GUIs Graphical User Interfaces SWING Containers Frame Panel
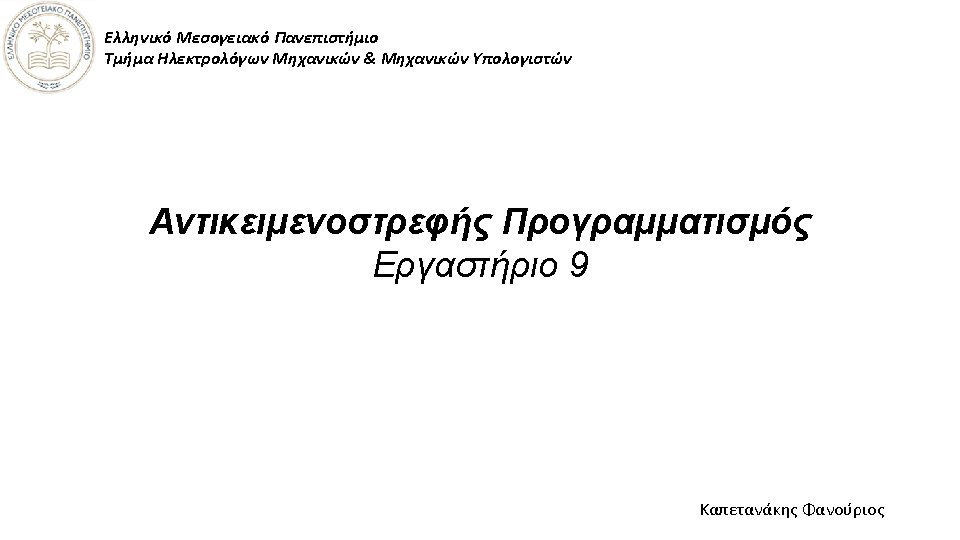
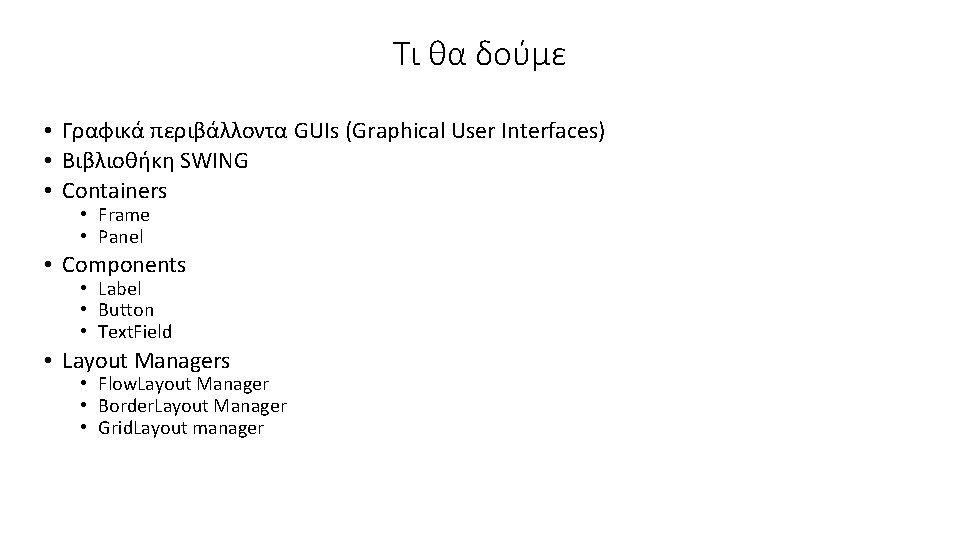
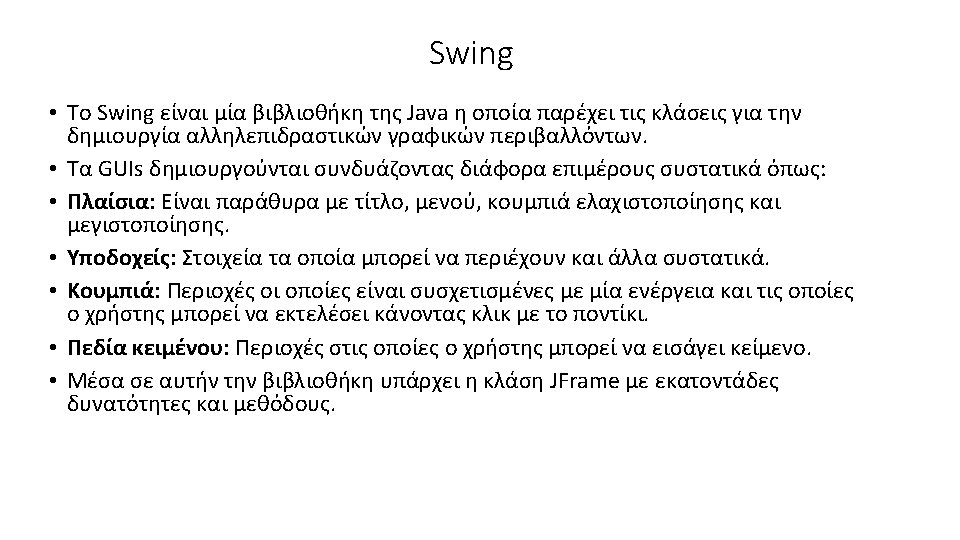
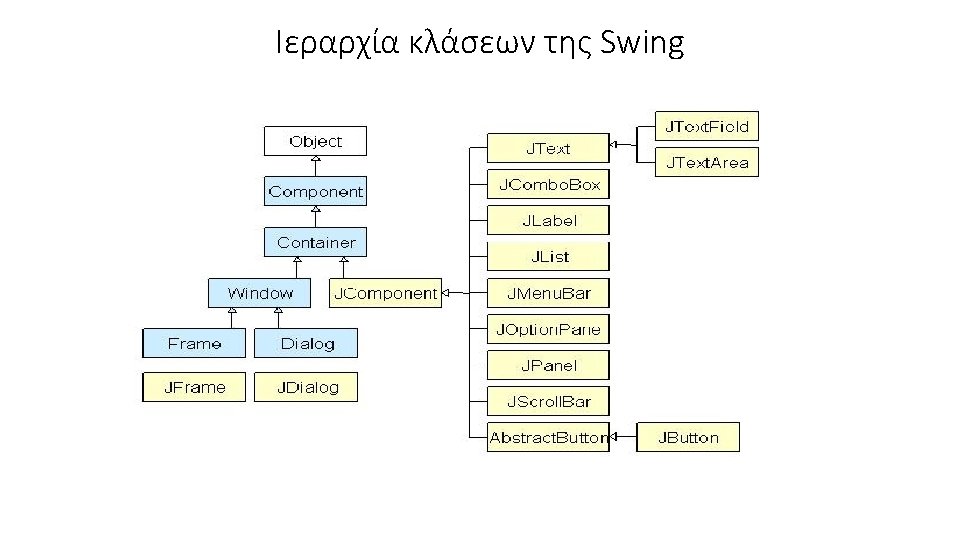
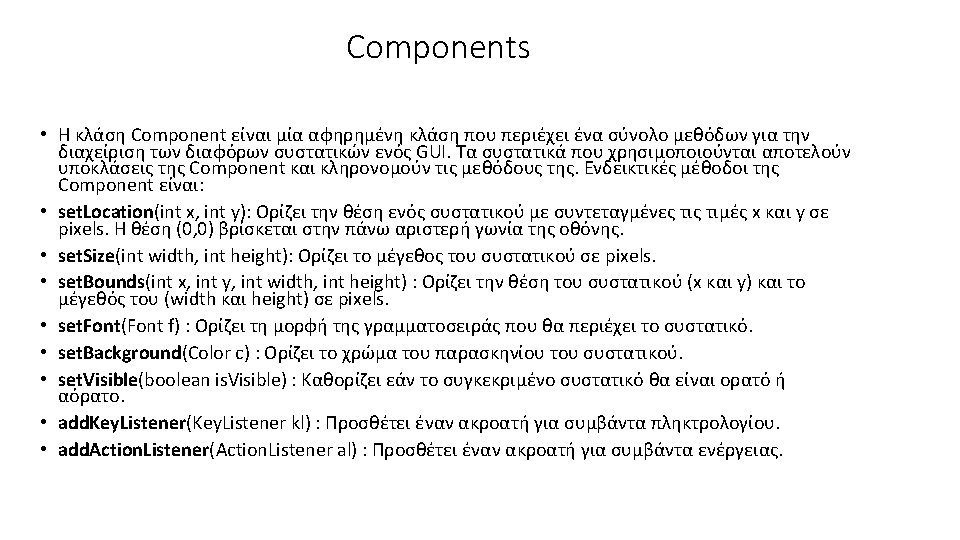
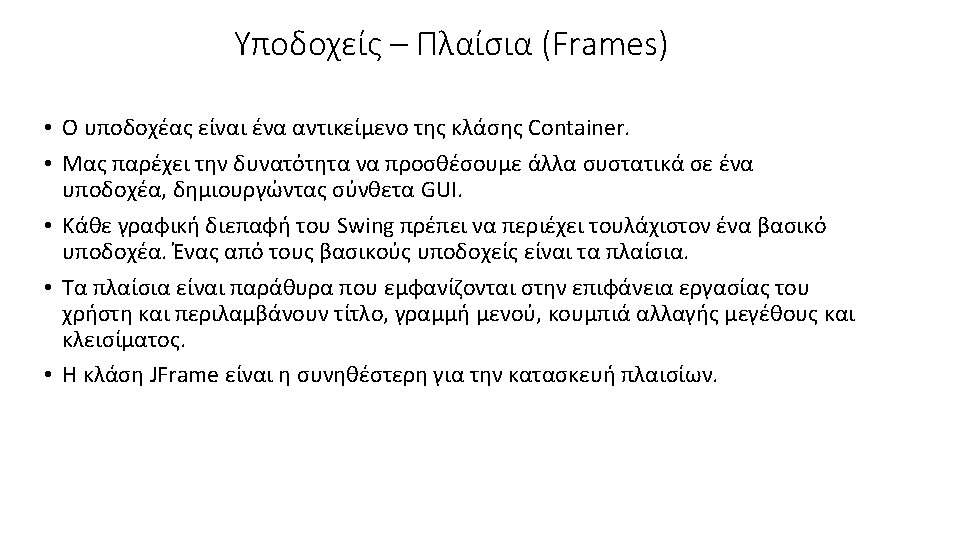
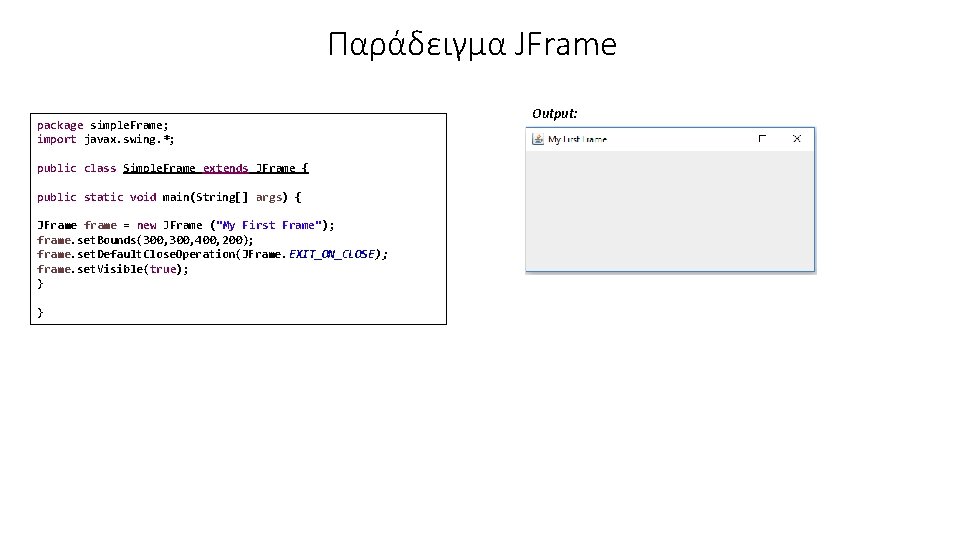
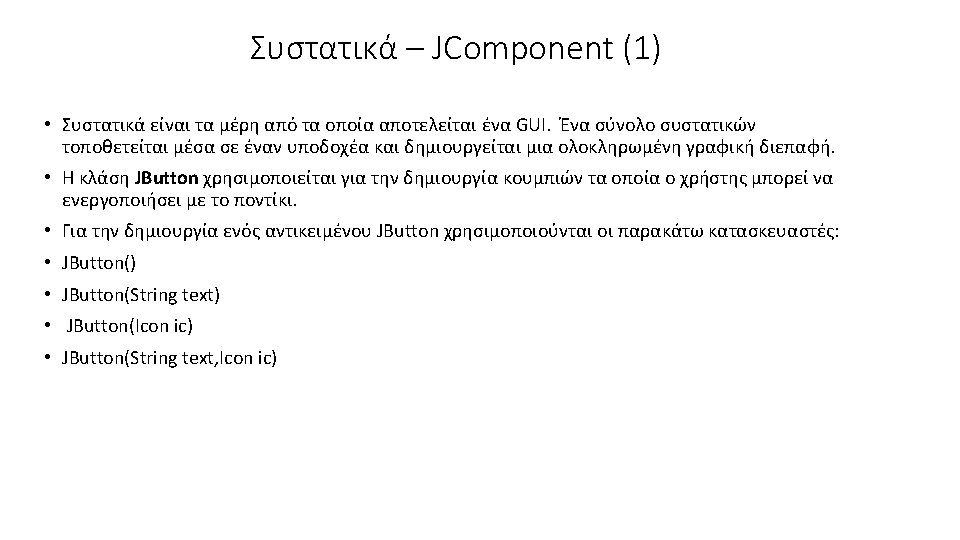
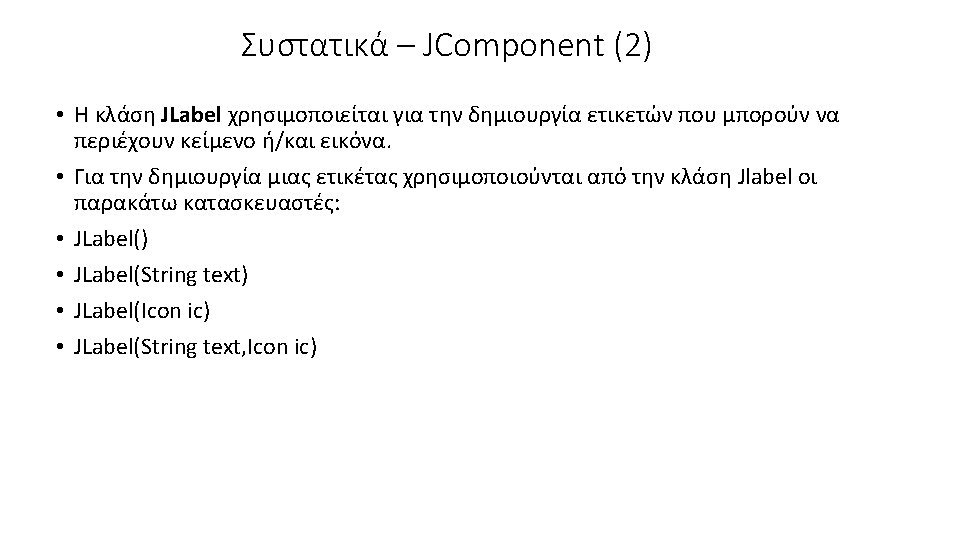
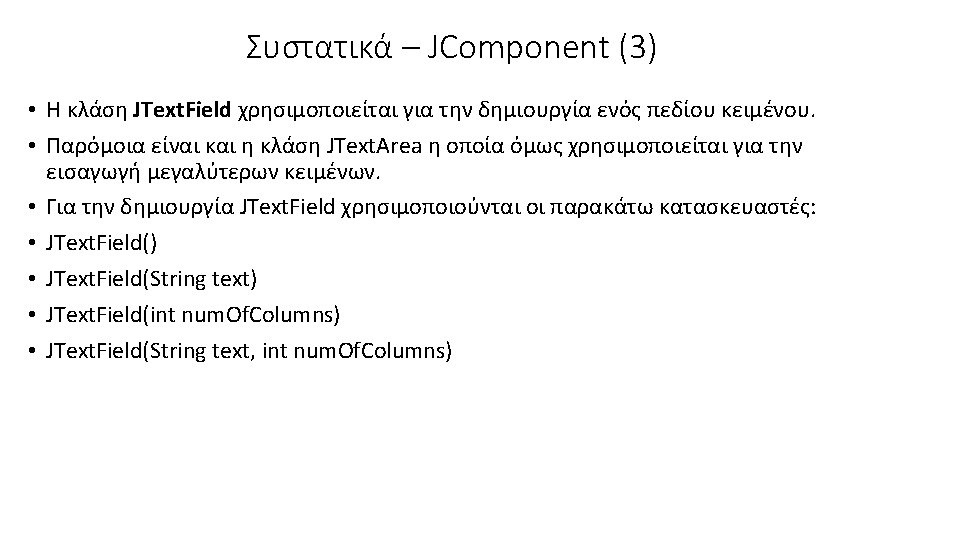
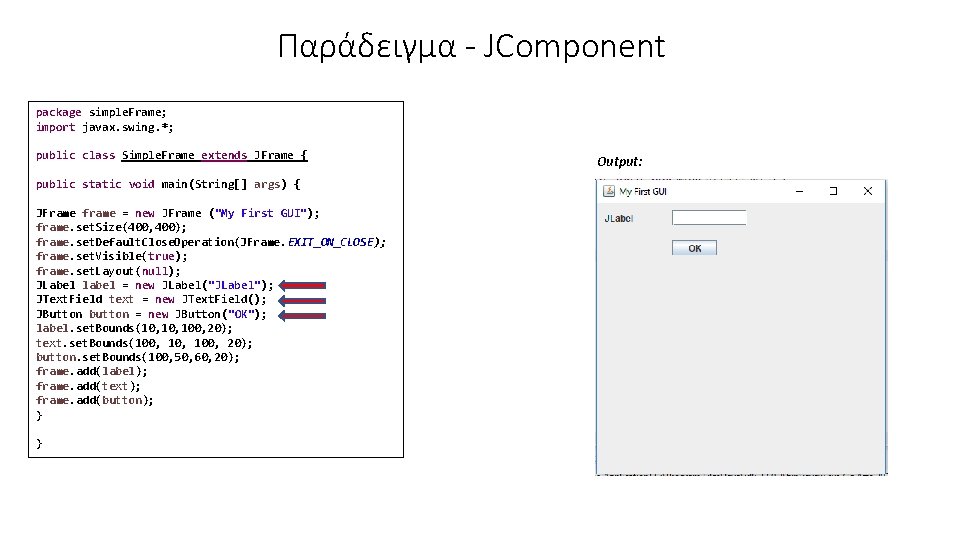
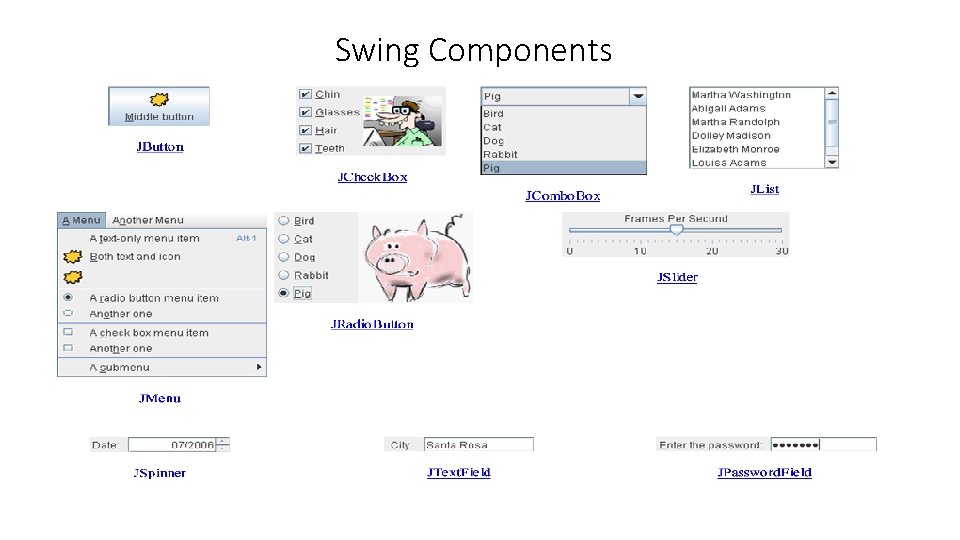
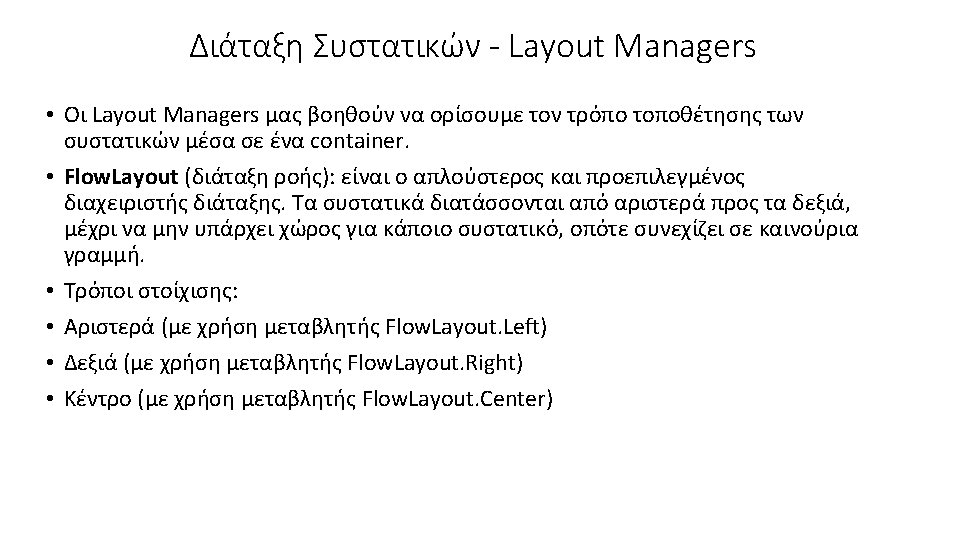
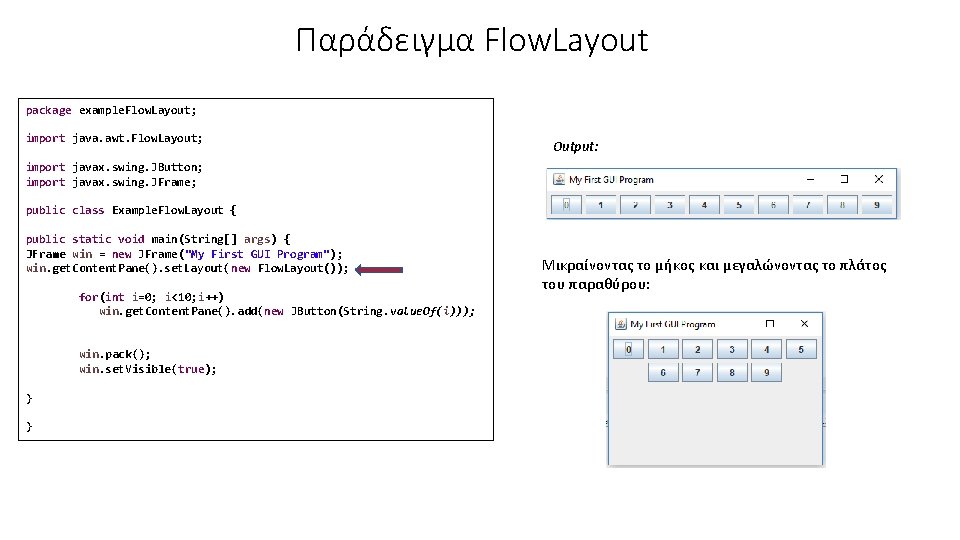
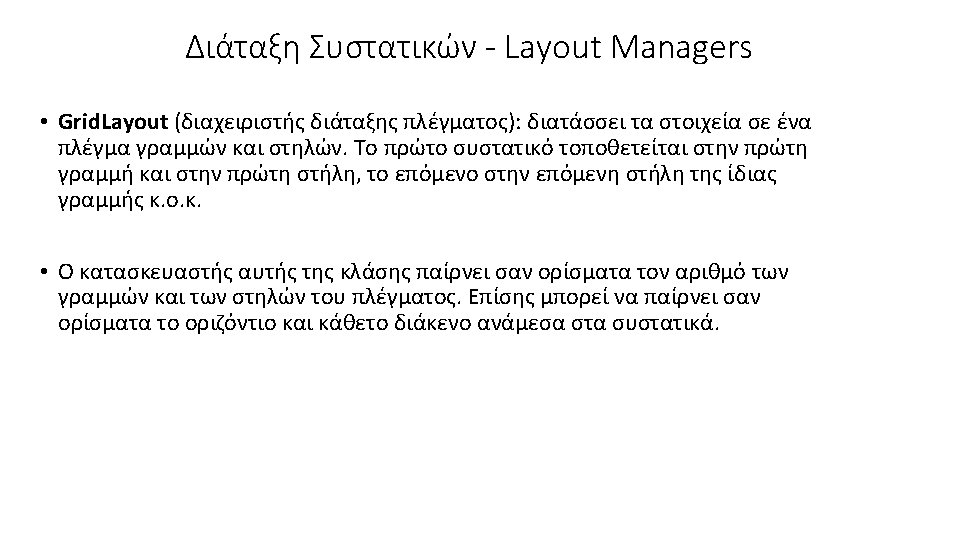
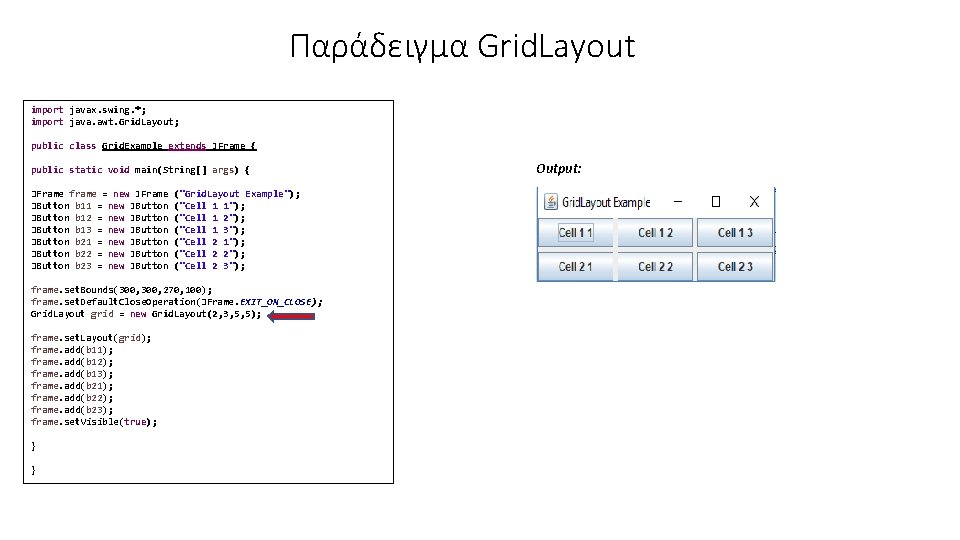
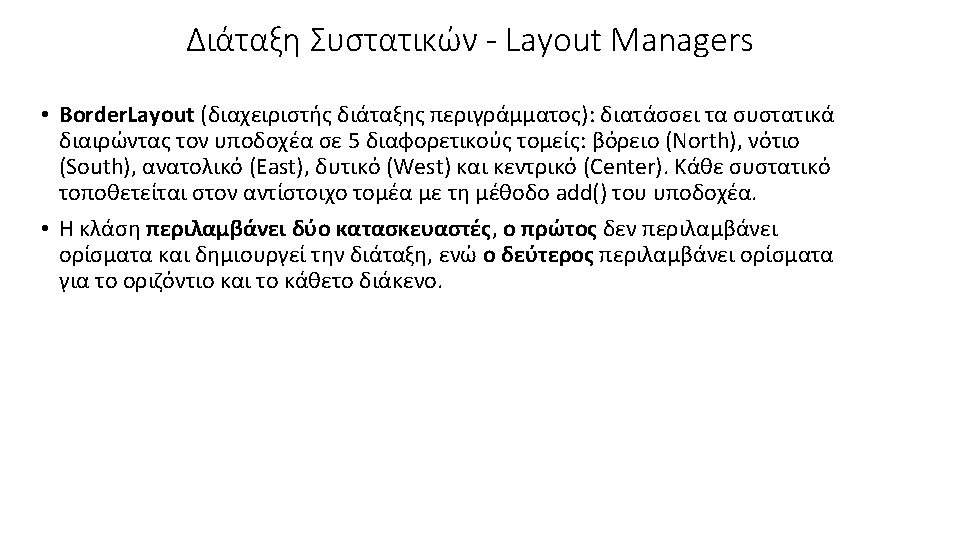
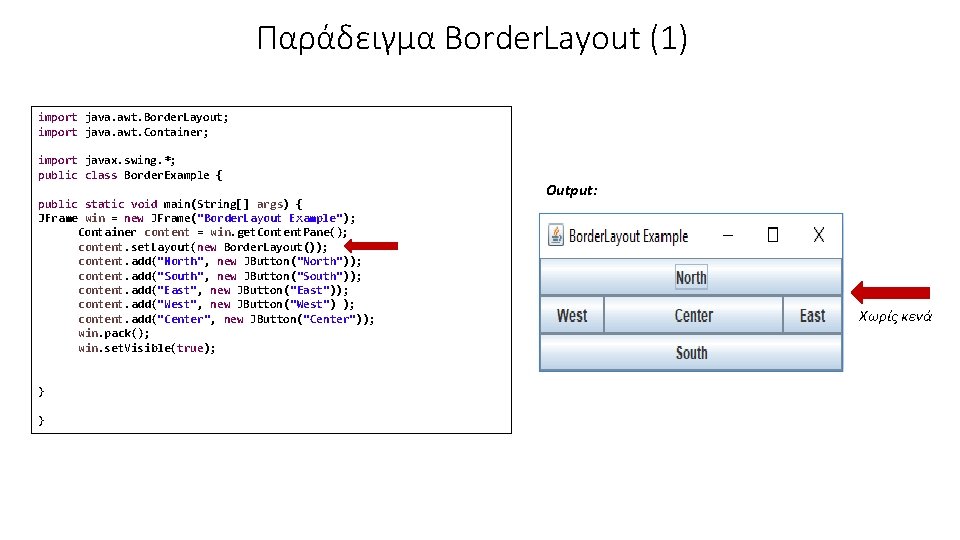
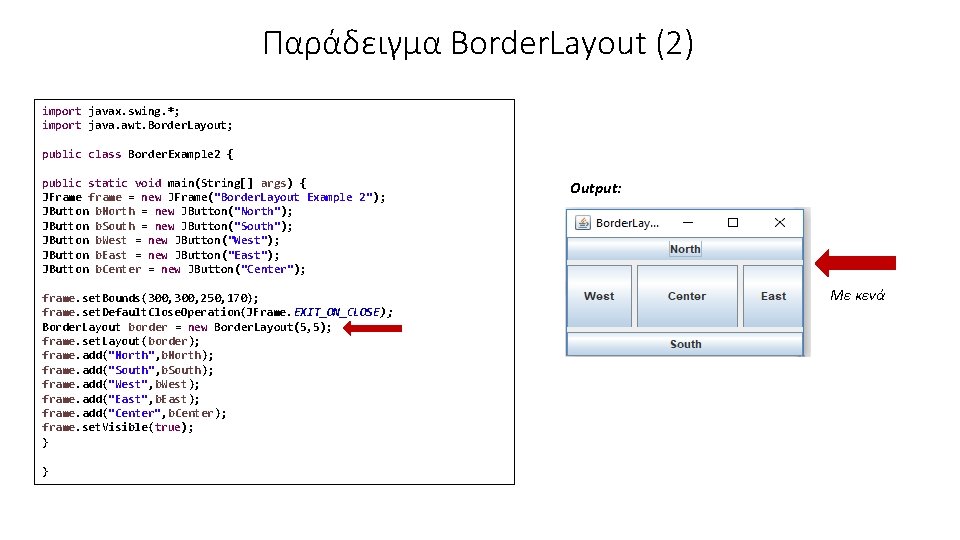
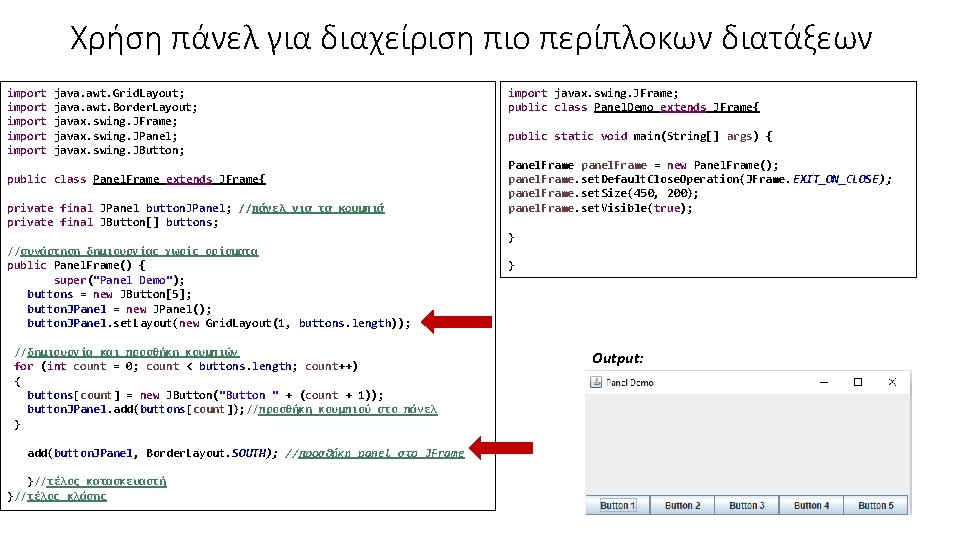
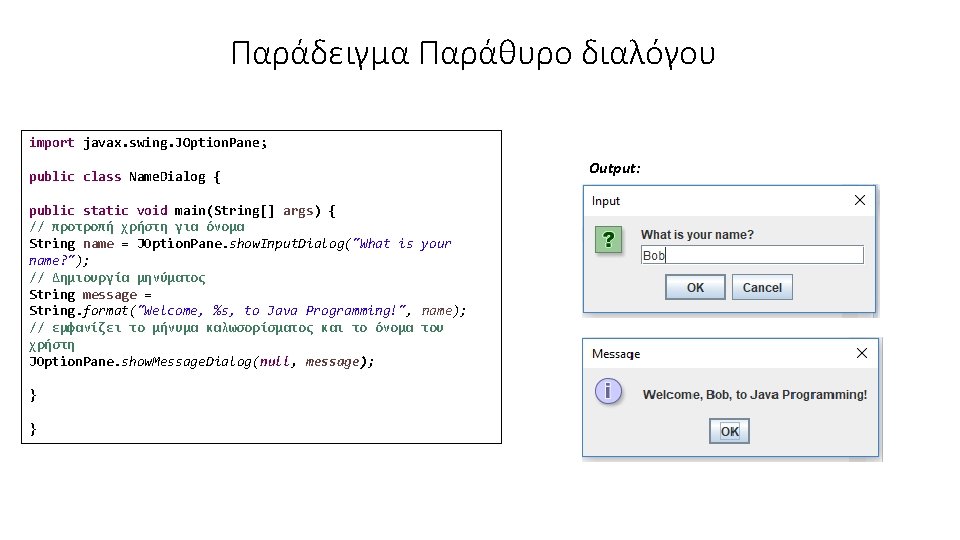
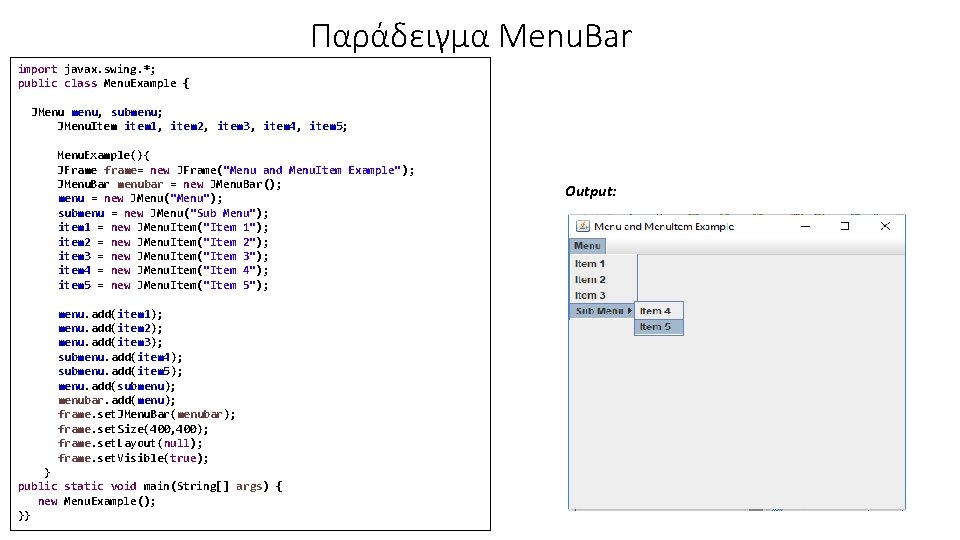
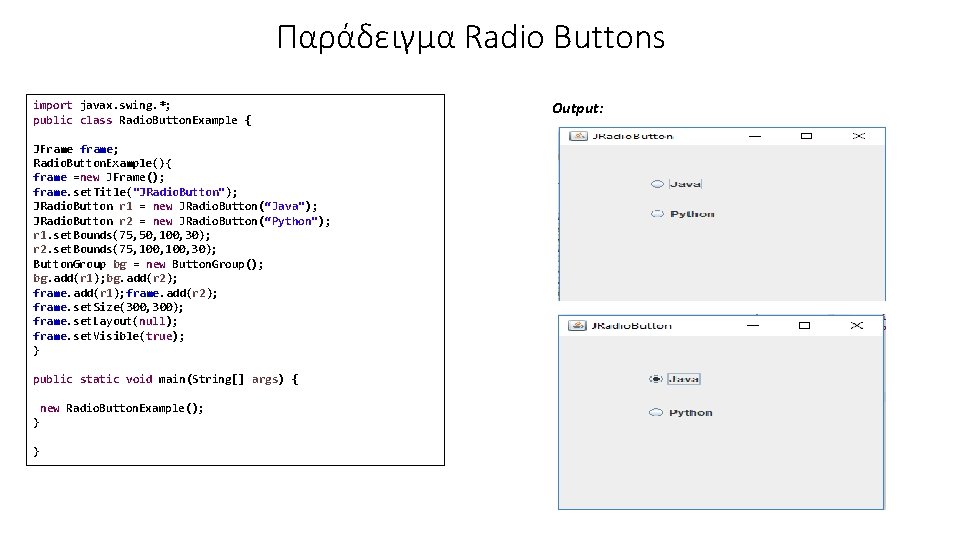
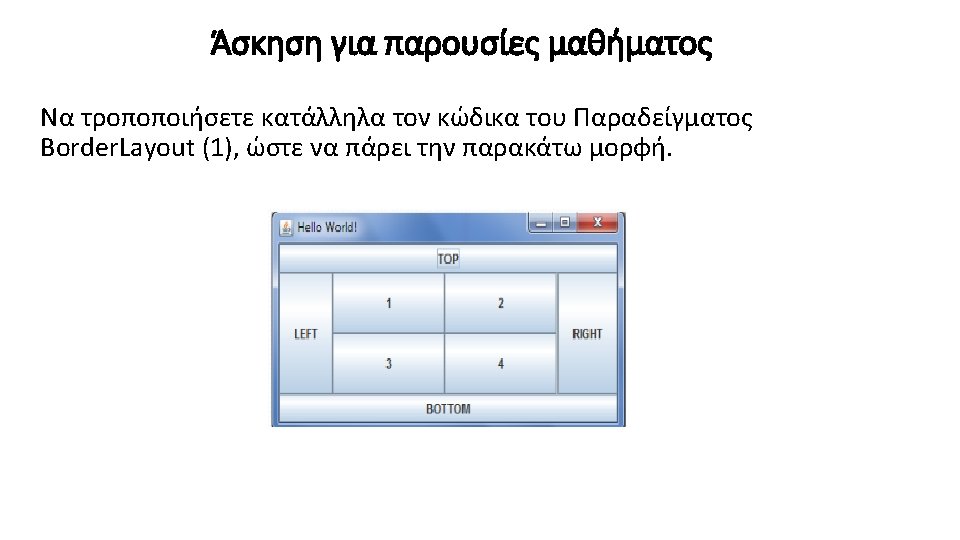
- Slides: 24
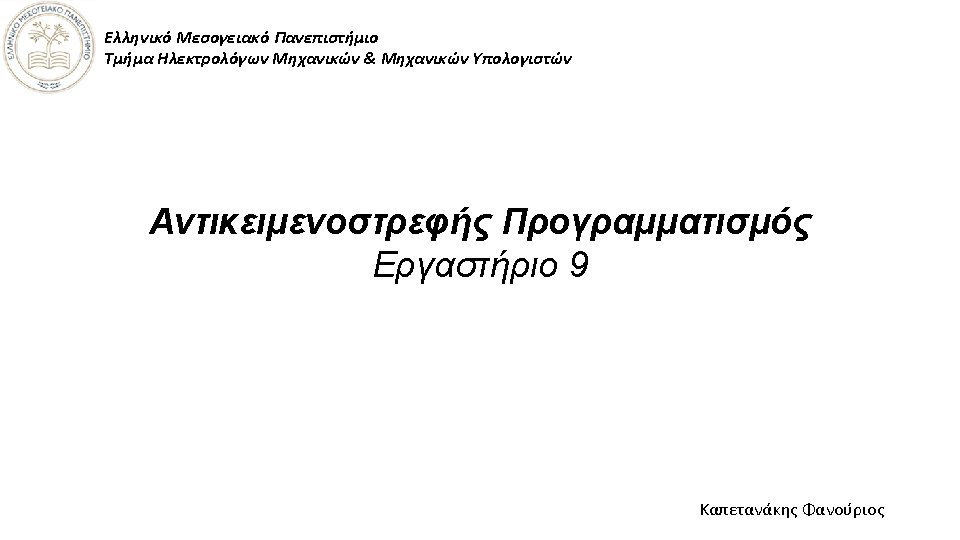
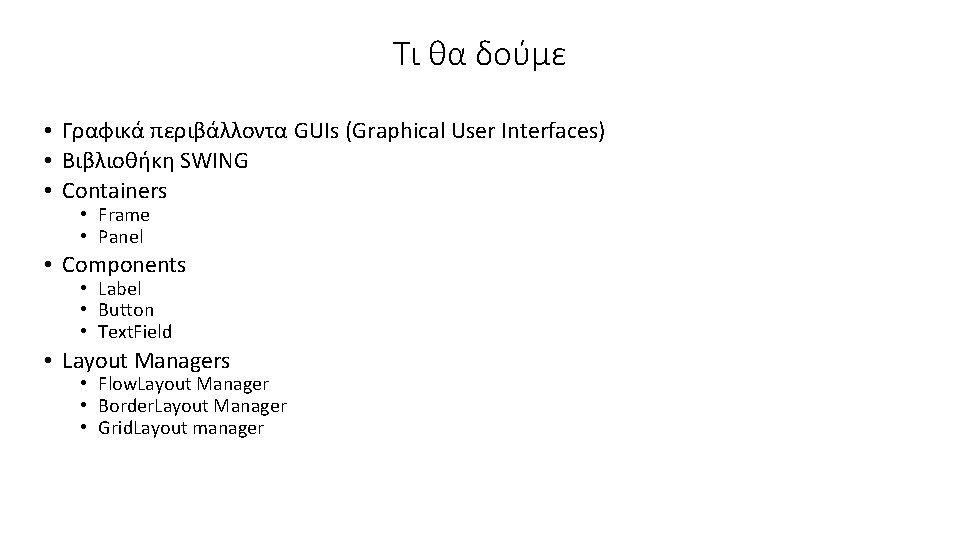
