Grid World Case Study n n n The
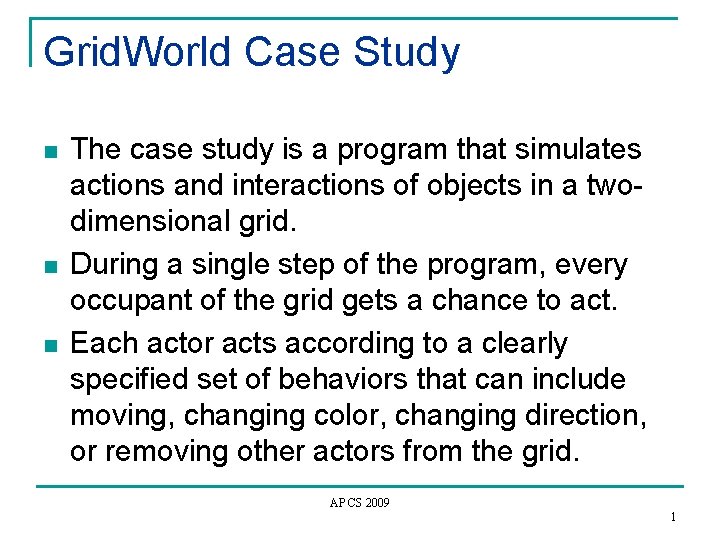
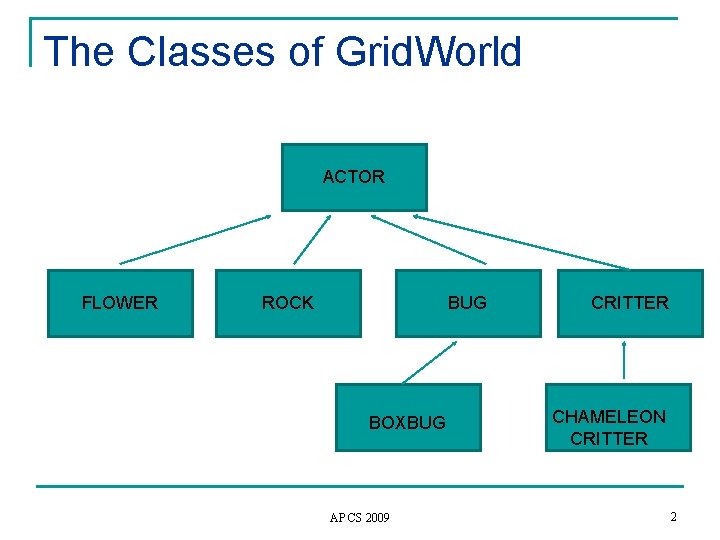
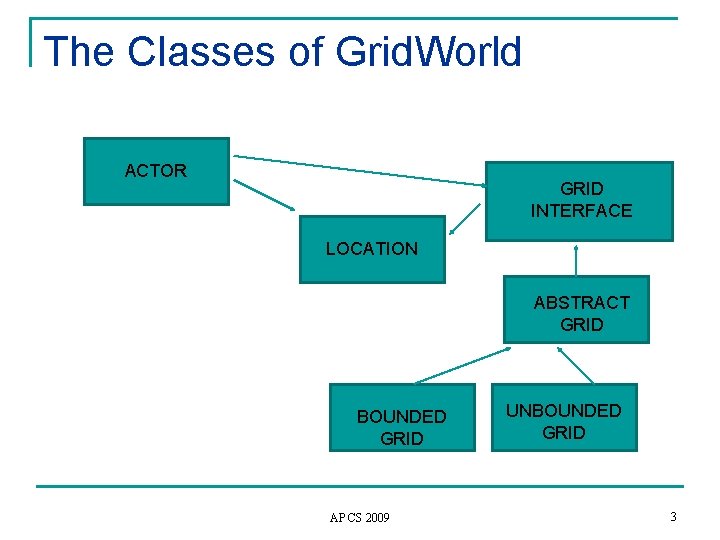
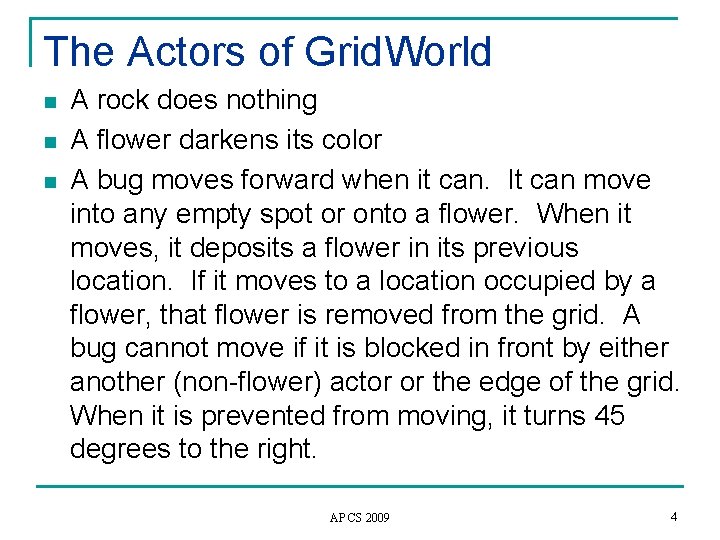
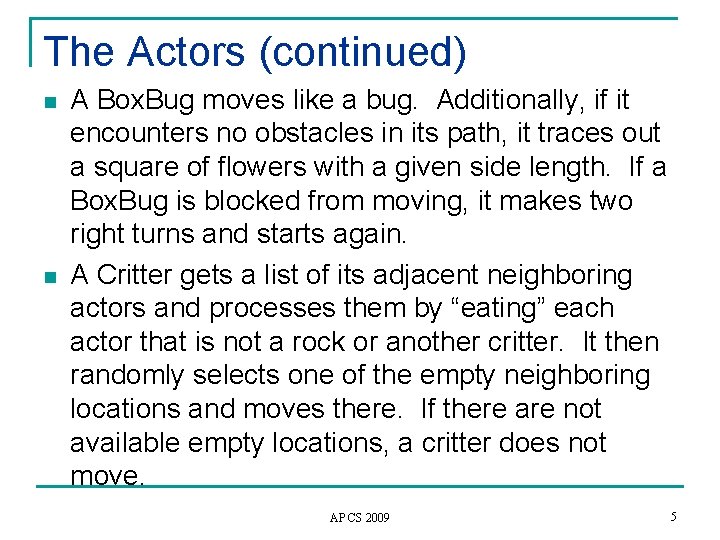
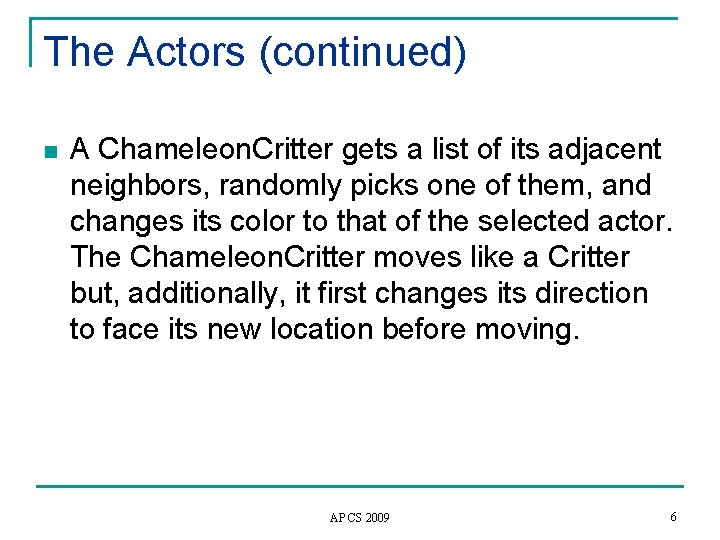
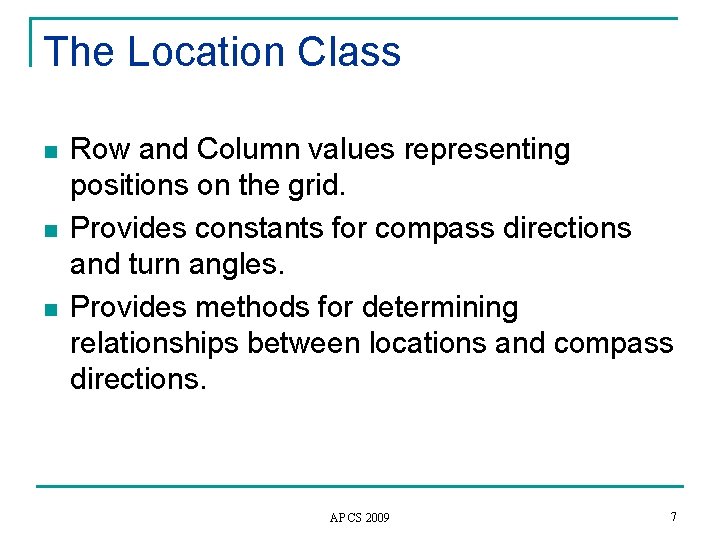
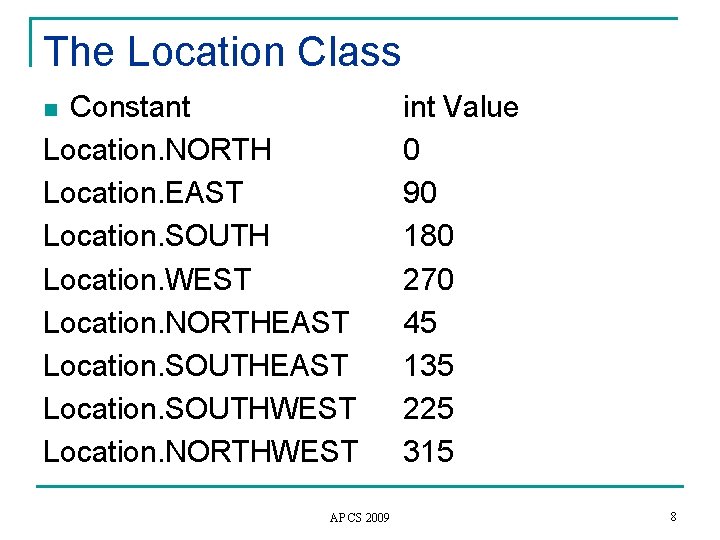
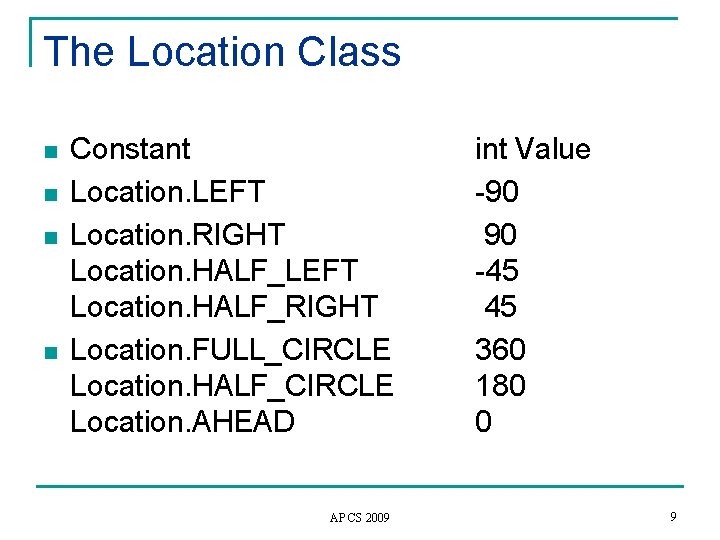
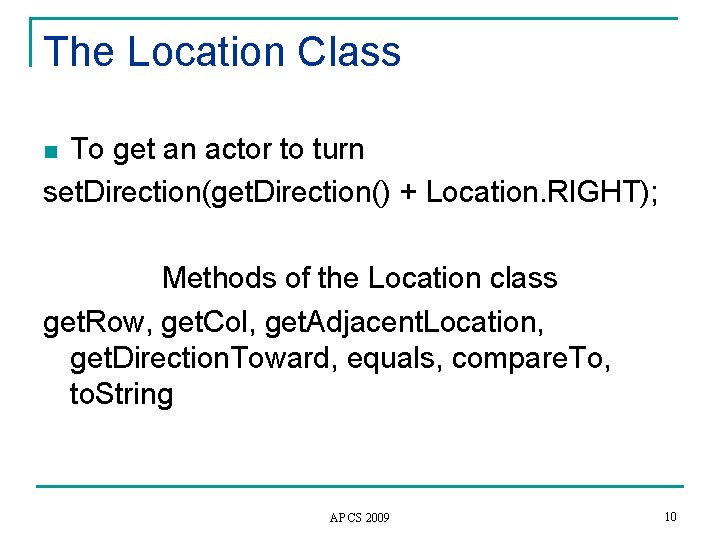
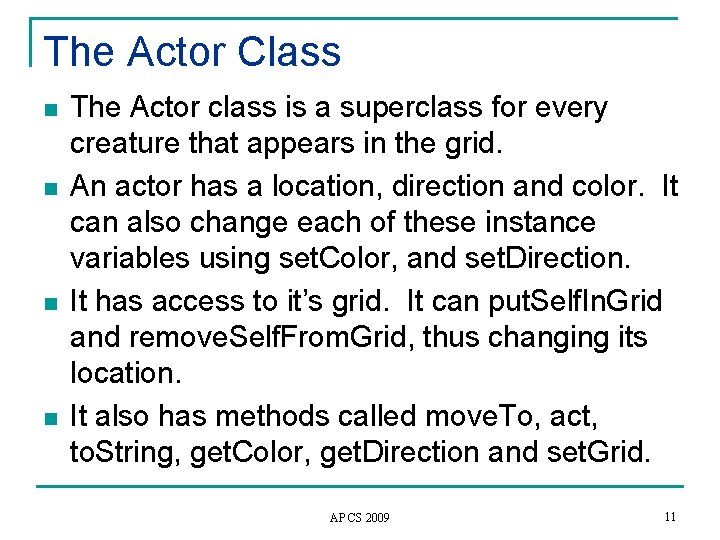
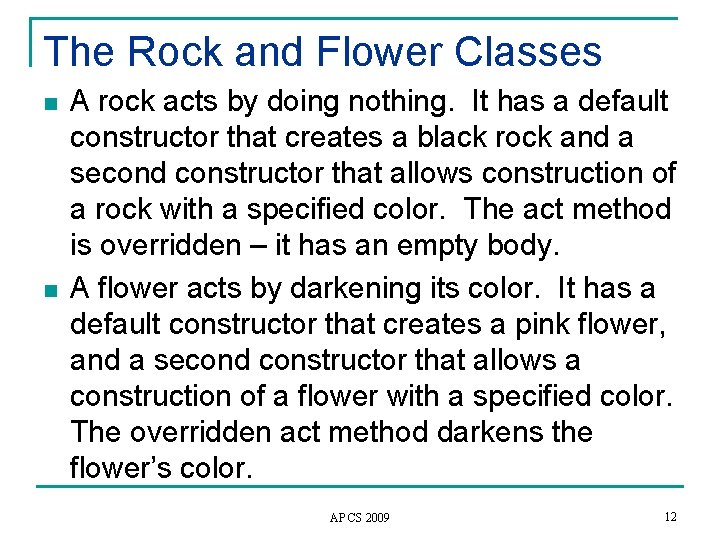
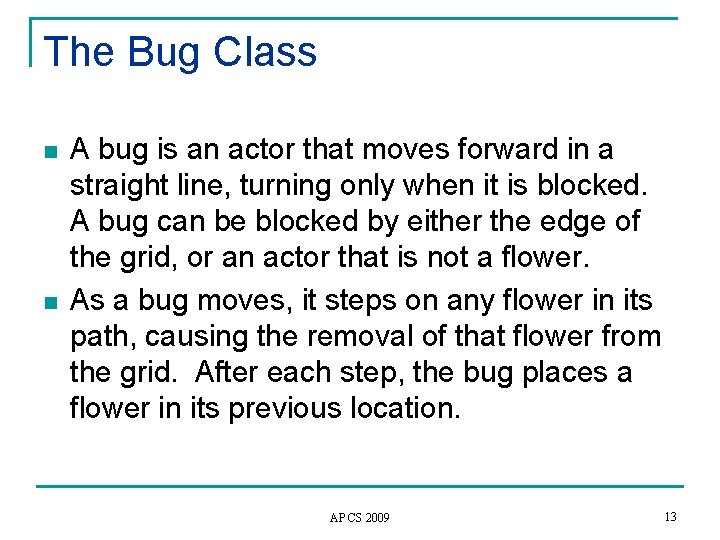
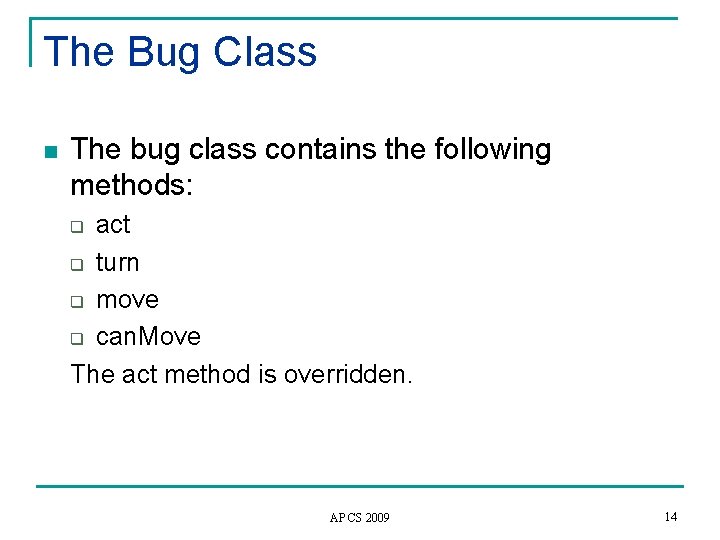
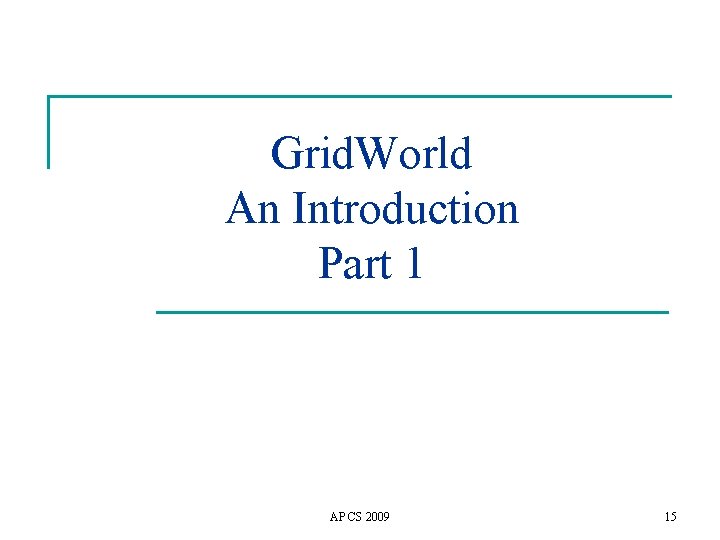
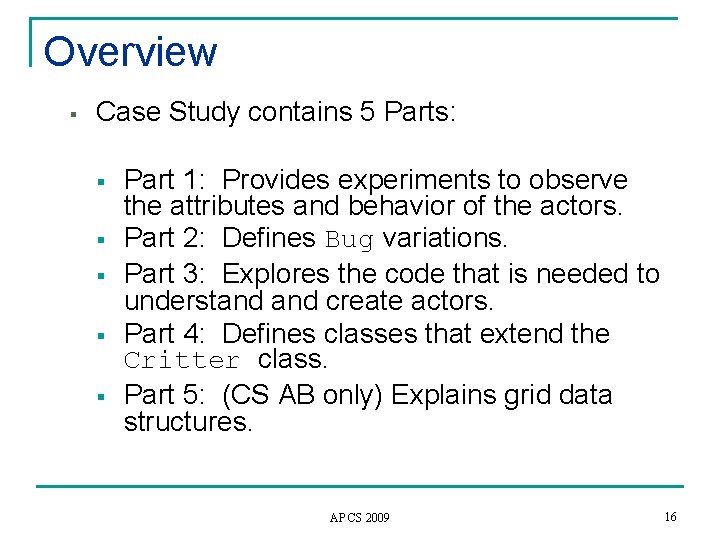
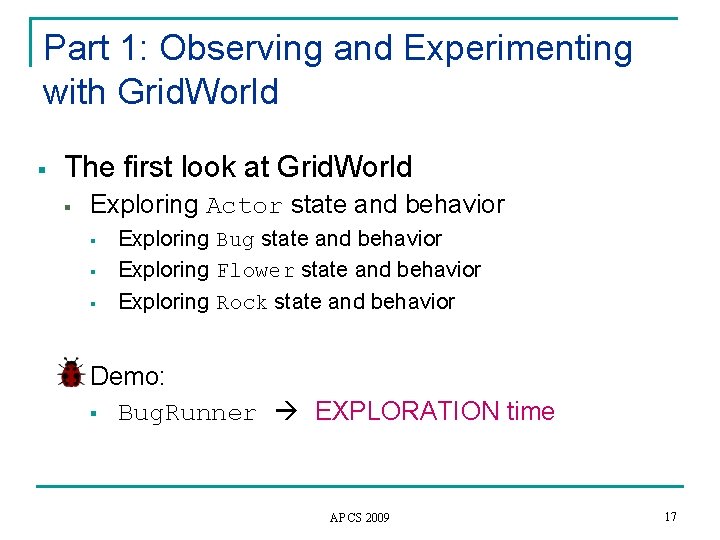
![Bug. Runner (code) public static void main(String[] args) { Actor. World world = new Bug. Runner (code) public static void main(String[] args) { Actor. World world = new](https://slidetodoc.com/presentation_image_h2/4892b38b92c4b0e6546fb34012c27c89/image-18.jpg)
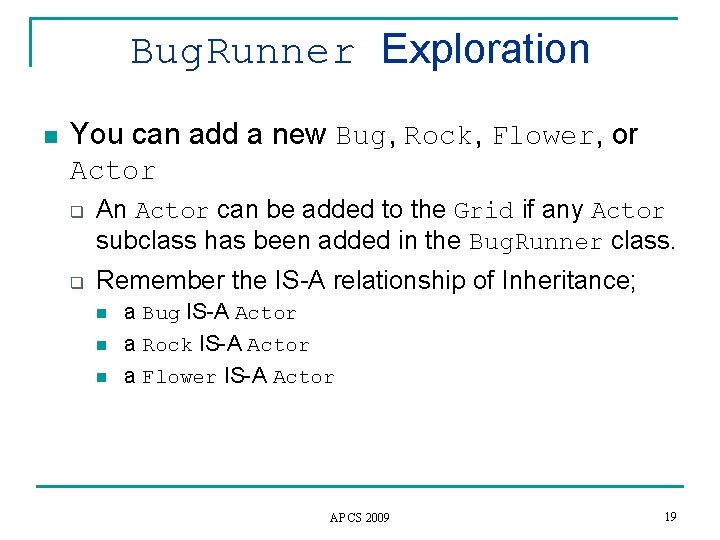
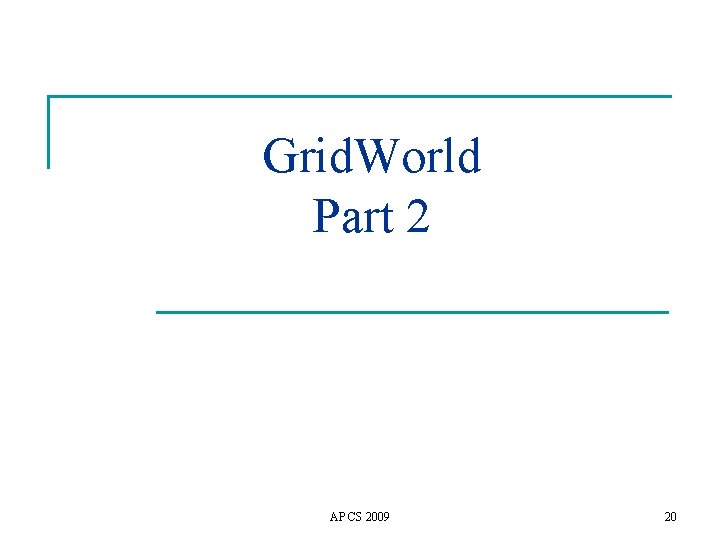
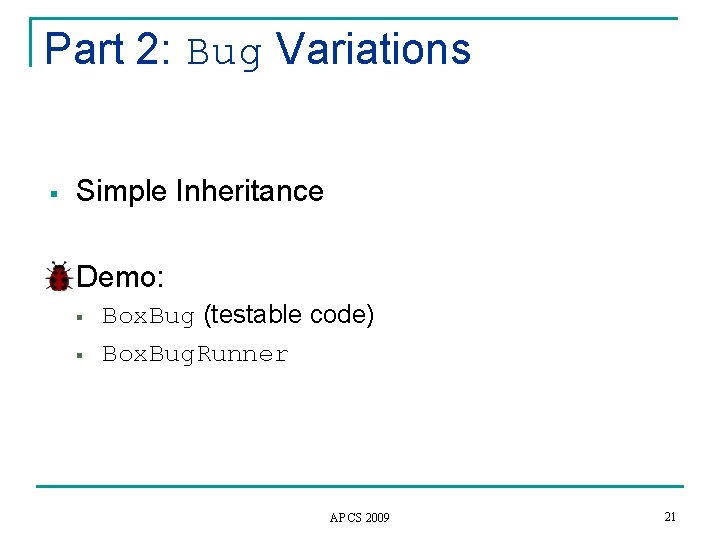
![Box. Bug. Runner public static void main(String[] args) { Actor. World world = new Box. Bug. Runner public static void main(String[] args) { Actor. World world = new](https://slidetodoc.com/presentation_image_h2/4892b38b92c4b0e6546fb34012c27c89/image-22.jpg)
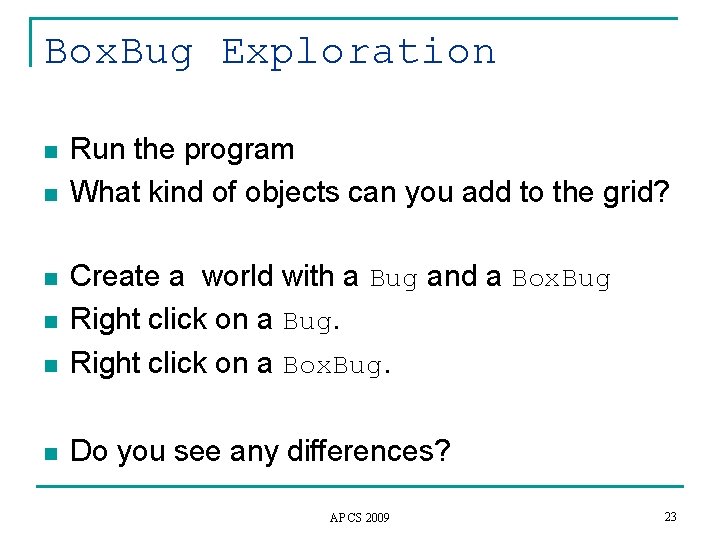
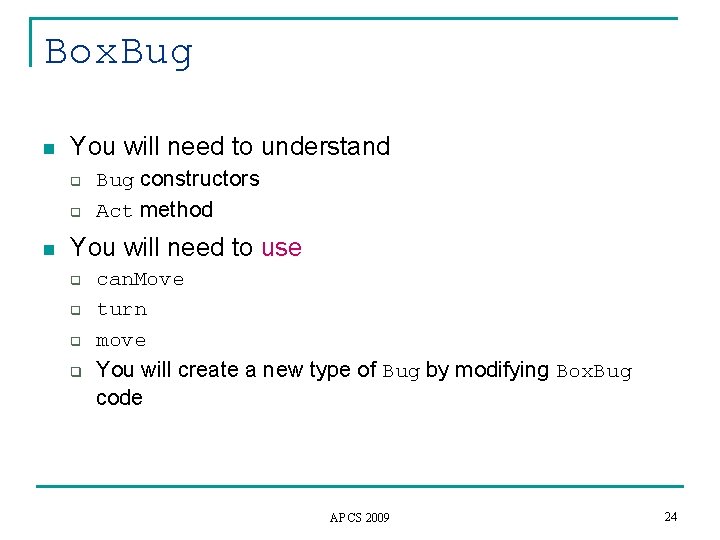
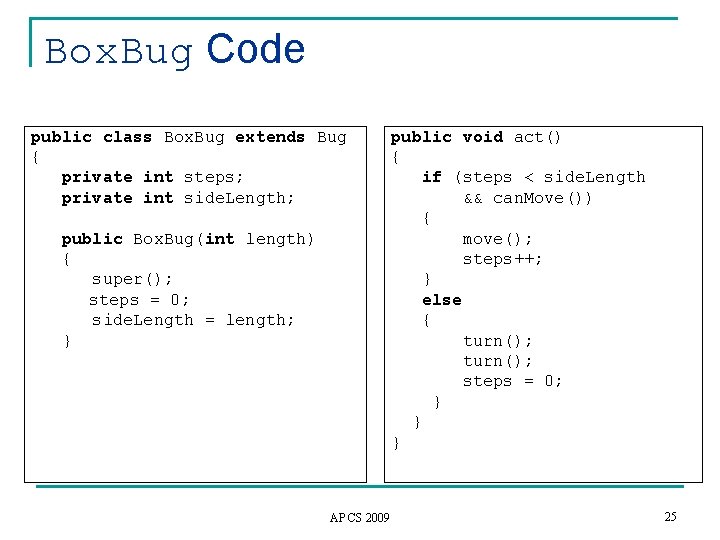
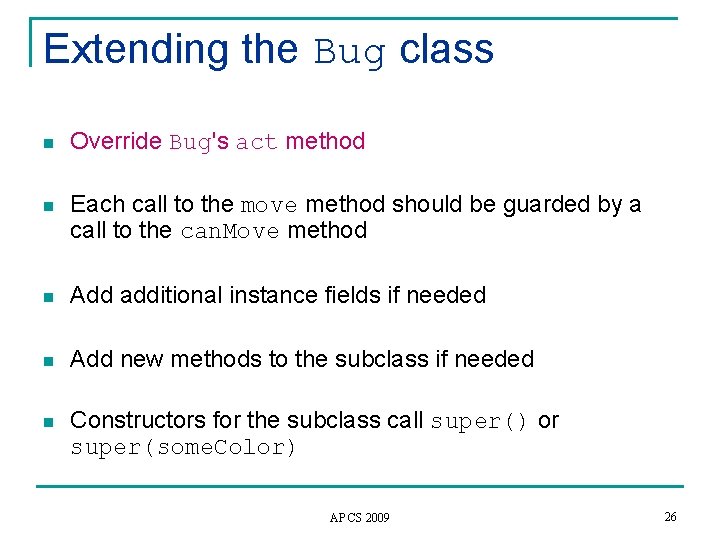
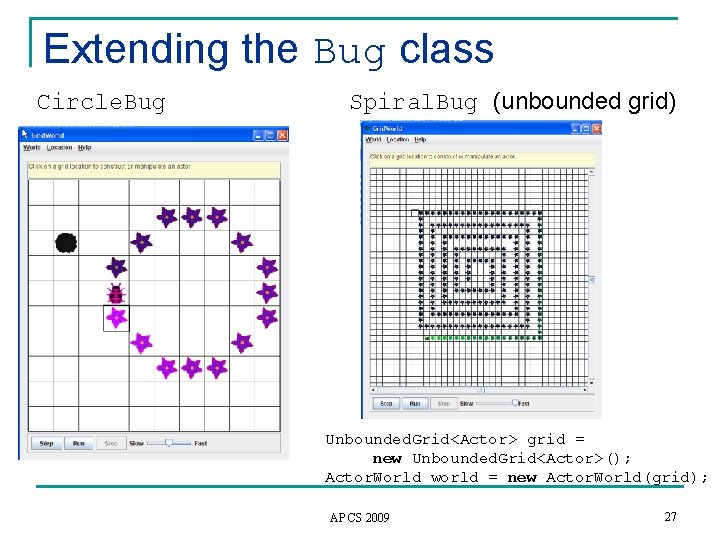
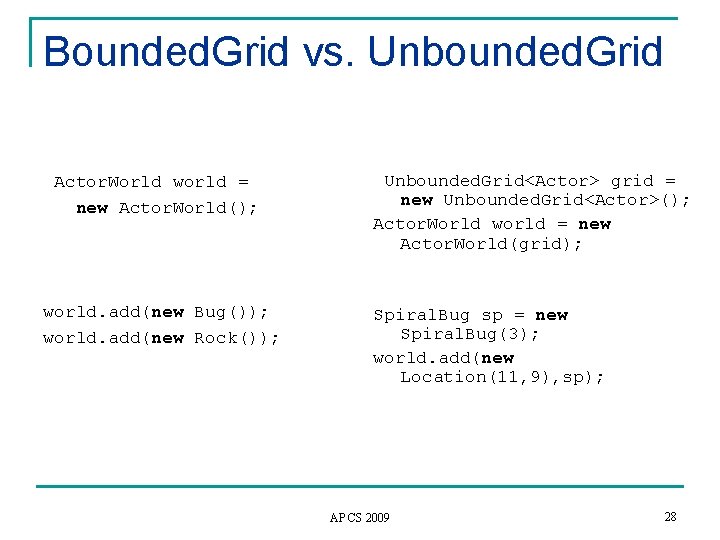
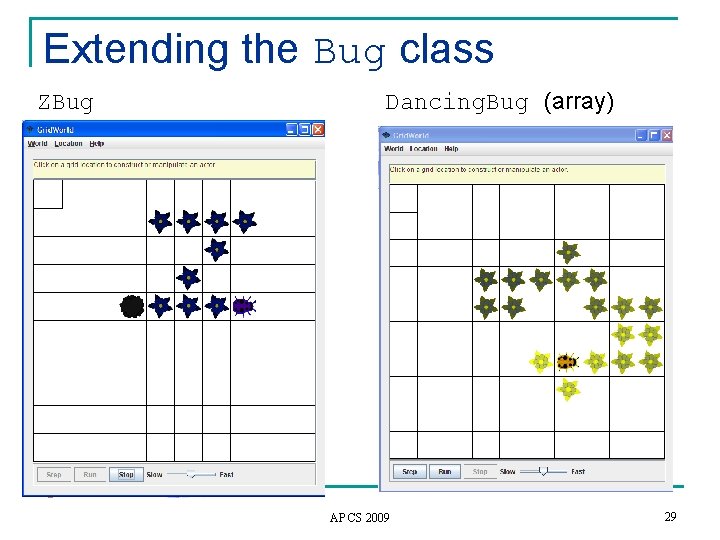
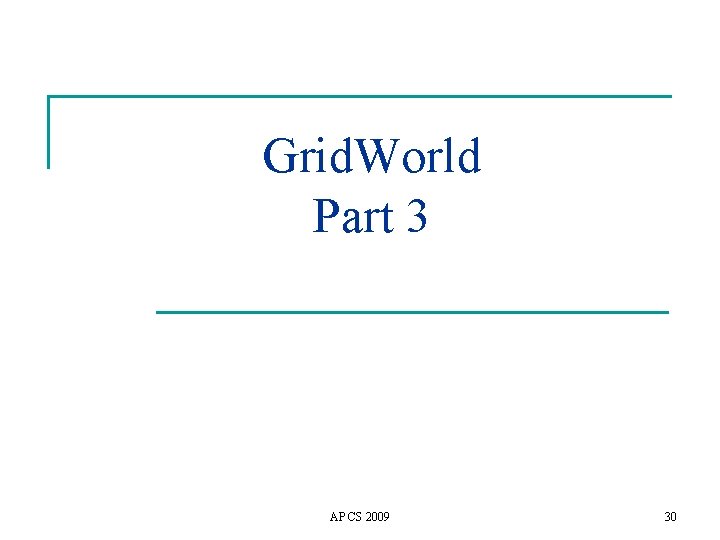
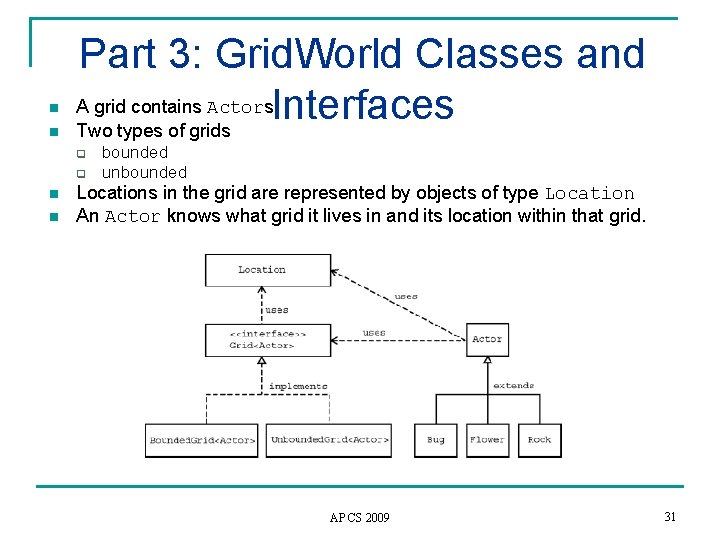
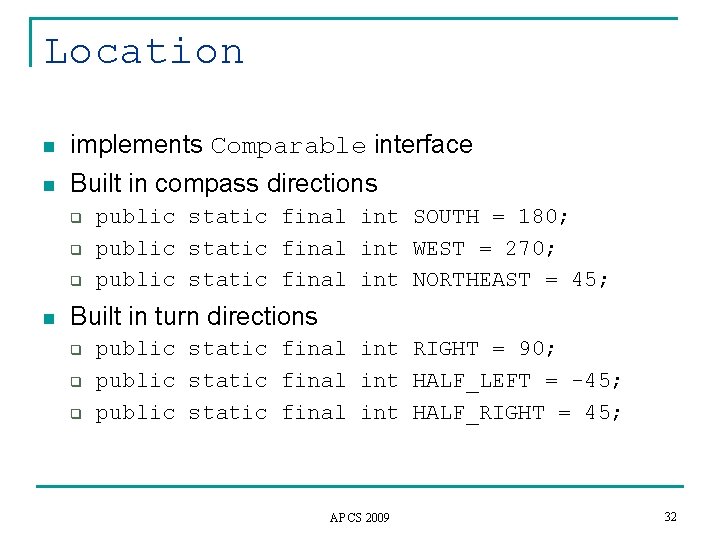
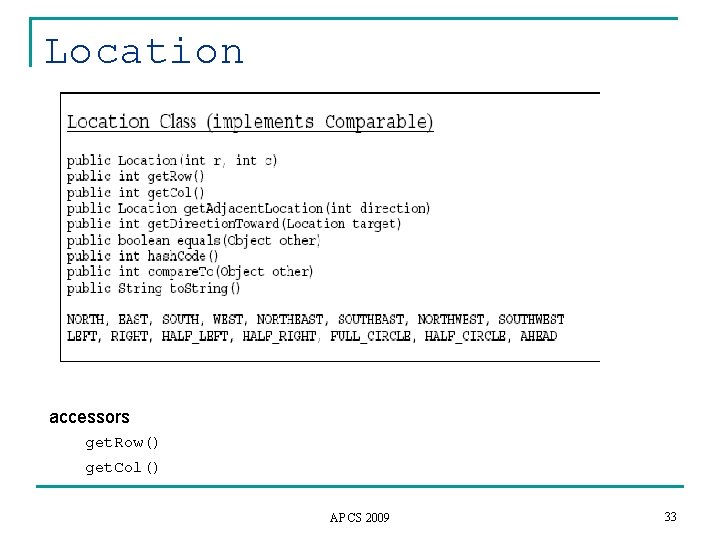
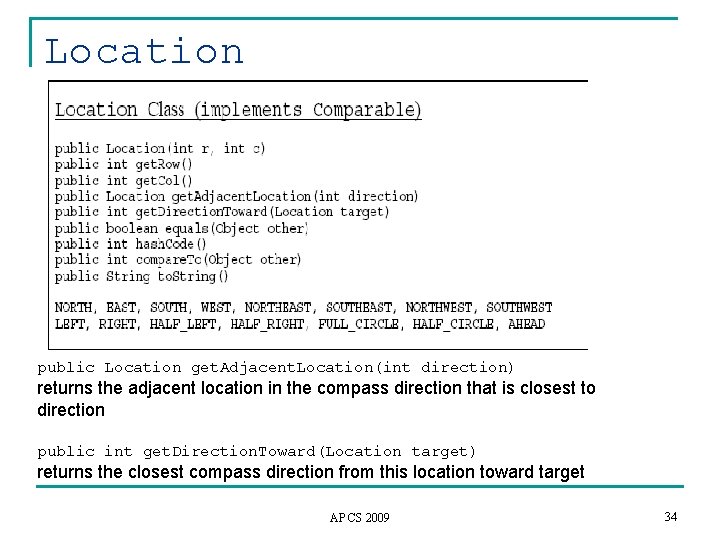
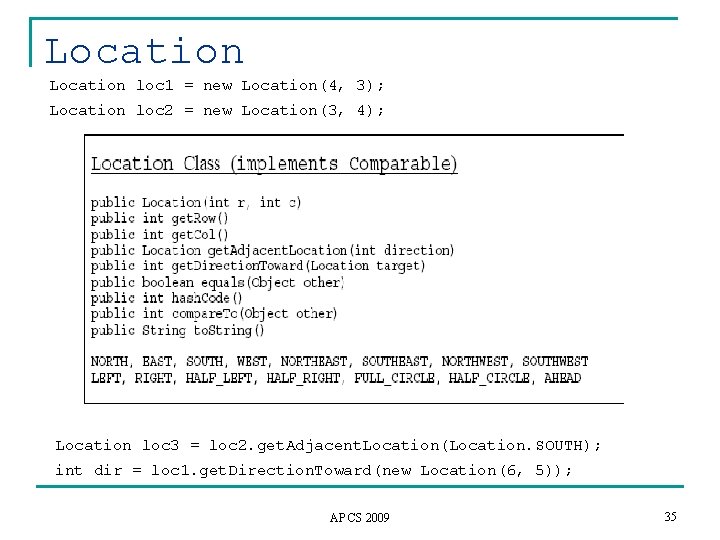
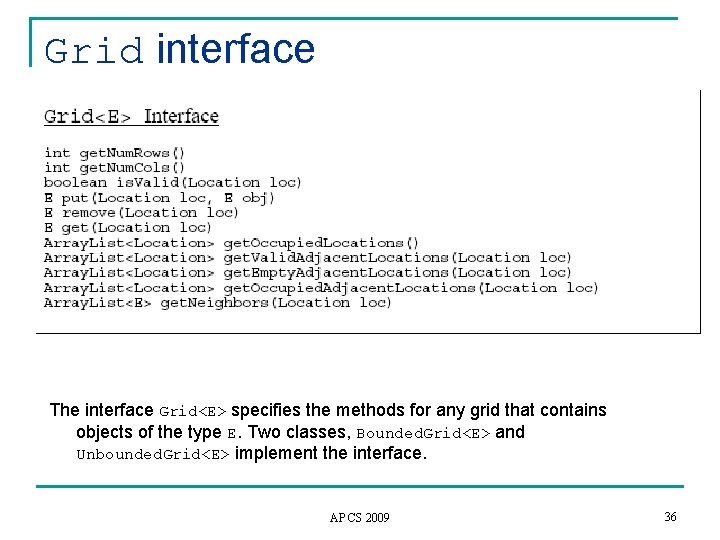
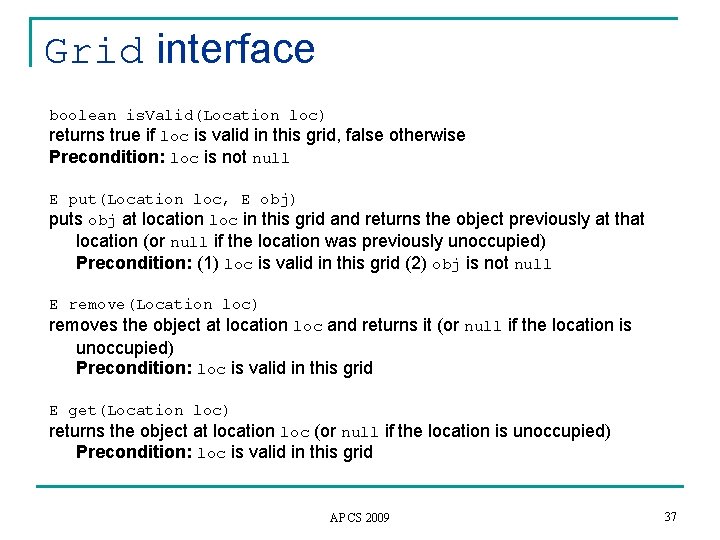
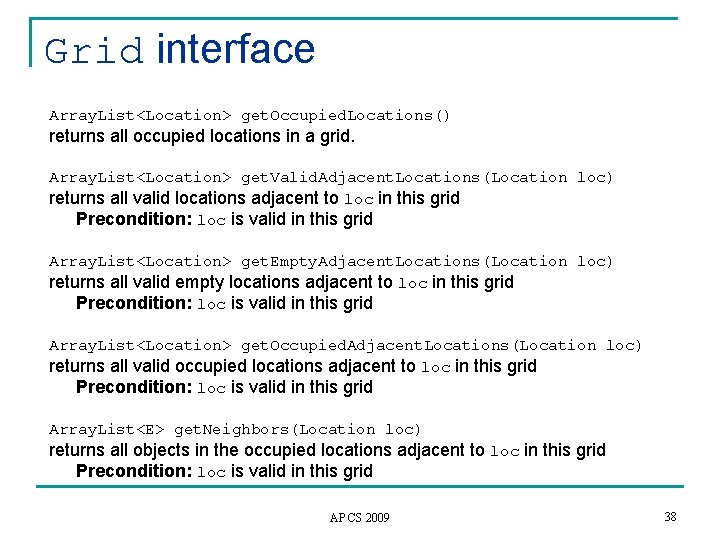
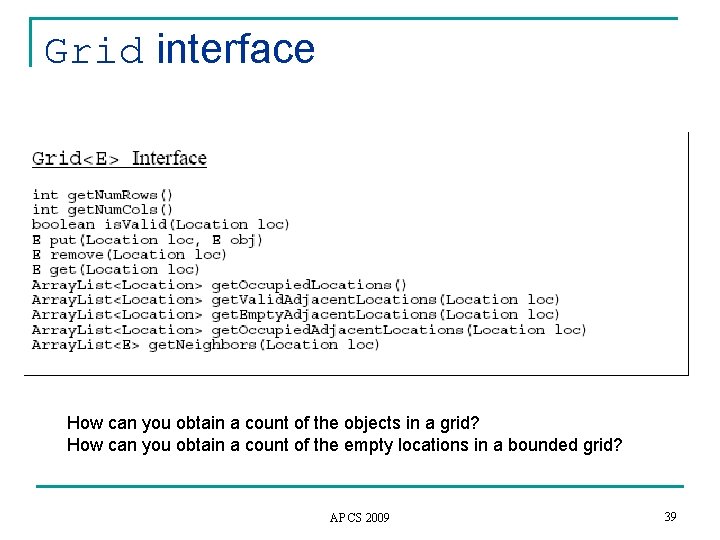
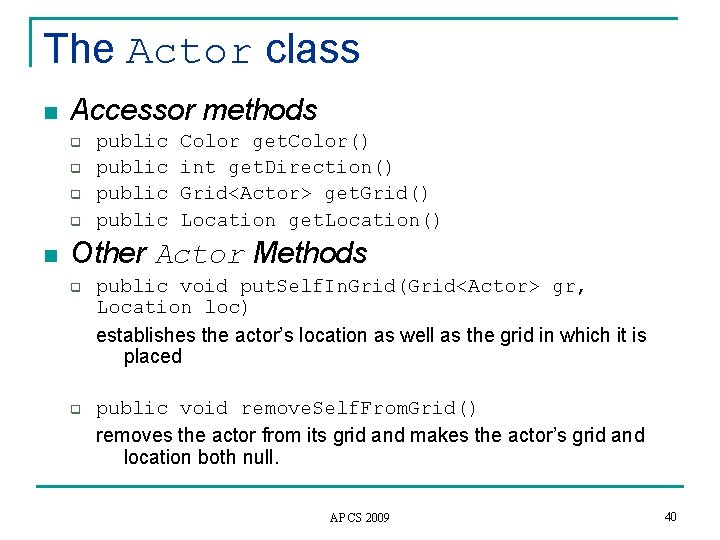
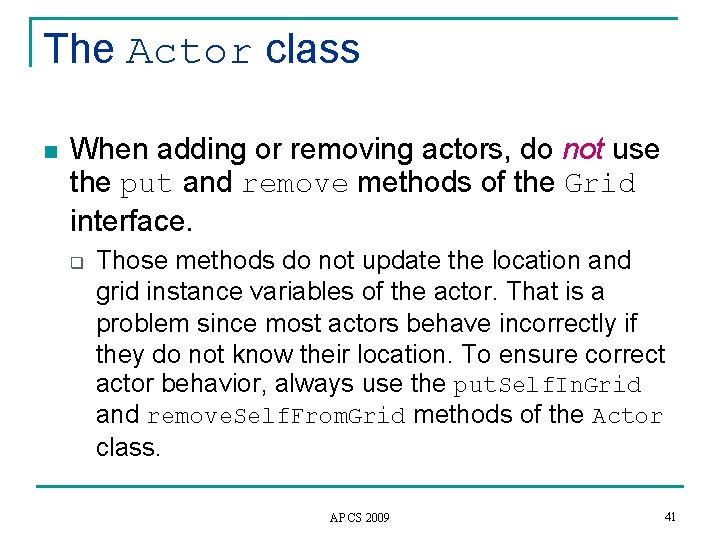
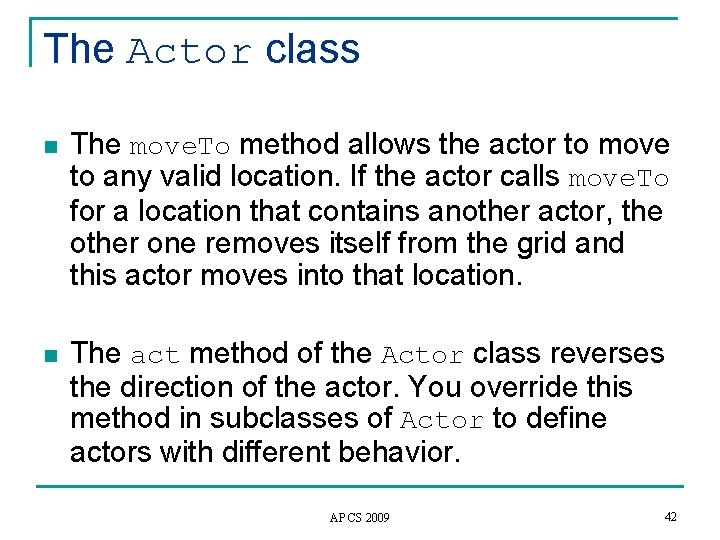
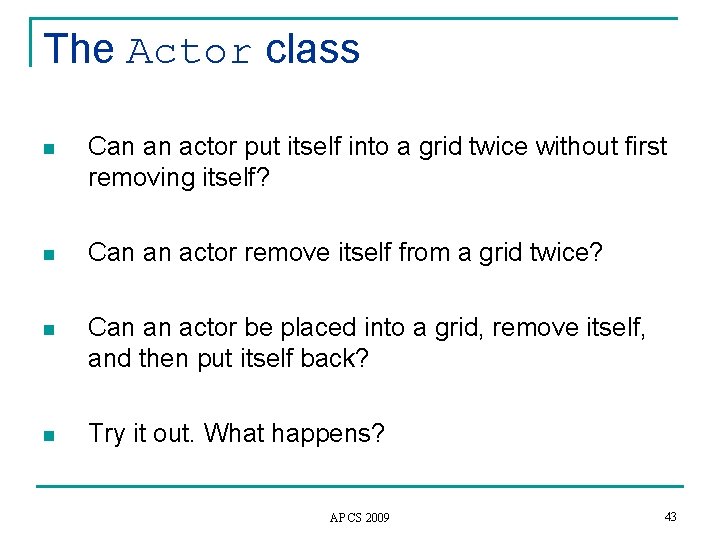
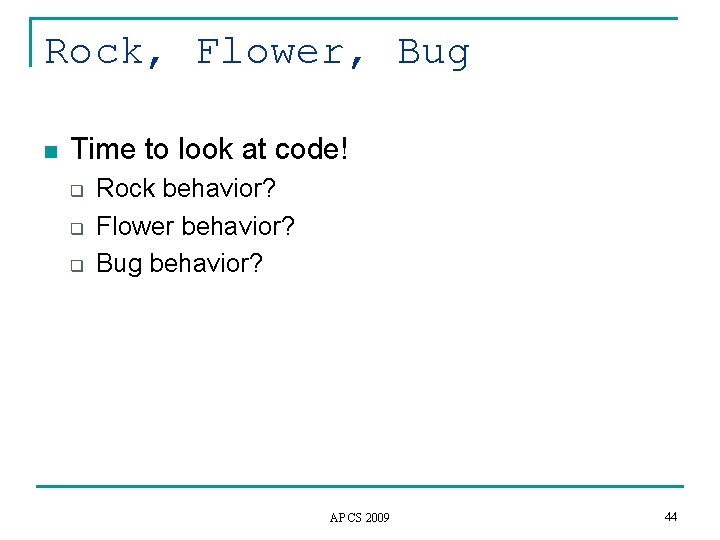
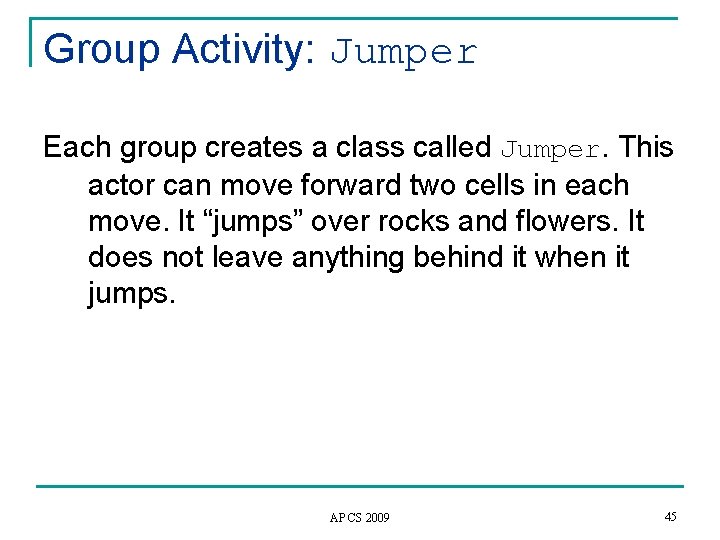
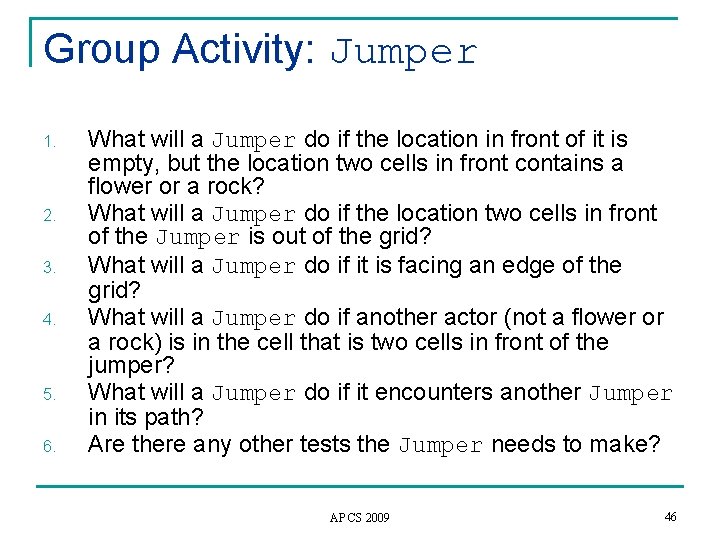
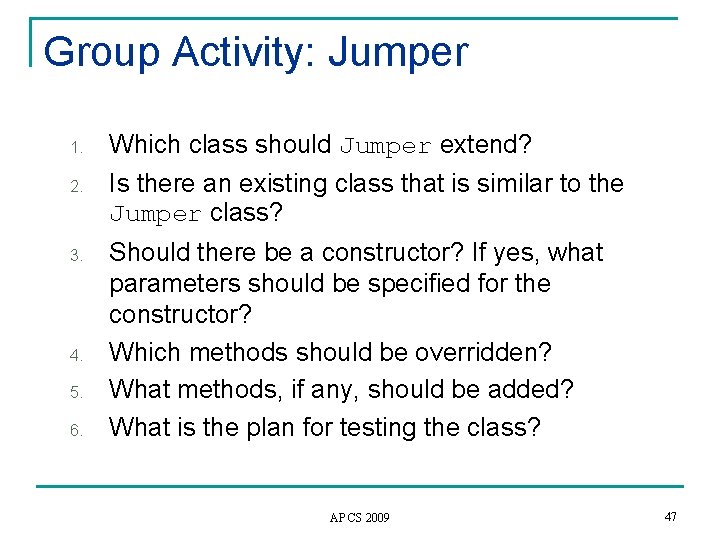
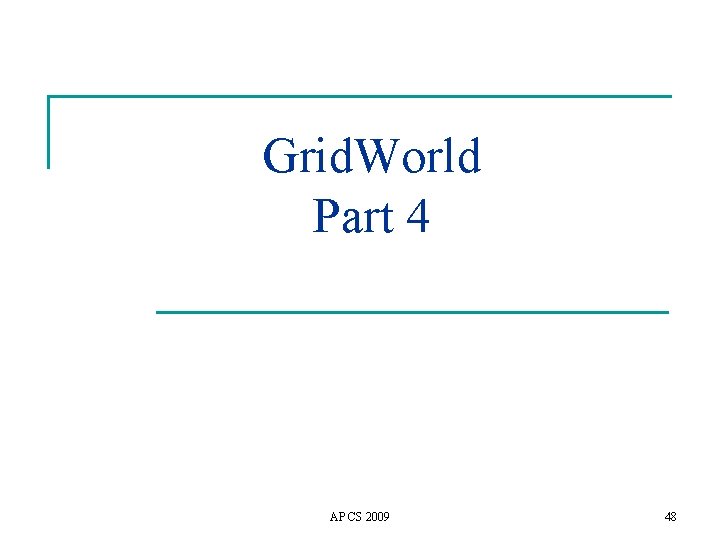
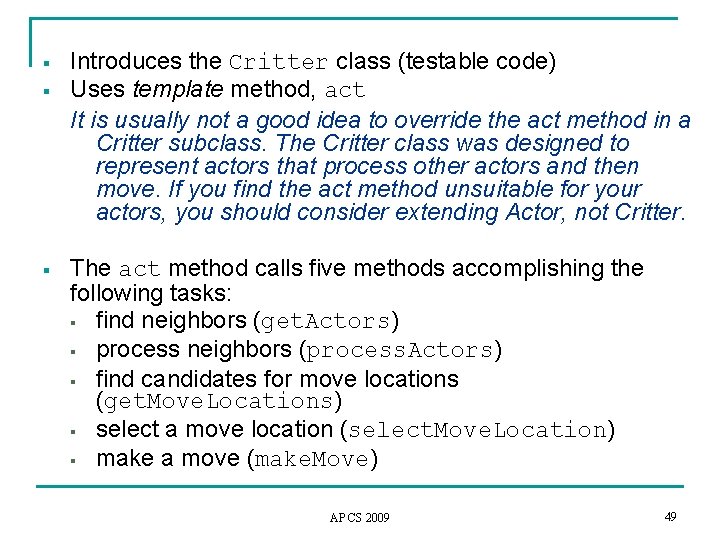
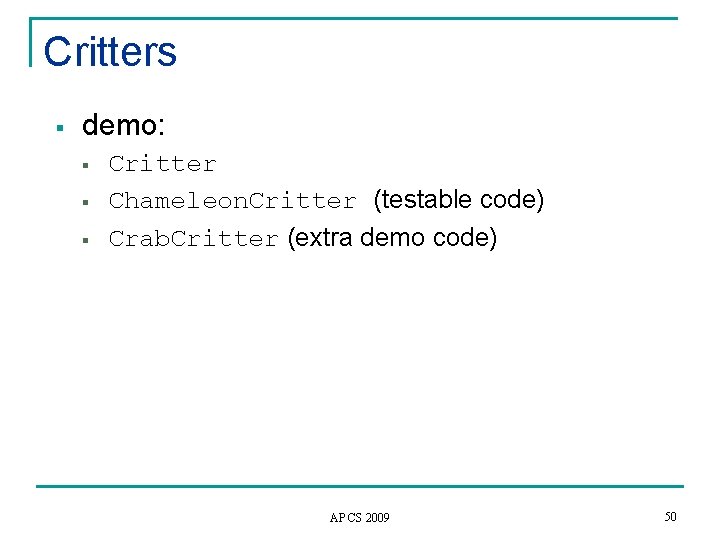
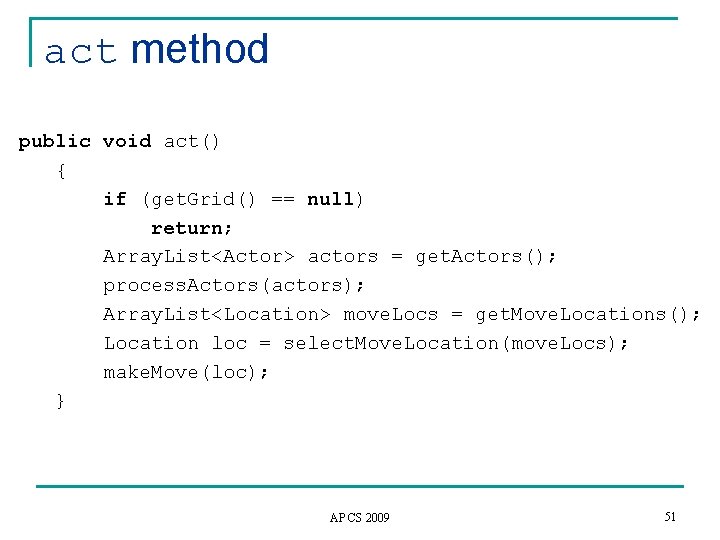
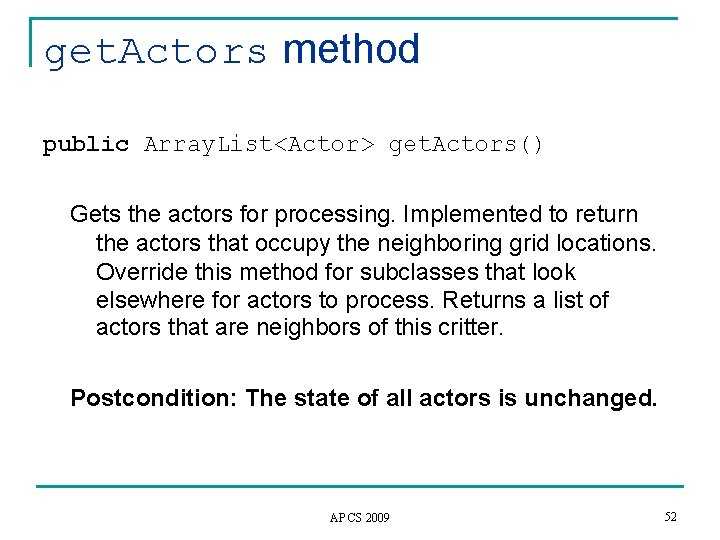
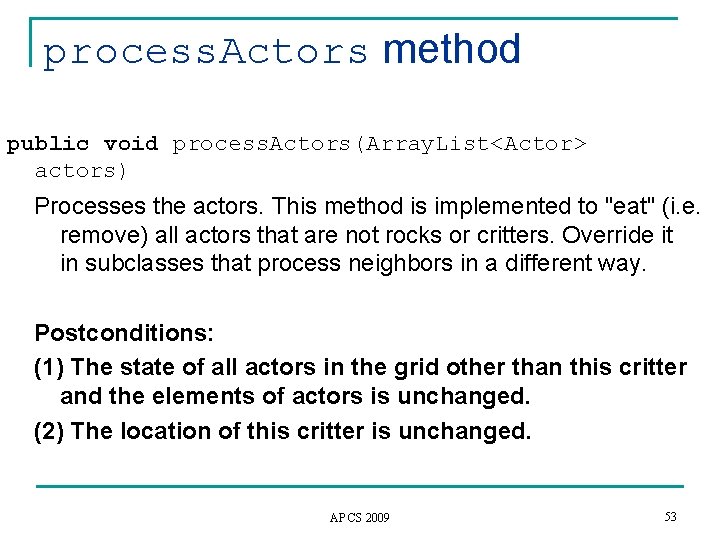
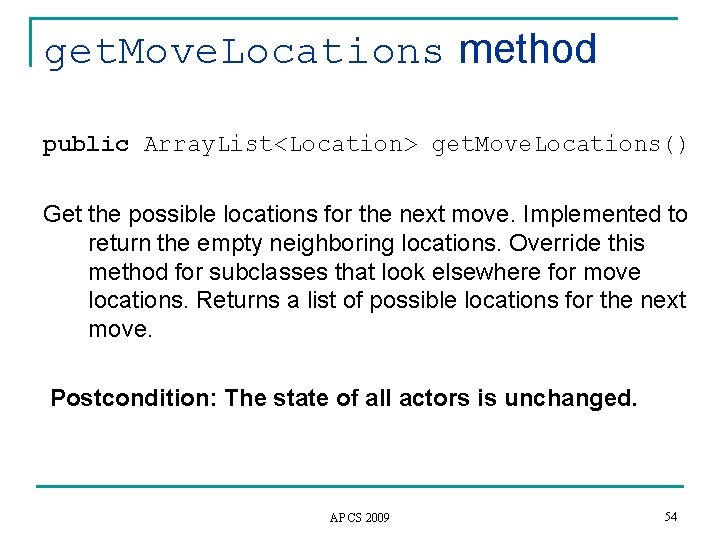
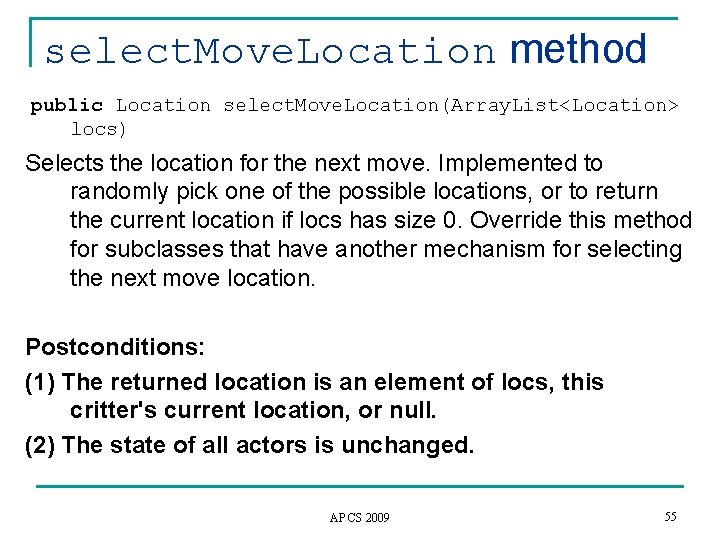
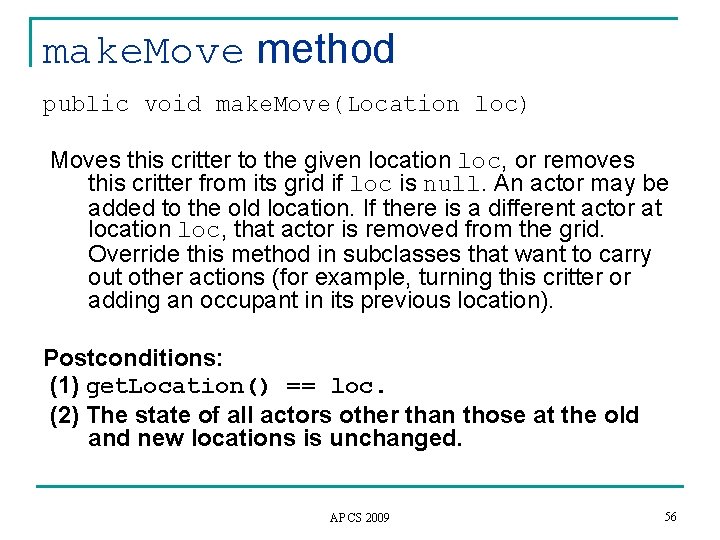
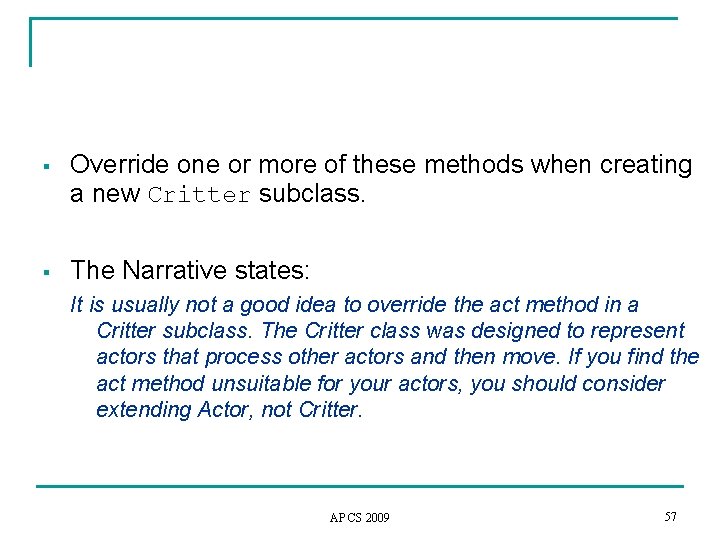
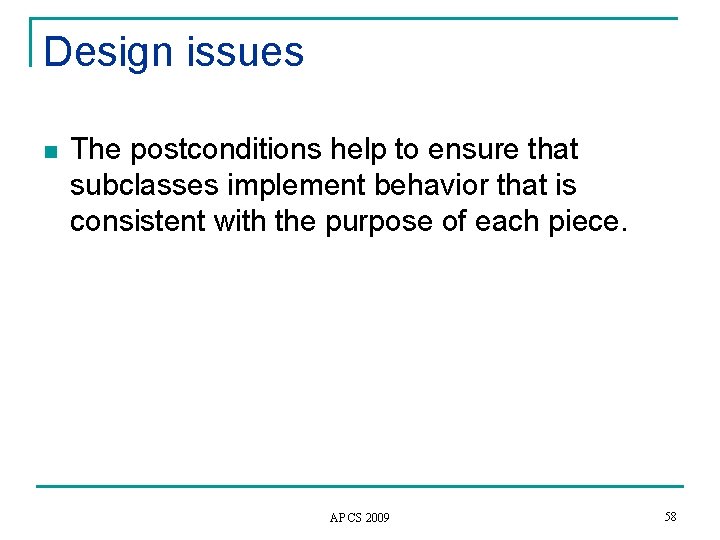
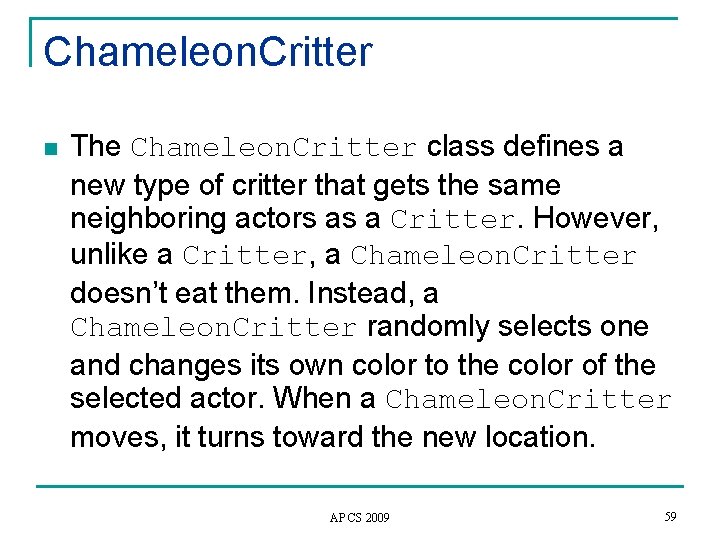
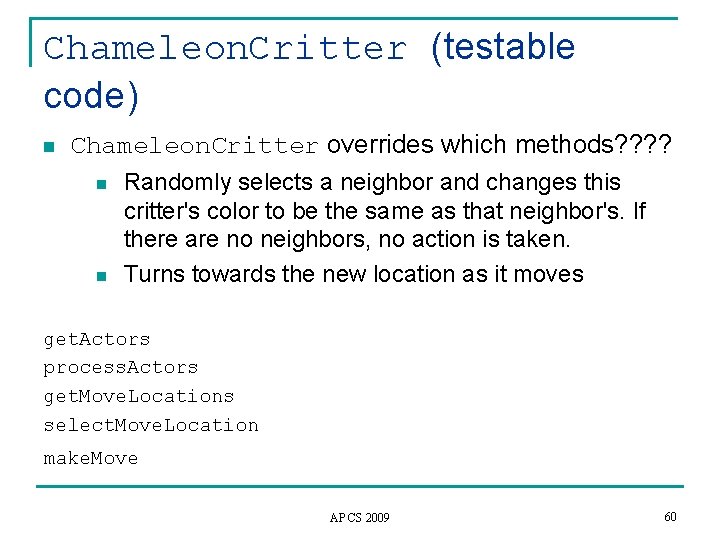
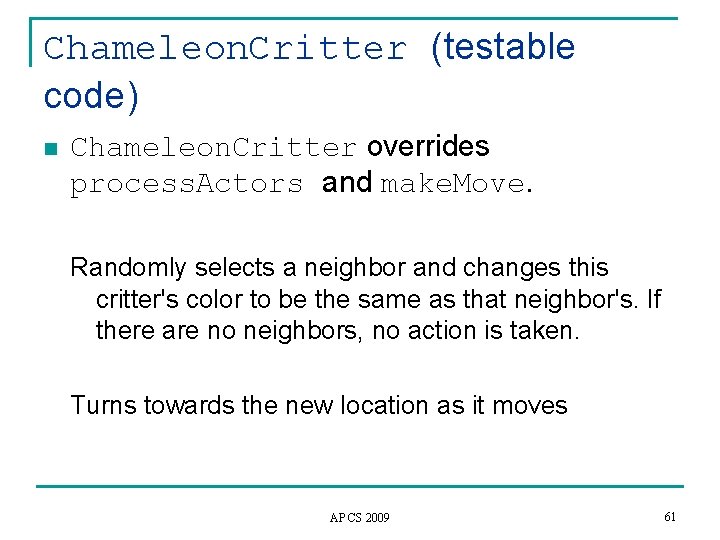
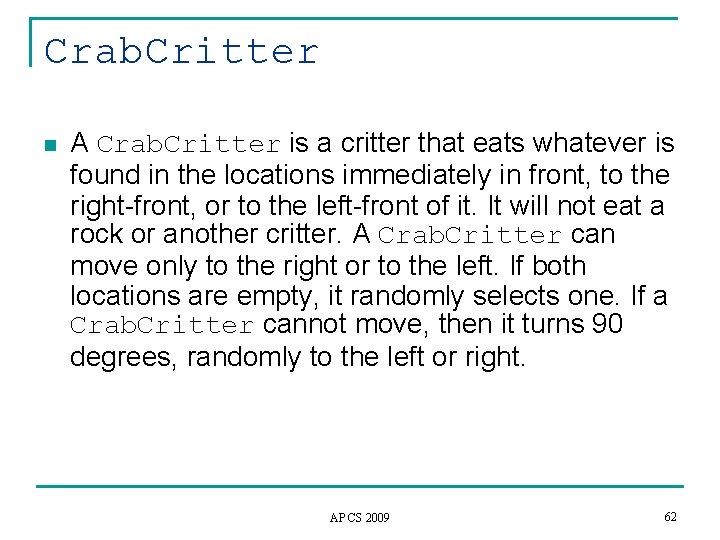
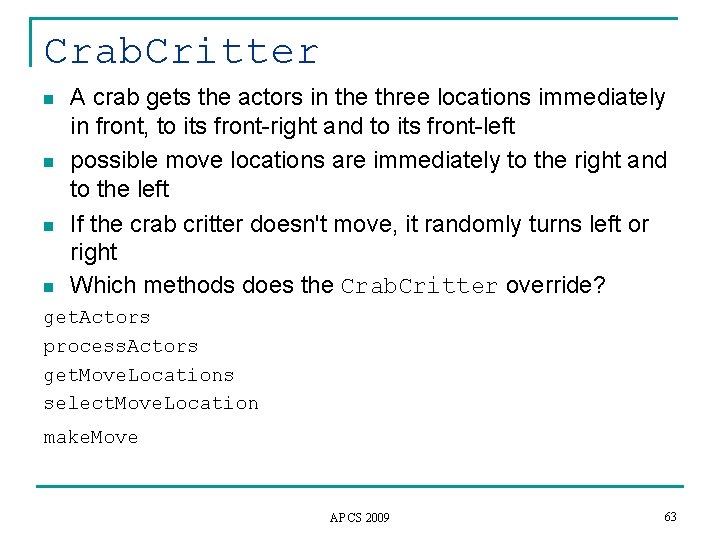
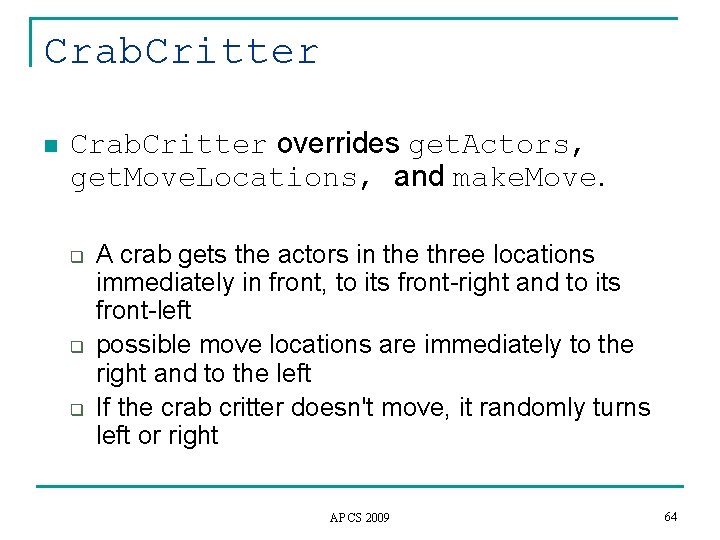
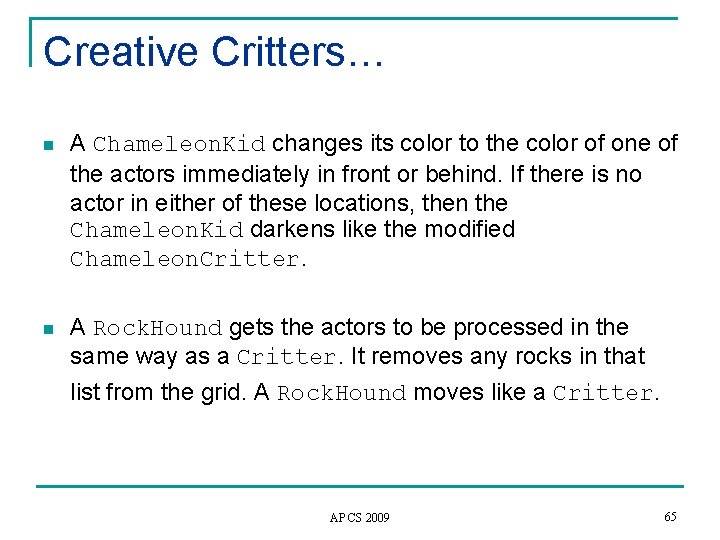
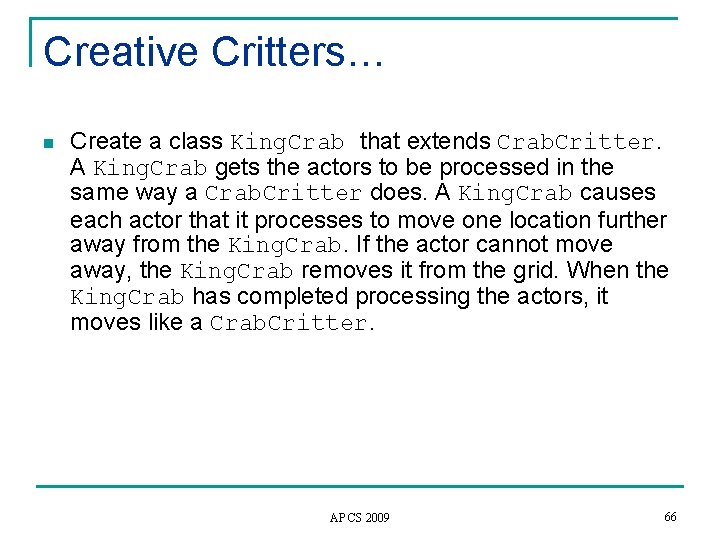
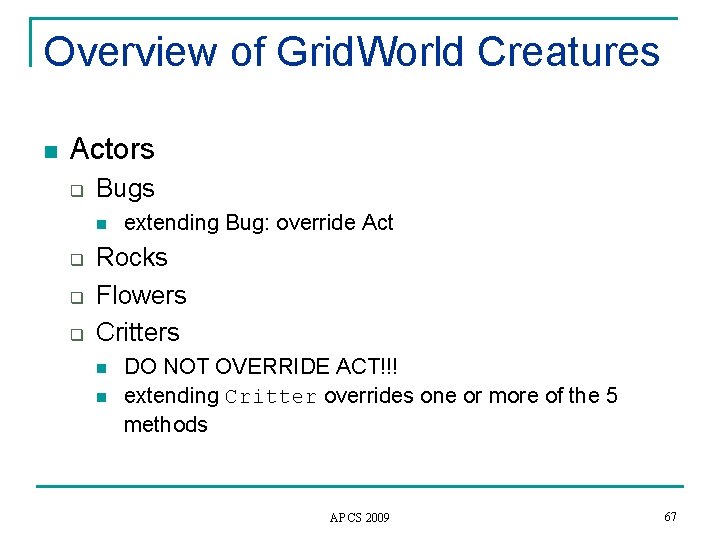
- Slides: 67
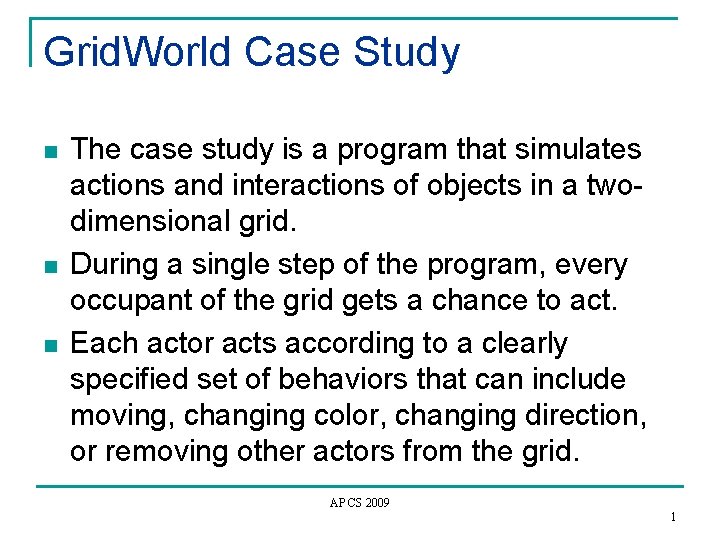
Grid. World Case Study n n n The case study is a program that simulates actions and interactions of objects in a twodimensional grid. During a single step of the program, every occupant of the grid gets a chance to act. Each actor acts according to a clearly specified set of behaviors that can include moving, changing color, changing direction, or removing other actors from the grid. AP CS 2009 1
