Implementing Concurrent R trees in Java Mehdi Kargar
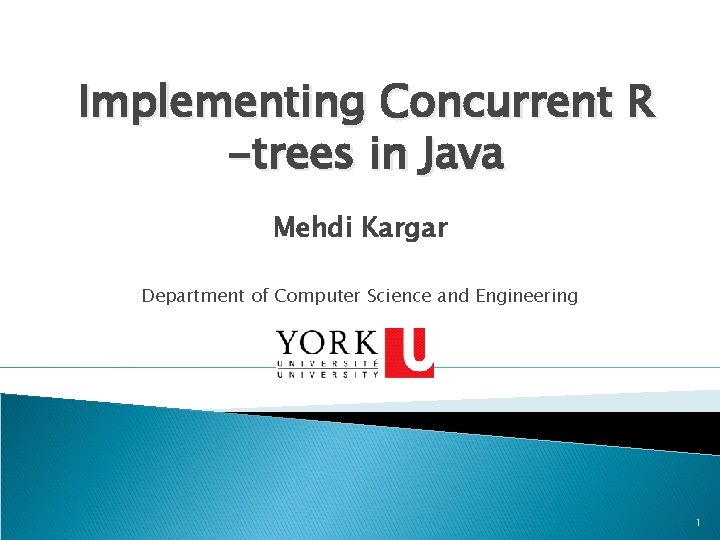
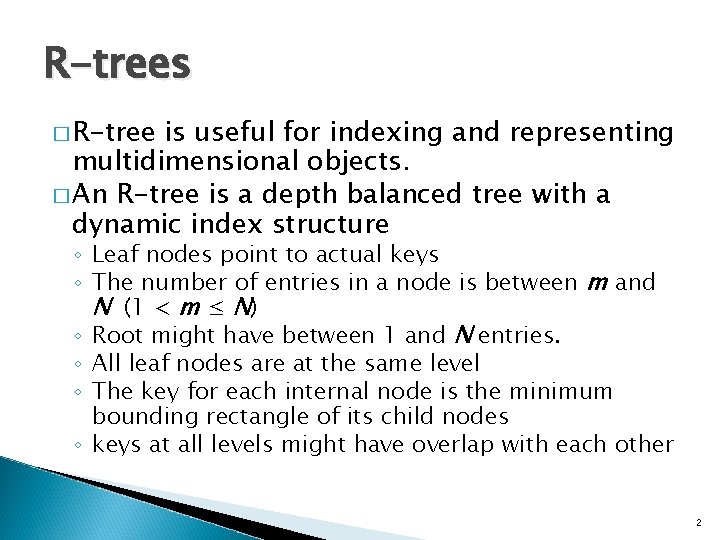
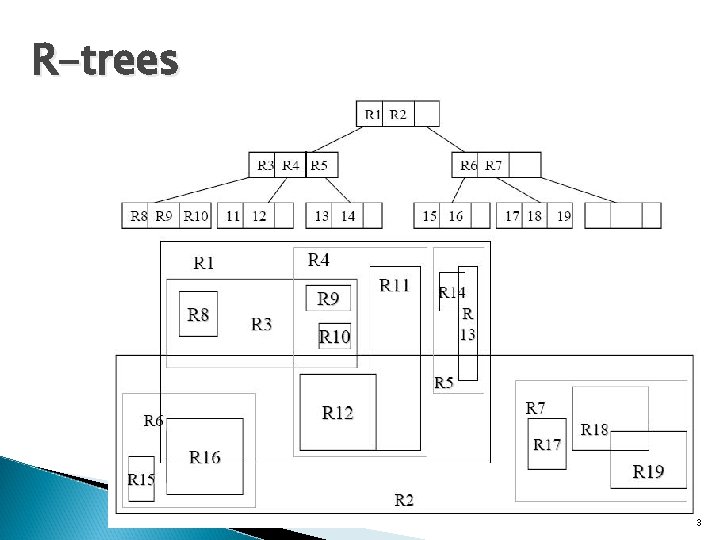
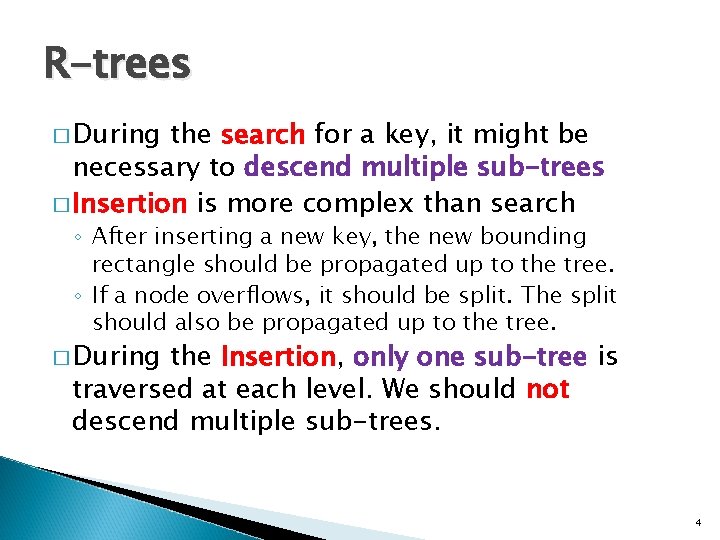
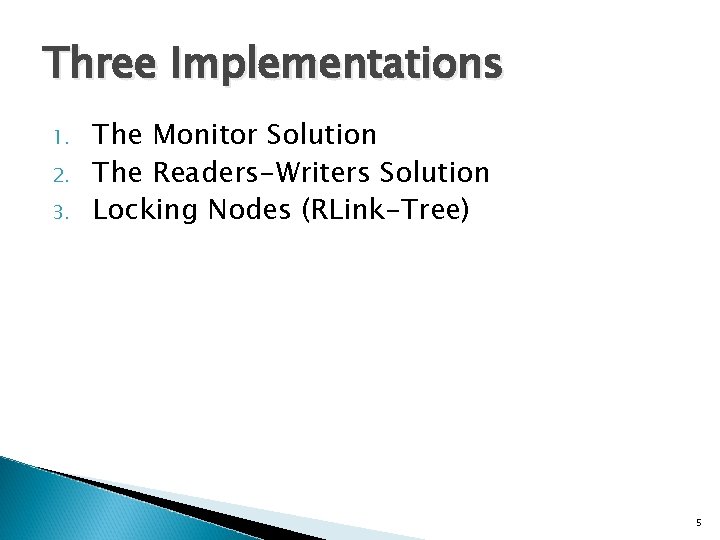
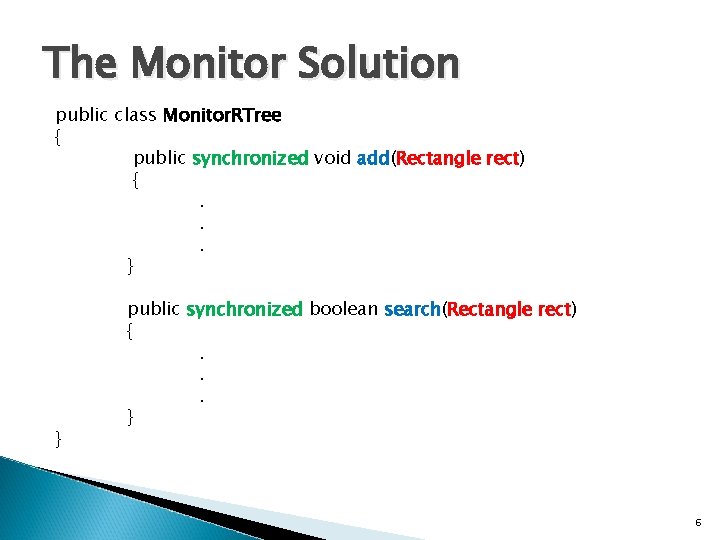
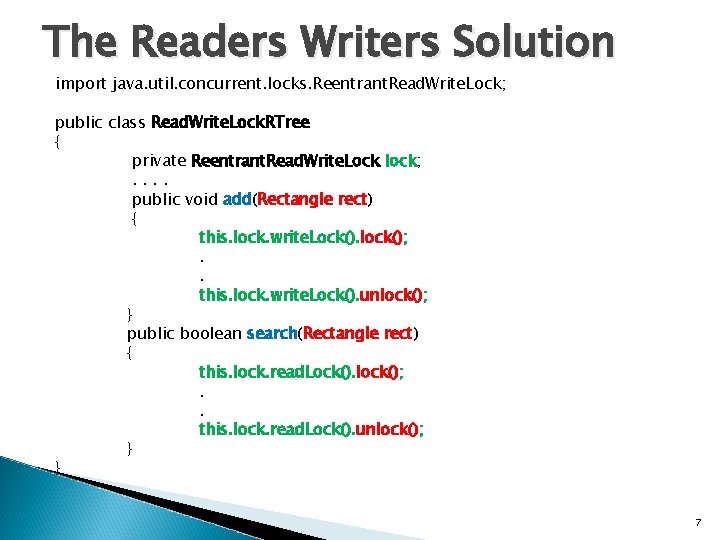
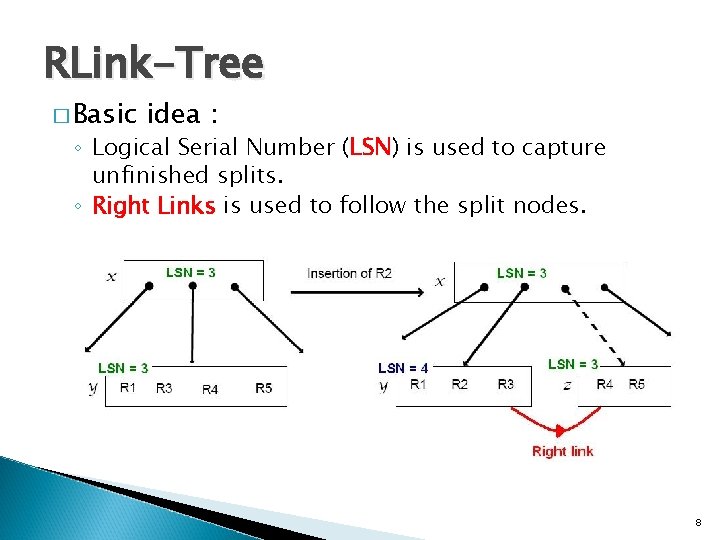
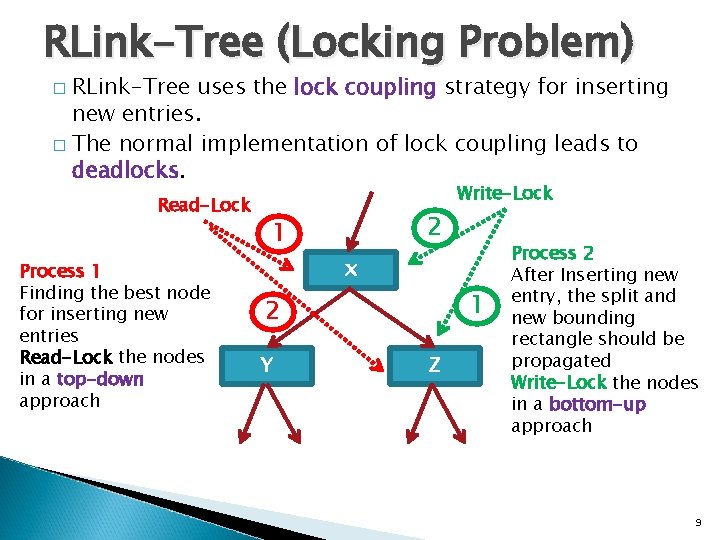
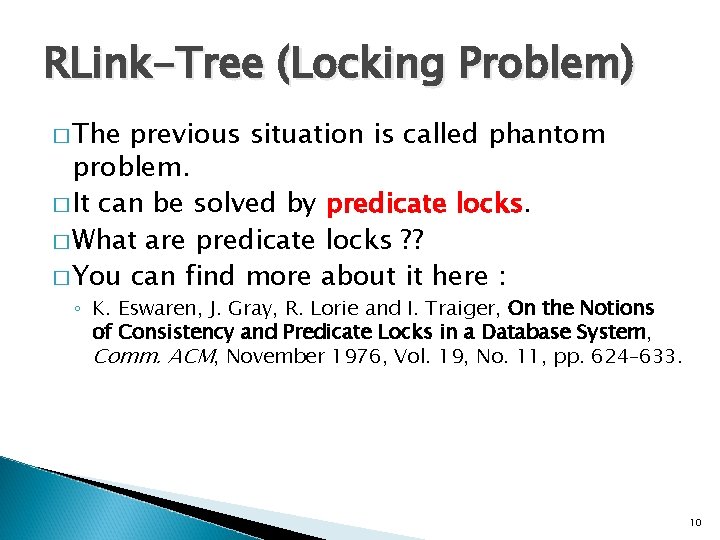
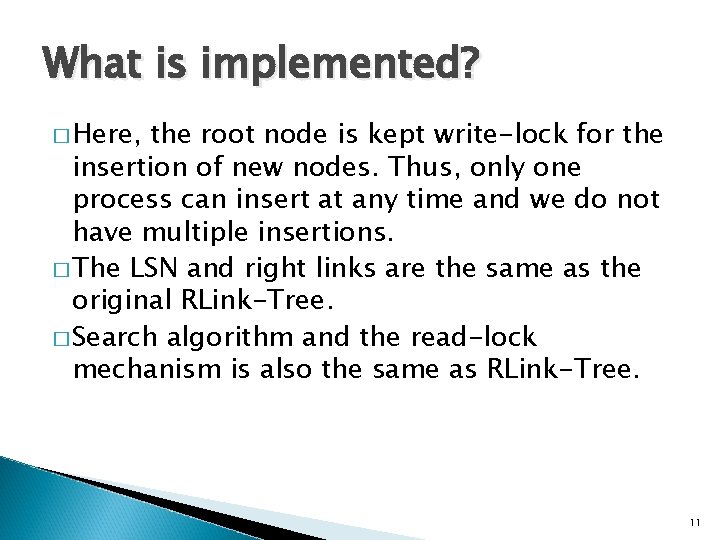
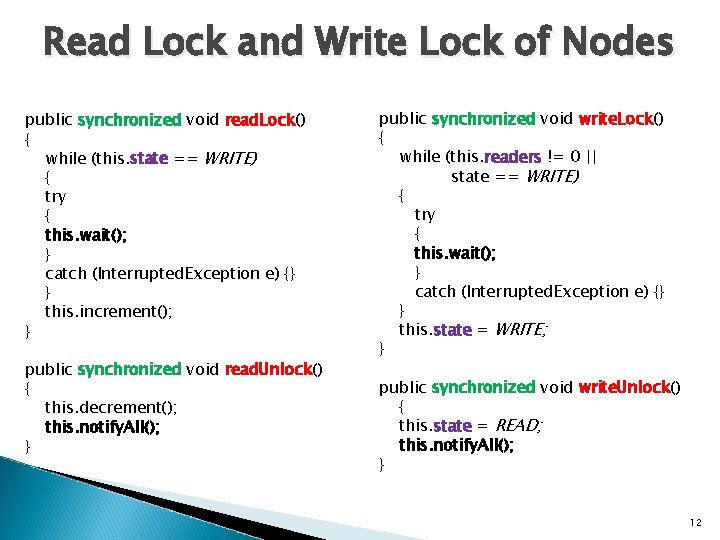
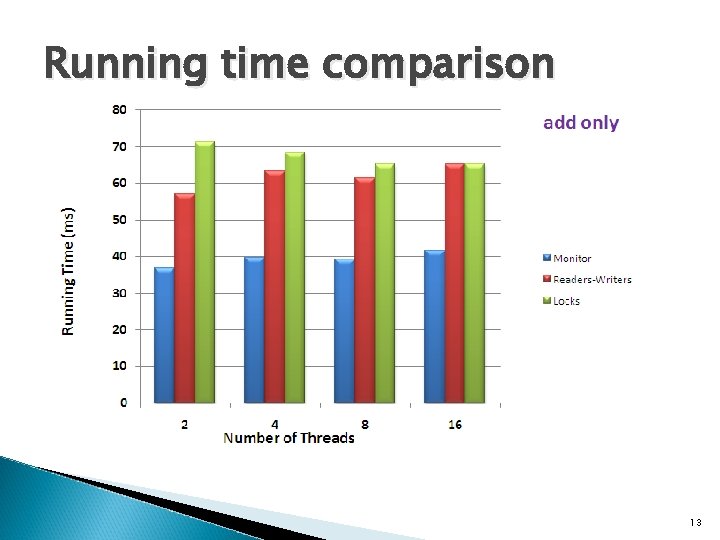
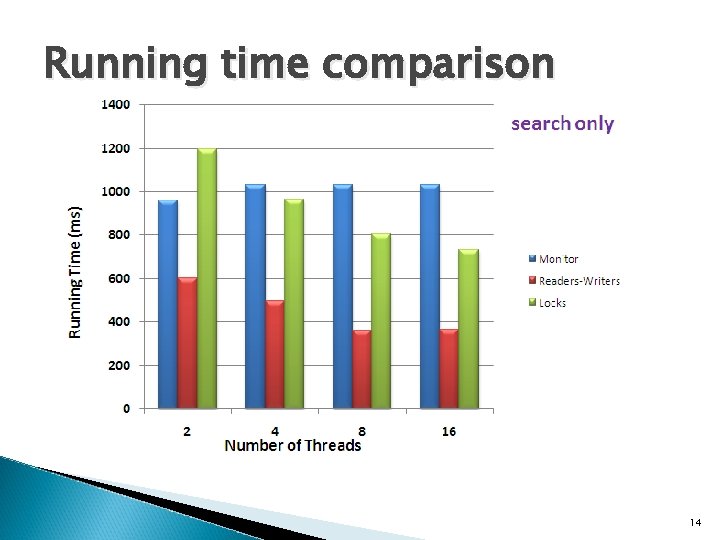
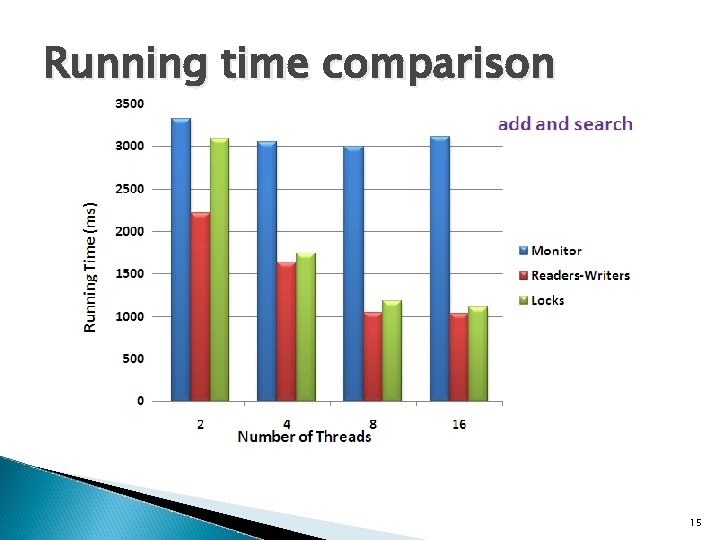
