include stdio h float add float a float
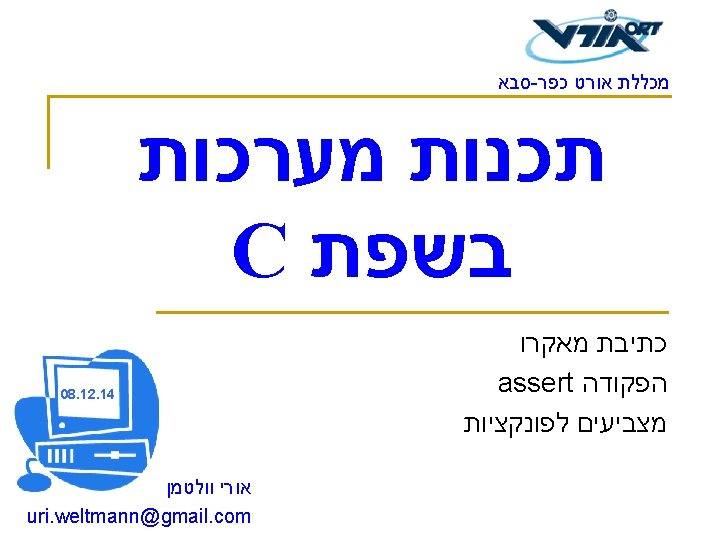
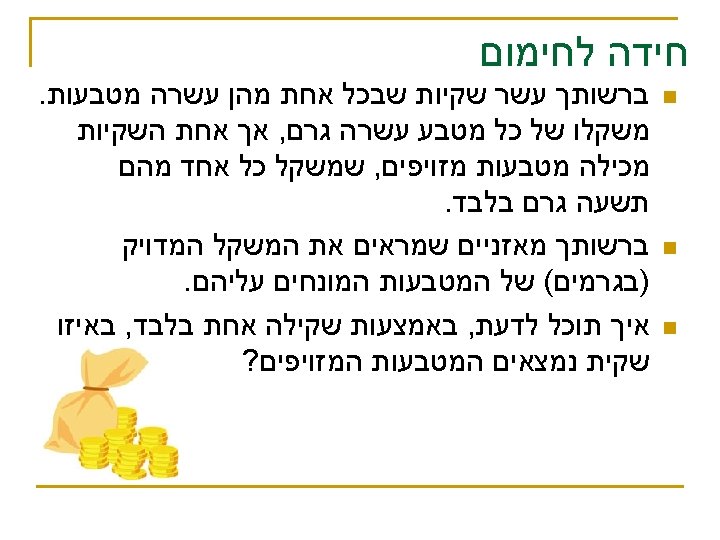
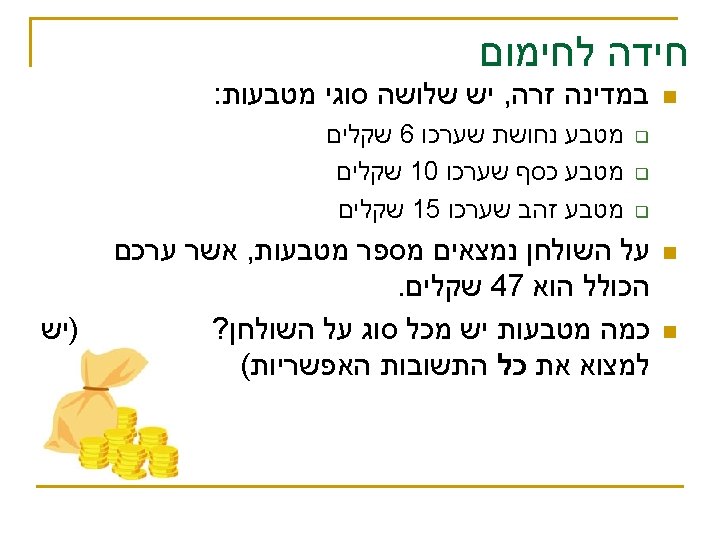
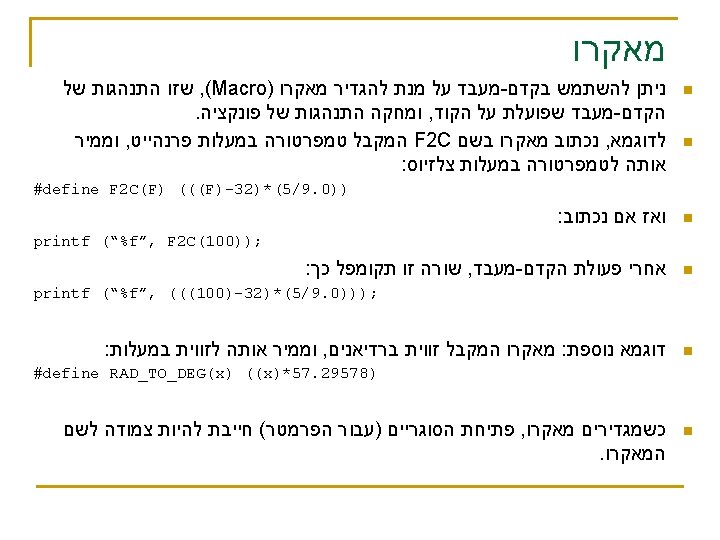
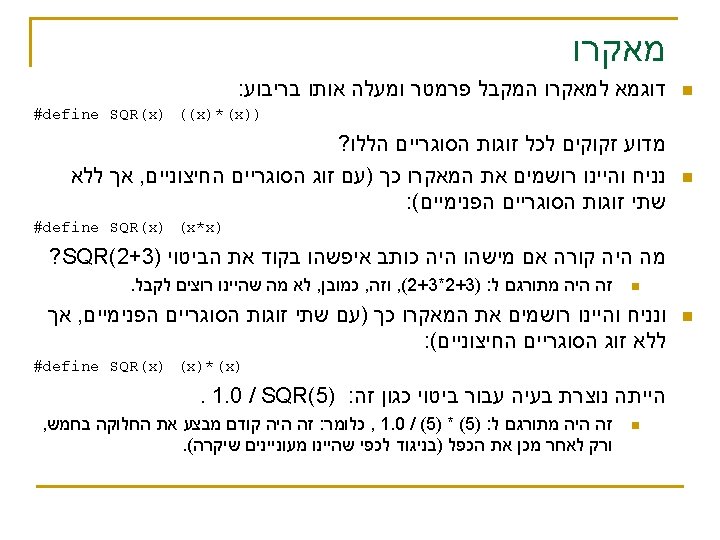
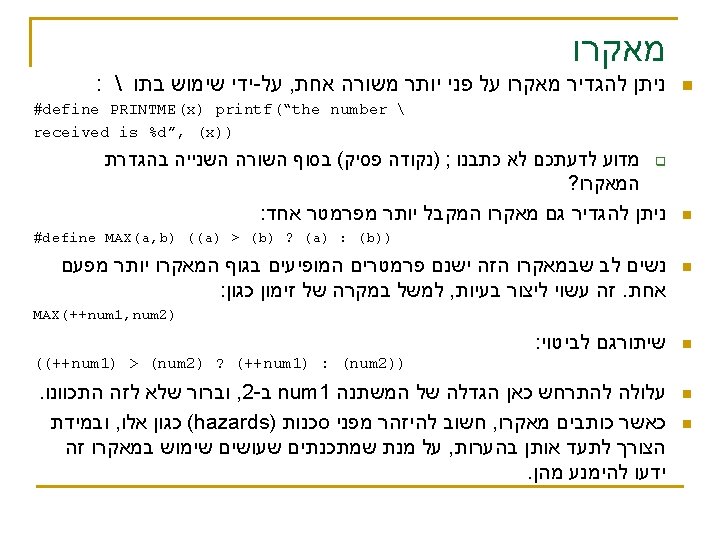
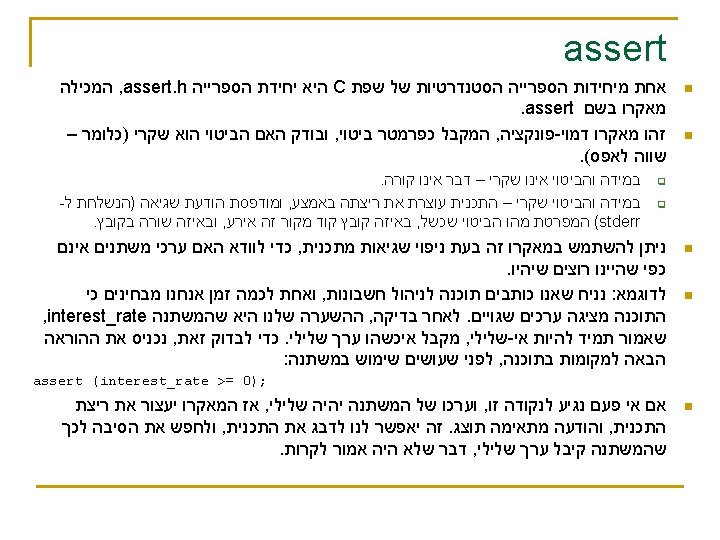
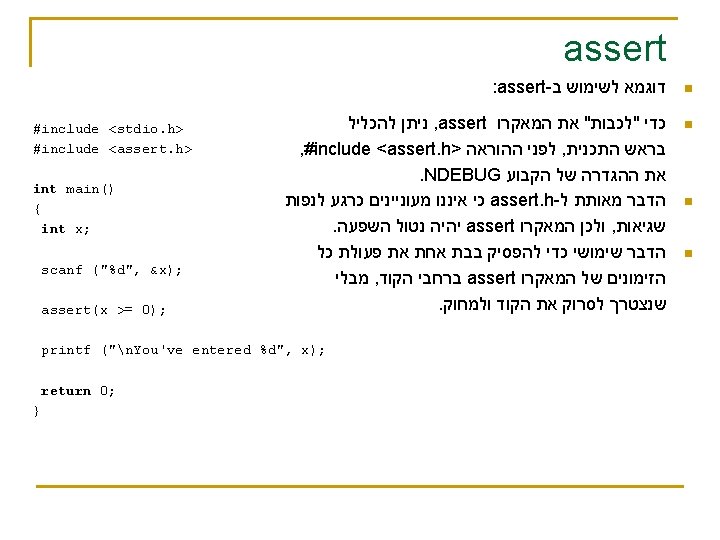
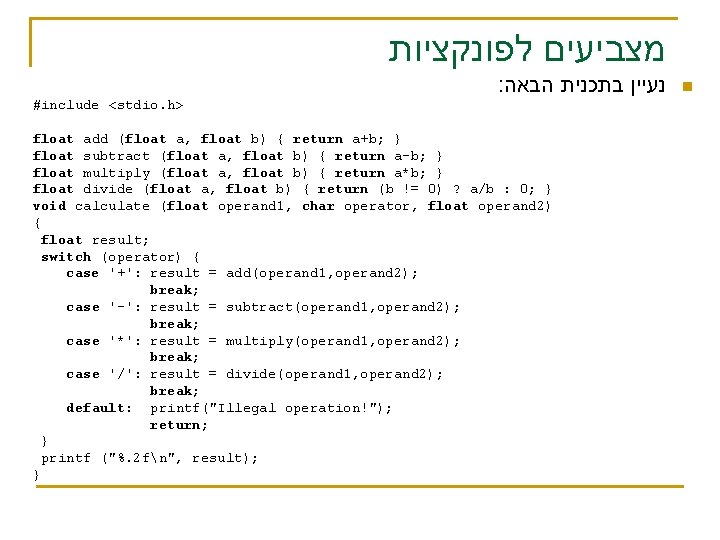
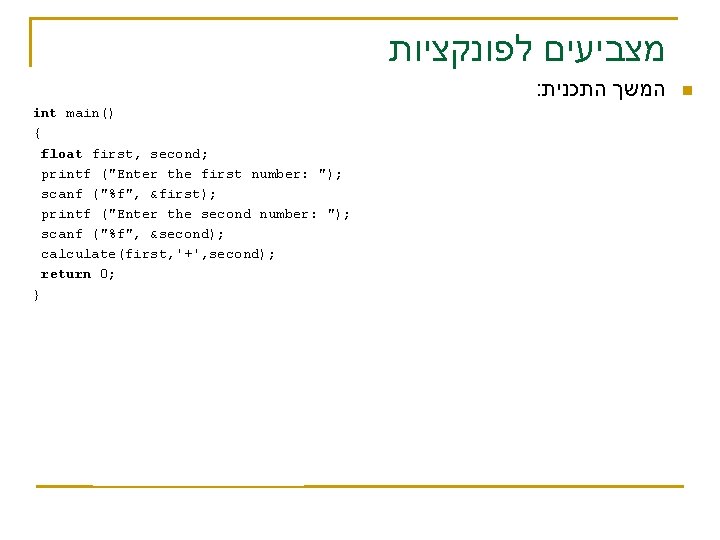
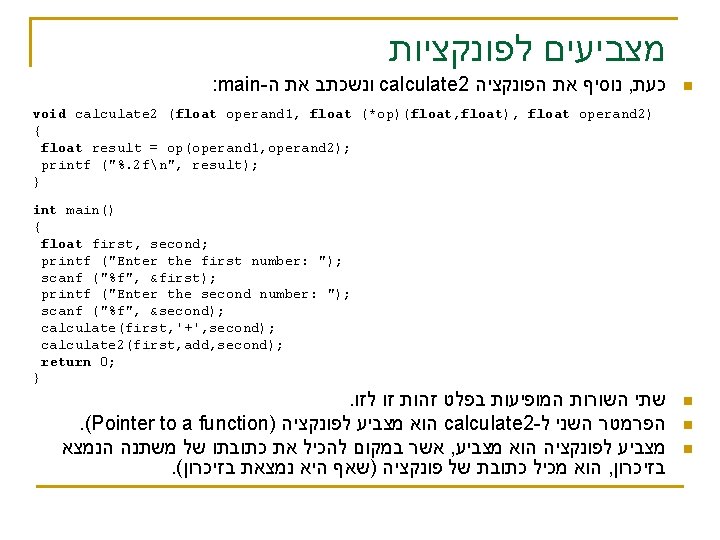
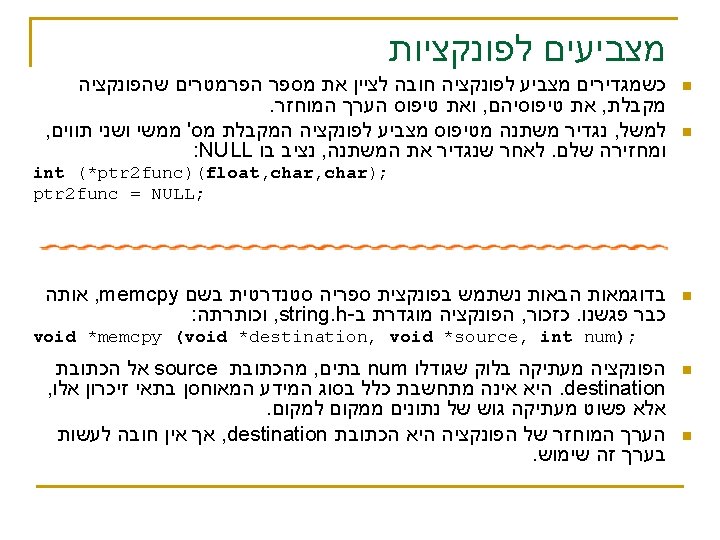
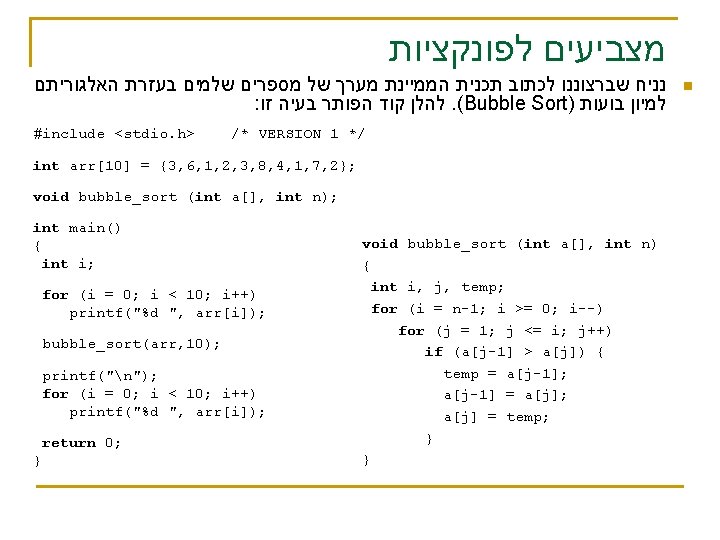
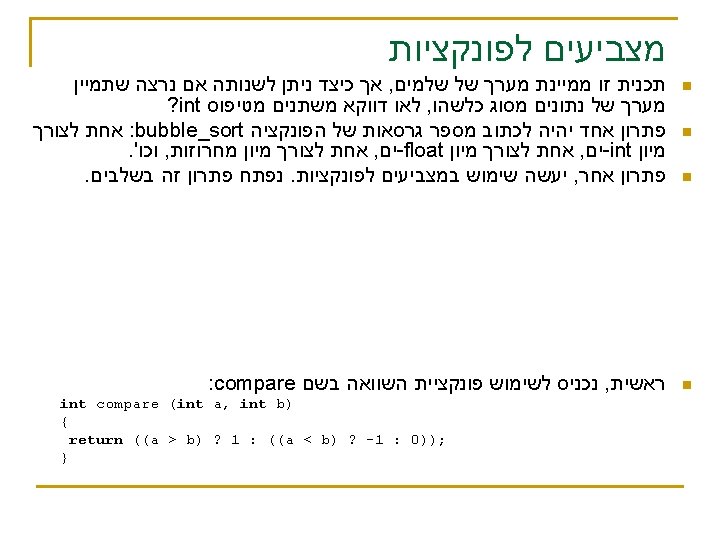
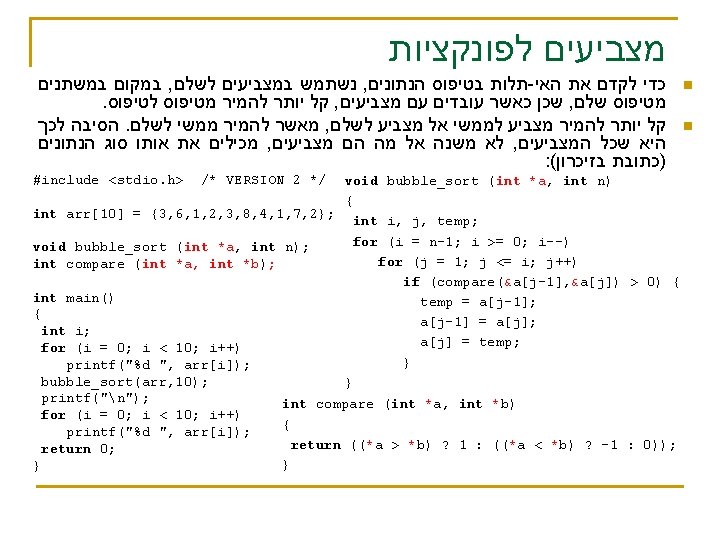
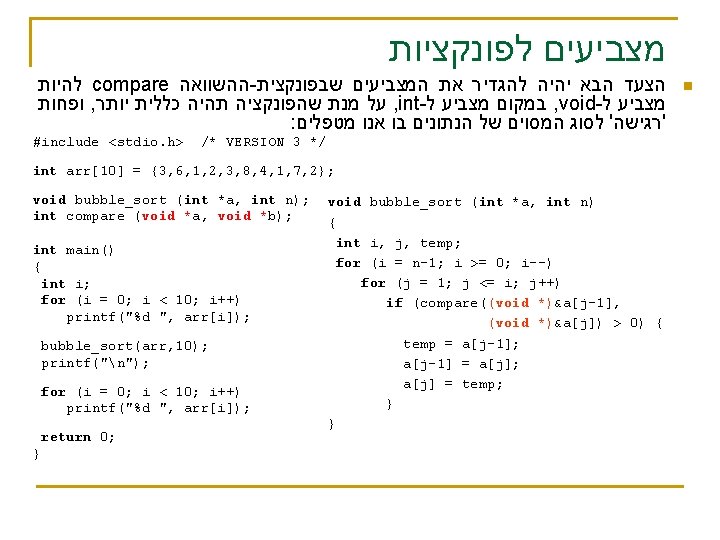
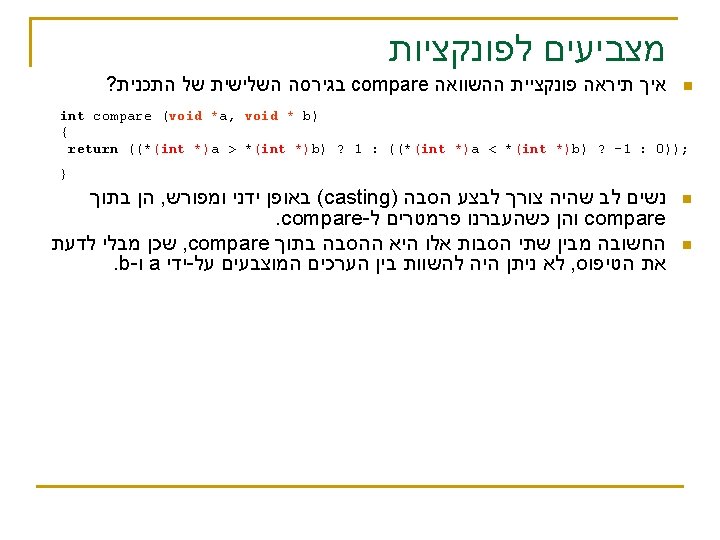
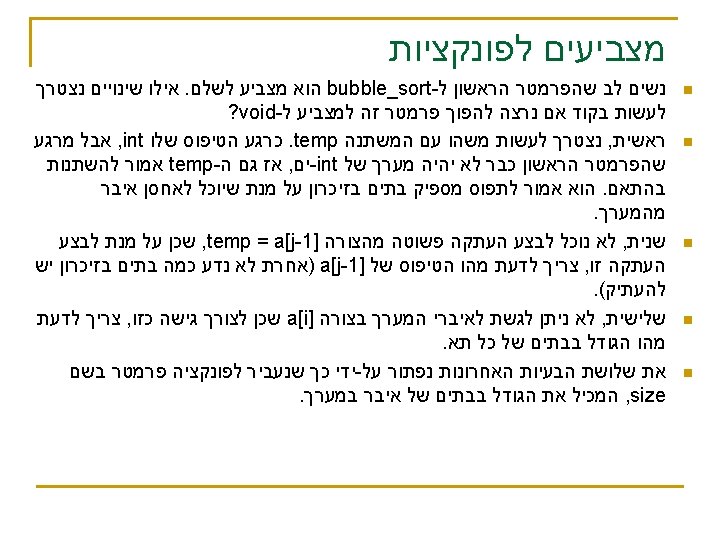
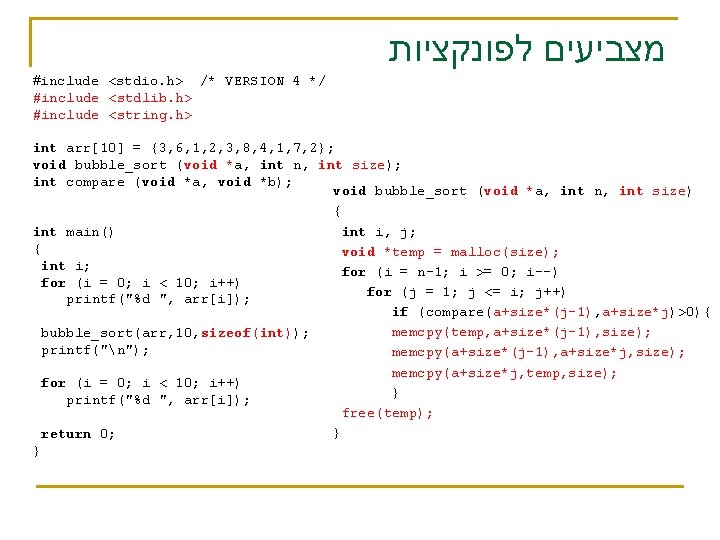
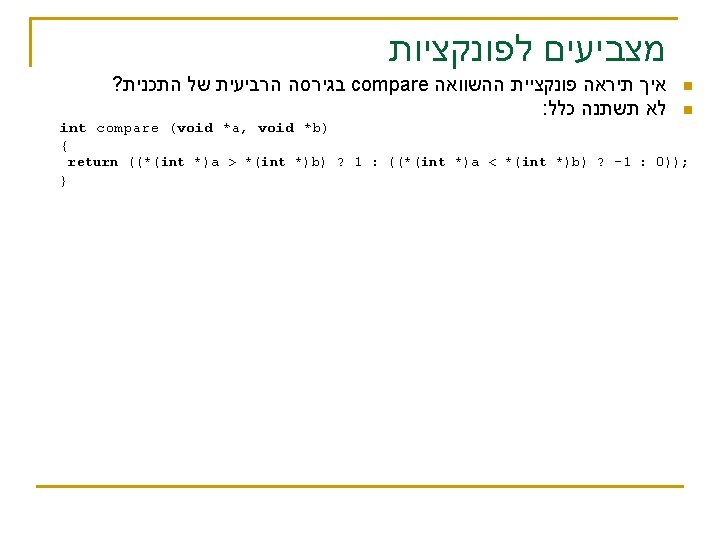
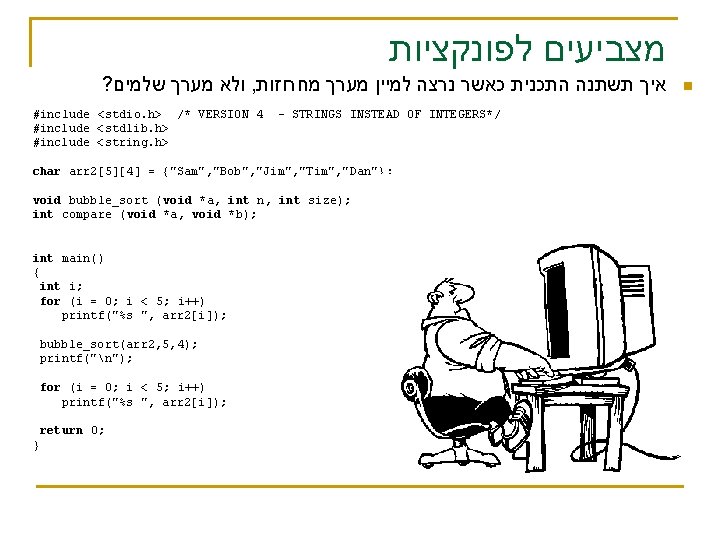
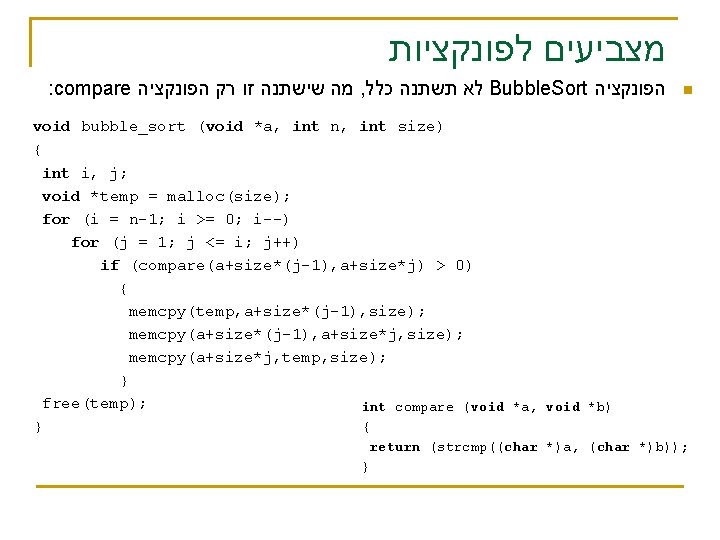
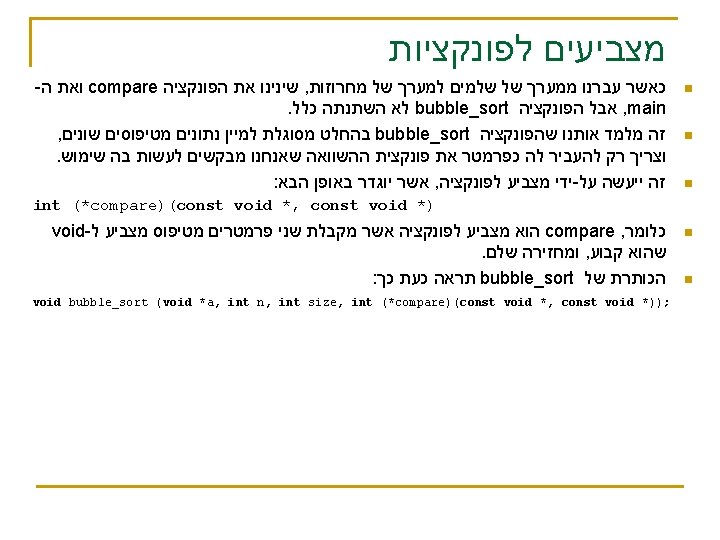