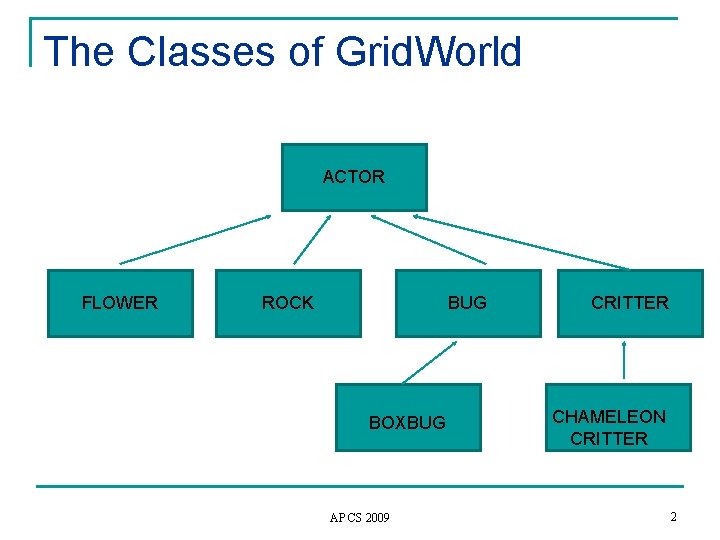
The Classes of Grid. World ACTOR FLOWER ROCK BUG BOXBUG AP CS 2009 CRITTER CHAMELEON CRITTER 2
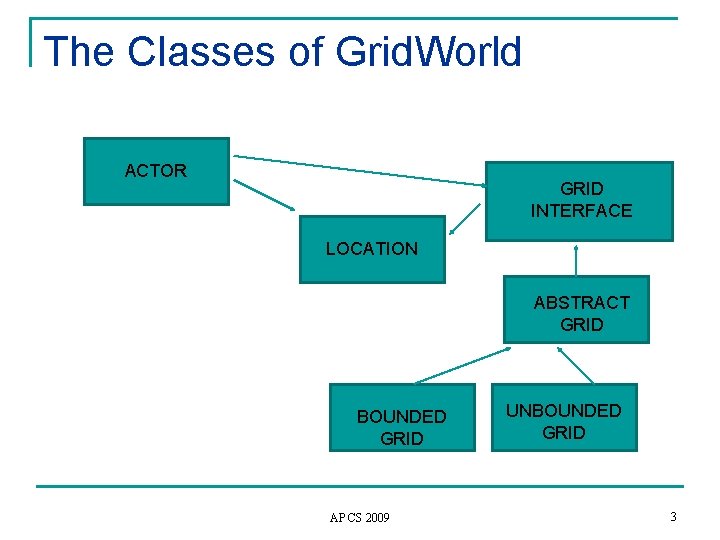
The Classes of Grid. World ACTOR GRID INTERFACE LOCATION ABSTRACT GRID BOUNDED GRID AP CS 2009 UNBOUNDED GRID 3
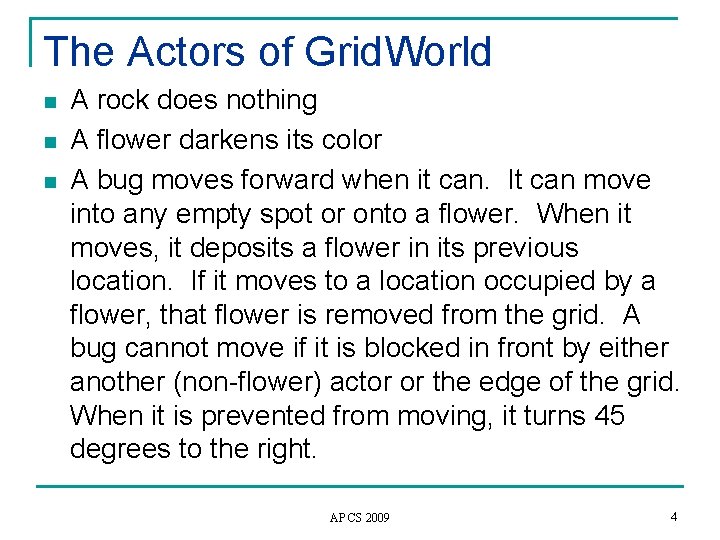
The Actors of Grid. World n n n A rock does nothing A flower darkens its color A bug moves forward when it can. It can move into any empty spot or onto a flower. When it moves, it deposits a flower in its previous location. If it moves to a location occupied by a flower, that flower is removed from the grid. A bug cannot move if it is blocked in front by either another (non-flower) actor or the edge of the grid. When it is prevented from moving, it turns 45 degrees to the right. AP CS 2009 4
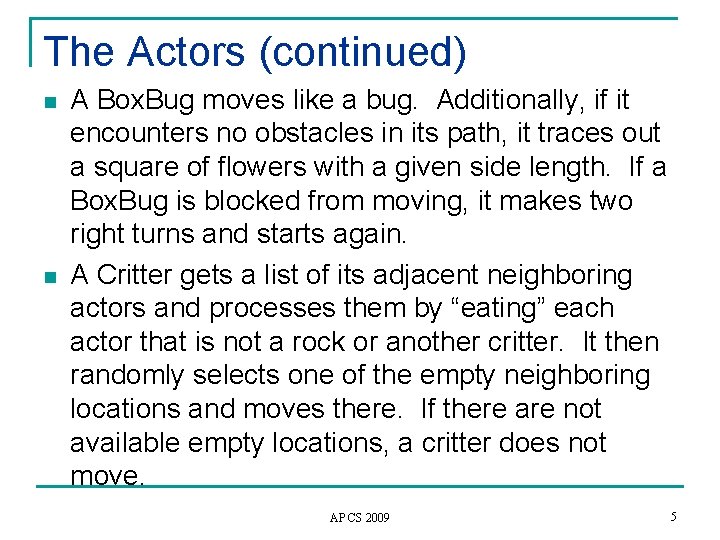
The Actors (continued) n n A Box. Bug moves like a bug. Additionally, if it encounters no obstacles in its path, it traces out a square of flowers with a given side length. If a Box. Bug is blocked from moving, it makes two right turns and starts again. A Critter gets a list of its adjacent neighboring actors and processes them by “eating” each actor that is not a rock or another critter. It then randomly selects one of the empty neighboring locations and moves there. If there are not available empty locations, a critter does not move. AP CS 2009 5
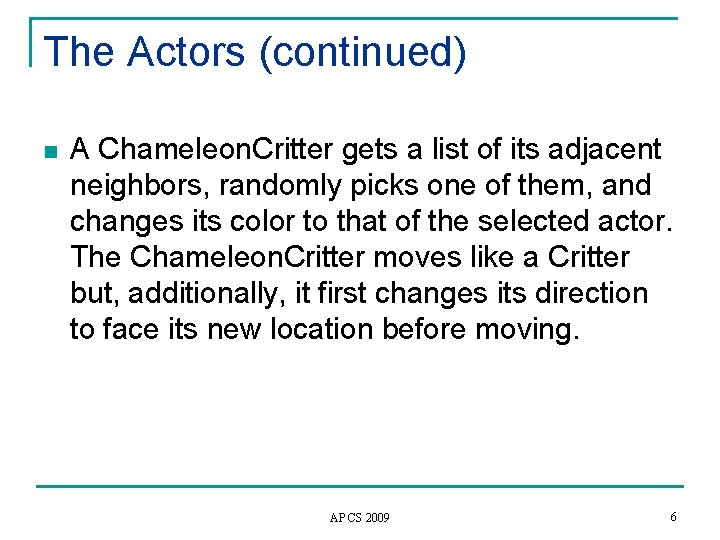
The Actors (continued) n A Chameleon. Critter gets a list of its adjacent neighbors, randomly picks one of them, and changes its color to that of the selected actor. The Chameleon. Critter moves like a Critter but, additionally, it first changes its direction to face its new location before moving. AP CS 2009 6
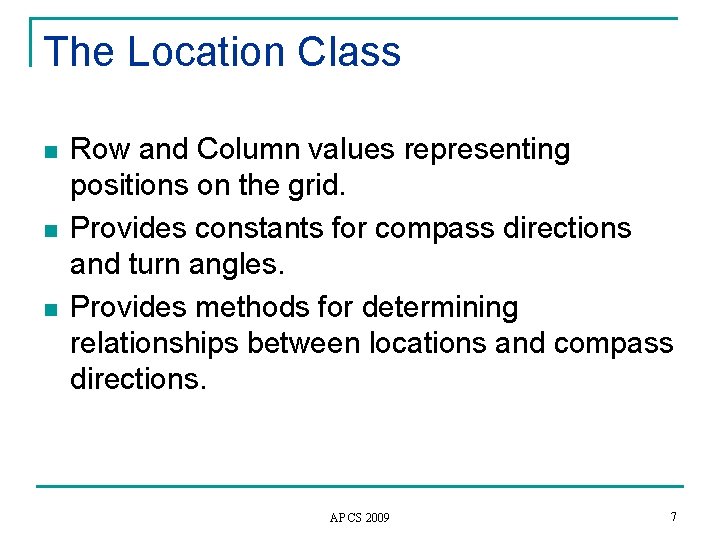
The Location Class n n n Row and Column values representing positions on the grid. Provides constants for compass directions and turn angles. Provides methods for determining relationships between locations and compass directions. AP CS 2009 7
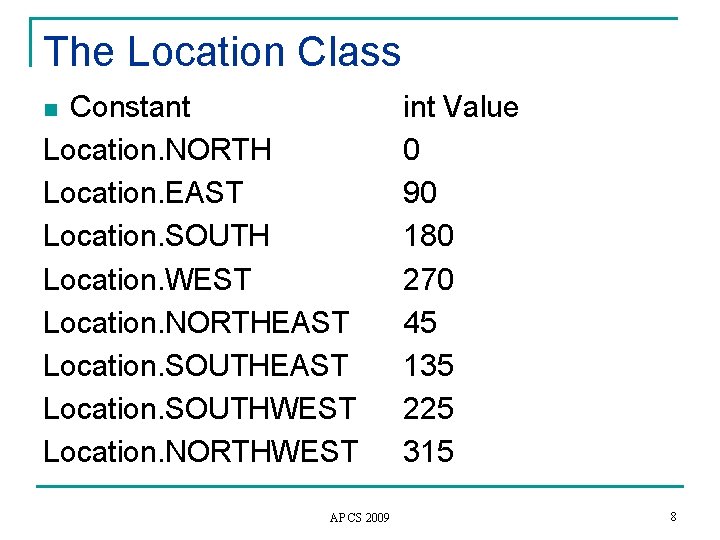
The Location Class Constant Location. NORTH Location. EAST Location. SOUTH Location. WEST Location. NORTHEAST Location. SOUTHWEST Location. NORTHWEST n AP CS 2009 int Value 0 90 180 270 45 135 225 315 8
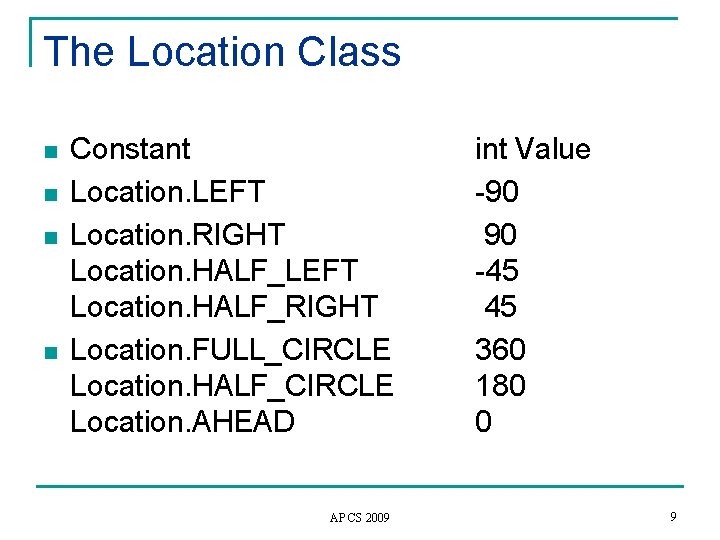
The Location Class n n Constant Location. LEFT Location. RIGHT Location. HALF_LEFT Location. HALF_RIGHT Location. FULL_CIRCLE Location. HALF_CIRCLE Location. AHEAD AP CS 2009 int Value -90 90 -45 45 360 180 0 9
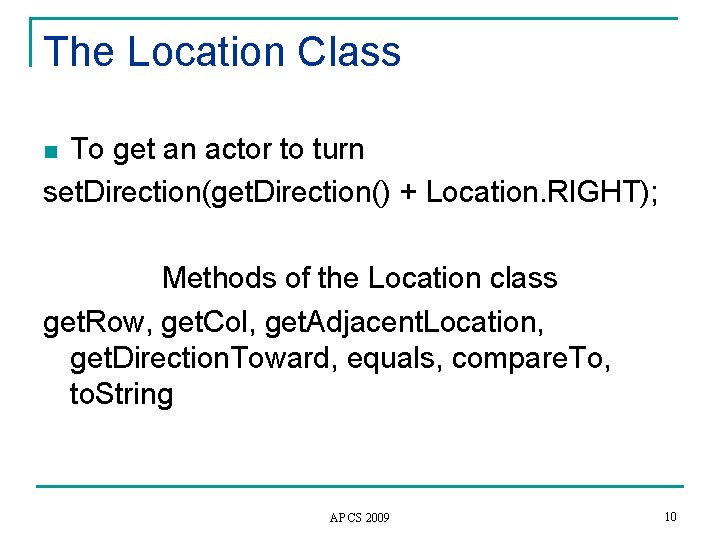
The Location Class To get an actor to turn set. Direction(get. Direction() + Location. RIGHT); n Methods of the Location class get. Row, get. Col, get. Adjacent. Location, get. Direction. Toward, equals, compare. To, to. String AP CS 2009 10
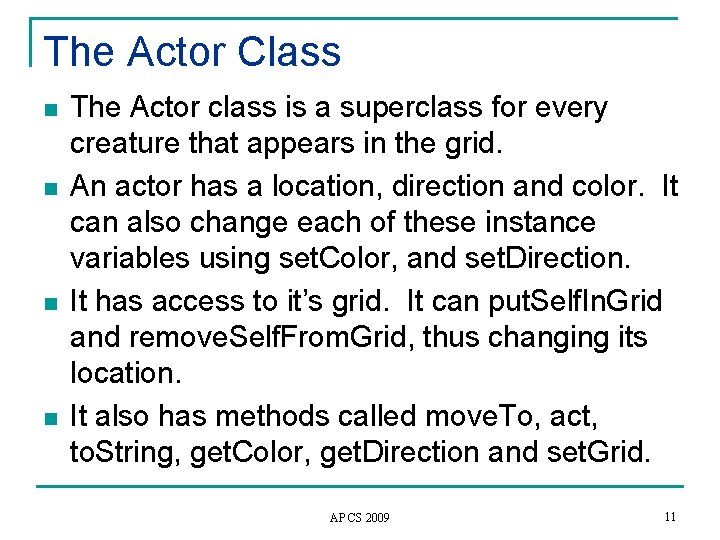
The Actor Class n n The Actor class is a superclass for every creature that appears in the grid. An actor has a location, direction and color. It can also change each of these instance variables using set. Color, and set. Direction. It has access to it’s grid. It can put. Self. In. Grid and remove. Self. From. Grid, thus changing its location. It also has methods called move. To, act, to. String, get. Color, get. Direction and set. Grid. AP CS 2009 11
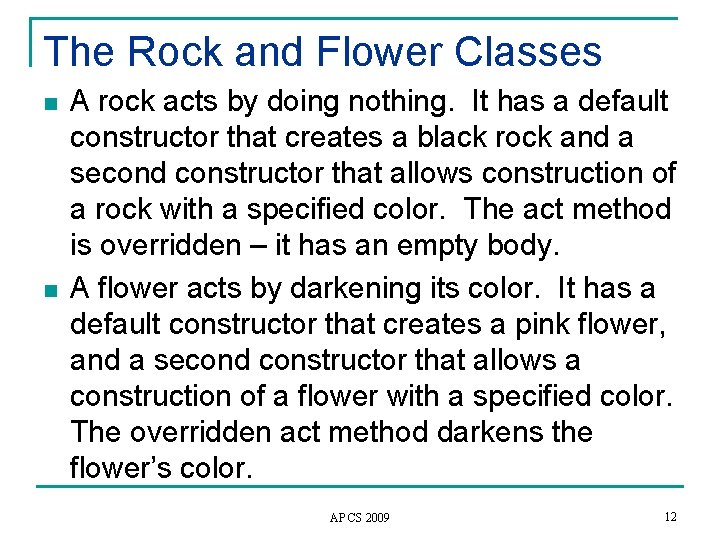
