FUNCTIONS IN C Topics Introduction to userdefined function
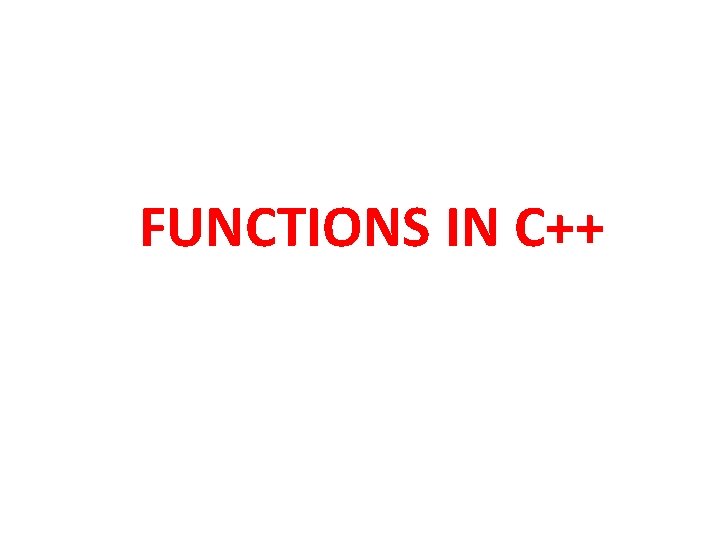
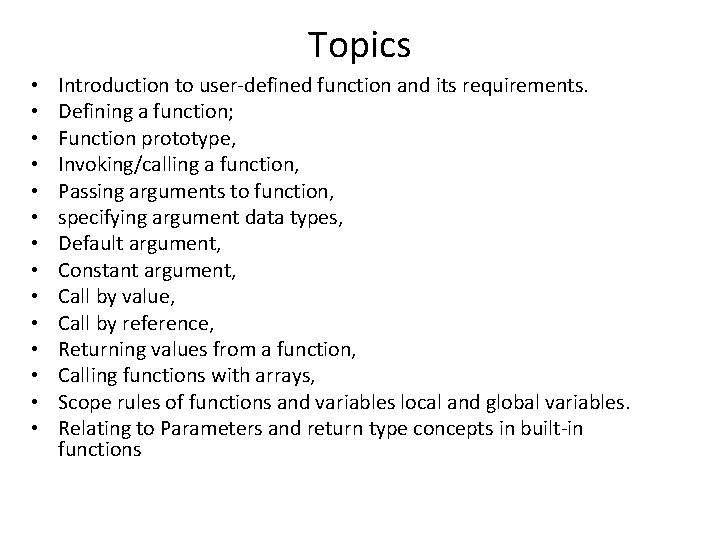
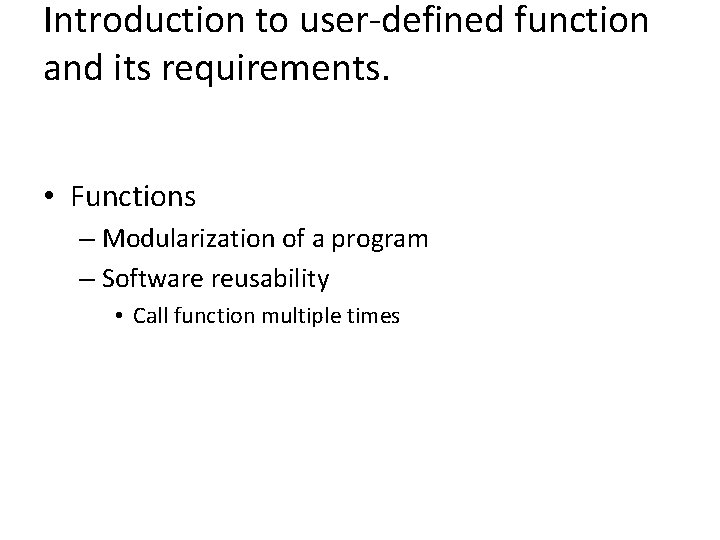
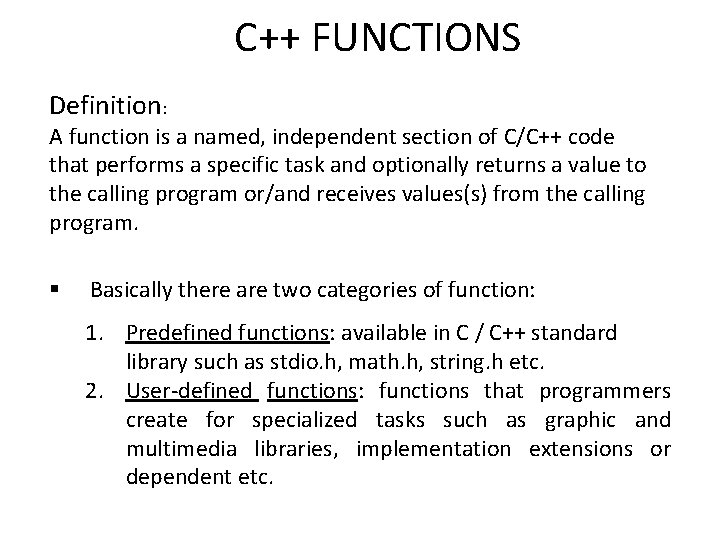
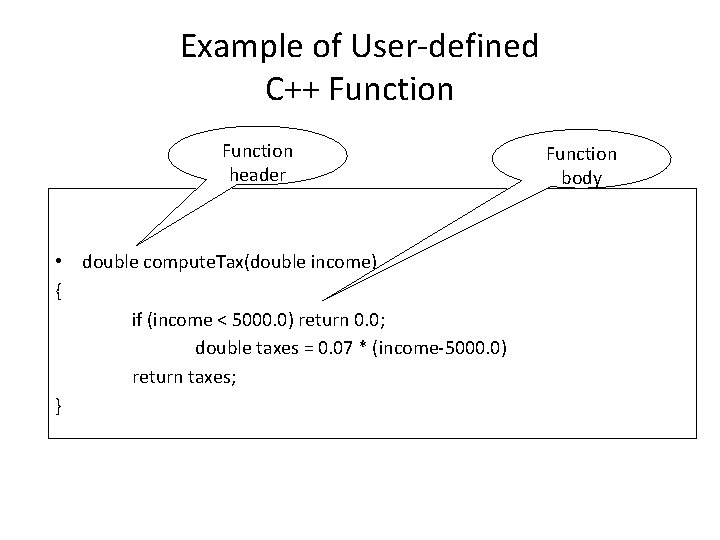
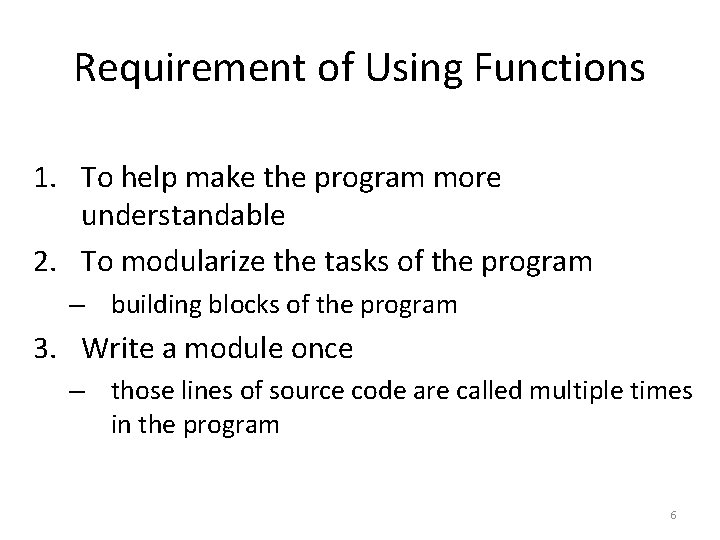
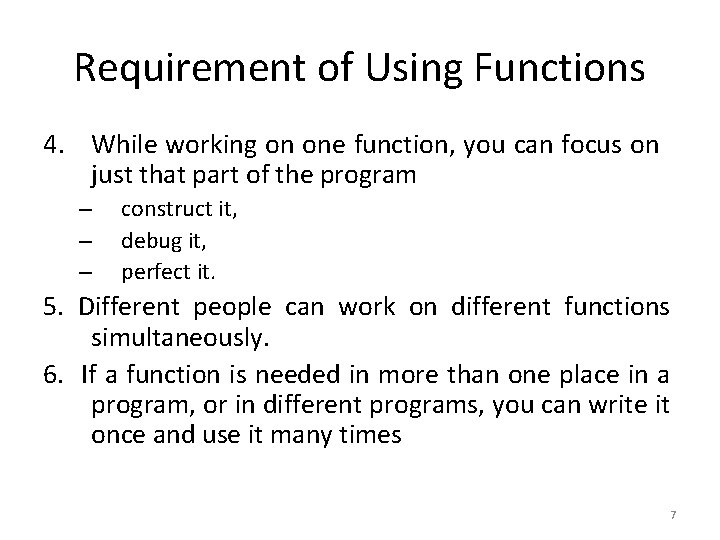
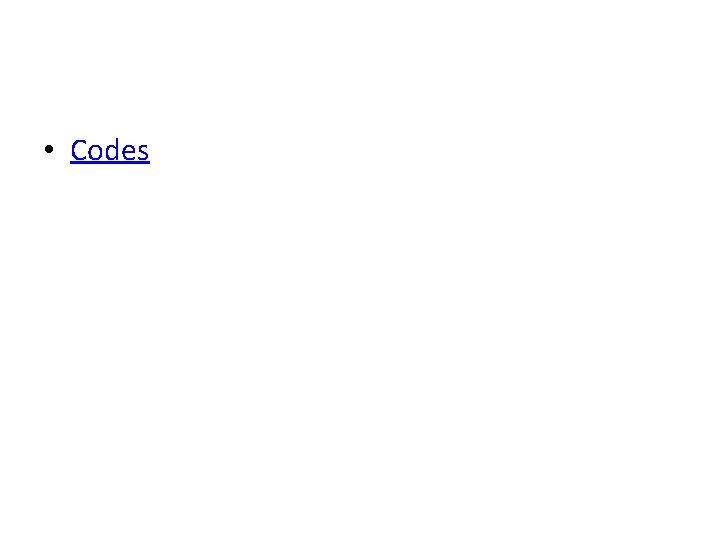
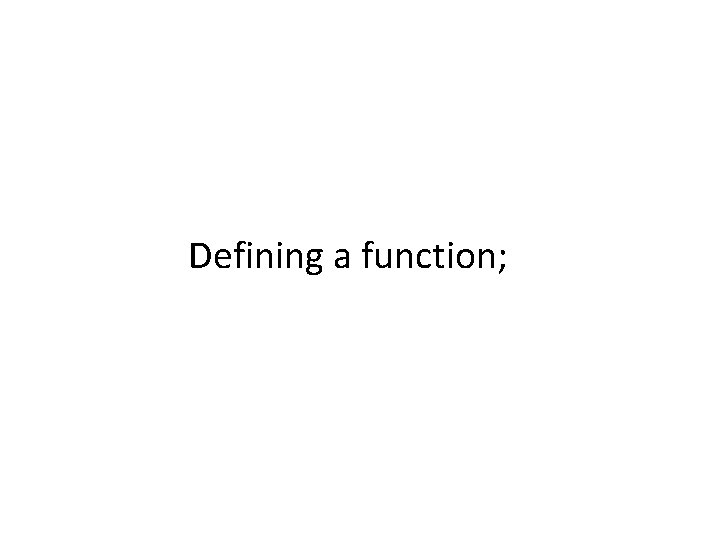
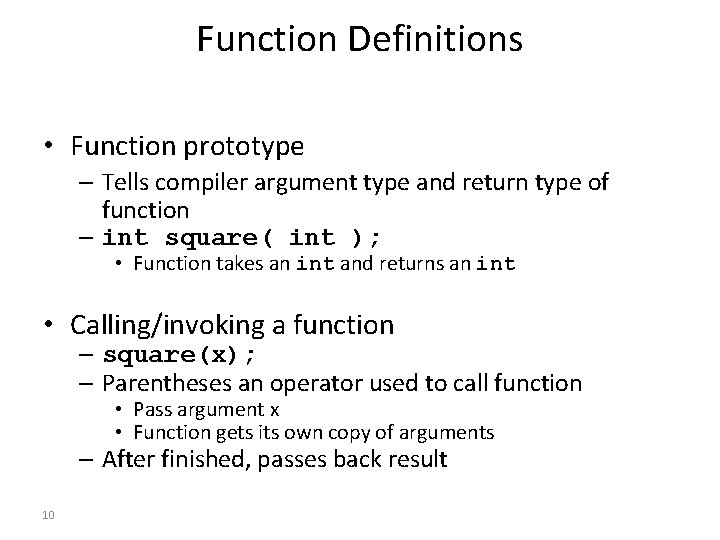
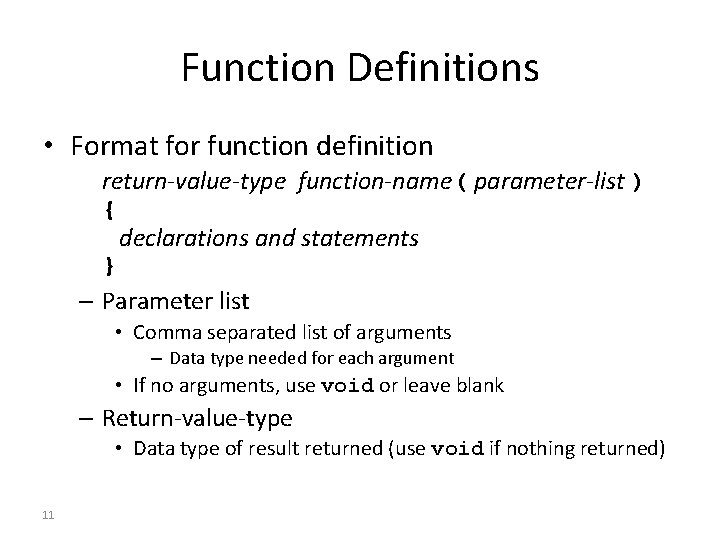
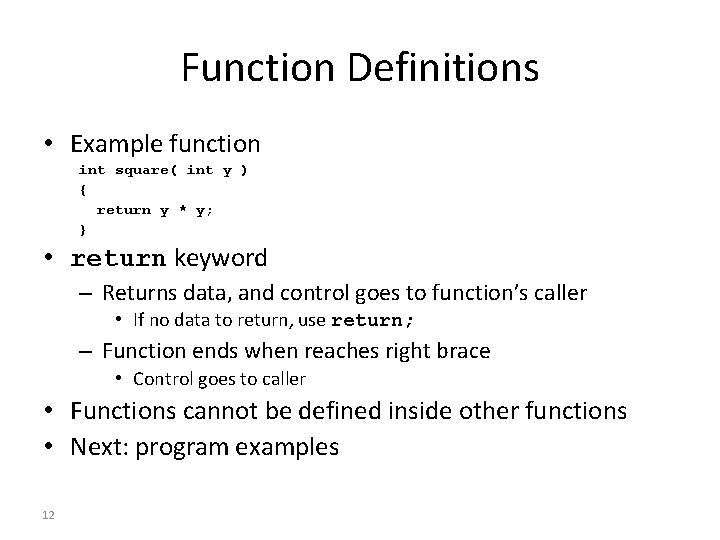
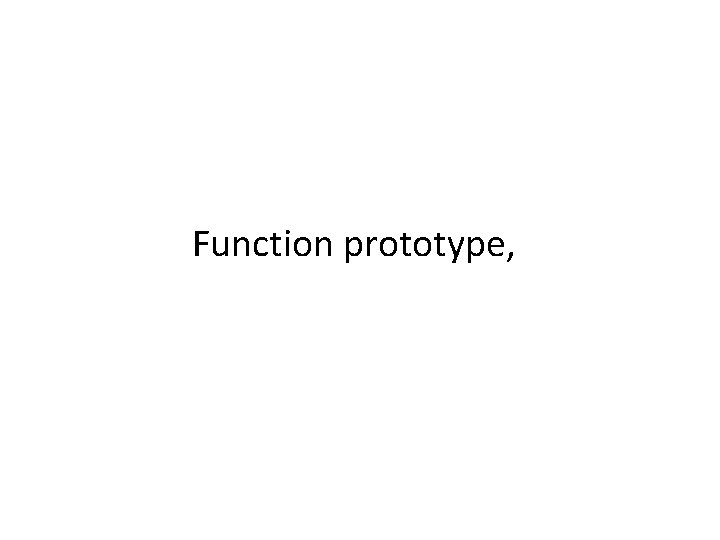
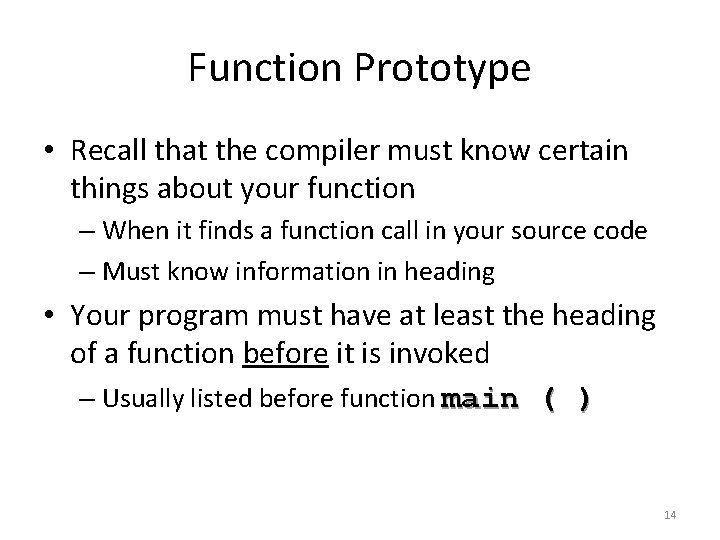
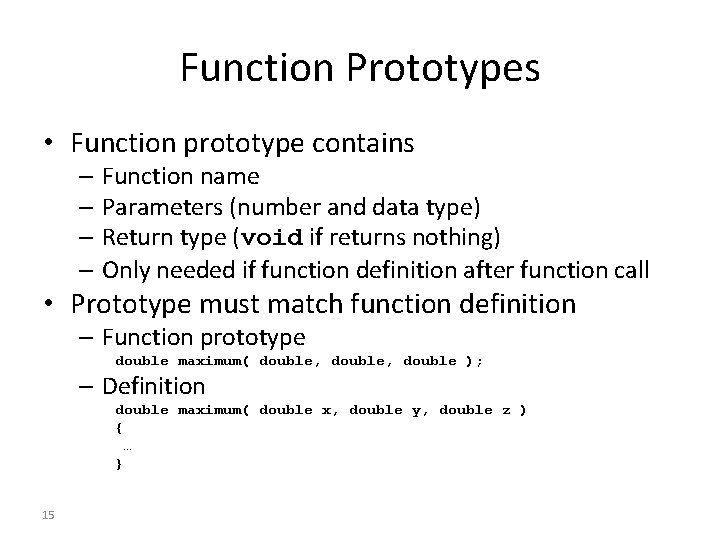
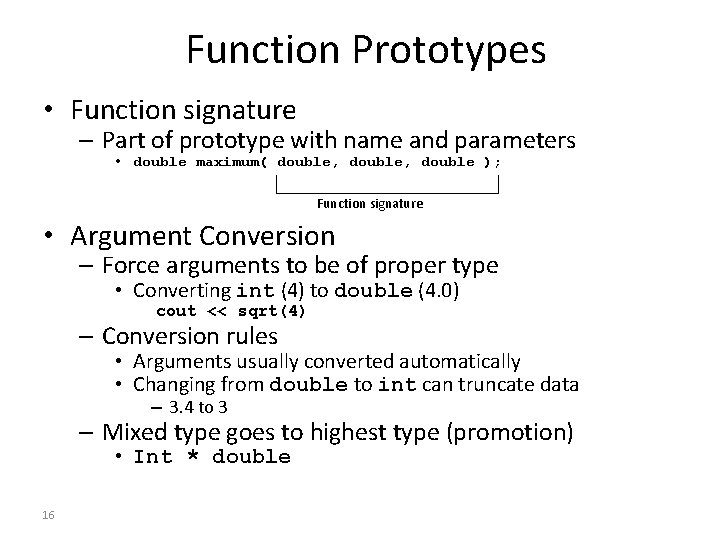
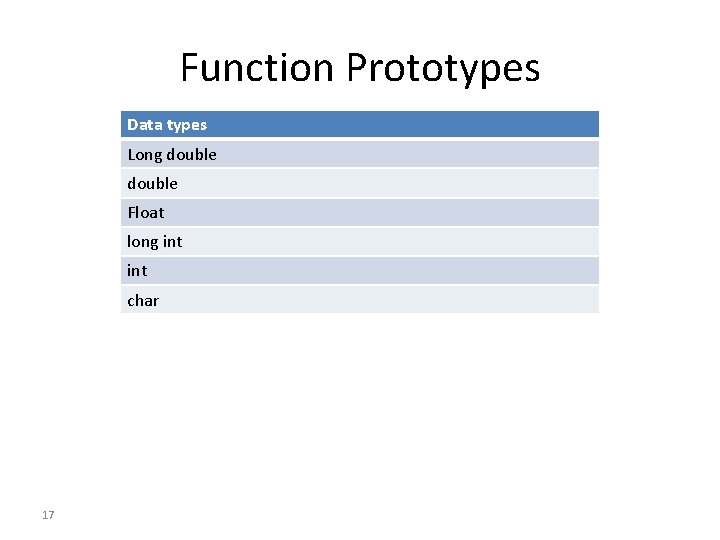
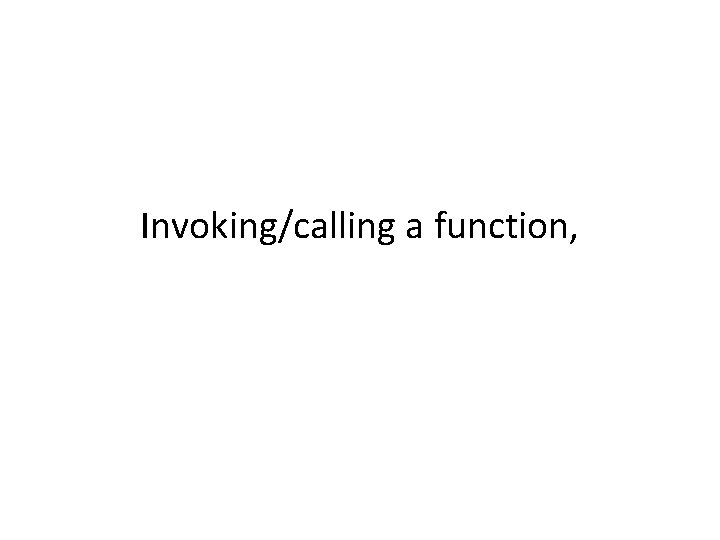
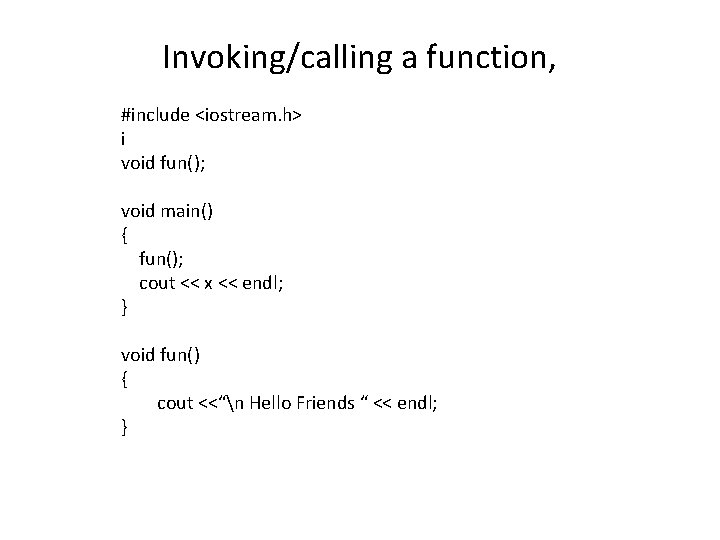
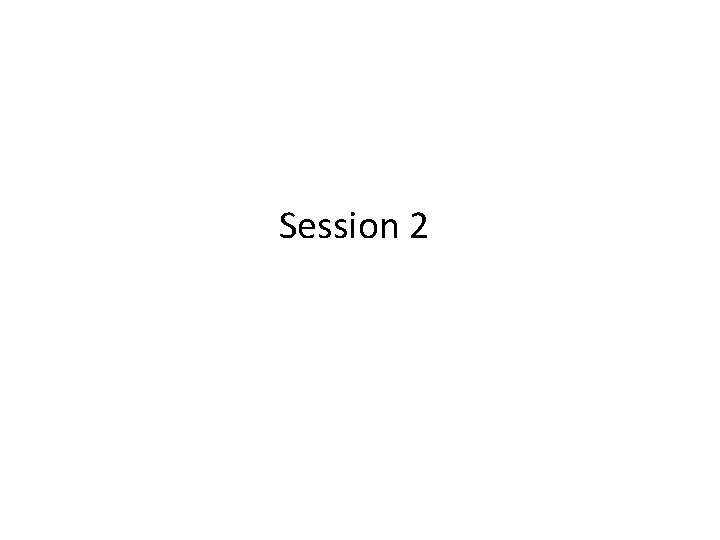
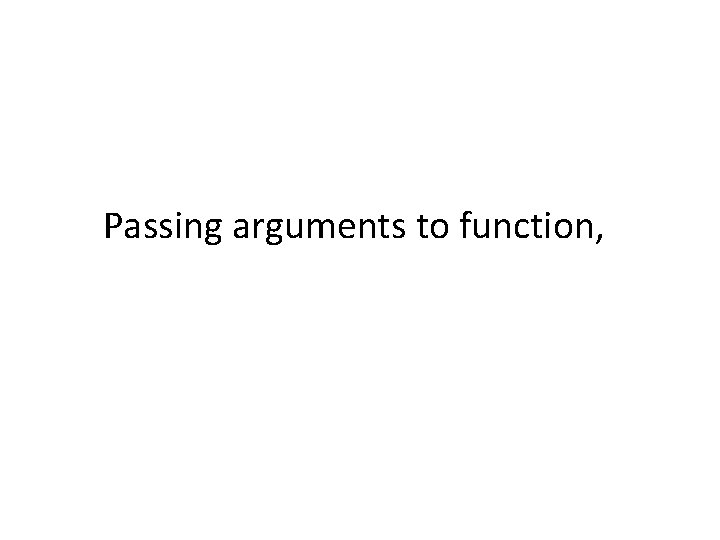
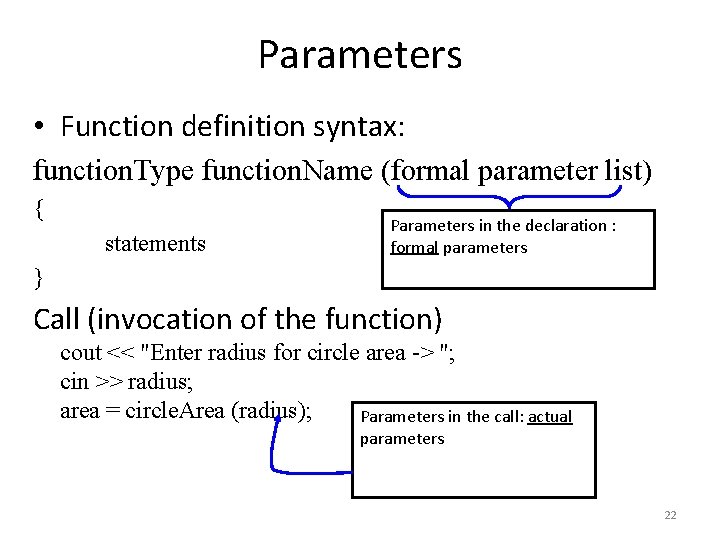
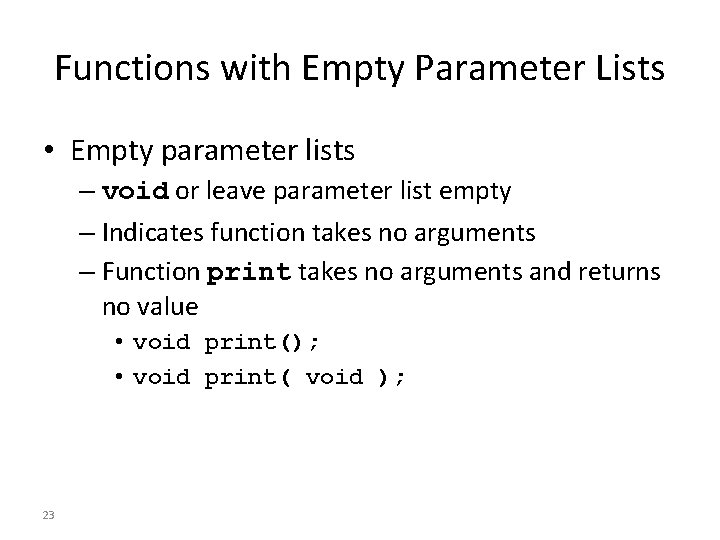
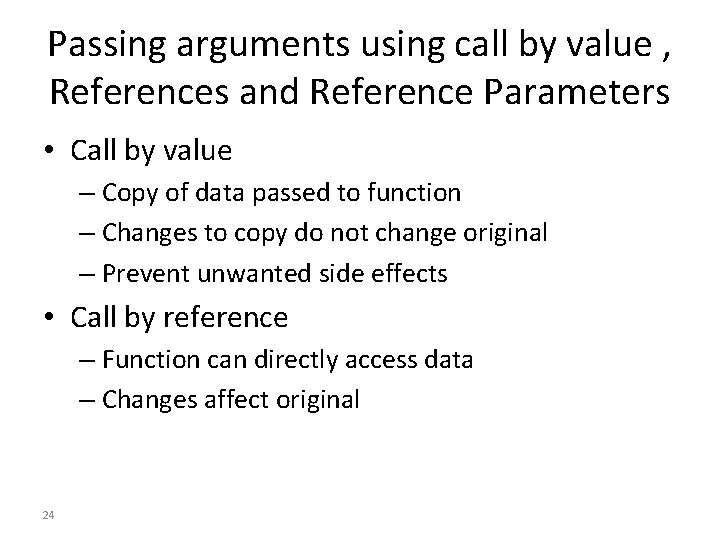
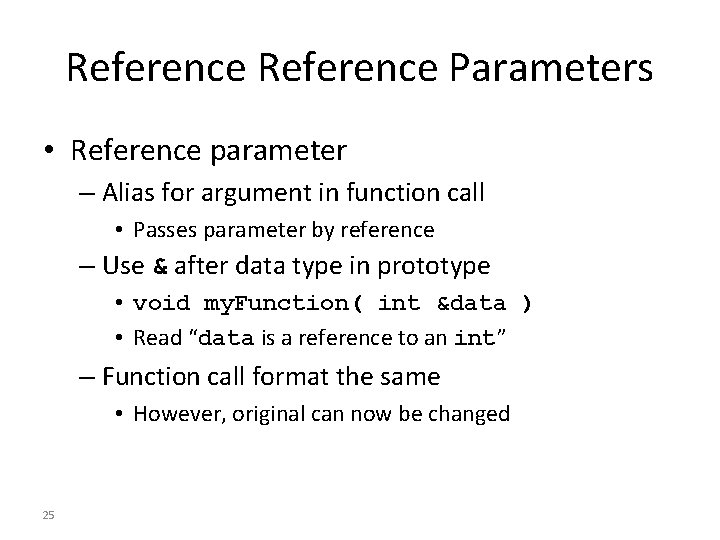
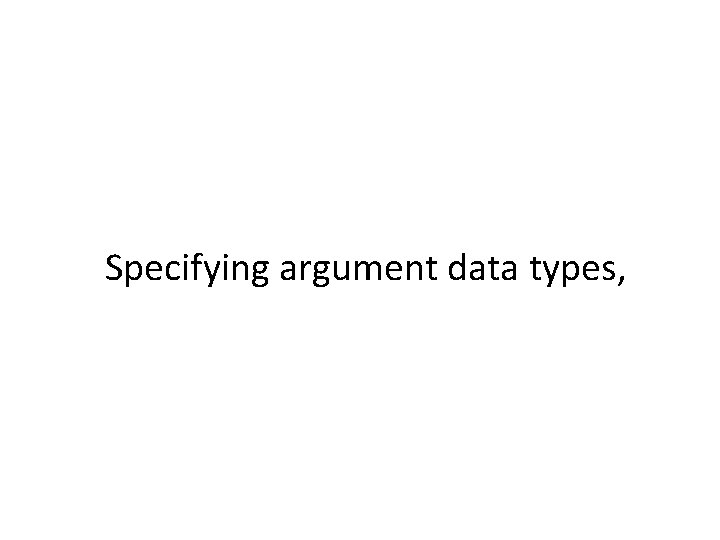
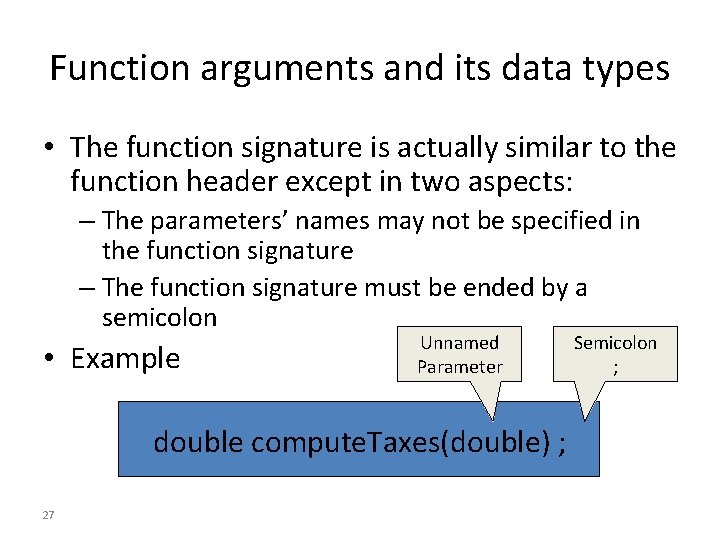
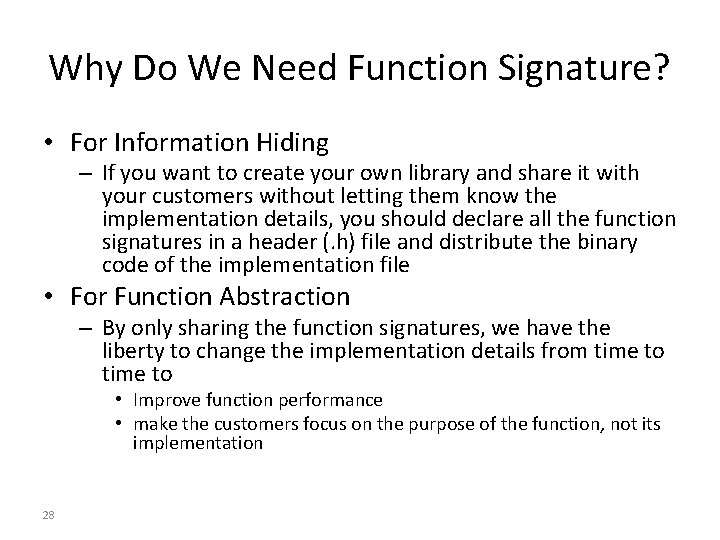
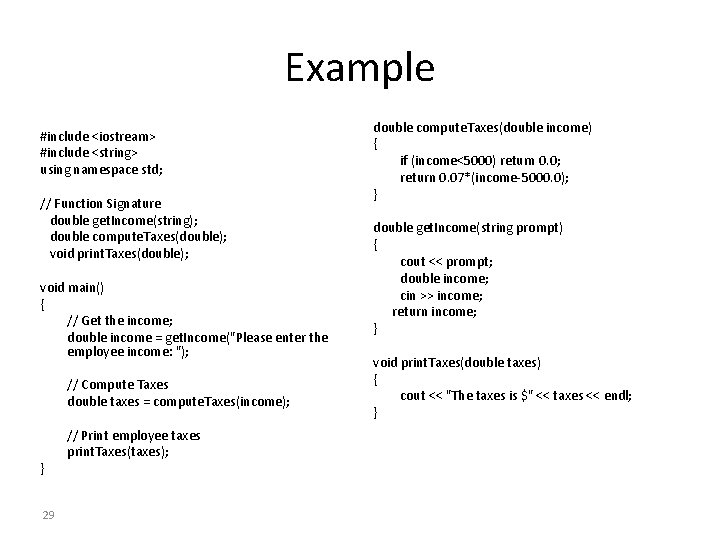
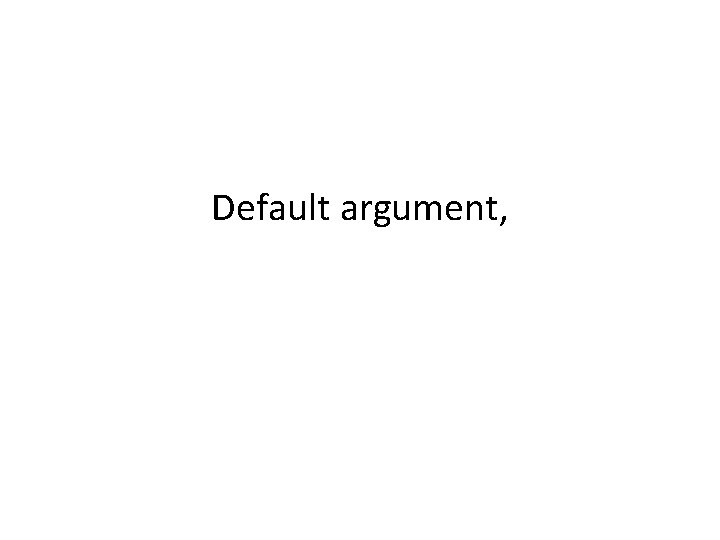
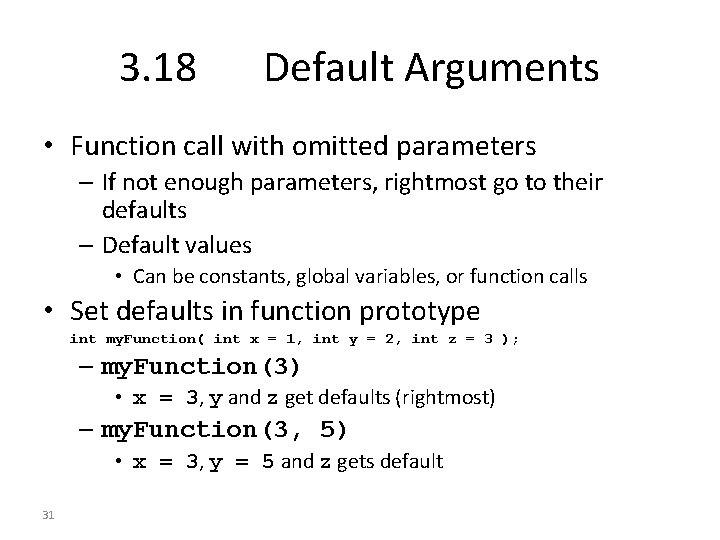
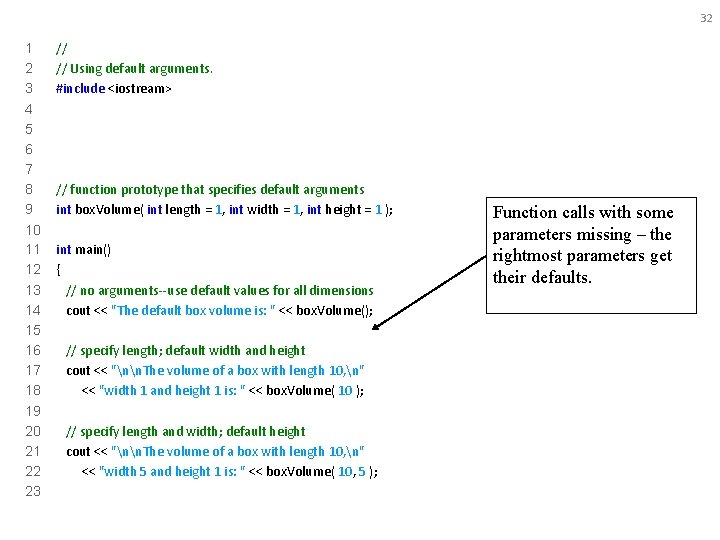
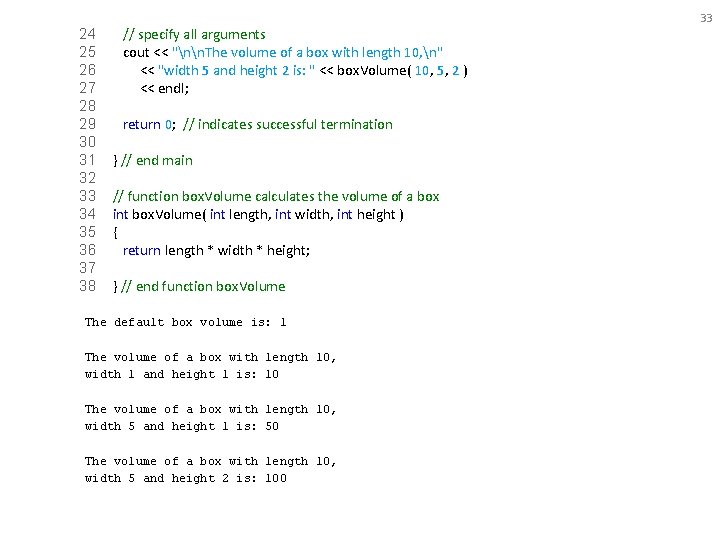
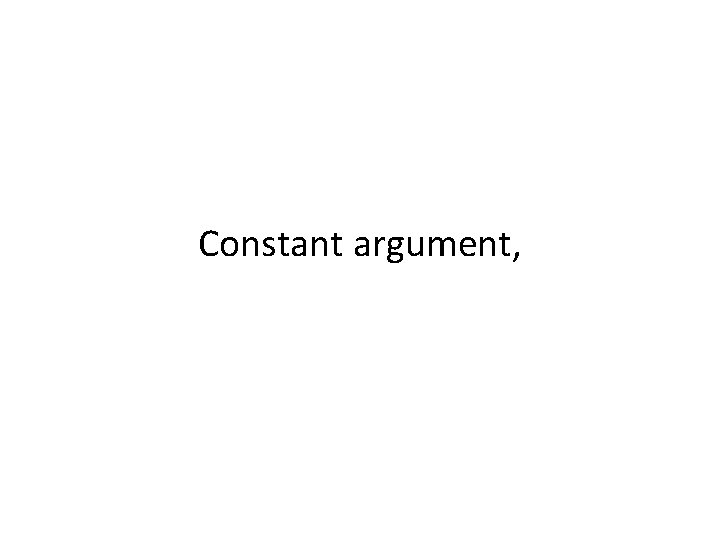
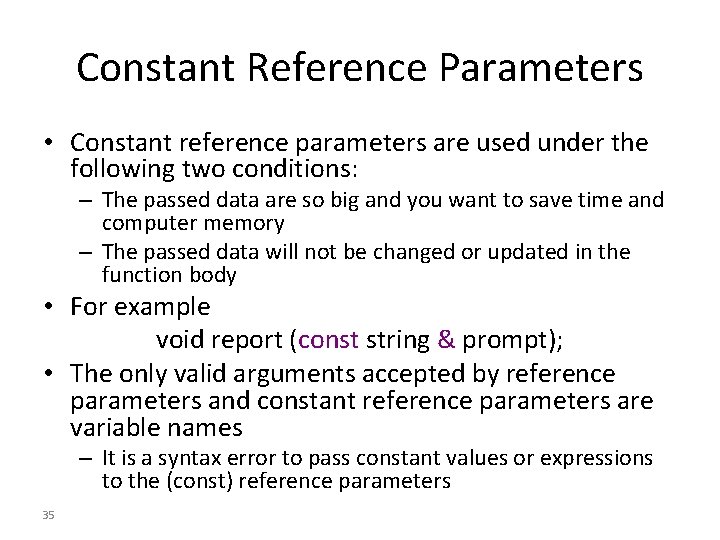
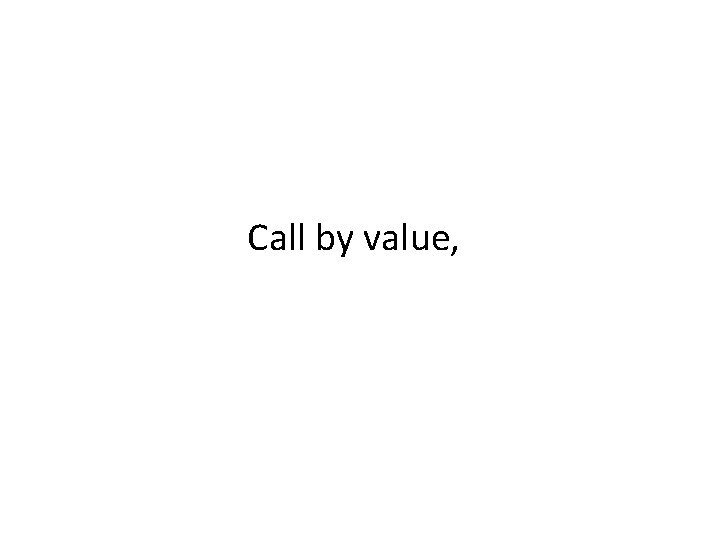
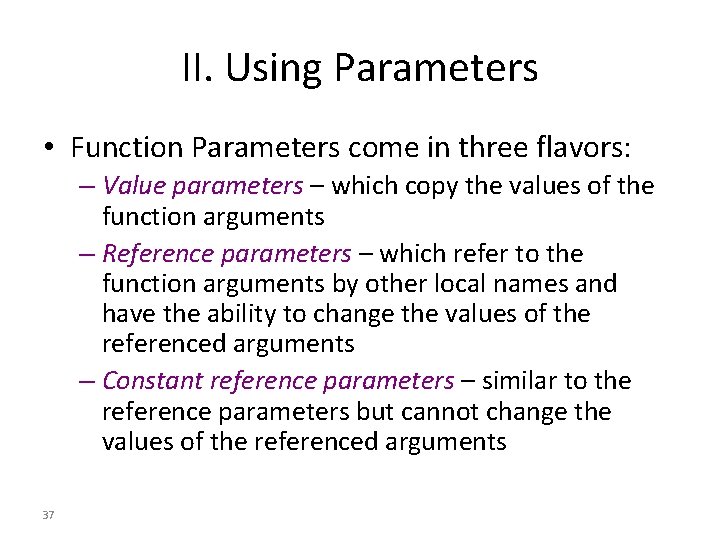
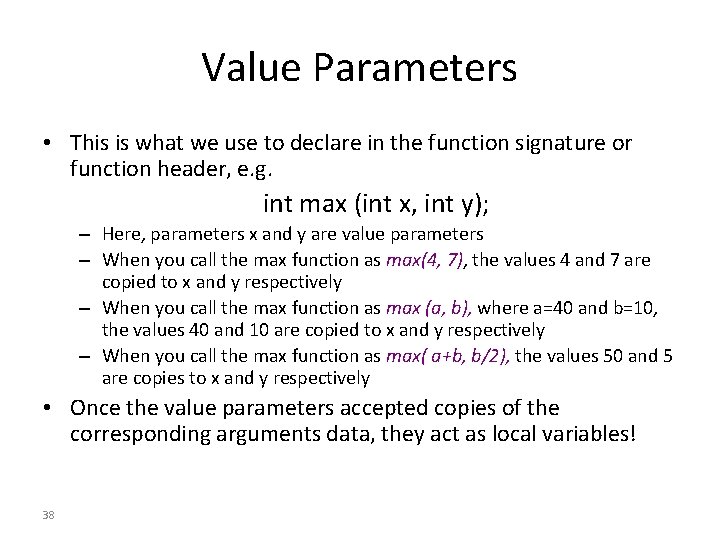
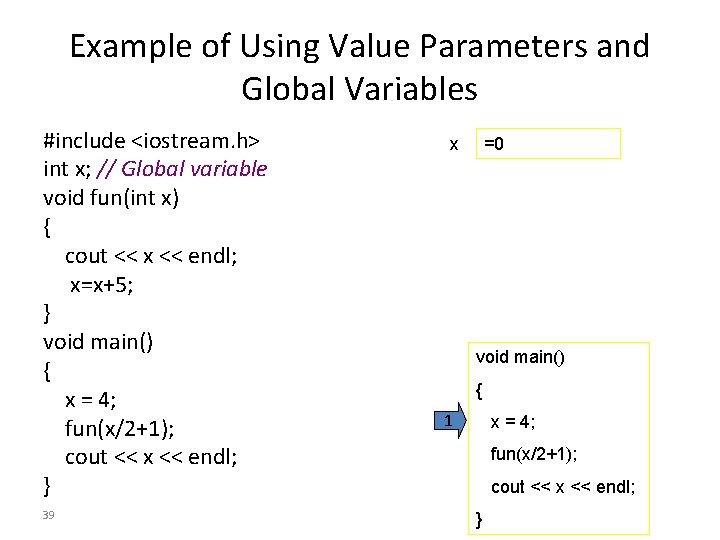
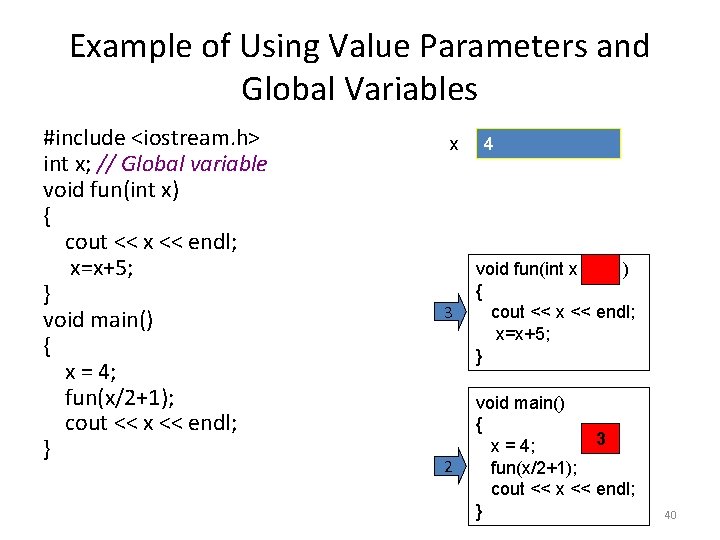
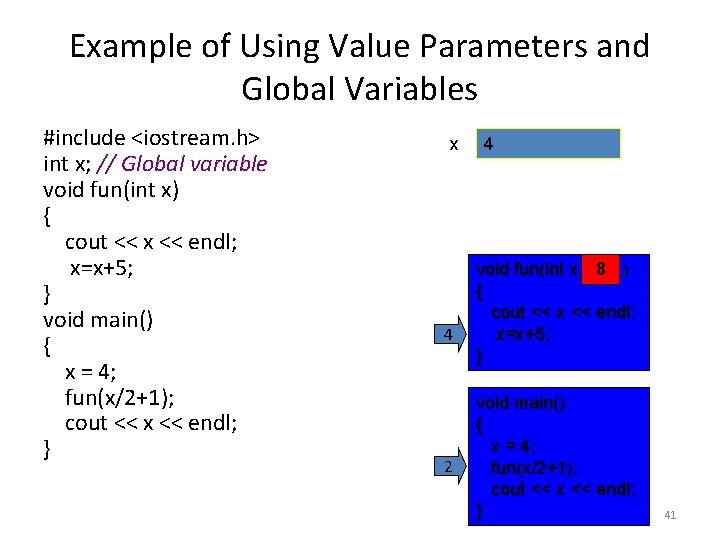
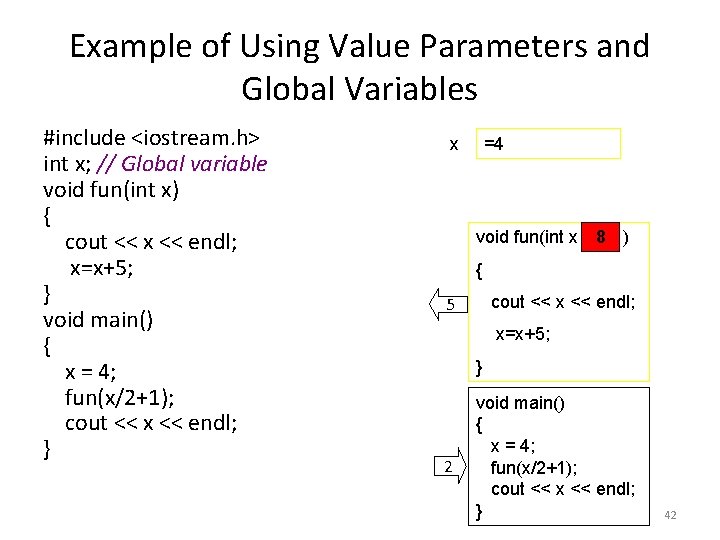
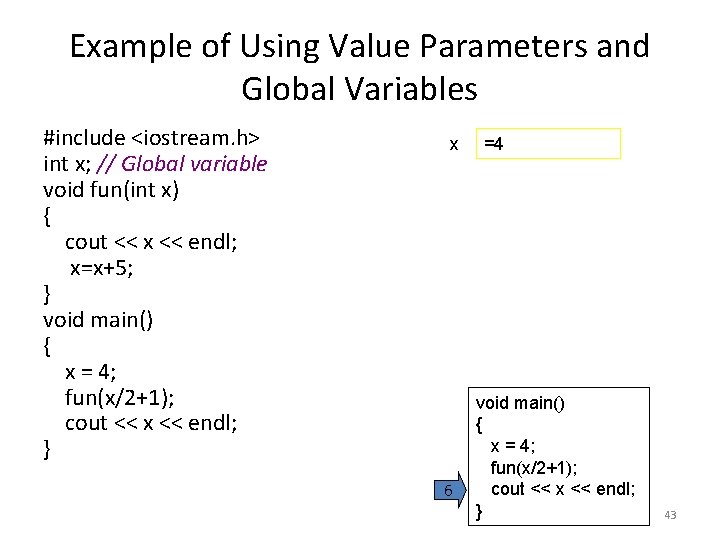
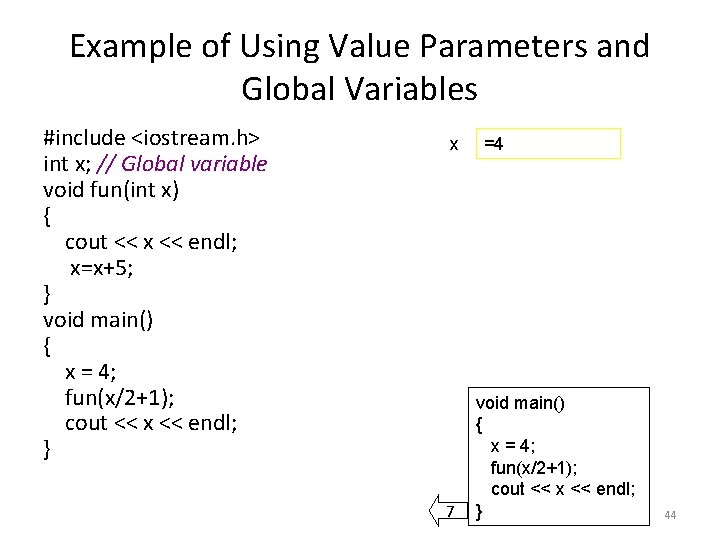
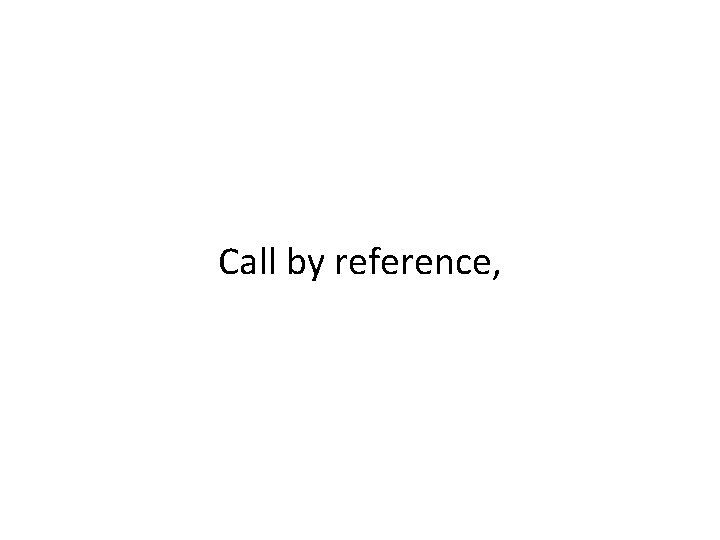
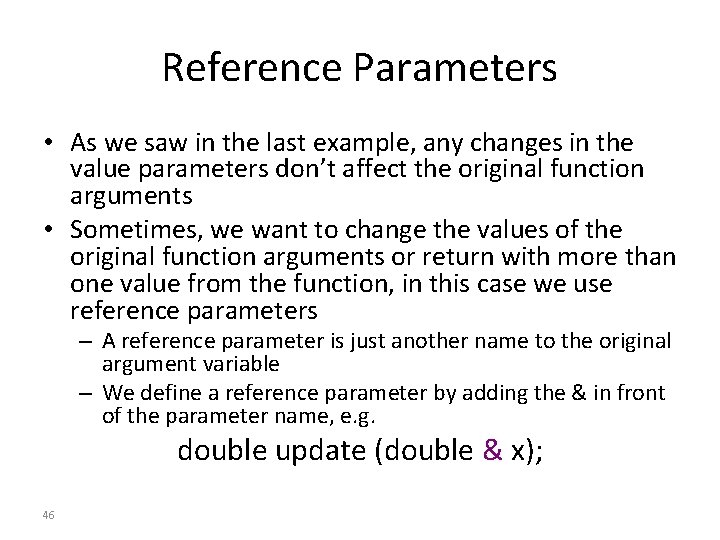
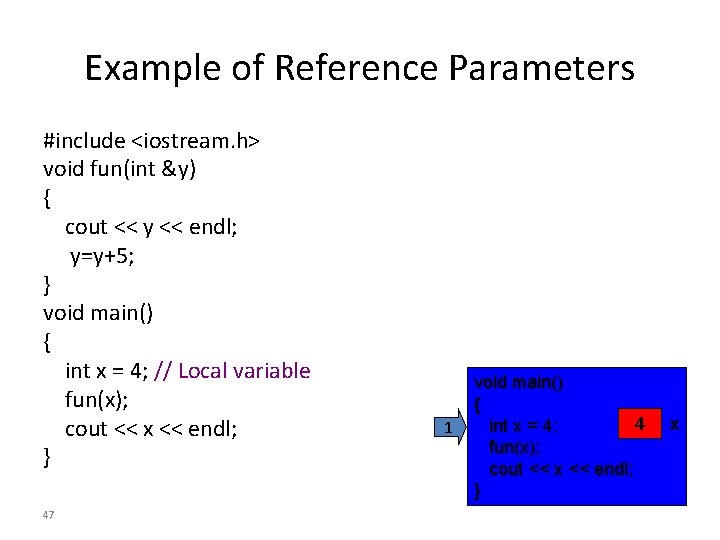
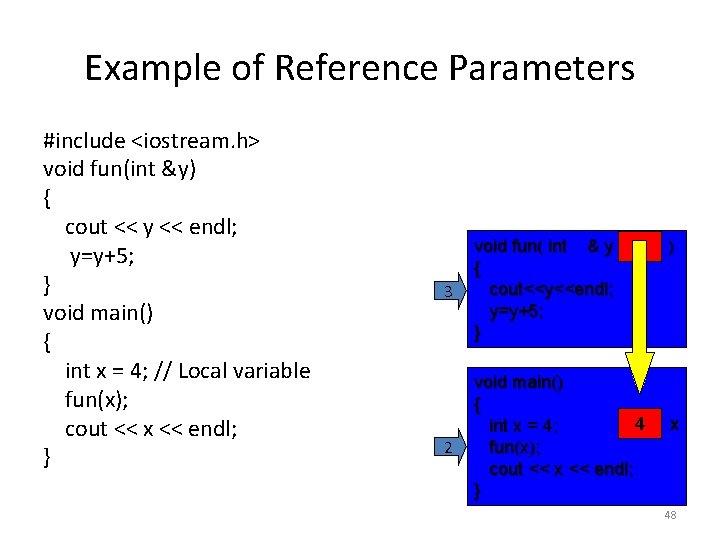
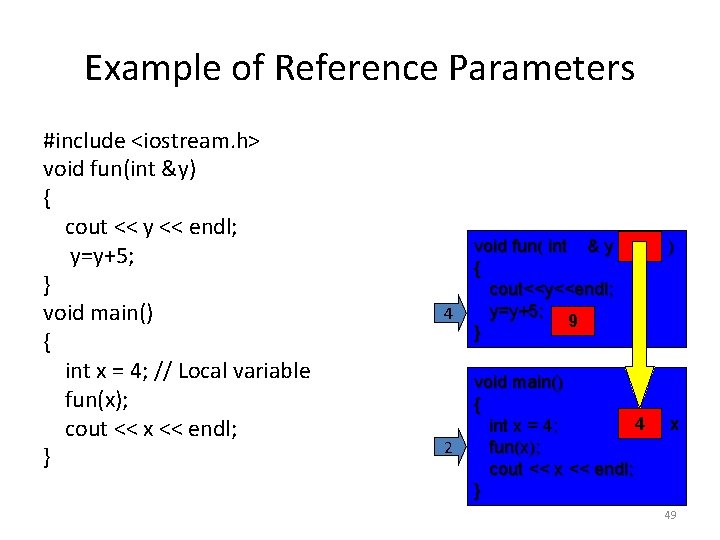
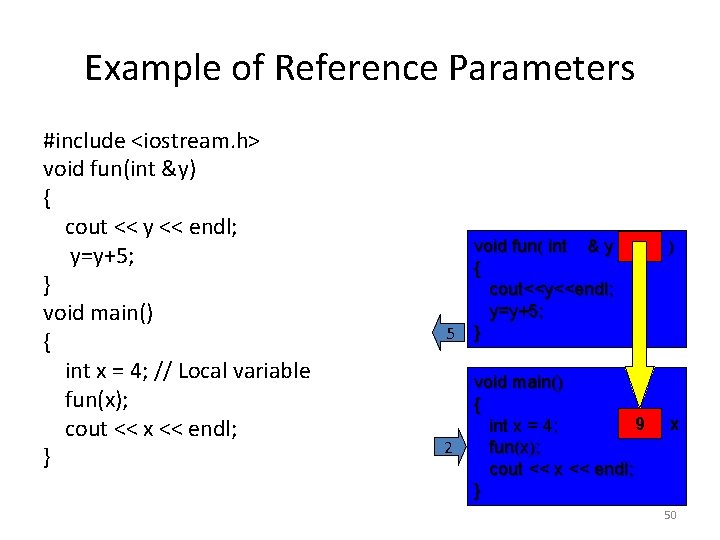
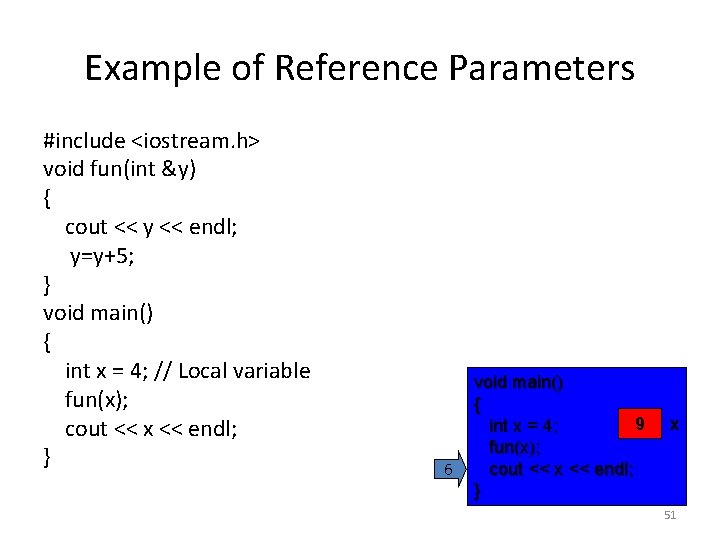
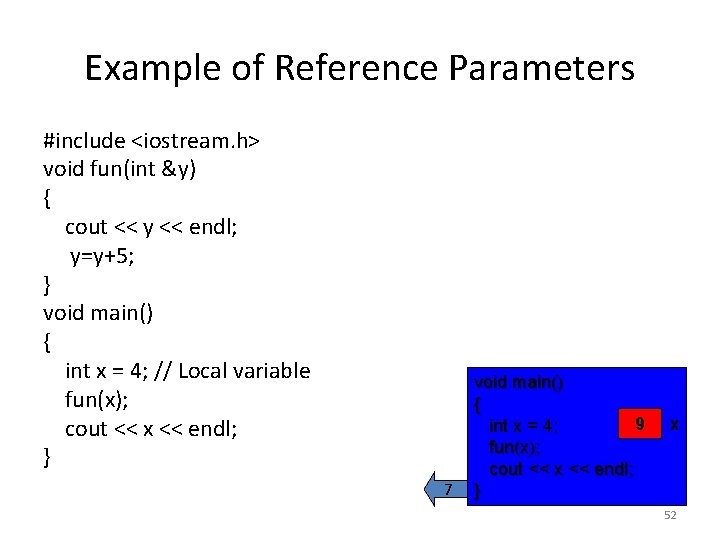
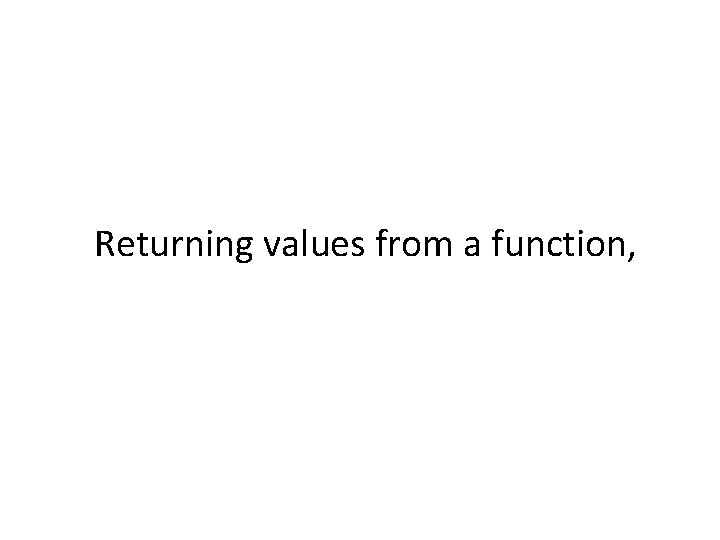
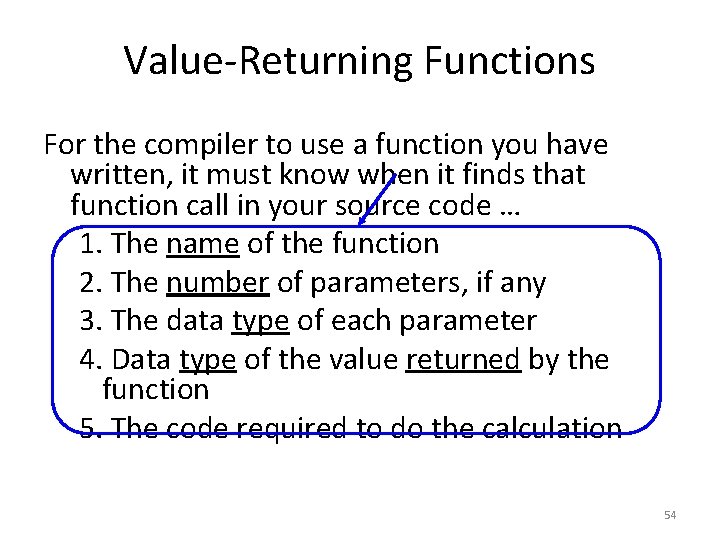
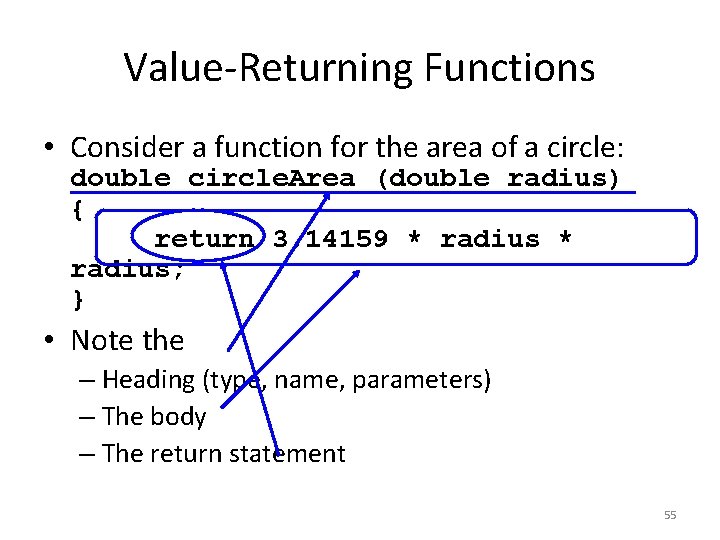
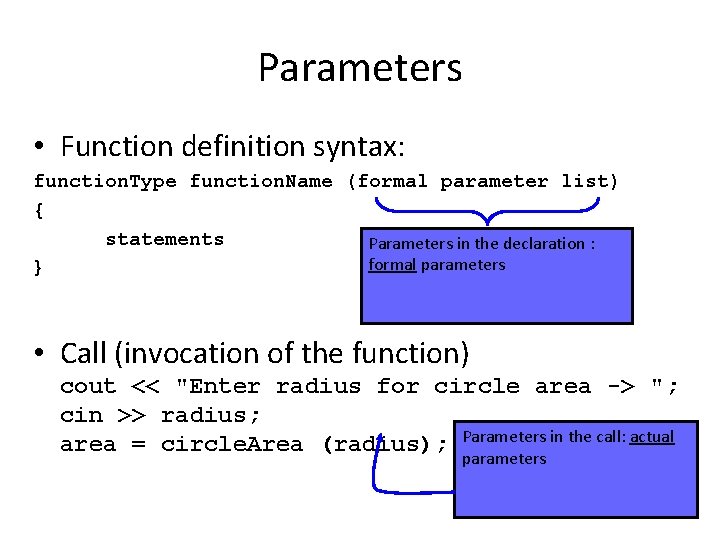
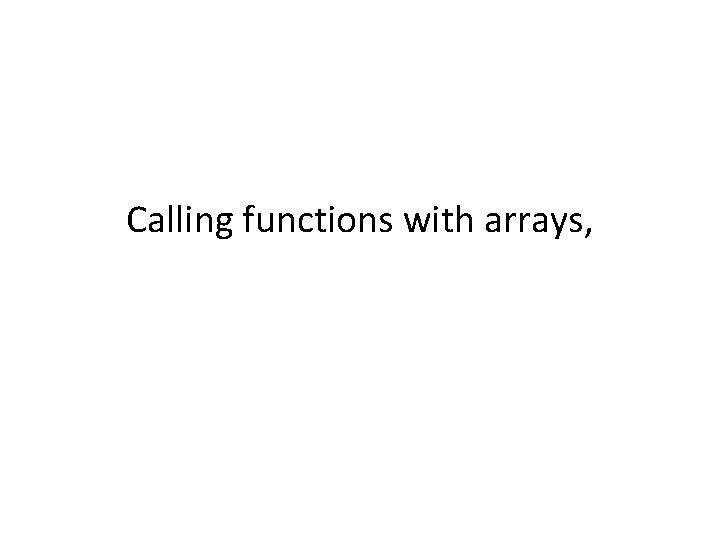
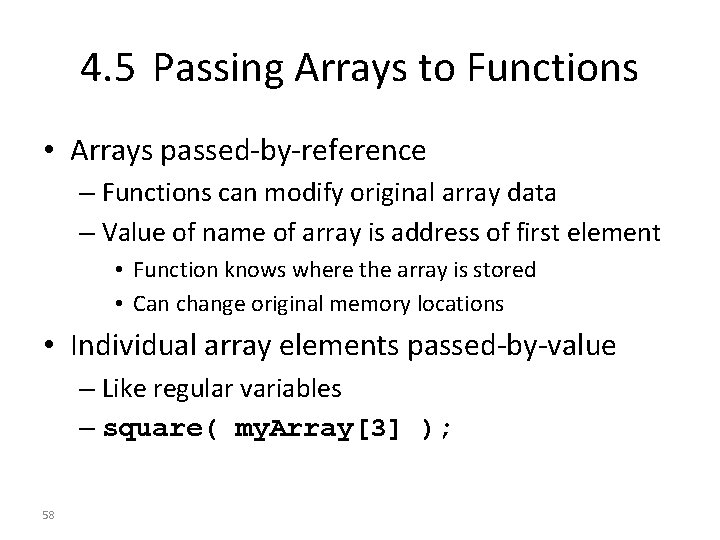
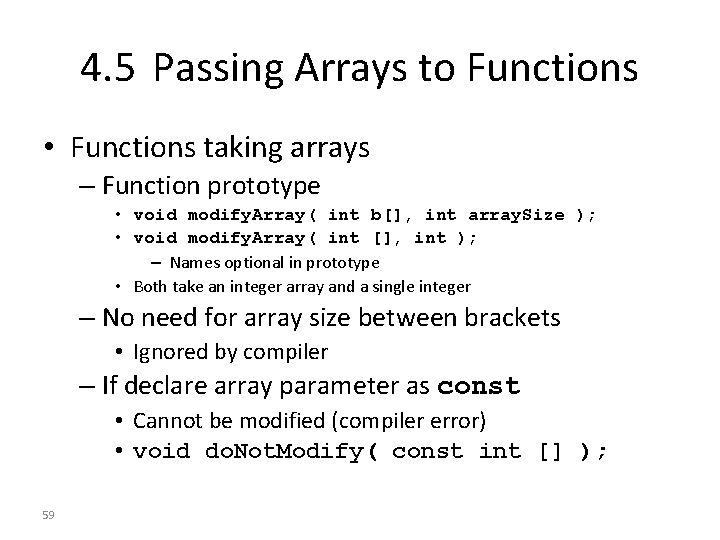
