Graphical User Interface Chapter Goals To understand the
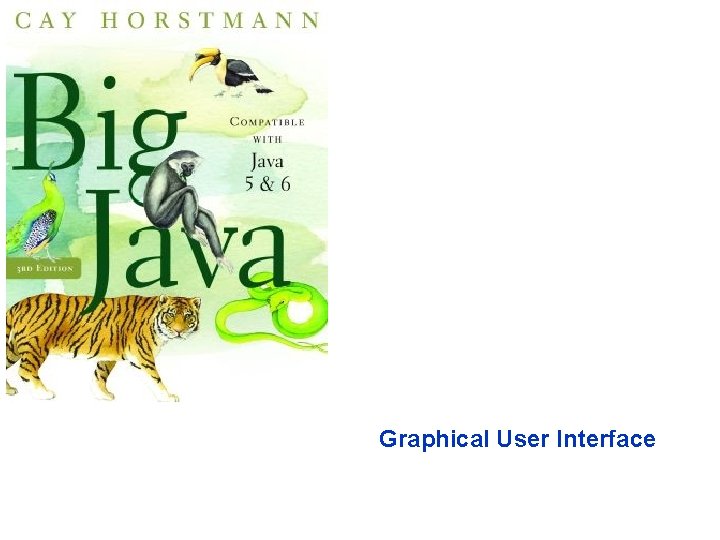
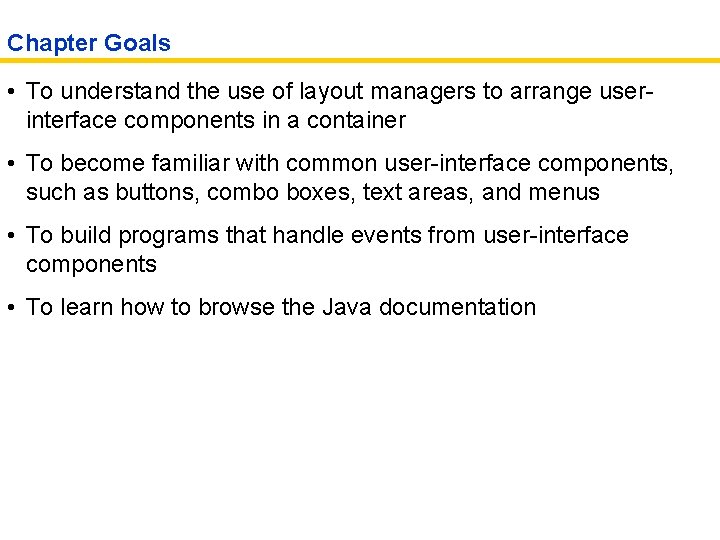
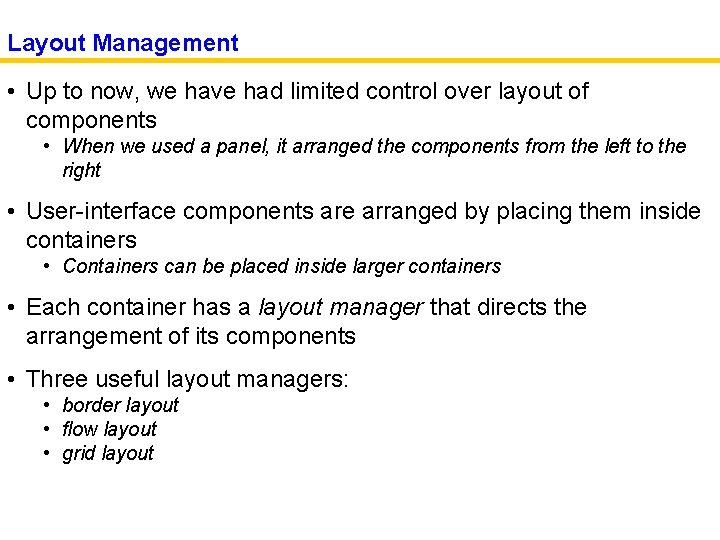
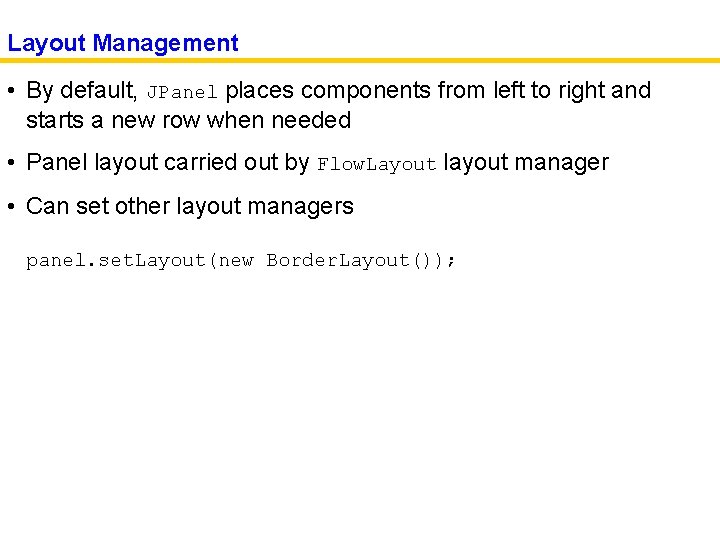
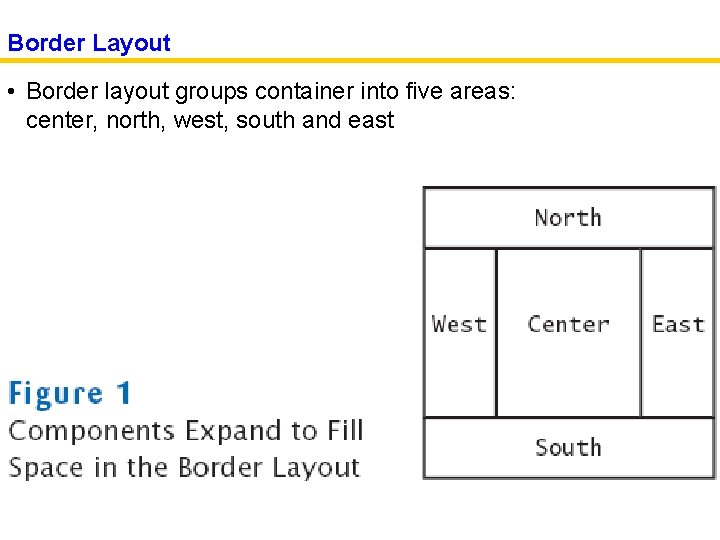
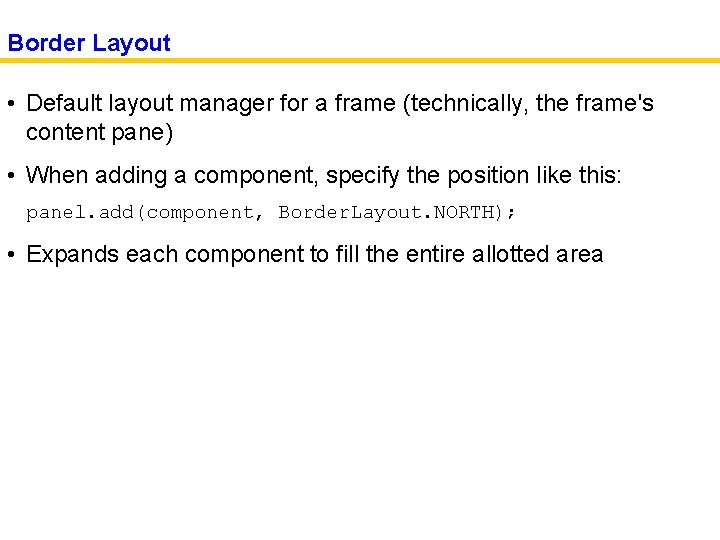
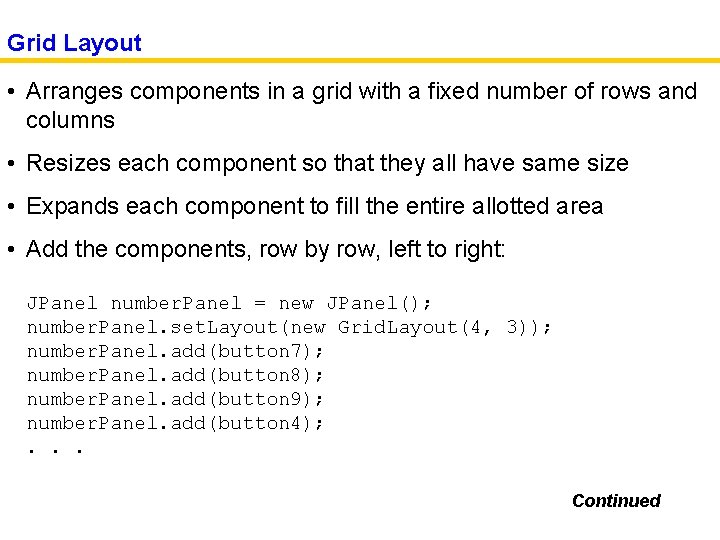
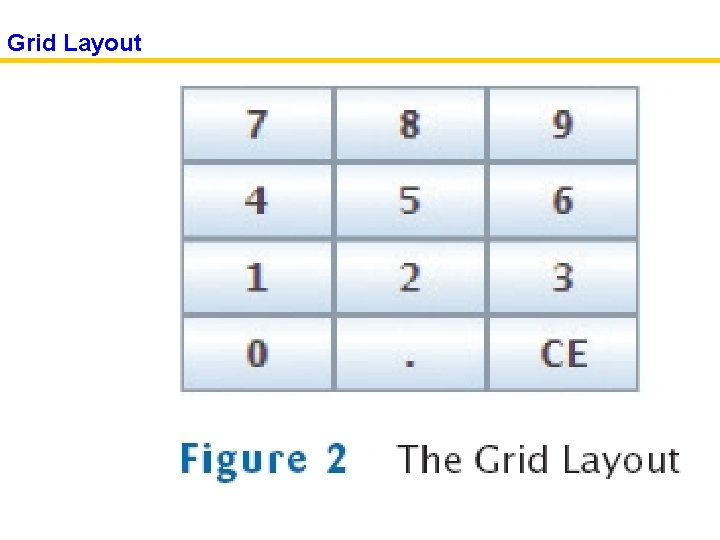
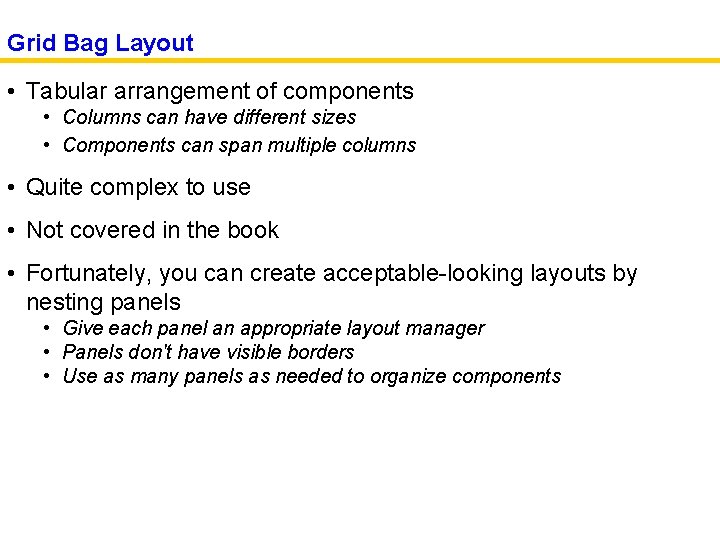
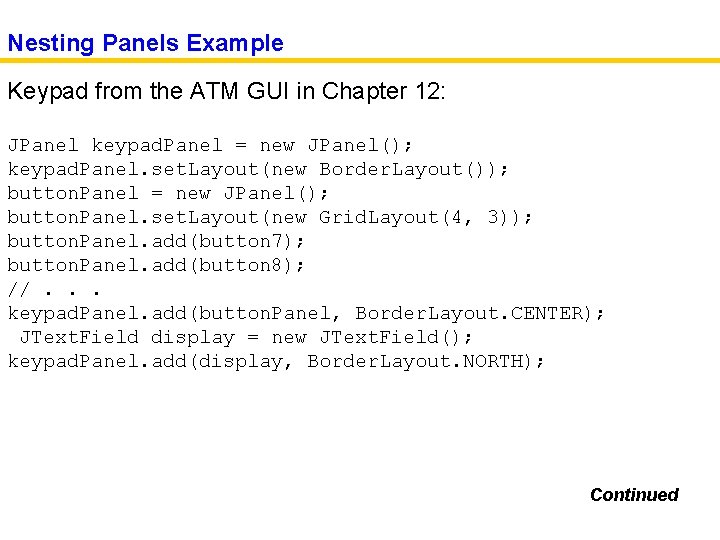
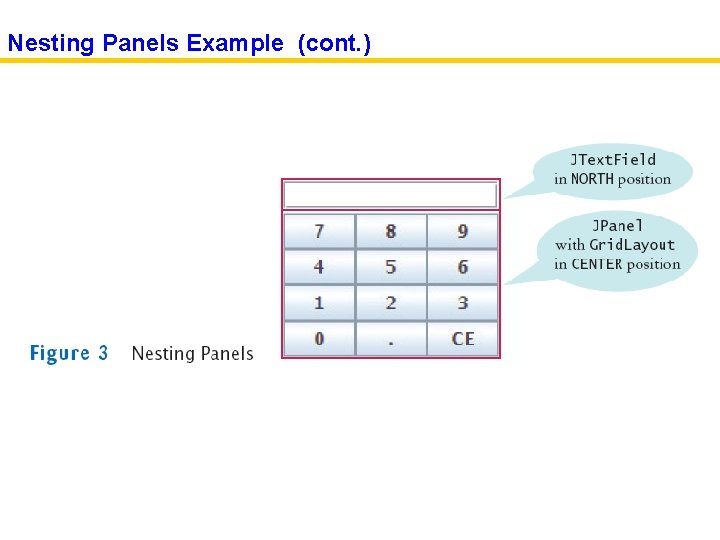
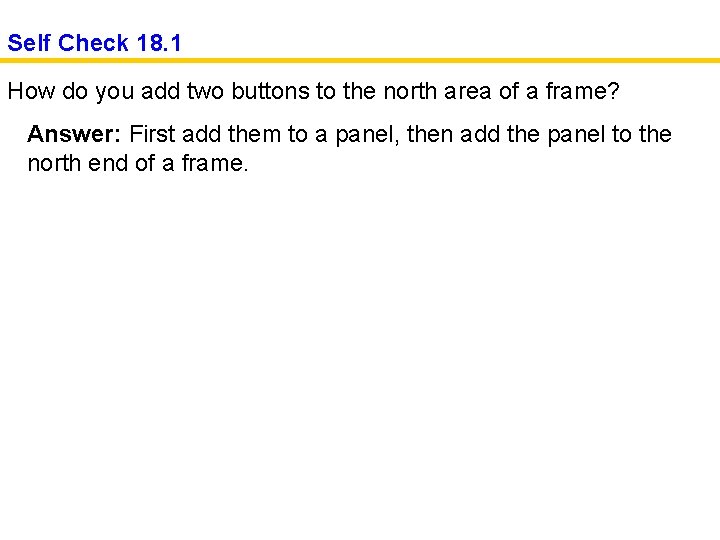
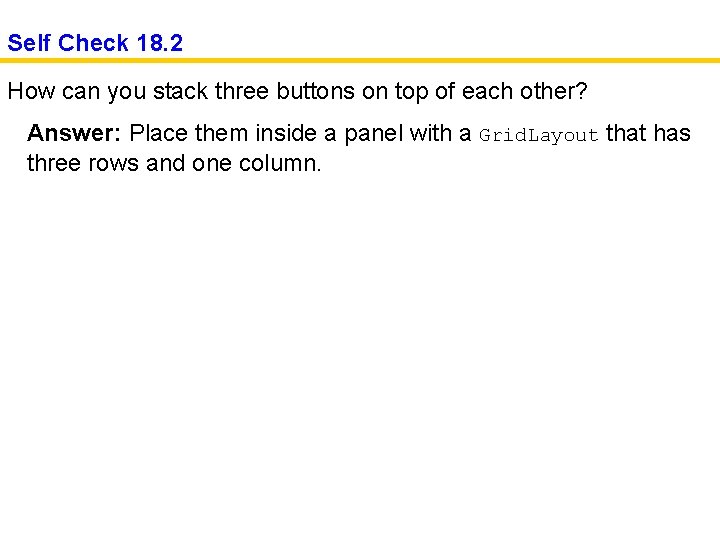
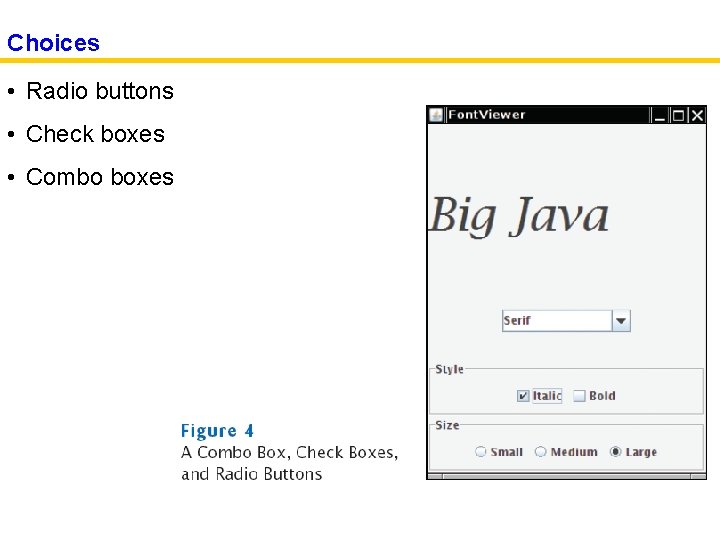
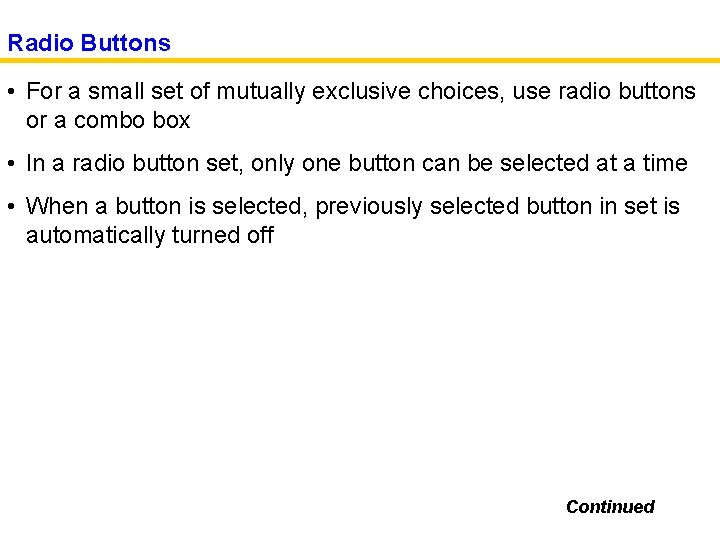
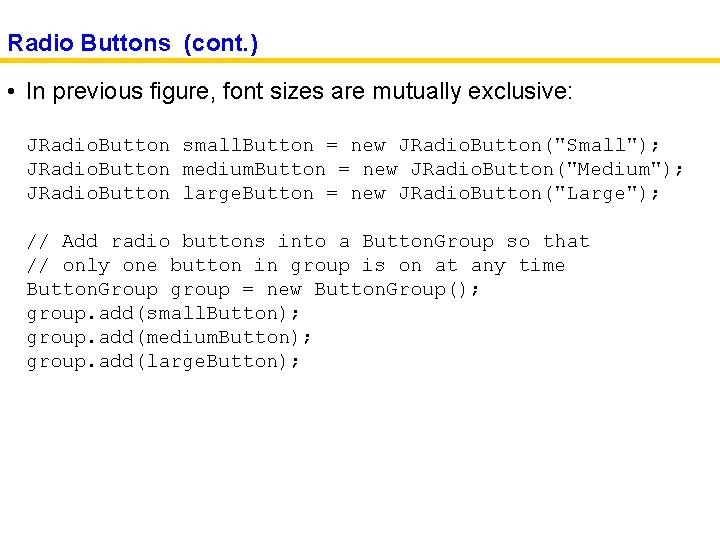
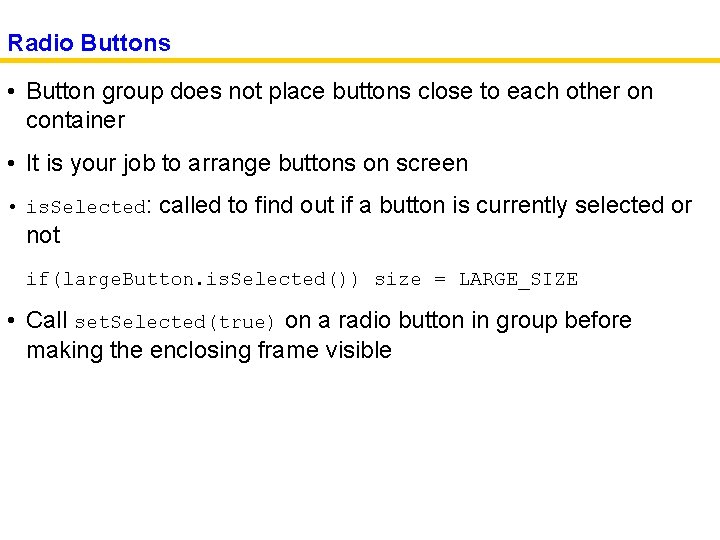
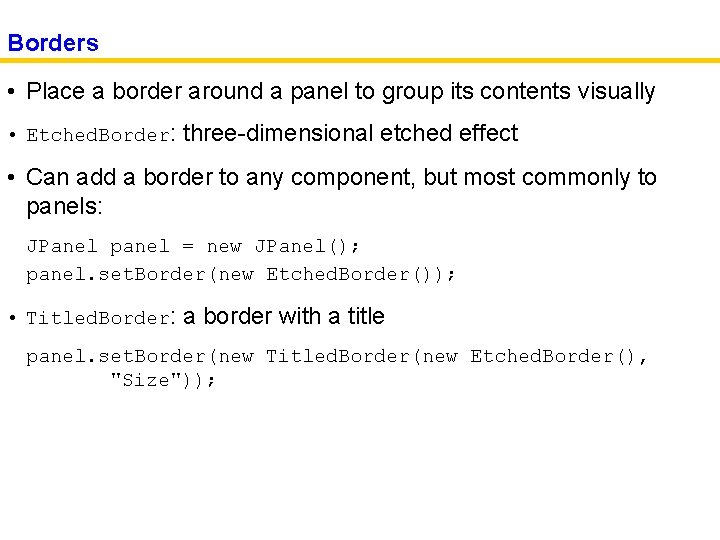
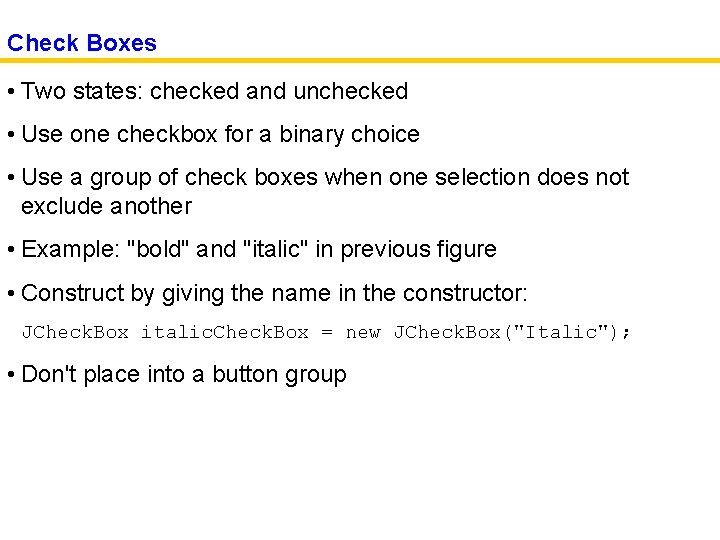
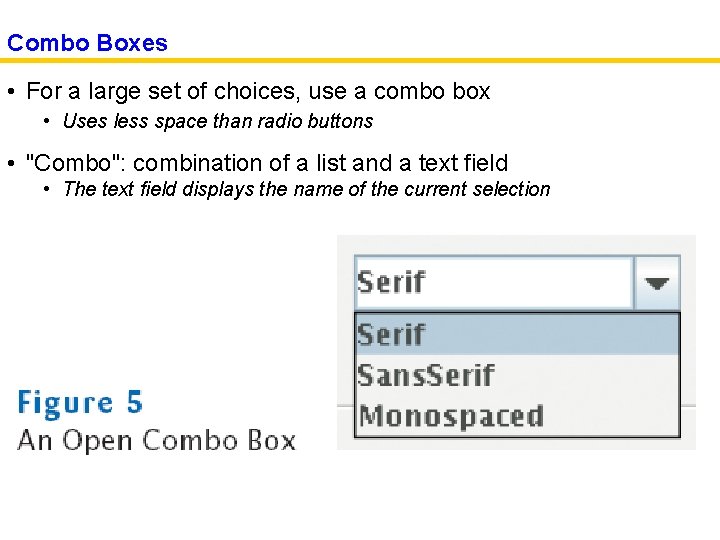
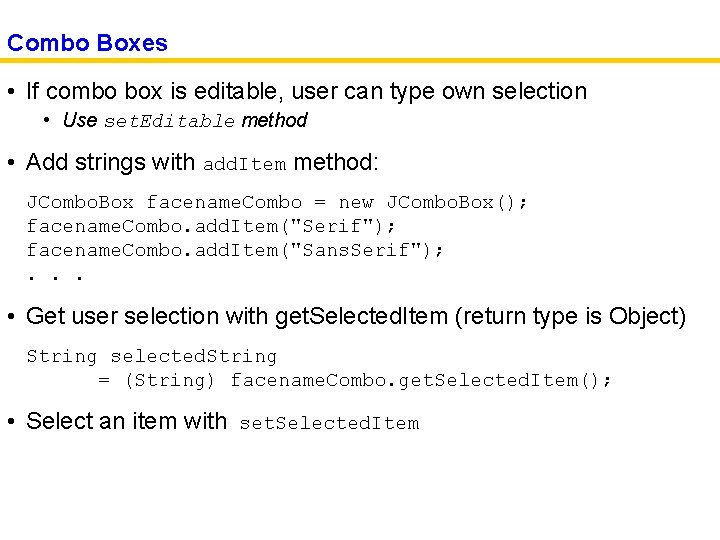
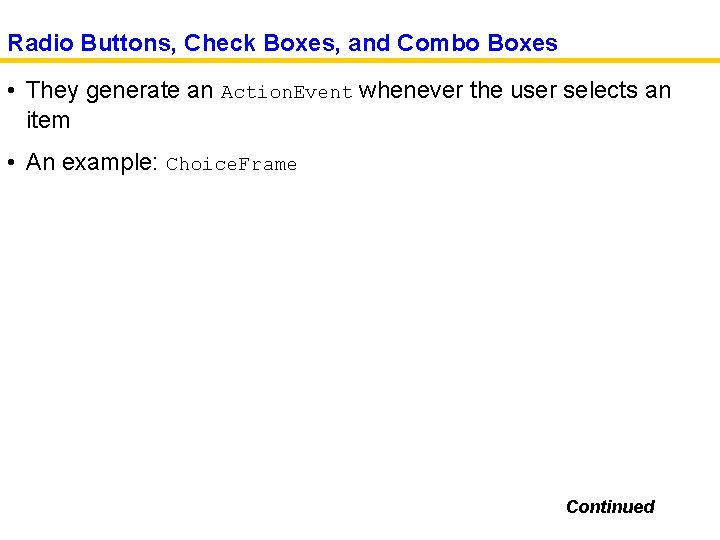
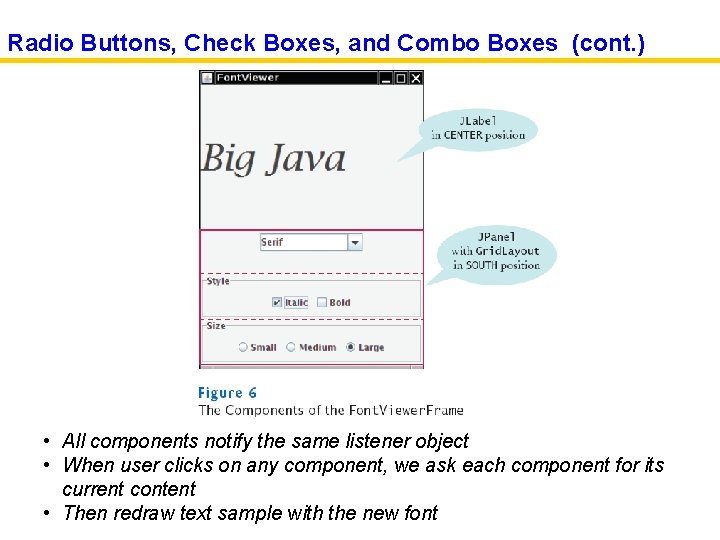
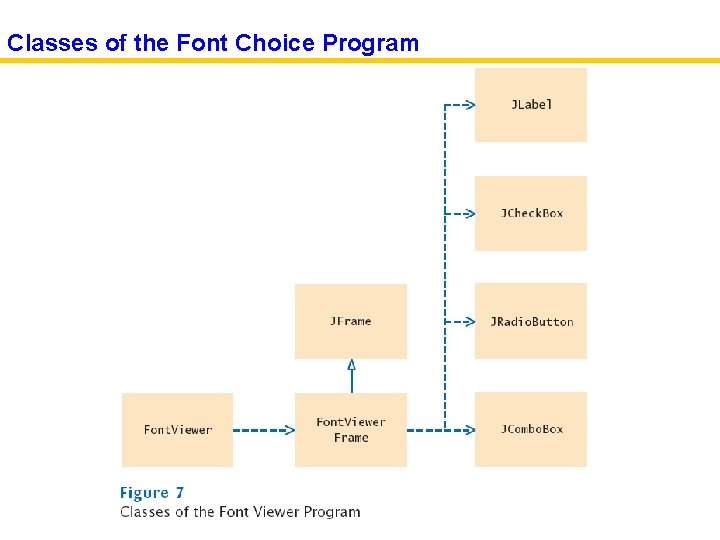
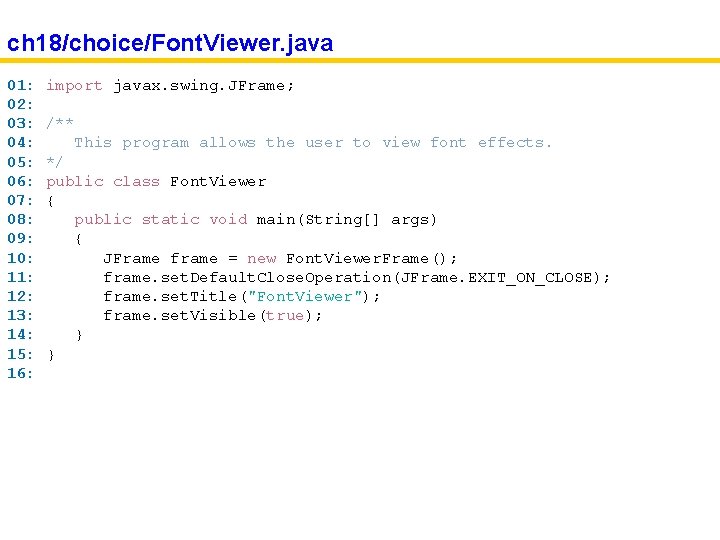
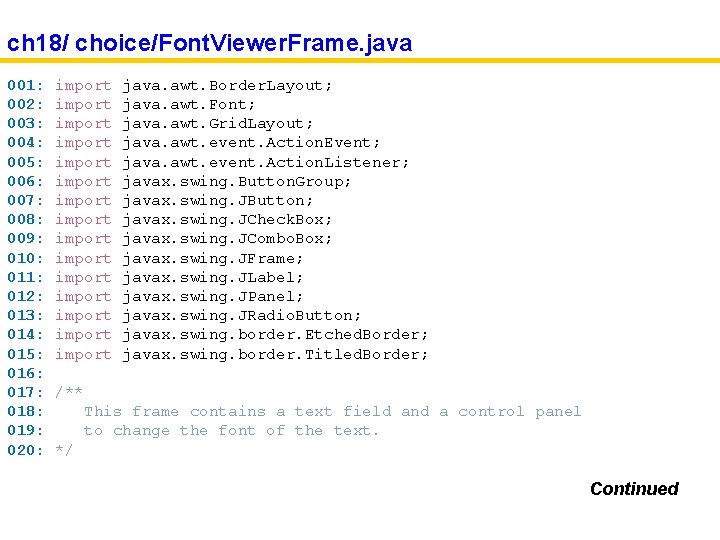
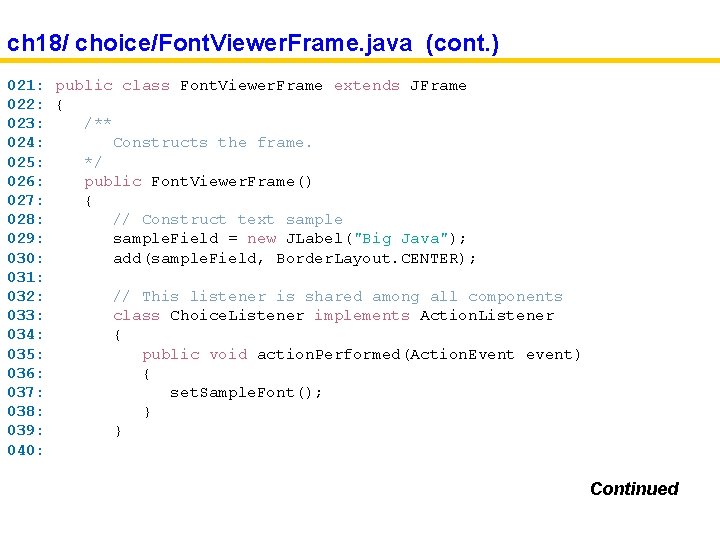
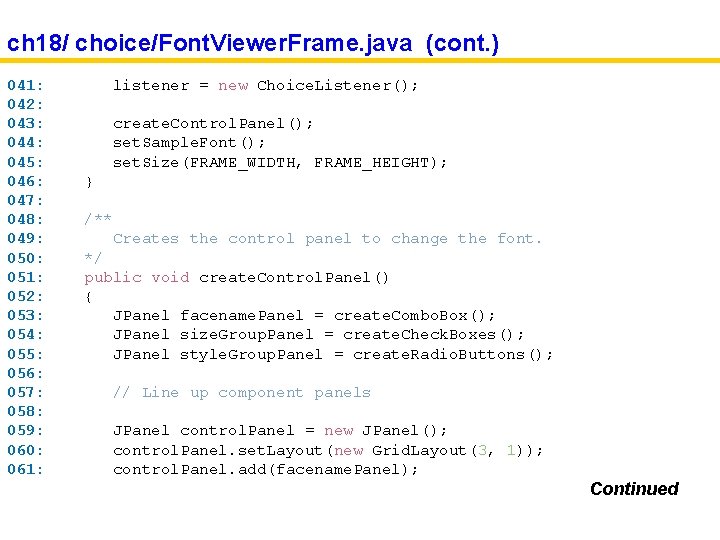
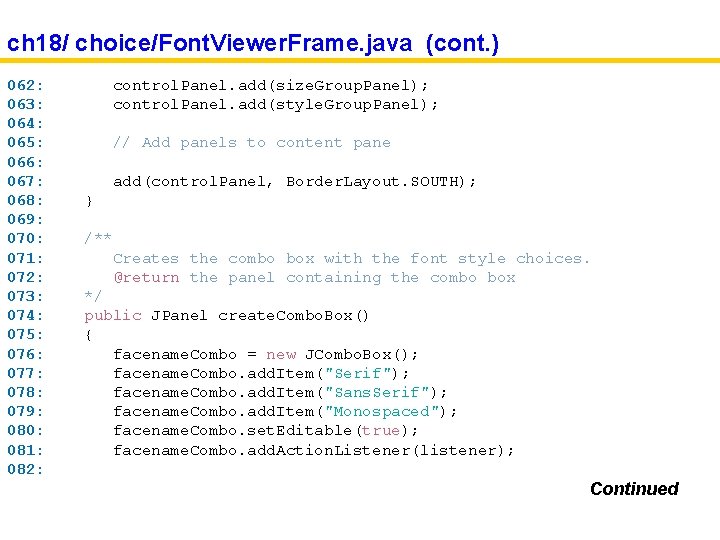
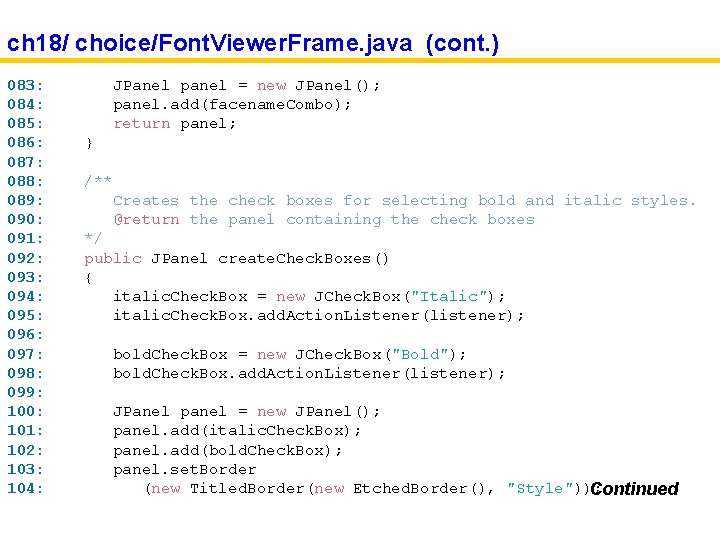
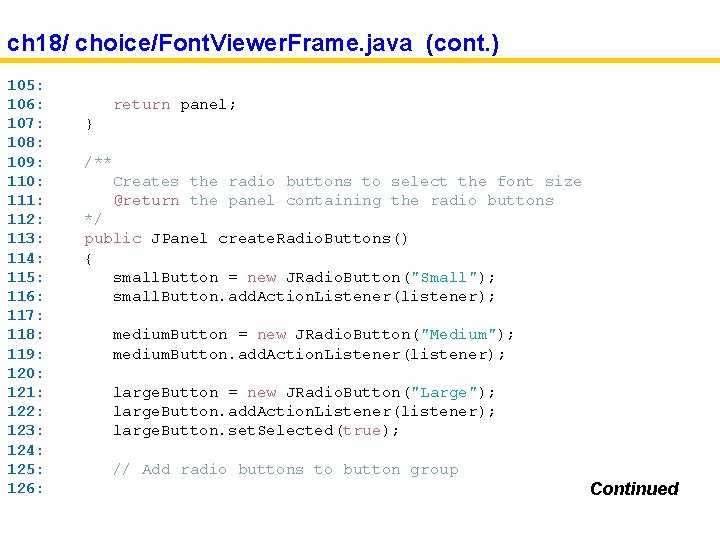
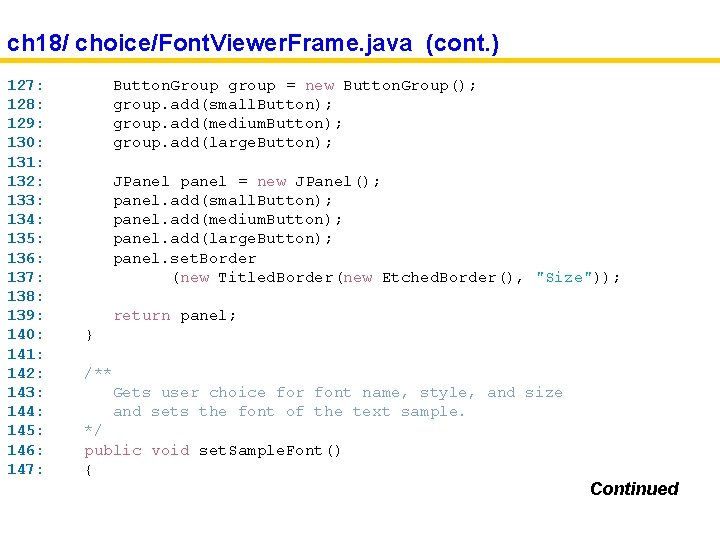
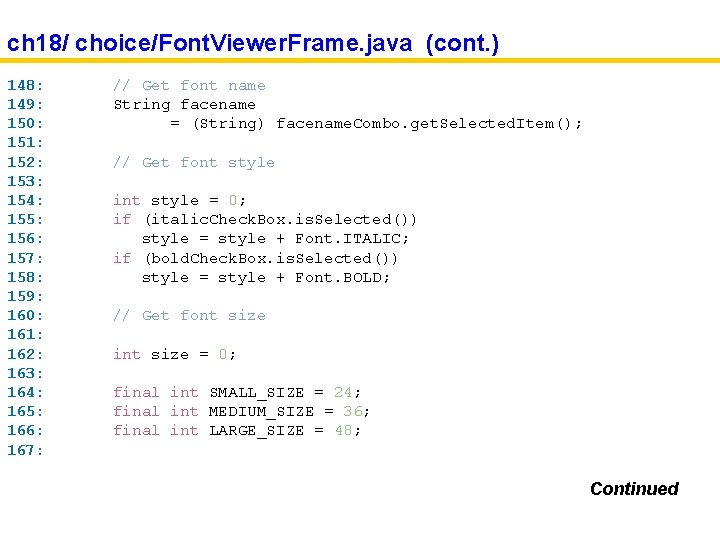
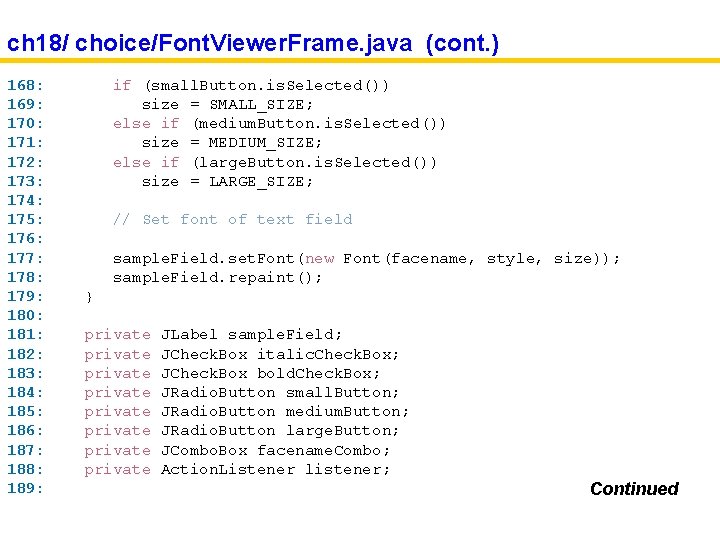
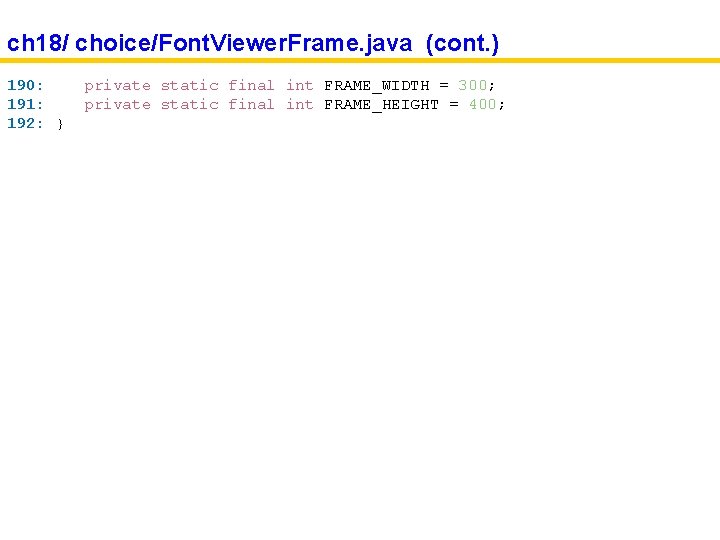
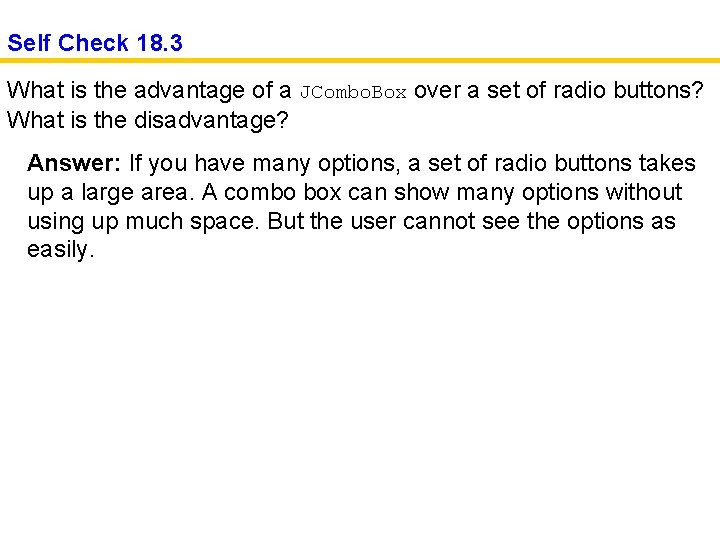
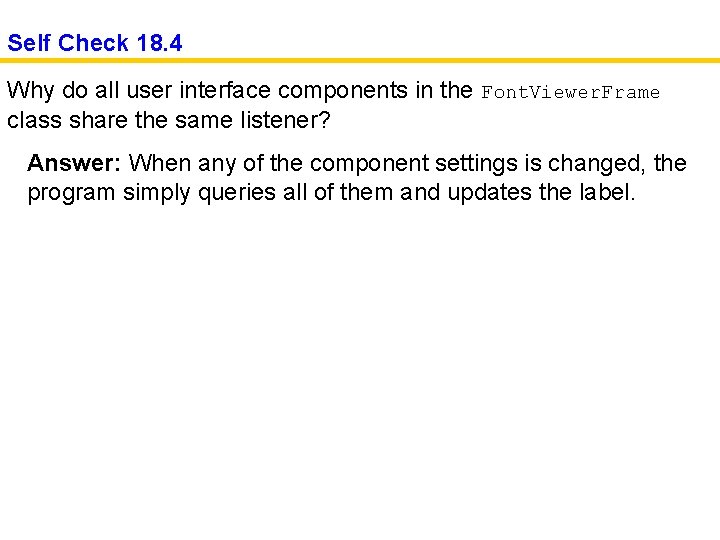
