Graphics Review Drawing Panel class Drawing Panel panel
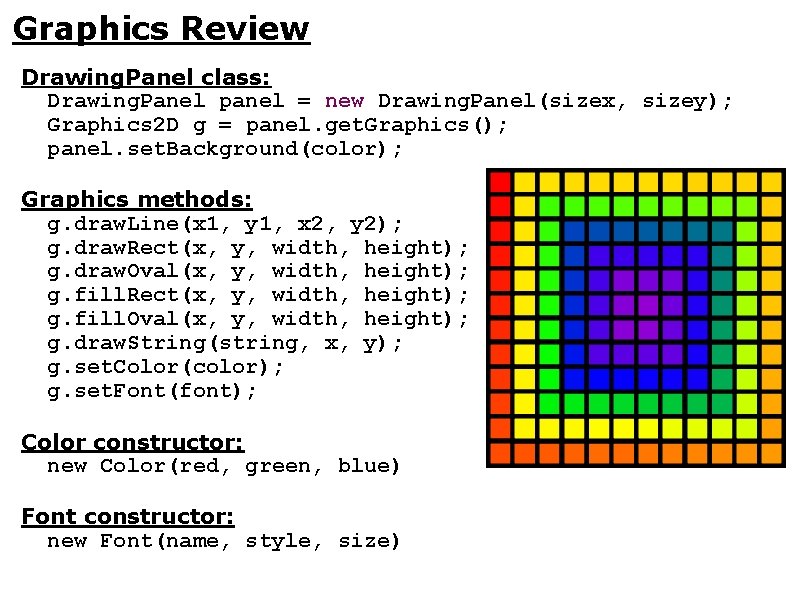
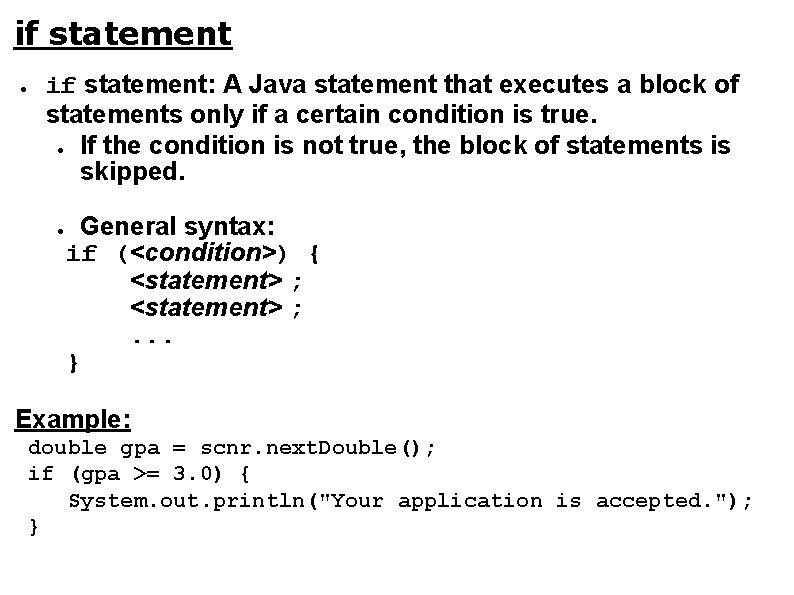
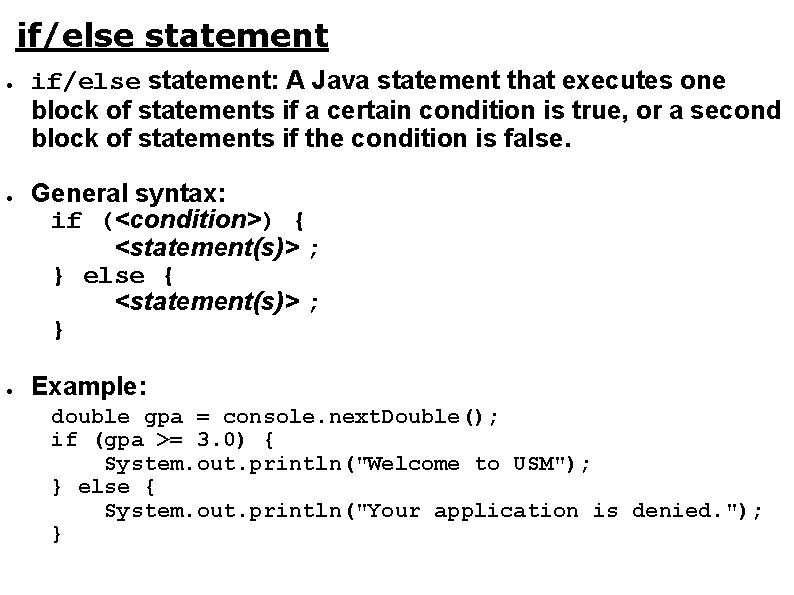
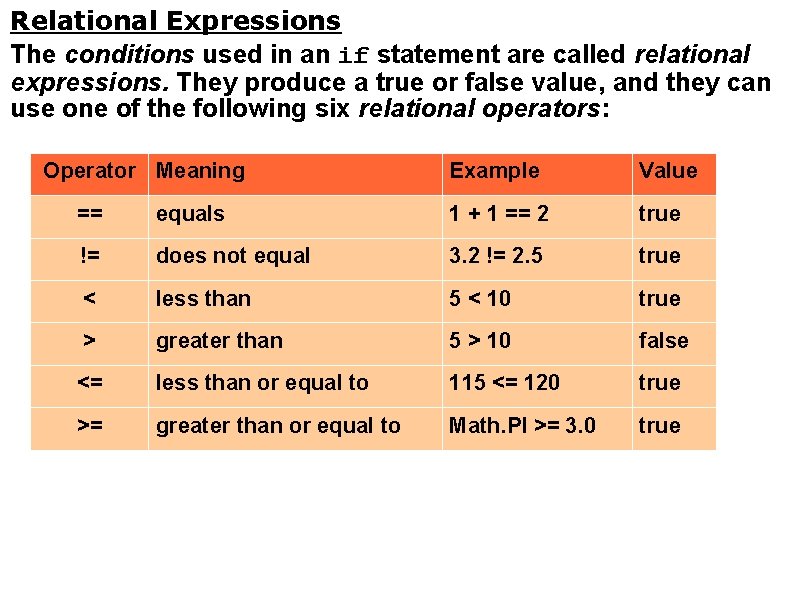
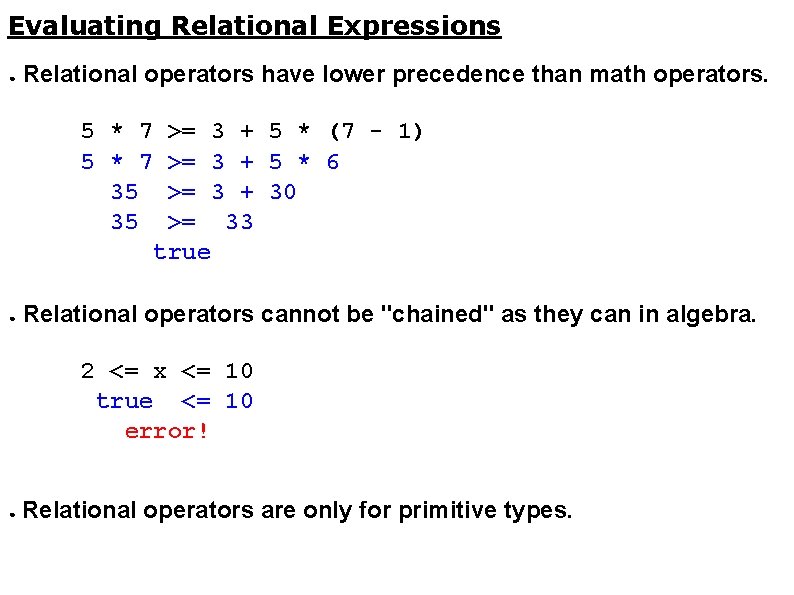
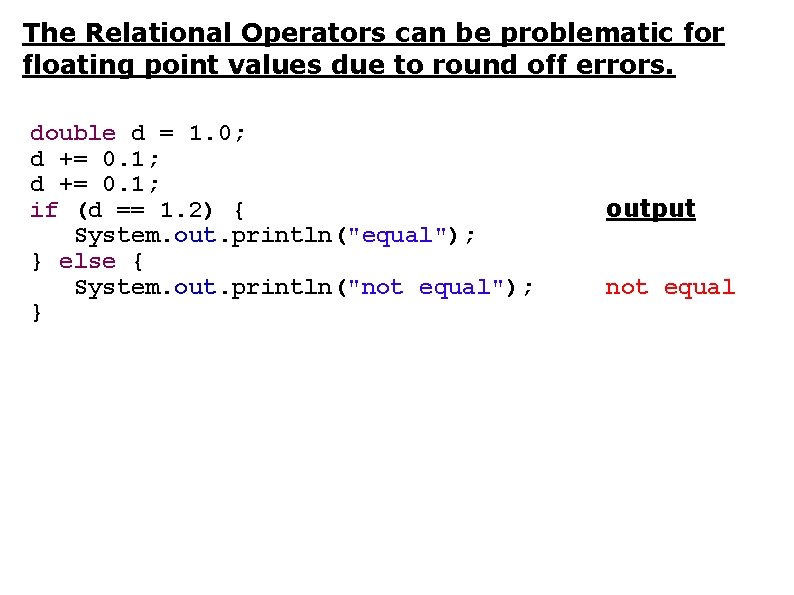
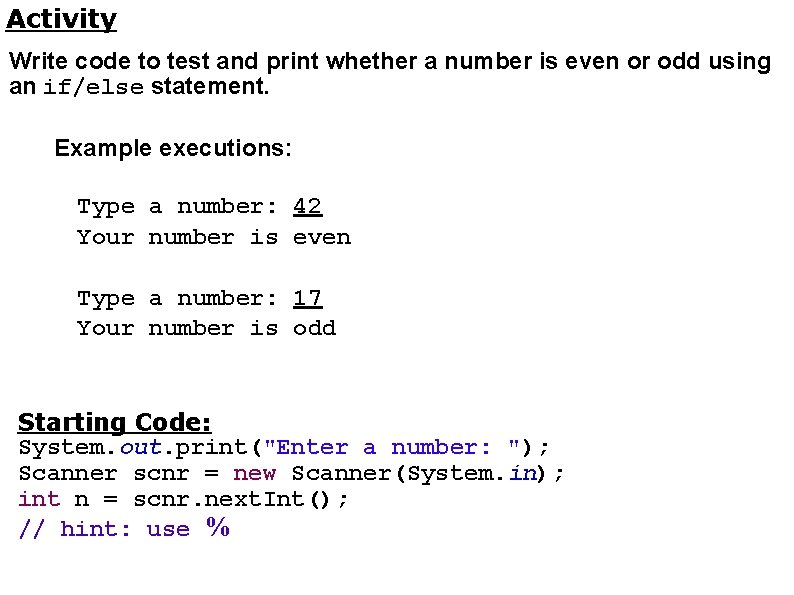
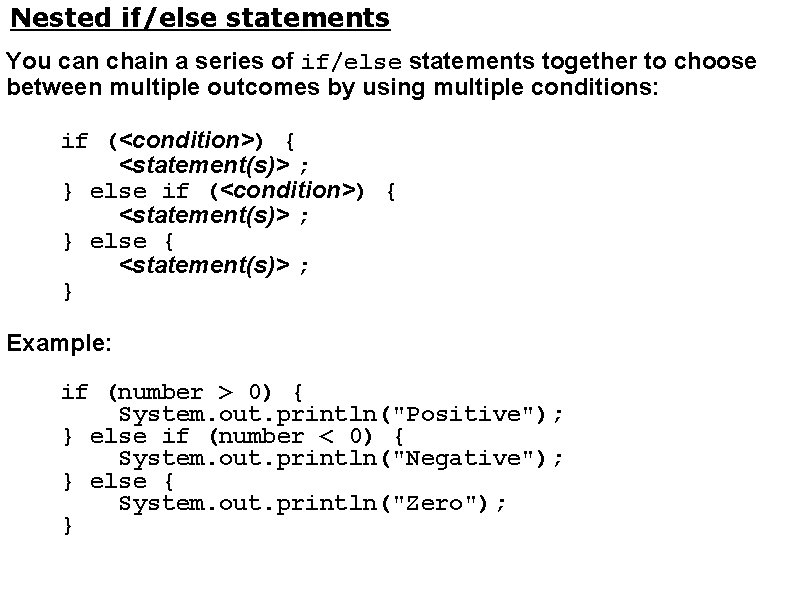
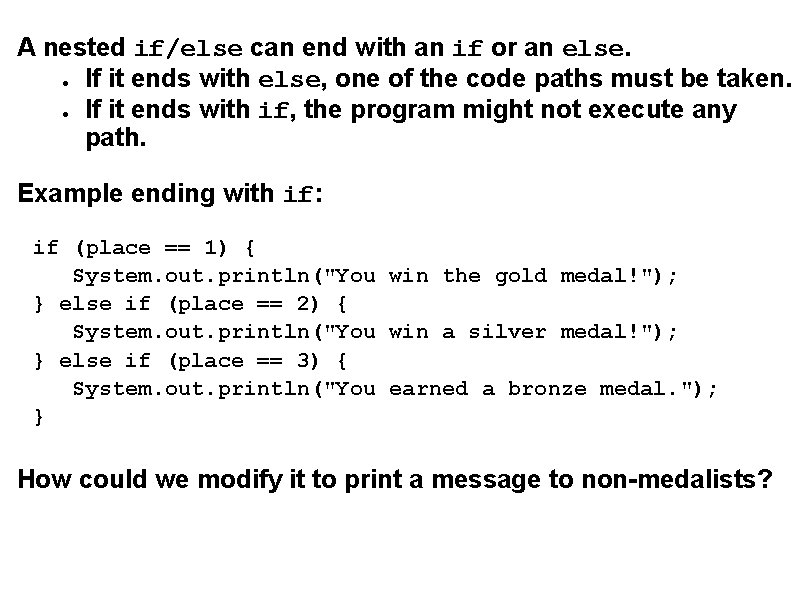
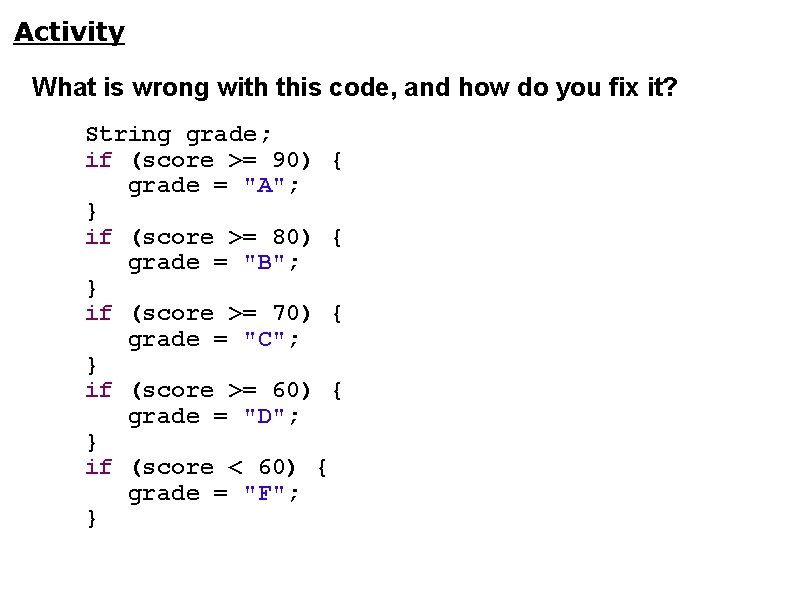
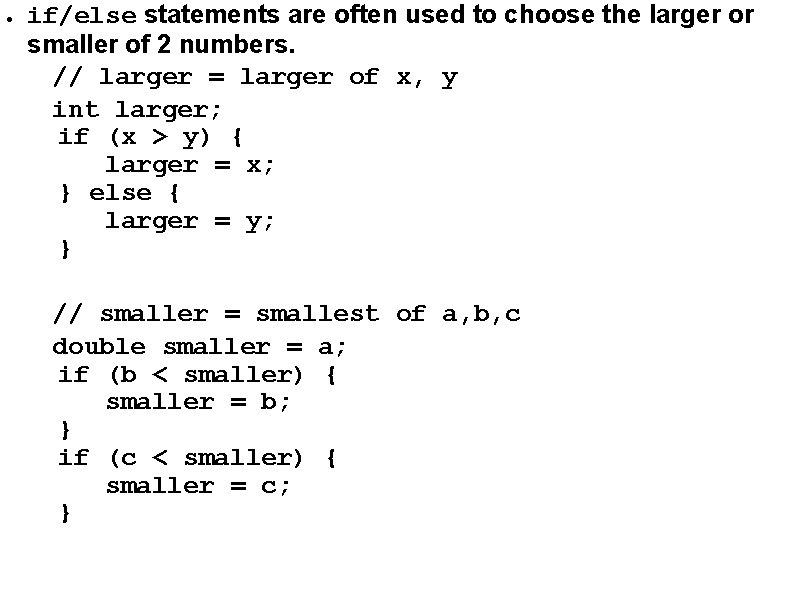
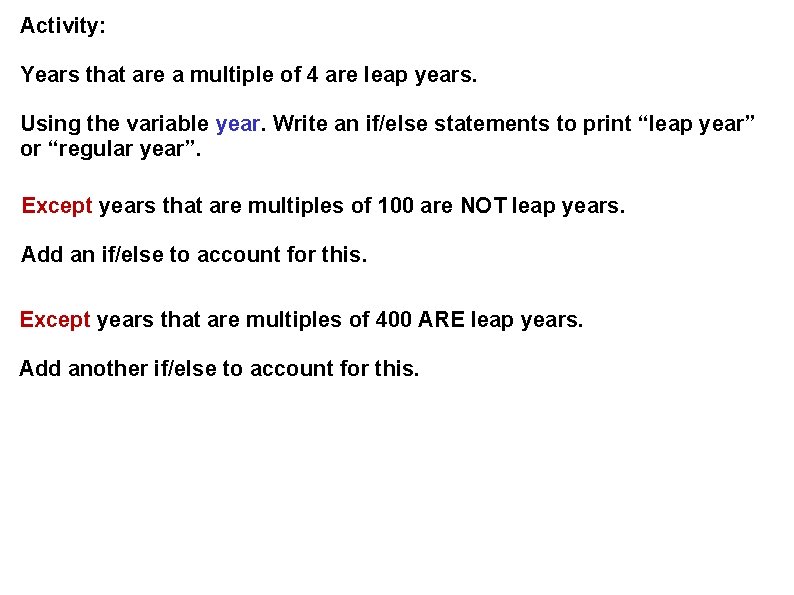
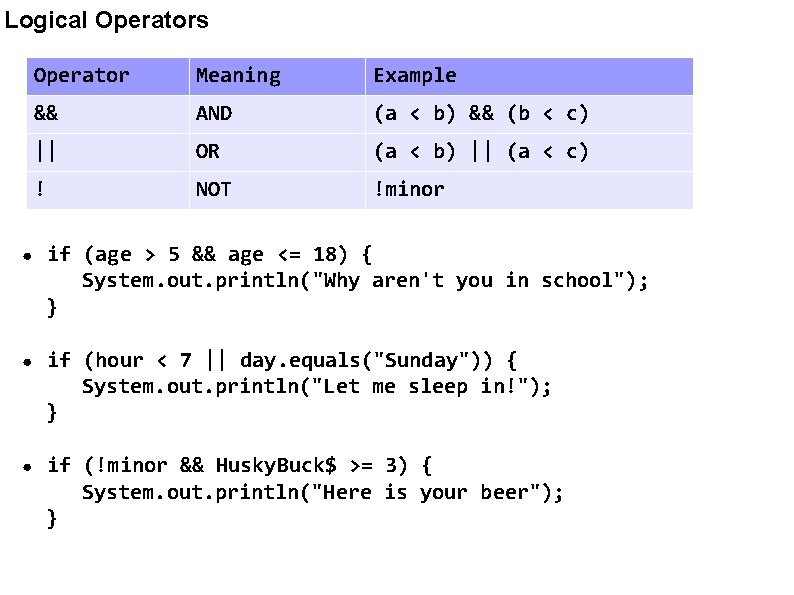