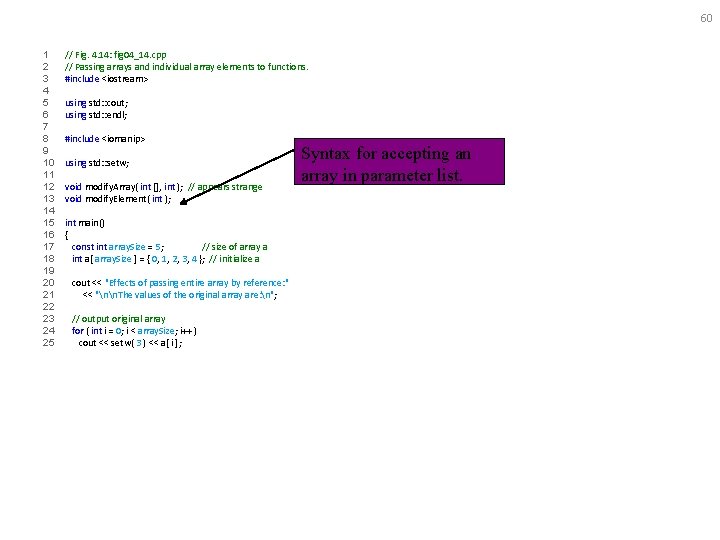
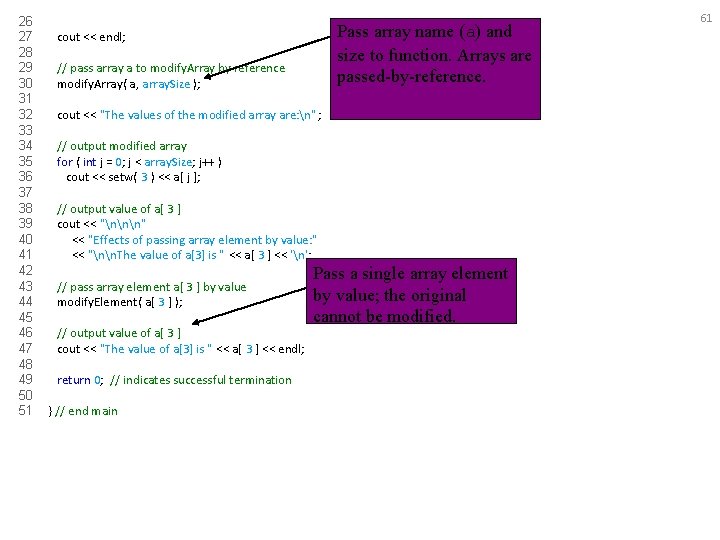
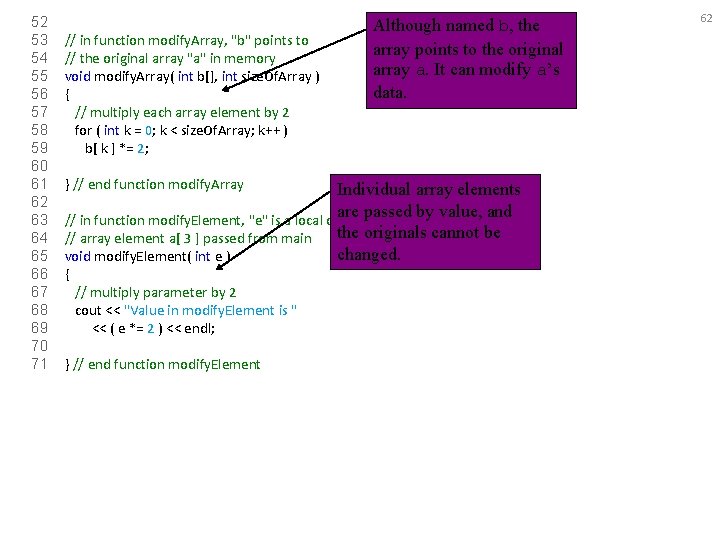
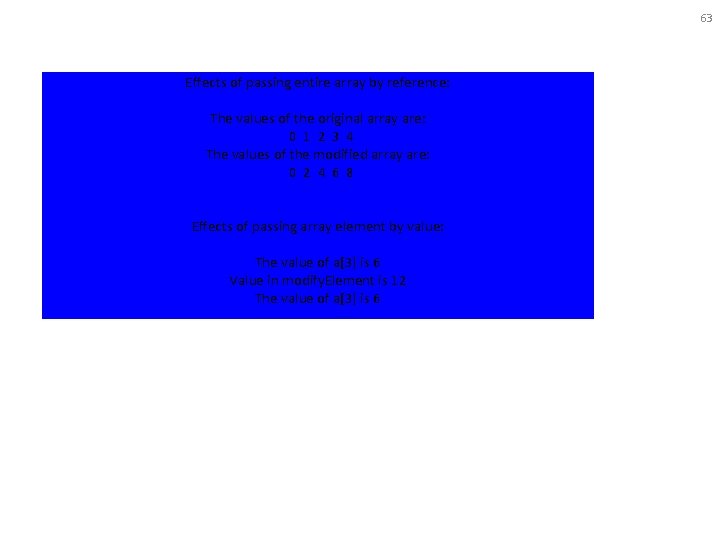
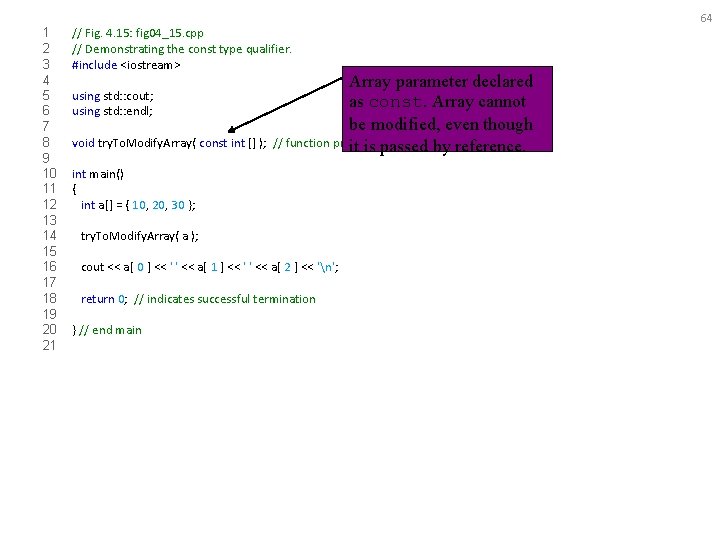
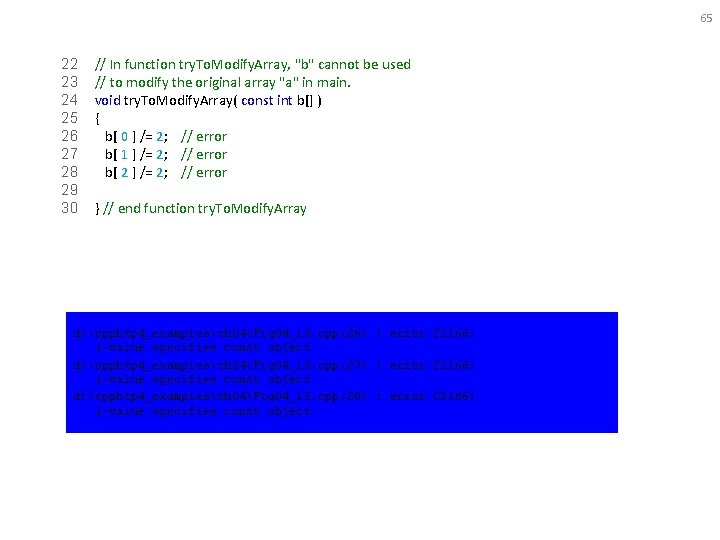
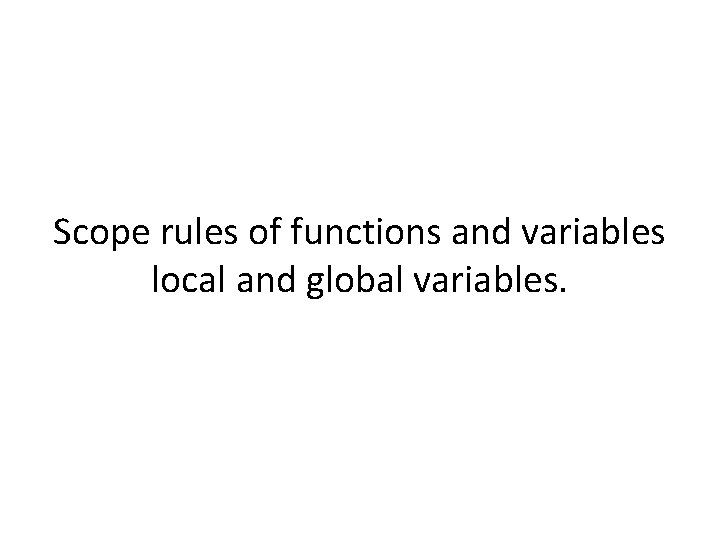
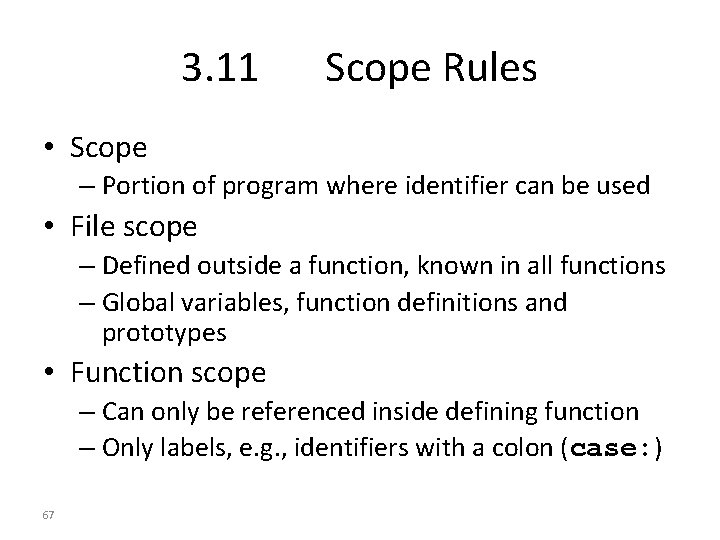
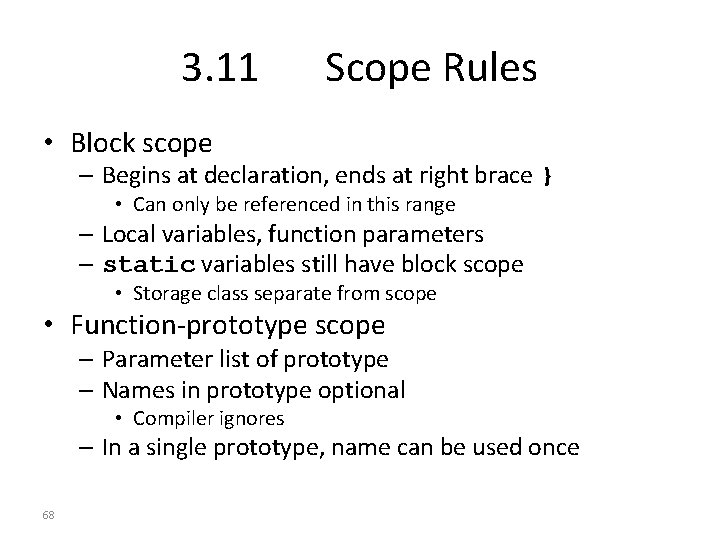
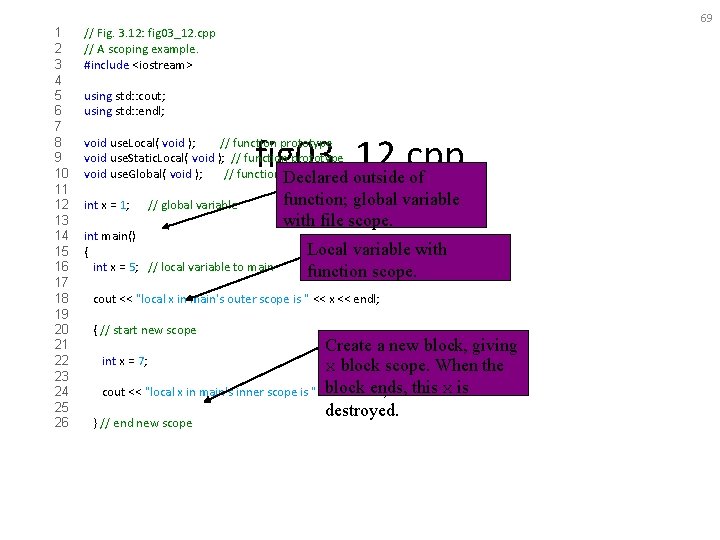
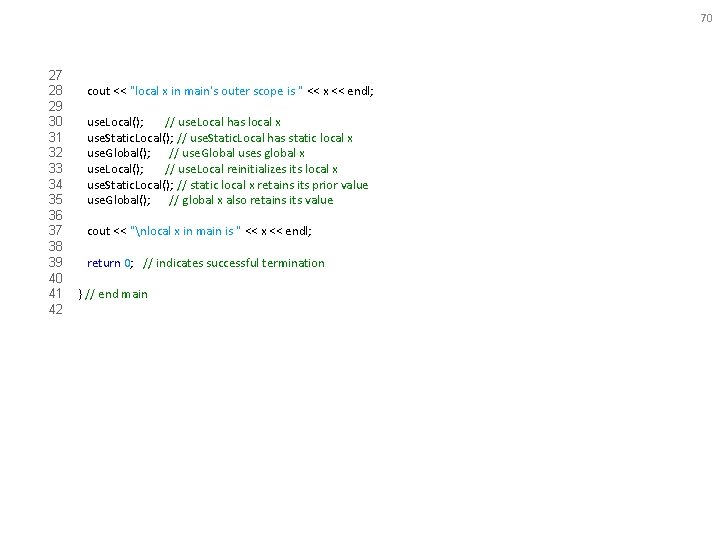
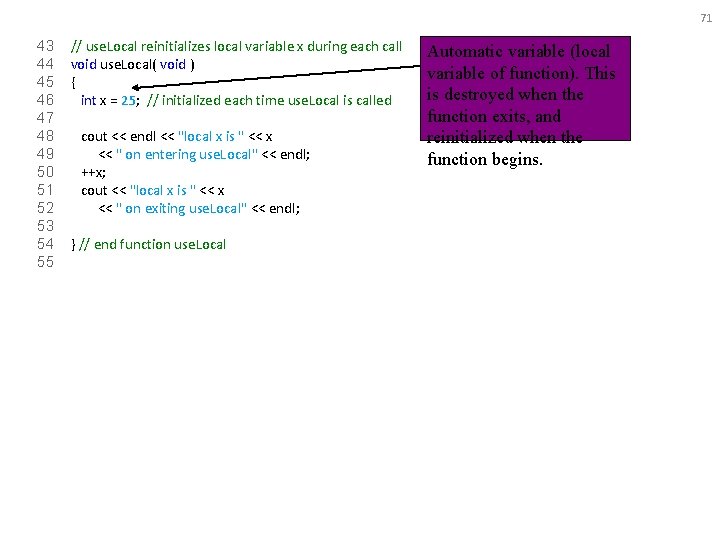
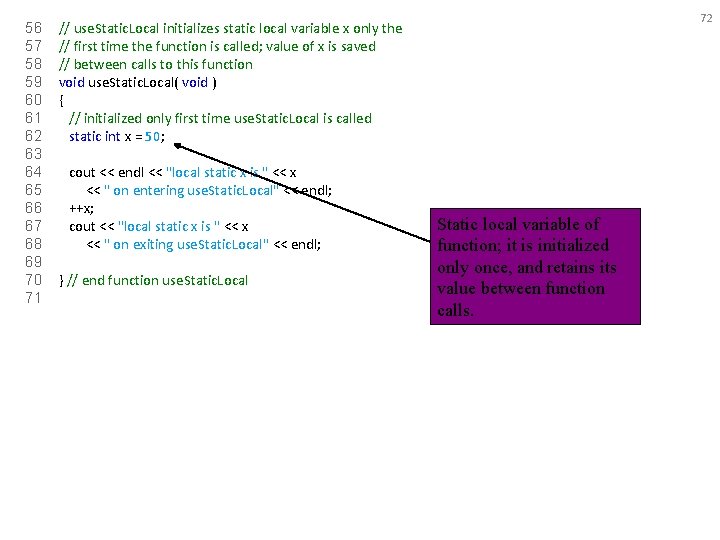
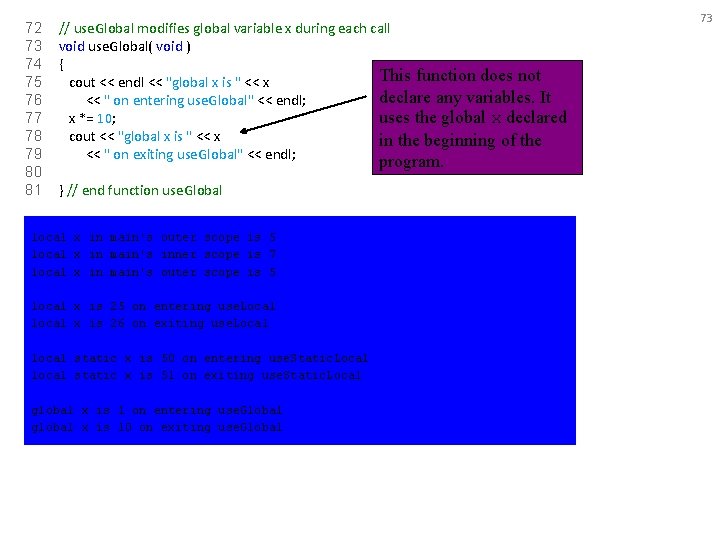
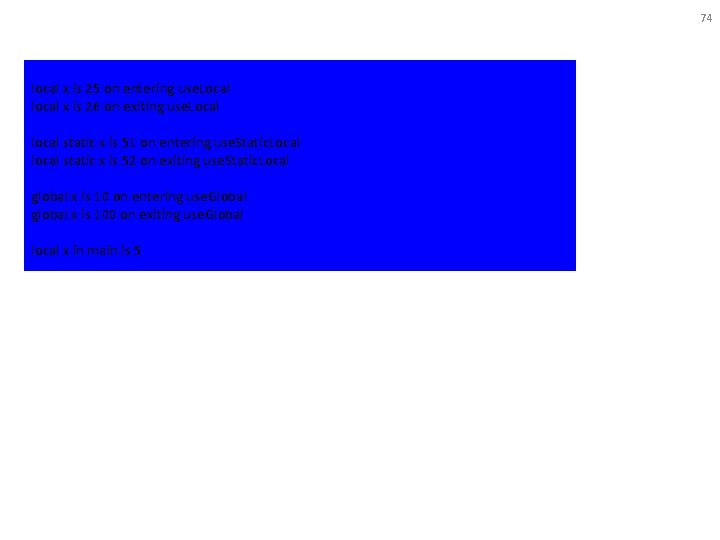
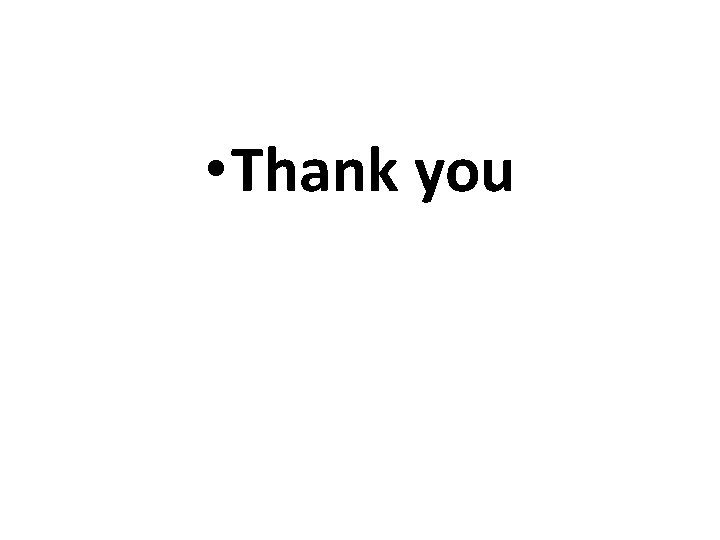
- Slides: 75
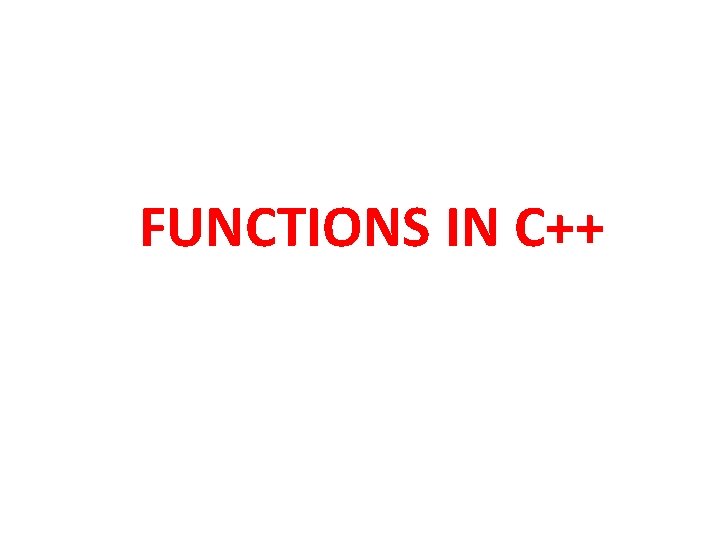
FUNCTIONS IN C++
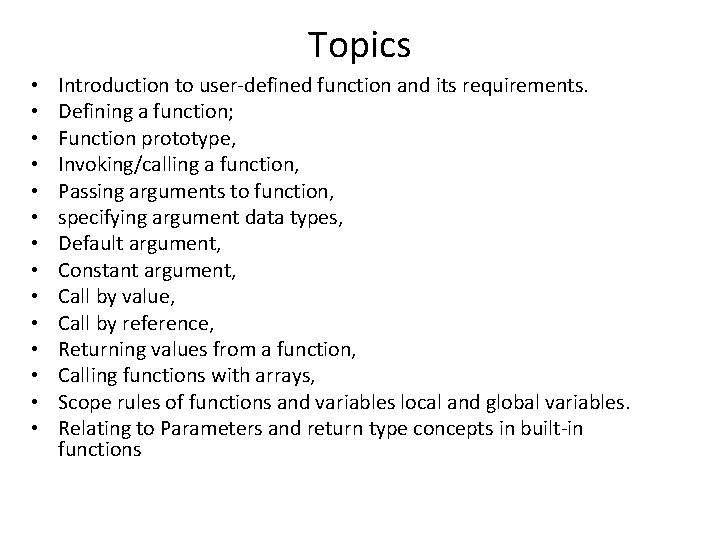
Topics • • • • Introduction to user-defined function and its requirements. Defining a function; Function prototype, Invoking/calling a function, Passing arguments to function, specifying argument data types, Default argument, Constant argument, Call by value, Call by reference, Returning values from a function, Calling functions with arrays, Scope rules of functions and variables local and global variables. Relating to Parameters and return type concepts in built-in functions
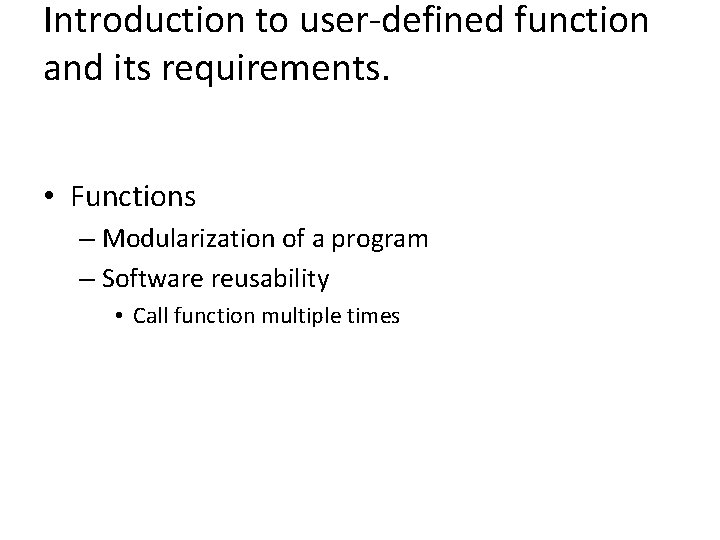
Introduction to user-defined function and its requirements. • Functions – Modularization of a program – Software reusability • Call function multiple times
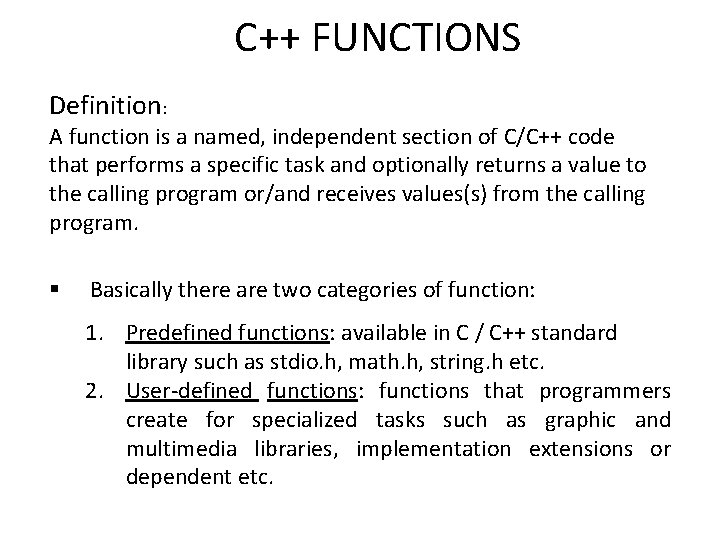
C++ FUNCTIONS Definition: A function is a named, independent section of C/C++ code that performs a specific task and optionally returns a value to the calling program or/and receives values(s) from the calling program. § Basically there are two categories of function: 1. Predefined functions: available in C / C++ standard library such as stdio. h, math. h, string. h etc. 2. User-defined functions: functions that programmers create for specialized tasks such as graphic and multimedia libraries, implementation extensions or dependent etc.
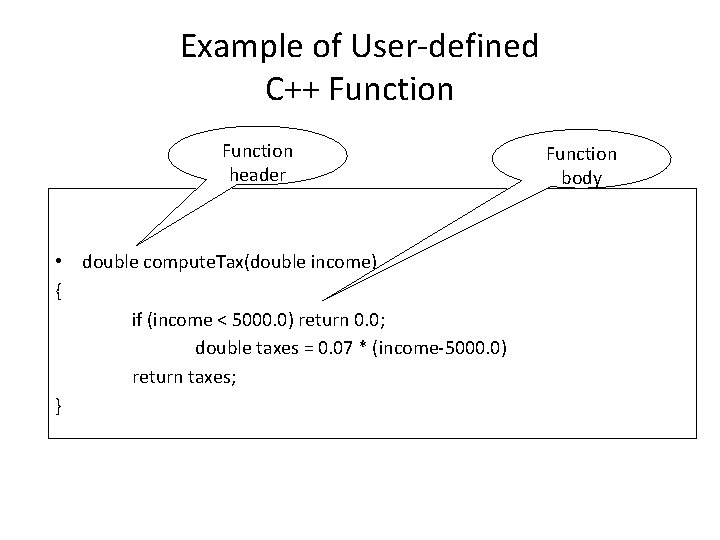
Example of User-defined C++ Function header • double compute. Tax(double income) { if (income < 5000. 0) return 0. 0; double taxes = 0. 07 * (income-5000. 0) return taxes; } Function body
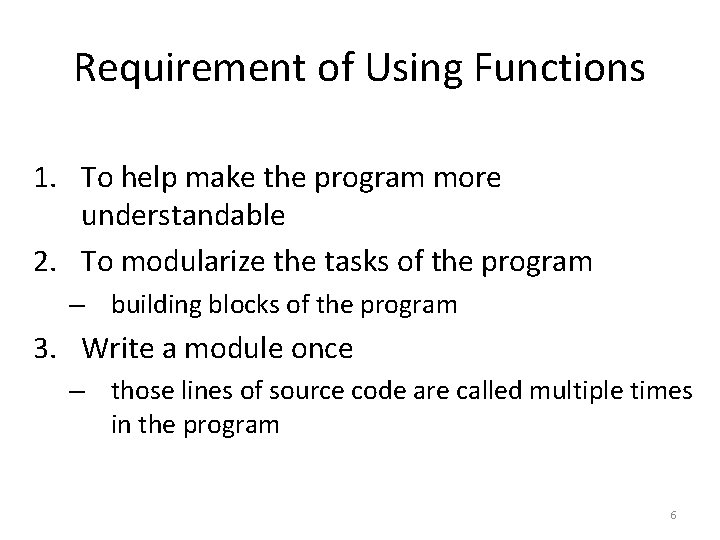
Requirement of Using Functions 1. To help make the program more understandable 2. To modularize the tasks of the program – building blocks of the program 3. Write a module once – those lines of source code are called multiple times in the program 6