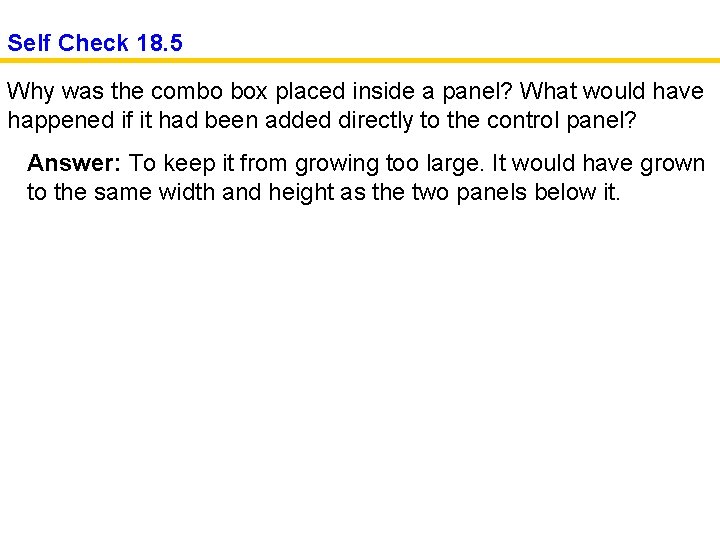
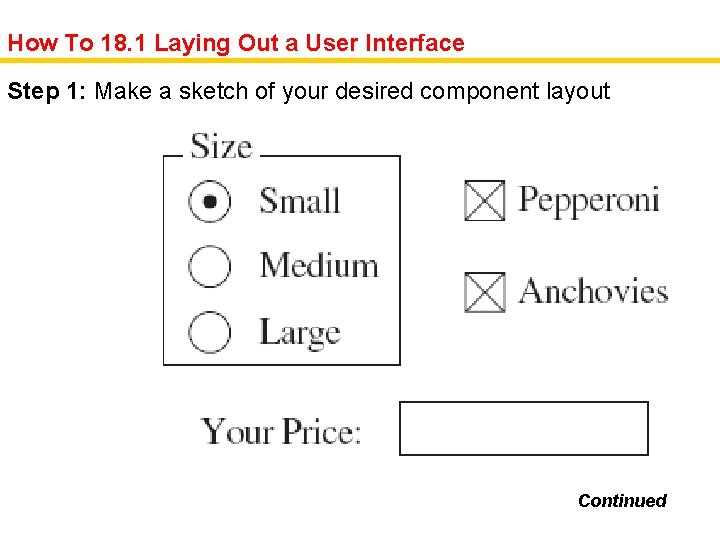
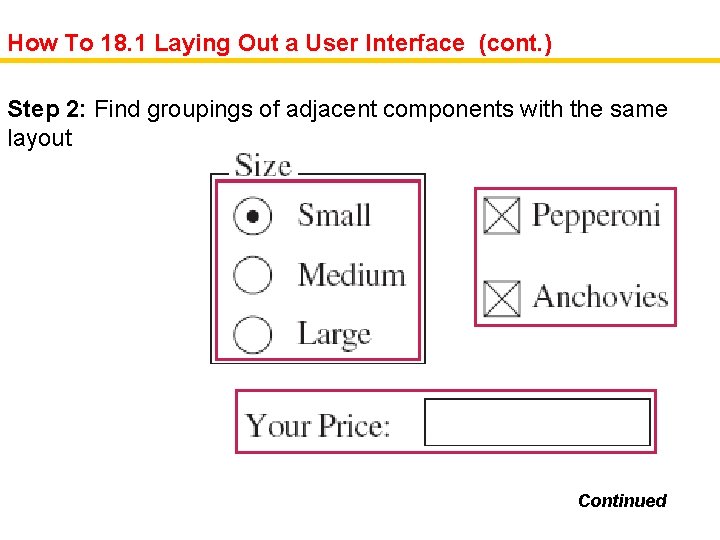
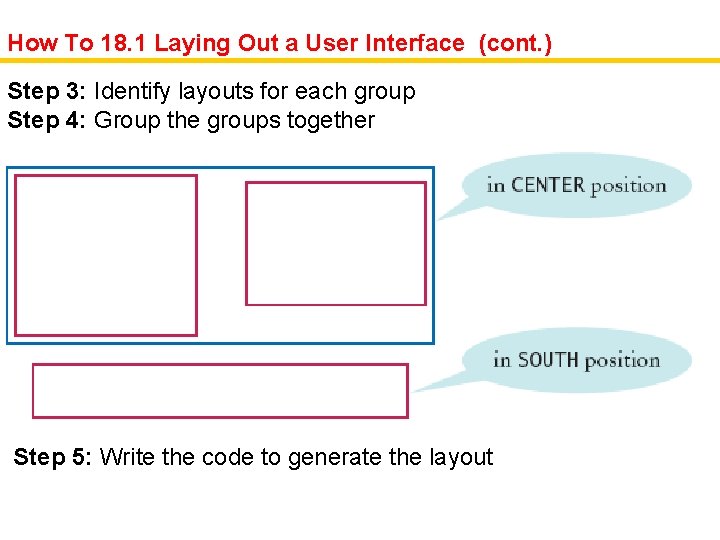
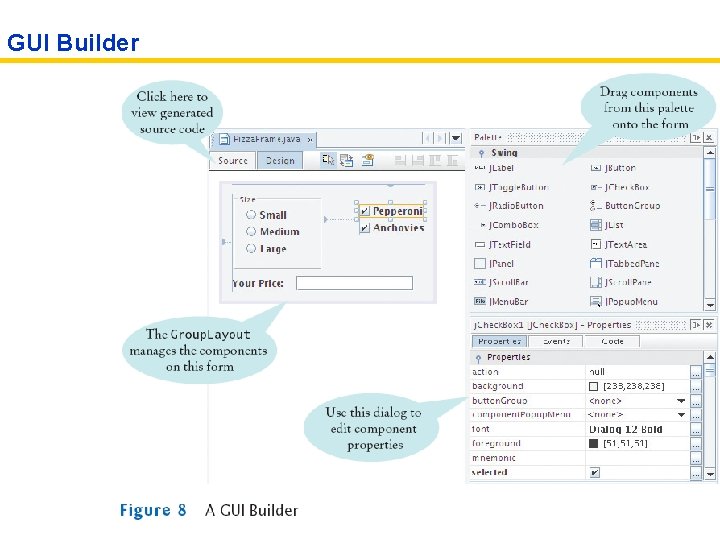
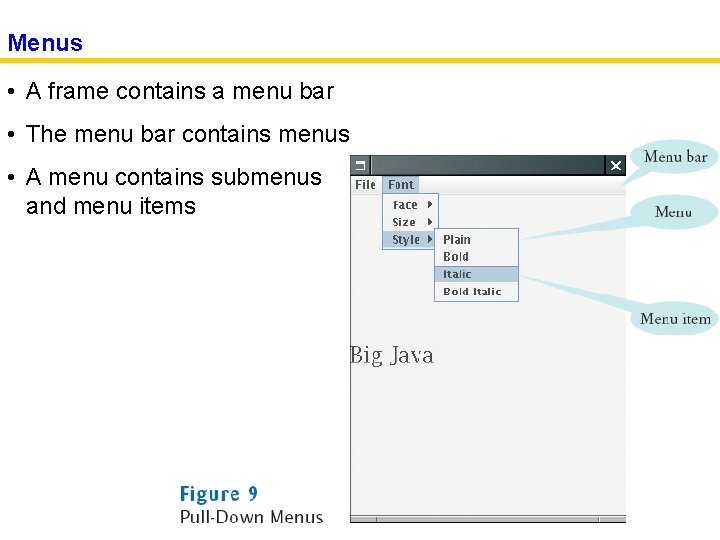
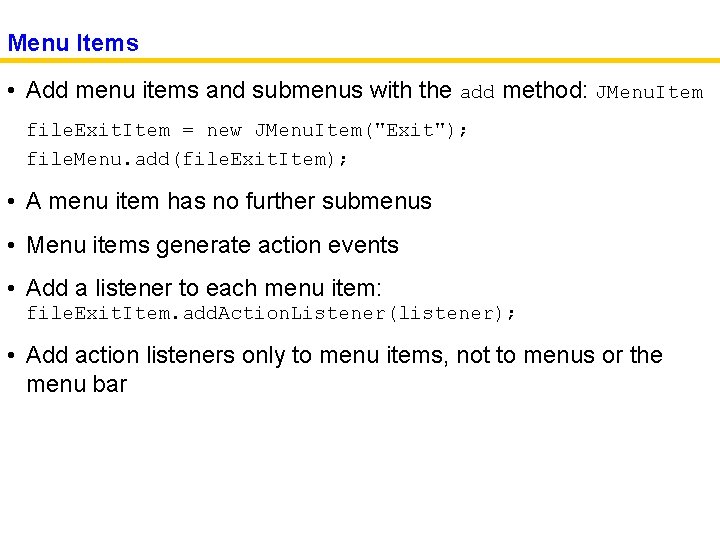
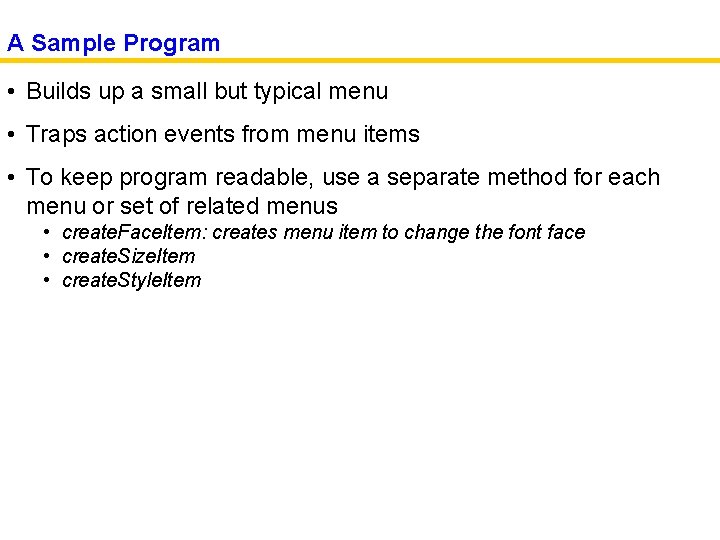
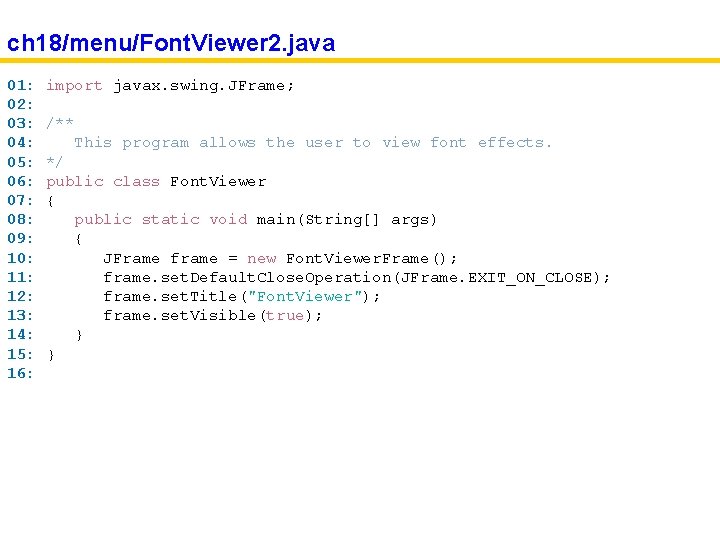
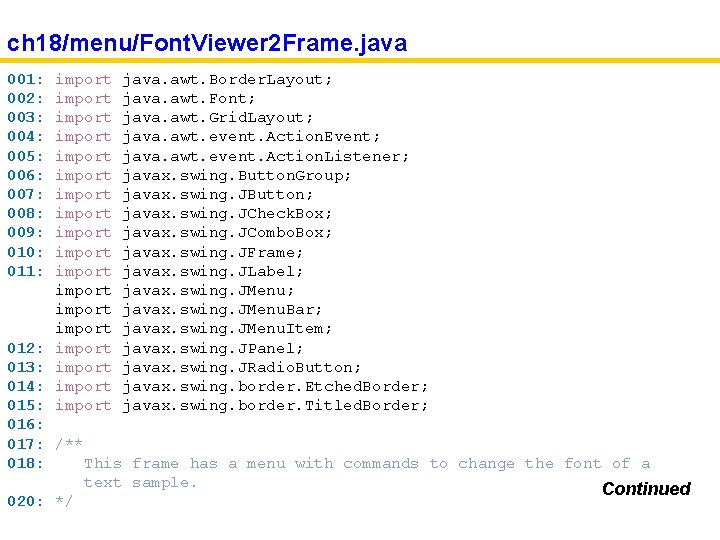
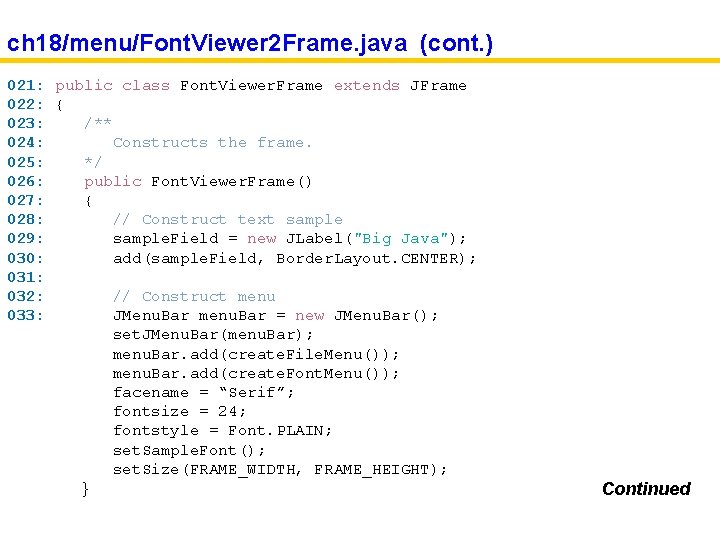
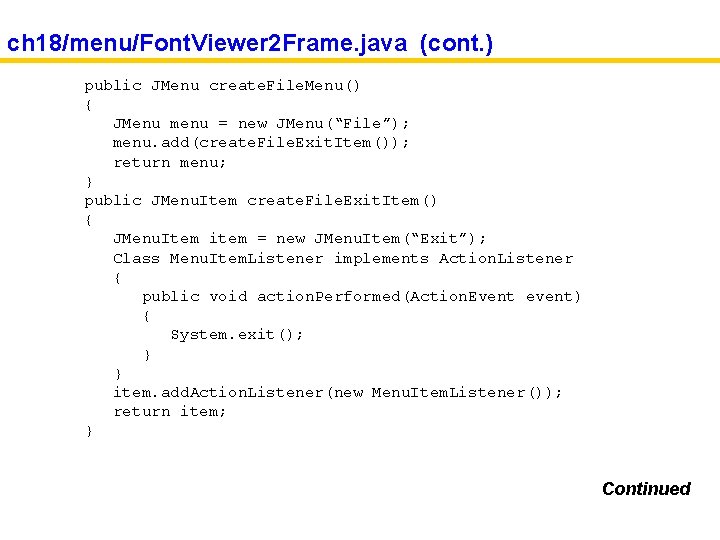
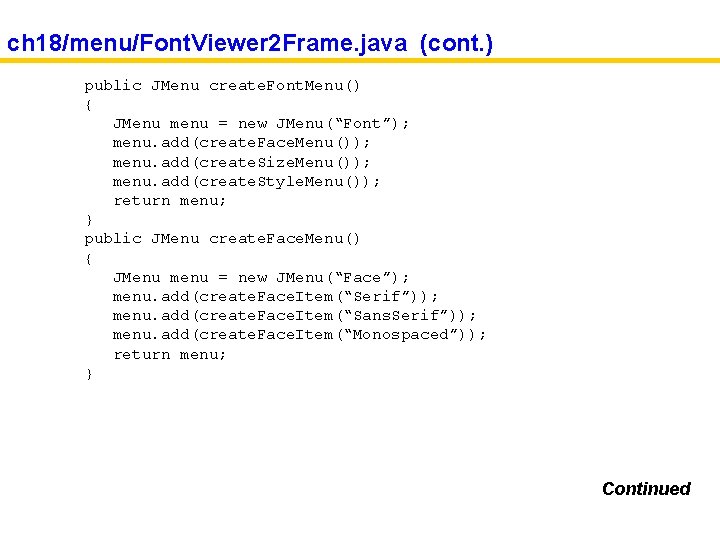
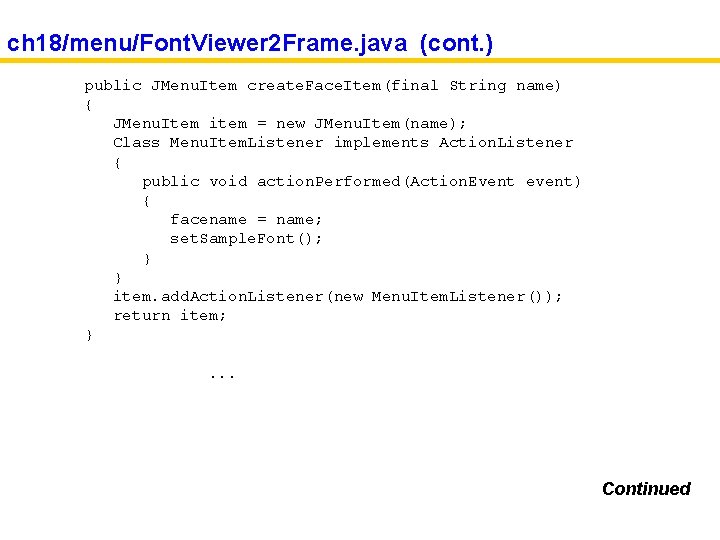
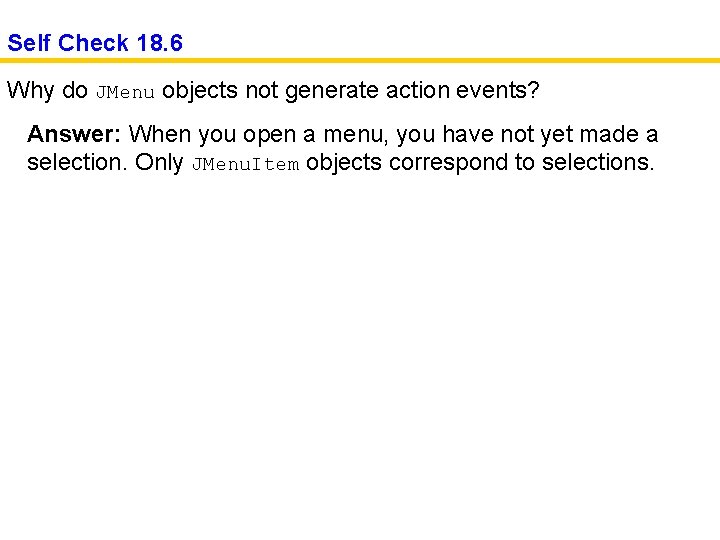
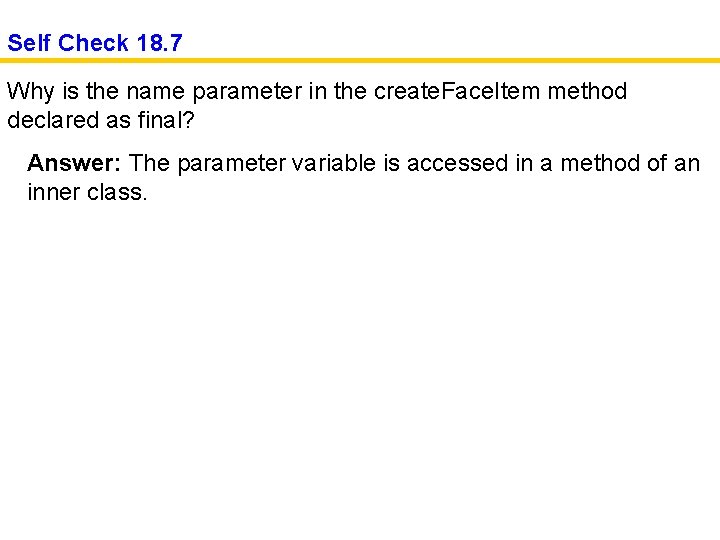
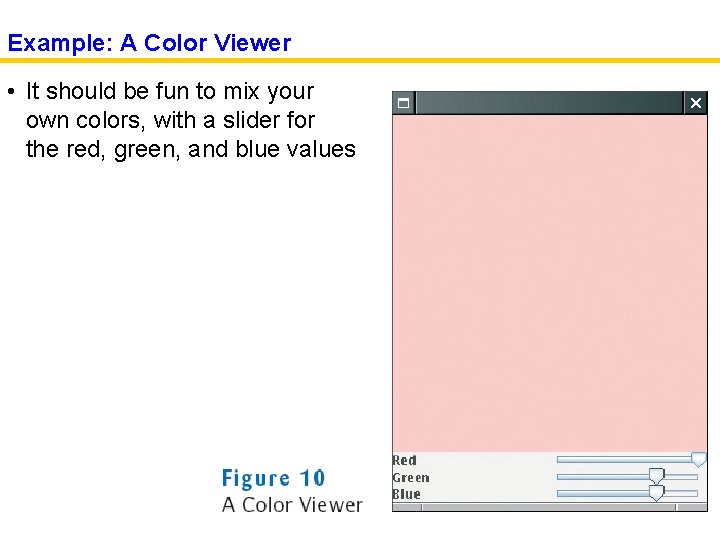
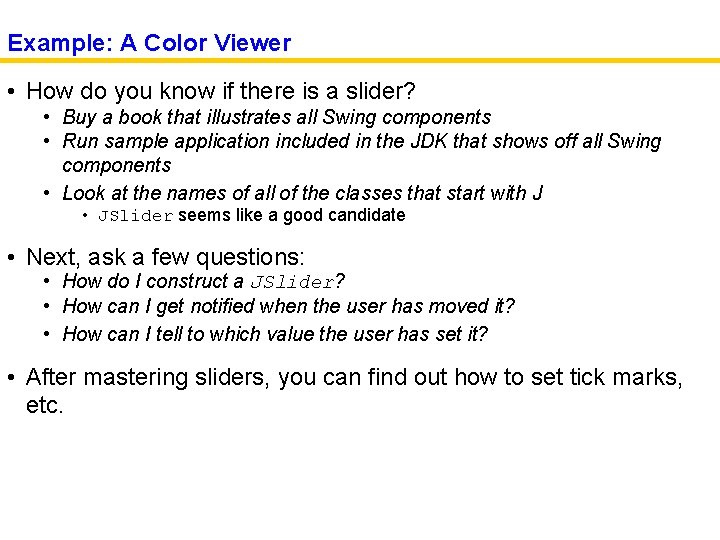
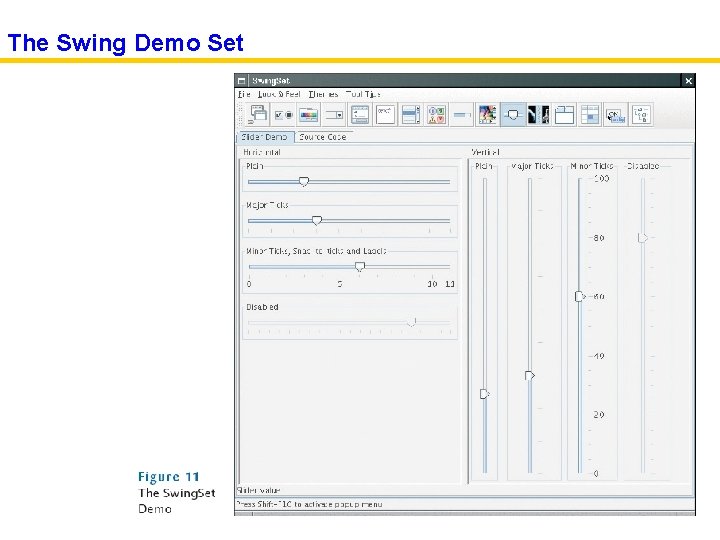
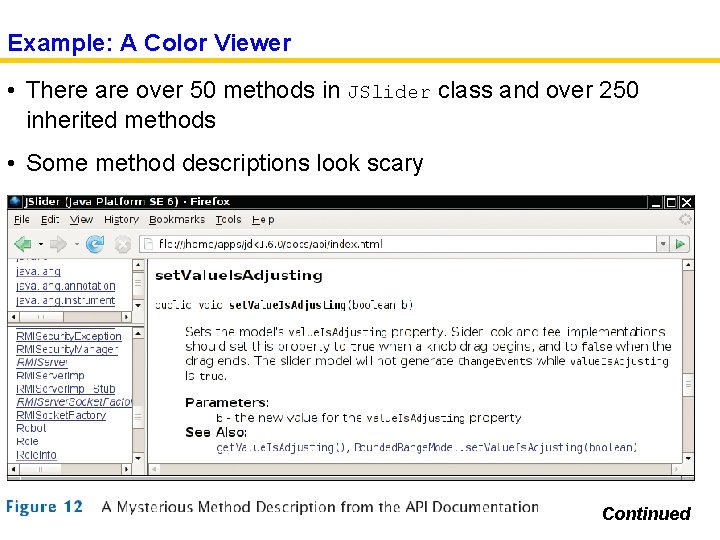
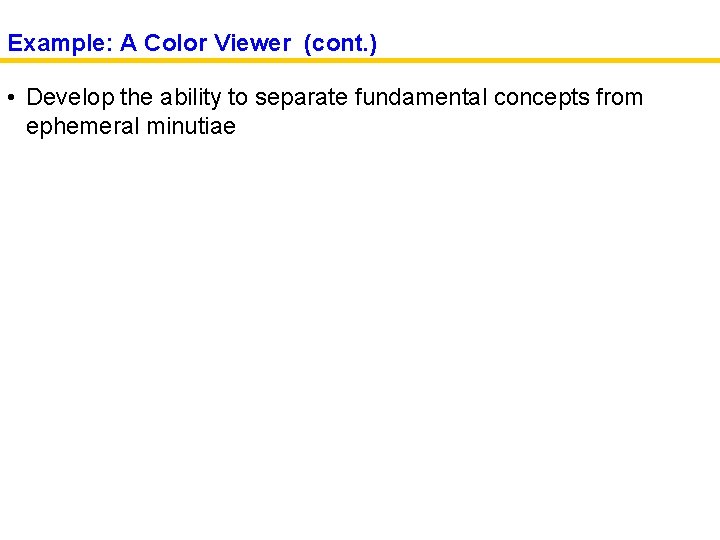
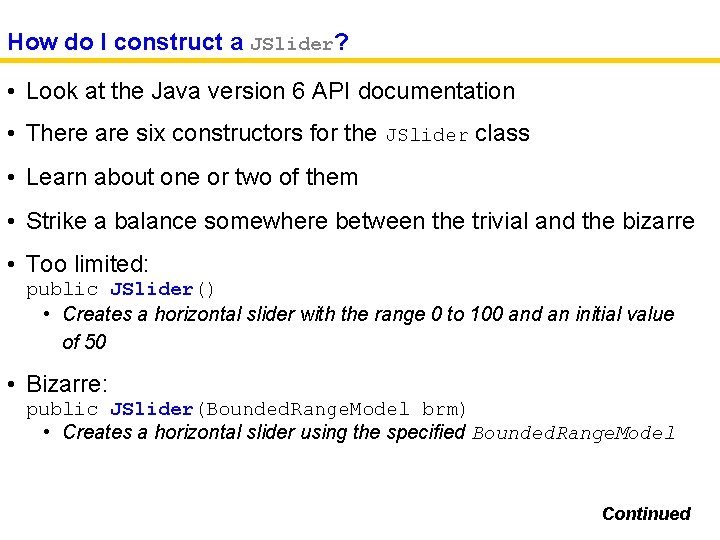
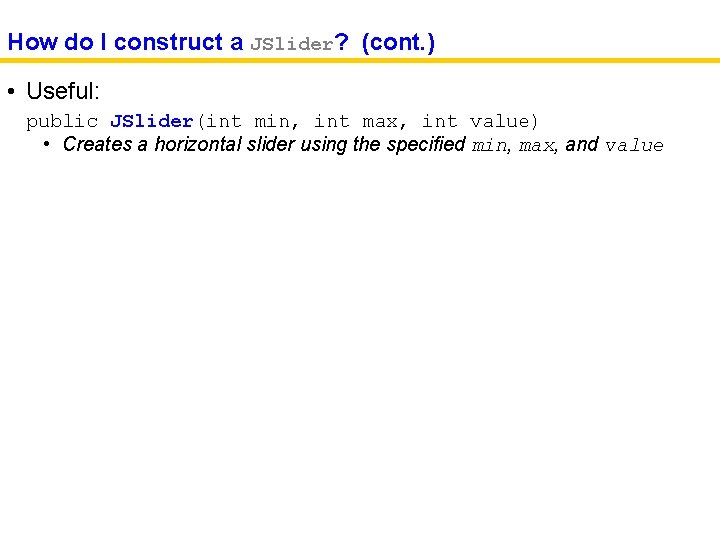
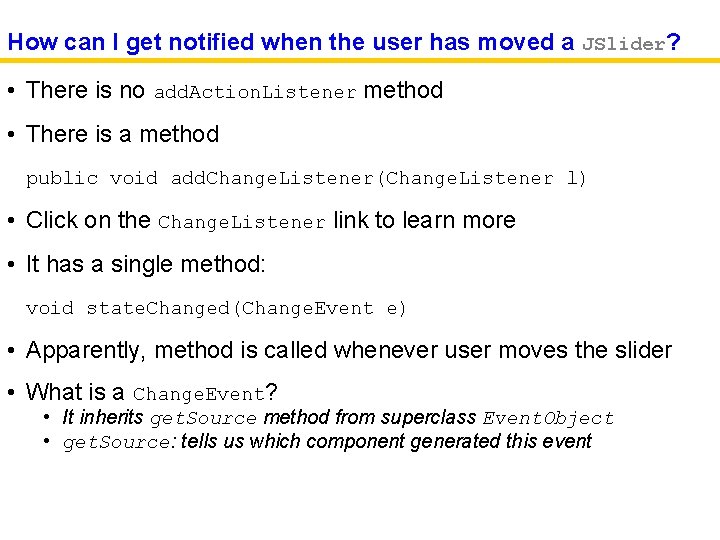
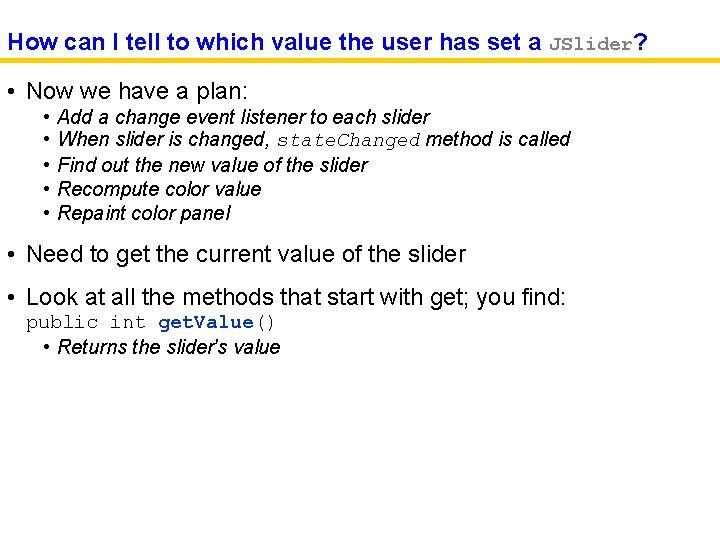
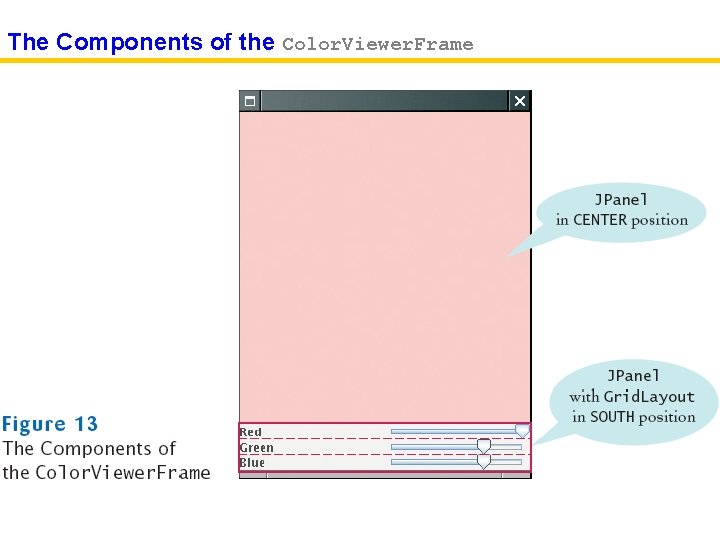
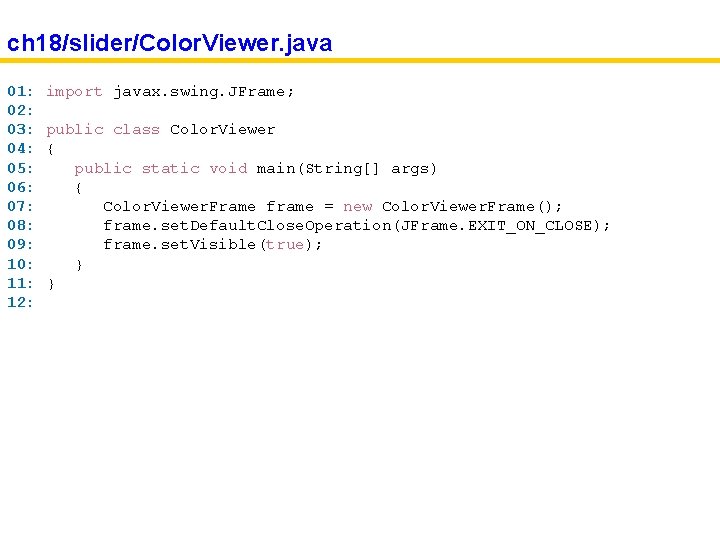
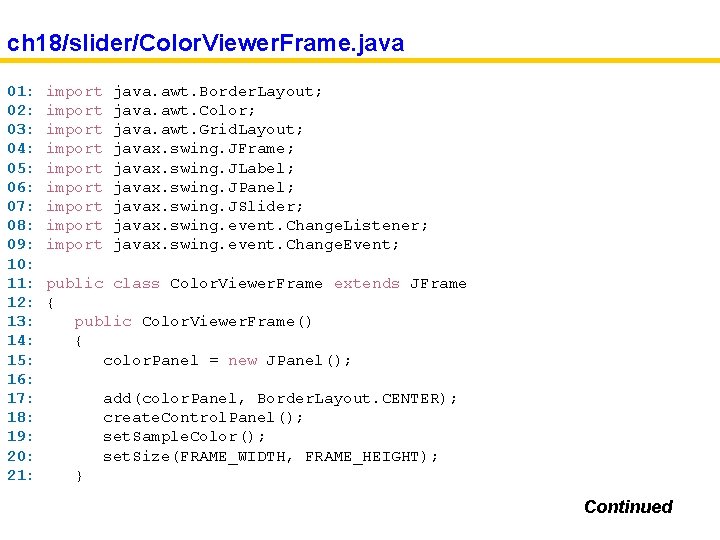
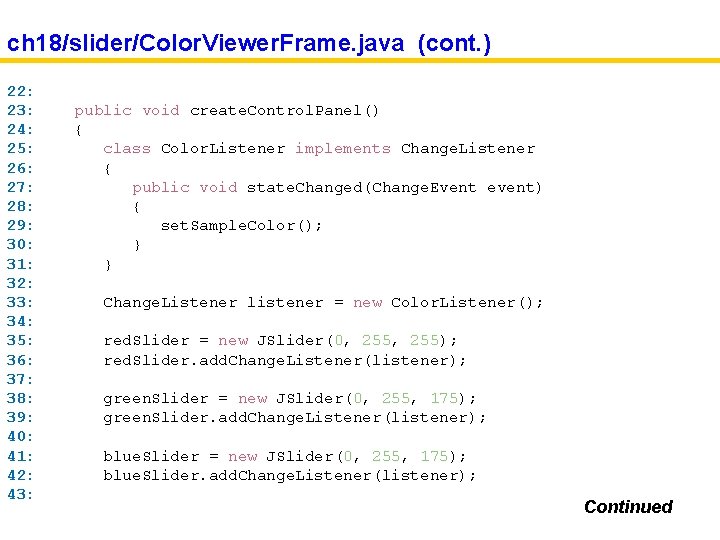
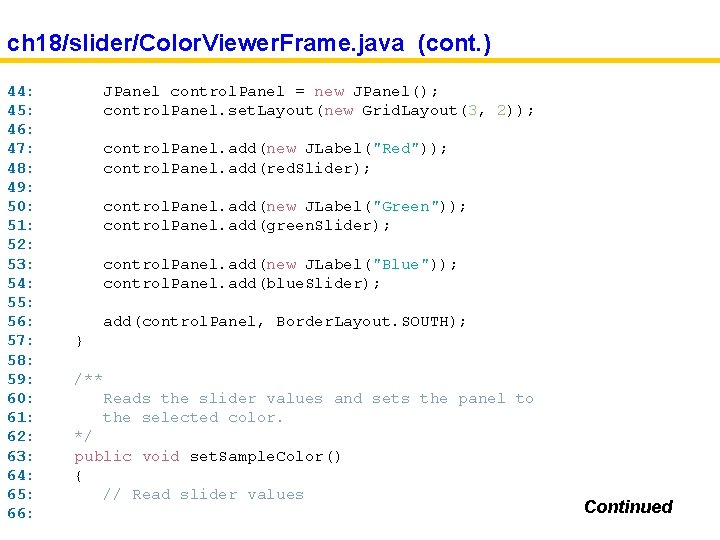
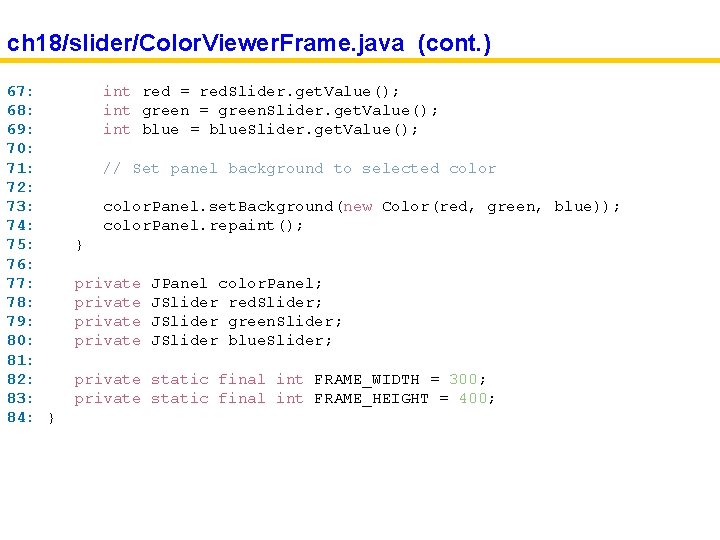
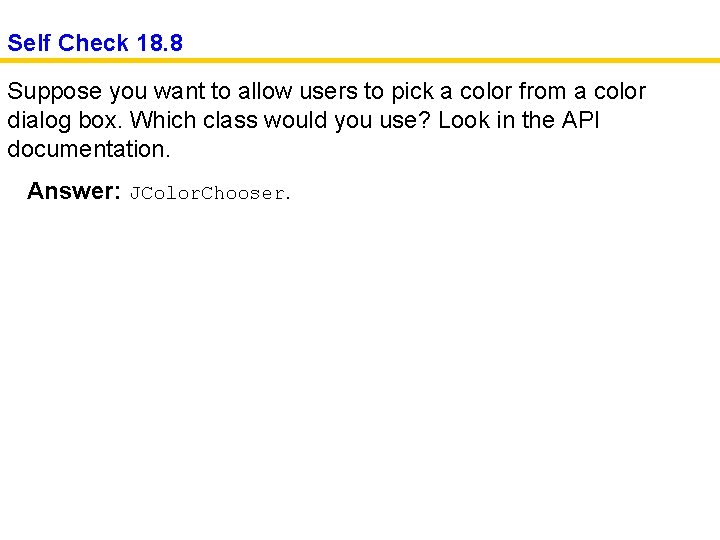
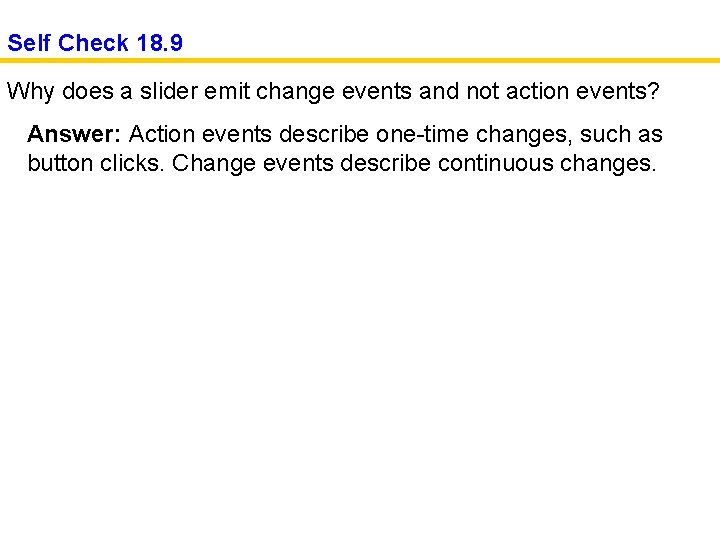
- Slides: 70
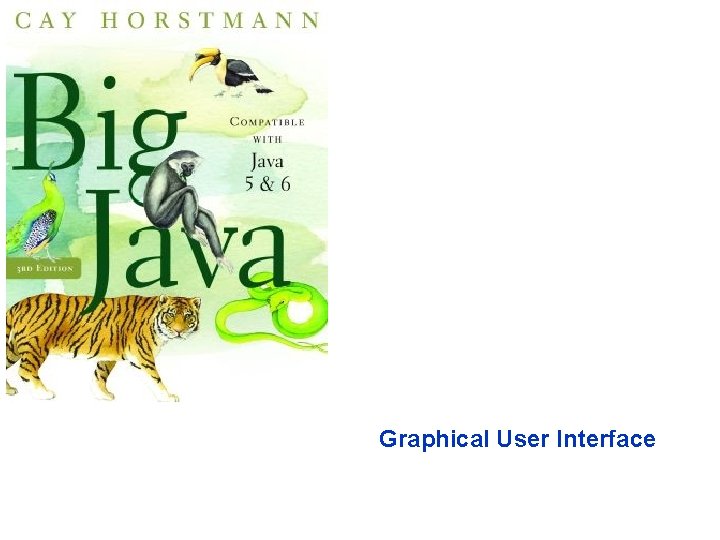
Graphical User Interface
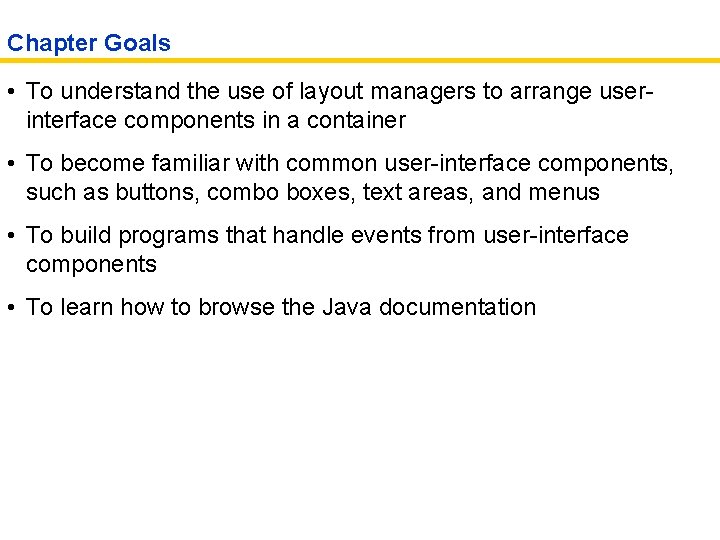
