Graphical User Interfaces in Haskell Koen Lindstrm Claessen
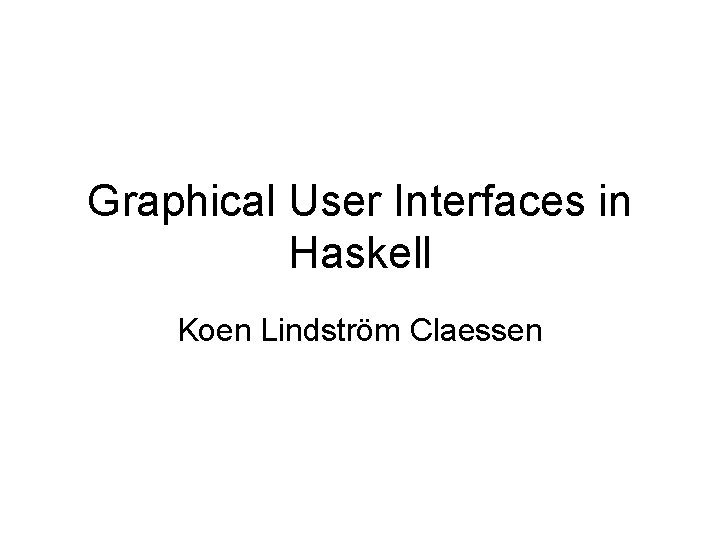
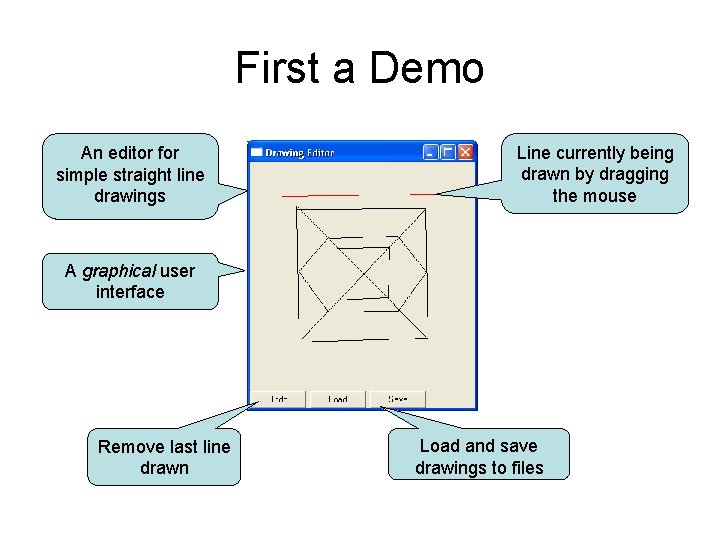
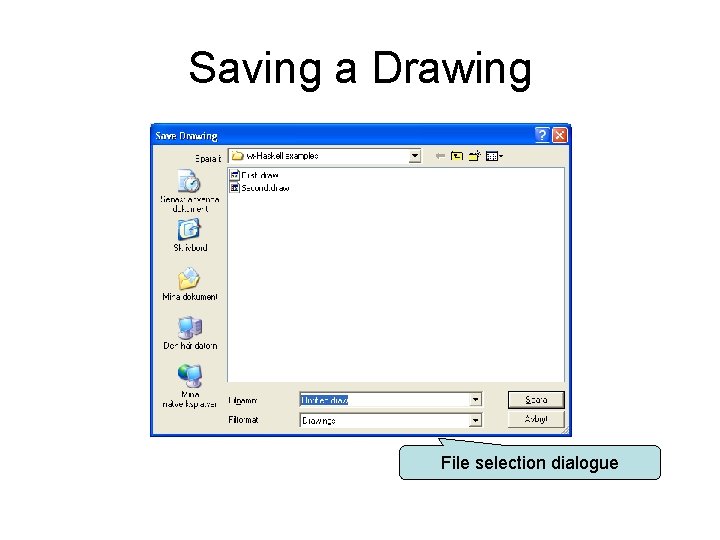
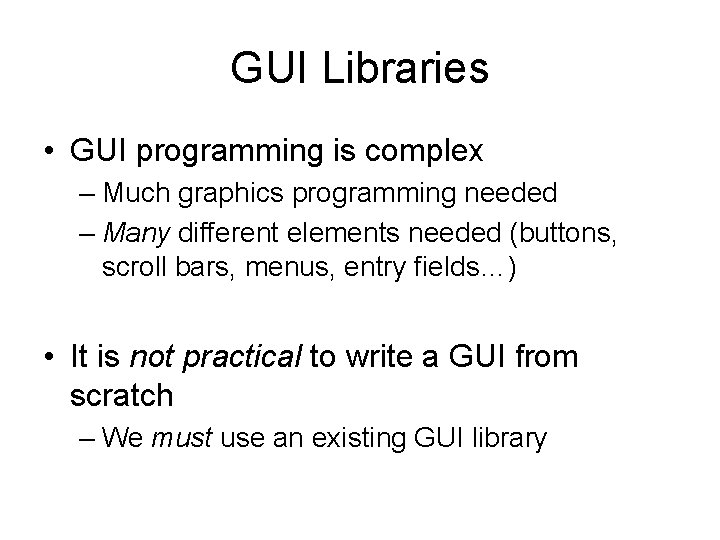
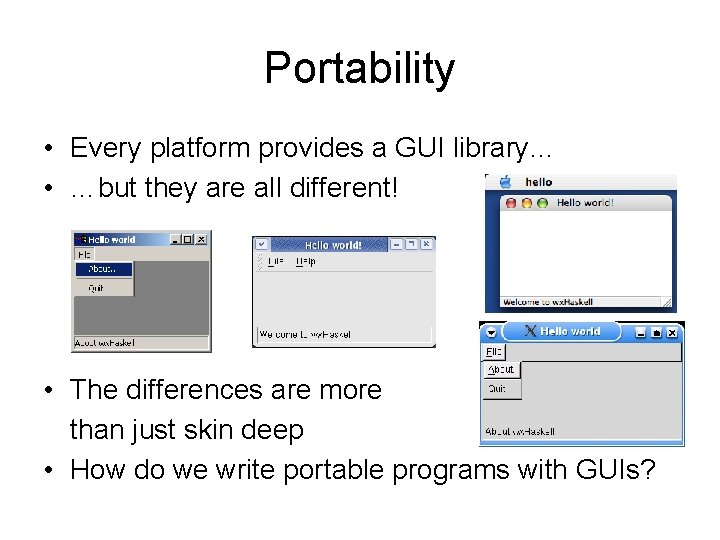
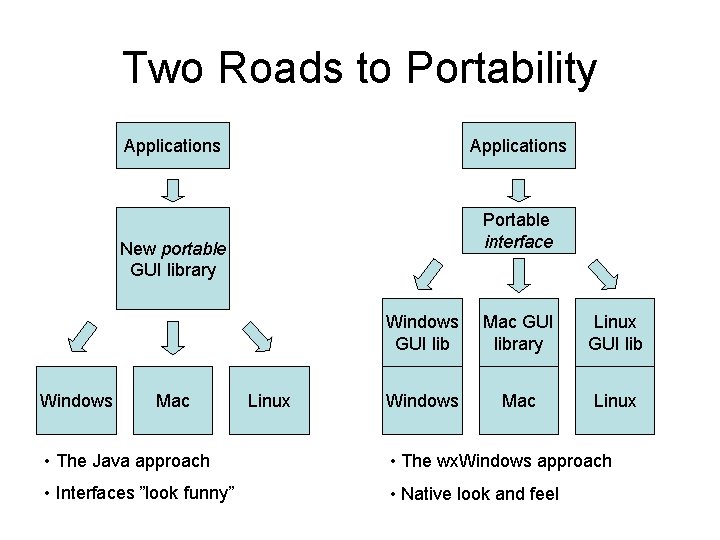
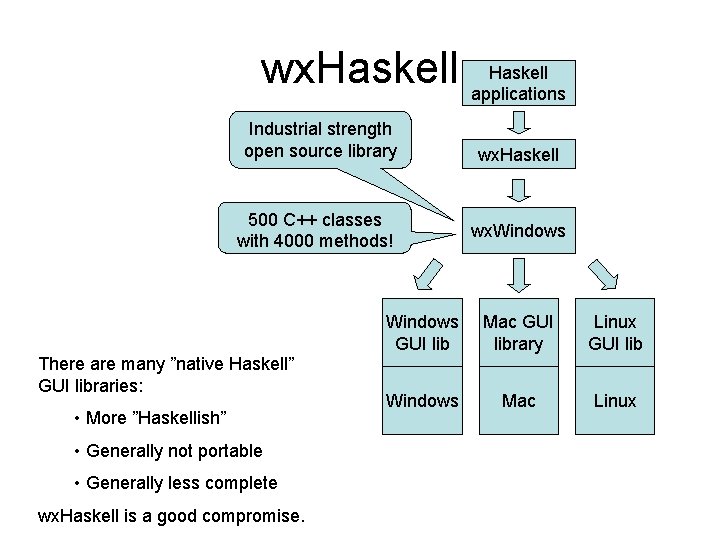
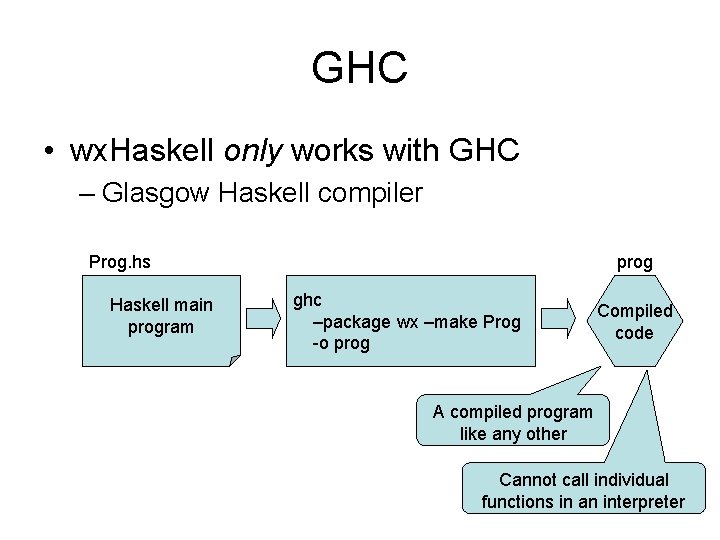
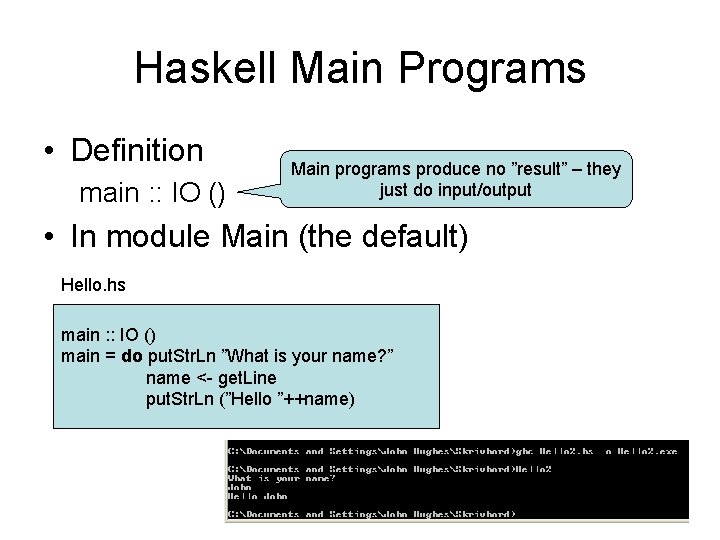
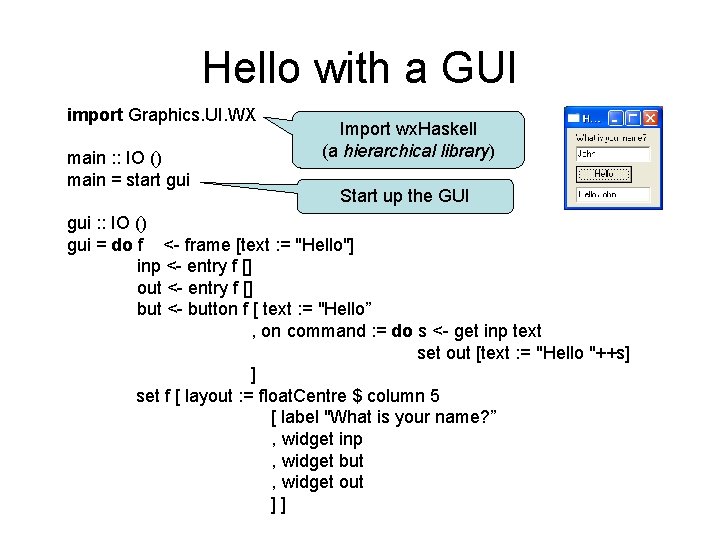
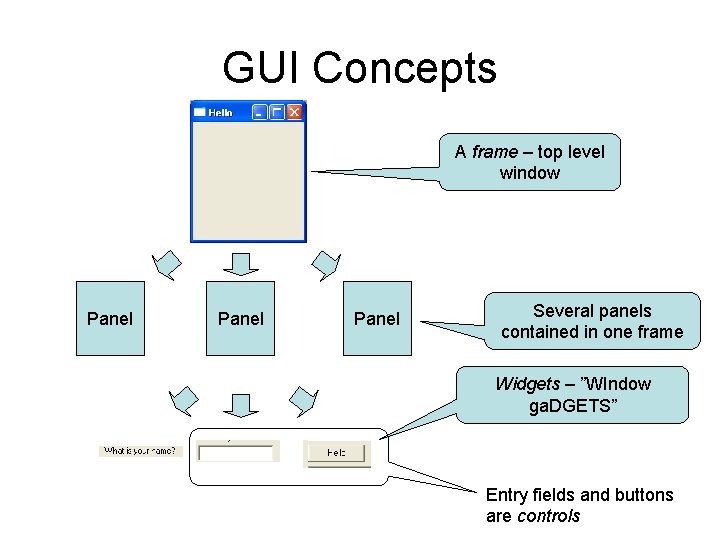
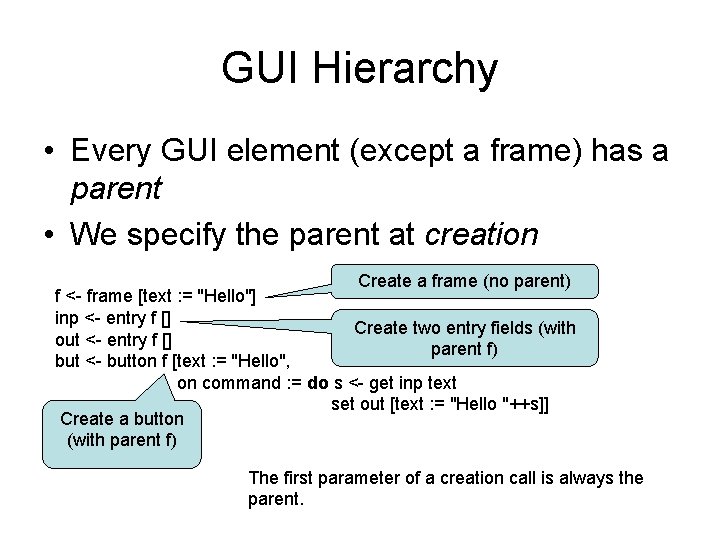
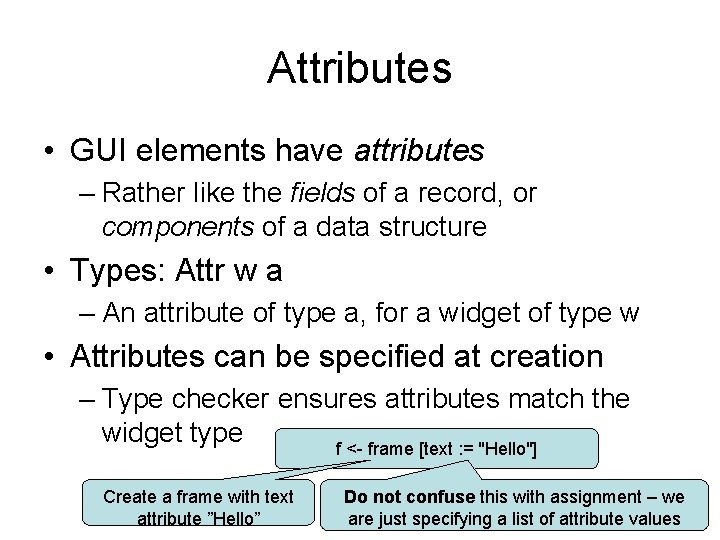
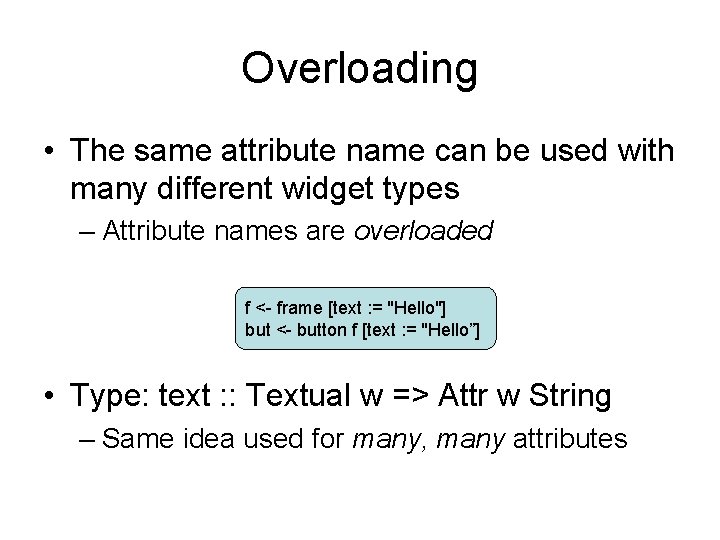
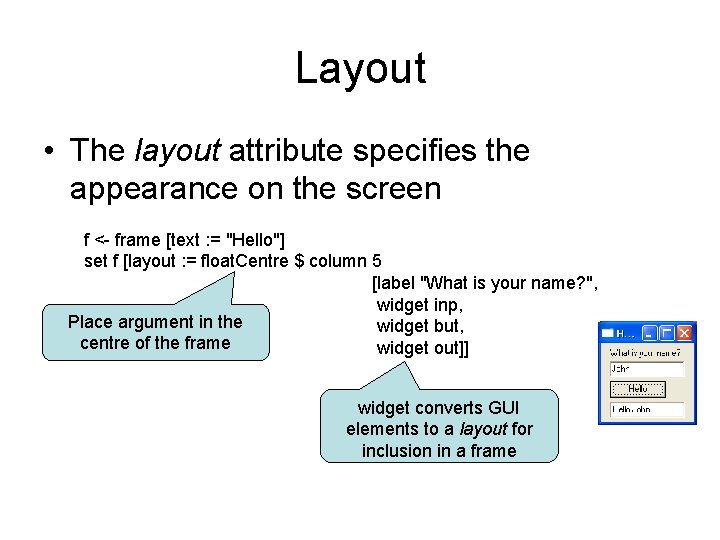
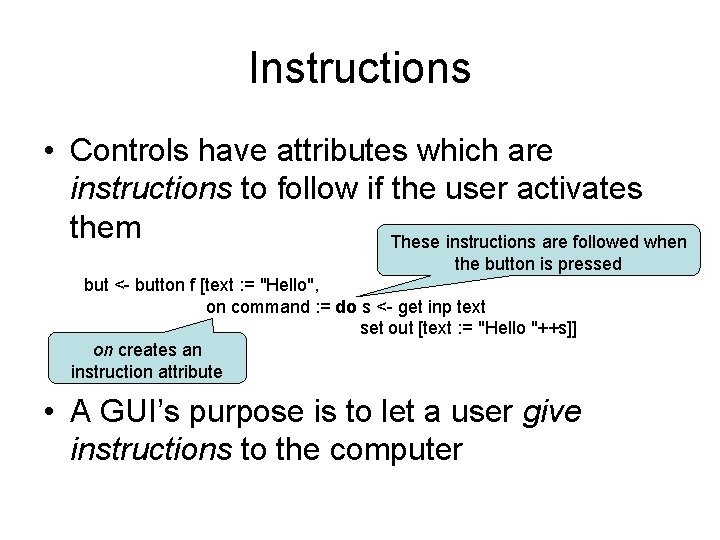
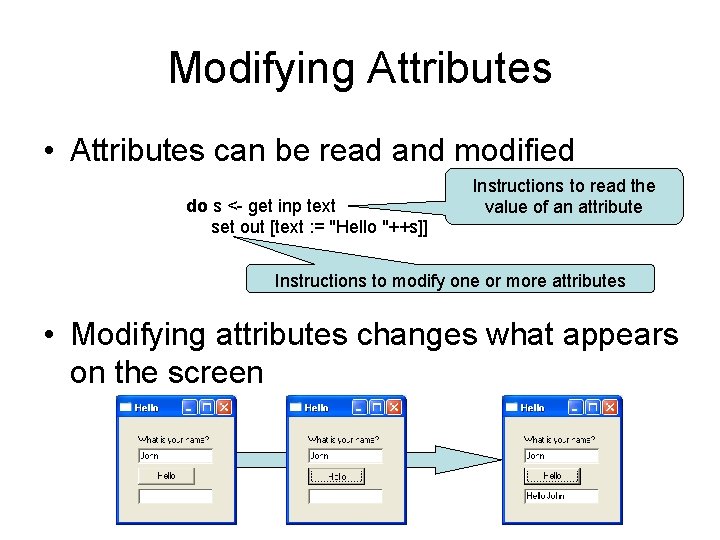
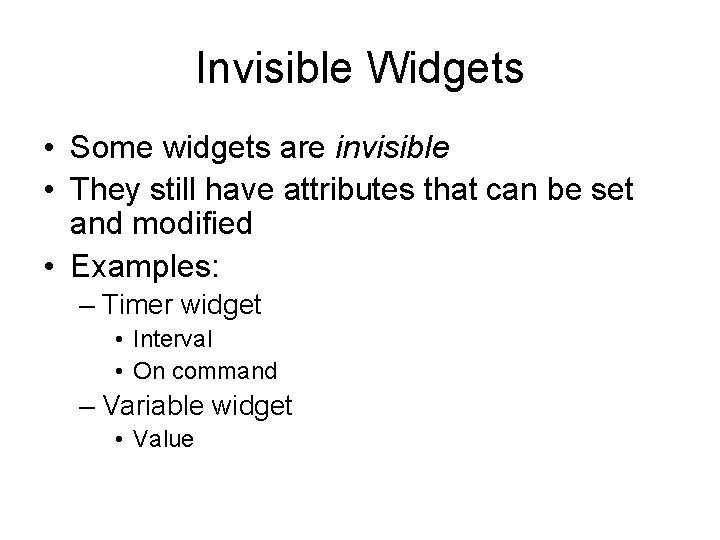
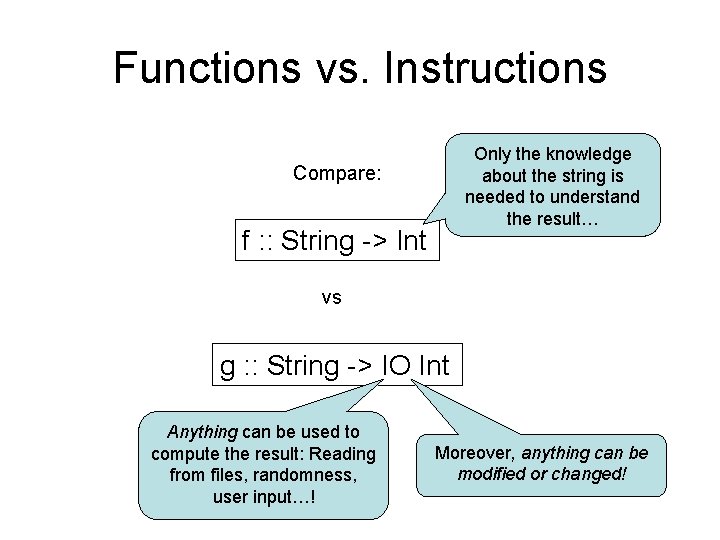
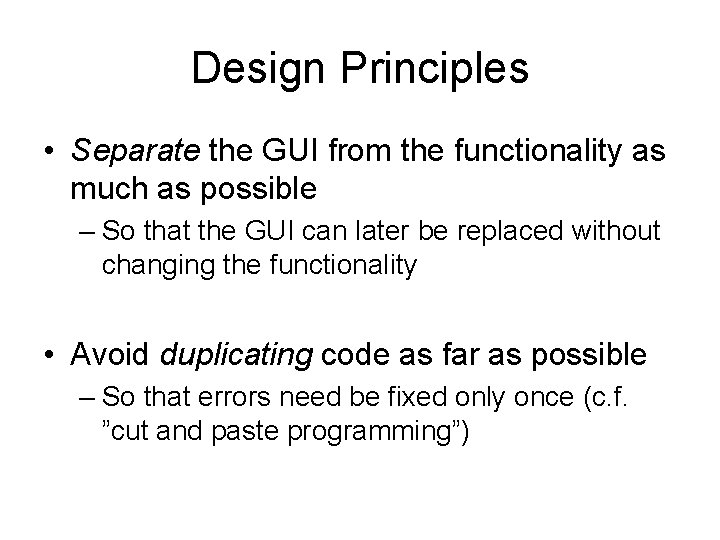
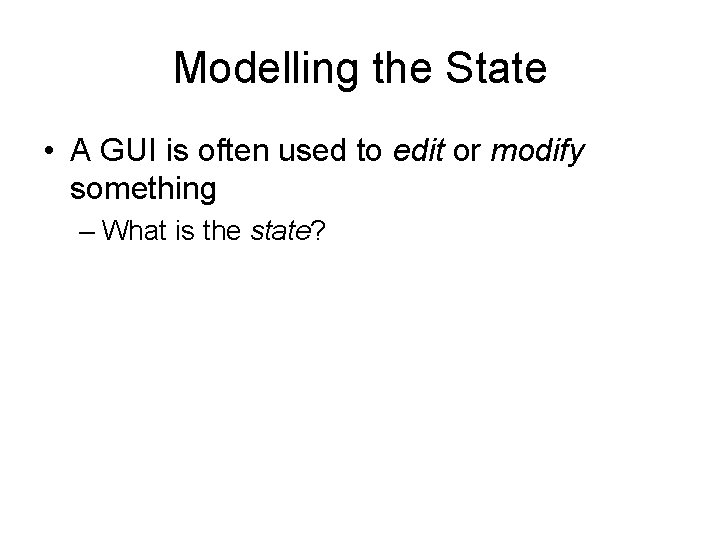
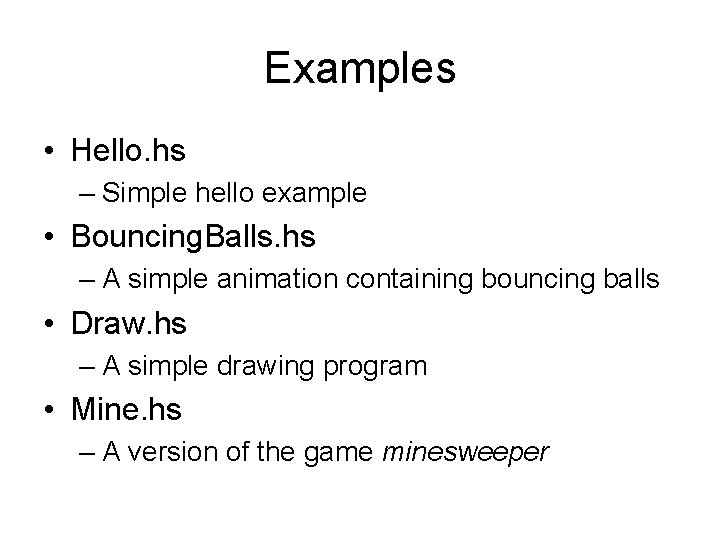
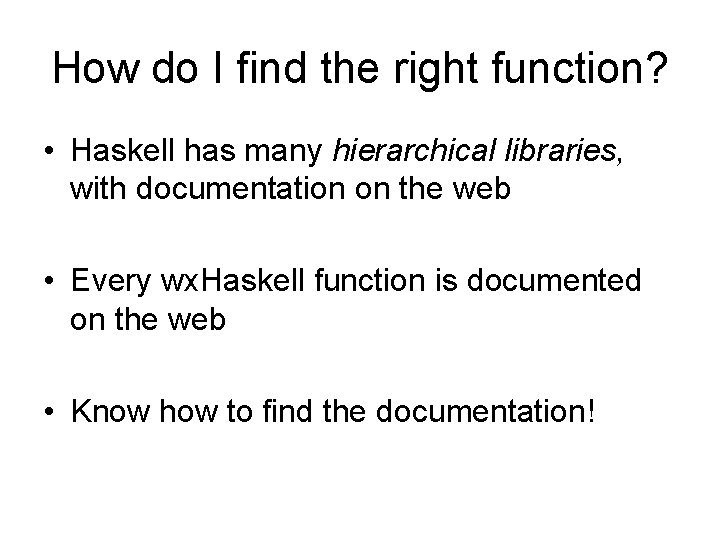
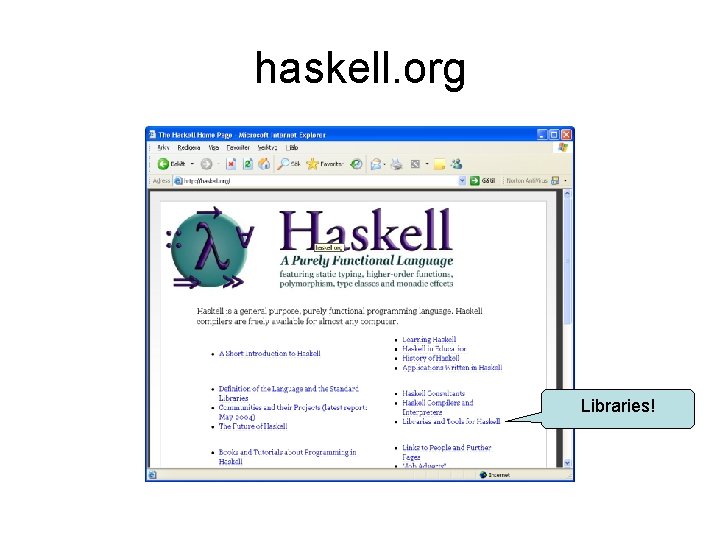
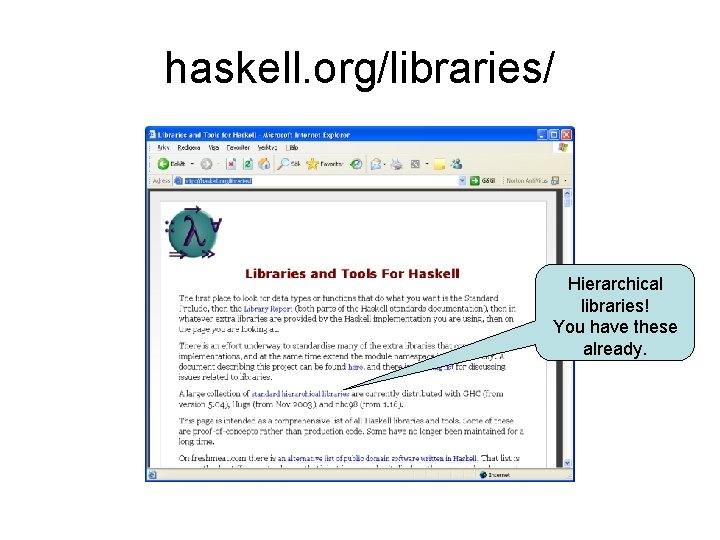
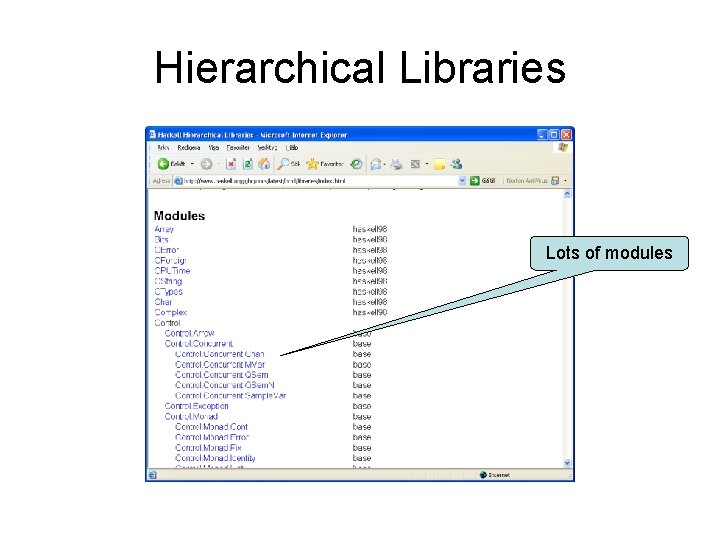
