FunctionsRecall 1 Parameter passing return void main int
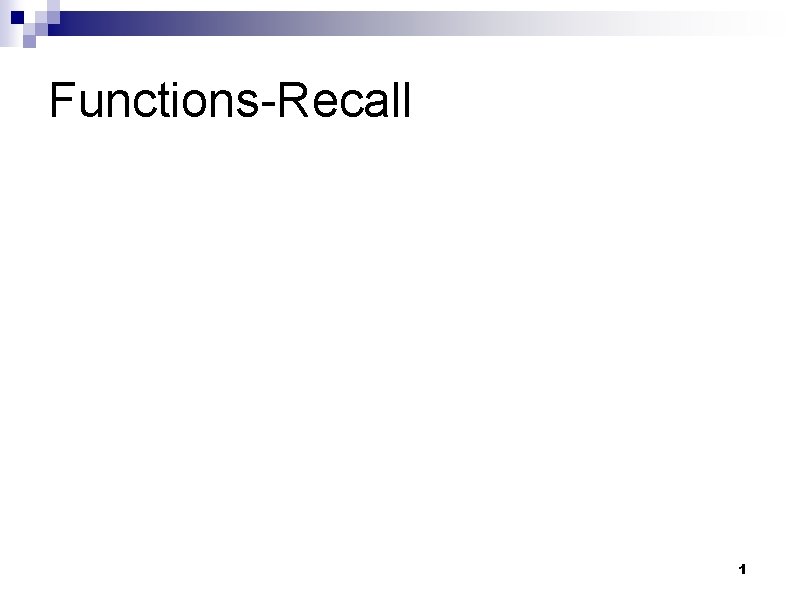
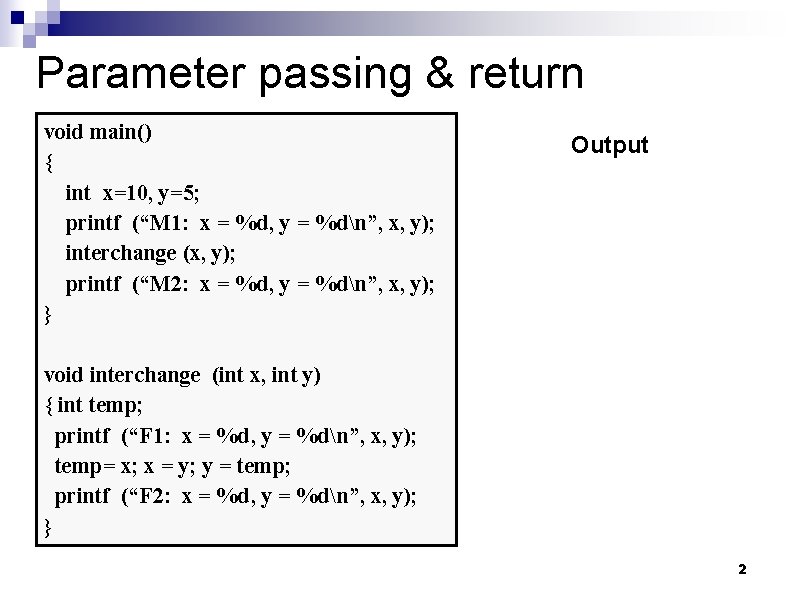
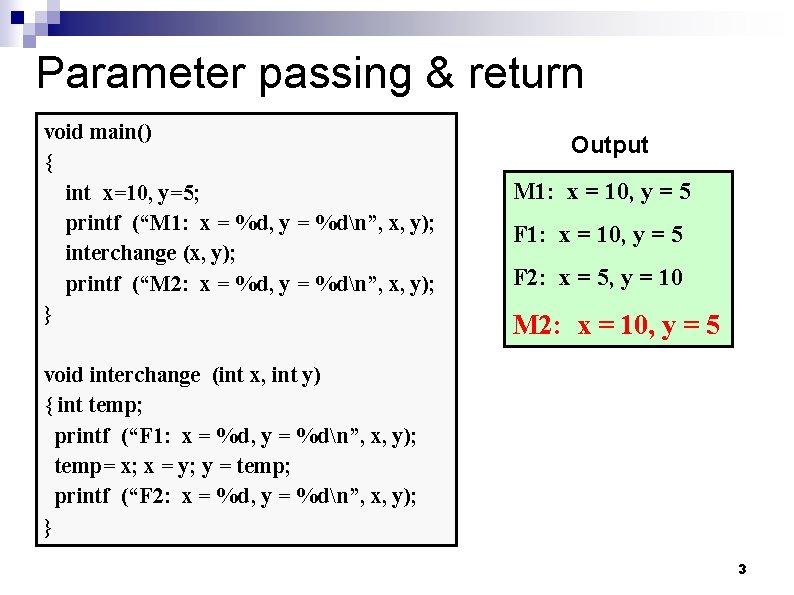
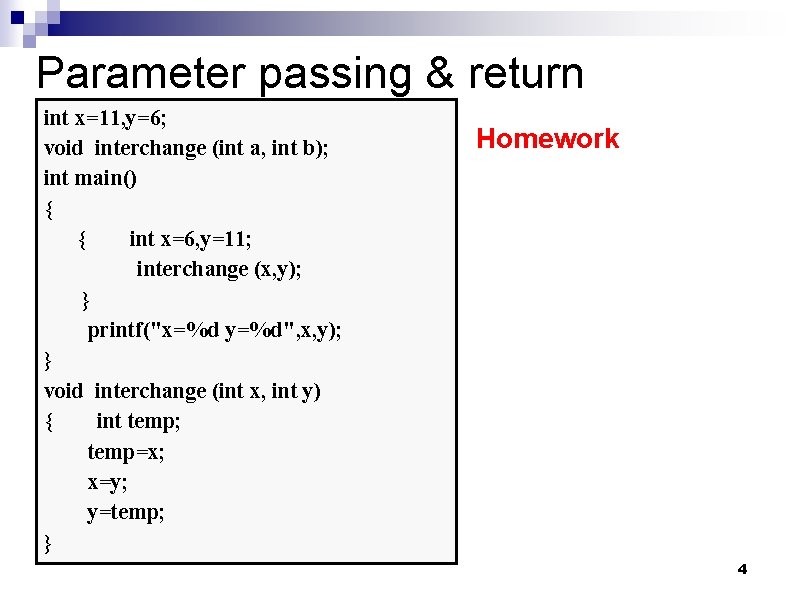
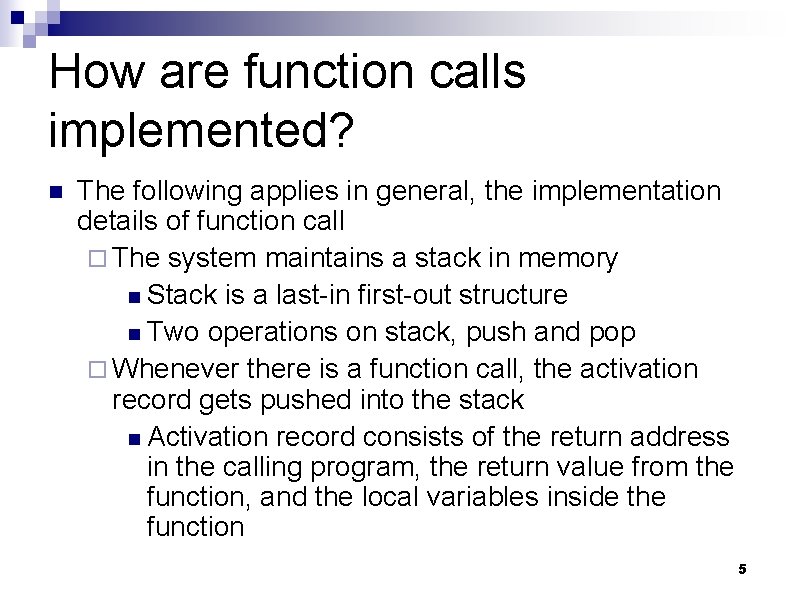
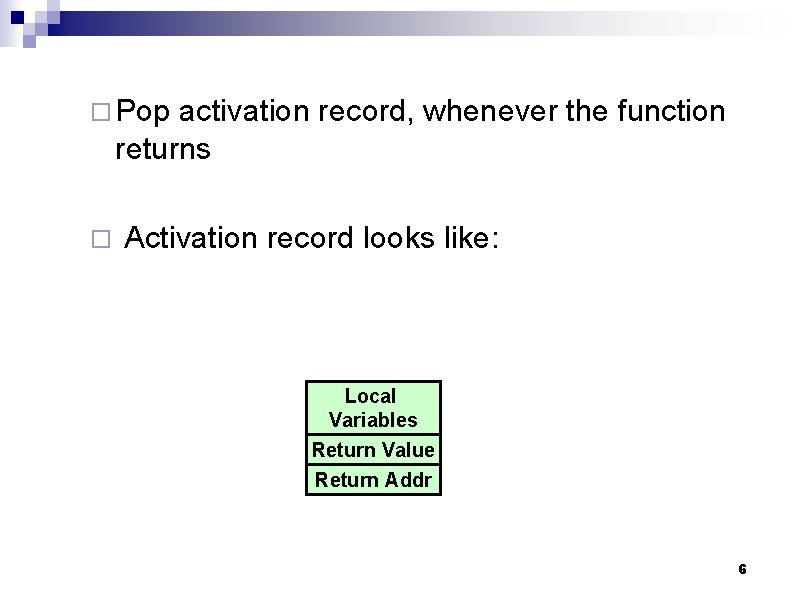
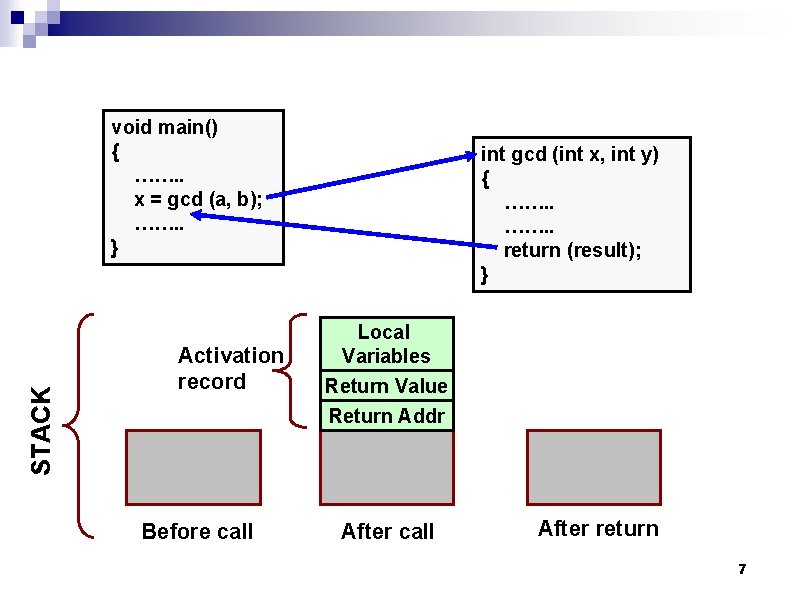
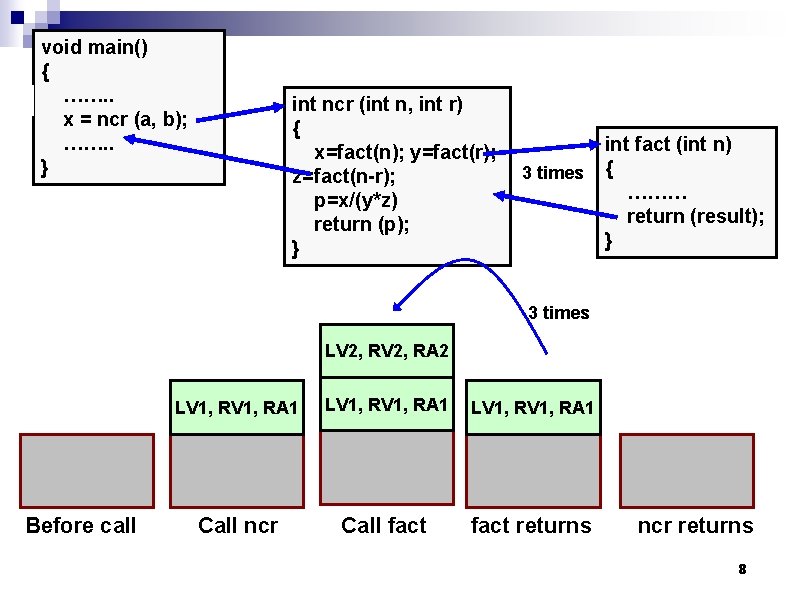
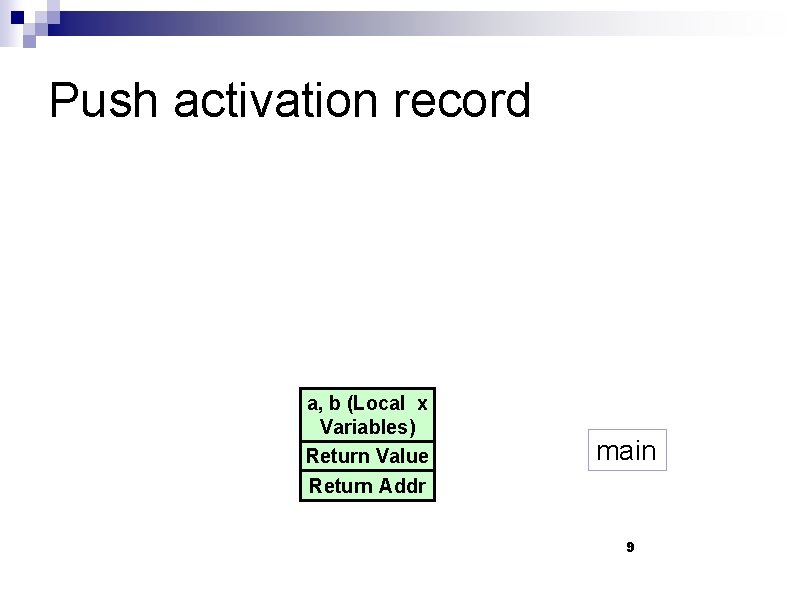
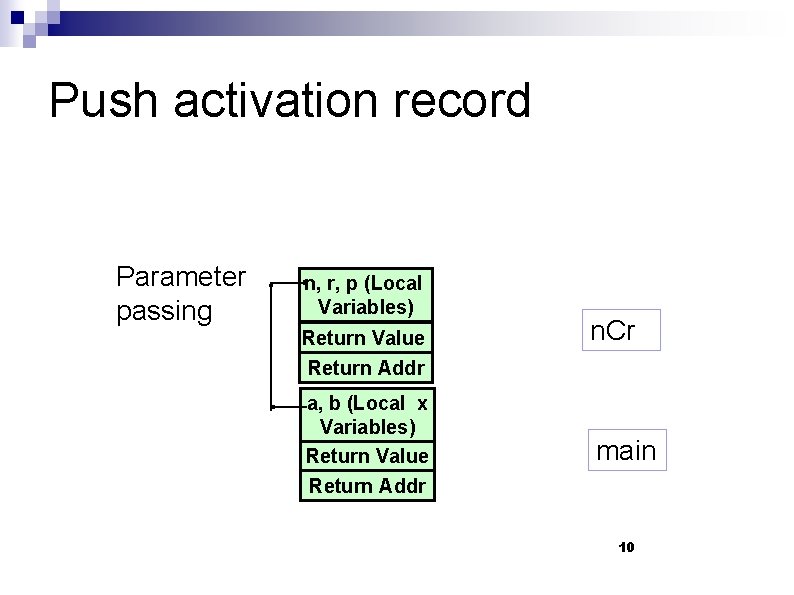
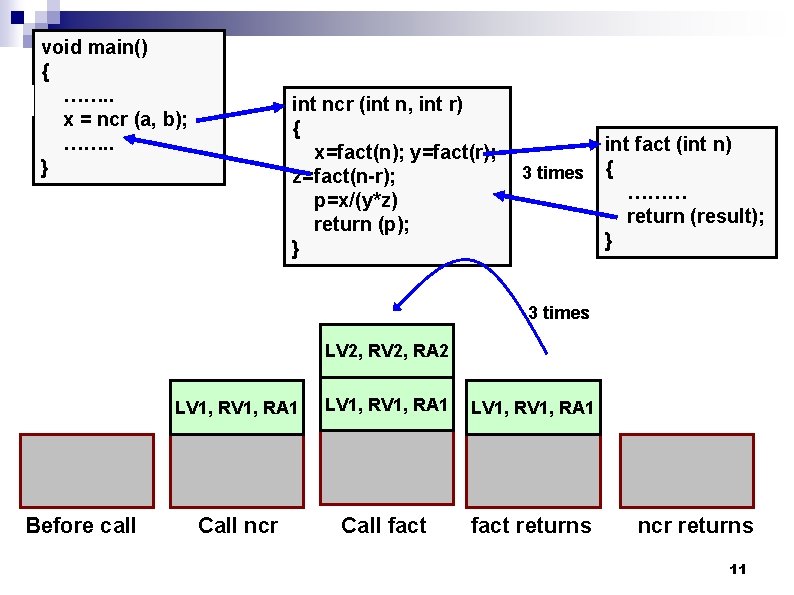
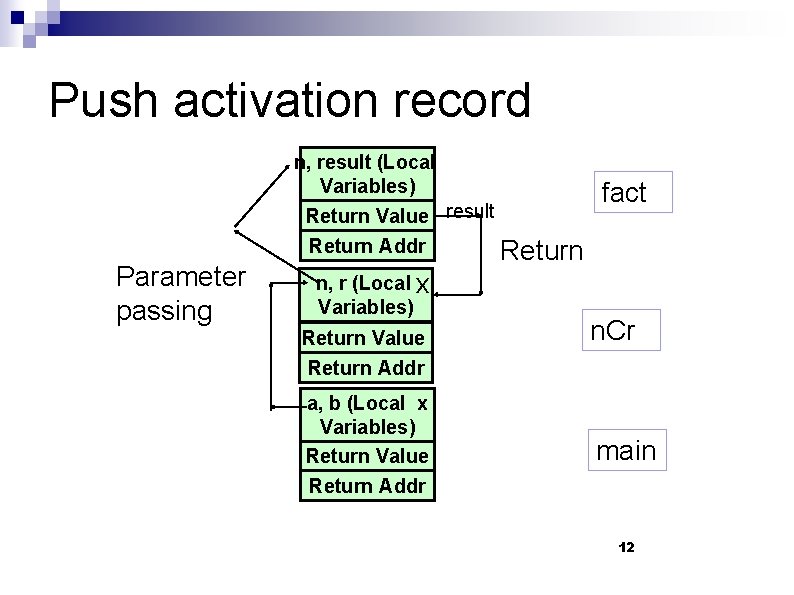
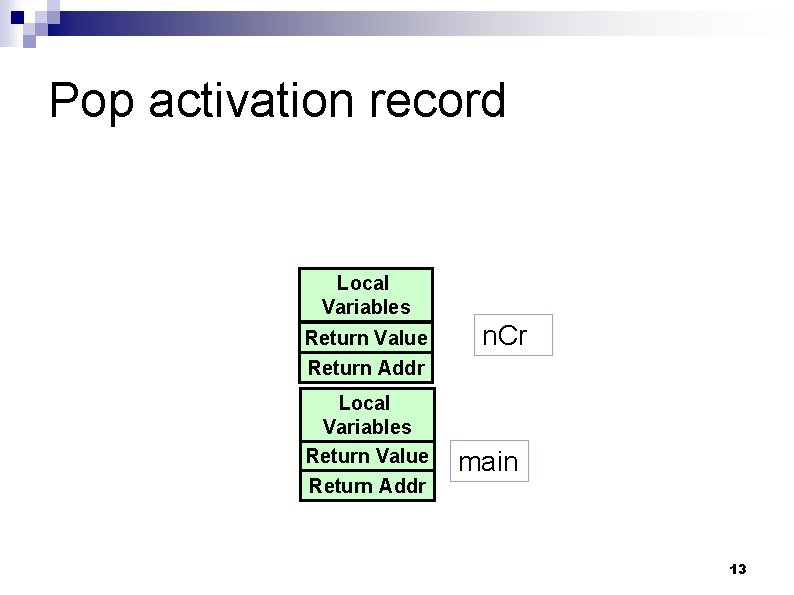
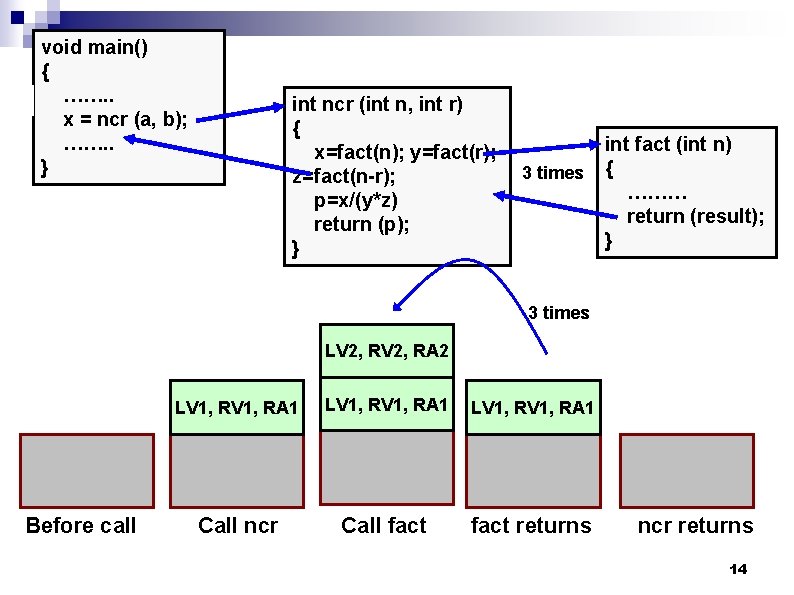
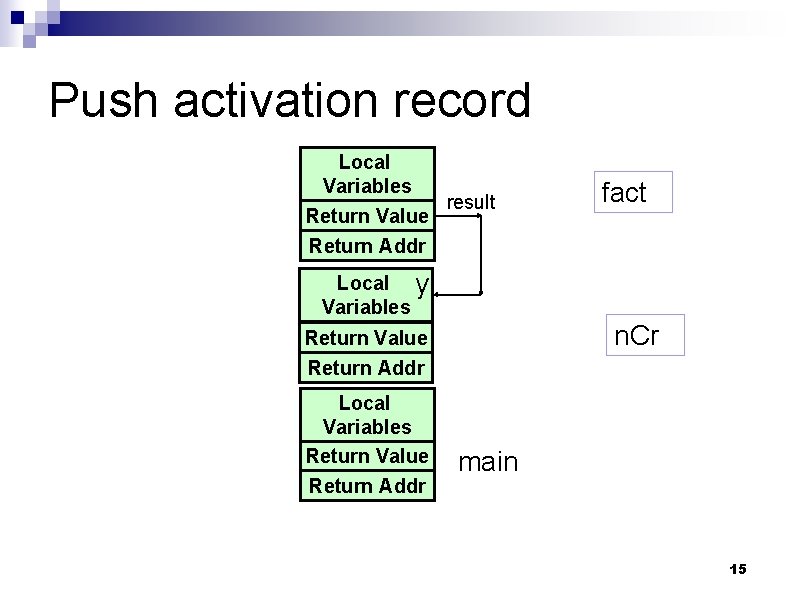
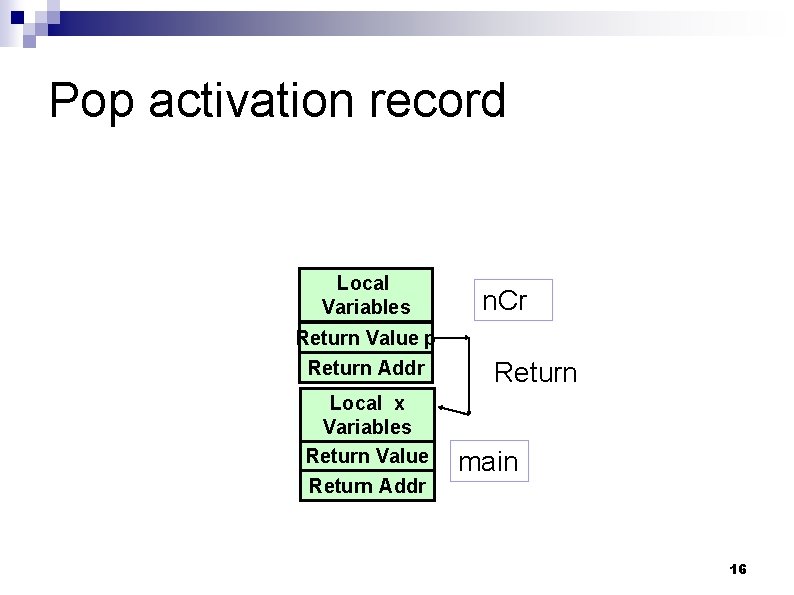
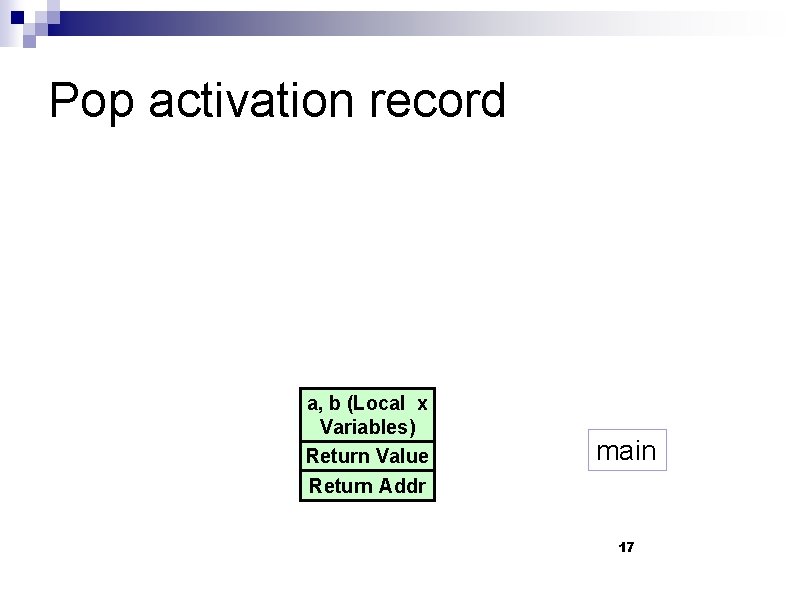
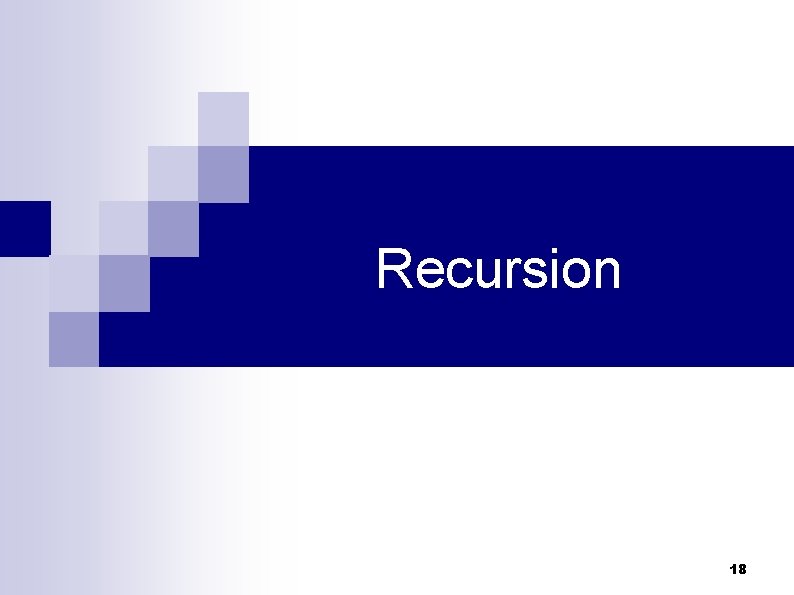
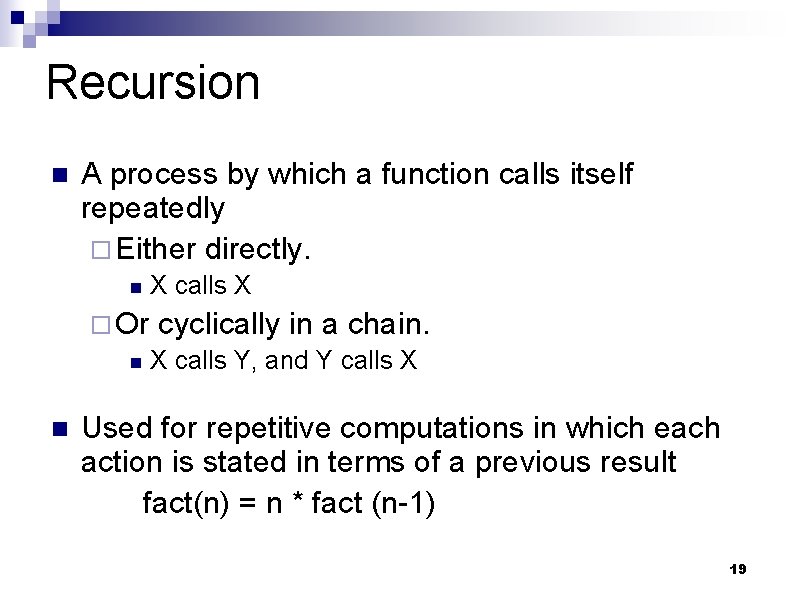
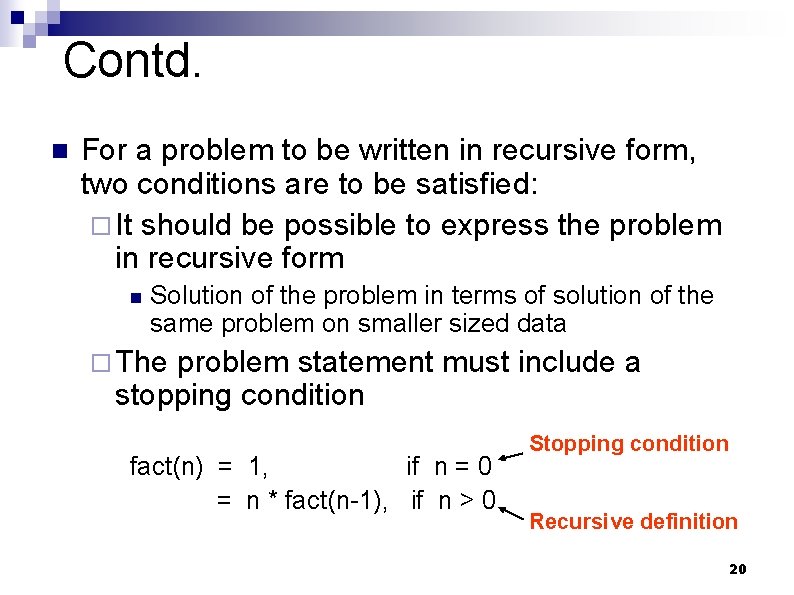
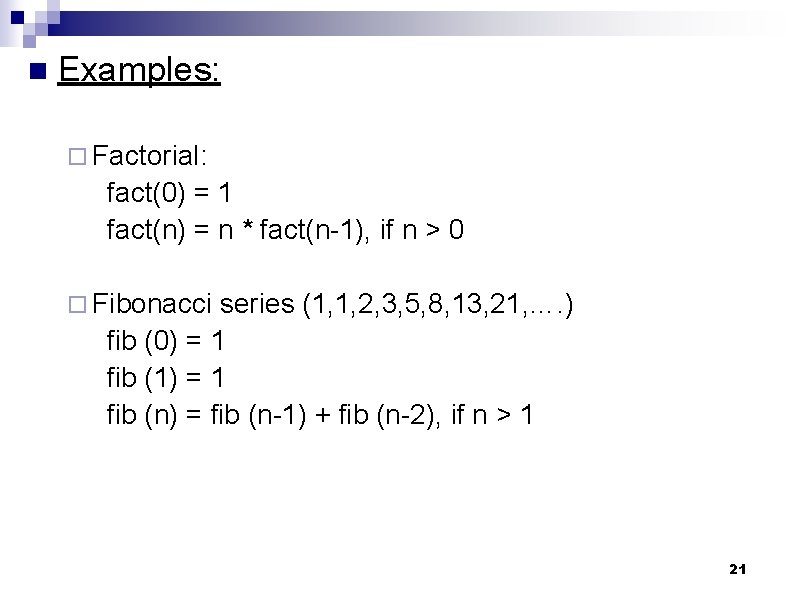
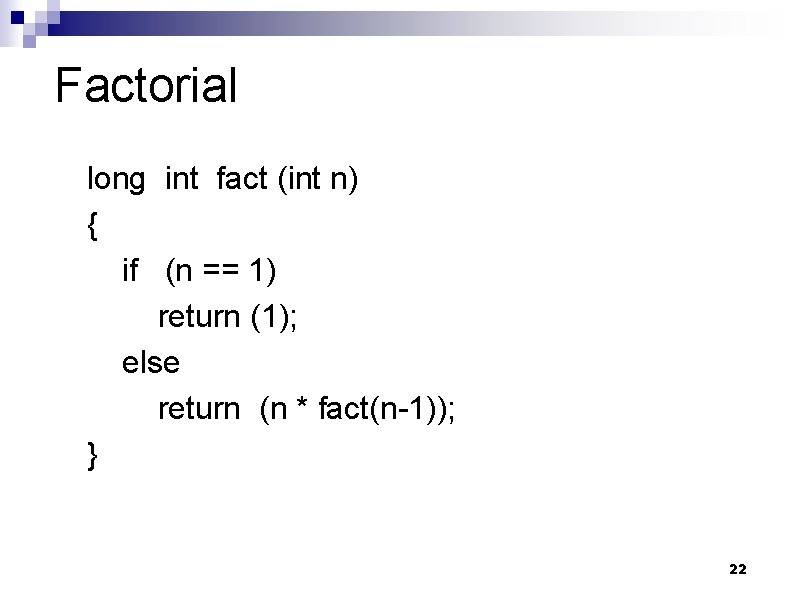
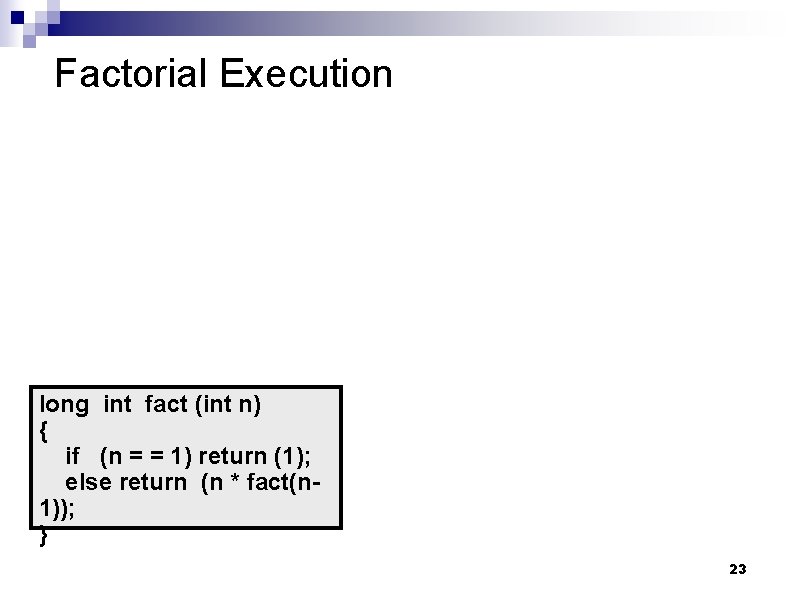
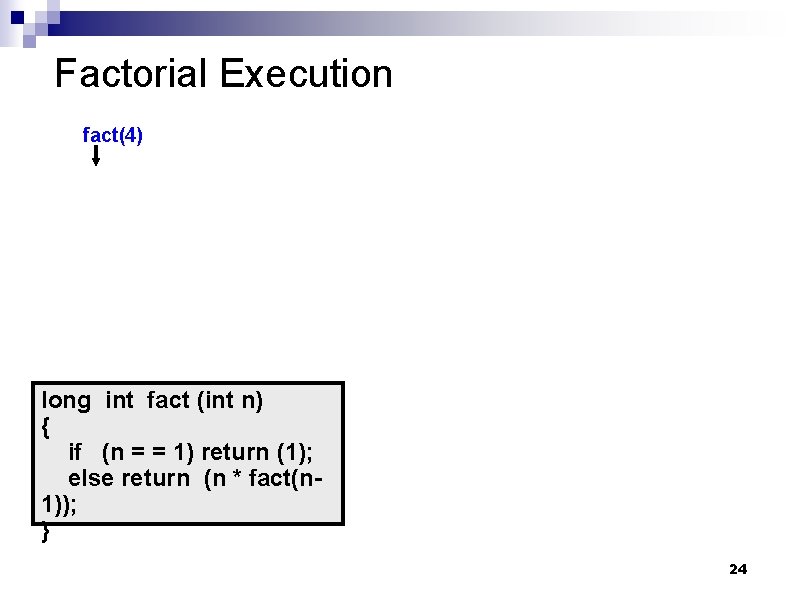
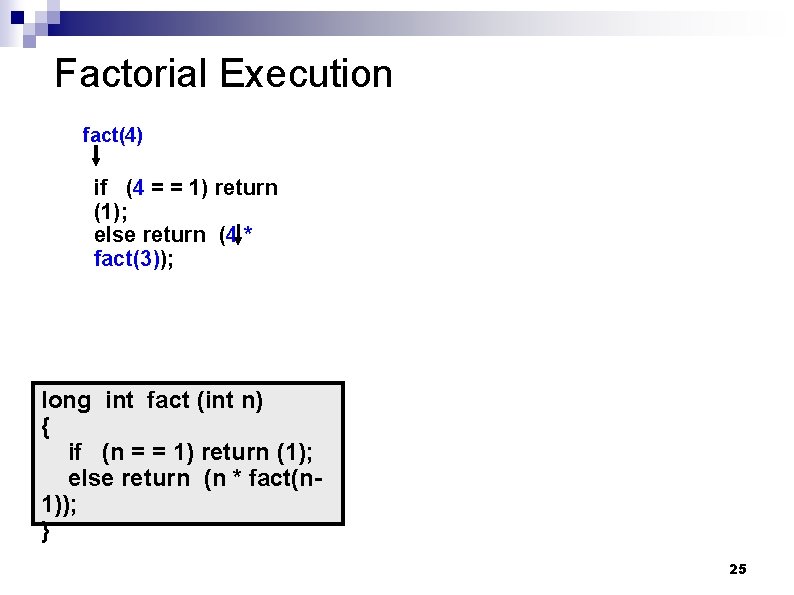
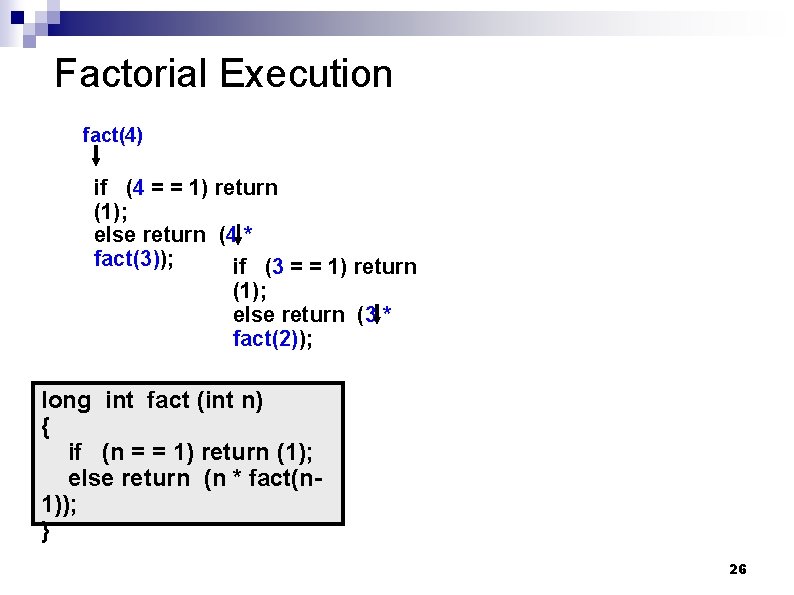
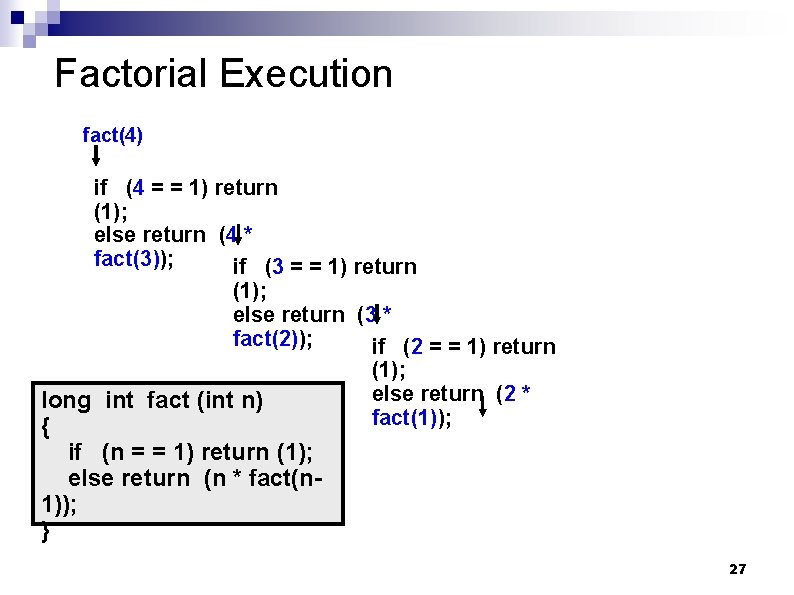
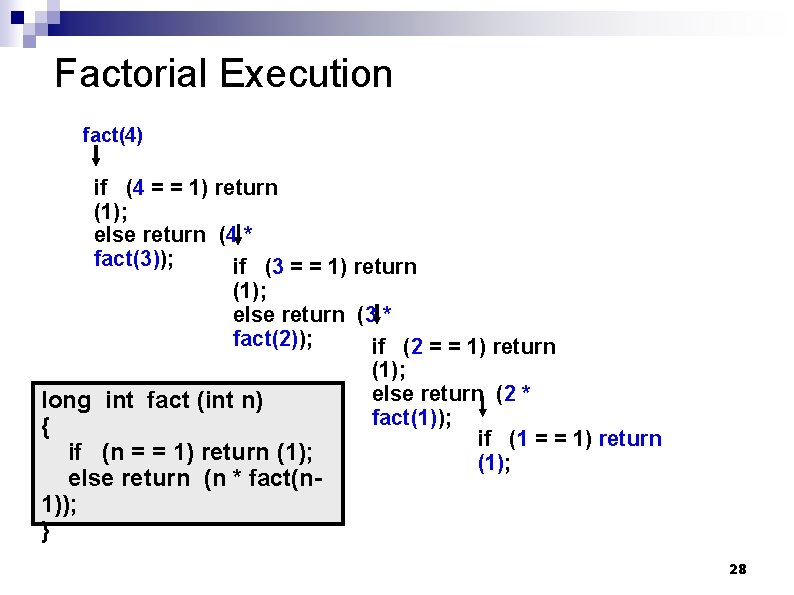
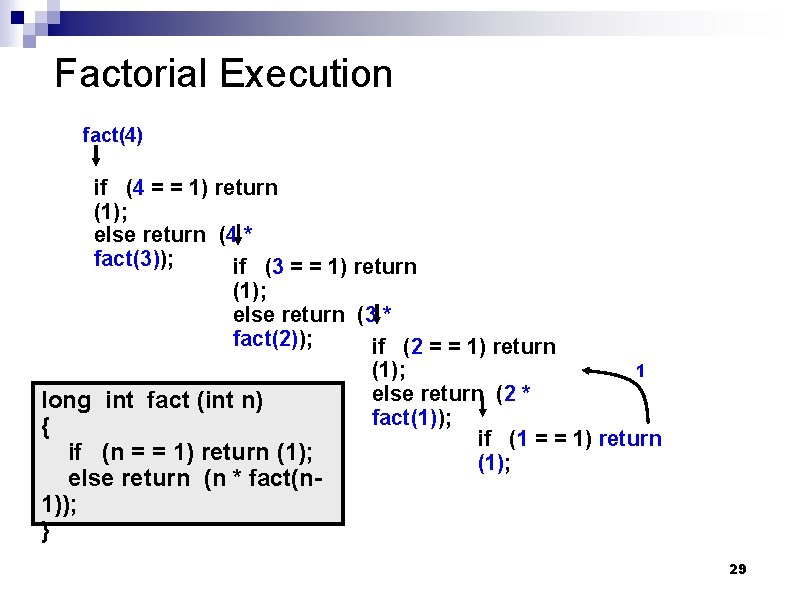
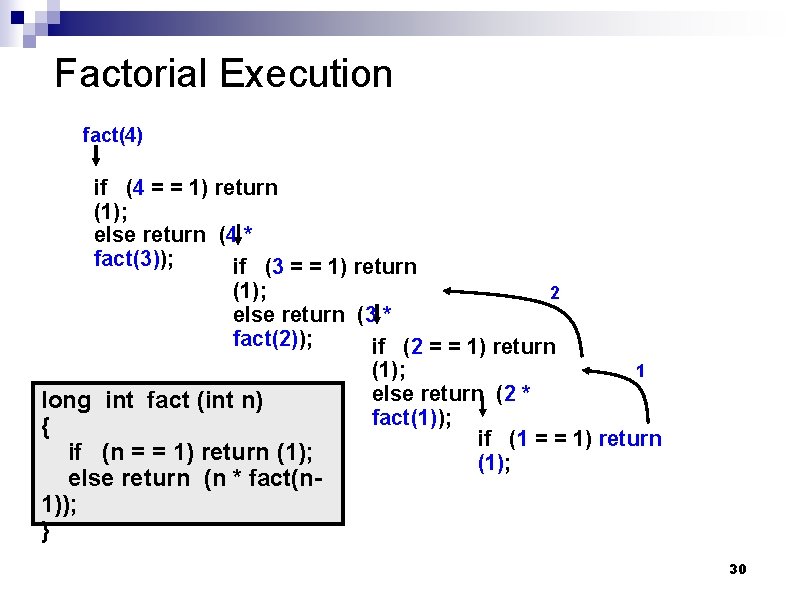
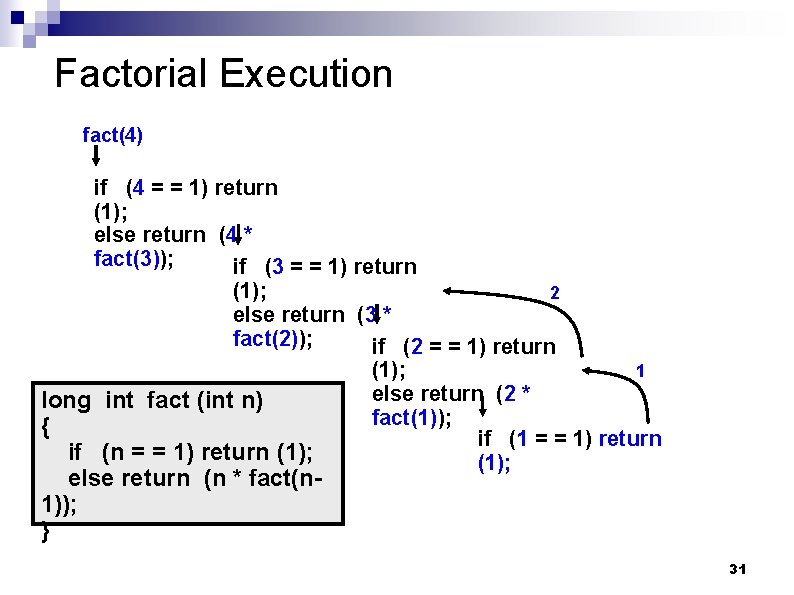
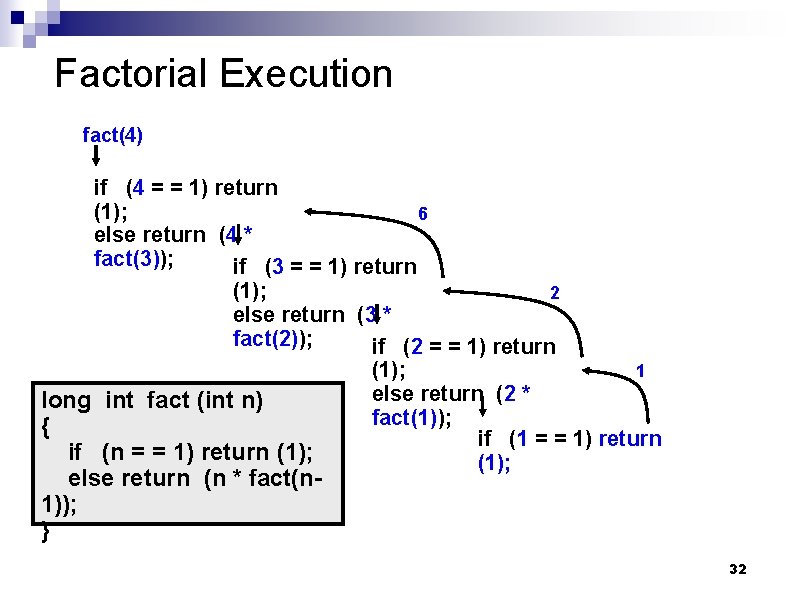
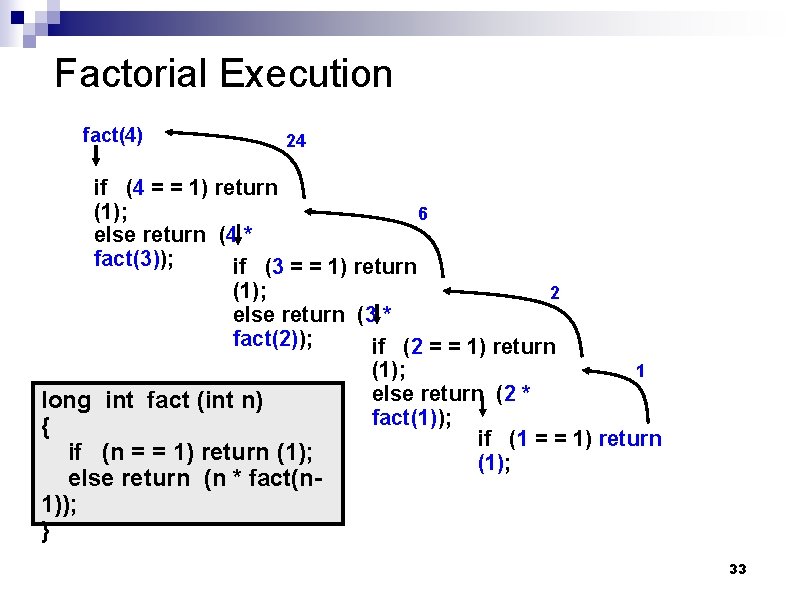
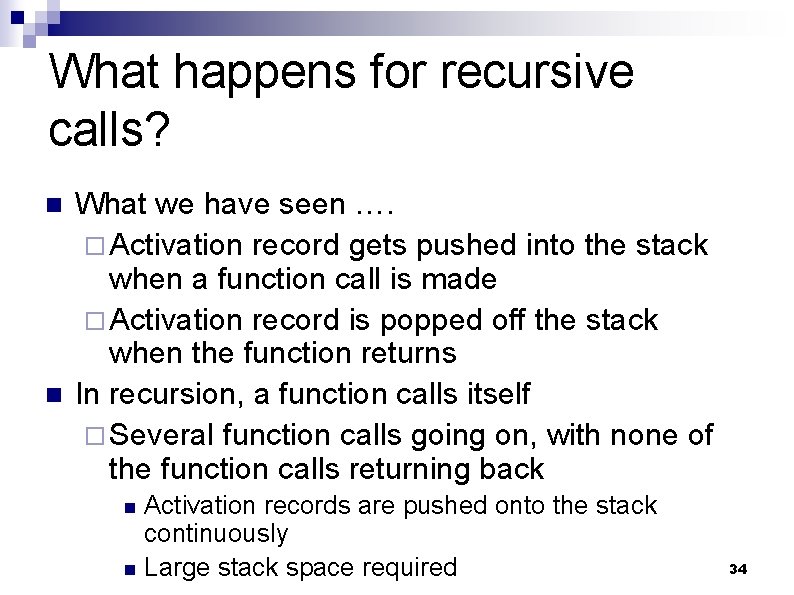
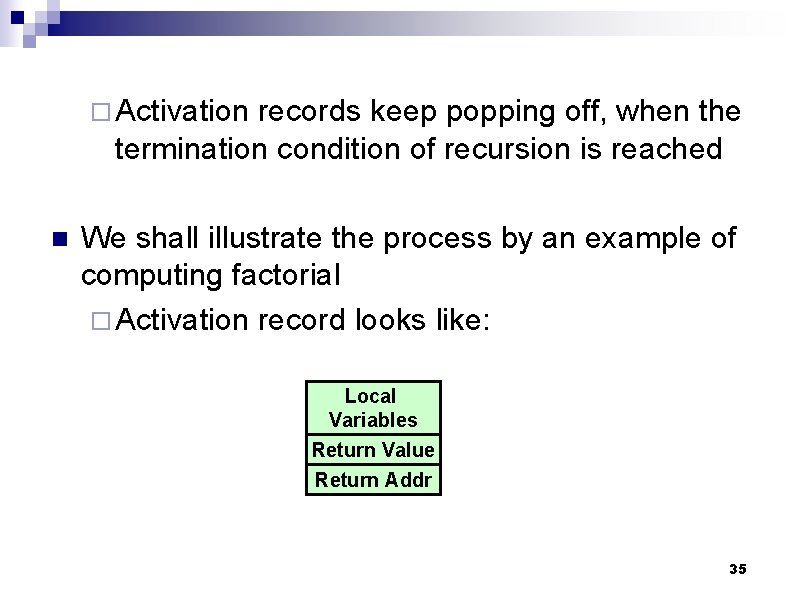
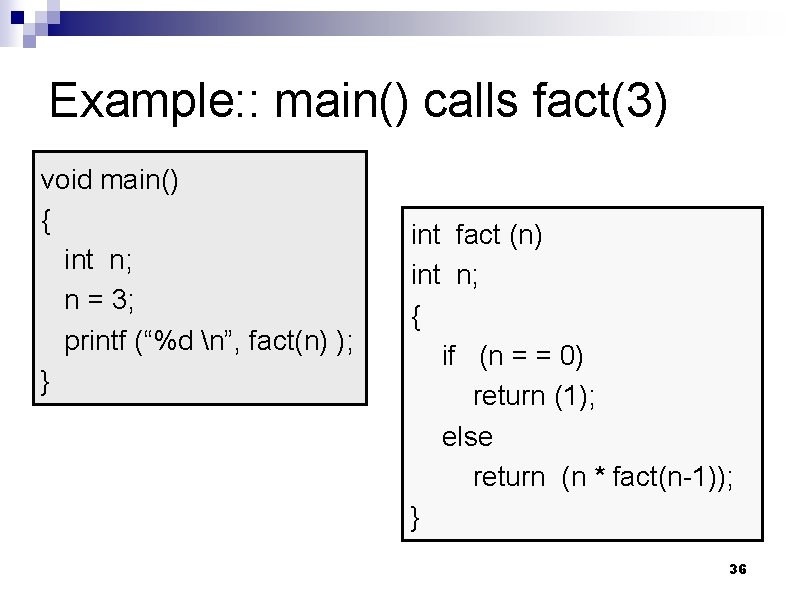
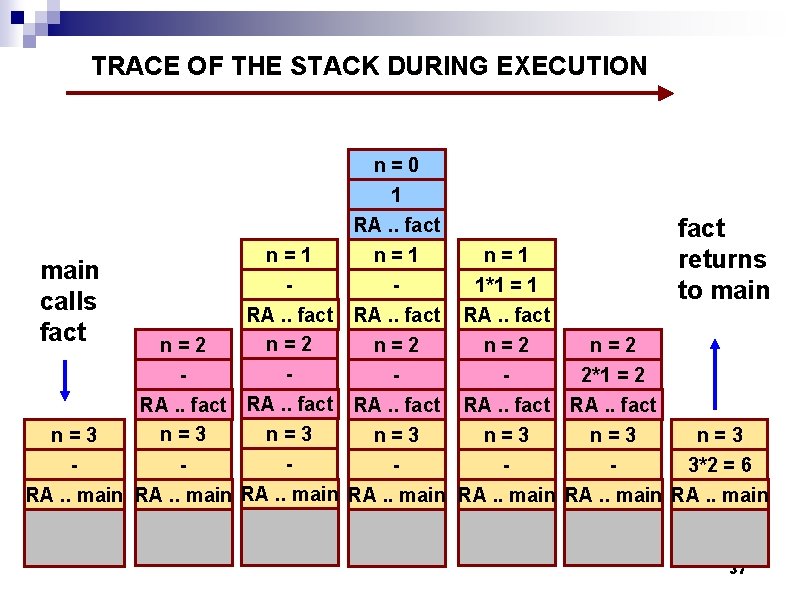
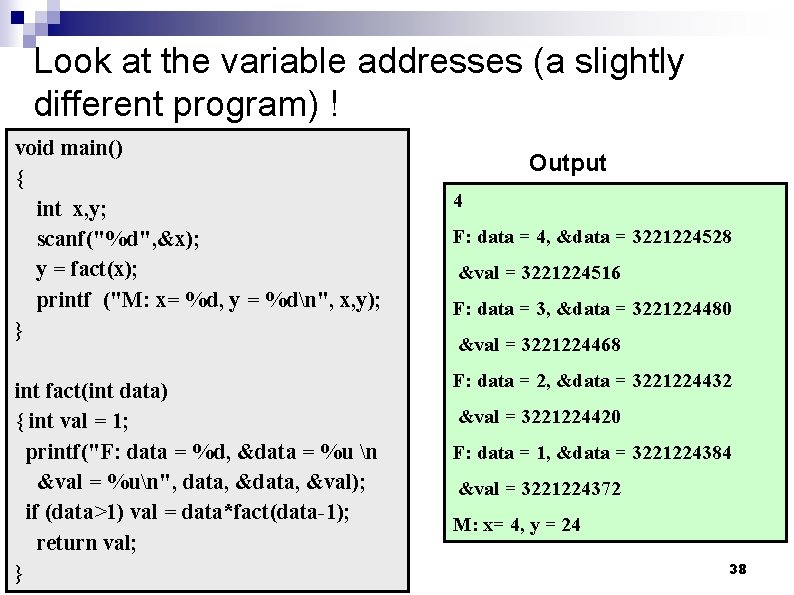
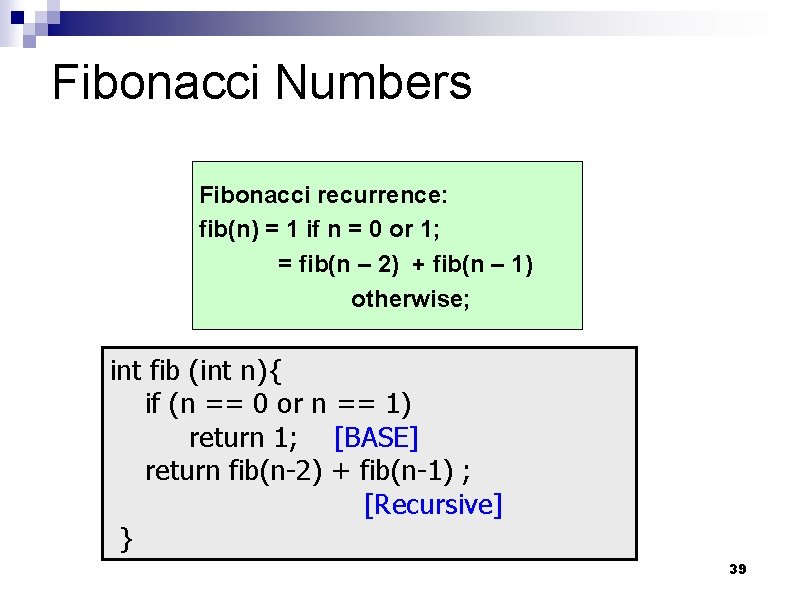
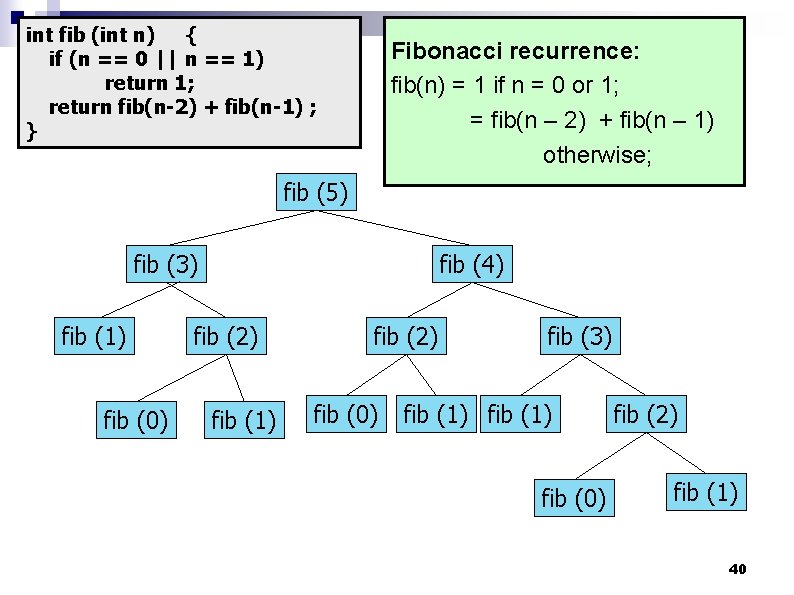
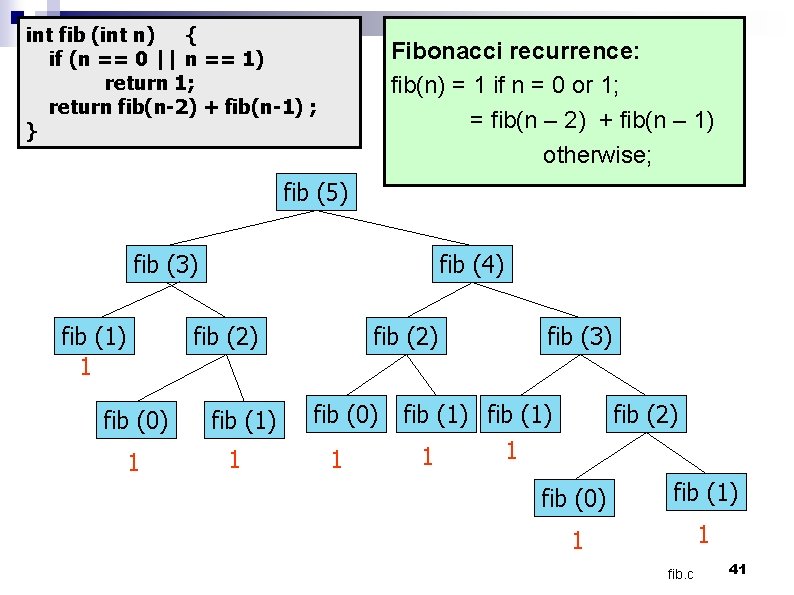
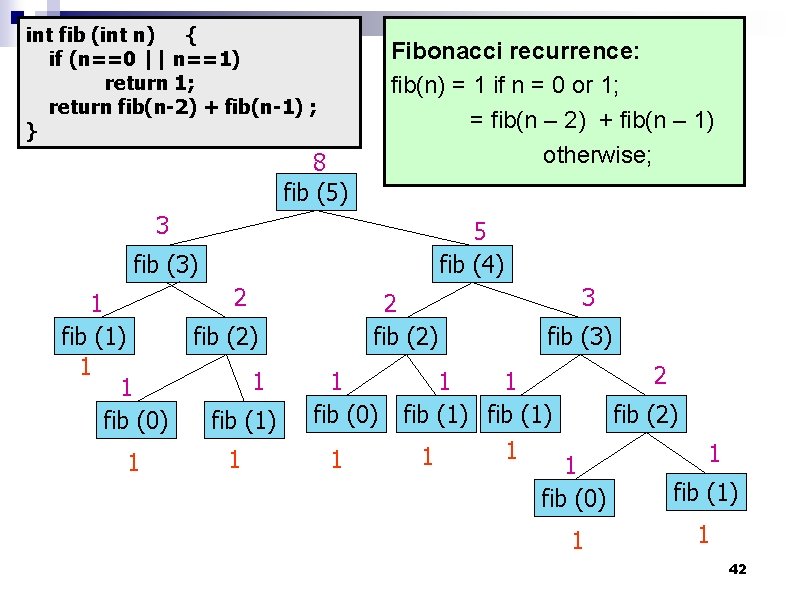
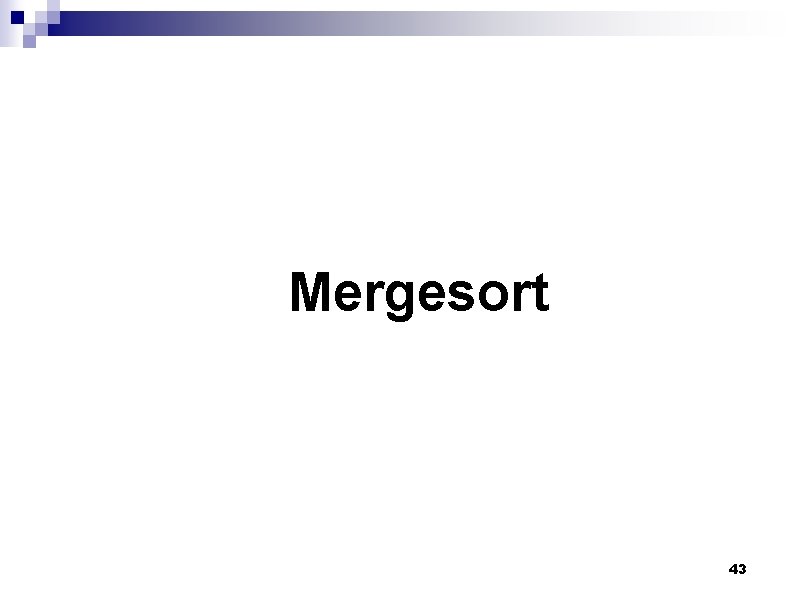
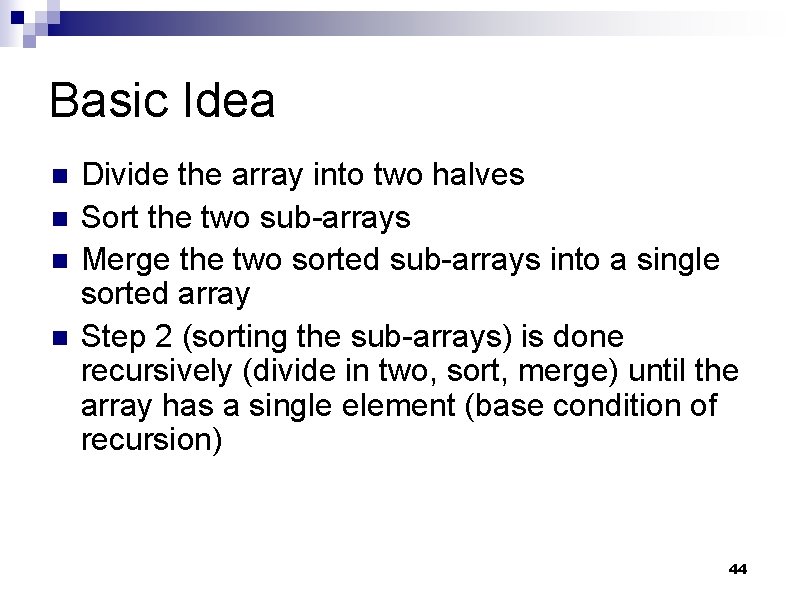
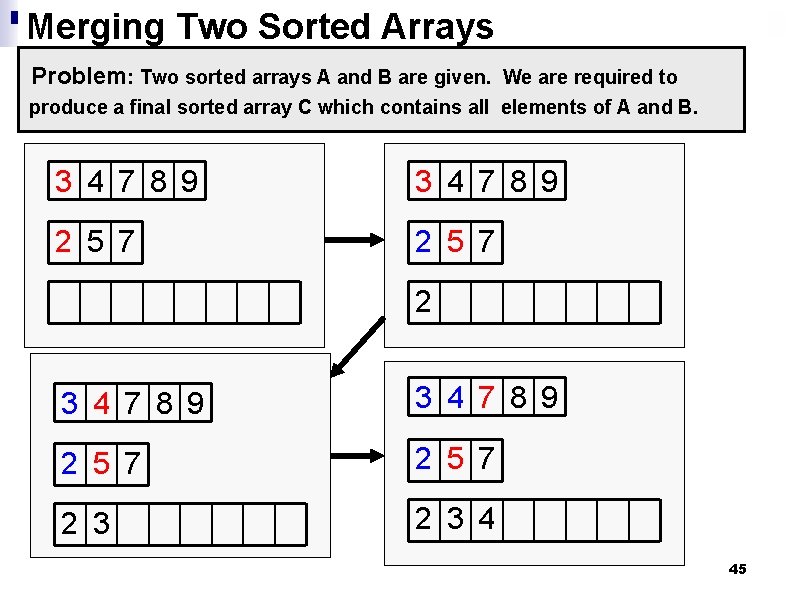