Chapter Goals • To understand the use of layout managers to arrange userinterface components in a container • To become familiar with common user-interface components, such as buttons, combo boxes, text areas, and menus • To build programs that handle events from user-interface components • To learn how to browse the Java documentation
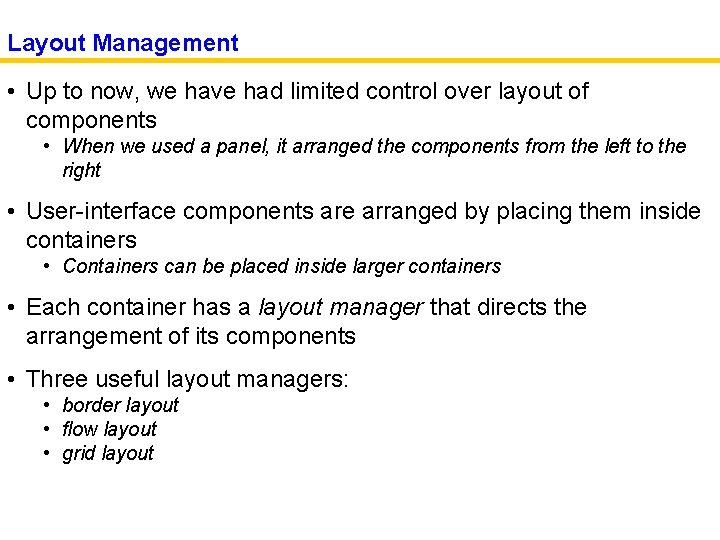
Layout Management • Up to now, we have had limited control over layout of components • When we used a panel, it arranged the components from the left to the right • User-interface components are arranged by placing them inside containers • Containers can be placed inside larger containers • Each container has a layout manager that directs the arrangement of its components • Three useful layout managers: • border layout • flow layout • grid layout
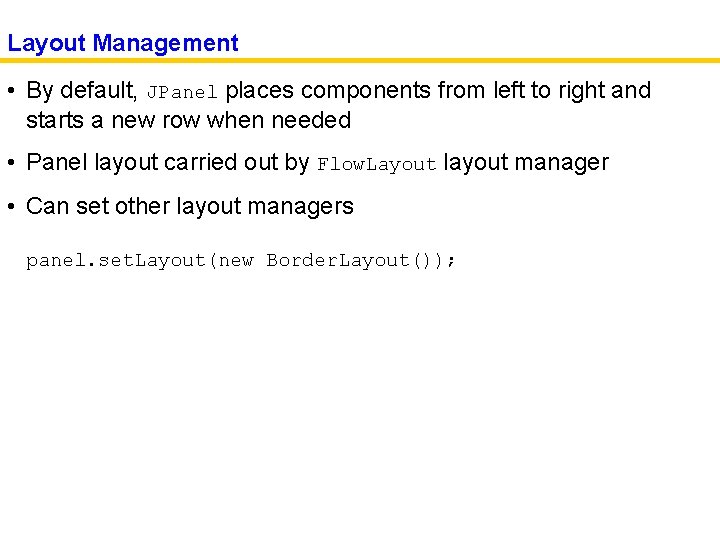
Layout Management • By default, JPanel places components from left to right and starts a new row when needed • Panel layout carried out by Flow. Layout layout manager • Can set other layout managers panel. set. Layout(new Border. Layout());
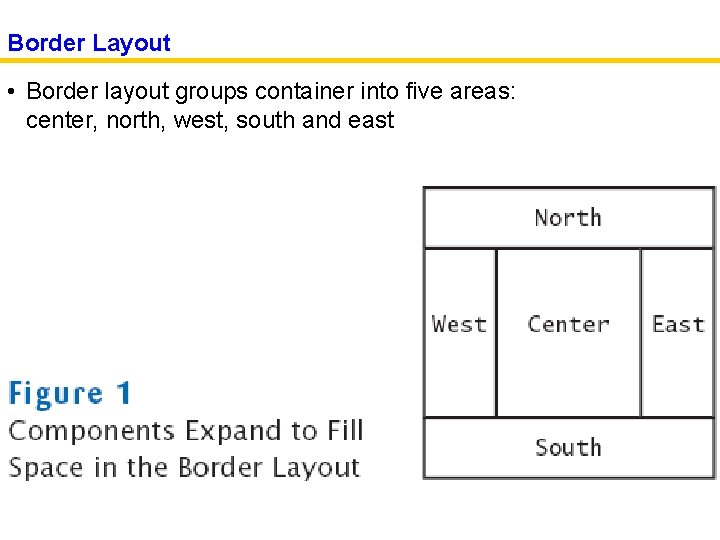
Border Layout • Border layout groups container into five areas: center, north, west, south and east
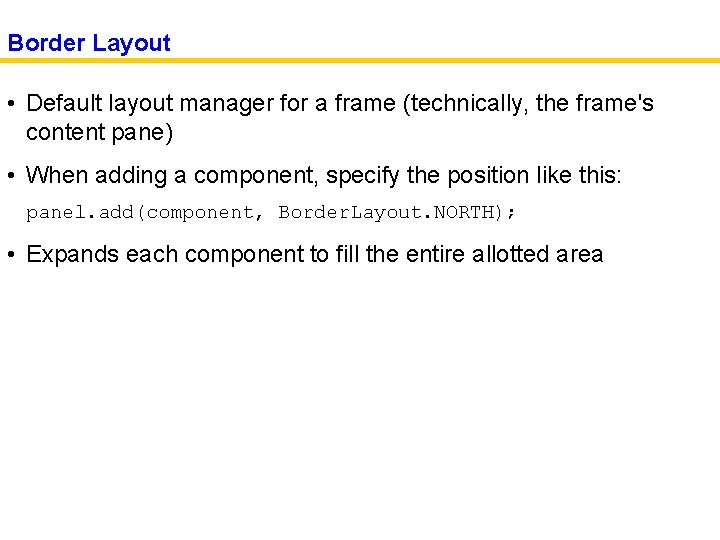
Border Layout • Default layout manager for a frame (technically, the frame's content pane) • When adding a component, specify the position like this: panel. add(component, Border. Layout. NORTH); • Expands each component to fill the entire allotted area
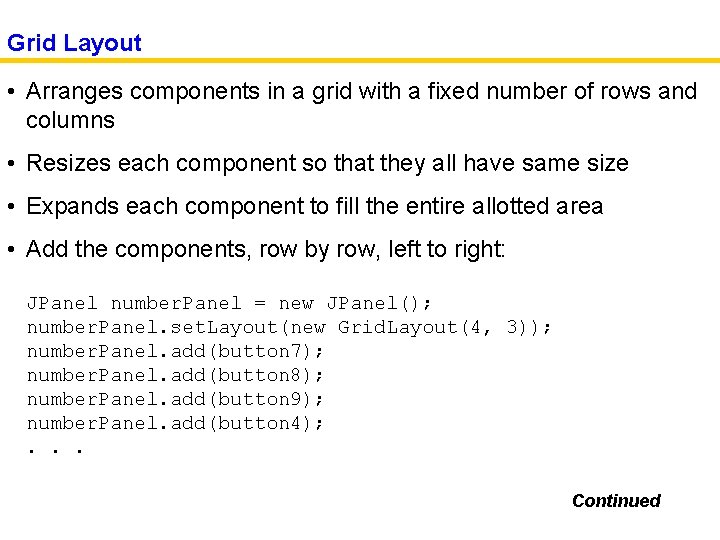