Τι θα δούμε • Γραφικά περιβάλλοντα GUIs (Graphical User Interfaces) • Βιβλιοθήκη SWING • Containers • Frame • Panel • Components • Label • Button • Text. Field • Layout Managers • Flow. Layout Manager • Border. Layout Manager • Grid. Layout manager
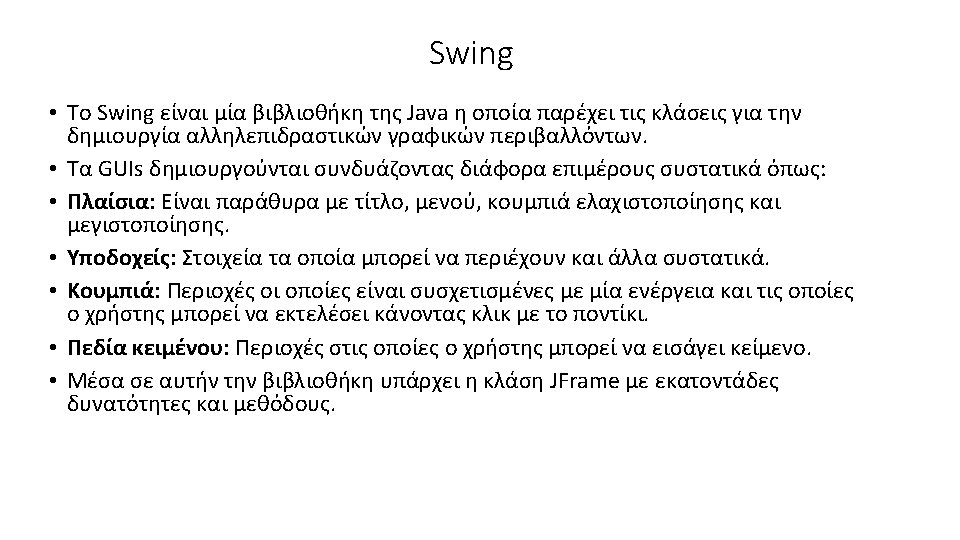
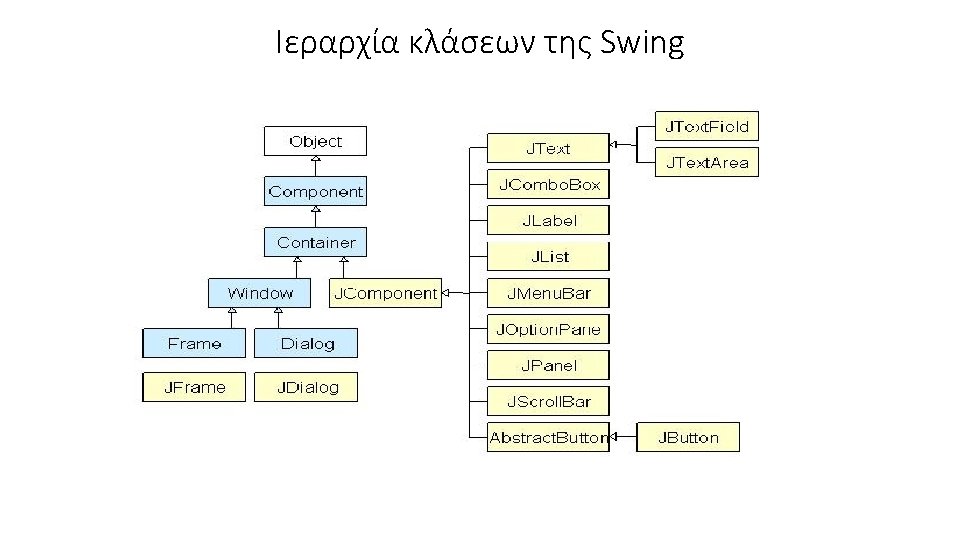
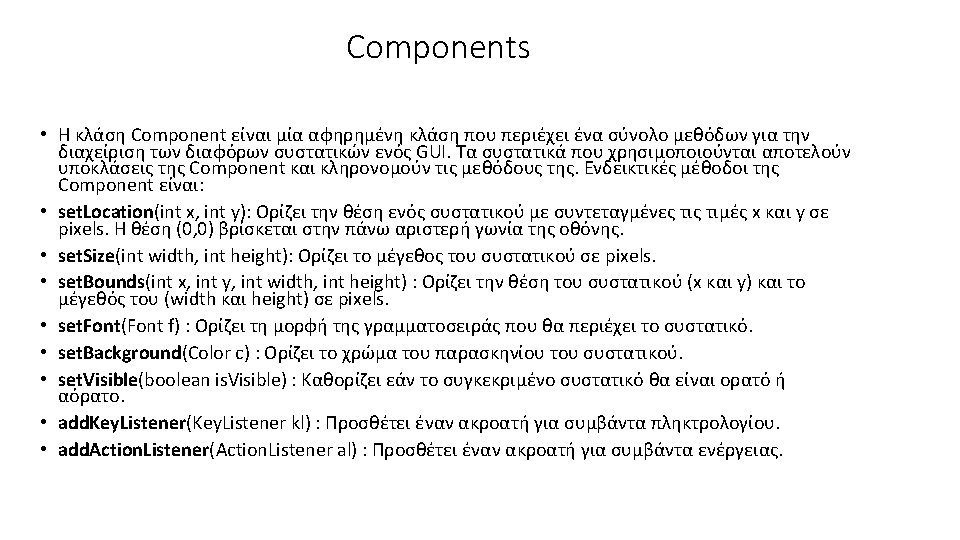
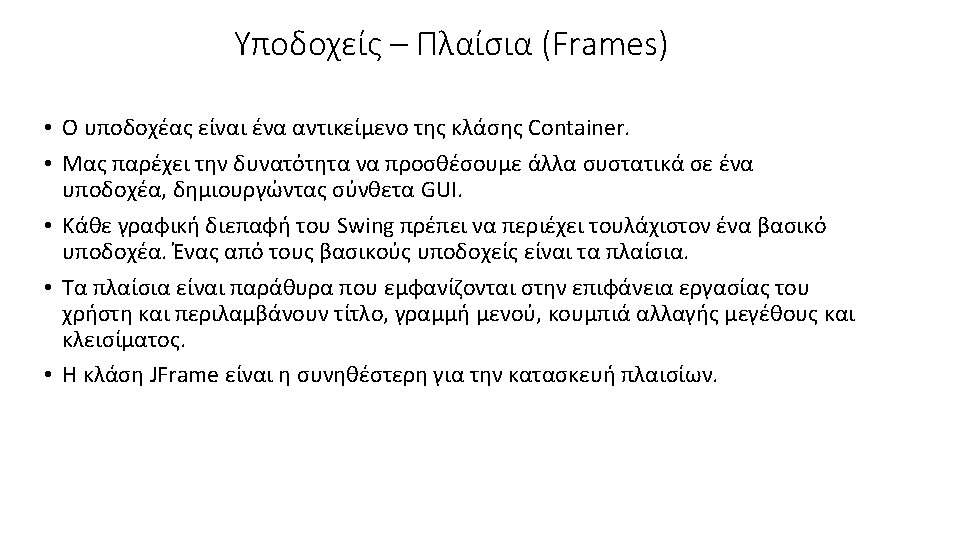
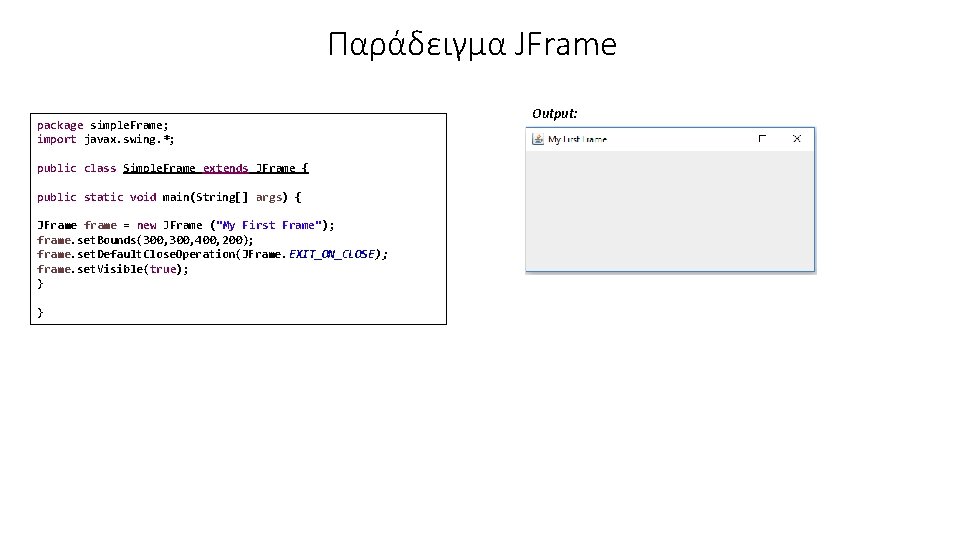
Παράδειγμα JFrame package simple. Frame; import javax. swing. *; public class Simple. Frame extends JFrame { public static void main(String[] args) { JFrame frame = new JFrame ("My First Frame"); frame. set. Bounds(300, 400, 200); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); frame. set. Visible(true); } } Output:
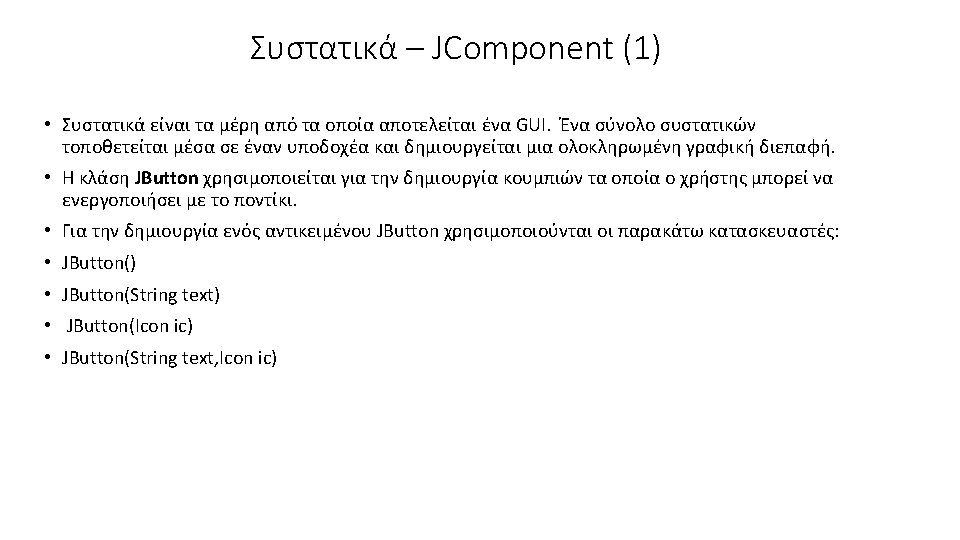
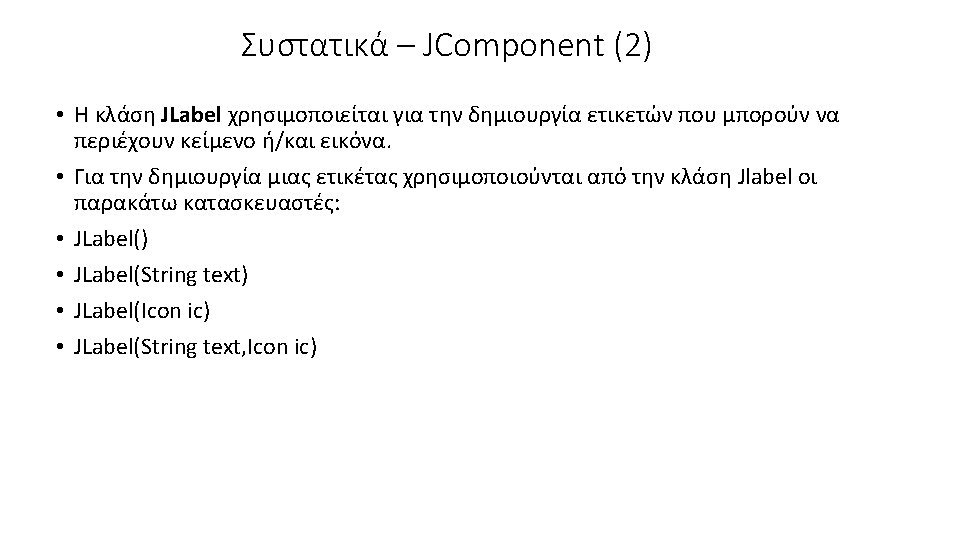
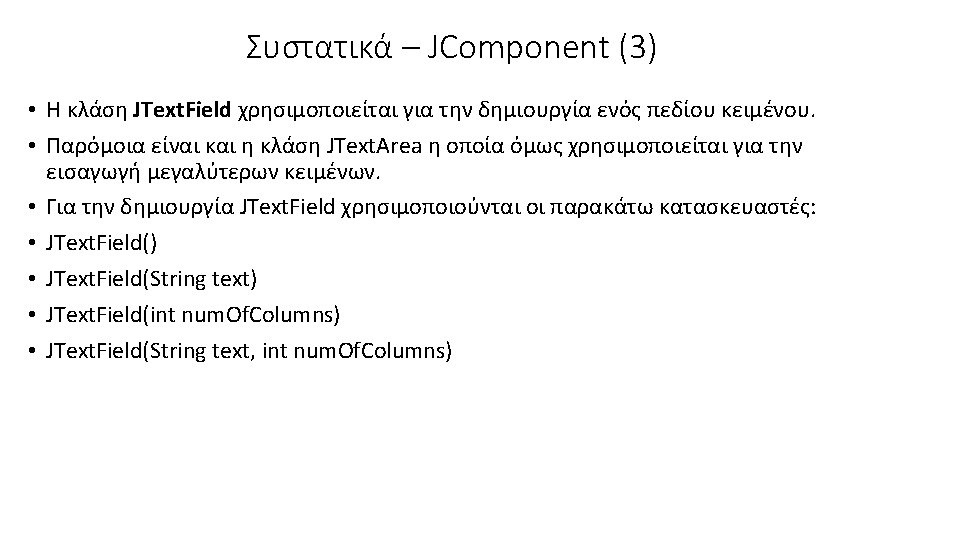
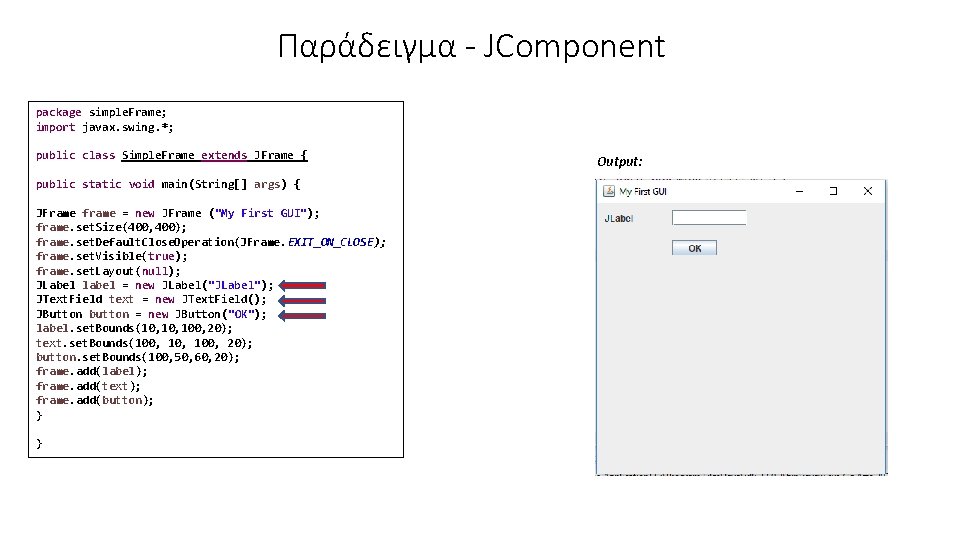
Παράδειγμα - JComponent package simple. Frame; import javax. swing. *; public class Simple. Frame extends JFrame { public static void main(String[] args) { JFrame frame = new JFrame ("My First GUI"); frame. set. Size(400, 400); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); frame. set. Visible(true); frame. set. Layout(null); JLabel