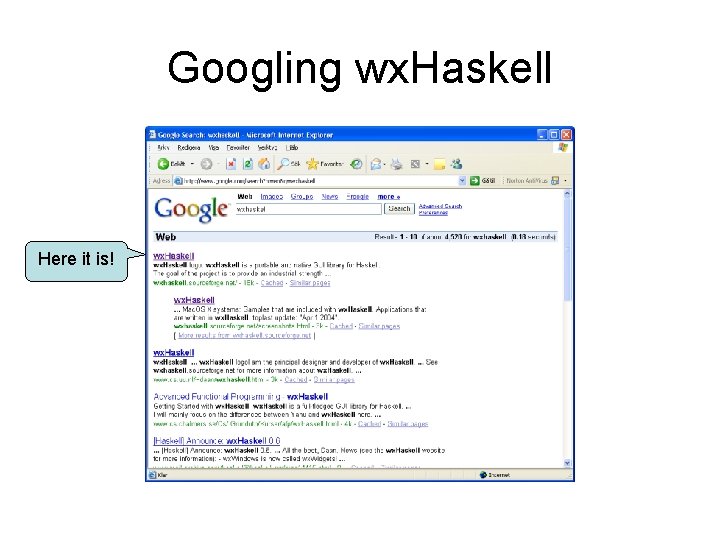
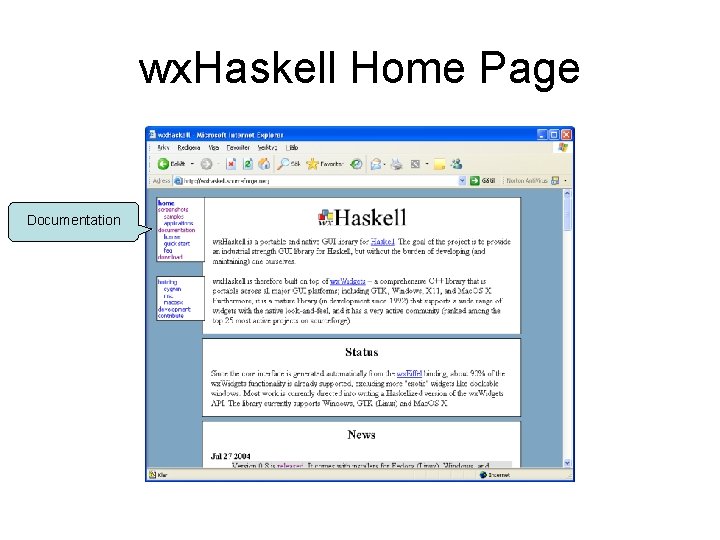
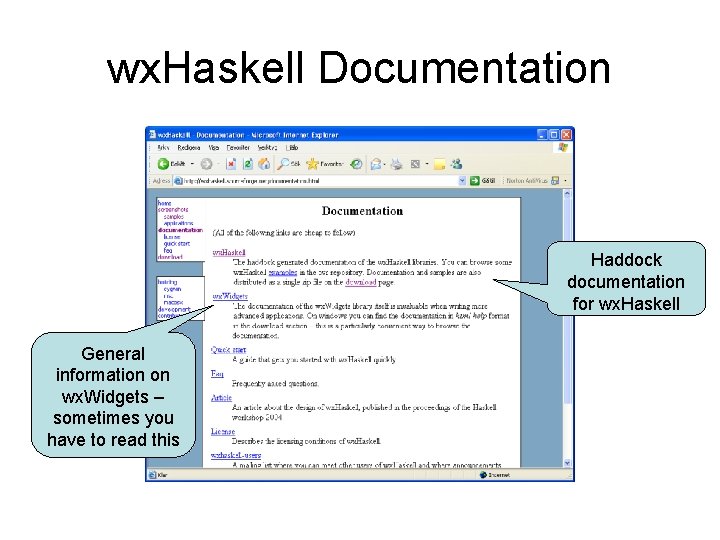
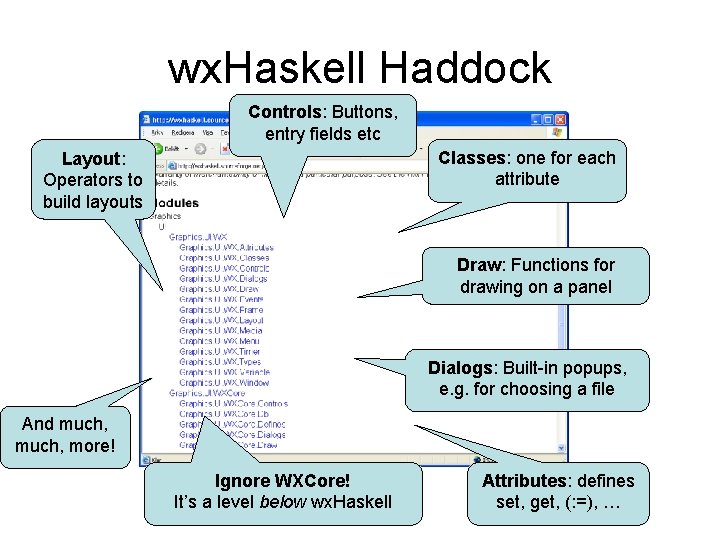
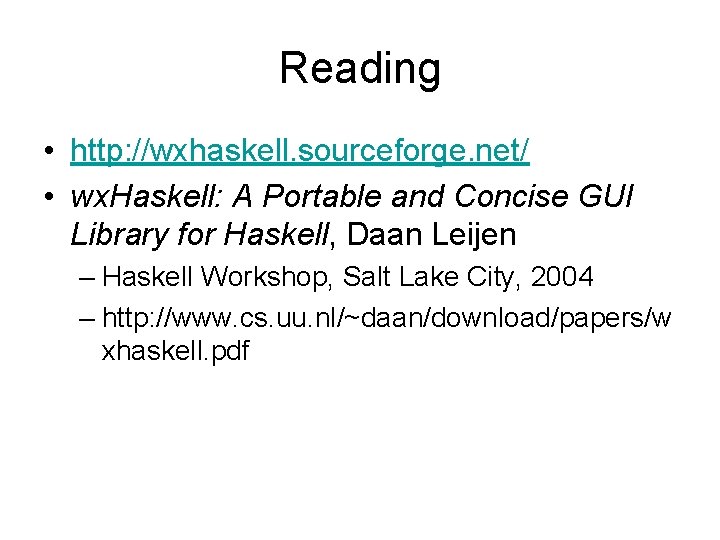
- Slides: 31
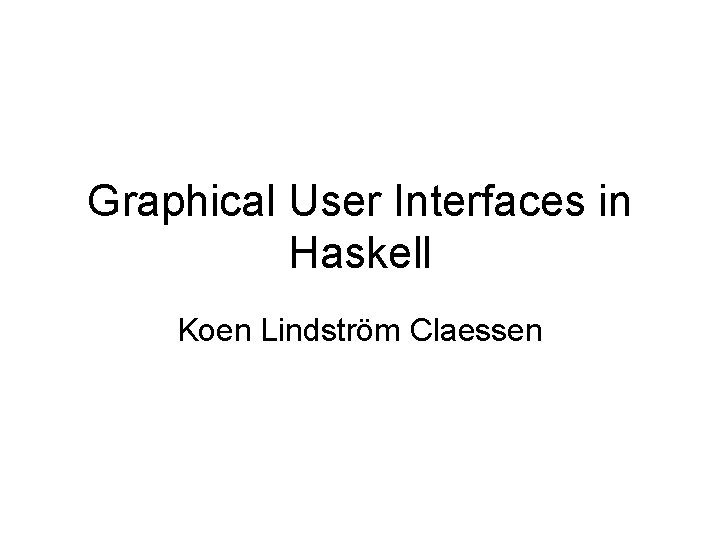
Graphical User Interfaces in Haskell Koen Lindström Claessen
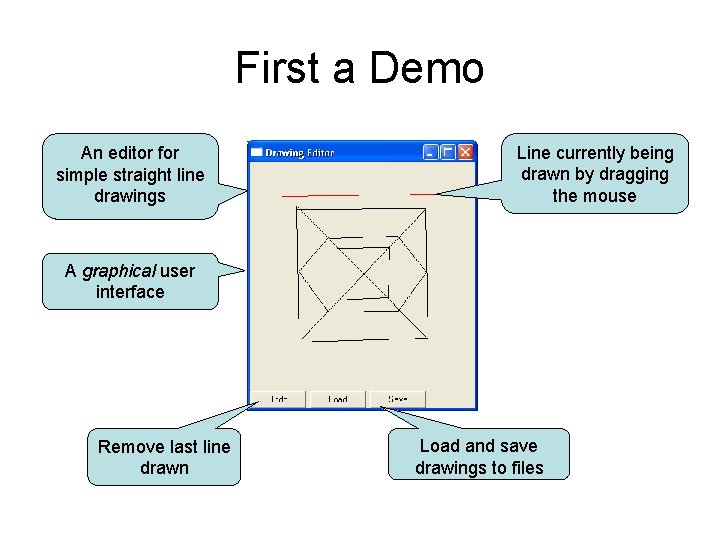
First a Demo An editor for simple straight line drawings Line currently being drawn by dragging the mouse A graphical user interface Remove last line drawn Load and save drawings to files
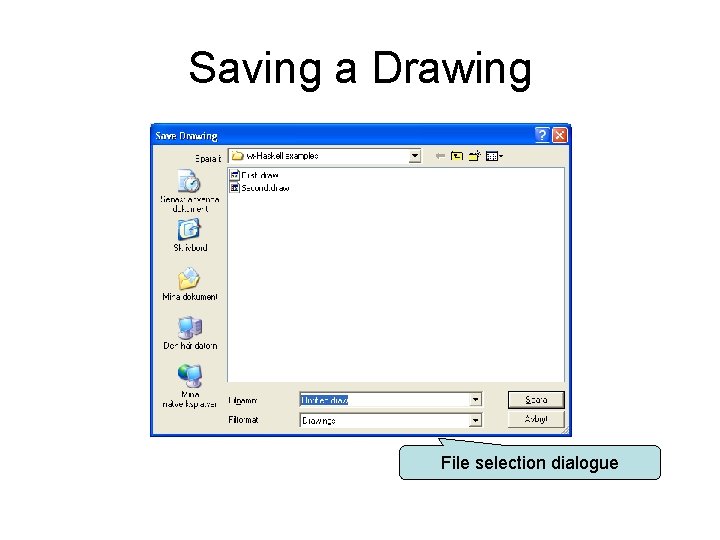
Saving a Drawing File selection dialogue
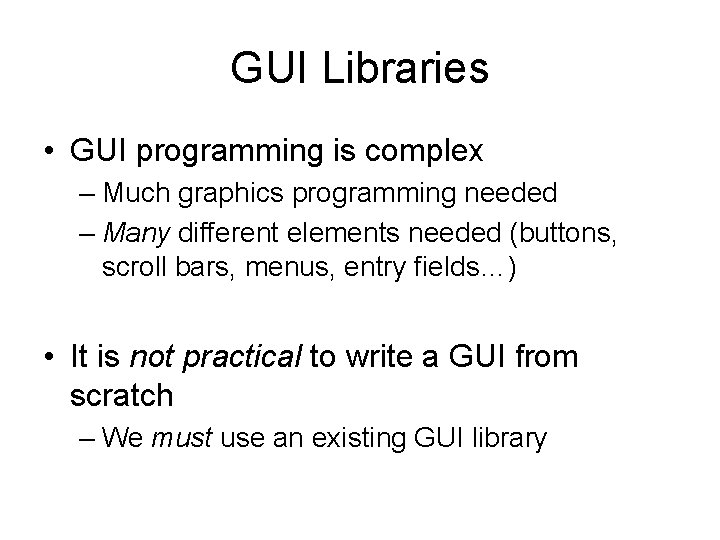
GUI Libraries • GUI programming is complex – Much graphics programming needed – Many different elements needed (buttons, scroll bars, menus, entry fields…) • It is not practical to write a GUI from scratch – We must use an existing GUI library
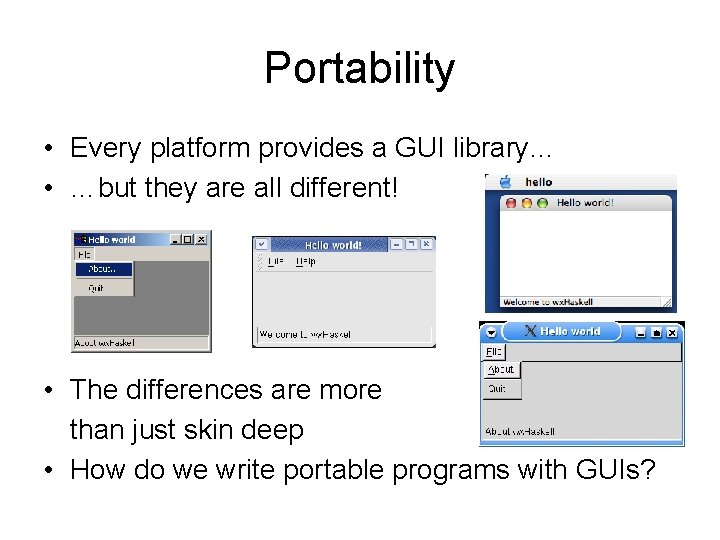
Portability • Every platform provides a GUI library… • …but they are all different! • The differences are more than just skin deep • How do we write portable programs with GUIs?
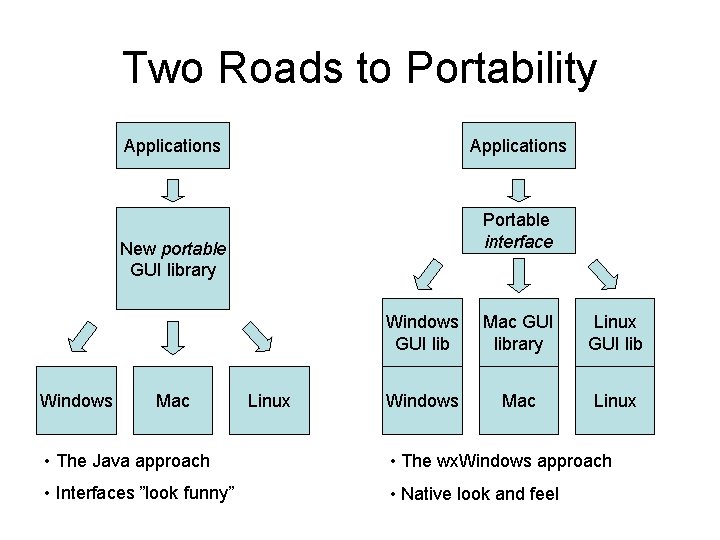
