GUI Components Structure Container Class Structure Component Class
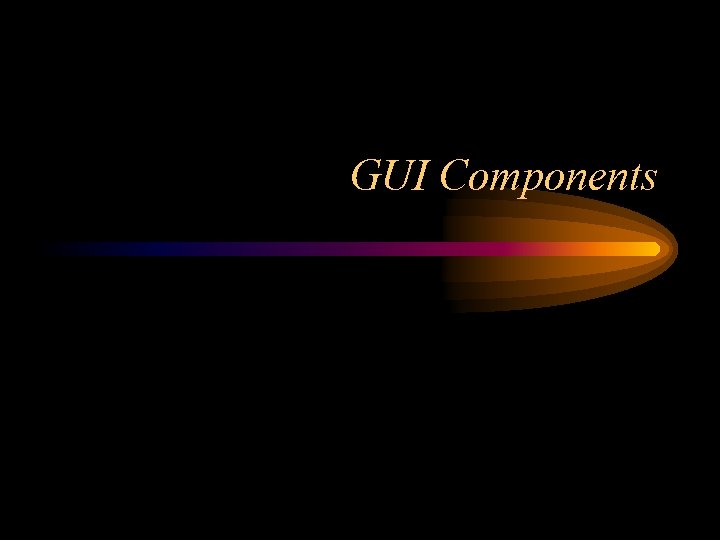
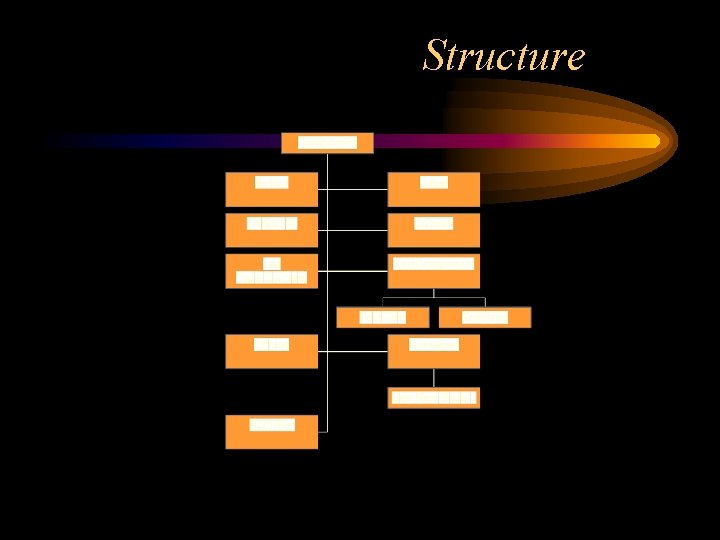
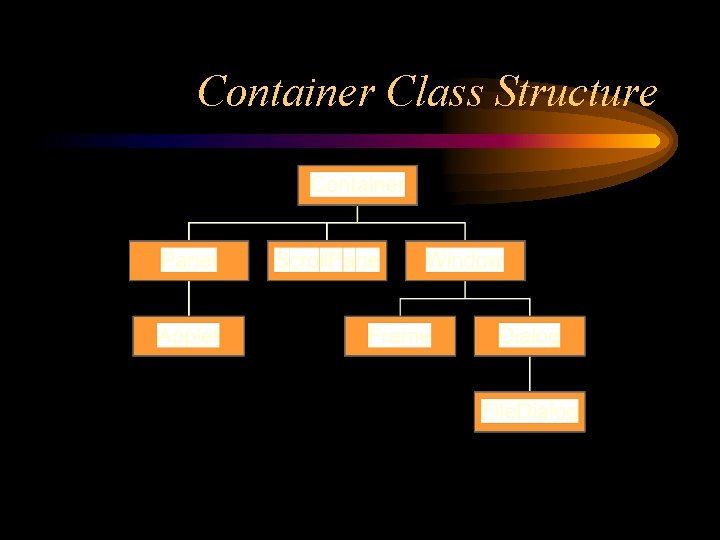
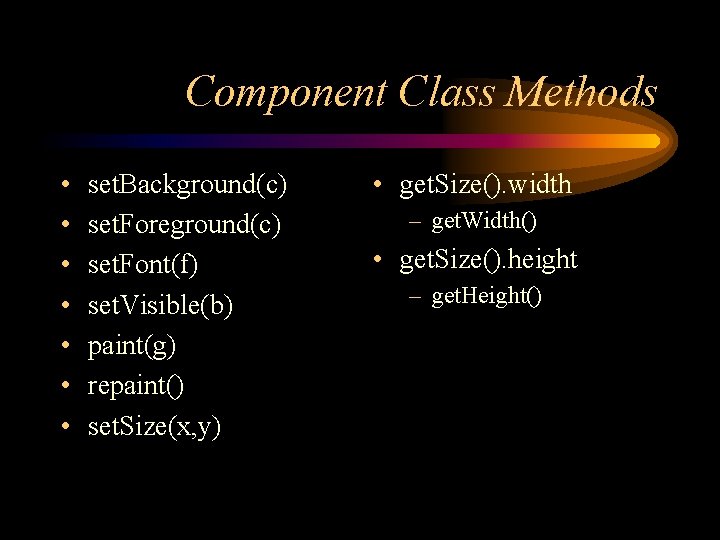
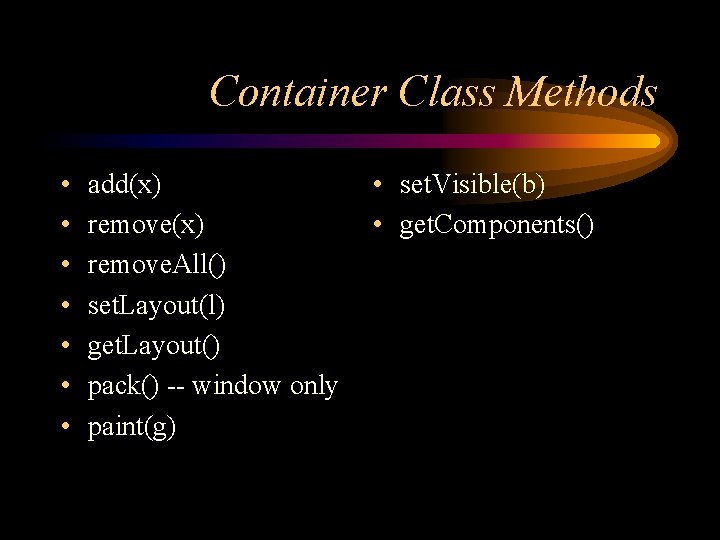
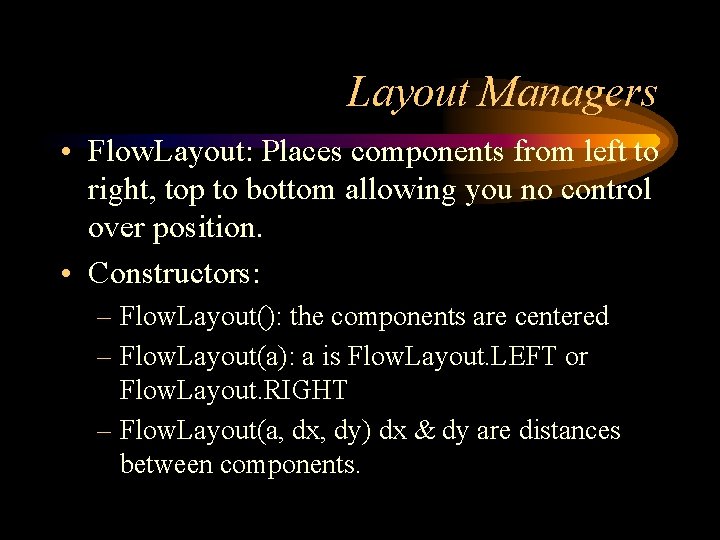
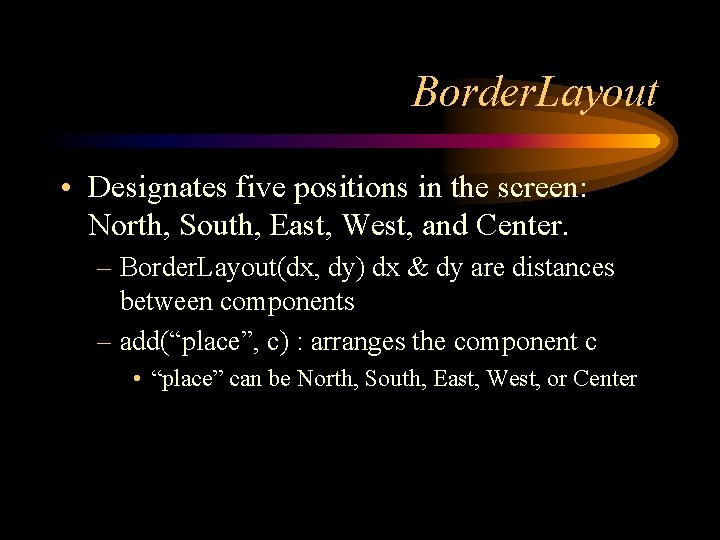
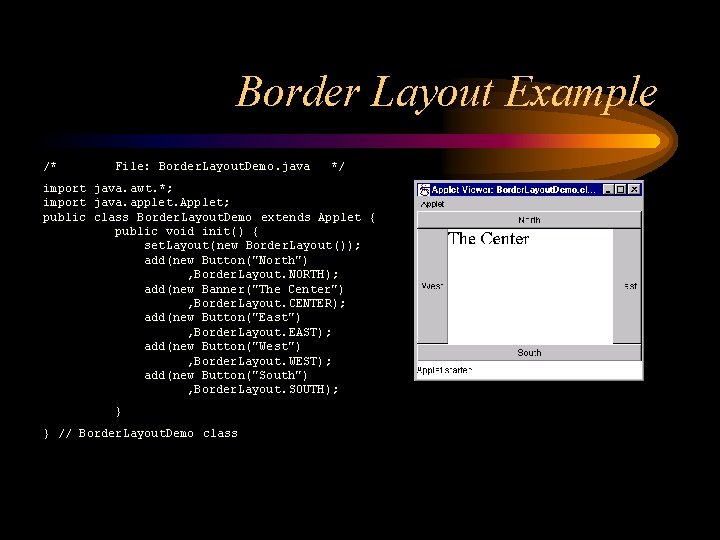
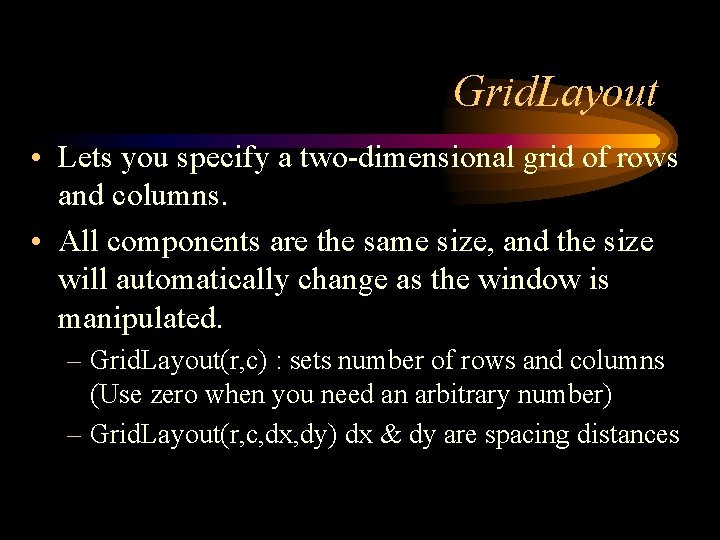
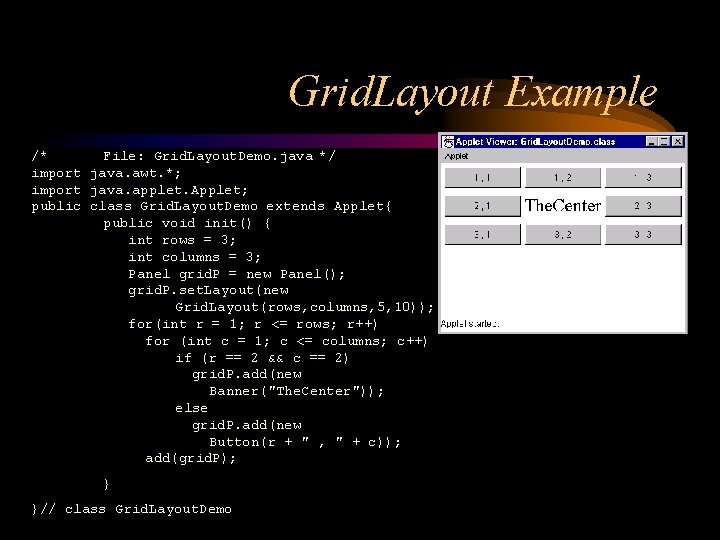
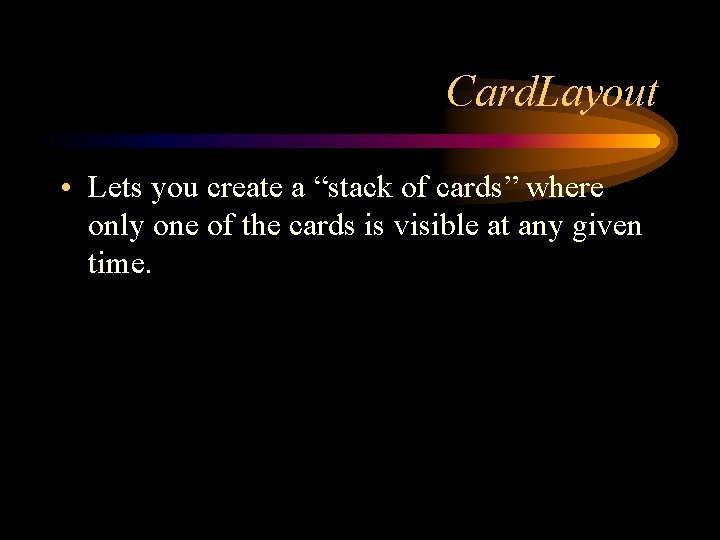
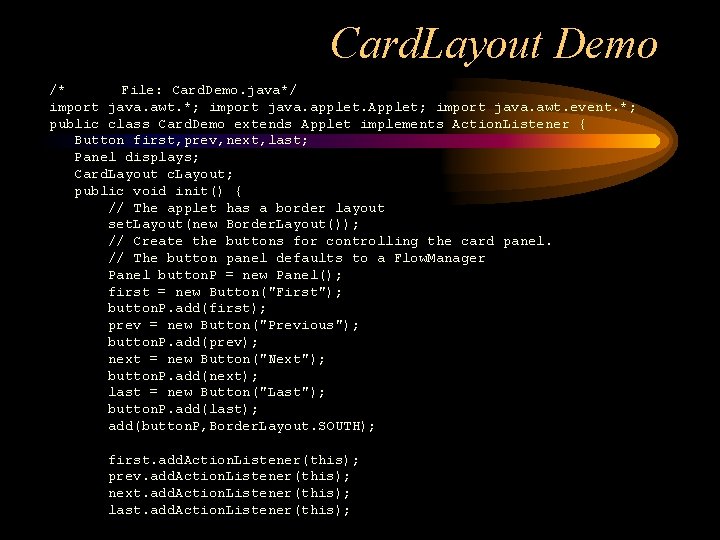
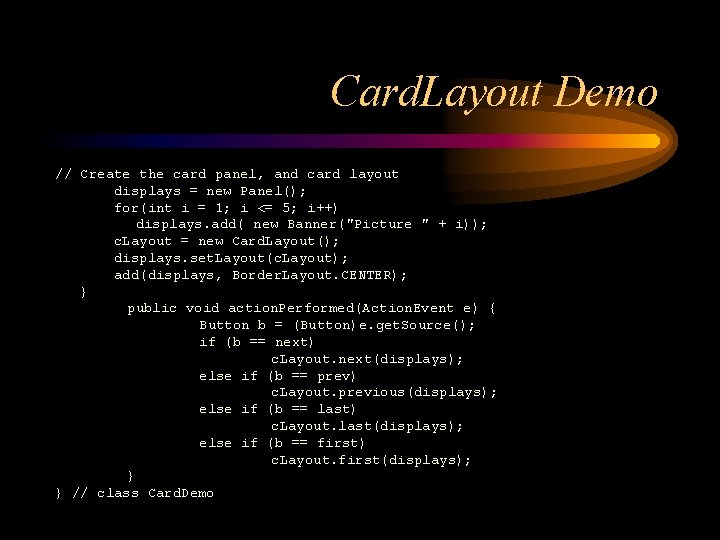
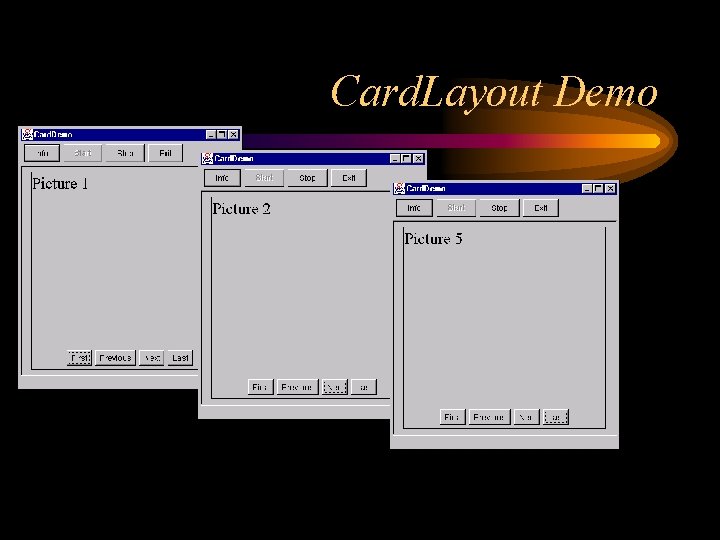
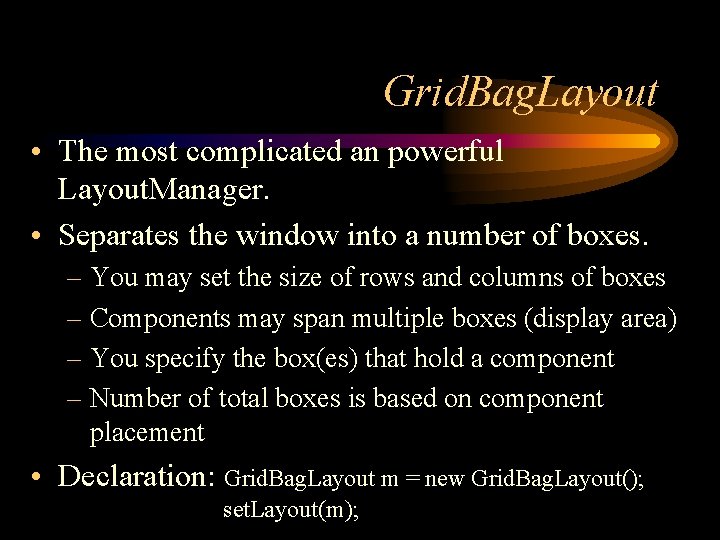
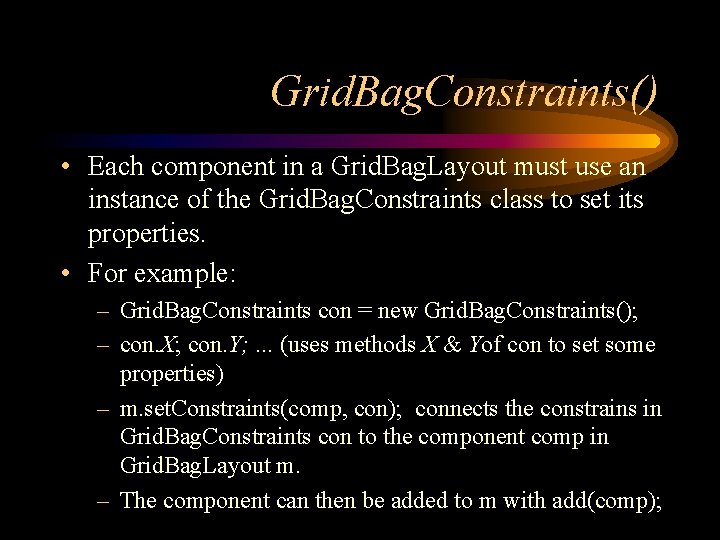
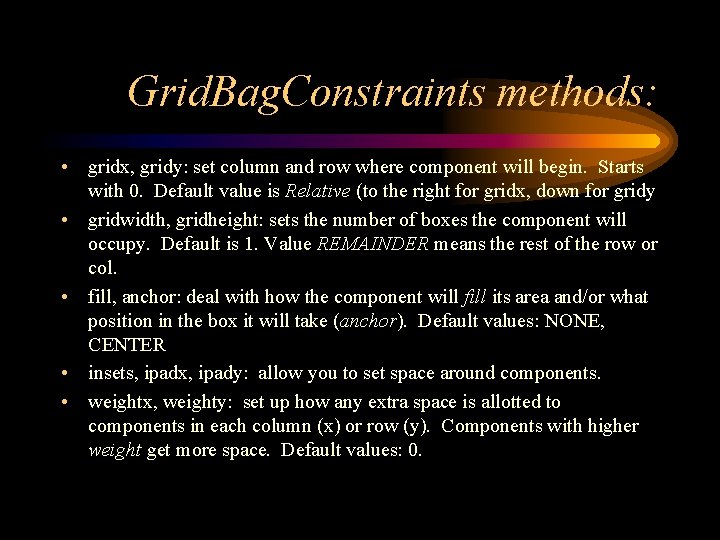
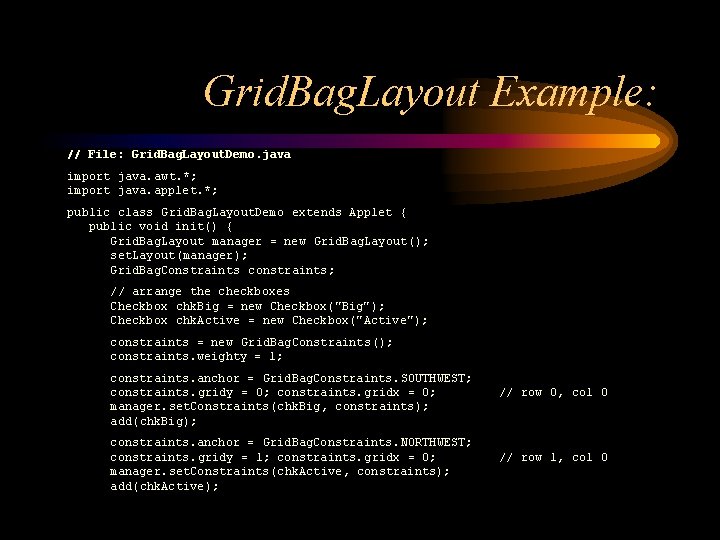
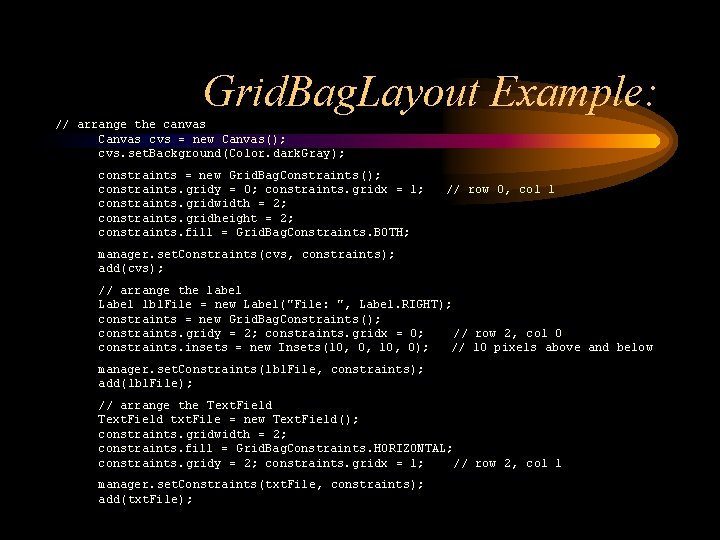
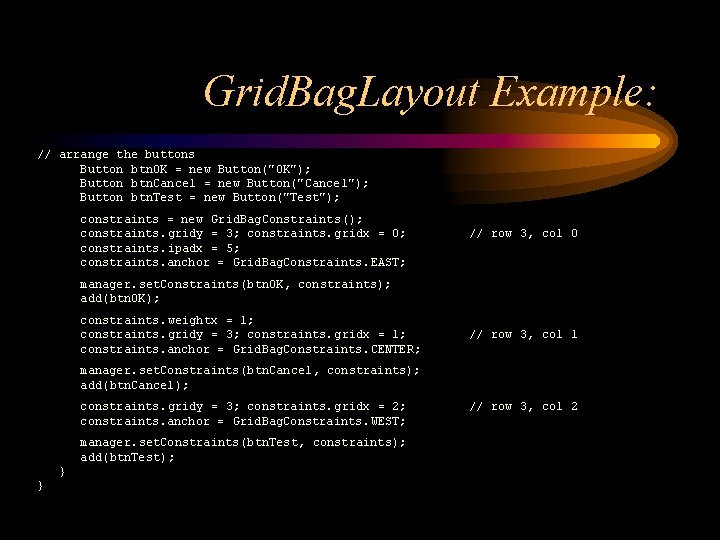
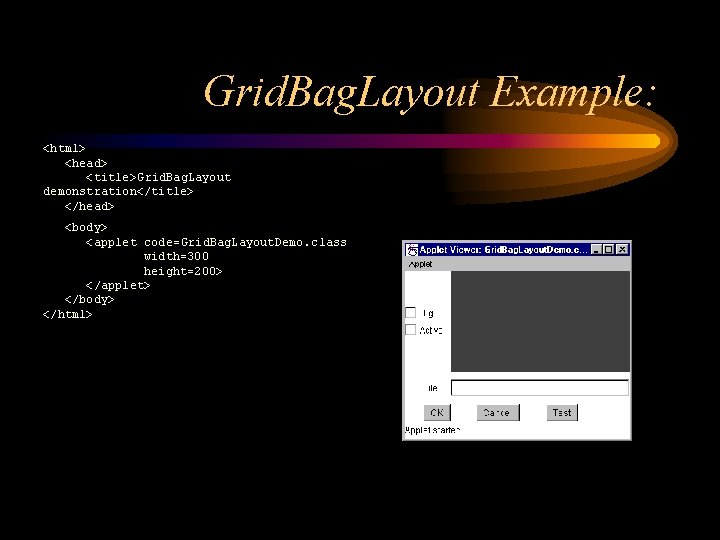
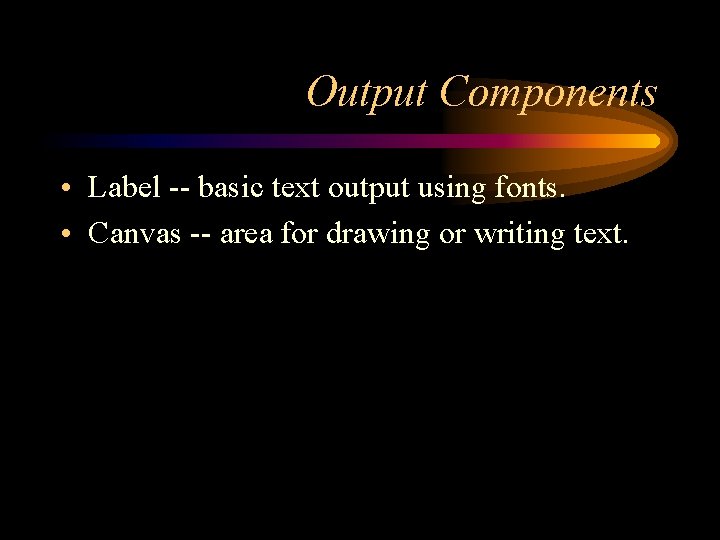
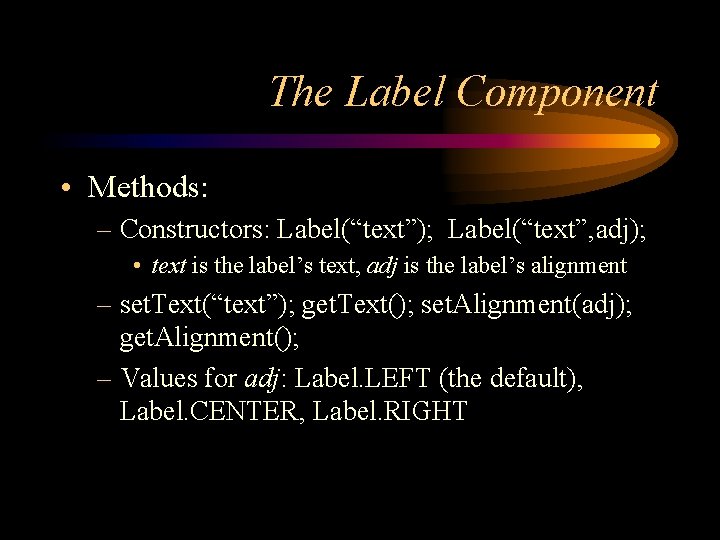
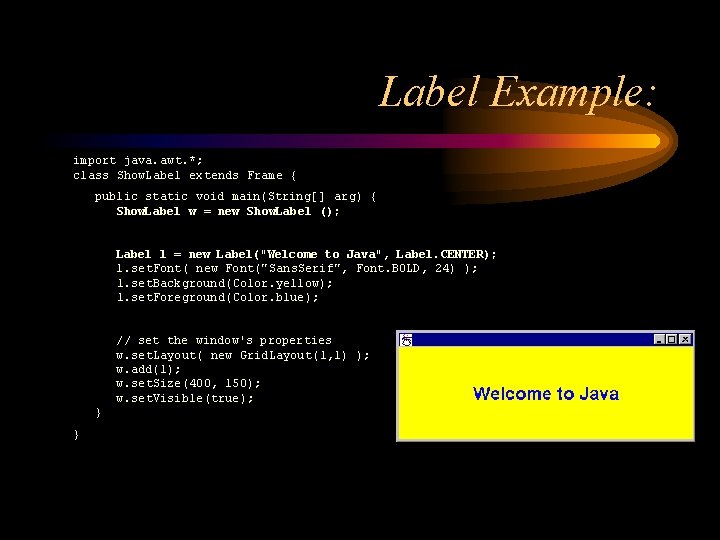
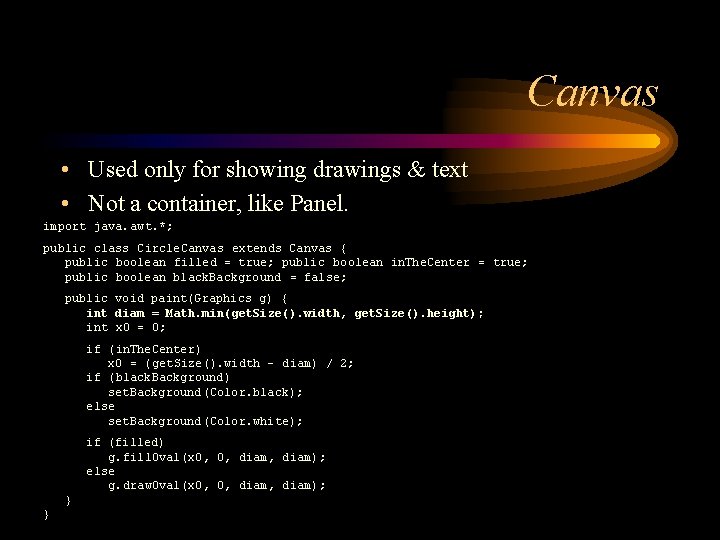
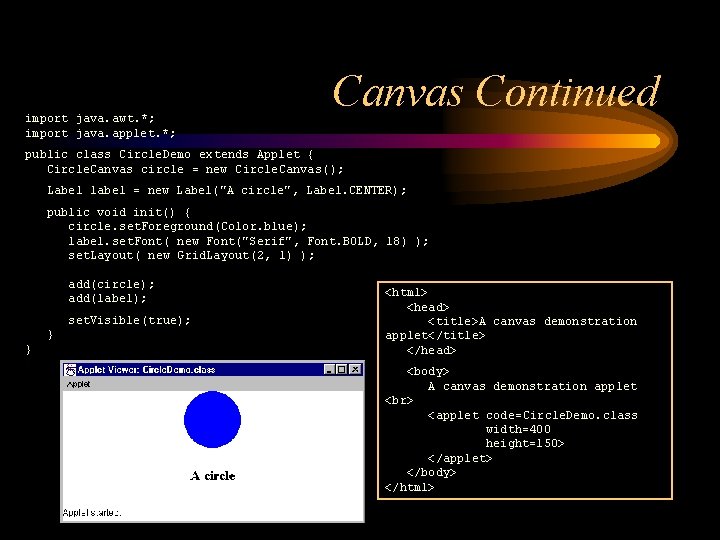
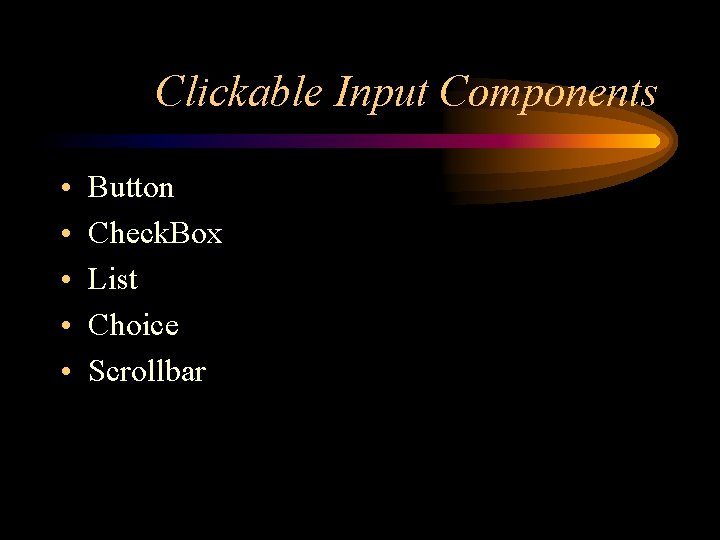
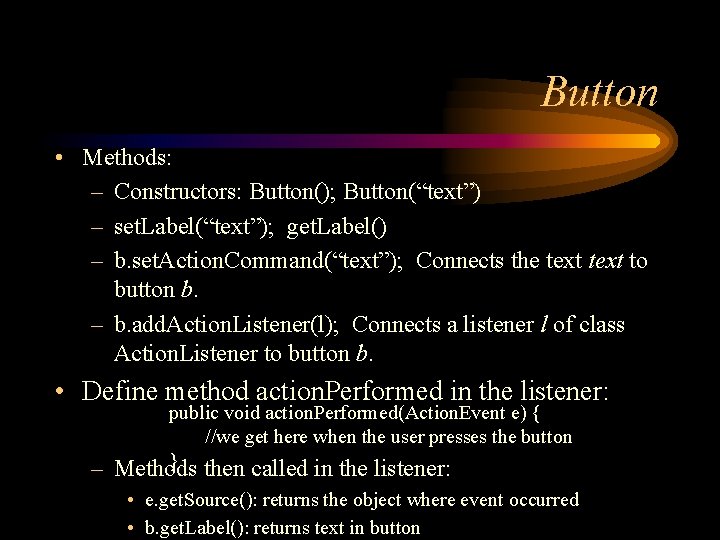
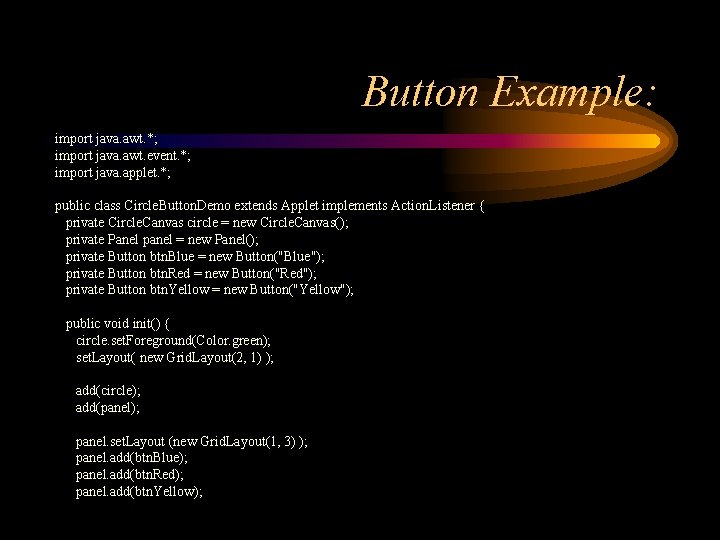
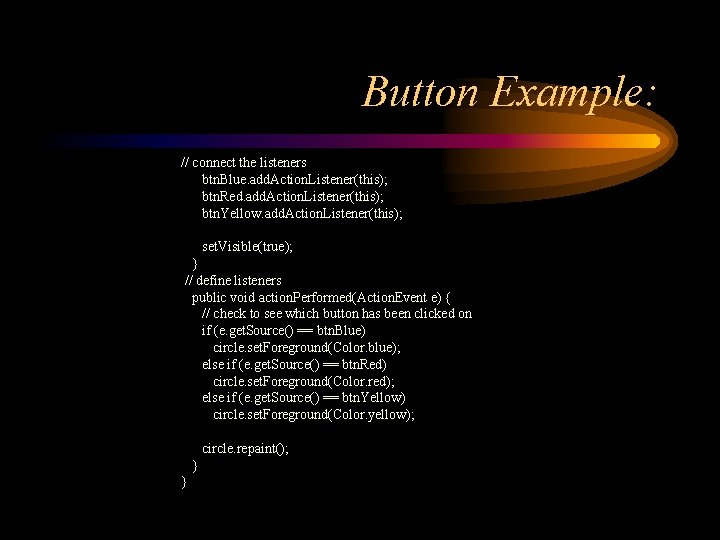
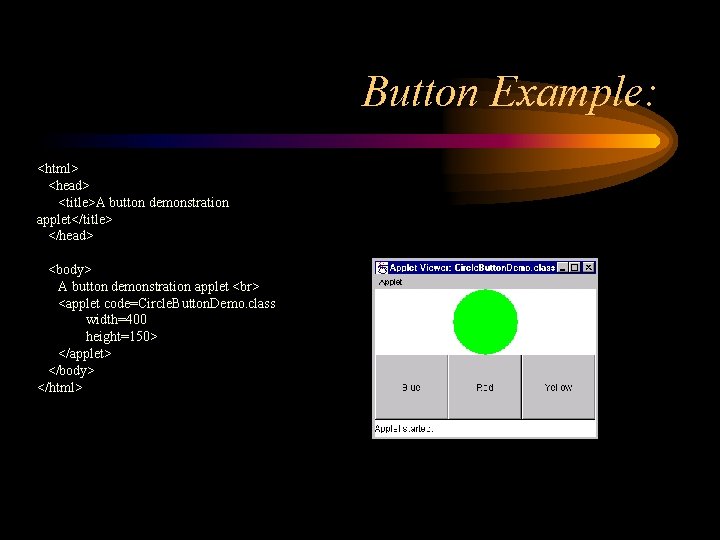
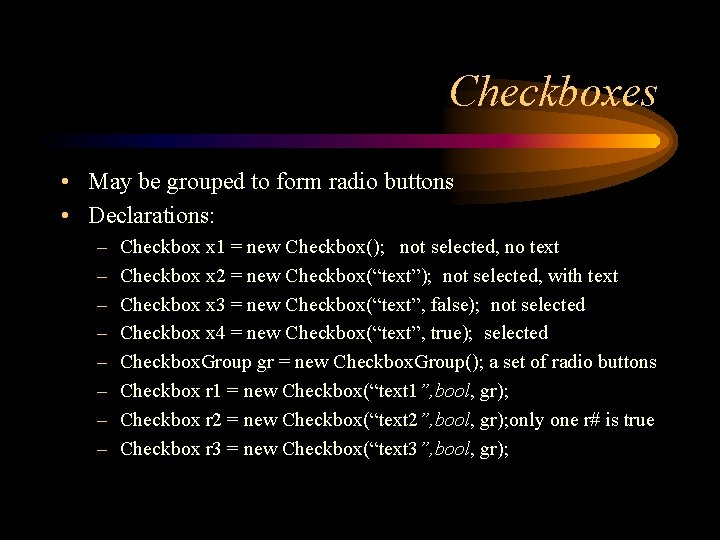
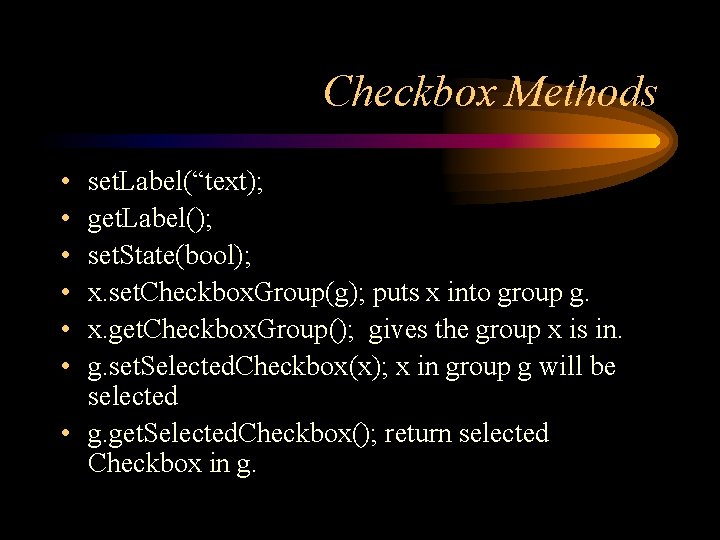