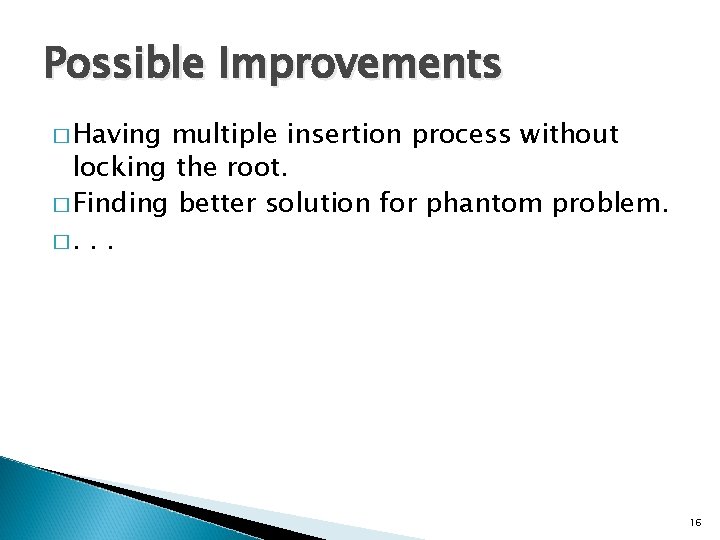
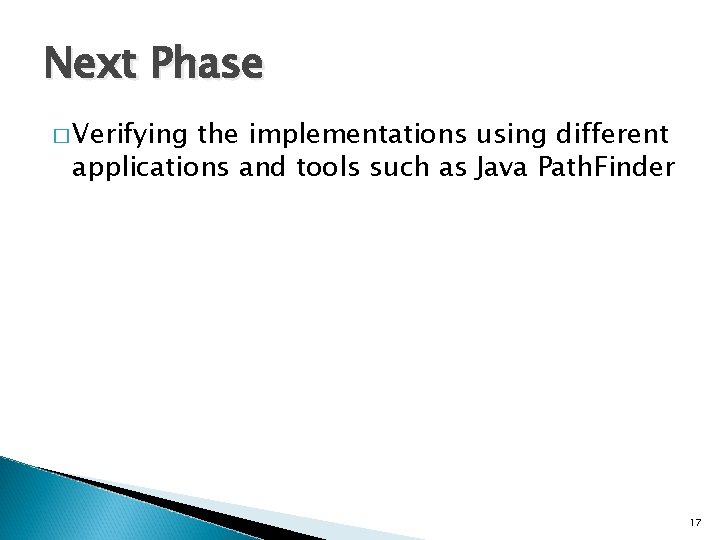
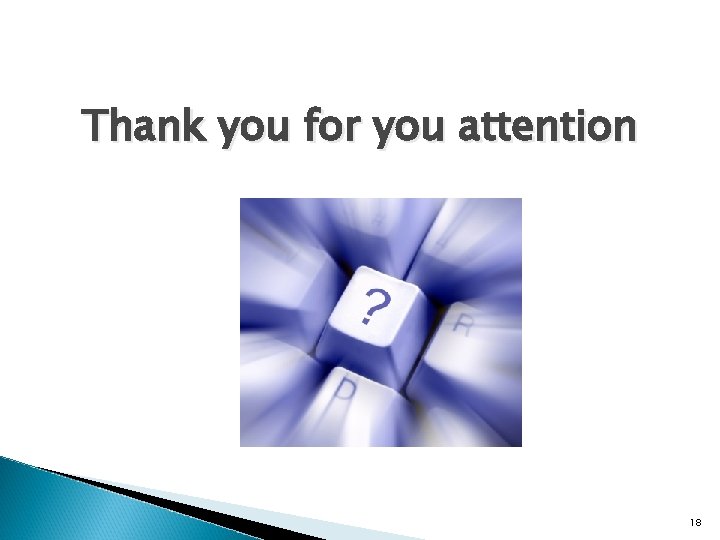
- Slides: 18
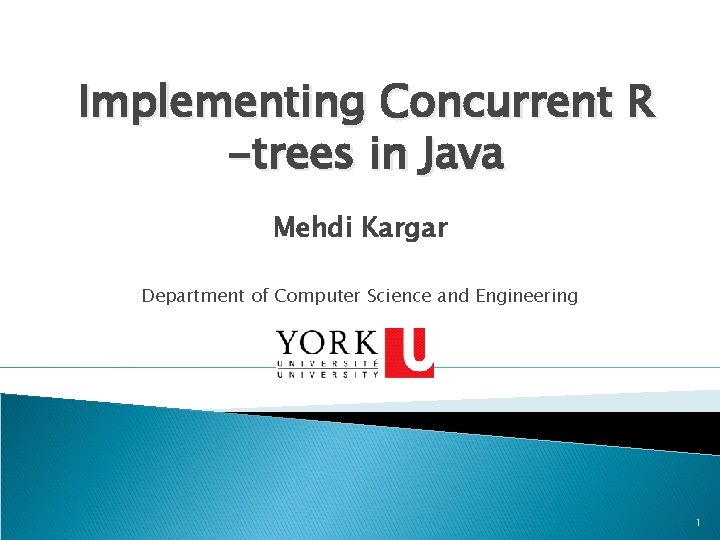
Implementing Concurrent R -trees in Java Mehdi Kargar Department of Computer Science and Engineering 1
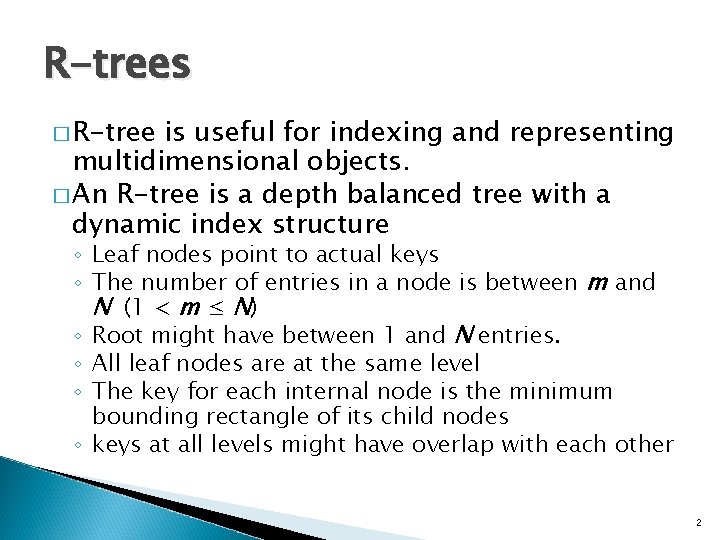
R-trees � R-tree is useful for indexing and representing multidimensional objects. � An R-tree is a depth balanced tree with a dynamic index structure ◦ Leaf nodes point to actual keys ◦ The number of entries in a node is between m and N (1 < m ≤ N) ◦ Root might have between 1 and N entries. ◦ All leaf nodes are at the same level ◦ The key for each internal node is the