Haskell References Learn You a Haskell for Great
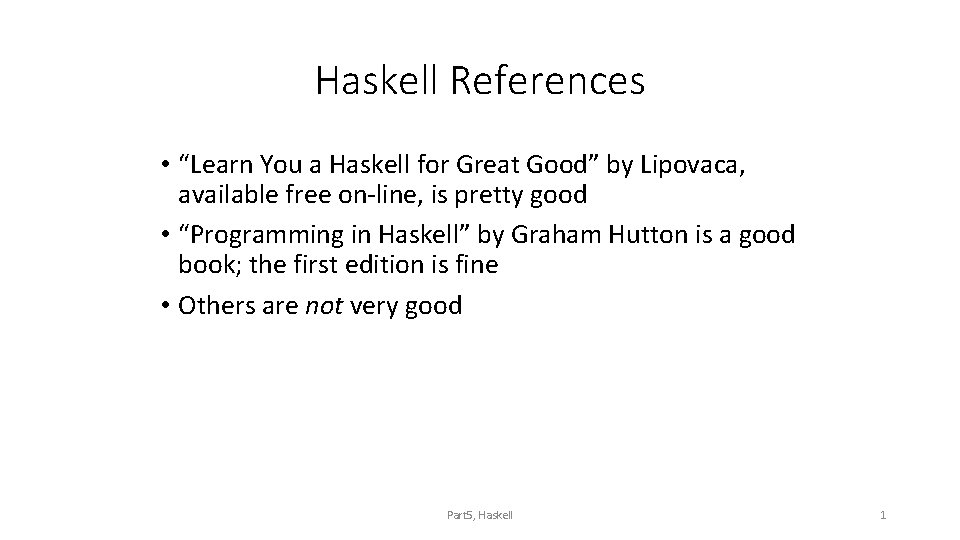
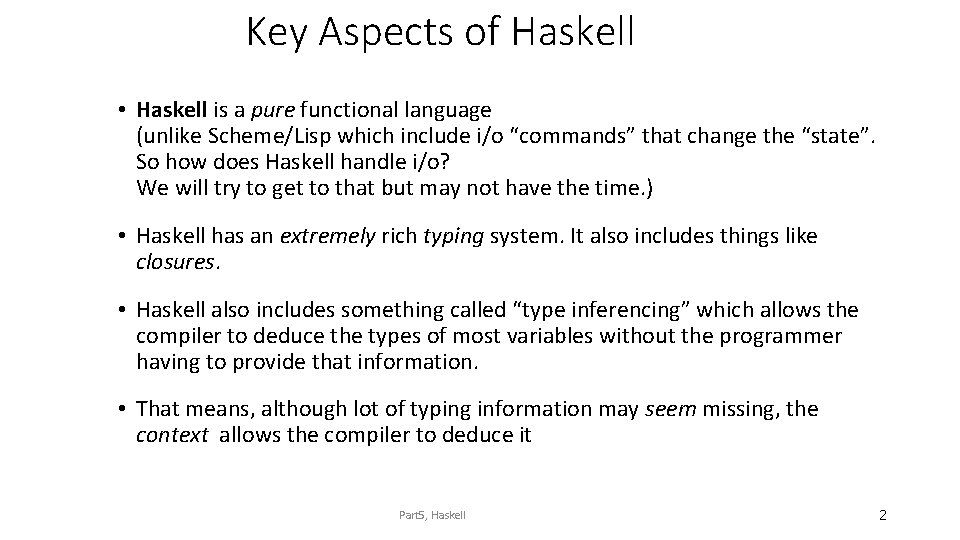
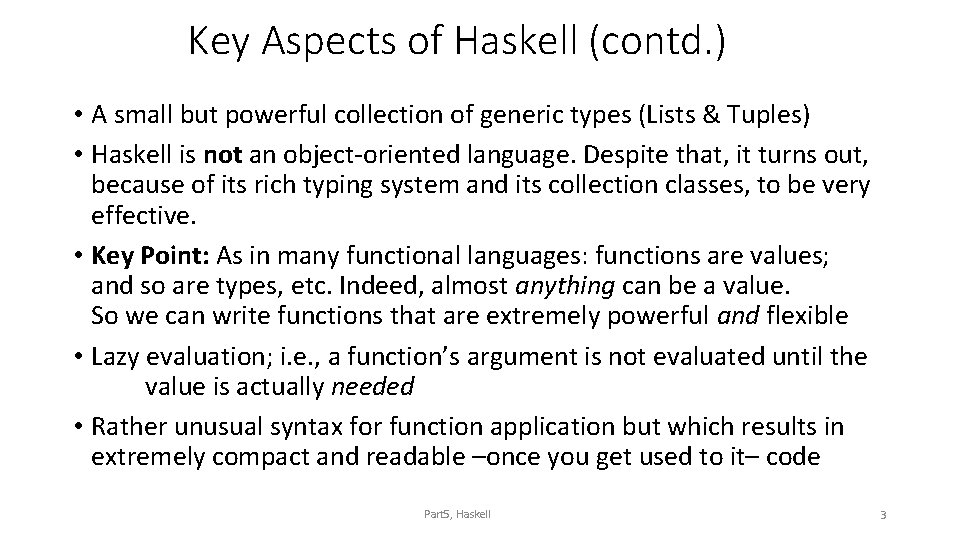
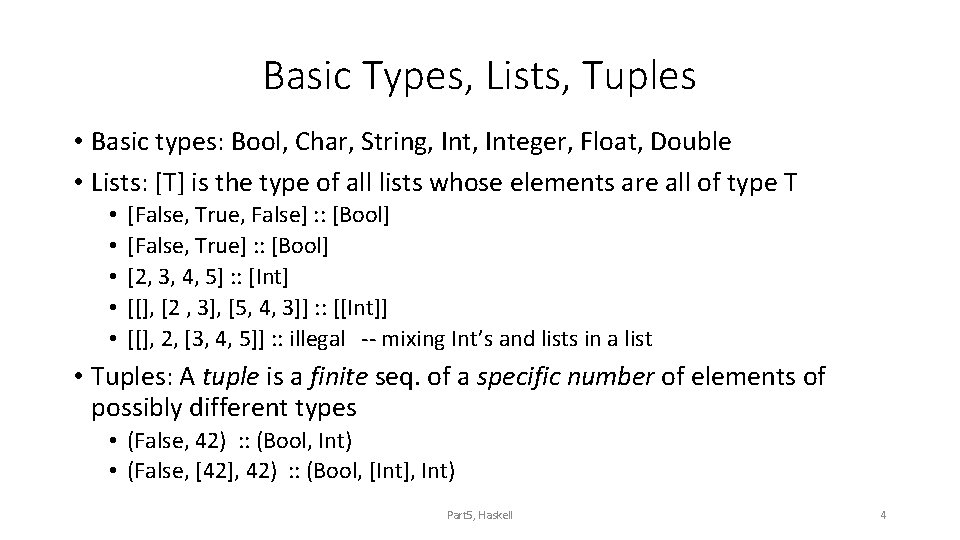
![Notation • “[ … ]” indicates list • “( … )” indicates tuple • Notation • “[ … ]” indicates list • “( … )” indicates tuple •](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-5.jpg)
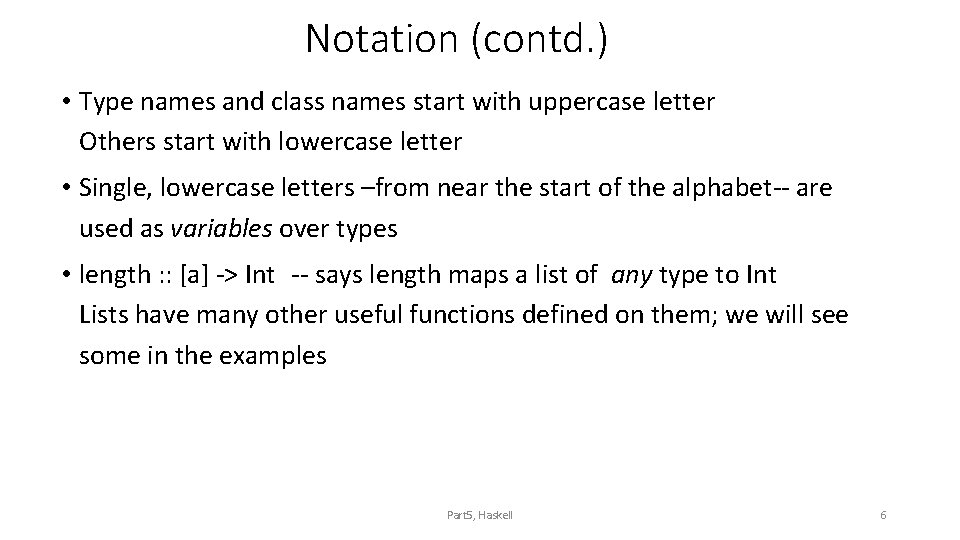
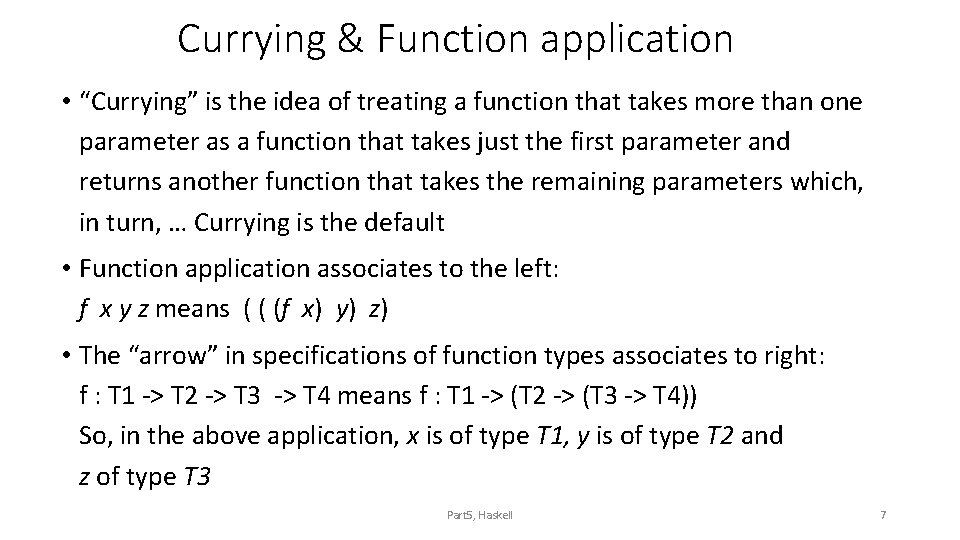
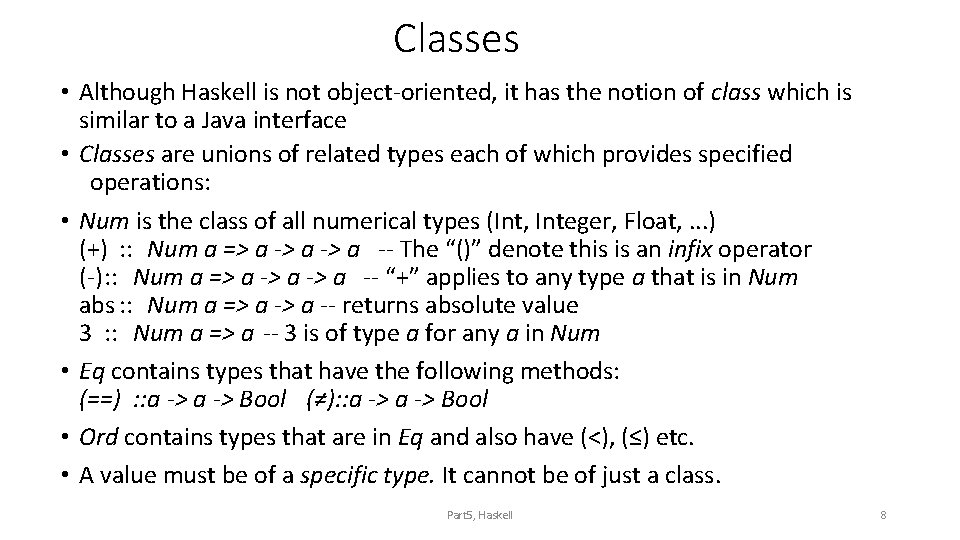
![A Simple Example • sum : : (Num a) => [a] -> a -- A Simple Example • sum : : (Num a) => [a] -> a --](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-9.jpg)
![Haskell library • length : : [a] -> Int -- says length maps a Haskell library • length : : [a] -> Int -- says length maps a](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-10.jpg)
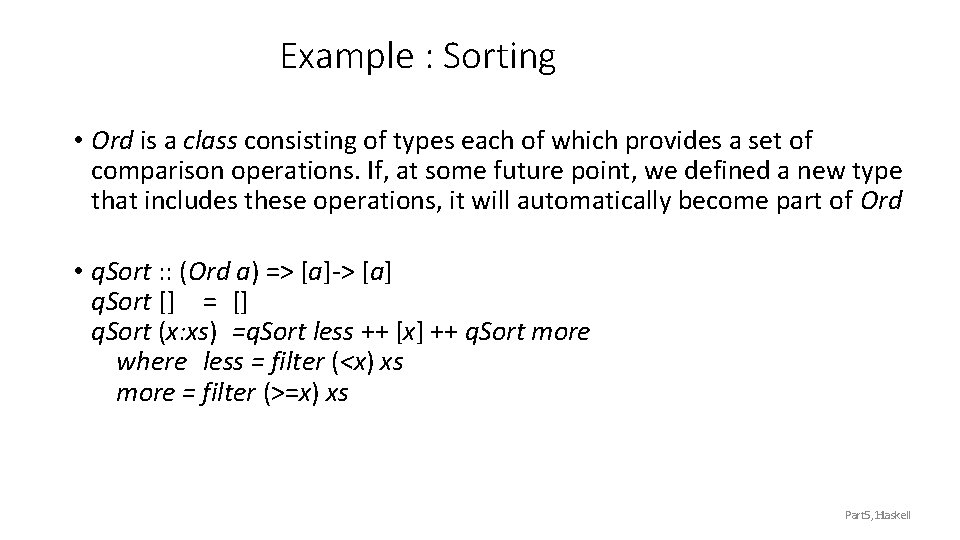
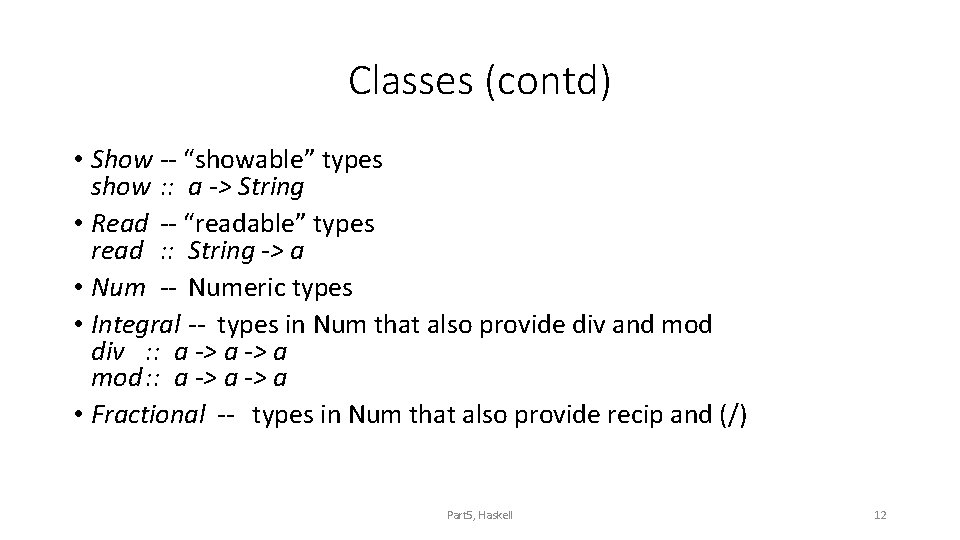
![Defining New Types New names for existing types: type String = [ Char ] Defining New Types New names for existing types: type String = [ Char ]](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-13.jpg)
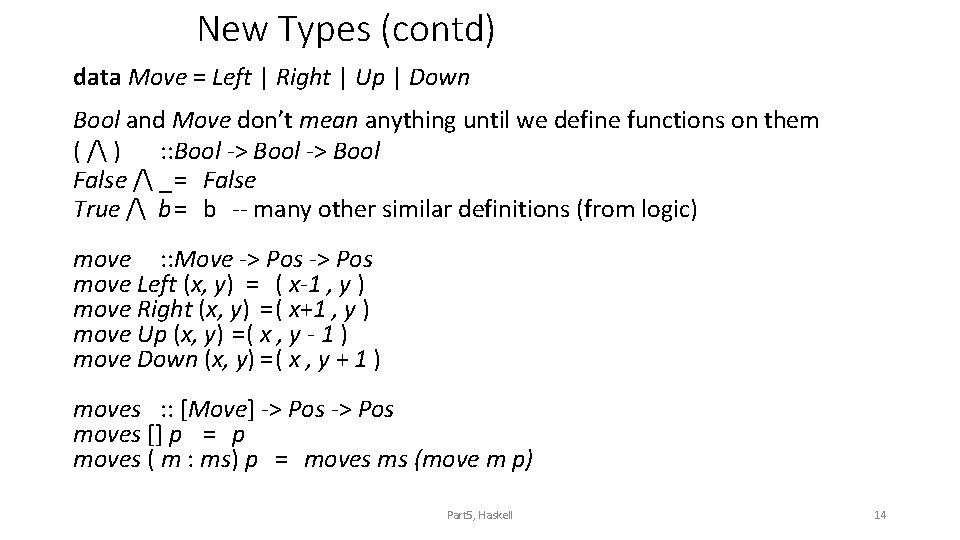
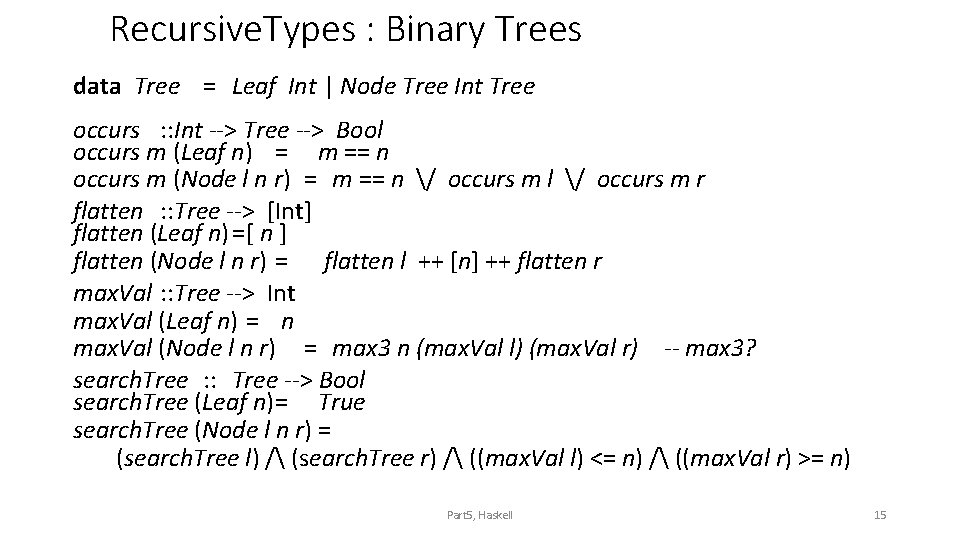
![Recursive. Types : Lists data [ a ]= [ ] | a : [ Recursive. Types : Lists data [ a ]= [ ] | a : [](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-16.jpg)
