Graphics Programming Polygon Filling Graphics Programming Java Threads
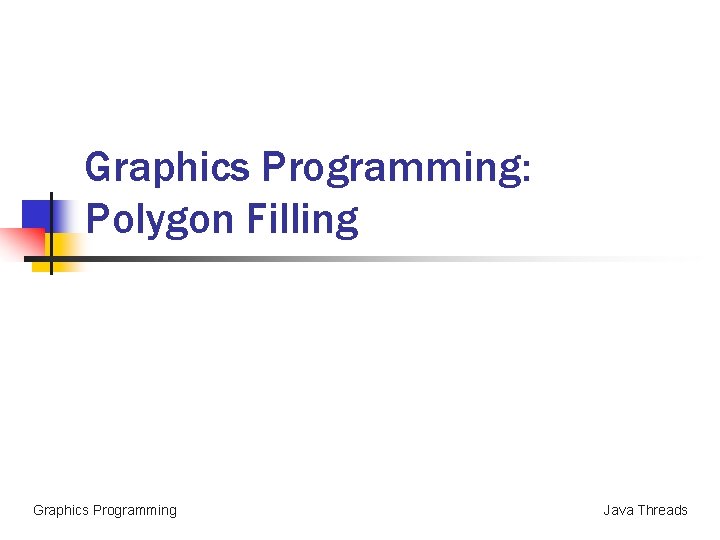
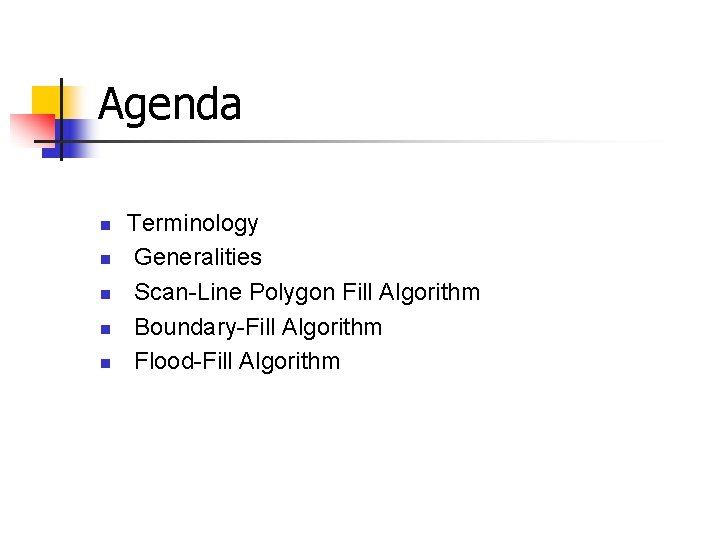
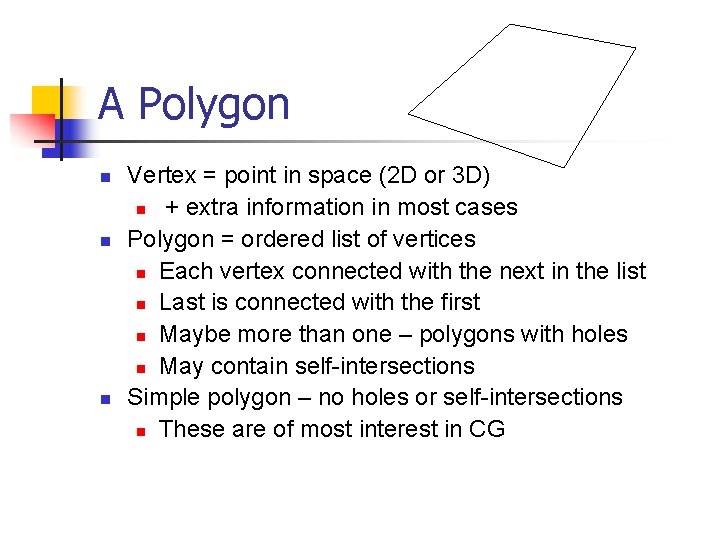
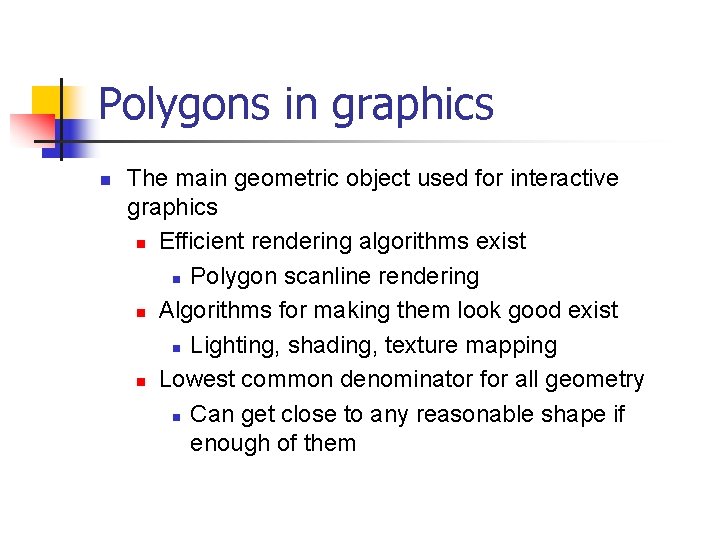
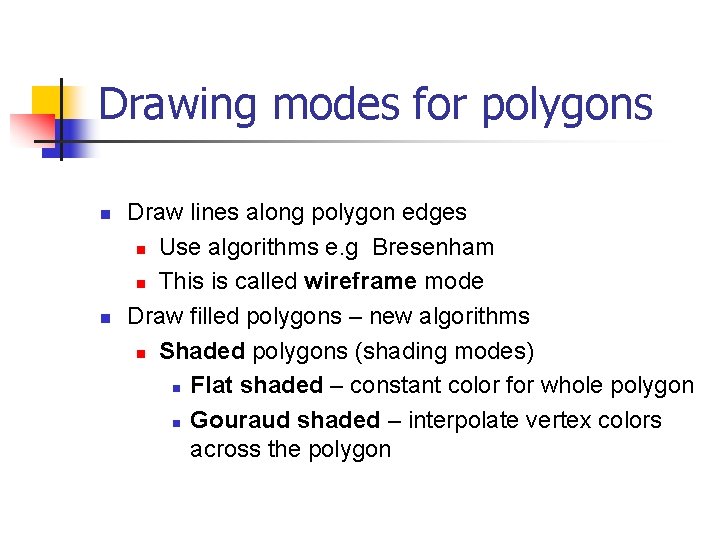
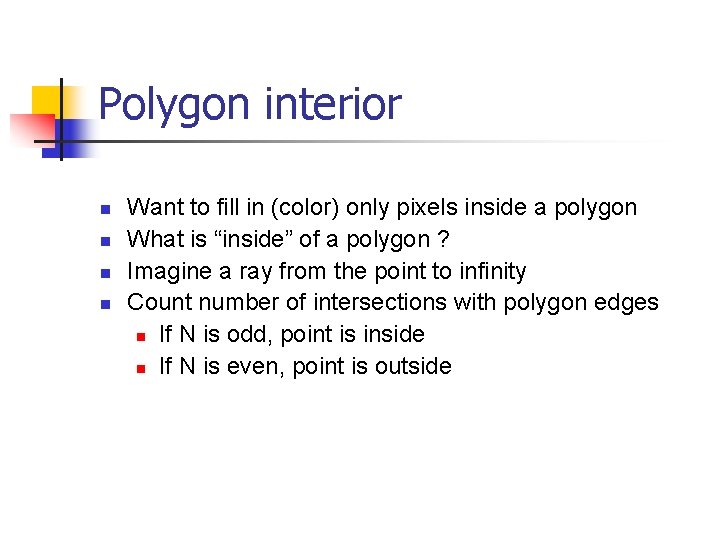
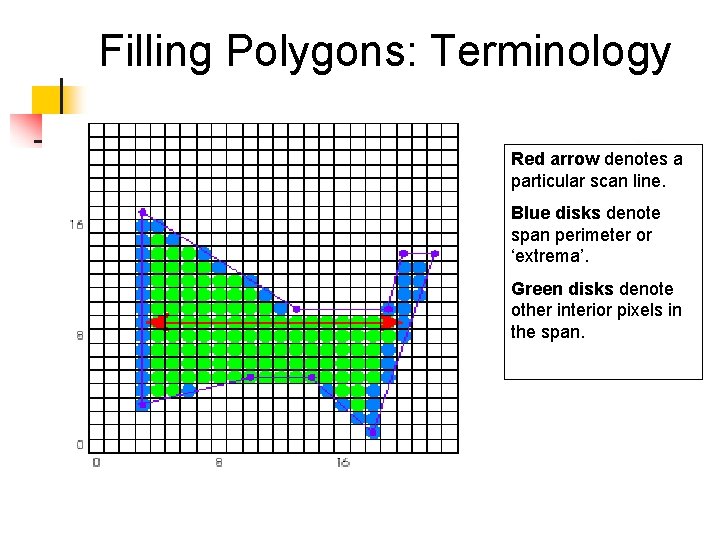
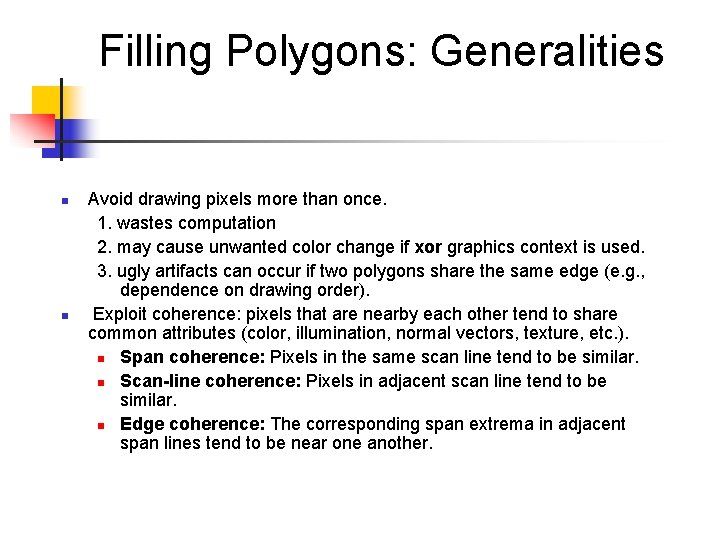
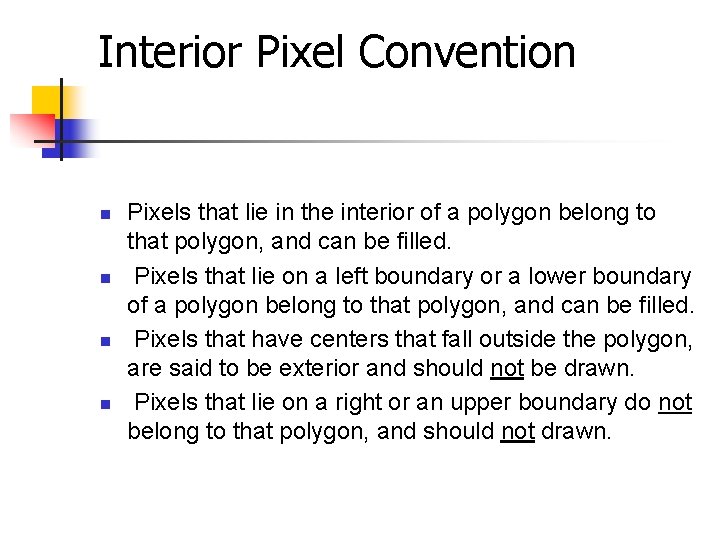
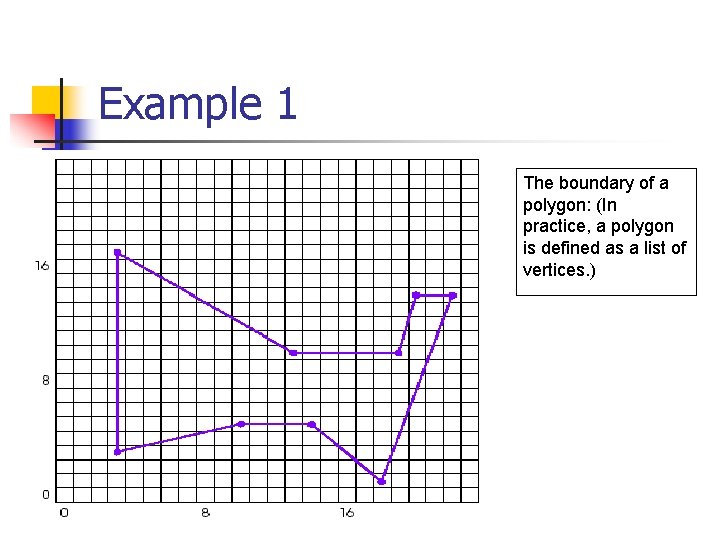
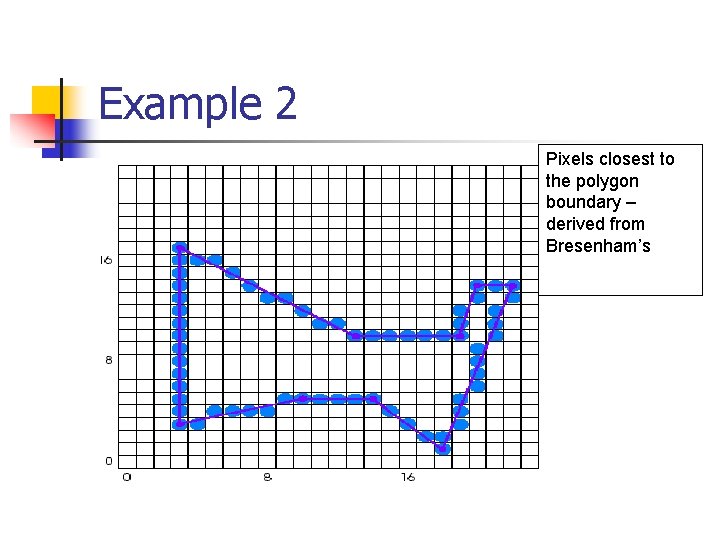
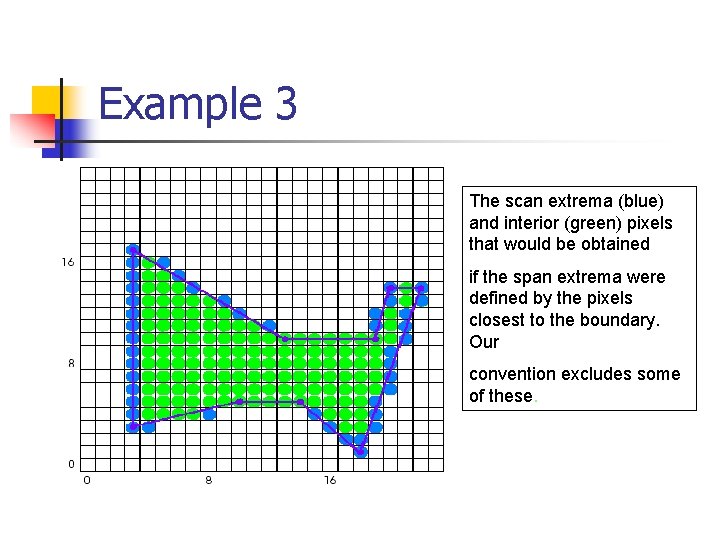
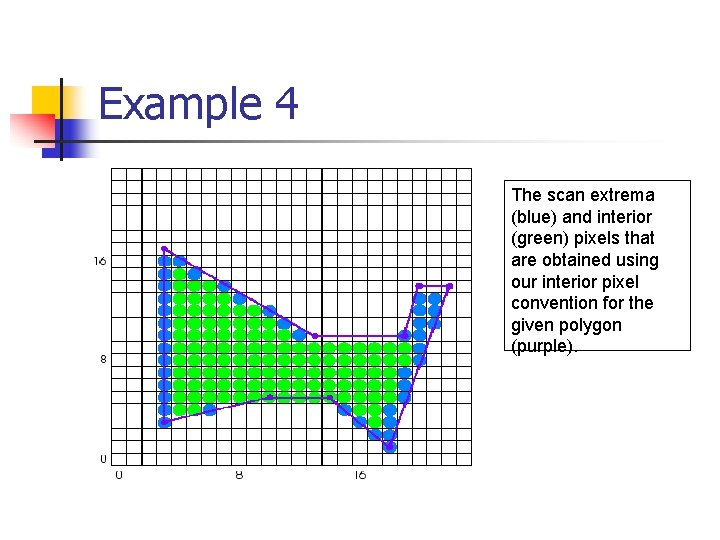
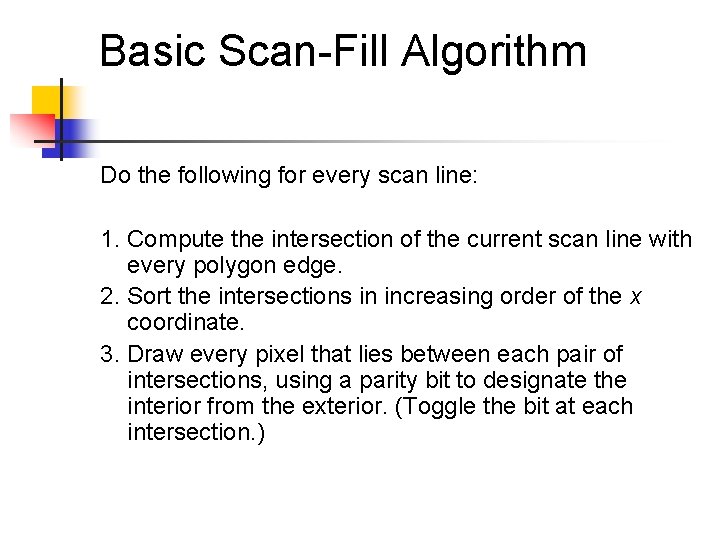