minimum bounding rectangle of its child nodes ◦ keys at all levels might have overlap with each other 2
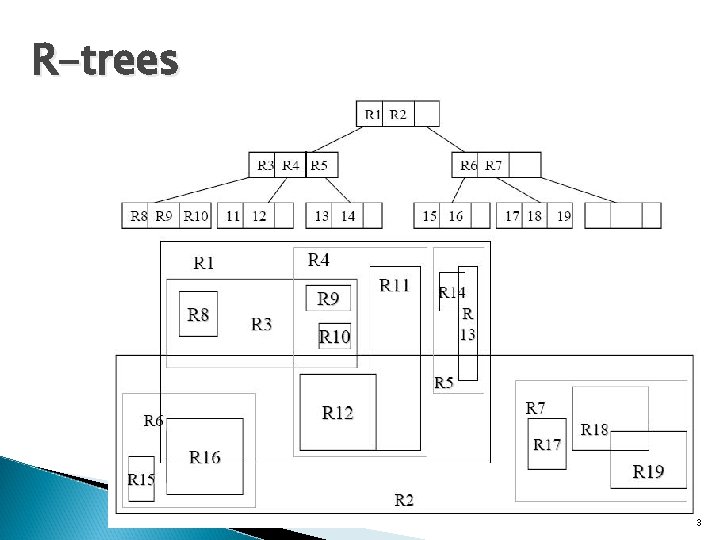
R-trees 3
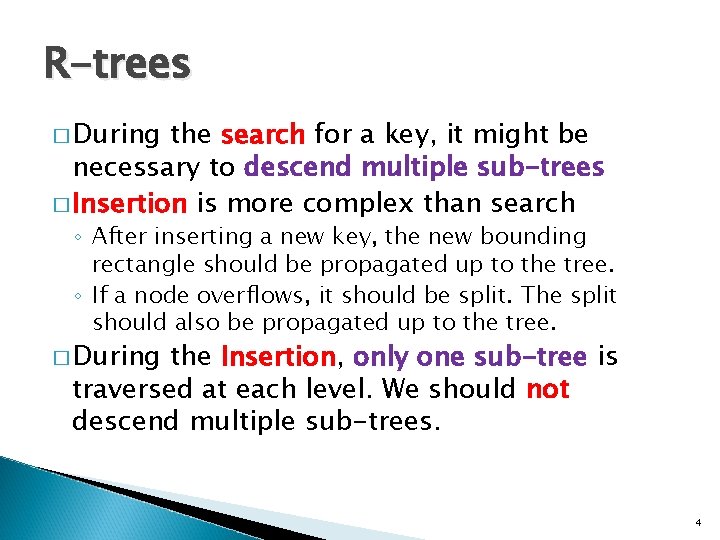
R-trees � During the search for a key, it might be necessary to descend multiple sub-trees � Insertion is more complex than search ◦ After inserting a new key, the new bounding rectangle should be propagated up to the tree. ◦ If a node overflows, it should be split. The split should also be propagated up to the tree. � During the Insertion, only one sub-tree is traversed at each level. We should not descend multiple sub-trees. 4
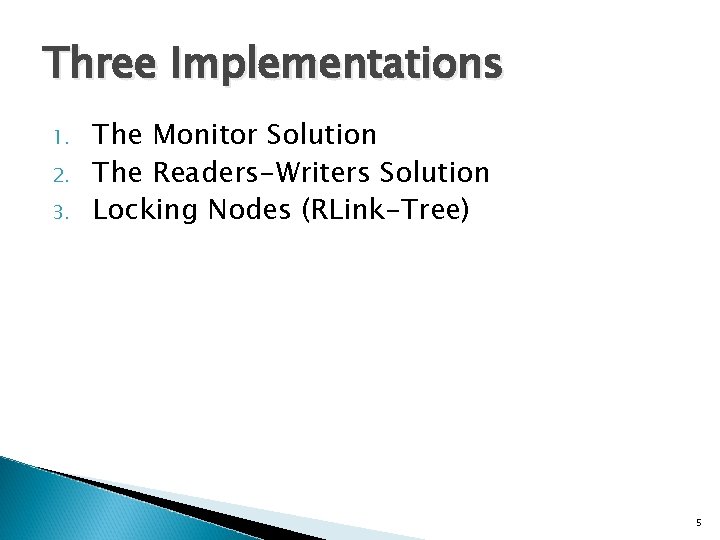