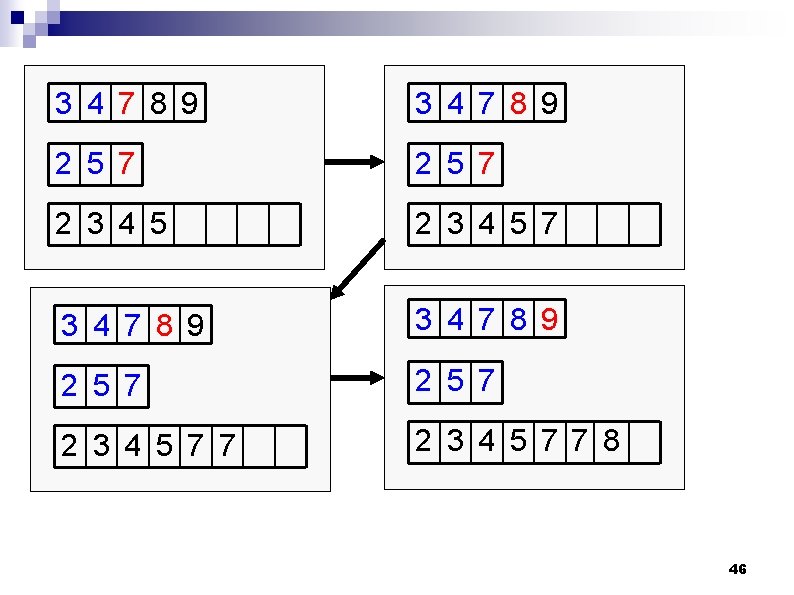
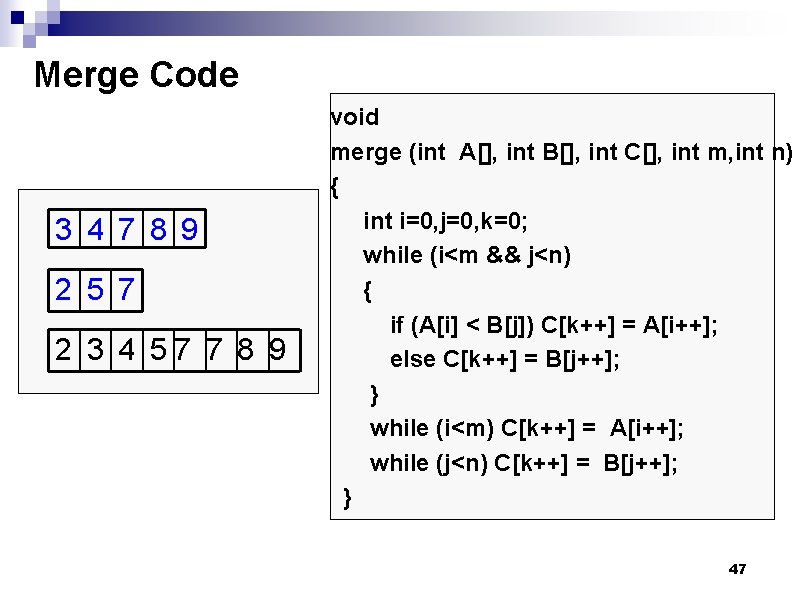
![Merge Sort: Sorting an array recursively void mergesort (int A[], int n) { int Merge Sort: Sorting an array recursively void mergesort (int A[], int n) { int](https://slidetodoc.com/presentation_image_h2/6d9c5cd009e63c476a8fe01339ec99cd/image-48.jpg)
- Slides: 48
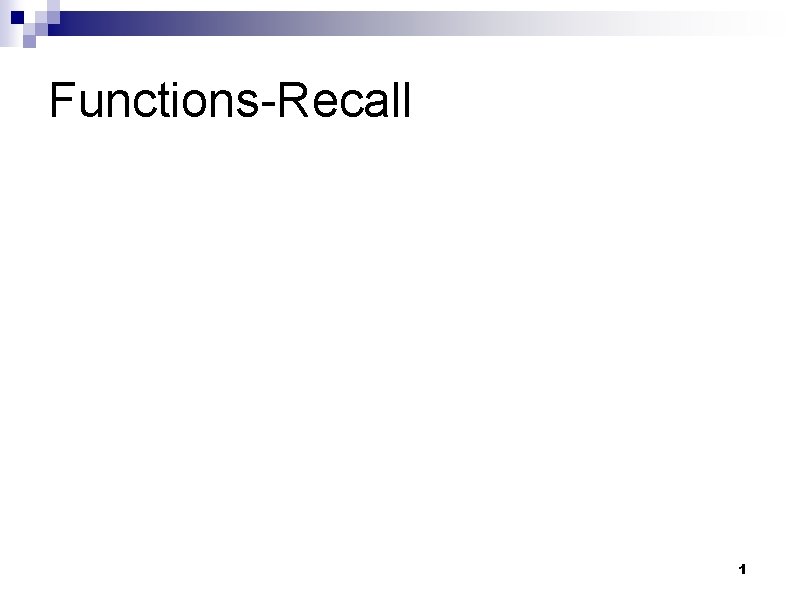
Functions-Recall 1
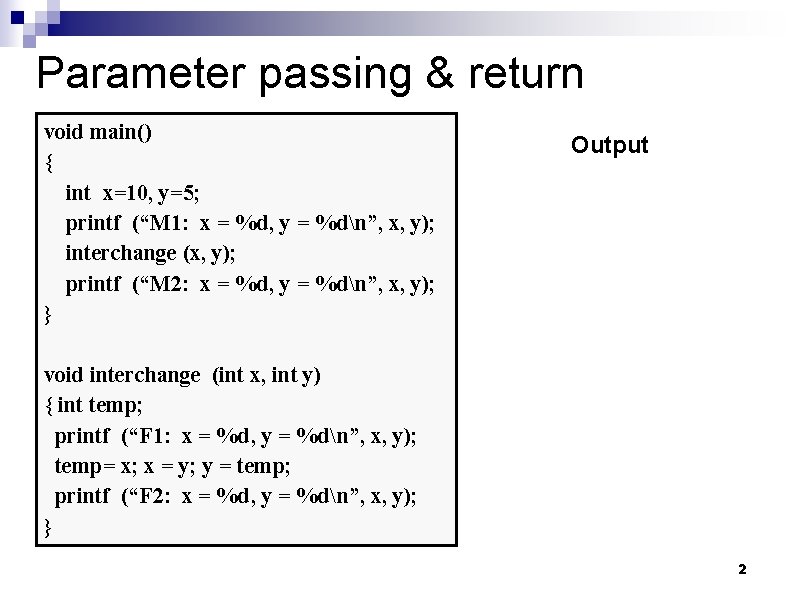
Parameter passing & return void main() { int x=10, y=5; printf (“M 1: x = %d, y = %dn”, x, y); interchange (x, y); printf (“M 2: x = %d, y = %dn”, x, y); } Output void interchange (int x, int y) { int temp; printf (“F 1: x = %d, y = %dn”, x, y); temp= x; x = y; y = temp; printf (“F 2: x = %d, y = %dn”, x, y); } 2
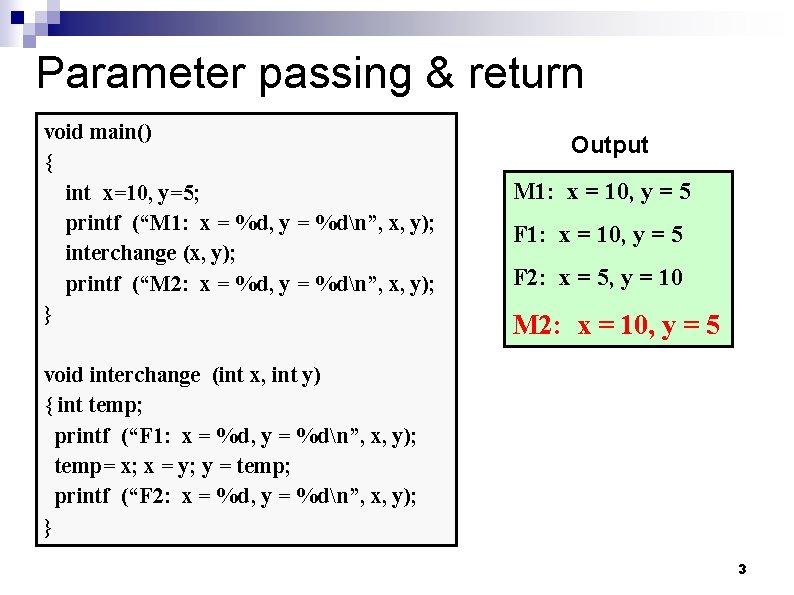
Parameter passing & return void main() { int x=10, y=5; printf (“M 1: x = %d, y = %dn”, x, y); interchange (x, y); printf (“M 2: x = %d, y = %dn”, x, y); } Output M 1: x = 10, y = 5 F 2: x = 5, y = 10 M 2: x = 10, y = 5 void interchange (int x, int y) { int temp; printf (“F 1: x = %d, y = %dn”, x, y); temp= x; x = y; y = temp; printf (“F 2: x = %d, y = %dn”, x, y); } 3
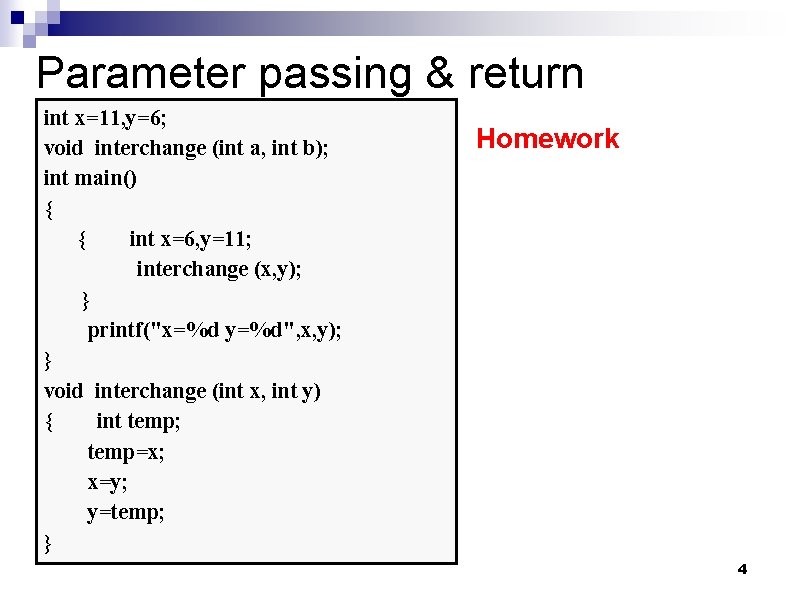
Parameter passing & return int x=11, y=6; void interchange (int a, int b); int main() { { int x=6, y=11; interchange (x, y); } printf("x=%d y=%d", x, y); } void interchange (int x, int y) { int temp; temp=x; x=y; y=temp; } Homework 4
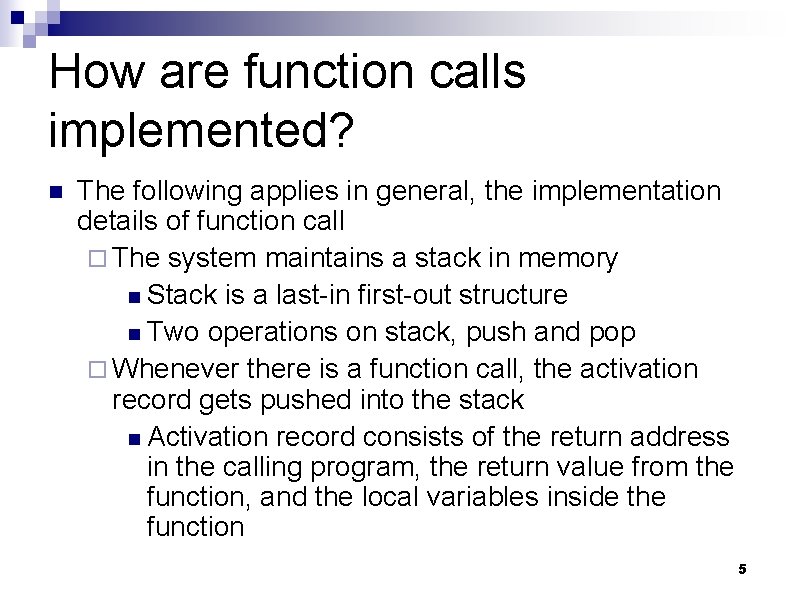
How are function calls implemented? n The following applies in general, the implementation details of function call ¨ The system maintains a stack in memory n Stack is a last-in first-out structure n Two operations on stack, push and pop ¨ Whenever there is a function call, the activation record gets pushed into the stack n Activation record consists of the return address in the calling program, the return value from the function, and the local variables inside the function 5