Grid Layout • Arranges components in a grid with a fixed number of rows and columns • Resizes each component so that they all have same size • Expands each component to fill the entire allotted area • Add the components, row by row, left to right: JPanel number. Panel = new JPanel(); number. Panel. set. Layout(new Grid. Layout(4, 3)); number. Panel. add(button 7); number. Panel. add(button 8); number. Panel. add(button 9); number. Panel. add(button 4); . . . Continued
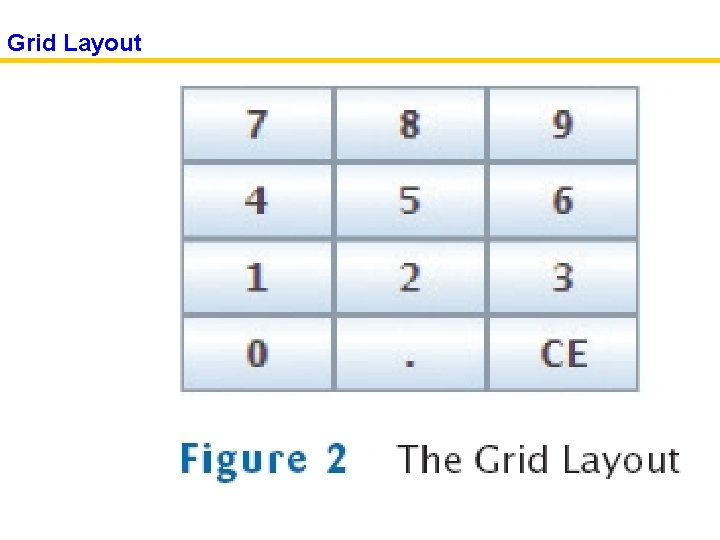
Grid Layout
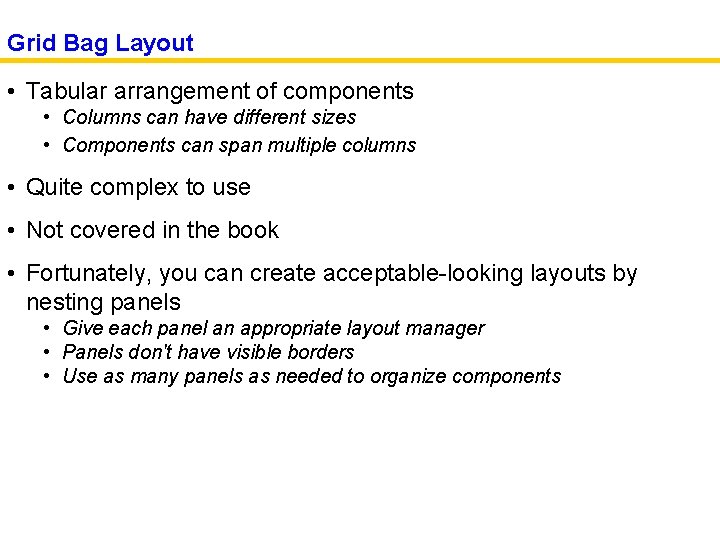
Grid Bag Layout • Tabular arrangement of components • Columns can have different sizes • Components can span multiple columns • Quite complex to use • Not covered in the book • Fortunately, you can create acceptable-looking layouts by nesting panels • Give each panel an appropriate layout manager • Panels don't have visible borders • Use as many panels as needed to organize components
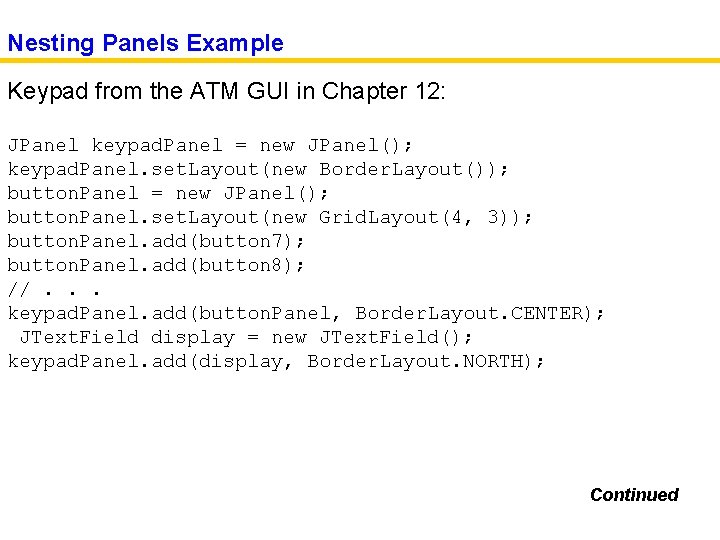
Nesting Panels Example Keypad from the ATM GUI in Chapter 12: JPanel keypad. Panel = new JPanel(); keypad. Panel. set. Layout(new Border. Layout()); button. Panel = new JPanel(); button. Panel. set. Layout(new Grid. Layout(4, 3)); button. Panel. add(button 7); button. Panel. add(button 8); //. . . keypad. Panel. add(button. Panel, Border. Layout. CENTER); JText. Field display = new JText. Field(); keypad. Panel. add(display, Border. Layout. NORTH); Continued
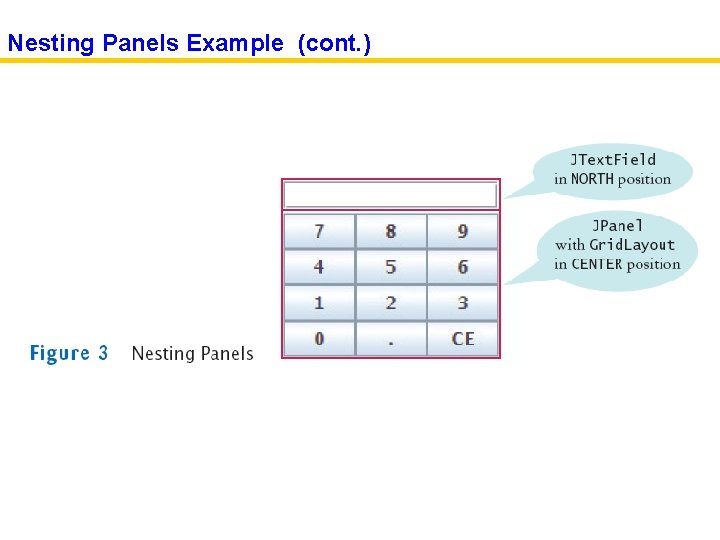
Nesting Panels Example (cont. )
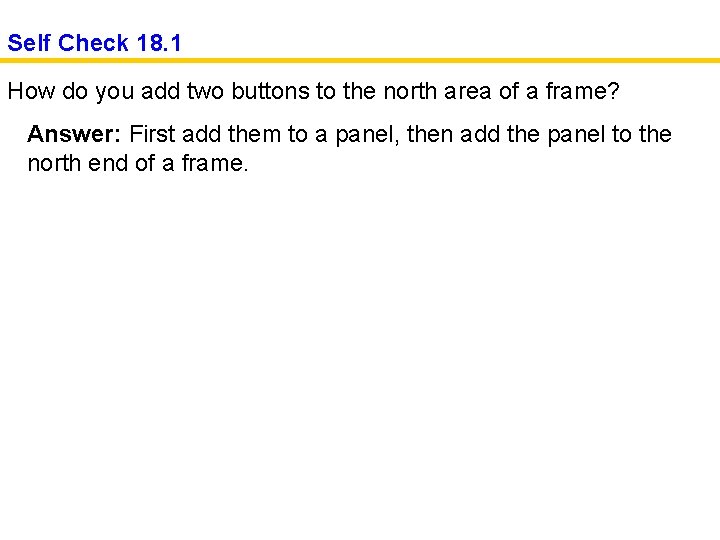
Self Check 18. 1 How do you add two buttons to the north area of a frame? Answer: First add them to a panel, then add the panel to the north end of a frame.
