Inheritance n Inheritance is a natural way to
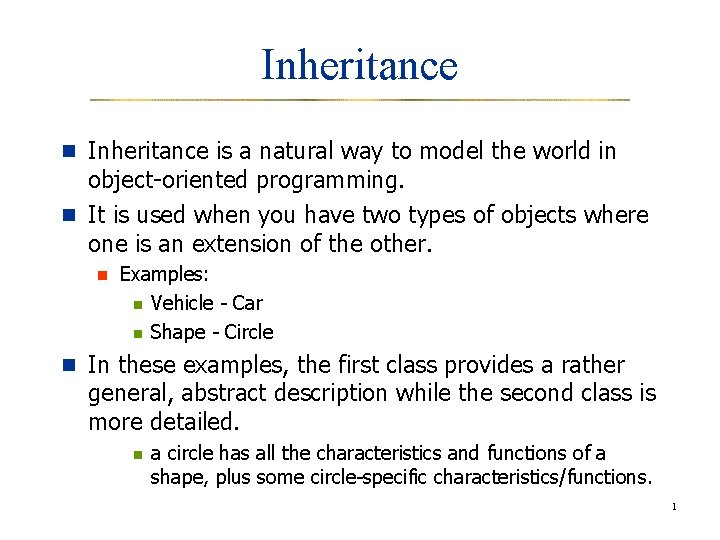
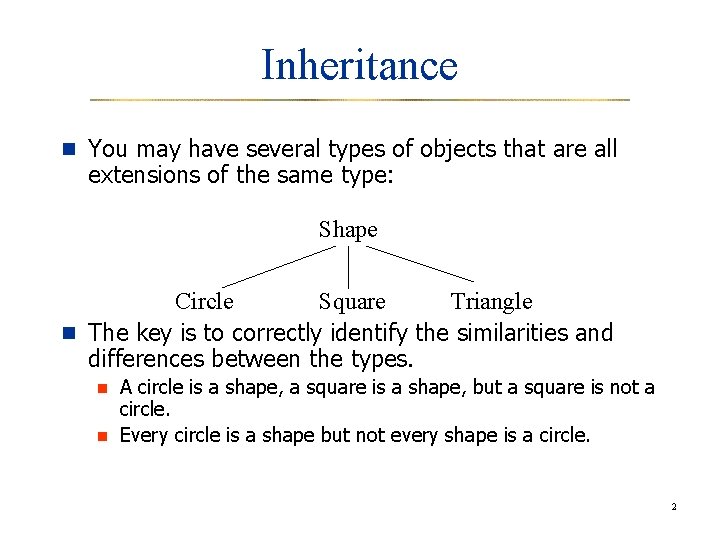
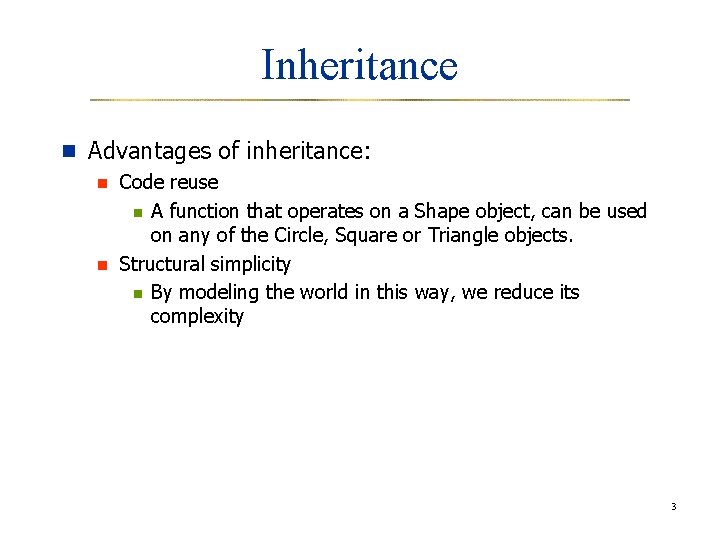
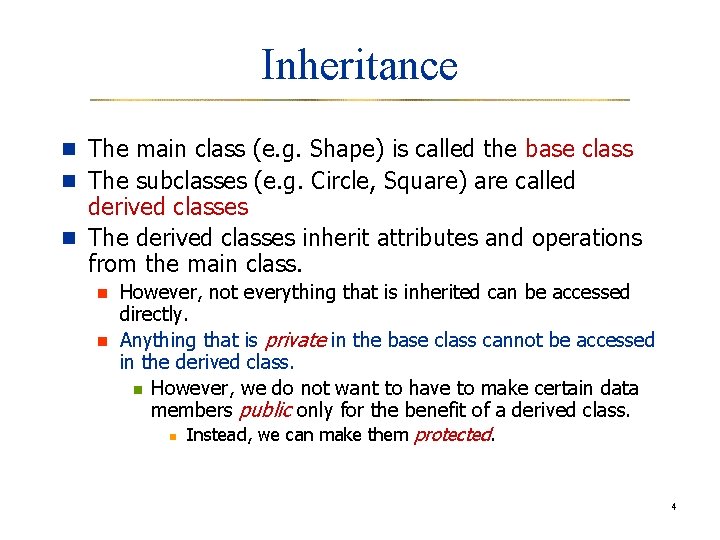
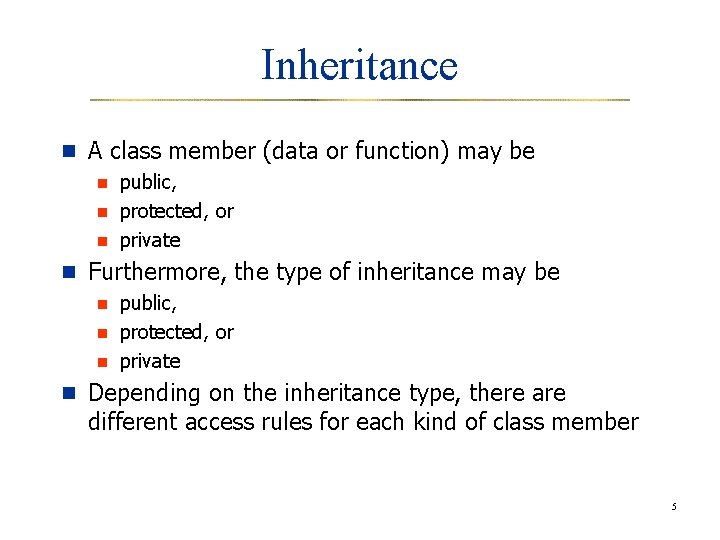
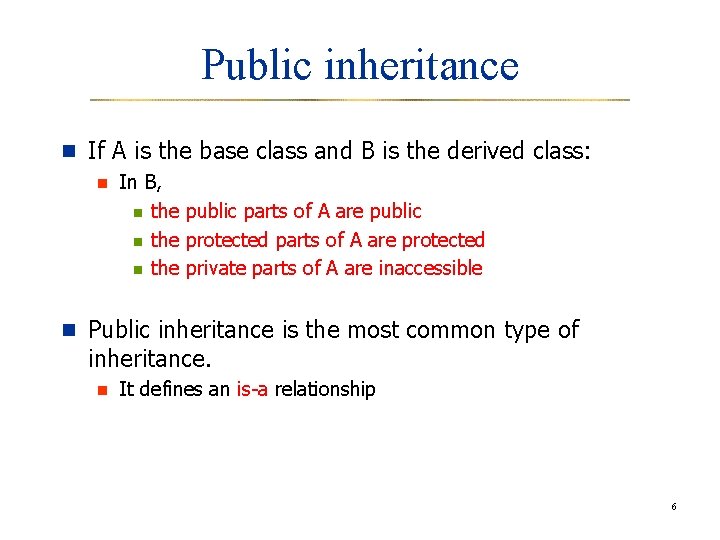
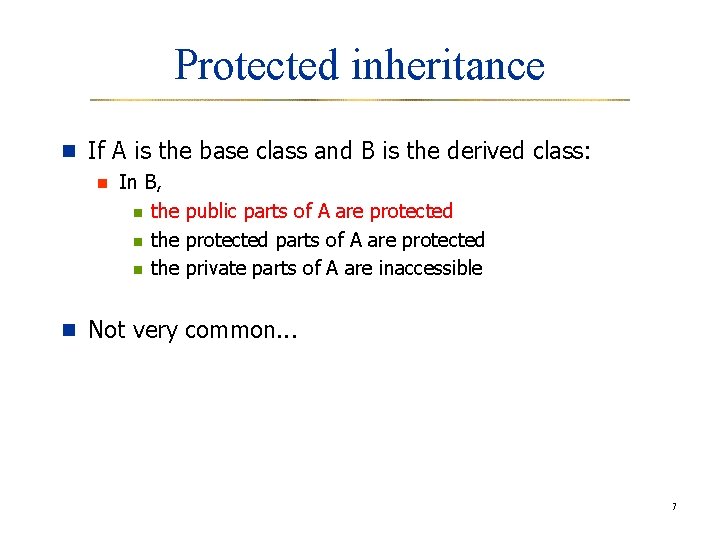
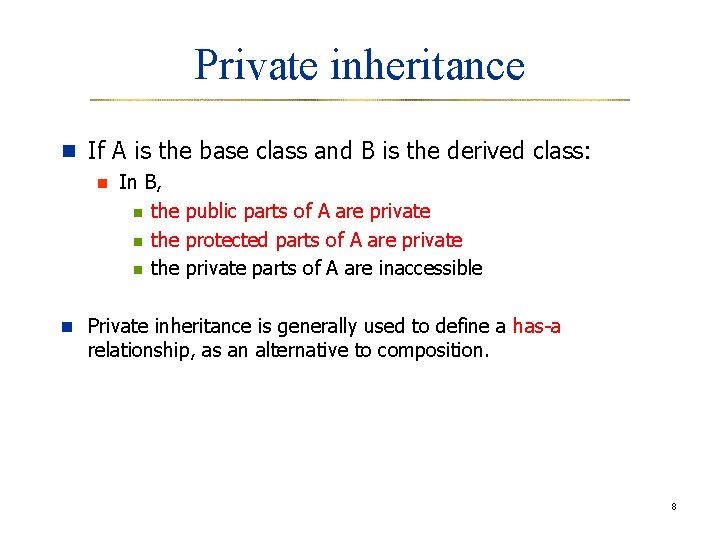
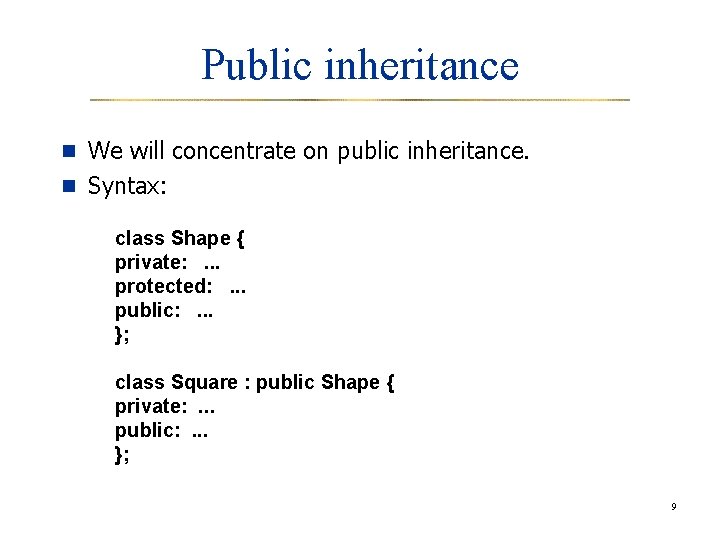
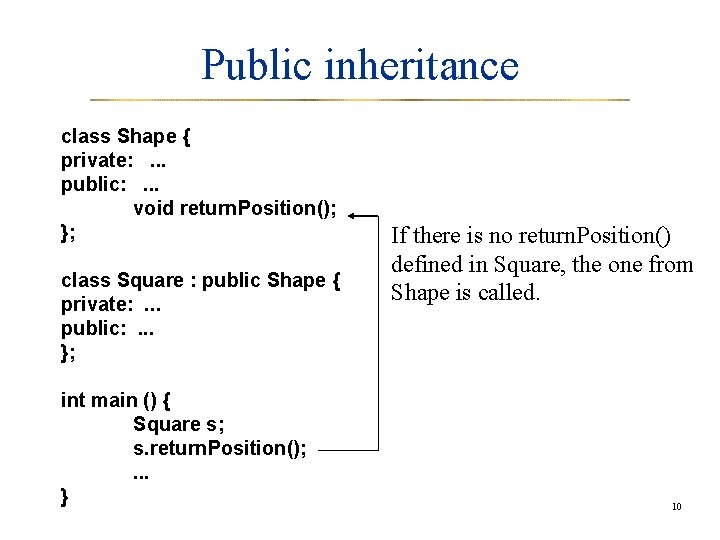
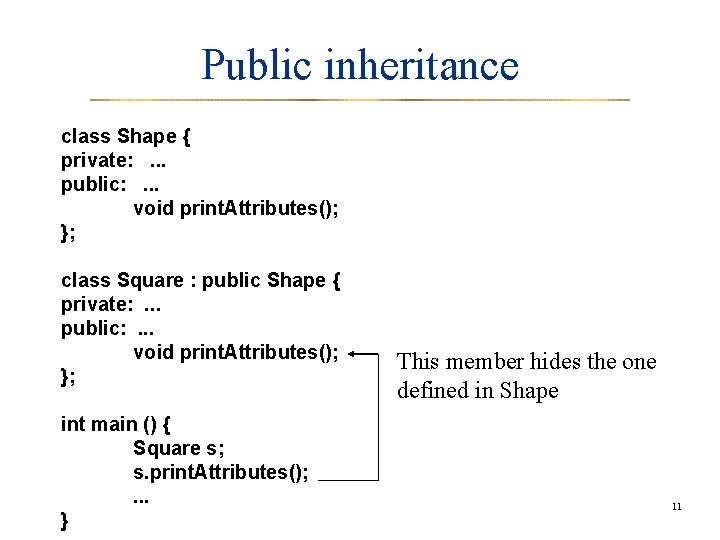
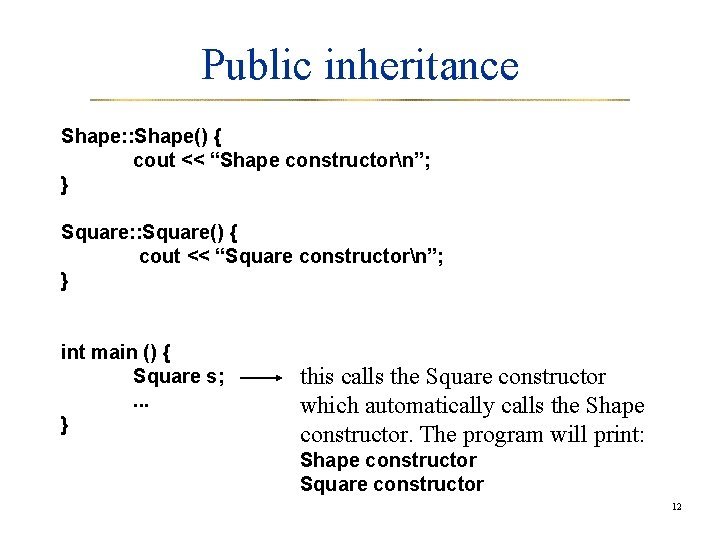
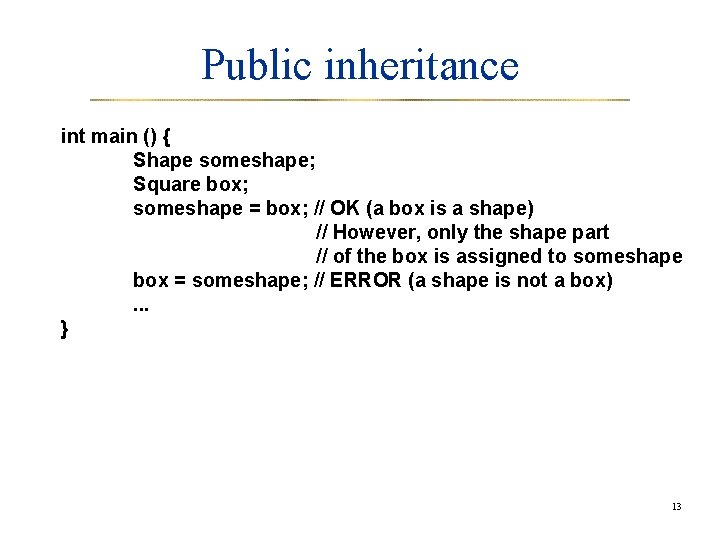
- Slides: 13
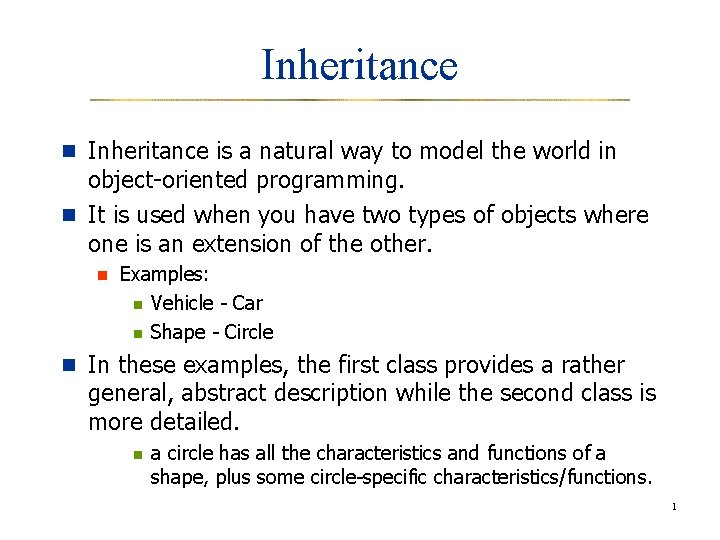
