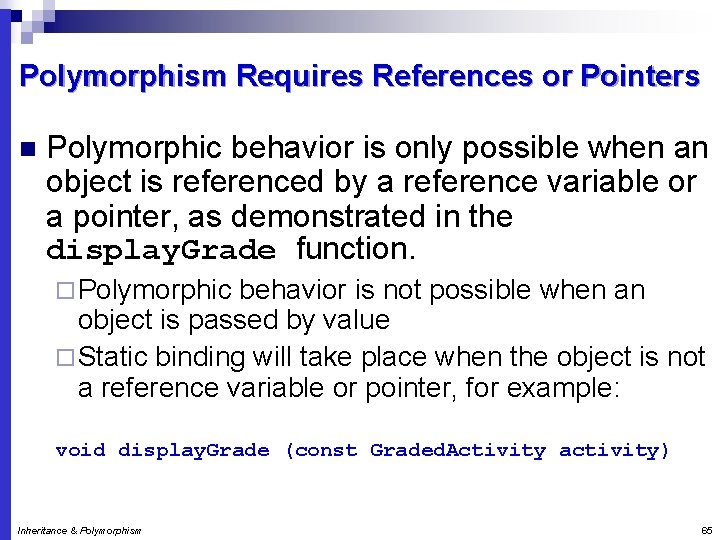
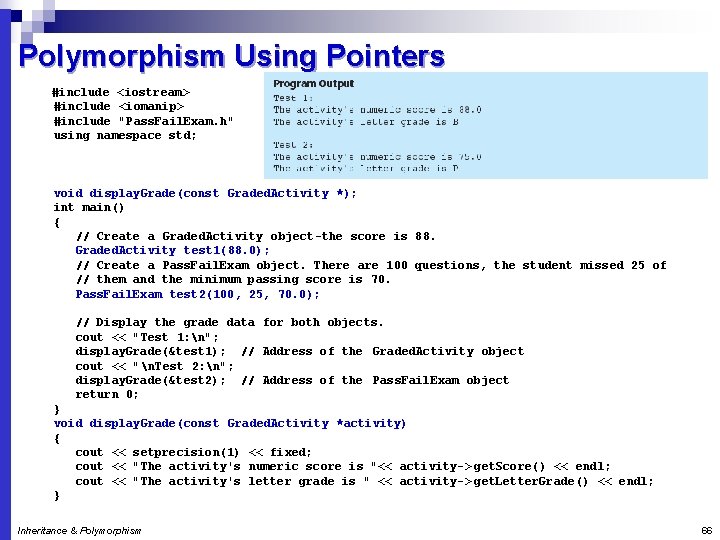
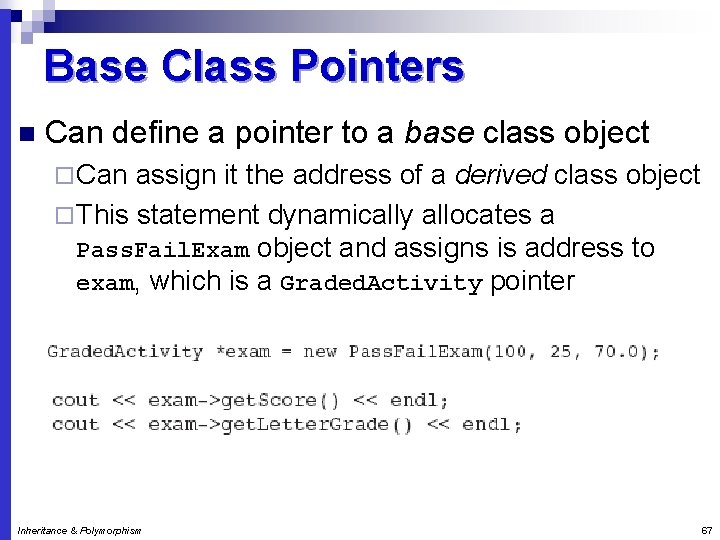
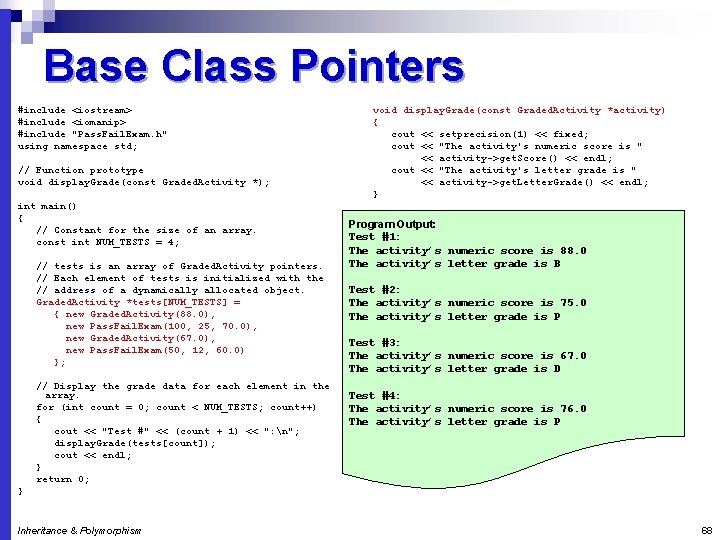
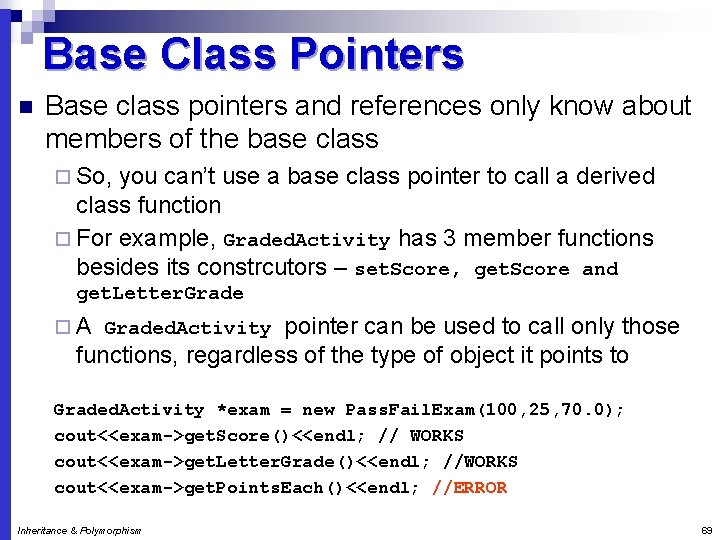
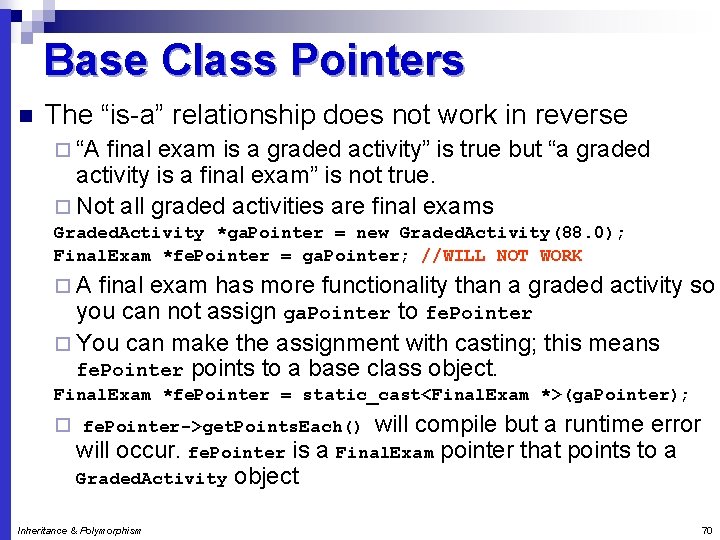
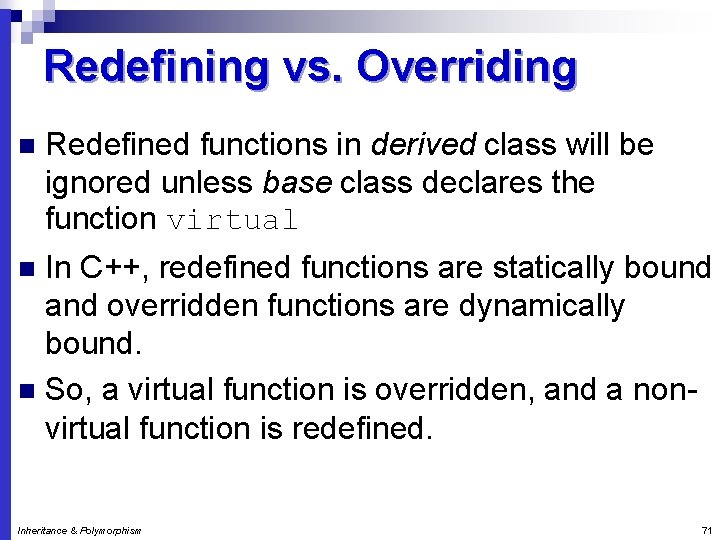
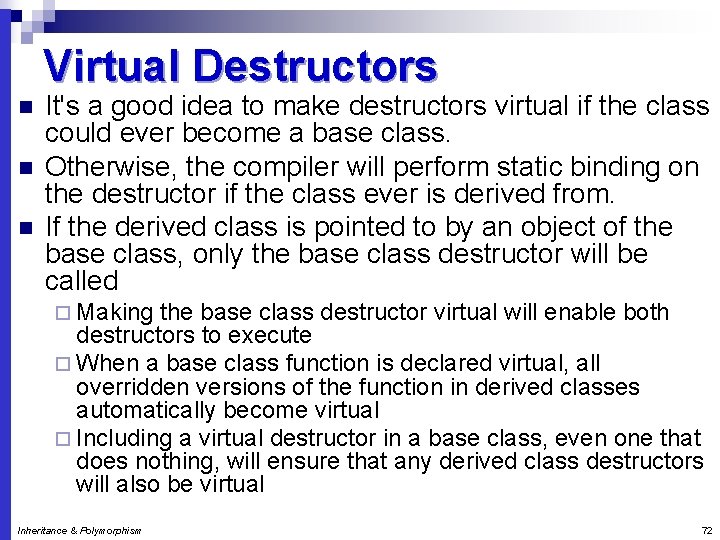
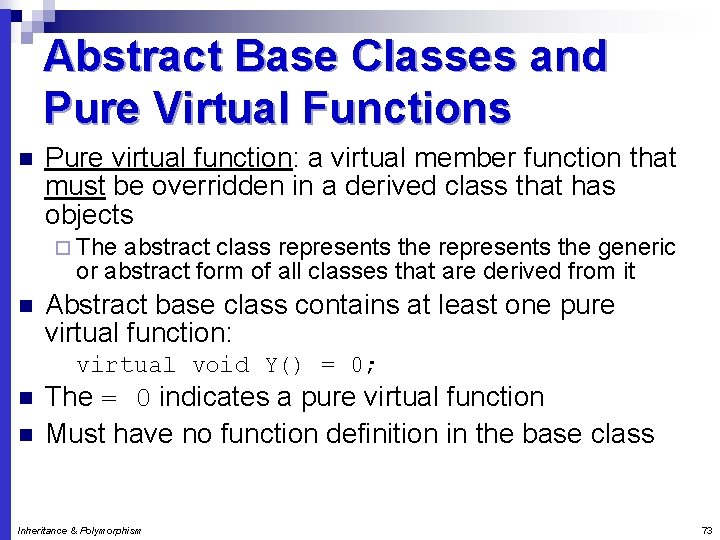
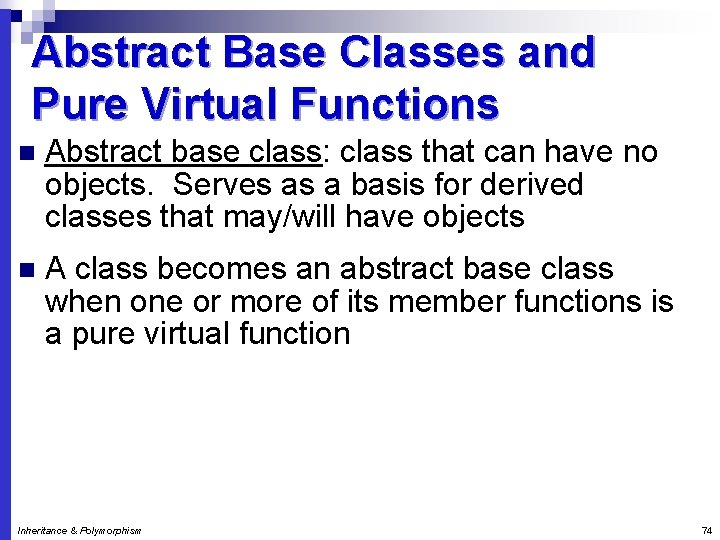
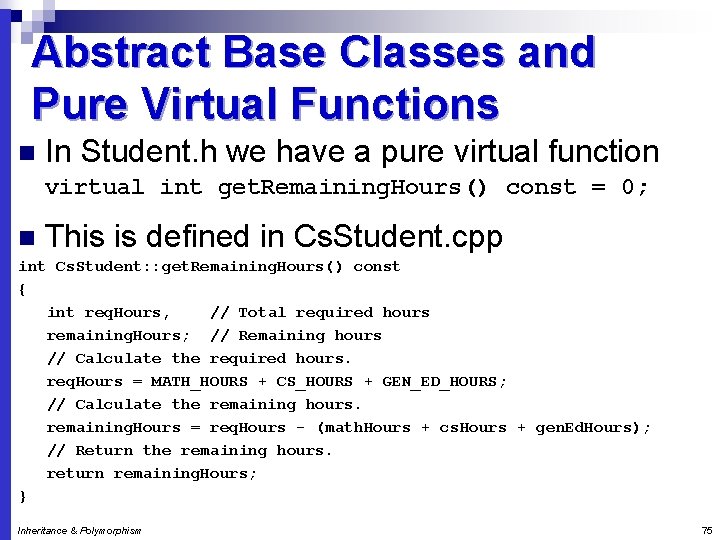
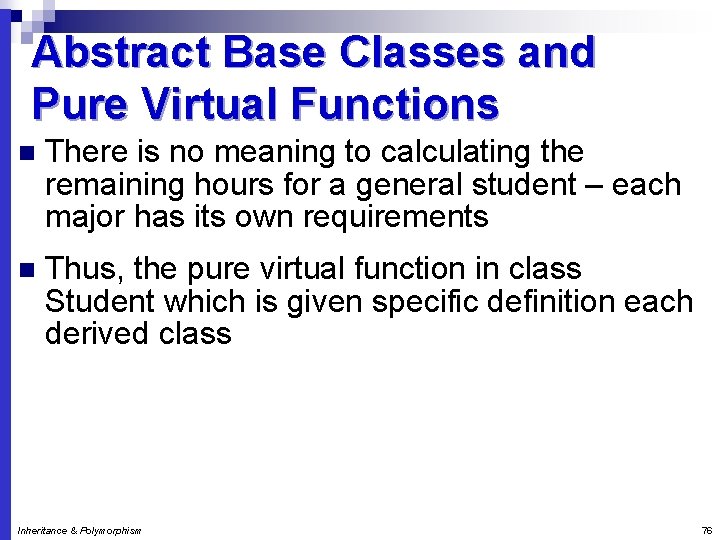
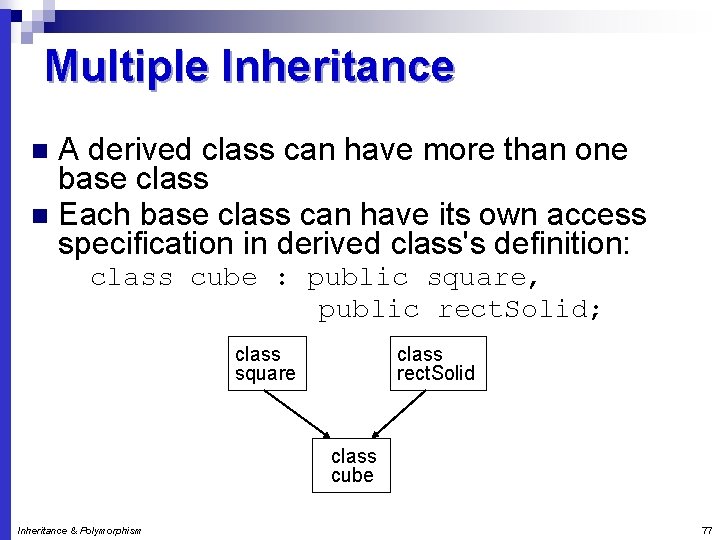
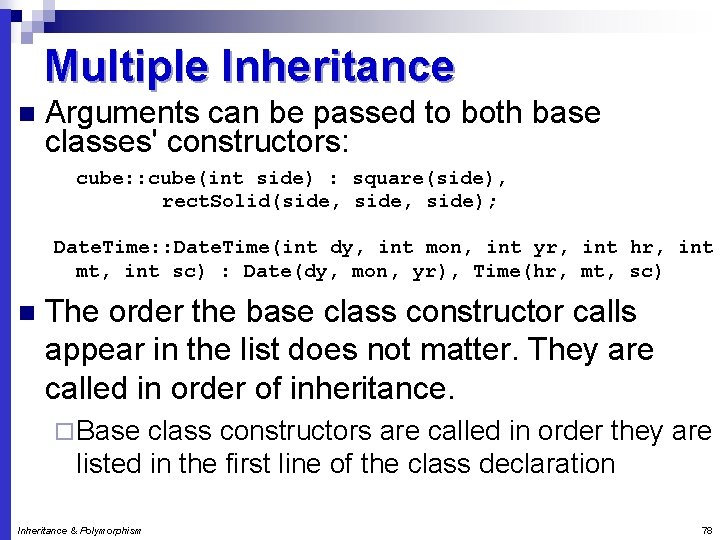
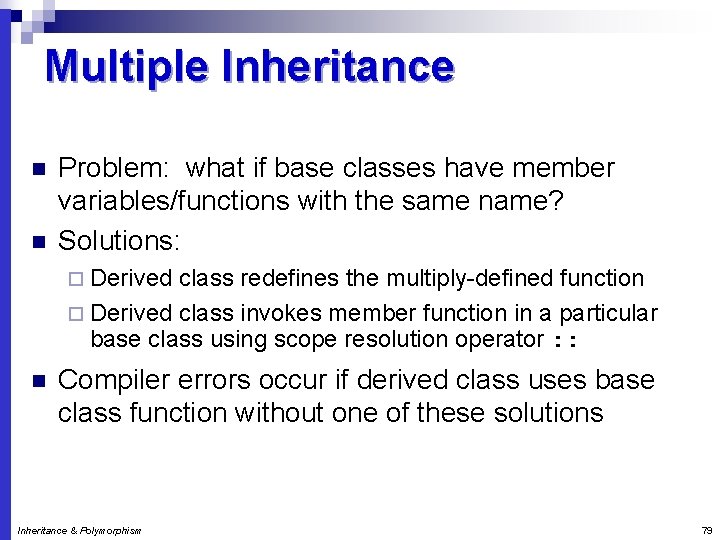
- Slides: 79
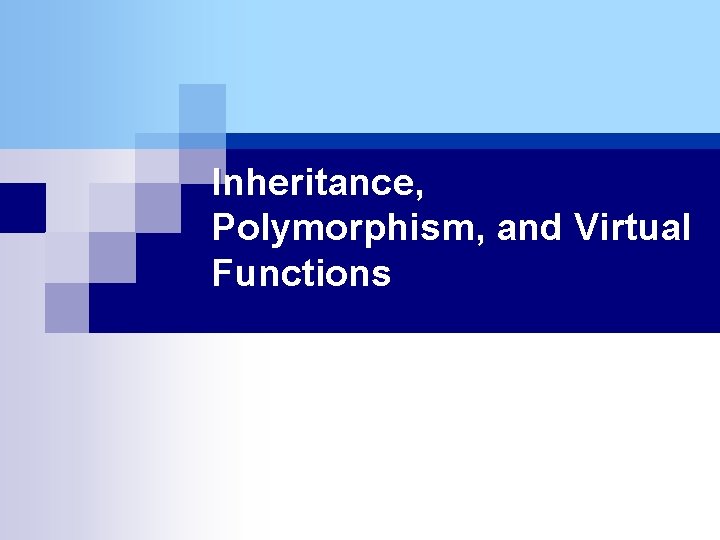
Inheritance, Polymorphism, and Virtual Functions
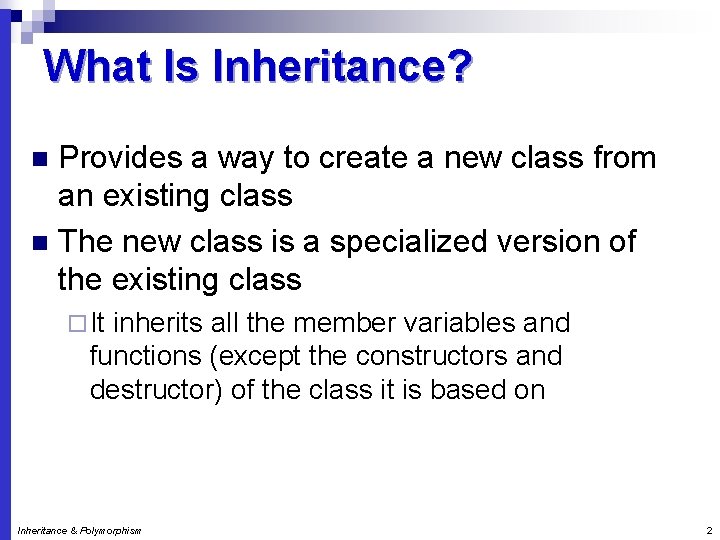
What Is Inheritance? Provides a way to create a new class from an existing class n The new class is a specialized version of the existing class n ¨ It inherits all the member variables and functions (except the constructors and destructor) of the class it is based on Inheritance & Polymorphism 2
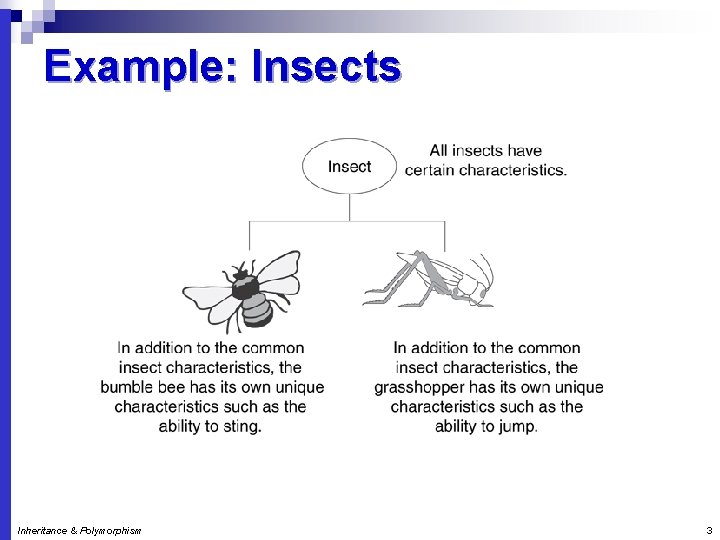
Example: Insects Inheritance & Polymorphism 3
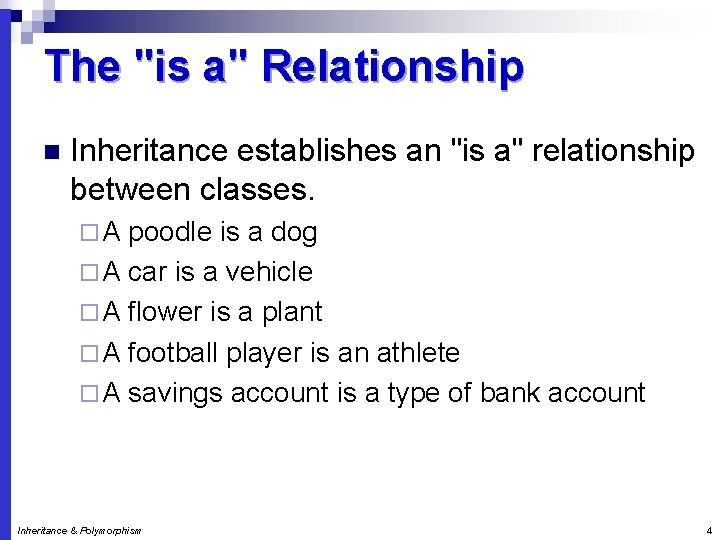
The "is a" Relationship n Inheritance establishes an "is a" relationship between classes. ¨A poodle is a dog ¨ A car is a vehicle ¨ A flower is a plant ¨ A football player is an athlete ¨ A savings account is a type of bank account Inheritance & Polymorphism 4
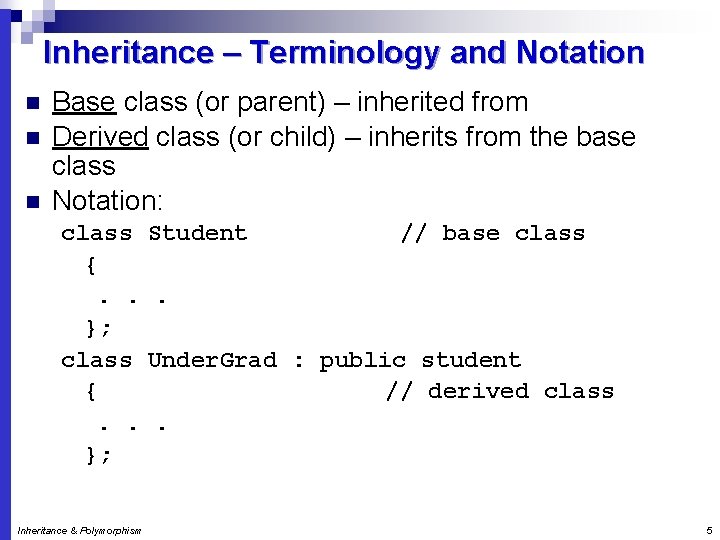
Inheritance – Terminology and Notation n Base class (or parent) – inherited from Derived class (or child) – inherits from the base class Notation: class {. . }; Inheritance & Polymorphism Student // base class . Under. Grad : public student // derived class. 5
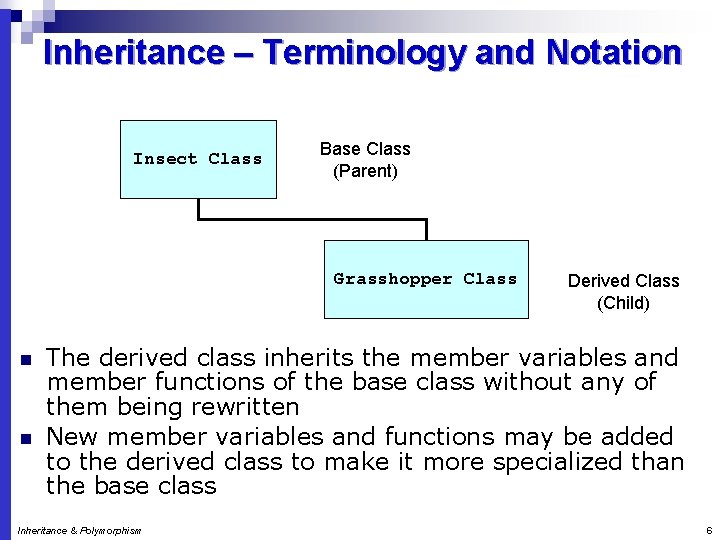
Inheritance – Terminology and Notation Insect Class Base Class (Parent) Grasshopper Class n n Derived Class (Child) The derived class inherits the member variables and member functions of the base class without any of them being rewritten New member variables and functions may be added to the derived class to make it more specialized than the base class Inheritance & Polymorphism 6
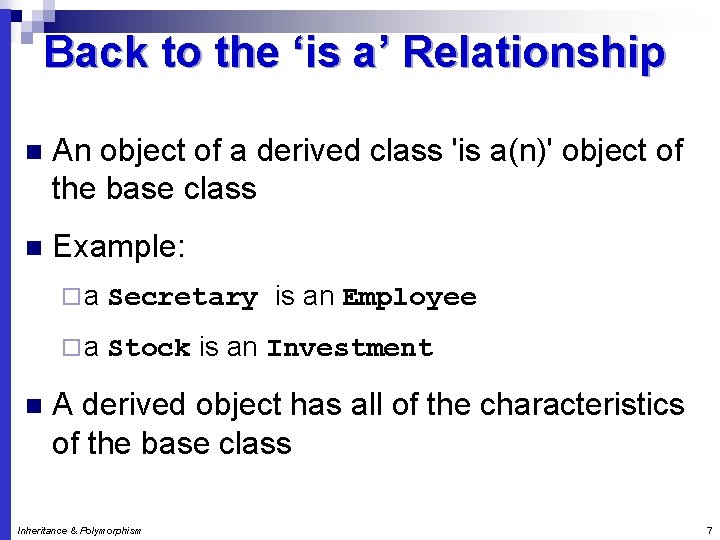
Back to the ‘is a’ Relationship n An object of a derived class 'is a(n)' object of the base class n Example: n ¨a Secretary is an Employee ¨a Stock is an Investment A derived object has all of the characteristics of the base class Inheritance & Polymorphism 7
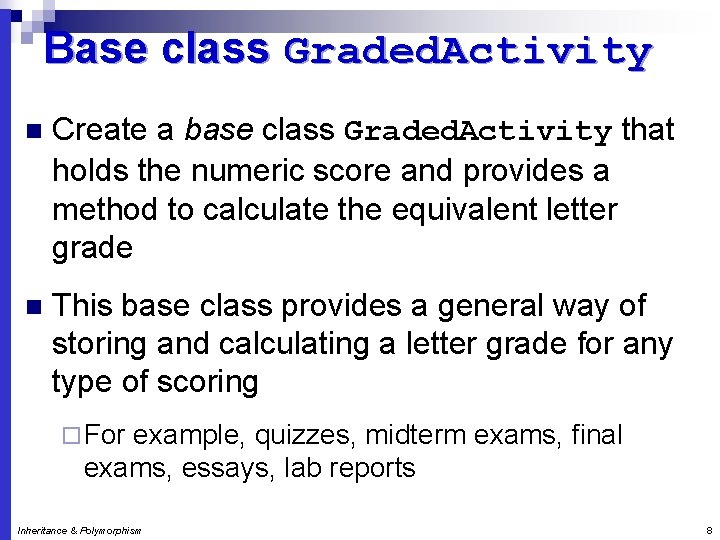
Base class Graded. Activity n Create a base class Graded. Activity that holds the numeric score and provides a method to calculate the equivalent letter grade n This base class provides a general way of storing and calculating a letter grade for any type of scoring ¨ For example, quizzes, midterm exams, final exams, essays, lab reports Inheritance & Polymorphism 8
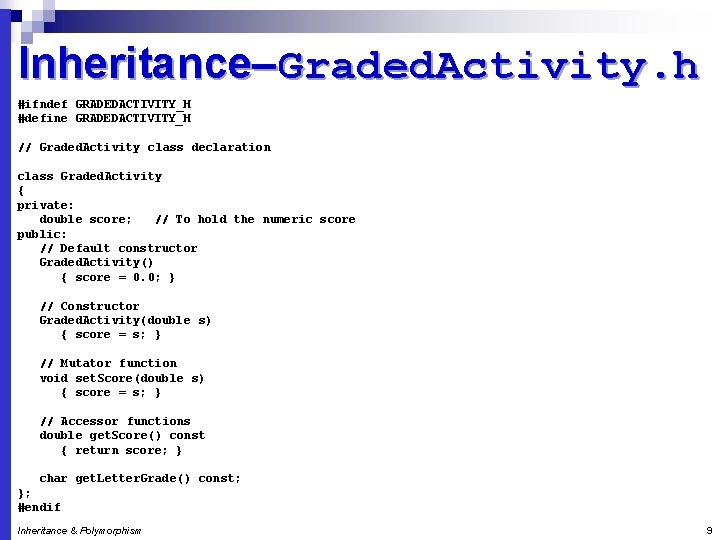
Inheritance–Graded. Activity. h #ifndef GRADEDACTIVITY_H #define GRADEDACTIVITY_H // Graded. Activity class declaration class Graded. Activity { private: double score; // To hold the numeric score public: // Default constructor Graded. Activity() { score = 0. 0; } // Constructor Graded. Activity(double s) { score = s; } // Mutator function void set. Score(double s) { score = s; } // Accessor functions double get. Score() const { return score; } char get. Letter. Grade() const; }; #endif Inheritance & Polymorphism 9
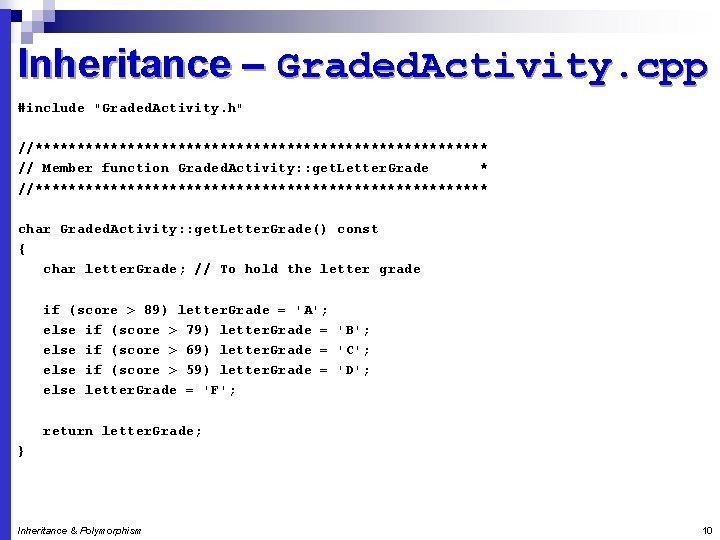
Inheritance – Graded. Activity. cpp #include "Graded. Activity. h" //*************************** // Member function Graded. Activity: : get. Letter. Grade * //*************************** char Graded. Activity: : get. Letter. Grade() const { char letter. Grade; // To hold the letter grade if (score > 89) letter. Grade = 'A'; else if (score > 79) letter. Grade = 'B'; else if (score > 69) letter. Grade = 'C'; else if (score > 59) letter. Grade = 'D'; else letter. Grade = 'F'; return letter. Grade; } Inheritance & Polymorphism 10
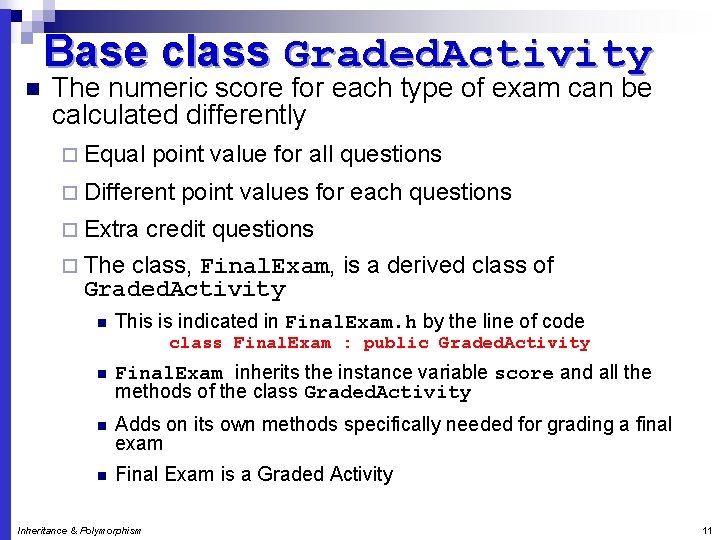
n Base class Graded. Activity The numeric score for each type of exam can be calculated differently ¨ Equal point value for all questions ¨ Different point values for each questions ¨ Extra credit questions ¨ The class, Final. Exam, is a derived class of Graded. Activity n This is indicated in Final. Exam. h by the line of code class Final. Exam : public Graded. Activity n Final. Exam inherits the instance variable score and all the methods of the class Graded. Activity n Adds on its own methods specifically needed for grading a final exam n Final Exam is a Graded Activity Inheritance & Polymorphism 11
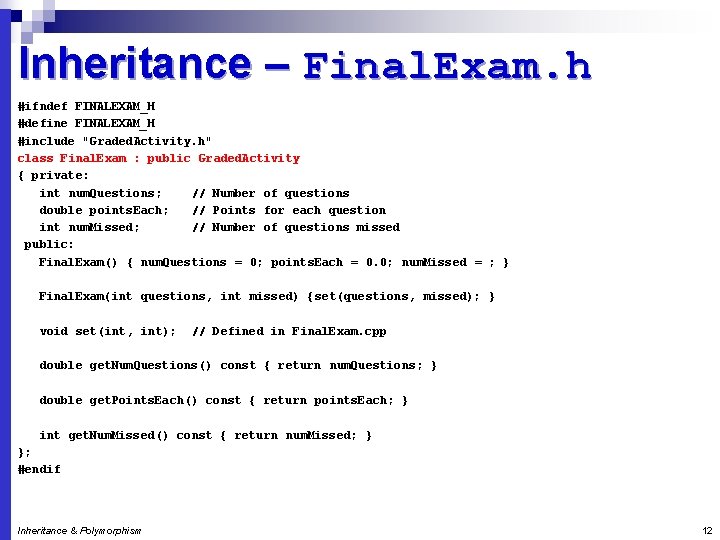