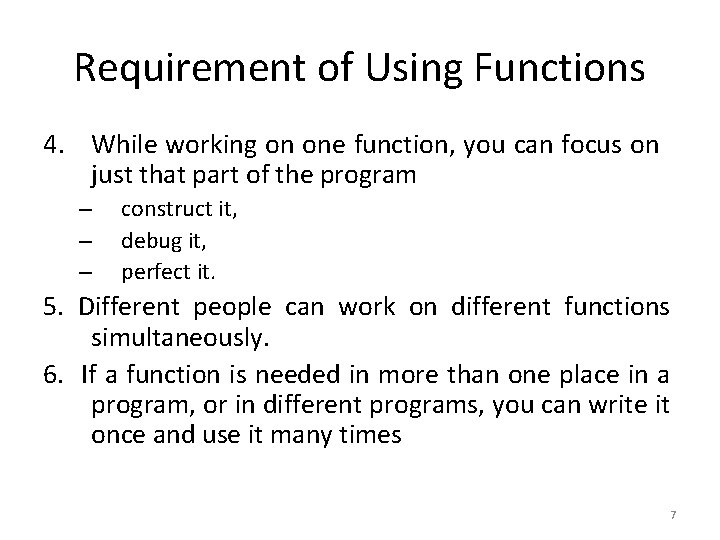
Requirement of Using Functions 4. While working on one function, you can focus on just that part of the program – – – construct it, debug it, perfect it. 5. Different people can work on different functions simultaneously. 6. If a function is needed in more than one place in a program, or in different programs, you can write it once and use it many times 7
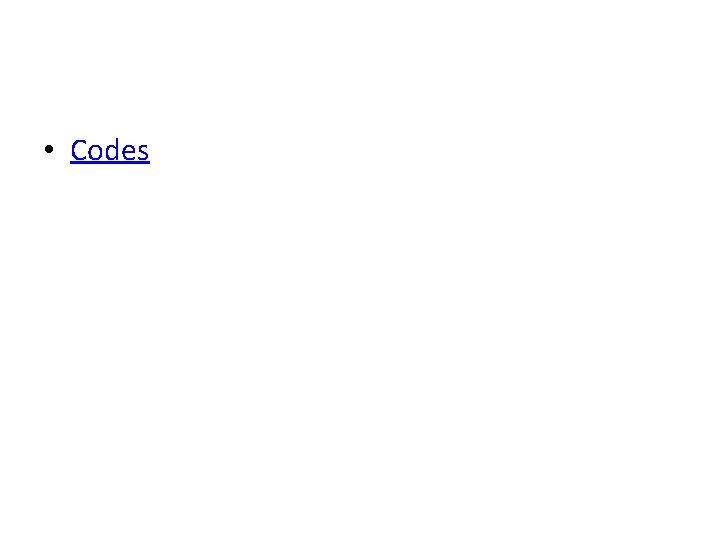
• Codes
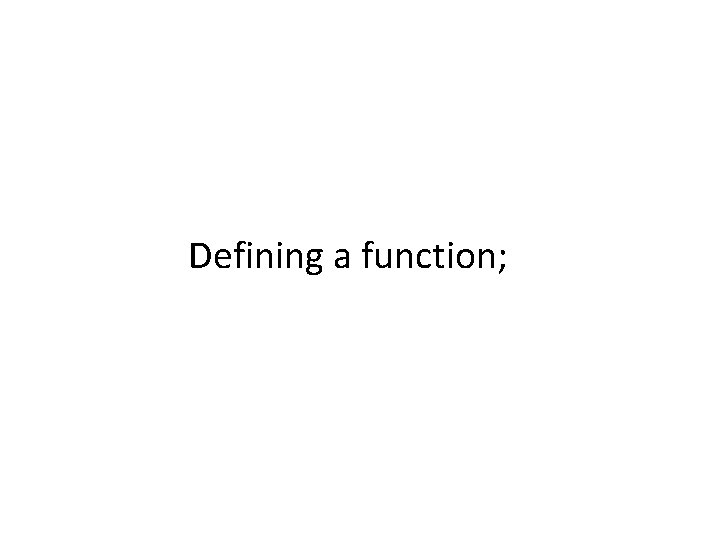
Defining a function;
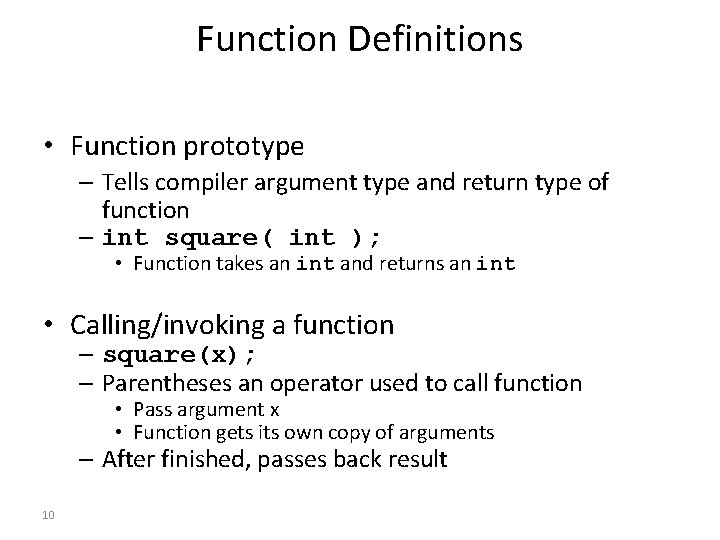
Function Definitions • Function prototype – Tells compiler argument type and return type of function – int square( int ); • Function takes an int and returns an int • Calling/invoking a function – square(x); – Parentheses an operator used to call function • Pass argument x • Function gets its own copy of arguments – After finished, passes back result 10
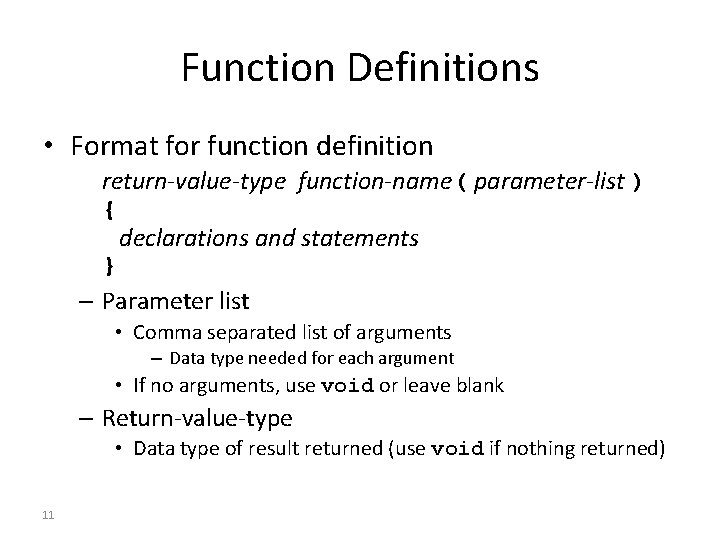
Function Definitions • Format for function definition return-value-type function-name( parameter-list ) { declarations and statements } – Parameter list • Comma separated list of arguments – Data type needed for each argument • If no arguments, use void or leave blank – Return-value-type • Data type of result returned (use void if nothing returned) 11
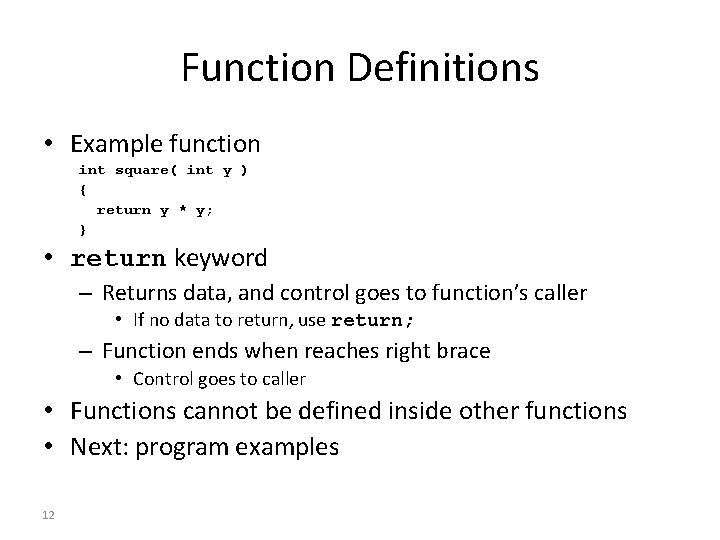
Function Definitions • Example function int square( int y ) { return y * y; } • return keyword – Returns data, and control goes to function’s caller • If no data to return, use return; – Function ends when reaches right brace • Control goes to caller • Functions cannot be defined inside other functions • Next: program examples 12
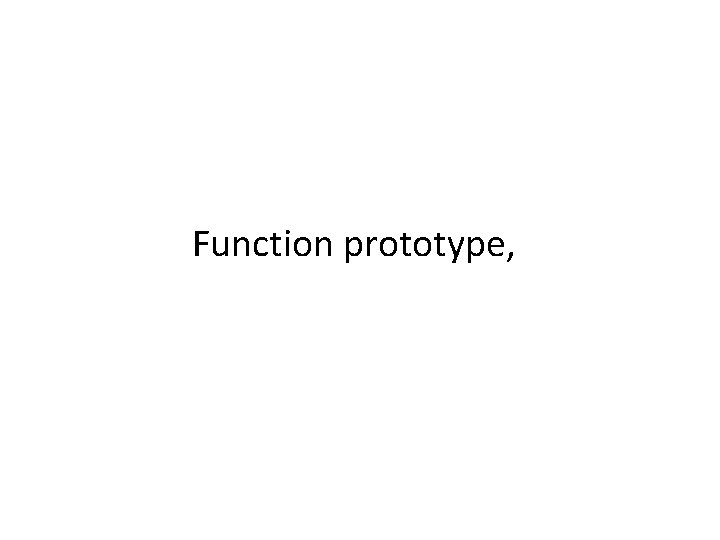
Function prototype,
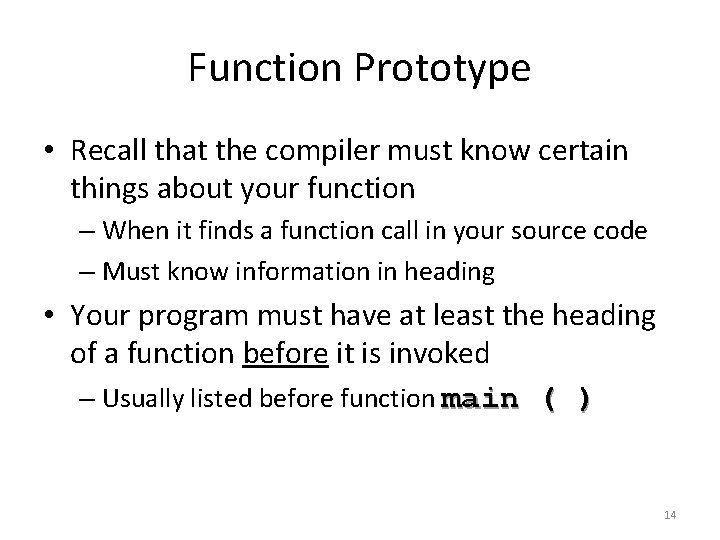
Function Prototype • Recall that the compiler must know certain things about your function – When it finds a function call in your source code – Must know information in heading • Your program must have at least the heading of a function before it is invoked – Usually listed before function main ( ) 14
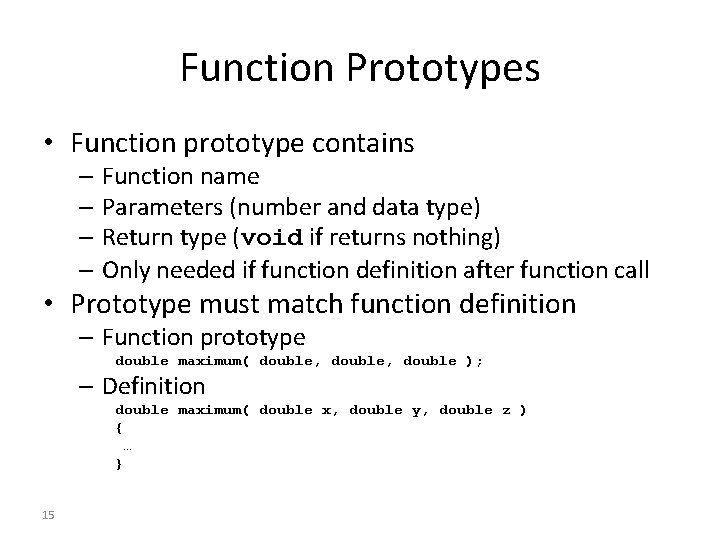
Function Prototypes • Function prototype contains – Function name – Parameters (number and data type) – Return type (void if returns nothing) – Only needed if function definition after function call • Prototype must match function definition – Function prototype double maximum( double, double ); – Definition double maximum( double x, double y, double z ) { … } 15
