G 64 ADS Advanced Data Structures Guoping Qiu
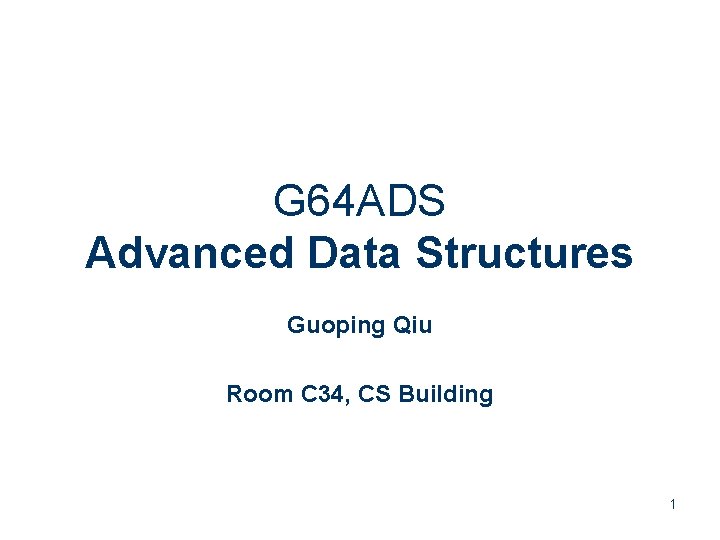
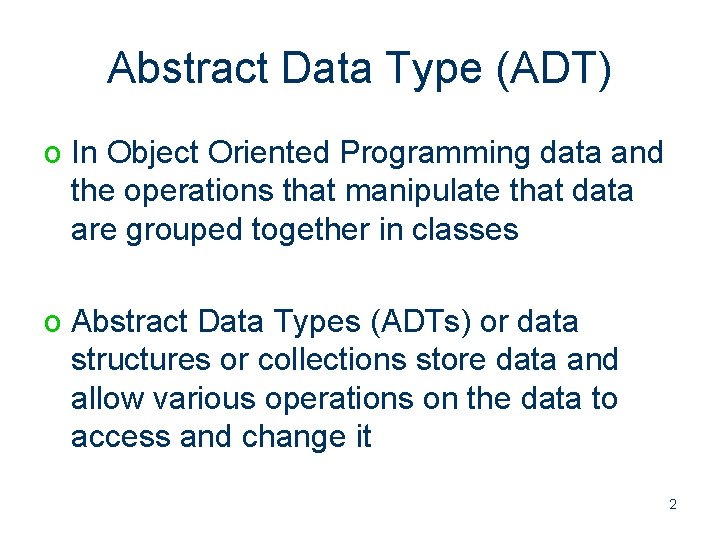
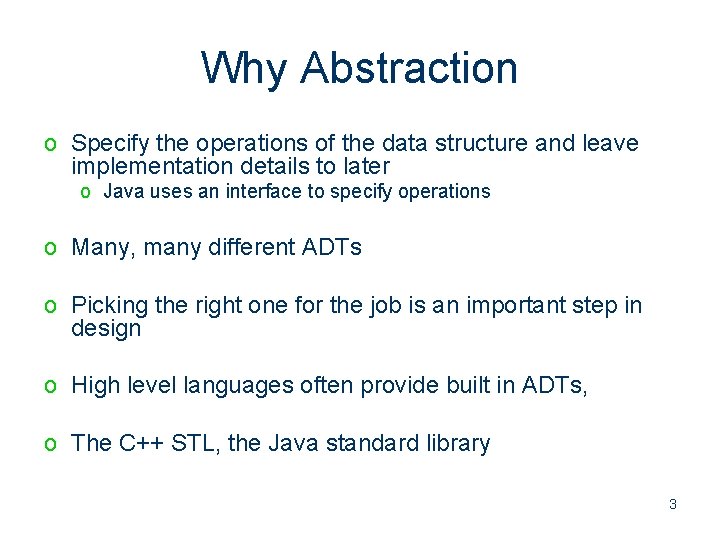
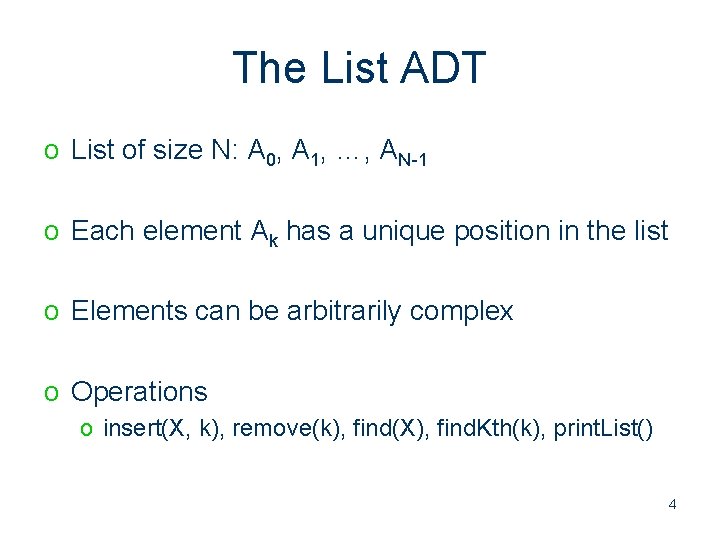
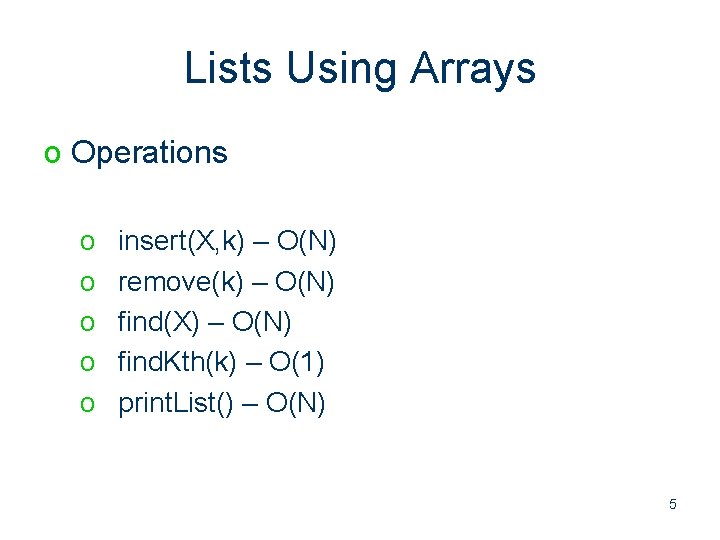
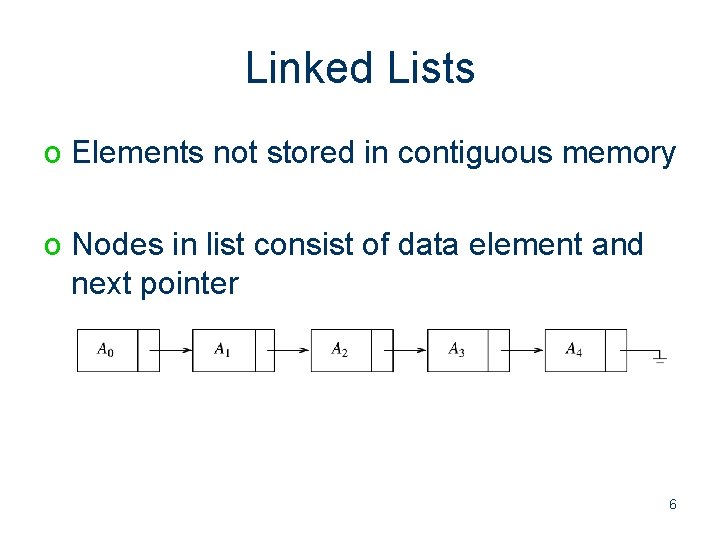
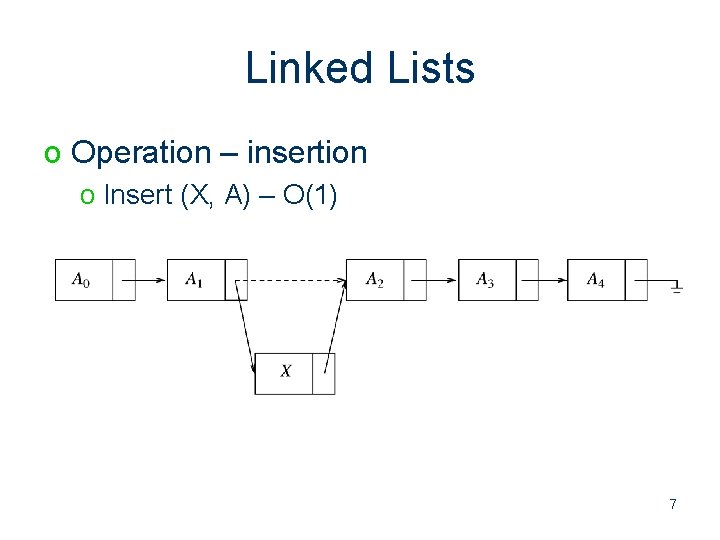
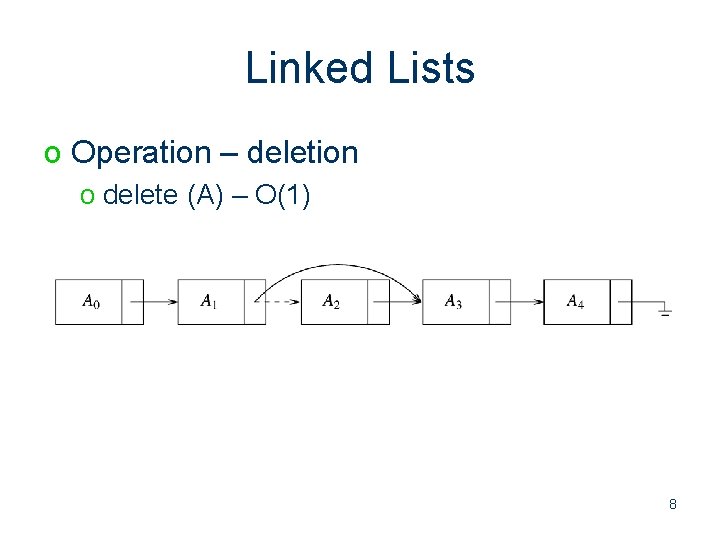
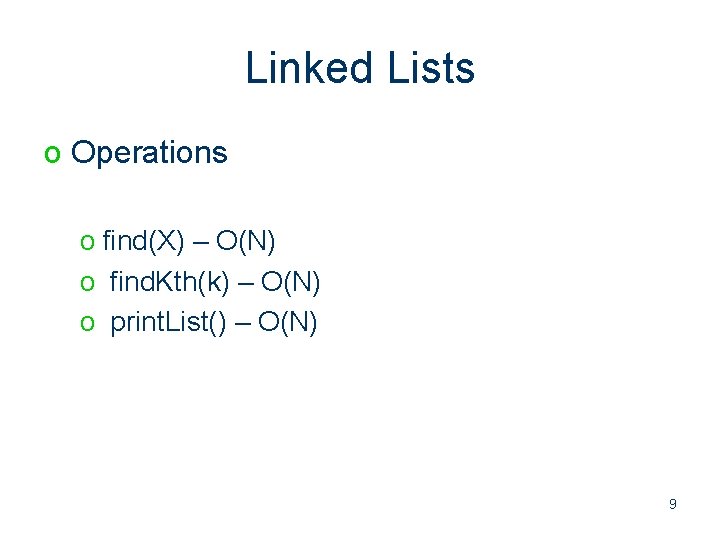
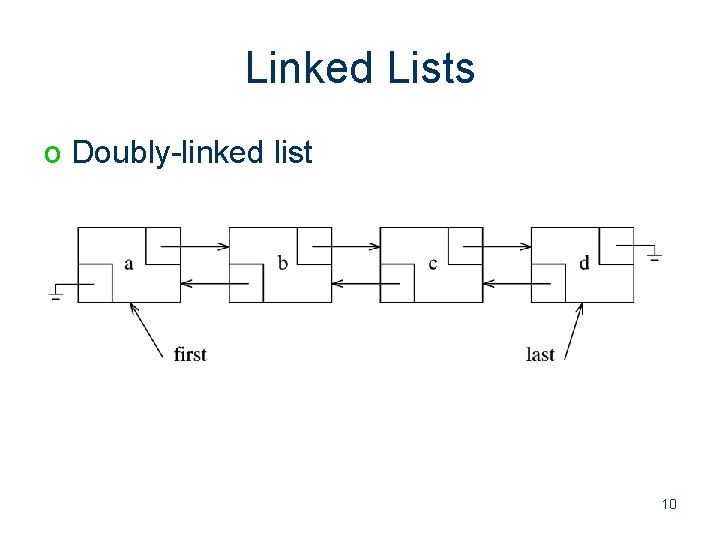
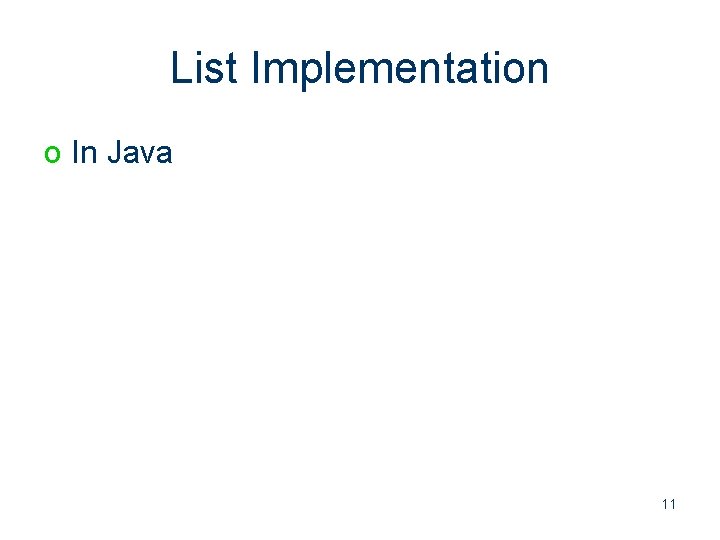
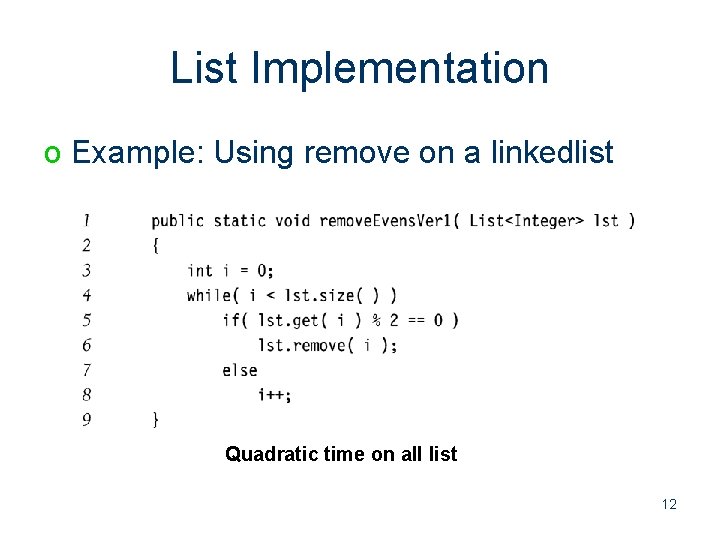
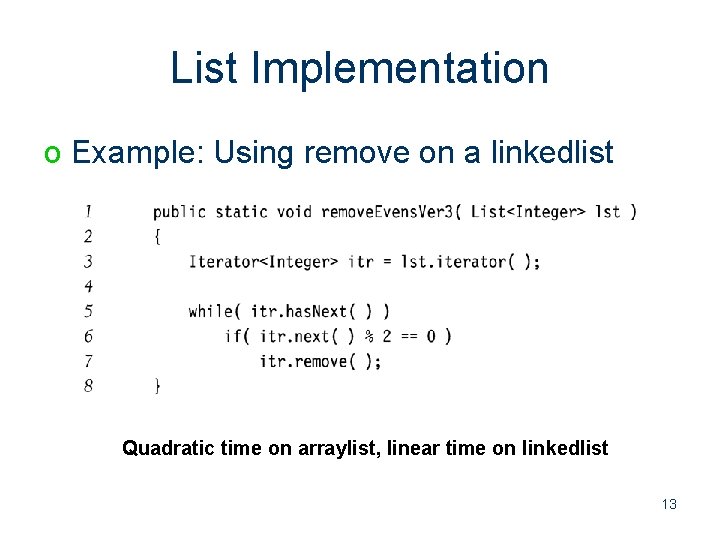