Inheritance – Final. Exam. h #ifndef FINALEXAM_H #define FINALEXAM_H #include "Graded. Activity. h" class Final. Exam : public Graded. Activity { private: int num. Questions; // Number of questions double points. Each; // Points for each question int num. Missed; // Number of questions missed public: Final. Exam() { num. Questions = 0; points. Each = 0. 0; num. Missed = ; } Final. Exam(int questions, int missed) {set(questions, missed); } void set(int, int); // Defined in Final. Exam. cpp double get. Num. Questions() const { return num. Questions; } double get. Points. Each() const { return points. Each; } int get. Num. Missed() const { return num. Missed; } }; #endif Inheritance & Polymorphism 12
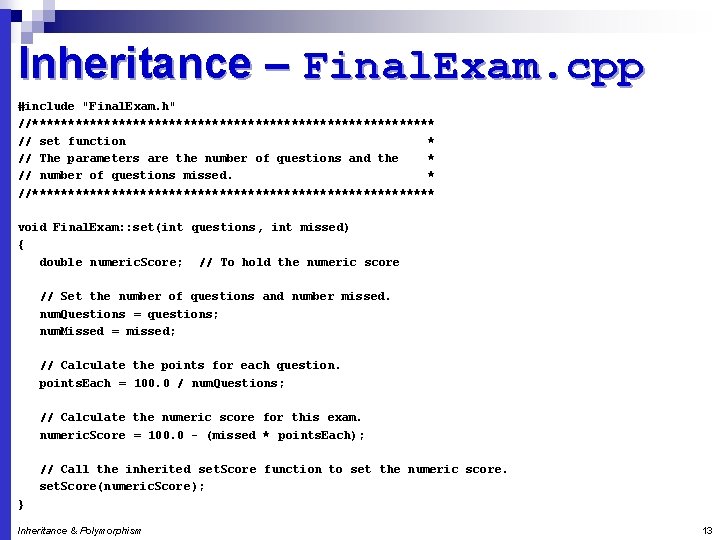
Inheritance – Final. Exam. cpp #include "Final. Exam. h" //**************************** // set function * // The parameters are the number of questions and the * // number of questions missed. * //**************************** void Final. Exam: : set(int questions, int missed) { double numeric. Score; // To hold the numeric score // Set the number of questions and number missed. num. Questions = questions; num. Missed = missed; // Calculate the points for each question. points. Each = 100. 0 / num. Questions; // Calculate the numeric score for this exam. numeric. Score = 100. 0 - (missed * points. Each); // Call the inherited set. Score function to set the numeric score. set. Score(numeric. Score); } Inheritance & Polymorphism 13
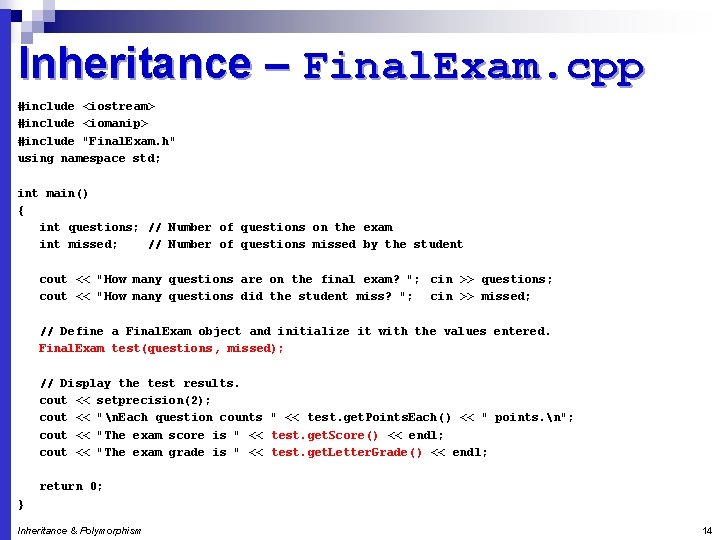
Inheritance – Final. Exam. cpp #include <iostream> #include <iomanip> #include "Final. Exam. h" using namespace std; int main() { int questions; // Number of questions on the exam int missed; // Number of questions missed by the student cout << "How many questions are on the final exam? "; cin >> questions; cout << "How many questions did the student miss? "; cin >> missed; // Define a Final. Exam object and initialize it with the values entered. Final. Exam test(questions, missed); // Display the test results. cout << setprecision(2); cout << "n. Each question counts " << test. get. Points. Each() << " points. n"; cout << "The exam score is " << test. get. Score() << endl; cout << "The exam grade is " << test. get. Letter. Grade() << endl; return 0; } Inheritance & Polymorphism 14
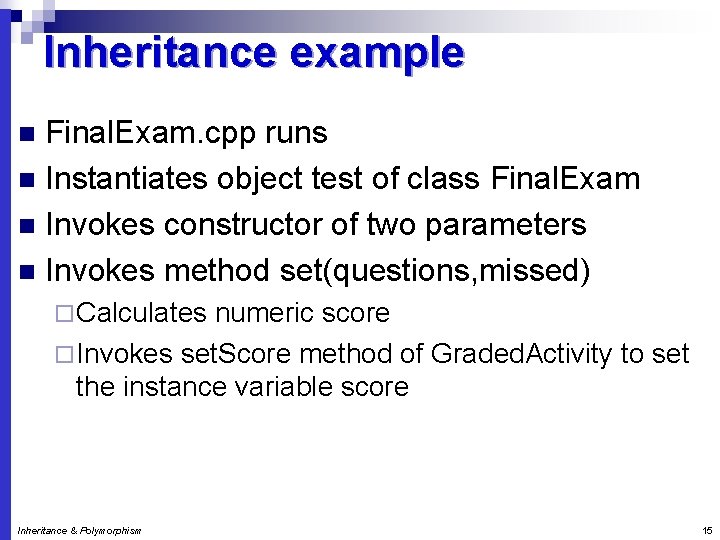
Inheritance example Final. Exam. cpp runs n Instantiates object test of class Final. Exam n Invokes constructor of two parameters n Invokes method set(questions, missed) n ¨ Calculates numeric score ¨ Invokes set. Score method of Graded. Activity to set the instance variable score Inheritance & Polymorphism 15
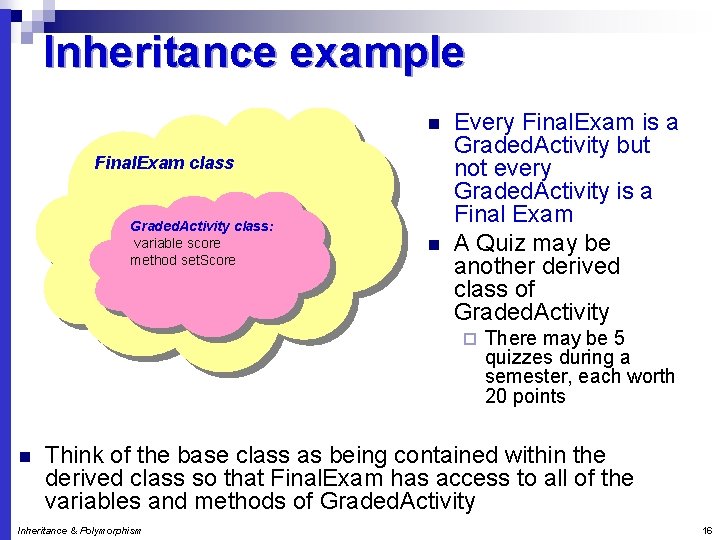
Inheritance example n Final. Exam class Graded. Activity class: variable score method set. Score n Every Final. Exam is a Graded. Activity but not every Graded. Activity is a Final Exam A Quiz may be another derived class of Graded. Activity ¨ n There may be 5 quizzes during a semester, each worth 20 points Think of the base class as being contained within the derived class so that Final. Exam has access to all of the variables and methods of Graded. Activity Inheritance & Polymorphism 16
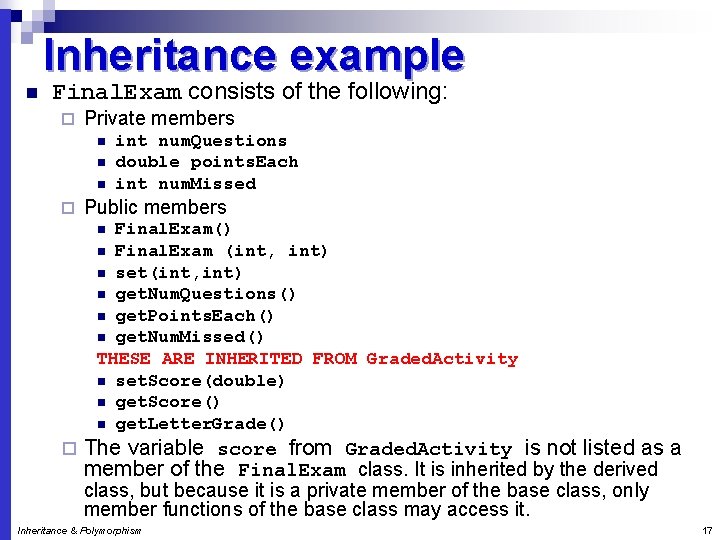
Inheritance example n Final. Exam consists of the following: ¨ Private members n n n ¨ int num. Questions double points. Each int num. Missed Public members Final. Exam() n Final. Exam (int, int) n set(int, int) n get. Num. Questions() n get. Points. Each() n get. Num. Missed() THESE ARE INHERITED FROM Graded. Activity n set. Score(double) n get. Score() n get. Letter. Grade() n ¨ The variable score from Graded. Activity is not listed as a member of the Final. Exam class. It is inherited by the derived class, but because it is a private member of the base class, only member functions of the base class may access it. Inheritance & Polymorphism 17
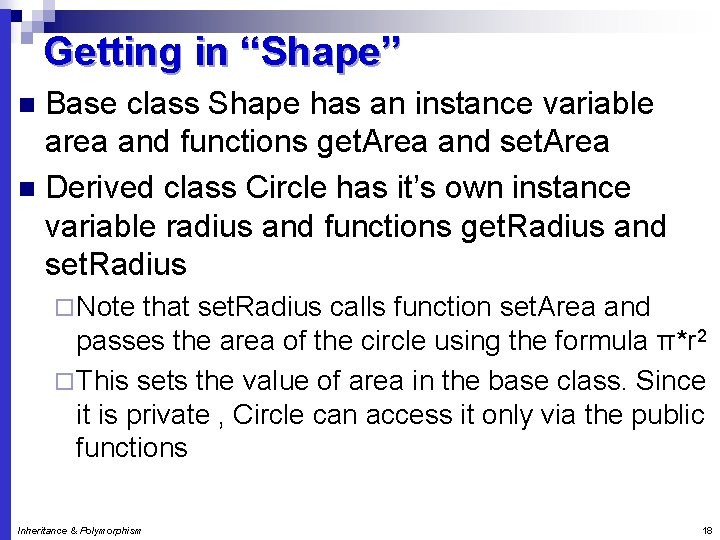
Getting in “Shape” Base class Shape has an instance variable area and functions get. Area and set. Area n Derived class Circle has it’s own instance variable radius and functions get. Radius and set. Radius n ¨ Note that set. Radius calls function set. Area and passes the area of the circle using the formula π*r 2 ¨ This sets the value of area in the base class. Since it is private , Circle can access it only via the public functions Inheritance & Polymorphism 18
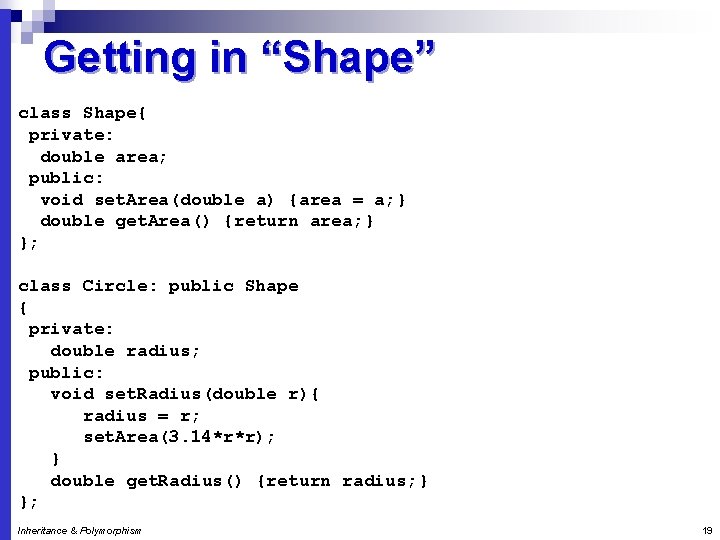
Getting in “Shape” class Shape{ private: double area; public: void set. Area(double a) {area = a; } double get. Area() {return area; } }; class Circle: public Shape { private: double radius; public: void set. Radius(double r){ radius = r; set. Area(3. 14*r*r); } double get. Radius() {return radius; } }; Inheritance & Polymorphism 19
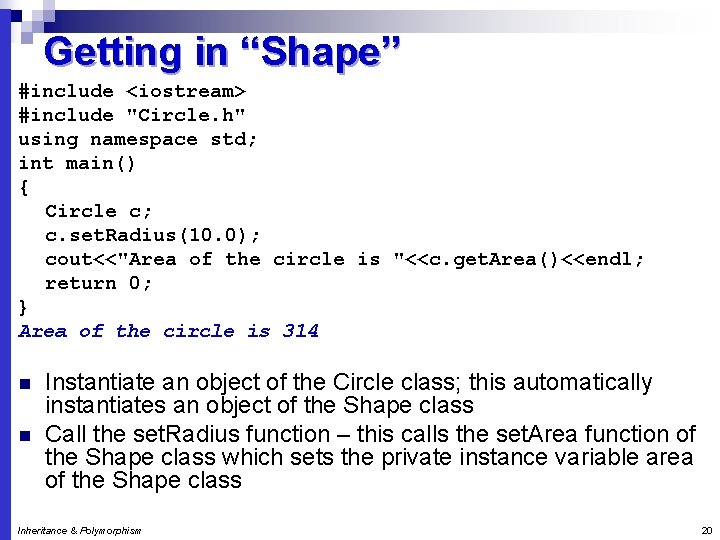