Inheritance n Inheritance is a natural way to model the world in object-oriented programming. n It is used when you have two types of objects where one is an extension of the other. n Examples: n Vehicle - Car n Shape - Circle n In these examples, the first class provides a rather general, abstract description while the second class is more detailed. n a circle has all the characteristics and functions of a shape, plus some circle-specific characteristics/functions. 1
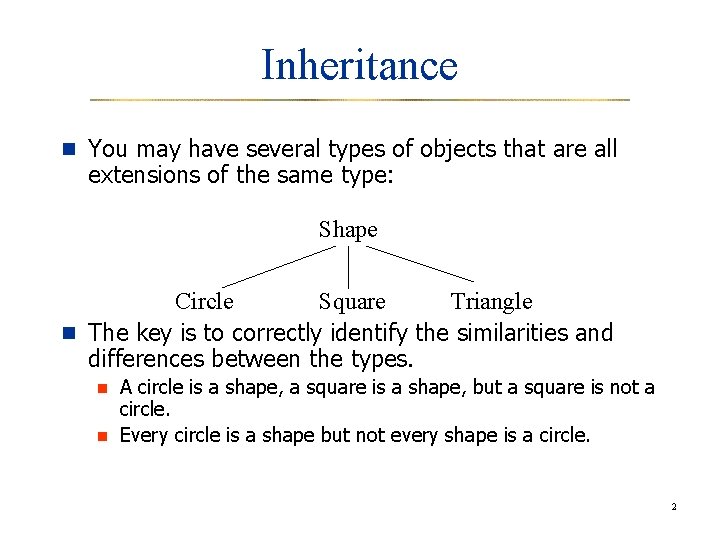
Inheritance n You may have several types of objects that are all extensions of the same type: Shape Circle Square Triangle n The key is