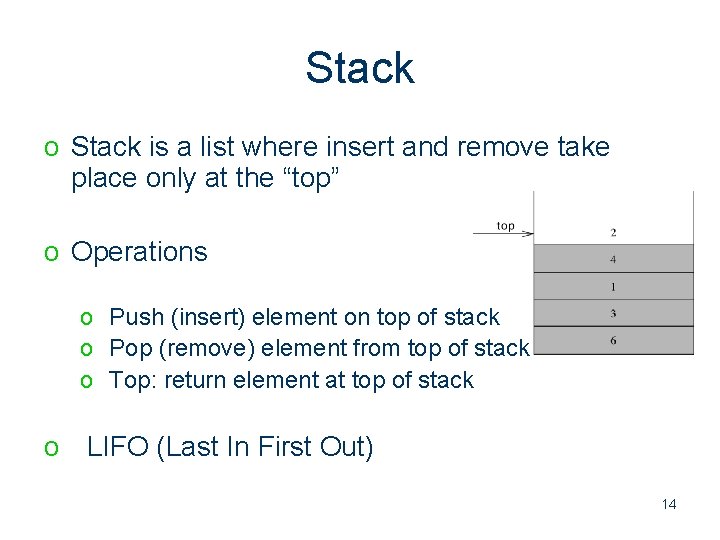
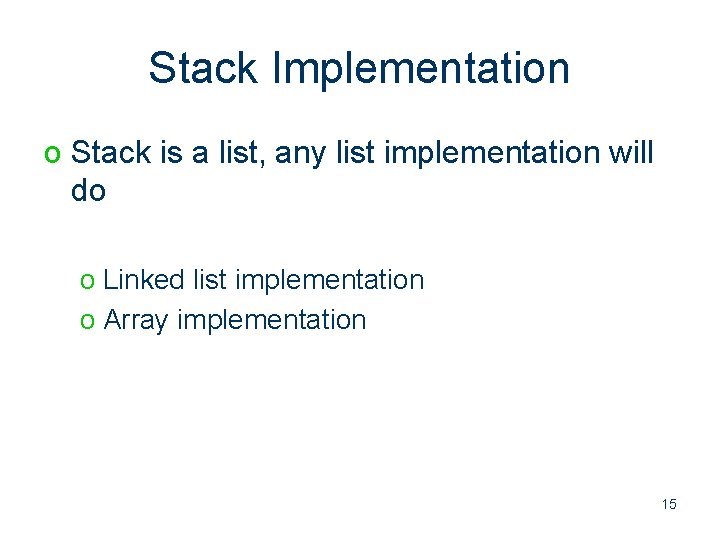
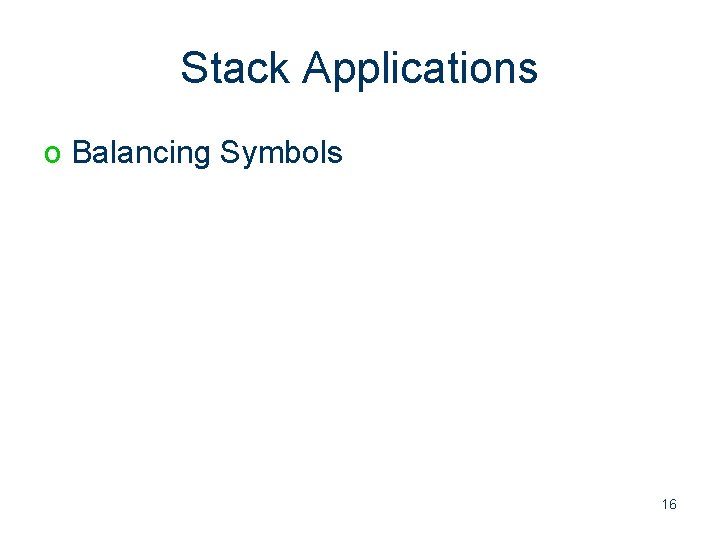
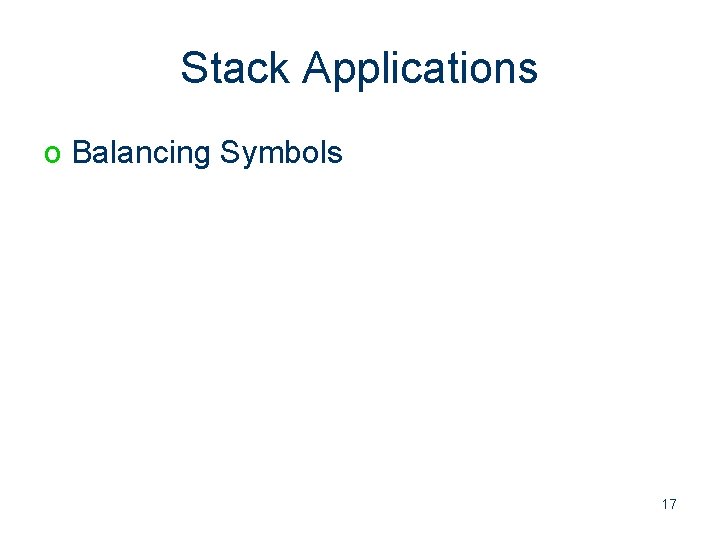
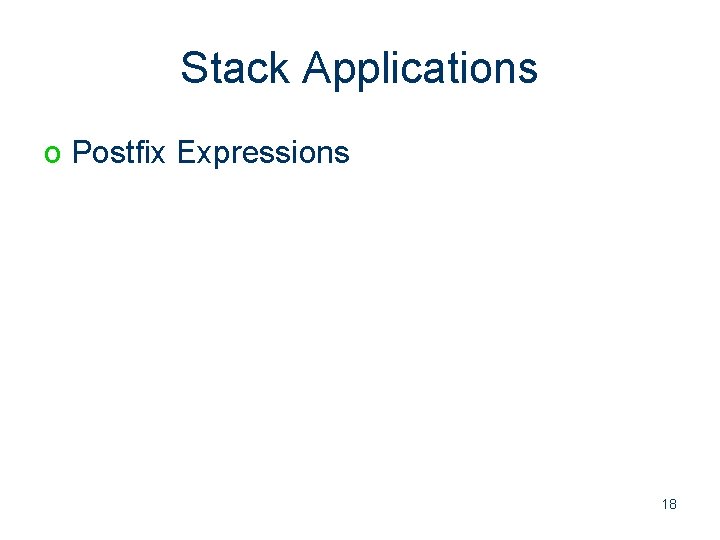
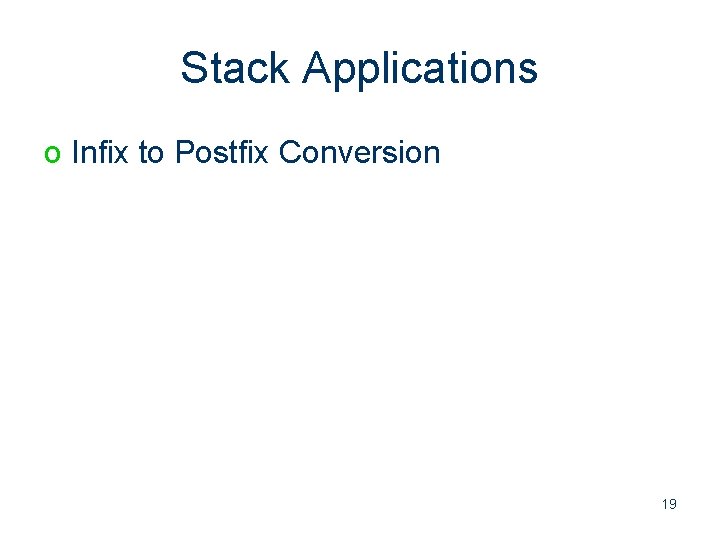
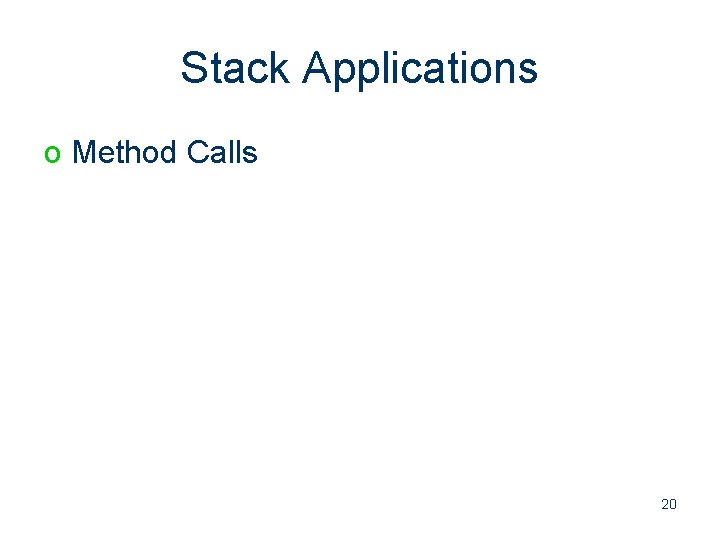
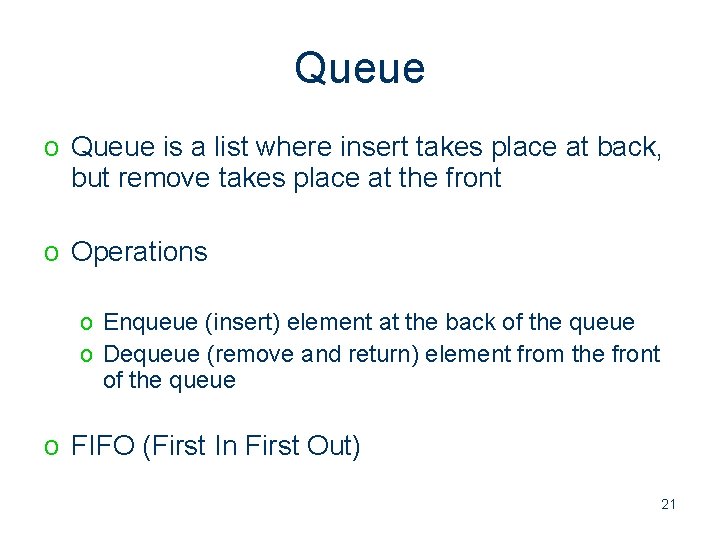
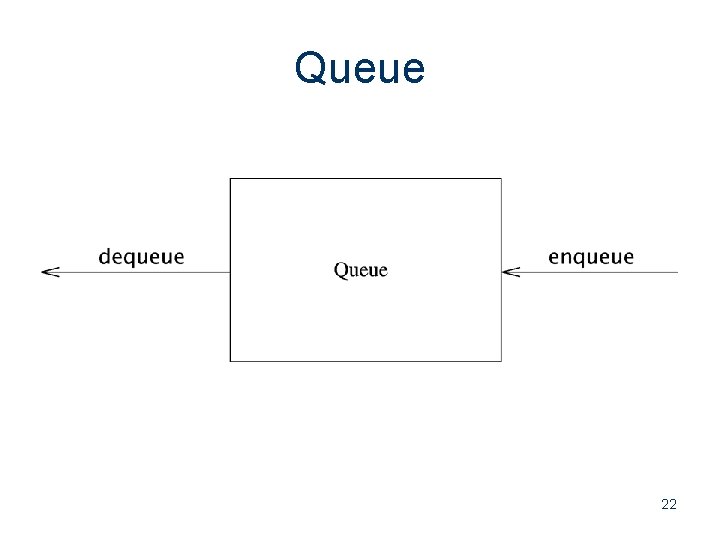
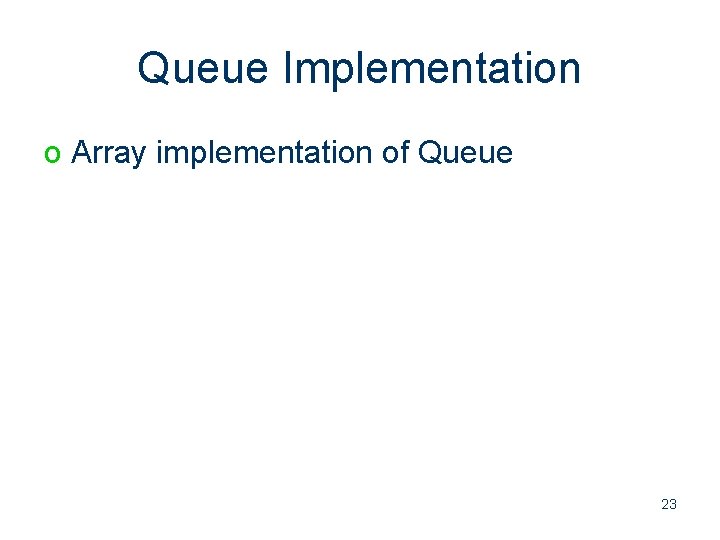
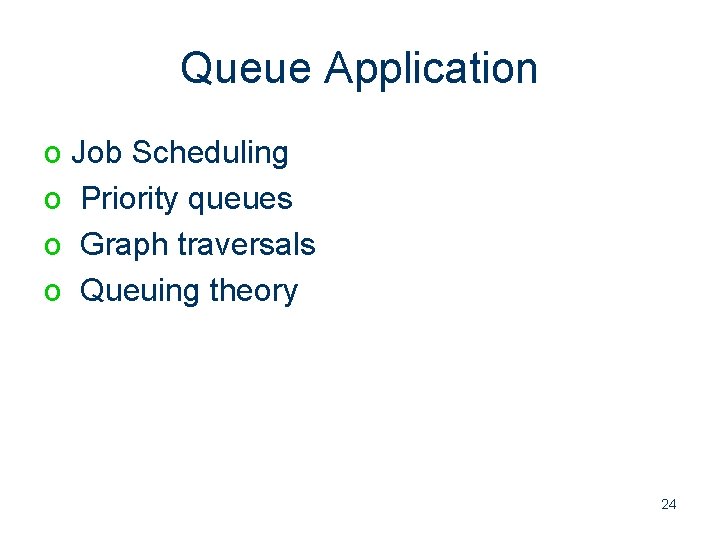
- Slides: 24
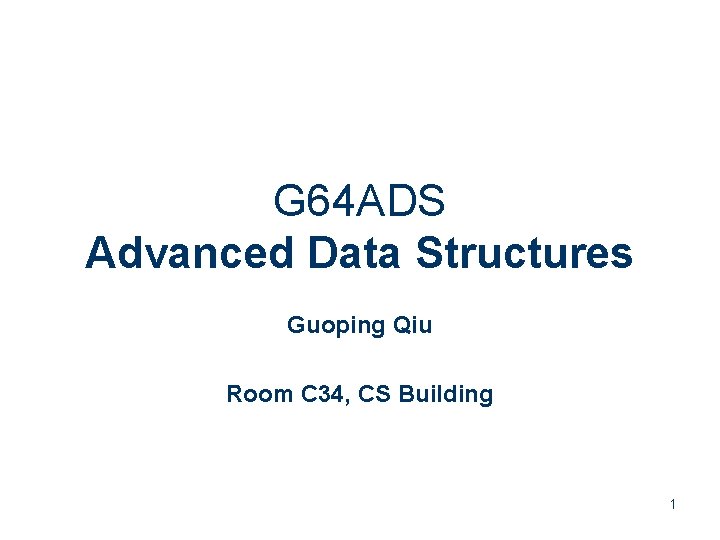
G 64 ADS Advanced Data Structures Guoping Qiu Room C 34, CS Building 1