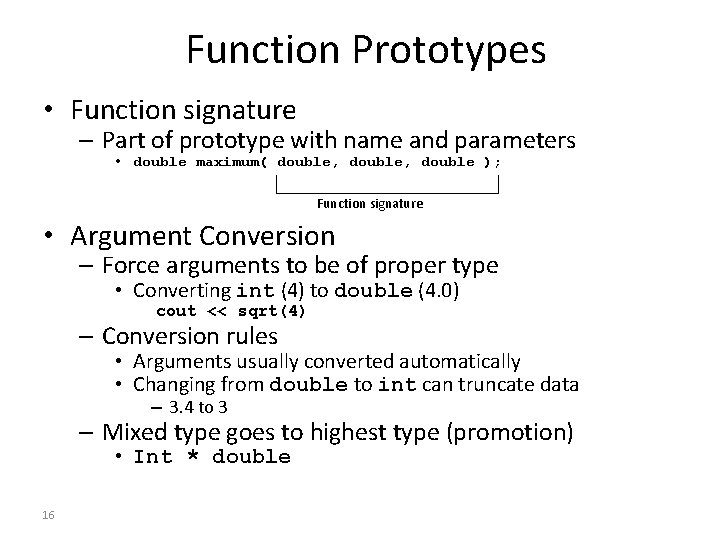
Function Prototypes • Function signature – Part of prototype with name and parameters • double maximum( double, double ); Function signature • Argument Conversion – Force arguments to be of proper type • Converting int (4) to double (4. 0) cout << sqrt(4) – Conversion rules • Arguments usually converted automatically • Changing from double to int can truncate data – 3. 4 to 3 – Mixed type goes to highest type (promotion) • Int * double 16
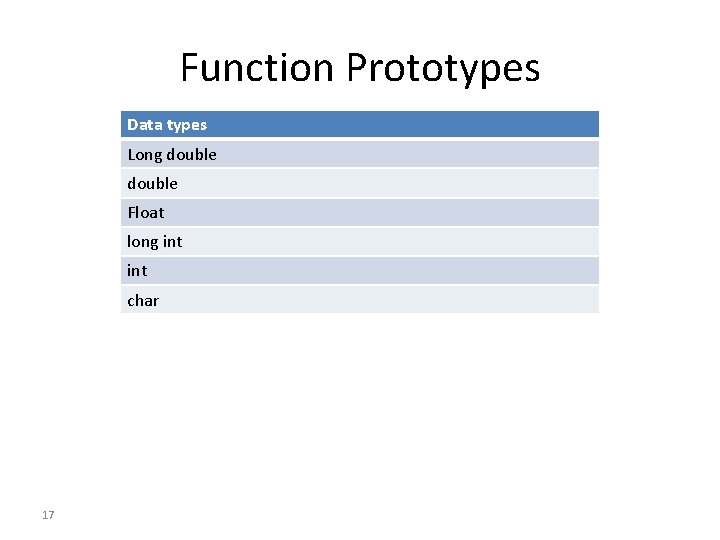
Function Prototypes Data types Long double Float long int char 17
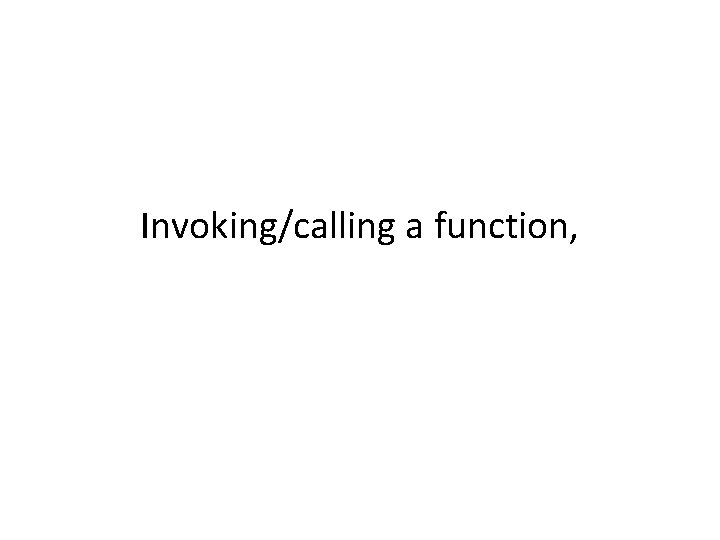
Invoking/calling a function,
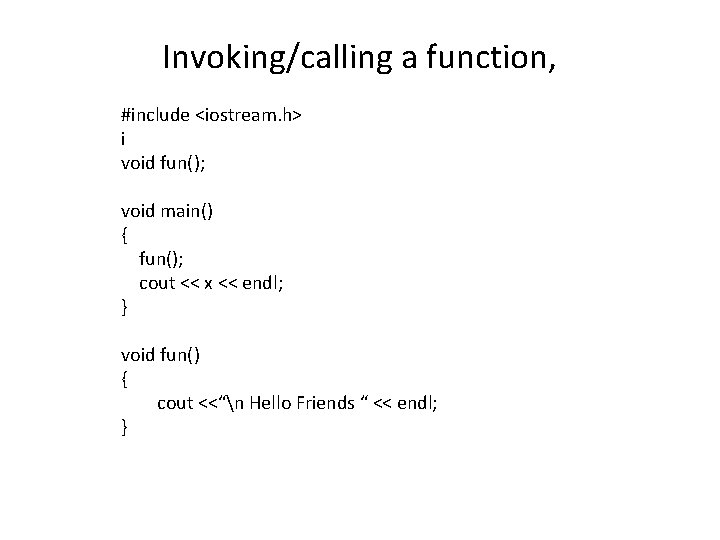
Invoking/calling a function, #include <iostream. h> i void fun(); void main() { fun(); cout << x << endl; } void fun() { cout <<“n Hello Friends “ << endl; }
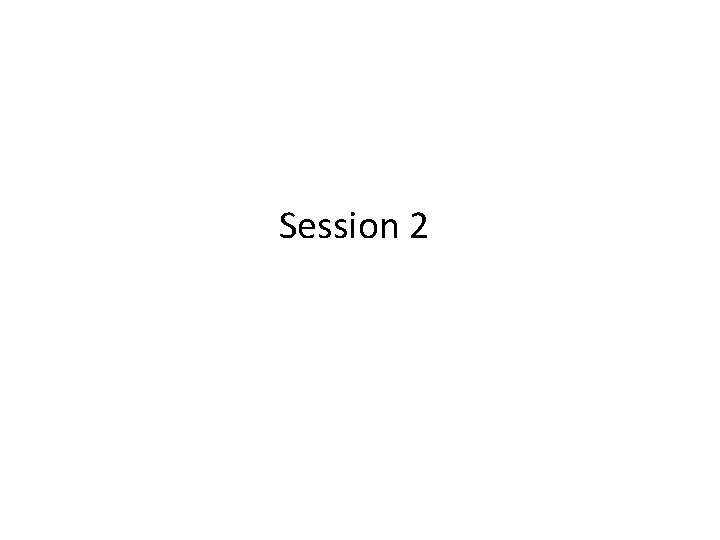
Session 2
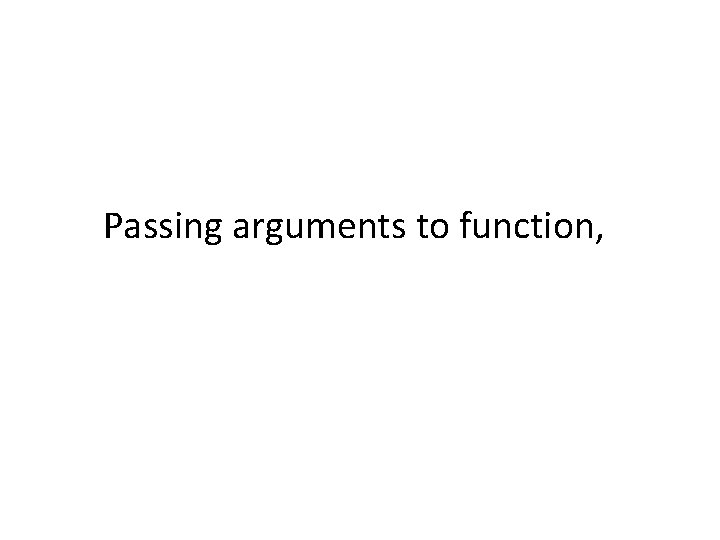
Passing arguments to function,
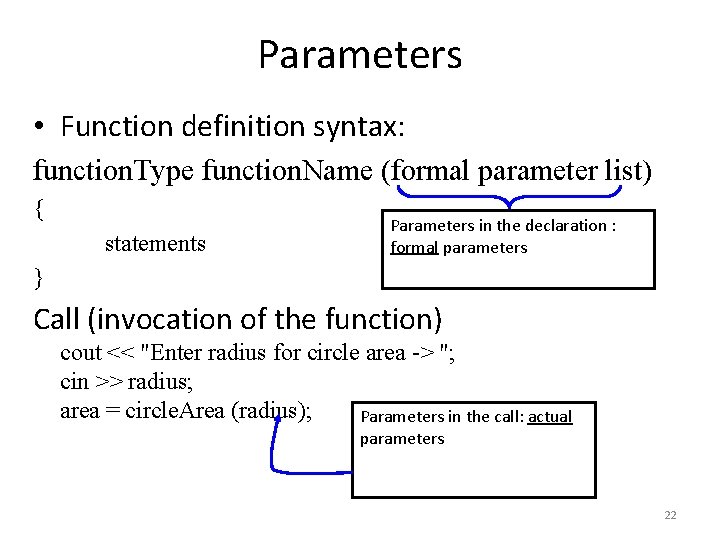
Parameters • Function definition syntax: function. Type function. Name (formal parameter list) { statements Parameters in the declaration : formal parameters } Call (invocation of the function) cout << "Enter radius for circle area -> "; cin >> radius; area = circle. Area (radius); Parameters in the call: actual parameters 22
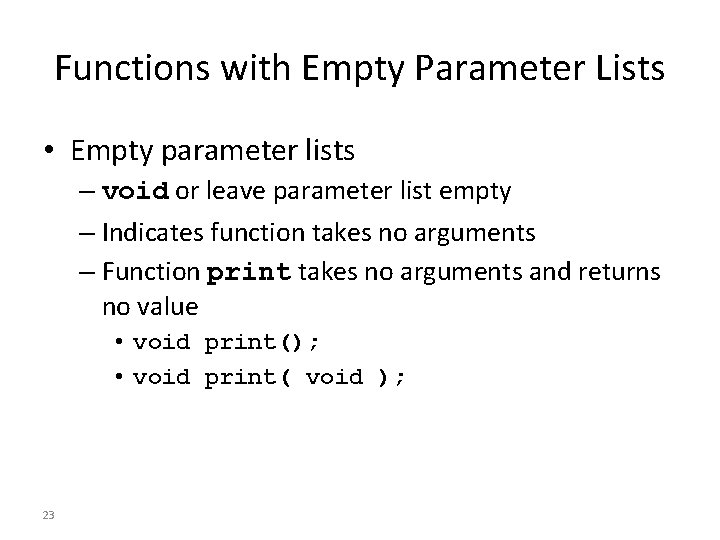
Functions with Empty Parameter Lists • Empty parameter lists – void or leave parameter list empty – Indicates function takes no arguments – Function print takes no arguments and returns no value • void print(); • void print( void ); 23
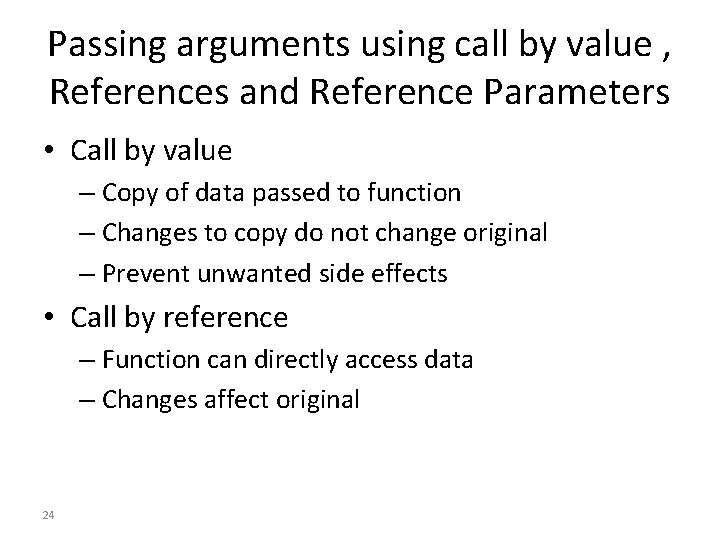
Passing arguments using call by value , References and Reference Parameters • Call by value – Copy of data passed to function – Changes to copy do not change original – Prevent unwanted side effects • Call by reference – Function can directly access data – Changes affect original 24
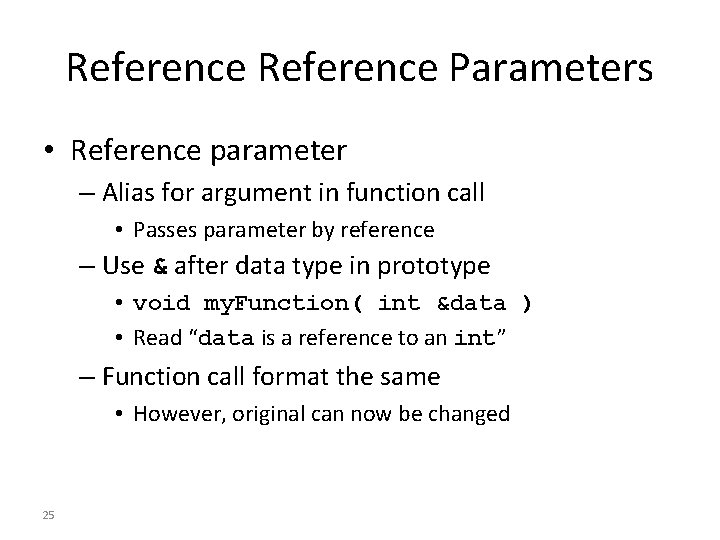
Reference Parameters • Reference parameter – Alias for argument in function call • Passes parameter by reference – Use & after data type in prototype • void my. Function( int &data ) • Read “data is a reference to an int” – Function call format the same • However, original can now be changed 25
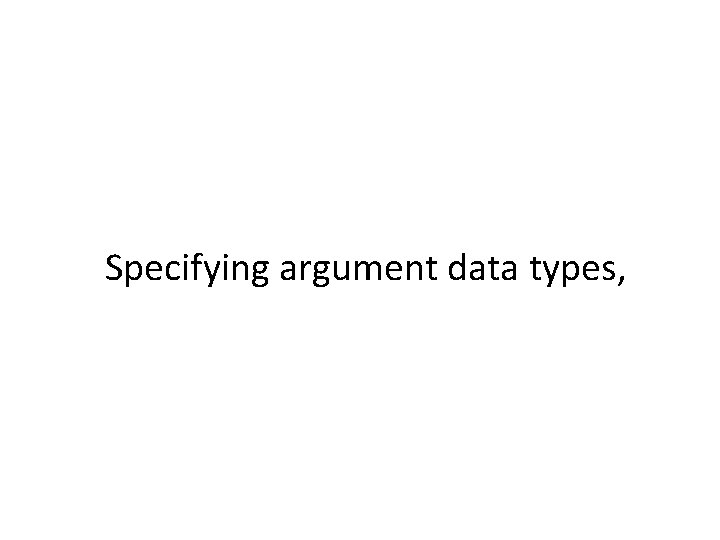
Specifying argument data types,