Getting in “Shape” #include <iostream> #include "Circle. h" using namespace std; int main() { Circle c; c. set. Radius(10. 0); cout<<"Area of the circle is "<<c. get. Area()<<endl; return 0; } Area of the circle is 314 n n Instantiate an object of the Circle class; this automatically instantiates an object of the Shape class Call the set. Radius function – this calls the set. Area function of the Shape class which sets the private instance variable area of the Shape class Inheritance & Polymorphism 20
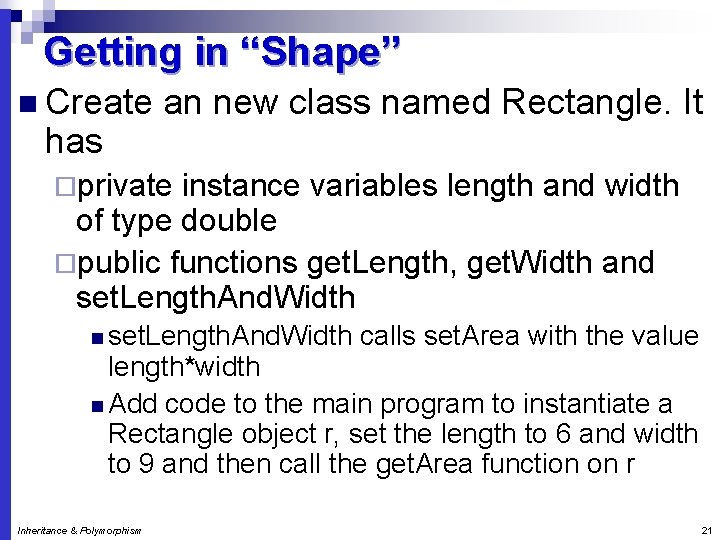
Getting in “Shape” n Create has an new class named Rectangle. It ¨private instance variables length and width of type double ¨public functions get. Length, get. Width and set. Length. And. Width n set. Length. And. Width calls set. Area with the value length*width n Add code to the main program to instantiate a Rectangle object r, set the length to 6 and width to 9 and then call the get. Area function on r Inheritance & Polymorphism 21
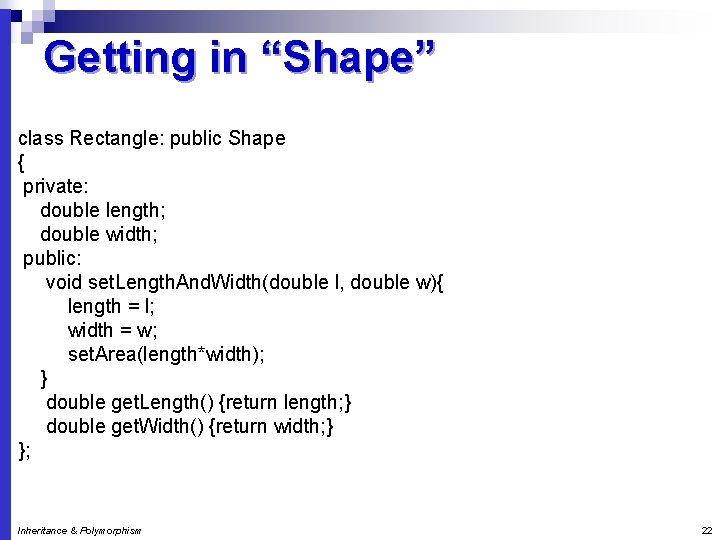
Getting in “Shape” class Rectangle: public Shape { private: double length; double width; public: void set. Length. And. Width(double l, double w){ length = l; width = w; set. Area(length*width); } double get. Length() {return length; } double get. Width() {return width; } }; Inheritance & Polymorphism 22
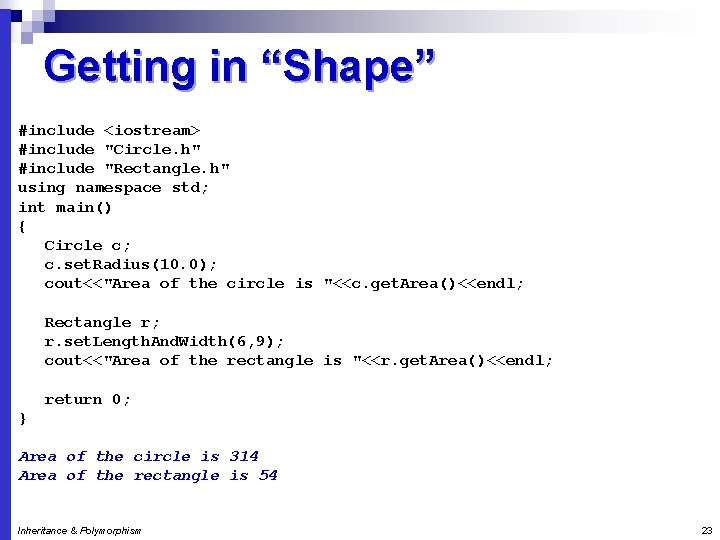
Getting in “Shape” #include <iostream> #include "Circle. h" #include "Rectangle. h" using namespace std; int main() { Circle c; c. set. Radius(10. 0); cout<<"Area of the circle is "<<c. get. Area()<<endl; Rectangle r; r. set. Length. And. Width(6, 9); cout<<"Area of the rectangle is "<<r. get. Area()<<endl; return 0; } Area of the circle is 314 Area of the rectangle is 54 Inheritance & Polymorphism 23
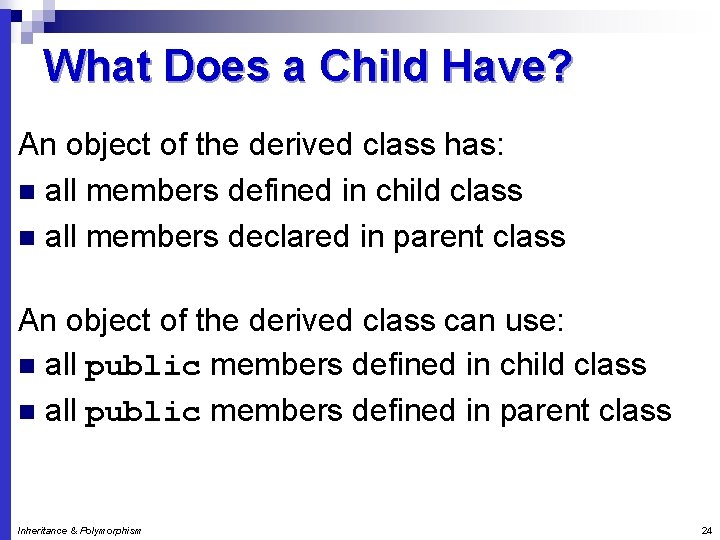
What Does a Child Have? An object of the derived class has: n all members defined in child class n all members declared in parent class An object of the derived class can use: n all public members defined in child class n all public members defined in parent class Inheritance & Polymorphism 24
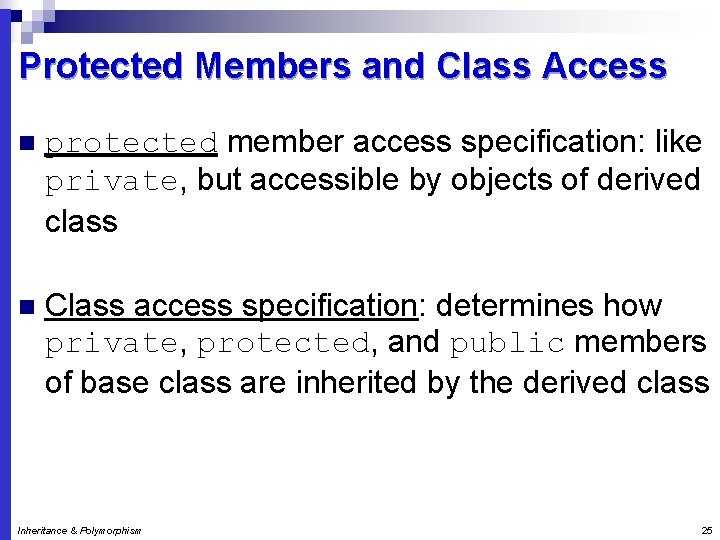
Protected Members and Class Access n protected member access specification: like private, but accessible by objects of derived class n Class access specification: determines how private, protected, and public members of base class are inherited by the derived class Inheritance & Polymorphism 25
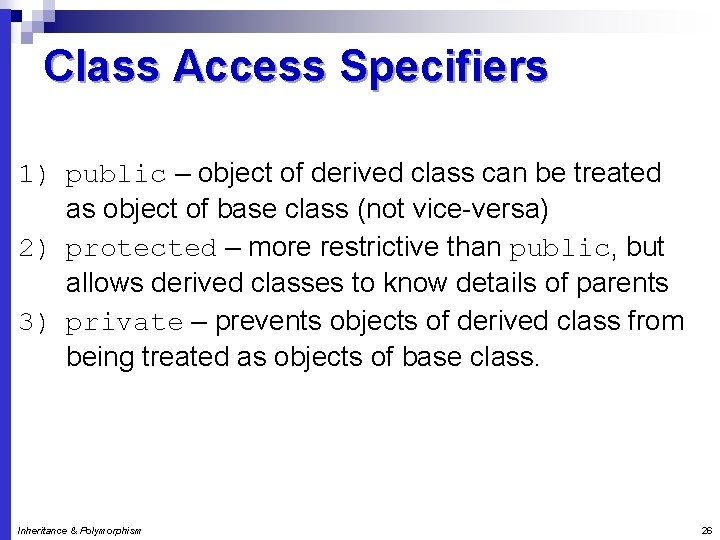
Class Access Specifiers 1) public – object of derived class can be treated as object of base class (not vice-versa) 2) protected – more restrictive than public, but allows derived classes to know details of parents 3) private – prevents objects of derived class from being treated as objects of base class. Inheritance & Polymorphism 26
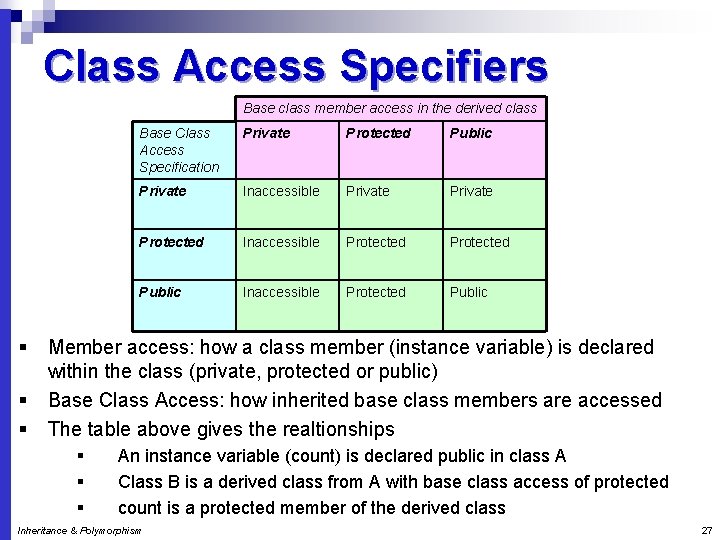
Class Access Specifiers Base class member access in the derived class § § § Base Class Access Specification Private Protected Public Private Inaccessible Private Protected Inaccessible Protected Public Member access: how a class member (instance variable) is declared within the class (private, protected or public) Base Class Access: how inherited base class members are accessed The table above gives the realtionships § § § An instance variable (count) is declared public in class A Class B is a derived class from A with base class access of protected count is a protected member of the derived class Inheritance & Polymorphism 27
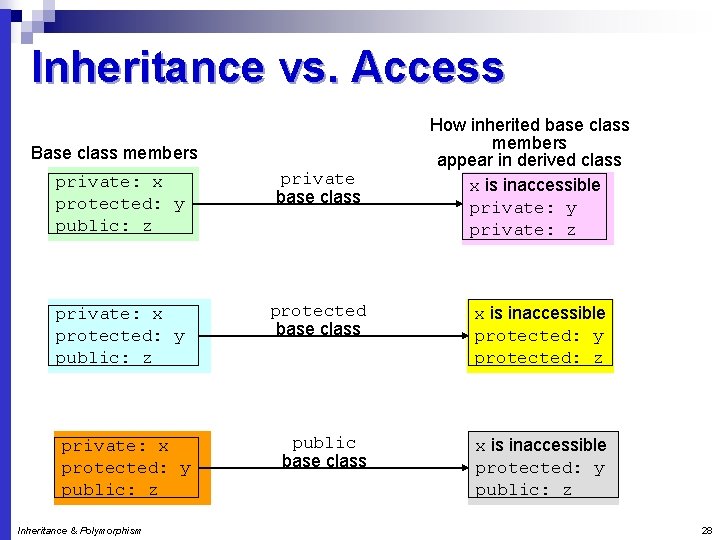
Inheritance vs. Access Base class members How inherited base class members appear in derived class x is inaccessible private: y private: z private: x protected: y public: z private base class private: x protected: y public: z protected base class x is inaccessible protected: y protected: z public base class x is inaccessible protected: y public: z private: x protected: y public: z Inheritance & Polymorphism 28
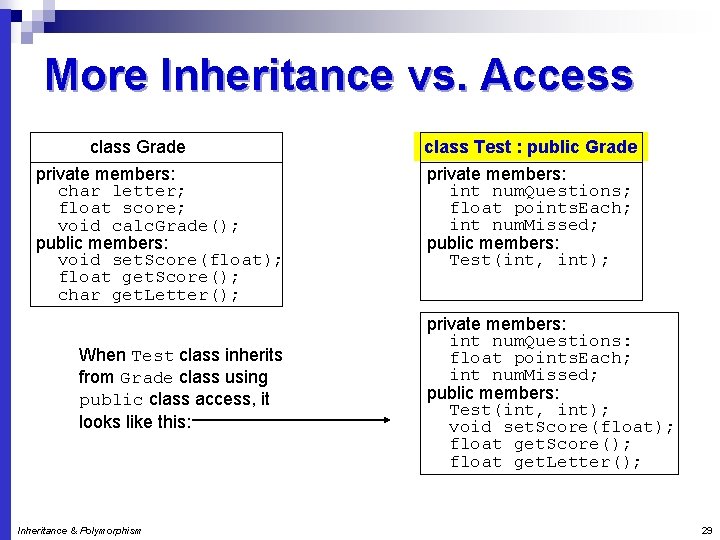
More Inheritance vs. Access class Grade private members: char letter; float score; void calc. Grade(); public members: void set. Score(float); float get. Score(); char get. Letter(); When Test class inherits from Grade class using public class access, it looks like this: Inheritance & Polymorphism class Test : public Grade private members: int num. Questions; float points. Each; int num. Missed; public members: Test(int, int); private members: int num. Questions: float points. Each; int num. Missed; public members: Test(int, int); void set. Score(float); float get. Score(); float get. Letter(); 29
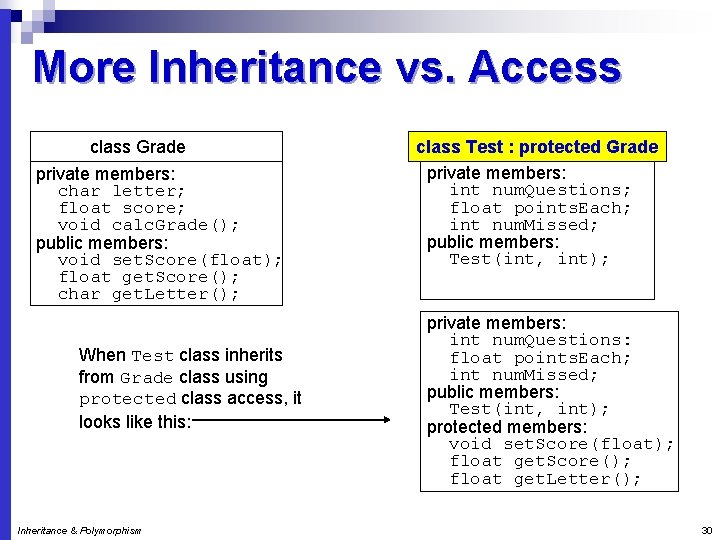
More Inheritance vs. Access class Grade private members: char letter; float score; void calc. Grade(); public members: void set. Score(float); float get. Score(); char get. Letter(); When Test class inherits from Grade class using protected class access, it looks like this: Inheritance & Polymorphism class Test : protected Grade private members: int num. Questions; float points. Each; int num. Missed; public members: Test(int, int); private members: int num. Questions: float points. Each; int num. Missed; public members: Test(int, int); protected members: void set. Score(float); float get. Score(); float get. Letter(); 30