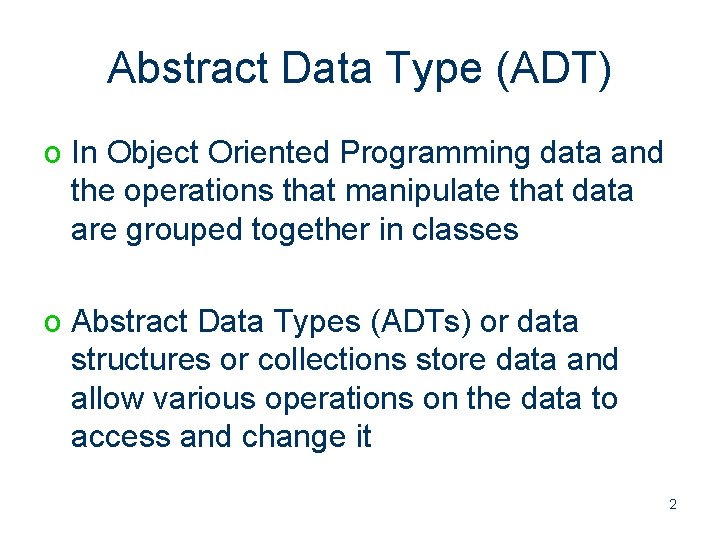
Abstract Data Type (ADT) o In Object Oriented Programming data and the operations that manipulate that data are grouped together in classes o Abstract Data Types (ADTs) or data structures or collections store data and allow various operations on the data to access and change it 2
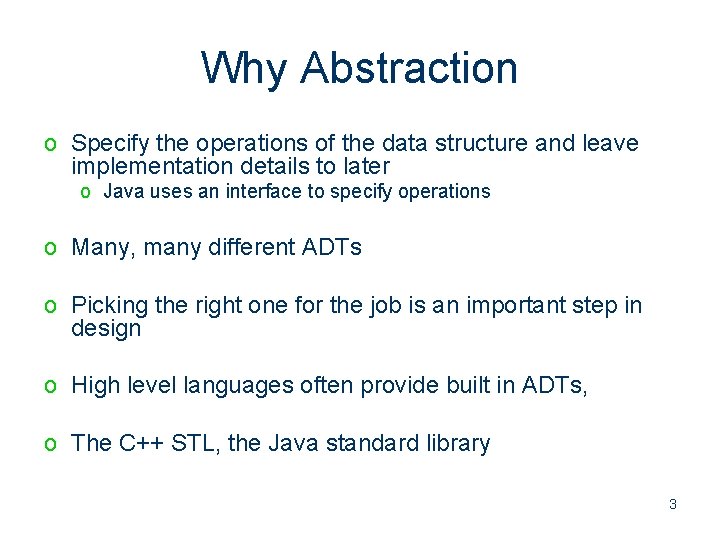
Why Abstraction o Specify the operations of the data structure and leave implementation details to later o Java uses an interface to specify operations o Many, many different ADTs o Picking the right one for the job is an important