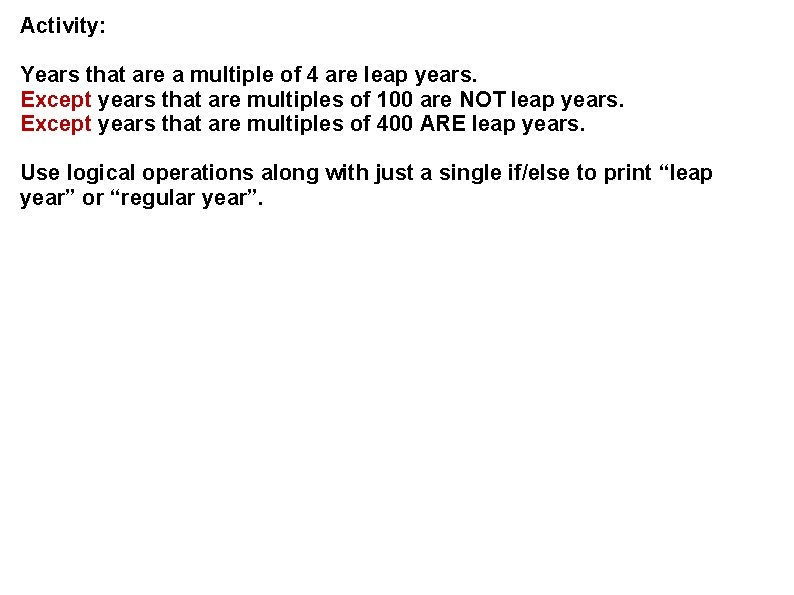
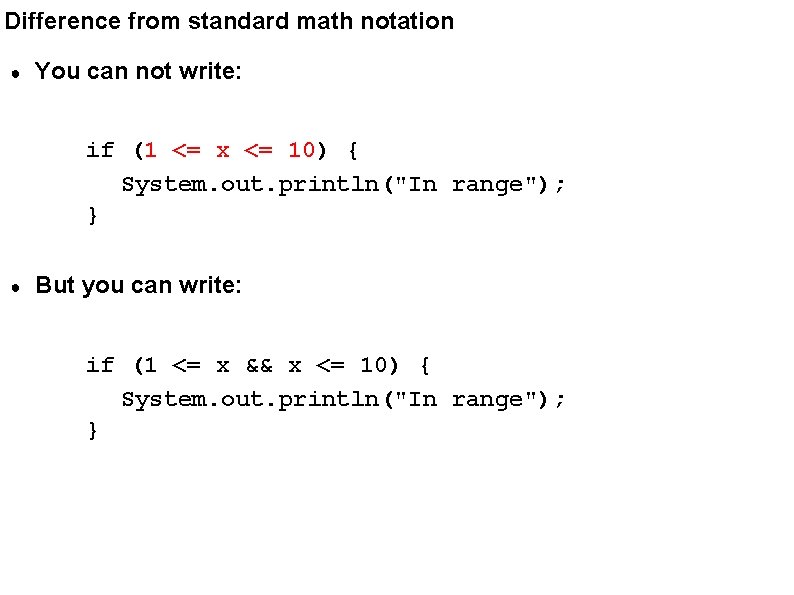
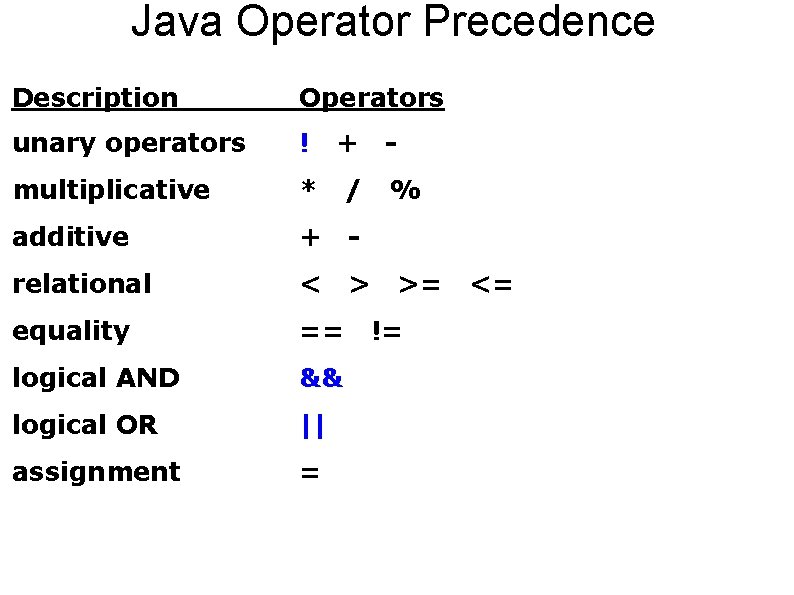
- Slides: 16
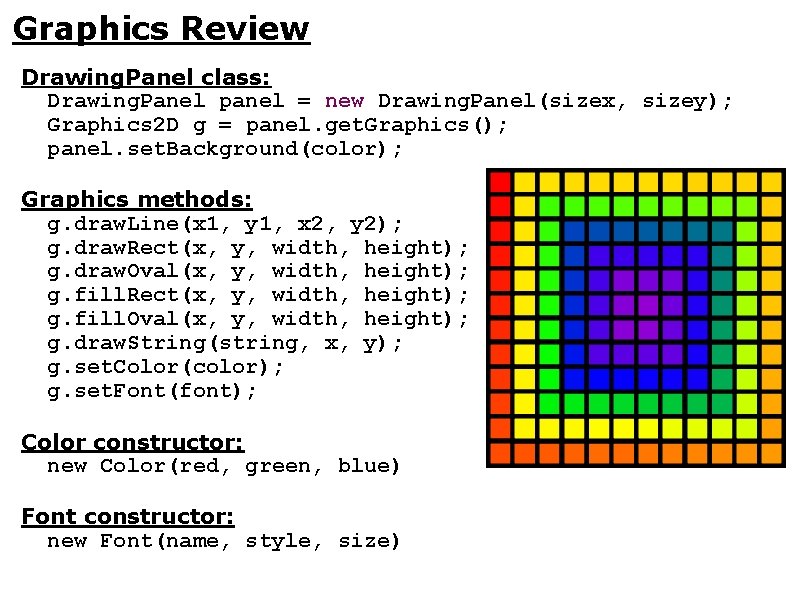
Graphics Review Drawing. Panel class: Drawing. Panel panel = new Drawing. Panel(sizex, sizey); Graphics 2 D g = panel. get. Graphics(); panel. set. Background(color); Graphics methods: g. draw. Line(x 1, y 1, x 2, y 2); g. draw. Rect(x, y, width, height); g. draw. Oval(x, y, width, height); g. fill. Rect(x, y, width, height); g. fill. Oval(x, y, width, height); g. draw. String(string, x, y); g. set. Color(color); g. set. Font(font); Color constructor: new Color(red, green, blue) Font constructor: new Font(name, style, size)