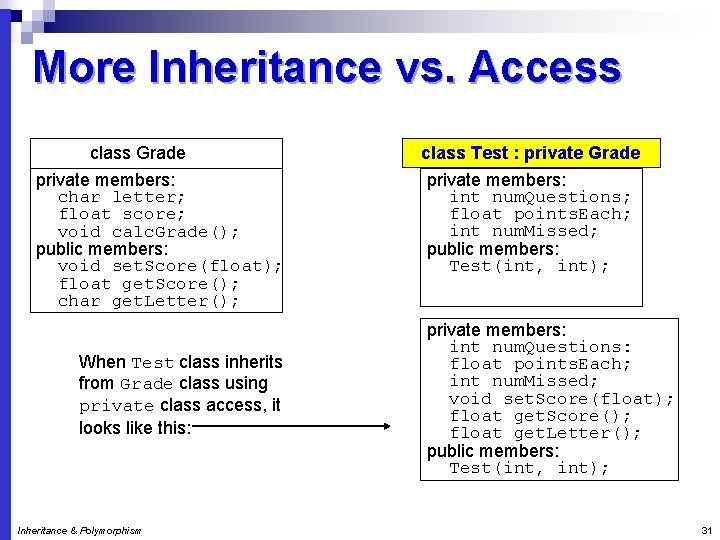
More Inheritance vs. Access class Grade private members: char letter; float score; void calc. Grade(); public members: void set. Score(float); float get. Score(); char get. Letter(); When Test class inherits from Grade class using private class access, it looks like this: Inheritance & Polymorphism class Test : private Grade private members: int num. Questions; float points. Each; int num. Missed; public members: Test(int, int); private members: int num. Questions: float points. Each; int num. Missed; void set. Score(float); float get. Score(); float get. Letter(); public members: Test(int, int); 31
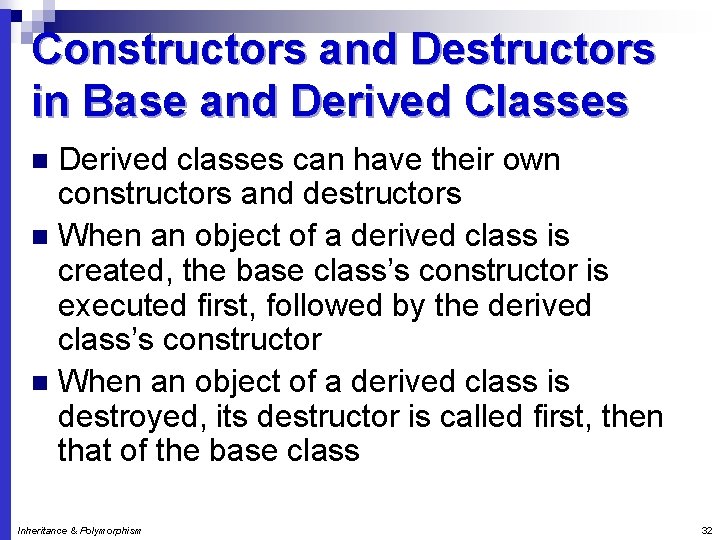
Constructors and Destructors in Base and Derived Classes Derived classes can have their own constructors and destructors n When an object of a derived class is created, the base class’s constructor is executed first, followed by the derived class’s constructor n When an object of a derived class is destroyed, its destructor is called first, then that of the base class n Inheritance & Polymorphism 32
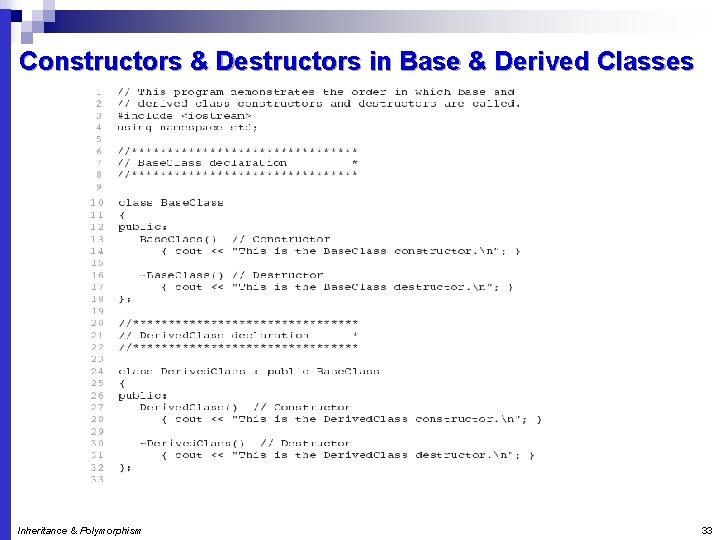
Constructors & Destructors in Base & Derived Classes Inheritance & Polymorphism 33
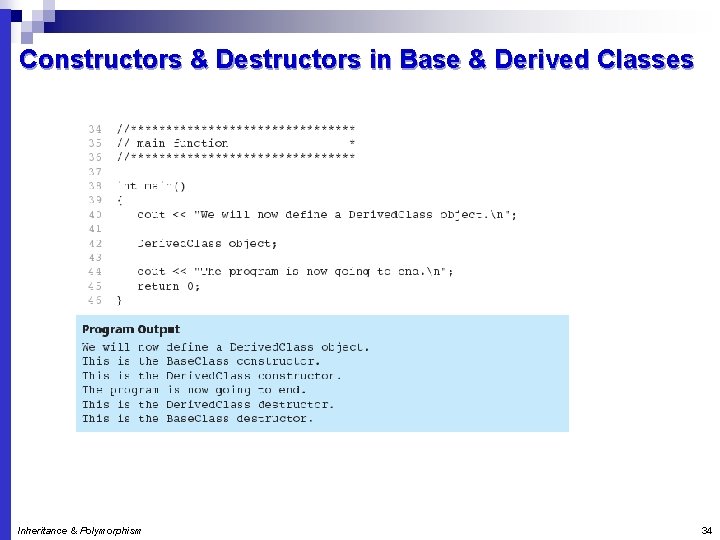
Constructors & Destructors in Base & Derived Classes Inheritance & Polymorphism 34
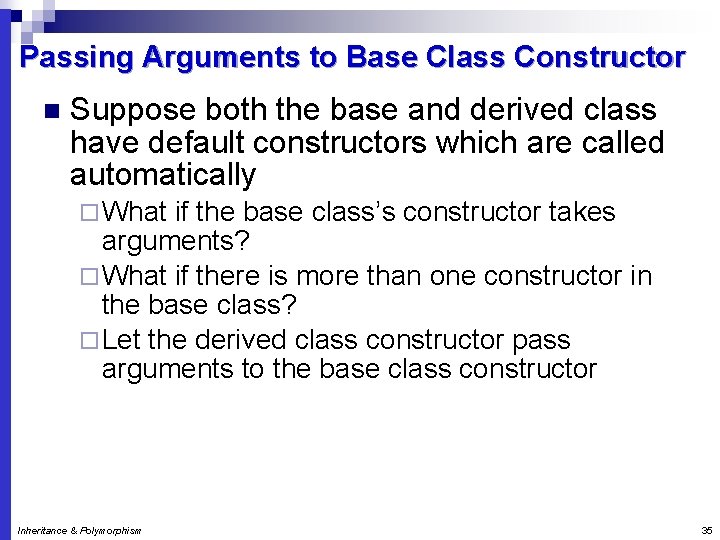
Passing Arguments to Base Class Constructor n Suppose both the base and derived class have default constructors which are called automatically ¨ What if the base class’s constructor takes arguments? ¨ What if there is more than one constructor in the base class? ¨ Let the derived class constructor pass arguments to the base class constructor Inheritance & Polymorphism 35
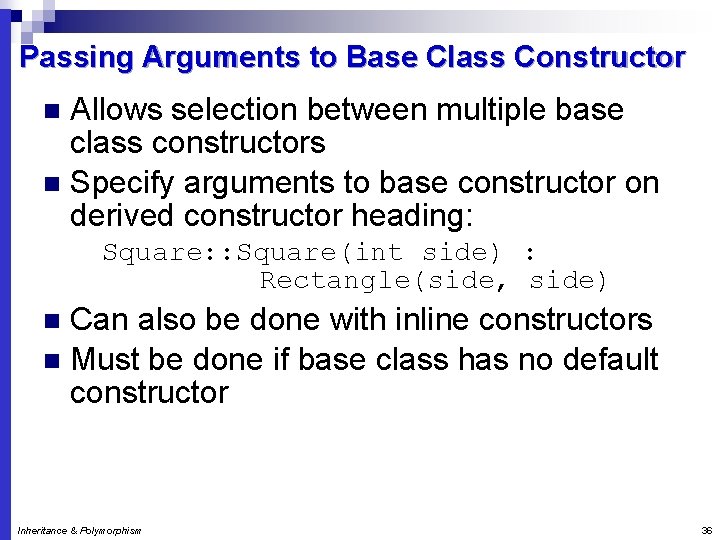
Passing Arguments to Base Class Constructor Allows selection between multiple base class constructors n Specify arguments to base constructor on derived constructor heading: n Square: : Square(int side) : Rectangle(side, side) Can also be done with inline constructors n Must be done if base class has no default constructor n Inheritance & Polymorphism 36
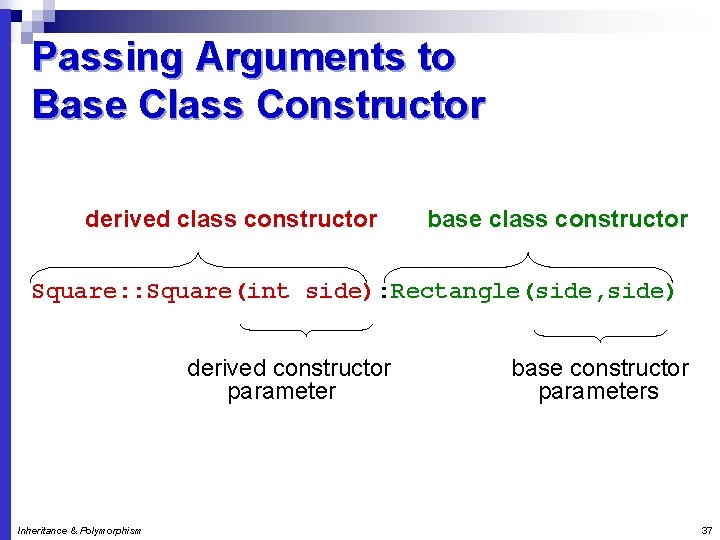
Passing Arguments to Base Class Constructor derived class constructor base class constructor Square: : Square(int side): Rectangle(side, side) derived constructor parameter Inheritance & Polymorphism base constructor parameters 37
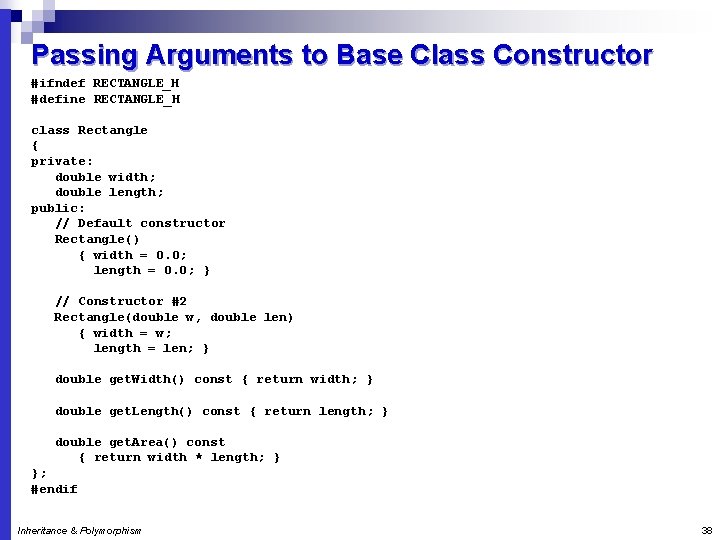
Passing Arguments to Base Class Constructor #ifndef RECTANGLE_H #define RECTANGLE_H class Rectangle { private: double width; double length; public: // Default constructor Rectangle() { width = 0. 0; length = 0. 0; } // Constructor #2 Rectangle(double w, double len) { width = w; length = len; } double get. Width() const { return width; } double get. Length() const { return length; } double get. Area() const { return width * length; } }; #endif Inheritance & Polymorphism 38
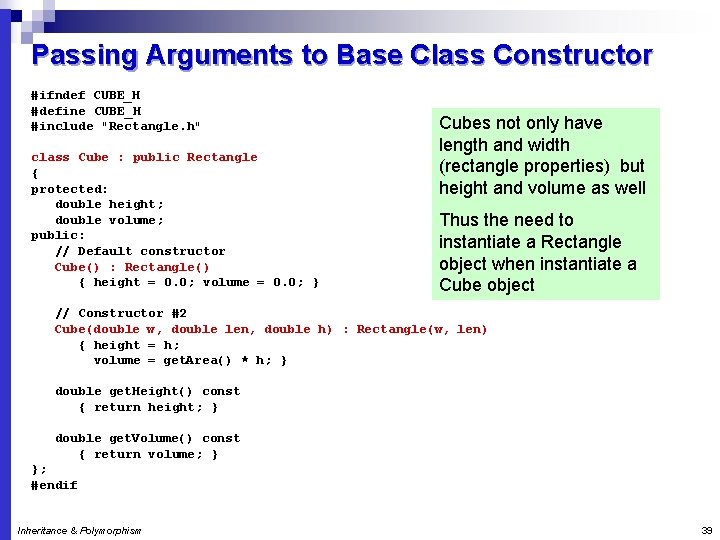
Passing Arguments to Base Class Constructor #ifndef CUBE_H #define CUBE_H #include "Rectangle. h" class Cube : public Rectangle { protected: double height; double volume; public: // Default constructor Cube() : Rectangle() { height = 0. 0; volume = 0. 0; } Cubes not only have length and width (rectangle properties) but height and volume as well Thus the need to instantiate a Rectangle object when instantiate a Cube object // Constructor #2 Cube(double w, double len, double h) : Rectangle(w, len) { height = h; volume = get. Area() * h; } double get. Height() const { return height; } double get. Volume() const { return volume; } }; #endif Inheritance & Polymorphism 39
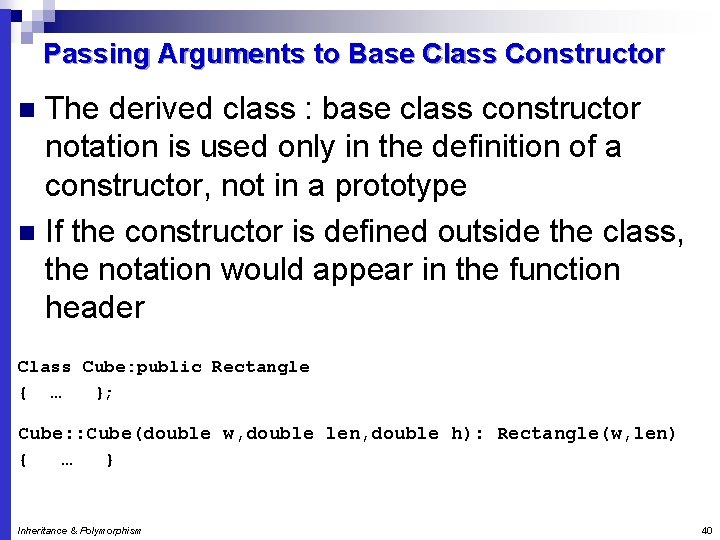
Passing Arguments to Base Class Constructor The derived class : base class constructor notation is used only in the definition of a constructor, not in a prototype n If the constructor is defined outside the class, the notation would appear in the function header n Class Cube: public Rectangle { … }; Cube: : Cube(double w, double len, double h): Rectangle(w, len) { … } Inheritance & Polymorphism 40
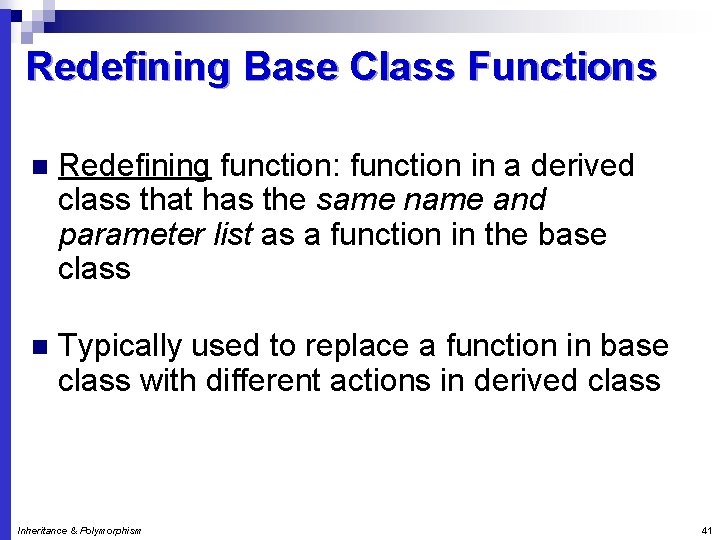
Redefining Base Class Functions n Redefining function: function in a derived class that has the same name and parameter list as a function in the base class n Typically used to replace a function in base class with different actions in derived class Inheritance & Polymorphism 41
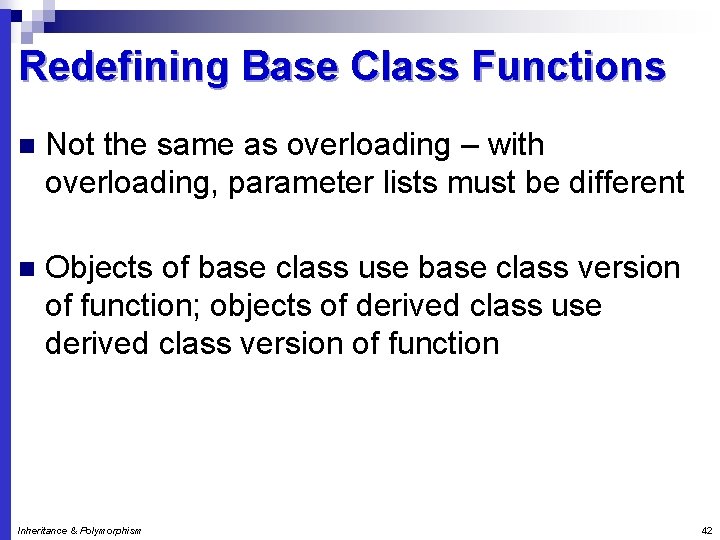
Redefining Base Class Functions n Not the same as overloading – with overloading, parameter lists must be different n Objects of base class use base class version of function; objects of derived class use derived class version of function Inheritance & Polymorphism 42
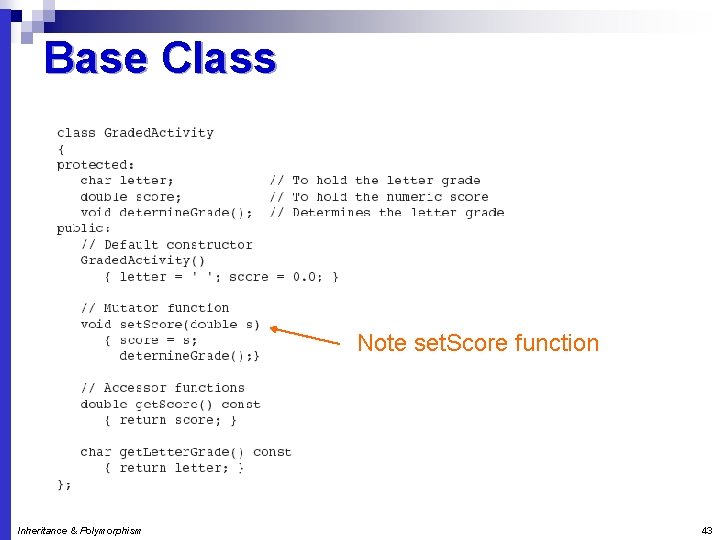