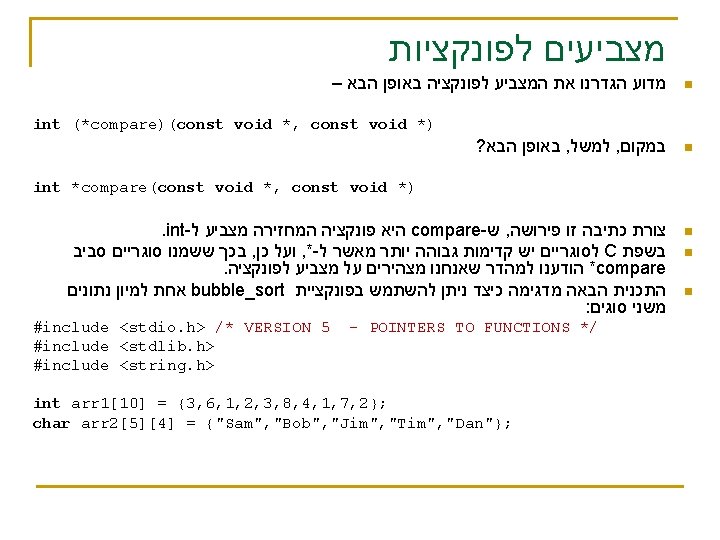
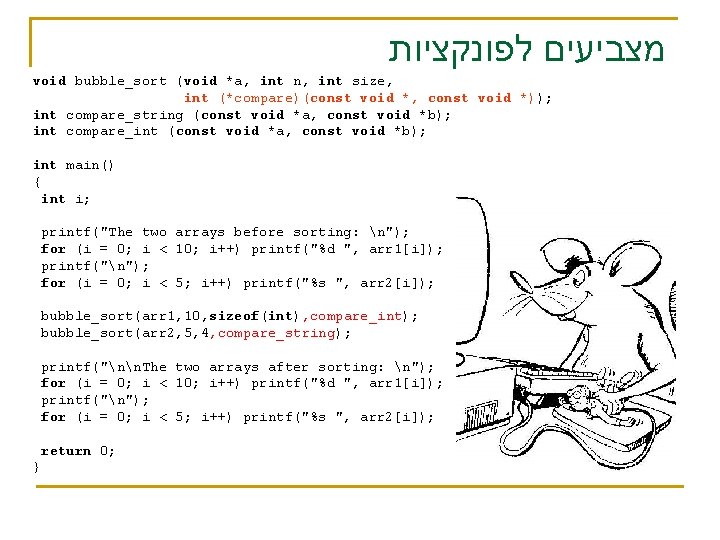
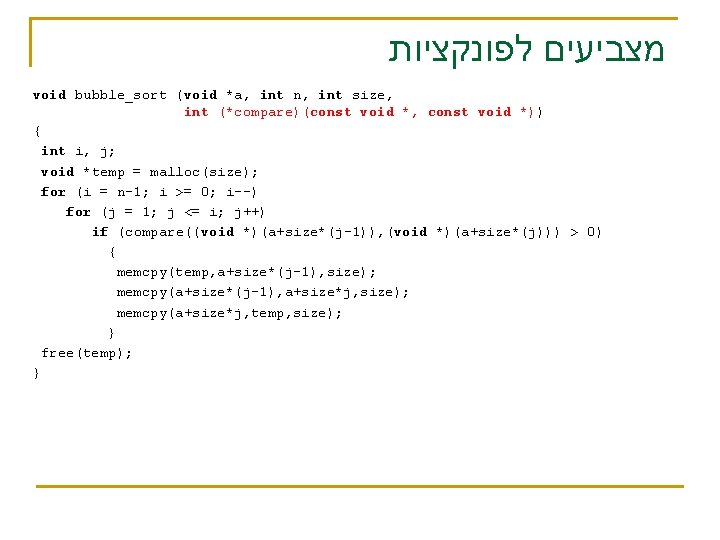
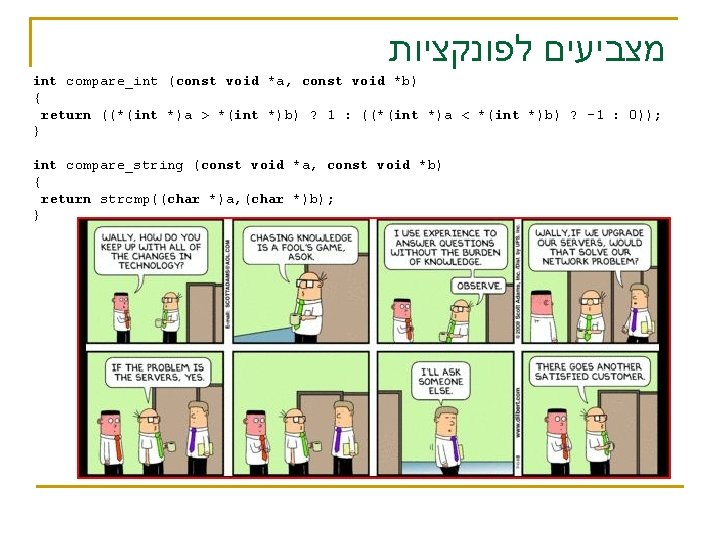
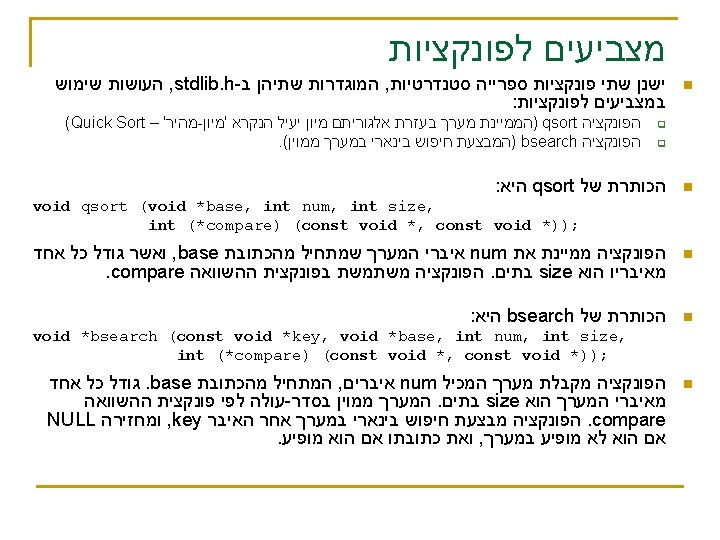
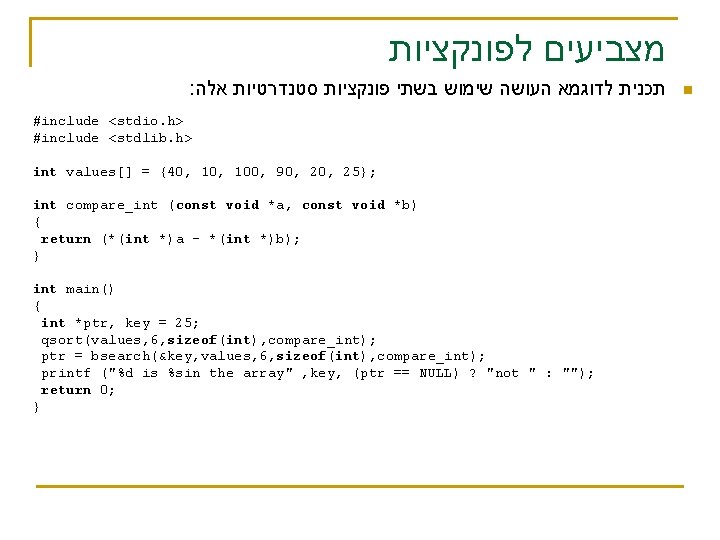
- Slides: 29
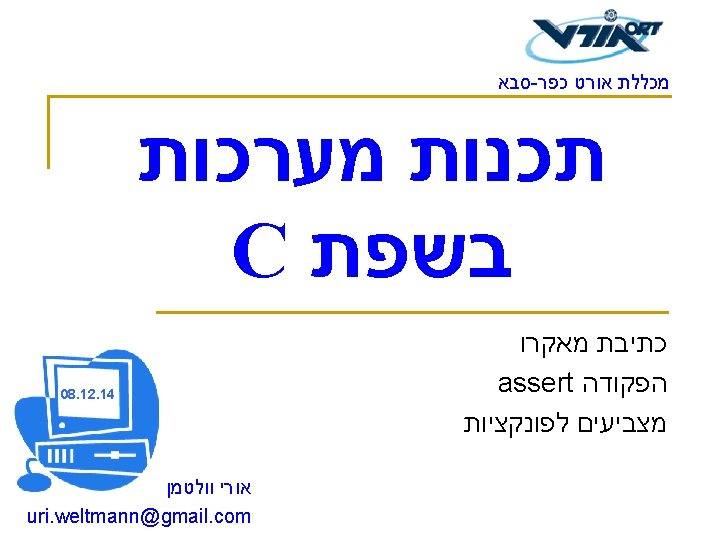
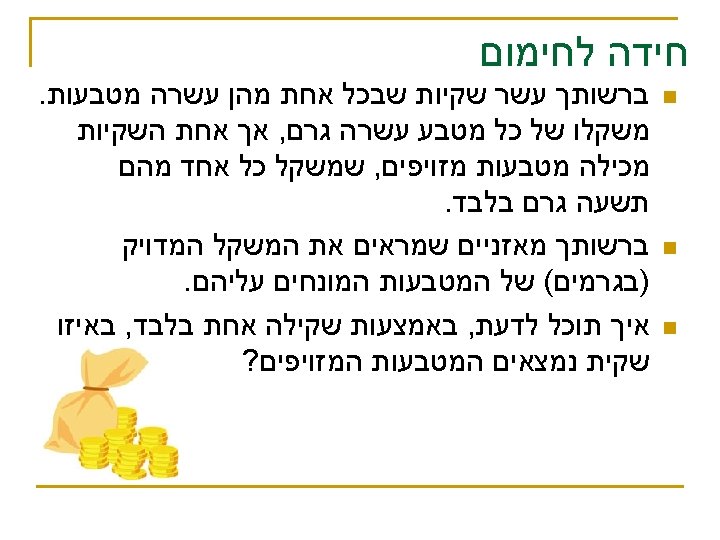
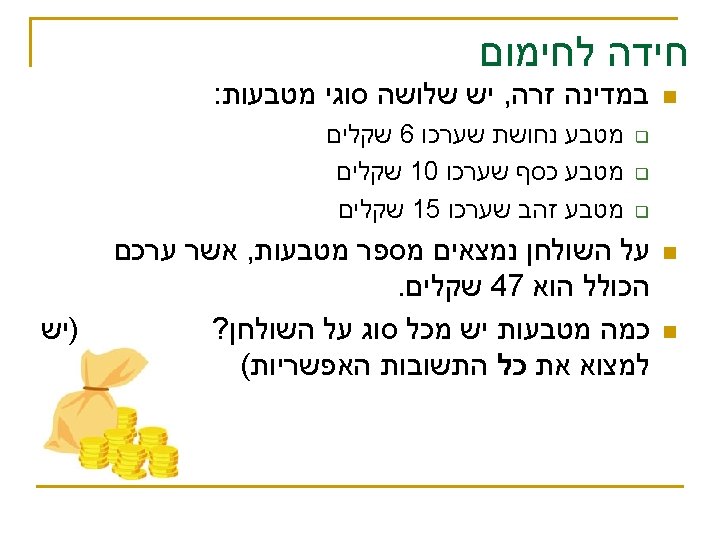
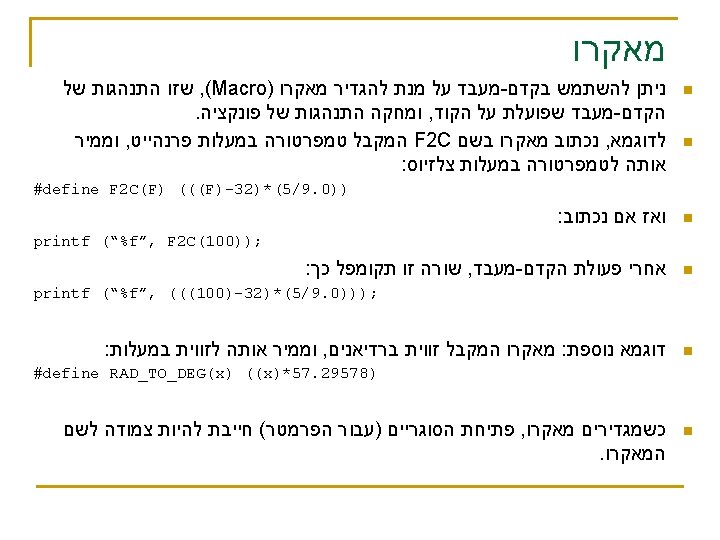
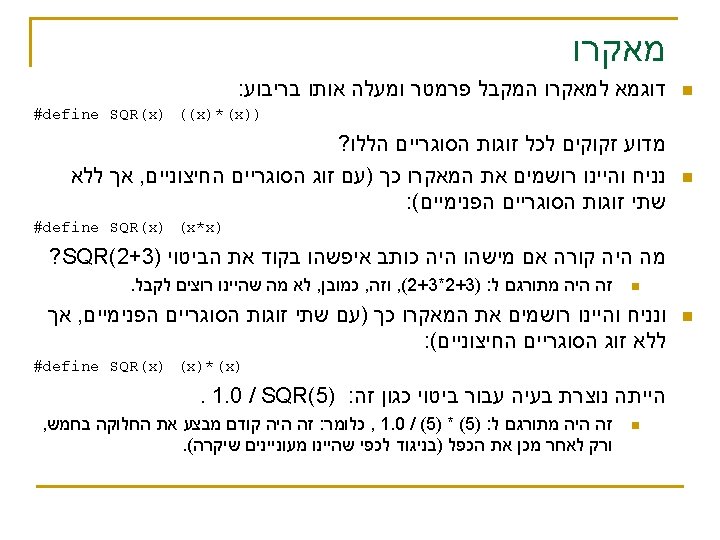
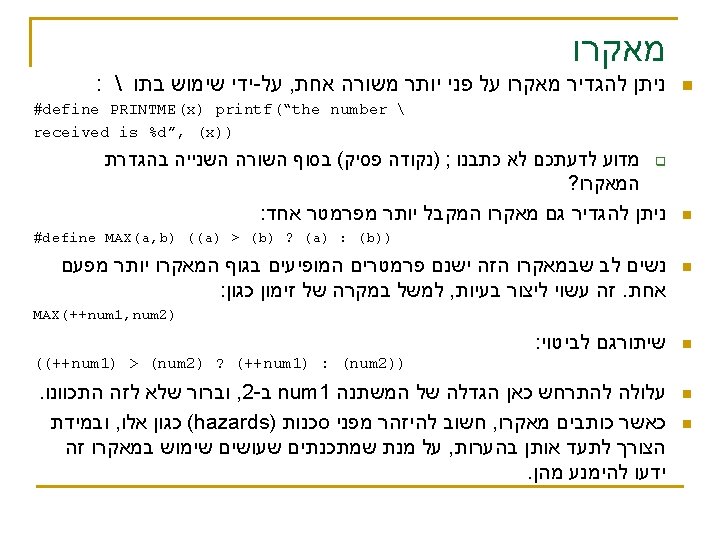
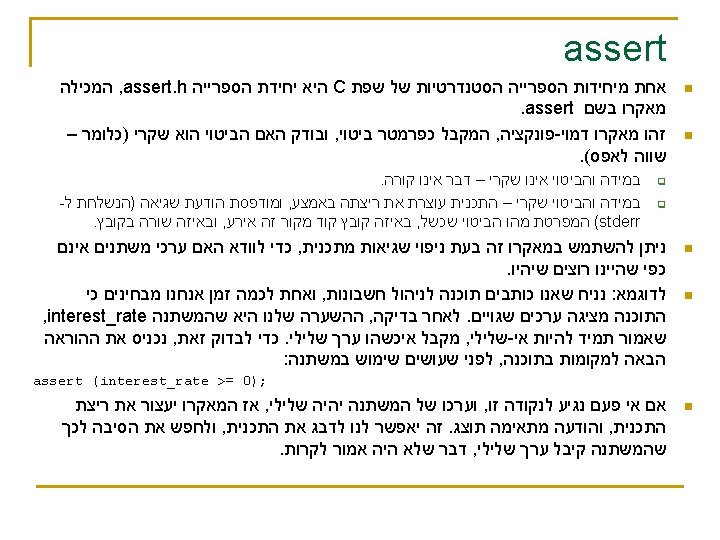
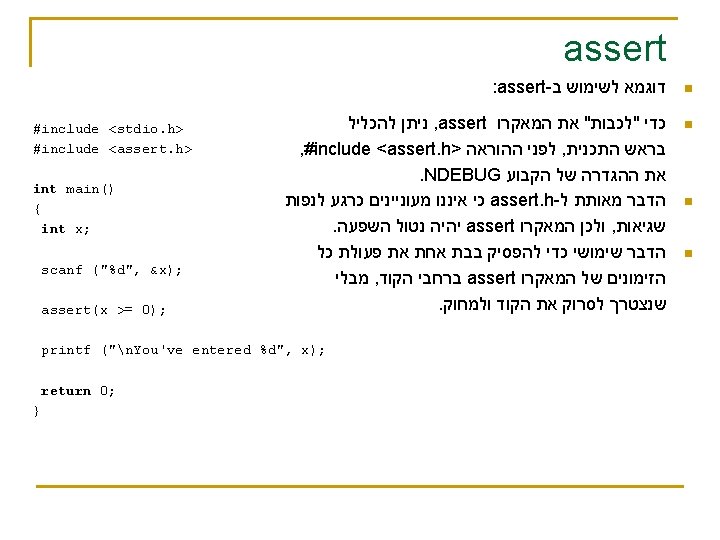
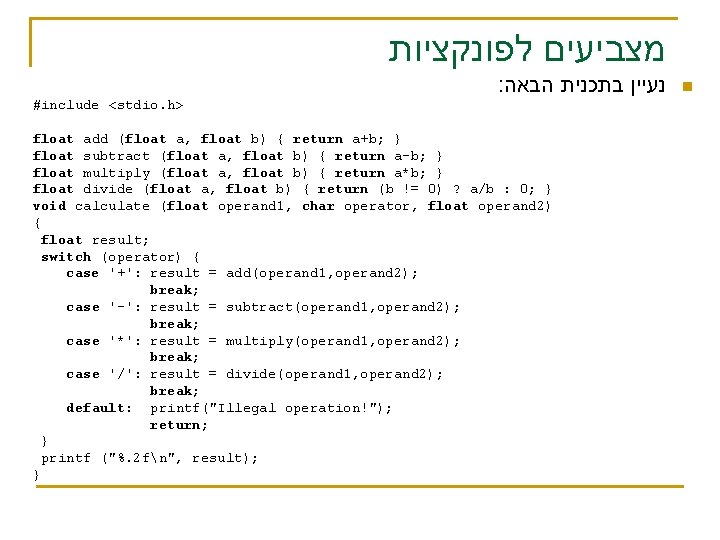
מצביעים לפונקציות : נעיין בתכנית הבאה #include <stdio. h> float add (float a, float b) { return a+b; } float subtract (float a, float b) { return a-b; } float multiply (float a, float b) { return a*b; } float divide (float a, float b) { return (b != 0) ? a/b : 0; } void calculate (float operand 1, char operator, float operand 2) { float result; switch (operator) { case '+': result = add(operand 1, operand 2); break; case '-': result = subtract(operand 1, operand 2); break; case '*': result = multiply(operand 1, operand 2); break; case '/': result = divide(operand 1, operand 2); break; default: printf("Illegal operation!"); return; } printf ("%. 2 fn", result); } n
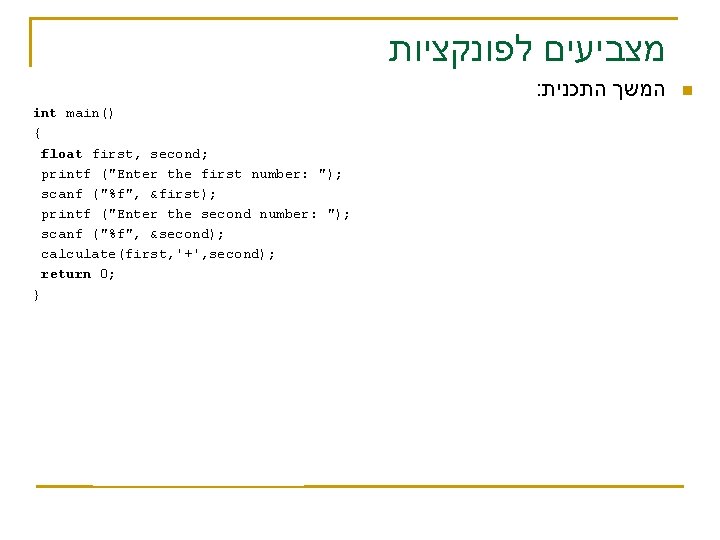
מצביעים לפונקציות : המשך התכנית int main() { float first, second; printf ("Enter the first number: "); scanf ("%f", &first); printf ("Enter the second number: "); scanf ("%f", &second); calculate(first, '+', second); return 0; } n
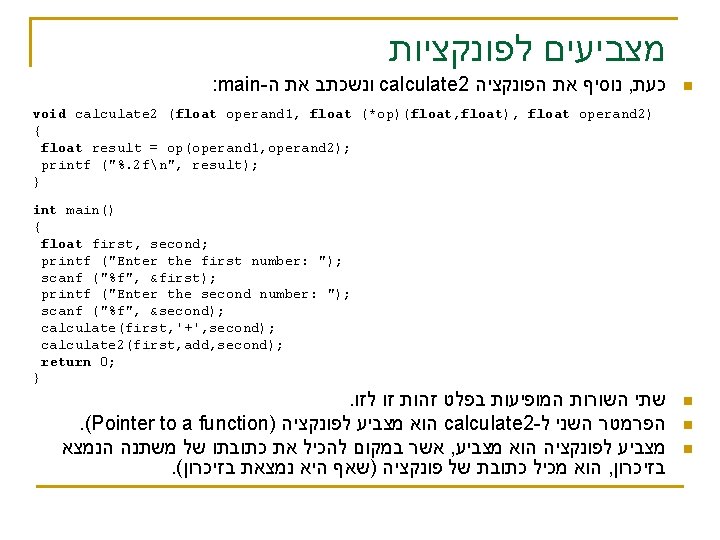
מצביעים לפונקציות : main- ונשכתב את ה calculate 2 נוסיף את הפונקציה , כעת n void calculate 2 (float operand 1, float (*op)(float, float), float operand 2) { float result = op(operand 1, operand 2); printf ("%. 2 fn", result); } int main() { float first, second; printf ("Enter the first number: "); scanf ("%f", &first); printf ("Enter the second number: "); scanf ("%f", &second); calculate(first, '+', second); calculate 