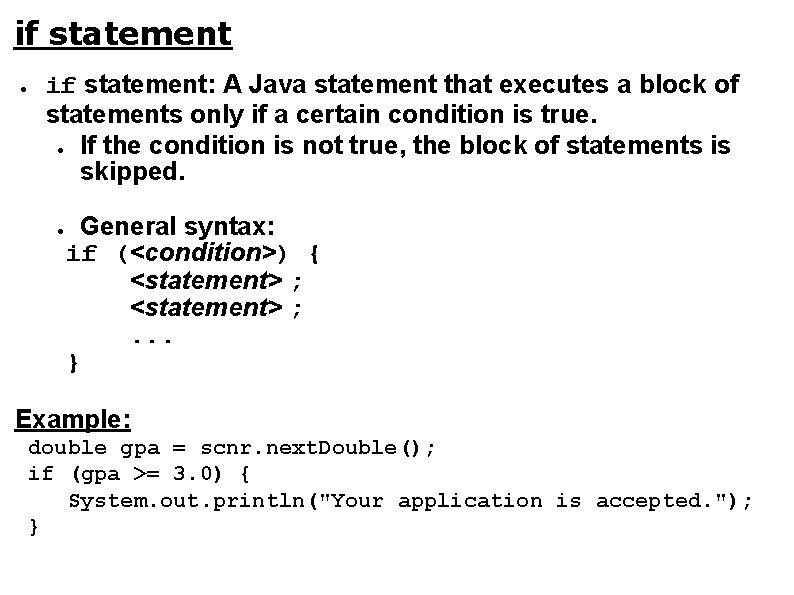
if statement ● if statement: A Java statement that executes a block of statements only if a certain condition is true. ● If the condition is not true, the block of statements is skipped. ● General syntax: if (<condition>) { <statement> ; . . . } Example: double gpa = scnr. next. Double(); if (gpa >= 3. 0) { System. out. println("Your application is accepted. "); }
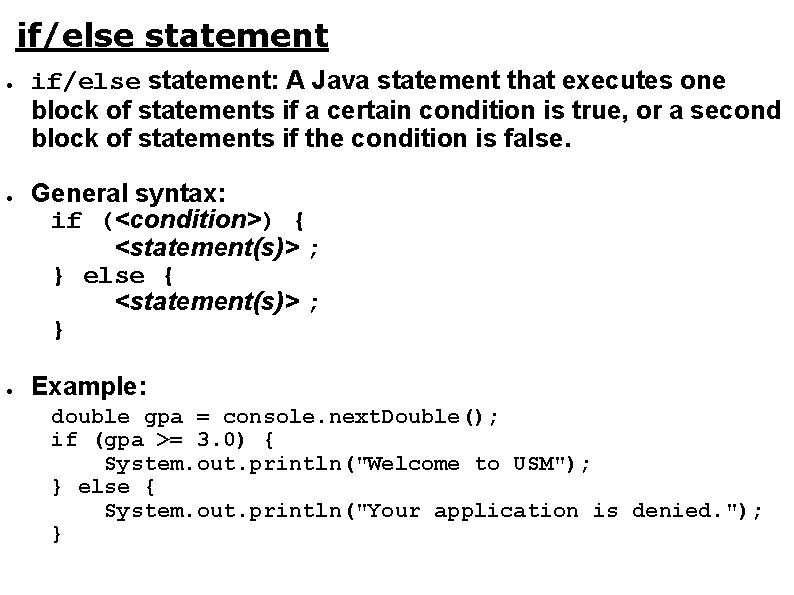