step in design o High level languages often provide built in ADTs, o The C++ STL, the Java standard library 3
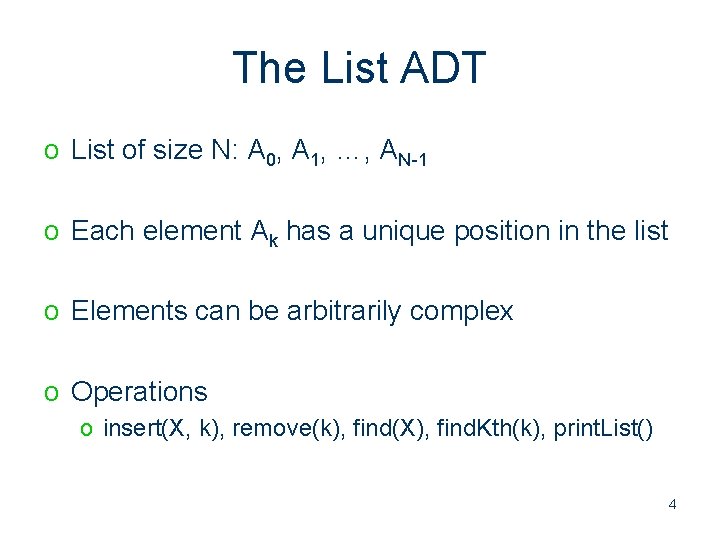
The List ADT o List of size N: A 0, A 1, …, AN-1 o Each element Ak has a unique position in the list o Elements can be arbitrarily complex o Operations o insert(X, k), remove(k), find(X), find. Kth(k), print. List() 4
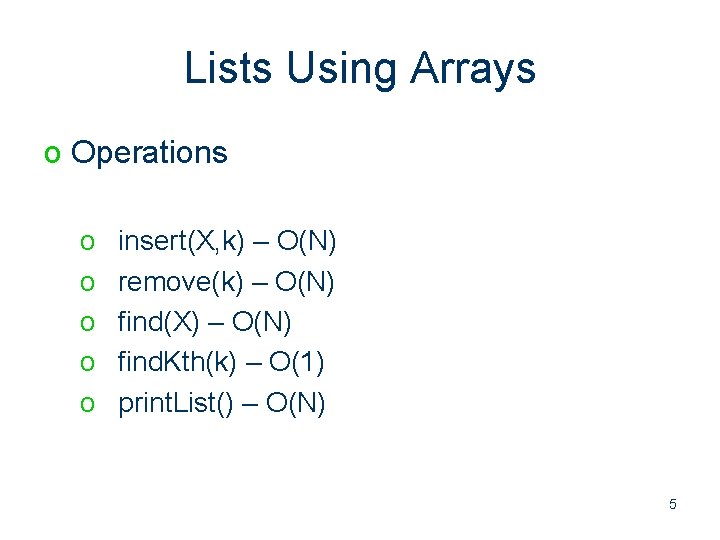
Lists Using Arrays o Operations o o o insert(X, k) – O(N) remove(k) – O(N) find(X) – O(N) find. Kth(k) – O(1) print. List() – O(N) 5
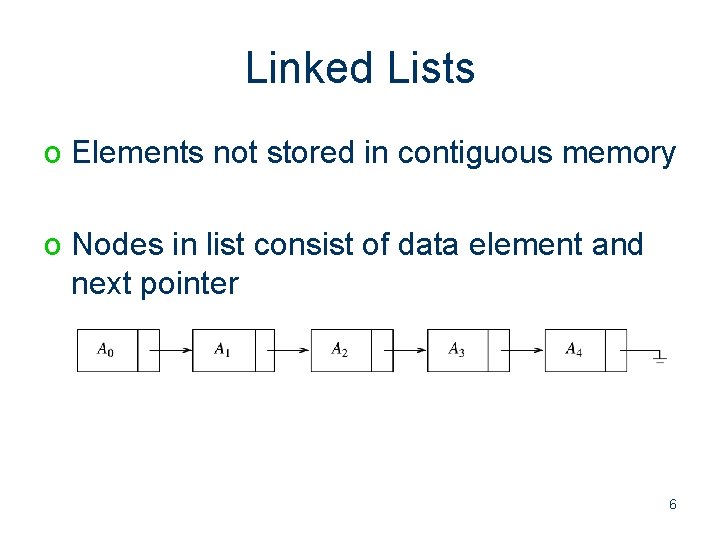