Base Class Note set. Score function Inheritance & Polymorphism 43
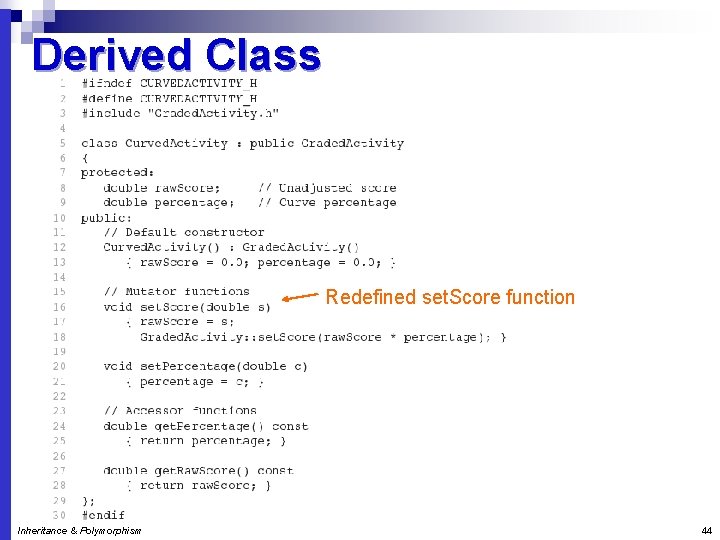
Derived Class Redefined set. Score function Inheritance & Polymorphism 44
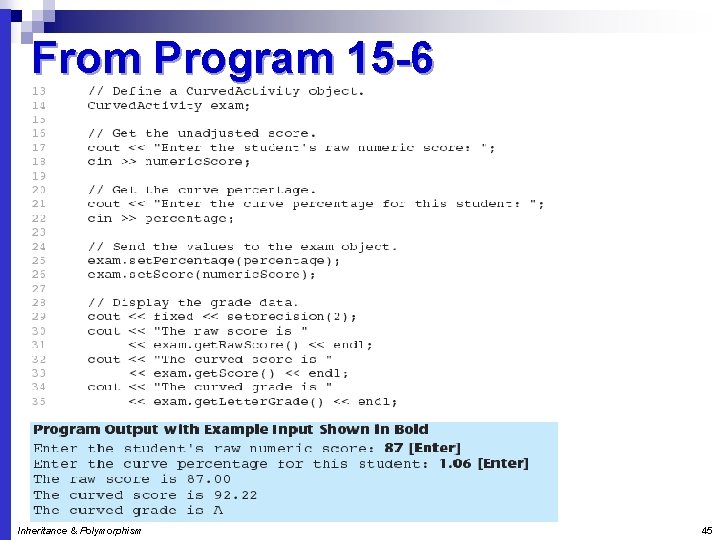
From Program 15 -6 Inheritance & Polymorphism 45
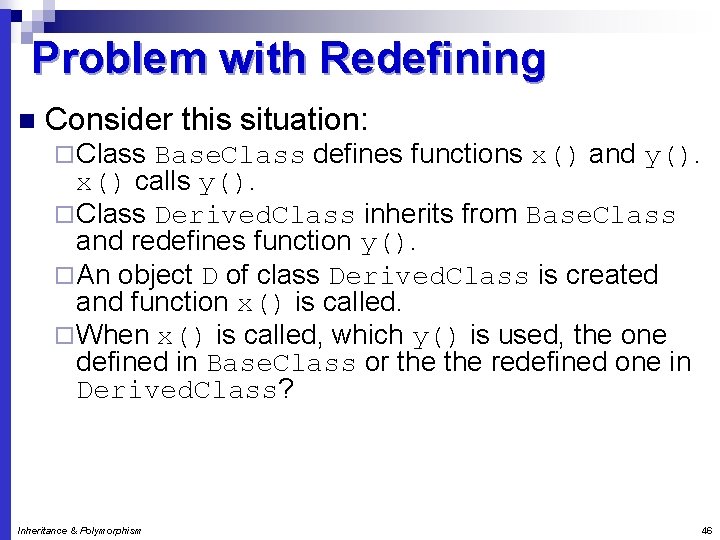
Problem with Redefining n Consider this situation: ¨ Class Base. Class defines functions x() and y(). x() calls y(). ¨ Class Derived. Class inherits from Base. Class and redefines function y(). ¨ An object D of class Derived. Class is created and function x() is called. ¨ When x() is called, which y() is used, the one defined in Base. Class or the redefined one in Derived. Class? Inheritance & Polymorphism 46
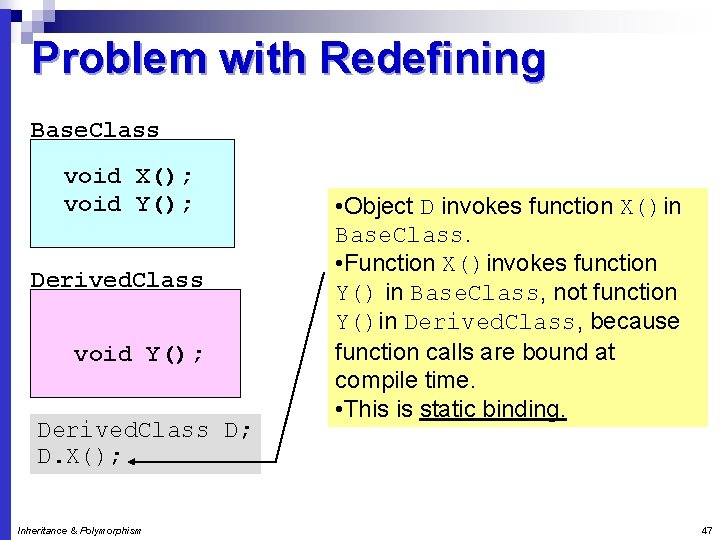
Problem with Redefining Base. Class void X(); void Y(); Derived. Class D; D. X(); Inheritance & Polymorphism • Object D invokes function X()in Base. Class. • Function X()invokes function Y() in Base. Class, not function Y()in Derived. Class, because function calls are bound at compile time. • This is static binding. 47
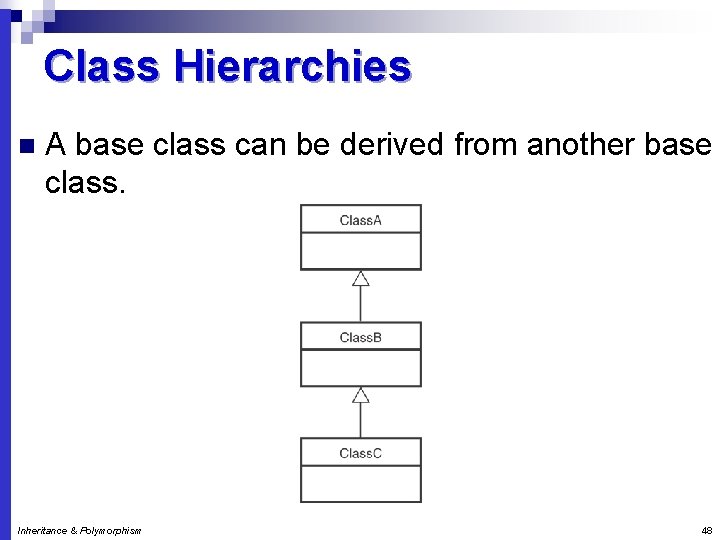
Class Hierarchies n A base class can be derived from another base class. Inheritance & Polymorphism 48
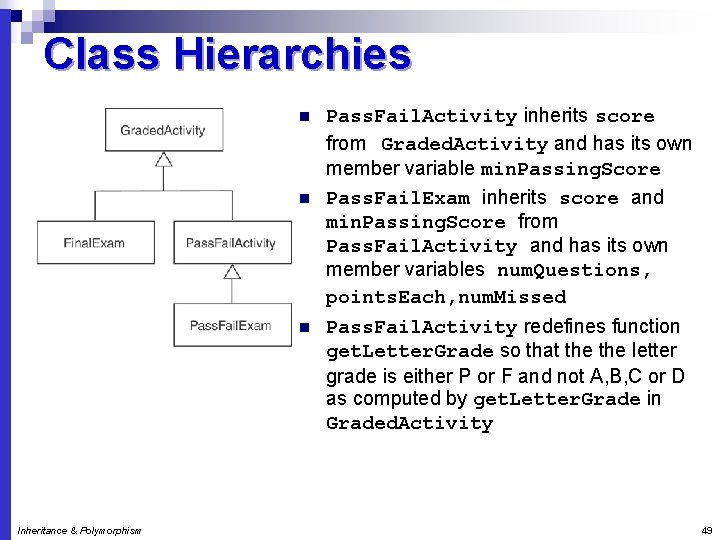
Class Hierarchies n n n Inheritance & Polymorphism Pass. Fail. Activity inherits score from Graded. Activity and has its own member variable min. Passing. Score Pass. Fail. Exam inherits score and min. Passing. Score from Pass. Fail. Activity and has its own member variables num. Questions, points. Each, num. Missed Pass. Fail. Activity redefines function get. Letter. Grade so that the letter grade is either P or F and not A, B, C or D as computed by get. Letter. Grade in Graded. Activity 49
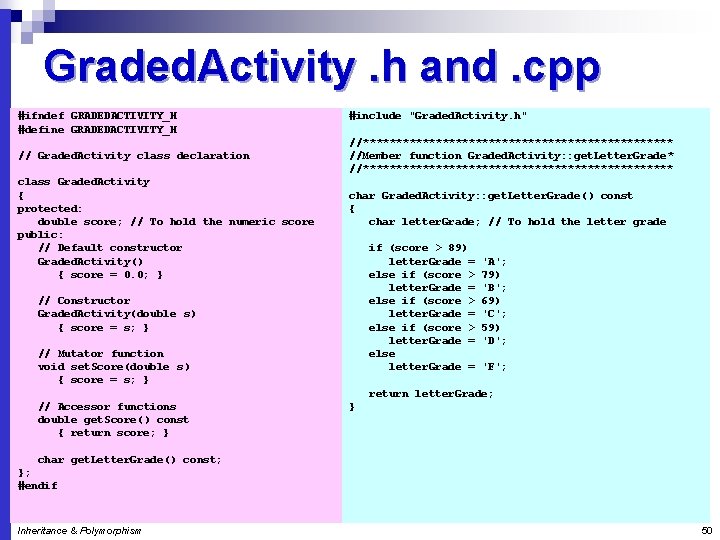
Graded. Activity. h and. cpp #ifndef GRADEDACTIVITY_H #define GRADEDACTIVITY_H // Graded. Activity class declaration class Graded. Activity { protected: double score; // To hold the numeric score public: // Default constructor Graded. Activity() { score = 0. 0; } #include "Graded. Activity. h" //************************ //Member function Graded. Activity: : get. Letter. Grade* //************************ char Graded. Activity: : get. Letter. Grade() const { char letter. Grade; // To hold the letter grade if (score > 89) letter. Grade = else if (score > letter. Grade = else letter. Grade = // Constructor Graded. Activity(double s) { score = s; } // Mutator function void set. Score(double s) { score = s; } 'A'; 79) 'B'; 69) 'C'; 59) 'D'; 'F'; return letter. Grade; // Accessor functions double get. Score() const { return score; } } char get. Letter. Grade() const; }; #endif Inheritance & Polymorphism 50
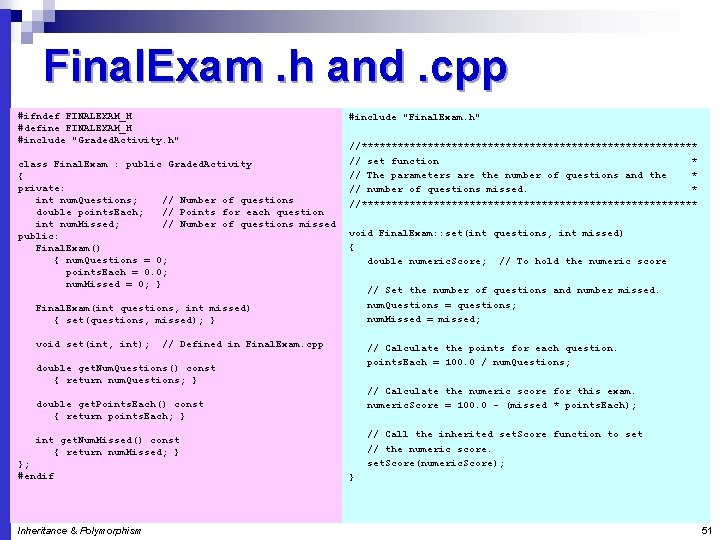
Final. Exam. h and. cpp #ifndef FINALEXAM_H #define FINALEXAM_H #include "Graded. Activity. h" class Final. Exam : public Graded. Activity { private: int num. Questions; // Number of questions double points. Each; // Points for each question int num. Missed; // Number of questions missed public: Final. Exam() { num. Questions = 0; points. Each = 0. 0; num. Missed = 0; } #include "Final. Exam. h" //**************************** // set function * // The parameters are the number of questions and the * // number of questions missed. * //**************************** void Final. Exam: : set(int questions, int missed) { double numeric. Score; // To hold the numeric score // Set the number of questions and number missed. num. Questions = questions; num. Missed = missed; Final. Exam(int questions, int missed) { set(questions, missed); } void set(int, int); // Defined in Final. Exam. cpp // Calculate the points for each question. points. Each = 100. 0 / num. Questions; double get. Num. Questions() const { return num. Questions; } // Calculate the numeric score for this exam. numeric. Score = 100. 0 - (missed * points. Each); double get. Points. Each() const { return points. Each; } // Call the inherited set. Score function to set // the numeric score. set. Score(numeric. Score); int get. Num. Missed() const { return num. Missed; } }; #endif Inheritance & Polymorphism } 51
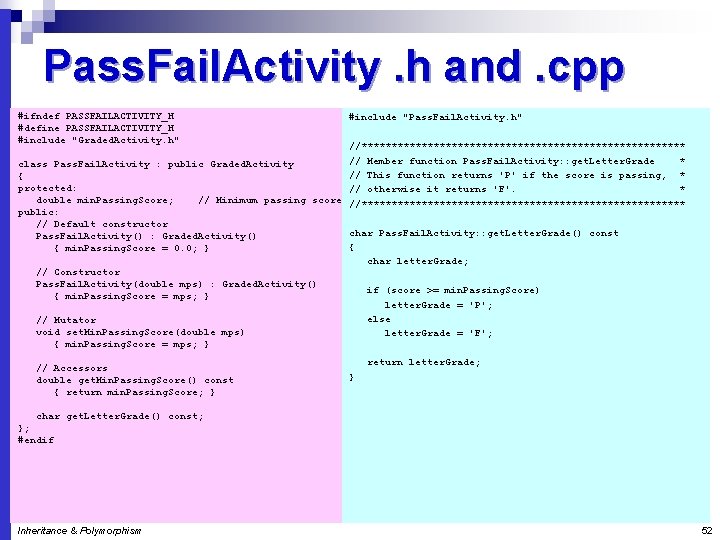
Pass. Fail. Activity. h and. cpp #ifndef PASSFAILACTIVITY_H #define PASSFAILACTIVITY_H #include "Graded. Activity. h" #include "Pass. Fail. Activity. h" //*************************** // Member function Pass. Fail. Activity: : get. Letter. Grade * class Pass. Fail. Activity : public Graded. Activity // This function returns 'P' if the score is passing, * { protected: // otherwise it returns 'F'. * double min. Passing. Score; // Minimum passing score. //*************************** public: // Default constructor char Pass. Fail. Activity: : get. Letter. Grade() const Pass. Fail. Activity() : Graded. Activity() { { min. Passing. Score = 0. 0; } char letter. Grade; // Constructor Pass. Fail. Activity(double mps) : Graded. Activity() if (score >= min. Passing. Score) { min. Passing. Score = mps; } letter. Grade = 'P'; else // Mutator void set. Min. Passing. Score(double mps) letter. Grade = 'F'; { min. Passing. Score = mps; } // Accessors double get. Min. Passing. Score() const { return min. Passing. Score; } return letter. Grade; } char get. Letter. Grade() const; }; #endif Inheritance & Polymorphism 52