if/else statement ● ● ● if/else statement: A Java statement that executes one block of statements if a certain condition is true, or a second block of statements if the condition is false. General syntax: if (<condition>) { <statement(s)> ; } else { <statement(s)> ; } Example: double gpa = console. next. Double(); if (gpa >= 3. 0) { System. out. println("Welcome to USM"); } else { System. out. println("Your application is denied. "); }
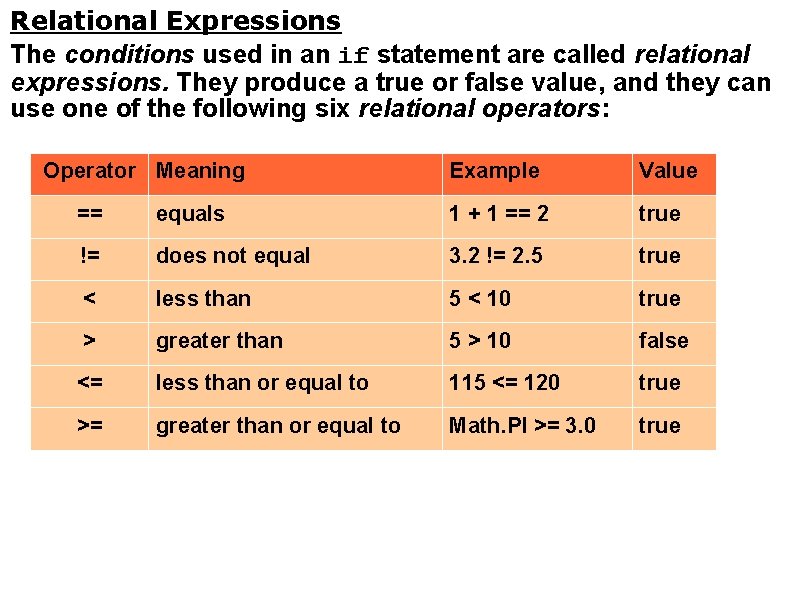
Relational Expressions The conditions used in an if statement are called relational expressions. They produce a true or false value, and they can use one of the following six relational operators: Operator Meaning Example Value == equals 1 + 1 == 2 true != does not equal 3. 