Three Implementations 1. 2. 3. The Monitor Solution The Readers-Writers Solution Locking Nodes (RLink-Tree) 5
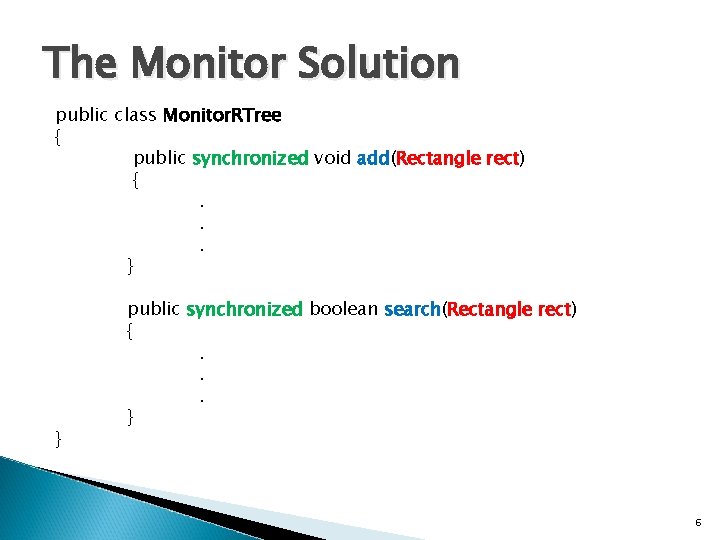
The Monitor Solution public class Monitor. RTree { public synchronized void add(Rectangle rect) {. . . } } public synchronized boolean search(Rectangle rect) {. . . } 6
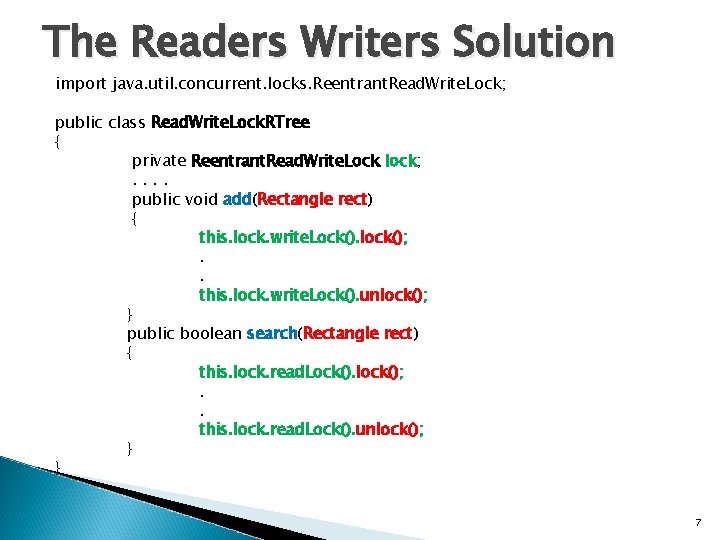
The Readers Writers Solution import java. util. concurrent. locks. Reentrant. Read. Write. Lock; public class Read. Write. Lock. RTree { private Reentrant. Read. Write. Lock lock; . . public void add(Rectangle rect) { this. lock. write. Lock(). lock(); . . this. lock. write. Lock(). unlock(); } public boolean search(Rectangle rect) { this. lock. read. Lock(). lock(); . . this. lock. read. Lock(). unlock(); } } 7