to correctly identify the similarities and differences between the types. n n A circle is a shape, a square is a shape, but a square is not a circle. Every circle is a shape but not every shape is a circle. 2
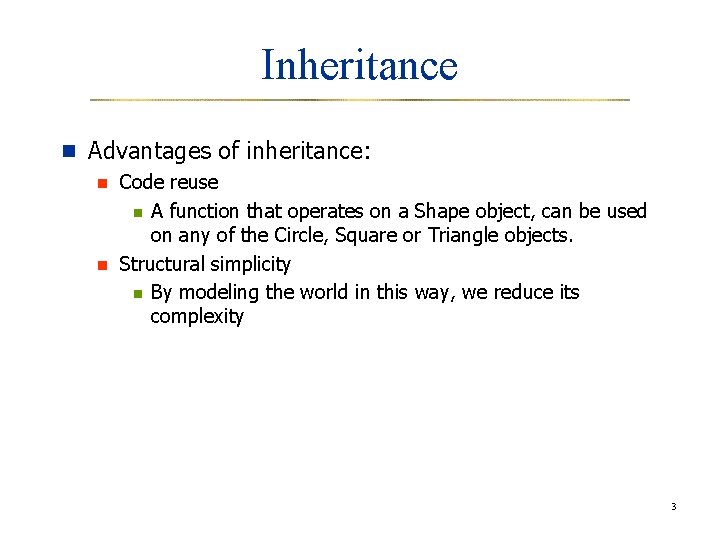
Inheritance n Advantages of inheritance: n Code reuse n A function that operates on a Shape object, can be used on any of the Circle, Square or Triangle objects. n Structural simplicity n By modeling the world in this way, we reduce its complexity 3
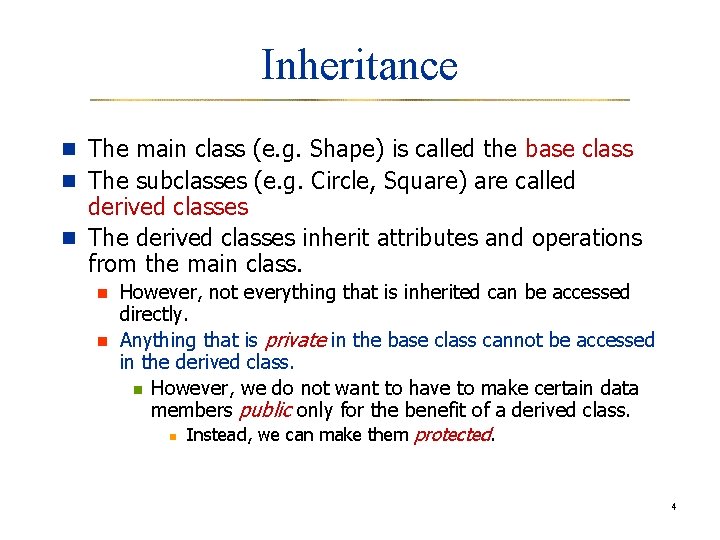
Inheritance n The main class (e. g. Shape) is called the base class n The subclasses (e. g. Circle, Square) are