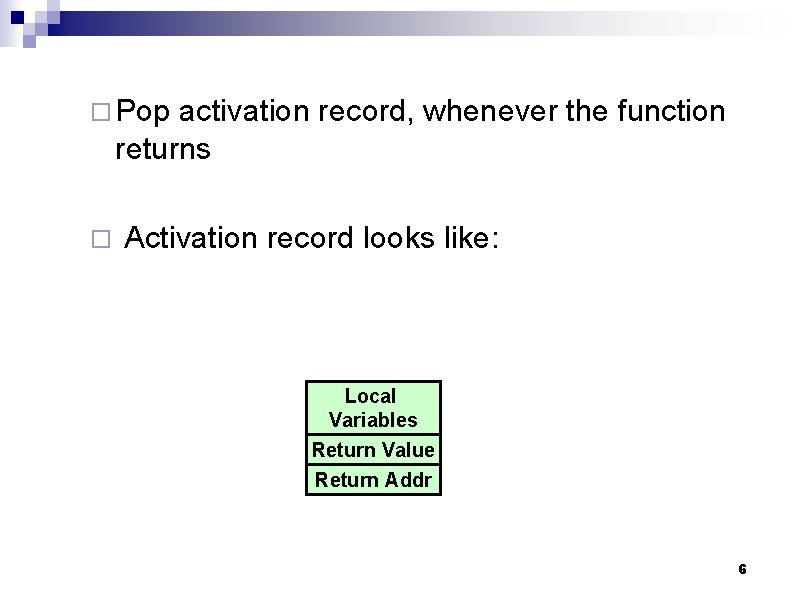
¨ Pop activation record, whenever the function returns ¨ Activation record looks like: Local Variables Return Value Return Addr 6
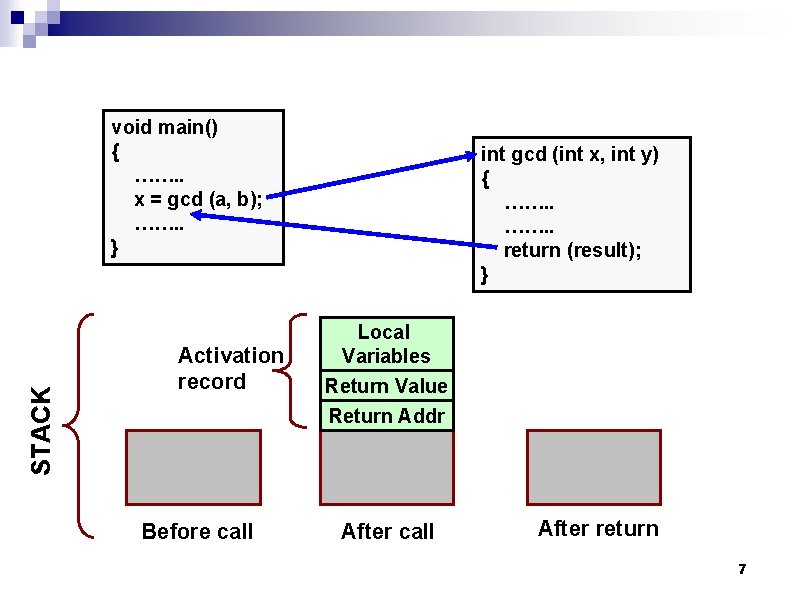
STACK void main() { ……. . x = gcd (a, b); ……. . } Activation record Before call int gcd (int x, int y) { ……. . return (result); } Local Variables Return Value Return Addr After call After return 7
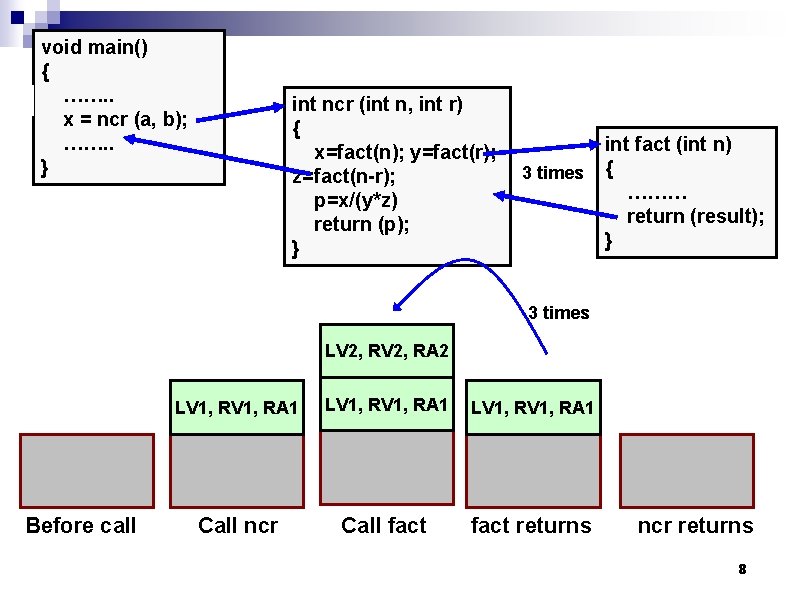
void main() { ……. . x = ncr (a, b); ……. . } int ncr (int n, int r) { x=fact(n); y=fact(r); z=fact(n-r); p=x/(y*z) return (p); } 3 times int fact (int n) { ……… return (result); } 3 times LV 2, RA 2 Before call LV 1, RV 1, RA 1 LV 1, RA 1 Call ncr Call fact returns ncr returns 8
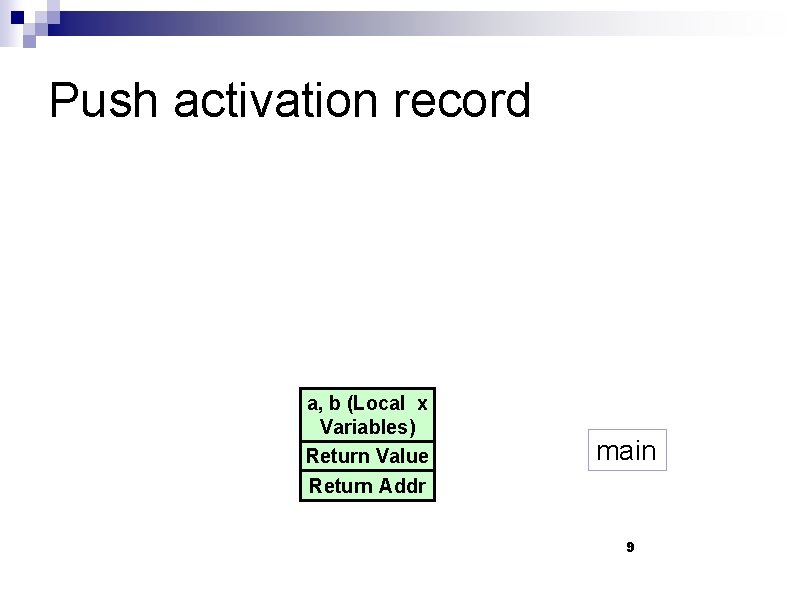
Push activation record a, b (Local x Variables) Return Value main Return Addr 9
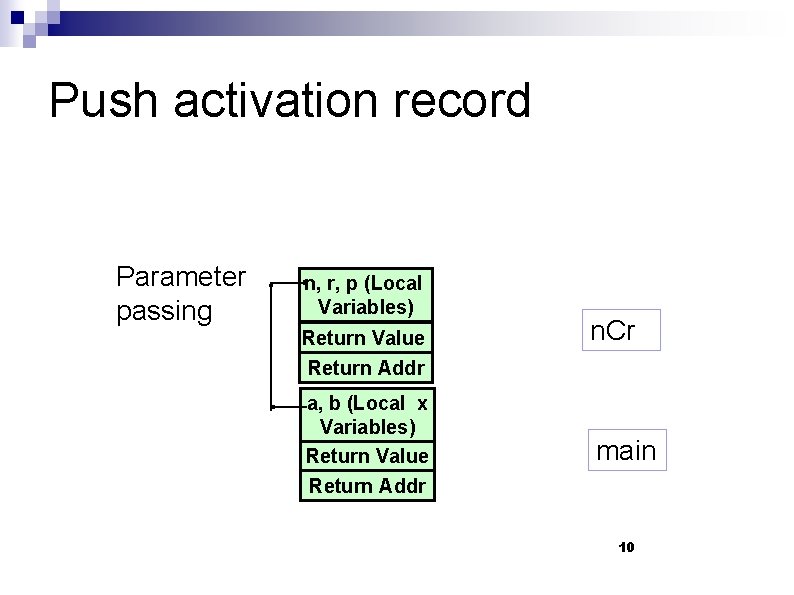
Push activation record Parameter passing n, r, p (Local Variables) Return Value Return Addr a, b (Local x Variables) Return Value n. Cr main Return Addr 10
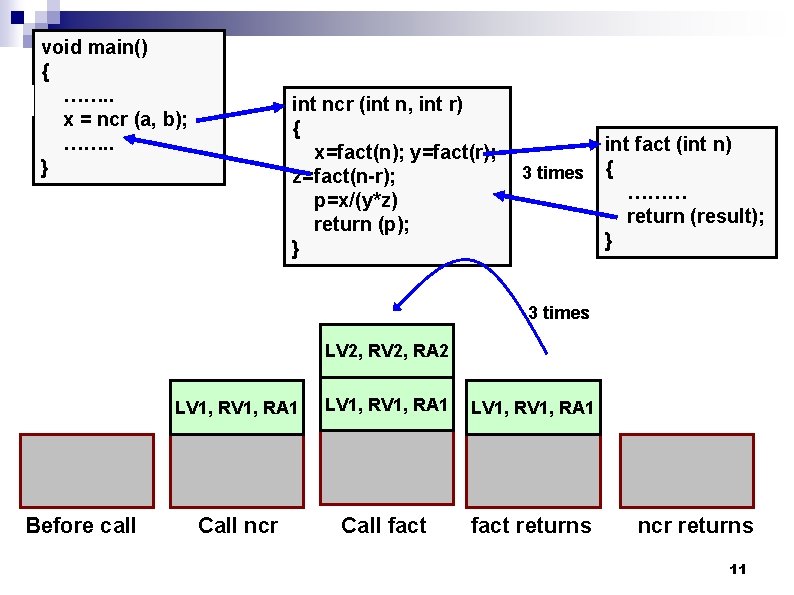
void main() { ……. . x = ncr (a, b); ……. . } int ncr (int n, int r) { x=fact(n); y=fact(r); z=fact(n-r); p=x/(y*z) return (p); } 3 times int fact (int n) { ……… return (result); } 3 times LV 2, RA 2 Before call LV 1, RV 1, RA 1 LV 1, RA 1 Call ncr Call fact returns ncr returns 11
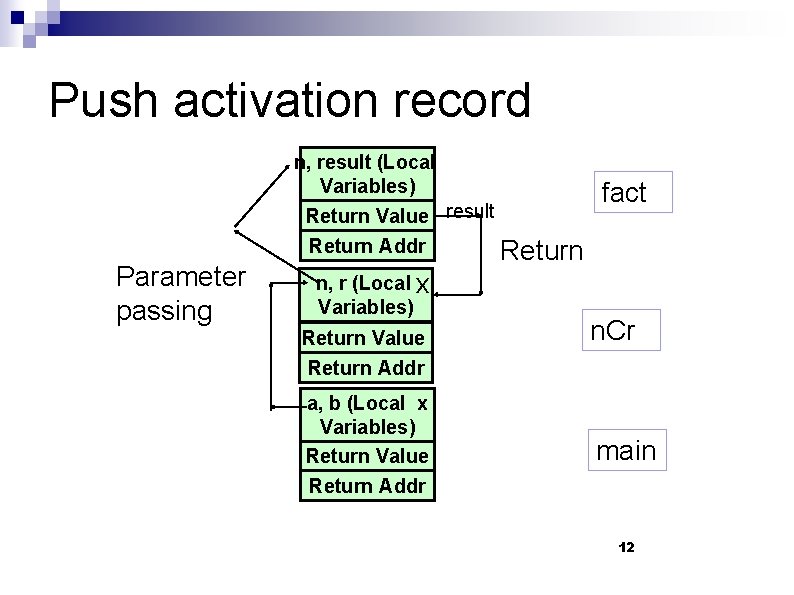
Push activation record n, result (Local Variables) Return Value result Return Addr Parameter passing n, r (Local x Variables) Return Value Return Addr a, b (Local x Variables) Return Value fact Return n. Cr main Return Addr 12
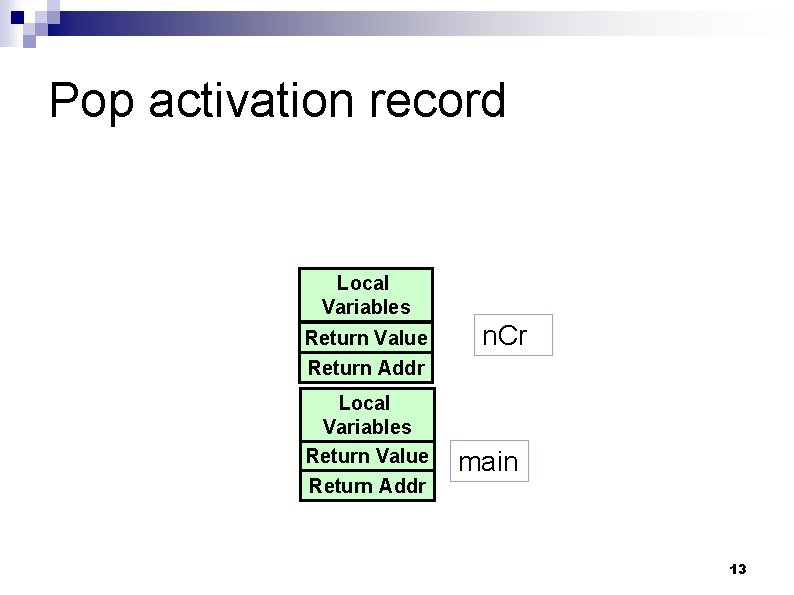
Pop activation record Local Variables Return Value Return Addr n. Cr main 13
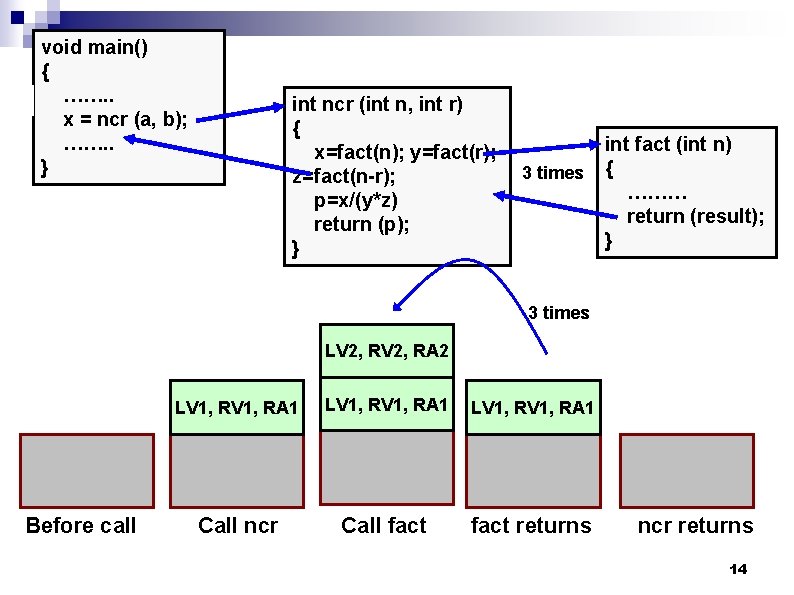