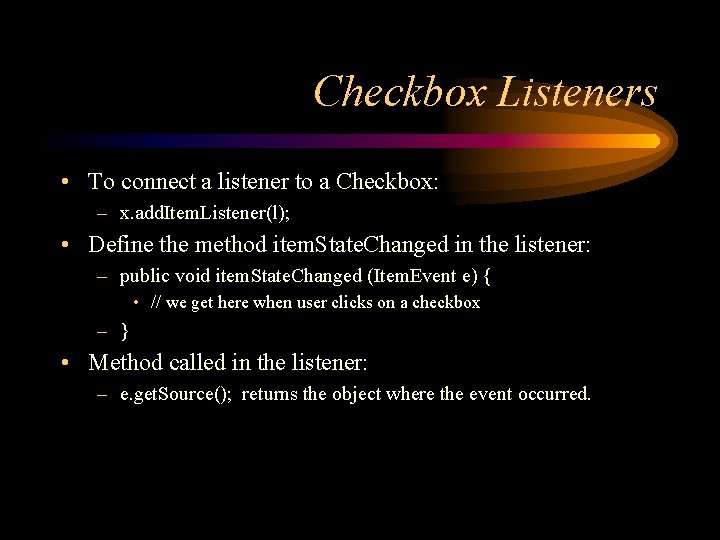
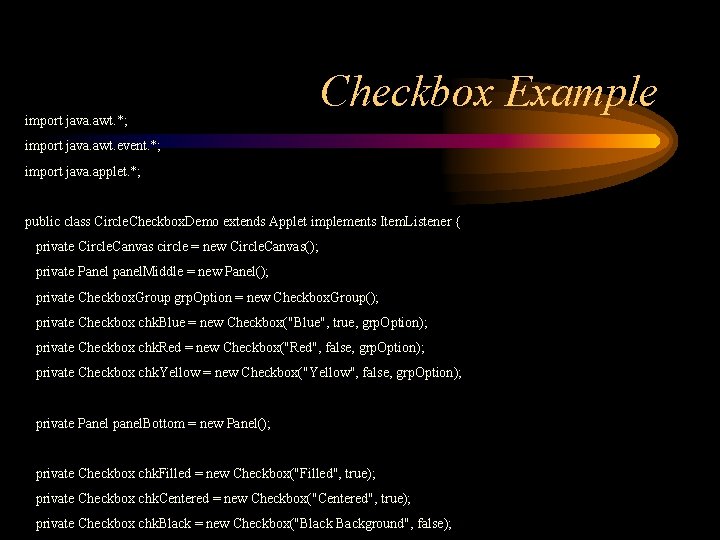
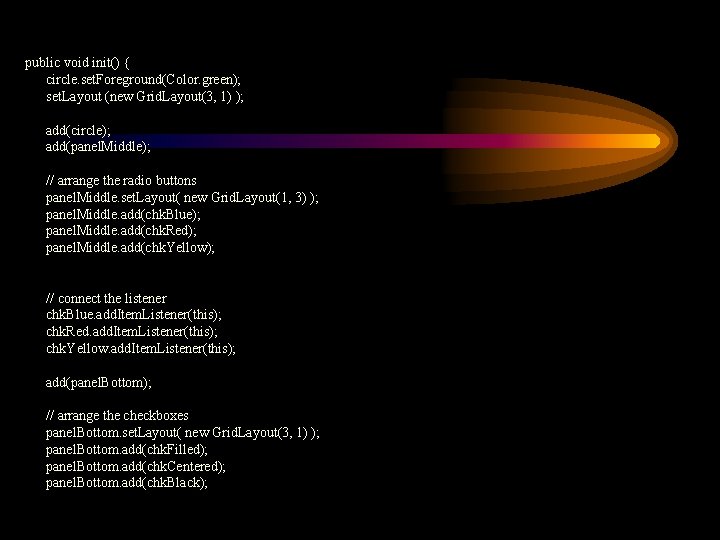
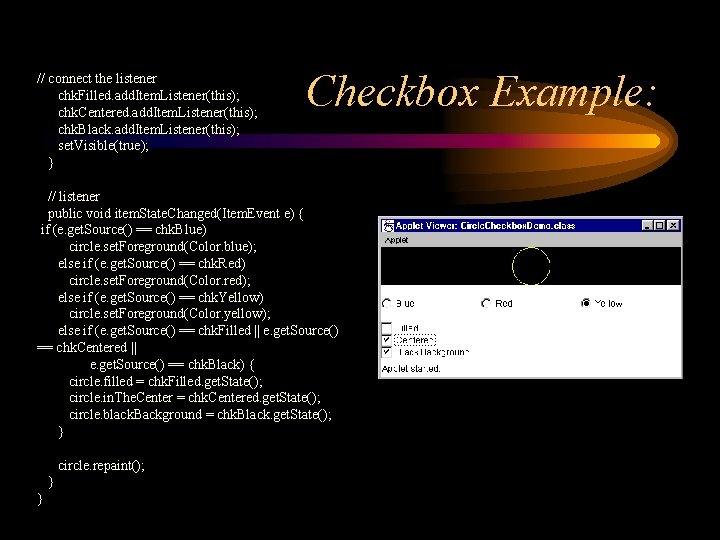
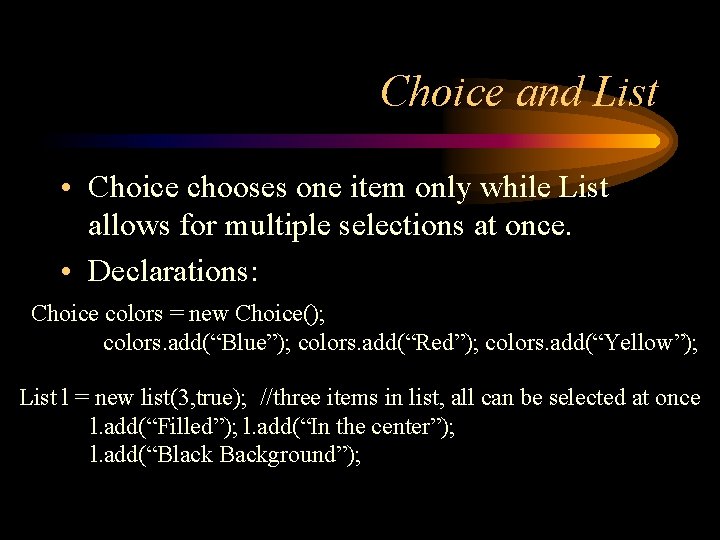
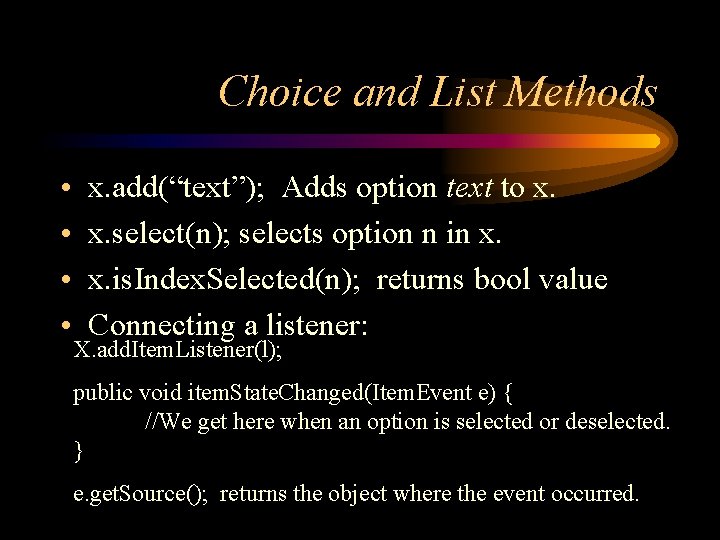
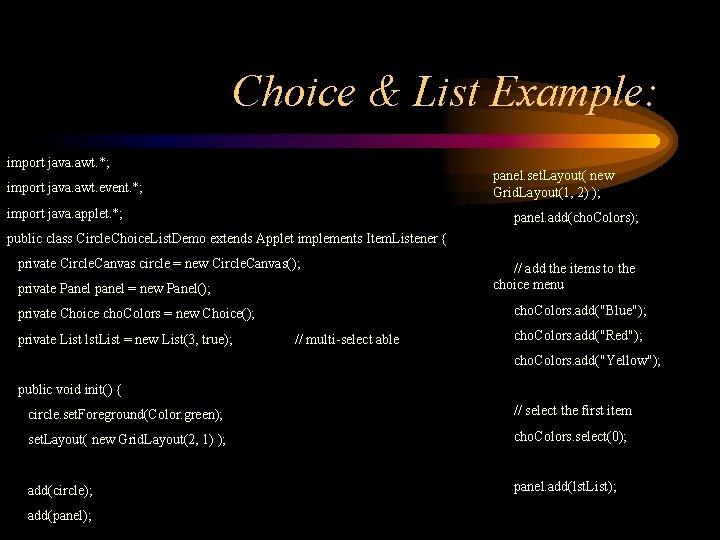
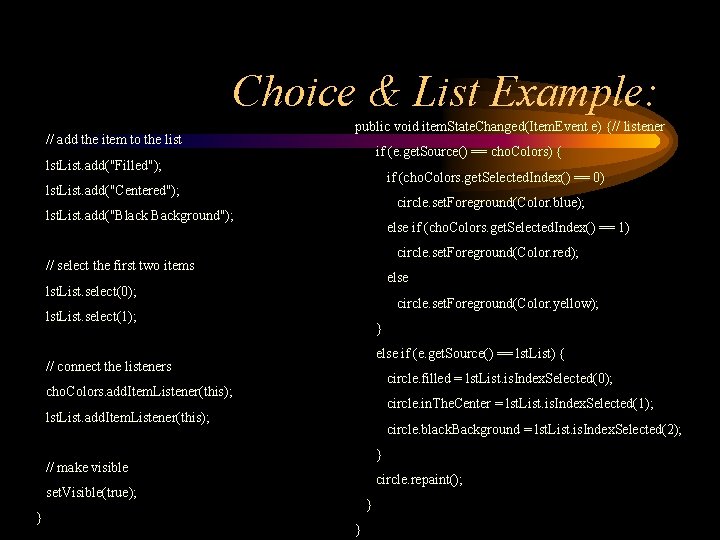
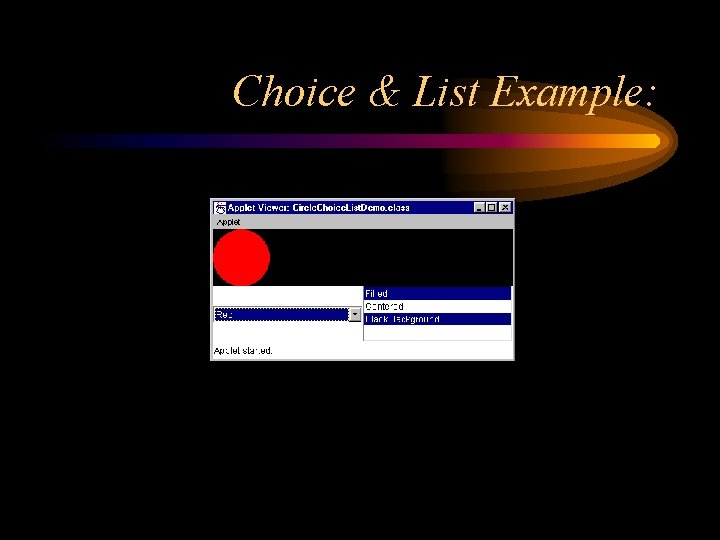
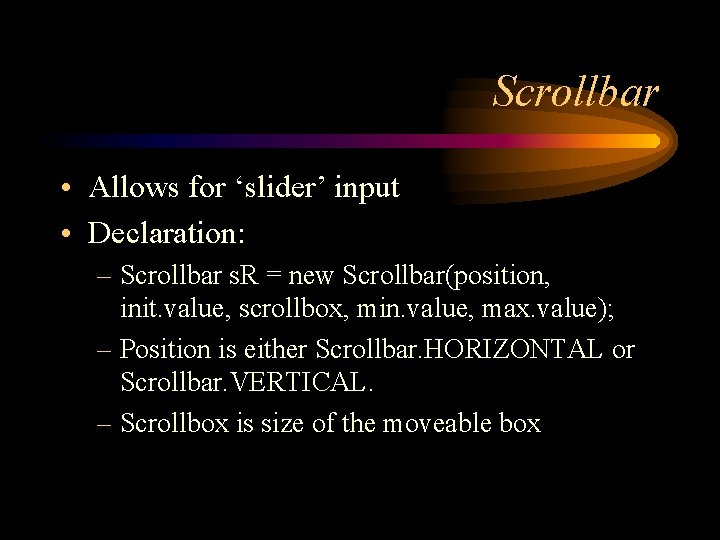
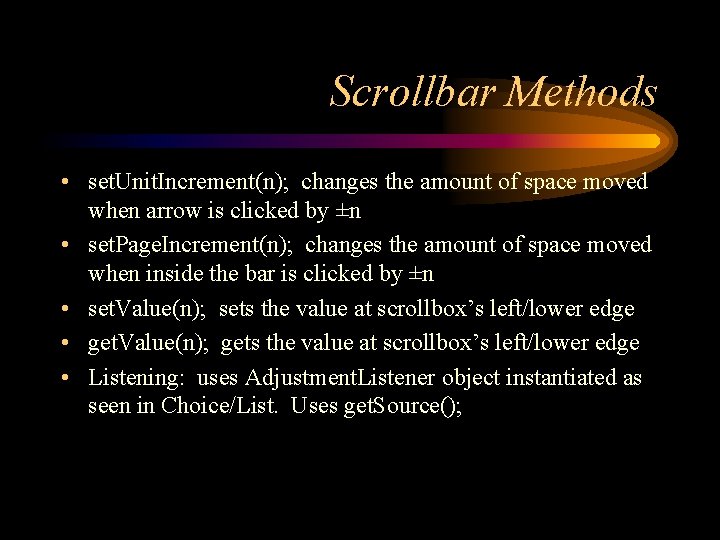
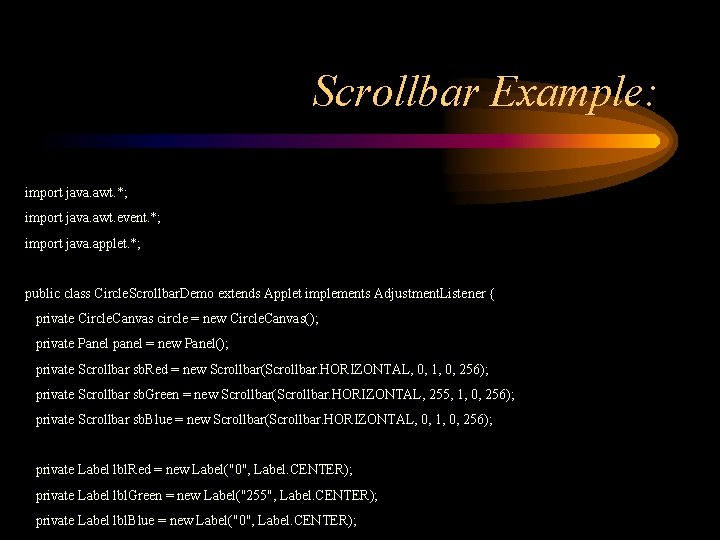
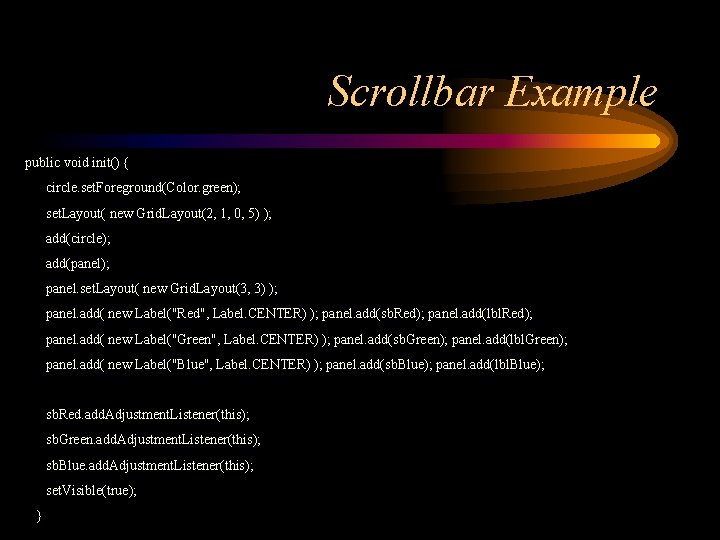
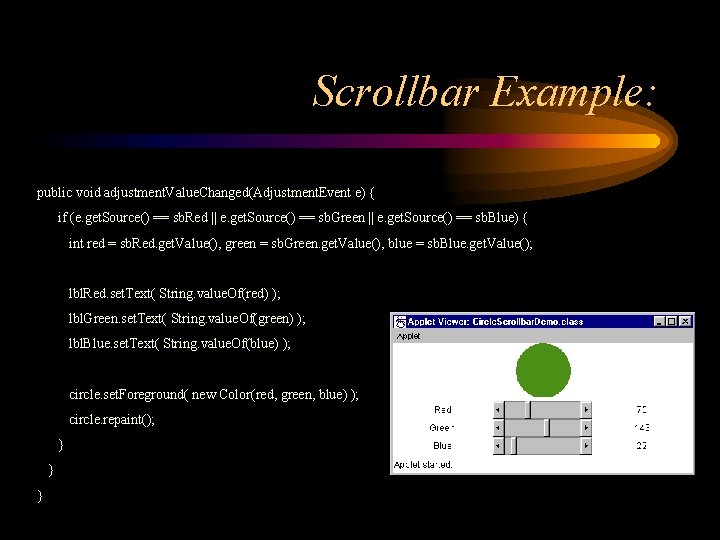
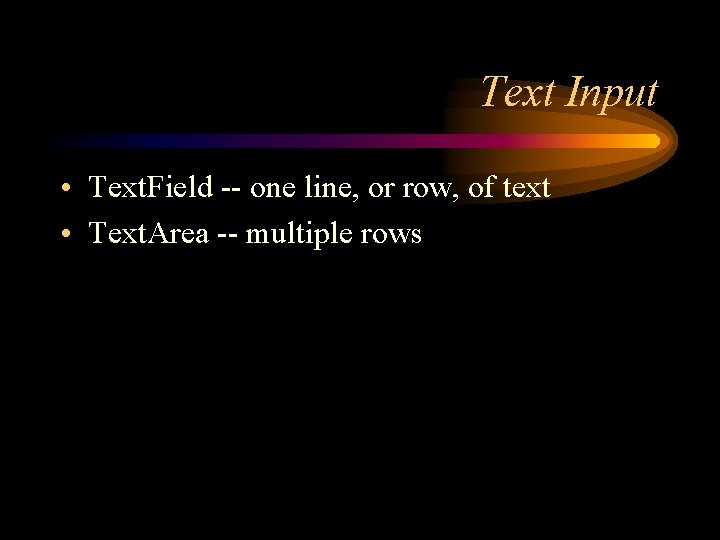
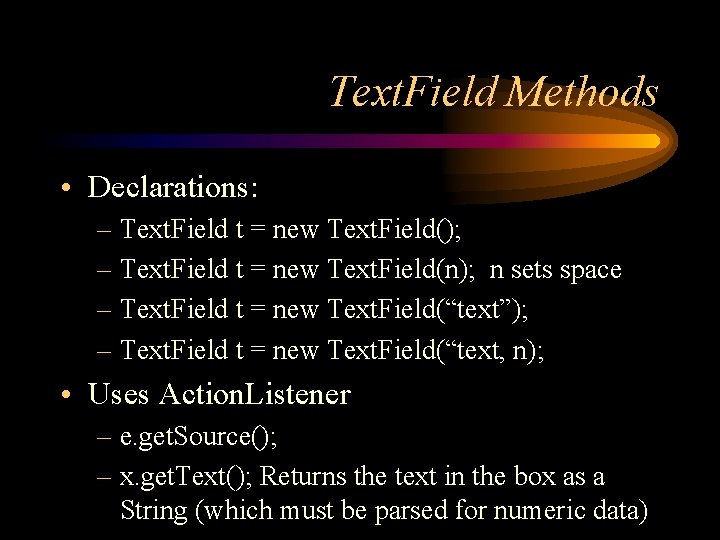
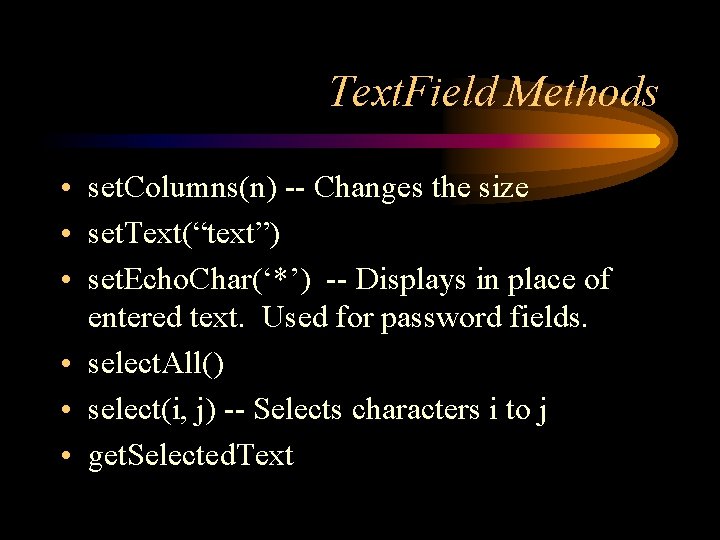
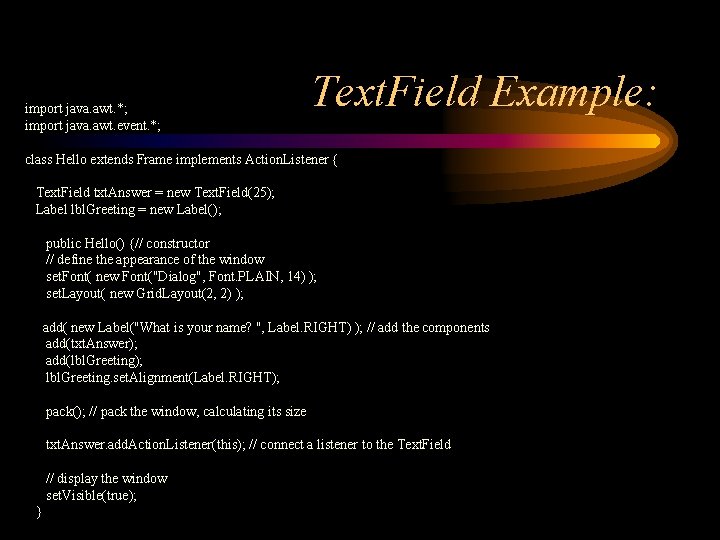
![Text. Field Example: public static void main(String[] arg) { Hello h = new Hello(); Text. Field Example: public static void main(String[] arg) { Hello h = new Hello();](https://slidetodoc.com/presentation_image/21468fe18b2ebd0c47c5b9b0c8764bcb/image-52.jpg)
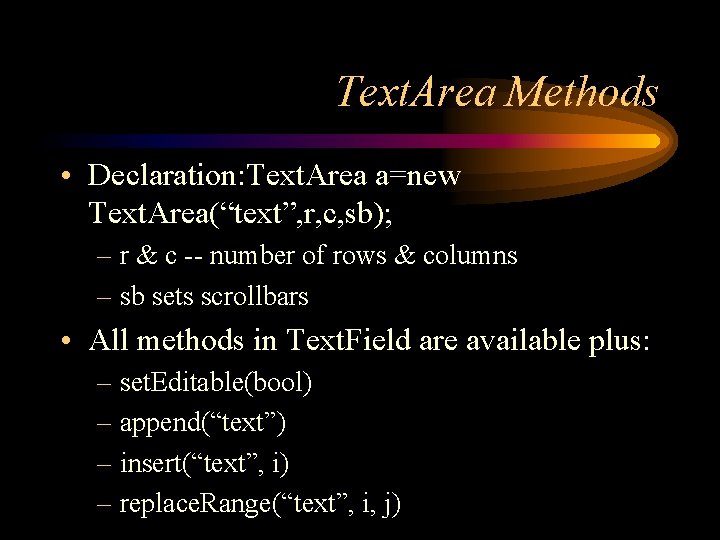
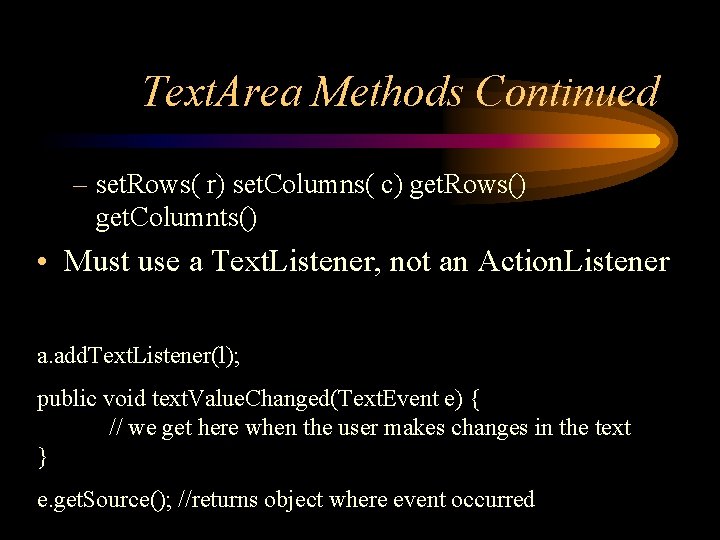
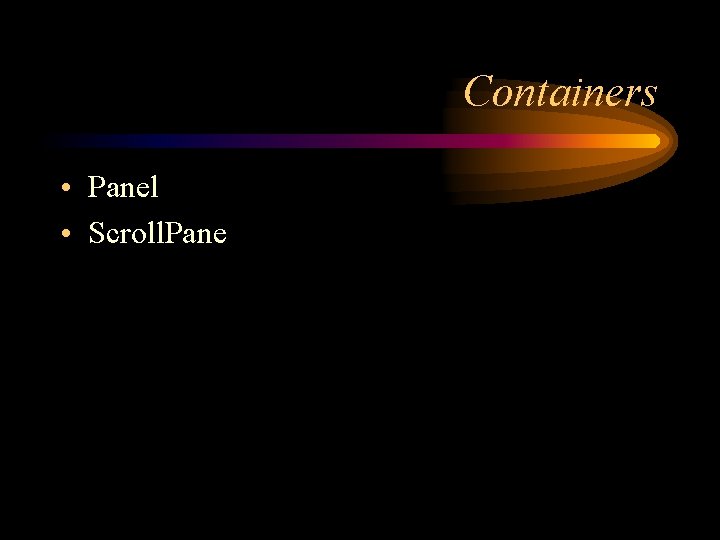
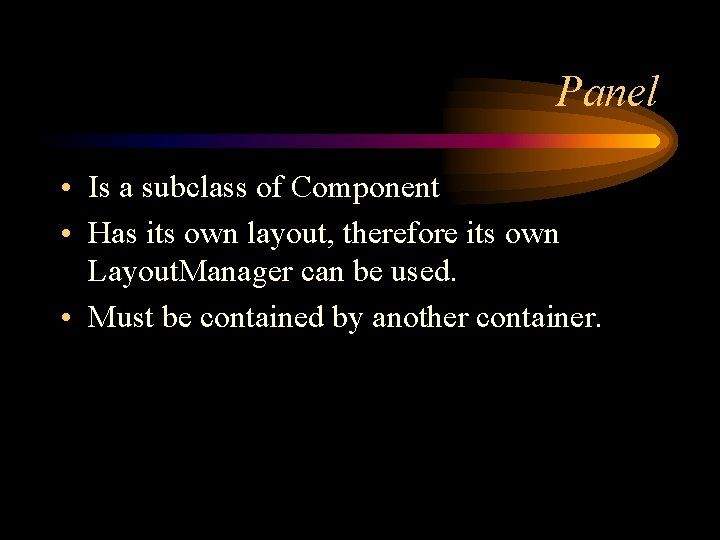
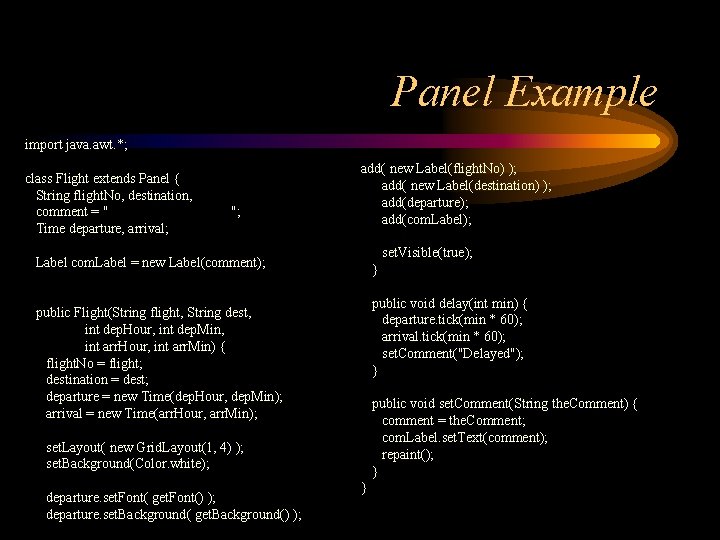
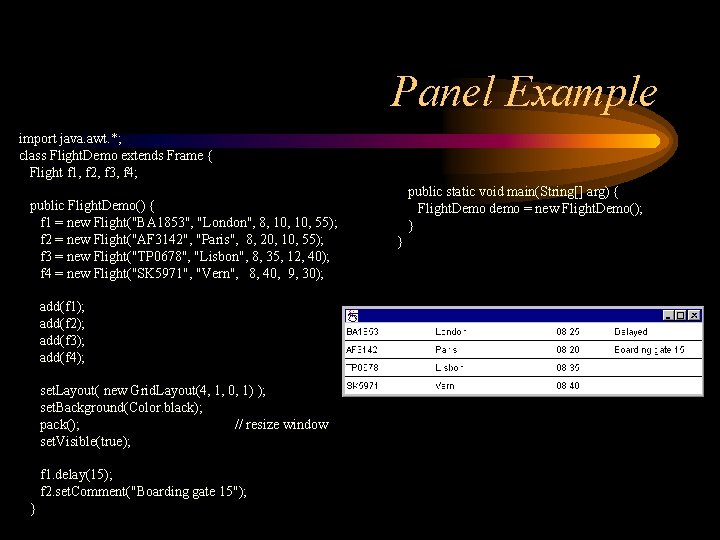
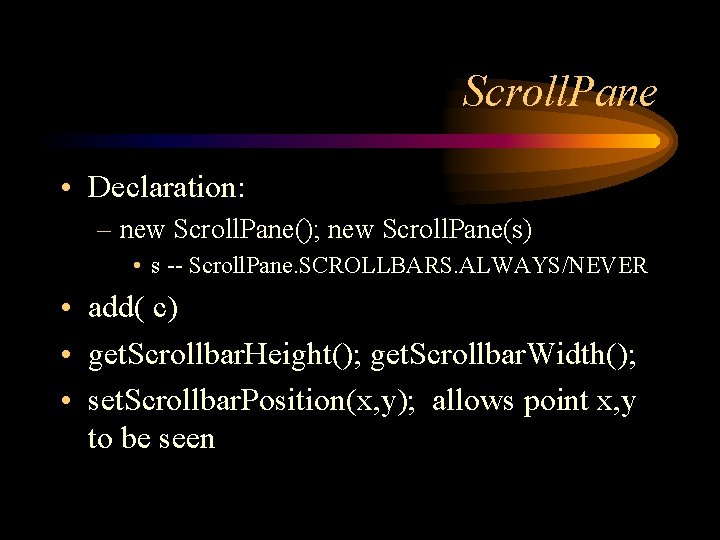
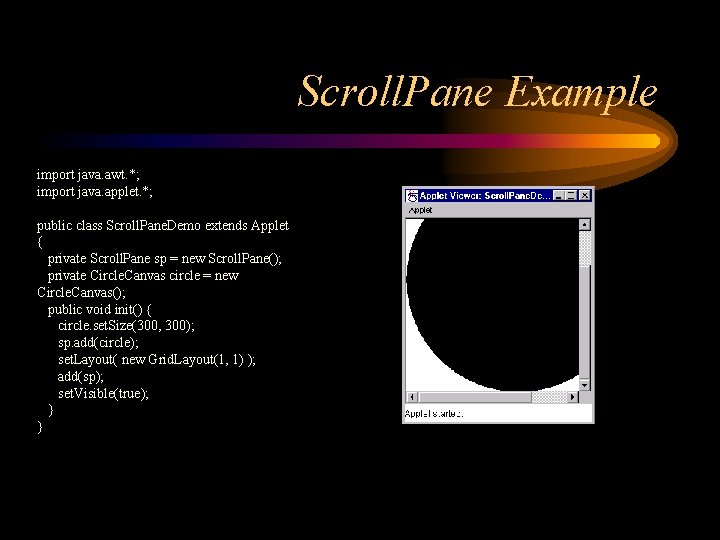
- Slides: 60
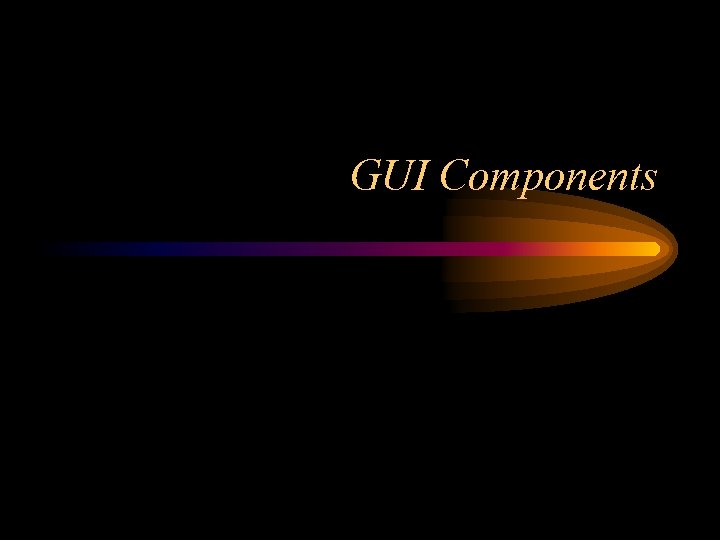
GUI Components