called derived classes n The derived classes inherit attributes and operations from the main class. n n However, not everything that is inherited can be accessed directly. Anything that is private in the base class cannot be accessed in the derived class. n However, we do not want to have to make certain data members public only for the benefit of a derived class. n Instead, we can make them protected. 4
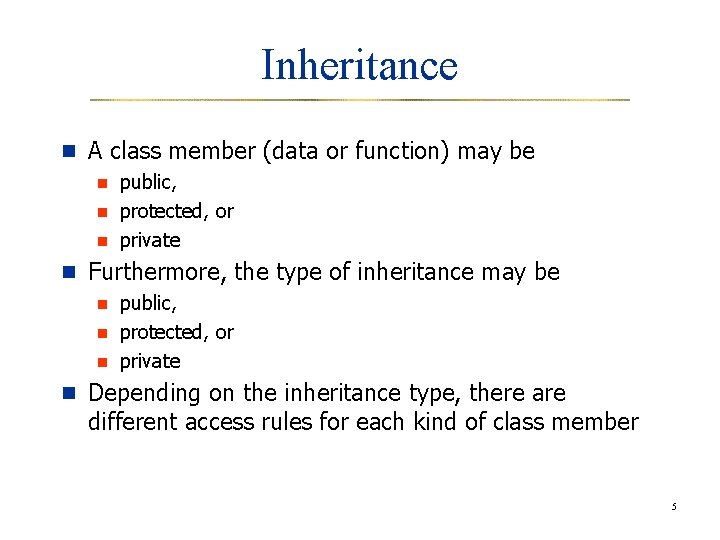
Inheritance n A class member (data or function) may be n public, n protected, or n private n Furthermore, the type of inheritance may be n public, n protected, or n private n Depending on the inheritance type, there are different access rules for each kind of class member 5