void main() { ……. . x = ncr (a, b); ……. . } int ncr (int n, int r) { x=fact(n); y=fact(r); z=fact(n-r); p=x/(y*z) return (p); } 3 times int fact (int n) { ……… return (result); } 3 times LV 2, RA 2 Before call LV 1, RV 1, RA 1 LV 1, RA 1 Call ncr Call fact returns ncr returns 14
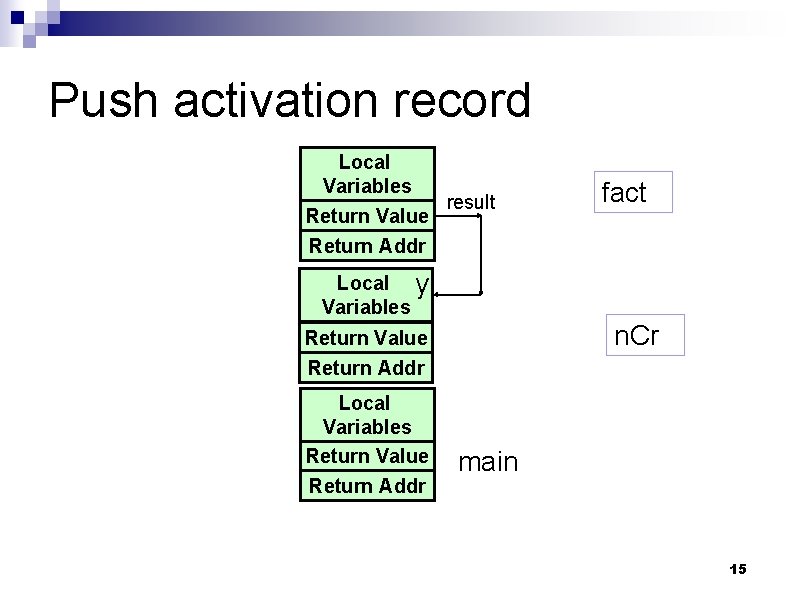
Push activation record Local Variables result Return Value fact Return Addr Local y Variables n. Cr Return Value Return Addr Local Variables Return Value Return Addr main 15
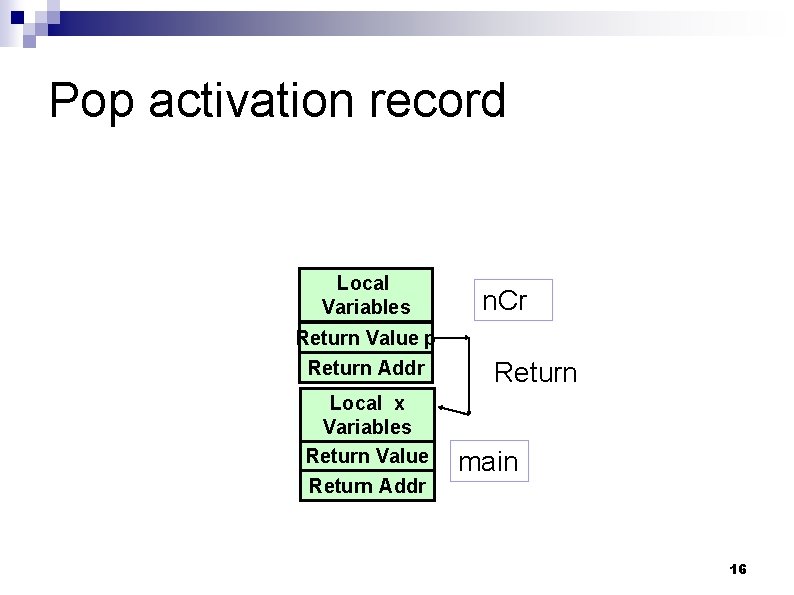
Pop activation record Local Variables Return Value p Return Addr Local x Variables Return Value Return Addr n. Cr Return main 16
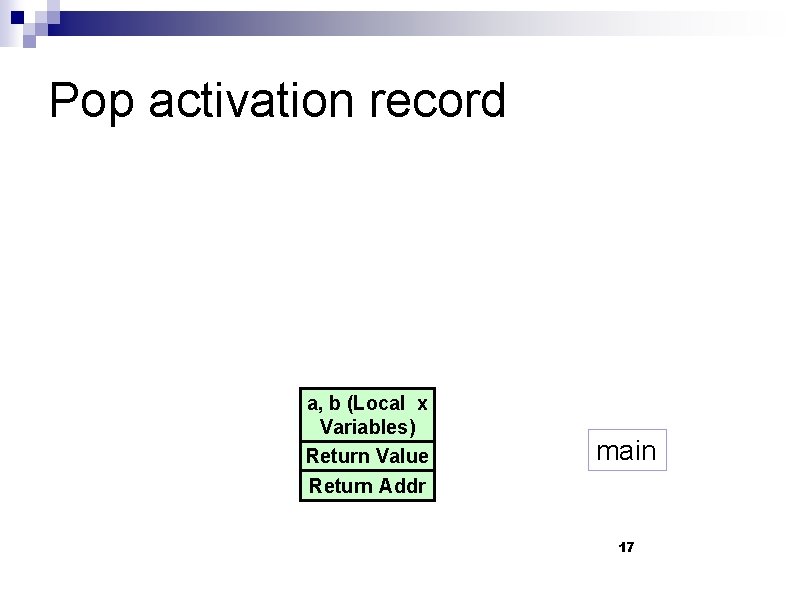
Pop activation record a, b (Local x Variables) Return Value main Return Addr 17
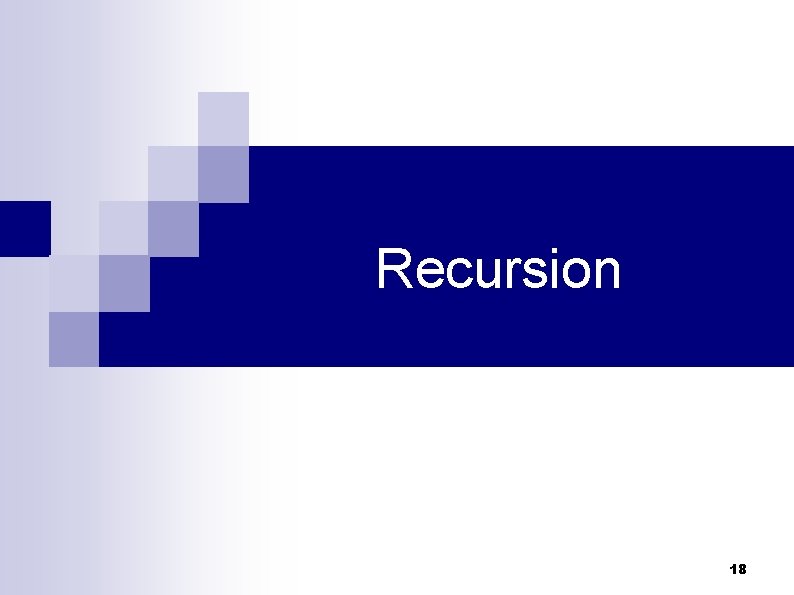
Recursion 18
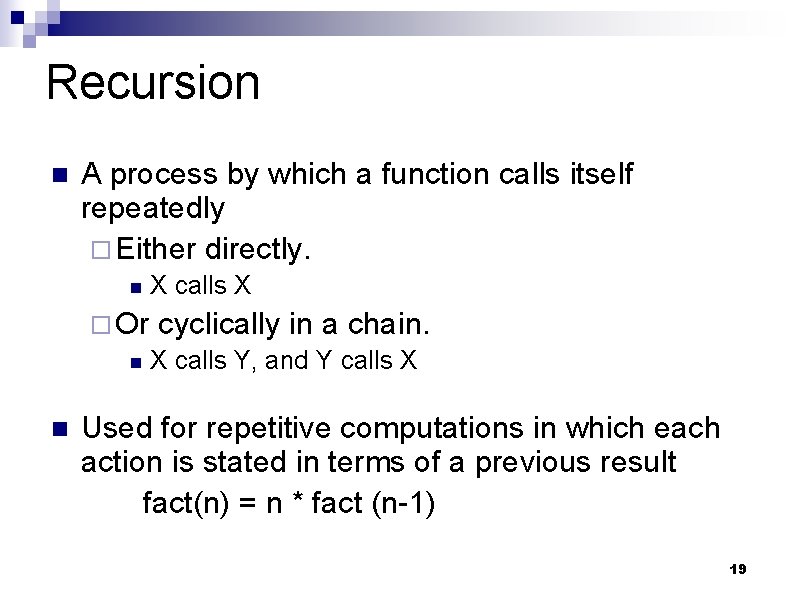
Recursion n A process by which a function calls itself repeatedly ¨ Either directly. n ¨ Or n n X calls X cyclically in a chain. X calls Y, and Y calls X Used for repetitive computations in which each action is stated in terms of a previous result fact(n) = n * fact (n-1) 19
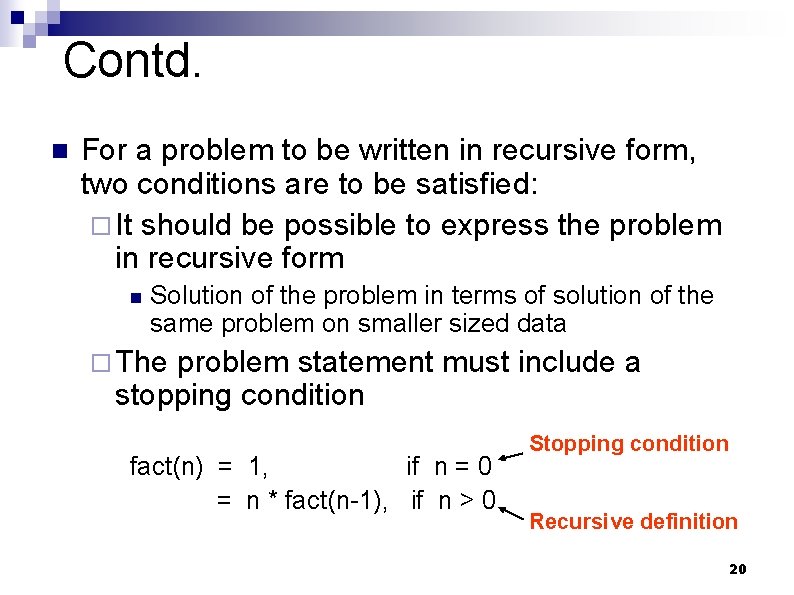
Contd. n For a problem to be written in recursive form, two conditions are to be satisfied: ¨ It should be possible to express the problem in recursive form n Solution of the problem in terms of solution of the same problem on smaller sized data ¨ The problem statement must include a stopping condition fact(n) = 1, if n = 0 = n * fact(n-1), if n > 0 Stopping condition Recursive definition 20
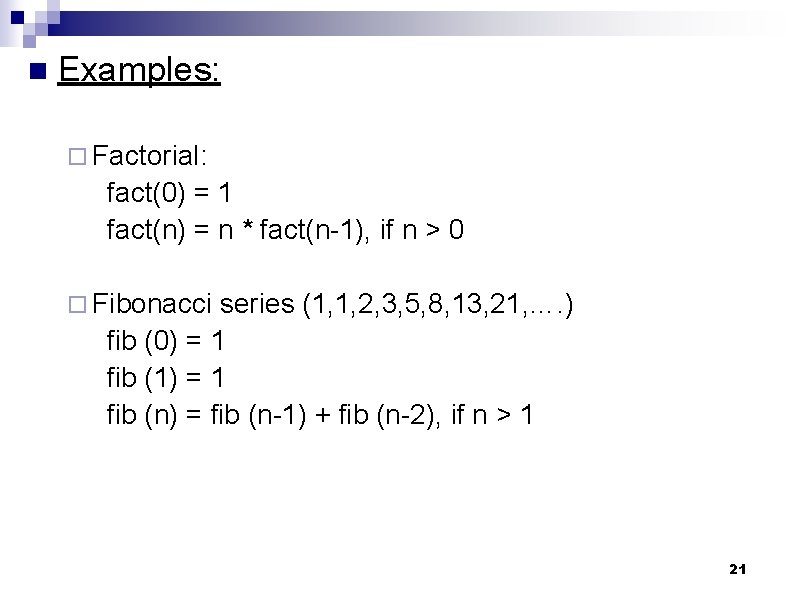
n Examples: ¨ Factorial: fact(0) = 1 fact(n) = n * fact(n-1), if n > 0 ¨ Fibonacci series (1, 1, 2, 3, 5, 8, 13, 21, …. ) fib (0) = 1 fib (1) = 1 fib (n) = fib (n-1) + fib (n-2), if n > 1 21
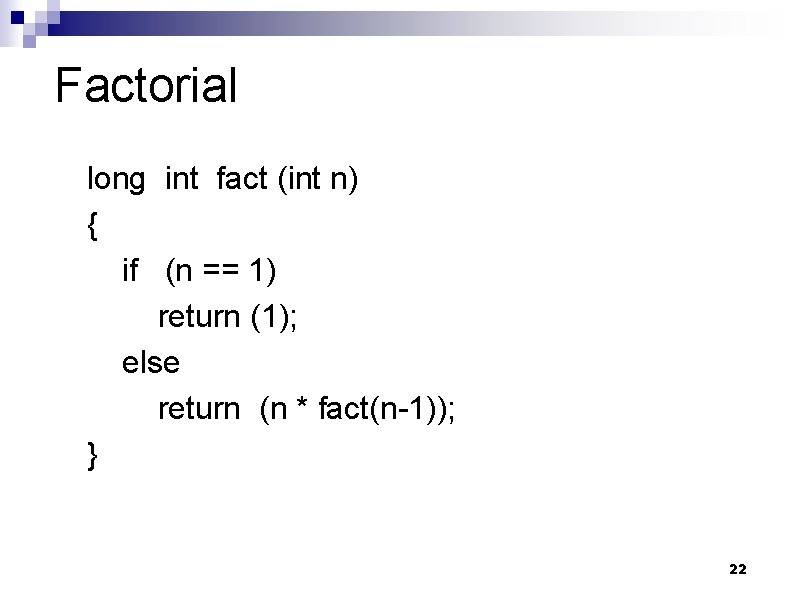
Factorial long int fact (int n) { if (n == 1) return (1); else return (n * fact(n-1)); } 22