![Sieve of Eratosthenes • primes : : [Int] primes = sieve [2. . ] Sieve of Eratosthenes • primes : : [Int] primes = sieve [2. . ]](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-17.jpg)
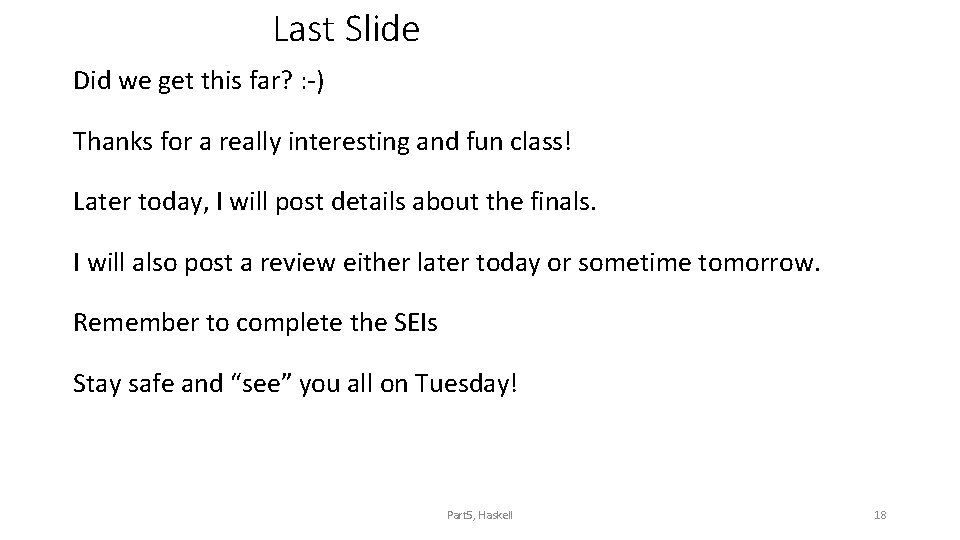
- Slides: 18
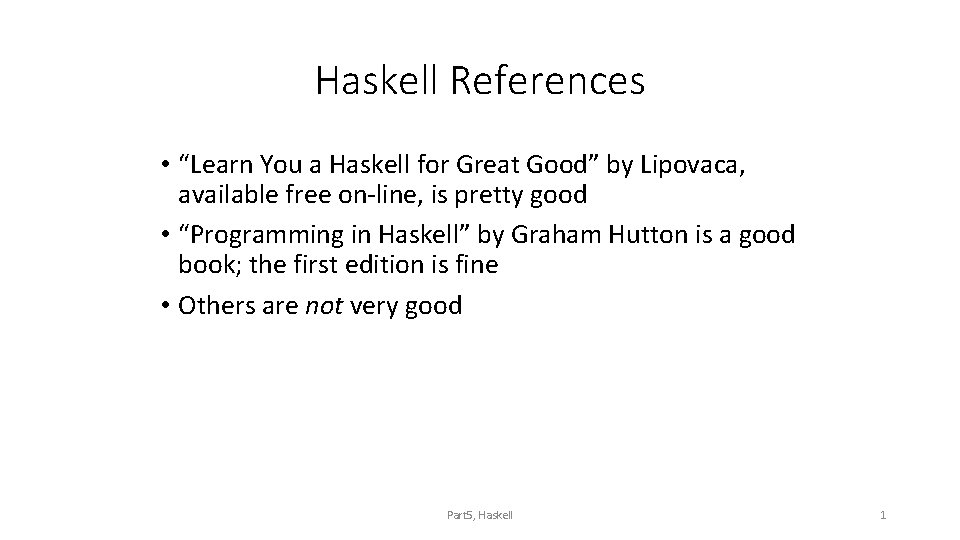
Haskell References • “Learn You a Haskell for Great Good” by Lipovaca, available free on-line, is pretty good • “Programming in Haskell” by Graham Hutton is a good book; the first edition is fine • Others are not very good Part 5, Haskell 1
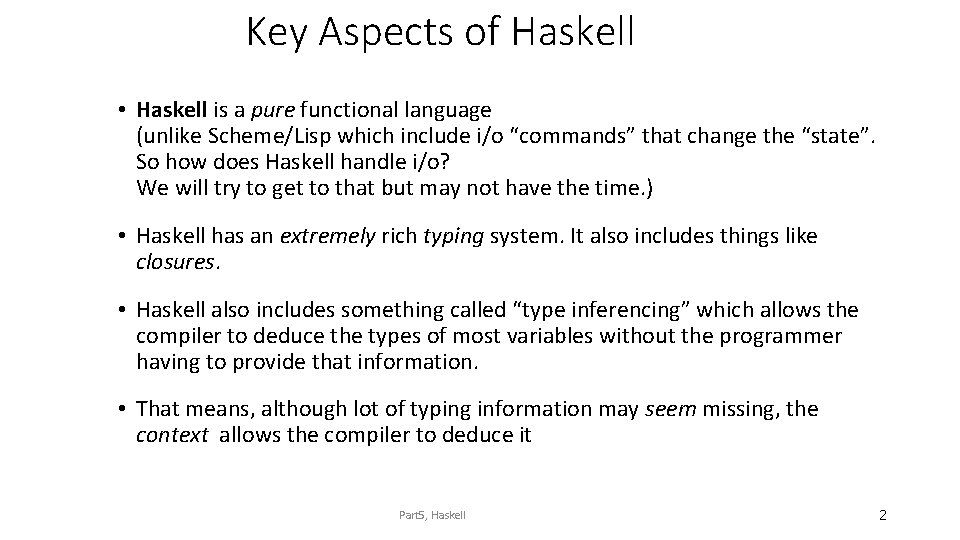
Key Aspects of Haskell • Haskell is a pure functional language (unlike Scheme/Lisp which include i/o “commands” that change the “state”. So how does Haskell handle i/o? We will try to get to that but may not have the time. ) • Haskell has an extremely rich typing system. It also includes things like closures. • Haskell also includes something called “type inferencing” which allows the compiler to deduce the types of most variables without the programmer having to provide that information. • That means, although lot of typing information may seem missing, the context allows the compiler to deduce it Part 5, Haskell 2
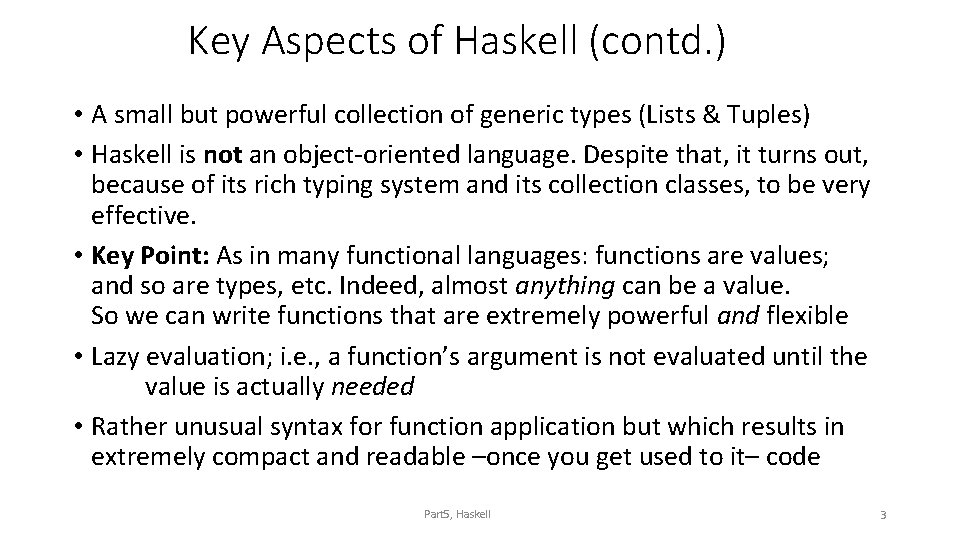
Key Aspects of Haskell (contd. ) • A small but powerful collection of generic types (Lists & Tuples) • Haskell is not an object-oriented language. Despite that, it turns out, because of its rich typing system and its collection classes, to be very effective. • Key Point: As