GUI Threading Explained and Verified Michael Ernst U
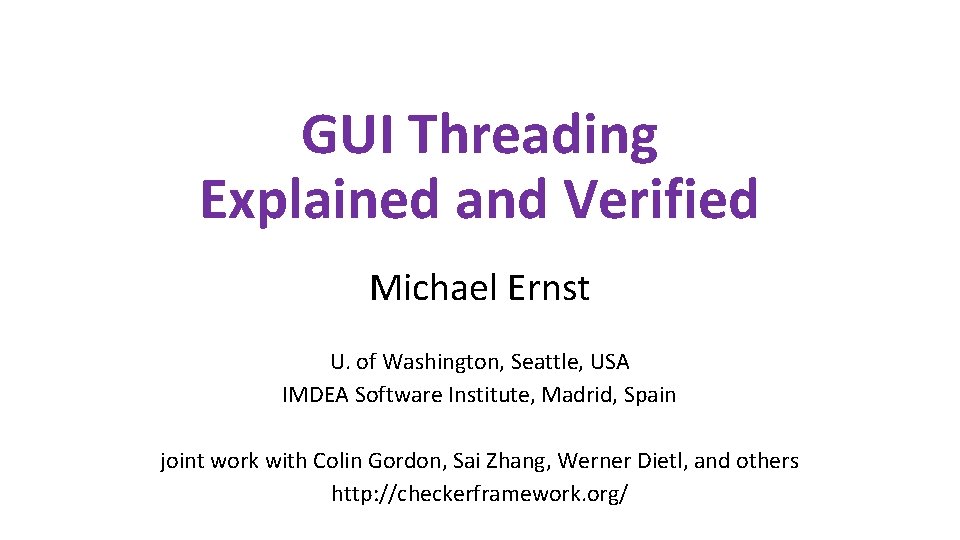
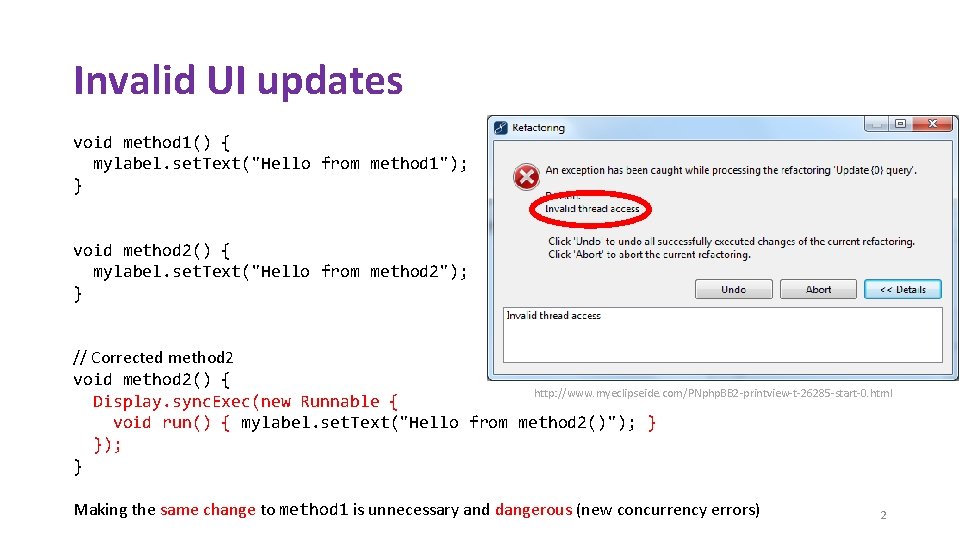
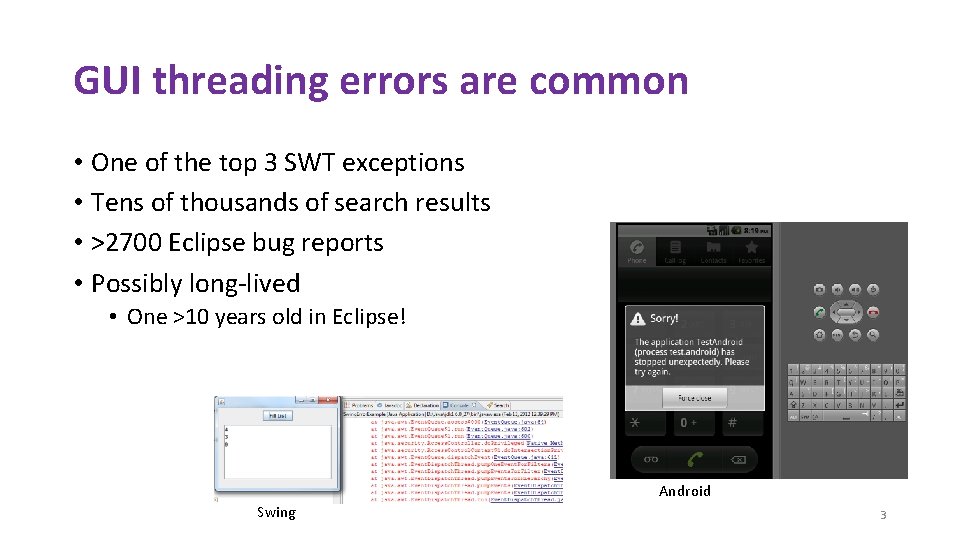
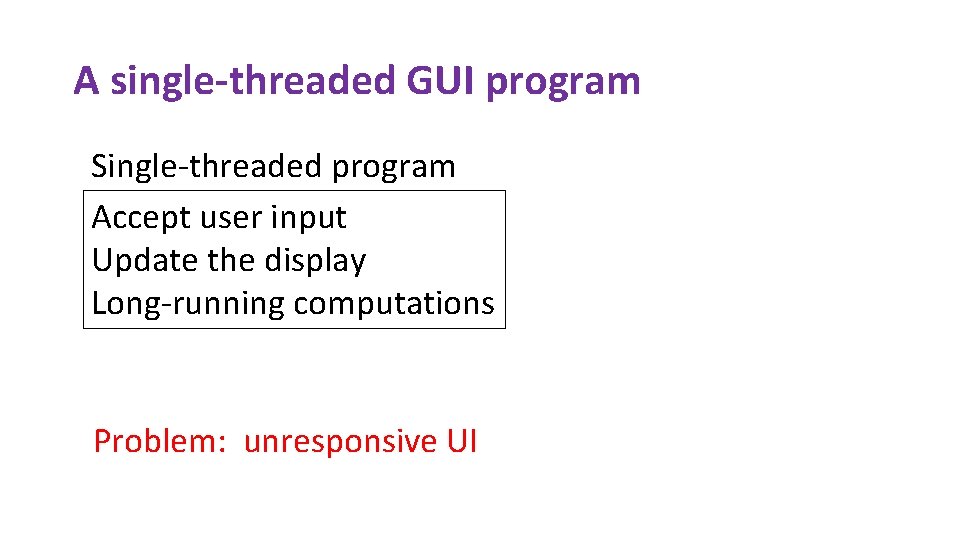
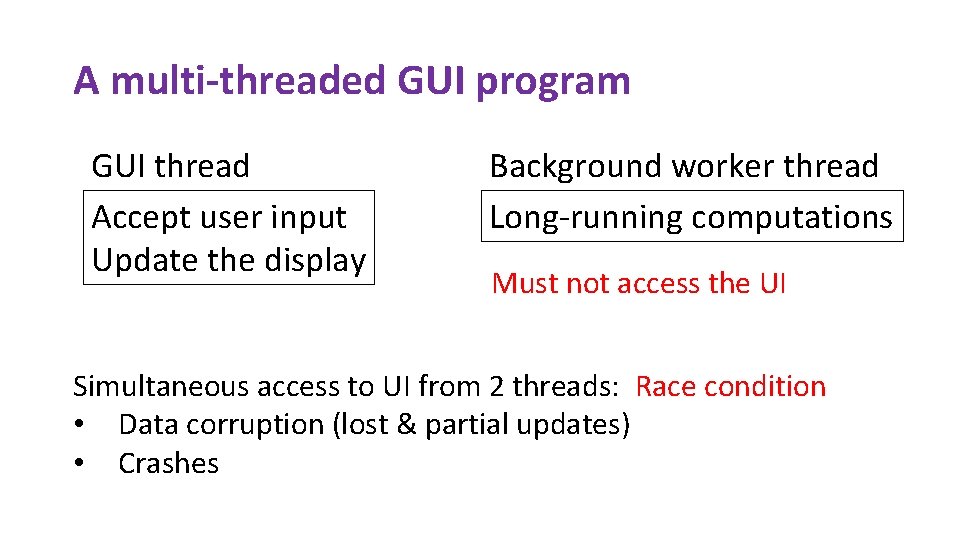
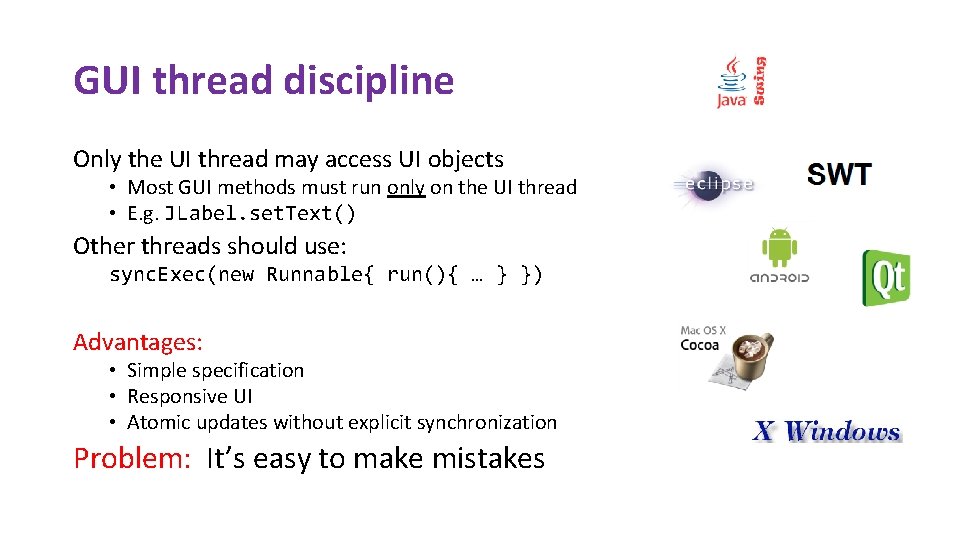
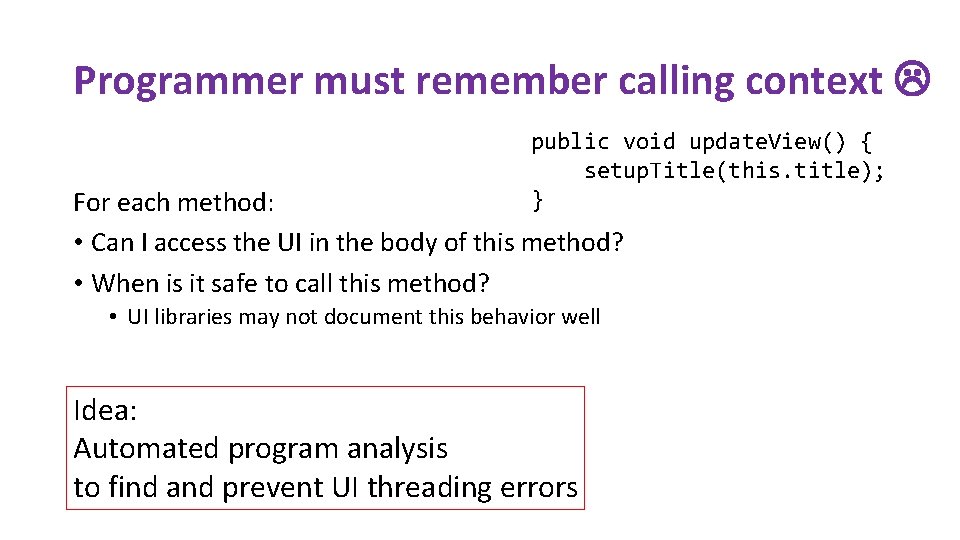
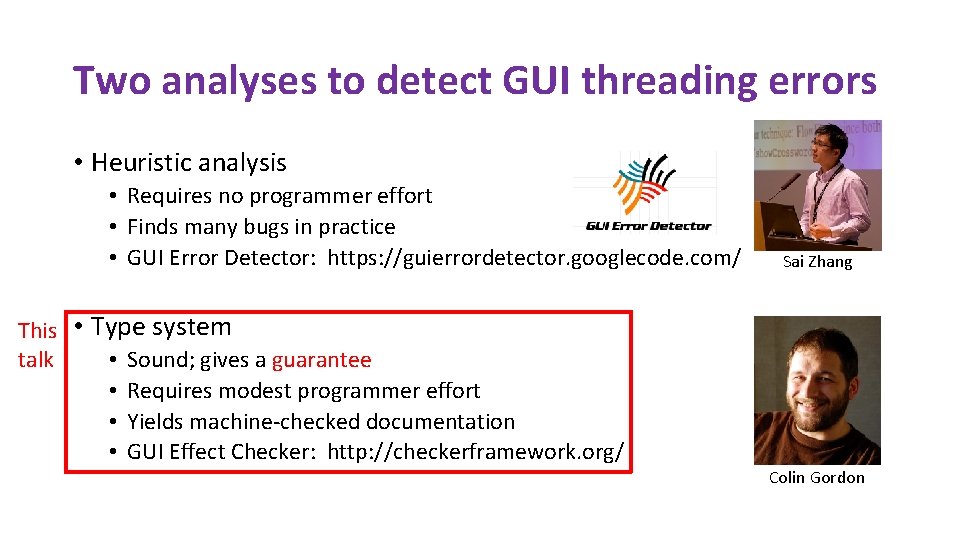
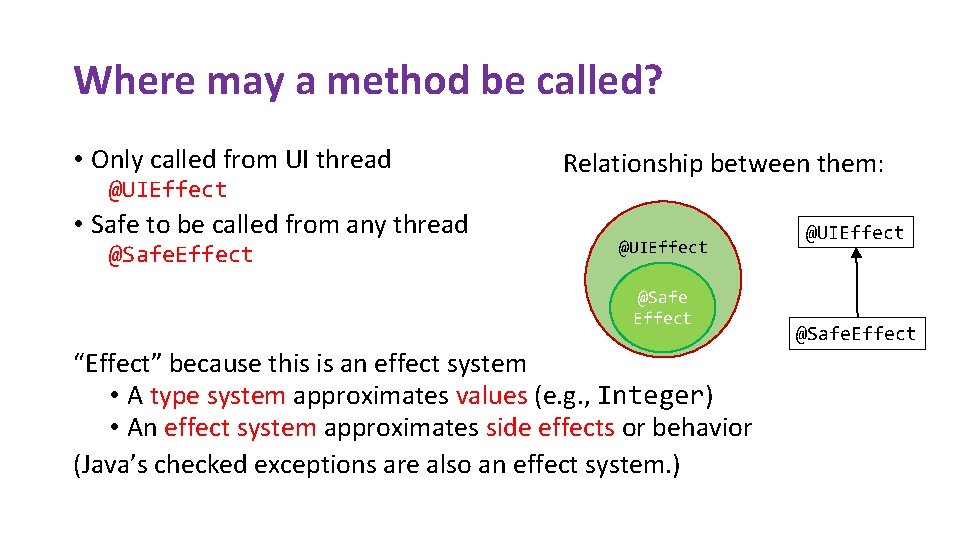
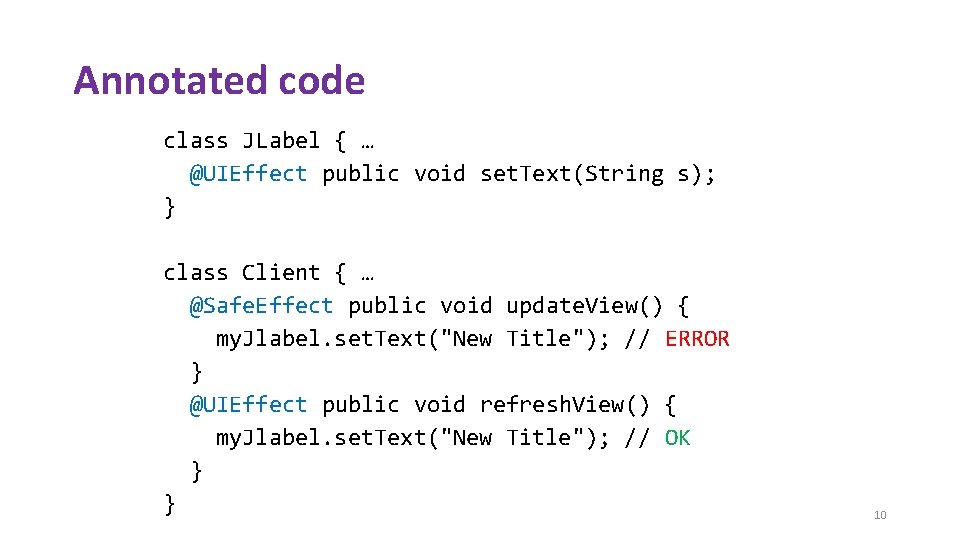
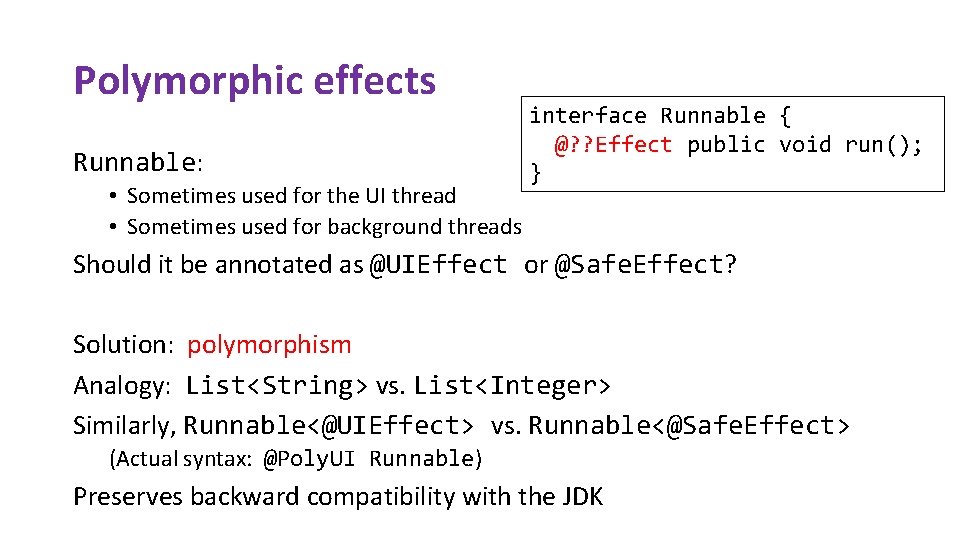
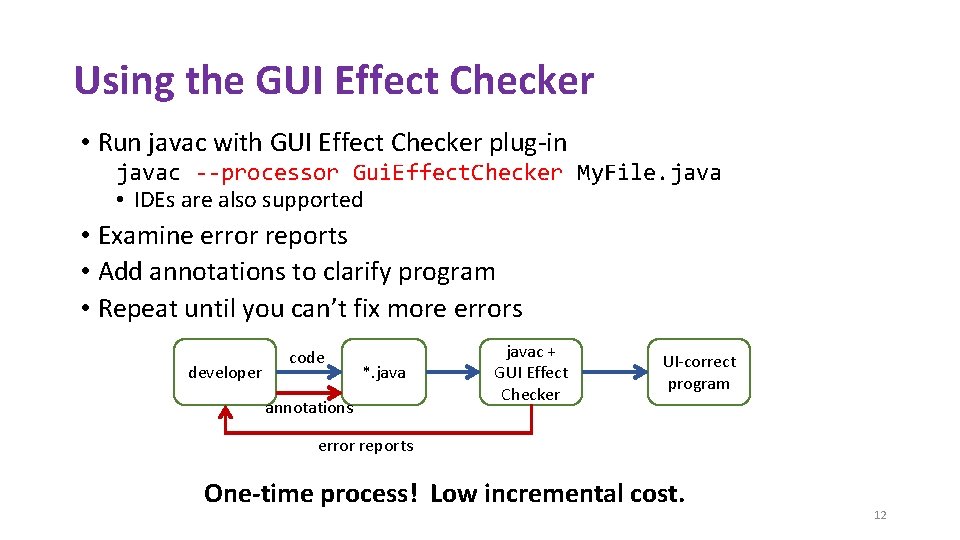
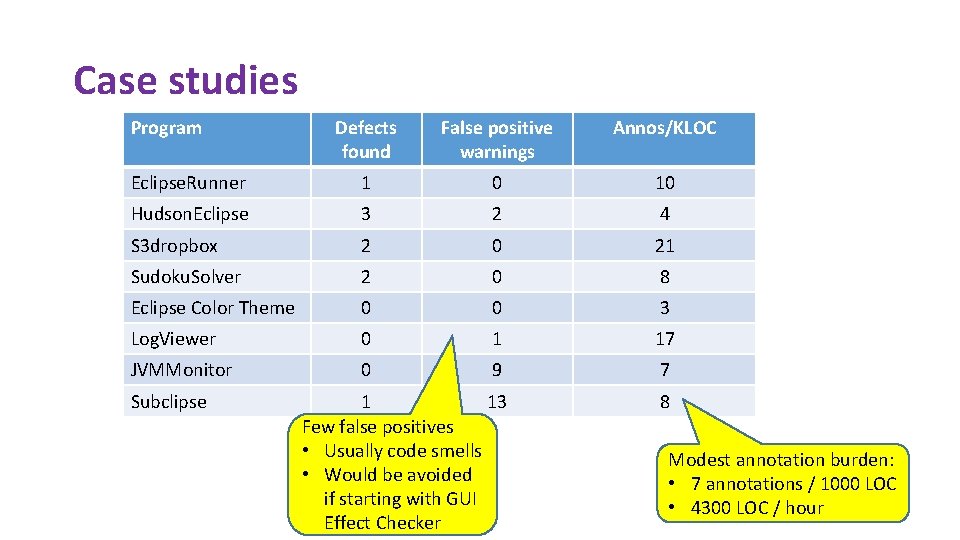
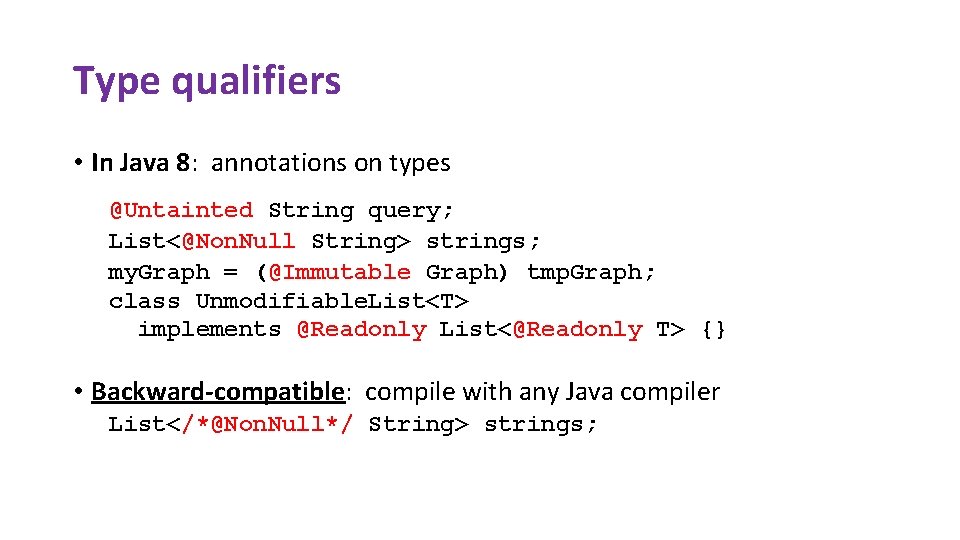
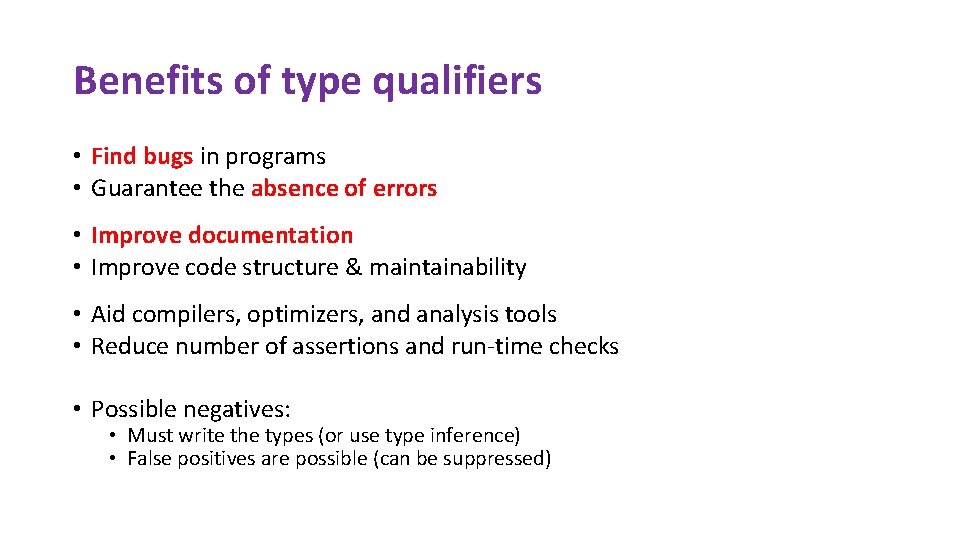
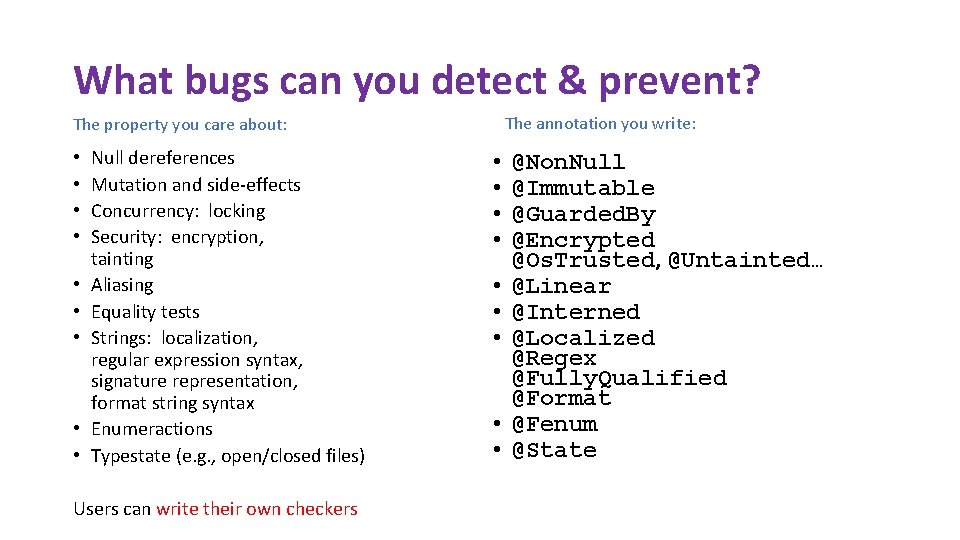
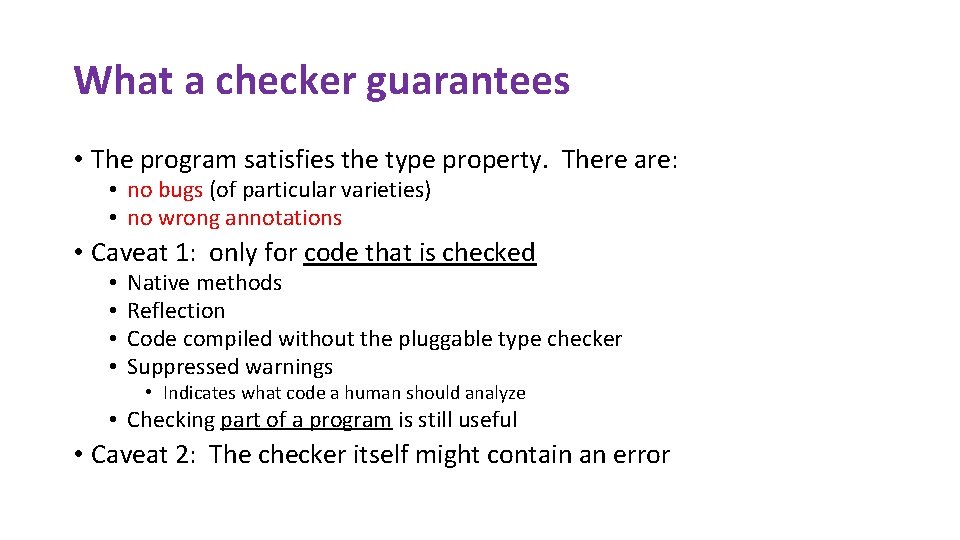
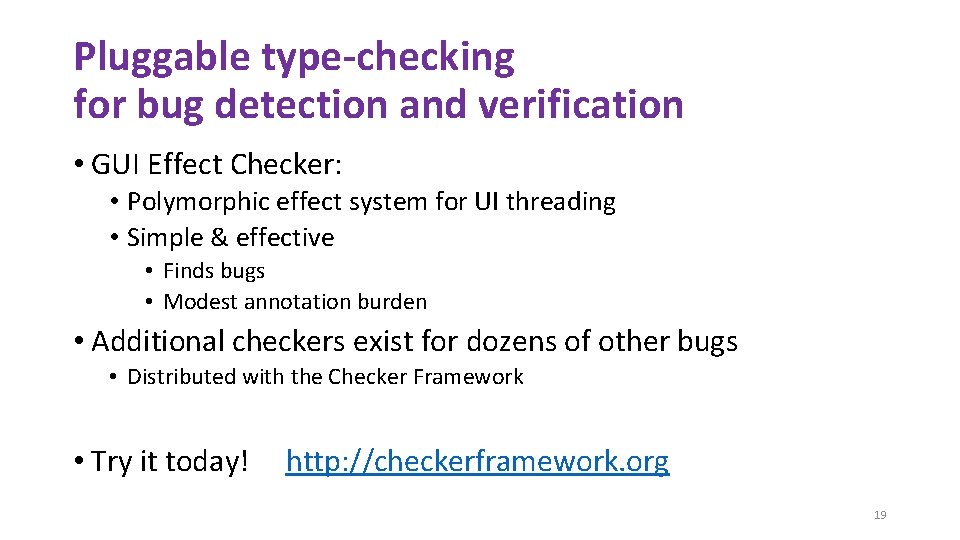
- Slides: 18
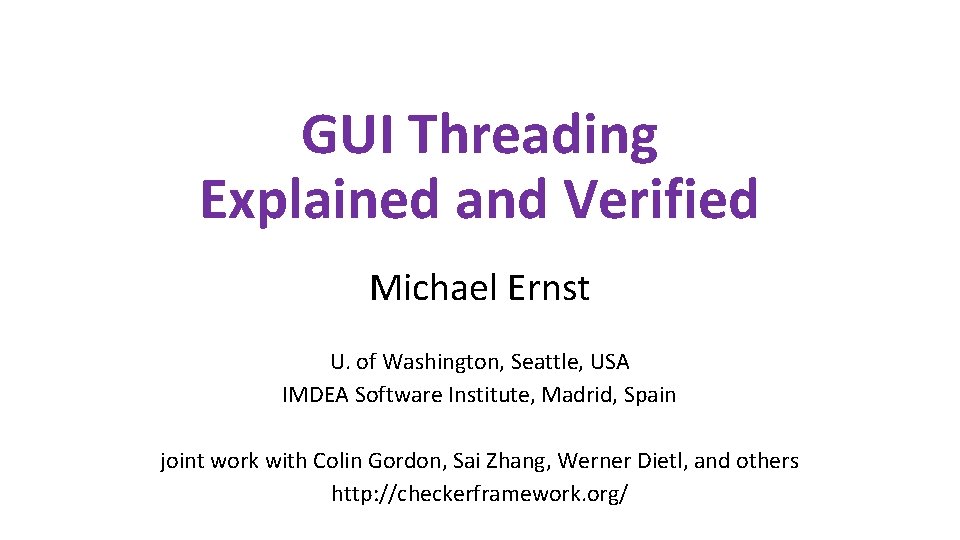
GUI Threading Explained and Verified Michael Ernst U. of Washington, Seattle, USA IMDEA Software Institute, Madrid, Spain joint work with Colin Gordon, Sai Zhang, Werner Dietl, and others http: //checkerframework. org/