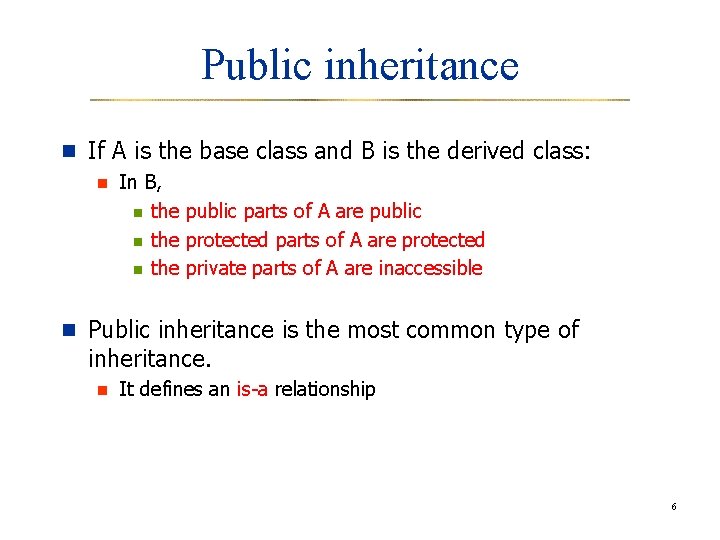
Public inheritance n If A is the base class and B is the derived class: n In B, n the public parts of A are public n the protected parts of A are protected n the private parts of A are inaccessible n Public inheritance is the most common type of inheritance. n It defines an is-a relationship 6
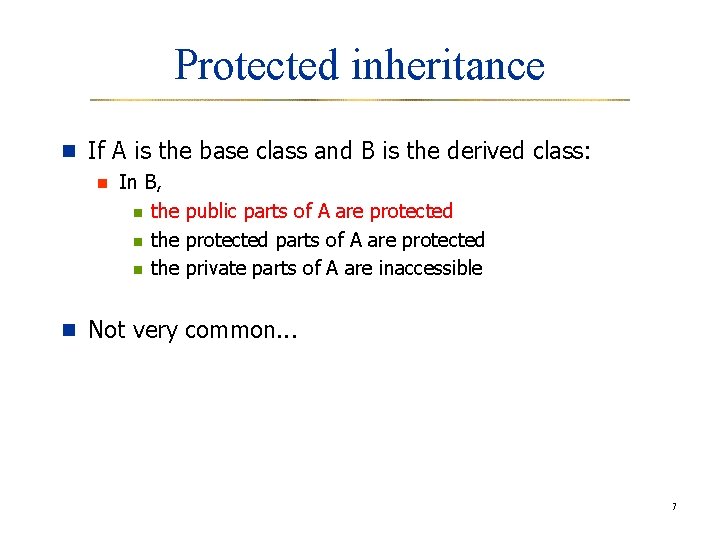
Protected inheritance n If A is the base class and B is the derived class: n In B, n the public parts of A are protected n the protected parts of A are protected n the private parts of A are inaccessible n Not very common. . . 7