in many functional languages: functions are values; and so are types, etc. Indeed, almost anything can be a value. So we can write functions that are extremely powerful and flexible • Lazy evaluation; i. e. , a function’s argument is not evaluated until the value is actually needed • Rather unusual syntax for function application but which results in extremely compact and readable –once you get used to it– code Part 5, Haskell 3
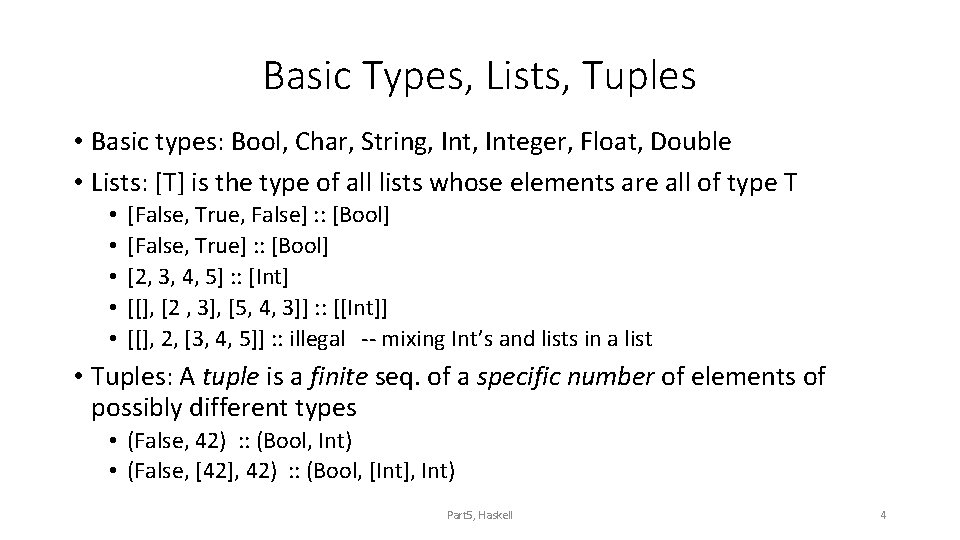
Basic Types, Lists, Tuples • Basic types: Bool, Char, String, Integer, Float, Double • Lists: [T] is the type of all lists whose elements are all of type T • • • [False, True, False] : : [Bool] [False, True] : : [Bool] [2, 3, 4, 5] : : [Int] [[], [2 , 3], [5, 4, 3]] : : [[Int]] [[], 2, [3, 4, 5]] : : illegal -- mixing Int’s and lists in a list • Tuples: A tuple is a finite seq. of a specific number of elements of possibly different types • (False, 42) : : (Bool, Int) • (False, [42], 42) : : (Bool, [Int], Int) Part 5, Haskell 4
![Notation indicates list indicates tuple Notation • “[ … ]” indicates list • “( … )” indicates tuple •](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-5.jpg)
Notation • “[ … ]” indicates list • “( … )” indicates tuple • “ xyz : : abc“ means “xyz is of type abc” • “ -> “ means “maps to” f : : [Int] -> (Bool, String) means “the function f maps a list of Int’s to a pair whose first component is a Bool and second is a String” • Function application