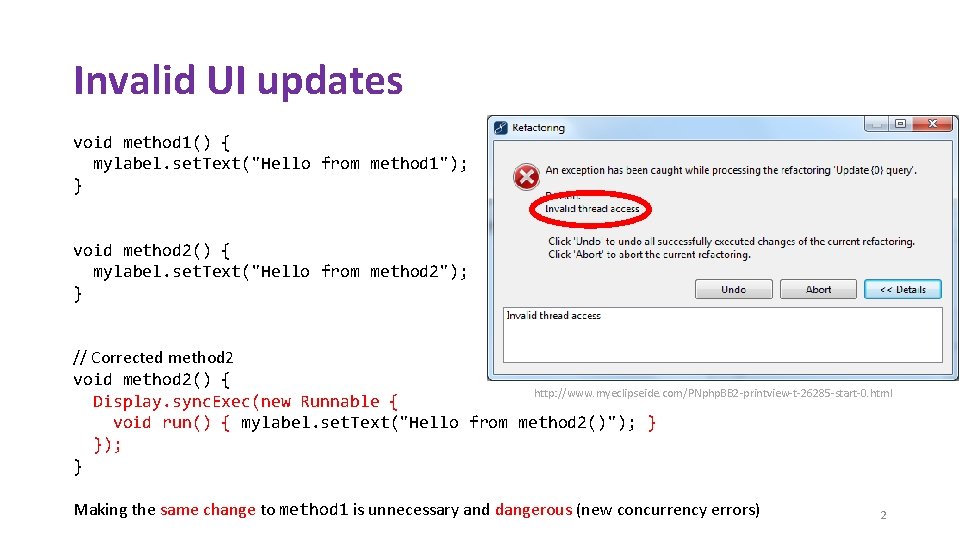
Invalid UI updates void method 1() { mylabel. set. Text("Hello from method 1"); } Hello from method 1 void method 2() { mylabel. set. Text("Hello from method 2"); } // Corrected method 2 void method 2() { http: //www. myeclipseide. com/PNphp. BB 2 -printview-t-26285 -start-0. html Display. sync. Exec(new Runnable { void run() { mylabel. set. Text("Hello from method 2()"); } }); } Making the same change to method 1 is unnecessary and dangerous (new concurrency errors) 2
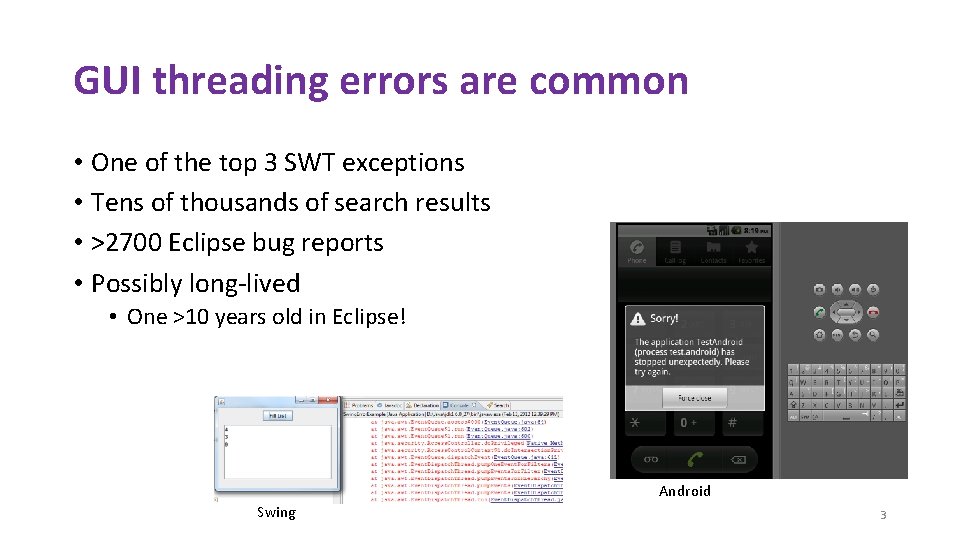
GUI threading errors are common • One of the top 3 SWT exceptions • Tens of thousands of search results • >2700 Eclipse bug reports • Possibly long-lived • One >10 years old in Eclipse! Android Swing 3
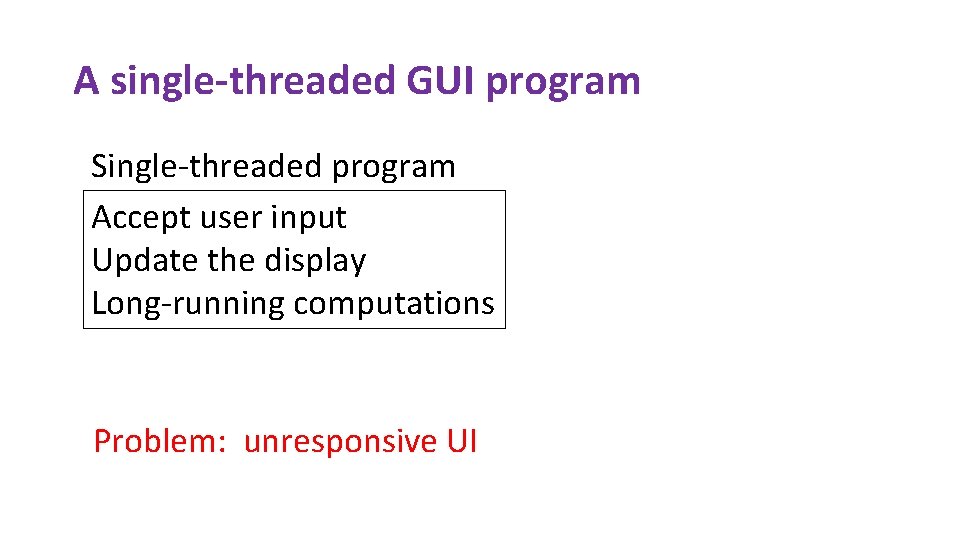
A single-threaded GUI program Single-threaded program Accept user input Update the display Long-running computations Problem: unresponsive UI
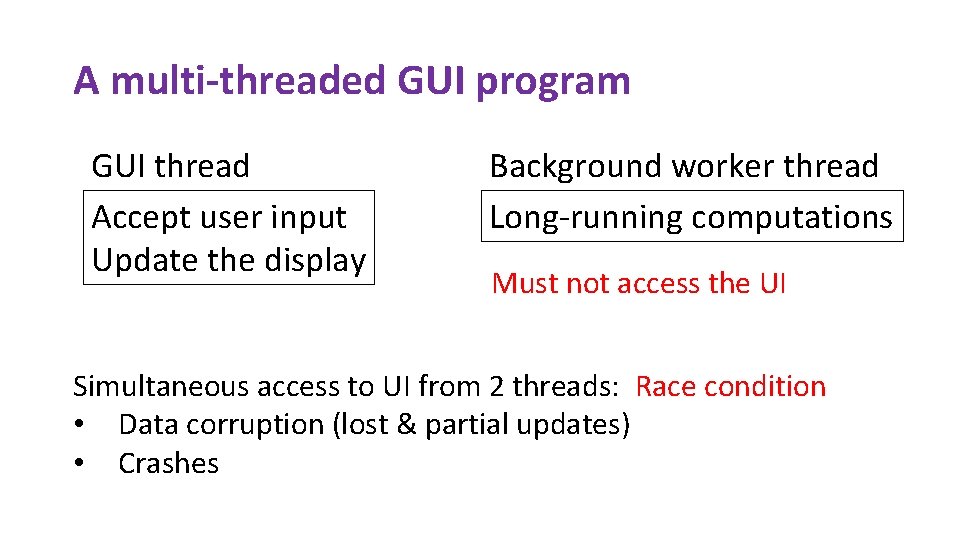