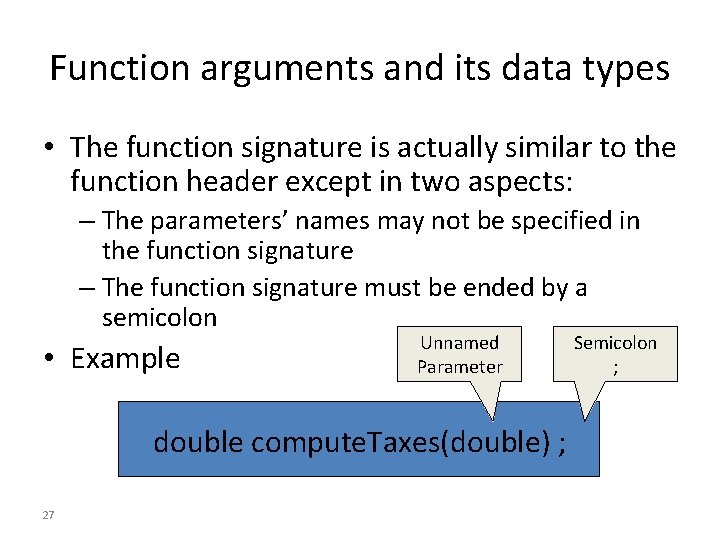
Function arguments and its data types • The function signature is actually similar to the function header except in two aspects: – The parameters’ names may not be specified in the function signature – The function signature must be ended by a semicolon • Example Unnamed Parameter double compute. Taxes(double) ; 27 Semicolon ;
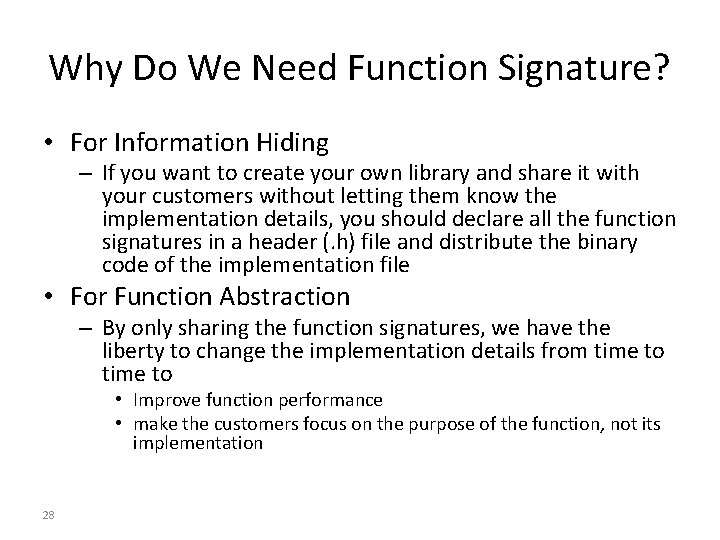
Why Do We Need Function Signature? • For Information Hiding – If you want to create your own library and share it with your customers without letting them know the implementation details, you should declare all the function signatures in a header (. h) file and distribute the binary code of the implementation file • For Function Abstraction – By only sharing the function signatures, we have the liberty to change the implementation details from time to • Improve function performance • make the customers focus on the purpose of the function, not its implementation 28
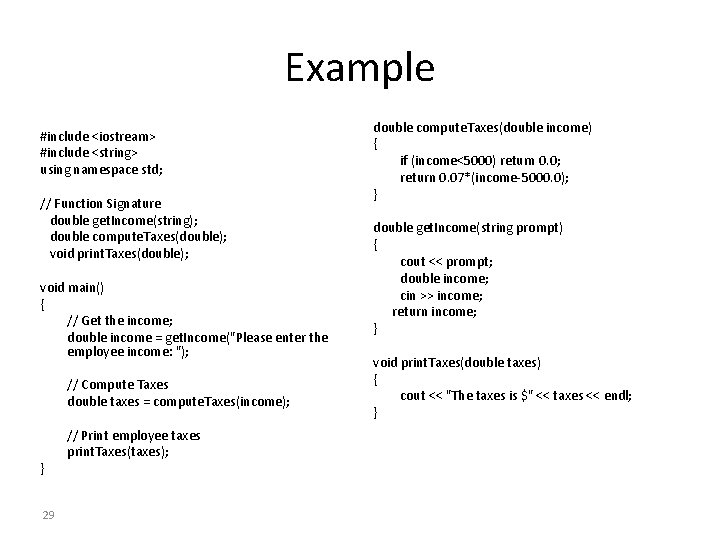
Example #include <iostream> #include <string> using namespace std; // Function Signature double get. Income(string); double compute. Taxes(double); void print. Taxes(double); void main() { // Get the income; double income = get. Income("Please enter the employee income: "); // Compute Taxes double taxes = compute. Taxes(income); } 29 // Print employee taxes print. Taxes(taxes); double compute. Taxes(double income) { if (income<5000) return 0. 0; return 0. 07*(income-5000. 0); } double get. Income(string prompt) { cout << prompt; double income; cin >> income; return income; } void print. Taxes(double taxes) { cout << "The taxes is $" << taxes << endl; }
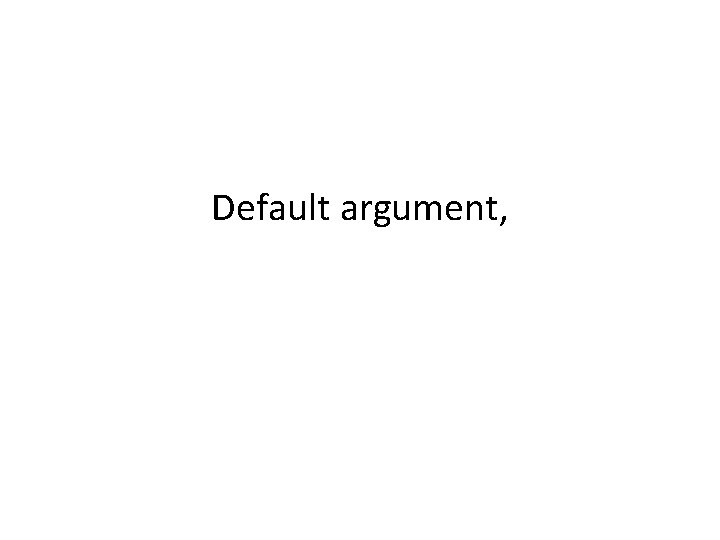
Default argument,
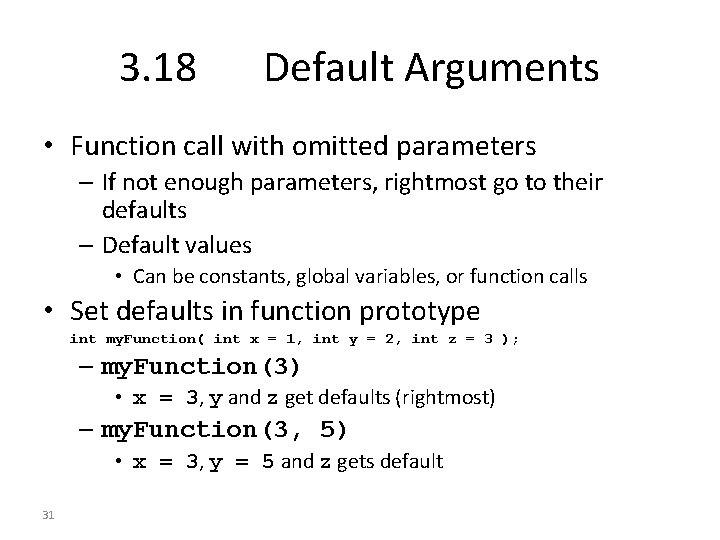
3. 18 Default Arguments • Function call with omitted parameters – If not enough parameters, rightmost go to their defaults – Default values • Can be constants, global variables, or function calls • Set defaults in function prototype int my. Function( int x = 1, int y = 2, int z = 3 ); – my. Function(3) • x = 3, y and z get defaults (rightmost) – my. Function(3, 5) • x = 3, y = 5 and z gets default 31
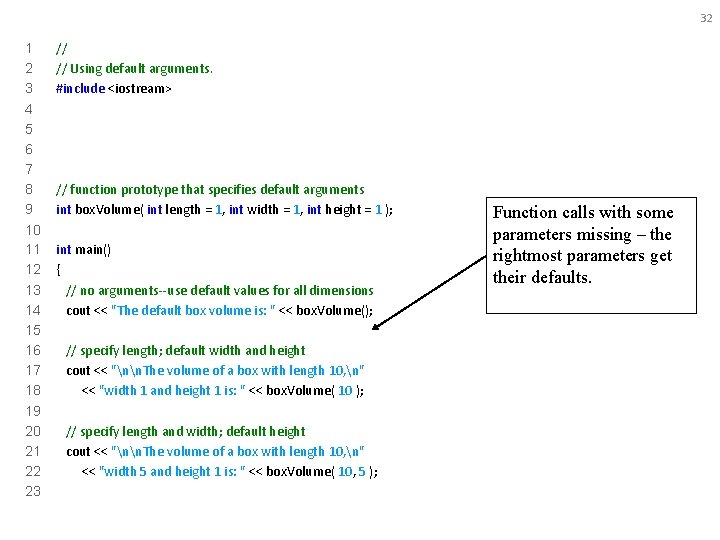