label = new JLabel("JLabel"); JText. Field text = new JText. Field(); JButton button = new JButton("OK"); label. set. Bounds(10, 100, 20); text. set. Bounds(100, 20); button. set. Bounds(100, 50, 60, 20); frame. add(label); frame. add(text); frame. add(button); } } Output:
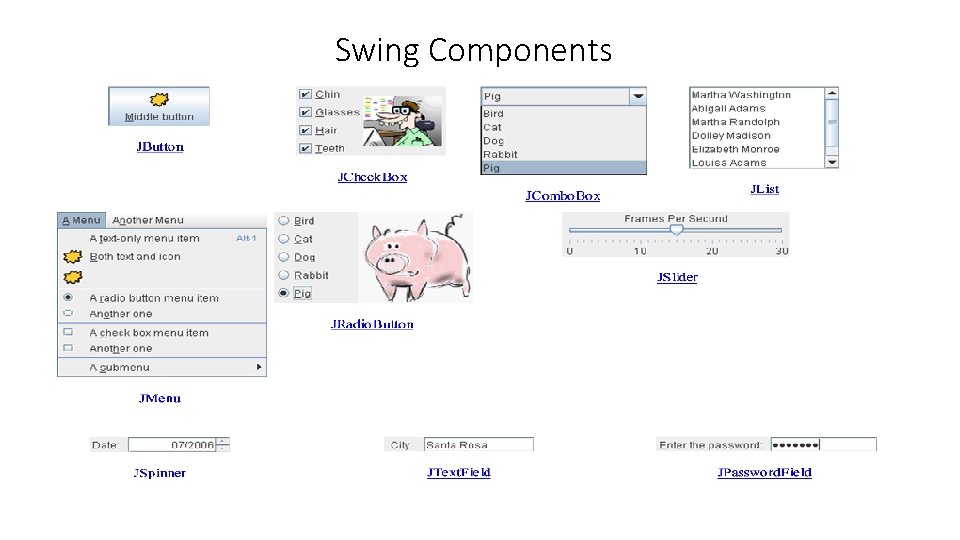
Swing Components
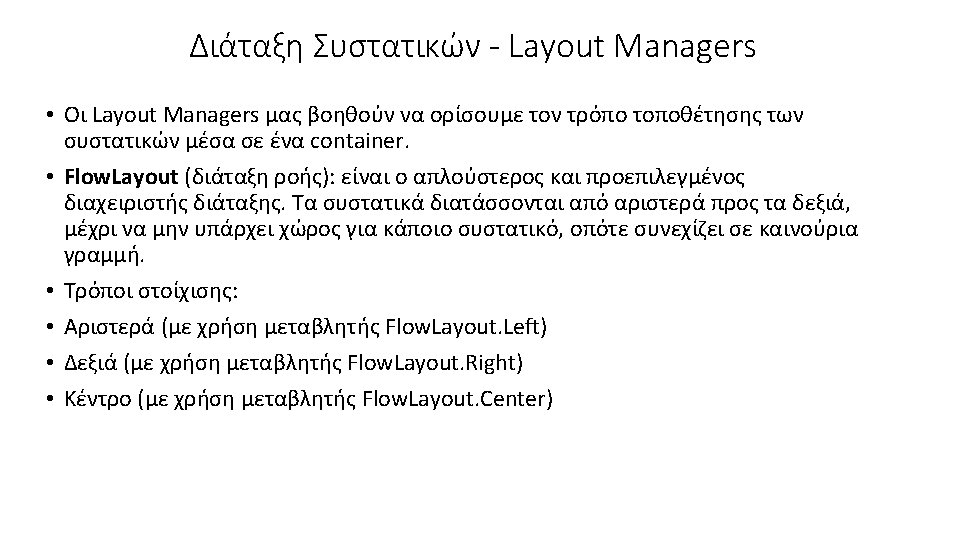
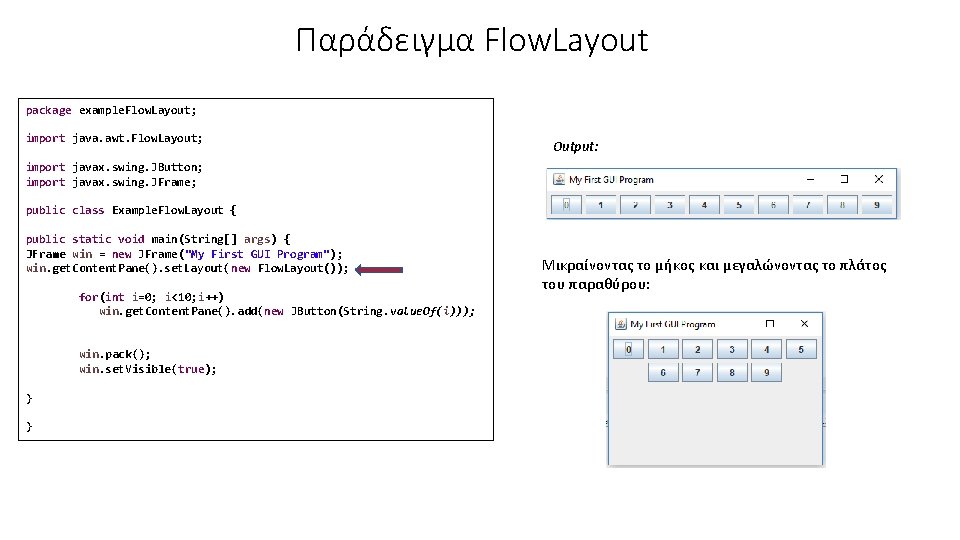
Παράδειγμα Flow. Layout package example. Flow. Layout; import java. awt. Flow. Layout; Output: import javax. swing. JButton; import javax. swing. JFrame; public class Example. Flow. Layout { public static void main(String[] args) { JFrame win = new JFrame("My First GUI Program"); win. get. Content. Pane(). set. Layout(new Flow. Layout()); for(int i=0; i<10; i++) win. get. Content. Pane(). add(new JButton(String. value. Of(i))); win. pack(); win. set. Visible(true); } } Μικραίνοντας το μήκος και μεγαλώνοντας το πλάτος του παραθύρου:
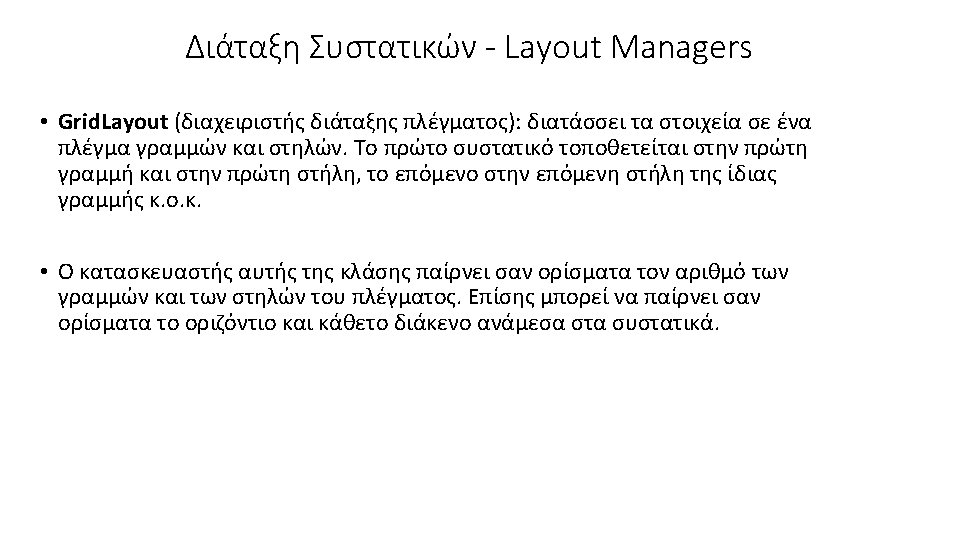
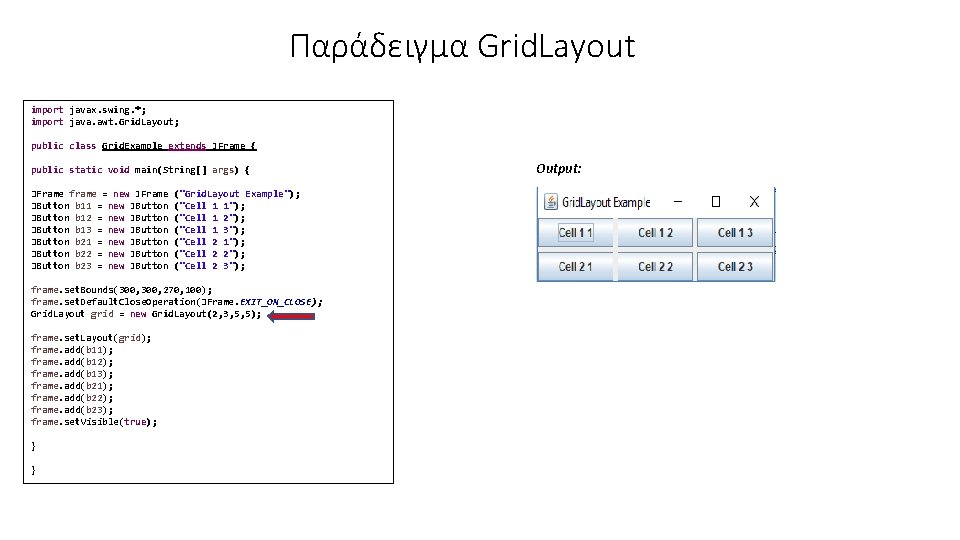
Παράδειγμα Grid. Layout import javax. swing. *; import java. awt. Grid. Layout; public class Grid. Example extends JFrame { public static void main(String[] args) { JFrame frame = new