A multi-threaded GUI program GUI thread Accept user input Update the display Background worker thread Long-running computations Must not access the UI Simultaneous access to UI from 2 threads: Race condition • Data corruption (lost & partial updates) • Crashes
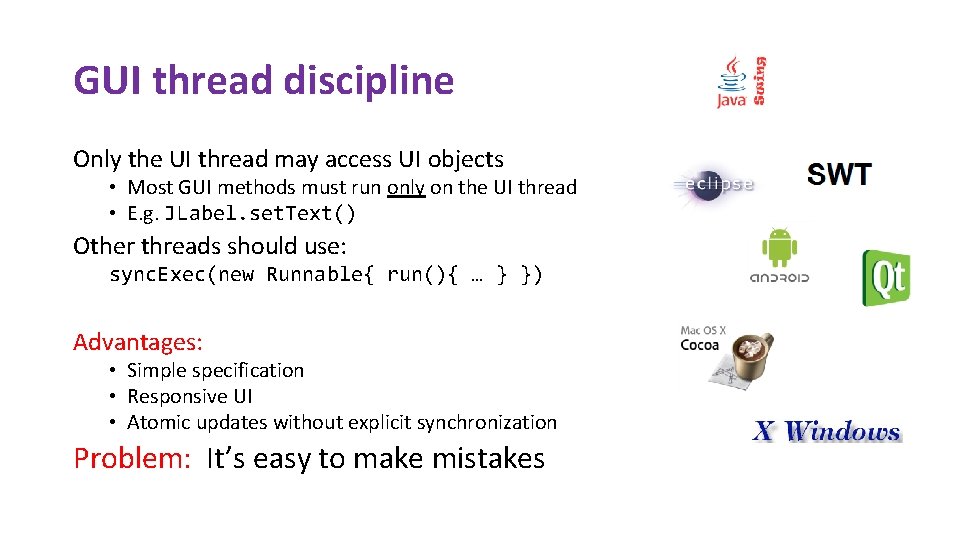
GUI thread discipline Only the UI thread may access UI objects • Most GUI methods must run only on the UI thread • E. g. JLabel. set. Text() Other threads should use: sync. Exec(new Runnable{ run(){ … } }) Advantages: • Simple specification • Responsive UI • Atomic updates without explicit synchronization Problem: It’s easy to make mistakes
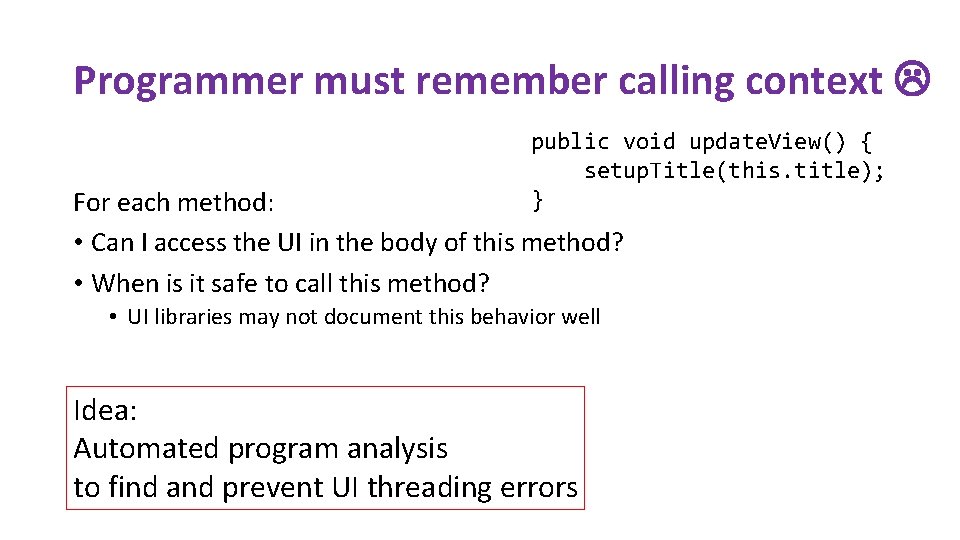
Programmer must remember calling context public void update. View() { setup. Title(this. title); } For each method: • Can I access the UI in the body of this method? • When is it safe to call this method? • UI libraries may not document this behavior well Idea: Automated program analysis to find and prevent UI threading errors
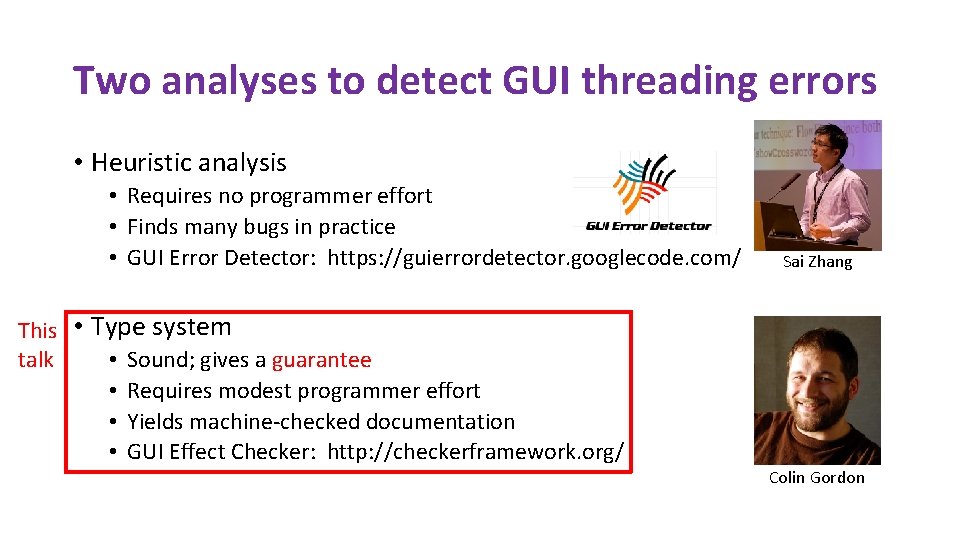