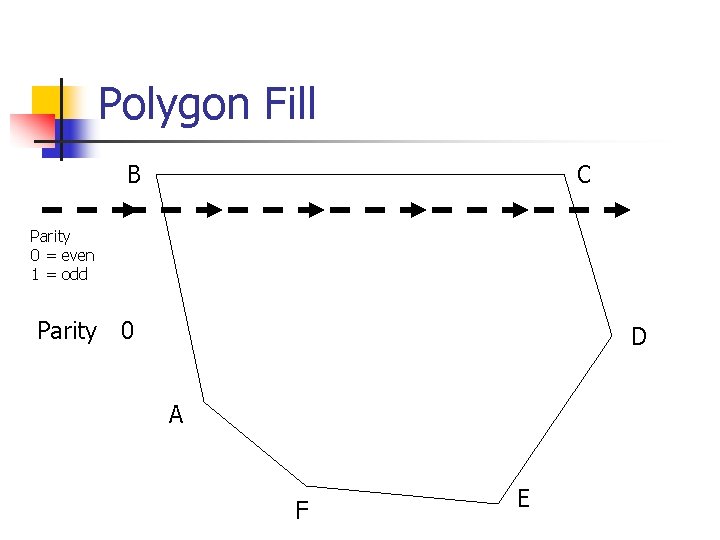
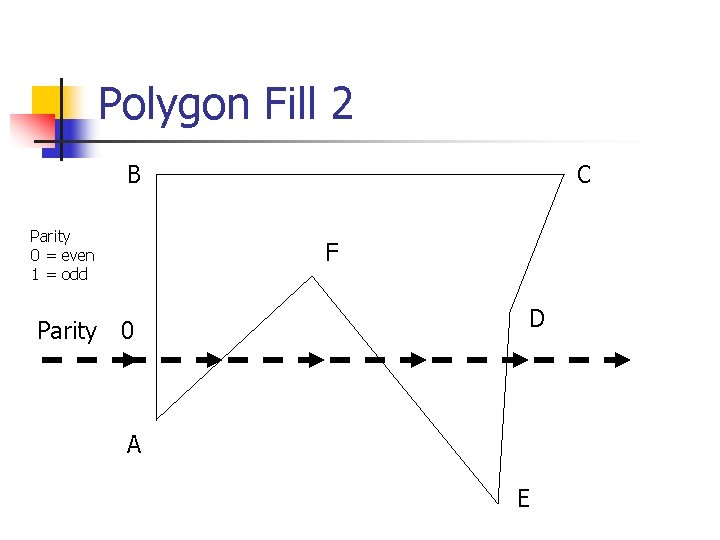
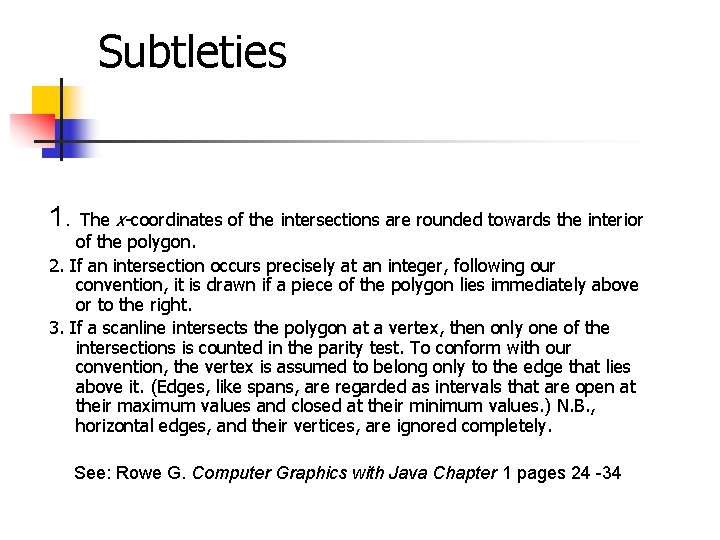
- Slides: 17
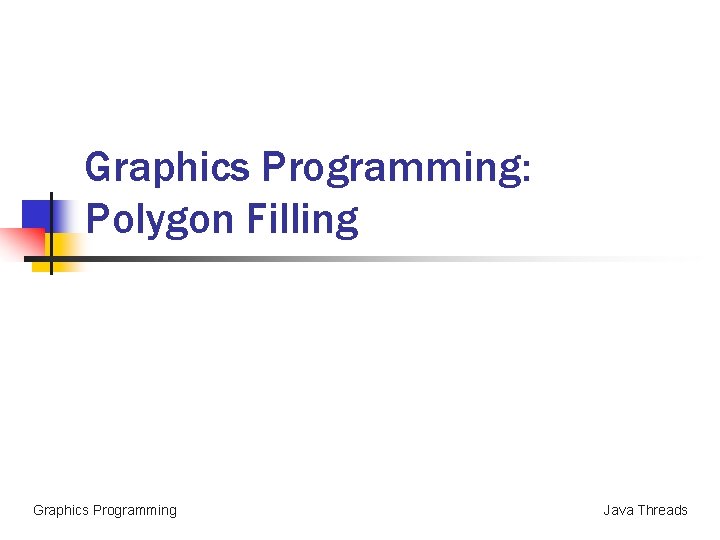
Graphics Programming: Polygon Filling Graphics Programming Java Threads
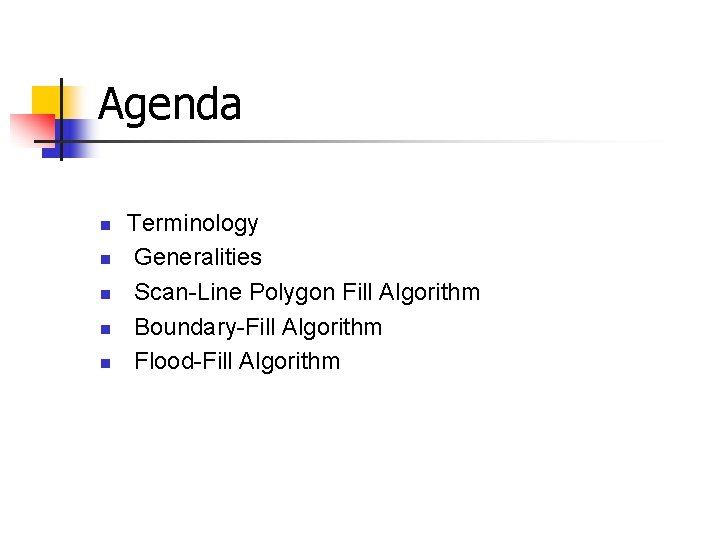
Agenda n n n Terminology Generalities Scan-Line Polygon Fill Algorithm Boundary-Fill Algorithm Flood-Fill Algorithm
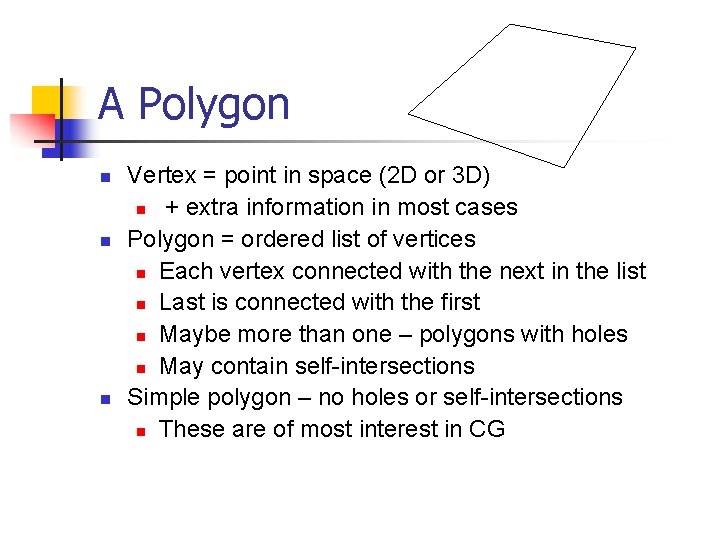
A Polygon n Vertex = point in space (2 D or 3 D) n + extra information in most cases Polygon = ordered list of vertices n Each vertex connected with the next in the list n Last is connected with