ICOM 4015 Advanced Programming Lecture 15 Dynamic Memory
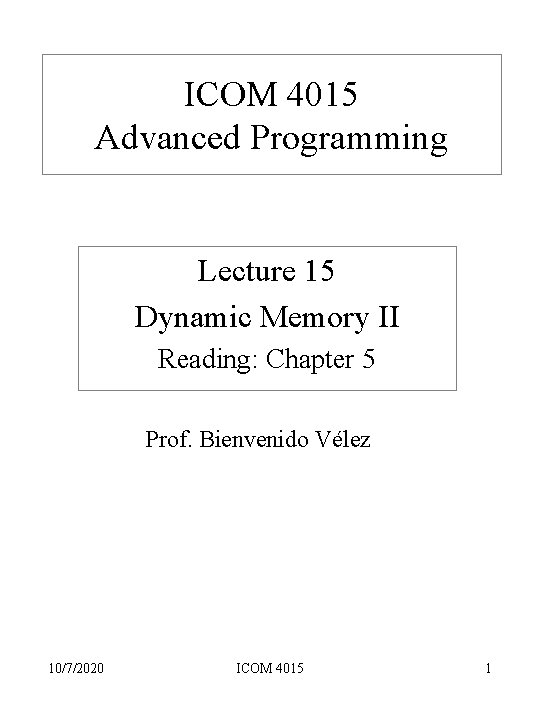
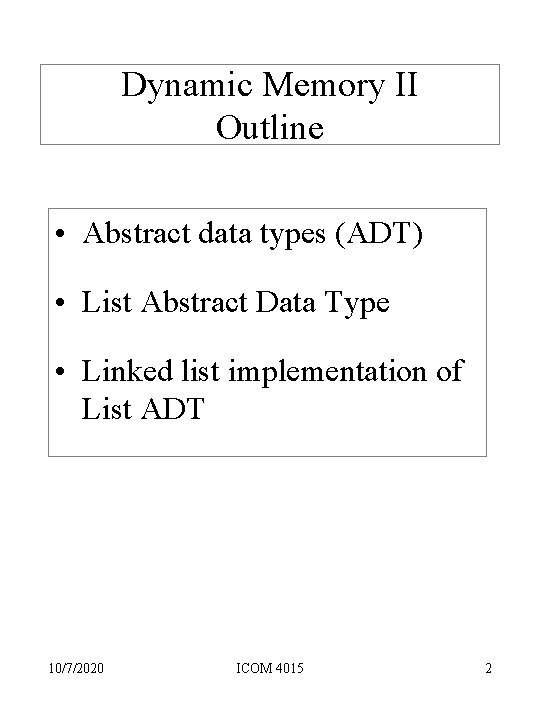
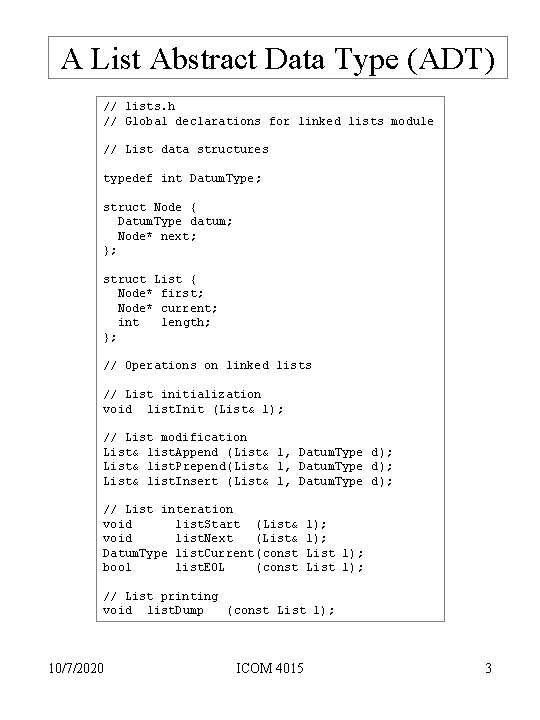
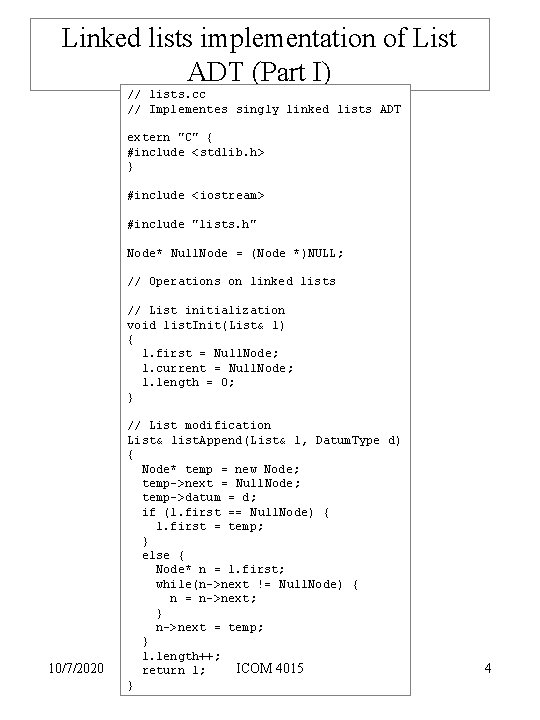
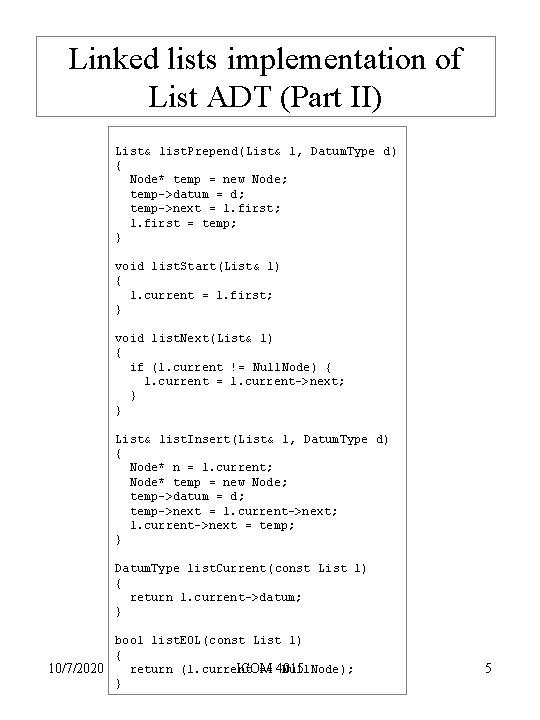
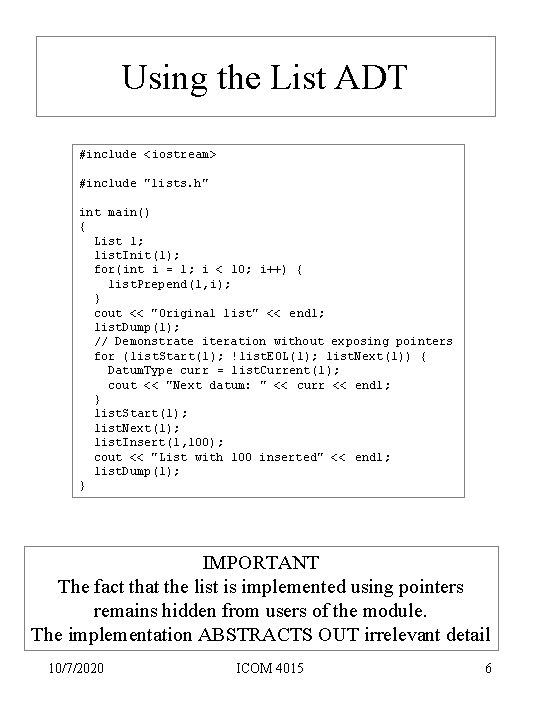
![List ADT Output [bvelez@amadeus] ~/icom 4015/lec 15 >>g++ lists. cc main. cc -o lists List ADT Output [bvelez@amadeus] ~/icom 4015/lec 15 >>g++ lists. cc main. cc -o lists](https://slidetodoc.com/presentation_image/fb95c12d31ad1584cd60a42b63e44b78/image-7.jpg)
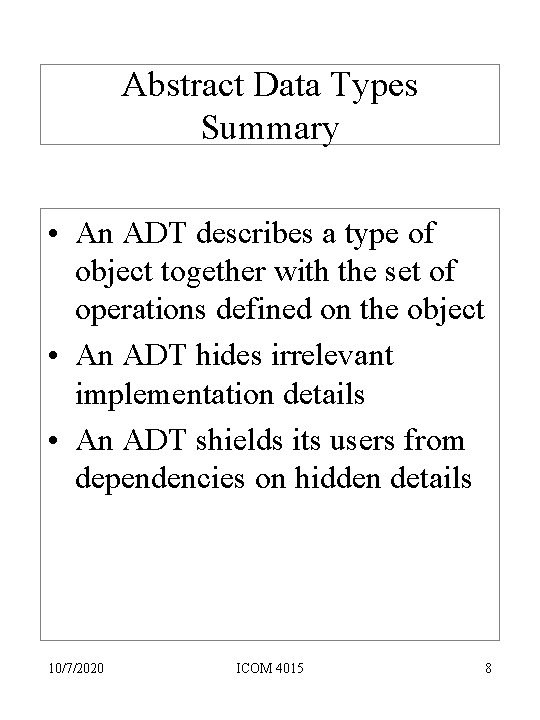
- Slides: 8
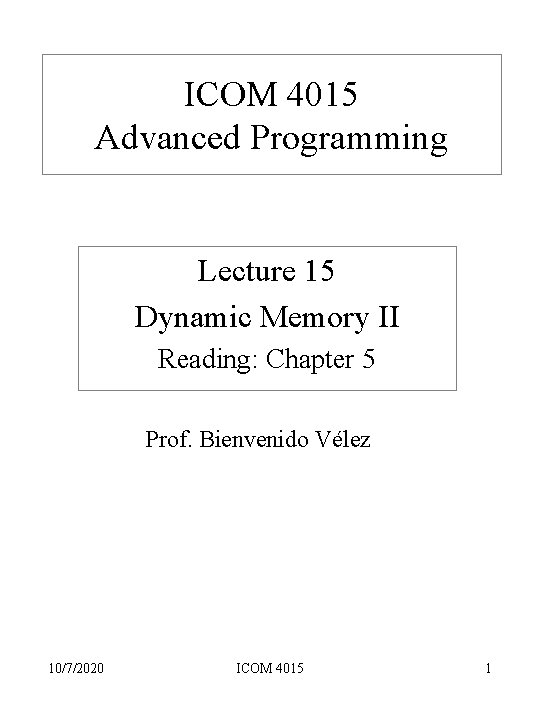
ICOM 4015 Advanced Programming Lecture 15 Dynamic Memory II Reading: Chapter 5 Prof. Bienvenido Vélez 10/7/2020 ICOM 4015 1
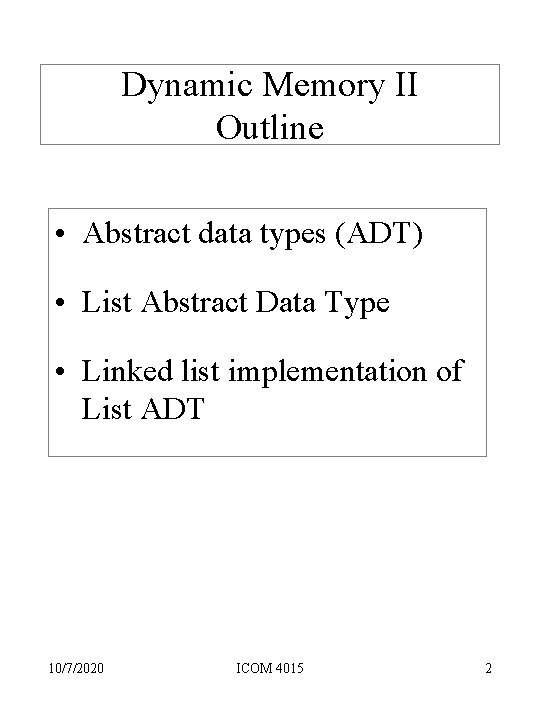
Dynamic Memory II Outline • Abstract data types (ADT) • List Abstract Data Type • Linked list implementation of List ADT 10/7/2020 ICOM 4015 2