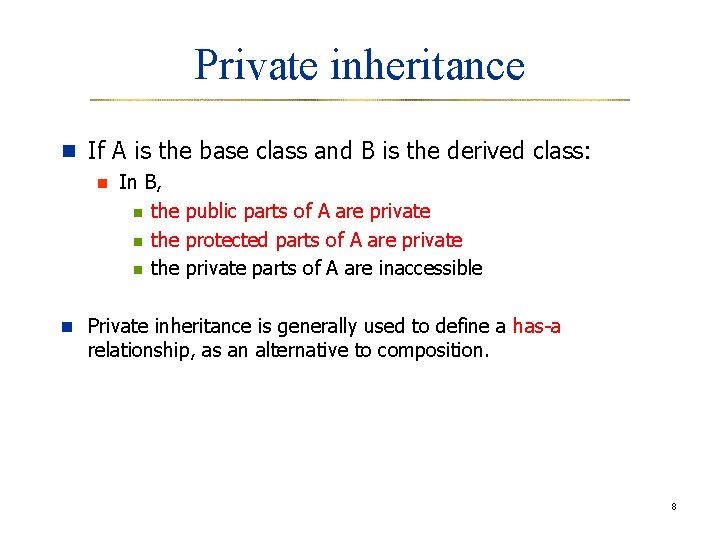
Private inheritance n If A is the base class and B is the derived class: n In B, n the public parts of A are private n the protected parts of A are private n the private parts of A are inaccessible n Private inheritance is generally used to define a has-a relationship, as an alternative to composition. 8
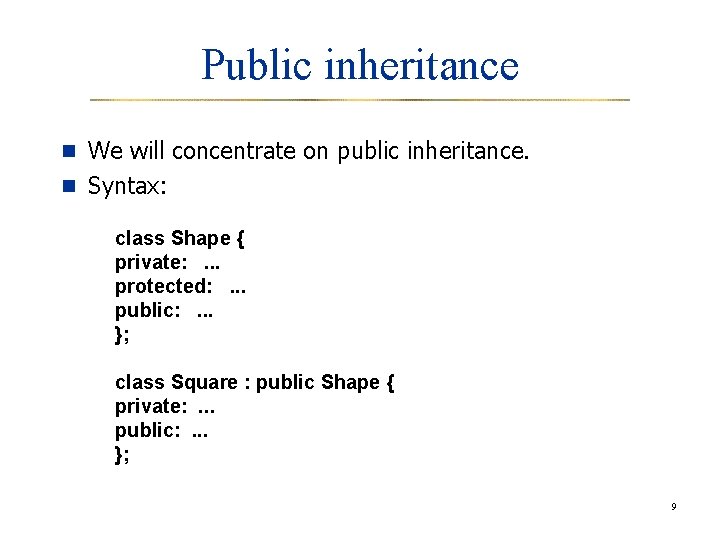
Public inheritance n We will concentrate on public inheritance. n Syntax: class Shape { private: . . . protected: . . . public: . . . }; class Square : public Shape { private: . . . public: . . . }; 9