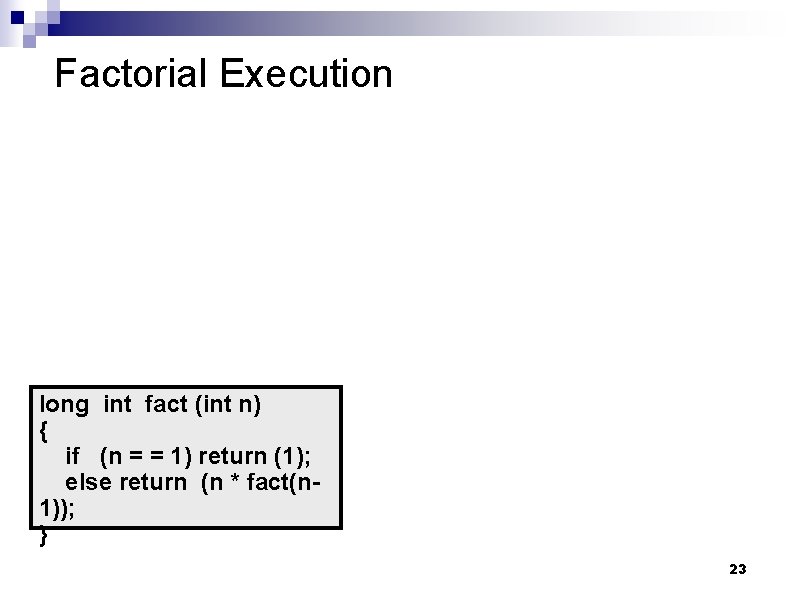
Factorial Execution long int fact (int n) { if (n = = 1) return (1); else return (n * fact(n 1)); } 23
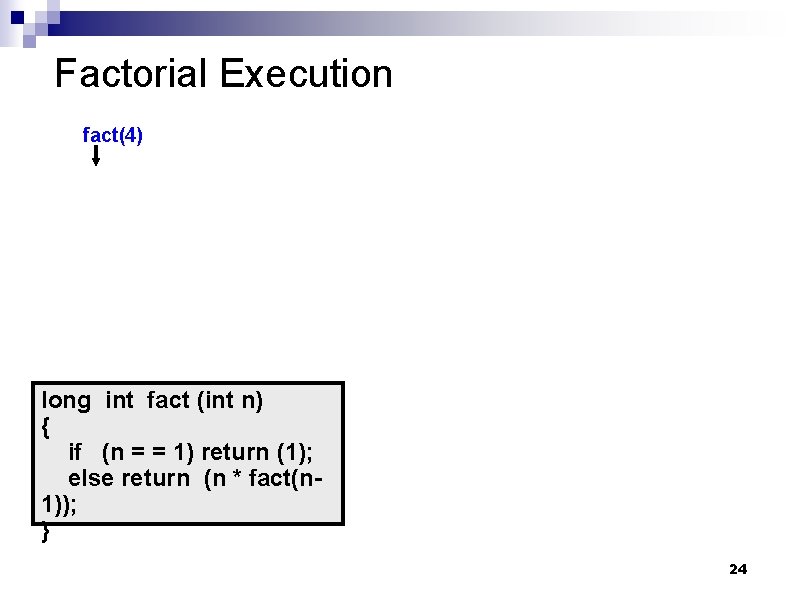
Factorial Execution fact(4) long int fact (int n) { if (n = = 1) return (1); else return (n * fact(n 1)); } 24
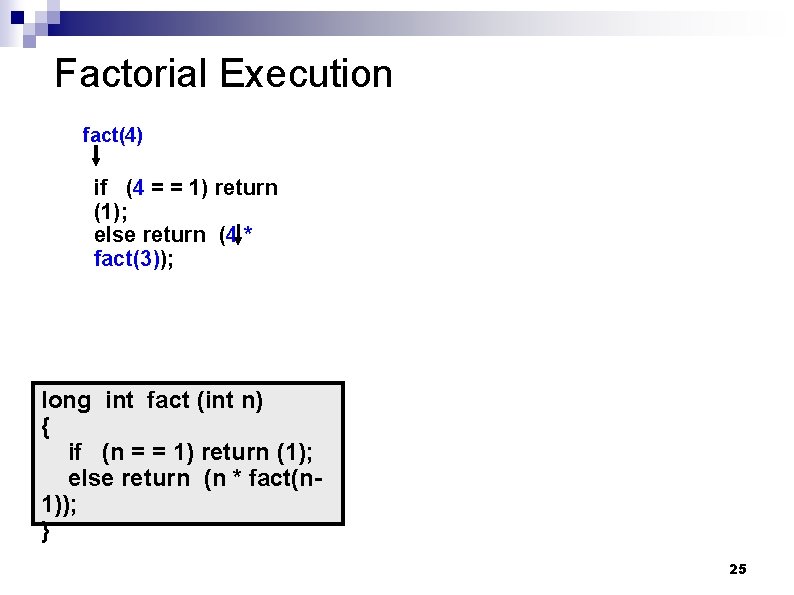
Factorial Execution fact(4) if (4 = = 1) return (1); else return (4 * fact(3)); long int fact (int n) { if (n = = 1) return (1); else return (n * fact(n 1)); } 25
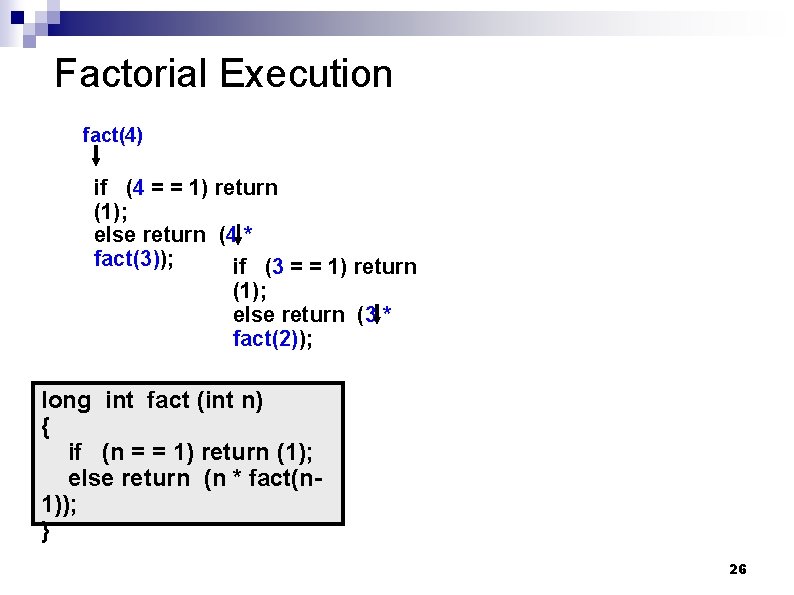
Factorial Execution fact(4) if (4 = = 1) return (1); else return (4 * fact(3)); if (3 = = 1) return (1); else return (3 * fact(2)); long int fact (int n) { if (n = = 1) return (1); else return (n * fact(n 1)); } 26
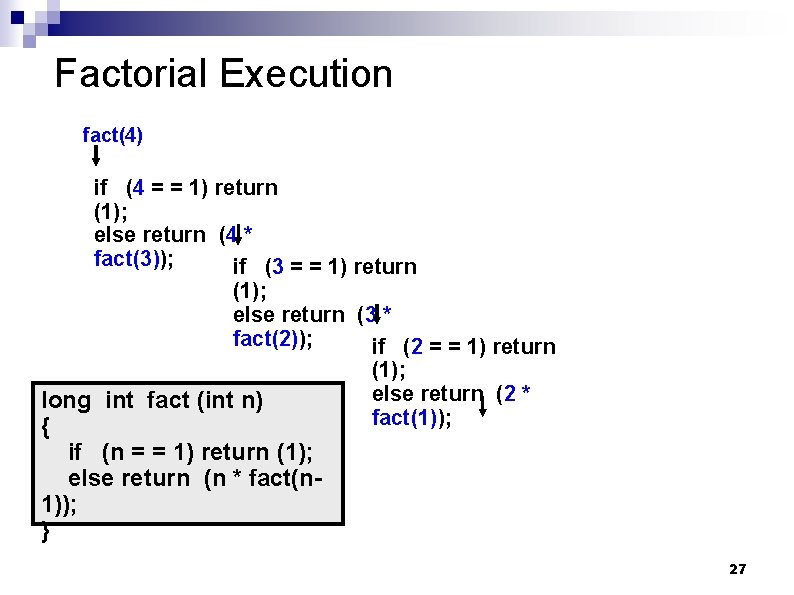
Factorial Execution fact(4) if (4 = = 1) return (1); else return (4 * fact(3)); if (3 = = 1) return (1); else return (3 * fact(2)); if (2 = = 1) return (1); else return (2 * long int fact (int n) fact(1)); { if (n = = 1) return (1); else return (n * fact(n 1)); } 27
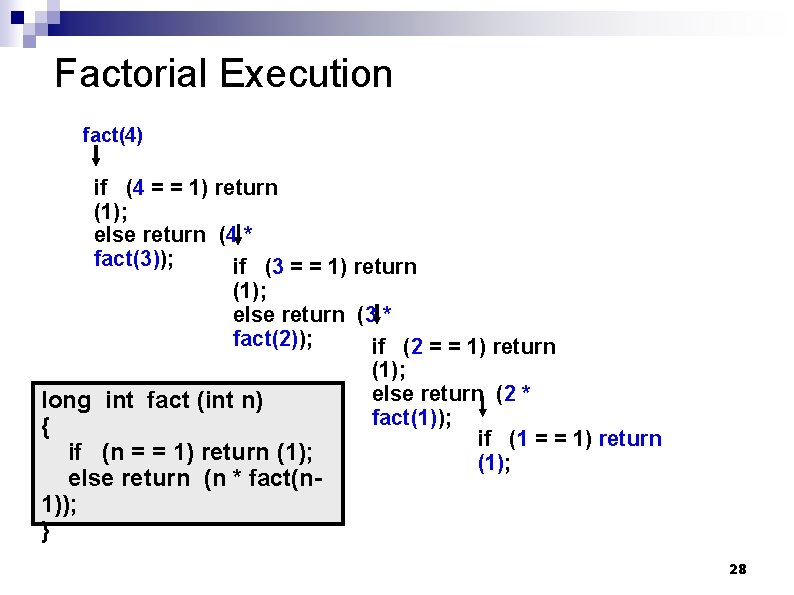
Factorial Execution fact(4) if (4 = = 1) return (1); else return (4 * fact(3)); if (3 = = 1) return (1); else return (3 * fact(2)); if (2 = = 1) return (1); else return (2 * long int fact (int n) fact(1)); { if (1 = = 1) return if (n = = 1) return (1); else return (n * fact(n 1)); } 28
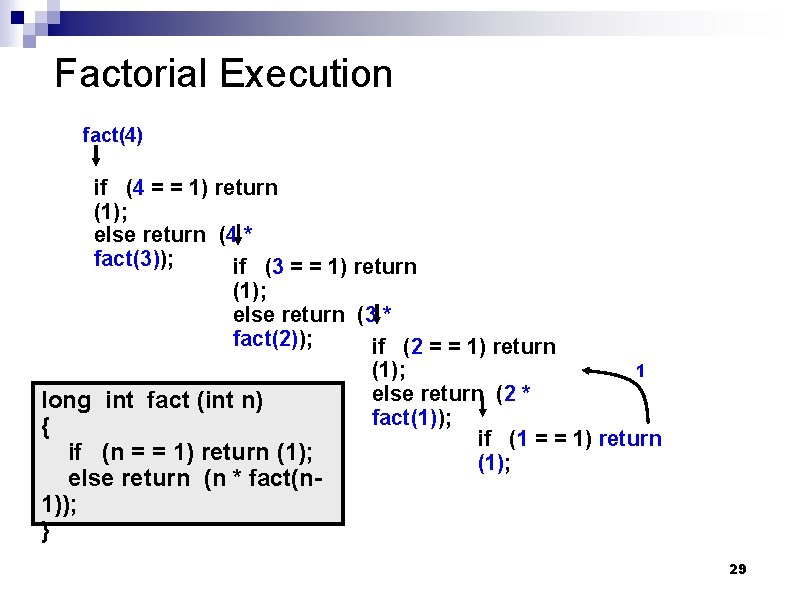
Factorial Execution fact(4) if (4 = = 1) return (1); else return (4 * fact(3)); if (3 = = 1) return (1); else return (3 * fact(2)); if (2 = = 1) return 1 (1); else return (2 * long int fact (int n) fact(1)); { if (1 = = 1) return if (n = = 1) return (1); else return (n * fact(n 1)); } 29
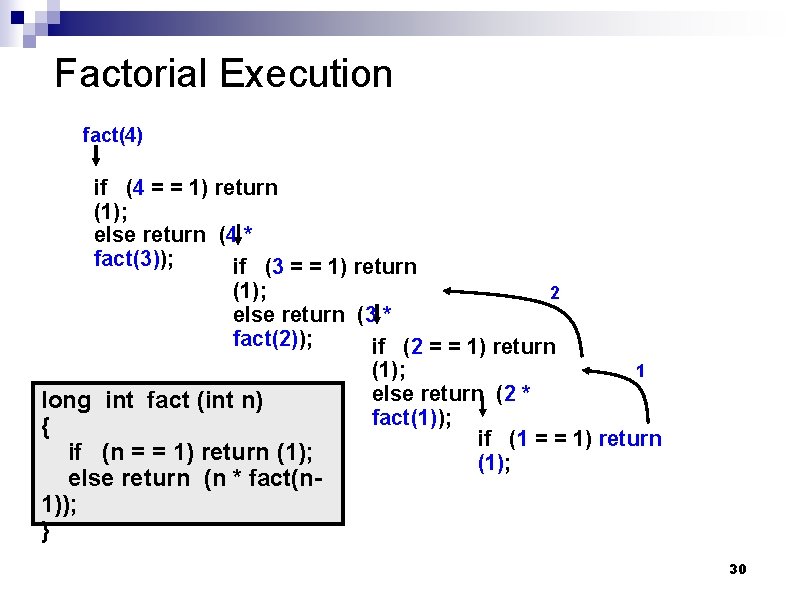