Two Roads to Portability Applications Portable interface New portable GUI library Windows Mac Linux Windows GUI lib Mac GUI library Linux GUI lib Windows Mac Linux • The Java approach • The wx. Windows approach • Interfaces ”look funny” • Native look and feel
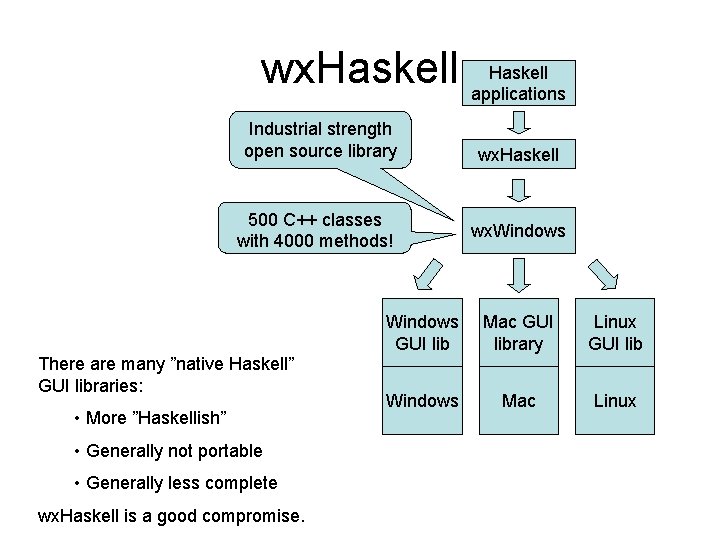
Haskell wx. Haskell applications Industrial strength open source library 500 C++ classes with 4000 methods! There are many ”native Haskell” GUI libraries: • More ”Haskellish” • Generally not portable • Generally less complete wx. Haskell is a good compromise. wx. Haskell wx. Windows GUI lib Mac GUI library Linux GUI lib Windows Mac Linux
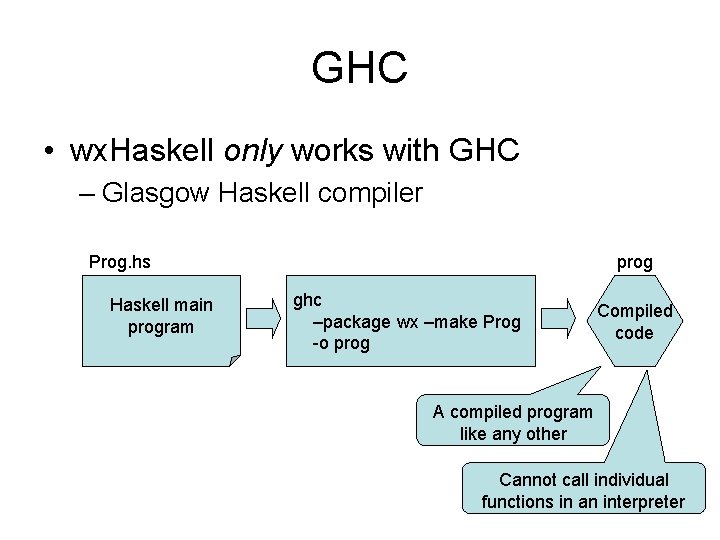
GHC • wx. Haskell only works with GHC – Glasgow Haskell compiler Prog. hs Haskell main program prog ghc –package wx –make Prog -o prog Compiled code A compiled program like any other Cannot call individual functions in an interpreter
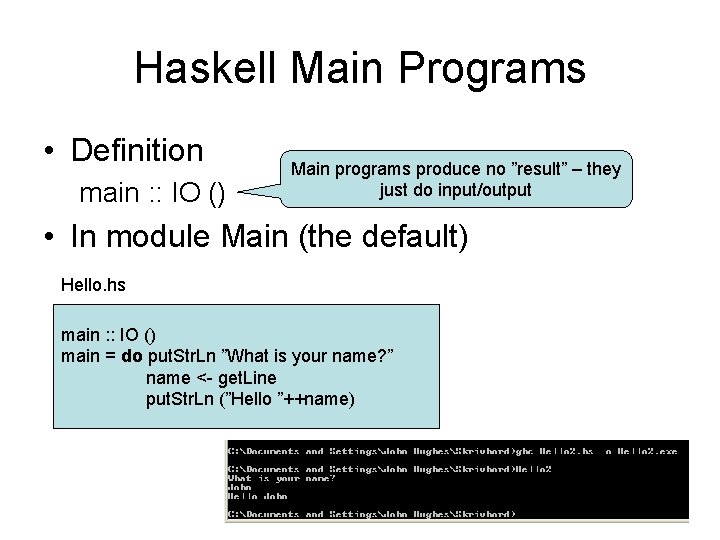
Haskell Main Programs • Definition main : : IO () Main programs produce no ”result” – they just do input/output • In module Main (the default) Hello. hs main : : IO () main = do put. Str. Ln ”What is your name? ” name <- get. Line put. Str. Ln (”Hello ”++name)
