How to Write a Mouse Listener Mouse events
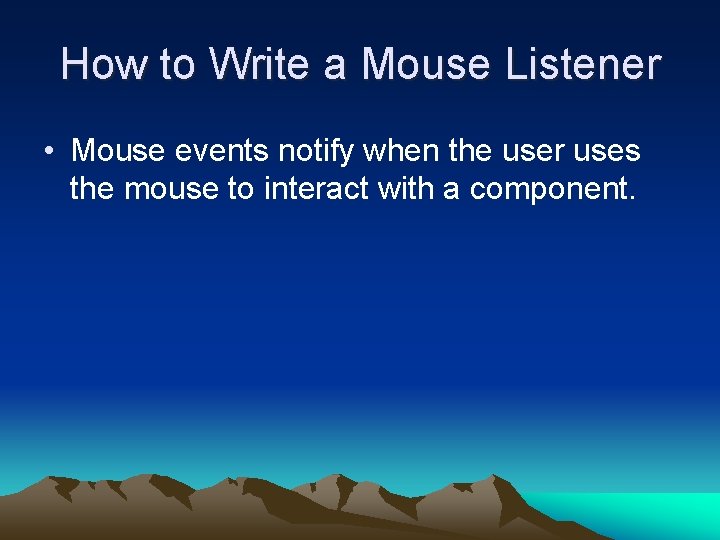
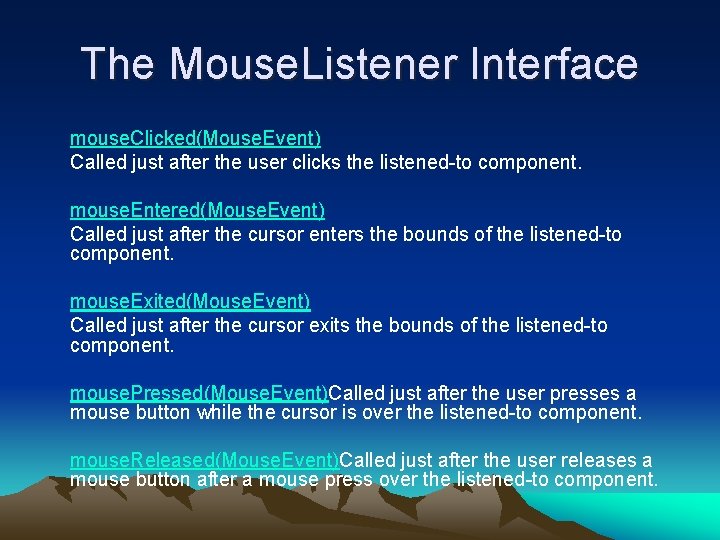
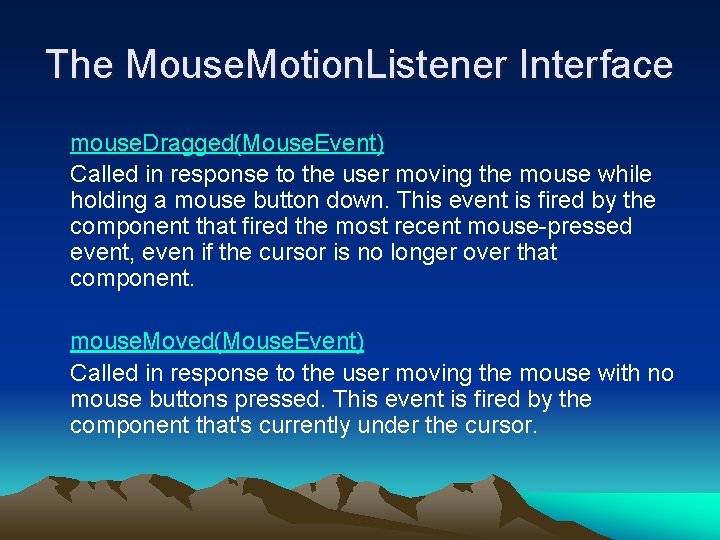
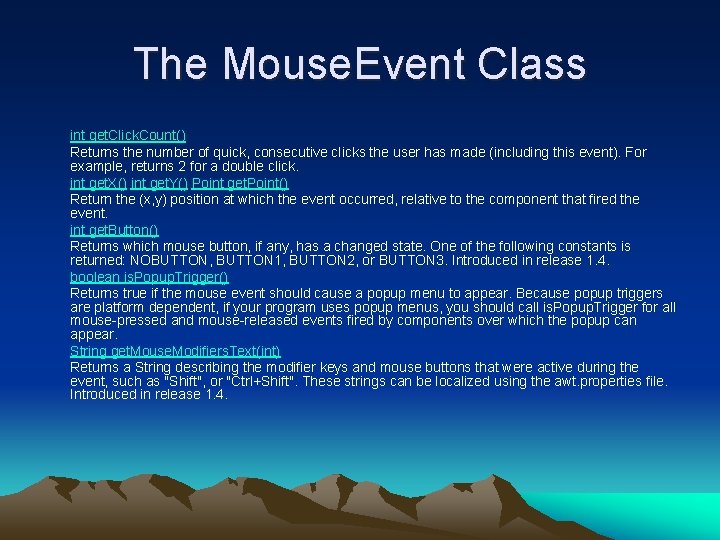
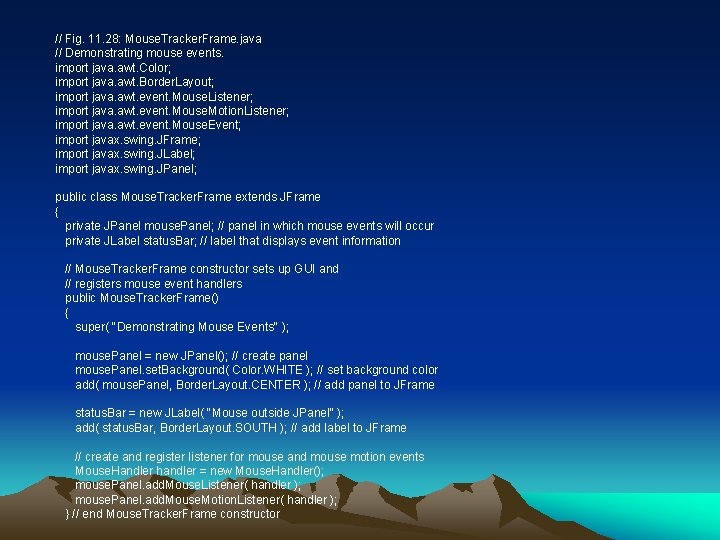
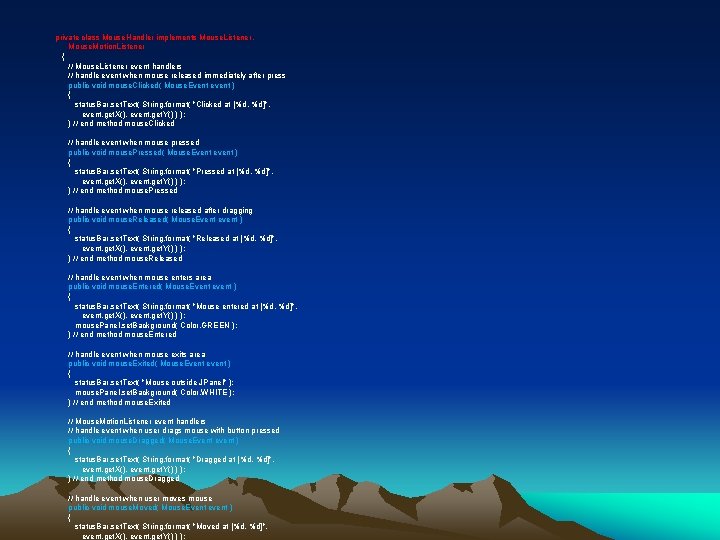
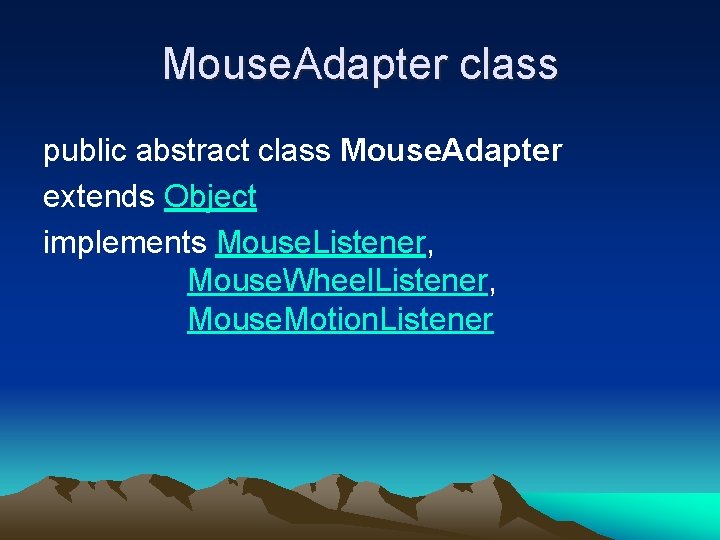
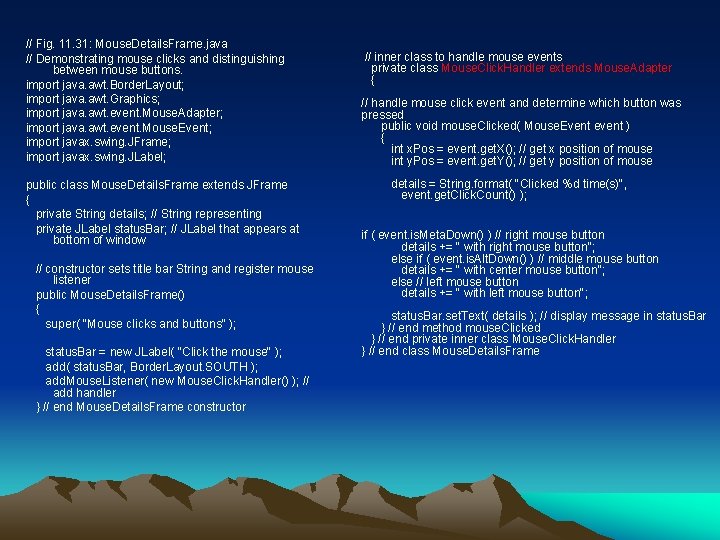
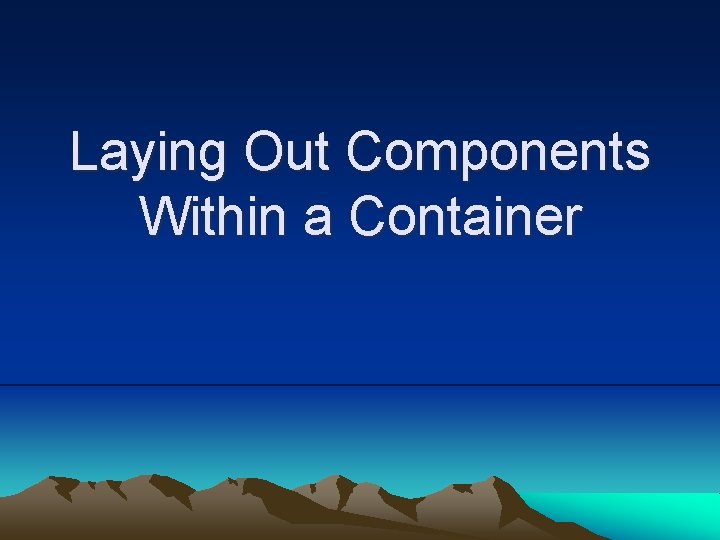
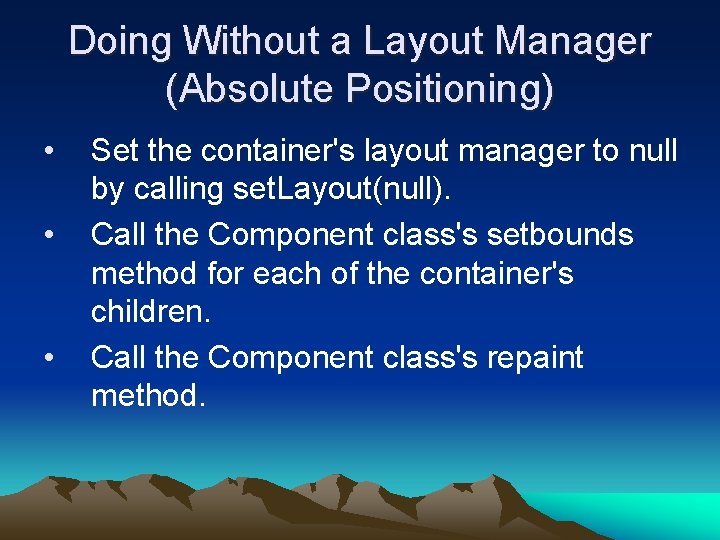
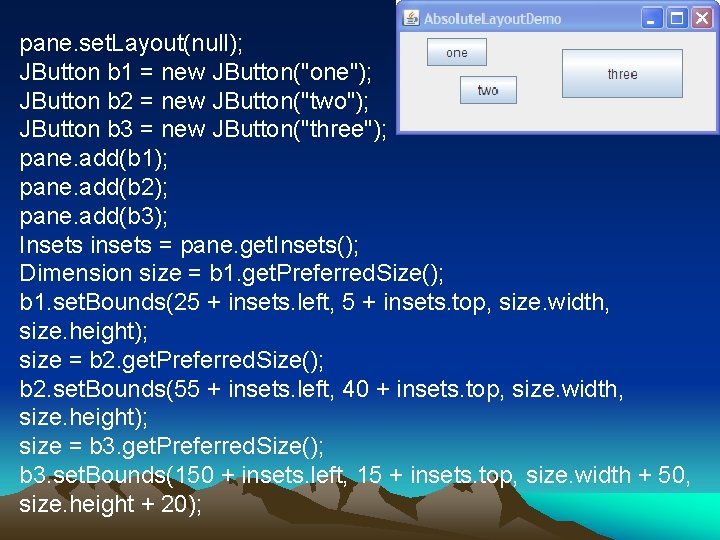
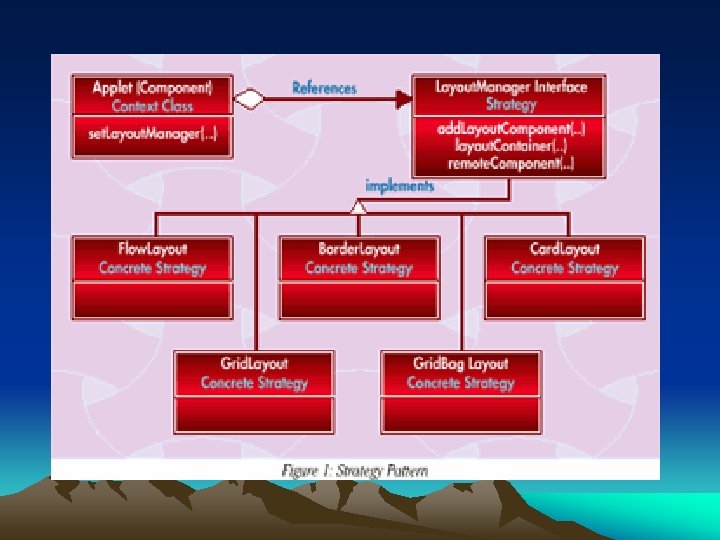
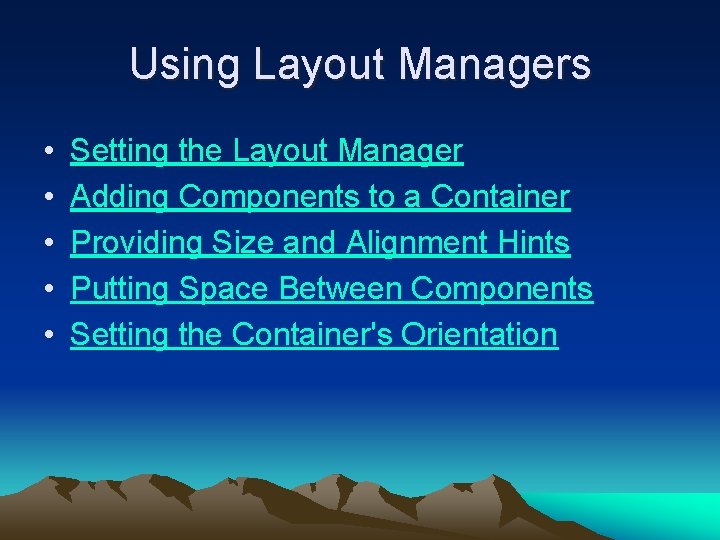
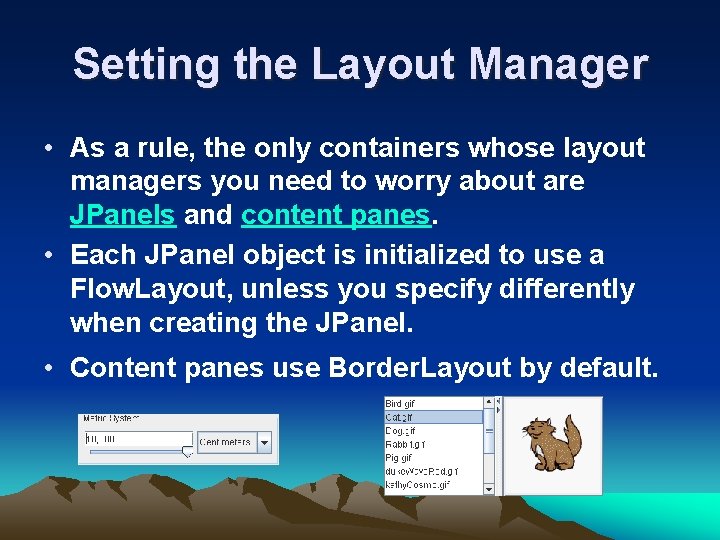
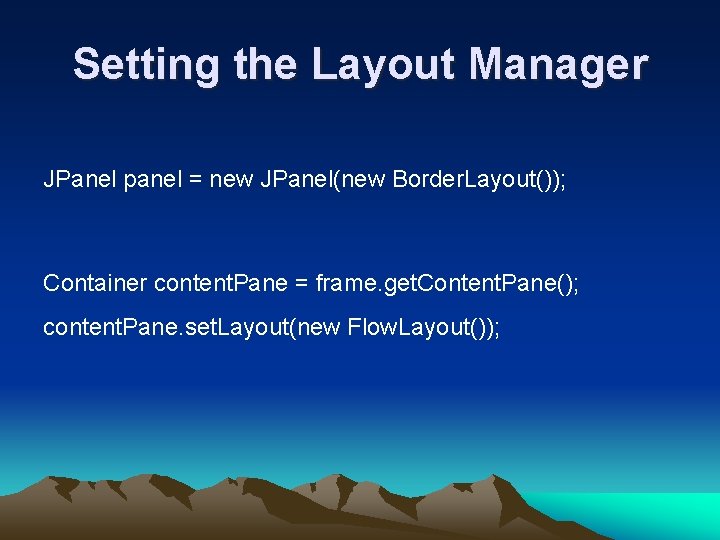
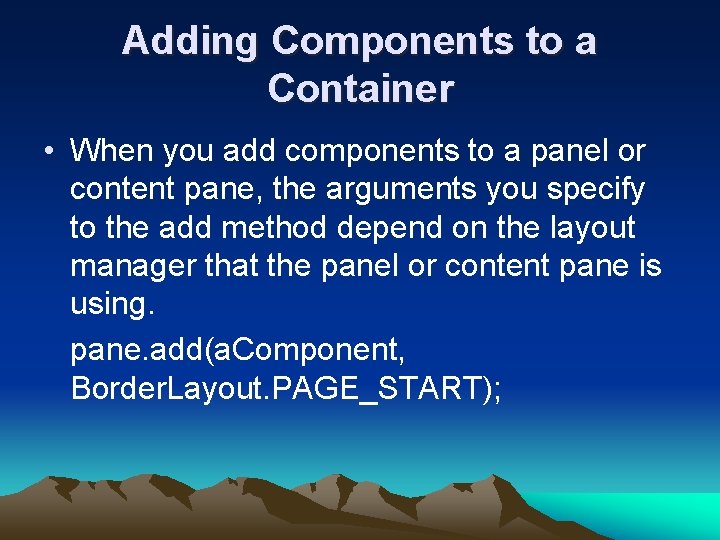
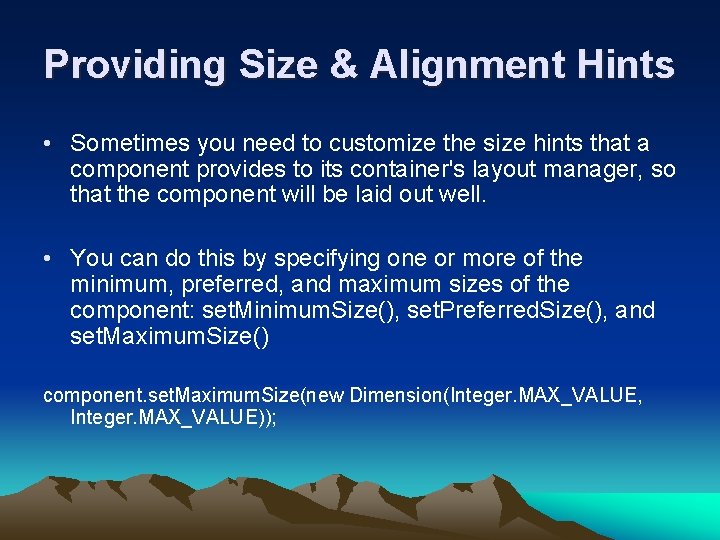
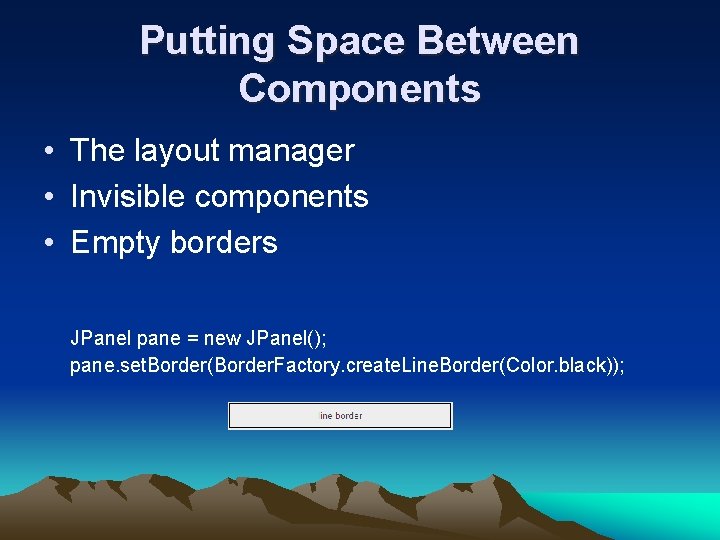
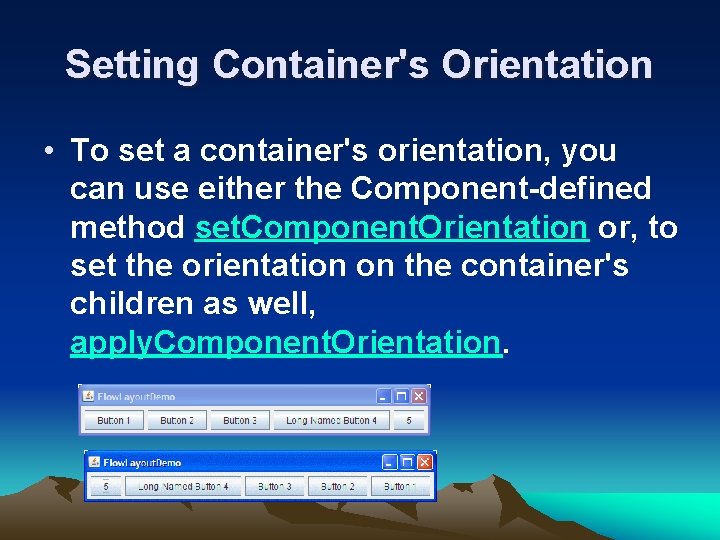
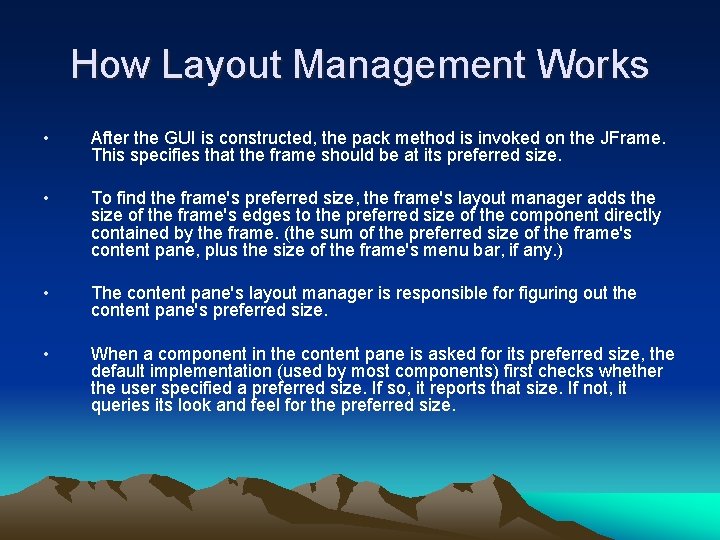
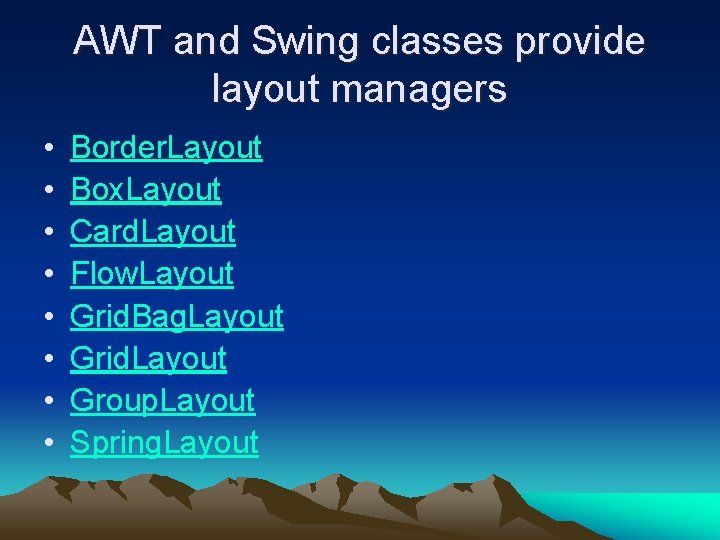
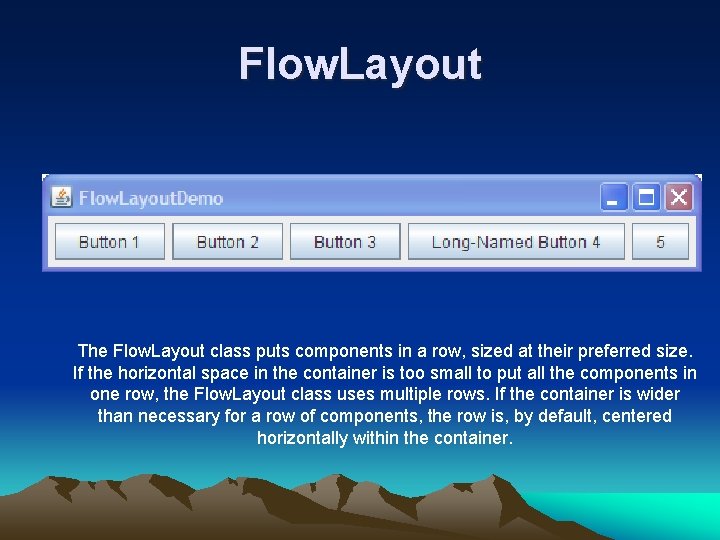
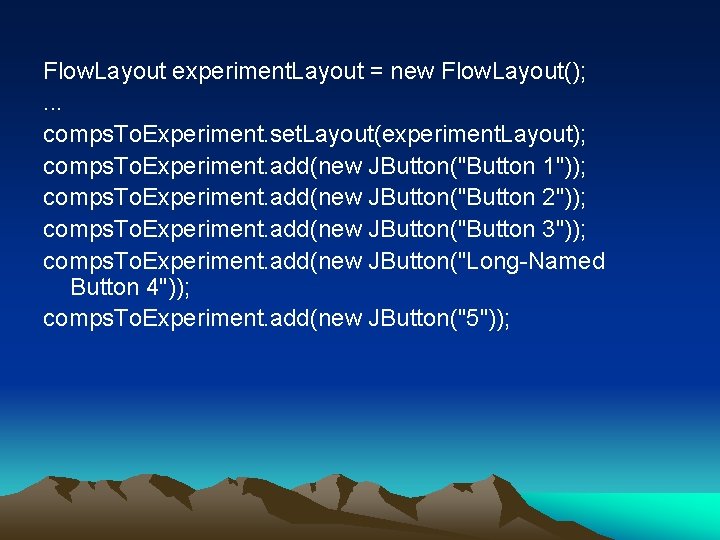