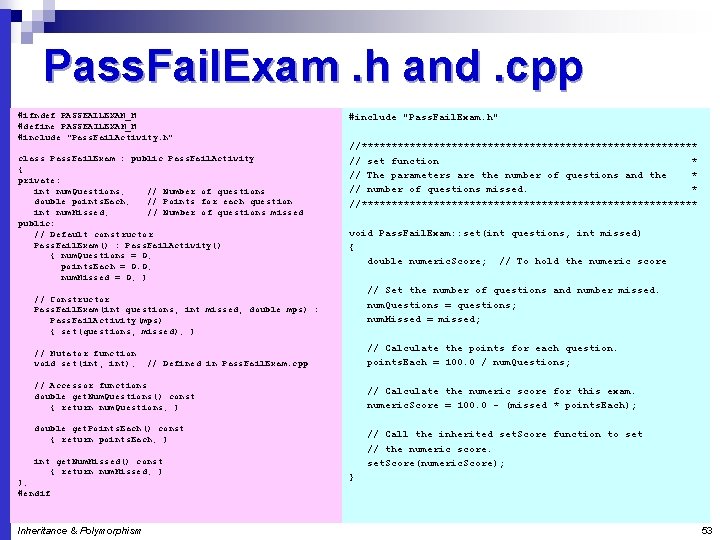
Pass. Fail. Exam. h and. cpp #ifndef PASSFAILEXAM_H #define PASSFAILEXAM_H #include "Pass. Fail. Activity. h" class Pass. Fail. Exam : public Pass. Fail. Activity { private: int num. Questions; // Number of questions double points. Each; // Points for each question int num. Missed; // Number of questions missed public: // Default constructor Pass. Fail. Exam() : Pass. Fail. Activity() { num. Questions = 0; points. Each = 0. 0; num. Missed = 0; } #include "Pass. Fail. Exam. h" //**************************** // set function * // The parameters are the number of questions and the * // number of questions missed. * //**************************** void Pass. Fail. Exam: : set(int questions, int missed) { double numeric. Score; // To hold the numeric score // Set the number of questions and number missed. num. Questions = questions; num. Missed = missed; // Constructor Pass. Fail. Exam(int questions, int missed, double mps) : Pass. Fail. Activity(mps) { set(questions, missed); } // Mutator function void set(int, int); // Calculate the points for each question. points. Each = 100. 0 / num. Questions; // Defined in Pass. Fail. Exam. cpp // Accessor functions double get. Num. Questions() const { return num. Questions; } // Calculate the numeric score for this exam. numeric. Score = 100. 0 - (missed * points. Each); double get. Points. Each() const { return points. Each; } int get. Num. Missed() const { return num. Missed; } }; #endif Inheritance & Polymorphism // Call the inherited set. Score function to set // the numeric score. set. Score(numeric. Score); } 53
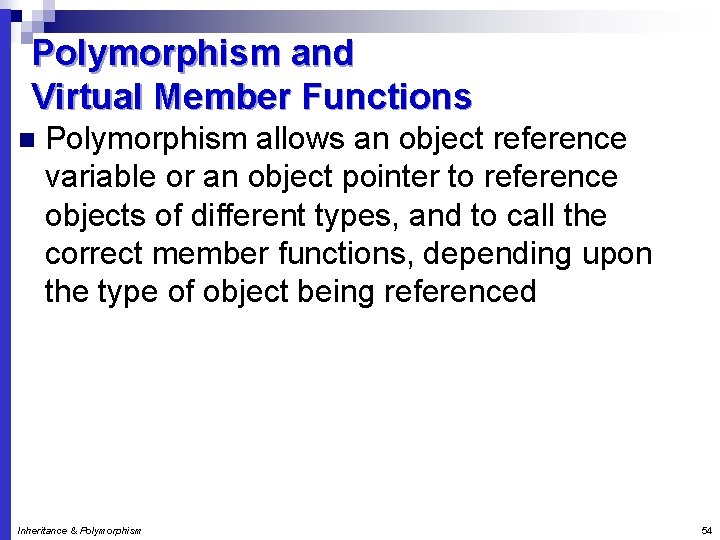
Polymorphism and Virtual Member Functions n Polymorphism allows an object reference variable or an object pointer to reference objects of different types, and to call the correct member functions, depending upon the type of object being referenced Inheritance & Polymorphism 54
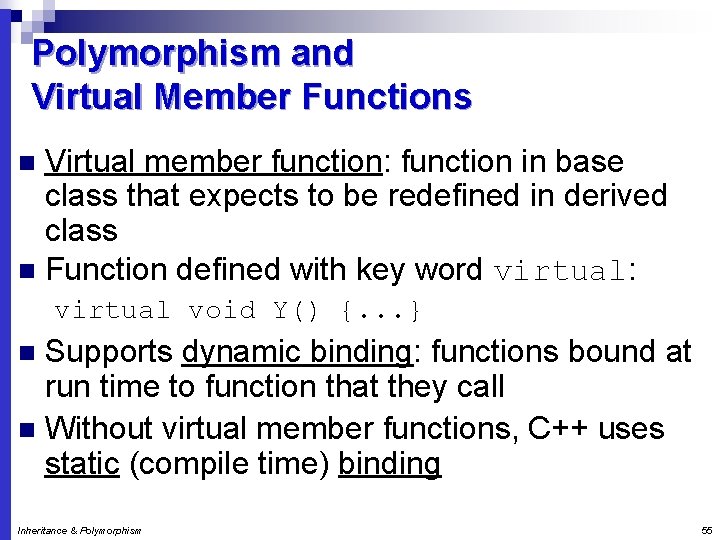
Polymorphism and Virtual Member Functions Virtual member function: function in base class that expects to be redefined in derived class n Function defined with key word virtual: n virtual void Y() {. . . } Supports dynamic binding: functions bound at run time to function that they call n Without virtual member functions, C++ uses static (compile time) binding n Inheritance & Polymorphism 55
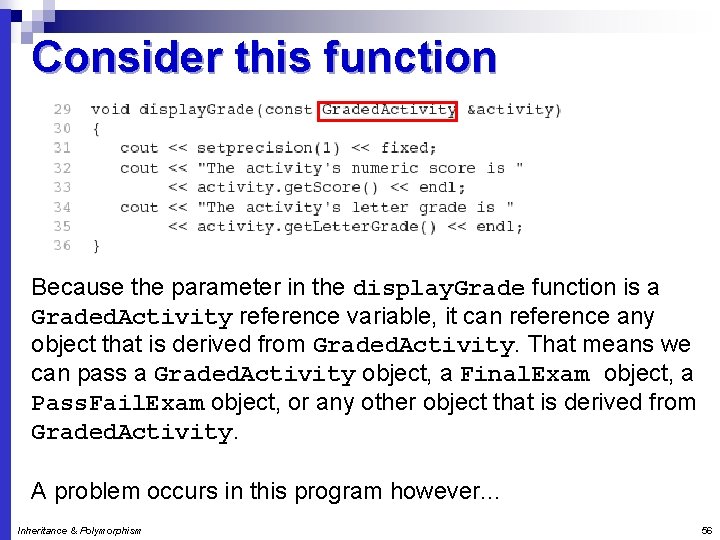
Consider this function Because the parameter in the display. Grade function is a Graded. Activity reference variable, it can reference any object that is derived from Graded. Activity. That means we can pass a Graded. Activity object, a Final. Exam object, a Pass. Fail. Exam object, or any other object that is derived from Graded. Activity. A problem occurs in this program however. . . Inheritance & Polymorphism 56
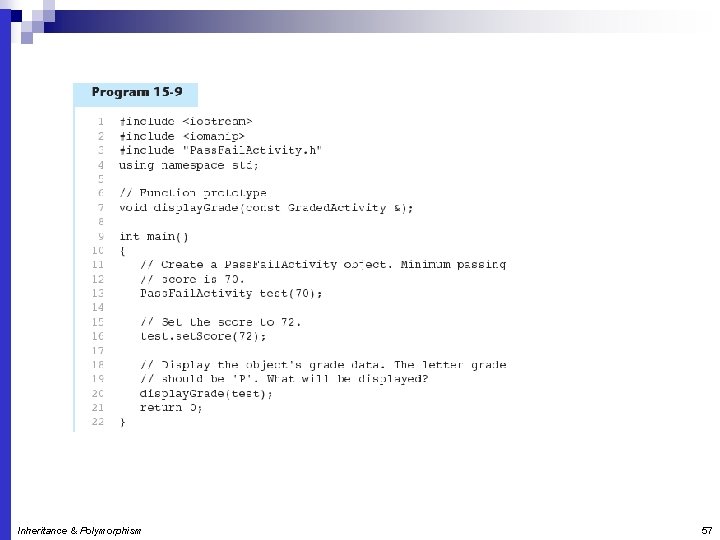
Inheritance & Polymorphism 57
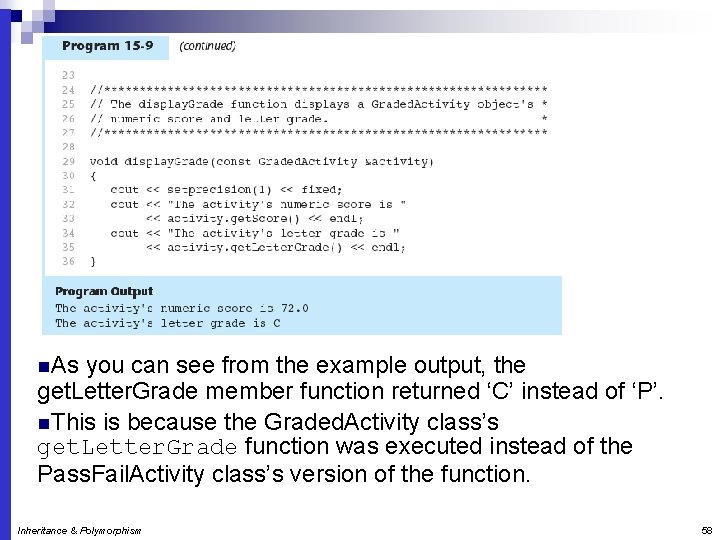
n. As you can see from the example output, the get. Letter. Grade member function returned ‘C’ instead of ‘P’. n. This is because the Graded. Activity class’s get. Letter. Grade function was executed instead of the Pass. Fail. Activity class’s version of the function. Inheritance & Polymorphism 58
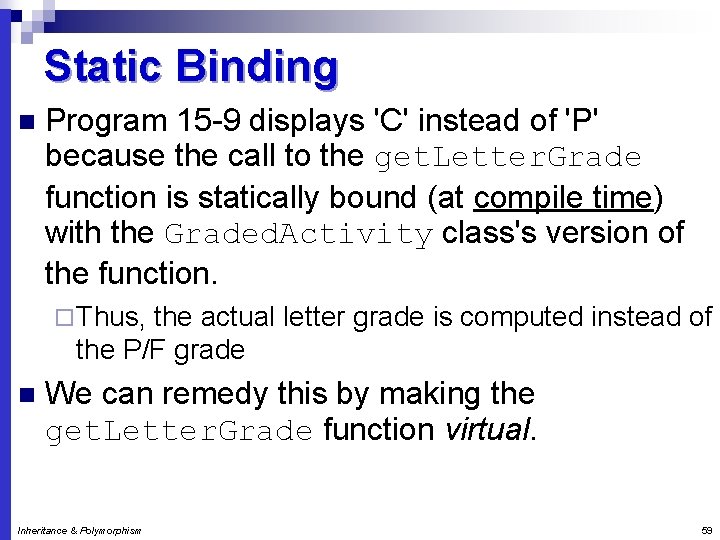
Static Binding n Program 15 -9 displays 'C' instead of 'P' because the call to the get. Letter. Grade function is statically bound (at compile time) with the Graded. Activity class's version of the function. ¨ Thus, the actual letter grade is computed instead of the P/F grade n We can remedy this by making the get. Letter. Grade function virtual. Inheritance & Polymorphism 59
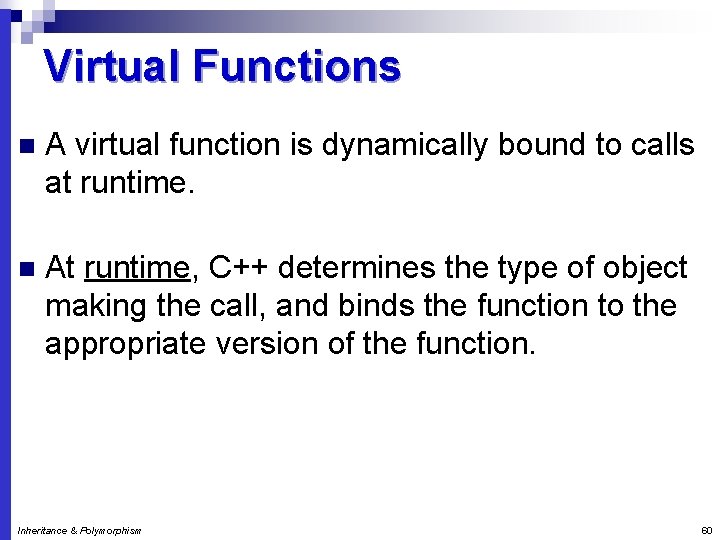
Virtual Functions n A virtual function is dynamically bound to calls at runtime. n At runtime, C++ determines the type of object making the call, and binds the function to the appropriate version of the function. Inheritance & Polymorphism 60
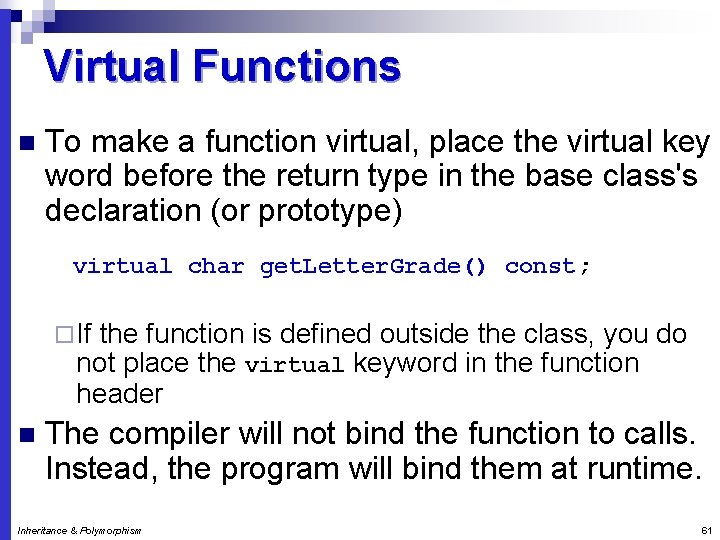
Virtual Functions n To make a function virtual, place the virtual key word before the return type in the base class's declaration (or prototype) virtual char get. Letter. Grade() const; ¨ If the function is defined outside the class, you do not place the virtual keyword in the function header n The compiler will not bind the function to calls. Instead, the program will bind them at runtime. Inheritance & Polymorphism 61
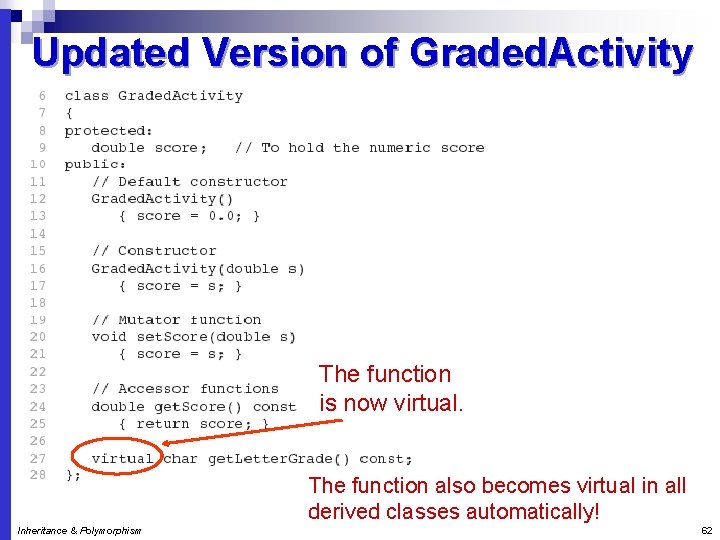