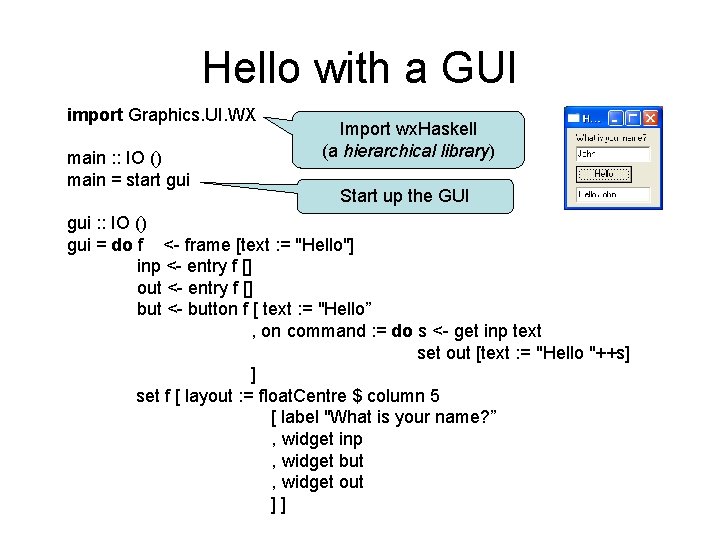
Hello with a GUI import Graphics. UI. WX main : : IO () main = start gui Import wx. Haskell (a hierarchical library) Start up the GUI gui : : IO () gui = do f <- frame [text : = "Hello"] inp <- entry f [] out <- entry f [] but <- button f [ text : = "Hello” , on command : = do s <- get inp text set out [text : = "Hello "++s] ] set f [ layout : = float. Centre $ column 5 [ label "What is your name? ” , widget inp , widget but , widget out ]]
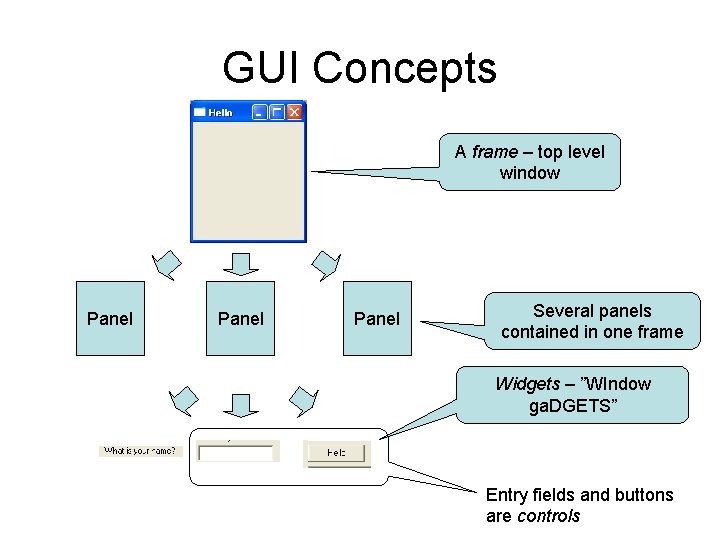
GUI Concepts A frame – top level window Panel Several panels contained in one frame Widgets – ”WIndow ga. DGETS” Entry fields and buttons are controls
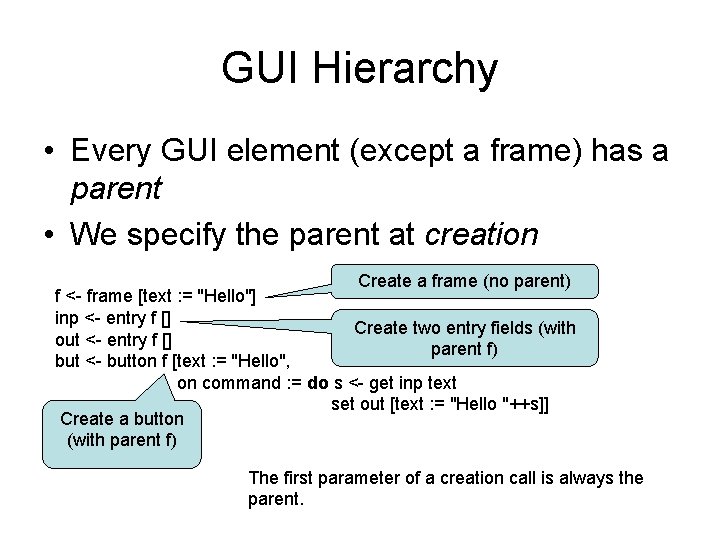
GUI Hierarchy • Every GUI element (except a frame) has a parent • We specify the parent at creation Create a frame (no parent) f <- frame [text : = "Hello"] inp <- entry f [] Create two entry fields (with out <- entry f [] parent f) but <- button f [text : = "Hello", on command : = do s <- get inp text set out [text : = "Hello "++s]] Create a button (with parent f) The first parameter of a creation call is always the parent.