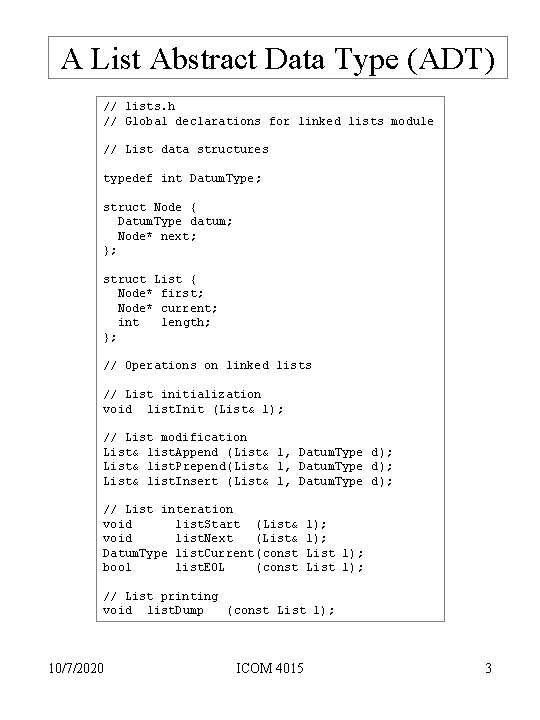
A List Abstract Data Type (ADT) // lists. h // Global declarations for linked lists module // List data structures typedef int Datum. Type; struct Node { Datum. Type datum; Node* next; }; struct List { Node* first; Node* current; int length; }; // Operations on linked lists // List initialization void list. Init (List& l); // List modification List& list. Append (List& l, Datum. Type d); List& list. Prepend(List& l, Datum. Type d); List& list. Insert (List& l, Datum. Type d); // List interation void list. Start (List& void list. Next (List& Datum. Type list. Current(const bool list. EOL (const l); List l); // List printing void list. Dump (const List l); 10/7/2020 ICOM 4015 3
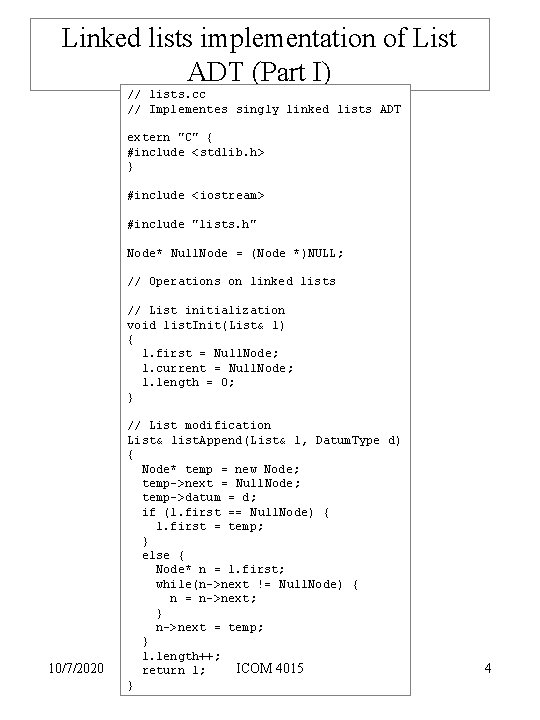
Linked lists implementation of List ADT (Part I) // lists. cc // Implementes singly linked lists ADT extern "C" { #include <stdlib. h> } #include <iostream> #include "lists. h" Node* Null. Node = (Node *)NULL; // Operations on linked lists // List initialization void list. Init(List& l) { l. first = Null. Node; l. current = Null. Node; l. length = 0; } 10/7/2020 // List modification List& list. Append(List& l, Datum. Type d) { Node* temp = new Node; temp->next = Null. Node; temp->datum = d; if (l. first == Null. Node) { l. first = temp; } else { Node* n = l. first; while(n->next != Null. Node) { n = n->next; } n->next = temp; } l. length++; ICOM 4015 return l; } 4
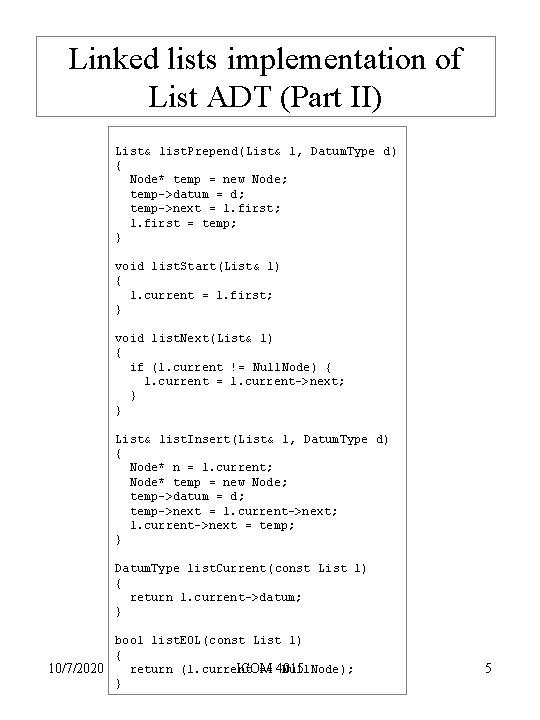
Linked lists implementation of List ADT (Part II) List& list. Prepend(List& l, Datum. Type d) { Node* temp = new Node; temp->datum = d; temp->next = l. first; l. first = temp; } void list. Start(List& l) { l. current = l. first; } void list. Next(List& l) { if (l. current != Null. Node) { l. current = l. current->next; } } List& list. Insert(List& l, Datum. Type d) { Node* n = l. current; Node* temp = new Node; temp->datum = d; temp->next = l. current->next; l. current->next = temp; } Datum. Type list. Current(const List l) { return l. current->datum; } bool list. EOL(const List l) { return (l. current == 4015 Null. Node); 10/7/2020 ICOM } 5
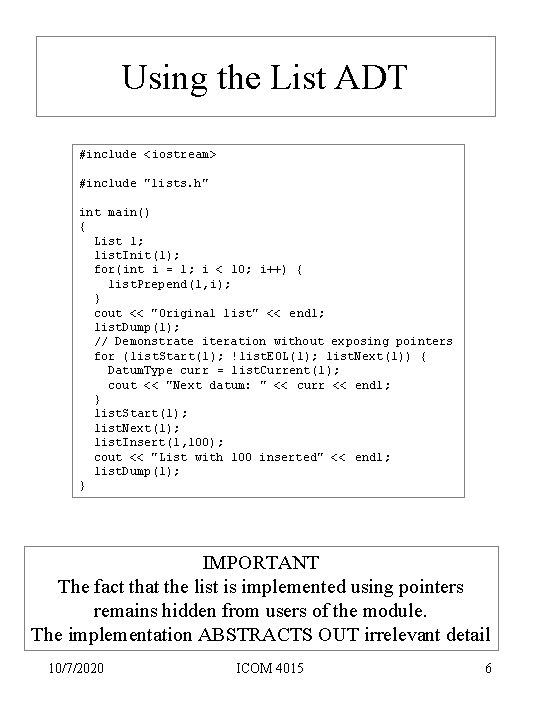
Using the List ADT #include <iostream> #include "lists. h" int main() { List l; list. Init(l); for(int i = 1; i < 10; i++) { list. Prepend(l, i); } cout << "Original list" << endl; list. Dump(l); // Demonstrate iteration without exposing pointers for (list. Start(l); !list. EOL(l); list. Next(l)) { Datum. Type curr = list. Current(l); cout << "Next datum: " << curr << endl; } list. Start(l); list. Next(l); list. Insert(l, 100); cout << "List with 100 inserted" << endl; list. Dump(l); } IMPORTANT The fact that the list is implemented using pointers remains hidden from users of the module. The implementation ABSTRACTS OUT irrelevant detail 10/7/2020 ICOM 4015 6