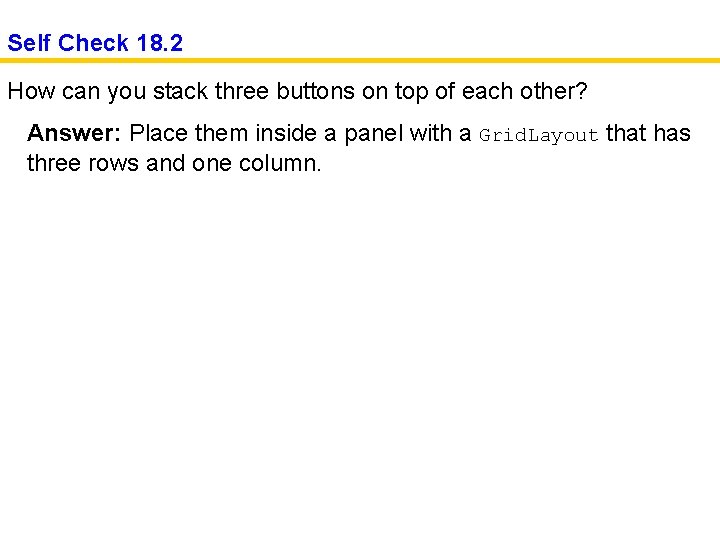
Self Check 18. 2 How can you stack three buttons on top of each other? Answer: Place them inside a panel with a Grid. Layout that has three rows and one column.
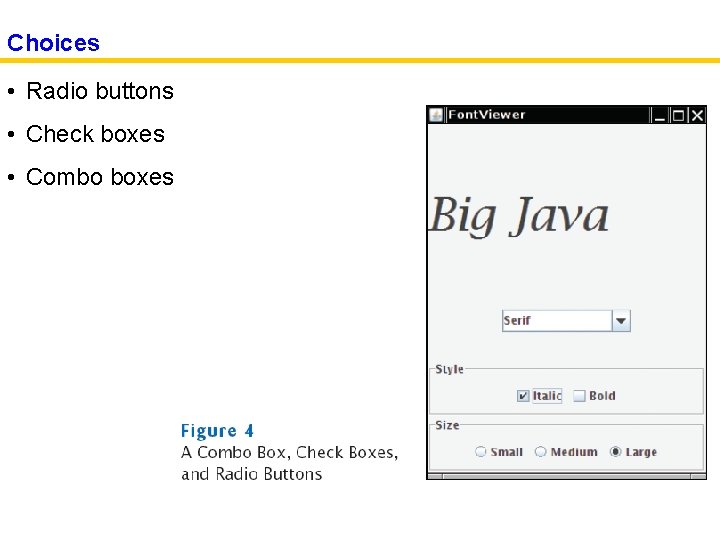
Choices • Radio buttons • Check boxes • Combo boxes
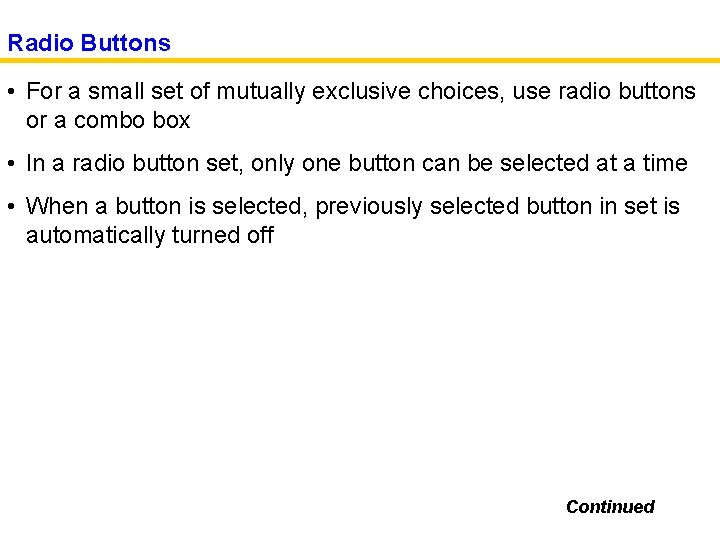
Radio Buttons • For a small set of mutually exclusive choices, use radio buttons or a combo box • In a radio button set, only one button can be selected at a time • When a button is selected, previously selected button in set is automatically turned off Continued
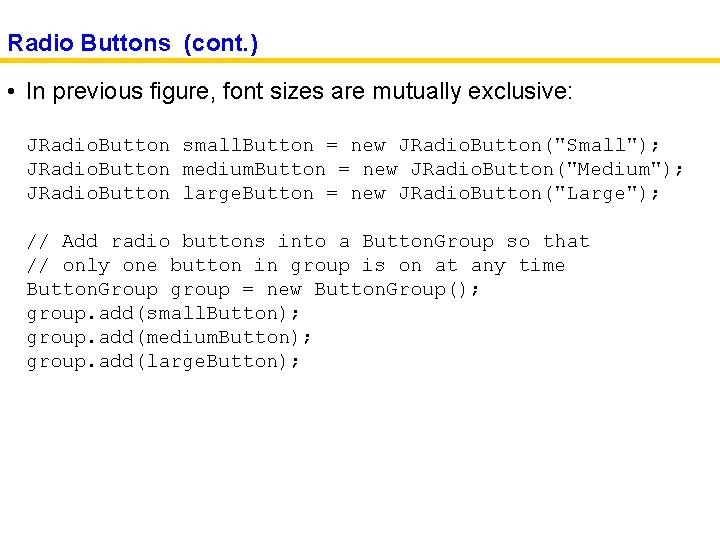
Radio Buttons (cont. ) • In previous figure, font sizes are mutually exclusive: JRadio. Button small. Button = new JRadio. Button("Small"); JRadio. Button medium. Button = new JRadio. Button("Medium"); JRadio. Button large. Button = new JRadio. Button("Large"); // Add radio buttons into a Button. Group so that // only one button in group is on at any time Button. Group group = new Button. Group(); group. add(small. Button); group. add(medium. Button); group. add(large. Button);
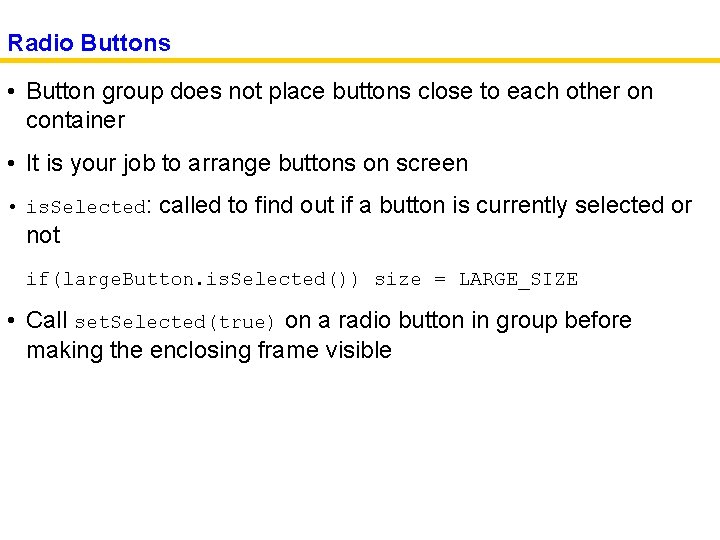
Radio Buttons • Button group does not place buttons close to each other on container • It is your job to arrange buttons on screen • is. Selected: called to find out if a button is currently selected or not if(large. Button. is. Selected()) size = LARGE_SIZE • Call set. Selected(true) on a radio button in group before making the enclosing frame visible
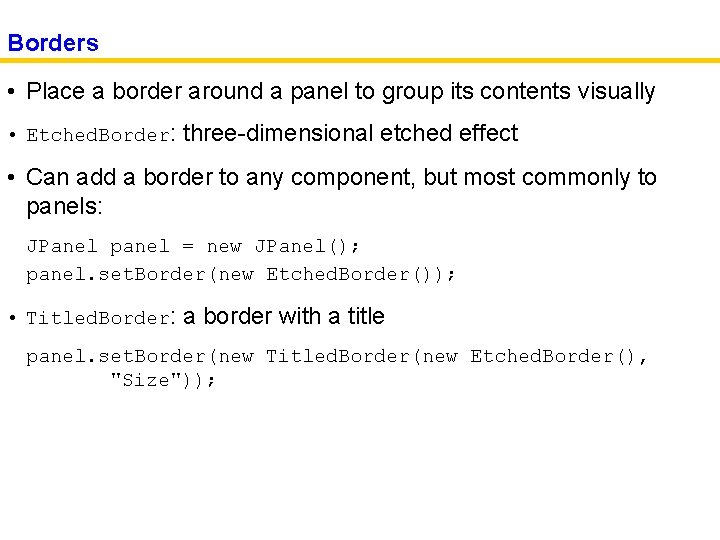
Borders • Place a border around a panel to group its contents visually • Etched. Border: three-dimensional etched effect • Can add a border to any component, but most commonly to panels: JPanel panel = new JPanel(); panel. set. Border(new Etched. Border()); • Titled. Border: a border with a title panel. set. Border(new Titled. Border(new Etched. Border(), "Size"));
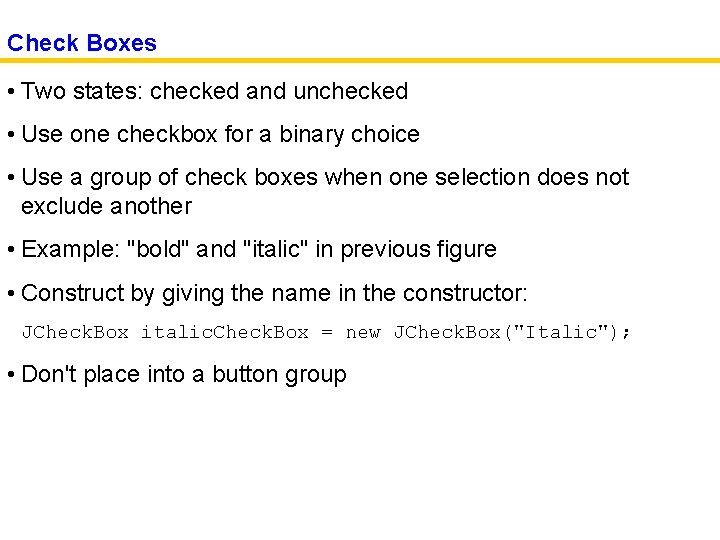
Check Boxes • Two states: checked and unchecked • Use one checkbox for a binary choice • Use a group of check boxes when one selection does not exclude another • Example: "bold" and "italic" in previous figure • Construct by giving the name in the constructor: JCheck. Box italic. Check. Box = new JCheck. Box("Italic"); • Don't place into a button group
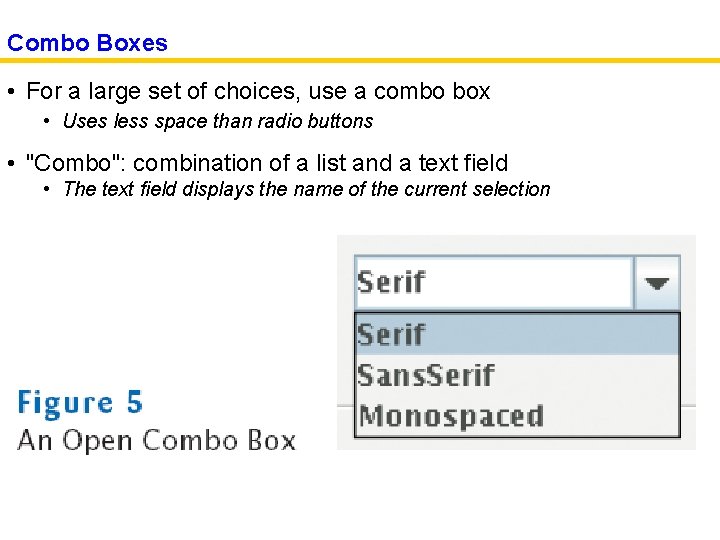
Combo Boxes • For a large set of choices, use a combo box • Uses less space than radio buttons • "Combo": combination of a list and a text field • The text field displays the name of the current selection
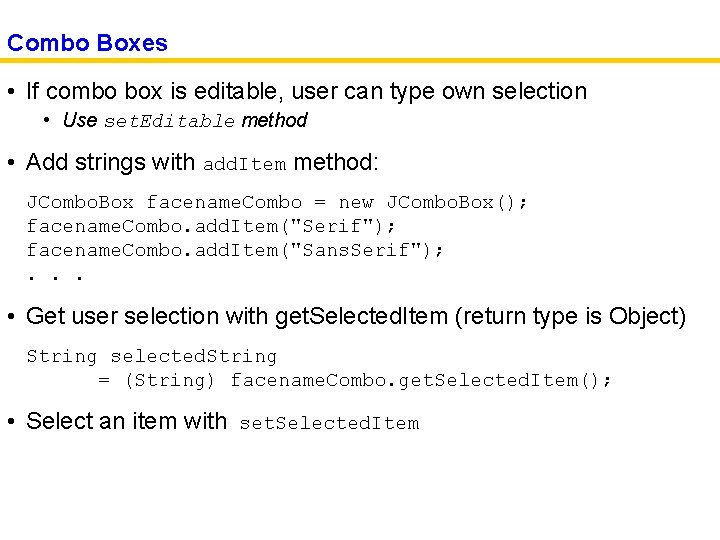
Combo Boxes • If combo box is editable, user can type own selection • Use set. Editable method • Add strings with add. Item method: JCombo. Box facename. Combo = new JCombo. Box(); facename. Combo. add. Item("Serif"); facename. Combo. add. Item("Sans. Serif"); . . . • Get user selection with get. Selected. Item (return type is Object) String selected. String = (String) facename. Combo. get. Selected. Item(); • Select an item with set. Selected. Item
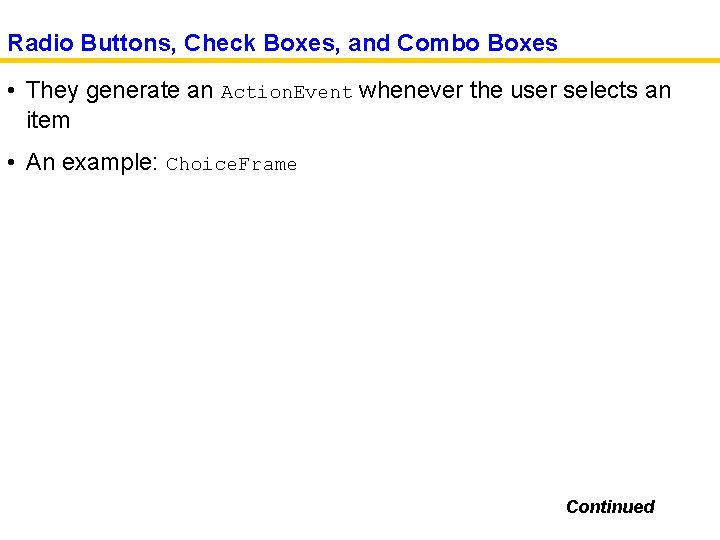
Radio Buttons, Check Boxes, and Combo Boxes • They generate an Action. Event whenever the user selects an item • An example: Choice. Frame Continued
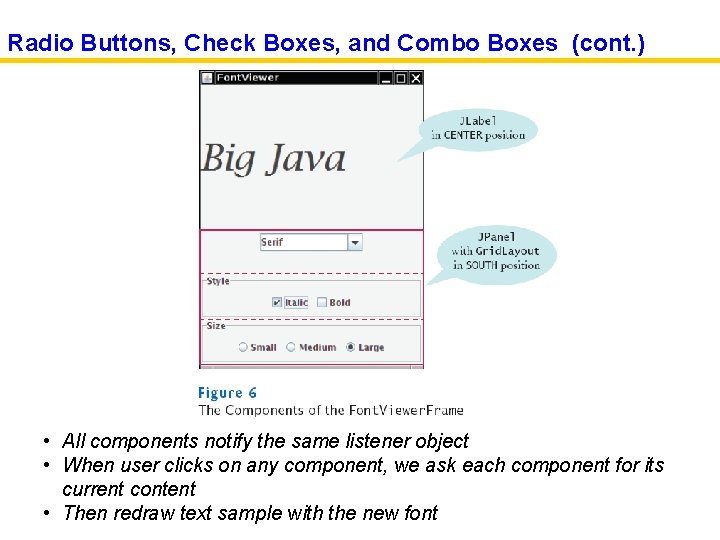
Radio Buttons, Check Boxes, and Combo Boxes (cont. ) • All components notify the same listener object • When user clicks on any component, we ask each component for its current content • Then redraw text sample with the new font
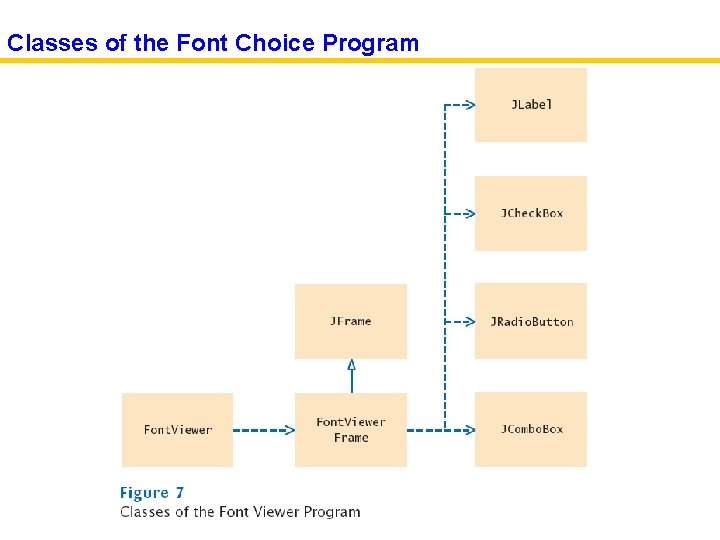
Classes of the Font Choice Program
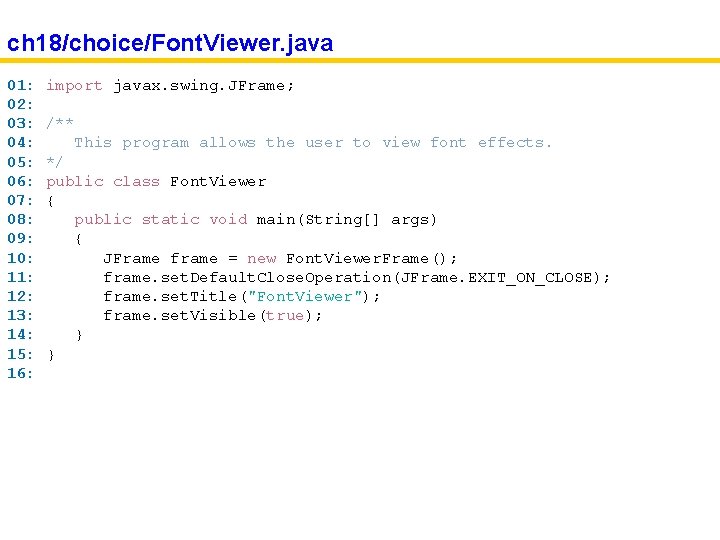
ch 18/choice/Font. Viewer. java 01: 02: 03: 04: 05: 06: 07: 08: 09: 10: 11: 12: 13: 14: 15: 16: import javax. swing. JFrame; /** This program allows the user to view font effects. */ public class Font. Viewer { public static void main(String[] args) { JFrame frame = new Font. Viewer. Frame(); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); frame. set. Title("Font. Viewer"); frame. set. Visible(true); } }
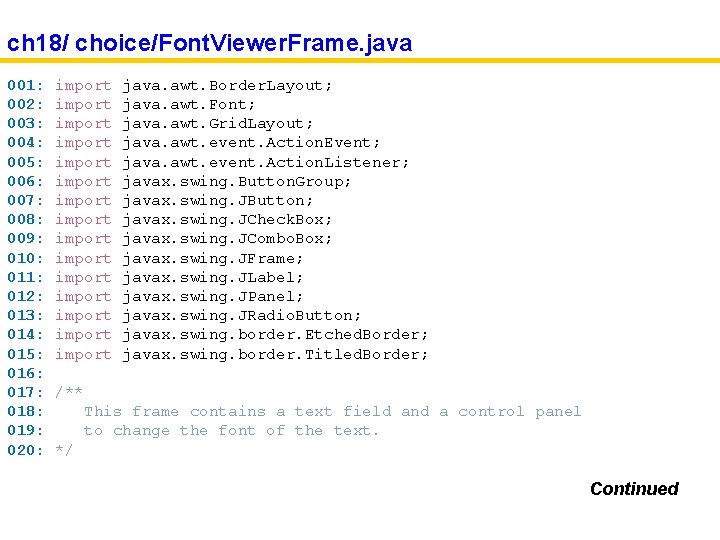
ch 18/ choice/Font. Viewer. Frame. java 001: 002: 003: 004: 005: 006: 007: 008: 009: 010: 011: 012: 013: 014: 015: 016: 017: 018: 019: 020: import import import import java. awt. Border. Layout; java. awt. Font; java. awt. Grid. Layout; java. awt. event. Action. Event; java. awt. event. Action. Listener; javax. swing. Button. Group; javax. swing. JButton; javax. swing. JCheck. Box; javax. swing. JCombo. Box; javax. swing. JFrame; javax. swing. JLabel; javax. swing. JPanel; javax. swing. JRadio. Button; javax. swing. border. Etched. Border; javax. swing. border. Titled. Border; /** This frame contains a text field and a control panel to change the font of the text. */ Continued
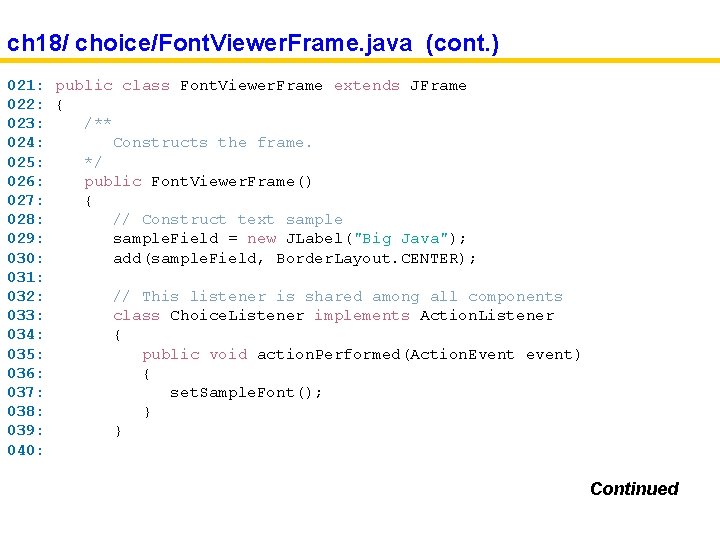
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 021: public class Font. Viewer. Frame extends JFrame 022: { 023: /** 024: Constructs the frame. 025: */ 026: public Font. Viewer. Frame() 027: { 028: // Construct text sample 029: sample. Field = new JLabel("Big Java"); 030: add(sample. Field, Border. Layout. CENTER); 031: 032: // This listener is shared among all components 033: class Choice. Listener implements Action. Listener 034: { 035: public void action. Performed(Action. Event event) 036: { 037: set. Sample. Font(); 038: } 039: } 040: Continued
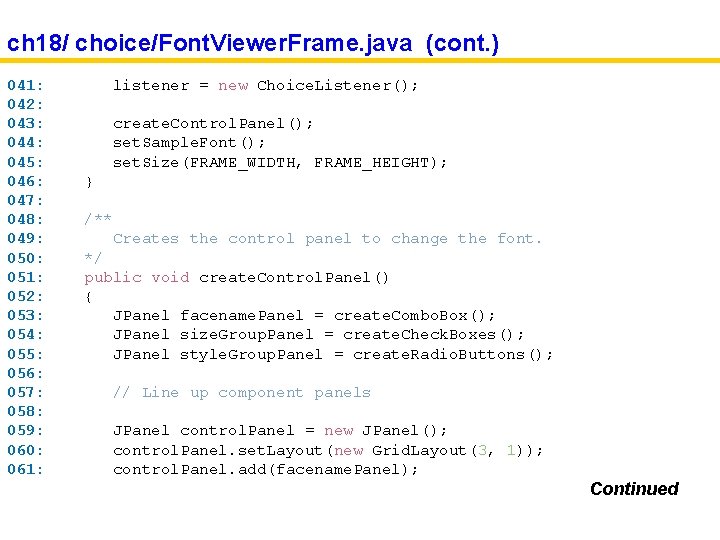