The Rock and Flower Classes n n A rock acts by doing nothing. It has a default constructor that creates a black rock and a second constructor that allows construction of a rock with a specified color. The act method is overridden – it has an empty body. A flower acts by darkening its color. It has a default constructor that creates a pink flower, and a second constructor that allows a construction of a flower with a specified color. The overridden act method darkens the flower’s color. AP CS 2009 12
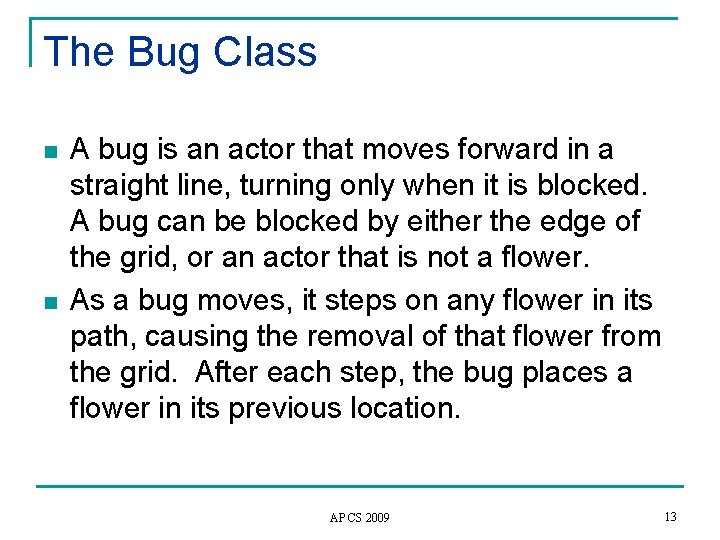
The Bug Class n n A bug is an actor that moves forward in a straight line, turning only when it is blocked. A bug can be blocked by either the edge of the grid, or an actor that is not a flower. As a bug moves, it steps on any flower in its path, causing the removal of that flower from the grid. After each step, the bug places a flower in its previous location. AP CS 2009 13
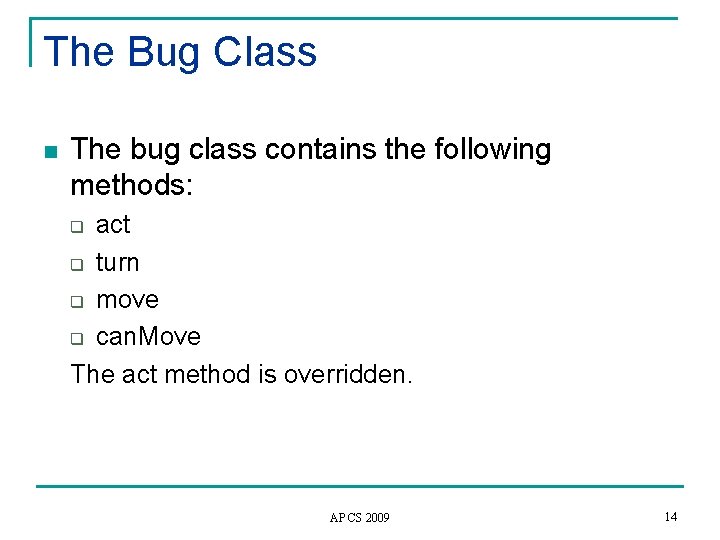
The Bug Class n The bug class contains the following methods: act q turn q move q can. Move The act method is overridden. q AP CS 2009 14
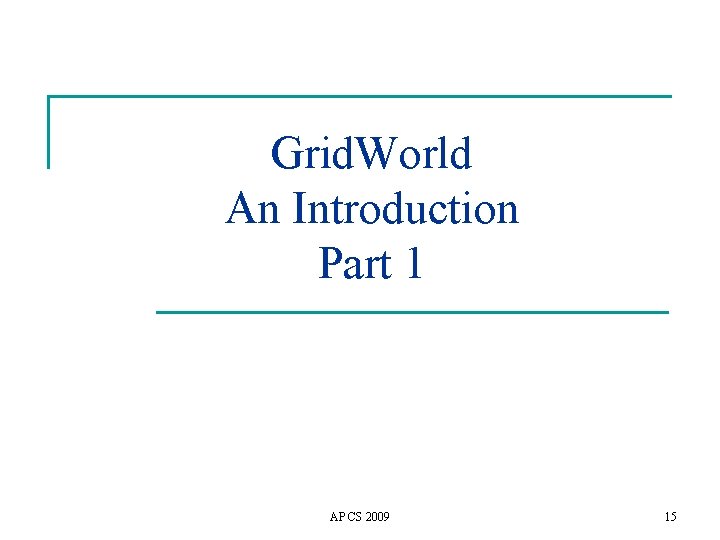
Grid. World An Introduction Part 1 AP CS 2009 15
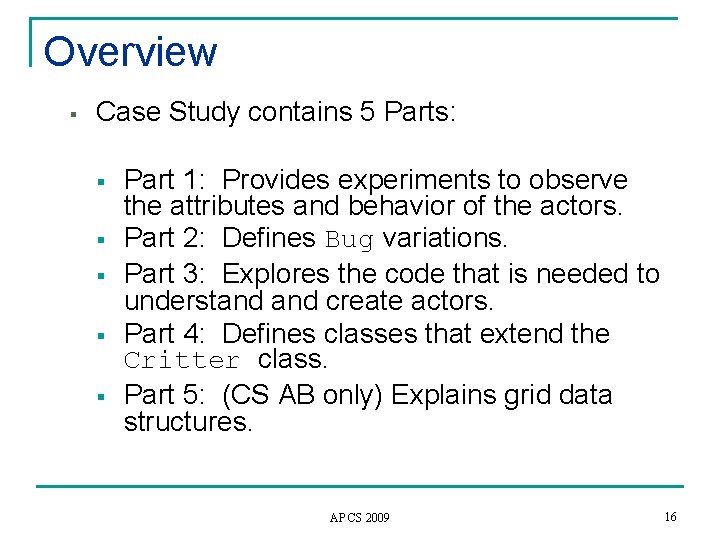
Overview § Case Study contains 5 Parts: § § § Part 1: Provides experiments to observe the attributes and behavior of the actors. Part 2: Defines Bug variations. Part 3: Explores the code that is needed to understand create actors. Part 4: Defines classes that extend the Critter class. Part 5: (CS AB only) Explains grid data structures. AP CS 2009 16
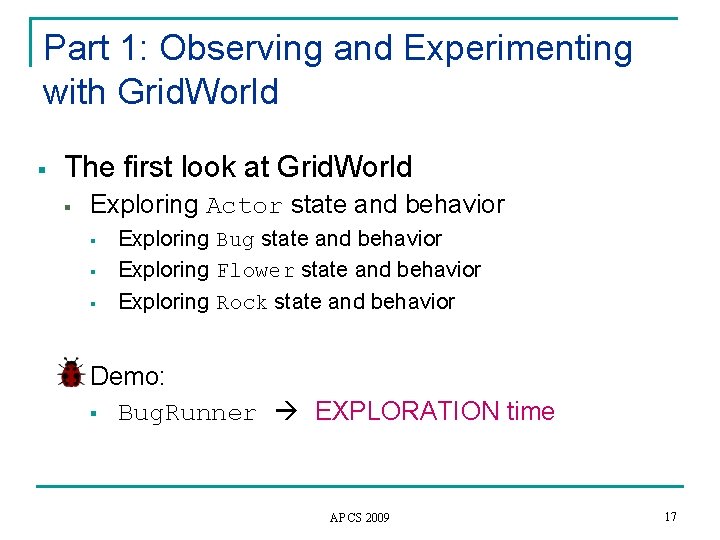
Part 1: Observing and Experimenting with Grid. World § The first look at Grid. World § Exploring Actor state and behavior § § Exploring Bug state and behavior Exploring Flower state and behavior Exploring Rock state and behavior Demo: § Bug. Runner EXPLORATION time AP CS 2009 17
![Bug Runner code public static void mainString args Actor World world new Bug. Runner (code) public static void main(String[] args) { Actor. World world = new](https://slidetodoc.com/presentation_image_h2/4892b38b92c4b0e6546fb34012c27c89/image-18.jpg)
Bug. Runner (code) public static void main(String[] args) { Actor. World world = new Actor. World(); world. add(new Bug()); world. add(new Rock()); world. show(); } AP CS 2009 18
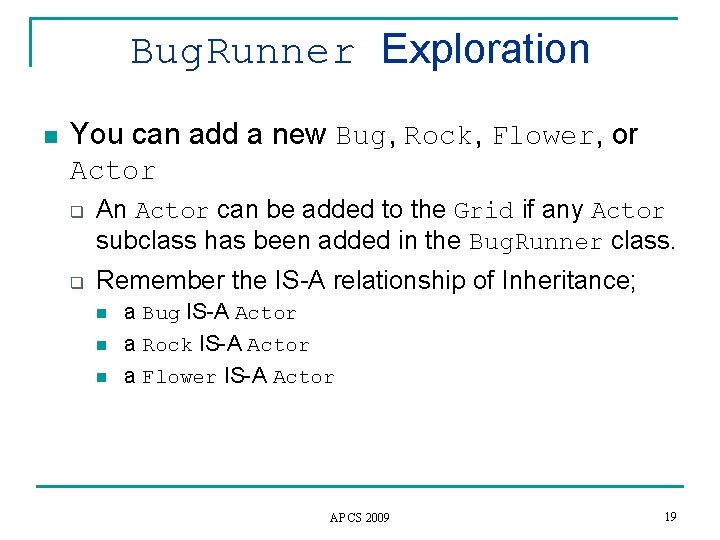
Bug. Runner Exploration n You can add a new Bug, Rock, Flower, or Actor q q An Actor can be added to the Grid if any Actor subclass has been added in the Bug. Runner class. Remember the IS-A relationship of Inheritance; n n n a Bug IS-A Actor a Rock IS-A Actor a Flower IS-A Actor AP CS 2009 19
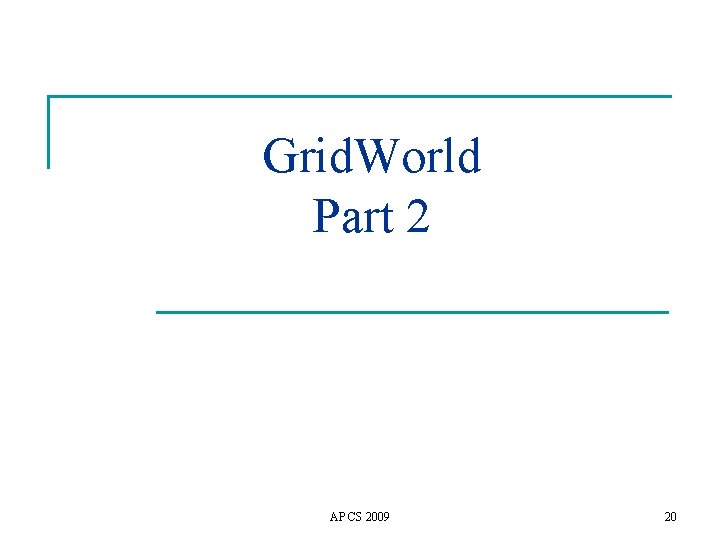
Grid. World Part 2 AP CS 2009 20
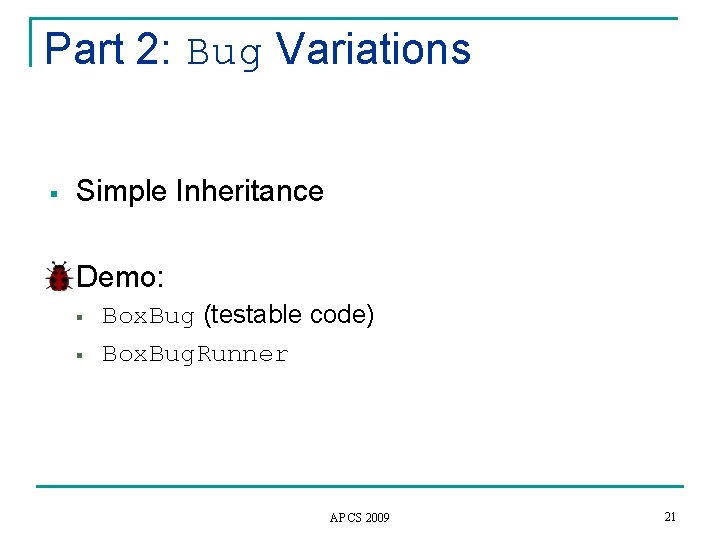
Part 2: Bug Variations § Simple Inheritance § Demo: § § Box. Bug (testable code) Box. Bug. Runner AP CS 2009 21
![Box Bug Runner public static void mainString args Actor World world new Box. Bug. Runner public static void main(String[] args) { Actor. World world = new](https://slidetodoc.com/presentation_image_h2/4892b38b92c4b0e6546fb34012c27c89/image-22.jpg)
Box. Bug. Runner public static void main(String[] args) { Actor. World world = new Actor. World(); Box. Bug alice = new Box. Bug(6); alice. set. Color(Color. ORANGE); Box. Bug bob = new Box. Bug(3); world. add(new Location(7, 8), alice); world. add(new Location(5, 5), bob); world. show(); } AP CS 2009 22
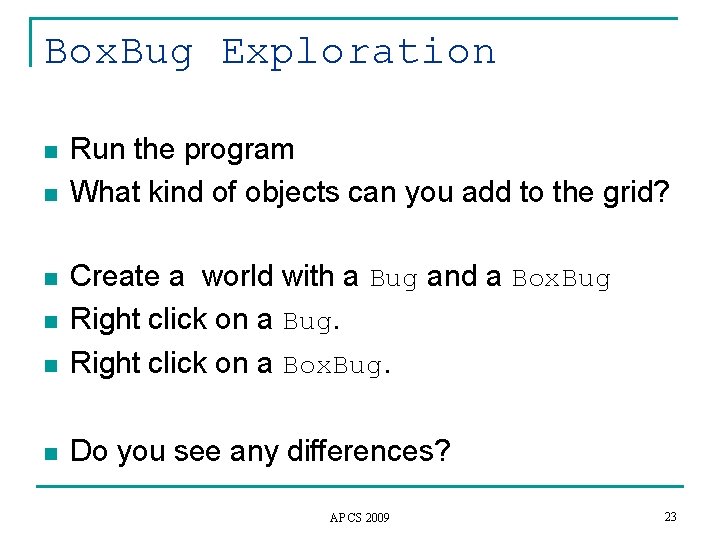
Box. Bug Exploration n n Run the program What kind of objects can you add to the grid? n Create a world with a Bug and a Box. Bug Right click on a Bug. Right click on a Box. Bug. n Do you see any differences? n n AP CS 2009 23