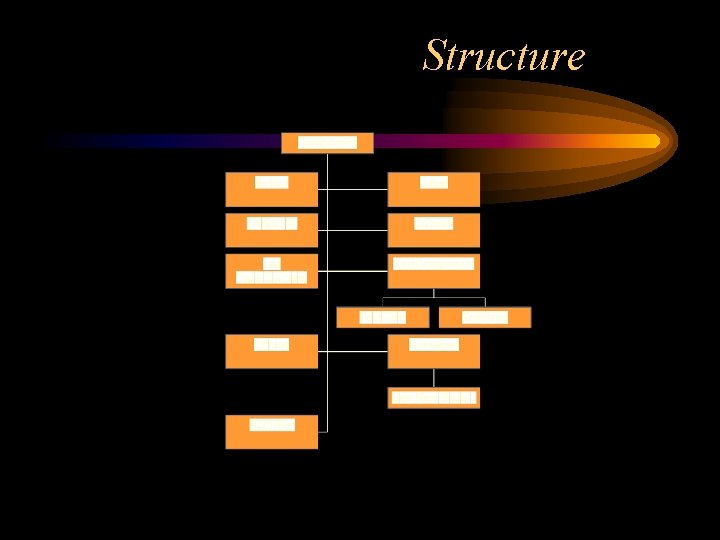
Structure
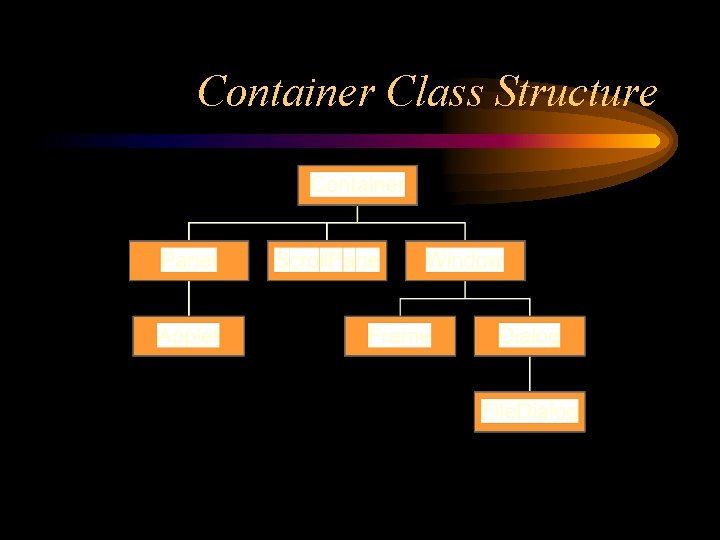
Container Class Structure
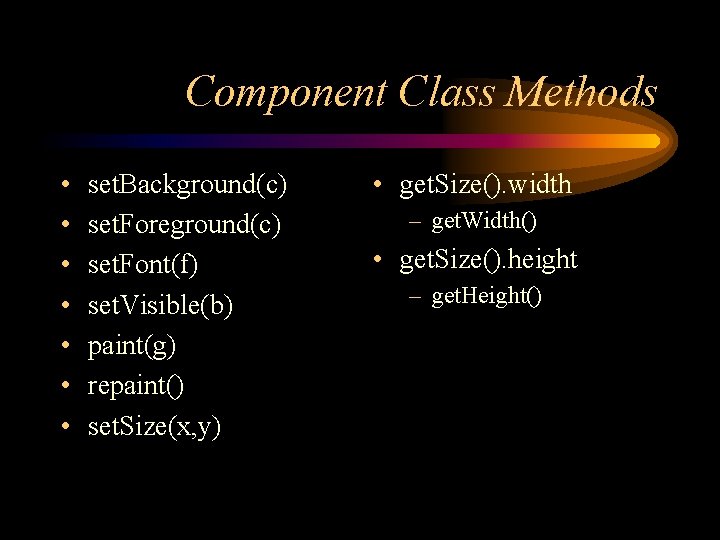
Component Class Methods • • set. Background(c) set. Foreground(c) set. Font(f) set. Visible(b) paint(g) repaint() set. Size(x, y) • get. Size(). width – get. Width() • get. Size(). height – get. Height()
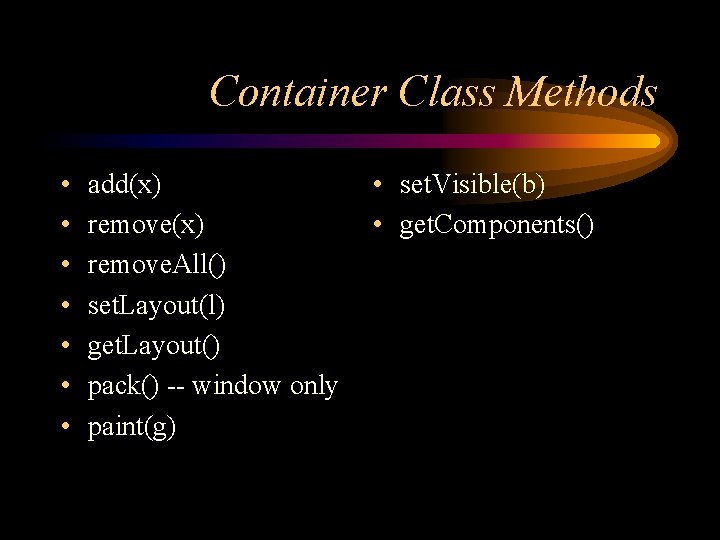
Container Class Methods • • add(x) remove. All() set. Layout(l) get. Layout() pack() -- window only paint(g) • set. Visible(b) • get. Components()
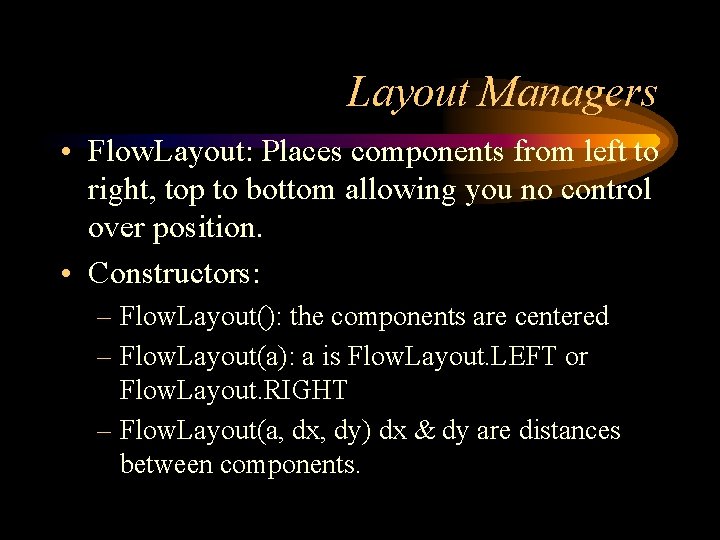
Layout Managers • Flow. Layout: Places components from left to right, top to bottom allowing you no control over position. • Constructors: – Flow. Layout(): the components are centered – Flow. Layout(a): a is Flow. Layout. LEFT or Flow. Layout. RIGHT – Flow. Layout(a, dx, dy) dx & dy are distances between components.
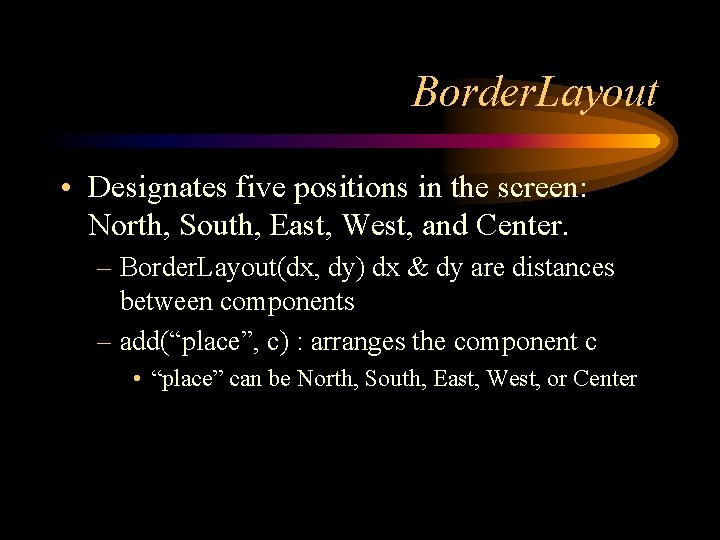
Border. Layout • Designates five positions in the screen: North, South, East, West, and Center. – Border. Layout(dx, dy) dx & dy are distances between components – add(“place”, c) : arranges the component c • “place” can be North, South, East, West, or Center
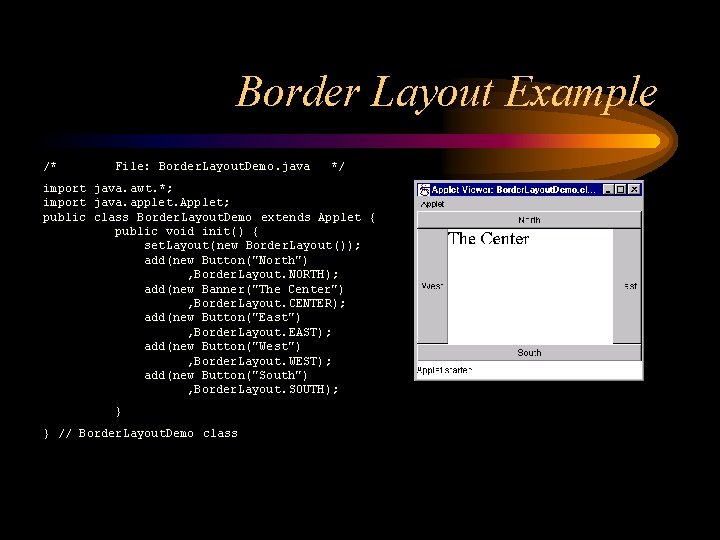