Two analyses to detect GUI threading errors • Heuristic analysis • Requires no programmer effort • Finds many bugs in practice • GUI Error Detector: https: //guierrordetector. googlecode. com/ Sai Zhang This • Type system talk • Sound; gives a guarantee • Requires modest programmer effort • Yields machine-checked documentation • GUI Effect Checker: http: //checkerframework. org/ Colin Gordon
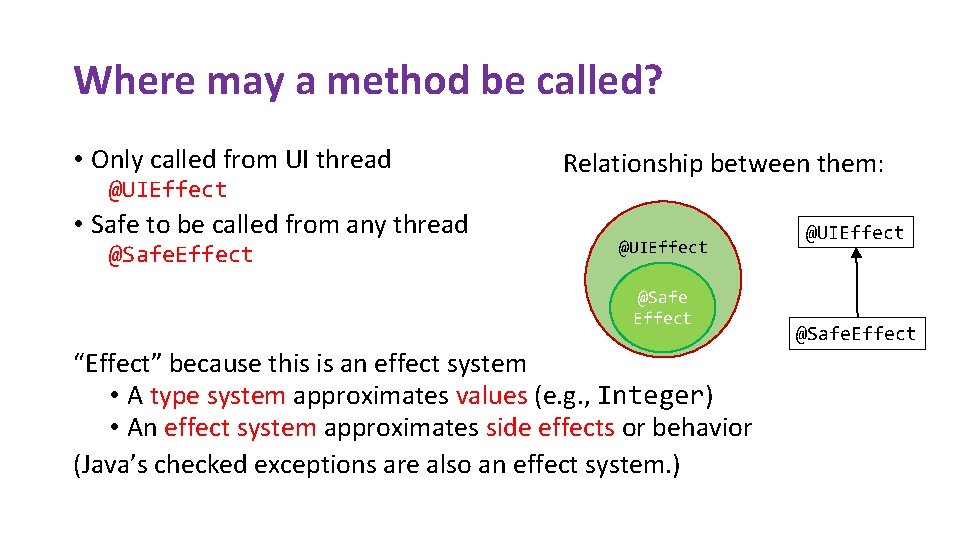
Where may a method be called? • Only called from UI thread @UIEffect • Safe to be called from any thread @Safe. Effect Relationship between them: @UIEffect @Safe Effect “Effect” because this is an effect system • A type system approximates values (e. g. , Integer) • An effect system approximates side effects or behavior (Java’s checked exceptions are also an effect system. ) @UIEffect @Safe. Effect
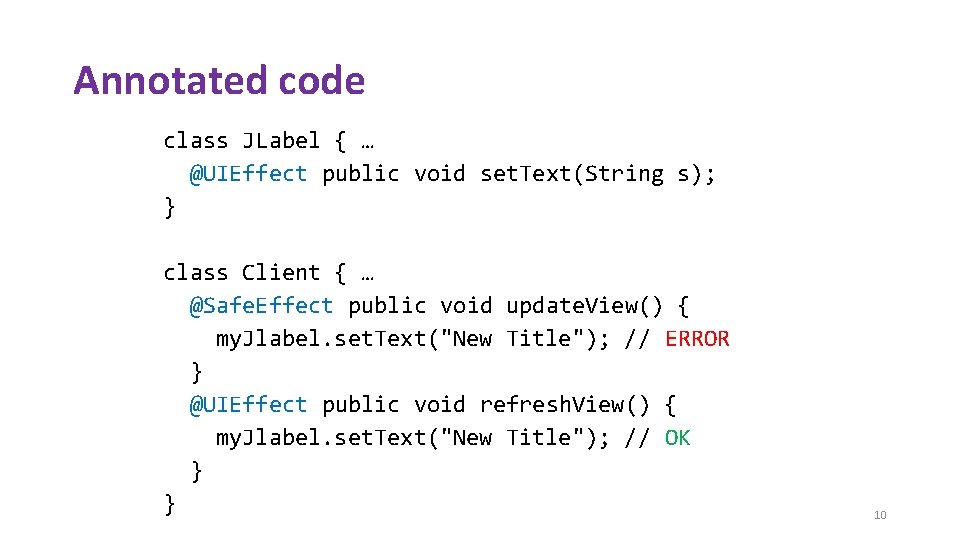