the first n Maybe more than one – polygons with holes n May contain self-intersections Simple polygon – no holes or self-intersections n These are of most interest in CG
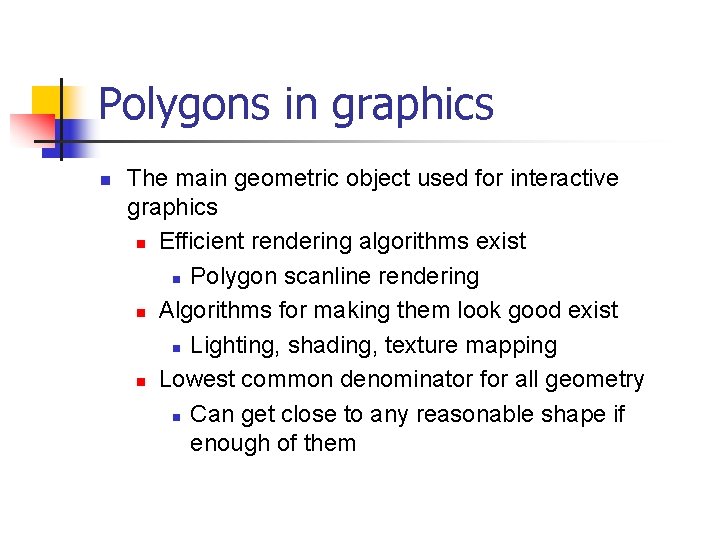
Polygons in graphics n The main geometric object used for interactive graphics n Efficient rendering algorithms exist n Polygon scanline rendering n Algorithms for making them look good exist n Lighting, shading, texture mapping n Lowest common denominator for all geometry n Can get close to any reasonable shape if enough of them
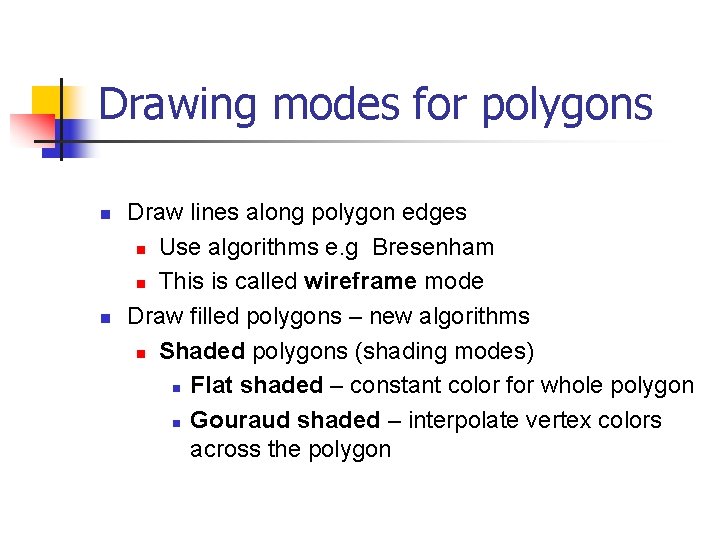