Factorial Execution fact(4) if (4 = = 1) return (1); else return (4 * fact(3)); if (3 = = 1) return (1); 2 else return (3 * fact(2)); if (2 = = 1) return 1 (1); else return (2 * long int fact (int n) fact(1)); { if (1 = = 1) return if (n = = 1) return (1); else return (n * fact(n 1)); } 30
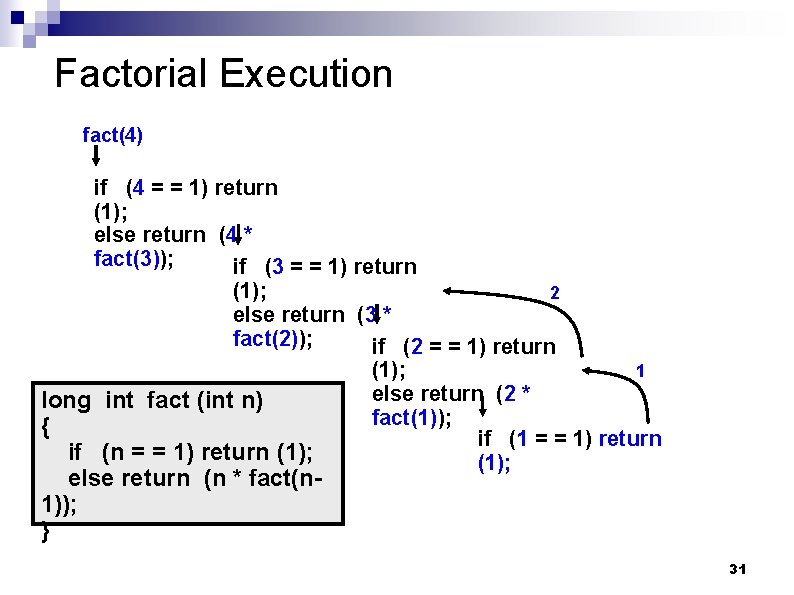
Factorial Execution fact(4) if (4 = = 1) return (1); else return (4 * fact(3)); if (3 = = 1) return (1); 2 else return (3 * fact(2)); if (2 = = 1) return 1 (1); else return (2 * long int fact (int n) fact(1)); { if (1 = = 1) return if (n = = 1) return (1); else return (n * fact(n 1)); } 31
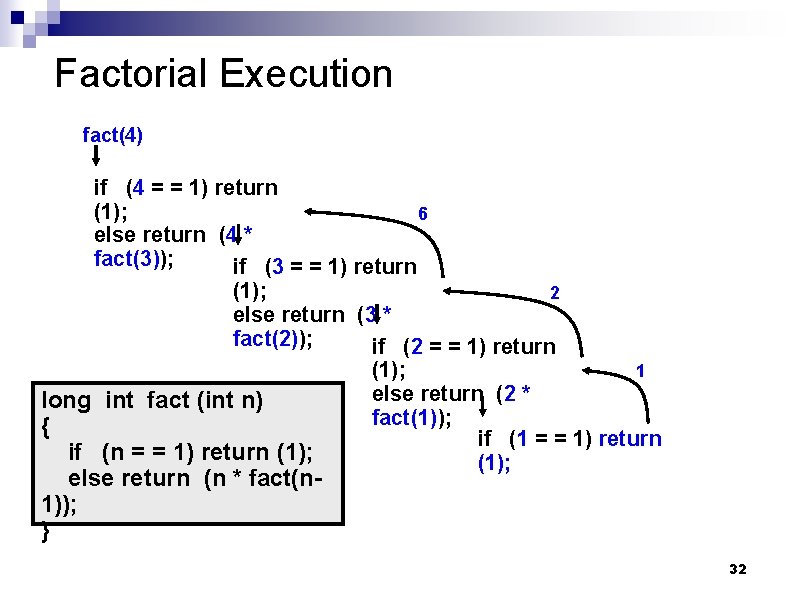
Factorial Execution fact(4) if (4 = = 1) return (1); 6 else return (4 * fact(3)); if (3 = = 1) return (1); 2 else return (3 * fact(2)); if (2 = = 1) return 1 (1); else return (2 * long int fact (int n) fact(1)); { if (1 = = 1) return if (n = = 1) return (1); else return (n * fact(n 1)); } 32
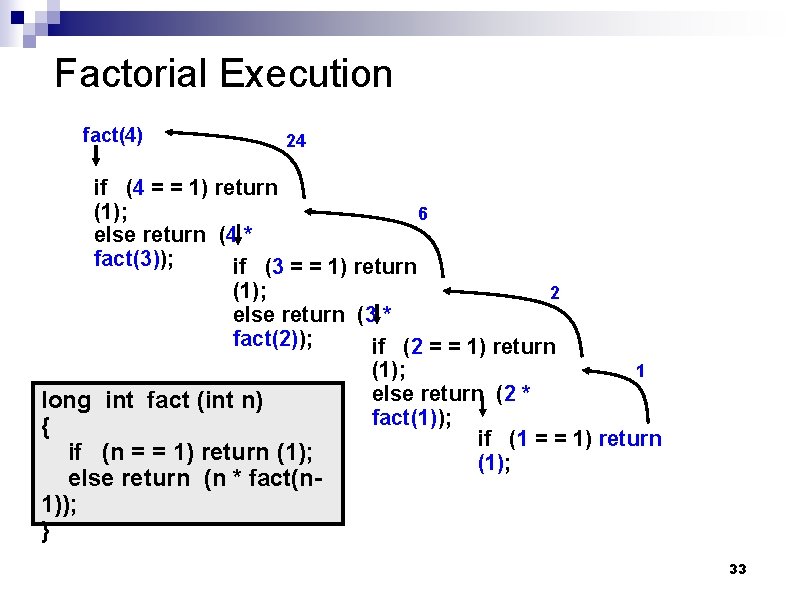
Factorial Execution fact(4) 24 if (4 = = 1) return (1); 6 else return (4 * fact(3)); if (3 = = 1) return (1); 2 else return (3 * fact(2)); if (2 = = 1) return 1 (1); else return (2 * long int fact (int n) fact(1)); { if (1 = = 1) return if (n = = 1) return (1); else return (n * fact(n 1)); } 33
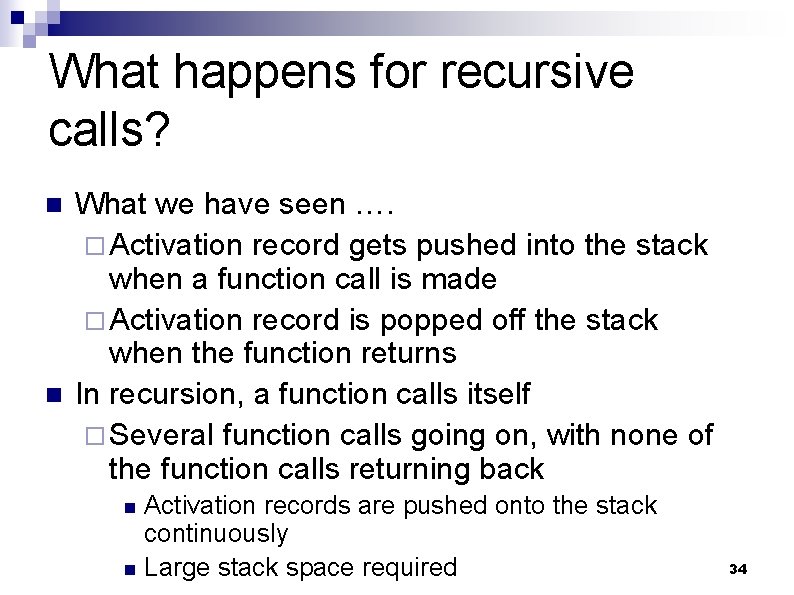
What happens for recursive calls? n n What we have seen …. ¨ Activation record gets pushed into the stack when a function call is made ¨ Activation record is popped off the stack when the function returns In recursion, a function calls itself ¨ Several function calls going on, with none of the function calls returning back Activation records are pushed onto the stack continuously n Large stack space required n 34
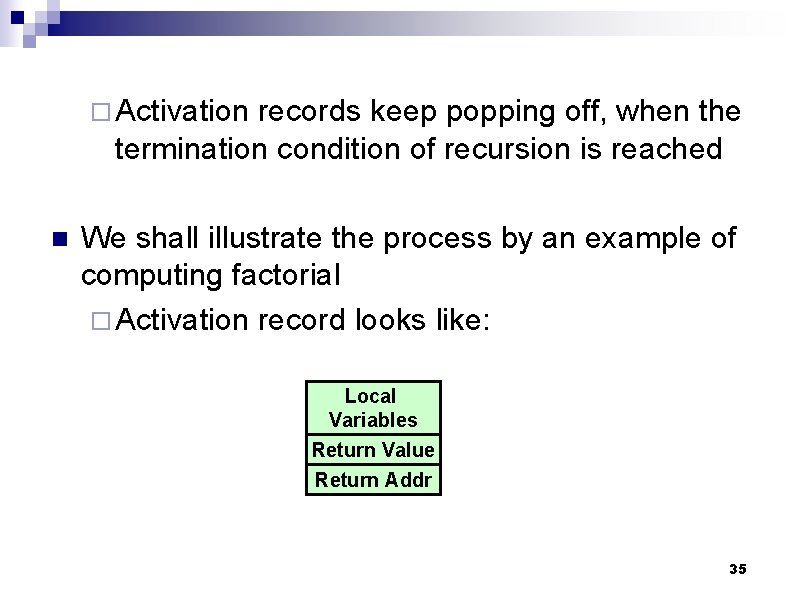
¨ Activation records keep popping off, when the termination condition of recursion is reached n We shall illustrate the process by an example of computing factorial ¨ Activation record looks like: Local Variables Return Value Return Addr 35