Border Layout Example /* File: Border. Layout. Demo. java */ import java. awt. *; import java. applet. Applet; public class Border. Layout. Demo extends Applet { public void init() { set. Layout(new Border. Layout()); add(new Button("North") , Border. Layout. NORTH); add(new Banner("The Center") , Border. Layout. CENTER); add(new Button("East") , Border. Layout. EAST); add(new Button("West") , Border. Layout. WEST); add(new Button("South") , Border. Layout. SOUTH); } } // Border. Layout. Demo class
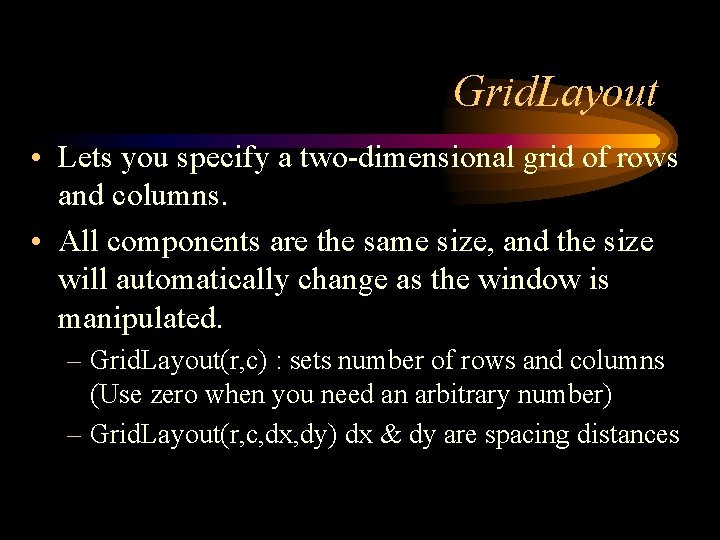
Grid. Layout • Lets you specify a two-dimensional grid of rows and columns. • All components are the same size, and the size will automatically change as the window is manipulated. – Grid. Layout(r, c) : sets number of rows and columns (Use zero when you need an arbitrary number) – Grid. Layout(r, c, dx, dy) dx & dy are spacing distances
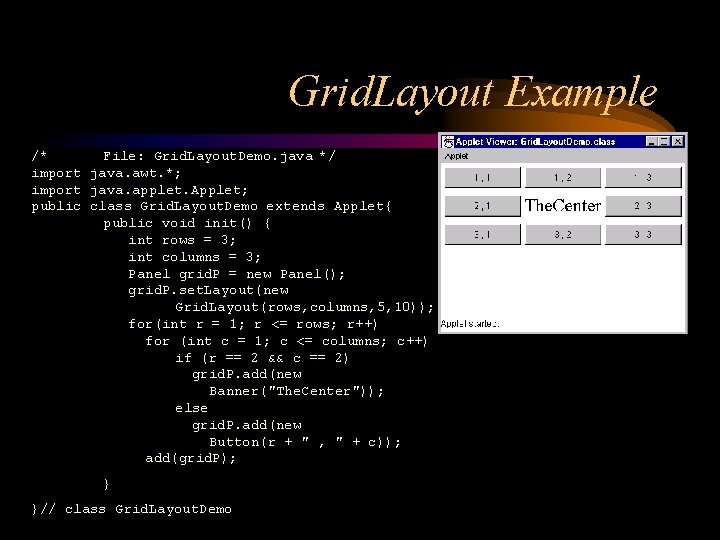
Grid. Layout Example /* File: Grid. Layout. Demo. java */ import java. awt. *; import java. applet. Applet; public class Grid. Layout. Demo extends Applet{ public void init() { int rows = 3; int columns = 3; Panel grid. P = new Panel(); grid. P. set. Layout(new Grid. Layout(rows, columns, 5, 10)); for(int r = 1; r <= rows; r++) for (int c = 1; c <= columns; c++) if (r == 2 && c == 2) grid. P. add(new Banner("The. Center")); else grid. P. add(new Button(r + " , " + c)); add(grid. P); } }// class Grid. Layout. Demo
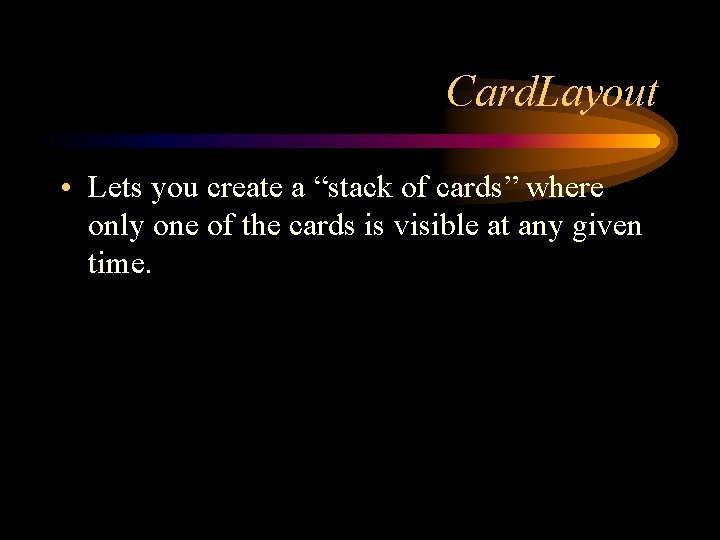
Card. Layout • Lets you create a “stack of cards” where only one of the cards is visible at any given time.