Drawing modes for polygons n n Draw lines along polygon edges n Use algorithms e. g Bresenham n This is called wireframe mode Draw filled polygons – new algorithms n Shaded polygons (shading modes) n Flat shaded – constant color for whole polygon n Gouraud shaded – interpolate vertex colors across the polygon
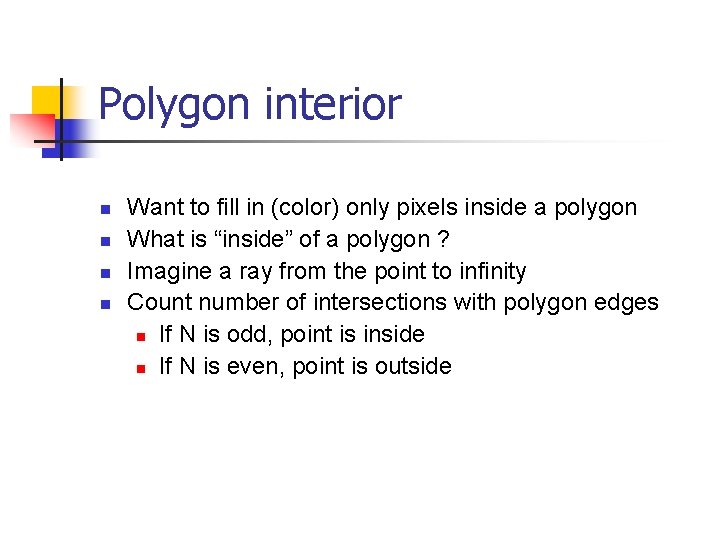
Polygon interior n n Want to fill in (color) only pixels inside a polygon What is “inside” of a polygon ? Imagine a ray from the point to infinity Count number of intersections with polygon edges n If N is odd, point is inside n If N is even, point is outside
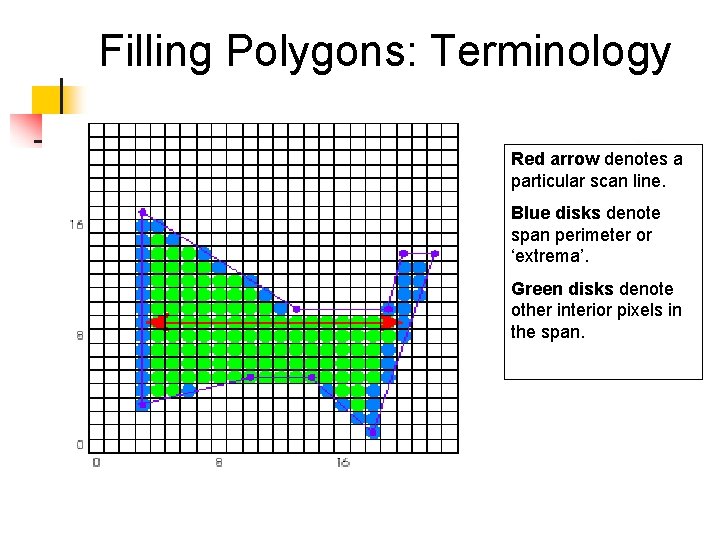