JFrame JButton b 11 = new JButton b 12 = new JButton b 13 = new JButton b 21 = new JButton b 22 = new JButton b 23 = new JButton ("Grid. Layout Example"); ("Cell 1 1"); ("Cell 1 2"); ("Cell 1 3"); ("Cell 2 1"); ("Cell 2 2"); ("Cell 2 3"); frame. set. Bounds(300, 270, 100); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); Grid. Layout grid = new Grid. Layout(2, 3, 5, 5); frame. set. Layout(grid); frame. add(b 11); frame. add(b 12); frame. add(b 13); frame. add(b 21); frame. add(b 22); frame. add(b 23); frame. set. Visible(true); } } Output:
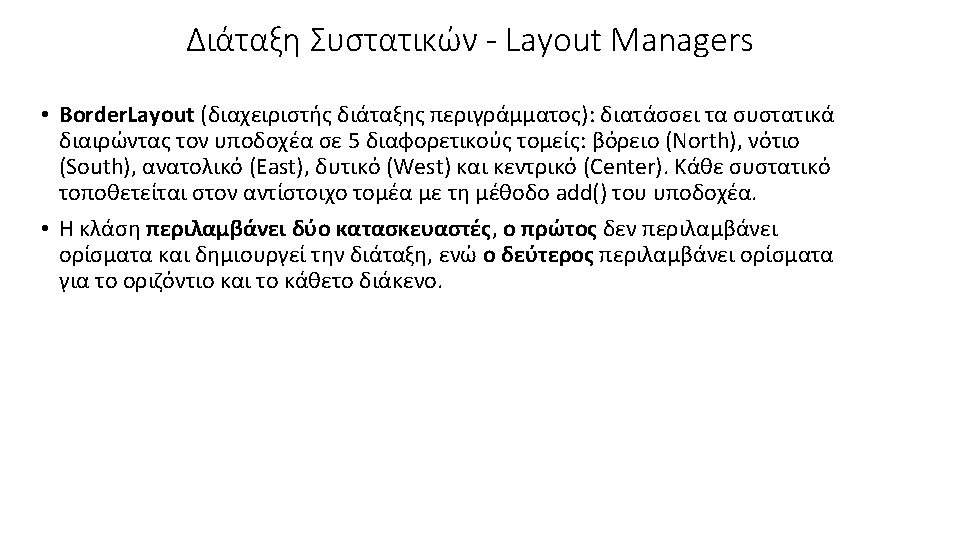
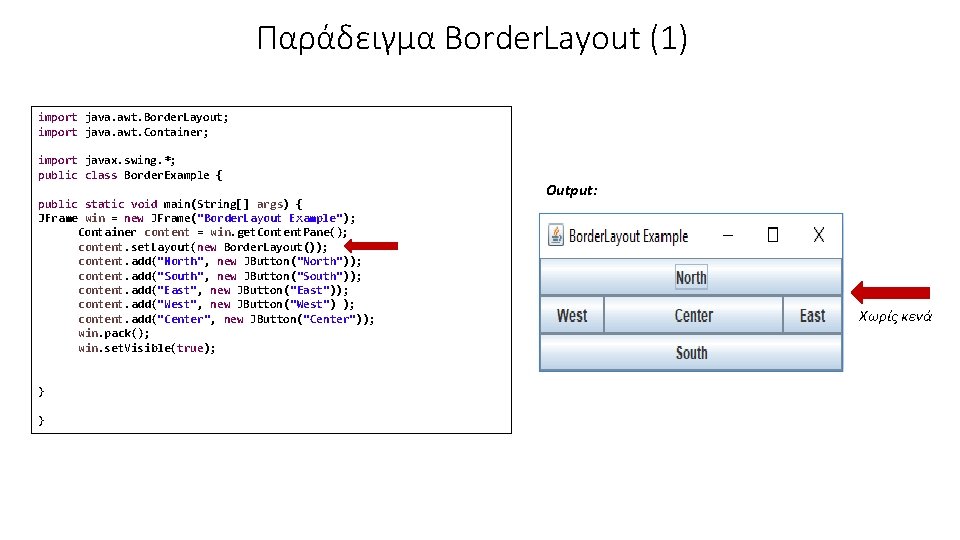
Παράδειγμα Border. Layout (1) import java. awt. Border. Layout; import java. awt. Container; import javax. swing. *; public class Border. Example { public static void main(String[] args) { JFrame win = new JFrame("Border. Layout Example"); Container content = win. get. Content. Pane(); content. set. Layout(new Border. Layout()); content. add("North", new JButton("North")); content. add("South", new JButton("South")); content. add("East", new JButton("East")); content. add("West", new JButton("West") ); content. add("Center", new JButton("Center")); win. pack(); win. set. Visible(true); } } Output: Χωρίς κενά