Linked Lists o Elements not stored in contiguous memory o Nodes in list consist of data element and next pointer 6
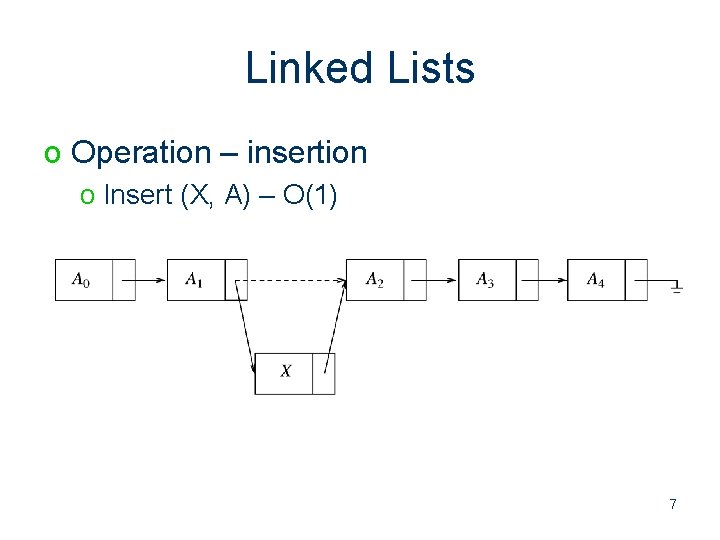
Linked Lists o Operation – insertion o Insert (X, A) – O(1) 7
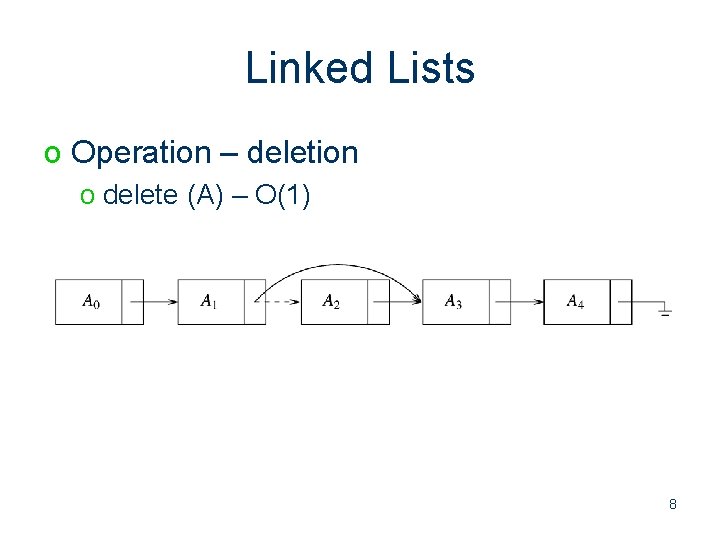
Linked Lists o Operation – deletion o delete (A) – O(1) 8
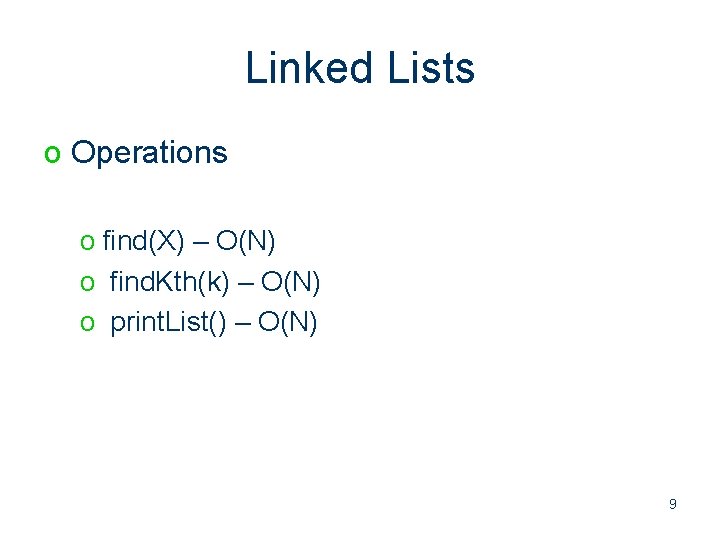
Linked Lists o Operations o find(X) – O(N) o find. Kth(k) – O(N) o print. List() – O(N) 9
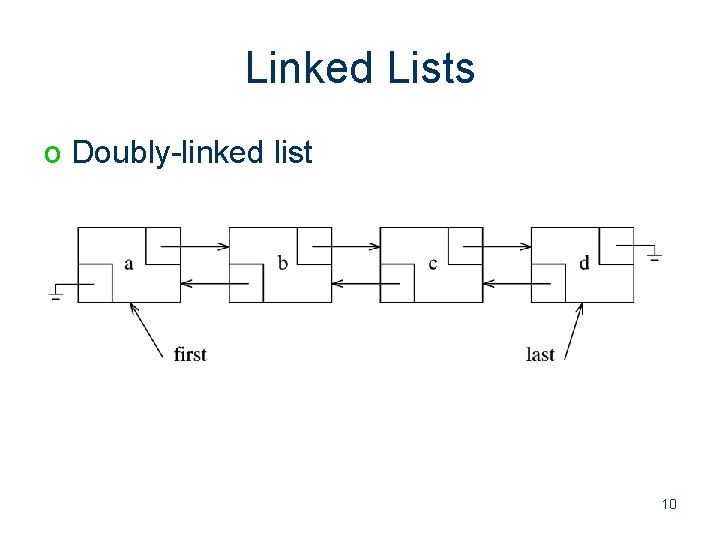
Linked Lists o Doubly-linked list 10
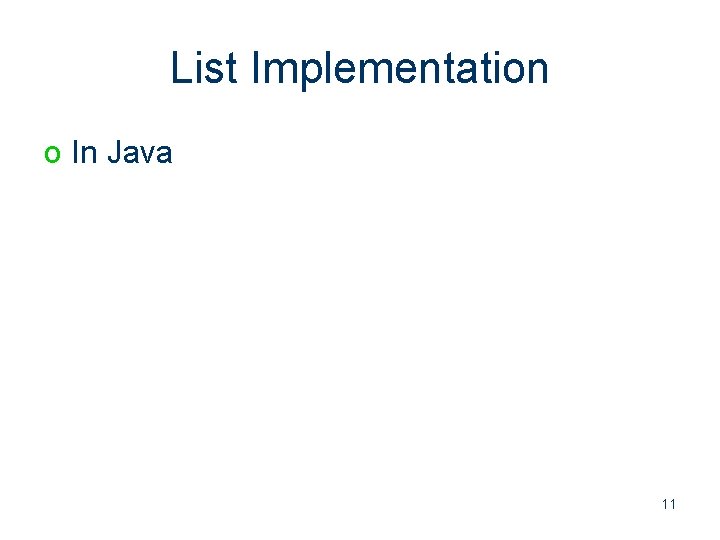
List Implementation o In Java 11