is indicated by whitespace If f is a function with two Int parameters, f 5 6 -- evaluates to the result of applying f to 5 and 6 • Binary operators such as “+” can be written as follows: (+) 5 6 -- same 5 + 6 Part 5, Haskell 5
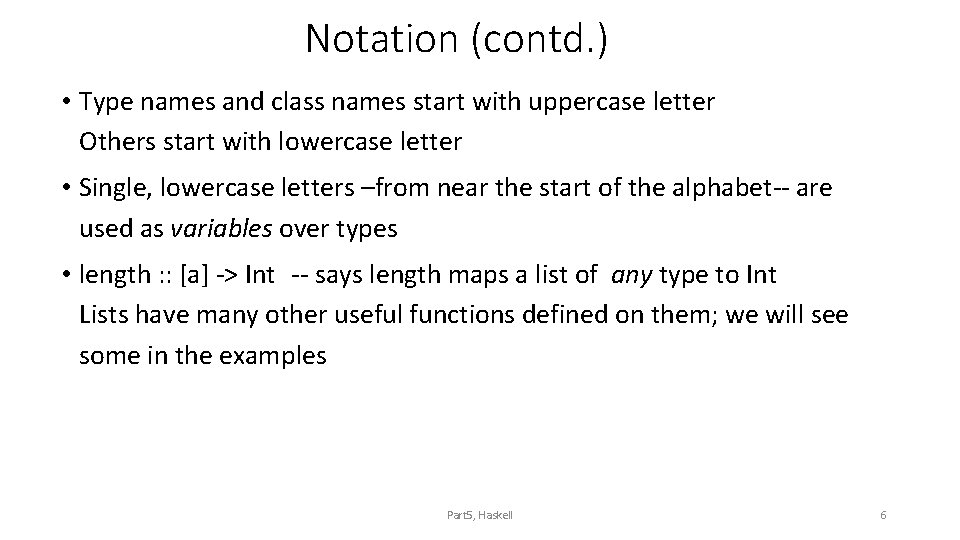
Notation (contd. ) • Type names and class names start with uppercase letter Others start with lowercase letter • Single, lowercase letters –from near the start of the alphabet-- are used as variables over types • length : : [a] -> Int -- says length maps a list of any type to Int Lists have many other useful functions defined on them; we will see some in the examples Part 5, Haskell 6
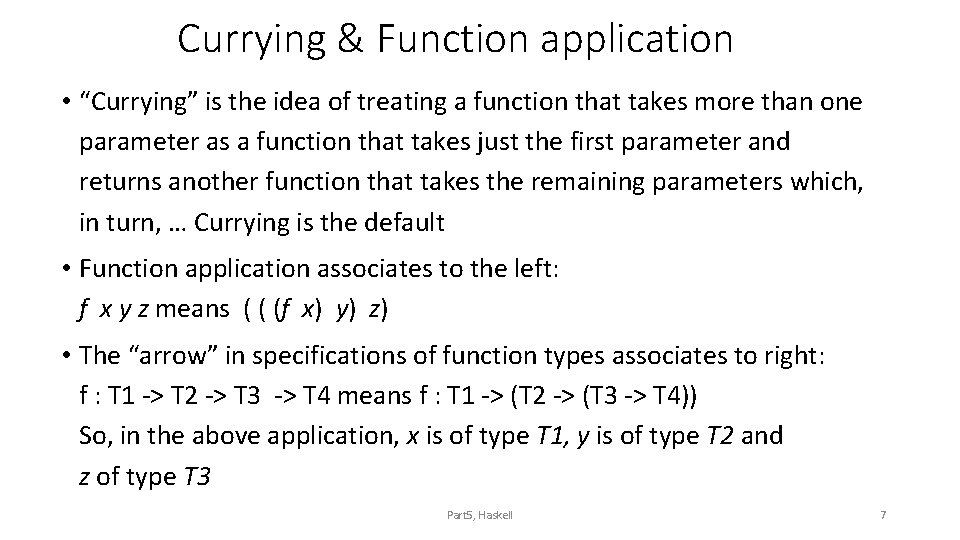
Currying & Function application • “Currying” is the idea of treating a function that takes more than one parameter as a function that takes just the first parameter and returns another function that takes the remaining parameters which, in turn, … Currying is the default • Function application associates to the left: f x y z means ( ( (f x) y) z) • The “arrow” in specifications of function types associates to right: f : T 1 -> T 2 -> T 3 -> T 4 means f : T 1 -> (T 2 -> (T 3 -> T 4)) So, in the above application, x is of type T 1, y is of type T 2 and z of type T 3 Part 5, Haskell 7
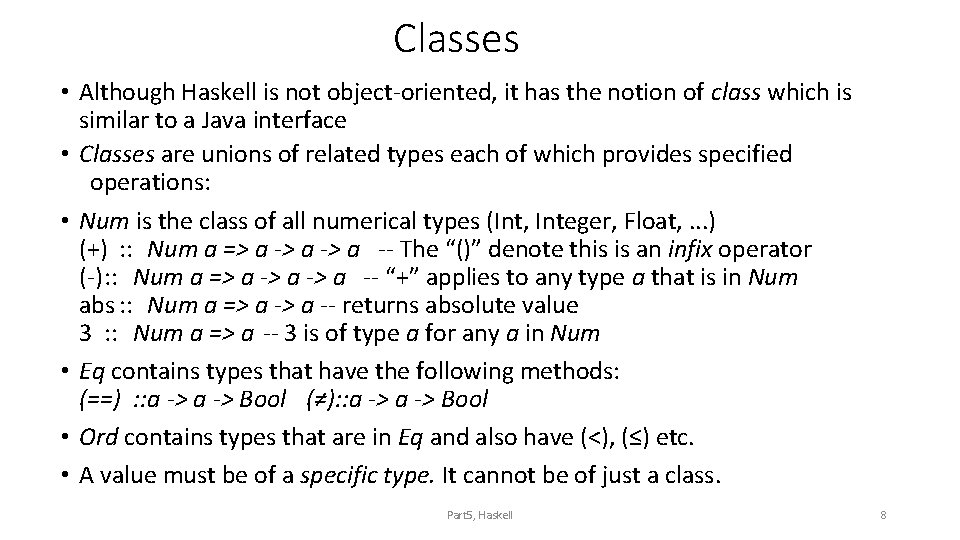