Annotated code class JLabel { … @UIEffect public void set. Text(String s); } class Client { … @Safe. Effect public void update. View() { my. Jlabel. set. Text("New Title"); // ERROR } @UIEffect public void refresh. View() { my. Jlabel. set. Text("New Title"); // OK } } 10
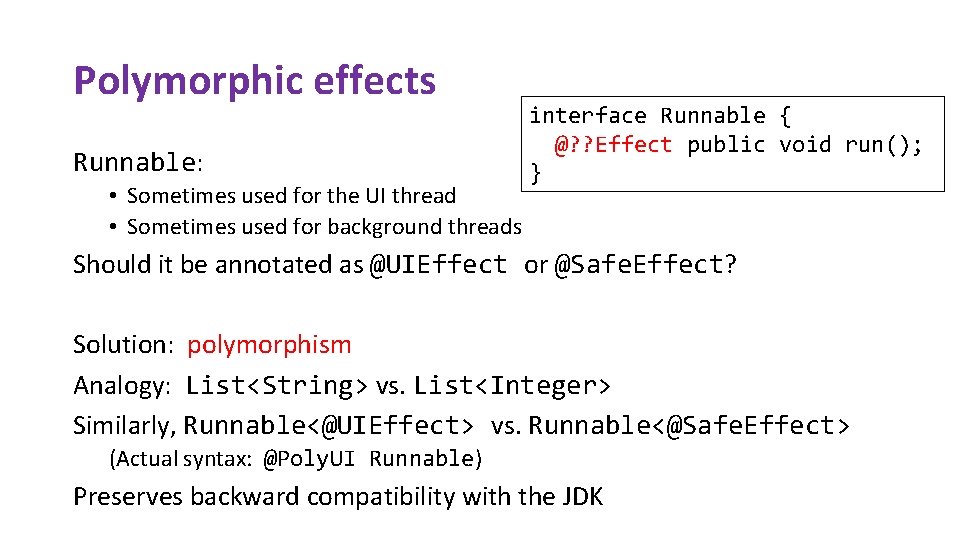
Polymorphic effects Runnable: • Sometimes used for the UI thread • Sometimes used for background threads interface Runnable { @? ? Effect public void run(); } Should it be annotated as @UIEffect or @Safe. Effect? Solution: polymorphism Analogy: List<String> vs. List<Integer> Similarly, Runnable<@UIEffect> vs. Runnable<@Safe. Effect> (Actual syntax: @Poly. UI Runnable) Preserves backward compatibility with the JDK
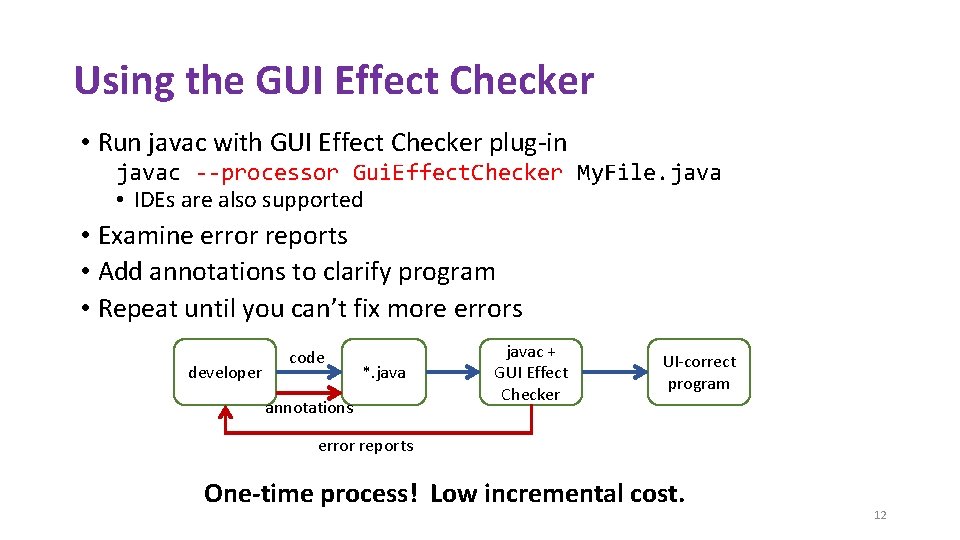
Using the GUI Effect Checker • Run javac with GUI Effect Checker plug-in javac --processor Gui. Effect. Checker My. File. java • IDEs are also supported • Examine error reports • Add annotations to clarify program • Repeat until you can’t fix more errors developer code *. java annotations javac + GUI Effect Checker UI-correct program error reports One-time process! Low incremental cost. 12