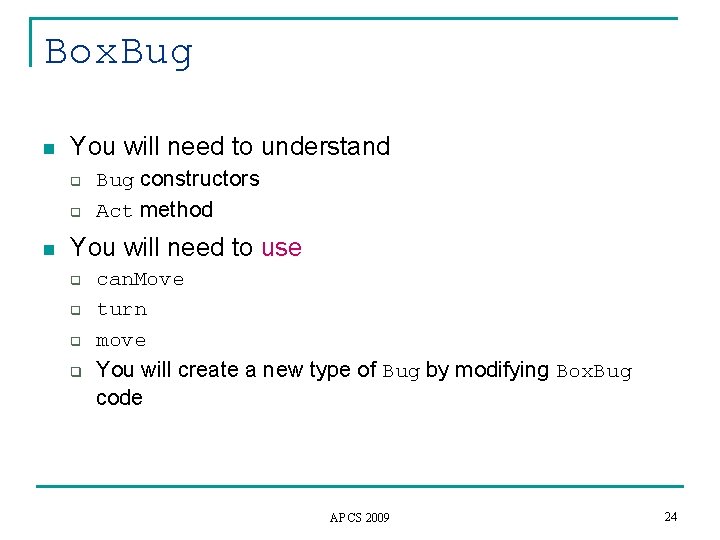
Box. Bug n n You will need to understand q Bug constructors q Act method You will need to use q q can. Move turn move You will create a new type of Bug by modifying Box. Bug code AP CS 2009 24
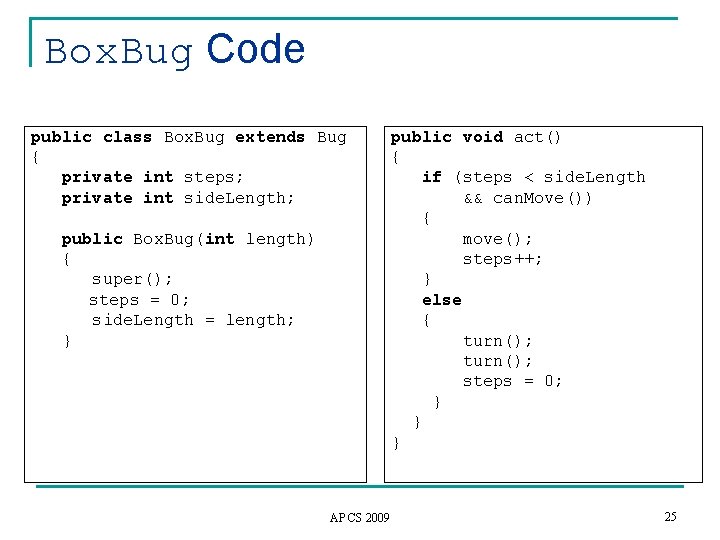
Box. Bug Code public class Box. Bug extends Bug { private int steps; private int side. Length; public Box. Bug(int length) { super(); steps = 0; side. Length = length; } AP CS 2009 public void act() { if (steps < side. Length && can. Move()) { move(); steps++; } else { turn(); steps = 0; } } } 25
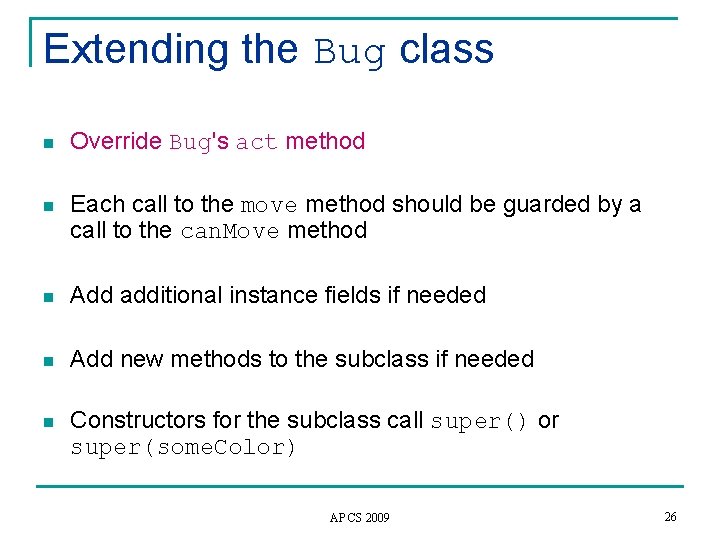
Extending the Bug class n Override Bug's act method n Each call to the move method should be guarded by a call to the can. Move method n Add additional instance fields if needed n Add new methods to the subclass if needed n Constructors for the subclass call super() or super(some. Color) AP CS 2009 26
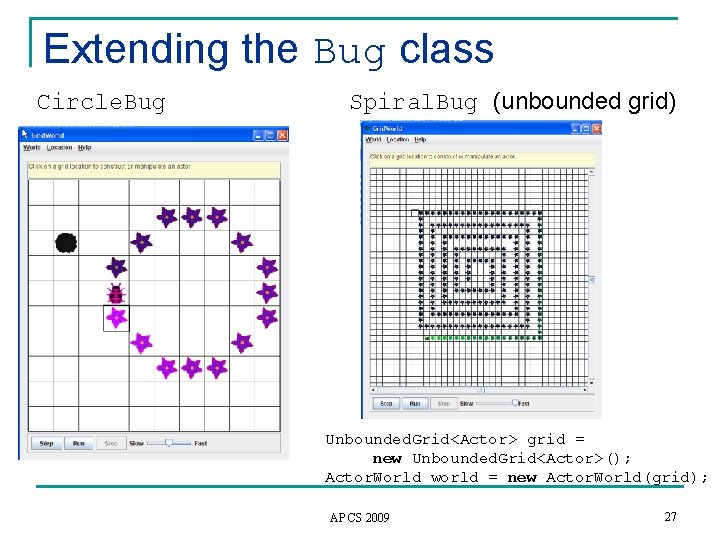
Extending the Bug class Circle. Bug Spiral. Bug (unbounded grid) Unbounded. Grid<Actor> grid = new Unbounded. Grid<Actor>(); Actor. World world = new Actor. World(grid); AP CS 2009 27
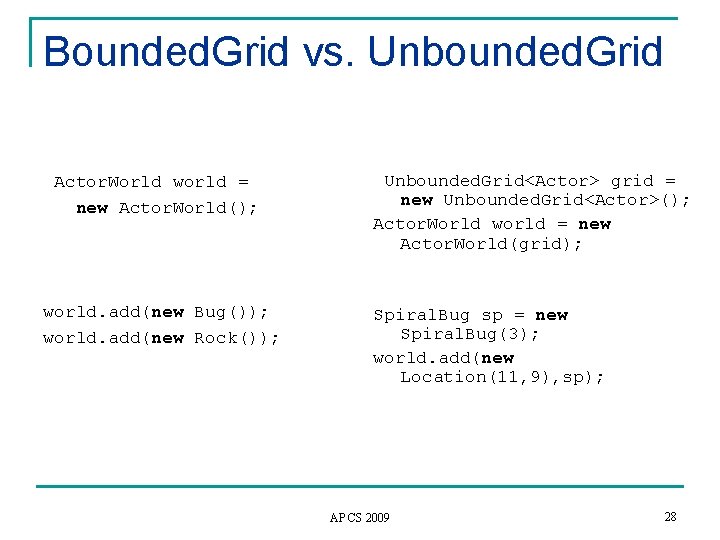
Bounded. Grid vs. Unbounded. Grid Actor. World world = new Actor. World(); world. add(new Bug()); world. add(new Rock()); Unbounded. Grid<Actor> grid = new Unbounded. Grid<Actor>(); Actor. World world = new Actor. World(grid); Spiral. Bug sp = new Spiral. Bug(3); world. add(new Location(11, 9), sp); AP CS 2009 28
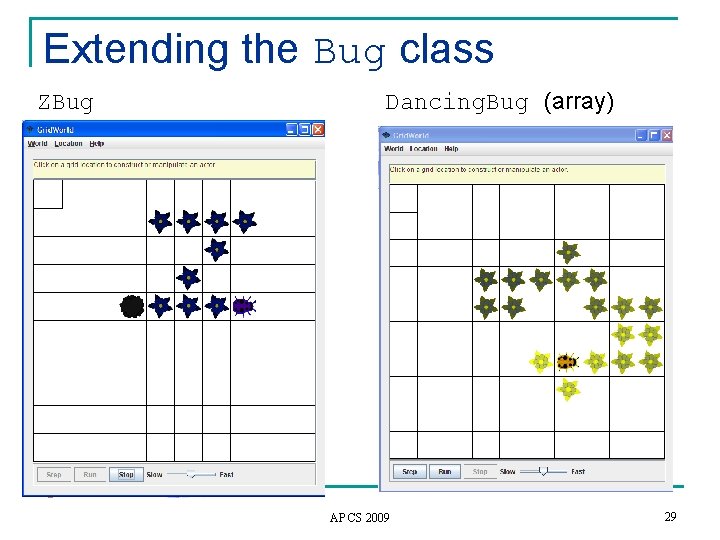
Extending the Bug class ZBug Dancing. Bug (array) AP CS 2009 29
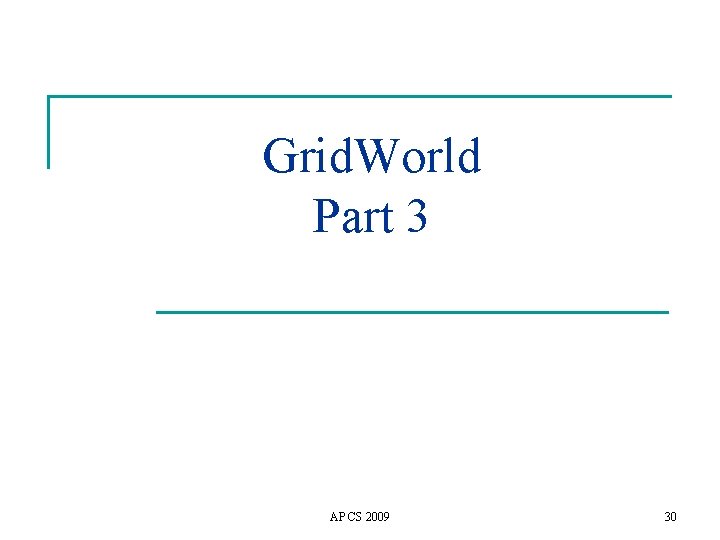
Grid. World Part 3 AP CS 2009 30
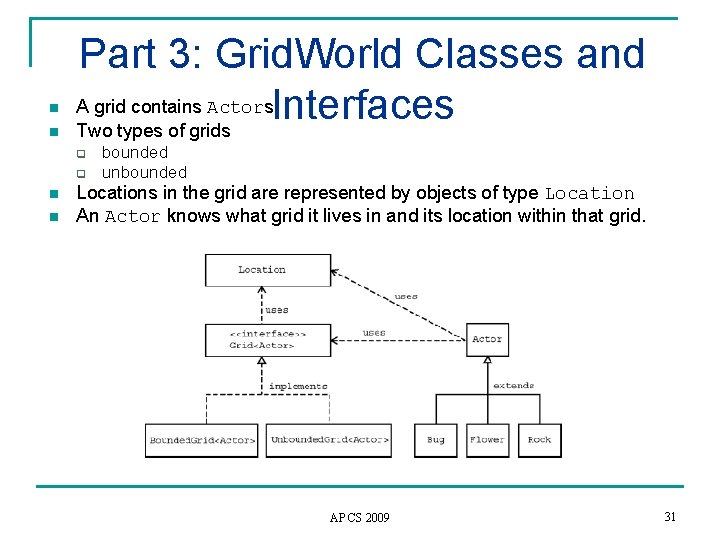
n n Part 3: Grid. World Classes and A grid contains Actors. Interfaces Two types of grids q q n n bounded unbounded Locations in the grid are represented by objects of type Location An Actor knows what grid it lives in and its location within that grid. AP CS 2009 31
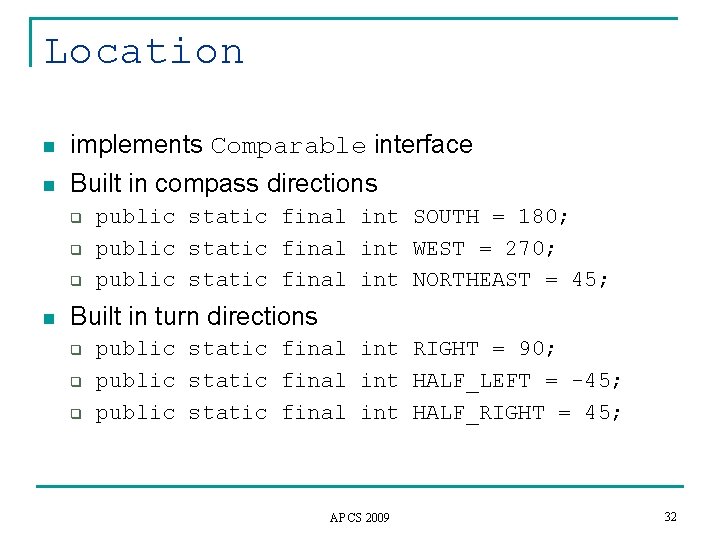
Location n implements Comparable interface n Built in compass directions q q q n public static final int SOUTH = 180; public static final int WEST = 270; public static final int NORTHEAST = 45; Built in turn directions q q q public static final int RIGHT = 90; public static final int HALF_LEFT = -45; public static final int HALF_RIGHT = 45; AP CS 2009 32
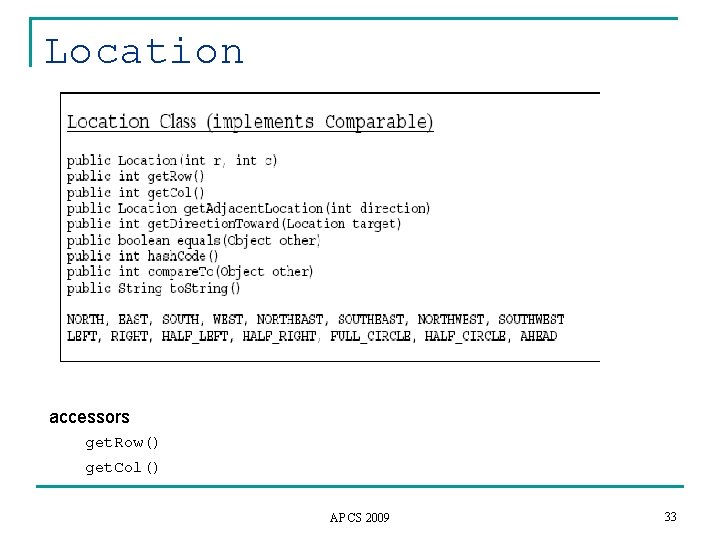
Location accessors get. Row() get. Col() AP CS 2009 33
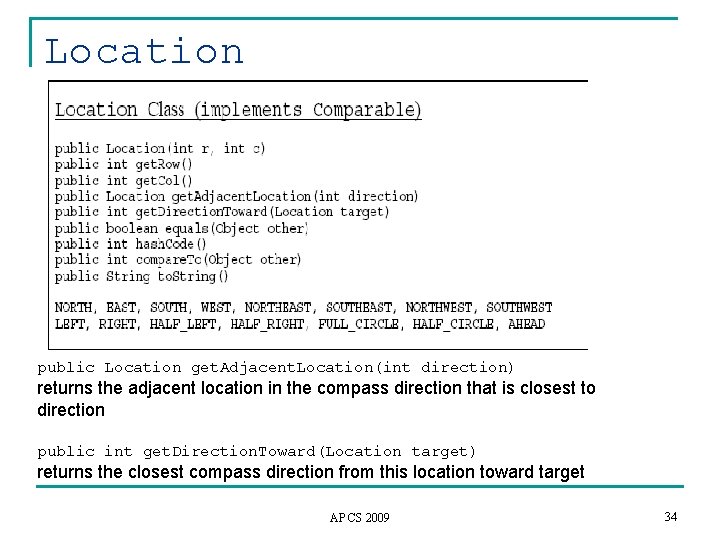
Location public Location get. Adjacent. Location(int direction) returns the adjacent location in the compass direction that is closest to direction public int get. Direction. Toward(Location target) returns the closest compass direction from this location toward target AP CS 2009 34
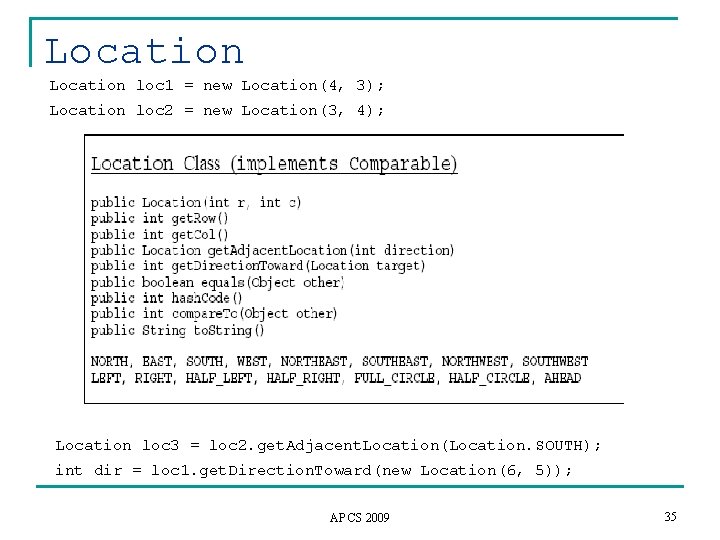
Location loc 1 = new Location(4, 3); Location loc 2 = new Location(3, 4); Location loc 3 = loc 2. get. Adjacent. Location(Location. SOUTH); int dir = loc 1. get. Direction. Toward(new Location(6, 5)); AP CS 2009 35
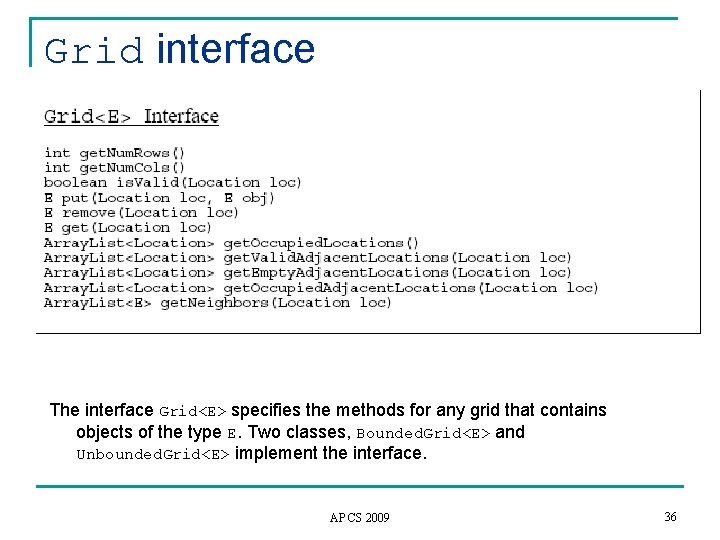
Grid interface The interface Grid<E> specifies the methods for any grid that contains objects of the type E. Two classes, Bounded. Grid<E> and Unbounded. Grid<E> implement the interface. AP CS 2009 36
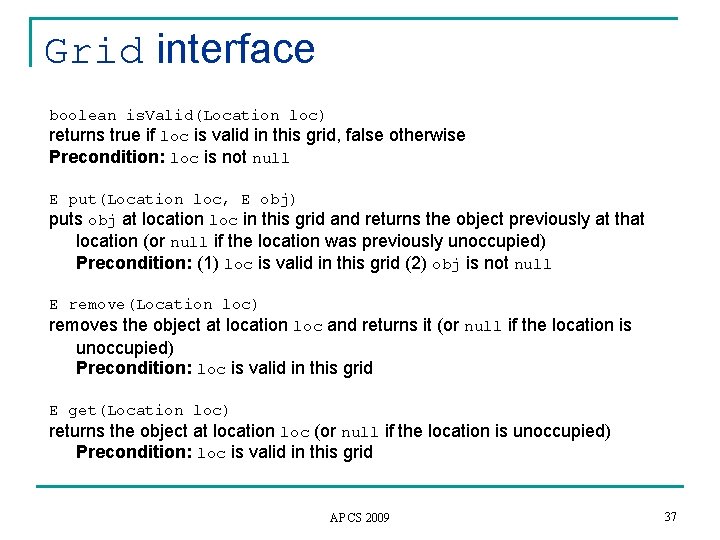