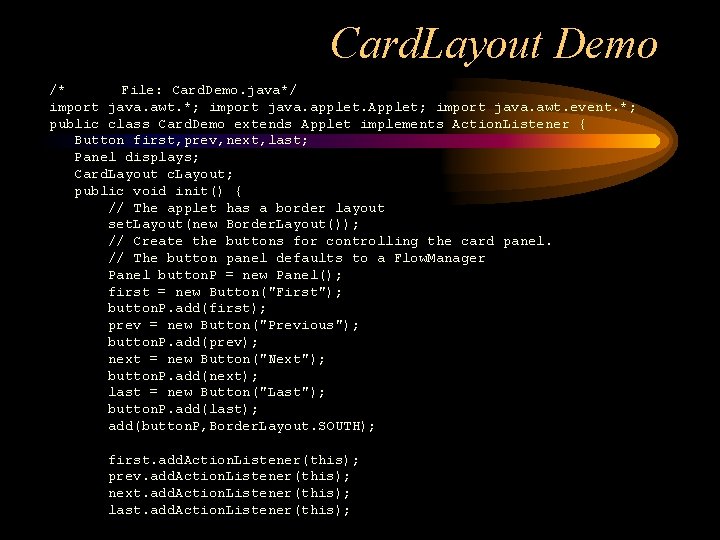
Card. Layout Demo /* File: Card. Demo. java*/ import java. awt. *; import java. applet. Applet; import java. awt. event. *; public class Card. Demo extends Applet implements Action. Listener { Button first, prev, next, last; Panel displays; Card. Layout c. Layout; public void init() { // The applet has a border layout set. Layout(new Border. Layout()); // Create the buttons for controlling the card panel. // The button panel defaults to a Flow. Manager Panel button. P = new Panel(); first = new Button("First"); button. P. add(first); prev = new Button("Previous"); button. P. add(prev); next = new Button("Next"); button. P. add(next); last = new Button("Last"); button. P. add(last); add(button. P, Border. Layout. SOUTH); first. add. Action. Listener(this); prev. add. Action. Listener(this); next. add. Action. Listener(this); last. add. Action. Listener(this);
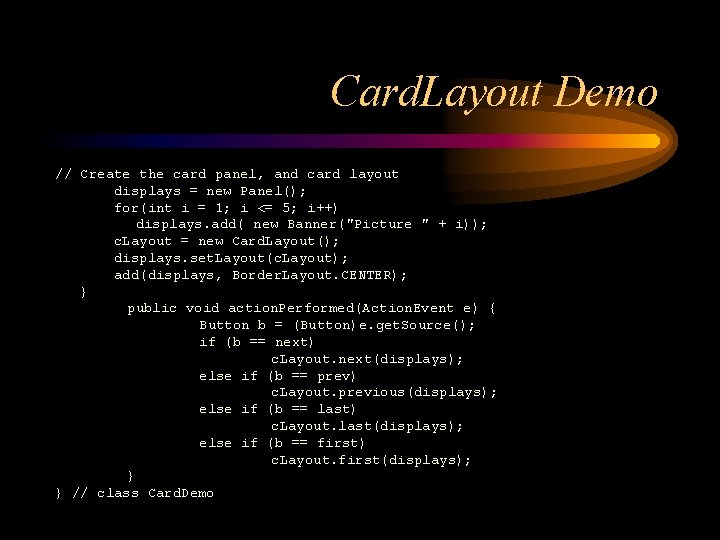
Card. Layout Demo // Create the card panel, and card layout displays = new Panel(); for(int i = 1; i <= 5; i++) displays. add( new Banner("Picture " + i)); c. Layout = new Card. Layout(); displays. set. Layout(c. Layout); add(displays, Border. Layout. CENTER); } public void action. Performed(Action. Event e) { Button b = (Button)e. get. Source(); if (b == next) c. Layout. next(displays); else if (b == prev) c. Layout. previous(displays); else if (b == last) c. Layout. last(displays); else if (b == first) c. Layout. first(displays); } } // class Card. Demo
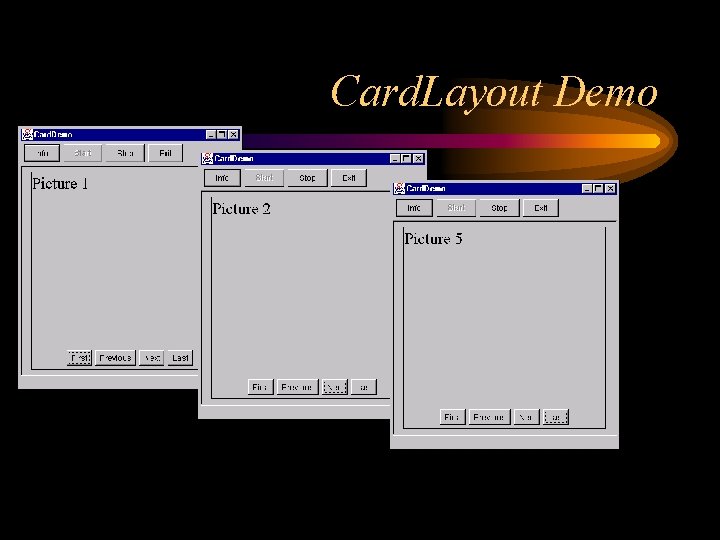
Card. Layout Demo
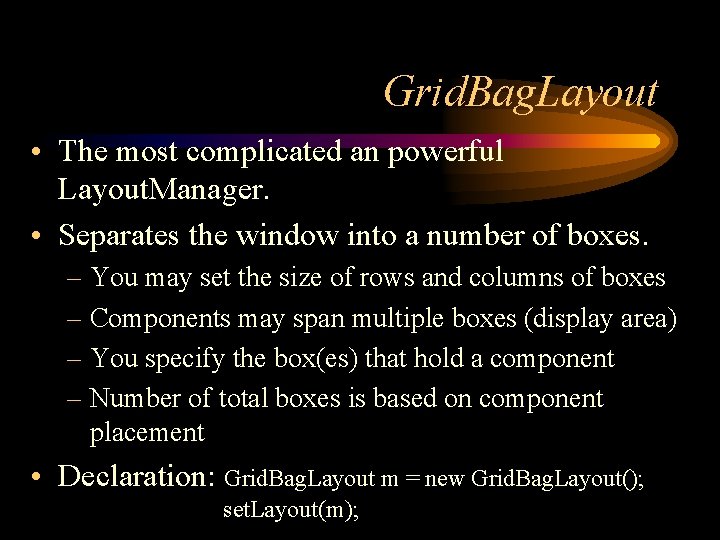
Grid. Bag. Layout • The most complicated an powerful Layout. Manager. • Separates the window into a number of boxes. – You may set the size of rows and columns of boxes – Components may span multiple boxes (display area) – You specify the box(es) that hold a component – Number of total boxes is based on component placement • Declaration: Grid. Bag. Layout m = new Grid. Bag. Layout(); set. Layout(m);
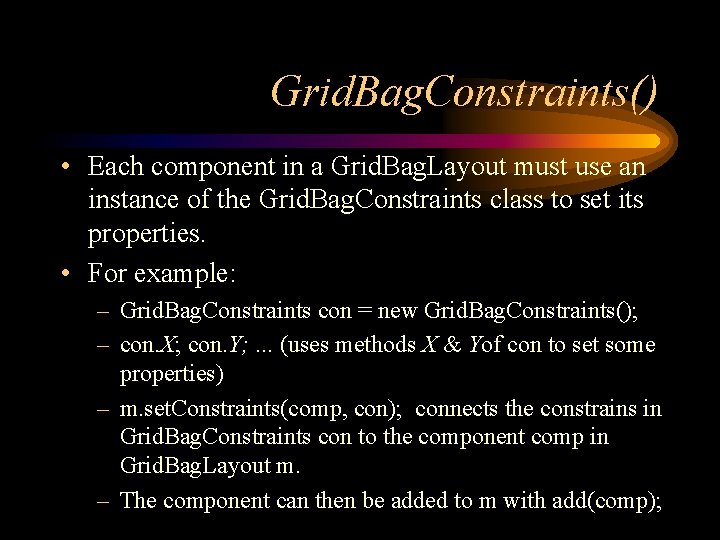
Grid. Bag. Constraints() • Each component in a Grid. Bag. Layout must use an instance of the Grid. Bag. Constraints class to set its properties. • For example: – Grid. Bag. Constraints con = new Grid. Bag. Constraints(); – con. X; con. Y; . . . (uses methods X & Yof con to set some properties) – m. set. Constraints(comp, con); connects the constrains in Grid. Bag. Constraints con to the component comp in Grid. Bag. Layout m. – The component can then be added to m with add(comp);
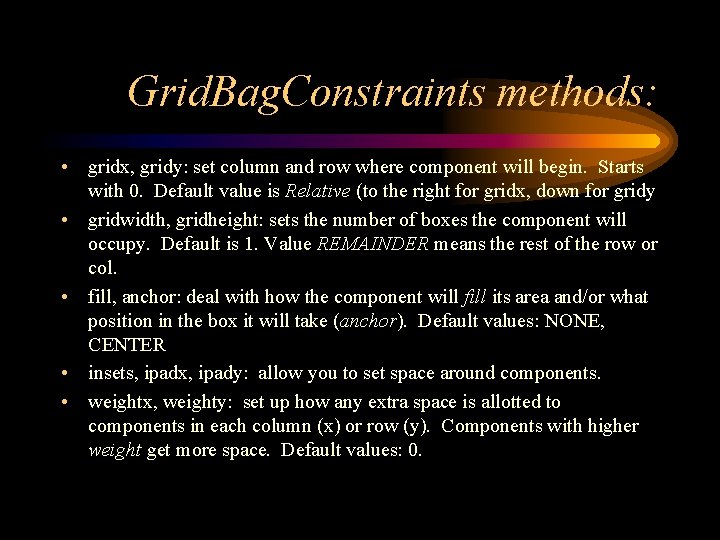
Grid. Bag. Constraints methods: • gridx, gridy: set column and row where component will begin. Starts with 0. Default value is Relative (to the right for gridx, down for gridy • gridwidth, gridheight: sets the number of boxes the component will occupy. Default is 1. Value REMAINDER means the rest of the row or col. • fill, anchor: deal with how the component will fill its area and/or what position in the box it will take (anchor). Default values: NONE, CENTER • insets, ipadx, ipady: allow you to set space around components. • weightx, weighty: set up how any extra space is allotted to components in each column (x) or row (y). Components with higher weight get more space. Default values: 0.
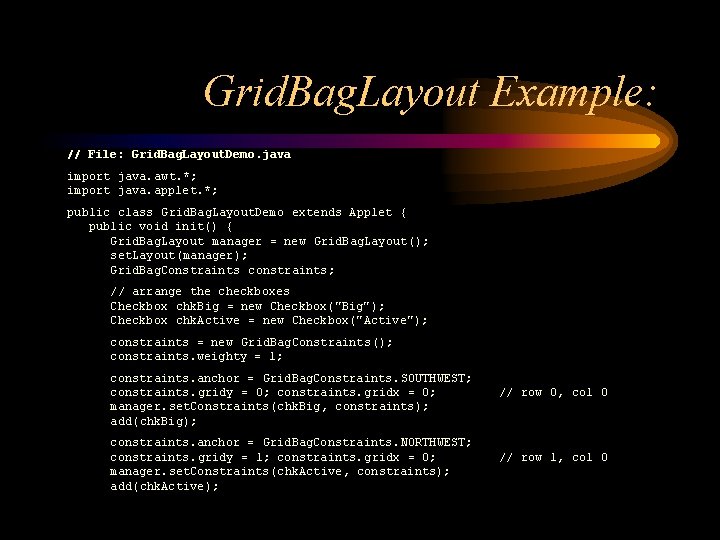
Grid. Bag. Layout Example: // File: Grid. Bag. Layout. Demo. java import java. awt. *; import java. applet. *; public class Grid. Bag. Layout. Demo extends Applet { public void init() { Grid. Bag. Layout manager = new Grid. Bag. Layout(); set. Layout(manager); Grid. Bag. Constraints constraints; // arrange the checkboxes Checkbox chk. Big = new Checkbox("Big"); Checkbox chk. Active = new Checkbox("Active"); constraints = new Grid. Bag. Constraints(); constraints. weighty = 1; constraints. anchor = Grid. Bag. Constraints. SOUTHWEST; constraints. gridy = 0; constraints. gridx = 0; manager. set. Constraints(chk. Big, constraints); add(chk. Big); constraints. anchor = Grid. Bag. Constraints. NORTHWEST; constraints. gridy = 1; constraints. gridx = 0; manager. set. Constraints(chk. Active, constraints); add(chk. Active); // row 0, col 0 // row 1, col 0
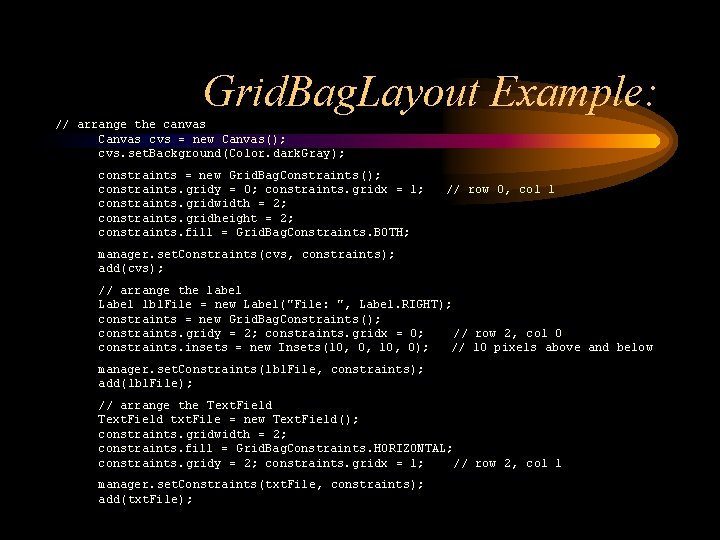
Grid. Bag. Layout Example: // arrange the canvas Canvas cvs = new Canvas(); cvs. set. Background(Color. dark. Gray); constraints = new Grid. Bag. Constraints(); constraints. gridy = 0; constraints. gridx = 1; constraints. gridwidth = 2; constraints. gridheight = 2; constraints. fill = Grid. Bag. Constraints. BOTH; // row 0, col 1 manager. set. Constraints(cvs, constraints); add(cvs); // arrange the label Label lbl. File = new Label("File: ", Label. RIGHT); constraints = new Grid. Bag. Constraints(); constraints. gridy = 2; constraints. gridx = 0; // row 2, col 0 constraints. insets = new Insets(10, 0, 10, 0); // 10 pixels above and below manager. set. Constraints(lbl. File, constraints); add(lbl. File); // arrange the Text. Field txt. File = new Text. Field(); constraints. gridwidth = 2; constraints. fill = Grid. Bag. Constraints. HORIZONTAL; constraints. gridy = 2; constraints. gridx = 1; // row 2, col 1 manager. set. Constraints(txt. File, constraints); add(txt. File);
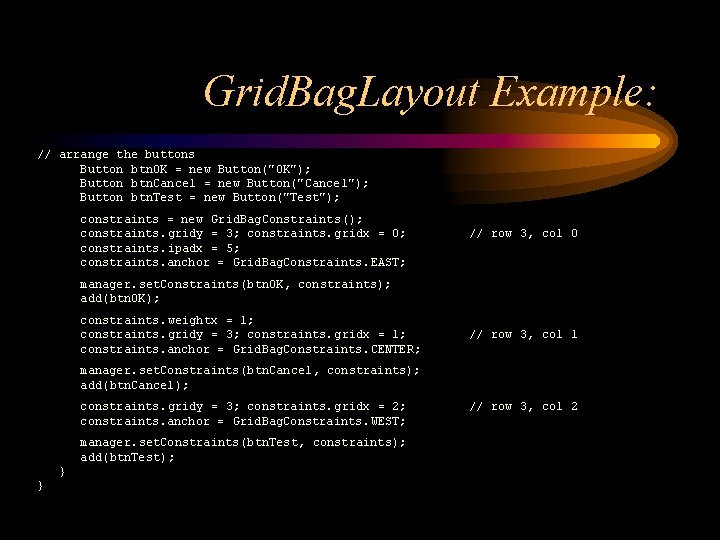
Grid. Bag. Layout Example: // arrange the buttons Button btn. OK = new Button("OK"); Button btn. Cancel = new Button("Cancel"); Button btn. Test = new Button("Test"); constraints = new Grid. Bag. Constraints(); constraints. gridy = 3; constraints. gridx = 0; constraints. ipadx = 5; constraints. anchor = Grid. Bag. Constraints. EAST; // row 3, col 0 manager. set. Constraints(btn. OK, constraints); add(btn. OK); constraints. weightx = 1; constraints. gridy = 3; constraints. gridx = 1; constraints. anchor = Grid. Bag. Constraints. CENTER; // row 3, col 1 manager. set. Constraints(btn. Cancel, constraints); add(btn. Cancel); constraints. gridy = 3; constraints. gridx = 2; constraints. anchor = Grid. Bag. Constraints. WEST; manager. set. Constraints(btn. Test, constraints); add(btn. Test); } } // row 3, col 2
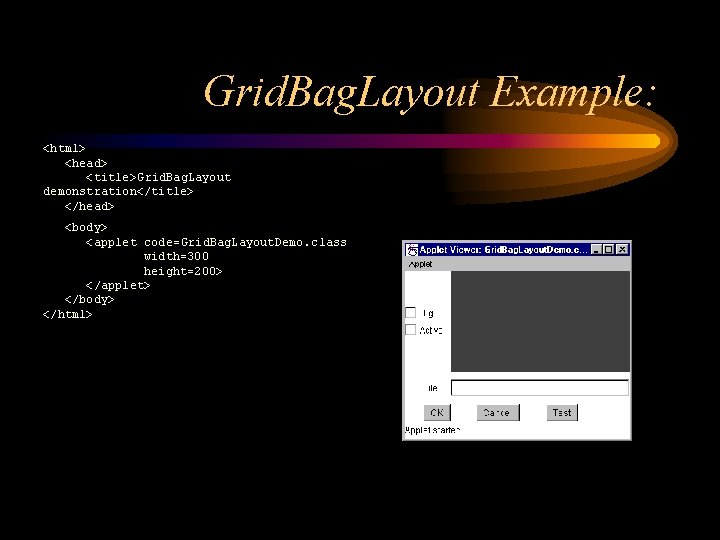
Grid. Bag. Layout Example: <html> <head> <title>Grid. Bag. Layout demonstration</title> </head> <body> <applet code=Grid. Bag. Layout. Demo. class width=300 height=200> </applet> </body> </html>
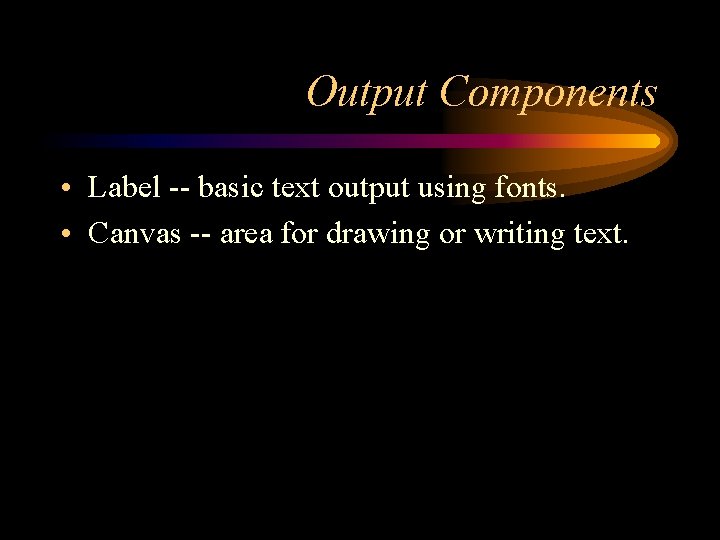
Output Components • Label -- basic text output using fonts. • Canvas -- area for drawing or writing text.