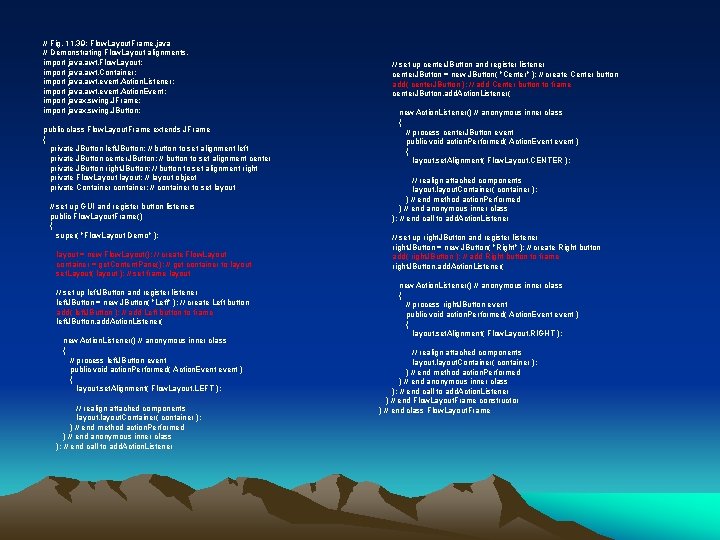
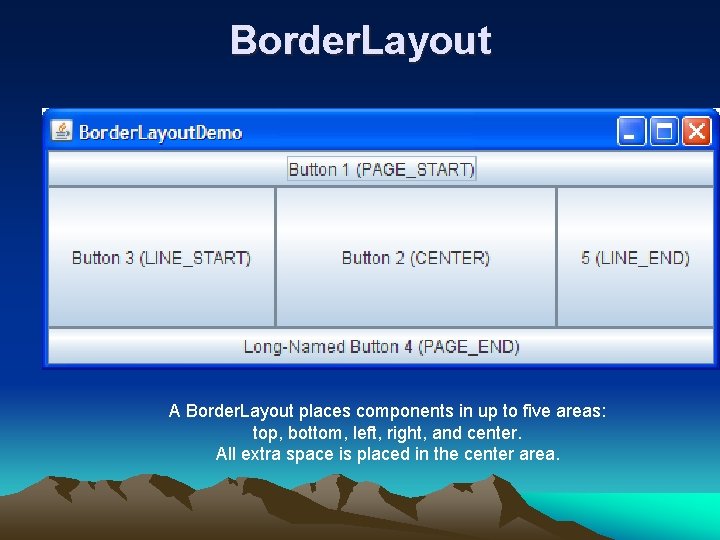
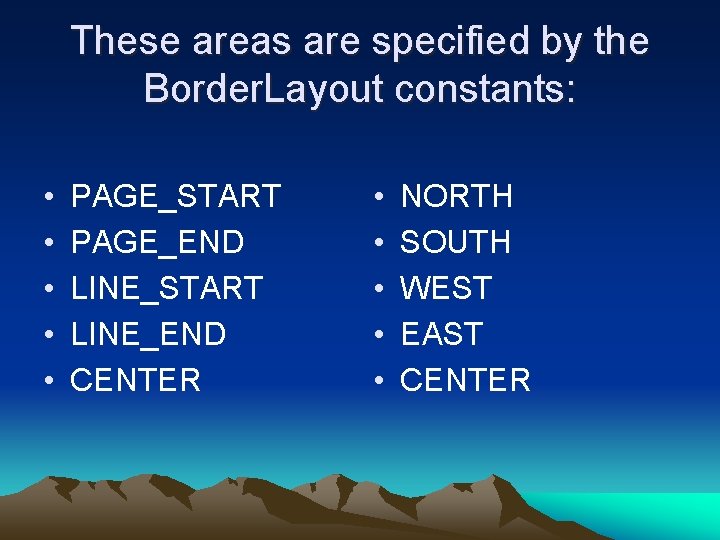
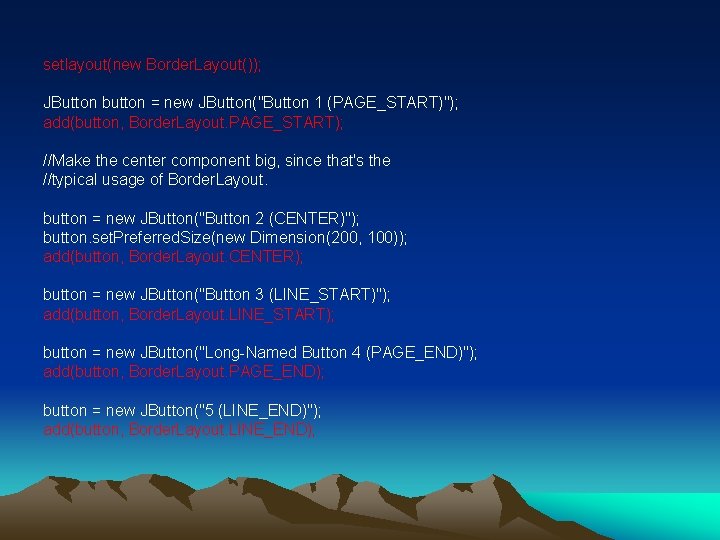
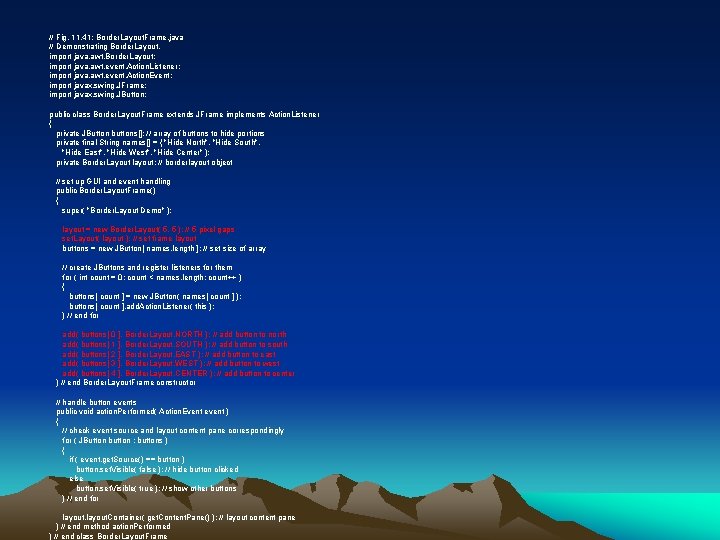
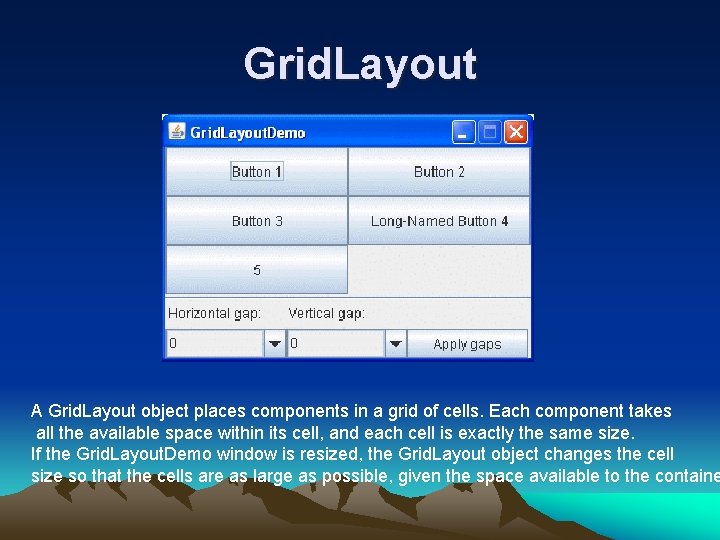
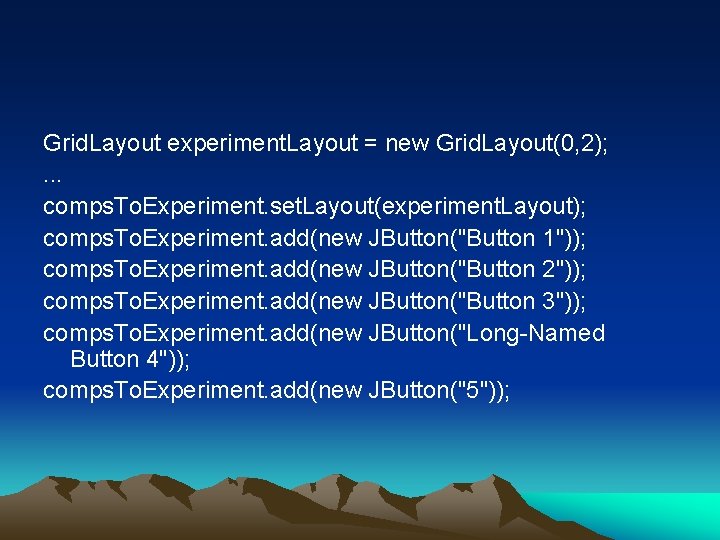
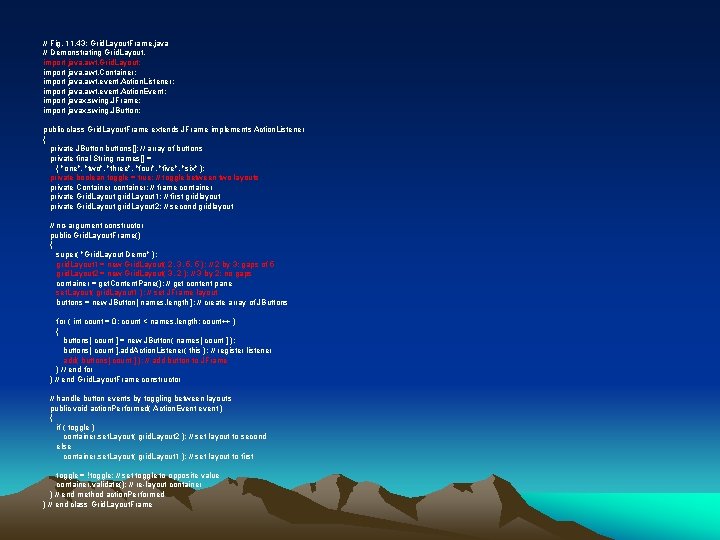
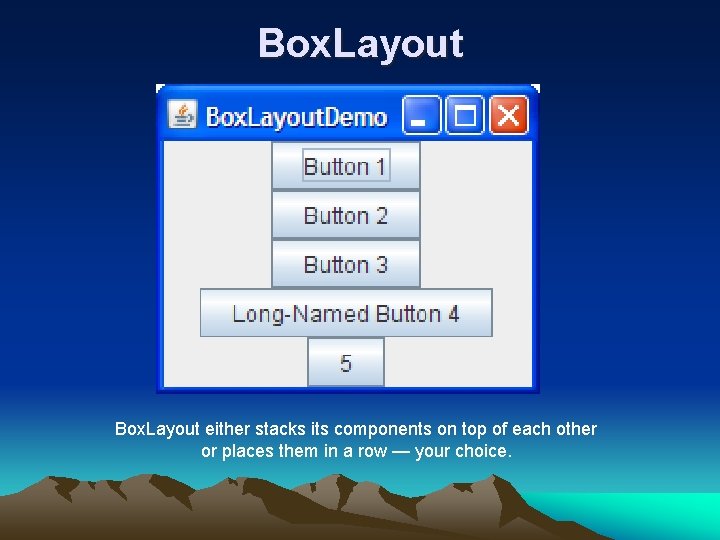
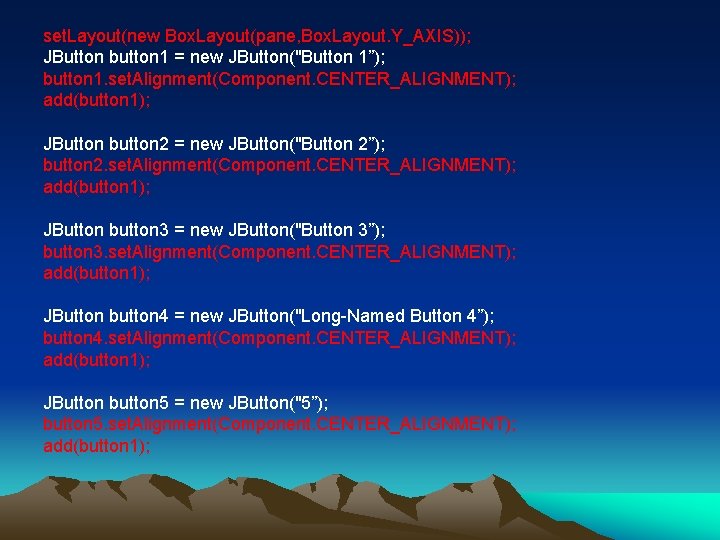
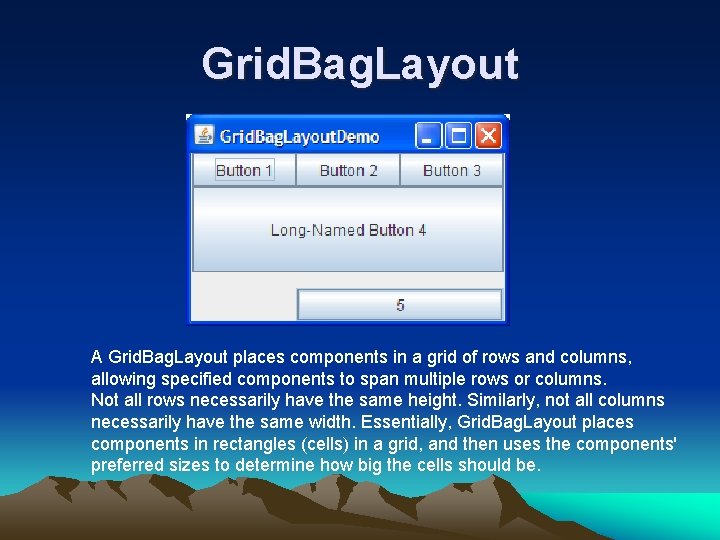
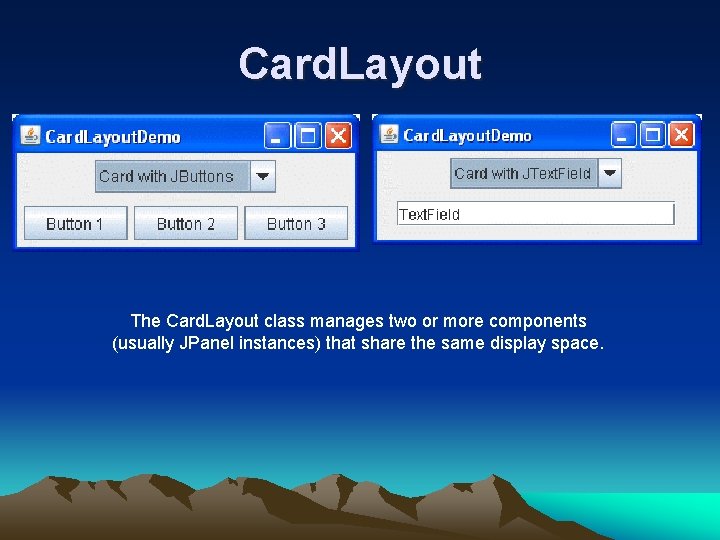
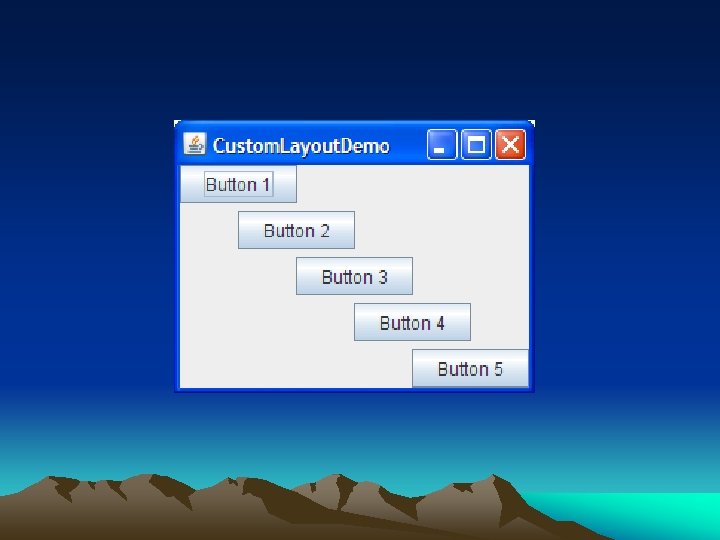
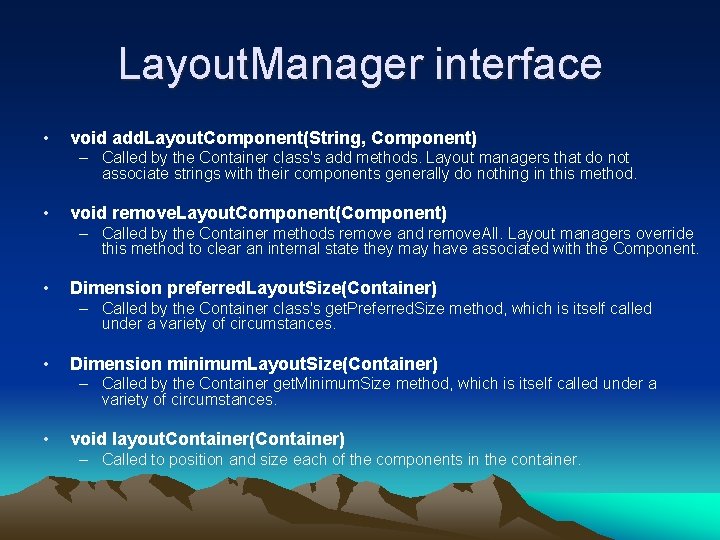
- Slides: 37
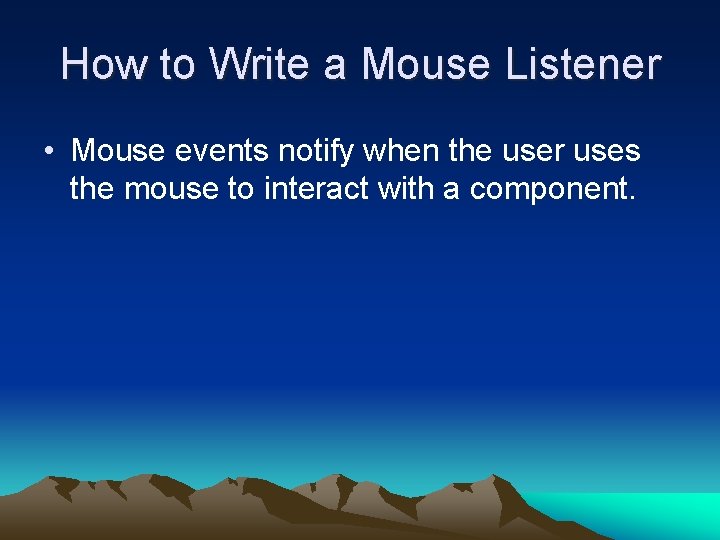
How to Write a Mouse Listener • Mouse events notify when the user uses the mouse to interact with a component.
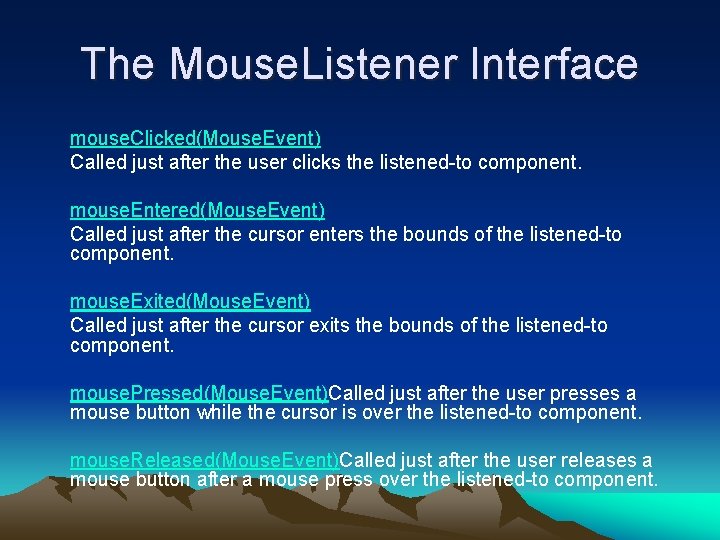
The Mouse. Listener Interface mouse. Clicked(Mouse. Event) Called just after the user clicks the listened-to component. mouse. Entered(Mouse. Event) Called just after the cursor enters the bounds of the listened-to