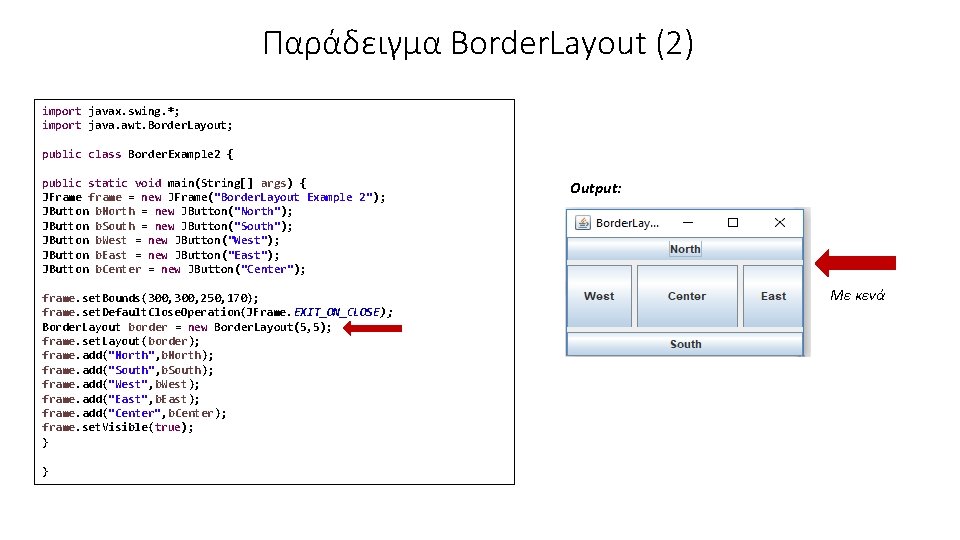
Παράδειγμα Border. Layout (2) import javax. swing. *; import java. awt. Border. Layout; public class Border. Example 2 { public static void main(String[] args) { JFrame frame = new JFrame("Border. Layout Example 2"); JButton b. North = new JButton("North"); JButton b. South = new JButton("South"); JButton b. West = new JButton("West"); JButton b. East = new JButton("East"); JButton b. Center = new JButton("Center"); frame. set. Bounds(300, 250, 170); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); Border. Layout border = new Border. Layout(5, 5); frame. set. Layout(border); frame. add("North", b. North); frame. add("South", b. South); frame. add("West", b. West); frame. add("East", b. East); frame. add("Center", b. Center); frame. set. Visible(true); } } Output: Με κενά
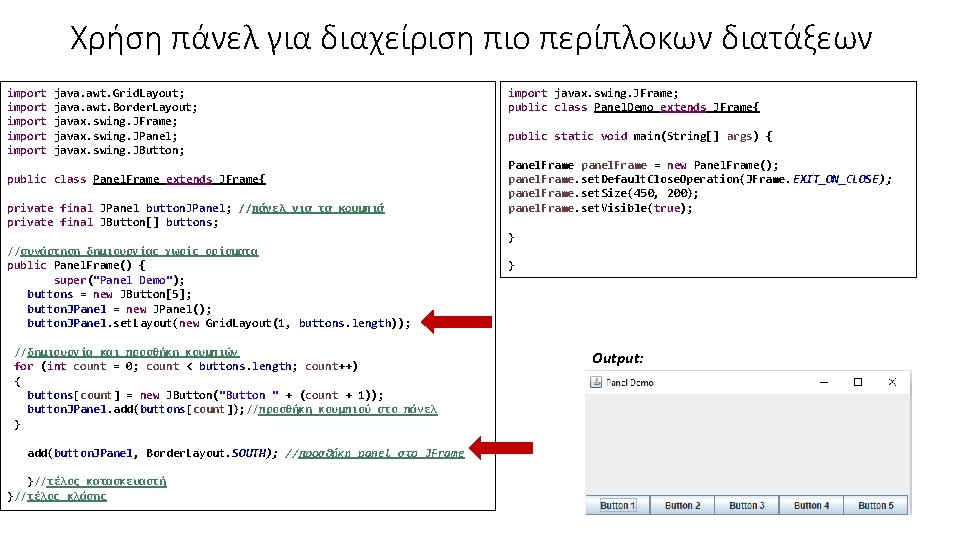
Χρήση πάνελ για διαχείριση πιο περίπλοκων διατάξεων import import java. awt. Grid. Layout; java. awt. Border. Layout; javax. swing. JFrame; javax. swing. JPanel; javax. swing. JButton; public class Panel. Frame extends JFrame{ private final JPanel button. JPanel; //πάνελ για τα κουμπιά private final