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 041: 042: 043: 044: 045: 046: 047: 048: 049: 050: 051: 052: 053: 054: 055: 056: 057: 058: 059: 060: 061: listener = new Choice. Listener(); create. Control. Panel(); set. Sample. Font(); set. Size(FRAME_WIDTH, FRAME_HEIGHT); } /** Creates the control panel to change the font. */ public void create. Control. Panel() { JPanel facename. Panel = create. Combo. Box(); JPanel size. Group. Panel = create. Check. Boxes(); JPanel style. Group. Panel = create. Radio. Buttons(); // Line up component panels JPanel control. Panel = new JPanel(); control. Panel. set. Layout(new Grid. Layout(3, 1)); control. Panel. add(facename. Panel); Continued
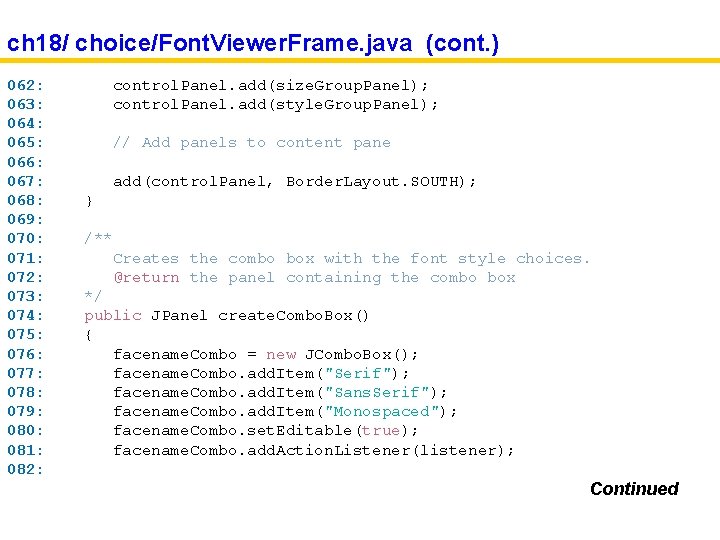
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 062: 063: 064: 065: 066: 067: 068: 069: 070: 071: 072: 073: 074: 075: 076: 077: 078: 079: 080: 081: 082: control. Panel. add(size. Group. Panel); control. Panel. add(style. Group. Panel); // Add panels to content pane add(control. Panel, Border. Layout. SOUTH); } /** Creates the combo box with the font style choices. @return the panel containing the combo box */ public JPanel create. Combo. Box() { facename. Combo = new JCombo. Box(); facename. Combo. add. Item("Serif"); facename. Combo. add. Item("Sans. Serif"); facename. Combo. add. Item("Monospaced"); facename. Combo. set. Editable(true); facename. Combo. add. Action. Listener(listener); Continued
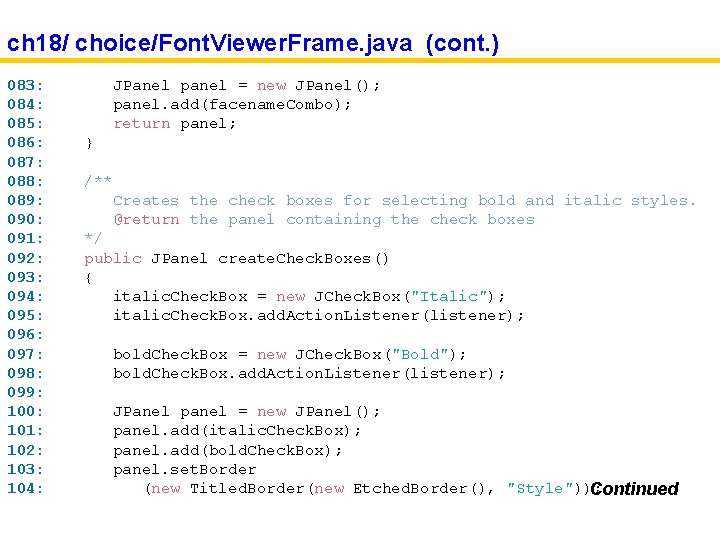
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 083: 084: 085: 086: 087: 088: 089: 090: 091: 092: 093: 094: 095: 096: 097: 098: 099: 100: 101: 102: 103: 104: JPanel panel = new JPanel(); panel. add(facename. Combo); return panel; } /** Creates the check boxes for selecting bold and italic styles. @return the panel containing the check boxes */ public JPanel create. Check. Boxes() { italic. Check. Box = new JCheck. Box("Italic"); italic. Check. Box. add. Action. Listener(listener); bold. Check. Box = new JCheck. Box("Bold"); bold. Check. Box. add. Action. Listener(listener); JPanel panel = new JPanel(); panel. add(italic. Check. Box); panel. add(bold. Check. Box); panel. set. Border (new Titled. Border(new Etched. Border(), "Style")); Continued
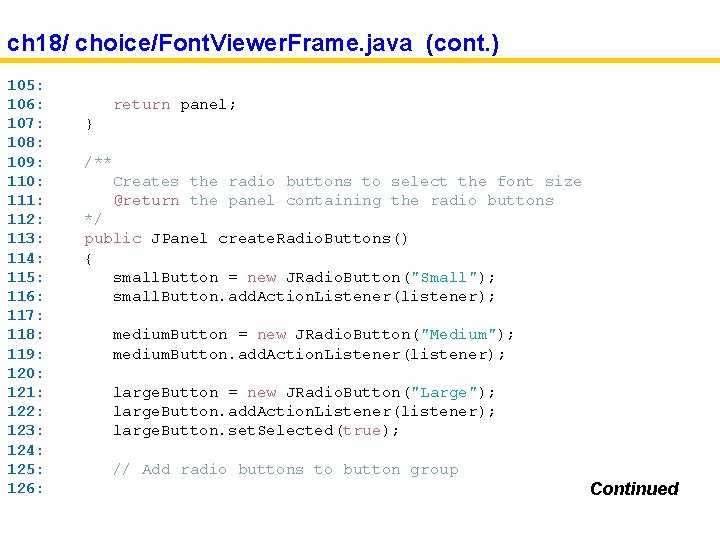
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 105: 106: 107: 108: 109: 110: 111: 112: 113: 114: 115: 116: 117: 118: 119: 120: 121: 122: 123: 124: 125: 126: return panel; } /** Creates the radio buttons to select the font size @return the panel containing the radio buttons */ public JPanel create. Radio. Buttons() { small. Button = new JRadio. Button("Small"); small. Button. add. Action. Listener(listener); medium. Button = new JRadio. Button("Medium"); medium. Button. add. Action. Listener(listener); large. Button = new JRadio. Button("Large"); large. Button. add. Action. Listener(listener); large. Button. set. Selected(true); // Add radio buttons to button group Continued
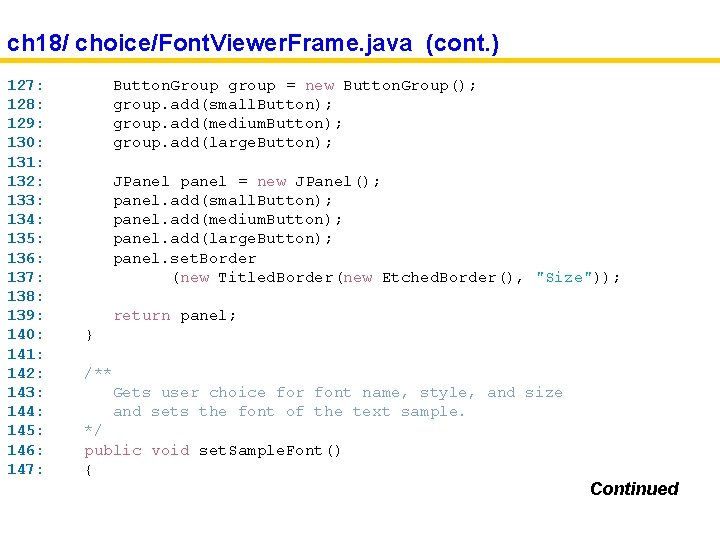
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 127: 128: 129: 130: 131: 132: 133: 134: 135: 136: 137: 138: 139: 140: 141: 142: 143: 144: 145: 146: 147: Button. Group group = new Button. Group(); group. add(small. Button); group. add(medium. Button); group. add(large. Button); JPanel panel = new JPanel(); panel. add(small. Button); panel. add(medium. Button); panel. add(large. Button); panel. set. Border (new Titled. Border(new Etched. Border(), "Size")); return panel; } /** Gets user choice for font name, style, and size and sets the font of the text sample. */ public void set. Sample. Font() { Continued
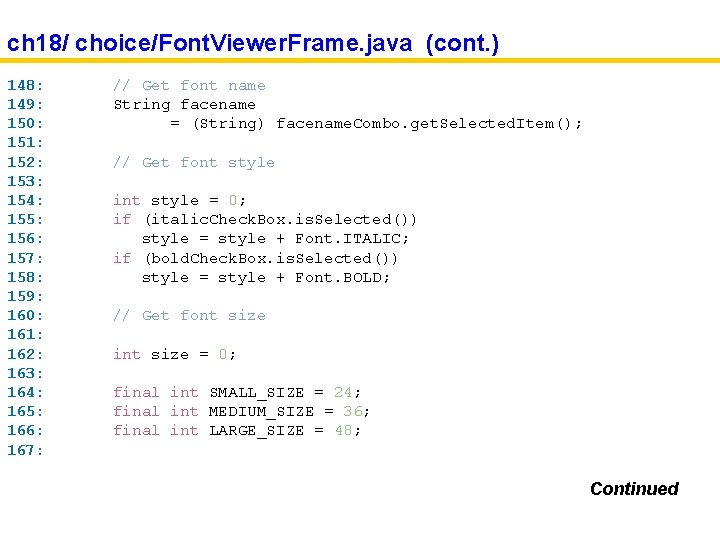
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 148: 149: 150: 151: 152: 153: 154: 155: 156: 157: 158: 159: 160: 161: 162: 163: 164: 165: 166: 167: // Get font name String facename = (String) facename. Combo. get. Selected. Item(); // Get font style int style = 0; if (italic. Check. Box. is. Selected()) style = style + Font. ITALIC; if (bold. Check. Box. is. Selected()) style = style + Font. BOLD; // Get font size int size = 0; final int SMALL_SIZE = 24; final int MEDIUM_SIZE = 36; final int LARGE_SIZE = 48; Continued
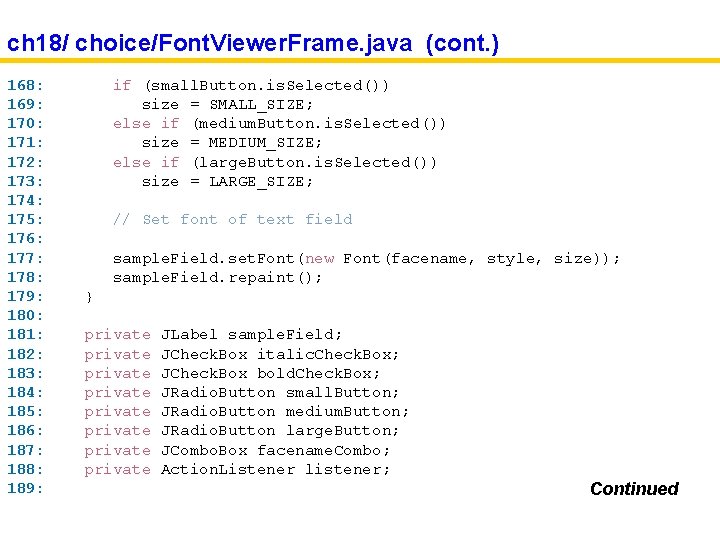
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 168: 169: 170: 171: 172: 173: 174: 175: 176: 177: 178: 179: 180: 181: 182: 183: 184: 185: 186: 187: 188: 189: if (small. Button. is. Selected()) size = SMALL_SIZE; else if (medium. Button. is. Selected()) size = MEDIUM_SIZE; else if (large. Button. is. Selected()) size = LARGE_SIZE; // Set font of text field sample. Field. set. Font(new Font(facename, style, size)); sample. Field. repaint(); } private private JLabel sample. Field; JCheck. Box italic. Check. Box; JCheck. Box bold. Check. Box; JRadio. Button small. Button; JRadio. Button medium. Button; JRadio. Button large. Button; JCombo. Box facename. Combo; Action. Listener listener; Continued
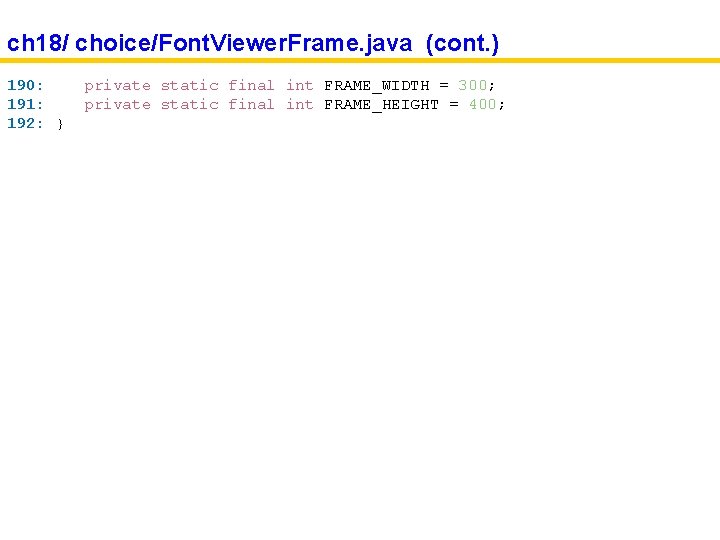
ch 18/ choice/Font. Viewer. Frame. java (cont. ) 190: 191: 192: } private static final int FRAME_WIDTH = 300; private static final int FRAME_HEIGHT = 400;
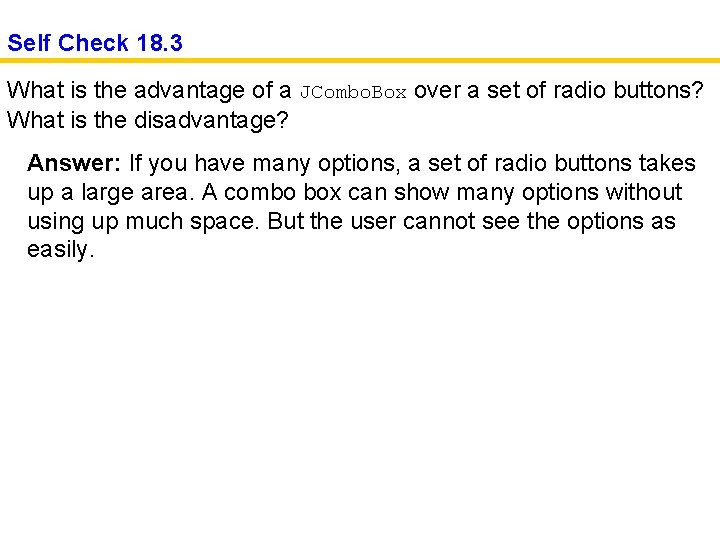
Self Check 18. 3 What is the advantage of a JCombo. Box over a set of radio buttons? What is the disadvantage? Answer: If you have many options, a set of radio buttons takes up a large area. A combo box can show many options without using up much space. But the user cannot see the options as easily.
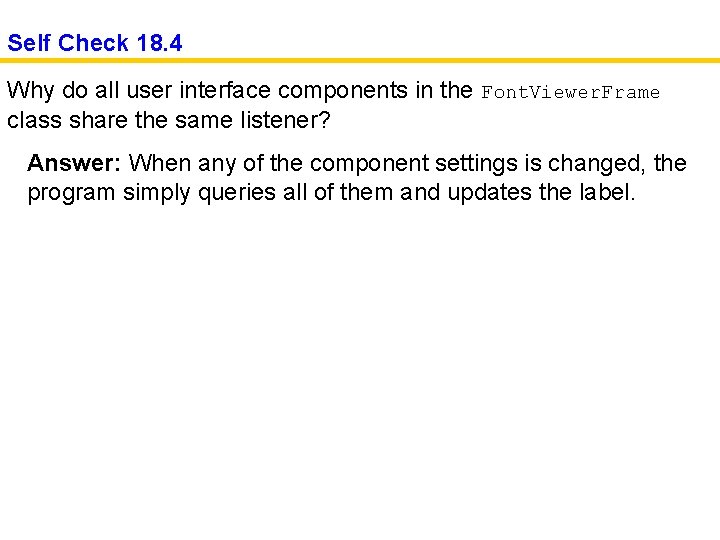
Self Check 18. 4 Why do all user interface components in the Font. Viewer. Frame class share the same listener? Answer: When any of the component settings is changed, the program simply queries all of them and updates the label.
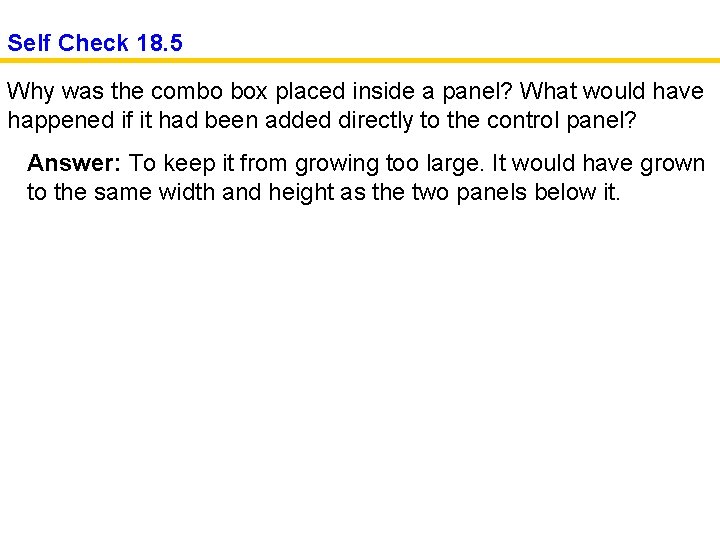
Self Check 18. 5 Why was the combo box placed inside a panel? What would have happened if it had been added directly to the control panel? Answer: To keep it from growing too large. It would have grown to the same width and height as the two panels below it.