component. mouse. Exited(Mouse. Event) Called just after the cursor exits the bounds of the listened-to component. mouse. Pressed(Mouse. Event)Called just after the user presses a mouse button while the cursor is over the listened-to component. mouse. Released(Mouse. Event)Called just after the user releases a mouse button after a mouse press over the listened-to component.
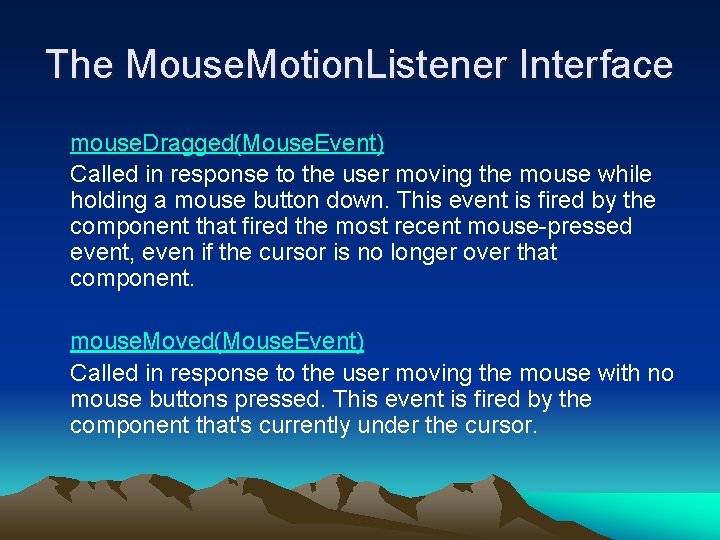
The Mouse. Motion. Listener Interface mouse. Dragged(Mouse. Event) Called in response to the user moving the mouse while holding a mouse button down. This event is fired by the component that fired the most recent mouse-pressed event, even if the cursor is no longer over that component. mouse. Moved(Mouse. Event) Called in response to the user moving the mouse with no mouse buttons pressed. This event is fired by the component that's currently under the cursor.
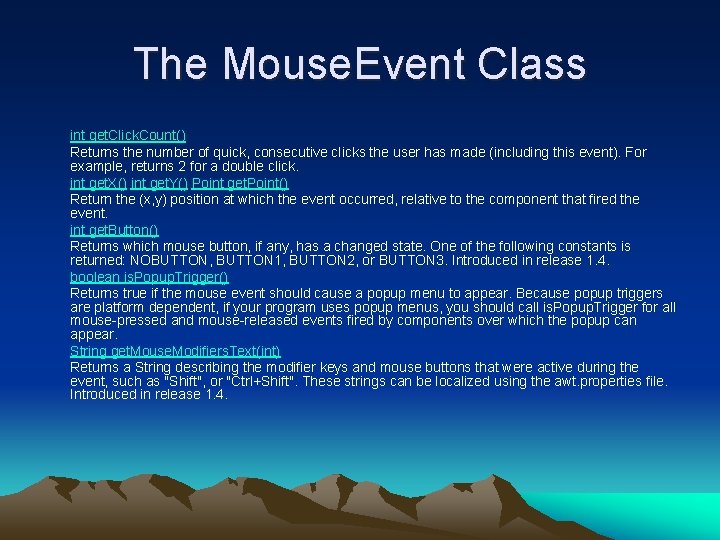
The Mouse. Event Class int get. Click. Count() Returns the number of quick, consecutive clicks the user has made (including this event). For example, returns 2 for a double click. int get. X() int get. Y() Point get. Point() Return the (x, y) position at which the event occurred, relative to the component that fired the event. int get. Button() Returns which mouse button, if any, has a changed state. One of the following constants is returned: NOBUTTON, BUTTON 1, BUTTON 2, or BUTTON 3. Introduced in release 1. 4. boolean is. Popup. Trigger() Returns true if the mouse event should cause a popup menu to appear. Because popup triggers are platform dependent, if your program uses popup menus, you should call is. Popup. Trigger for all mouse-pressed and mouse-released events fired by components over which the popup can appear. String get. Mouse. Modifiers. Text(int) Returns a String describing the modifier keys and mouse buttons that were active during the event, such as "Shift", or "Ctrl+Shift". These strings can be localized using the awt. properties file. Introduced in release 1. 4.