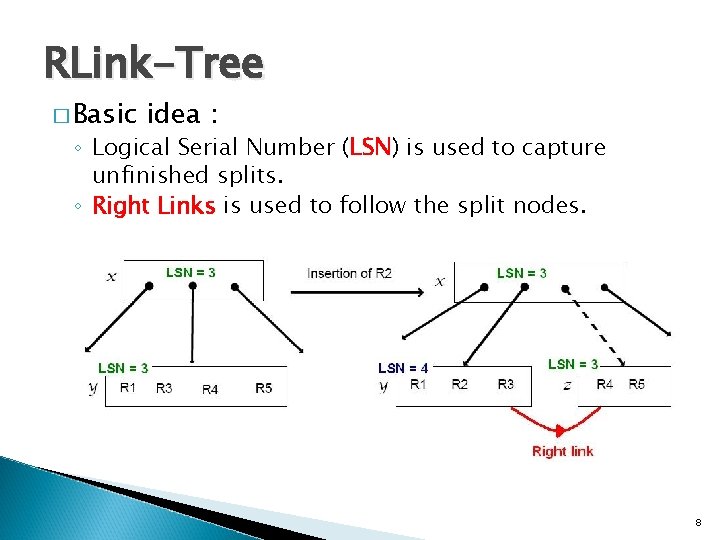
RLink-Tree � Basic idea : ◦ Logical Serial Number (LSN) is used to capture unfinished splits. ◦ Right Links is used to follow the split nodes. 8
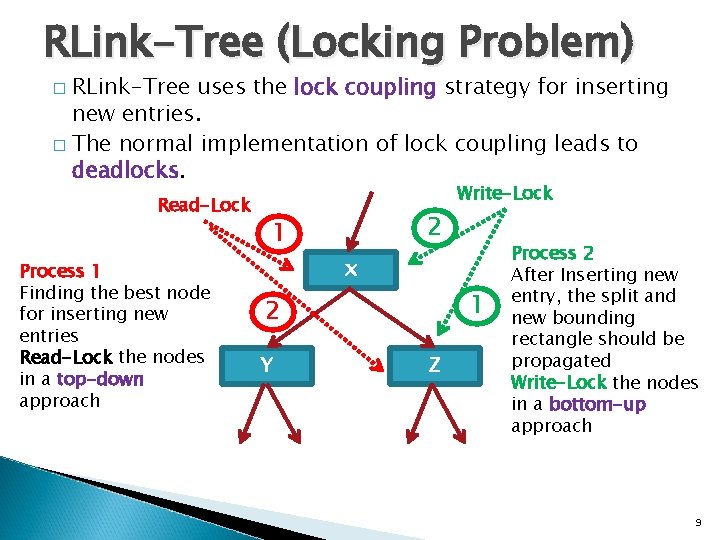
RLink-Tree (Locking Problem) RLink-Tree uses the lock coupling strategy for inserting new entries. � The normal implementation of lock coupling leads to deadlocks. � Read-Lock Process 1 Finding the best node for inserting new entries Read-Lock the nodes in a top-down approach Write-Lock 2 1 x 1 2 Y Z Process 2 After Inserting new entry, the split and new bounding rectangle should be propagated Write-Lock the nodes in a bottom-up approach 9