Updated Version of Graded. Activity The function is now virtual. The function also becomes virtual in all derived classes automatically! Inheritance & Polymorphism 62
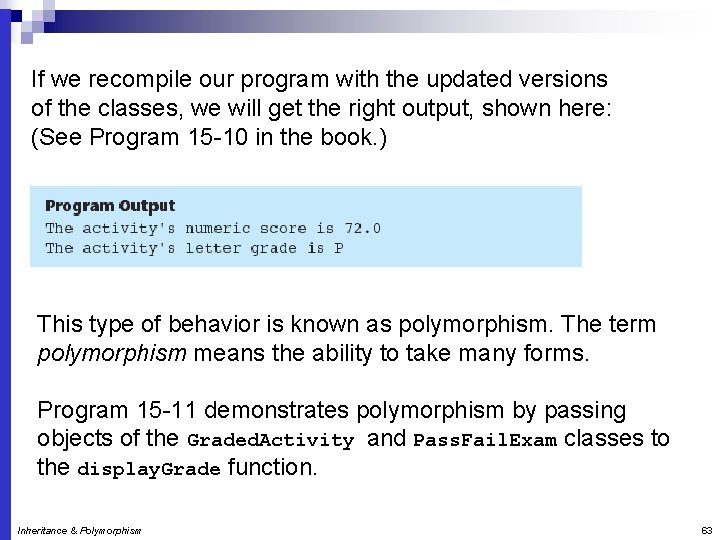
If we recompile our program with the updated versions of the classes, we will get the right output, shown here: (See Program 15 -10 in the book. ) This type of behavior is known as polymorphism. The term polymorphism means the ability to take many forms. Program 15 -11 demonstrates polymorphism by passing objects of the Graded. Activity and Pass. Fail. Exam classes to the display. Grade function. Inheritance & Polymorphism 63
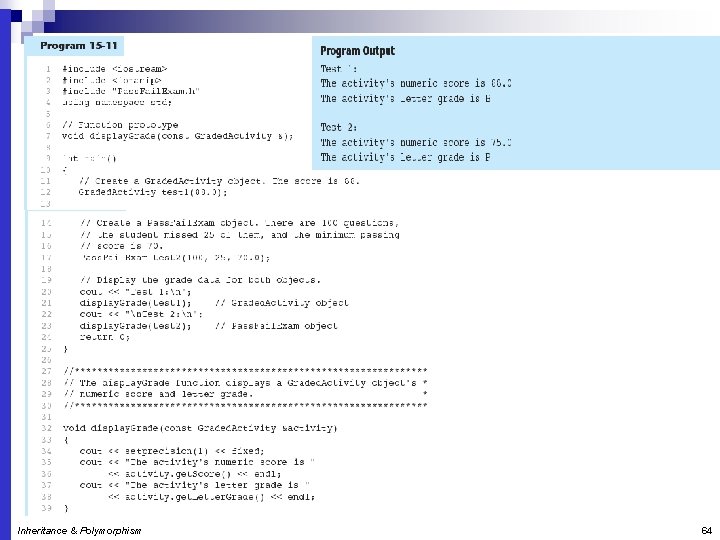
Inheritance & Polymorphism 64
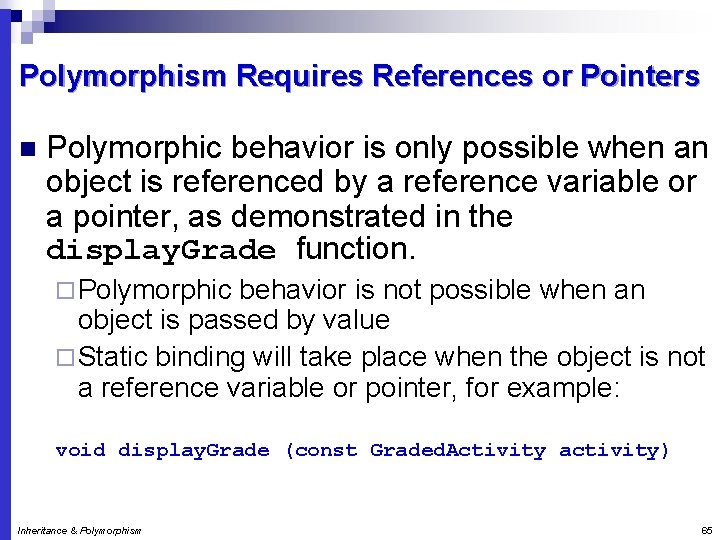
Polymorphism Requires References or Pointers n Polymorphic behavior is only possible when an object is referenced by a reference variable or a pointer, as demonstrated in the display. Grade function. ¨ Polymorphic behavior is not possible when an object is passed by value ¨ Static binding will take place when the object is not a reference variable or pointer, for example: void display. Grade (const Graded. Activity activity) Inheritance & Polymorphism 65
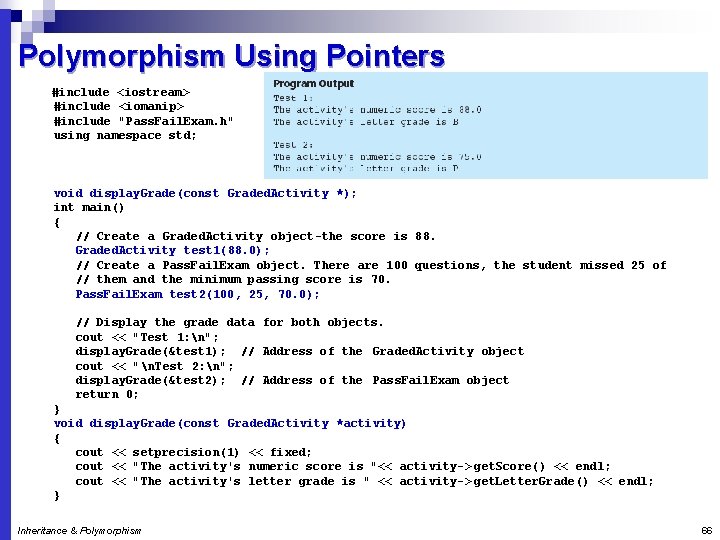
Polymorphism Using Pointers #include <iostream> #include <iomanip> #include "Pass. Fail. Exam. h" using namespace std; void display. Grade(const Graded. Activity *); int main() { // Create a Graded. Activity object-the score is 88. Graded. Activity test 1(88. 0); // Create a Pass. Fail. Exam object. There are 100 questions, the student missed 25 of // them and the minimum passing score is 70. Pass. Fail. Exam test 2(100, 25, 70. 0); // Display the grade data for both objects. cout << "Test 1: n"; display. Grade(&test 1); // Address of the Graded. Activity object cout << "n. Test 2: n"; display. Grade(&test 2); // Address of the Pass. Fail. Exam object return 0; } void display. Grade(const Graded. Activity *activity) { cout << setprecision(1) << fixed; cout << "The activity's numeric score is "<< activity-> get. Score() << endl; cout << "The activity's letter grade is " << activity-> get. Letter. Grade() << endl; } Inheritance & Polymorphism 66
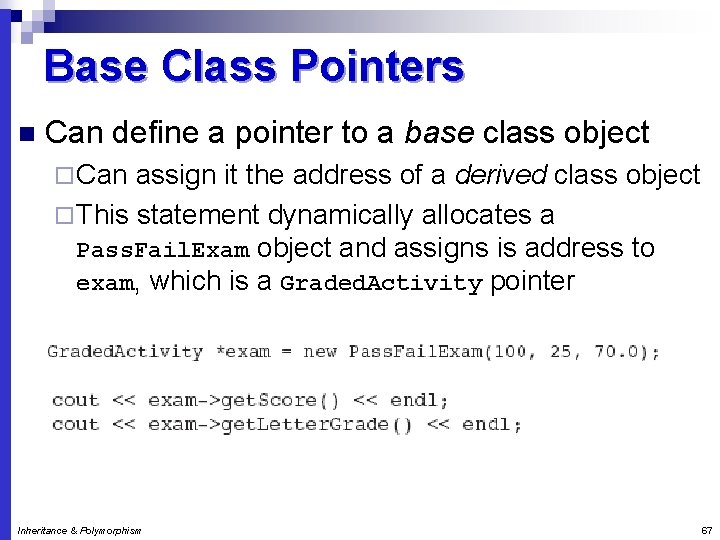
Base Class Pointers n Can define a pointer to a base class object ¨ Can assign it the address of a derived class object ¨ This statement dynamically allocates a Pass. Fail. Exam object and assigns is address to exam, which is a Graded. Activity pointer Inheritance & Polymorphism 67
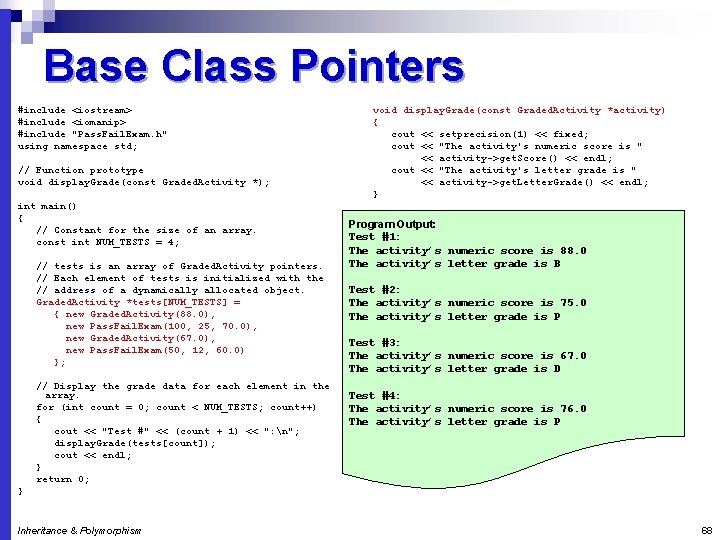
Base Class Pointers #include <iostream> #include <iomanip> #include "Pass. Fail. Exam. h" using namespace std; // Function prototype void display. Grade(const Graded. Activity *); int main() { // Constant for the size of an array. const int NUM_TESTS = 4; // tests is an array of Graded. Activity pointers. // Each element of tests is initialized with the // address of a dynamically allocated object. Graded. Activity *tests[NUM_TESTS] = { new Graded. Activity(88. 0), new Pass. Fail. Exam(100, 25, 70. 0), new Graded. Activity(67. 0), new Pass. Fail. Exam(50, 12, 60. 0) }; // Display the grade data for each element in the array. for (int count = 0; count < NUM_TESTS; count++) { cout << "Test #" << (count + 1) << ": n"; display. Grade(tests[count]); cout << endl; } return 0; void display. Grade(const Graded. Activity *activity) { cout << setprecision(1) << fixed; cout << "The activity's numeric score is " << activity->get. Score() << endl; cout << "The activity's letter grade is " << activity->get. Letter. Grade() << endl; } Program Output: Test #1: The activity’s numeric score is 88. 0 The activity’s letter grade is B Test #2: The activity’s numeric score is 75. 0 The activity’s letter grade is P Test #3: The activity’s numeric score is 67. 0 The activity’s letter grade is D Test #4: The activity’s numeric score is 76. 0 The activity’s letter grade is P } Inheritance & Polymorphism 68
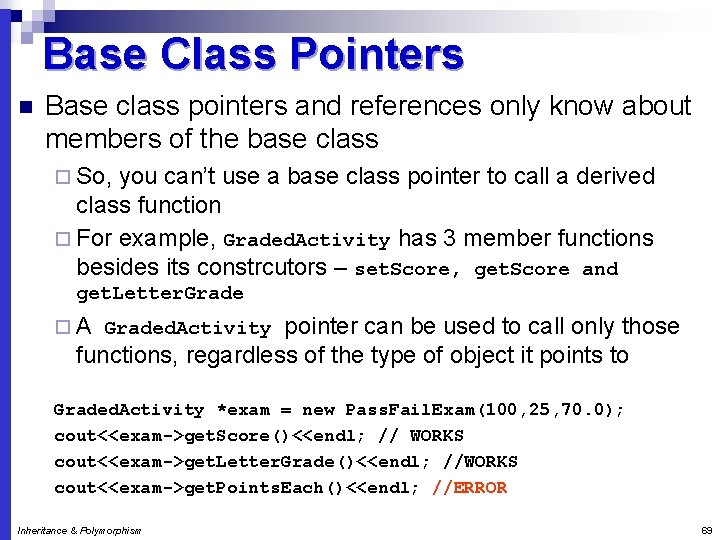
Base Class Pointers n Base class pointers and references only know about members of the base class ¨ So, you can’t use a base class pointer to call a derived class function ¨ For example, Graded. Activity has 3 member functions besides its constrcutors – set. Score, get. Score and get. Letter. Grade ¨ A Graded. Activity pointer can be used to call only those functions, regardless of the type of object it points to Graded. Activity *exam = new Pass. Fail. Exam(100, 25, 70. 0); cout<<exam->get. Score()<<endl; // WORKS cout<<exam->get. Letter. Grade()<<endl; //WORKS cout<<exam->get. Points. Each()<<endl; //ERROR Inheritance & Polymorphism 69
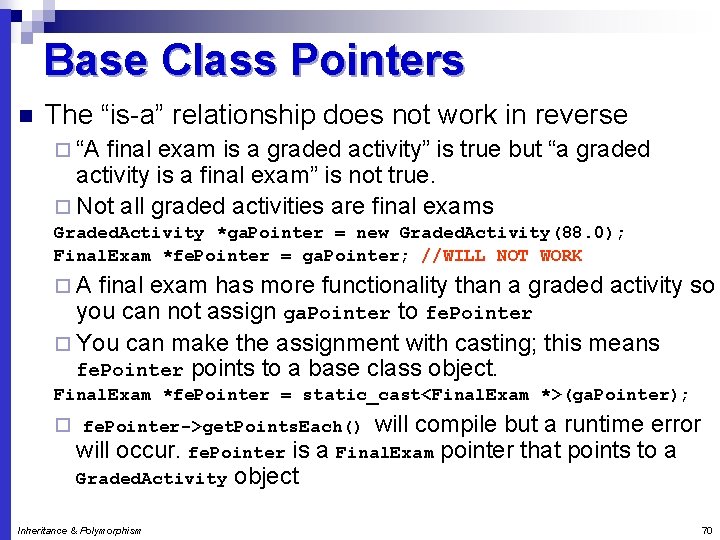