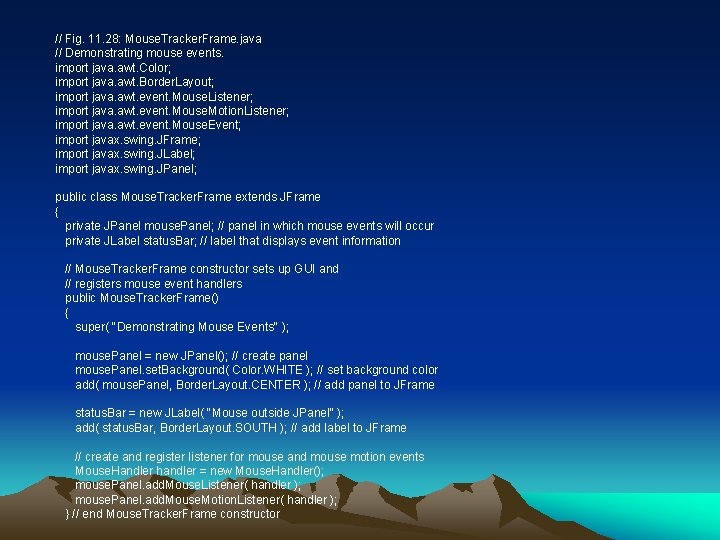
// Fig. 11. 28: Mouse. Tracker. Frame. java // Demonstrating mouse events. import java. awt. Color; import java. awt. Border. Layout; import java. awt. event. Mouse. Listener; import java. awt. event. Mouse. Motion. Listener; import java. awt. event. Mouse. Event; import javax. swing. JFrame; import javax. swing. JLabel; import javax. swing. JPanel; public class Mouse. Tracker. Frame extends JFrame { private JPanel mouse. Panel; // panel in which mouse events will occur private JLabel status. Bar; // label that displays event information // Mouse. Tracker. Frame constructor sets up GUI and // registers mouse event handlers public Mouse. Tracker. Frame() { super( "Demonstrating Mouse Events" ); mouse. Panel = new JPanel(); // create panel mouse. Panel. set. Background( Color. WHITE ); // set background color add( mouse. Panel, Border. Layout. CENTER ); // add panel to JFrame status. Bar = new JLabel( "Mouse outside JPanel" ); add( status. Bar, Border. Layout. SOUTH ); // add label to JFrame // create and register listener for mouse and mouse motion events Mouse. Handler handler = new Mouse. Handler(); mouse. Panel. add. Mouse. Listener( handler ); mouse. Panel. add. Mouse. Motion. Listener( handler ); } // end Mouse. Tracker. Frame constructor
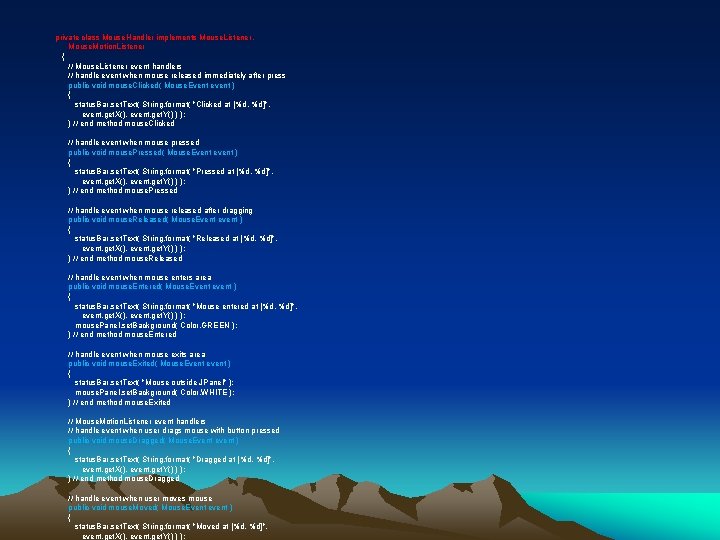
private class Mouse. Handler implements Mouse. Listener, Mouse. Motion. Listener { // Mouse. Listener event handlers // handle event when mouse released immediately after press public void mouse. Clicked( Mouse. Event event ) { status. Bar. set. Text( String. format( "Clicked at [%d, %d]", event. get. X(), event. get. Y() ) ); } // end method mouse. Clicked // handle event when mouse pressed public void mouse. Pressed( Mouse. Event event ) { status. Bar. set. Text( String. format( "Pressed at [%d, %d]", event. get. X(), event. get. Y() ) ); } // end method mouse. Pressed // handle event when mouse released after dragging public void mouse. Released( Mouse. Event event ) { status. Bar. set. Text( String. format( "Released at [%d, %d]", event. get. X(), event. get. Y() ) ); } // end method mouse. Released // handle event when mouse enters area public void mouse. Entered( Mouse. Event event ) { status. Bar. set. Text( String. format( "Mouse entered at [%d, %d]", event. get. X(), event. get. Y() ) ); mouse. Panel. set. Background( Color. GREEN ); } // end method mouse. Entered // handle event when mouse exits area public void mouse. Exited( Mouse. Event event ) { status. Bar. set. Text( "Mouse outside JPanel" ); mouse. Panel. set. Background( Color. WHITE ); } // end method mouse. Exited // Mouse. Motion. Listener event handlers // handle event when user drags mouse with button pressed public void mouse. Dragged( Mouse. Event event ) { status. Bar. set. Text( String. format( "Dragged at [%d, %d]", event. get. X(), event. get. Y() ) ); } // end method mouse. Dragged // handle event when user moves mouse public void mouse. Moved( Mouse. Event event ) { status. Bar. set. Text( String. format( "Moved at [%d, %d]", event. get. X(), event. get. Y() ) );
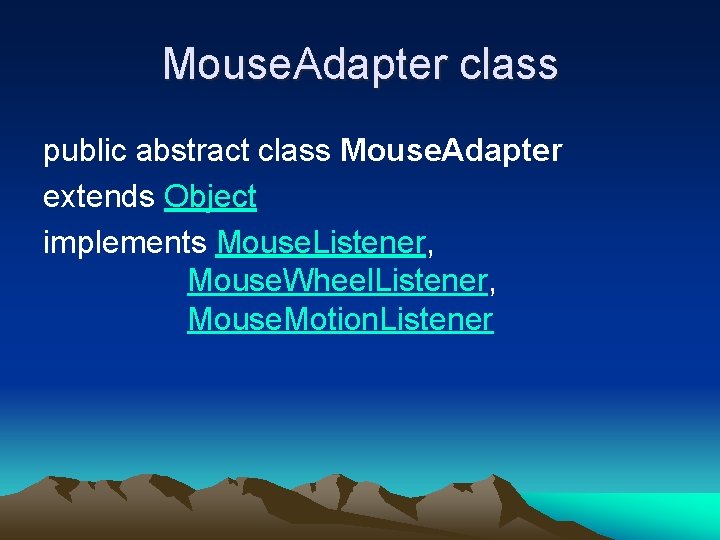
Mouse. Adapter class public abstract class Mouse. Adapter extends Object implements Mouse. Listener, Mouse. Wheel. Listener, Mouse. Motion. Listener
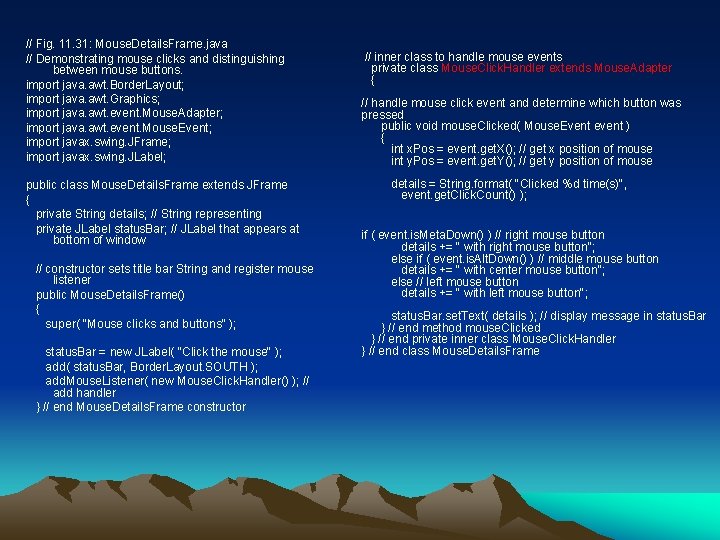
// Fig. 11. 31: Mouse. Details. Frame. java // Demonstrating mouse clicks and distinguishing between mouse buttons. import java. awt. Border. Layout; import java. awt. Graphics; import java. awt. event. Mouse. Adapter; import java. awt. event. Mouse. Event; import javax. swing. JFrame; import javax. swing. JLabel; public class Mouse. Details. Frame extends JFrame { private String details; // String representing private JLabel status. Bar; // JLabel that appears at bottom of window // constructor sets title bar String and register mouse listener public Mouse. Details. Frame() { super( "Mouse clicks and buttons" ); status. Bar = new JLabel( "Click the mouse" ); add( status. Bar, Border. Layout. SOUTH ); add. Mouse. Listener( new Mouse. Click. Handler() ); // add handler } // end Mouse. Details. Frame constructor // inner class to handle mouse events private class Mouse. Click. Handler extends Mouse. Adapter { // handle mouse click event and determine which button was pressed public void mouse. Clicked( Mouse. Event event ) { int x. Pos = event. get. X(); // get x position of mouse int y. Pos = event. get. Y(); // get y position of mouse details = String. format( "Clicked %d time(s)", event. get. Click. Count() ); if ( event. is. Meta. Down() ) // right mouse button details += " with right mouse button"; else if ( event. is. Alt. Down() ) // middle mouse button details += " with center mouse button"; else // left mouse button details += " with left mouse button"; status. Bar. set. Text( details ); // display message in status. Bar } // end method mouse. Clicked } // end private inner class Mouse. Click. Handler } // end class Mouse. Details. Frame
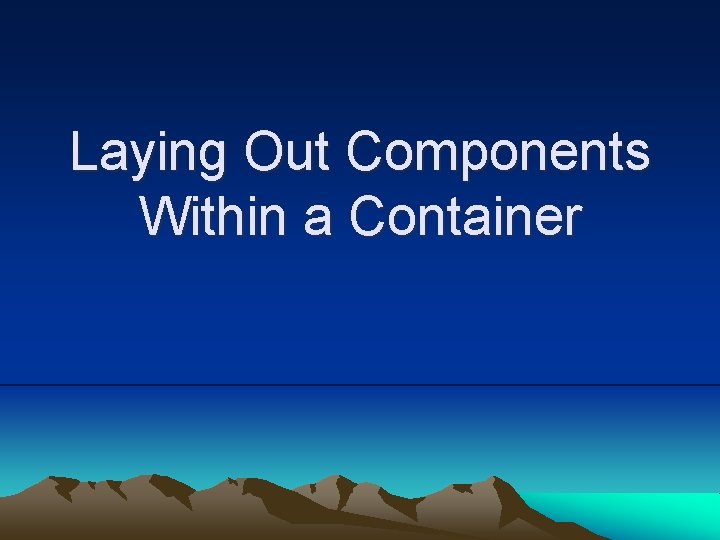
Laying Out Components Within a Container
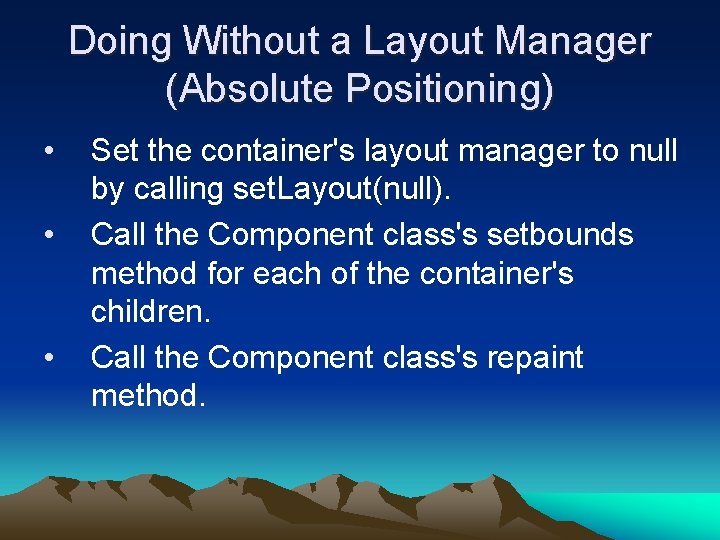
Doing Without a Layout Manager (Absolute Positioning) • • • Set the container's layout manager to null by calling set. Layout(null). Call the Component class's setbounds method for each of the container's children. Call the Component class's repaint method.
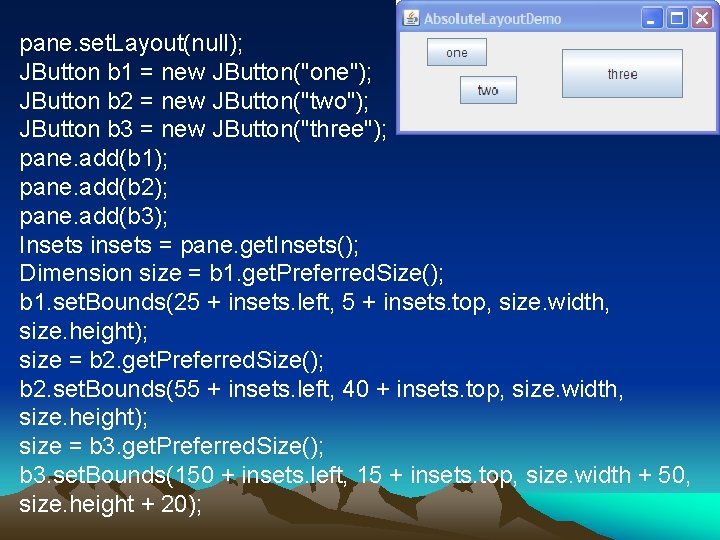
pane. set. Layout(null); JButton b 1 = new JButton("one"); JButton b 2 = new JButton("two"); JButton b 3 = new JButton("three"); pane. add(b 1); pane. add(b 2); pane. add(b 3); Insets insets = pane. get. Insets(); Dimension size = b 1. get. Preferred. Size(); b 1. set. Bounds(25 + insets. left, 5 + insets. top, size. width, size. height); size = b 2. get. Preferred. Size(); b 2. set. Bounds(55 + insets. left, 40 + insets. top, size. width, size. height); size = b 3. get. Preferred. Size(); b 3. set. Bounds(150 + insets. left, 15 + insets. top, size. width + 50, size. height + 20);
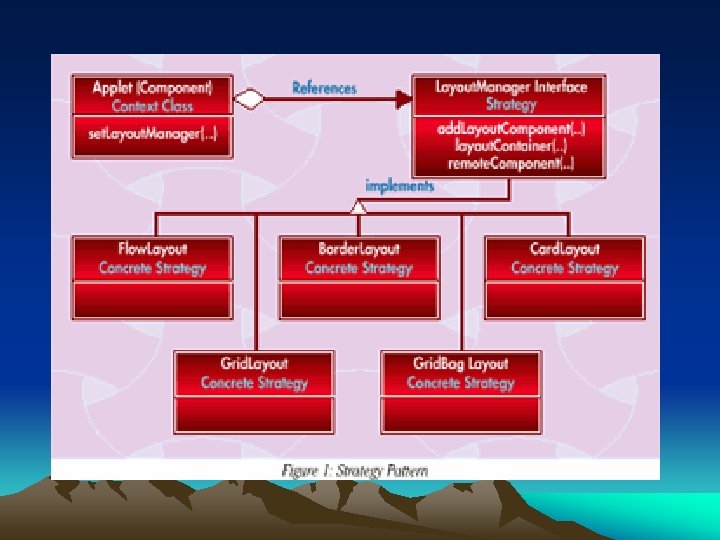
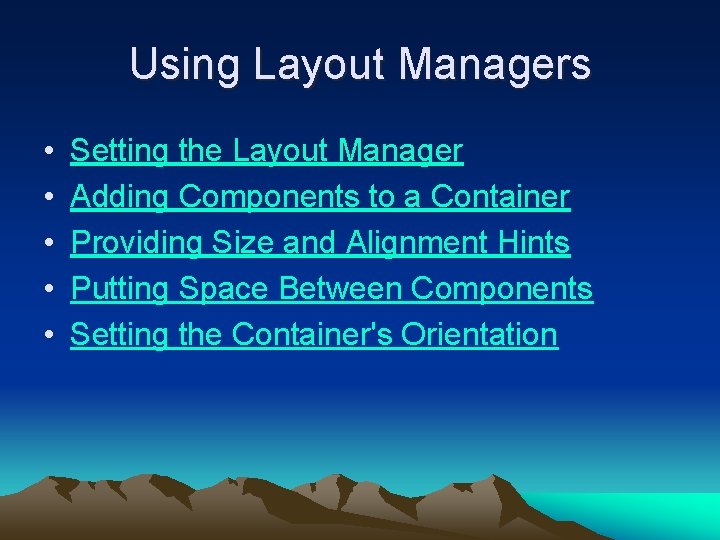
Using Layout Managers • • • Setting the Layout Manager Adding Components to a Container Providing Size and Alignment Hints Putting Space Between Components Setting the Container's Orientation
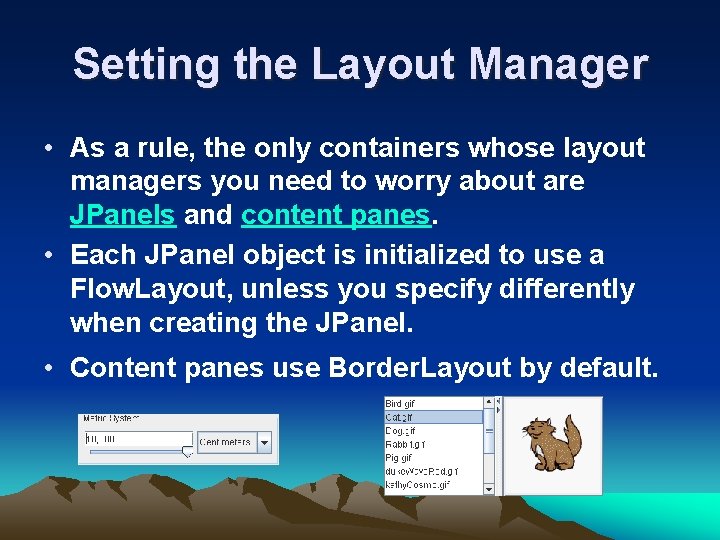
Setting the Layout Manager • As a rule, the only containers whose layout managers you need to worry about are JPanels and content panes. • Each JPanel object is initialized to use a Flow. Layout, unless you specify differently when creating the JPanel. • Content panes use Border. Layout by default.