Filling Polygons: Terminology Red arrow denotes a particular scan line. Blue disks denote span perimeter or ‘extrema’. Green disks denote other interior pixels in the span.
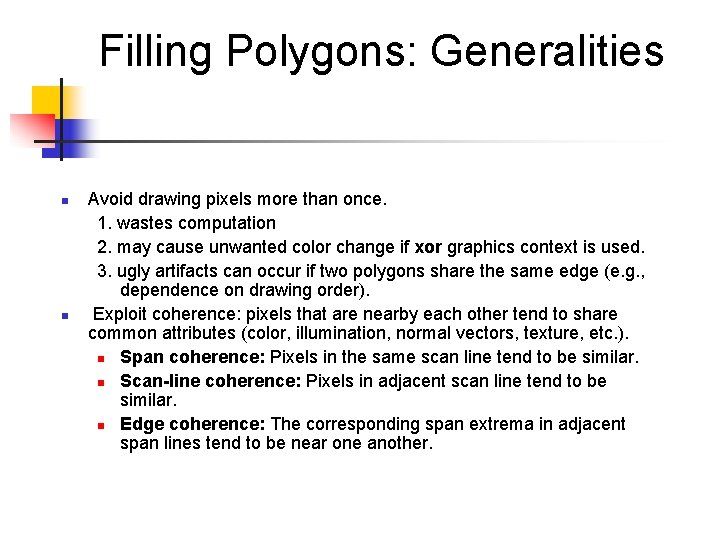
Filling Polygons: Generalities n n Avoid drawing pixels more than once. 1. wastes computation 2. may cause unwanted color change if xor graphics context is used. 3. ugly artifacts can occur if two polygons share the same edge (e. g. , dependence on drawing order). Exploit coherence: pixels that are nearby each other tend to share common attributes (color, illumination, normal vectors, texture, etc. ). n Span coherence: Pixels in the same scan line tend to be similar. n