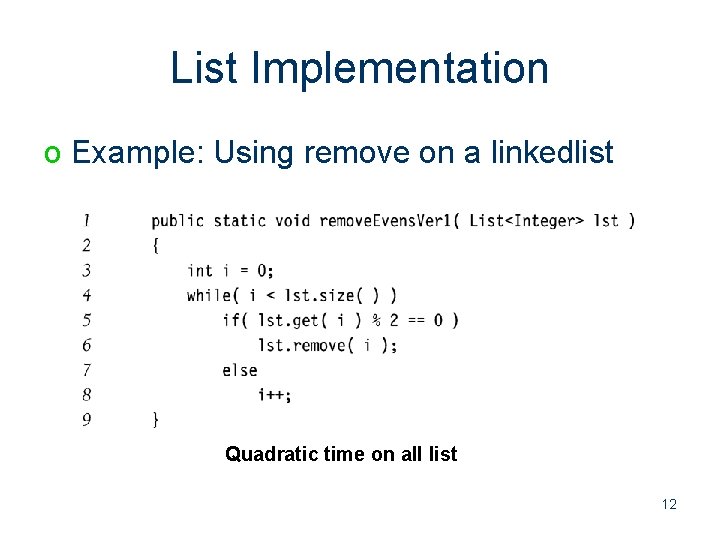
List Implementation o Example: Using remove on a linkedlist Quadratic time on all list 12
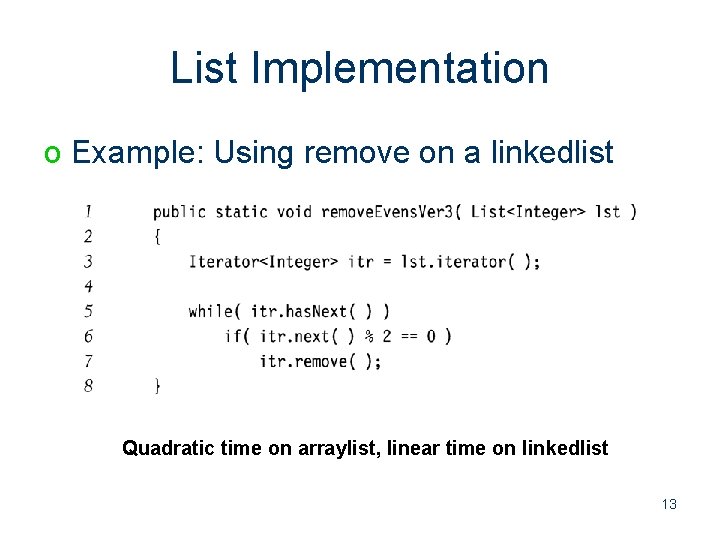
List Implementation o Example: Using remove on a linkedlist Quadratic time on arraylist, linear time on linkedlist 13
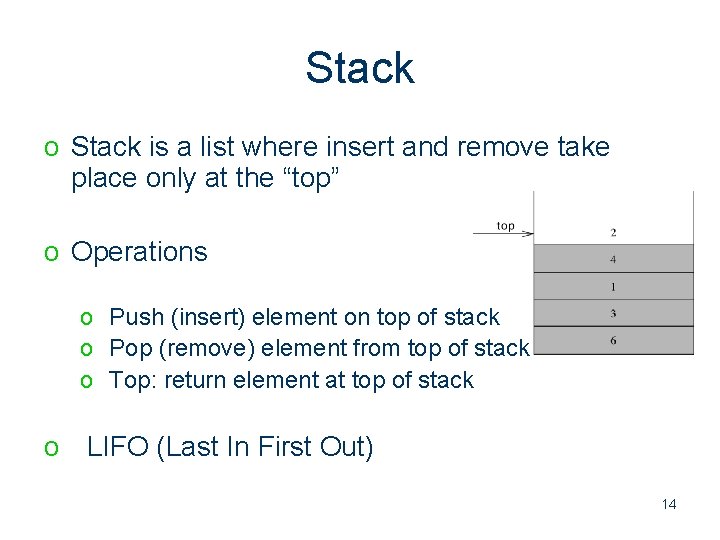
Stack o Stack is a list where insert and remove take place only at the “top” o Operations o Push (insert) element on top of stack o Pop (remove) element from top of stack o Top: return element at top of stack o LIFO (Last In First Out) 14