32 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 // // Using default arguments. #include <iostream> // function prototype that specifies default arguments int box. Volume( int length = 1, int width = 1, int height = 1 ); int main() { // no arguments--use default values for all dimensions cout << "The default box volume is: " << box. Volume(); // specify length; default width and height cout << "nn. The volume of a box with length 10, n" << "width 1 and height 1 is: " << box. Volume( 10 ); // specify length and width; default height cout << "nn. The volume of a box with length 10, n" << "width 5 and height 1 is: " << box. Volume( 10, 5 ); Function calls with some parameters missing – the rightmost parameters get their defaults.
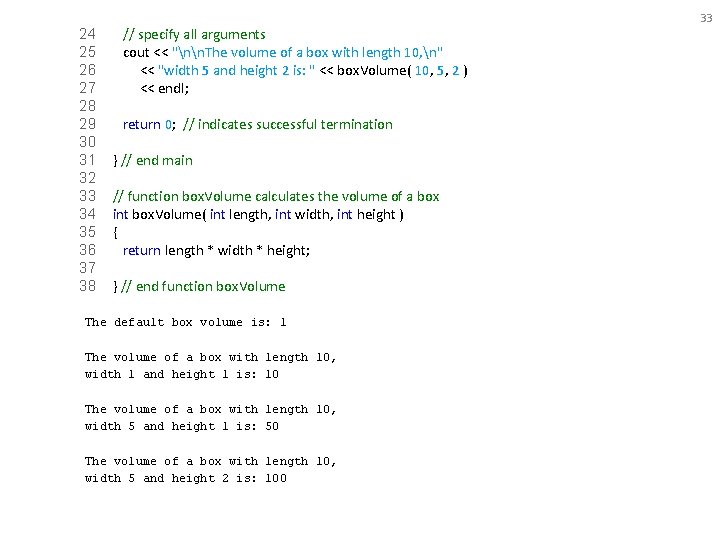
24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 // specify all arguments cout << "nn. The volume of a box with length 10, n" << "width 5 and height 2 is: " << box. Volume( 10, 5, 2 ) << endl; return 0; // indicates successful termination } // end main // function box. Volume calculates the volume of a box int box. Volume( int length, int width, int height ) { return length * width * height; } // end function box. Volume The default box volume is: 1 The volume of a box with length 10, width 1 and height 1 is: 10 The volume of a box with length 10, width 5 and height 1 is: 50 The volume of a box with length 10, width 5 and height 2 is: 100 33
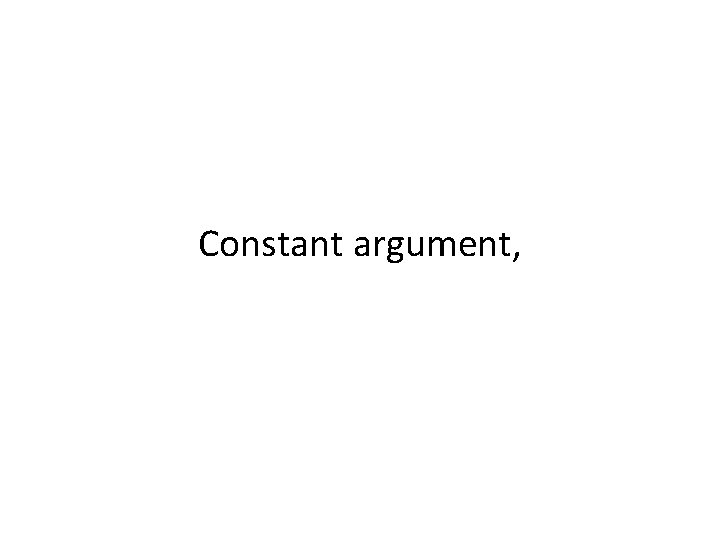
Constant argument,
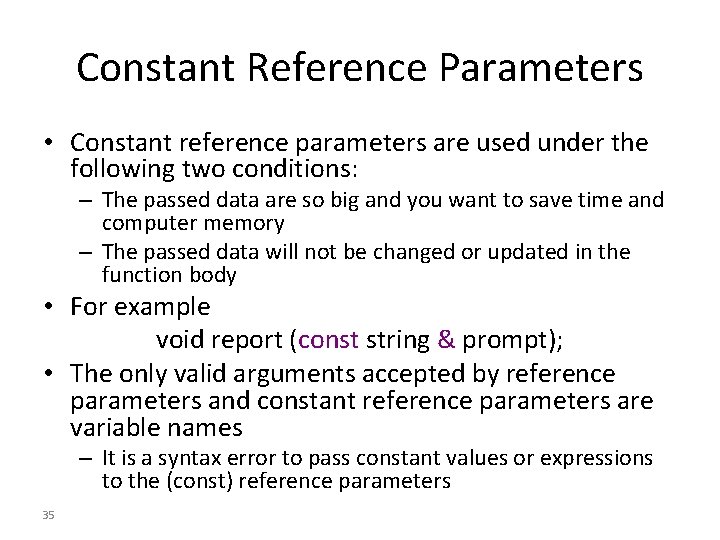
Constant Reference Parameters • Constant reference parameters are used under the following two conditions: – The passed data are so big and you want to save time and computer memory – The passed data will not be changed or updated in the function body • For example void report (const string & prompt); • The only valid arguments accepted by reference parameters and constant reference parameters are variable names – It is a syntax error to pass constant values or expressions to the (const) reference parameters 35
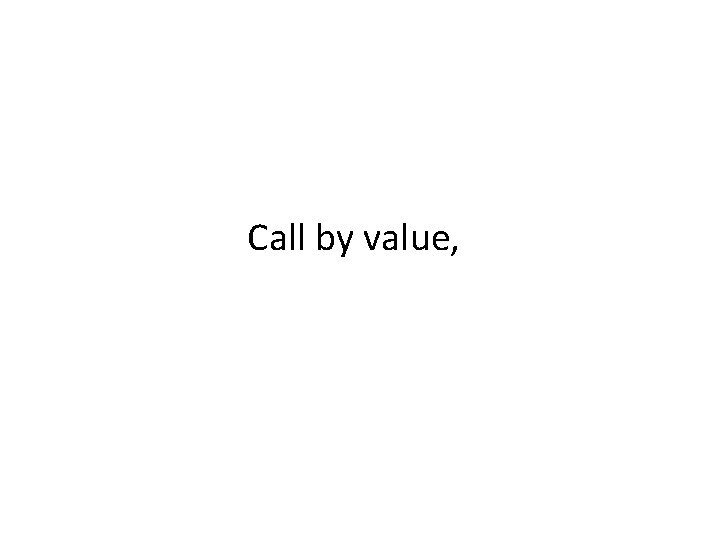
Call by value,
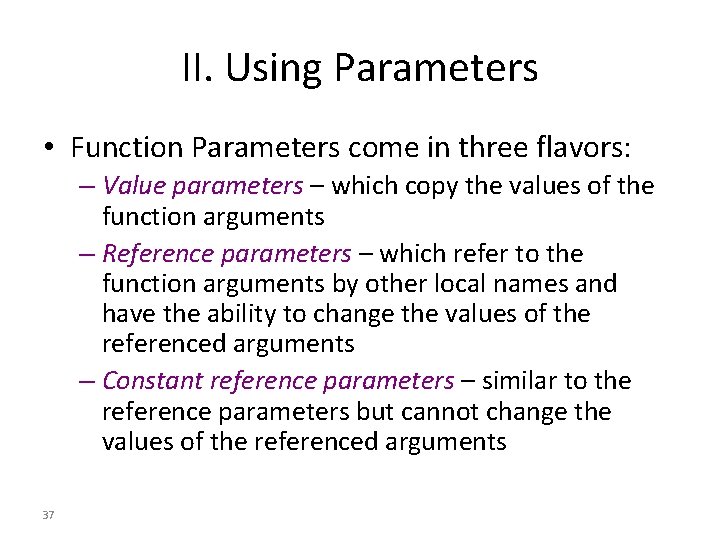
II. Using Parameters • Function Parameters come in three flavors: – Value parameters – which copy the values of the function arguments – Reference parameters – which refer to the function arguments by other local names and have the ability to change the values of the referenced arguments – Constant reference parameters – similar to the reference parameters but cannot change the values of the referenced arguments 37
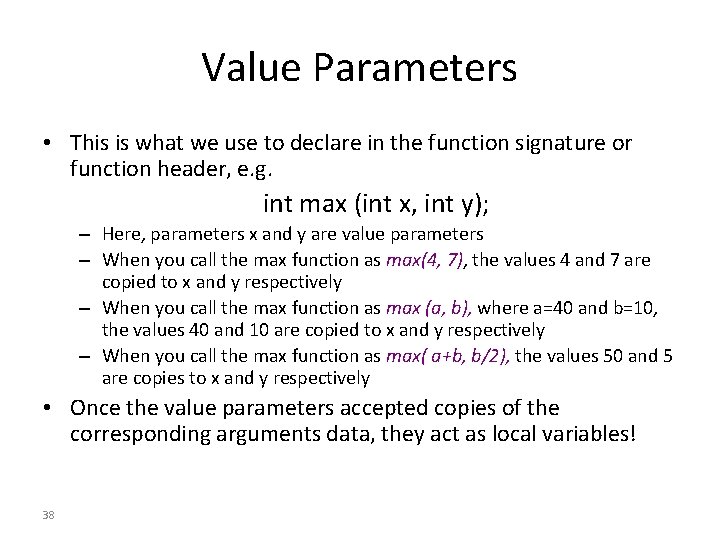