2(first, add, second); return 0; } . שתי השורות המופיעות בפלט זהות זו לזו . (Pointer to a function) הוא מצביע לפונקציה calculate 2 - הפרמטר השני ל אשר במקום להכיל את כתובתו של משתנה הנמצא , מצביע לפונקציה הוא מצביע . ( הוא מכיל כתובת של פונקציה )שאף היא נמצאת בזיכרון , בזיכרון n n n
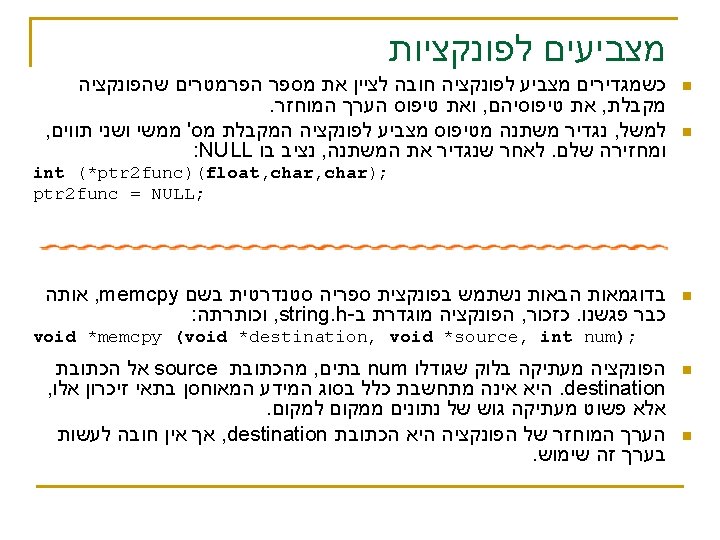
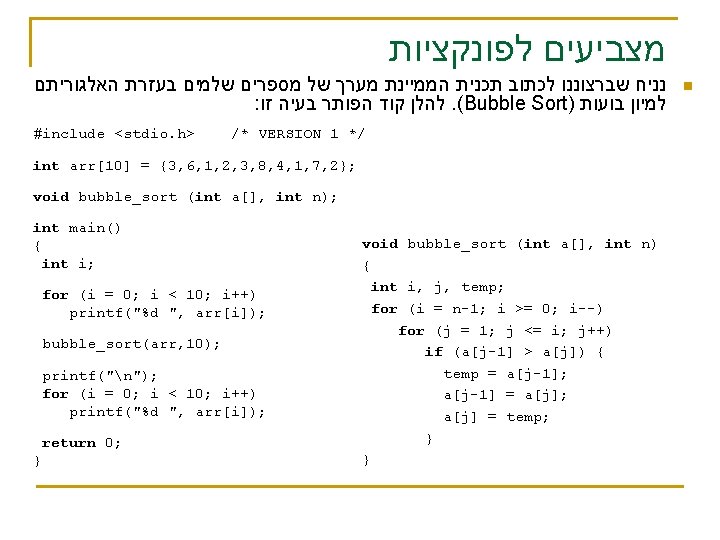
מצביעים לפונקציות נניח שברצוננו לכתוב תכנית הממיינת מערך של מספרים שלמים בעזרת האלגוריתם : להלן קוד הפותר בעיה זו. (Bubble Sort) למיון בועות #include <stdio. h> /* VERSION 1 */ int arr[10] = {3, 6, 1, 2, 3, 8, 4, 1, 7, 2}; void bubble_sort (int a[], int n); int main() { int i; for (i = 0; i < 10; i++) printf("%d ", arr[i]); bubble_sort(arr, 10); printf("n"); for (i = 0; i < 10; i++) printf("%d ", arr[i]); return 0; } void bubble_sort (int a[], int n) { int i, j, temp; for (i = n-1; i >= 0; i--) for (j = 1; j <= i; j++) if (a[j-1] > a[j]) { temp = a[j-1]; a[j-1] = a[j]; a[j] = temp; } } n