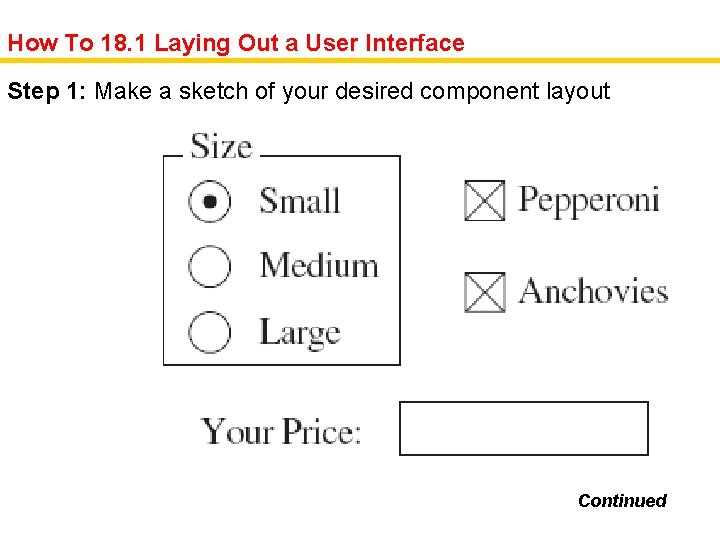
How To 18. 1 Laying Out a User Interface Step 1: Make a sketch of your desired component layout Continued
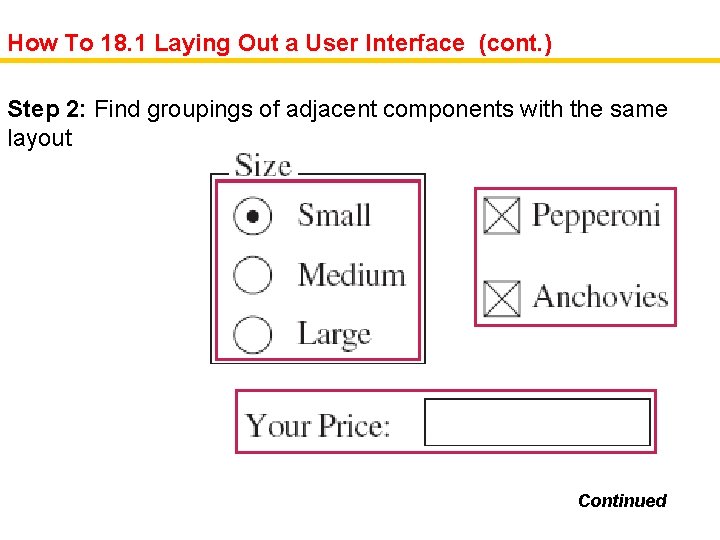
How To 18. 1 Laying Out a User Interface (cont. ) Step 2: Find groupings of adjacent components with the same layout Continued
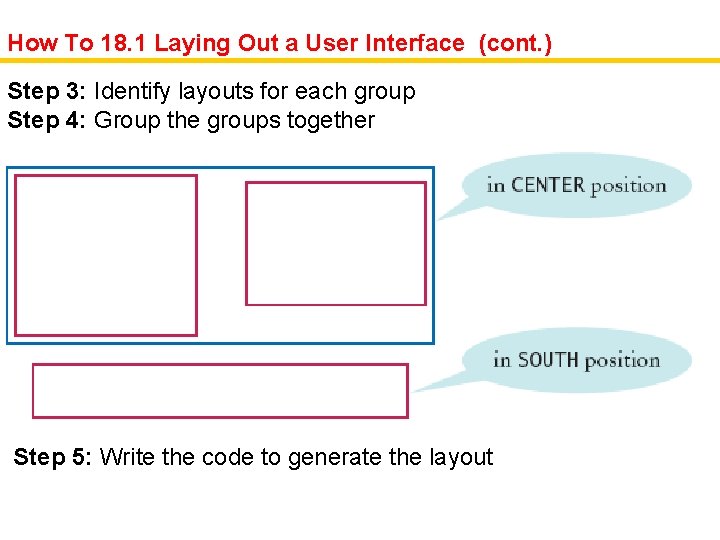
How To 18. 1 Laying Out a User Interface (cont. ) Step 3: Identify layouts for each group Step 4: Group the groups together Step 5: Write the code to generate the layout
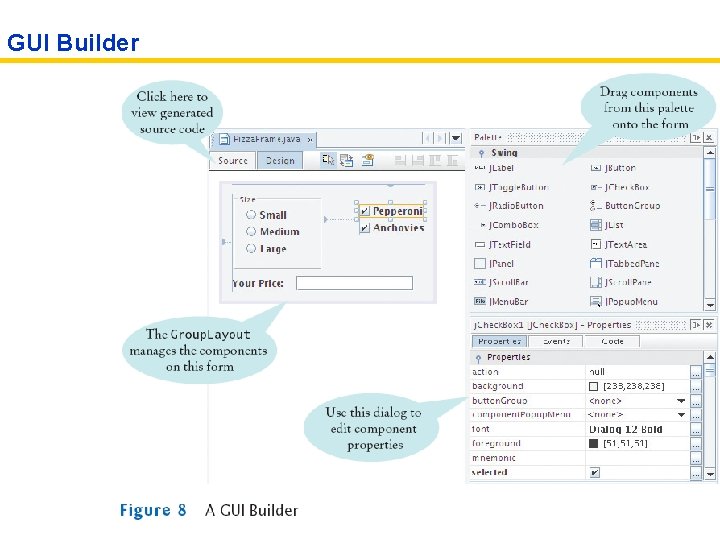
GUI Builder
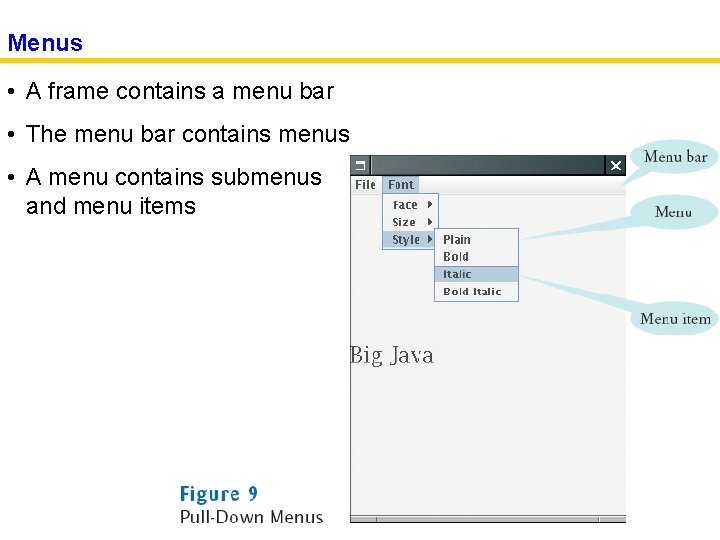
Menus • A frame contains a menu bar • The menu bar contains menus • A menu contains submenus and menu items
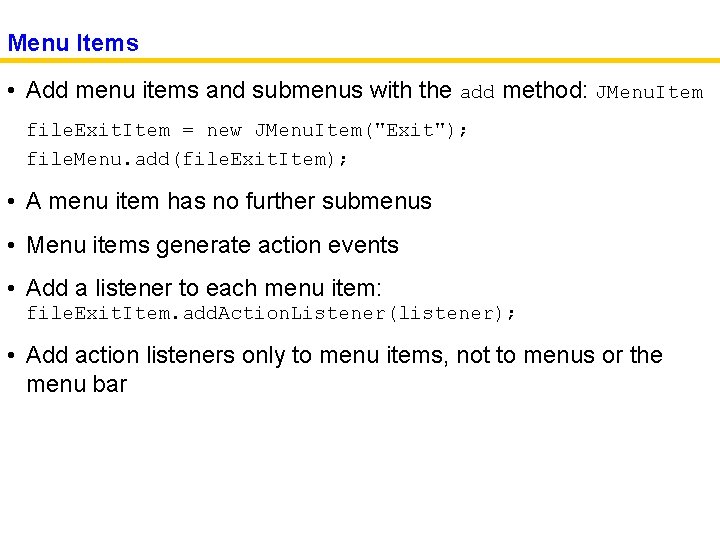
Menu Items • Add menu items and submenus with the add method: JMenu. Item file. Exit. Item = new JMenu. Item("Exit"); file. Menu. add(file. Exit. Item); • A menu item has no further submenus • Menu items generate action events • Add a listener to each menu item: file. Exit. Item. add. Action. Listener(listener); • Add action listeners only to menu items, not to menus or the menu bar
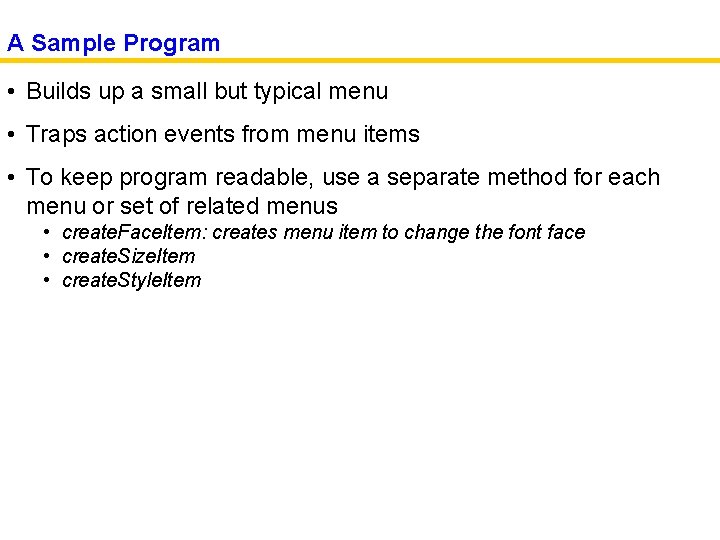
A Sample Program • Builds up a small but typical menu • Traps action events from menu items • To keep program readable, use a separate method for each menu or set of related menus • create. Face. Item: creates menu item to change the font face • create. Size. Item • create. Style. Item
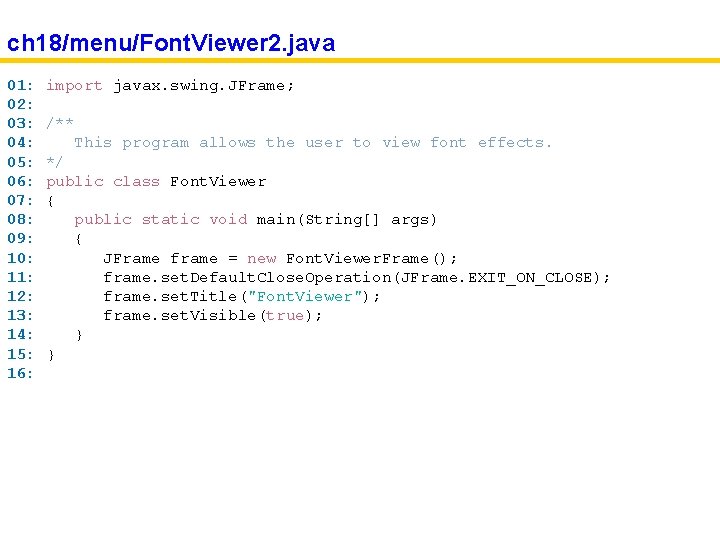
ch 18/menu/Font. Viewer 2. java 01: 02: 03: 04: 05: 06: 07: 08: 09: 10: 11: 12: 13: 14: 15: 16: import javax. swing. JFrame; /** This program allows the user to view font effects. */ public class Font. Viewer { public static void main(String[] args) { JFrame frame = new Font. Viewer. Frame(); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); frame. set. Title("Font. Viewer"); frame. set. Visible(true); } }
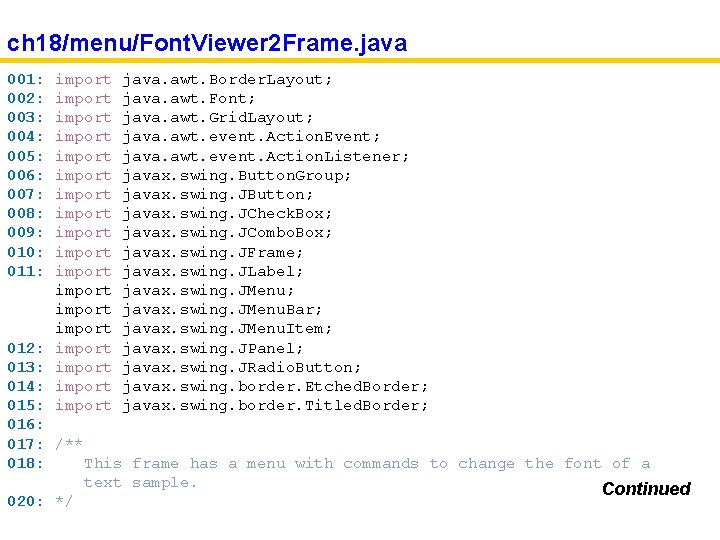
ch 18/menu/Font. Viewer 2 Frame. java 001: 002: 003: 004: 005: 006: 007: 008: 009: 010: 011: import import import import import java. awt. Border. Layout; java. awt. Font; java. awt. Grid. Layout; java. awt. event. Action. Event; java. awt. event. Action. Listener; javax. swing. Button. Group; javax. swing. JButton; javax. swing. JCheck. Box; javax. swing. JCombo. Box; javax. swing. JFrame; javax. swing. JLabel; javax. swing. JMenu. Bar; javax. swing. JMenu. Item; javax. swing. JPanel; javax. swing. JRadio. Button; javax. swing. border. Etched. Border; javax. swing. border. Titled. Border; 012: 013: 014: 015: 016: 017: /** 018: This frame has a menu with commands to change the font of a text sample. Continued 020: */
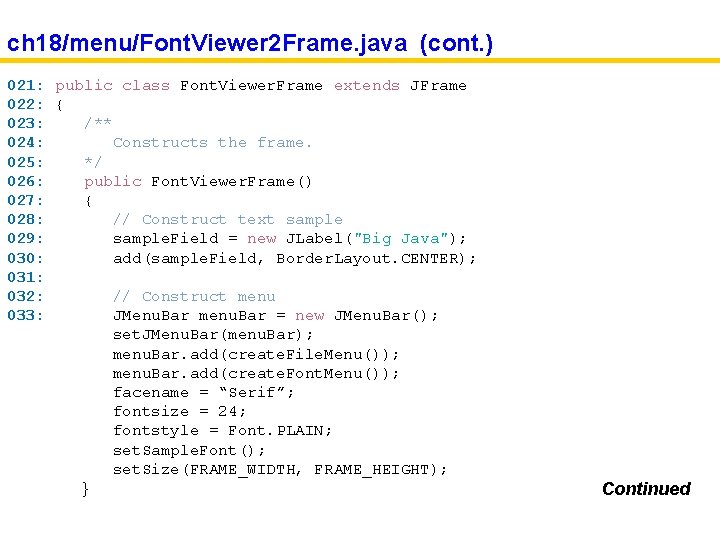
ch 18/menu/Font. Viewer 2 Frame. java (cont. ) 021: public class Font. Viewer. Frame extends JFrame 022: { 023: /** 024: Constructs the frame. 025: */ 026: public Font. Viewer. Frame() 027: { 028: // Construct text sample 029: sample. Field = new JLabel("Big Java"); 030: add(sample. Field, Border. Layout. CENTER); 031: 032: // Construct menu 033: JMenu. Bar menu. Bar = new JMenu. Bar(); set. JMenu. Bar(menu. Bar); menu. Bar. add(create. File. Menu()); menu. Bar. add(create. Font. Menu()); facename = “Serif”; fontsize = 24; fontstyle = Font. PLAIN; set. Sample. Font(); set. Size(FRAME_WIDTH, FRAME_HEIGHT); } Continued
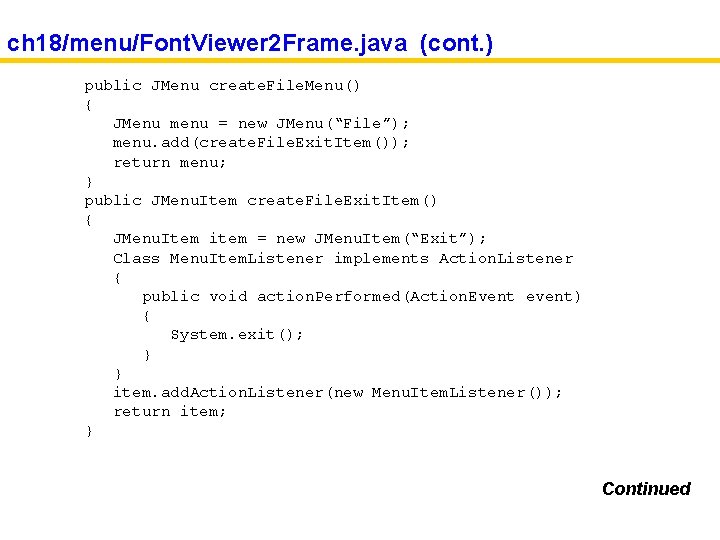
ch 18/menu/Font. Viewer 2 Frame. java (cont. ) public JMenu create. File. Menu() { JMenu menu = new JMenu(“File”); menu. add(create. File. Exit. Item()); return menu; } public JMenu. Item create. File. Exit. Item() { JMenu. Item item = new JMenu. Item(“Exit”); Class Menu. Item. Listener implements Action. Listener { public void action. Performed(Action. Event event) { System. exit(); } } item. add. Action. Listener(new Menu. Item. Listener()); return item; } Continued
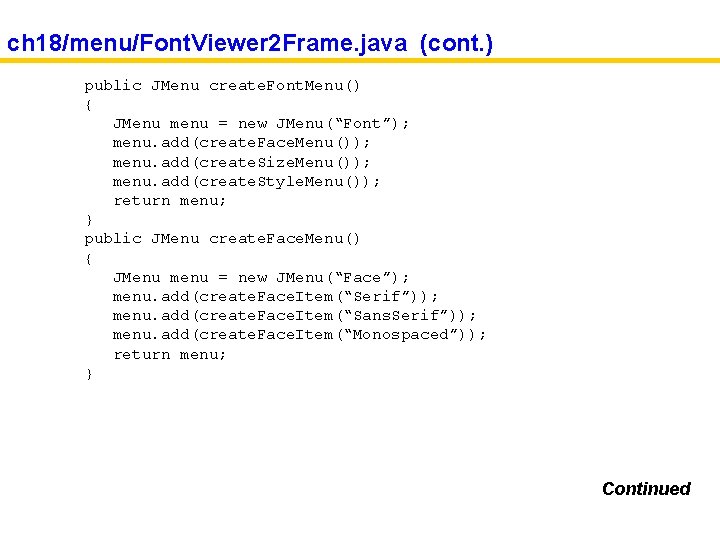
ch 18/menu/Font. Viewer 2 Frame. java (cont. ) public JMenu create. Font. Menu() { JMenu menu = new JMenu(“Font”); menu. add(create. Face. Menu()); menu. add(create. Size. Menu()); menu. add(create. Style. Menu()); return menu; } public JMenu create. Face. Menu() { JMenu menu = new JMenu(“Face”); menu. add(create. Face. Item(“Serif”)); menu. add(create. Face. Item(“Sans. Serif”)); menu. add(create. Face. Item(“Monospaced”)); return menu; } Continued
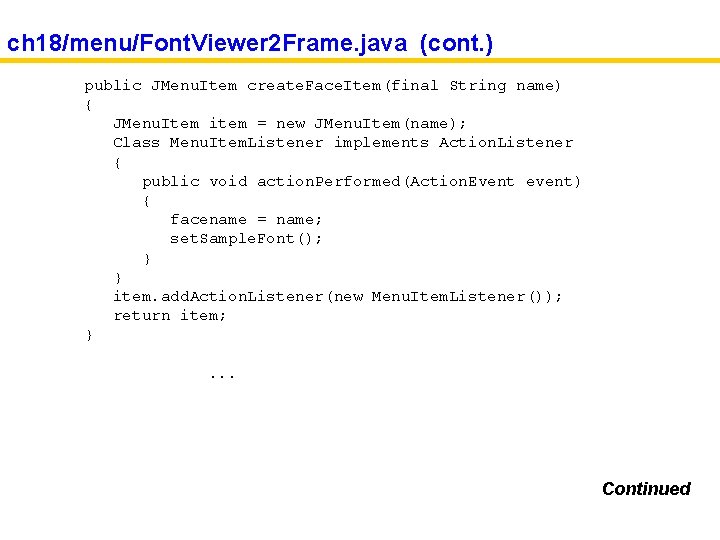
ch 18/menu/Font. Viewer 2 Frame. java (cont. ) public JMenu. Item create. Face. Item(final String name) { JMenu. Item item = new JMenu. Item(name); Class Menu. Item. Listener implements Action. Listener { public void action. Performed(Action. Event event) { facename = name; set. Sample. Font(); } } item. add. Action. Listener(new Menu. Item. Listener()); return item; }. . . Continued
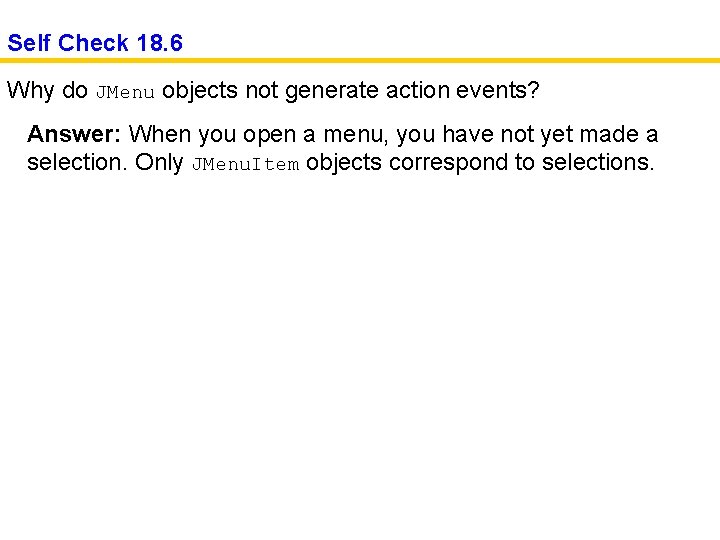
Self Check 18. 6 Why do JMenu objects not generate action events? Answer: When you open a menu, you have not yet made a selection. Only JMenu. Item objects correspond to selections.
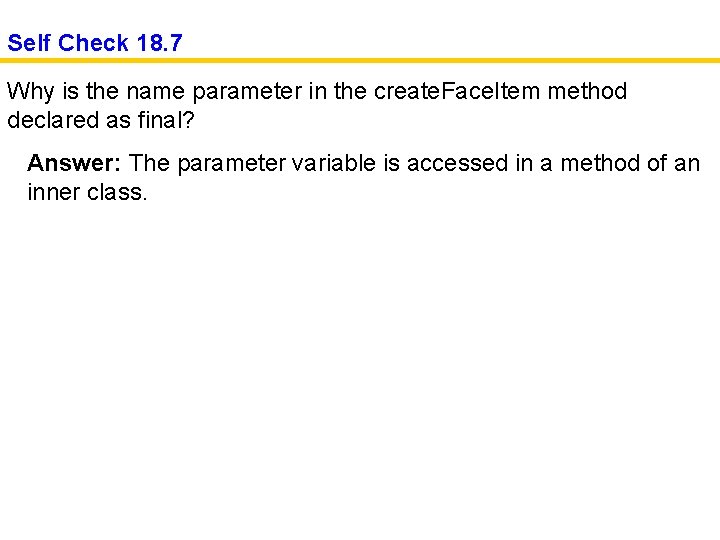
Self Check 18. 7 Why is the name parameter in the create. Face. Item method declared as final? Answer: The parameter variable is accessed in a method of an inner class.
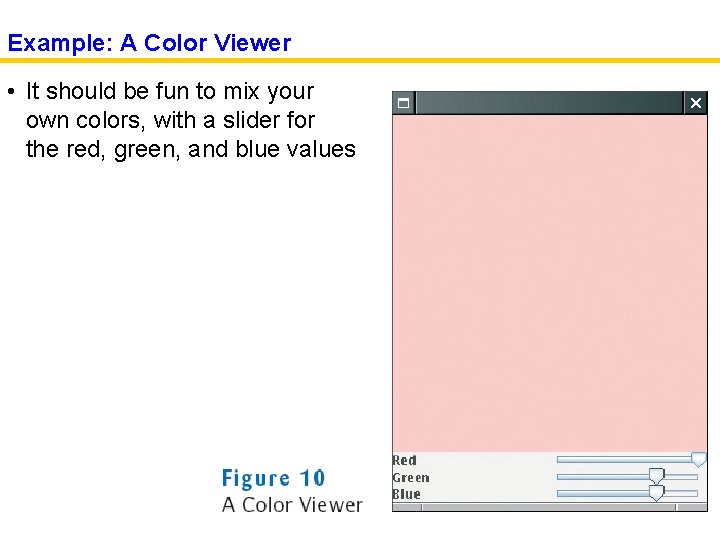
Example: A Color Viewer • It should be fun to mix your own colors, with a slider for the red, green, and blue values
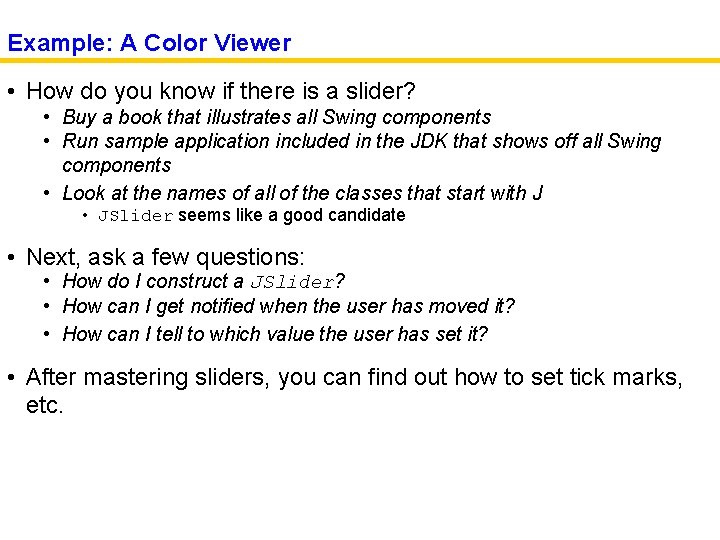
Example: A Color Viewer • How do you know if there is a slider? • Buy a book that illustrates all Swing components • Run sample application included in the JDK that shows off all Swing components • Look at the names of all of the classes that start with J • JSlider seems like a good candidate • Next, ask a few questions: • How do I construct a JSlider? • How can I get notified when the user has moved it? • How can I tell to which value the user has set it? • After mastering sliders, you can find out how to set tick marks, etc.