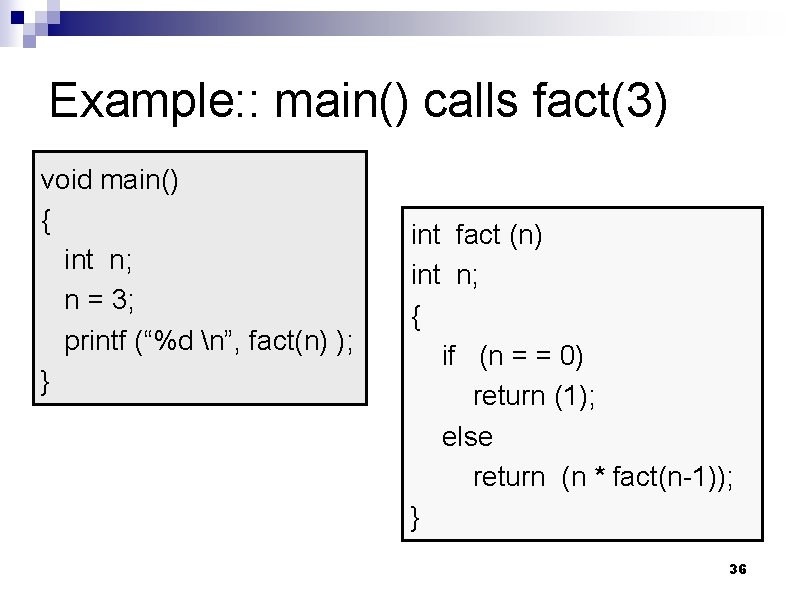
Example: : main() calls fact(3) void main() { int n; n = 3; printf (“%d n”, fact(n) ); } int fact (n) int n; { if (n = = 0) return (1); else return (n * fact(n-1)); } 36
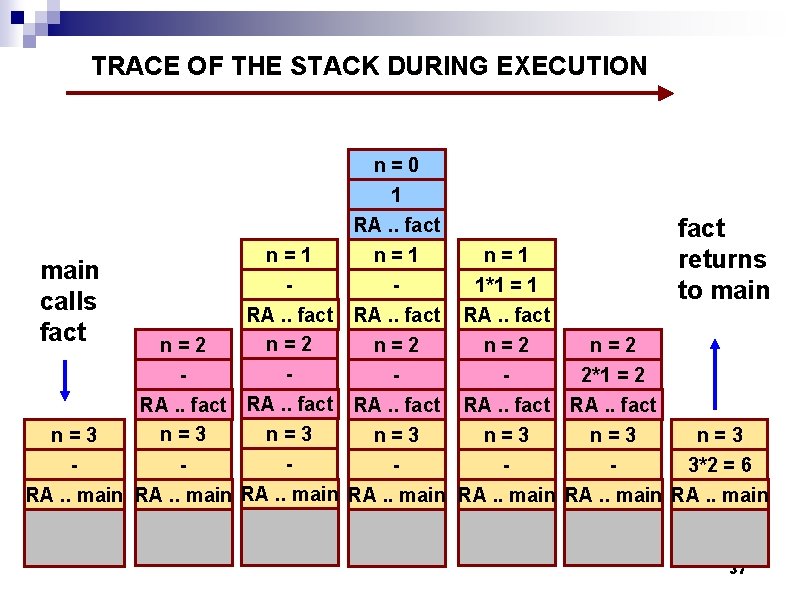
TRACE OF THE STACK DURING EXECUTION n=0 1 main calls fact n=1 n=2 RA. . fact n=1 RA. . fact n=2 RA. . fact returns to main n=1 1*1 = 1 RA. . fact n=2 2*1 = 2 RA. . fact n=3 n=3 3*2 = 6 RA. . main RA. . main 37
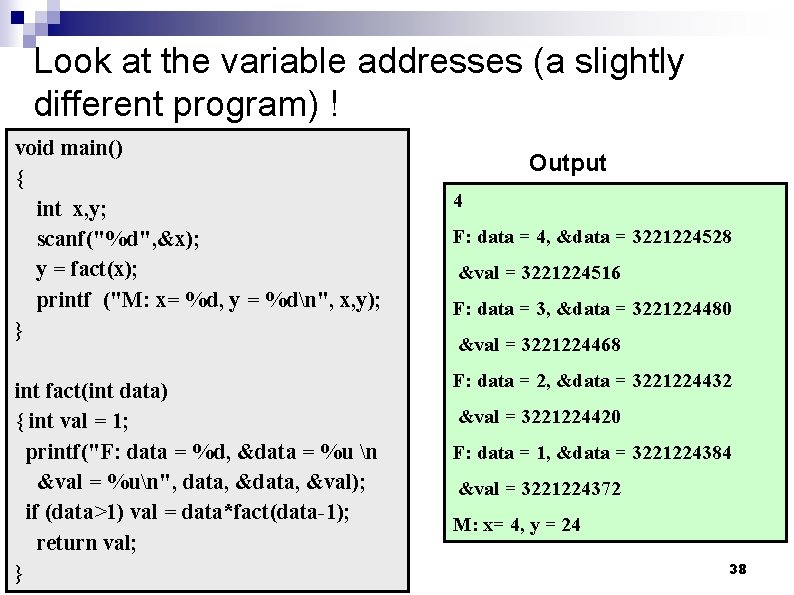
Look at the variable addresses (a slightly different program) ! void main() { int x, y; scanf("%d", &x); y = fact(x); printf ("M: x= %d, y = %dn", x, y); } int fact(int data) { int val = 1; printf("F: data = %d, &data = %u n &val = %un", data, &val); if (data>1) val = data*fact(data-1); return val; } Output 4 F: data = 4, &data = 3221224528 &val = 3221224516 F: data = 3, &data = 3221224480 &val = 3221224468 F: data = 2, &data = 3221224432 &val = 3221224420 F: data = 1, &data = 3221224384 &val = 3221224372 M: x= 4, y = 24 38
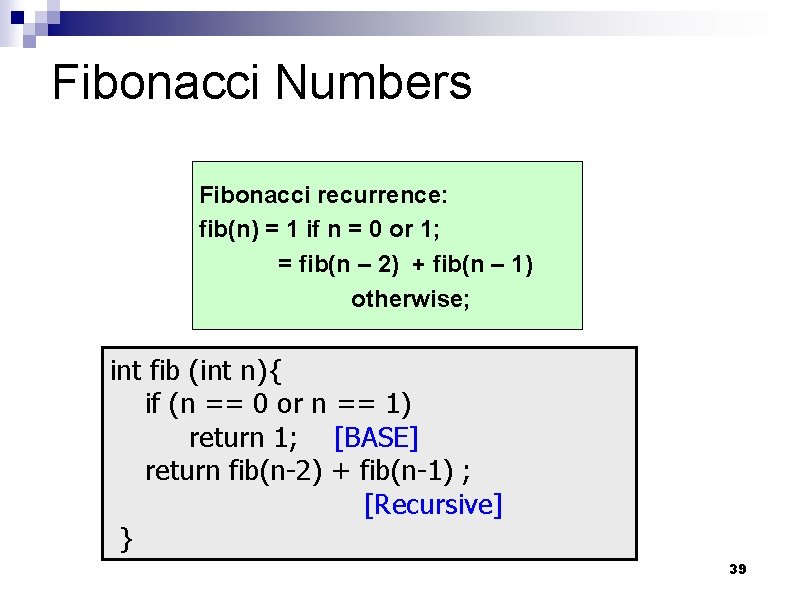
Fibonacci Numbers Fibonacci recurrence: fib(n) = 1 if n = 0 or 1; = fib(n – 2) + fib(n – 1) otherwise; int fib (int n){ if (n == 0 or n == 1) return 1; [BASE] return fib(n-2) + fib(n-1) ; [Recursive] } 39
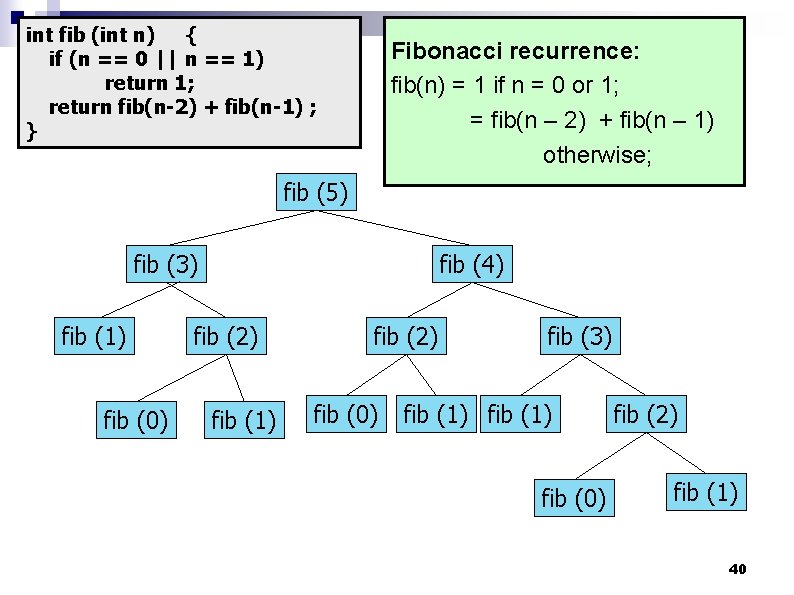
int fib (int n) { if (n == 0 || n == 1) return 1; return fib(n-2) + fib(n-1) ; } Fibonacci recurrence: fib(n) = 1 if n = 0 or 1; = fib(n – 2) + fib(n – 1) otherwise; fib (5) fib (3) fib (1) fib (0) fib (4) fib (2) fib (1) fib (2) fib (0) fib (3) fib (1) fib (0) fib (2) fib (1) 40
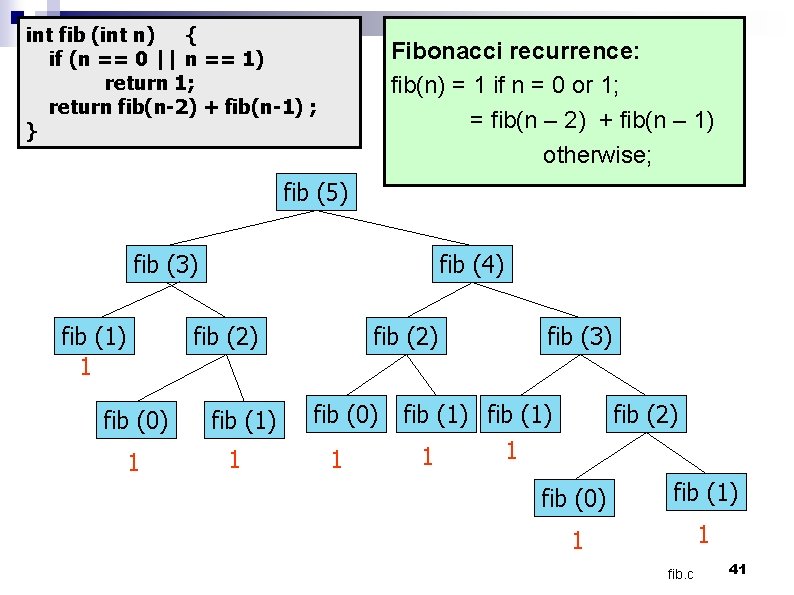
int fib (int n) { if (n == 0 || n == 1) return 1; return fib(n-2) + fib(n-1) ; } Fibonacci recurrence: fib(n) = 1 if n = 0 or 1; = fib(n – 2) + fib(n – 1) otherwise; fib (5) fib (3) fib (1) 1 fib (4) fib (2) fib (0) 1 fib (1) 1 fib (2) fib (0) 1 fib (3) fib (1) 1 1 fib (2) fib (0) fib (1) 1 1 fib. c 41