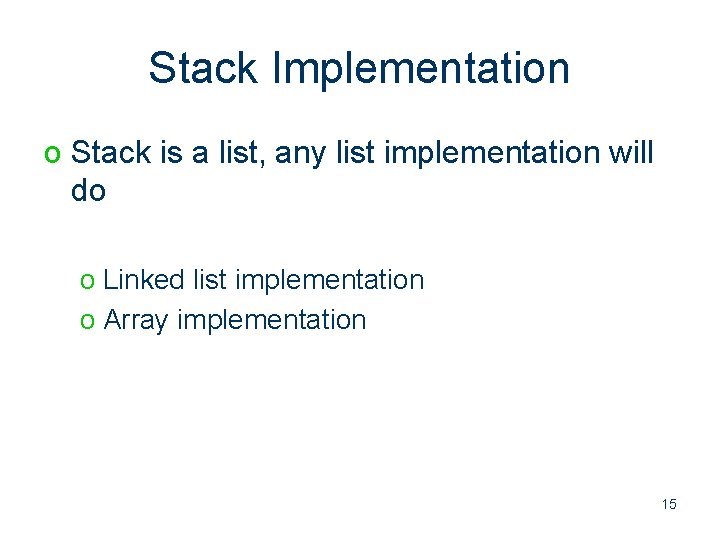
Stack Implementation o Stack is a list, any list implementation will do o Linked list implementation o Array implementation 15
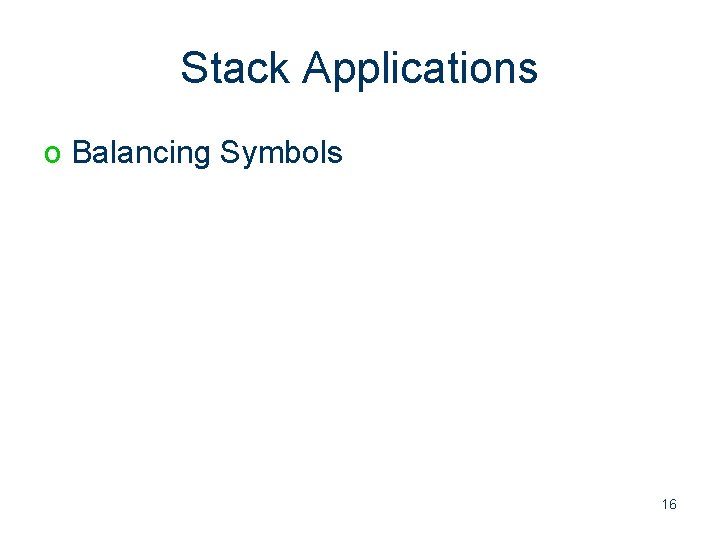
Stack Applications o Balancing Symbols 16
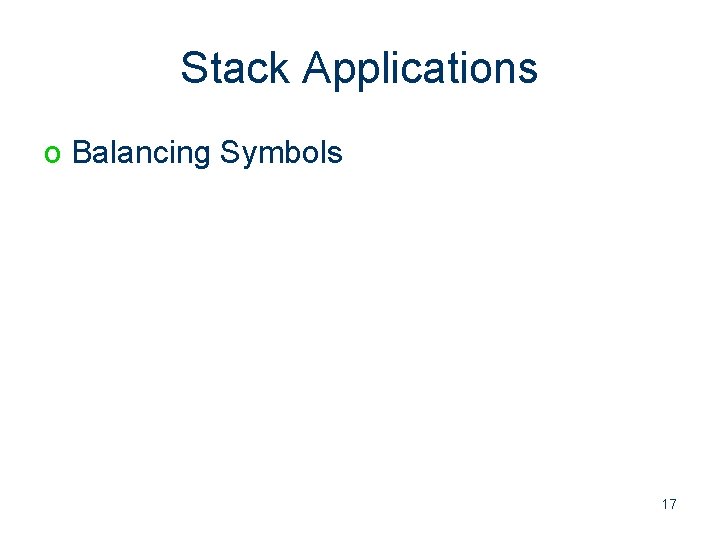
Stack Applications o Balancing Symbols 17
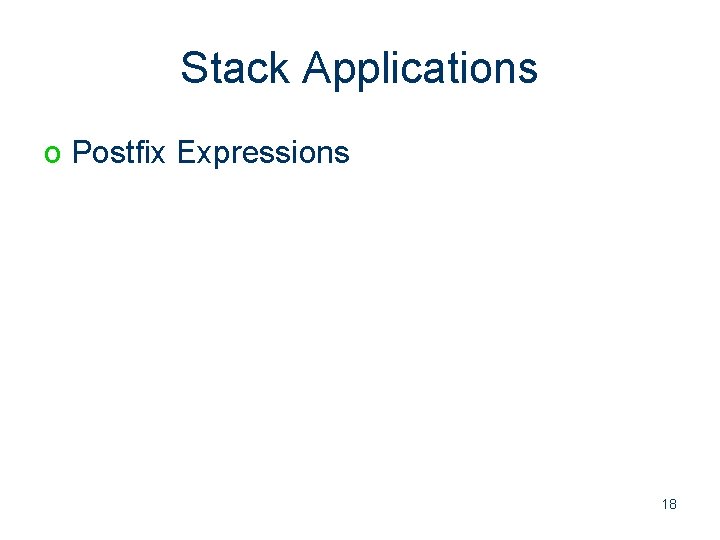
Stack Applications o Postfix Expressions 18
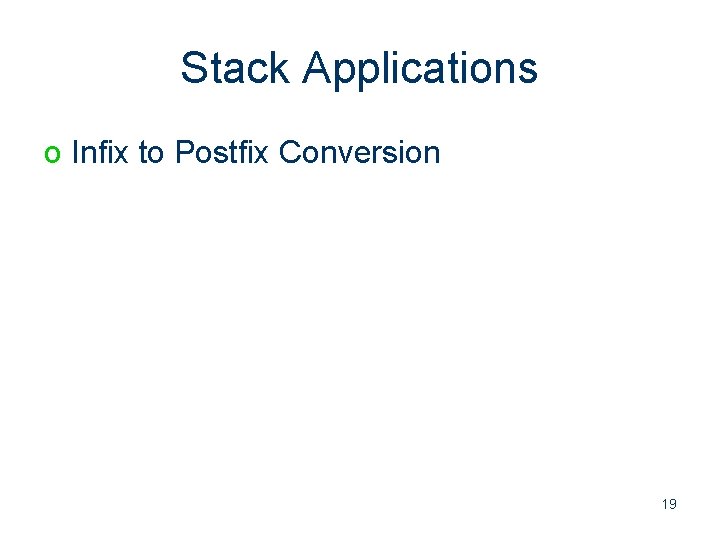
Stack Applications o Infix to Postfix Conversion 19
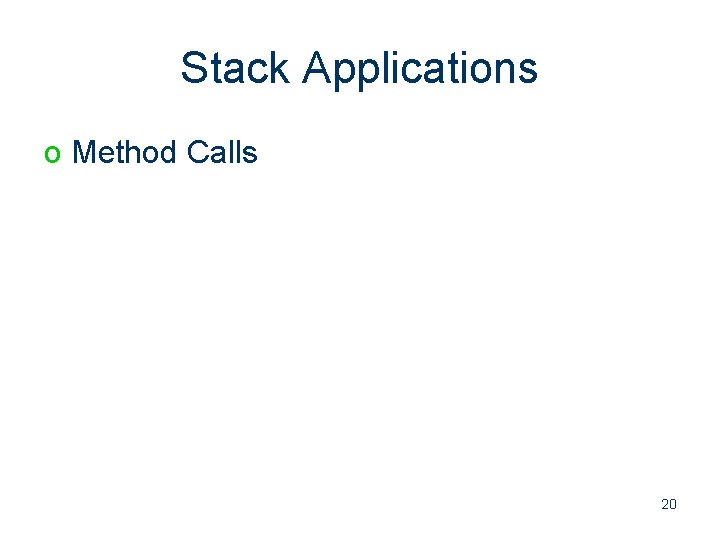
Stack Applications o Method Calls 20