Grid interface boolean is. Valid(Location loc) returns true if loc is valid in this grid, false otherwise Precondition: loc is not null E put(Location loc, E obj) puts obj at location loc in this grid and returns the object previously at that location (or null if the location was previously unoccupied) Precondition: (1) loc is valid in this grid (2) obj is not null E remove(Location loc) removes the object at location loc and returns it (or null if the location is unoccupied) Precondition: loc is valid in this grid E get(Location loc) returns the object at location loc (or null if the location is unoccupied) Precondition: loc is valid in this grid AP CS 2009 37
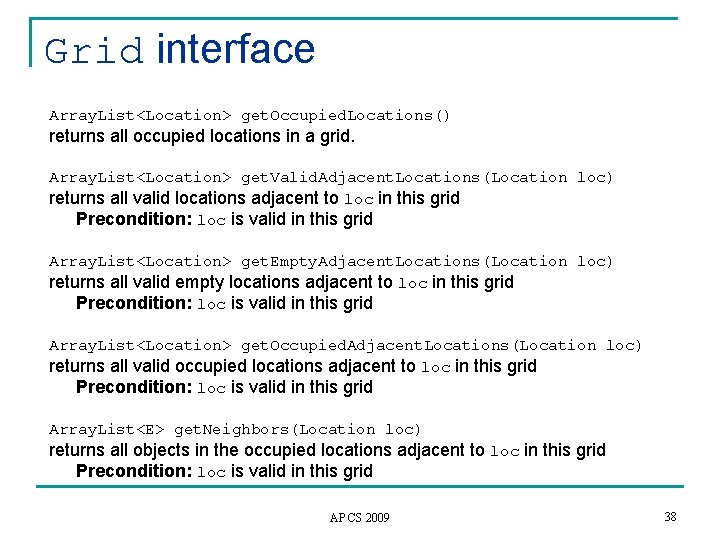
Grid interface Array. List<Location> get. Occupied. Locations() returns all occupied locations in a grid. Array. List<Location> get. Valid. Adjacent. Locations(Location loc) returns all valid locations adjacent to loc in this grid Precondition: loc is valid in this grid Array. List<Location> get. Empty. Adjacent. Locations(Location loc) returns all valid empty locations adjacent to loc in this grid Precondition: loc is valid in this grid Array. List<Location> get. Occupied. Adjacent. Locations(Location loc) returns all valid occupied locations adjacent to loc in this grid Precondition: loc is valid in this grid Array. List<E> get. Neighbors(Location loc) returns all objects in the occupied locations adjacent to loc in this grid Precondition: loc is valid in this grid AP CS 2009 38
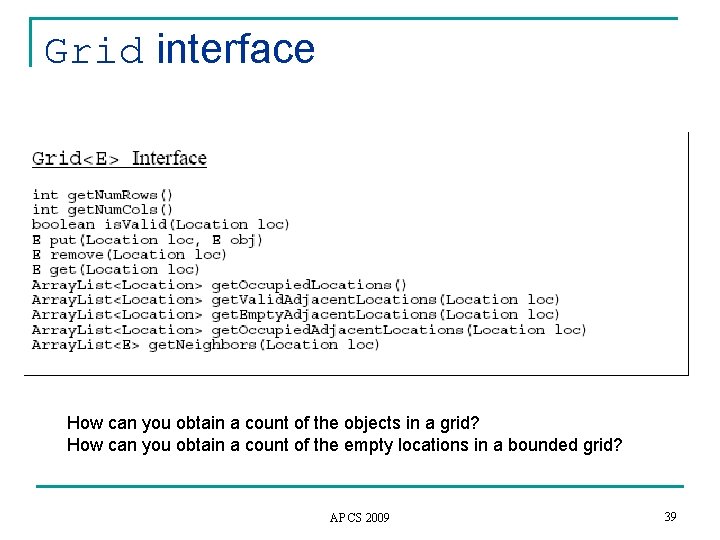
Grid interface How can you obtain a count of the objects in a grid? How can you obtain a count of the empty locations in a bounded grid? AP CS 2009 39
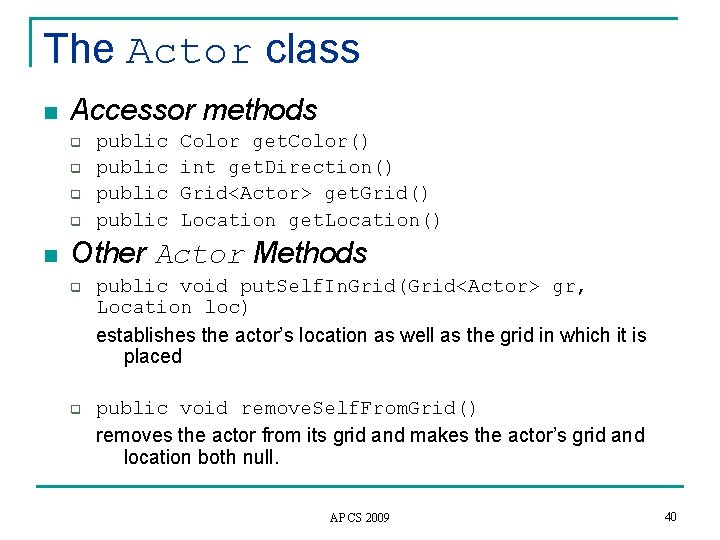
The Actor class n Accessor methods q q n public Color get. Color() int get. Direction() Grid<Actor> get. Grid() Location get. Location() Other Actor Methods q public void put. Self. In. Grid(Grid<Actor> gr, Location loc) establishes the actor’s location as well as the grid in which it is placed q public void remove. Self. From. Grid() removes the actor from its grid and makes the actor’s grid and location both null. AP CS 2009 40
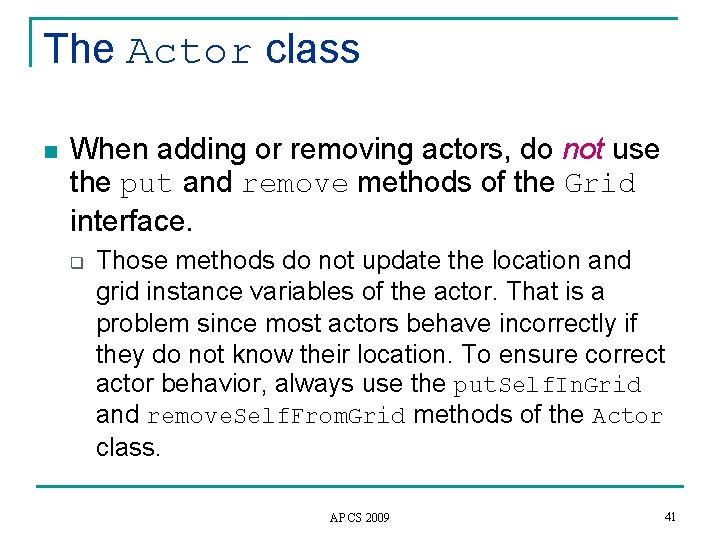
The Actor class n When adding or removing actors, do not use the put and remove methods of the Grid interface. q Those methods do not update the location and grid instance variables of the actor. That is a problem since most actors behave incorrectly if they do not know their location. To ensure correct actor behavior, always use the put. Self. In. Grid and remove. Self. From. Grid methods of the Actor class. AP CS 2009 41
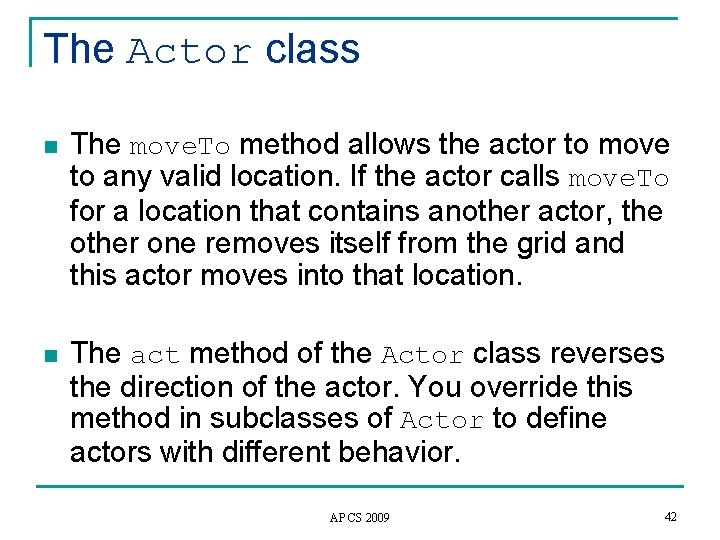
The Actor class n The move. To method allows the actor to move to any valid location. If the actor calls move. To for a location that contains another actor, the other one removes itself from the grid and this actor moves into that location. n The act method of the Actor class reverses the direction of the actor. You override this method in subclasses of Actor to define actors with different behavior. AP CS 2009 42
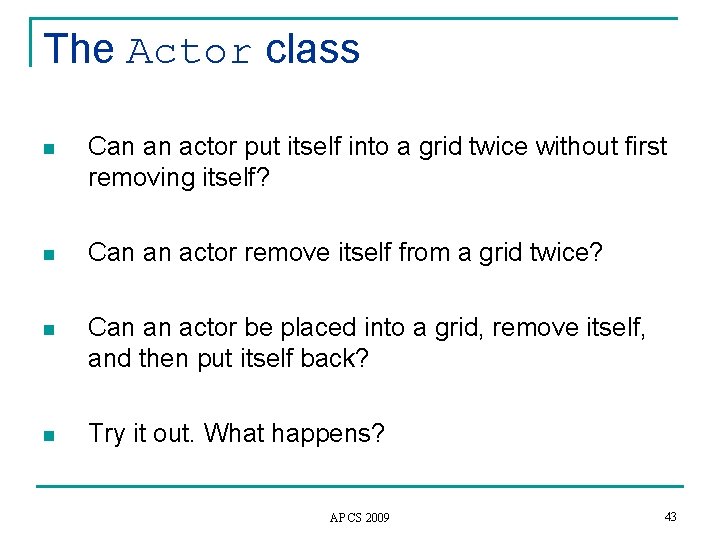
The Actor class n Can an actor put itself into a grid twice without first removing itself? n Can an actor remove itself from a grid twice? n Can an actor be placed into a grid, remove itself, and then put itself back? n Try it out. What happens? AP CS 2009 43
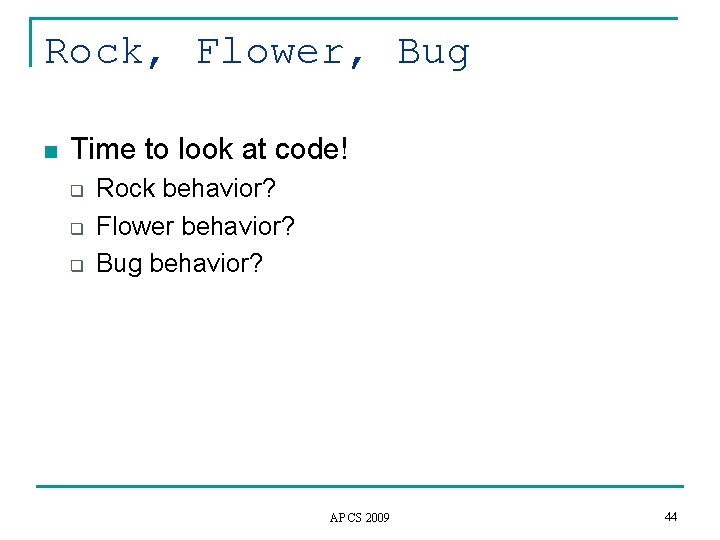
Rock, Flower, Bug n Time to look at code! q q q Rock behavior? Flower behavior? Bug behavior? AP CS 2009 44
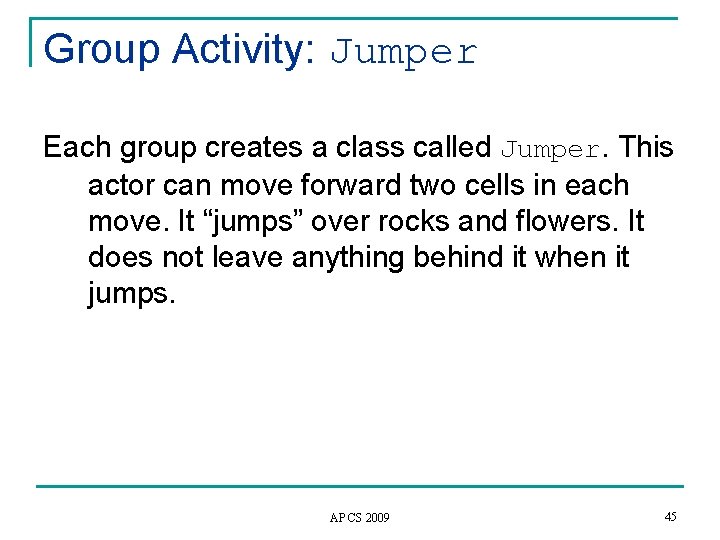
Group Activity: Jumper Each group creates a class called Jumper. This actor can move forward two cells in each move. It “jumps” over rocks and flowers. It does not leave anything behind it when it jumps. AP CS 2009 45
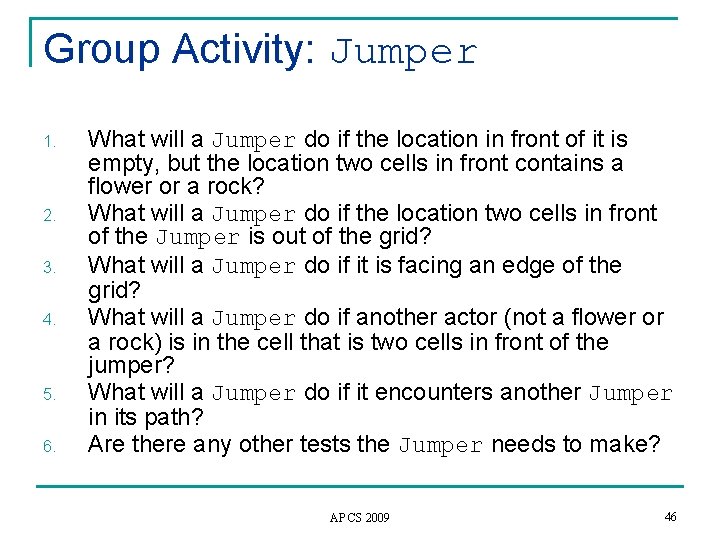