![List ADT Output bvelezamadeus icom 4015lec 15 g lists cc main cc o lists List ADT Output [bvelez@amadeus] ~/icom 4015/lec 15 >>g++ lists. cc main. cc -o lists](https://slidetodoc.com/presentation_image/fb95c12d31ad1584cd60a42b63e44b78/image-7.jpg)
List ADT Output [bvelez@amadeus] ~/icom 4015/lec 15 >>g++ lists. cc main. cc -o lists [bvelez@amadeus] ~/icom 4015/lec 15 >>lists Original list 9 8 7 6 5 4 3 2 1 Next datum: 9 Next datum: 8 Next datum: 7 Next datum: 6 Next datum: 5 Next datum: 4 Next datum: 3 Next datum: 2 Next datum: 1 List with 100 inserted 9 8 100 7 6 5 4 3 2 1 [bvelez@amadeus] ~/icom 4015/lec 15 >> 10/7/2020 ICOM 4015 7
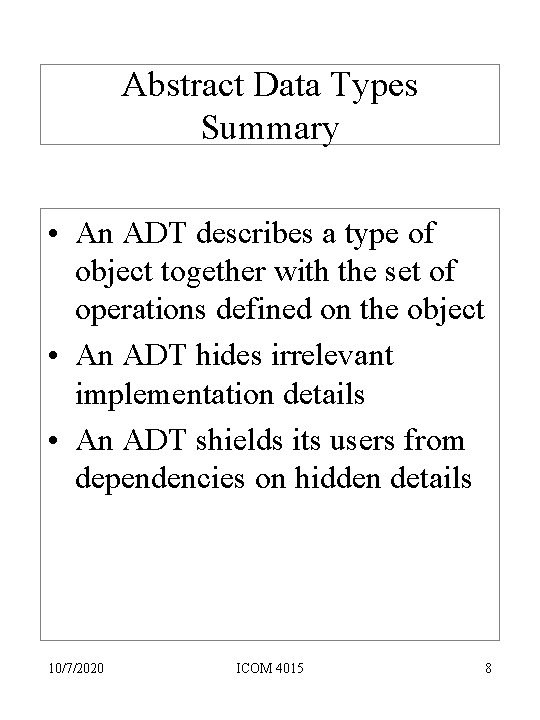
Abstract Data Types Summary • An ADT describes a type of object together with the set of operations defined on the object • An ADT hides irrelevant implementation details • An ADT shields its users from dependencies on hidden details 10/7/2020 ICOM 4015 8