2 != 2. 5 true < less than 5 < 10 true > greater than 5 > 10 false <= less than or equal to 115 <= 120 true >= greater than or equal to Math. PI >= 3. 0 true
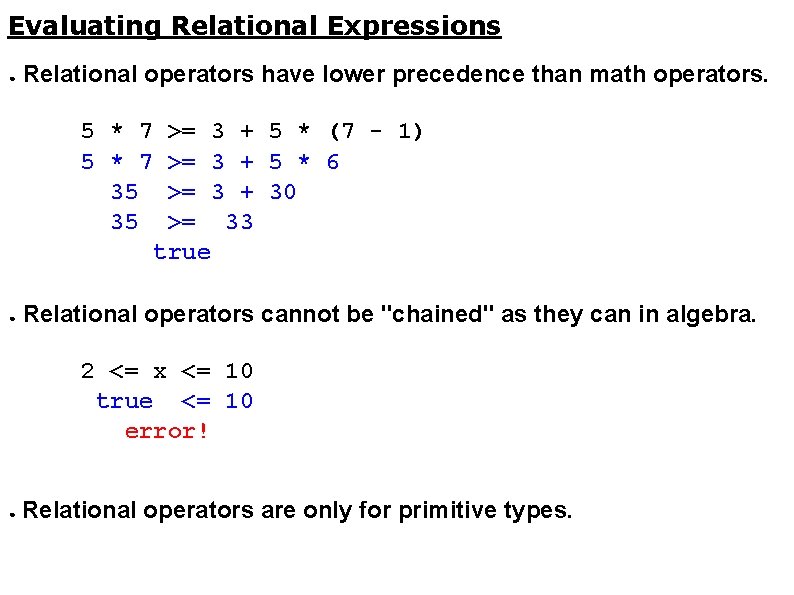
Evaluating Relational Expressions ● Relational operators have lower precedence than math operators. 5 * 7 35 35 >= 3 + 5 * (7 - 1) >= 3 + 5 * 6 >= 3 + 30 >= 33 true ● Relational operators cannot be "chained" as they can in algebra. 2 <= x <= 10 true <= 10 error! ● Relational operators are only for primitive types.