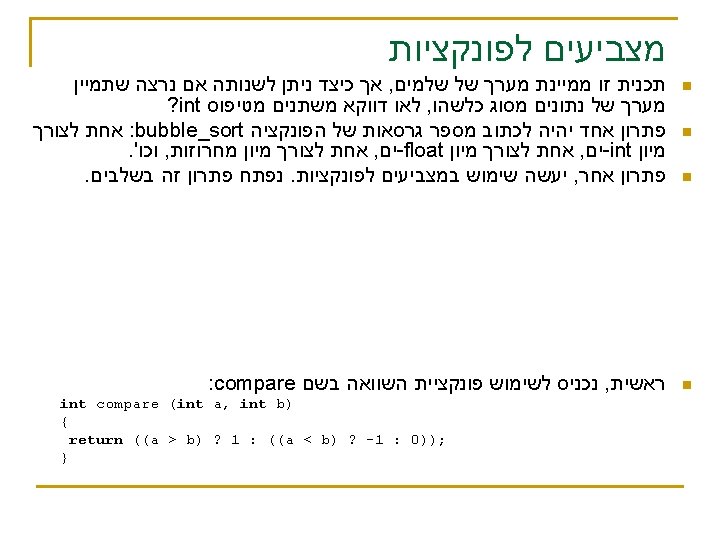
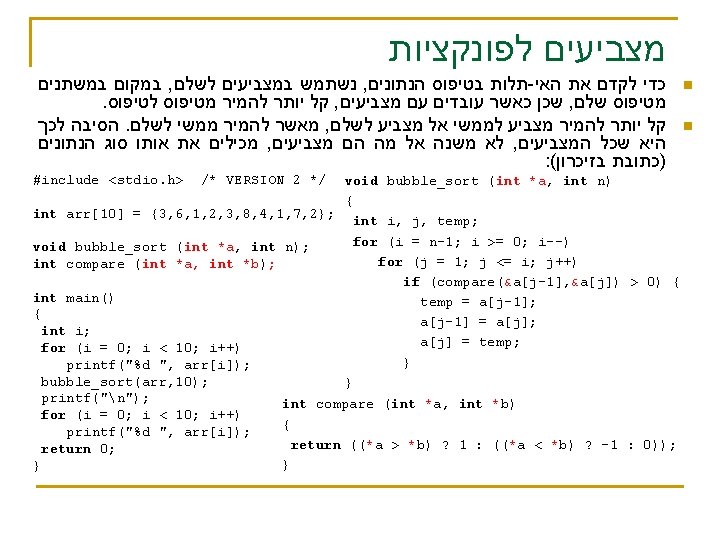
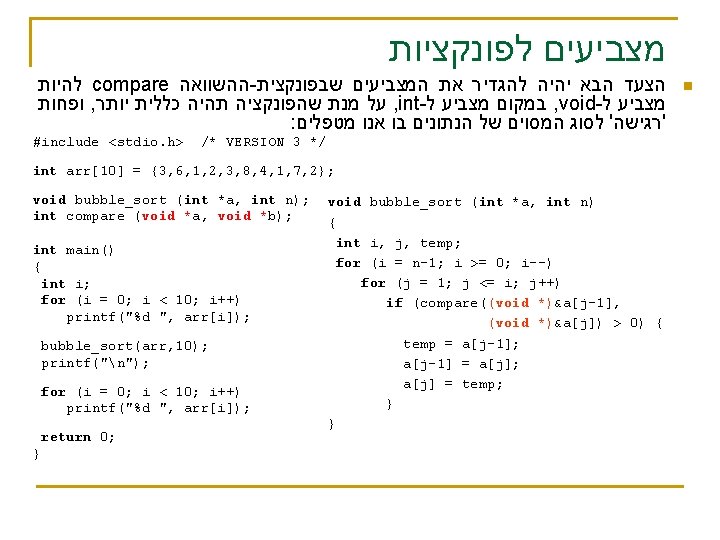
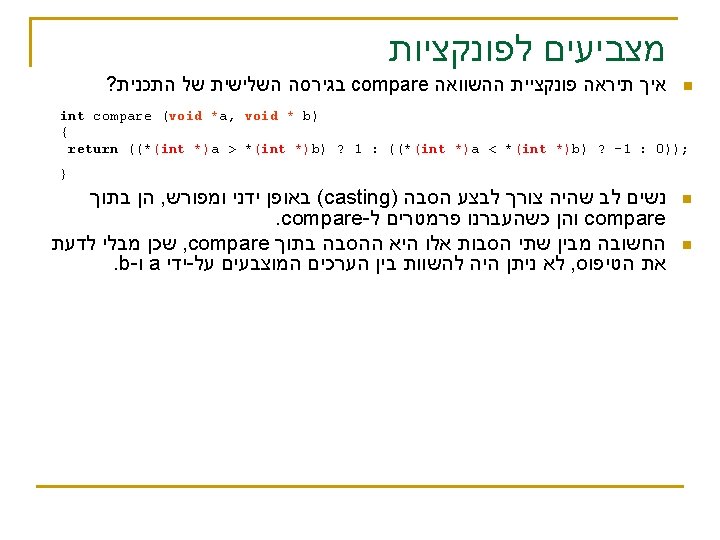
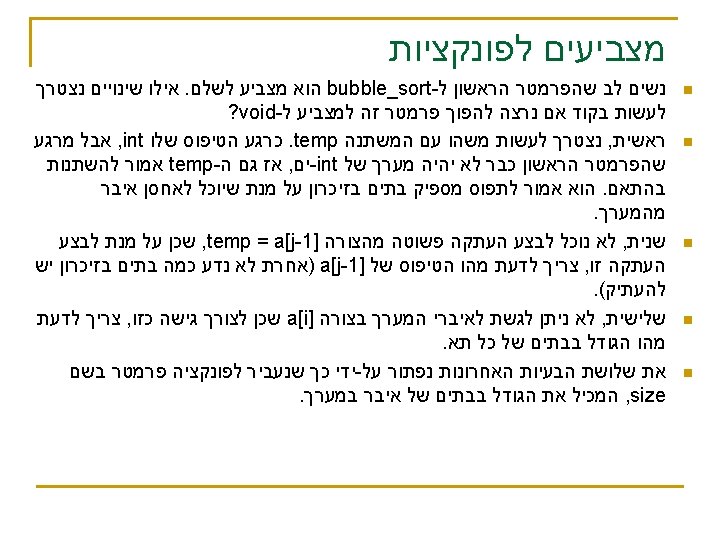
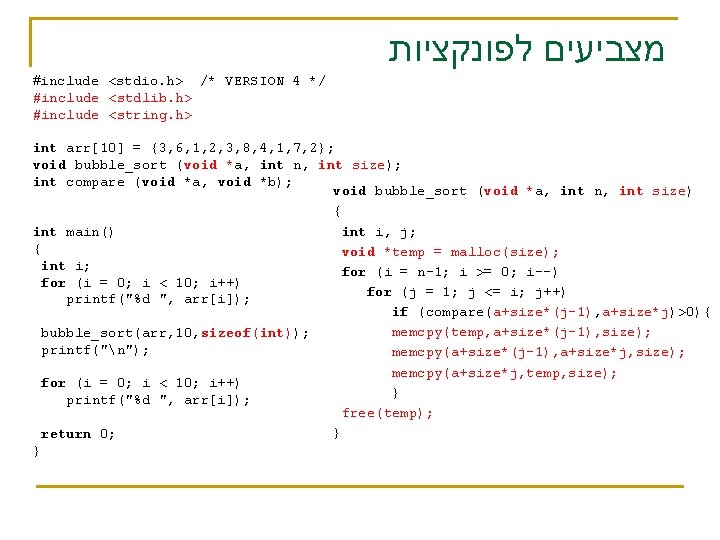
מצביעים לפונקציות #include <stdio. h> /* VERSION 4 */ #include <stdlib. h> #include <string. h> int arr[10] = {3, 6, 1, 2, 3, 8, 4, 1, 7, 2}; void bubble_sort (void *a, int n, int size); int compare (void *a, void *b); void bubble_sort (void *a, int n, int size) { int i, j; int main() { void *temp = malloc(size); int i; for (i = n-1; i >= 0; i--) for (i = 0; i < 10; i++) for (j = 1; j <= i; j++) printf("%d ", arr[i]); if (compare(a+size*(j-1), a+size*j)>0){ memcpy(temp, a+size*(j-1), size); bubble_sort(arr, 10, sizeof(int)); printf("n"); memcpy(a+size*(j-1), a+size*j, size); memcpy(a+size*j, temp, size); for (i = 0; i < 10; i++) } printf("%d ", arr[i]); free(temp); } return 0; }
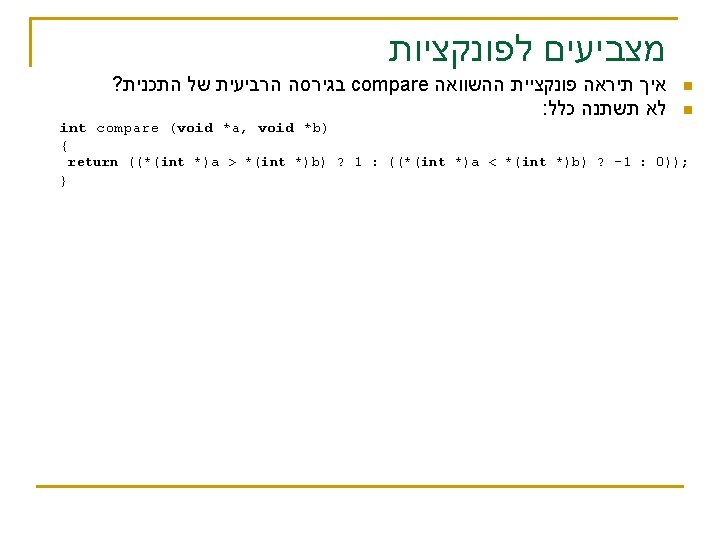
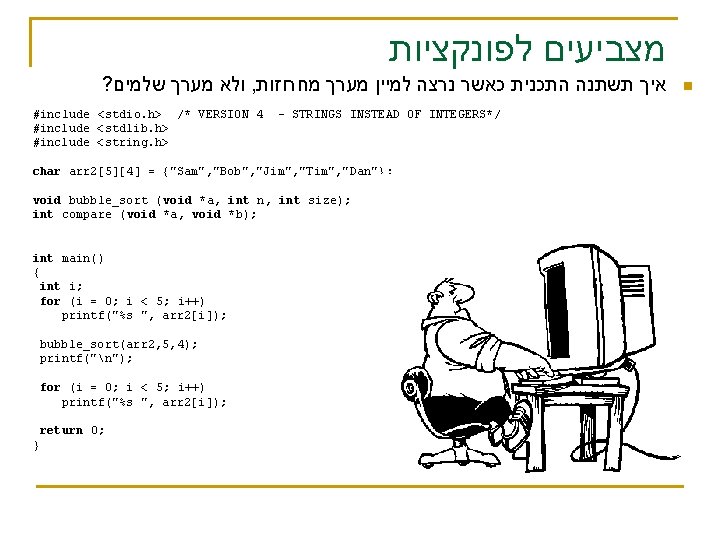