JButton[] buttons; import javax. swing. JFrame; public class Panel. Demo extends JFrame{ public static void main(String[] args) { Panel. Frame panel. Frame = new Panel. Frame(); panel. Frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); panel. Frame. set. Size(450, 200); panel. Frame. set. Visible(true); } //συνάρτηση δημιουργίας χωρίς ορίσματα public Panel. Frame() { super("Panel Demo"); buttons = new JButton[5]; button. JPanel = new JPanel(); button. JPanel. set. Layout(new Grid. Layout(1, buttons. length)); //δημιουργία και προσθήκη κουμπιών for (int count = 0; count < buttons. length; count++) { buttons[count] = new JButton("Button " + (count + 1)); button. JPanel. add(buttons[count]); //προσθήκη κουμπιού στο πάνελ } add(button. JPanel, Border. Layout. SOUTH); //προσθήκη panel στο JFrame }//τέλος κατασκευαστή }//τέλος κλάσης } Output:
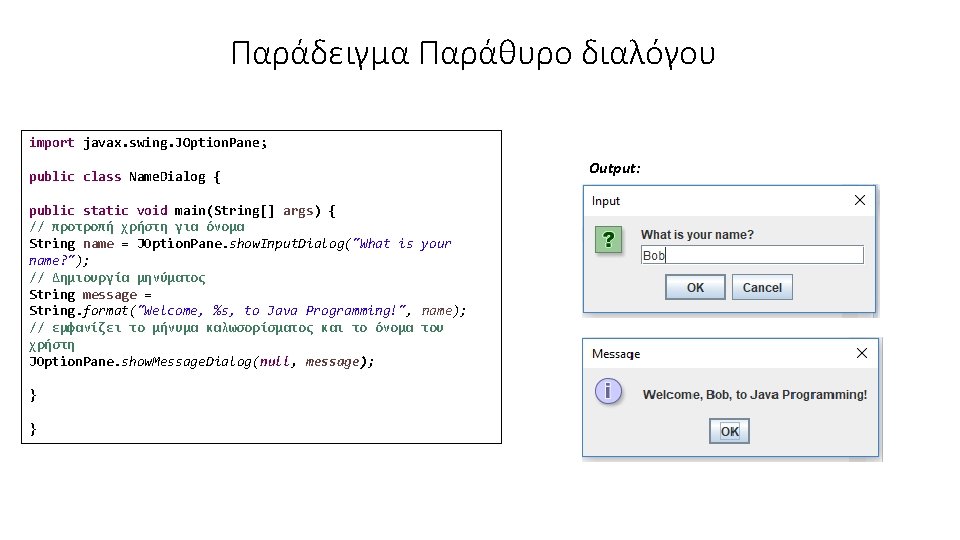
Παράδειγμα Παράθυρο διαλόγου import javax. swing. JOption. Pane; public class Name. Dialog { public static void main(String[] args) { // προτροπή χρήστη για όνομα String name = JOption. Pane. show. Input. Dialog("What is your name? "); // Δημιουργία μηνύματος String message = String. format("Welcome, %s, to Java Programming!", name); // εμφανίζει το μήνυμα καλωσορίσματος και το όνομα του χρήστη JOption. Pane. show. Message. Dialog(null, message); } } Output:
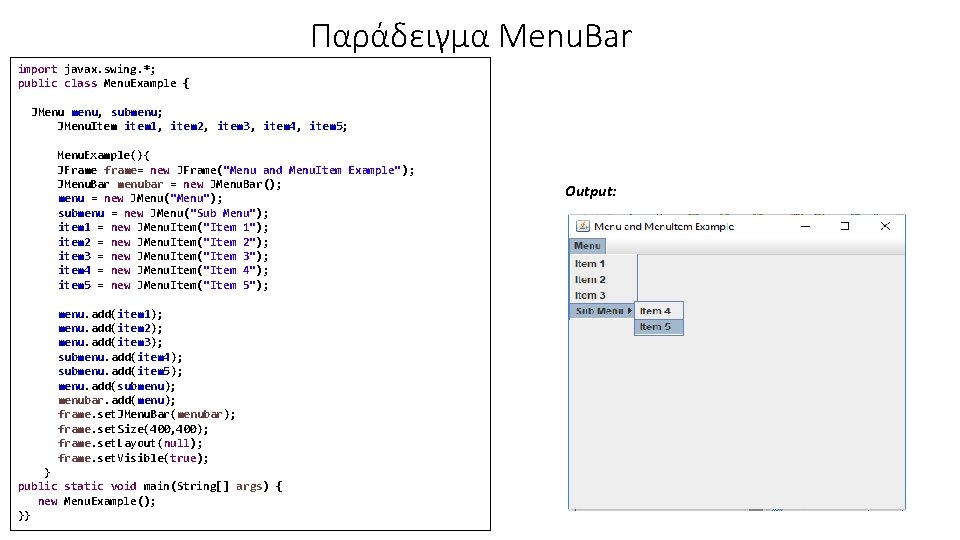
Παράδειγμα Menu. Bar import javax. swing. *; public class Menu. Example { JMenu menu, submenu; JMenu. Item item 1, item 2, item 3, item 4, item 5; Menu. Example(){ JFrame frame= new JFrame("Menu and Menu. Item Example"); JMenu. Bar menubar = new JMenu. Bar(); menu = new JMenu("Menu"); submenu = new JMenu("Sub Menu"); item 1 = new JMenu. Item("Item 1"); item 2 = new JMenu. Item("Item 2"); item 3 = new JMenu. Item("Item 3"); item 4 = new JMenu. Item("Item 4"); item 5 = new JMenu. Item("Item 5"); menu. add(item 1); menu. add(item 2); menu. add(item 3); submenu. add(item 4); submenu. add(item 5); menu. add(submenu); menubar. add(menu); frame. set. JMenu. Bar(menubar); frame. set. Size(400, 400); frame. set. Layout(null); frame. set. Visible(true); } public static void main(String[] args) { new Menu. Example(); }} Output:
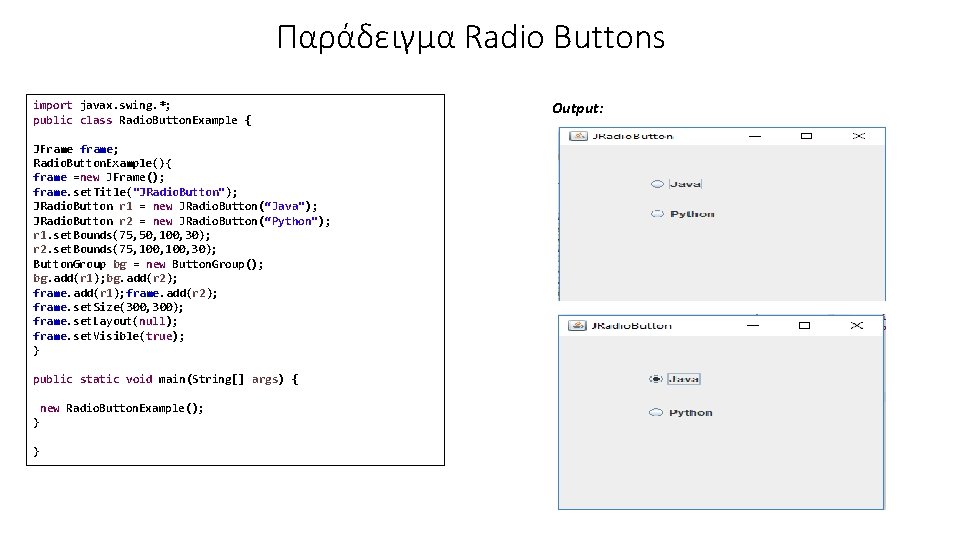