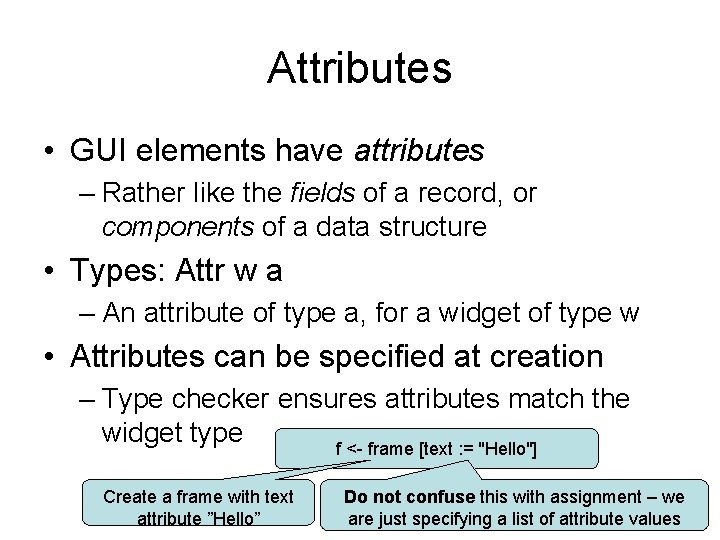
Attributes • GUI elements have attributes – Rather like the fields of a record, or components of a data structure • Types: Attr w a – An attribute of type a, for a widget of type w • Attributes can be specified at creation – Type checker ensures attributes match the widget type f <- frame [text : = "Hello"] Create a frame with text attribute ”Hello” Do not confuse this with assignment – we are just specifying a list of attribute values
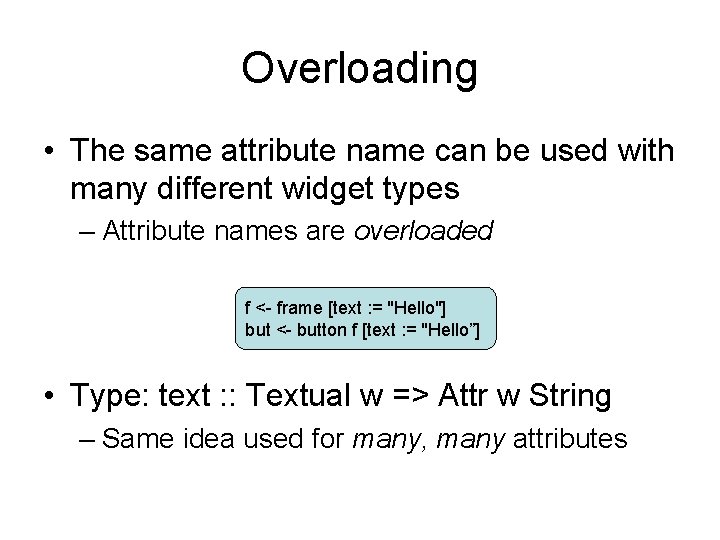
Overloading • The same attribute name can be used with many different widget types – Attribute names are overloaded f <- frame [text : = "Hello"] but <- button f [text : = "Hello”] • Type: text : : Textual w => Attr w String – Same idea used for many, many attributes
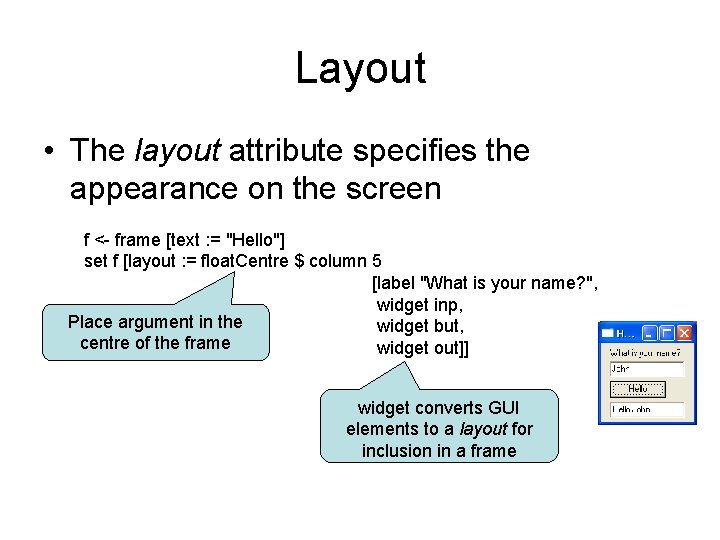
Layout • The layout attribute specifies the appearance on the screen f <- frame [text : = "Hello"] set f [layout : = float. Centre $ column 5 [label "What is your name? ", widget inp, Place argument in the widget but, centre of the frame widget out]] widget converts GUI elements to a layout for inclusion in a frame