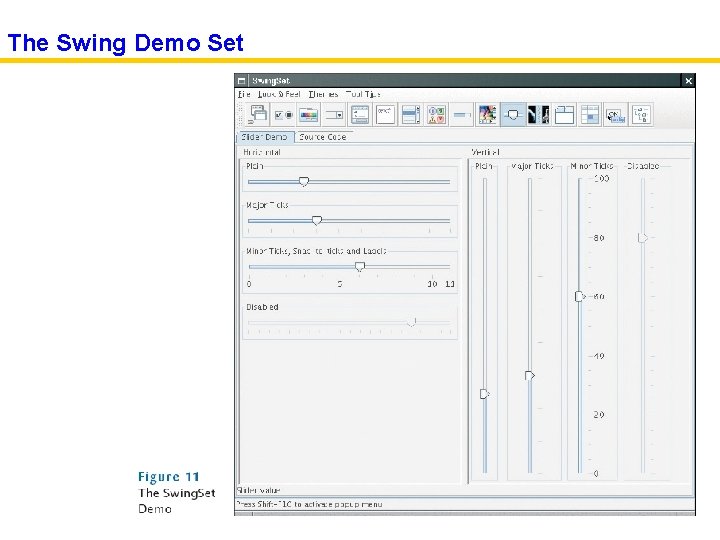
The Swing Demo Set
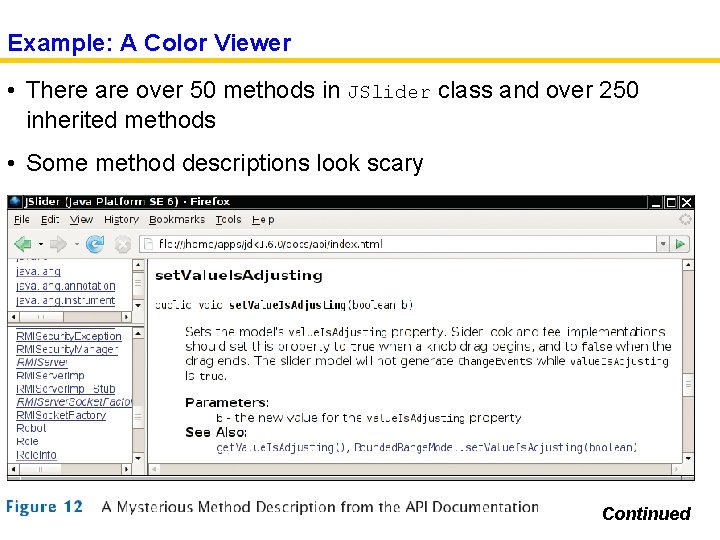
Example: A Color Viewer • There are over 50 methods in JSlider class and over 250 inherited methods • Some method descriptions look scary Continued
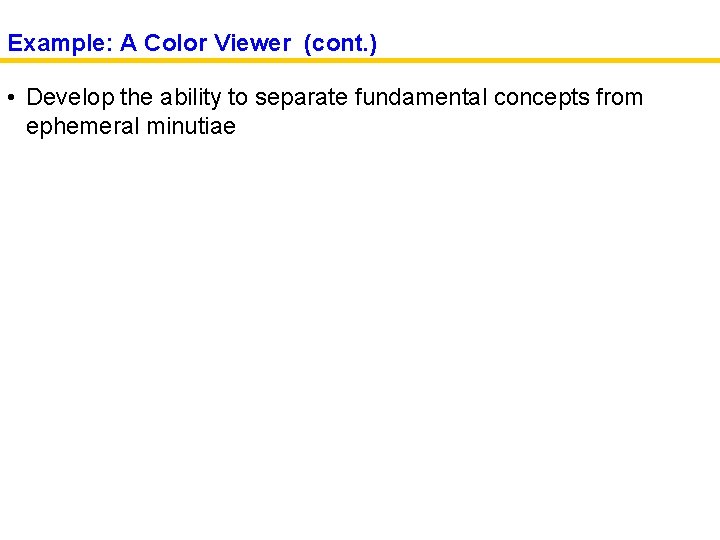
Example: A Color Viewer (cont. ) • Develop the ability to separate fundamental concepts from ephemeral minutiae
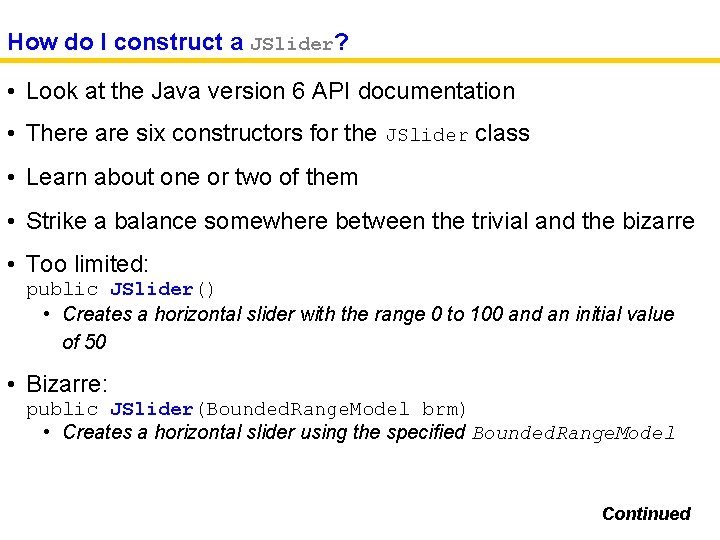
How do I construct a JSlider? • Look at the Java version 6 API documentation • There are six constructors for the JSlider class • Learn about one or two of them • Strike a balance somewhere between the trivial and the bizarre • Too limited: public JSlider() • Creates a horizontal slider with the range 0 to 100 and an initial value of 50 • Bizarre: public JSlider(Bounded. Range. Model brm) • Creates a horizontal slider using the specified Bounded. Range. Model Continued
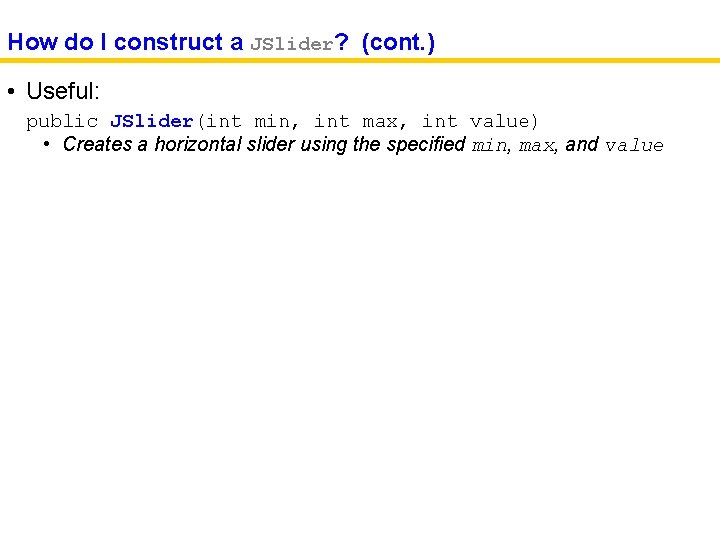
How do I construct a JSlider? (cont. ) • Useful: public JSlider(int min, int max, int value) • Creates a horizontal slider using the specified min, max, and value
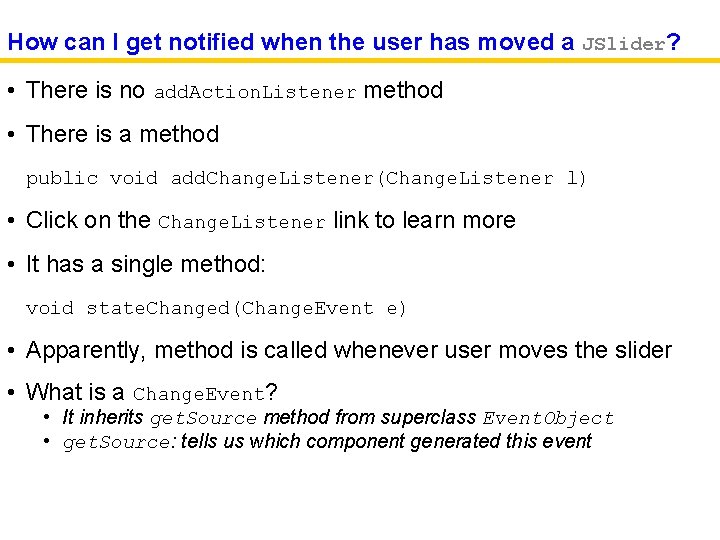
How can I get notified when the user has moved a JSlider? • There is no add. Action. Listener method • There is a method public void add. Change. Listener(Change. Listener l) • Click on the Change. Listener link to learn more • It has a single method: void state. Changed(Change. Event e) • Apparently, method is called whenever user moves the slider • What is a Change. Event? • It inherits get. Source method from superclass Event. Object • get. Source: tells us which component generated this event
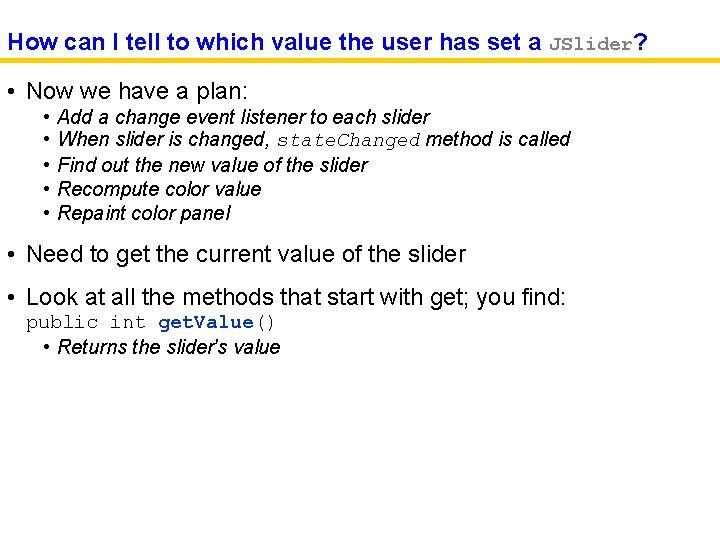
How can I tell to which value the user has set a JSlider? • Now we have a plan: • • • Add a change event listener to each slider When slider is changed, state. Changed method is called Find out the new value of the slider Recompute color value Repaint color panel • Need to get the current value of the slider • Look at all the methods that start with get; you find: public int get. Value() • Returns the slider's value
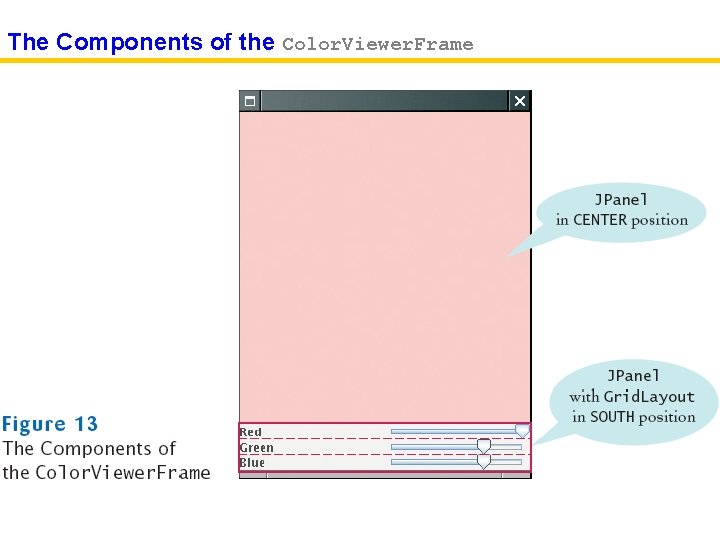
The Components of the Color. Viewer. Frame
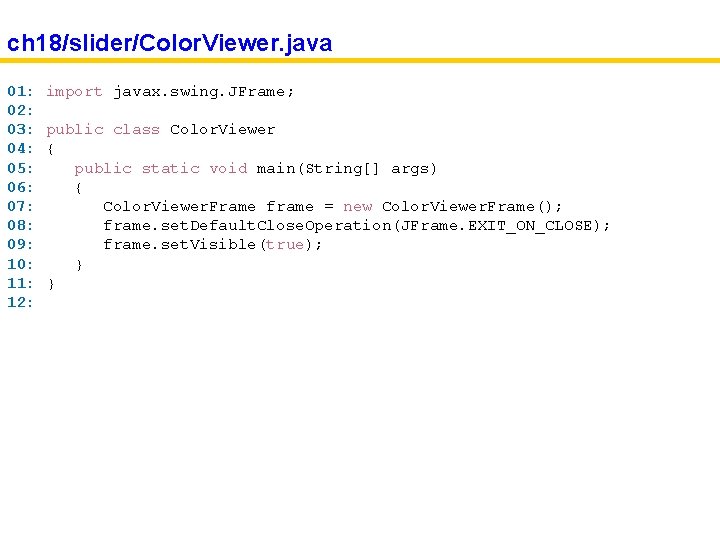
ch 18/slider/Color. Viewer. java 01: 02: 03: 04: 05: 06: 07: 08: 09: 10: 11: 12: import javax. swing. JFrame; public class Color. Viewer { public static void main(String[] args) { Color. Viewer. Frame frame = new Color. Viewer. Frame(); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); frame. set. Visible(true); } }
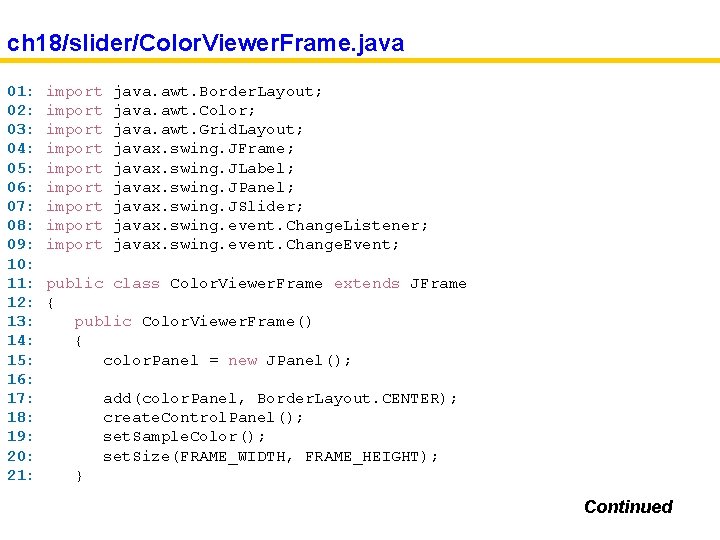
ch 18/slider/Color. Viewer. Frame. java 01: 02: 03: 04: 05: 06: 07: 08: 09: 10: 11: 12: 13: 14: 15: 16: 17: 18: 19: 20: 21: import import import java. awt. Border. Layout; java. awt. Color; java. awt. Grid. Layout; javax. swing. JFrame; javax. swing. JLabel; javax. swing. JPanel; javax. swing. JSlider; javax. swing. event. Change. Listener; javax. swing. event. Change. Event; public class Color. Viewer. Frame extends JFrame { public Color. Viewer. Frame() { color. Panel = new JPanel(); add(color. Panel, Border. Layout. CENTER); create. Control. Panel(); set. Sample. Color(); set. Size(FRAME_WIDTH, FRAME_HEIGHT); } Continued
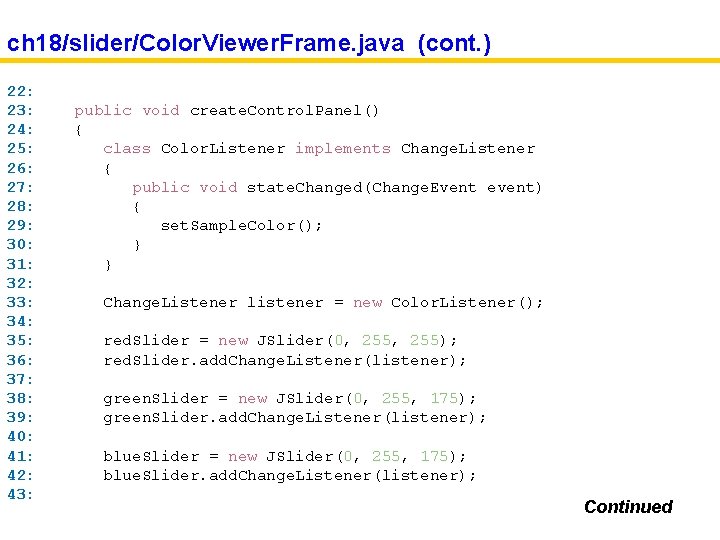
ch 18/slider/Color. Viewer. Frame. java (cont. ) 22: 23: 24: 25: 26: 27: 28: 29: 30: 31: 32: 33: 34: 35: 36: 37: 38: 39: 40: 41: 42: 43: public void create. Control. Panel() { class Color. Listener implements Change. Listener { public void state. Changed(Change. Event event) { set. Sample. Color(); } } Change. Listener listener = new Color. Listener(); red. Slider = new JSlider(0, 255); red. Slider. add. Change. Listener(listener); green. Slider = new JSlider(0, 255, 175); green. Slider. add. Change. Listener(listener); blue. Slider = new JSlider(0, 255, 175); blue. Slider. add. Change. Listener(listener); Continued
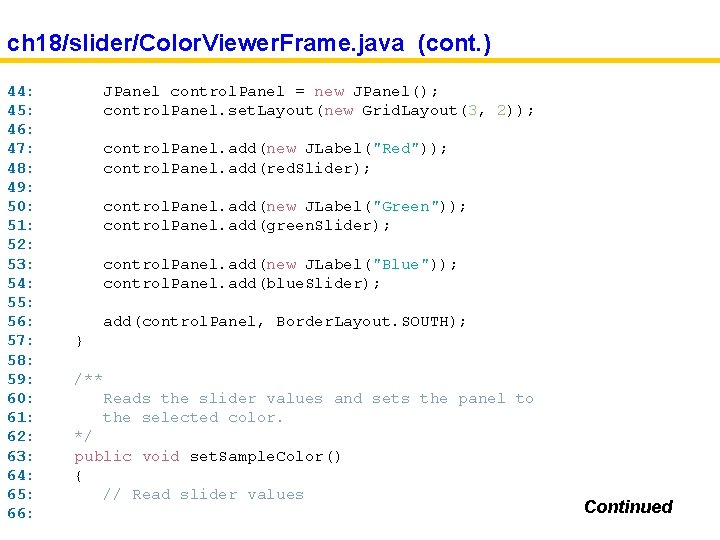
ch 18/slider/Color. Viewer. Frame. java (cont. ) 44: 45: 46: 47: 48: 49: 50: 51: 52: 53: 54: 55: 56: 57: 58: 59: 60: 61: 62: 63: 64: 65: 66: JPanel control. Panel = new JPanel(); control. Panel. set. Layout(new Grid. Layout(3, 2)); control. Panel. add(new JLabel("Red")); control. Panel. add(red. Slider); control. Panel. add(new JLabel("Green")); control. Panel. add(green. Slider); control. Panel. add(new JLabel("Blue")); control. Panel. add(blue. Slider); add(control. Panel, Border. Layout. SOUTH); } /** Reads the slider values and sets the panel to the selected color. */ public void set. Sample. Color() { // Read slider values Continued
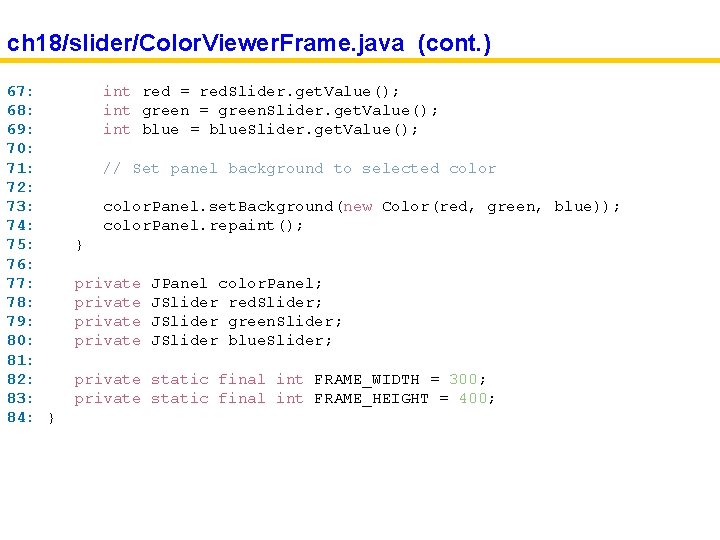
ch 18/slider/Color. Viewer. Frame. java (cont. ) 67: 68: 69: 70: 71: 72: 73: 74: 75: 76: 77: 78: 79: 80: 81: 82: 83: 84: } int red = red. Slider. get. Value(); int green = green. Slider. get. Value(); int blue = blue. Slider. get. Value(); // Set panel background to selected color. Panel. set. Background(new Color(red, green, blue)); color. Panel. repaint(); } private JPanel color. Panel; JSlider red. Slider; JSlider green. Slider; JSlider blue. Slider; private static final int FRAME_WIDTH = 300; private static final int FRAME_HEIGHT = 400;
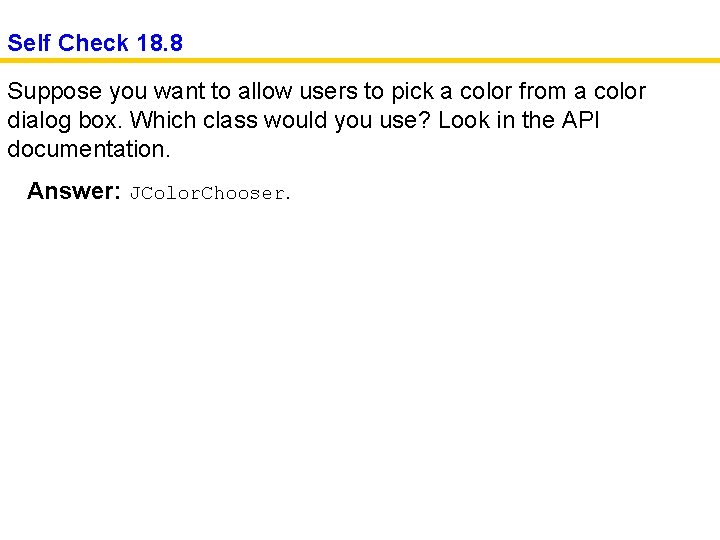
Self Check 18. 8 Suppose you want to allow users to pick a color from a color dialog box. Which class would you use? Look in the API documentation. Answer: JColor. Chooser.
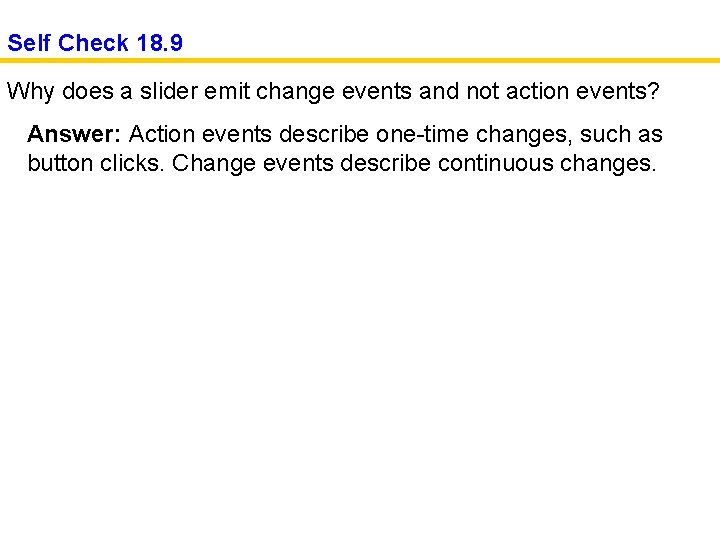
Self Check 18. 9 Why does a slider emit change events and not action events? Answer: Action events describe one-time changes, such as button clicks. Change events describe continuous changes.