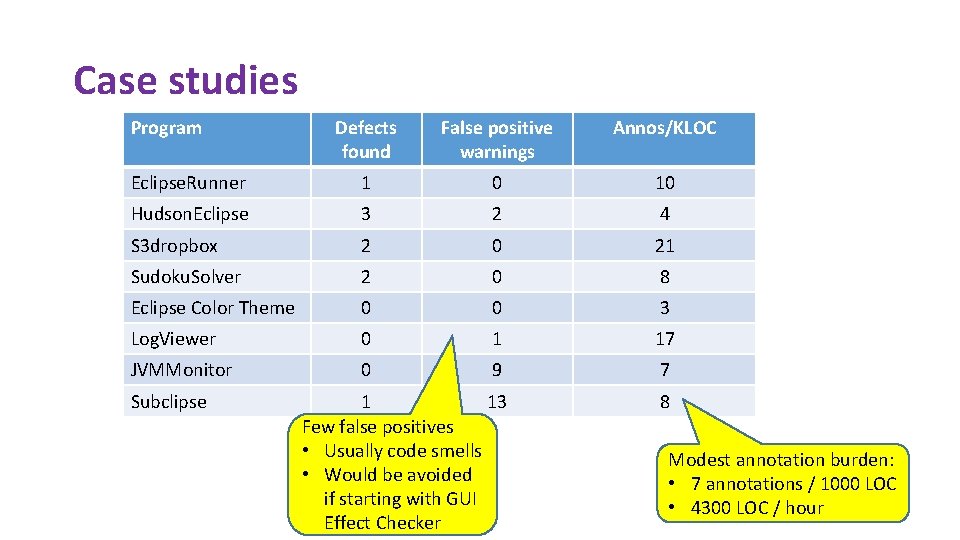
Case studies Program Defects found False positive warnings Annos/KLOC Eclipse. Runner 1 0 10 Hudson. Eclipse 3 2 4 S 3 dropbox 2 0 21 Sudoku. Solver 2 0 8 Eclipse Color Theme 0 0 3 Log. Viewer 0 1 17 JVMMonitor 0 9 7 1 13 Few false positives • Usually code smells • Would be avoided if starting with GUI Effect Checker 8 Subclipse Modest annotation burden: • 7 annotations / 1000 LOC • 4300 LOC / hour
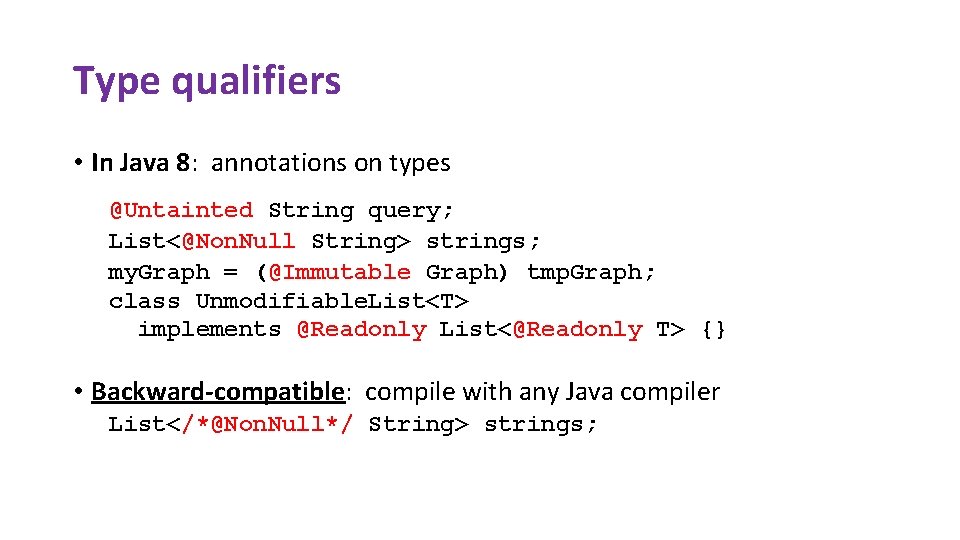
Type qualifiers • In Java 8: annotations on types @Untainted String query; List<@Non. Null String> strings; my. Graph = (@Immutable Graph) tmp. Graph; class Unmodifiable. List<T> implements @Readonly List<@Readonly T> {} • Backward-compatible: compile with any Java compiler List</*@Non. Null*/ String> strings;
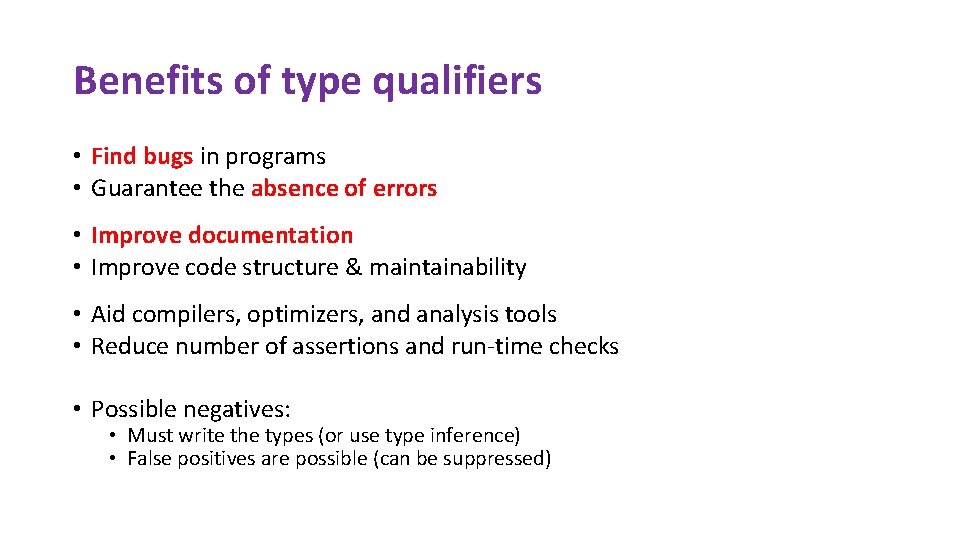
Benefits of type qualifiers • Find bugs in programs • Guarantee the absence of errors • Improve documentation • Improve code structure & maintainability • Aid compilers, optimizers, and