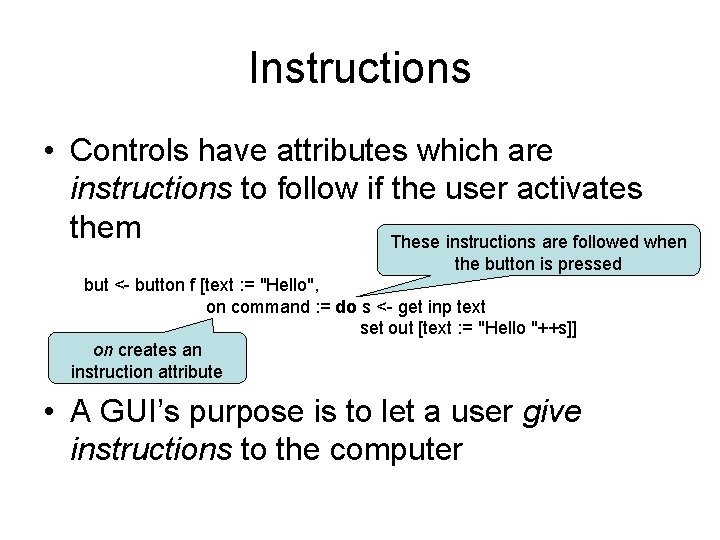
Instructions • Controls have attributes which are instructions to follow if the user activates them These instructions are followed when the button is pressed but <- button f [text : = "Hello", on command : = do s <- get inp text set out [text : = "Hello "++s]] on creates an instruction attribute • A GUI’s purpose is to let a user give instructions to the computer
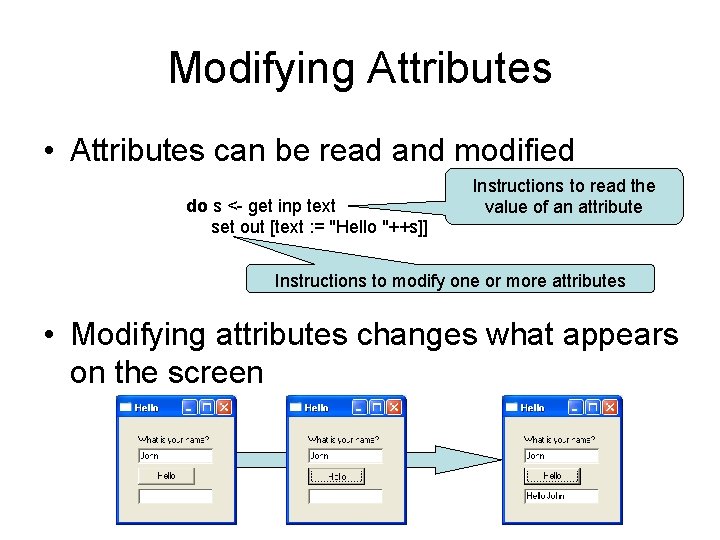
Modifying Attributes • Attributes can be read and modified do s <- get inp text set out [text : = "Hello "++s]] Instructions to read the value of an attribute Instructions to modify one or more attributes • Modifying attributes changes what appears on the screen
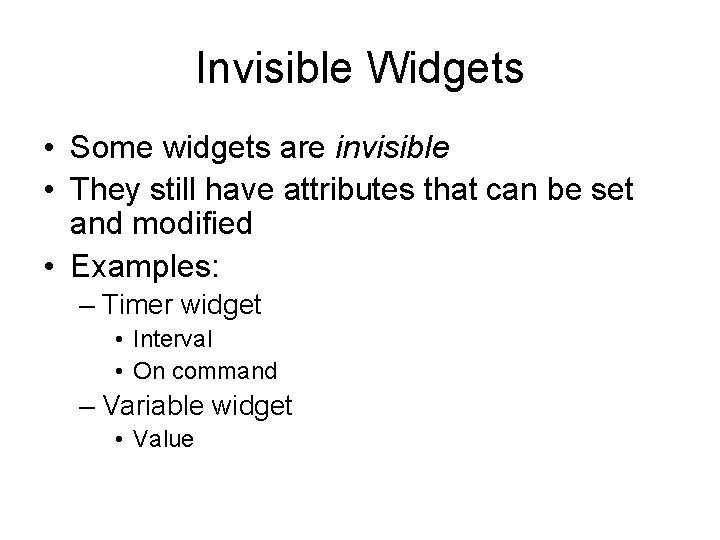
Invisible Widgets • Some widgets are invisible • They still have attributes that can be set and modified • Examples: – Timer widget • Interval • On command – Variable widget • Value
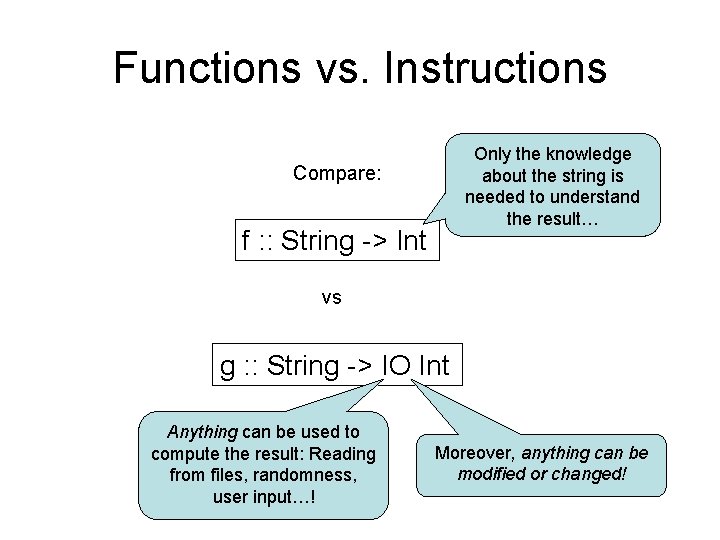