Group Activity: Jumper 1. 2. 3. 4. 5. 6. What will a Jumper do if the location in front of it is empty, but the location two cells in front contains a flower or a rock? What will a Jumper do if the location two cells in front of the Jumper is out of the grid? What will a Jumper do if it is facing an edge of the grid? What will a Jumper do if another actor (not a flower or a rock) is in the cell that is two cells in front of the jumper? What will a Jumper do if it encounters another Jumper in its path? Are there any other tests the Jumper needs to make? AP CS 2009 46
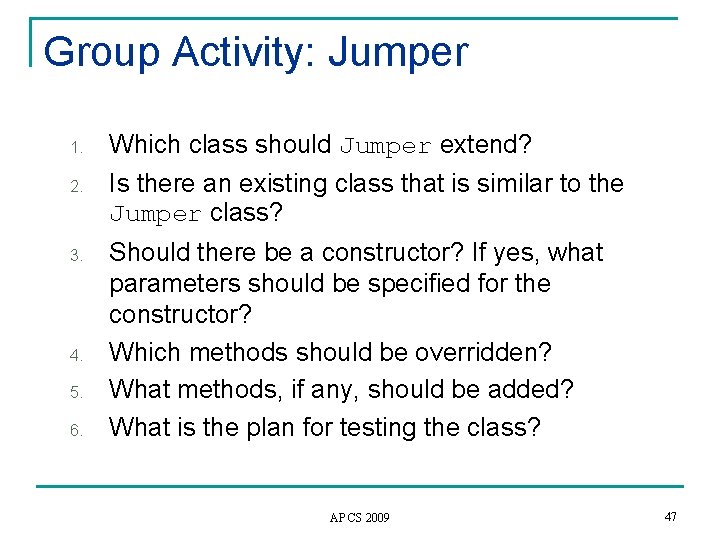
Group Activity: Jumper 1. 2. 3. 4. 5. 6. Which class should Jumper extend? Is there an existing class that is similar to the Jumper class? Should there be a constructor? If yes, what parameters should be specified for the constructor? Which methods should be overridden? What methods, if any, should be added? What is the plan for testing the class? AP CS 2009 47
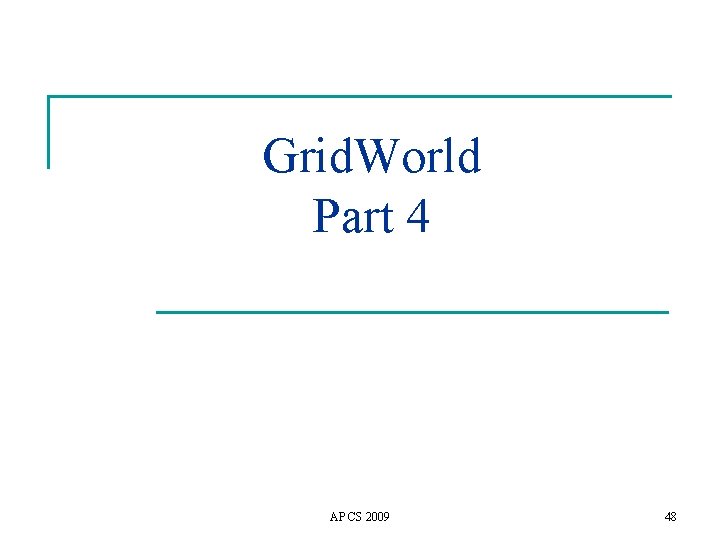
Grid. World Part 4 AP CS 2009 48
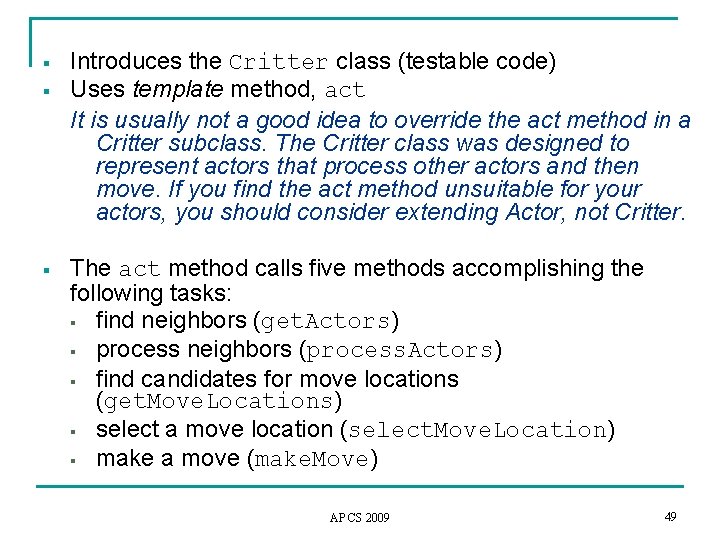
§ § § Introduces the Critter class (testable code) Uses template method, act It is usually not a good idea to override the act method in a Critter subclass. The Critter class was designed to represent actors that process other actors and then move. If you find the act method unsuitable for your actors, you should consider extending Actor, not Critter. The act method calls five methods accomplishing the following tasks: § find neighbors (get. Actors) § process neighbors (process. Actors) § find candidates for move locations (get. Move. Locations) § select a move location (select. Move. Location) § make a move (make. Move) AP CS 2009 49
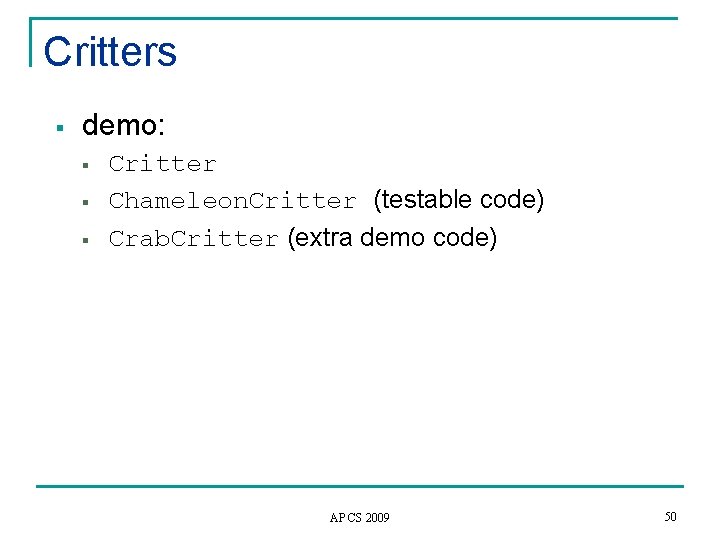
Critters § demo: § § § Critter Chameleon. Critter (testable code) Crab. Critter (extra demo code) AP CS 2009 50
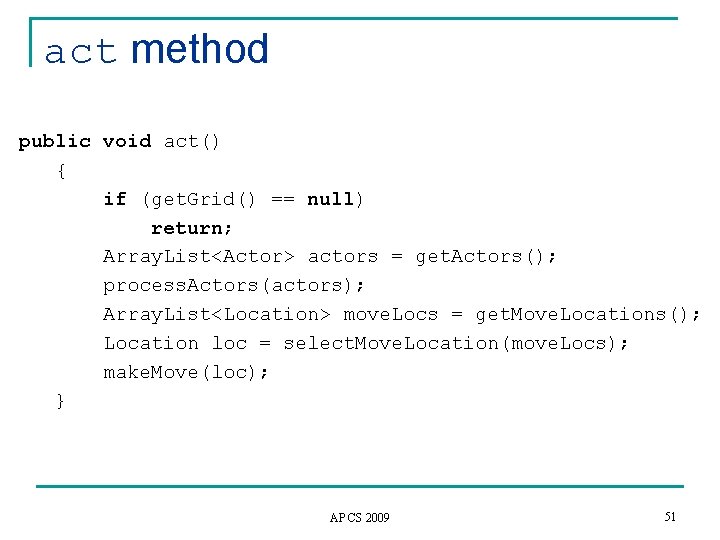
act method public void act() { if (get. Grid() == null) return; Array. List<Actor> actors = get. Actors(); process. Actors(actors); Array. List<Location> move. Locs = get. Move. Locations(); Location loc = select. Move. Location(move. Locs); make. Move(loc); } AP CS 2009 51
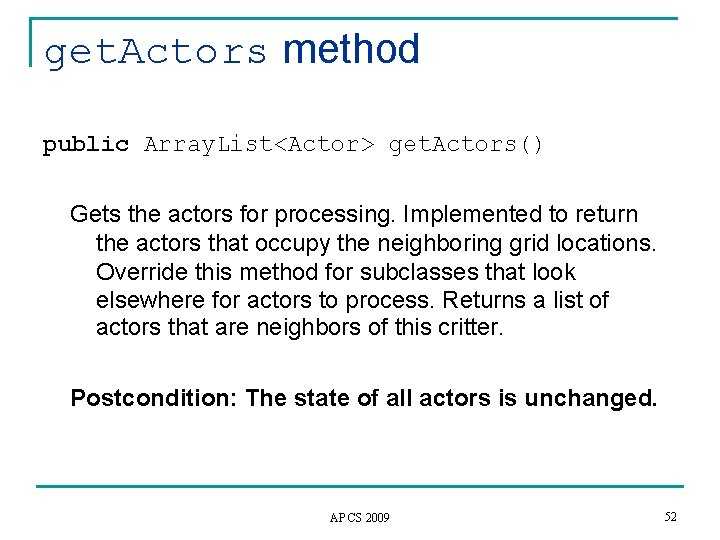
get. Actors method public Array. List<Actor> get. Actors() Gets the actors for processing. Implemented to return the actors that occupy the neighboring grid locations. Override this method for subclasses that look elsewhere for actors to process. Returns a list of actors that are neighbors of this critter. Postcondition: The state of all actors is unchanged. AP CS 2009 52
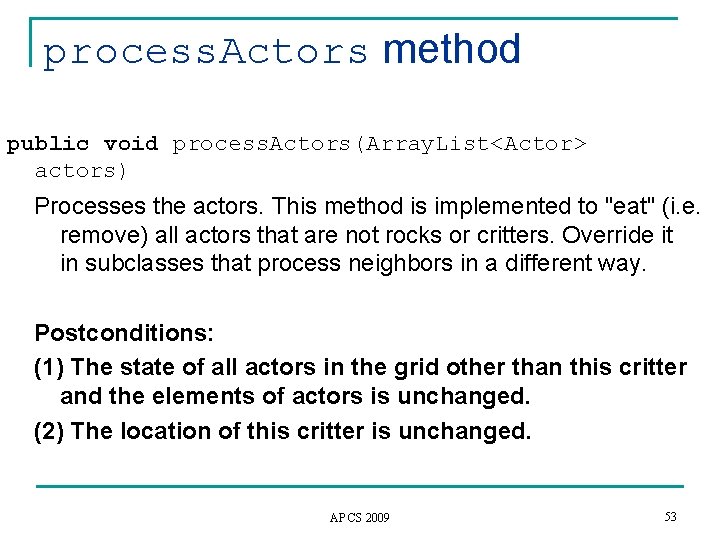
process. Actors method public void process. Actors(Array. List<Actor> actors) Processes the actors. This method is implemented to "eat" (i. e. remove) all actors that are not rocks or critters. Override it in subclasses that process neighbors in a different way. Postconditions: (1) The state of all actors in the grid other than this critter and the elements of actors is unchanged. (2) The location of this critter is unchanged. AP CS 2009 53
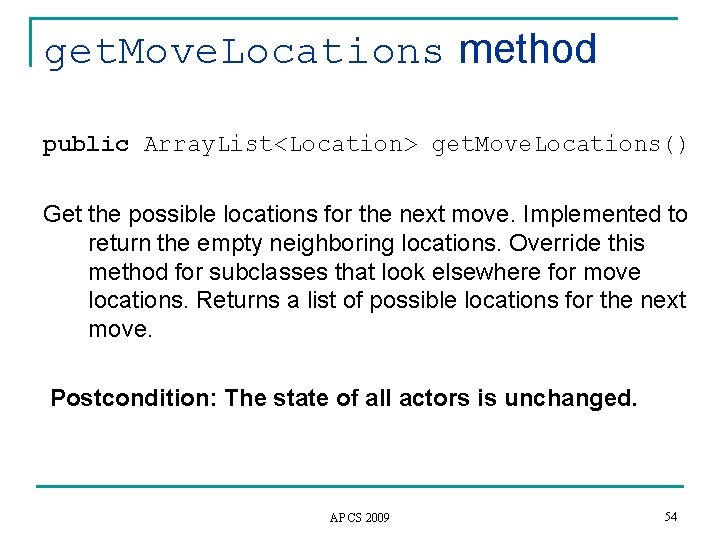
get. Move. Locations method public Array. List<Location> get. Move. Locations() Get the possible locations for the next move. Implemented to return the empty neighboring locations. Override this method for subclasses that look elsewhere for move locations. Returns a list of possible locations for the next move. Postcondition: The state of all actors is unchanged. AP CS 2009 54
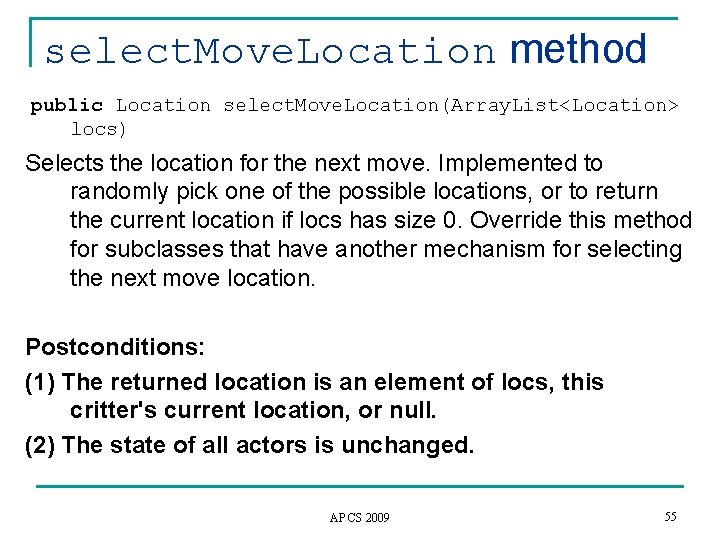