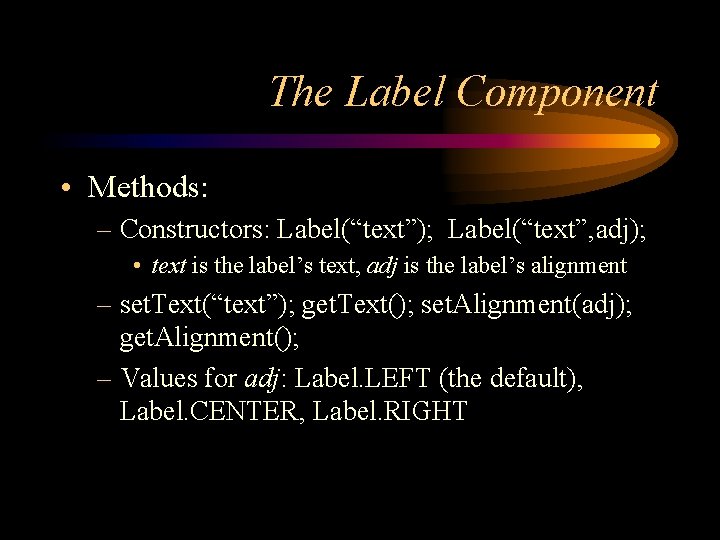
The Label Component • Methods: – Constructors: Label(“text”); Label(“text”, adj); • text is the label’s text, adj is the label’s alignment – set. Text(“text”); get. Text(); set. Alignment(adj); get. Alignment(); – Values for adj: Label. LEFT (the default), Label. CENTER, Label. RIGHT
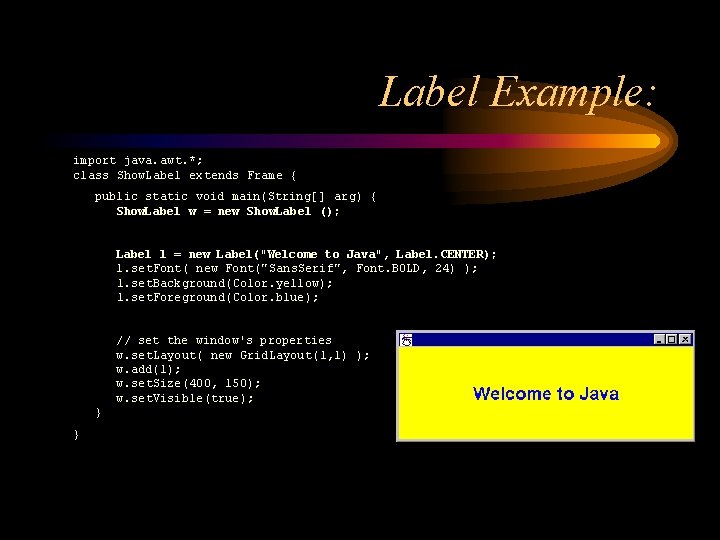
Label Example: import java. awt. *; class Show. Label extends Frame { public static void main(String[] arg) { Show. Label w = new Show. Label (); Label l = new Label("Welcome to Java", Label. CENTER); l. set. Font( new Font("Sans. Serif", Font. BOLD, 24) ); l. set. Background(Color. yellow); l. set. Foreground(Color. blue); // set the window's properties w. set. Layout( new Grid. Layout(1, 1) ); w. add(l); w. set. Size(400, 150); w. set. Visible(true); } }
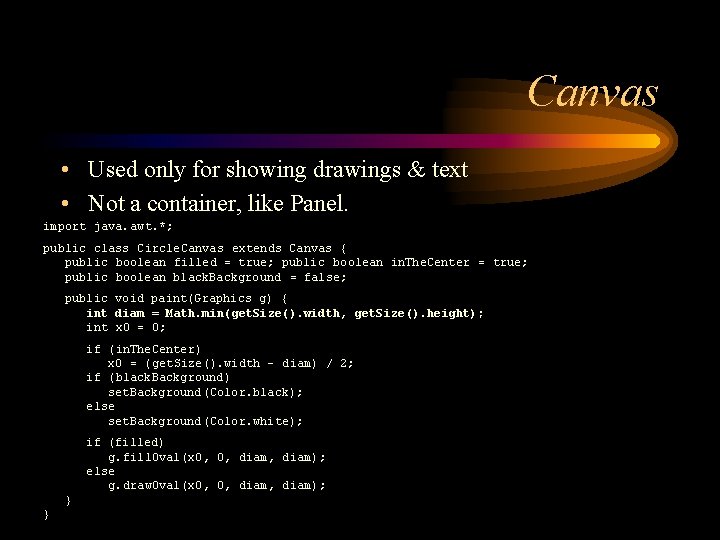
Canvas • Used only for showing drawings & text • Not a container, like Panel. import java. awt. *; public class Circle. Canvas extends Canvas { public boolean filled = true; public boolean in. The. Center = true; public boolean black. Background = false; public void paint(Graphics g) { int diam = Math. min(get. Size(). width, get. Size(). height); int x 0 = 0; if (in. The. Center) x 0 = (get. Size(). width - diam) / 2; if (black. Background) set. Background(Color. black); else set. Background(Color. white); if (filled) g. fill. Oval(x 0, 0, diam); else g. draw. Oval(x 0, 0, diam); } }
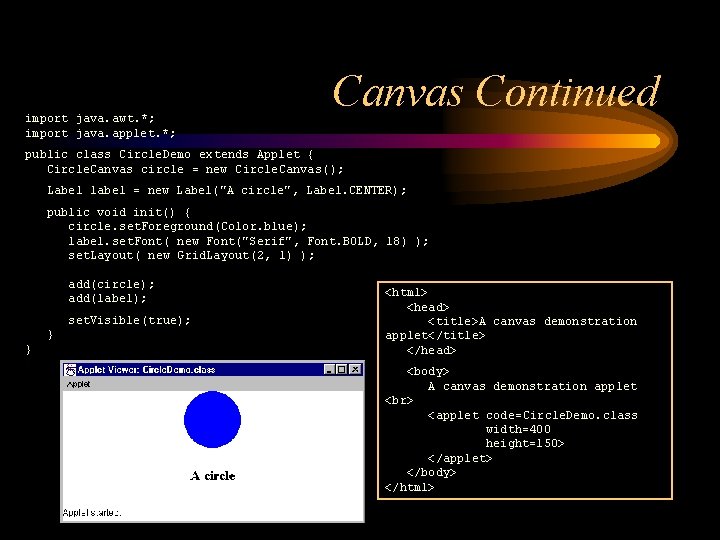
import java. awt. *; import java. applet. *; Canvas Continued public class Circle. Demo extends Applet { Circle. Canvas circle = new Circle. Canvas(); Label label = new Label("A circle", Label. CENTER); public void init() { circle. set. Foreground(Color. blue); label. set. Font( new Font("Serif", Font. BOLD, 18) ); set. Layout( new Grid. Layout(2, 1) ); add(circle); add(label); set. Visible(true); } } <html> <head> <title>A canvas demonstration applet</title> </head> <body> A canvas demonstration applet <applet code=Circle. Demo. class width=400 height=150> </applet> </body> </html>
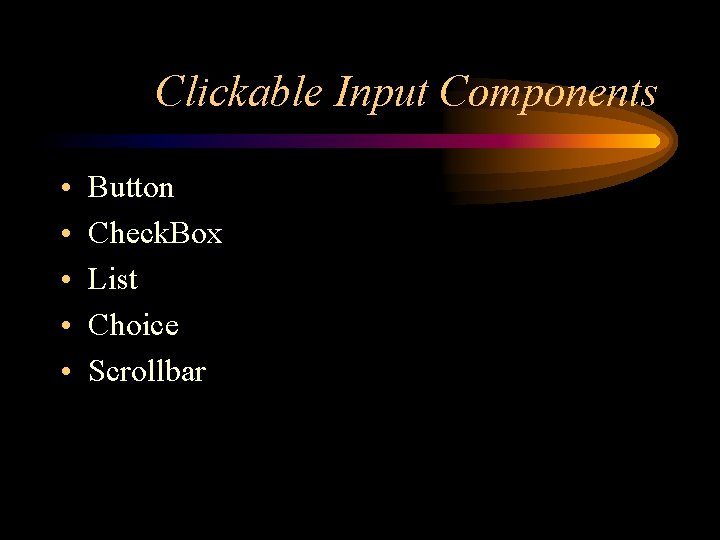
Clickable Input Components • • • Button Check. Box List Choice Scrollbar
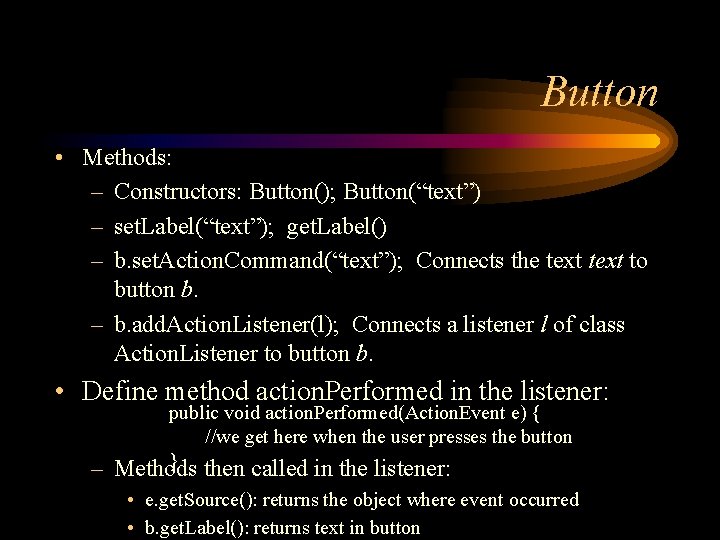
Button • Methods: – Constructors: Button(); Button(“text”) – set. Label(“text”); get. Label() – b. set. Action. Command(“text”); Connects the text to button b. – b. add. Action. Listener(l); Connects a listener l of class Action. Listener to button b. • Define method action. Performed in the listener: – public void action. Performed(Action. Event e) { //we get here when the user presses the button } then called in the listener: Methods • e. get. Source(): returns the object where event occurred • b. get. Label(): returns text in button
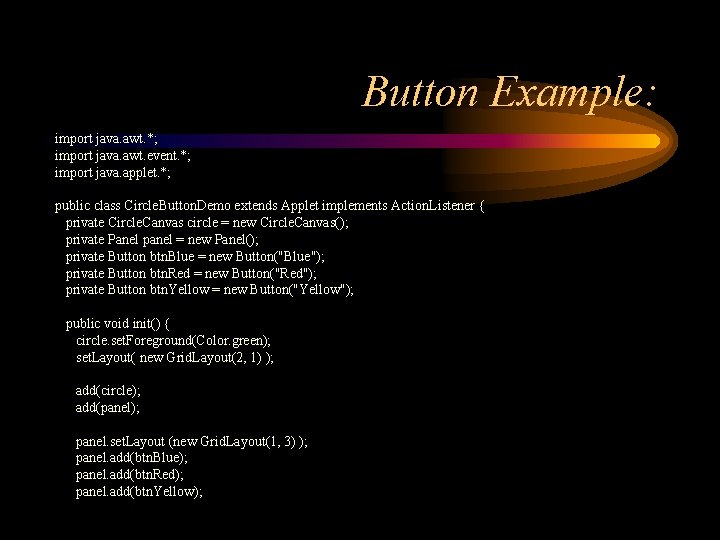
Button Example: import java. awt. *; import java. awt. event. *; import java. applet. *; public class Circle. Button. Demo extends Applet implements Action. Listener { private Circle. Canvas circle = new Circle. Canvas(); private Panel panel = new Panel(); private Button btn. Blue = new Button("Blue"); private Button btn. Red = new Button("Red"); private Button btn. Yellow = new Button("Yellow"); public void init() { circle. set. Foreground(Color. green); set. Layout( new Grid. Layout(2, 1) ); add(circle); add(panel); panel. set. Layout (new Grid. Layout(1, 3) ); panel. add(btn. Blue); panel. add(btn. Red); panel. add(btn. Yellow);
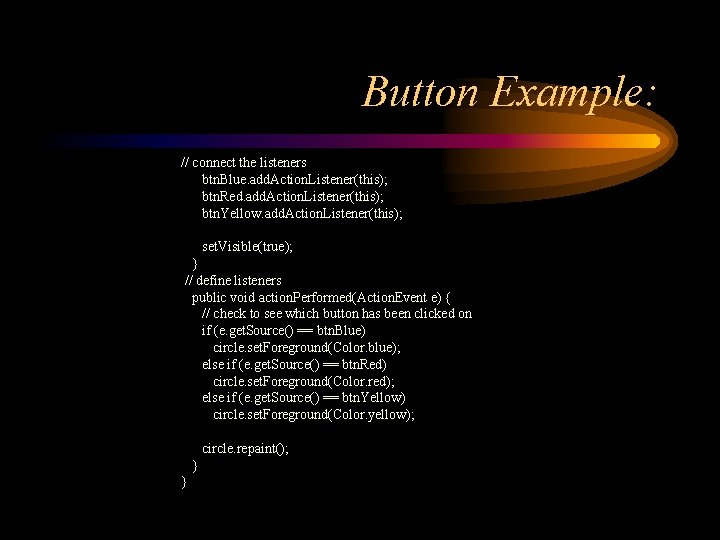
Button Example: // connect the listeners btn. Blue. add. Action. Listener(this); btn. Red. add. Action. Listener(this); btn. Yellow. add. Action. Listener(this); set. Visible(true); } // define listeners public void action. Performed(Action. Event e) { // check to see which button has been clicked on if (e. get. Source() == btn. Blue) circle. set. Foreground(Color. blue); else if (e. get. Source() == btn. Red) circle. set. Foreground(Color. red); else if (e. get. Source() == btn. Yellow) circle. set. Foreground(Color. yellow); circle. repaint(); } }
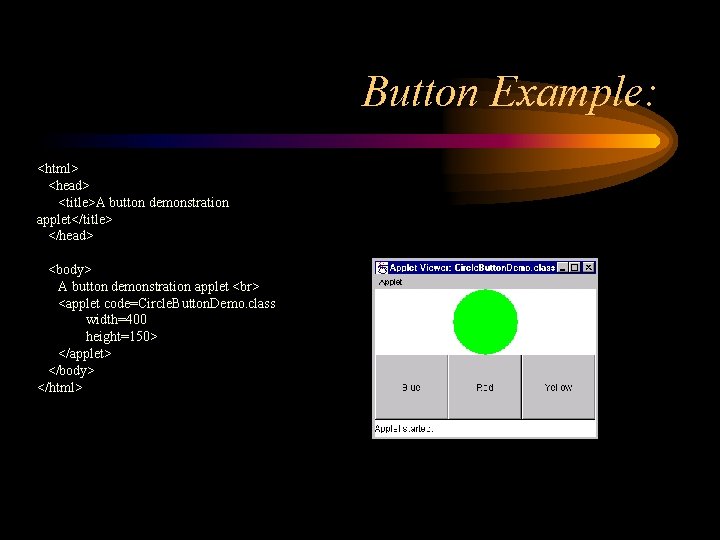
Button Example: <html> <head> <title>A button demonstration applet</title> </head> <body> A button demonstration applet <applet code=Circle. Button. Demo. class width=400 height=150> </applet> </body> </html>
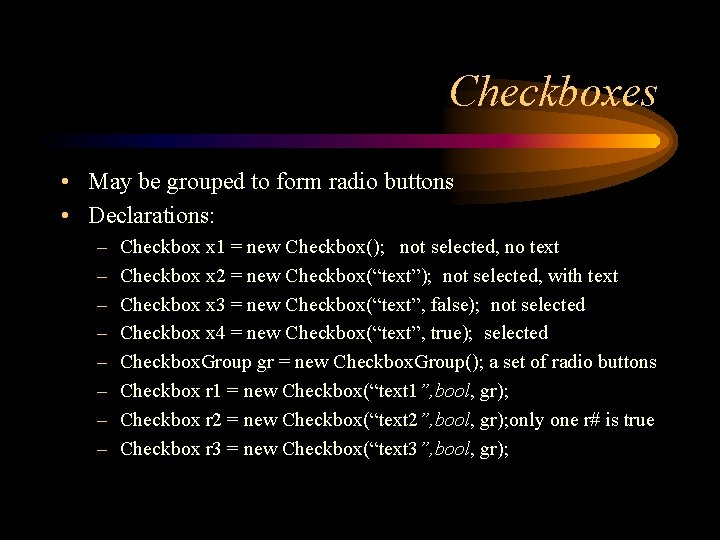
Checkboxes • May be grouped to form radio buttons • Declarations: – – – – Checkbox x 1 = new Checkbox(); not selected, no text Checkbox x 2 = new Checkbox(“text”); not selected, with text Checkbox x 3 = new Checkbox(“text”, false); not selected Checkbox x 4 = new Checkbox(“text”, true); selected Checkbox. Group gr = new Checkbox. Group(); a set of radio buttons Checkbox r 1 = new Checkbox(“text 1”, bool, gr); Checkbox r 2 = new Checkbox(“text 2”, bool, gr); only one r# is true Checkbox r 3 = new Checkbox(“text 3”, bool, gr);
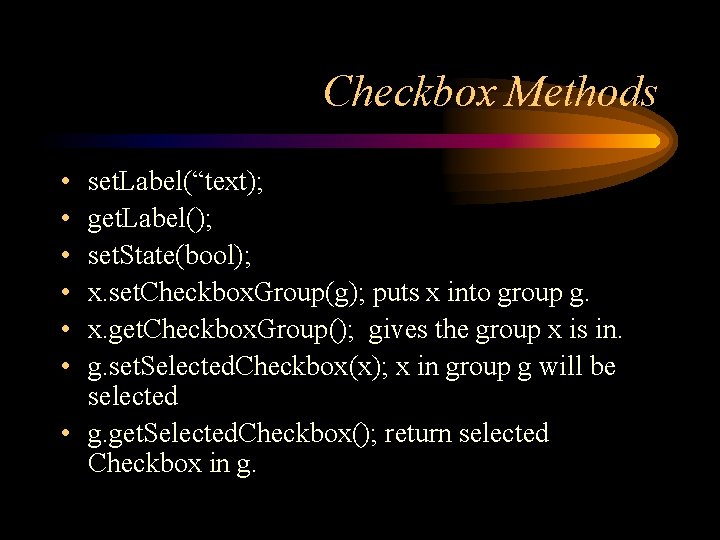
Checkbox Methods • • • set. Label(“text); get. Label(); set. State(bool); x. set. Checkbox. Group(g); puts x into group g. x. get. Checkbox. Group(); gives the group x is in. g. set. Selected. Checkbox(x); x in group g will be selected • g. get. Selected. Checkbox(); return selected Checkbox in g.
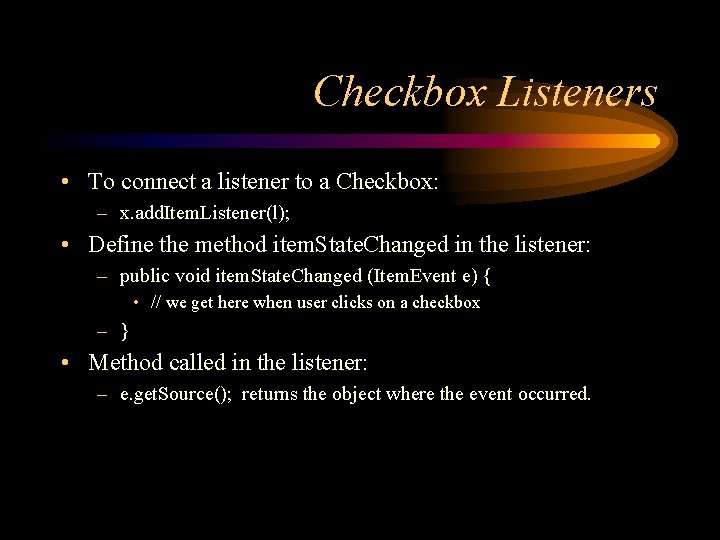
Checkbox Listeners • To connect a listener to a Checkbox: – x. add. Item. Listener(l); • Define the method item. State. Changed in the listener: – public void item. State. Changed (Item. Event e) { • // we get here when user clicks on a checkbox – } • Method called in the listener: – e. get. Source(); returns the object where the event occurred.