Functions vs. Instructions Only the knowledge about the string is needed to understand the result… Compare: f : : String -> Int vs g : : String -> IO Int Anything can be used to compute the result: Reading from files, randomness, user input…! Moreover, anything can be modified or changed!
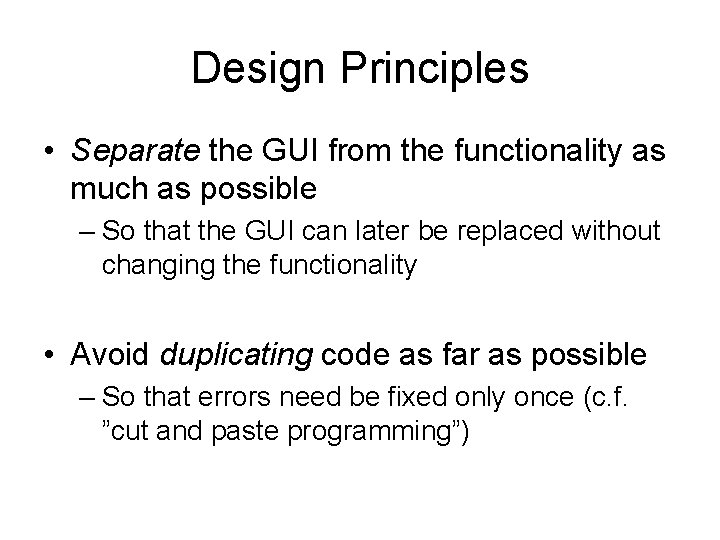
Design Principles • Separate the GUI from the functionality as much as possible – So that the GUI can later be replaced without changing the functionality • Avoid duplicating code as far as possible – So that errors need be fixed only once (c. f. ”cut and paste programming”)
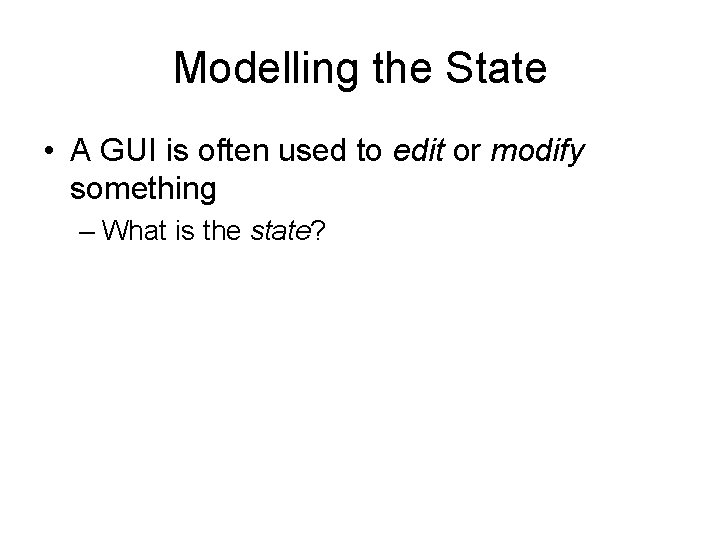
Modelling the State • A GUI is often used to edit or modify something – What is the state?
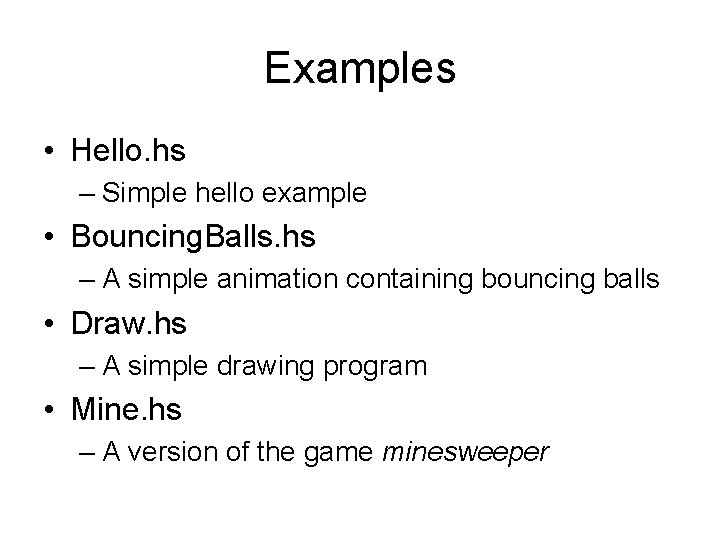
Examples • Hello. hs – Simple hello example • Bouncing. Balls. hs – A simple animation containing bouncing balls • Draw. hs – A simple drawing program • Mine. hs – A version of the game minesweeper
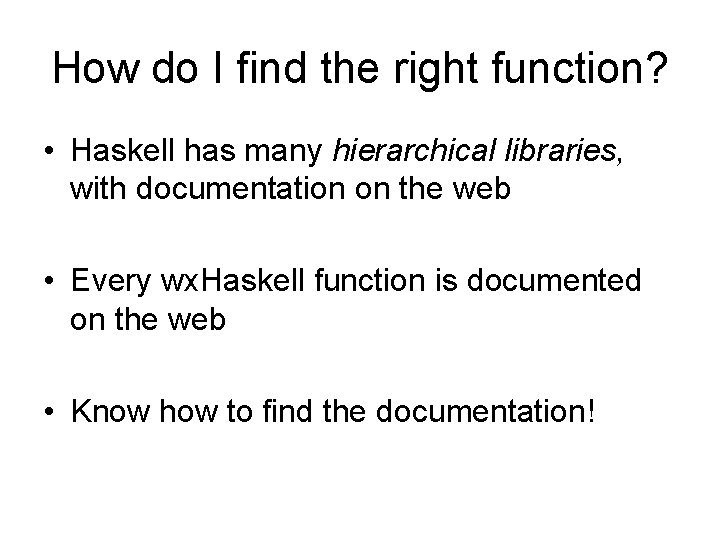
How do I find the right function? • Haskell has many hierarchical libraries, with documentation on the web • Every wx. Haskell function is documented on the web • Know how to find the documentation!