Value Parameters • This is what we use to declare in the function signature or function header, e. g. int max (int x, int y); – Here, parameters x and y are value parameters – When you call the max function as max(4, 7) the values 4 and 7 are copied to x and y respectively – When you call the max function as max (a, b), where a=40 and b=10, the values 40 and 10 are copied to x and y respectively – When you call the max function as max( a+b, b/2), the values 50 and 5 are copies to x and y respectively • Once the value parameters accepted copies of the corresponding arguments data, they act as local variables! 38
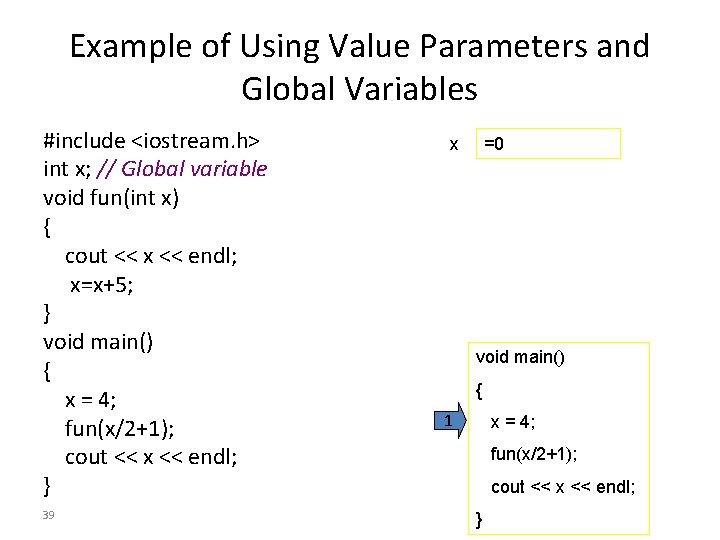
Example of Using Value Parameters and Global Variables #include <iostream. h> int x; // Global variable void fun(int x) { cout << x << endl; x=x+5; } void main() { x = 4; fun(x/2+1); cout << x << endl; } 39 =0 x void main() { 1 x = 4; fun(x/2+1); cout << x << endl; }
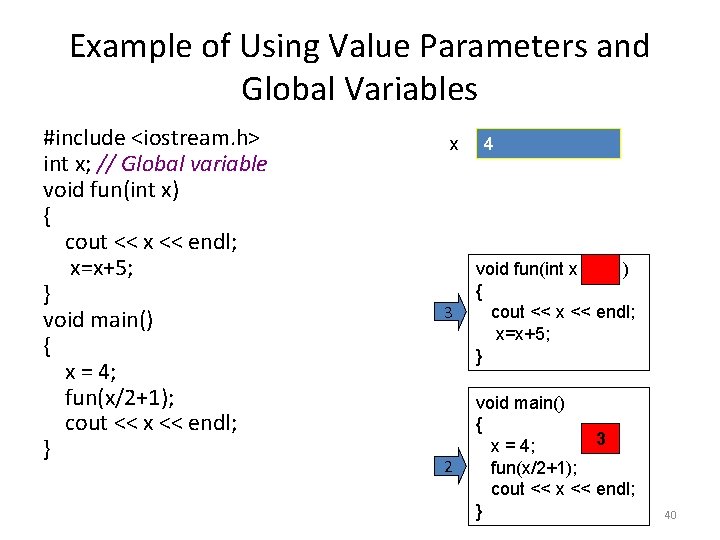
Example of Using Value Parameters and Global Variables #include <iostream. h> int x; // Global variable void fun(int x) { cout << x << endl; x=x+5; } void main() { x = 4; fun(x/2+1); cout << x << endl; } x 3 2 4 void fun(int x ) { cout << x << endl; x=x+5; } void main() { 3 x = 4; fun(x/2+1); cout << x << endl; } 40
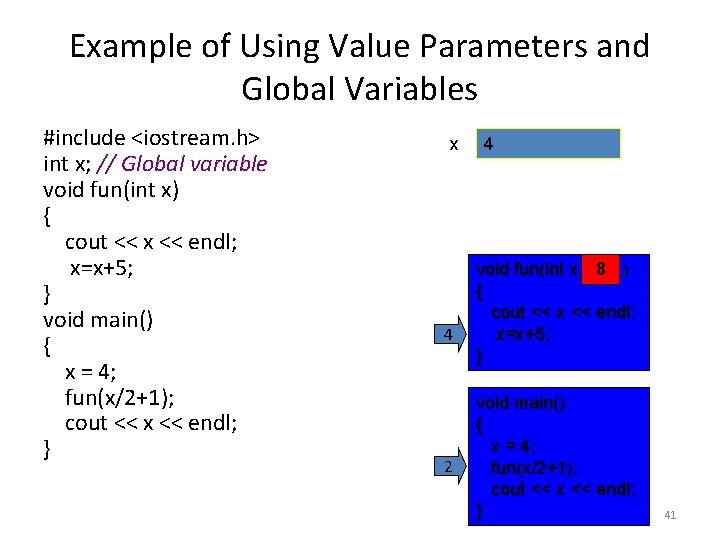
Example of Using Value Parameters and Global Variables #include <iostream. h> int x; // Global variable void fun(int x) { cout << x << endl; x=x+5; } void main() { x = 4; fun(x/2+1); cout << x << endl; } x 4 2 4 void fun(int x 8 3 ) { cout << x << endl; x=x+5; } void main() { x = 4; fun(x/2+1); cout << x << endl; } 41
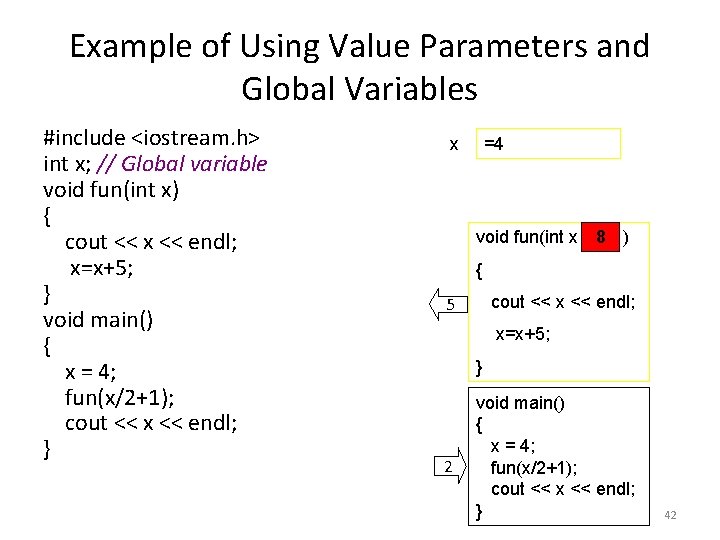
Example of Using Value Parameters and Global Variables #include <iostream. h> int x; // Global variable void fun(int x) { cout << x << endl; x=x+5; } void main() { x = 4; fun(x/2+1); cout << x << endl; } =4 x void fun(int x 8 ) { cout << x << endl; 5 x=x+5; } 2 void main() { x = 4; fun(x/2+1); cout << x << endl; } 42
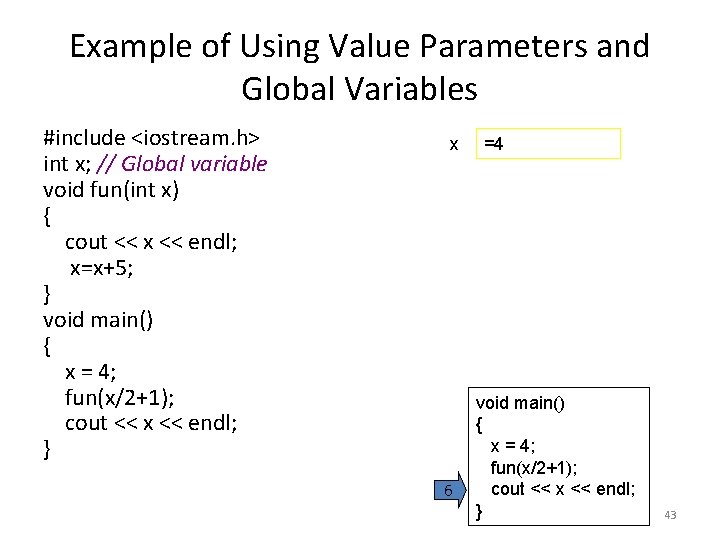
Example of Using Value Parameters and Global Variables #include <iostream. h> int x; // Global variable void fun(int x) { cout << x << endl; x=x+5; } void main() { x = 4; fun(x/2+1); cout << x << endl; } x 6 =4 void main() { x = 4; fun(x/2+1); cout << x << endl; } 43
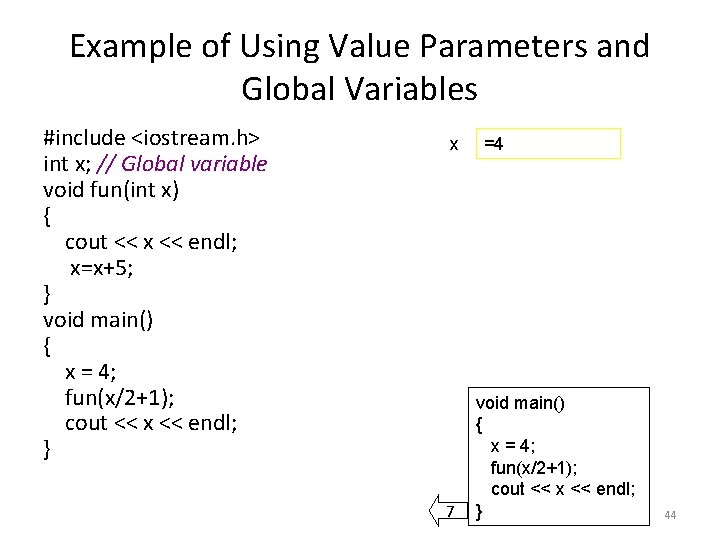
Example of Using Value Parameters and Global Variables #include <iostream. h> int x; // Global variable void fun(int x) { cout << x << endl; x=x+5; } void main() { x = 4; fun(x/2+1); cout << x << endl; } x 7 =4 void main() { x = 4; fun(x/2+1); cout << x << endl; } 44