select. Move. Location method public Location select. Move. Location(Array. List<Location> locs) Selects the location for the next move. Implemented to randomly pick one of the possible locations, or to return the current location if locs has size 0. Override this method for subclasses that have another mechanism for selecting the next move location. Postconditions: (1) The returned location is an element of locs, this critter's current location, or null. (2) The state of all actors is unchanged. AP CS 2009 55
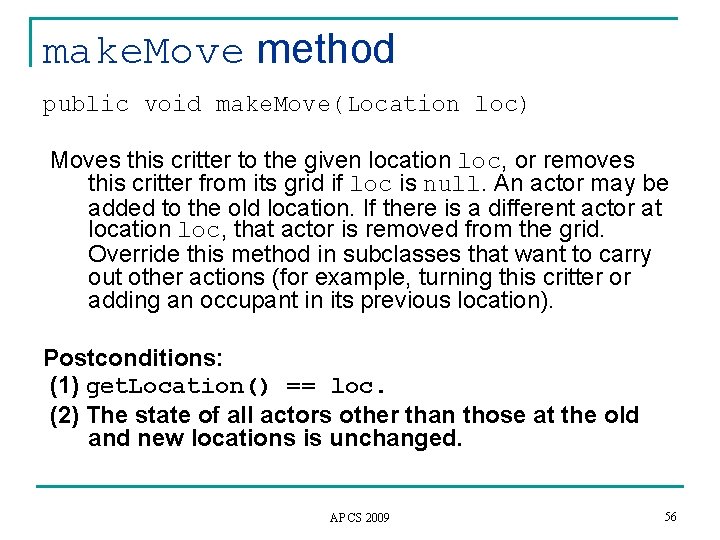
make. Move method public void make. Move(Location loc) Moves this critter to the given location loc, or removes this critter from its grid if loc is null. An actor may be added to the old location. If there is a different actor at location loc, that actor is removed from the grid. Override this method in subclasses that want to carry out other actions (for example, turning this critter or adding an occupant in its previous location). Postconditions: (1) get. Location() == loc. (2) The state of all actors other than those at the old and new locations is unchanged. AP CS 2009 56
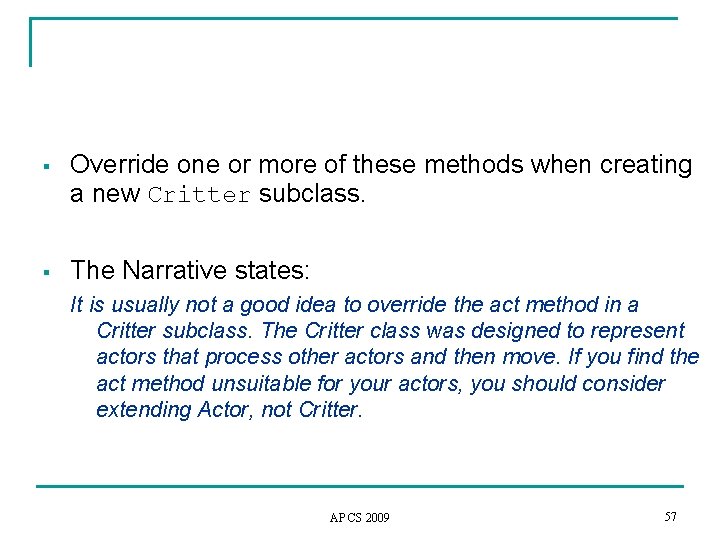
§ Override one or more of these methods when creating a new Critter subclass. § The Narrative states: It is usually not a good idea to override the act method in a Critter subclass. The Critter class was designed to represent actors that process other actors and then move. If you find the act method unsuitable for your actors, you should consider extending Actor, not Critter. AP CS 2009 57
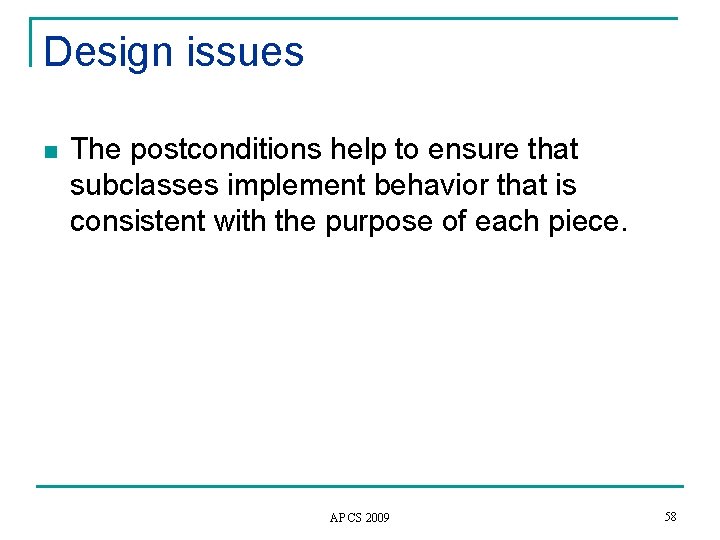
Design issues n The postconditions help to ensure that subclasses implement behavior that is consistent with the purpose of each piece. AP CS 2009 58
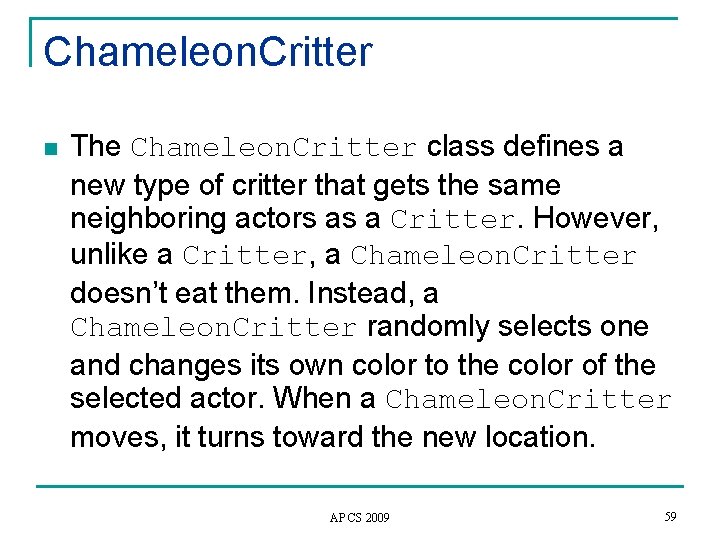
Chameleon. Critter n The Chameleon. Critter class defines a new type of critter that gets the same neighboring actors as a Critter. However, unlike a Critter, a Chameleon. Critter doesn’t eat them. Instead, a Chameleon. Critter randomly selects one and changes its own color to the color of the selected actor. When a Chameleon. Critter moves, it turns toward the new location. AP CS 2009 59
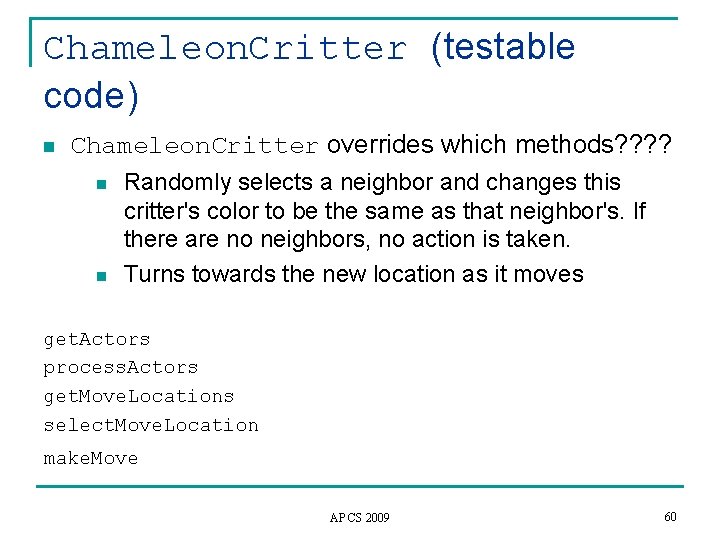
Chameleon. Critter (testable code) n Chameleon. Critter overrides which methods? ? n n Randomly selects a neighbor and changes this critter's color to be the same as that neighbor's. If there are no neighbors, no action is taken. Turns towards the new location as it moves get. Actors process. Actors get. Move. Locations select. Move. Location make. Move AP CS 2009 60
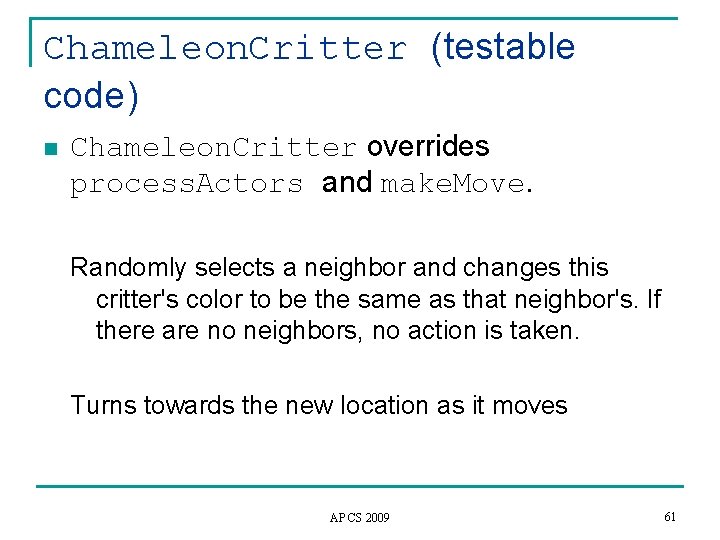
Chameleon. Critter (testable code) n Chameleon. Critter overrides process. Actors and make. Move. Randomly selects a neighbor and changes this critter's color to be the same as that neighbor's. If there are no neighbors, no action is taken. Turns towards the new location as it moves AP CS 2009 61
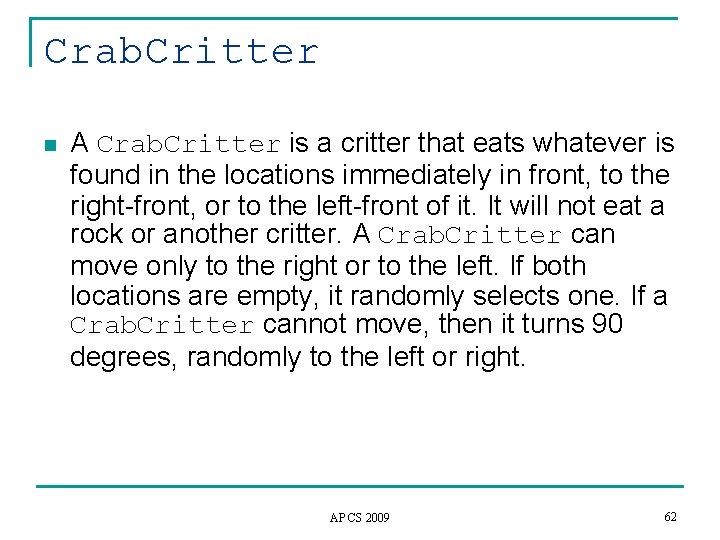
Crab. Critter n A Crab. Critter is a critter that eats whatever is found in the locations immediately in front, to the right-front, or to the left-front of it. It will not eat a rock or another critter. A Crab. Critter can move only to the right or to the left. If both locations are empty, it randomly selects one. If a Crab. Critter cannot move, then it turns 90 degrees, randomly to the left or right. AP CS 2009 62
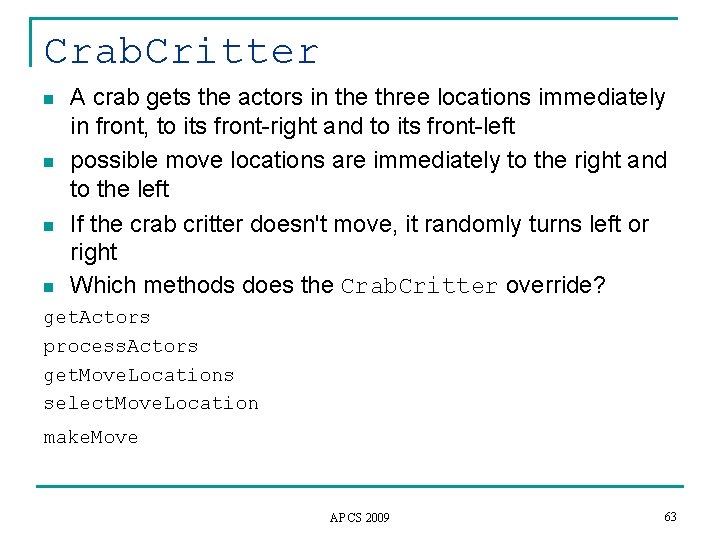