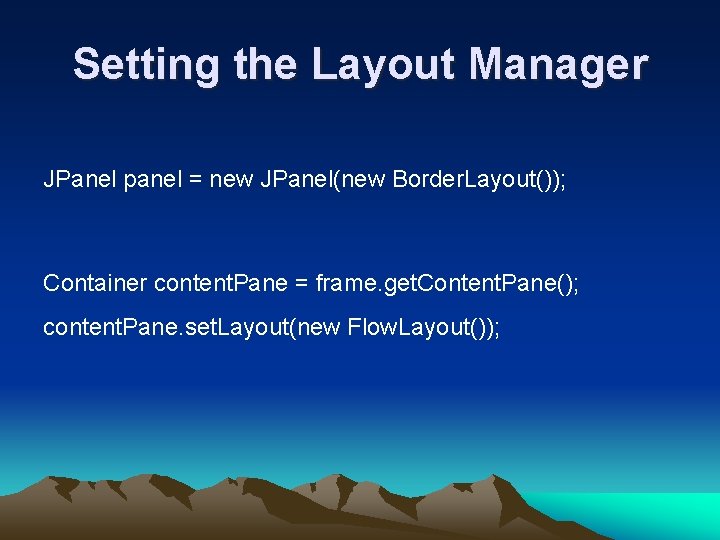
Setting the Layout Manager JPanel panel = new JPanel(new Border. Layout()); Container content. Pane = frame. get. Content. Pane(); content. Pane. set. Layout(new Flow. Layout());
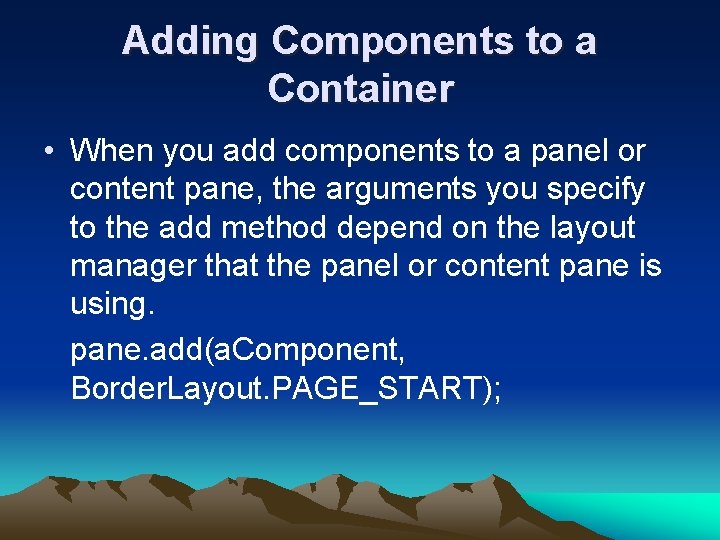
Adding Components to a Container • When you add components to a panel or content pane, the arguments you specify to the add method depend on the layout manager that the panel or content pane is using. pane. add(a. Component, Border. Layout. PAGE_START);
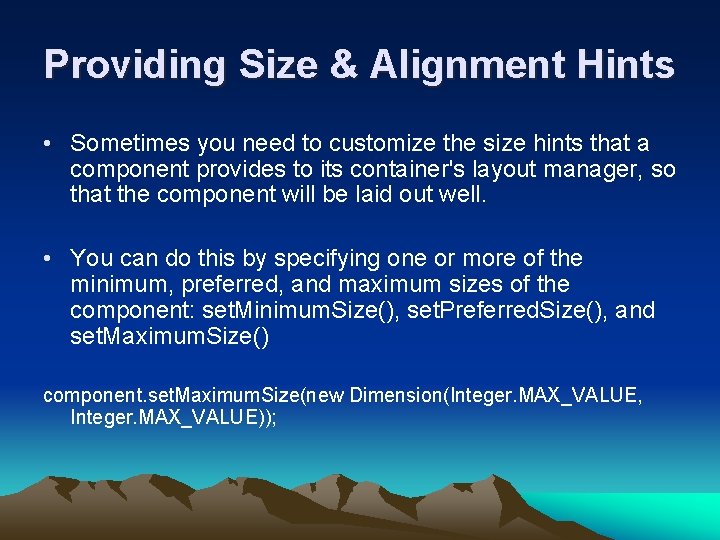
Providing Size & Alignment Hints • Sometimes you need to customize the size hints that a component provides to its container's layout manager, so that the component will be laid out well. • You can do this by specifying one or more of the minimum, preferred, and maximum sizes of the component: set. Minimum. Size(), set. Preferred. Size(), and set. Maximum. Size() component. set. Maximum. Size(new Dimension(Integer. MAX_VALUE, Integer. MAX_VALUE));
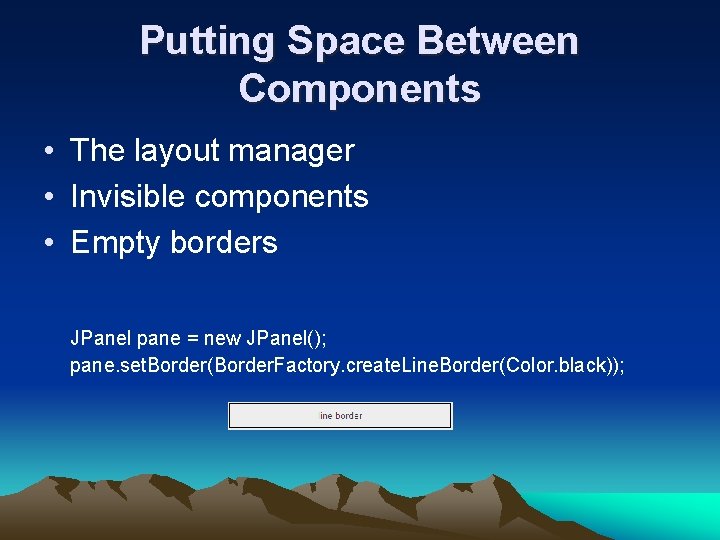
Putting Space Between Components • The layout manager • Invisible components • Empty borders JPanel pane = new JPanel(); pane. set. Border(Border. Factory. create. Line. Border(Color. black));
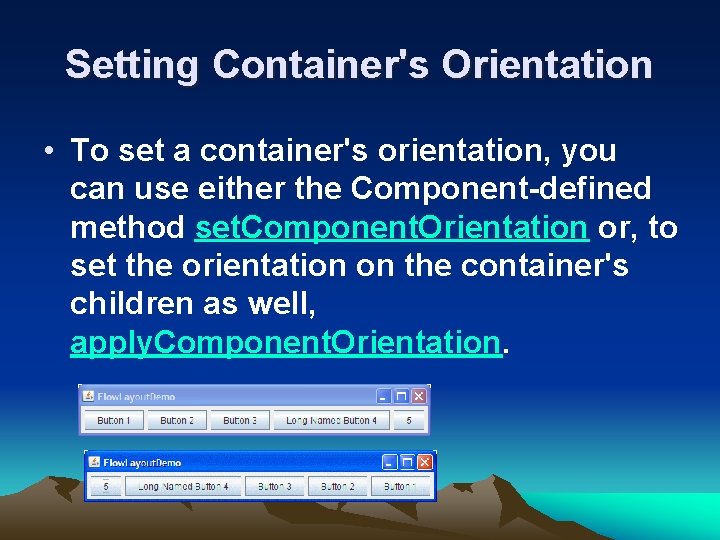
Setting Container's Orientation • To set a container's orientation, you can use either the Component-defined method set. Component. Orientation or, to set the orientation on the container's children as well, apply. Component. Orientation.
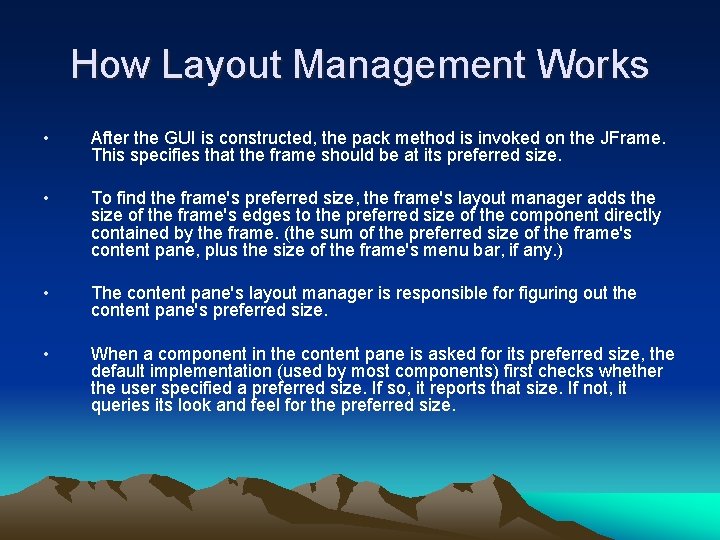
How Layout Management Works • After the GUI is constructed, the pack method is invoked on the JFrame. This specifies that the frame should be at its preferred size. • To find the frame's preferred size, the frame's layout manager adds the size of the frame's edges to the preferred size of the component directly contained by the frame. (the sum of the preferred size of the frame's content pane, plus the size of the frame's menu bar, if any. ) • The content pane's layout manager is responsible for figuring out the content pane's preferred size. • When a component in the content pane is asked for its preferred size, the default implementation (used by most components) first checks whether the user specified a preferred size. If so, it reports that size. If not, it queries its look and feel for the preferred size.
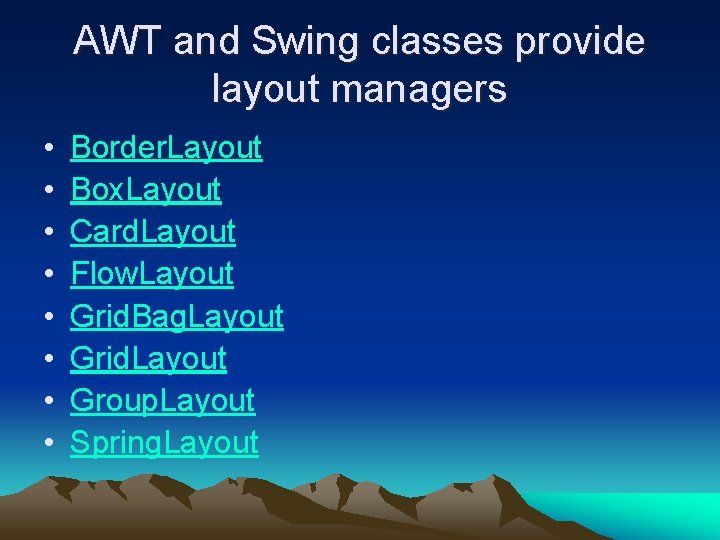
AWT and Swing classes provide layout managers • • Border. Layout Box. Layout Card. Layout Flow. Layout Grid. Bag. Layout Grid. Layout Group. Layout Spring. Layout
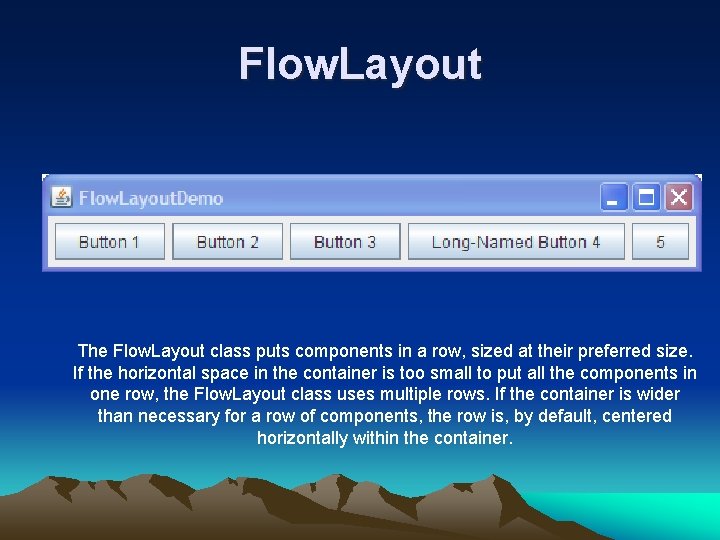
Flow. Layout The Flow. Layout class puts components in a row, sized at their preferred size. If the horizontal space in the container is too small to put all the components in one row, the Flow. Layout class uses multiple rows. If the container is wider than necessary for a row of components, the row is, by default, centered horizontally within the container.