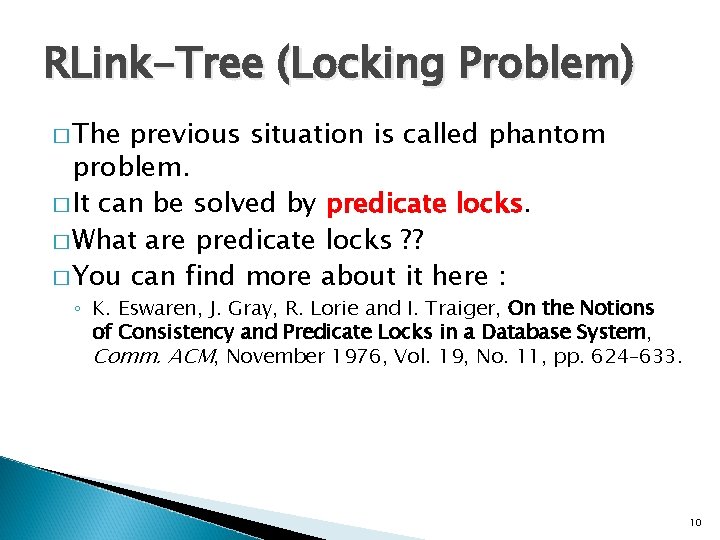
RLink-Tree (Locking Problem) � The previous situation is called phantom problem. � It can be solved by predicate locks. � What are predicate locks ? ? � You can find more about it here : ◦ K. Eswaren, J. Gray, R. Lorie and I. Traiger, On the Notions of Consistency and Predicate Locks in a Database System, Comm. ACM, November 1976, Vol. 19, No. 11, pp. 624– 633. 10
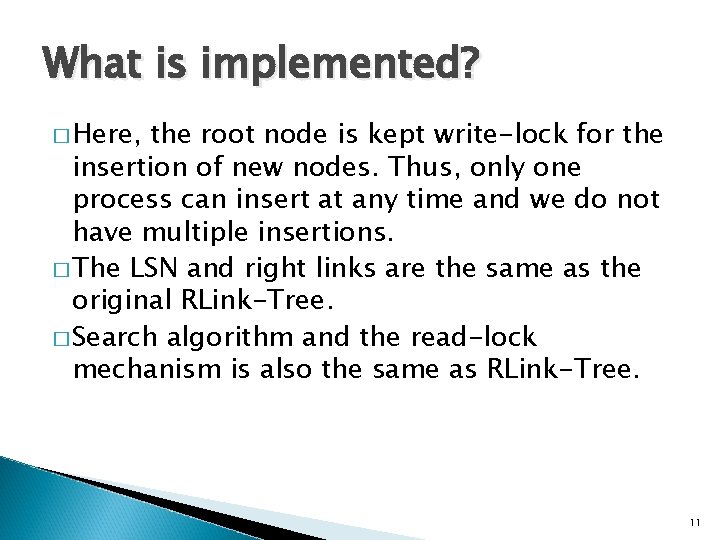
What is implemented? � Here, the root node is kept write-lock for the insertion of new nodes. Thus, only one process can insert at any time and we do