מצביעים לפונקציות ? ולא מערך שלמים , איך תשתנה התכנית כאשר נרצה למיין מערך מחרוזות #include <stdio. h> /* VERSION 4 #include <stdlib. h> #include <string. h> - STRINGS INSTEAD OF INTEGERS*/ char arr 2[5][4] = {"Sam", "Bob", "Jim", "Tim", "Dan"}; void bubble_sort (void *a, int n, int size); int compare (void *a, void *b); int main() { int i; for (i = 0; i < 5; i++) printf("%s ", arr 2[i]); bubble_sort(arr 2, 5, 4); printf("n"); for (i = 0; i < 5; i++) printf("%s ", arr 2[i]); return 0; } n
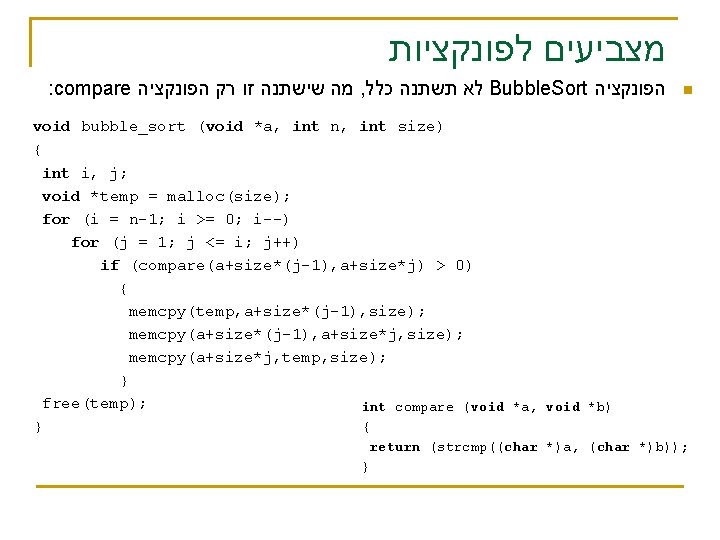
מצביעים לפונקציות : compare מה שישתנה זו רק הפונקציה , לא תשתנה כלל Bubble. Sort הפונקציה n void bubble_sort (void *a, int n, int size) { int i, j; void *temp = malloc(size); for (i = n-1; i >= 0; i--) for (j = 1; j <= i; j++) if (compare(a+size*(j-1), a+size*j) > 0) { memcpy(temp, a+size*(j-1), size); memcpy(a+size*(j-1), a+size*j, size); memcpy(a+size*j, temp, size); } free(temp); int compare (void *a, void *b) { } return (strcmp((char *)a, (char *)b)); }