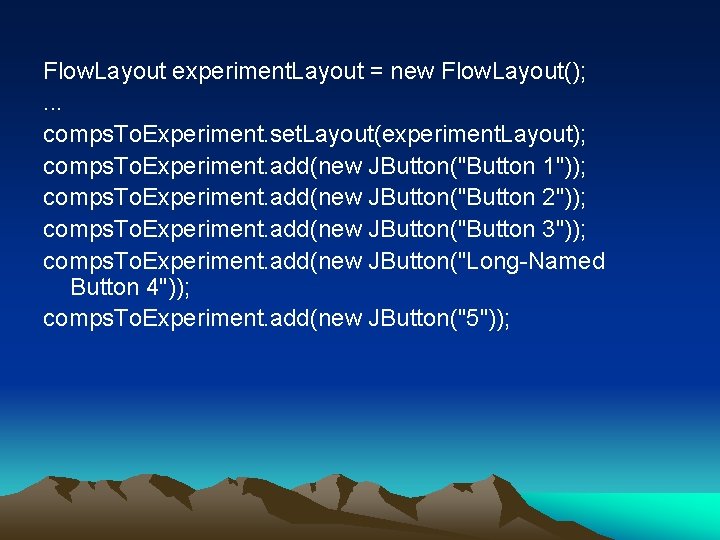
Flow. Layout experiment. Layout = new Flow. Layout(); . . . comps. To. Experiment. set. Layout(experiment. Layout); comps. To. Experiment. add(new JButton("Button 1")); comps. To. Experiment. add(new JButton("Button 2")); comps. To. Experiment. add(new JButton("Button 3")); comps. To. Experiment. add(new JButton("Long-Named Button 4")); comps. To. Experiment. add(new JButton("5"));
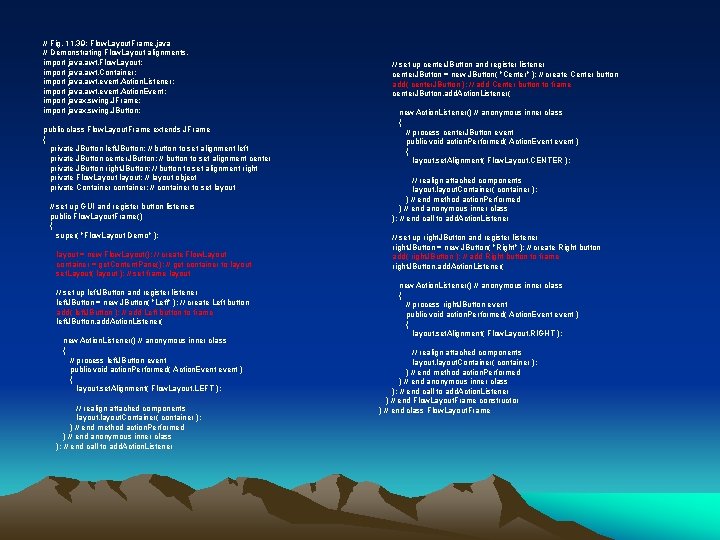
// Fig. 11. 39: Flow. Layout. Frame. java // Demonstrating Flow. Layout alignments. import java. awt. Flow. Layout; import java. awt. Container; import java. awt. event. Action. Listener; import java. awt. event. Action. Event; import javax. swing. JFrame; import javax. swing. JButton; public class Flow. Layout. Frame extends JFrame { private JButton left. JButton; // button to set alignment left private JButton center. JButton; // button to set alignment center private JButton right. JButton; // button to set alignment right private Flow. Layout layout; // layout object private Container container; // container to set layout // set up GUI and register button listeners public Flow. Layout. Frame() { super( "Flow. Layout Demo" ); layout = new Flow. Layout(); // create Flow. Layout container = get. Content. Pane(); // get container to layout set. Layout( layout ); // set frame layout // set up left. JButton and register listener left. JButton = new JButton( "Left" ); // create Left button add( left. JButton ); // add Left button to frame left. JButton. add. Action. Listener( new Action. Listener() // anonymous inner class { // process left. JButton event public void action. Performed( Action. Event event ) { layout. set. Alignment( Flow. Layout. LEFT ); // realign attached components layout. Container( container ); } // end method action. Performed } // end anonymous inner class ); // end call to add. Action. Listener // set up center. JButton and register listener center. JButton = new JButton( "Center" ); // create Center button add( center. JButton ); // add Center button to frame center. JButton. add. Action. Listener( new Action. Listener() // anonymous inner class { // process center. JButton event public void action. Performed( Action. Event event ) { layout. set. Alignment( Flow. Layout. CENTER ); // realign attached components layout. Container( container ); } // end method action. Performed } // end anonymous inner class ); // end call to add. Action. Listener // set up right. JButton and register listener right. JButton = new JButton( "Right" ); // create Right button add( right. JButton ); // add Right button to frame right. JButton. add. Action. Listener( new Action. Listener() // anonymous inner class { // process right. JButton event public void action. Performed( Action. Event event ) { layout. set. Alignment( Flow. Layout. RIGHT ); // realign attached components layout. Container( container ); } // end method action. Performed } // end anonymous inner class ); // end call to add. Action. Listener } // end Flow. Layout. Frame constructor } // end class Flow. Layout. Frame
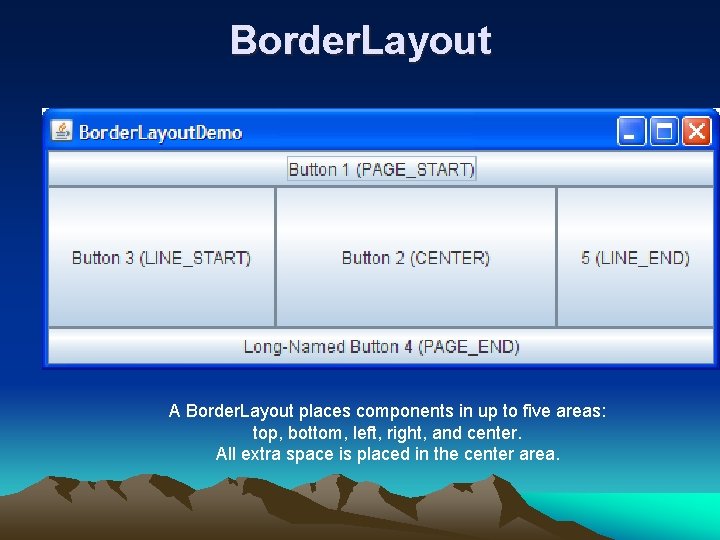
Border. Layout A Border. Layout places components in up to five areas: top, bottom, left, right, and center. All extra space is placed in the center area.
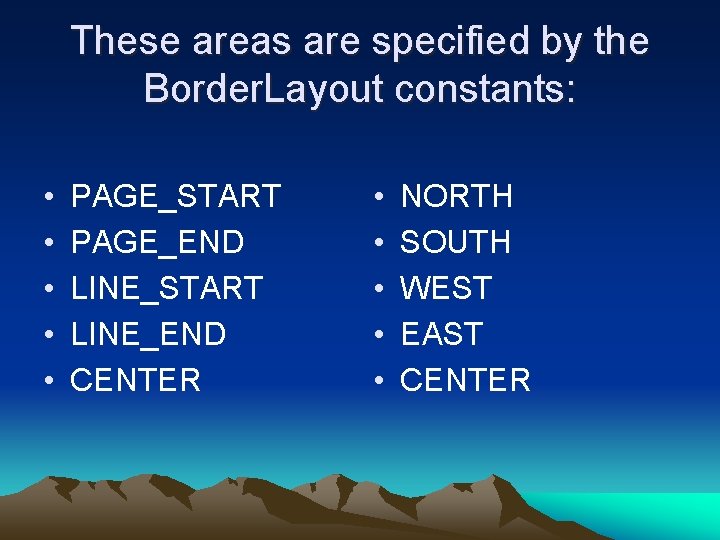
These areas are specified by the Border. Layout constants: • • • PAGE_START PAGE_END LINE_START LINE_END CENTER • • • NORTH SOUTH WEST EAST CENTER
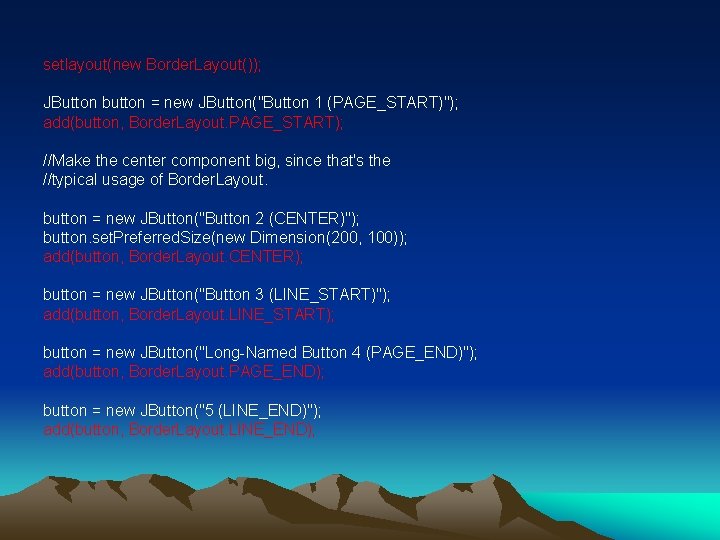
setlayout(new Border. Layout()); JButton button = new JButton("Button 1 (PAGE_START)"); add(button, Border. Layout. PAGE_START); //Make the center component big, since that's the //typical usage of Border. Layout. button = new JButton("Button 2 (CENTER)"); button. set. Preferred. Size(new Dimension(200, 100)); add(button, Border. Layout. CENTER); button = new JButton("Button 3 (LINE_START)"); add(button, Border. Layout. LINE_START); button = new JButton("Long-Named Button 4 (PAGE_END)"); add(button, Border. Layout. PAGE_END); button = new JButton("5 (LINE_END)"); add(button, Border. Layout. LINE_END);
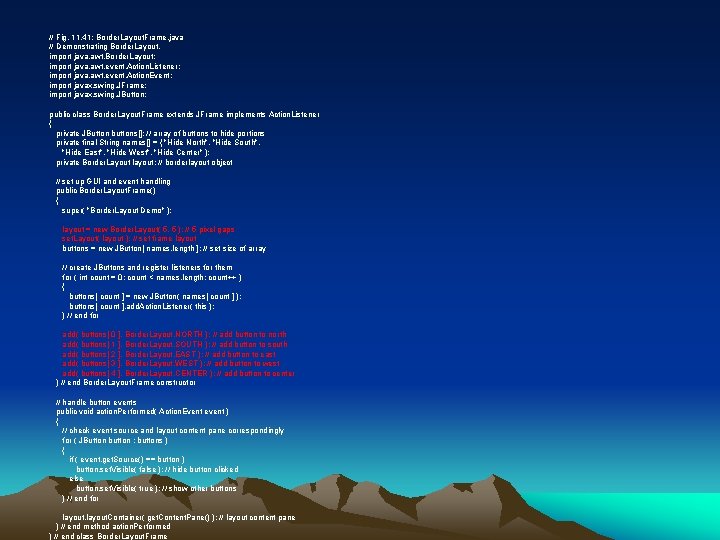
// Fig. 11. 41: Border. Layout. Frame. java // Demonstrating Border. Layout. import java. awt. Border. Layout; import java. awt. event. Action. Listener; import java. awt. event. Action. Event; import javax. swing. JFrame; import javax. swing. JButton; public class Border. Layout. Frame extends JFrame implements Action. Listener { private JButton buttons[]; // array of buttons to hide portions private final String names[] = { "Hide North", "Hide South", "Hide East", "Hide West", "Hide Center" }; private Border. Layout layout; // borderlayout object // set up GUI and event handling public Border. Layout. Frame() { super( "Border. Layout Demo" ); layout = new Border. Layout( 5, 5 ); // 5 pixel gaps set. Layout( layout ); // set frame layout buttons = new JButton[ names. length ]; // set size of array // create JButtons and register listeners for them for ( int count = 0; count < names. length; count++ ) { buttons[ count ] = new JButton( names[ count ] ); buttons[ count ]. add. Action. Listener( this ); } // end for add( buttons[ 0 ], Border. Layout. NORTH ); // add button to north add( buttons[ 1 ], Border. Layout. SOUTH ); // add button to south add( buttons[ 2 ], Border. Layout. EAST ); // add button to east add( buttons[ 3 ], Border. Layout. WEST ); // add button to west add( buttons[ 4 ], Border. Layout. CENTER ); // add button to center } // end Border. Layout. Frame constructor // handle button events public void action. Performed( Action. Event event ) { // check event source and layout content pane correspondingly for ( JButton button : buttons ) { if ( event. get. Source() == button ) button. set. Visible( false ); // hide button clicked else button. set. Visible( true ); // show other buttons } // end for layout. Container( get. Content. Pane() ); // layout content pane } // end method action. Performed } // end class Border. Layout. Frame