Παράδειγμα Radio Buttons import javax. swing. *; public class Radio. Button. Example { JFrame frame; Radio. Button. Example(){ frame =new JFrame(); frame. set. Title("JRadio. Button"); JRadio. Button r 1 = new JRadio. Button(“Java"); JRadio. Button r 2 = new JRadio. Button(“Python"); r 1. set. Bounds(75, 50, 100, 30); r 2. set. Bounds(75, 100, 30); Button. Group bg = new Button. Group(); bg. add(r 1); bg. add(r 2); frame. add(r 1); frame. add(r 2); frame. set. Size(300, 300); frame. set. Layout(null); frame. set. Visible(true); } public static void main(String[] args) { new Radio. Button. Example(); } } Output:
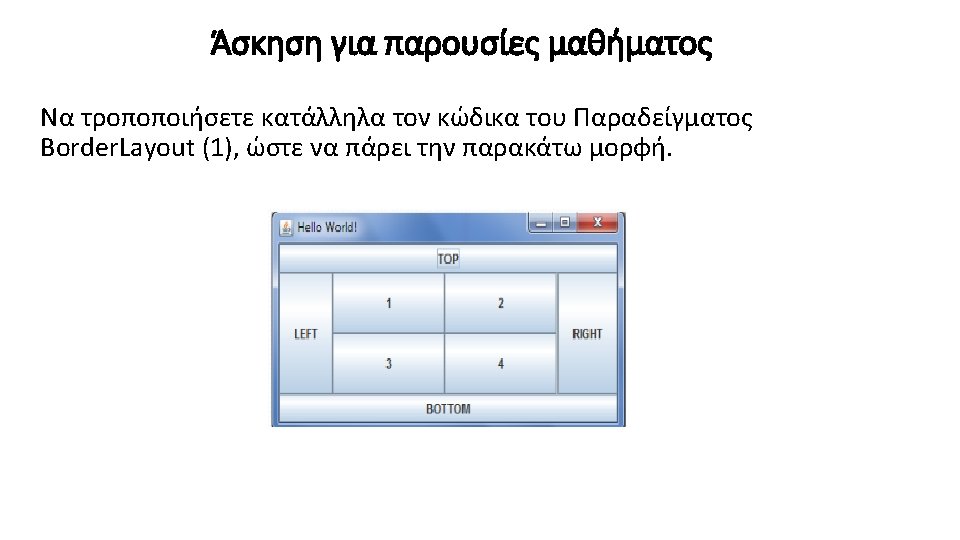
Java gui for r
Java swing container
Swing graphics
Why are user interfaces hard to implement
Heuristic evaluation of user interfaces
User interfaces design dc
[web user interface]
Graphics and guis with matlab
What are the characteristics of gui
Java swing panel
Java swing panel
Continuous panel vs discontinuous panel
Panel zıt panel
Graphical user interface design principles
Component name
User interface history
Graphical user interface
Characteristics of graphical user interface
Java user interface
Graphical user interface in data structures
Graphical user interface testing
History of user interface
Components of graphical user interface
Graphical user interface design principles
Frame relay frame format