Scan-line coherence: Pixels in adjacent scan line tend to be similar. n Edge coherence: The corresponding span extrema in adjacent span lines tend to be near one another.
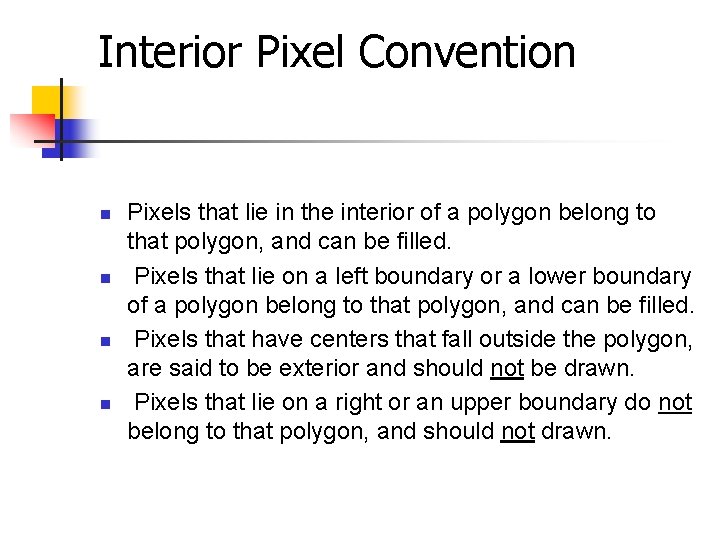
Interior Pixel Convention n n Pixels that lie in the interior of a polygon belong to that polygon, and can be filled. Pixels that lie on a left boundary or a lower boundary of a polygon belong to that polygon, and can be filled. Pixels that have centers that fall outside the polygon, are said to be exterior and should not be drawn. Pixels that lie on a right or an upper boundary do not belong to that polygon, and should not drawn.