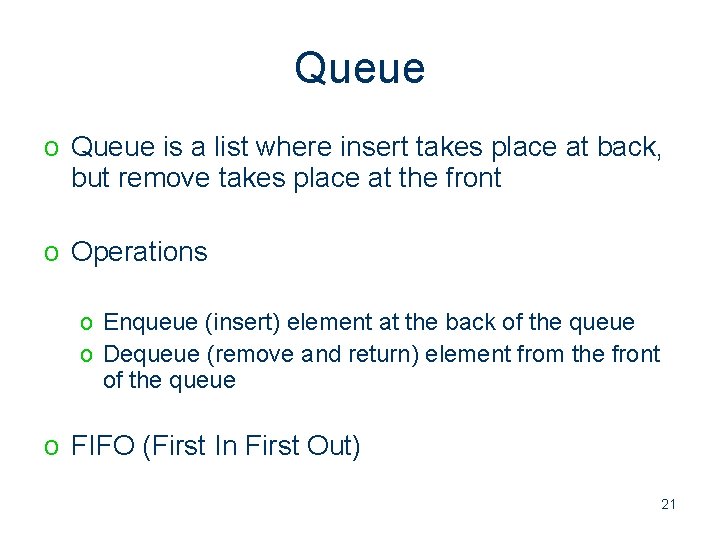
Queue o Queue is a list where insert takes place at back, but remove takes place at the front o Operations o Enqueue (insert) element at the back of the queue o Dequeue (remove and return) element from the front of the queue o FIFO (First In First Out) 21
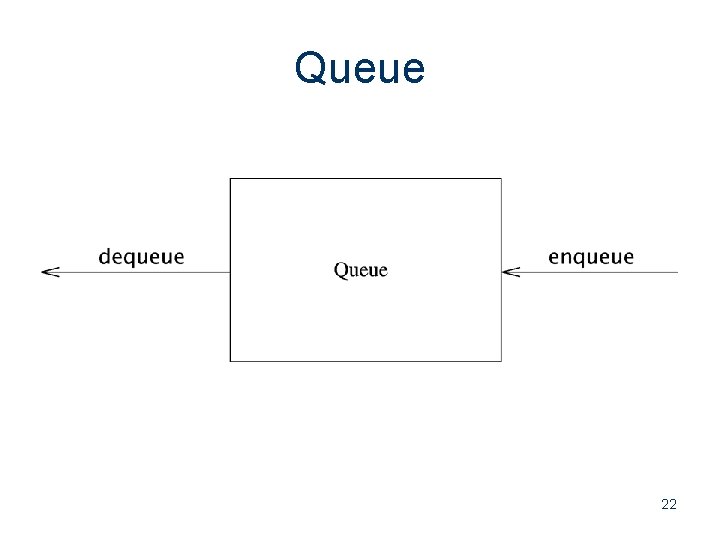
Queue 22
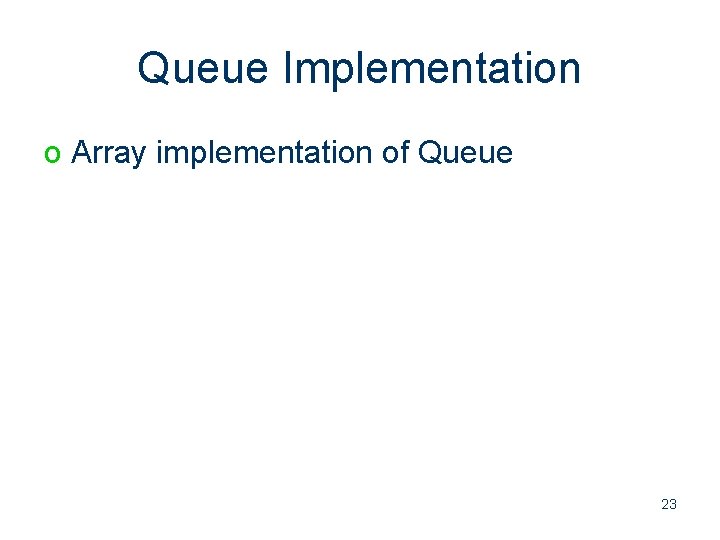
Queue Implementation o Array implementation of Queue 23
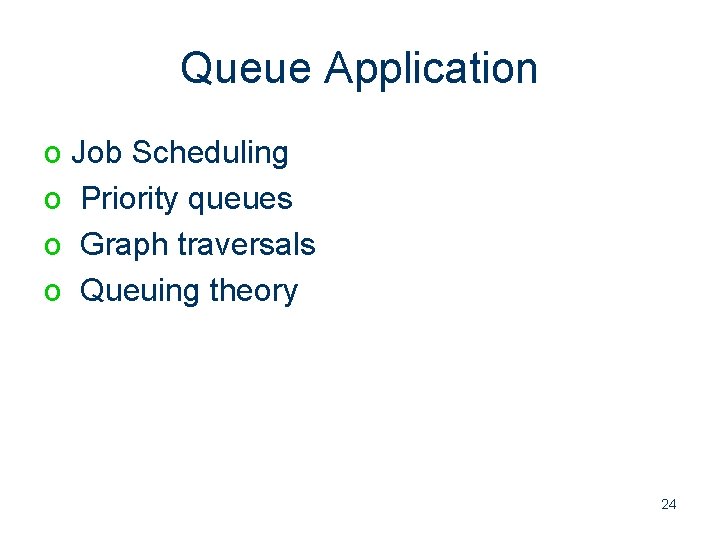
Queue Application o Job Scheduling o Priority queues o Graph traversals o Queuing theory 24