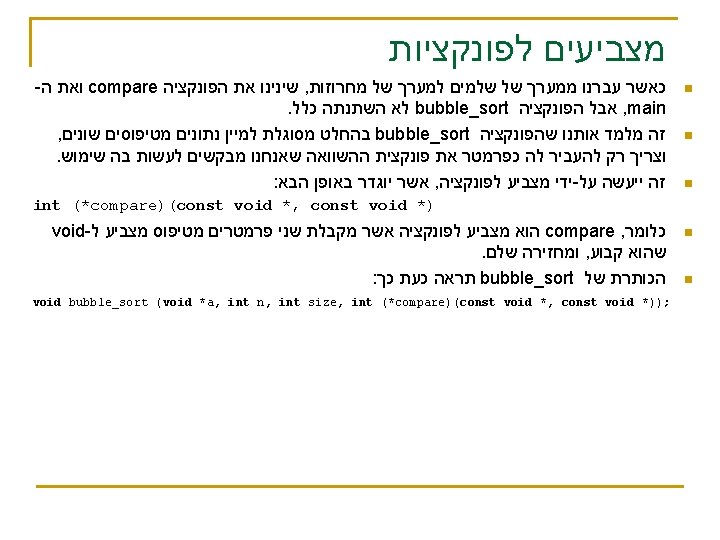
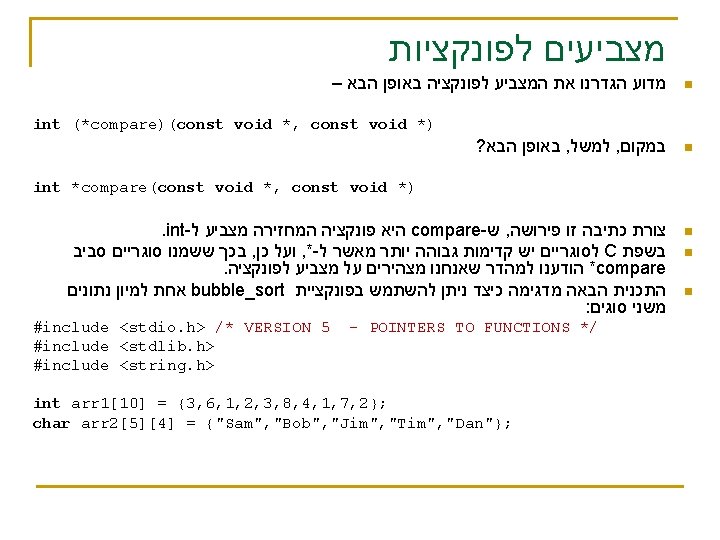
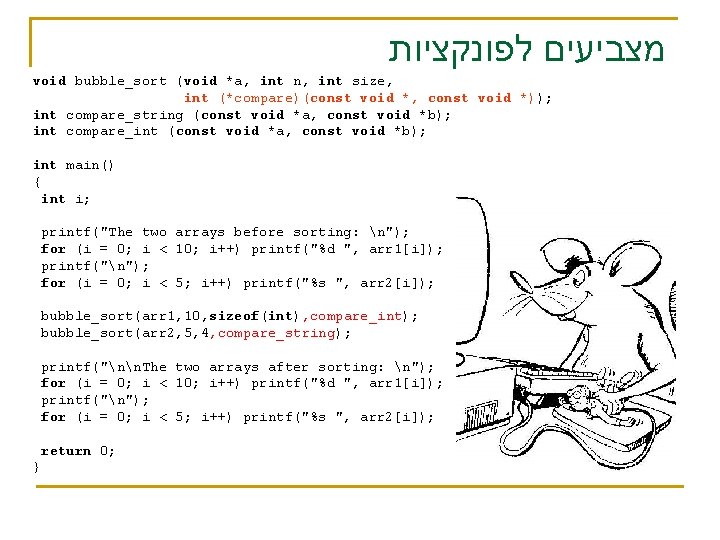
מצביעים לפונקציות void bubble_sort (void *a, int n, int size, int (*compare)(const void *, const void *)); int compare_string (const void *a, const void *b); int compare_int (const void *a, const void *b); int main() { int i; printf("The two arrays before sorting: n"); for (i = 0; i < 10; i++) printf("%d ", arr 1[i]); printf("n"); for (i = 0; i < 5; i++) printf("%s ", arr 2[i]); bubble_sort(arr 1, 10, sizeof(int), compare_int); bubble_sort(arr 2, 5, 4, compare_string); printf("nn. The two arrays after sorting: n"); for (i = 0; i < 10; i++) printf("%d ", arr 1[i]); printf("n"); for (i = 0; i < 5; i++) printf("%s ", arr 2[i]); return 0; }
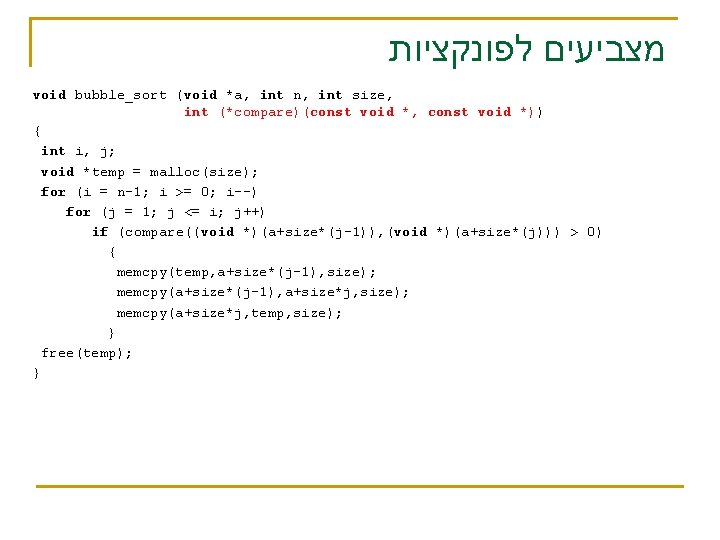
מצביעים לפונקציות void bubble_sort (void *a, int n, int size, int (*compare)(const void *, const void *)) { int i, j; void *temp = malloc(size); for (i = n-1; i >= 0; i--) for (j = 1; j <= i; j++) if (compare((void *)(a+size*(j-1)), (void *)(a+size*(j))) > 0) { memcpy(temp, a+size*(j-1), size); memcpy(a+size*(j-1), a+size*j, size); memcpy(a+size*j, temp, size); } free(temp); }
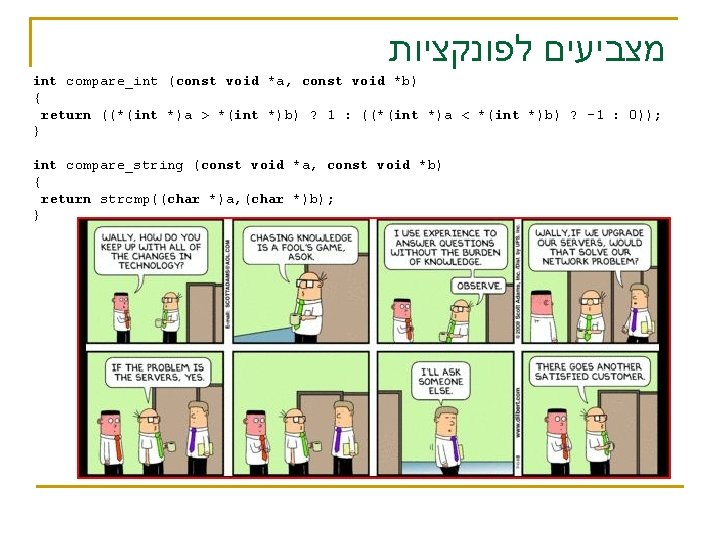
מצביעים לפונקציות int compare_int (const void *a, const void *b) { return ((*(int *)a > *(int *)b) ? 1 : ((*(int *)a < *(int *)b) ? -1 : 0)); } int compare_string (const void *a, const void *b) { return strcmp((char *)a, (char *)b); }
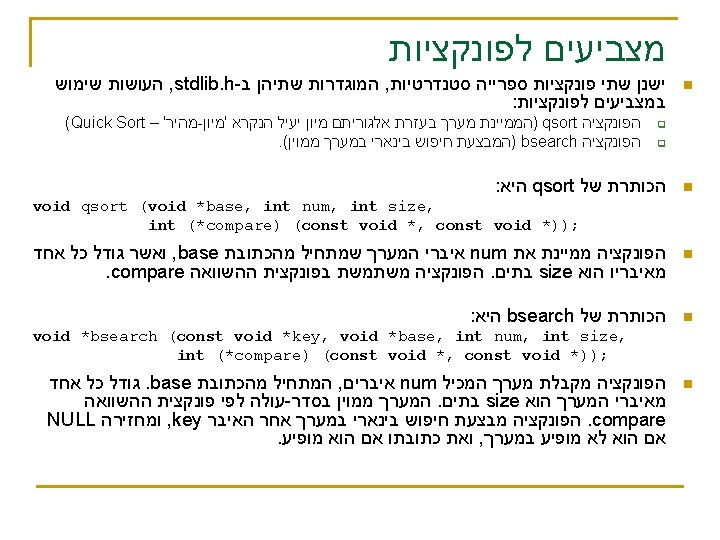
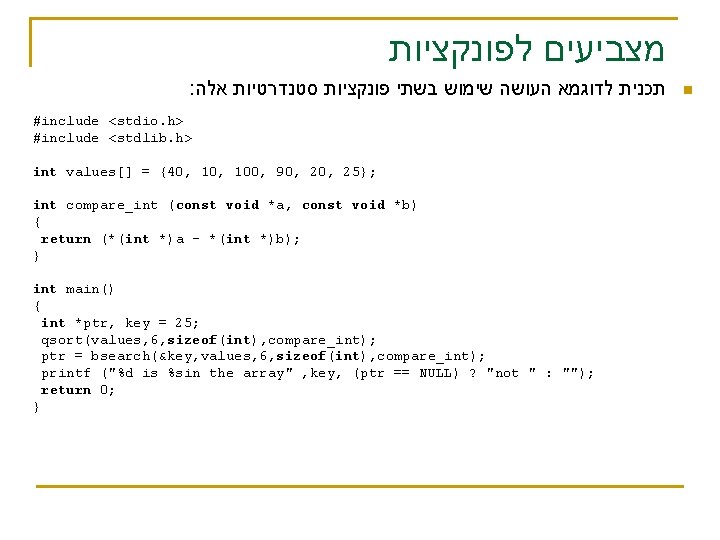
מצביעים לפונקציות : תכנית לדוגמא העושה שימוש בשתי פונקציות סטנדרטיות אלה #include <stdio. h> #include <stdlib. h> int values[] = {40, 100, 90, 25}; int compare_int (const void *a, const void *b) { return (*(int *)a - *(int *)b); } int main() { int *ptr, key = 25; qsort(values, 6, sizeof(int), compare_int); ptr = bsearch(&key, values, 6, sizeof(int), compare_int); printf ("%d is %sin the array" , key, (ptr == NULL) ? "not " : ""); return 0; } n