analysis tools • Reduce number of assertions and run-time checks • Possible negatives: • Must write the types (or use type inference) • False positives are possible (can be suppressed)
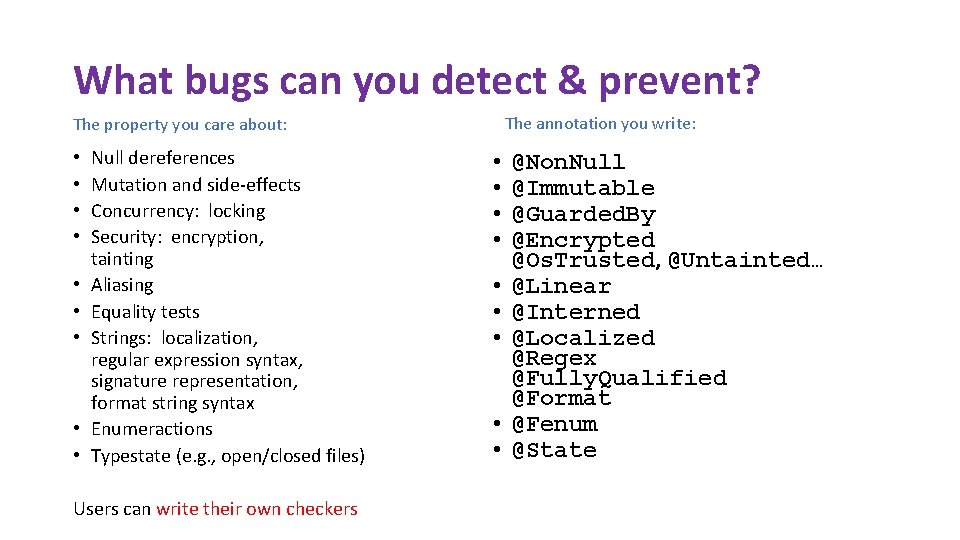
What bugs can you detect & prevent? The annotation you write: The property you care about: • • • Null dereferences Mutation and side-effects Concurrency: locking Security: encryption, tainting Aliasing Equality tests Strings: localization, regular expression syntax, signature representation, format string syntax Enumeractions Typestate (e. g. , open/closed files) Users can write their own checkers • • • @Non. Null @Immutable @Guarded. By @Encrypted @Os. Trusted, @Untainted… @Linear @Interned @Localized @Regex @Fully. Qualified @Format @Fenum @State
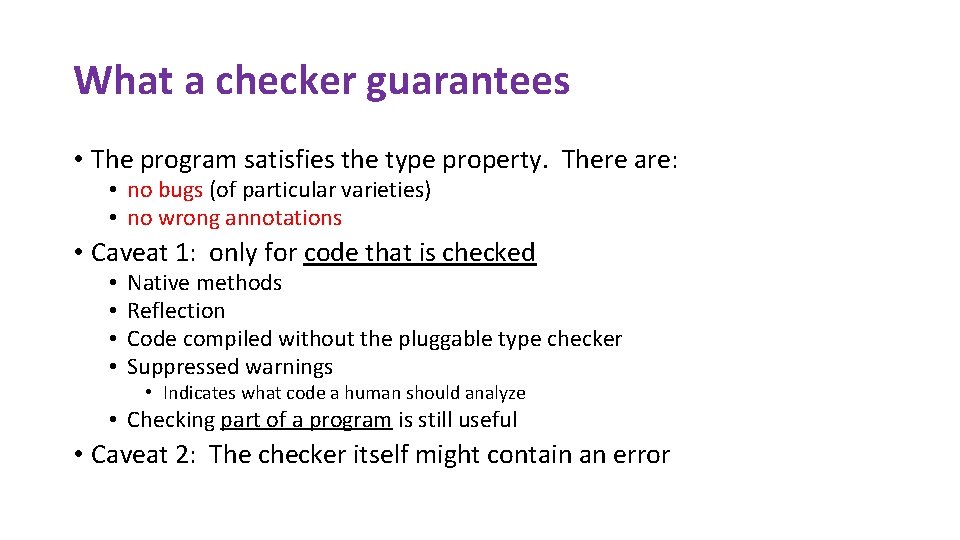
What a checker guarantees • The program satisfies the type property. There are: • no bugs (of particular varieties) • no wrong annotations • Caveat 1: only for code that is checked • • Native methods