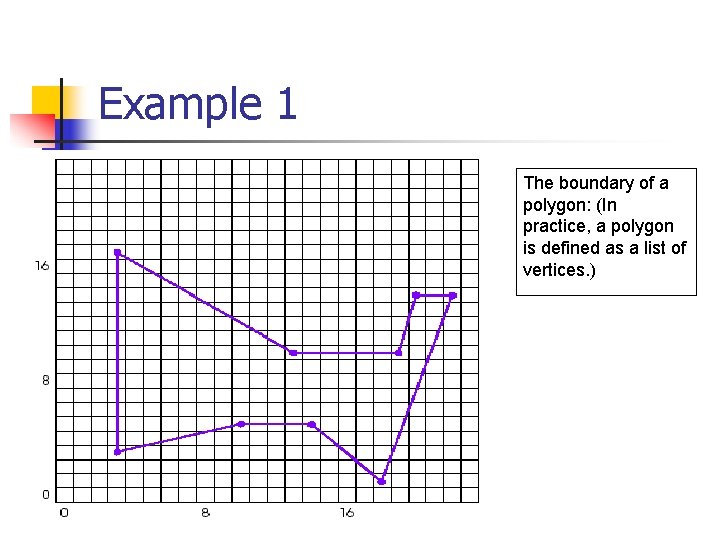
Example 1 The boundary of a polygon: (In practice, a polygon is defined as a list of vertices. )
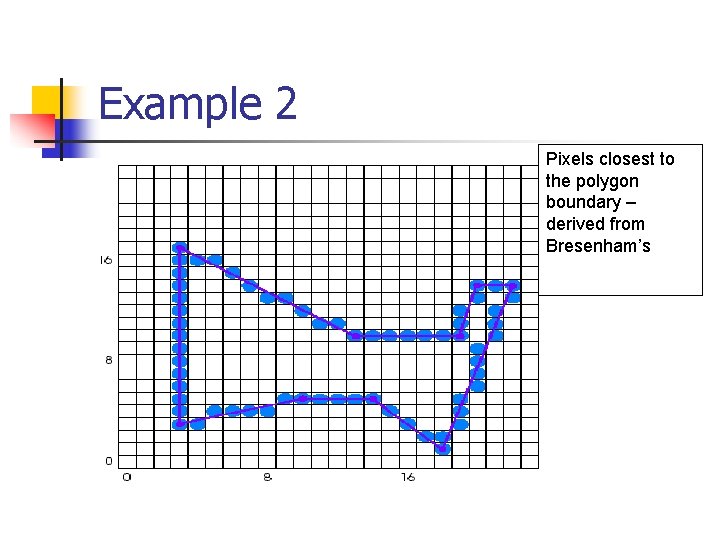
Example 2 Pixels closest to the polygon boundary – derived from Bresenham’s
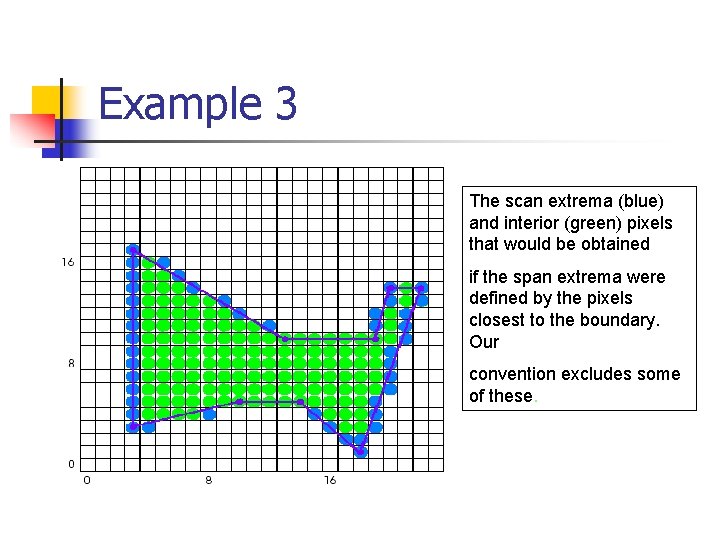
Example 3 The scan extrema (blue) and interior (green) pixels that would be obtained if the span extrema were defined by the pixels closest to the boundary. Our convention excludes some of these.
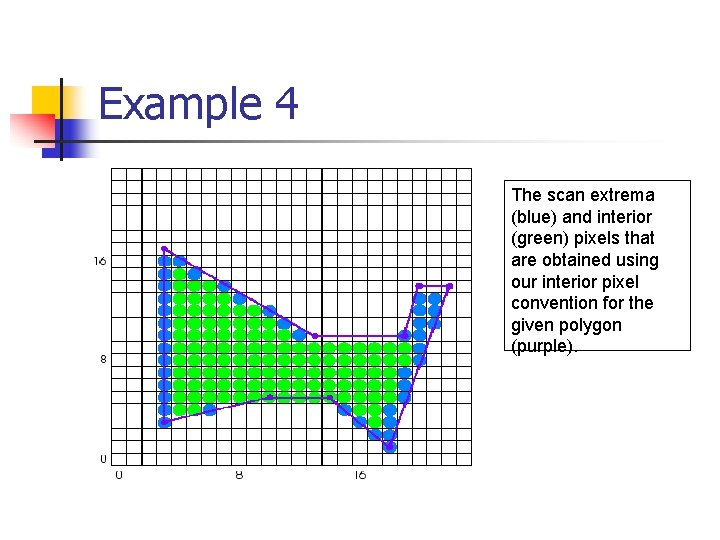
Example 4 The scan extrema (blue) and interior (green) pixels that are obtained using our interior pixel convention for the given polygon (purple).