Classes • Although Haskell is not object-oriented, it has the notion of class which is similar to a Java interface • Classes are unions of related types each of which provides specified operations: • Num is the class of all numerical types (Int, Integer, Float, . . . ) (+) : : Num a => a -> a -- The “()” denote this is an infix operator (-): : Num a => a -> a -- “+” applies to any type a that is in Num abs : : Num a => a -- returns absolute value 3 : : Num a => a -- 3 is of type a for any a in Num • Eq contains types that have the following methods: (==) : : a -> Bool (≠): : a -> Bool • Ord contains types that are in Eq and also have (<), (≤) etc. • A value must be of a specific type. It cannot be of just a class. Part 5, Haskell 8
![A Simple Example sum Num a a a A Simple Example • sum : : (Num a) => [a] -> a --](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-9.jpg)
A Simple Example • sum : : (Num a) => [a] -> a -- sum takes a list of a’s and returns an a; -- a is a type in Num which includes all numerical types • sum [] = 0 -- sum of empty list is 0 sum (n: ns) = n + sum ns -- of non-empty list is first element plus sum of rest -- “: ” is also called the “cons” operator -- The parentheses are used to avoid precedence problems In other words, sum takes an arg. of type [Int] or [Double], etc. , and returns a value of type Int or Double, etc. This is a common way to define functions on lists – by cases, one for the null list and one for the non-null list. Part 5, Haskell 9
![Haskell library length a Int says length maps a Haskell library • length : : [a] -> Int -- says length maps a](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-10.jpg)