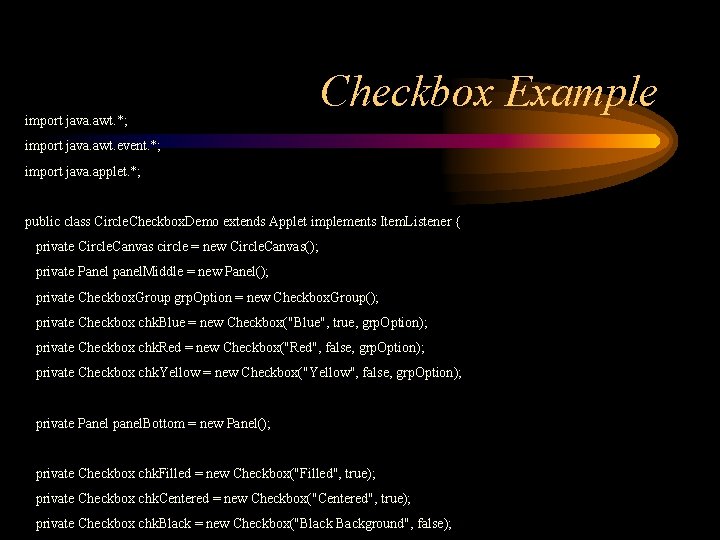
import java. awt. *; Checkbox Example import java. awt. event. *; import java. applet. *; public class Circle. Checkbox. Demo extends Applet implements Item. Listener { private Circle. Canvas circle = new Circle. Canvas(); private Panel panel. Middle = new Panel(); private Checkbox. Group grp. Option = new Checkbox. Group(); private Checkbox chk. Blue = new Checkbox("Blue", true, grp. Option); private Checkbox chk. Red = new Checkbox("Red", false, grp. Option); private Checkbox chk. Yellow = new Checkbox("Yellow", false, grp. Option); private Panel panel. Bottom = new Panel(); private Checkbox chk. Filled = new Checkbox("Filled", true); private Checkbox chk. Centered = new Checkbox("Centered", true); private Checkbox chk. Black = new Checkbox("Black Background", false);
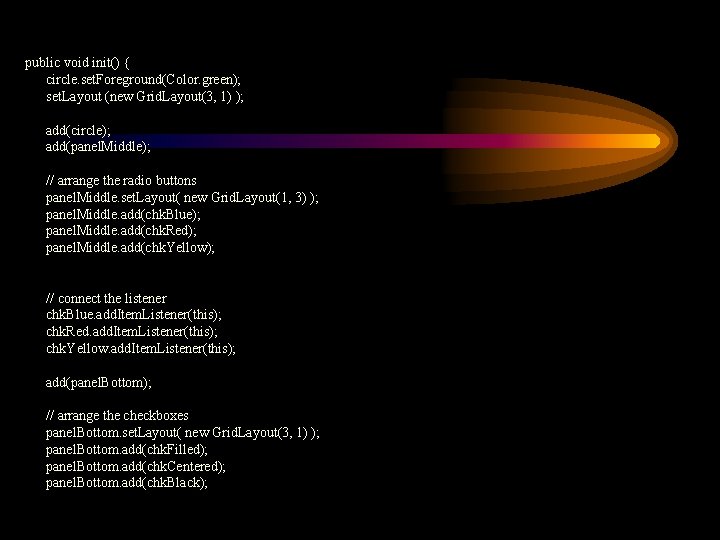
public void init() { circle. set. Foreground(Color. green); set. Layout (new Grid. Layout(3, 1) ); add(circle); add(panel. Middle); // arrange the radio buttons panel. Middle. set. Layout( new Grid. Layout(1, 3) ); panel. Middle. add(chk. Blue); panel. Middle. add(chk. Red); panel. Middle. add(chk. Yellow); // connect the listener chk. Blue. add. Item. Listener(this); chk. Red. add. Item. Listener(this); chk. Yellow. add. Item. Listener(this); add(panel. Bottom); // arrange the checkboxes panel. Bottom. set. Layout( new Grid. Layout(3, 1) ); panel. Bottom. add(chk. Filled); panel. Bottom. add(chk. Centered); panel. Bottom. add(chk. Black);
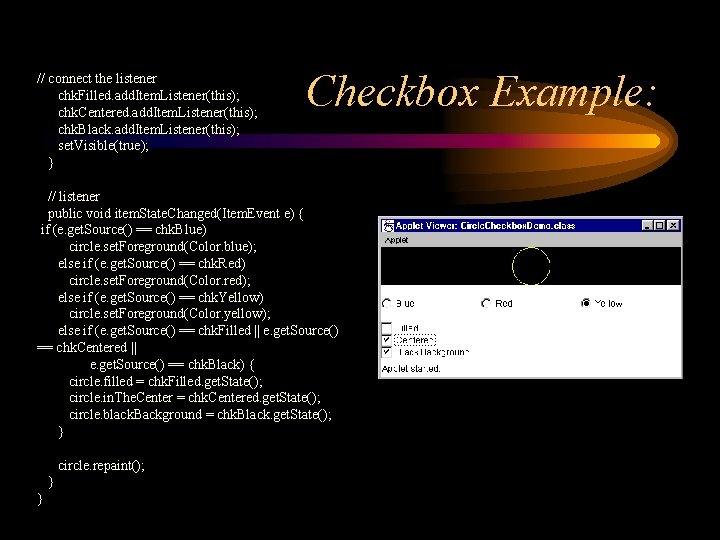
// connect the listener chk. Filled. add. Item. Listener(this); chk. Centered. add. Item. Listener(this); chk. Black. add. Item. Listener(this); set. Visible(true); } Checkbox Example: // listener public void item. State. Changed(Item. Event e) { if (e. get. Source() == chk. Blue) circle. set. Foreground(Color. blue); else if (e. get. Source() == chk. Red) circle. set. Foreground(Color. red); else if (e. get. Source() == chk. Yellow) circle. set. Foreground(Color. yellow); else if (e. get. Source() == chk. Filled || e. get. Source() == chk. Centered || e. get. Source() == chk. Black) { circle. filled = chk. Filled. get. State(); circle. in. The. Center = chk. Centered. get. State(); circle. black. Background = chk. Black. get. State(); } circle. repaint(); } }
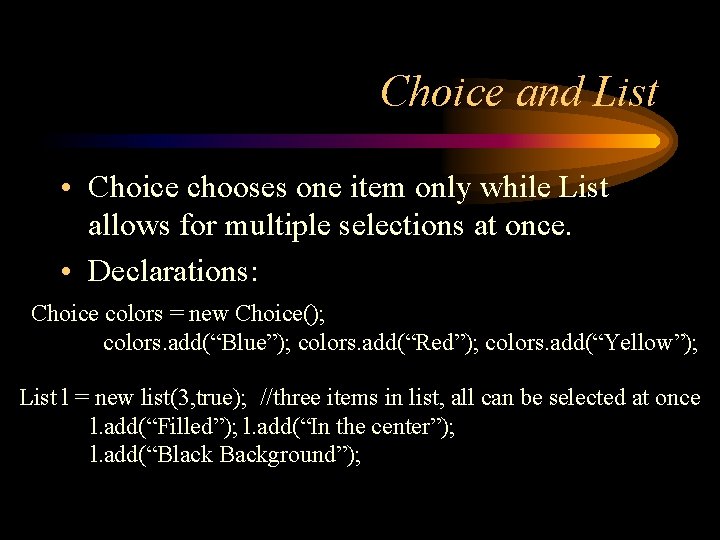
Choice and List • Choice chooses one item only while List allows for multiple selections at once. • Declarations: Choice colors = new Choice(); colors. add(“Blue”); colors. add(“Red”); colors. add(“Yellow”); List l = new list(3, true); //three items in list, all can be selected at once l. add(“Filled”); l. add(“In the center”); l. add(“Black Background”);
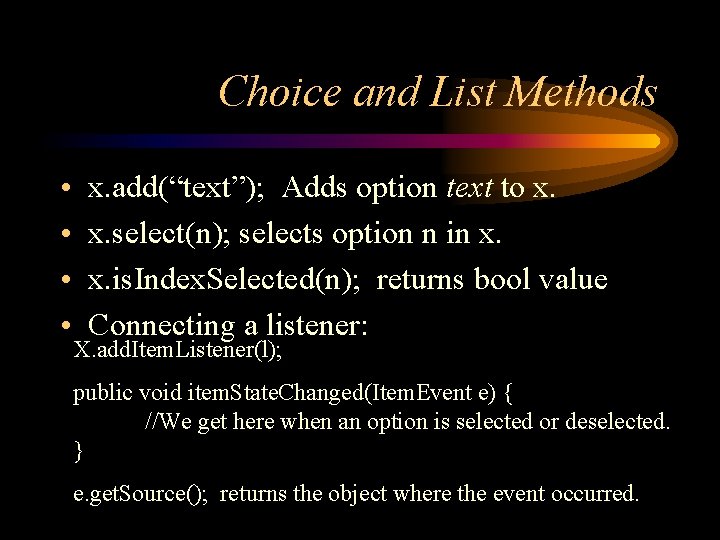
Choice and List Methods • • x. add(“text”); Adds option text to x. x. select(n); selects option n in x. x. is. Index. Selected(n); returns bool value Connecting a listener: X. add. Item. Listener(l); public void item. State. Changed(Item. Event e) { //We get here when an option is selected or deselected. } e. get. Source(); returns the object where the event occurred.
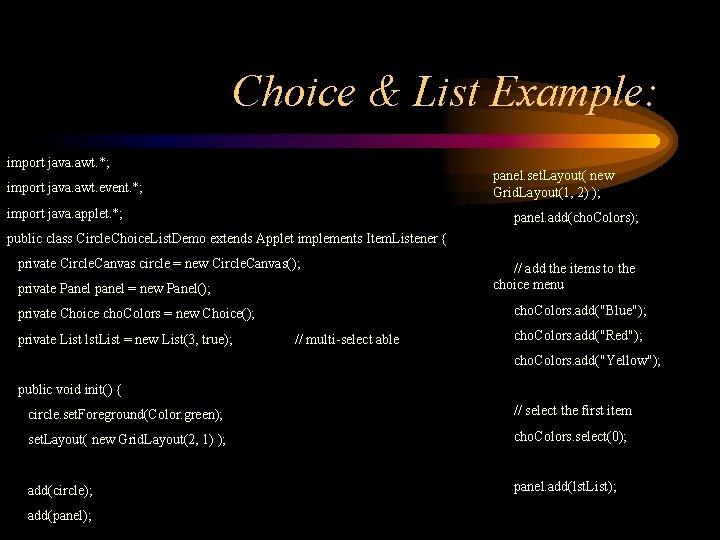
Choice & List Example: import java. awt. *; panel. set. Layout( new Grid. Layout(1, 2) ); import java. awt. event. *; import java. applet. *; panel. add(cho. Colors); public class Circle. Choice. List. Demo extends Applet implements Item. Listener { private Circle. Canvas circle = new Circle. Canvas(); private Panel panel = new Panel(); cho. Colors. add("Blue"); private Choice cho. Colors = new Choice(); private List lst. List = new List(3, true); // add the items to the choice menu // multi-select able cho. Colors. add("Red"); cho. Colors. add("Yellow"); public void init() { circle. set. Foreground(Color. green); // select the first item set. Layout( new Grid. Layout(2, 1) ); cho. Colors. select(0); add(circle); panel. add(lst. List); add(panel);
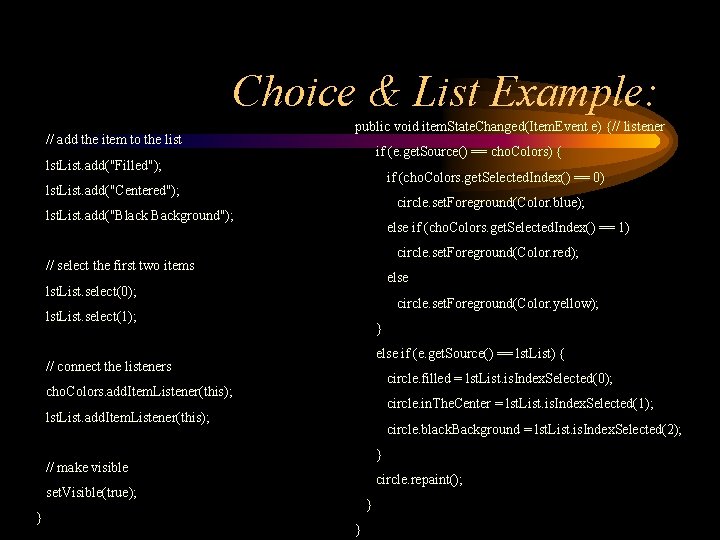
Choice & List Example: // add the item to the list public void item. State. Changed(Item. Event e) {// listener if (e. get. Source() == cho. Colors) { lst. List. add("Filled"); if (cho. Colors. get. Selected. Index() == 0) lst. List. add("Centered"); circle. set. Foreground(Color. blue); lst. List. add("Black Background"); else if (cho. Colors. get. Selected. Index() == 1) circle. set. Foreground(Color. red); // select the first two items else lst. List. select(0); circle. set. Foreground(Color. yellow); lst. List. select(1); } else if (e. get. Source() == lst. List) { // connect the listeners circle. filled = lst. List. is. Index. Selected(0); cho. Colors. add. Item. Listener(this); circle. in. The. Center = lst. List. is. Index. Selected(1); lst. List. add. Item. Listener(this); circle. black. Background = lst. List. is. Index. Selected(2); } // make visible circle. repaint(); set. Visible(true); } } }
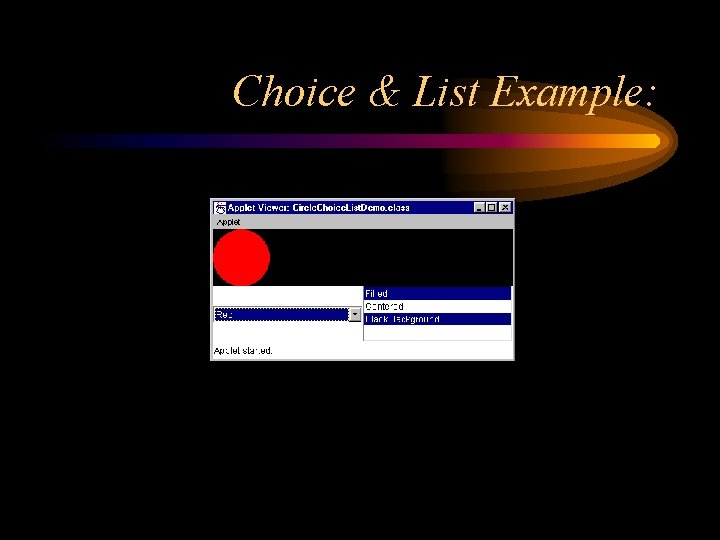
Choice & List Example:
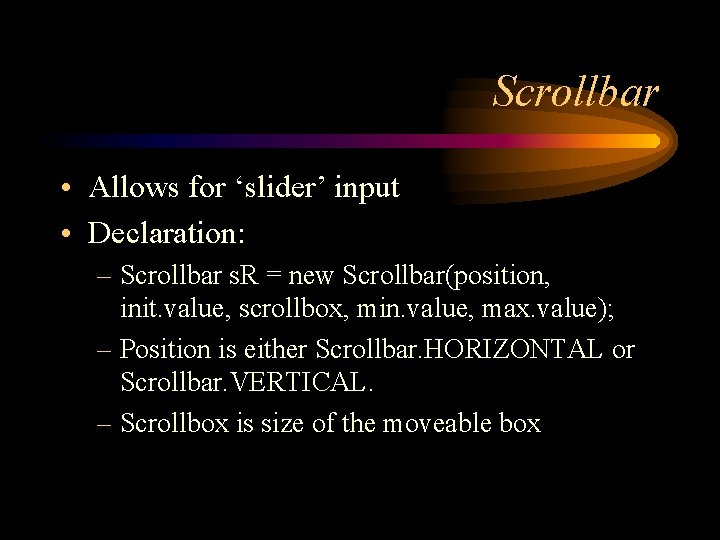
Scrollbar • Allows for ‘slider’ input • Declaration: – Scrollbar s. R = new Scrollbar(position, init. value, scrollbox, min. value, max. value); – Position is either Scrollbar. HORIZONTAL or Scrollbar. VERTICAL. – Scrollbox is size of the moveable box
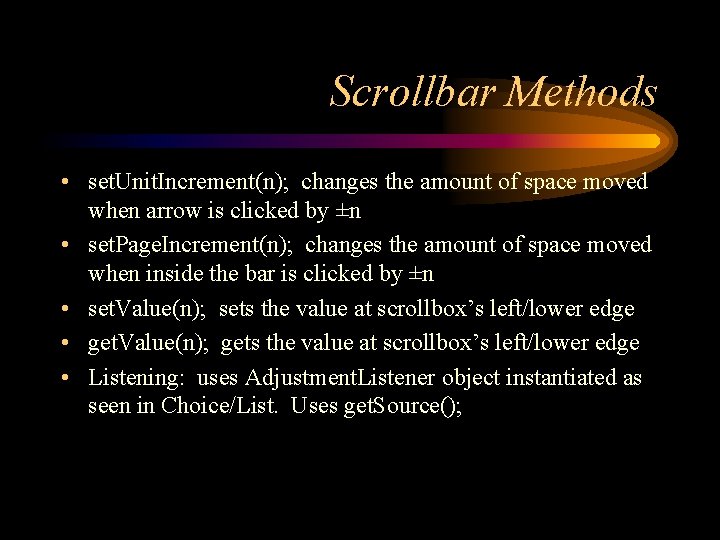
Scrollbar Methods • set. Unit. Increment(n); changes the amount of space moved when arrow is clicked by ±n • set. Page. Increment(n); changes the amount of space moved when inside the bar is clicked by ±n • set. Value(n); sets the value at scrollbox’s left/lower edge • get. Value(n); gets the value at scrollbox’s left/lower edge • Listening: uses Adjustment. Listener object instantiated as seen in Choice/List. Uses get. Source();
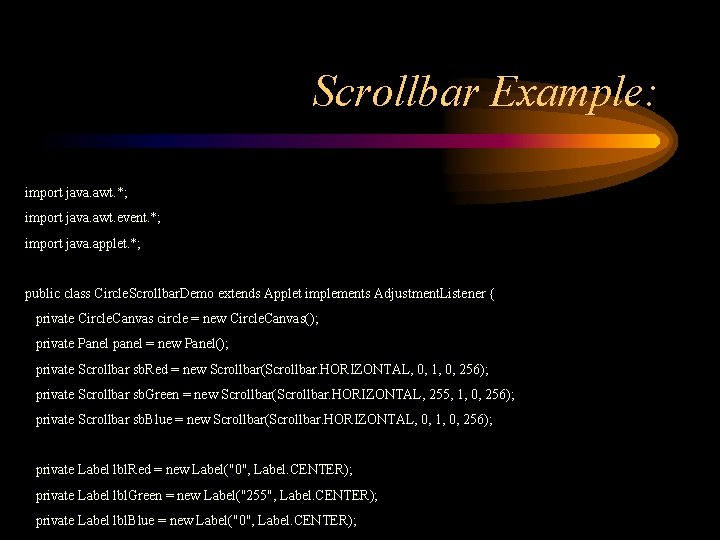
Scrollbar Example: import java. awt. *; import java. awt. event. *; import java. applet. *; public class Circle. Scrollbar. Demo extends Applet implements Adjustment. Listener { private Circle. Canvas circle = new Circle. Canvas(); private Panel panel = new Panel(); private Scrollbar sb. Red = new Scrollbar(Scrollbar. HORIZONTAL, 0, 1, 0, 256); private Scrollbar sb. Green = new Scrollbar(Scrollbar. HORIZONTAL, 255, 1, 0, 256); private Scrollbar sb. Blue = new Scrollbar(Scrollbar. HORIZONTAL, 0, 1, 0, 256); private Label lbl. Red = new Label("0", Label. CENTER); private Label lbl. Green = new Label("255", Label. CENTER); private Label lbl. Blue = new Label("0", Label. CENTER);
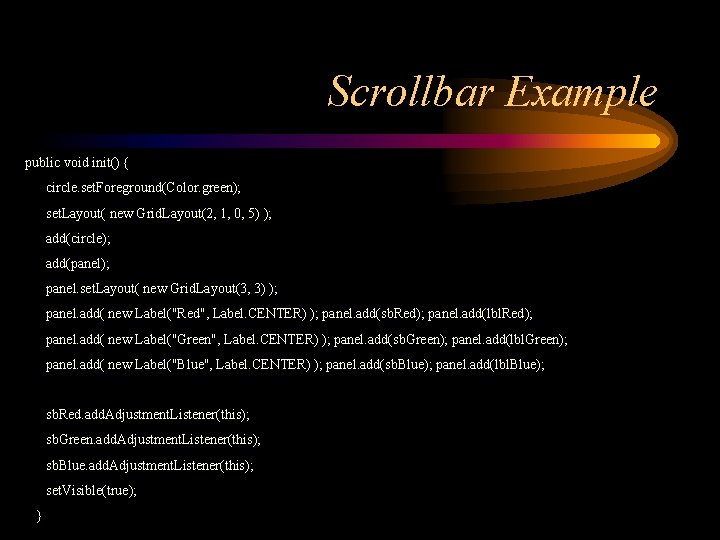