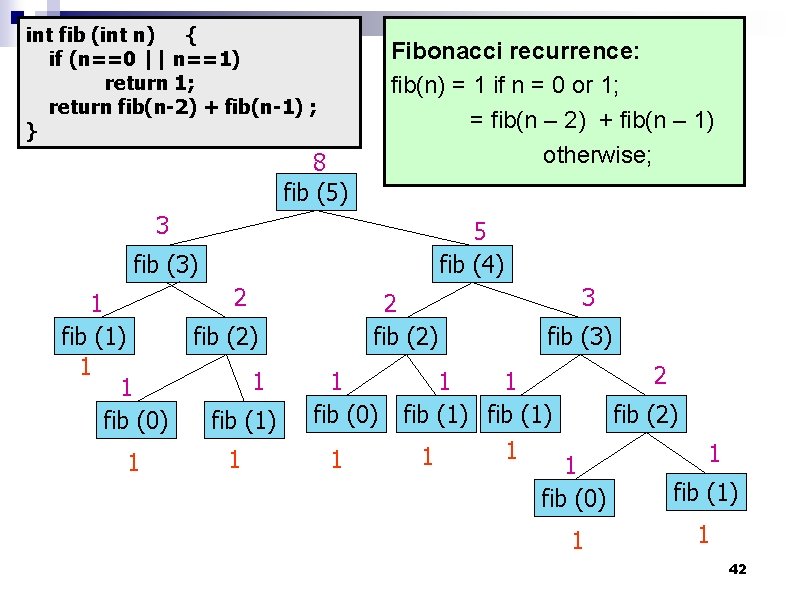
int fib (int n) { if (n==0 || n==1) return 1; return fib(n-2) + fib(n-1) ; } Fibonacci recurrence: fib(n) = 1 if n = 0 or 1; = fib(n – 2) + fib(n – 1) otherwise; 8 fib (5) 3 5 fib (4) fib (3) 1 fib (1) 1 1 fib (0) 1 2 2 fib (2) 1 fib (1) 1 1 fib (0) 1 3 fib (3) 2 1 1 fib (1) 1 1 fib (2) 1 1 fib (0) fib (1) 1 1 42
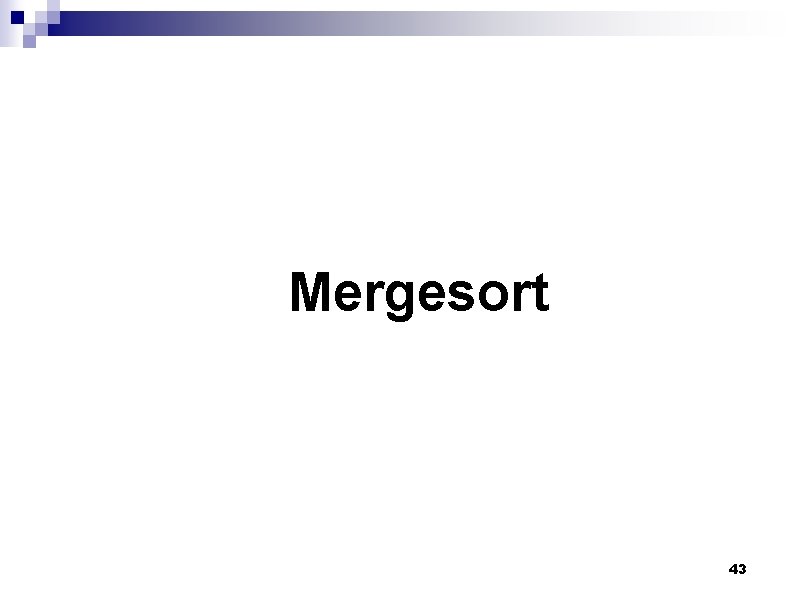
Mergesort 43
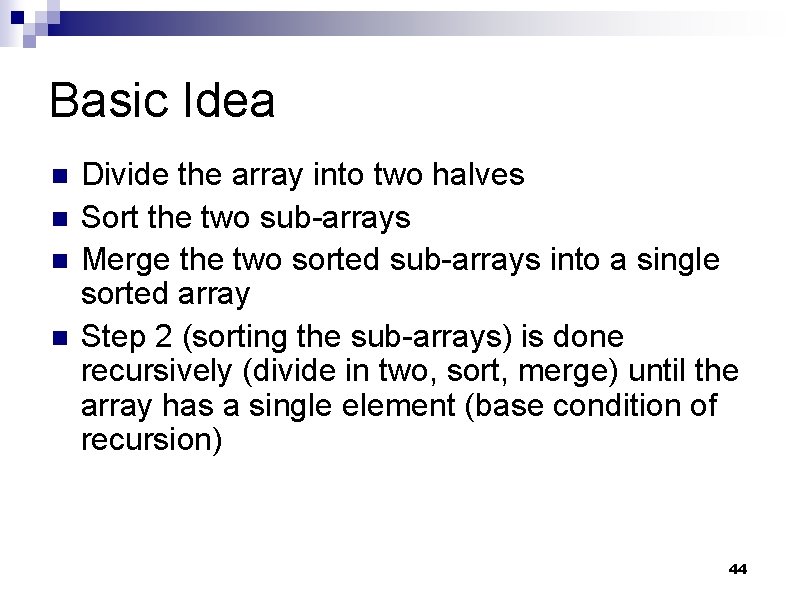
Basic Idea n n Divide the array into two halves Sort the two sub-arrays Merge the two sorted sub-arrays into a single sorted array Step 2 (sorting the sub-arrays) is done recursively (divide in two, sort, merge) until the array has a single element (base condition of recursion) 44
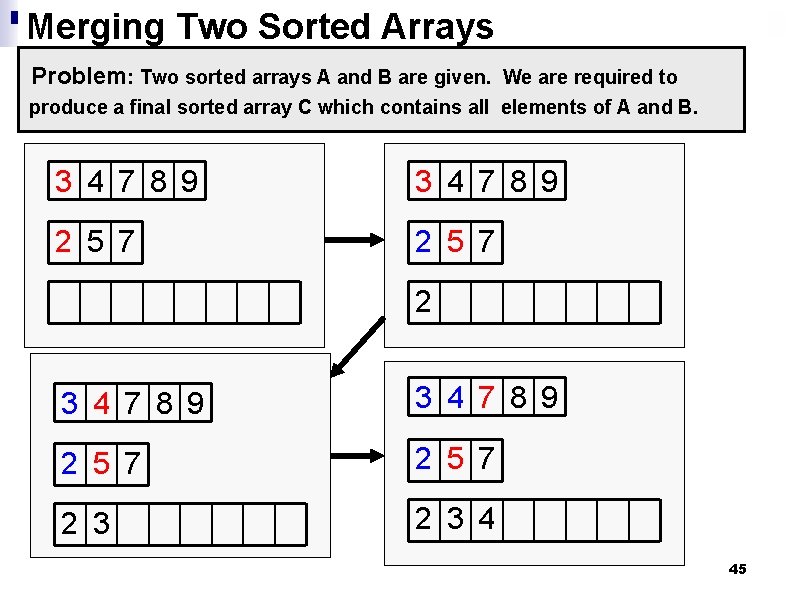
Merging Two Sorted Arrays Problem: Two sorted arrays A and B are given. We are required to produce a final sorted array C which contains all elements of A and B. 3 4 7 8 9 2 5 7 2 3 2 3 4 45
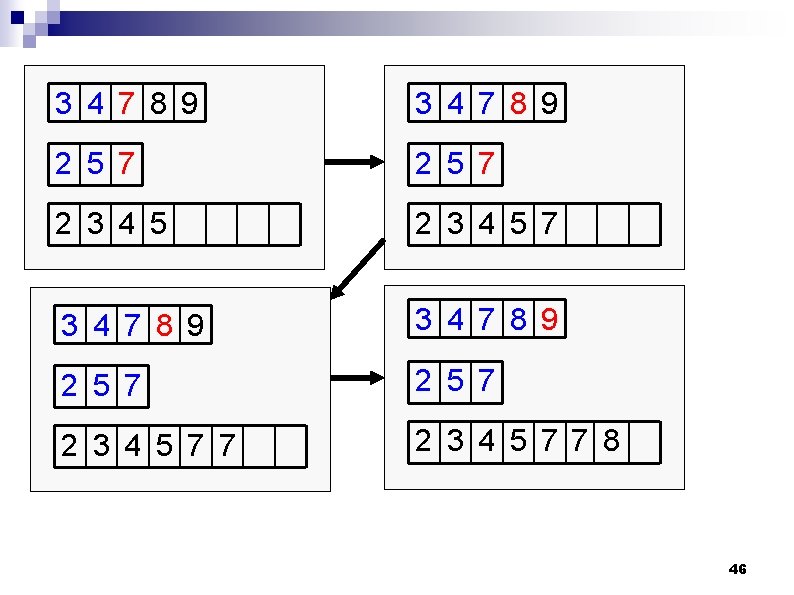
3 4 7 8 9 2 5 7 2 3 4 5 7 7 8 46
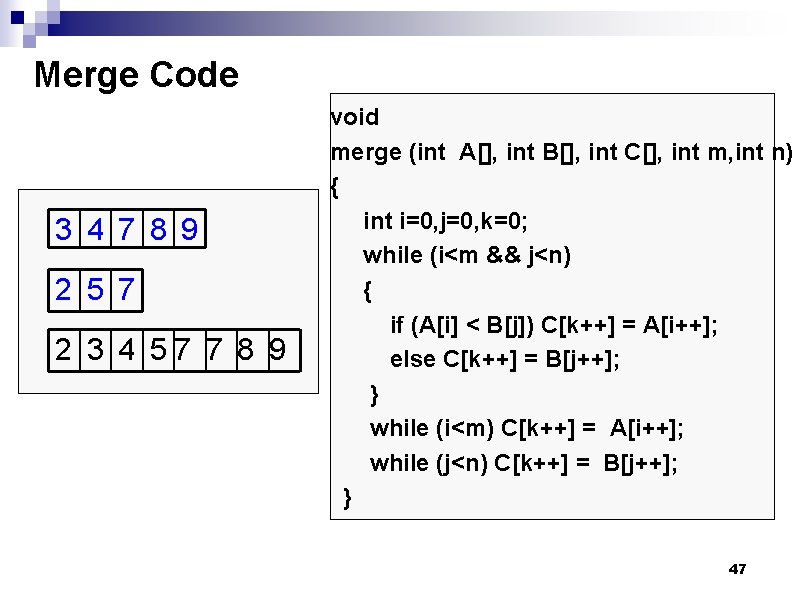
Merge Code 3 4 7 8 9 2 5 7 2 3 4 57 7 8 9 void merge (int A[], int B[], int C[], int m, int n) { int i=0, j=0, k=0; while (i<m && j<n) { if (A[i] < B[j]) C[k++] = A[i++]; else C[k++] = B[j++]; } while (i<m) C[k++] = A[i++]; while (j<n) C[k++] = B[j++]; } 47
![Merge Sort Sorting an array recursively void mergesort int A int n int Merge Sort: Sorting an array recursively void mergesort (int A[], int n) { int](https://slidetodoc.com/presentation_image_h2/6d9c5cd009e63c476a8fe01339ec99cd/image-48.jpg)
Merge Sort: Sorting an array recursively void mergesort (int A[], int n) { int i, j, B[max]; if (n <= 1) return; i = n/2; mergesort(A, i); mergesort(A+i, n-i); merge(A, A+i, B, i, n-i); for (j=0; j<n; j++) A[j] = B[j]; free(B); } 48