Haskell library • length : : [a] -> Int -- says length maps a list of any type to Int null, any, elem, head, tail, last, take. While, filter. . . Lists have many other useful functions defined on them • But the Haskell primitives are so powerful that we could easily define these functions from scratch as we will see • Filter: filter : : (a -> Bool) -> [a] --- what does this say? filter p xs = [ x | x <- xs, p x ] -- the result is a list of all those elements of xs that satisfy p Part 5, Haskell 10
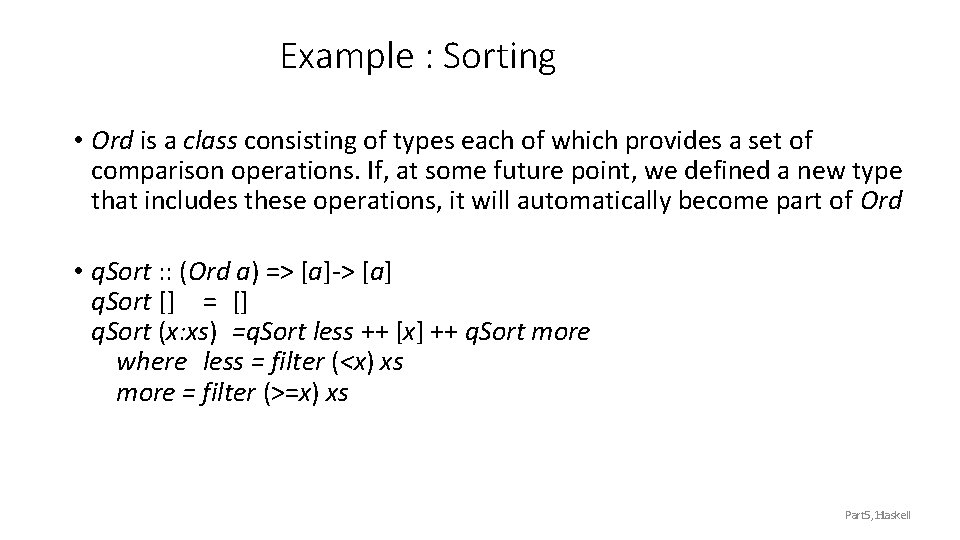
Example : Sorting • Ord is a class consisting of types each of which provides a set of comparison operations. If, at some future point, we defined a new type that includes these operations, it will automatically become part of Ord • q. Sort : : (Ord a) => [a]-> [a] q. Sort [] = [] q. Sort (x: xs) =q. Sort less ++ [x] ++ q. Sort more where less = filter (<x) xs more = filter (>=x) xs Part 5, 11 Haskell
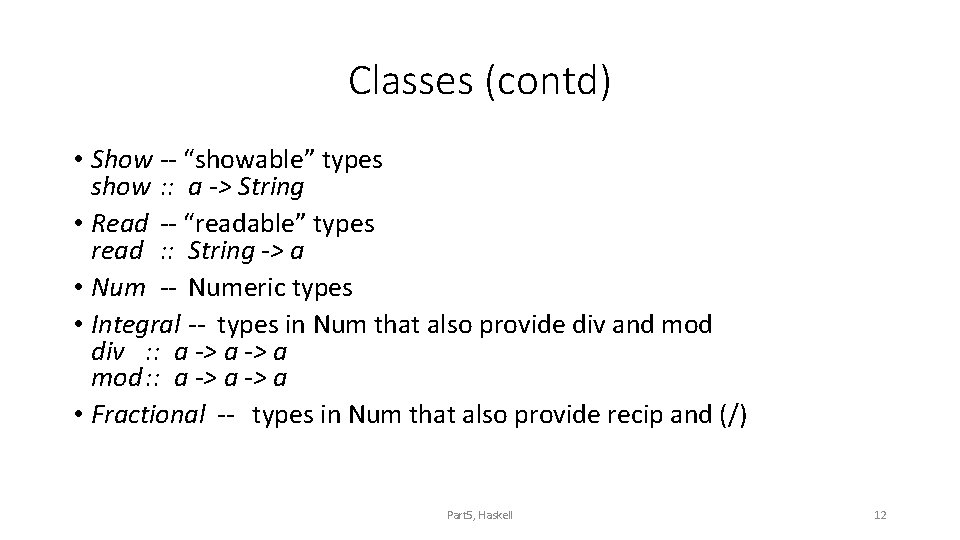
Classes (contd) • Show -- “showable” types show : : a -> String • Read -- “readable” types read : : String -> a • Num -- Numeric types • Integral -- types in Num that also provide div and mod div : : a -> a mod : : a -> a • Fractional -- types in Num that also provide recip and (/) Part 5, Haskell 12