not have multiple insertions. � The LSN and right links are the same as the original RLink-Tree. � Search algorithm and the read-lock mechanism is also the same as RLink-Tree. 11
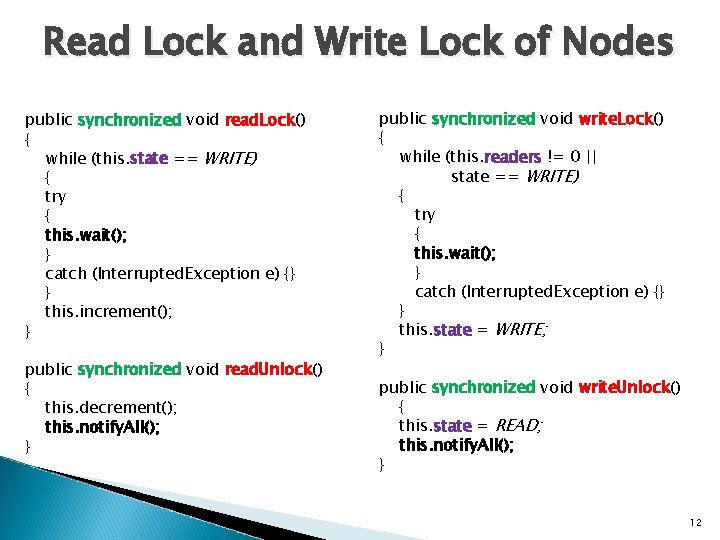
Read Lock and Write Lock of Nodes public synchronized void read. Lock() { while (this. state == WRITE) { try { this. wait(); } catch (Interrupted. Exception e) {} } this. increment(); } public synchronized void read. Unlock() { this. decrement(); this. notify. All(); } public synchronized void write. Lock() { while (this. readers != 0 || state == WRITE) { try { this. wait(); } catch (Interrupted. Exception e) {} } this. state = WRITE; } public synchronized void write. Unlock() { this. state = READ; this. notify. All(); } 12