Crab. Critter n n A crab gets the actors in the three locations immediately in front, to its front-right and to its front-left possible move locations are immediately to the right and to the left If the crab critter doesn't move, it randomly turns left or right Which methods does the Crab. Critter override? get. Actors process. Actors get. Move. Locations select. Move. Location make. Move AP CS 2009 63
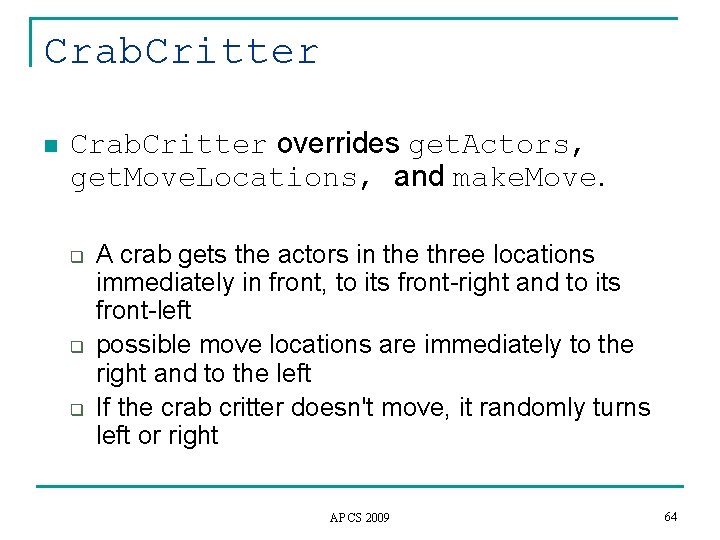
Crab. Critter n Crab. Critter overrides get. Actors, get. Move. Locations, and make. Move. q q q A crab gets the actors in the three locations immediately in front, to its front-right and to its front-left possible move locations are immediately to the right and to the left If the crab critter doesn't move, it randomly turns left or right AP CS 2009 64
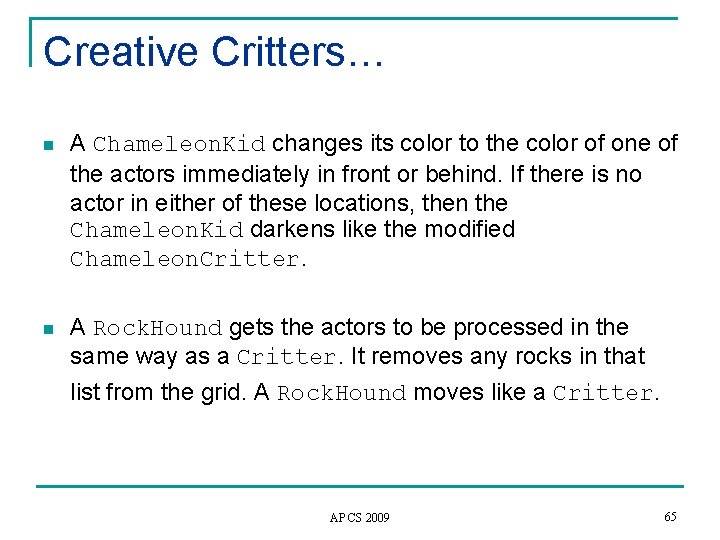
Creative Critters… n A Chameleon. Kid changes its color to the color of one of the actors immediately in front or behind. If there is no actor in either of these locations, then the Chameleon. Kid darkens like the modified Chameleon. Critter. n A Rock. Hound gets the actors to be processed in the same way as a Critter. It removes any rocks in that list from the grid. A Rock. Hound moves like a Critter. AP CS 2009 65
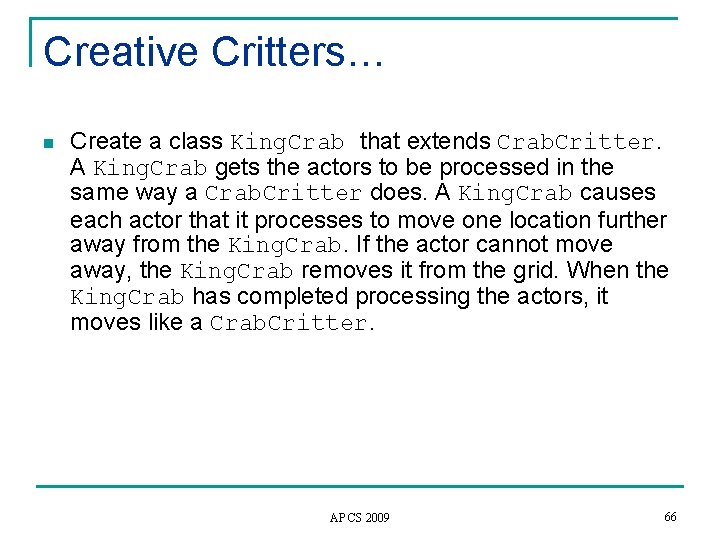
Creative Critters… n Create a class King. Crab that extends Crab. Critter. A King. Crab gets the actors to be processed in the same way a Crab. Critter does. A King. Crab causes each actor that it processes to move one location further away from the King. Crab. If the actor cannot move away, the King. Crab removes it from the grid. When the King. Crab has completed processing the actors, it moves like a Crab. Critter. AP CS 2009 66
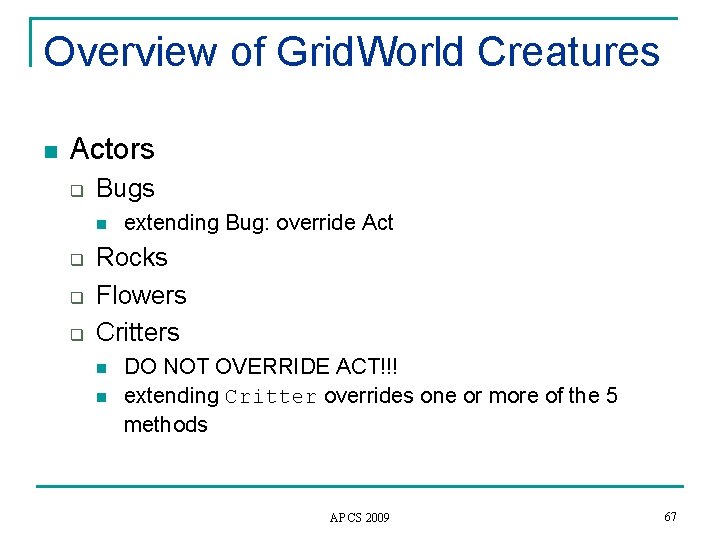
Overview of Grid. World Creatures n Actors q Bugs n q q q extending Bug: override Act Rocks Flowers Critters n n DO NOT OVERRIDE ACT!!! extending Critter overrides one or more of the 5 methods AP CS 2009 67
Gridworld case study
Grid world case study
Best case worst case average case
Hershey's erp failure
World bank case study
Pin grid array
Hát kết hợp bộ gõ cơ thể
Ng-html
Bổ thể
Tỉ lệ cơ thể trẻ em
Chó sói
Chụp tư thế worms-breton
Hát lên người ơi alleluia
Các môn thể thao bắt đầu bằng tiếng nhảy
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Cong thức tính động năng
Trời xanh đây là của chúng ta thể thơ
Cách giải mật thư tọa độ
Phép trừ bù
Phản ứng thế ankan
Các châu lục và đại dương trên thế giới
Thơ thất ngôn tứ tuyệt đường luật
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Cái miệng xinh xinh thế chỉ nói điều hay thôi
Vẽ hình chiếu vuông góc của vật thể sau
Biện pháp chống mỏi cơ
đặc điểm cơ thể của người tối cổ
V. c c
Vẽ hình chiếu đứng bằng cạnh của vật thể
Vẽ hình chiếu vuông góc của vật thể sau
Thẻ vin
đại từ thay thế
điện thế nghỉ
Tư thế ngồi viết
Diễn thế sinh thái là
Các loại đột biến cấu trúc nhiễm sắc thể
Số.nguyên tố
Tư thế ngồi viết
Lời thề hippocrates
Thiếu nhi thế giới liên hoan
ưu thế lai là gì
Khi nào hổ mẹ dạy hổ con săn mồi
Khi nào hổ mẹ dạy hổ con săn mồi
Sơ đồ cơ thể người
Từ ngữ thể hiện lòng nhân hậu
Thế nào là mạng điện lắp đặt kiểu nổi
Long case and short case
Average case complexity of binary search
Glennan building cwru
Bubble sort best case and worst case
Bubble sort best case and worst case
Bubble sort best case and worst case
The ambiguous case for the law of sines
Zara information system
Yelp elite benefits
The kf case study
Beth anders
Action research vs case study
Volkswagen of america: managing it priorities
Plant and machinery valuation report
Mini case study example
Starbucks foreign direct investment
Case study 11
Notasi uml
Illustrative case study example
Tuna for lunch case study