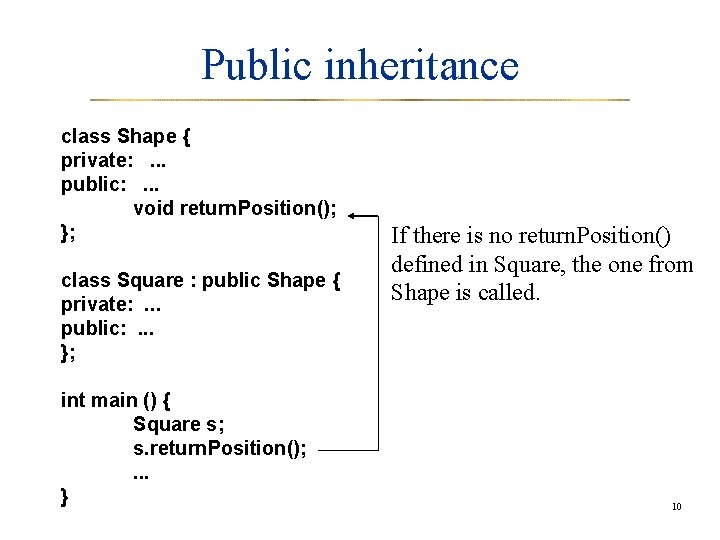
Public inheritance class Shape { private: . . . public: . . . void return. Position(); }; class Square : public Shape { private: . . . public: . . . }; int main () { Square s; s. return. Position(); . . . } If there is no return. Position() defined in Square, the one from Shape is called. 10
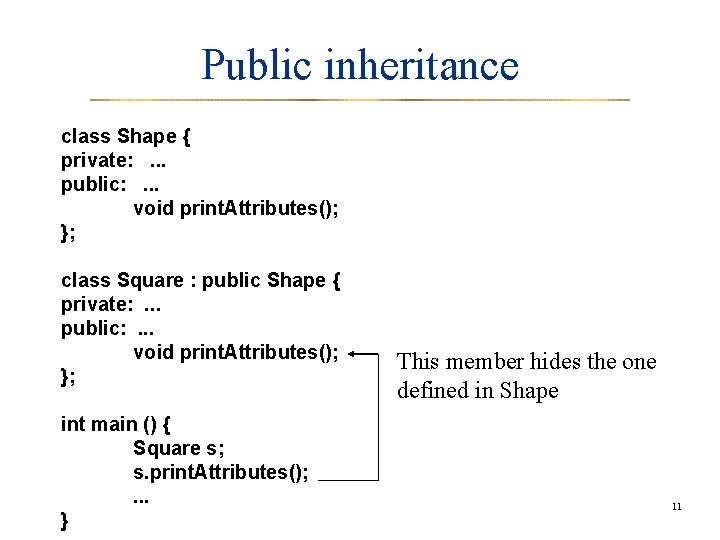
Public inheritance class Shape { private: . . . public: . . . void print. Attributes(); }; class Square : public Shape { private: . . . public: . . . void print. Attributes(); }; int main () { Square s; s. print. Attributes(); . . . } This member hides the one defined in Shape 11