Reflection Code compiled without the pluggable type checker Suppressed warnings • Indicates what code a human should analyze • Checking part of a program is still useful • Caveat 2: The checker itself might contain an error
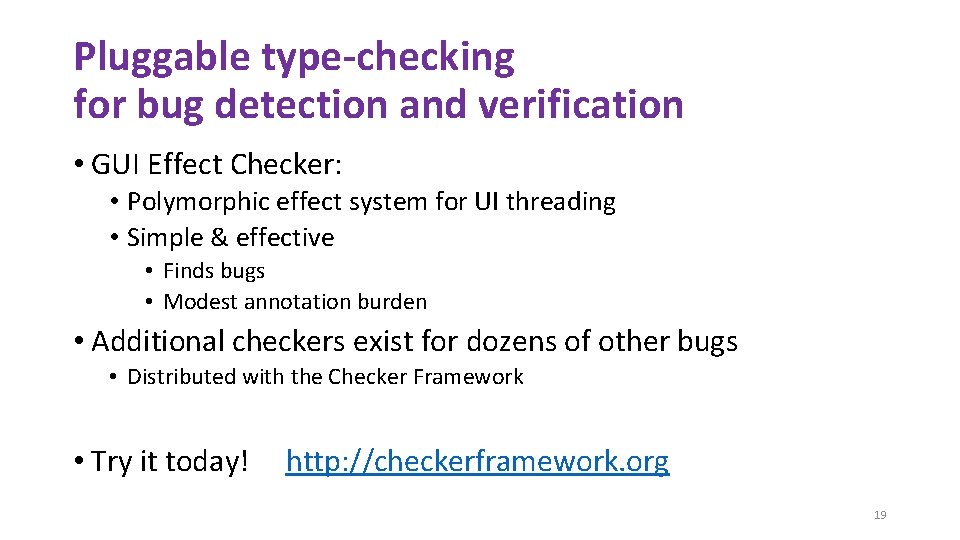
Pluggable type-checking for bug detection and verification • GUI Effect Checker: • Polymorphic effect system for UI threading • Simple & effective • Finds bugs • Modest annotation burden • Additional checkers exist for dozens of other bugs • Distributed with the Checker Framework • Try it today! http: //checkerframework. org 19
Ge gi gue gui güe güi
Michael d. ernst
Verified credentials uthsc
Sonitrol chicagoland west
What is stock verification
Drive wheel sewing machine
List of cutting tools in mechanical workshop
Threading building blocks
Hyper threading
Unreal engine multithreading
Interlocked pipeline
Com threading model
Protein threading
Hyper threading
Gore machine
Hyper threading
Hyper threading
Max ernst forest and dove
Ernst and young client portal