Base Class Pointers n The “is-a” relationship does not work in reverse ¨ “A final exam is a graded activity” is true but “a graded activity is a final exam” is not true. ¨ Not all graded activities are final exams Graded. Activity *ga. Pointer = new Graded. Activity(88. 0); Final. Exam *fe. Pointer = ga. Pointer; //WILL NOT WORK ¨A final exam has more functionality than a graded activity so you can not assign ga. Pointer to fe. Pointer ¨ You can make the assignment with casting; this means fe. Pointer points to a base class object. Final. Exam *fe. Pointer = static_cast<Final. Exam *>(ga. Pointer); will compile but a runtime error will occur. fe. Pointer is a Final. Exam pointer that points to a Graded. Activity object ¨ fe. Pointer->get. Points. Each() Inheritance & Polymorphism 70
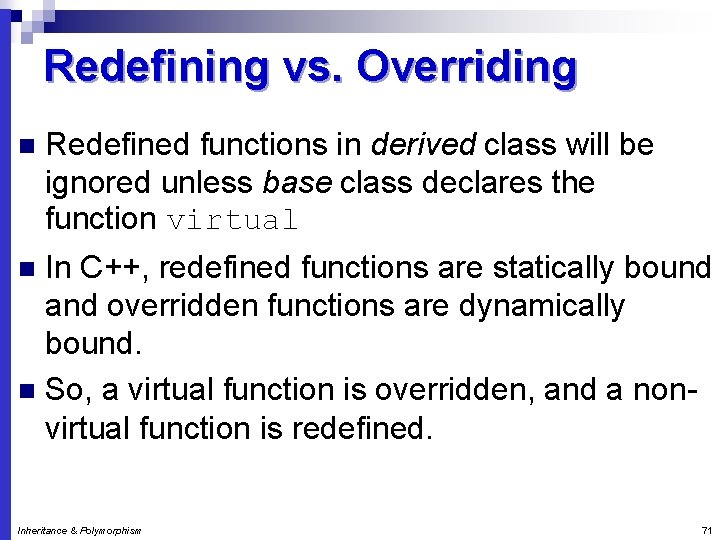
Redefining vs. Overriding n Redefined functions in derived class will be ignored unless base class declares the function virtual In C++, redefined functions are statically bound and overridden functions are dynamically bound. n So, a virtual function is overridden, and a nonvirtual function is redefined. n Inheritance & Polymorphism 71
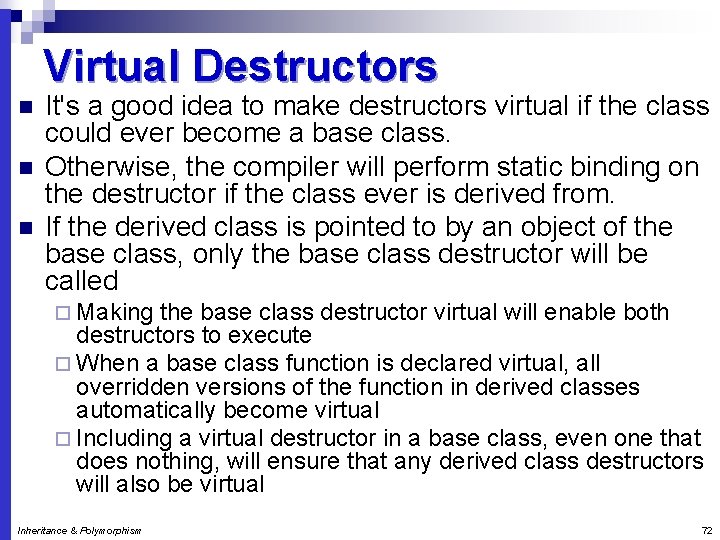
Virtual Destructors n n n It's a good idea to make destructors virtual if the class could ever become a base class. Otherwise, the compiler will perform static binding on the destructor if the class ever is derived from. If the derived class is pointed to by an object of the base class, only the base class destructor will be called ¨ Making the base class destructor virtual will enable both destructors to execute ¨ When a base class function is declared virtual, all overridden versions of the function in derived classes automatically become virtual ¨ Including a virtual destructor in a base class, even one that does nothing, will ensure that any derived class destructors will also be virtual Inheritance & Polymorphism 72
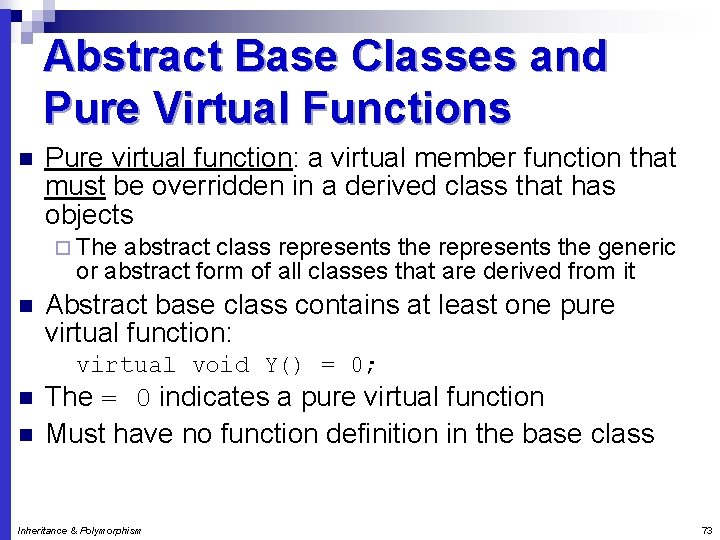
Abstract Base Classes and Pure Virtual Functions n Pure virtual function: a virtual member function that must be overridden in a derived class that has objects ¨ The abstract class represents the generic or abstract form of all classes that are derived from it n Abstract base class contains at least one pure virtual function: virtual void Y() = 0; n n The = 0 indicates a pure virtual function Must have no function definition in the base class Inheritance & Polymorphism 73
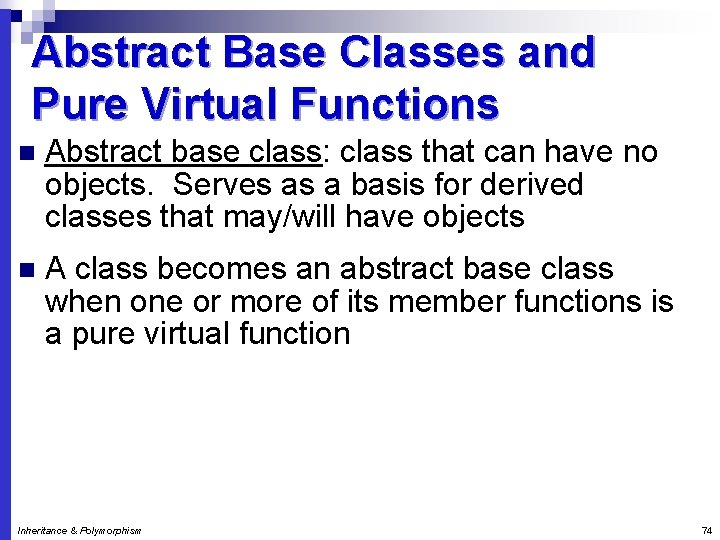
Abstract Base Classes and Pure Virtual Functions n Abstract base class: class that can have no objects. Serves as a basis for derived classes that may/will have objects n A class becomes an abstract base class when one or more of its member functions is a pure virtual function Inheritance & Polymorphism 74
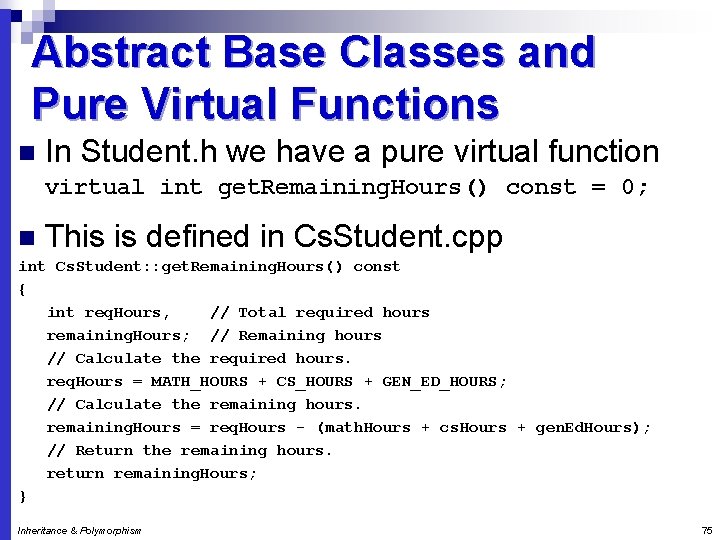
Abstract Base Classes and Pure Virtual Functions n In Student. h we have a pure virtual function virtual int get. Remaining. Hours() const = 0; n This is defined in Cs. Student. cpp int Cs. Student: : get. Remaining. Hours() const { int req. Hours, // Total required hours remaining. Hours; // Remaining hours // Calculate the required hours. req. Hours = MATH_HOURS + CS_HOURS + GEN_ED_HOURS; // Calculate the remaining hours. remaining. Hours = req. Hours - (math. Hours + cs. Hours + gen. Ed. Hours); // Return the remaining hours. return remaining. Hours; } Inheritance & Polymorphism 75
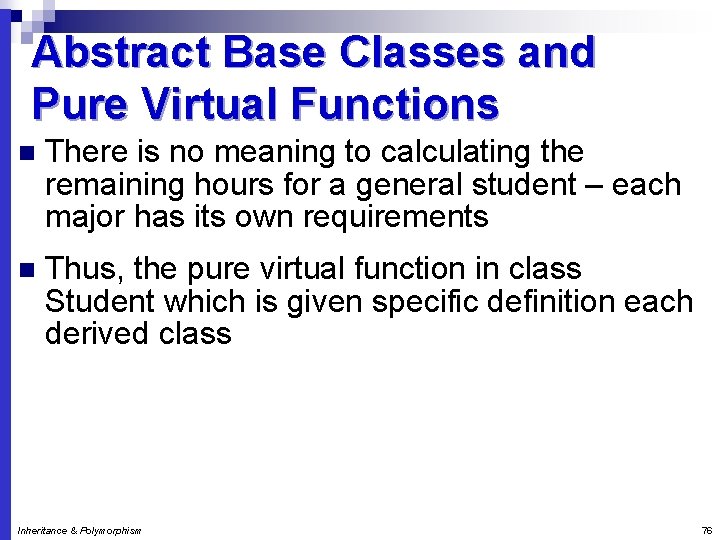
Abstract Base Classes and Pure Virtual Functions n There is no meaning to calculating the remaining hours for a general student – each major has its own requirements n Thus, the pure virtual function in class Student which is given specific definition each derived class Inheritance & Polymorphism 76
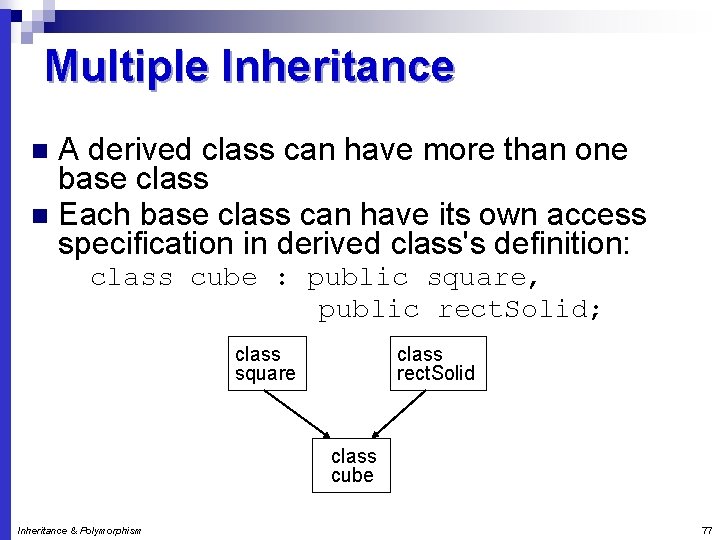
Multiple Inheritance A derived class can have more than one base class n Each base class can have its own access specification in derived class's definition: n class cube : public square, public rect. Solid; class square class rect. Solid class cube Inheritance & Polymorphism 77
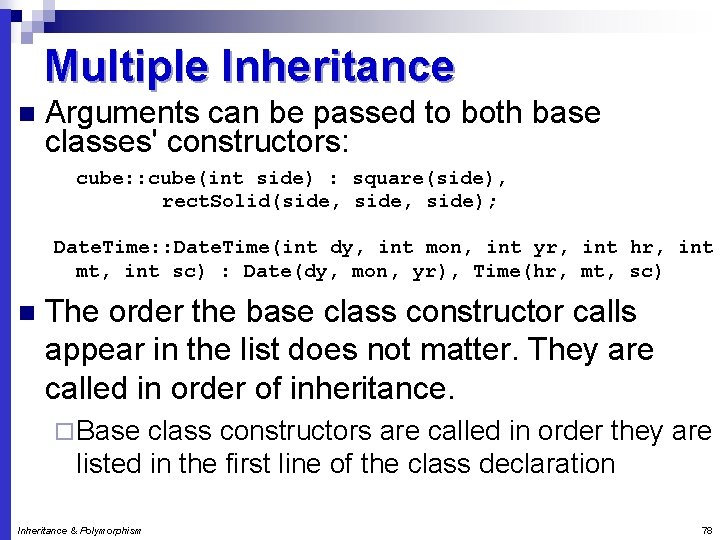
Multiple Inheritance n Arguments can be passed to both base classes' constructors: cube: : cube(int side) : square(side), rect. Solid(side, side); Date. Time: : Date. Time(int dy, int mon, int yr, int hr, int mt, int sc) : Date(dy, mon, yr), Time(hr, mt, sc) n The order the base class constructor calls appear in the list does not matter. They are called in order of inheritance. ¨ Base class constructors are called in order they are listed in the first line of the class declaration Inheritance & Polymorphism 78
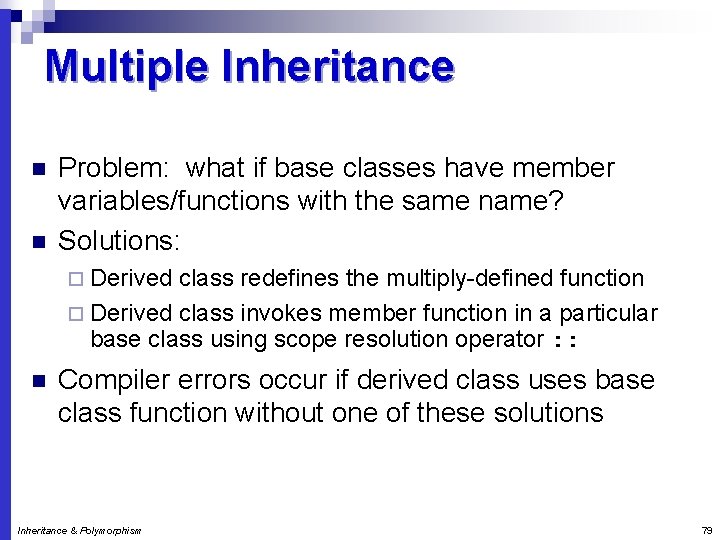
Multiple Inheritance n n Problem: what if base classes have member variables/functions with the same name? Solutions: ¨ Derived class redefines the multiply-defined function ¨ Derived class invokes member function in a particular base class using scope resolution operator : : n Compiler errors occur if derived class uses base class function without one of these solutions Inheritance & Polymorphism 79