![Defining New Types New names for existing types type String Char Defining New Types New names for existing types: type String = [ Char ]](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-13.jpg)
Defining New Types New names for existing types: type String = [ Char ] -- this is how String is defined type Pos = ( Int, Int ) New types: data Bool = False | True Part 5, Haskell 13
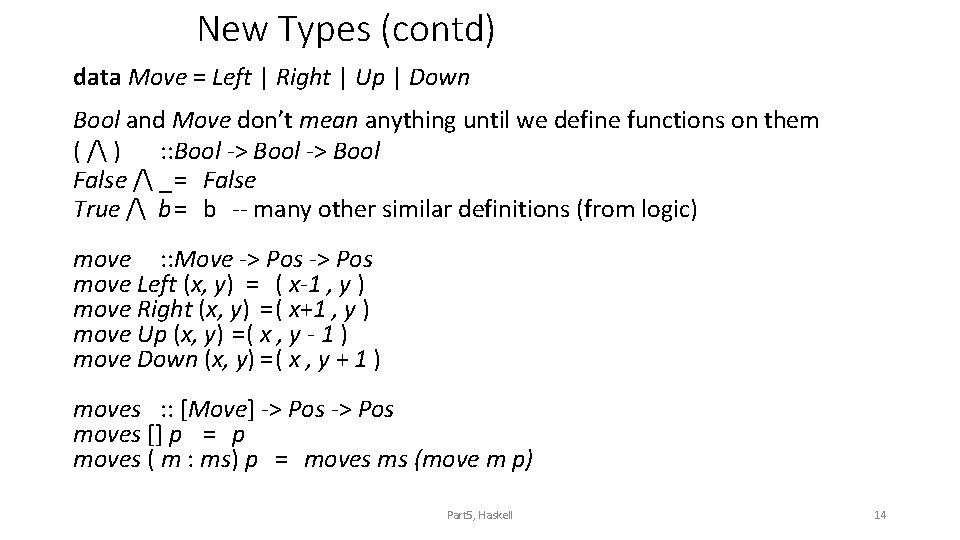
New Types (contd) data Move = Left | Right | Up | Down Bool and Move don’t mean anything until we define functions on them ( / ) : : Bool -> Bool False / _= False True / b= b -- many other similar definitions (from logic) move : : Move -> Pos move Left (x, y) = ( x-1 , y ) move Right (x, y) =( x+1 , y ) move Up (x, y) =( x , y - 1 ) move Down (x, y) =( x , y + 1 ) moves : : [Move] -> Pos moves [] p = p moves ( m : ms) p = moves ms (move m p) Part 5, Haskell 14
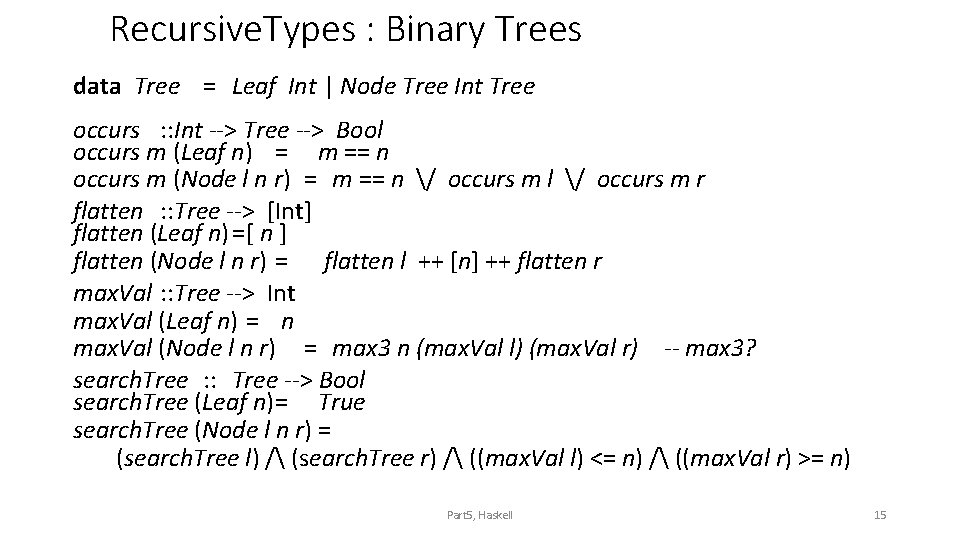
Recursive. Types : Binary Trees data Tree = Leaf Int | Node Tree Int Tree occurs : : Int --> Tree --> Bool occurs m (Leaf n) = m == n occurs m (Node l n r) = m == n / occurs m l / occurs m r flatten : : Tree --> [Int] flatten (Leaf n)=[ n ] flatten (Node l n r) = flatten l ++ [n] ++ flatten r max. Val : : Tree --> Int max. Val (Leaf n) = n max. Val (Node l n r) = max 3 n (max. Val l) (max. Val r) -- max 3? search. Tree : : Tree --> Bool search. Tree (Leaf n)= True search. Tree (Node l n r) = (search. Tree l) / (search. Tree r) / ((max. Val l) <= n) / ((max. Val r) >= n) Part 5, Haskell 15