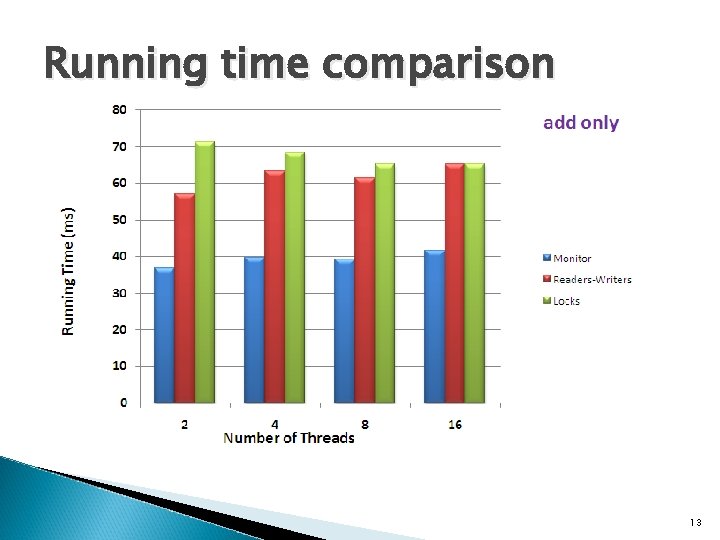
Running time comparison 13
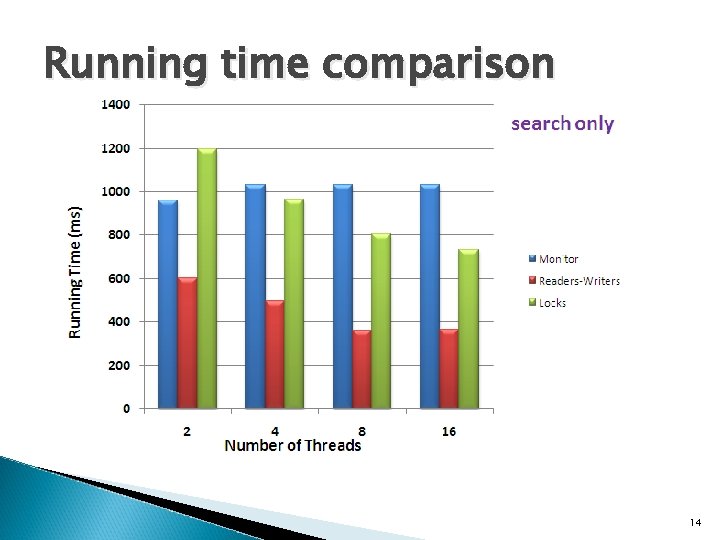
Running time comparison 14
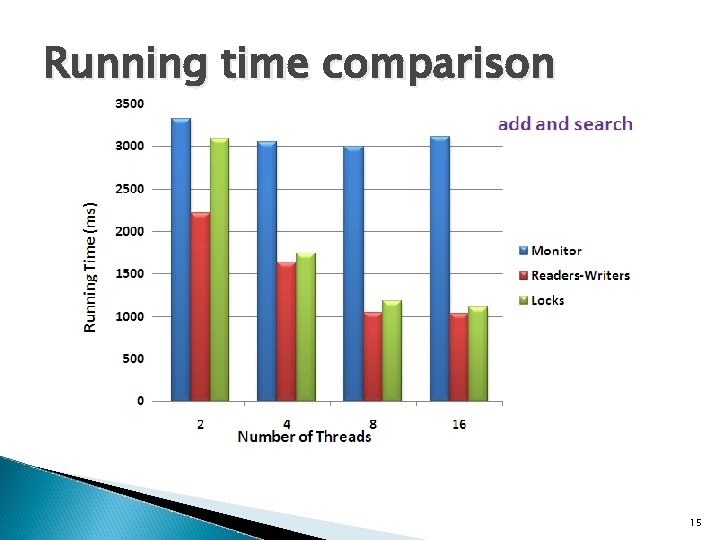
Running time comparison 15
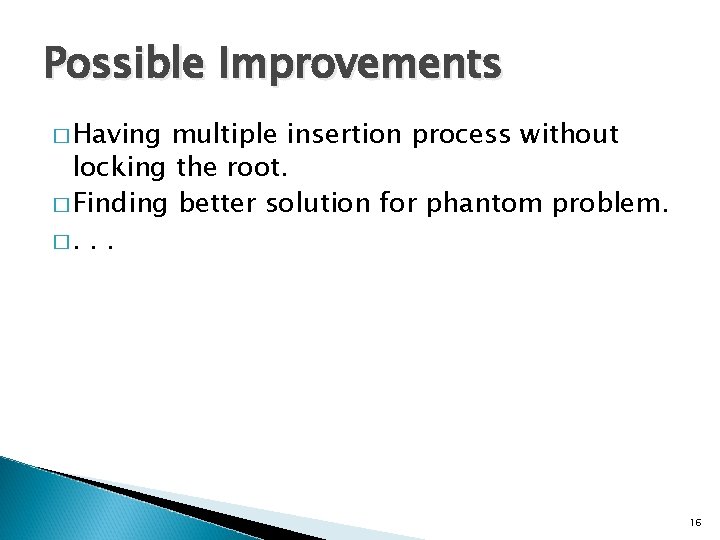
Possible Improvements � Having multiple insertion process without locking the root. � Finding better solution for phantom problem. �. . . 16
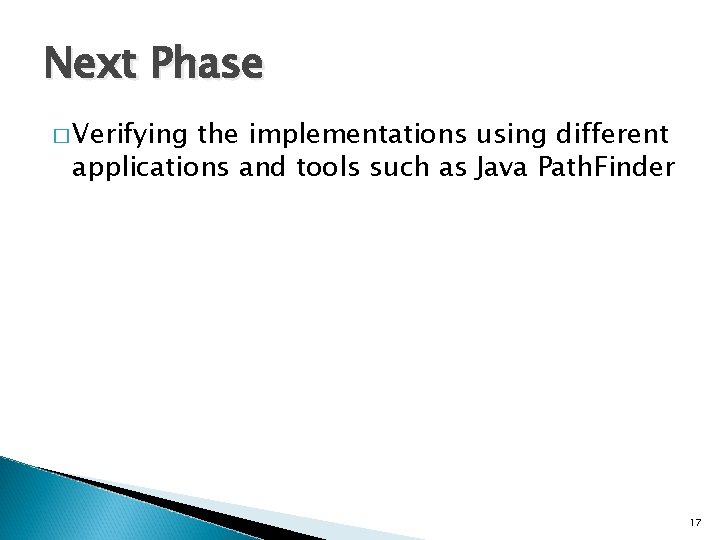
Next Phase � Verifying the implementations using different applications and tools such as Java Path. Finder 17
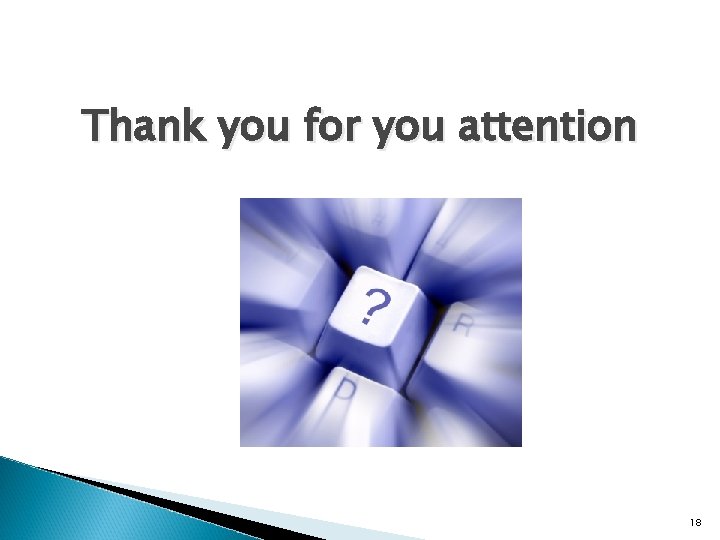
Thank you for you attention 18