Scrollbar Example public void init() { circle. set. Foreground(Color. green); set. Layout( new Grid. Layout(2, 1, 0, 5) ); add(circle); add(panel); panel. set. Layout( new Grid. Layout(3, 3) ); panel. add( new Label("Red", Label. CENTER) ); panel. add(sb. Red); panel. add(lbl. Red); panel. add( new Label("Green", Label. CENTER) ); panel. add(sb. Green); panel. add(lbl. Green); panel. add( new Label("Blue", Label. CENTER) ); panel. add(sb. Blue); panel. add(lbl. Blue); sb. Red. add. Adjustment. Listener(this); sb. Green. add. Adjustment. Listener(this); sb. Blue. add. Adjustment. Listener(this); set. Visible(true); }
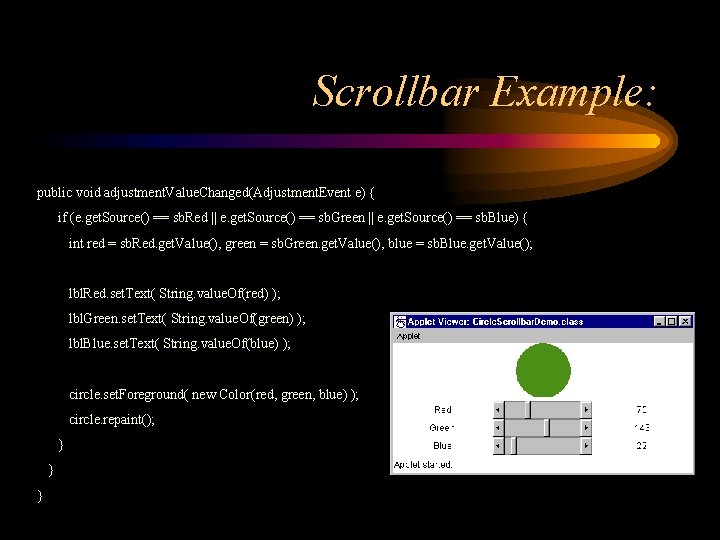
Scrollbar Example: public void adjustment. Value. Changed(Adjustment. Event e) { if (e. get. Source() == sb. Red || e. get. Source() == sb. Green || e. get. Source() == sb. Blue) { int red = sb. Red. get. Value(), green = sb. Green. get. Value(), blue = sb. Blue. get. Value(); lbl. Red. set. Text( String. value. Of(red) ); lbl. Green. set. Text( String. value. Of(green) ); lbl. Blue. set. Text( String. value. Of(blue) ); circle. set. Foreground( new Color(red, green, blue) ); circle. repaint(); } } }
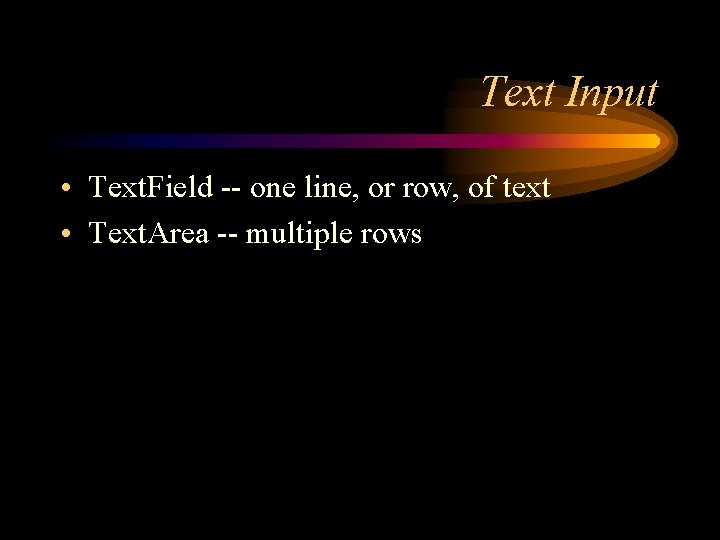
Text Input • Text. Field -- one line, or row, of text • Text. Area -- multiple rows
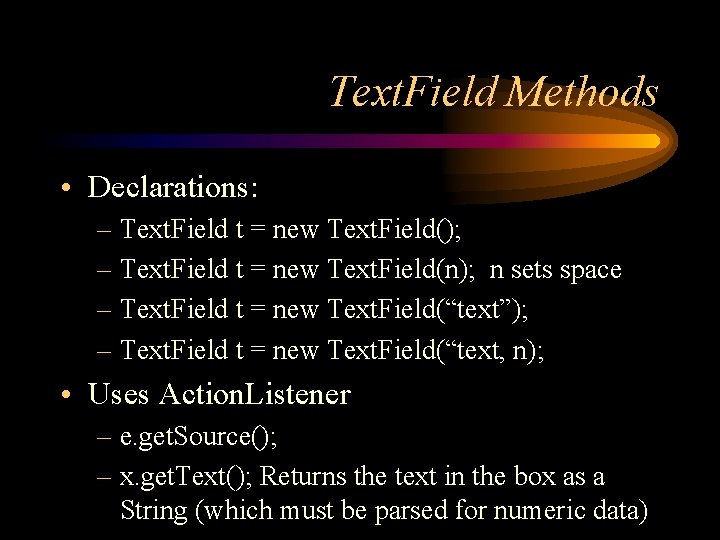
Text. Field Methods • Declarations: – Text. Field t = new Text. Field(); – Text. Field t = new Text. Field(n); n sets space – Text. Field t = new Text. Field(“text”); – Text. Field t = new Text. Field(“text, n); • Uses Action. Listener – e. get. Source(); – x. get. Text(); Returns the text in the box as a String (which must be parsed for numeric data)
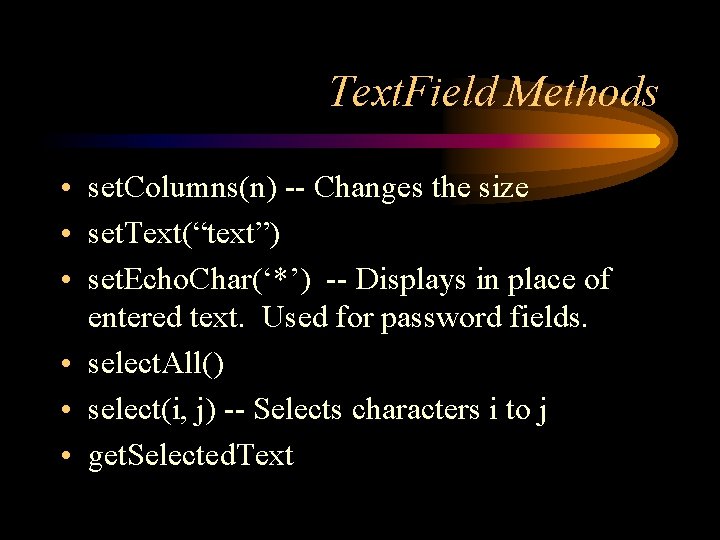
Text. Field Methods • set. Columns(n) -- Changes the size • set. Text(“text”) • set. Echo. Char(‘*’) -- Displays in place of entered text. Used for password fields. • select. All() • select(i, j) -- Selects characters i to j • get. Selected. Text
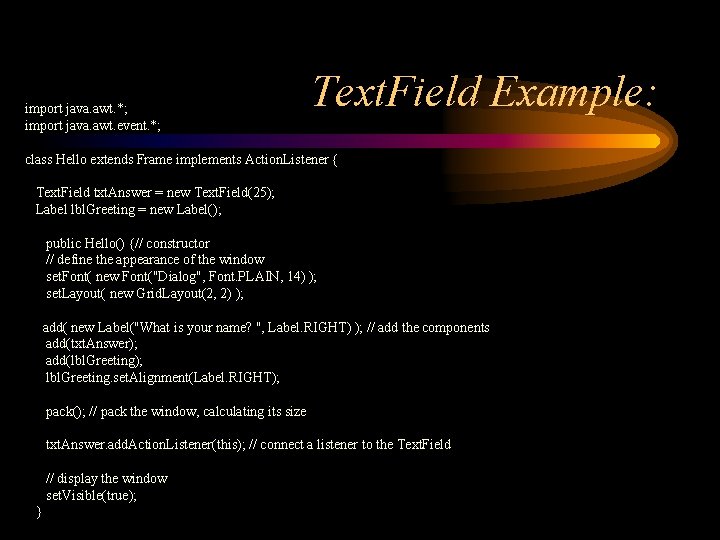
import java. awt. *; import java. awt. event. *; Text. Field Example: class Hello extends Frame implements Action. Listener { Text. Field txt. Answer = new Text. Field(25); Label lbl. Greeting = new Label(); public Hello() {// constructor // define the appearance of the window set. Font( new Font("Dialog", Font. PLAIN, 14) ); set. Layout( new Grid. Layout(2, 2) ); add( new Label("What is your name? ", Label. RIGHT) ); // add the components add(txt. Answer); add(lbl. Greeting); lbl. Greeting. set. Alignment(Label. RIGHT); pack(); // pack the window, calculating its size txt. Answer. add. Action. Listener(this); // connect a listener to the Text. Field // display the window set. Visible(true); }
![Text Field Example public static void mainString arg Hello h new Hello Text. Field Example: public static void main(String[] arg) { Hello h = new Hello();](https://slidetodoc.com/presentation_image/21468fe18b2ebd0c47c5b9b0c8764bcb/image-52.jpg)
Text. Field Example: public static void main(String[] arg) { Hello h = new Hello(); } // listeners public void action. Performed(Action. Event e) { // this method is called when an event occurs if (e. get. Source() == txt. Answer) { String name = txt. Answer. get. Text(); lbl. Greeting. set. Text("Welcome " + name + "!"); } } }
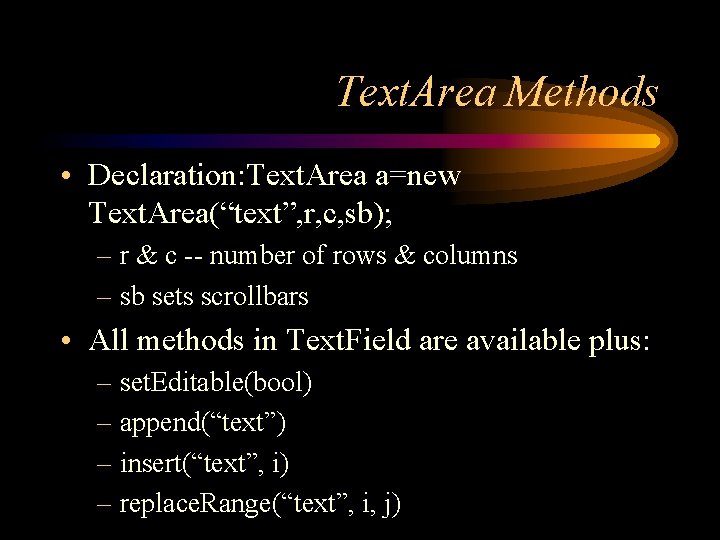
Text. Area Methods • Declaration: Text. Area a=new Text. Area(“text”, r, c, sb); – r & c -- number of rows & columns – sb sets scrollbars • All methods in Text. Field are available plus: – set. Editable(bool) – append(“text”) – insert(“text”, i) – replace. Range(“text”, i, j)
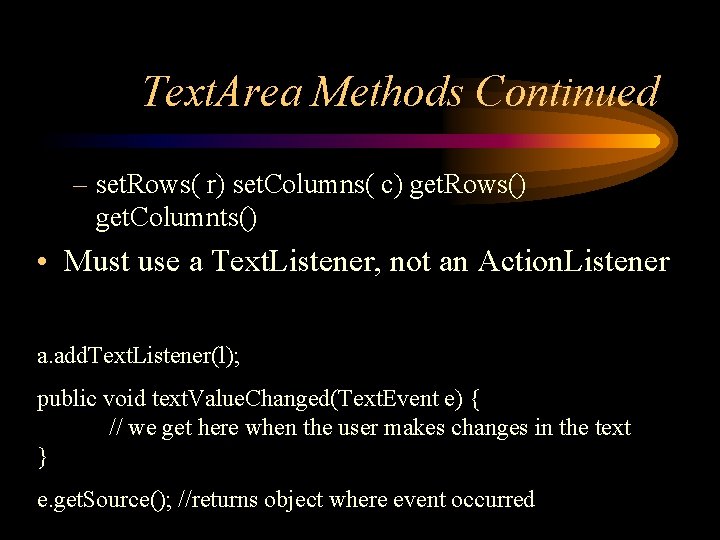
Text. Area Methods Continued – set. Rows( r) set. Columns( c) get. Rows() get. Columnts() • Must use a Text. Listener, not an Action. Listener a. add. Text. Listener(l); public void text. Value. Changed(Text. Event e) { // we get here when the user makes changes in the text } e. get. Source(); //returns object where event occurred
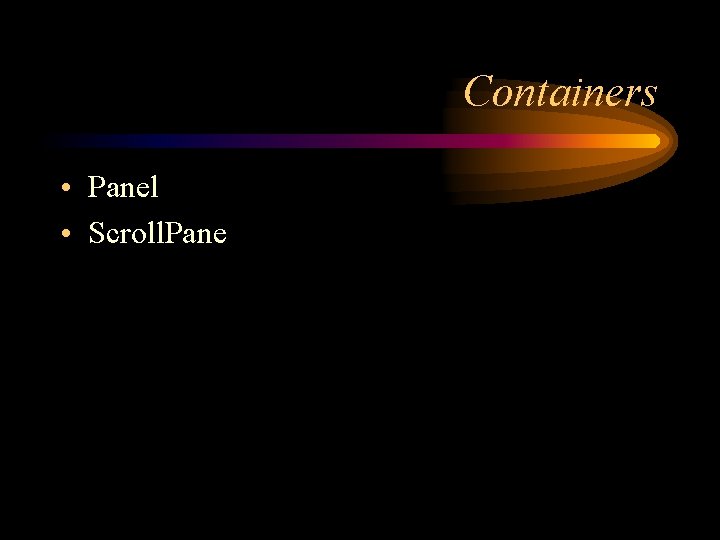
Containers • Panel • Scroll. Pane
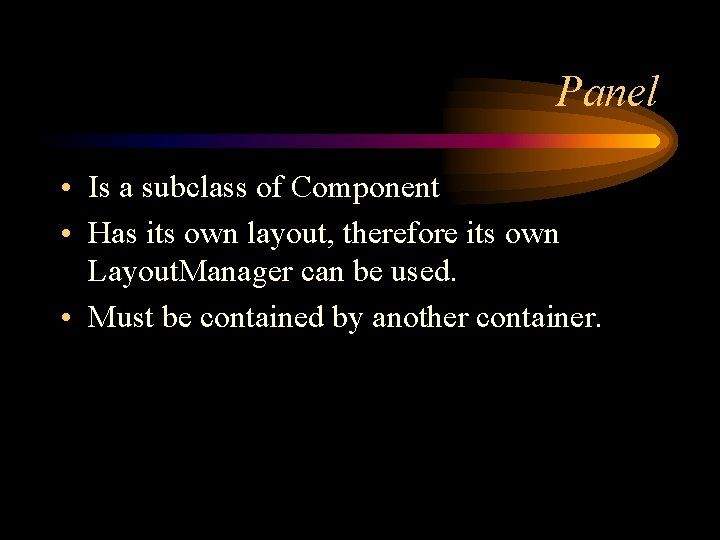
Panel • Is a subclass of Component • Has its own layout, therefore its own Layout. Manager can be used. • Must be contained by another container.
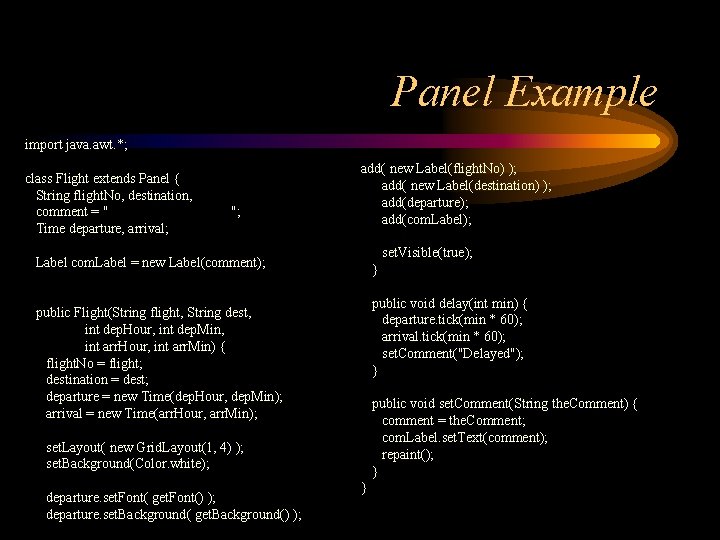
Panel Example import java. awt. *; class Flight extends Panel { String flight. No, destination, comment = " Time departure, arrival; "; add( new Label(flight. No) ); add( new Label(destination) ); add(departure); add(com. Label); set. Visible(true); Label com. Label = new Label(comment); } public void delay(int min) { departure. tick(min * 60); arrival. tick(min * 60); set. Comment("Delayed"); } public Flight(String flight, String dest, int dep. Hour, int dep. Min, int arr. Hour, int arr. Min) { flight. No = flight; destination = dest; departure = new Time(dep. Hour, dep. Min); arrival = new Time(arr. Hour, arr. Min); public void set. Comment(String the. Comment) { comment = the. Comment; com. Label. set. Text(comment); repaint(); } set. Layout( new Grid. Layout(1, 4) ); set. Background(Color. white); departure. set. Font( get. Font() ); departure. set. Background( get. Background() ); }
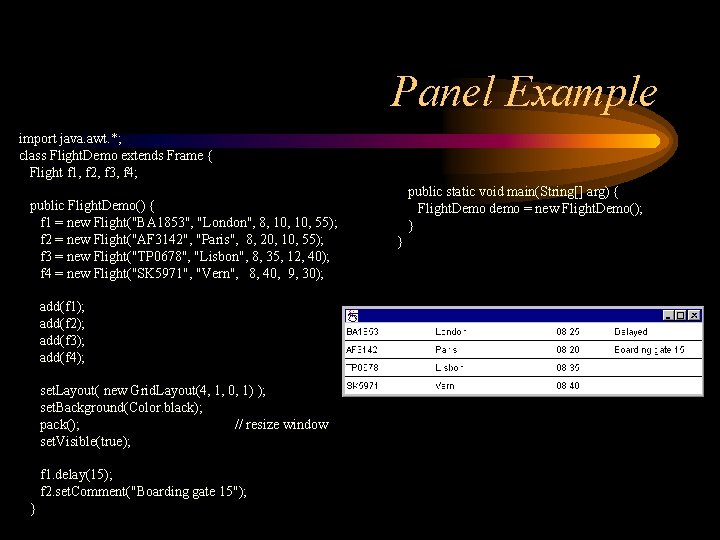
Panel Example import java. awt. *; class Flight. Demo extends Frame { Flight f 1, f 2, f 3, f 4; public Flight. Demo() { f 1 = new Flight("BA 1853", "London", 8, 10, 55); f 2 = new Flight("AF 3142", "Paris", 8, 20, 10, 55); f 3 = new Flight("TP 0678", "Lisbon", 8, 35, 12, 40); f 4 = new Flight("SK 5971", "Vern", 8, 40, 9, 30); add(f 1); add(f 2); add(f 3); add(f 4); set. Layout( new Grid. Layout(4, 1, 0, 1) ); set. Background(Color. black); pack(); // resize window set. Visible(true); f 1. delay(15); f 2. set. Comment("Boarding gate 15"); } public static void main(String[] arg) { Flight. Demo demo = new Flight. Demo(); } }
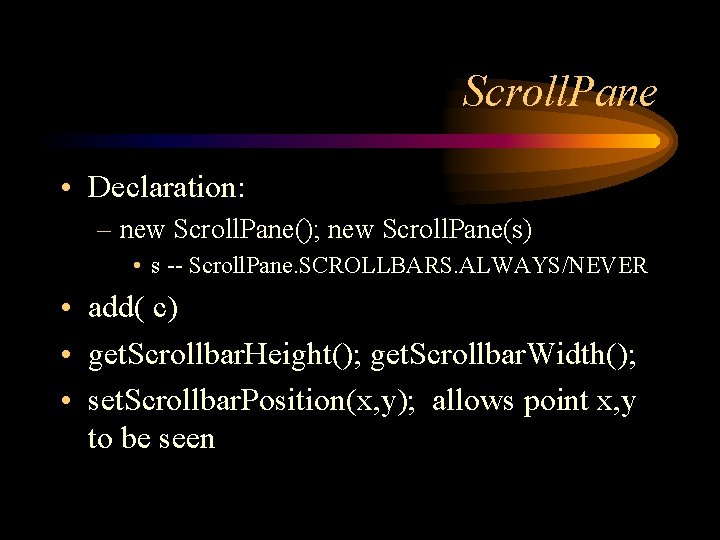
Scroll. Pane • Declaration: – new Scroll. Pane(); new Scroll. Pane(s) • s -- Scroll. Pane. SCROLLBARS. ALWAYS/NEVER • add( c) • get. Scrollbar. Height(); get. Scrollbar. Width(); • set. Scrollbar. Position(x, y); allows point x, y to be seen
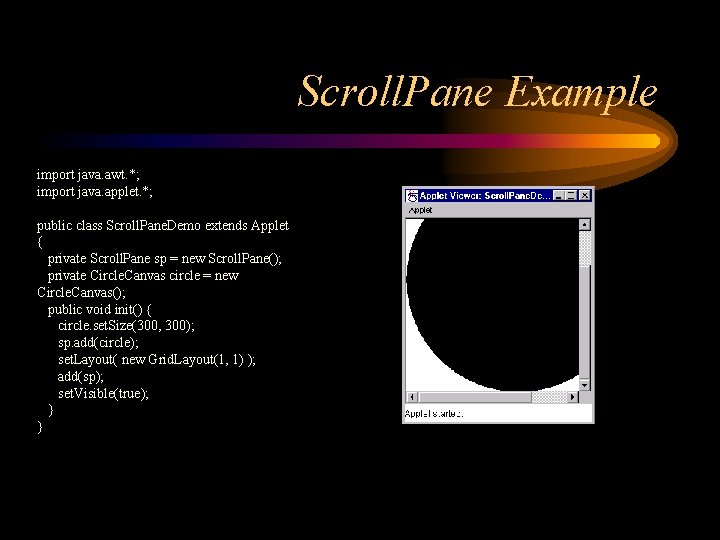
Scroll. Pane Example import java. awt. *; import java. applet. *; public class Scroll. Pane. Demo extends Applet { private Scroll. Pane sp = new Scroll. Pane(); private Circle. Canvas circle = new Circle. Canvas(); public void init() { circle. set. Size(300, 300); sp. add(circle); set. Layout( new Grid. Layout(1, 1) ); add(sp); set. Visible(true); } }