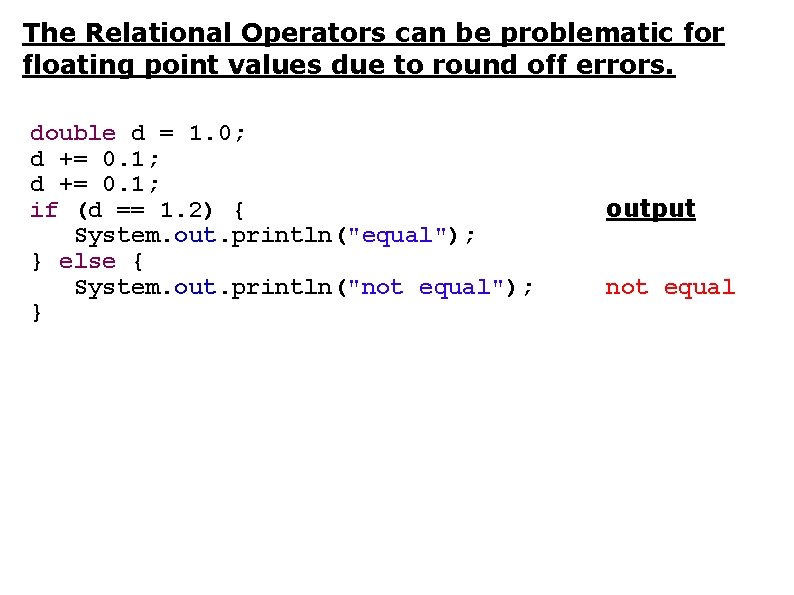
The Relational Operators can be problematic for floating point values due to round off errors. double d = 1. 0; d += 0. 1; if (d == 1. 2) { System. out. println("equal"); } else { System. out. println("not equal"); } output not equal
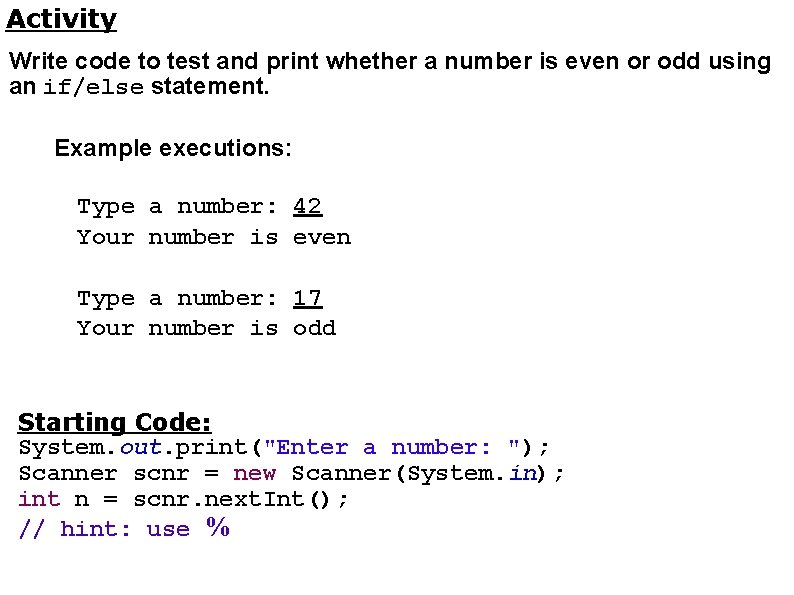
Activity Write code to test and print whether a number is even or odd using an if/else statement. Example executions: Type a number: 42 Your number is even Type a number: 17 Your number is odd Starting Code: System. out. print("Enter a number: "); Scanner scnr = new Scanner(System. in); int n = scnr. next. Int(); // hint: use %
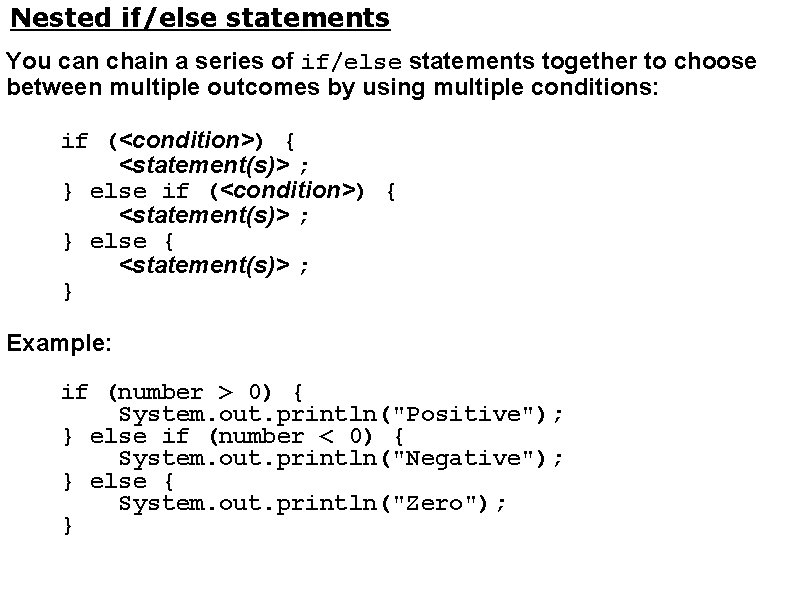
Nested if/else statements You can chain a series of if/else statements together to choose between multiple outcomes by using multiple conditions: if (<condition>) { <statement(s)> ; } else { <statement(s)> ; } Example: if (number > 0) { System. out. println("Positive"); } else if (number < 0) { System. out. println("Negative"); } else { System. out. println("Zero"); }
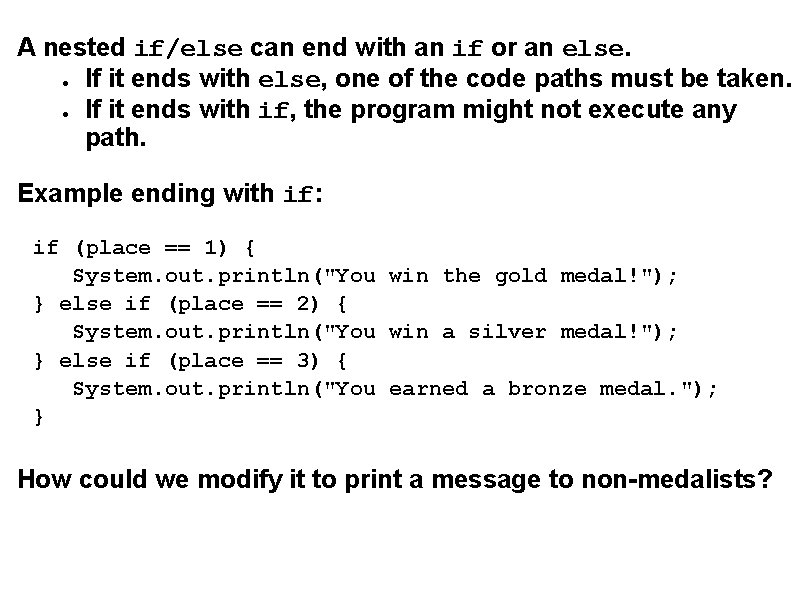
A nested if/else can end with an if or an else. ● If it ends with else, one of the code paths must be taken. ● If it ends with if, the program might not execute any path. Example ending with if: if (place == 1) { System. out. println("You win the gold medal!"); } else if (place == 2) { System. out. println("You win a silver medal!"); } else if (place == 3) { System. out. println("You earned a bronze medal. "); } How could we modify it to print a message to non-medalists?