![Recursive Types Lists data a a Recursive. Types : Lists data [ a ]= [ ] | a : [](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-16.jpg)
Recursive. Types : Lists data [ a ]= [ ] | a : [ a ] null : : [ a ] --> Bool null [ ] = True null (__: __)= False zip : : [ a ] --> [ b ] --> [ (a , b)] zip __ [ ] == [ ] zip [ ] __ == [ ] zip (x : xs) (y : ys ) == ( x, y) : zip xs ys take. While : : (a --> Bool) --> [a] take. While __ [ ] = [ ] take. While p (x: : xs) = | p x = x : take. While p xs I otherwise = [ ] And *many* other functions Part 5, Haskell 16
![Sieve of Eratosthenes primes Int primes sieve 2 Sieve of Eratosthenes • primes : : [Int] primes = sieve [2. . ]](https://slidetodoc.com/presentation_image_h2/49e11d1d0eab04b0685c5995c86e8051/image-17.jpg)
Sieve of Eratosthenes • primes : : [Int] primes = sieve [2. . ] -- [2. . ] is an infinite list!. . . but generated lazily sieve : : [Int] -> [Int] sieve (p: xs) =p : sieve [x | x <- xs, x `mod` p /= 0] • > primes [2, 3, 5, 7, 11, 13, 17, . . . - - keeps going till you stop it • take. While (< 10) primes -- take While : : (Int -> Bool) -> [a]--> [a] [2, 3, 5, 7] Part 5, Haskell 17
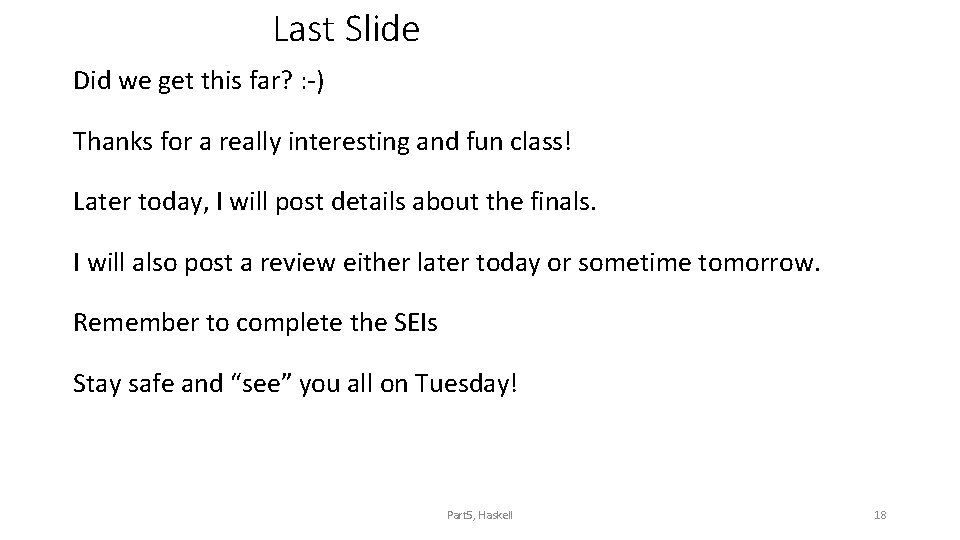
Last Slide Did we get this far? : -) Thanks for a really interesting and fun class! Later today, I will post details about the finals. I will also post a review either later today or sometime tomorrow. Remember to complete the SEIs Stay safe and “see” you all on Tuesday! Part 5, Haskell 18