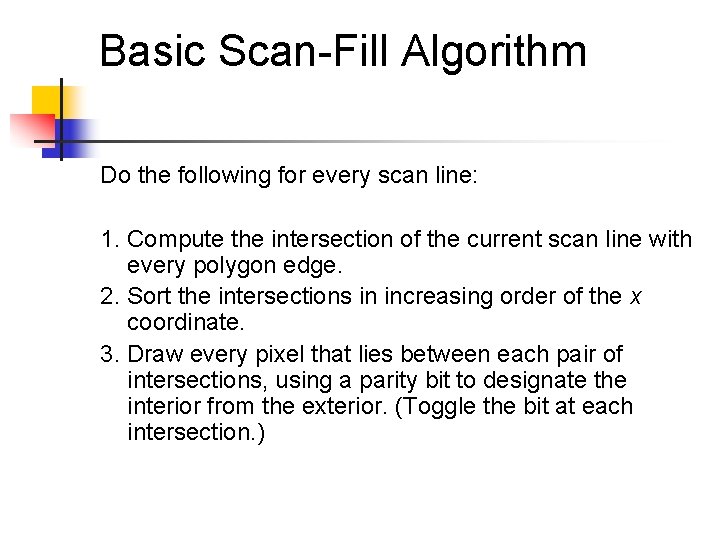
Basic Scan-Fill Algorithm Do the following for every scan line: 1. Compute the intersection of the current scan line with every polygon edge. 2. Sort the intersections in increasing order of the x coordinate. 3. Draw every pixel that lies between each pair of intersections, using a parity bit to designate the interior from the exterior. (Toggle the bit at each intersection. )
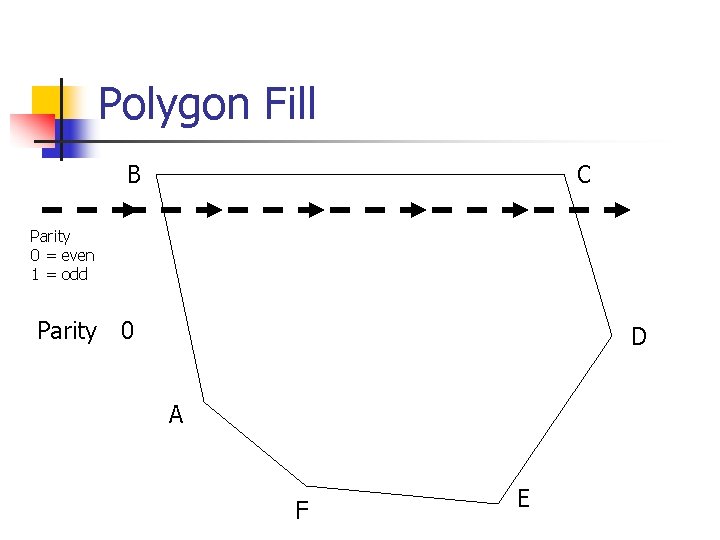
Polygon Fill B C Parity 0 = even 1 = odd Parity 1 0 D A F E
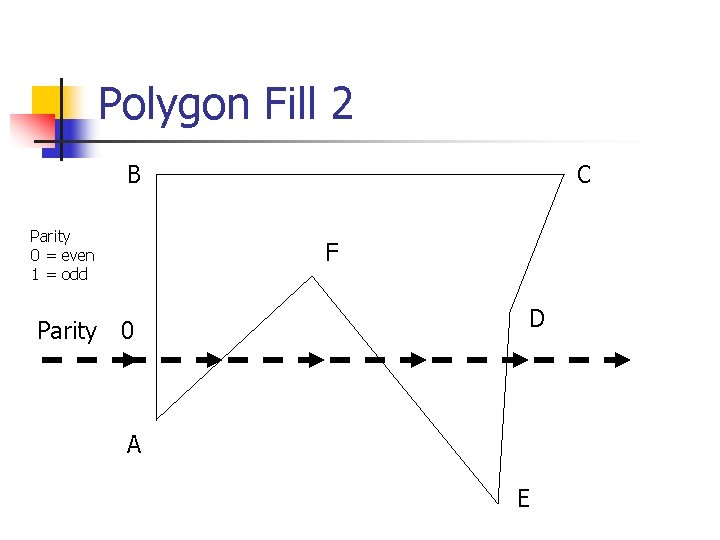
Polygon Fill 2 B Parity 0 = even 1 = odd Parity C F 1 0 D A E
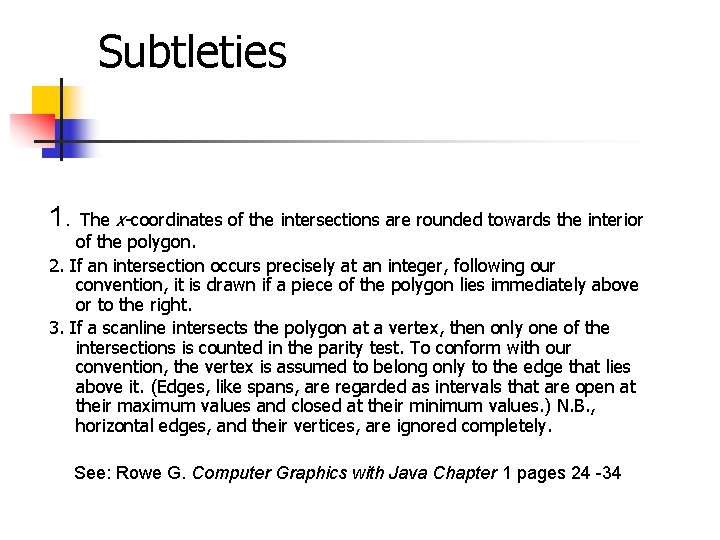
Subtleties 1. The x-coordinates of the intersections are rounded towards the interior of the polygon. 2. If an intersection occurs precisely at an integer, following our convention, it is drawn if a piece of the polygon lies immediately above or to the right. 3. If a scanline intersects the polygon at a vertex, then only one of the intersections is counted in the parity test. To conform with our convention, the vertex is assumed to belong only to the edge that lies above it. (Edges, like spans, are regarded as intervals that are open at their maximum values and closed at their minimum values. ) N. B. , horizontal edges, and their vertices, are ignored completely. See: Rowe G. Computer Graphics with Java Chapter 1 pages 24 -34