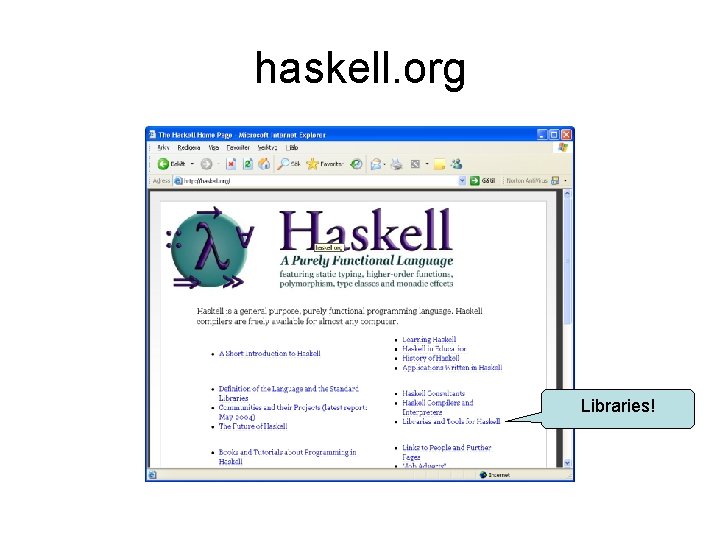
haskell. org Libraries!
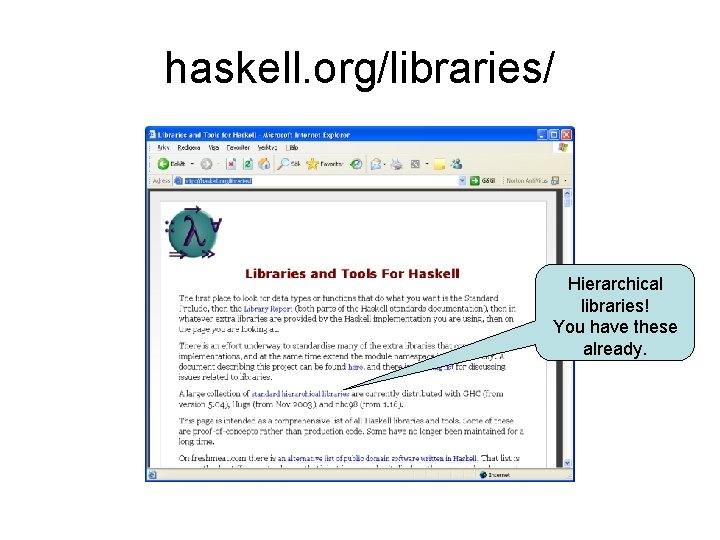
haskell. org/libraries/ Hierarchical libraries! You have these already.
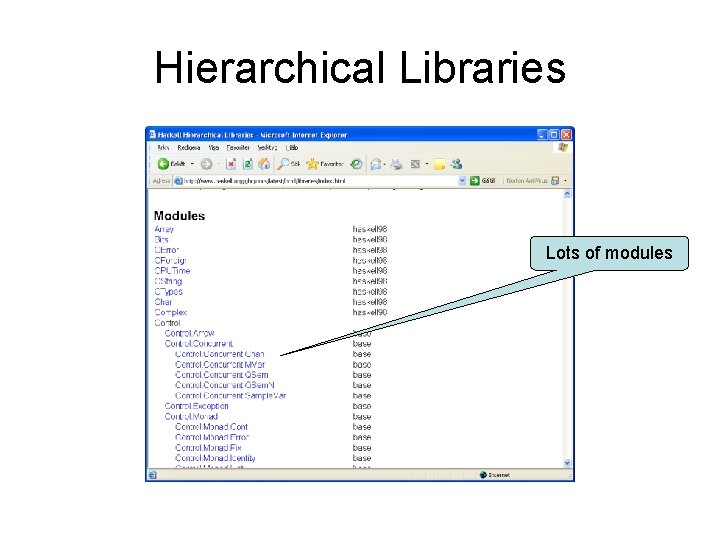
Hierarchical Libraries Lots of modules
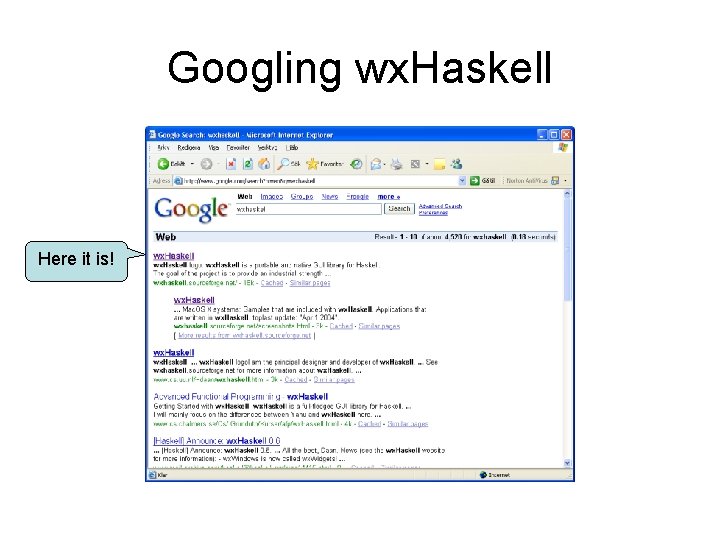
Googling wx. Haskell Here it is!
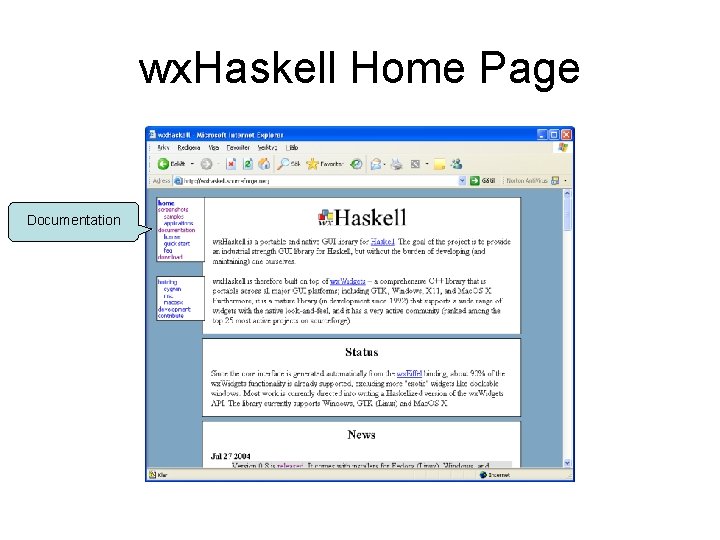
wx. Haskell Home Page Documentation
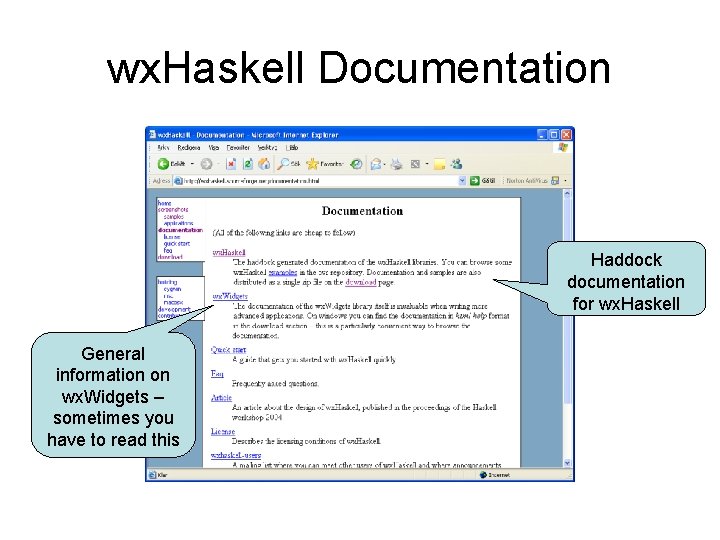
wx. Haskell Documentation Haddock documentation for wx. Haskell General information on wx. Widgets – sometimes you have to read this
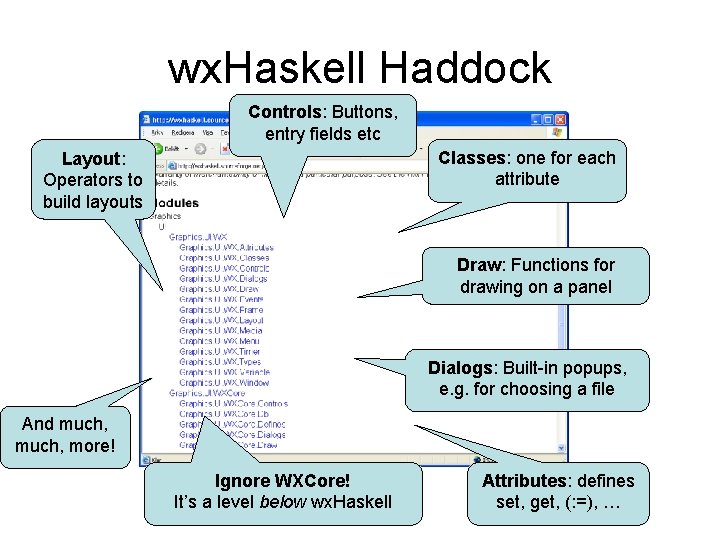
wx. Haskell Haddock Controls: Buttons, entry fields etc Classes: one for each attribute Layout: Operators to build layouts Draw: Functions for drawing on a panel Dialogs: Built-in popups, e. g. for choosing a file And much, more! Ignore WXCore! It’s a level below wx. Haskell Attributes: defines set, get, (: =), …
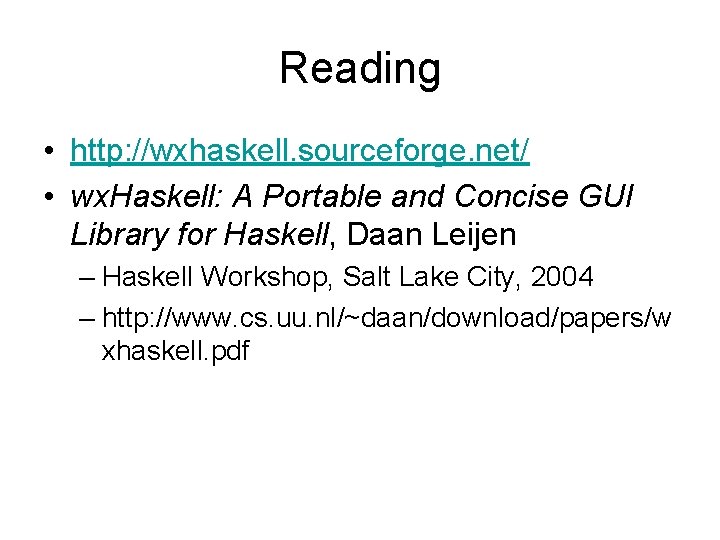
Reading • http: //wxhaskell. sourceforge. net/ • wx. Haskell: A Portable and Concise GUI Library for Haskell, Daan Leijen – Haskell Workshop, Salt Lake City, 2004 – http: //www. cs. uu. nl/~daan/download/papers/w xhaskell. pdf