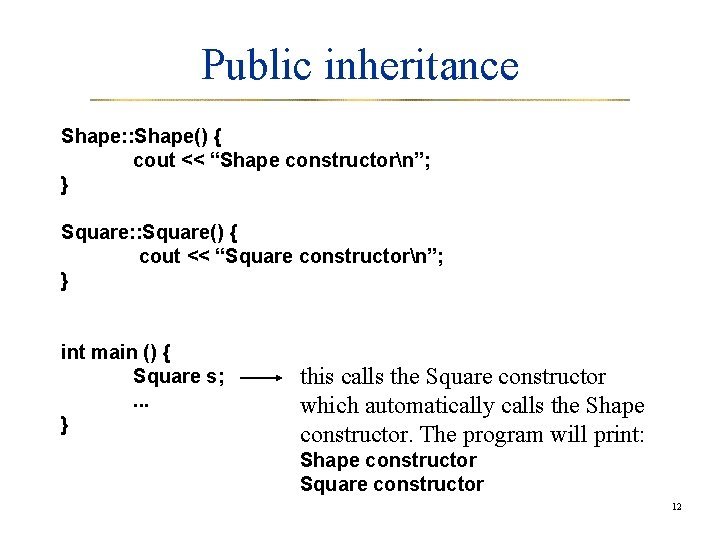
Public inheritance Shape: : Shape() { cout << “Shape constructorn”; } Square: : Square() { cout << “Square constructorn”; } int main () { Square s; . . . } this calls the Square constructor which automatically calls the Shape constructor. The program will print: Shape constructor Square constructor 12
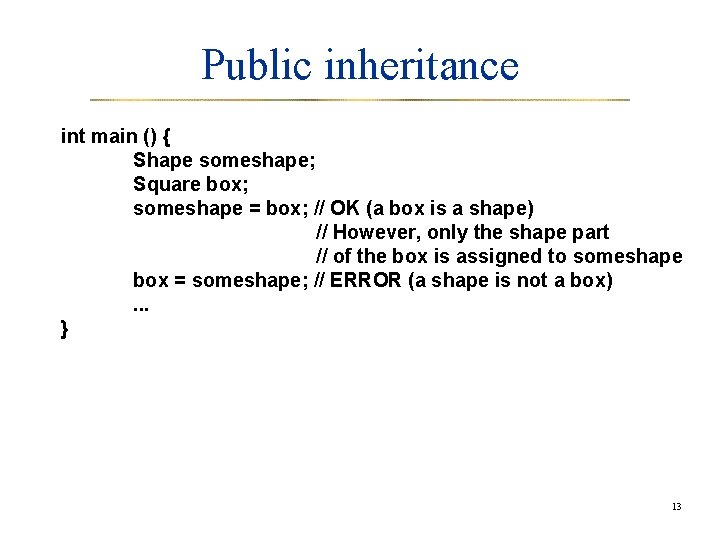
Public inheritance int main () { Shape someshape; Square box; someshape = box; // OK (a box is a shape) // However, only the shape part // of the box is assigned to someshape box = someshape; // ERROR (a shape is not a box). . . } 13