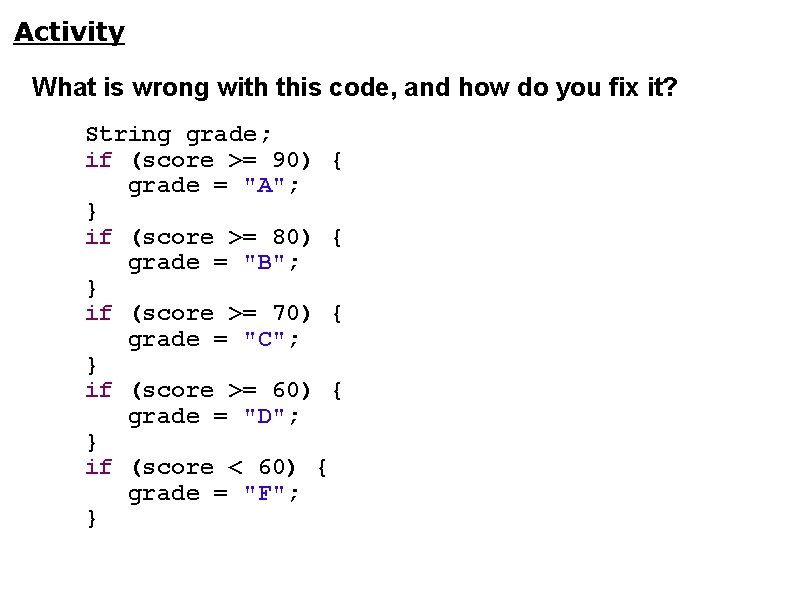
Activity What is wrong with this code, and how do you fix it? String grade; if (score >= 90) { grade = "A"; } if (score >= 80) { grade = "B"; } if (score >= 70) { grade = "C"; } if (score >= 60) { grade = "D"; } if (score < 60) { grade = "F"; }
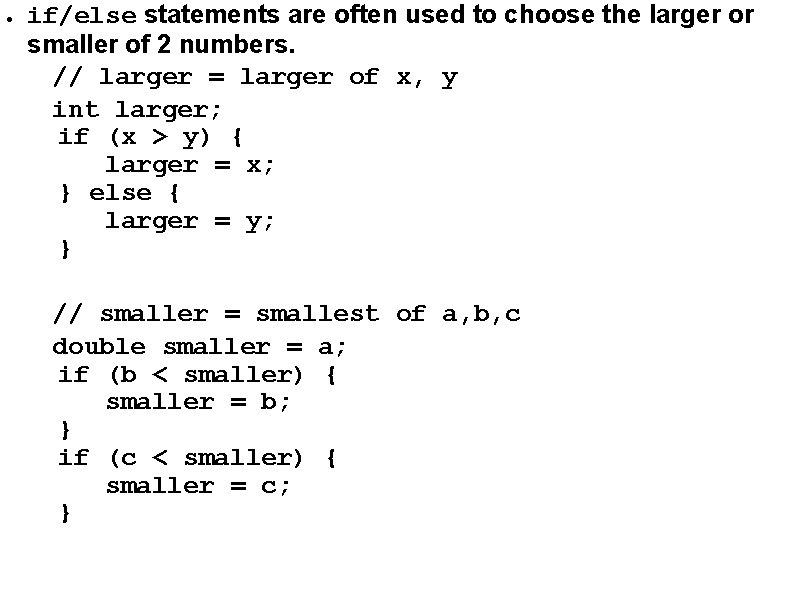
● if/else statements are often used to choose the larger or smaller of 2 numbers. // larger = larger of x, y int larger; if (x > y) { larger = x; } else { larger = y; } // smaller = smallest of a, b, c double smaller = a; if (b < smaller) { smaller = b; } if (c < smaller) { smaller = c; }
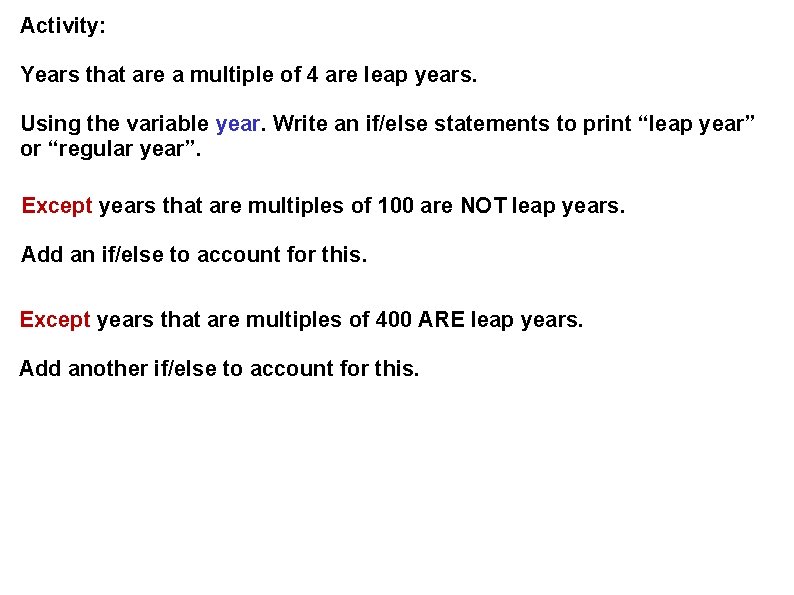
Activity: Years that are a multiple of 4 are leap years. Using the variable year. Write an if/else statements to print “leap year” or “regular year”. Except years that are multiples of 100 are NOT leap years. Add an if/else to account for this. Except years that are multiples of 400 ARE leap years. Add another if/else to account for this.