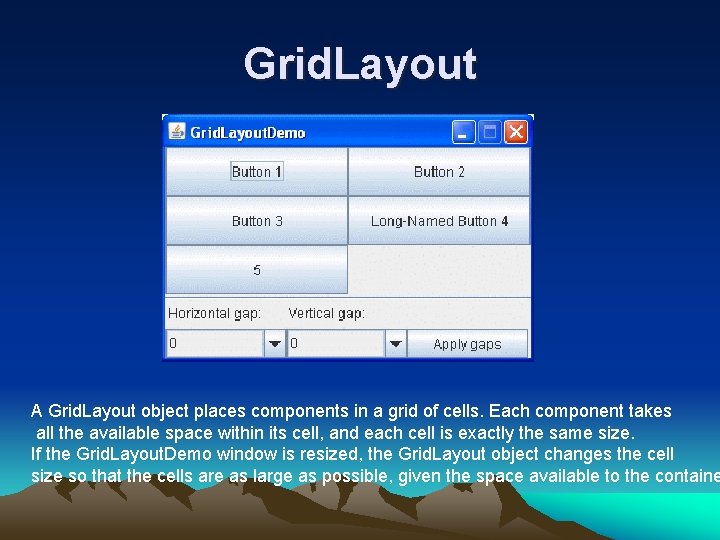
Grid. Layout A Grid. Layout object places components in a grid of cells. Each component takes all the available space within its cell, and each cell is exactly the same size. If the Grid. Layout. Demo window is resized, the Grid. Layout object changes the cell size so that the cells are as large as possible, given the space available to the containe
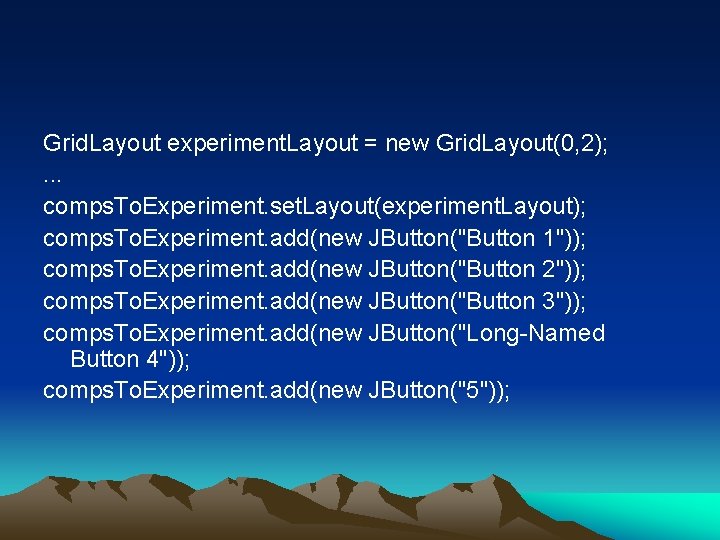
Grid. Layout experiment. Layout = new Grid. Layout(0, 2); . . . comps. To. Experiment. set. Layout(experiment. Layout); comps. To. Experiment. add(new JButton("Button 1")); comps. To. Experiment. add(new JButton("Button 2")); comps. To. Experiment. add(new JButton("Button 3")); comps. To. Experiment. add(new JButton("Long-Named Button 4")); comps. To. Experiment. add(new JButton("5"));
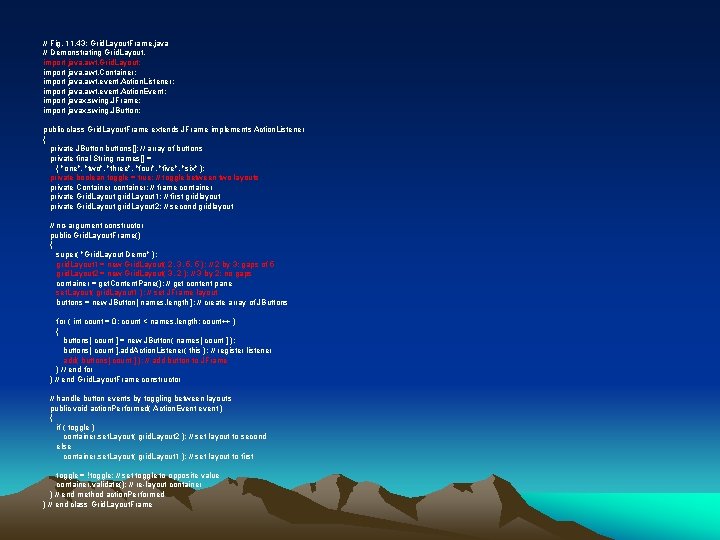
// Fig. 11. 43: Grid. Layout. Frame. java // Demonstrating Grid. Layout. import java. awt. Grid. Layout; import java. awt. Container; import java. awt. event. Action. Listener; import java. awt. event. Action. Event; import javax. swing. JFrame; import javax. swing. JButton; public class Grid. Layout. Frame extends JFrame implements Action. Listener { private JButton buttons[]; // array of buttons private final String names[] = { "one", "two", "three", "four", "five", "six" }; private boolean toggle = true; // toggle between two layouts private Container container; // frame container private Grid. Layout grid. Layout 1; // first gridlayout private Grid. Layout grid. Layout 2; // second gridlayout // no-argument constructor public Grid. Layout. Frame() { super( "Grid. Layout Demo" ); grid. Layout 1 = new Grid. Layout( 2, 3, 5, 5 ); // 2 by 3; gaps of 5 grid. Layout 2 = new Grid. Layout( 3, 2 ); // 3 by 2; no gaps container = get. Content. Pane(); // get content pane set. Layout( grid. Layout 1 ); // set JFrame layout buttons = new JButton[ names. length ]; // create array of JButtons for ( int count = 0; count < names. length; count++ ) { buttons[ count ] = new JButton( names[ count ] ); buttons[ count ]. add. Action. Listener( this ); // register listener add( buttons[ count ] ); // add button to JFrame } // end for } // end Grid. Layout. Frame constructor // handle button events by toggling between layouts public void action. Performed( Action. Event event ) { if ( toggle ) container. set. Layout( grid. Layout 2 ); // set layout to second else container. set. Layout( grid. Layout 1 ); // set layout to first toggle = !toggle; // set toggle to opposite value container. validate(); // re-layout container } // end method action. Performed } // end class Grid. Layout. Frame
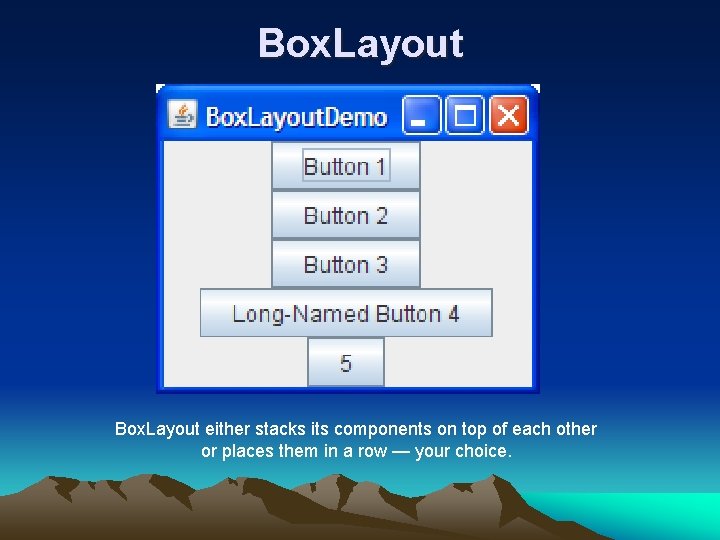
Box. Layout either stacks its components on top of each other or places them in a row — your choice.
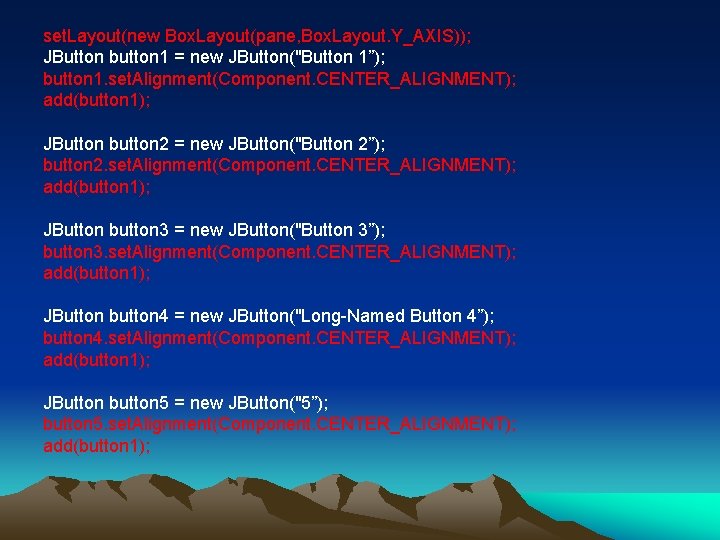
set. Layout(new Box. Layout(pane, Box. Layout. Y_AXIS)); JButton button 1 = new JButton("Button 1”); button 1. set. Alignment(Component. CENTER_ALIGNMENT); add(button 1); JButton button 2 = new JButton("Button 2”); button 2. set. Alignment(Component. CENTER_ALIGNMENT); add(button 1); JButton button 3 = new JButton("Button 3”); button 3. set. Alignment(Component. CENTER_ALIGNMENT); add(button 1); JButton button 4 = new JButton("Long-Named Button 4”); button 4. set. Alignment(Component. CENTER_ALIGNMENT); add(button 1); JButton button 5 = new JButton("5”); button 5. set. Alignment(Component. CENTER_ALIGNMENT); add(button 1);
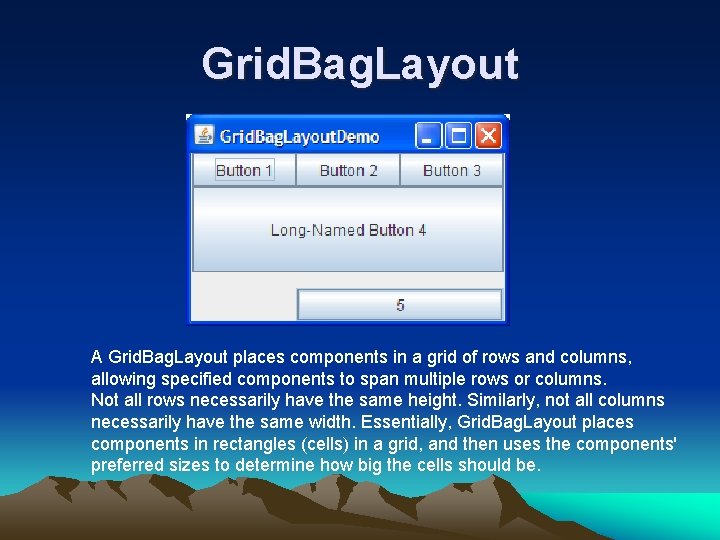
Grid. Bag. Layout A Grid. Bag. Layout places components in a grid of rows and columns, allowing specified components to span multiple rows or columns. Not all rows necessarily have the same height. Similarly, not all columns necessarily have the same width. Essentially, Grid. Bag. Layout places components in rectangles (cells) in a grid, and then uses the components' preferred sizes to determine how big the cells should be.
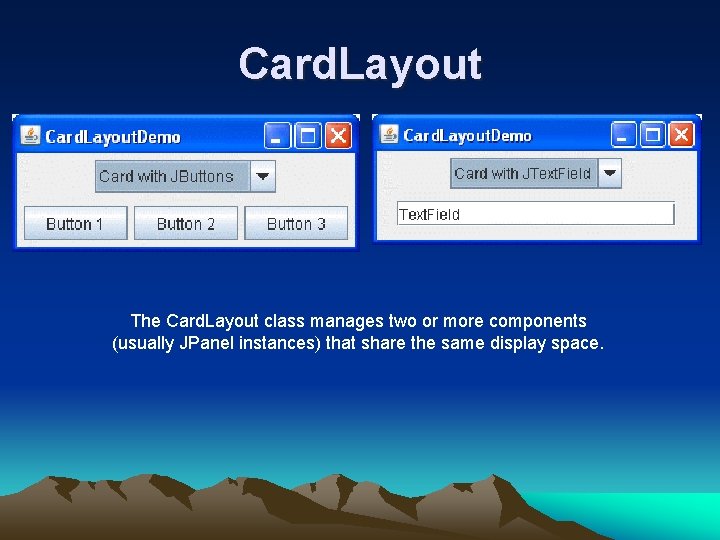
Card. Layout The Card. Layout class manages two or more components (usually JPanel instances) that share the same display space.
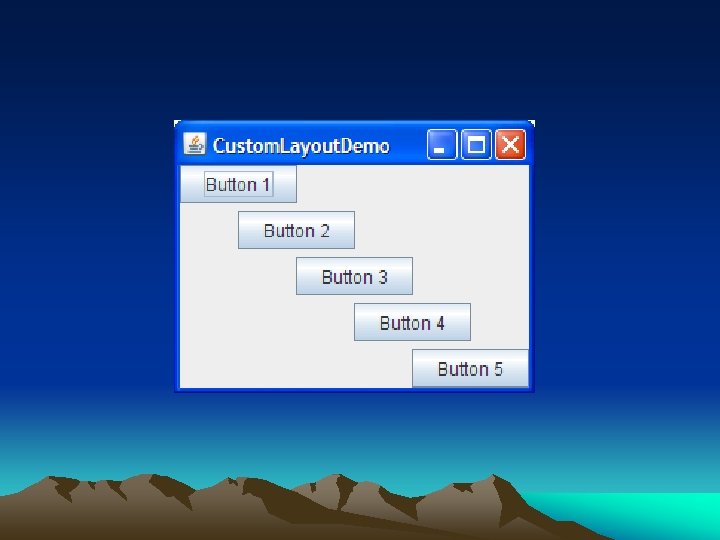
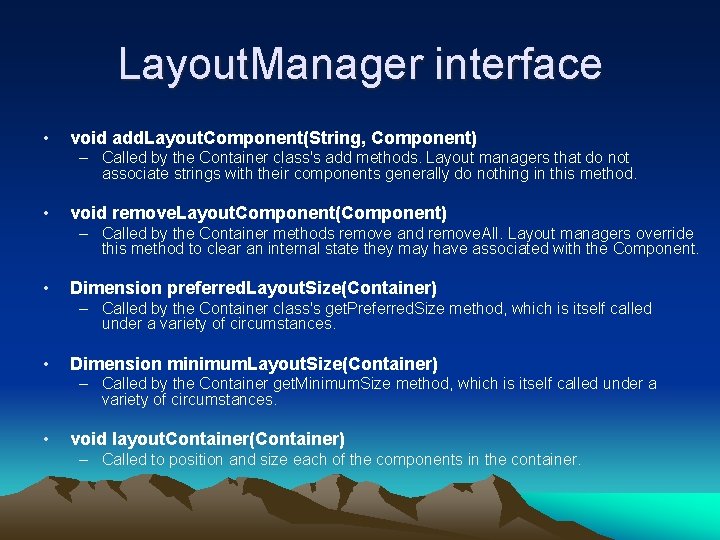
Layout. Manager interface • void add. Layout. Component(String, Component) – Called by the Container class's add methods. Layout managers that do not associate strings with their components generally do nothing in this method. • void remove. Layout. Component(Component) – Called by the Container methods remove and remove. All. Layout managers override this method to clear an internal state they may have associated with the Component. • Dimension preferred. Layout. Size(Container) – Called by the Container class's get. Preferred. Size method, which is itself called under a variety of circumstances. • Dimension minimum. Layout. Size(Container) – Called by the Container get. Minimum. Size method, which is itself called under a variety of circumstances. • void layout. Container(Container) – Called to position and size each of the components in the container.