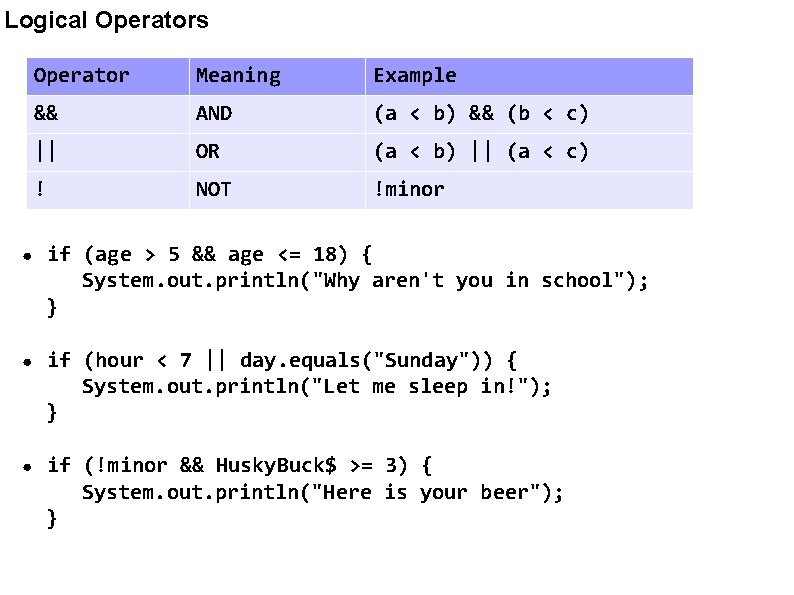
Logical Operators Operator Meaning Example && AND (a < b) && (b < c) || OR (a < b) || (a < c) ! NOT !minor ● if (age > 5 && age <= 18) { System. out. println("Why aren't you in school"); } ● if (hour < 7 || day. equals("Sunday")) { System. out. println("Let me sleep in!"); } ● if (!minor && Husky. Buck$ >= 3) { System. out. println("Here is your beer"); }
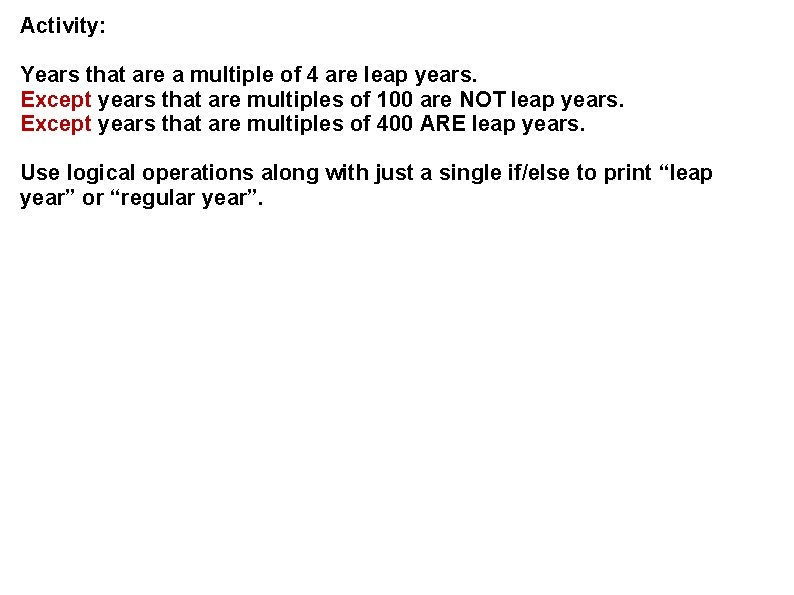
Activity: Years that are a multiple of 4 are leap years. Except years that are multiples of 100 are NOT leap years. Except years that are multiples of 400 ARE leap years. Use logical operations along with just a single if/else to print “leap year” or “regular year”.
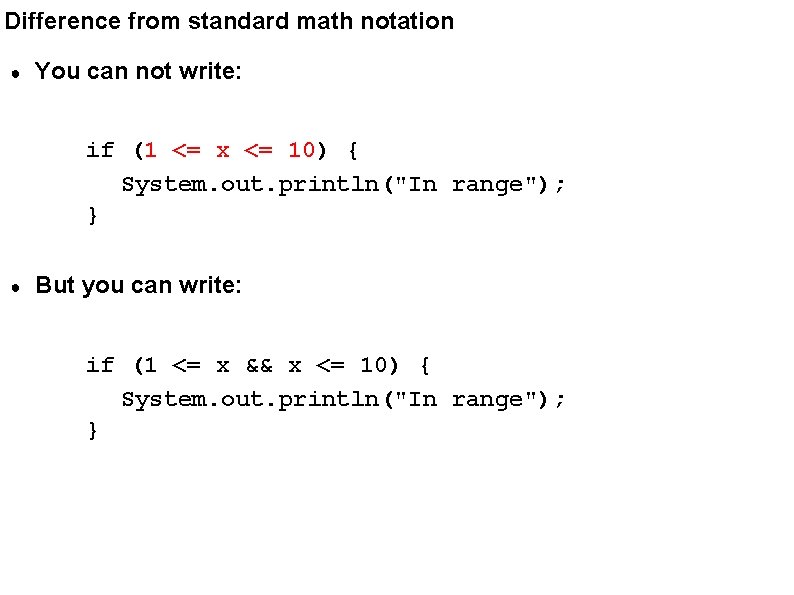
Difference from standard math notation ● You can not write: if (1 <= x <= 10) { System. out. println("In range"); } ● But you can write: if (1 <= x && x <= 10) { System. out. println("In range"); }
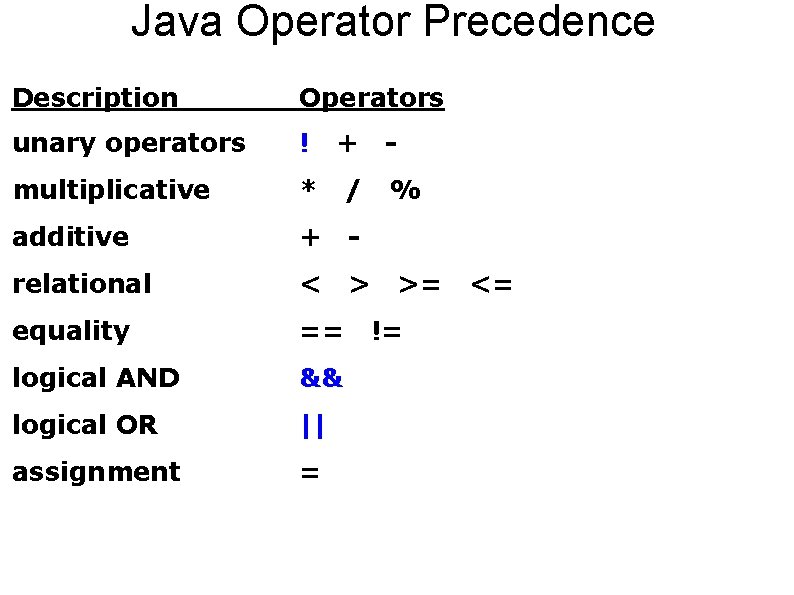
Java Operator Precedence Description Operators unary operators ! + multiplicative * / additive + - relational < > equality == logical AND && logical OR || assignment = % >= != <=