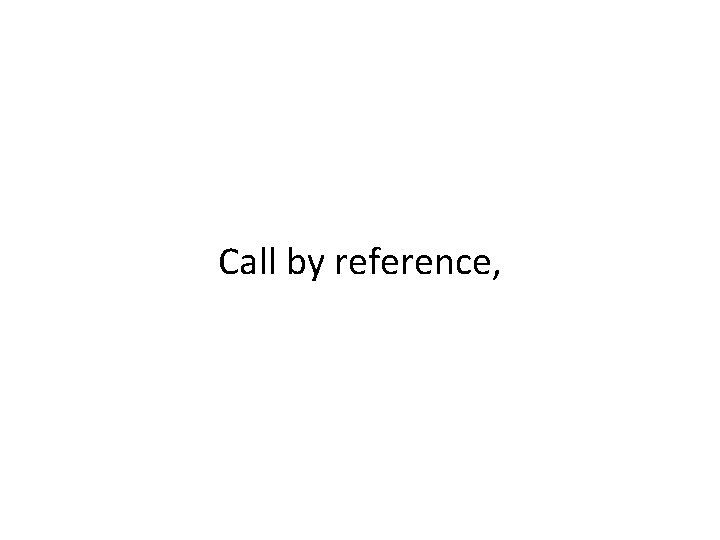
Call by reference,
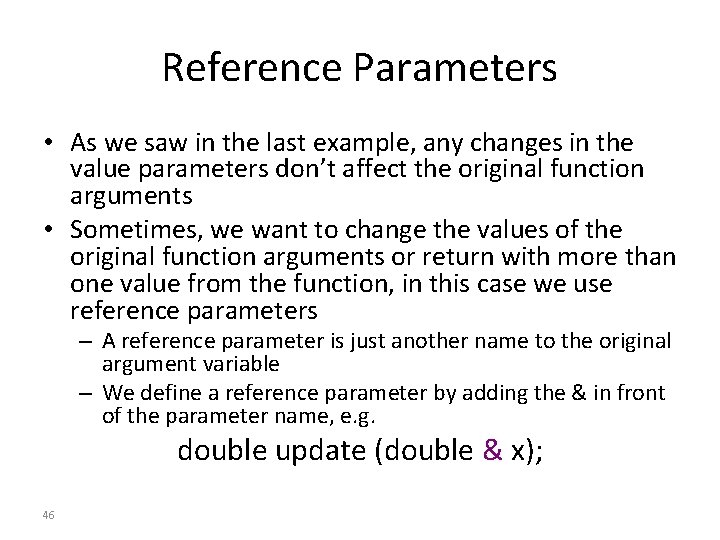
Reference Parameters • As we saw in the last example, any changes in the value parameters don’t affect the original function arguments • Sometimes, we want to change the values of the original function arguments or return with more than one value from the function, in this case we use reference parameters – A reference parameter is just another name to the original argument variable – We define a reference parameter by adding the & in front of the parameter name, e. g. double update (double & x); 46
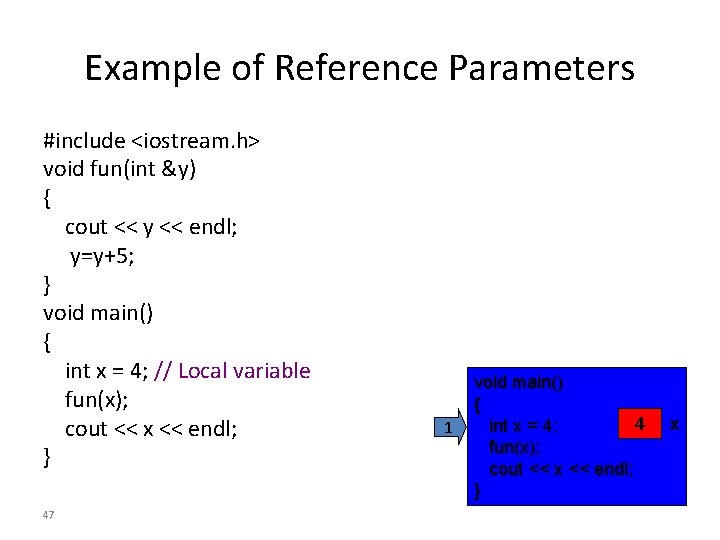
Example of Reference Parameters #include <iostream. h> void fun(int &y) { cout << y << endl; y=y+5; } void main() { int x = 4; // Local variable fun(x); cout << x << endl; } 47 1 void main() { ? 4 int x = 4; fun(x); cout << x << endl; } x
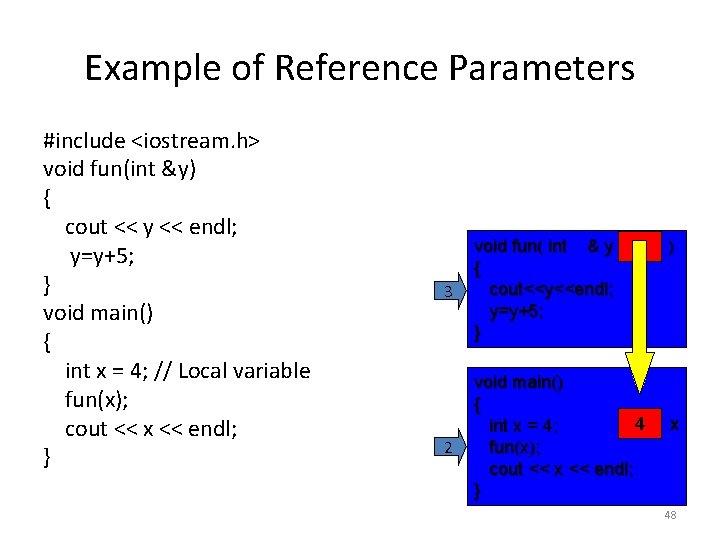
Example of Reference Parameters #include <iostream. h> void fun(int &y) { cout << y << endl; y=y+5; } void main() { int x = 4; // Local variable fun(x); cout << x << endl; } 3 2 void fun( int & y { cout<<y<<endl; y=y+5; } void main() { ? 4 int x = 4; fun(x); cout << x << endl; } ) x 48
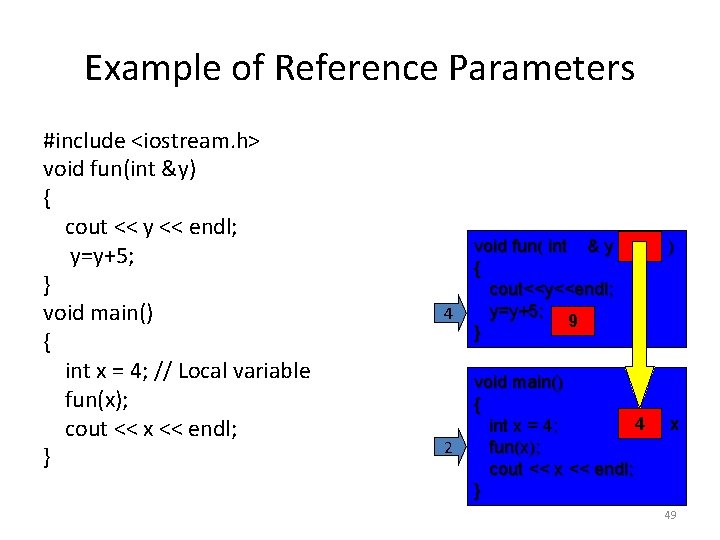
Example of Reference Parameters #include <iostream. h> void fun(int &y) { cout << y << endl; y=y+5; } void main() { int x = 4; // Local variable fun(x); cout << x << endl; } 4 2 void fun( int & y { cout<<y<<endl; y=y+5; 9 } void main() { ? 4 int x = 4; fun(x); cout << x << endl; } ) x 49
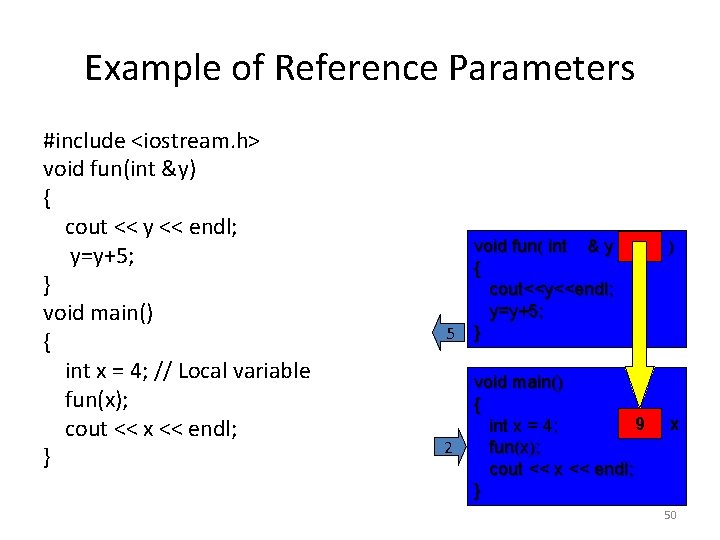
Example of Reference Parameters #include <iostream. h> void fun(int &y) { cout << y << endl; y=y+5; } void main() { int x = 4; // Local variable fun(x); cout << x << endl; } 5 2 void fun( int & y { cout<<y<<endl; y=y+5; } void main() { ? 9 int x = 4; fun(x); cout << x << endl; } ) x 50
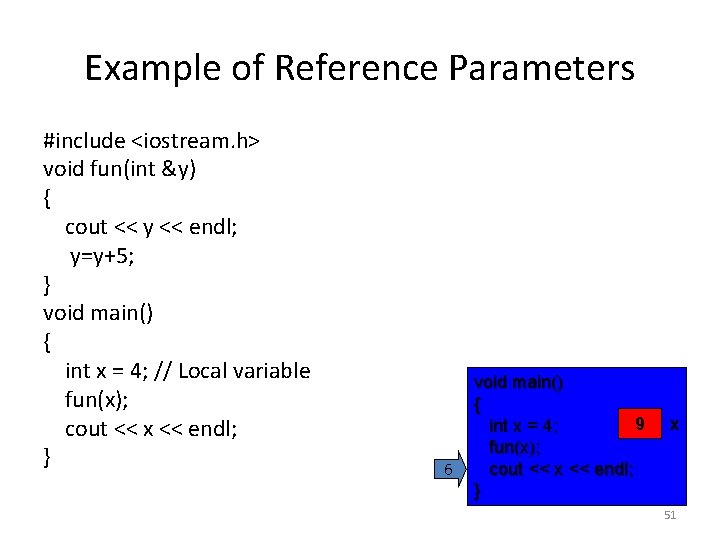
Example of Reference Parameters #include <iostream. h> void fun(int &y) { cout << y << endl; y=y+5; } void main() { int x = 4; // Local variable fun(x); cout << x << endl; } 6 void main() { ? 9 int x = 4; fun(x); cout << x << endl; } x 51
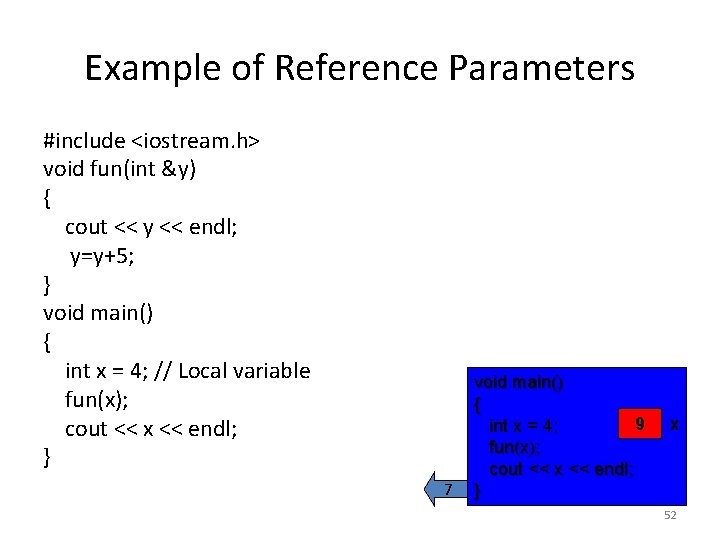
Example of Reference Parameters #include <iostream. h> void fun(int &y) { cout << y << endl; y=y+5; } void main() { int x = 4; // Local variable fun(x); cout << x << endl; } 7 void main() { ? 9 int x = 4; fun(x); cout << x << endl; } x 52
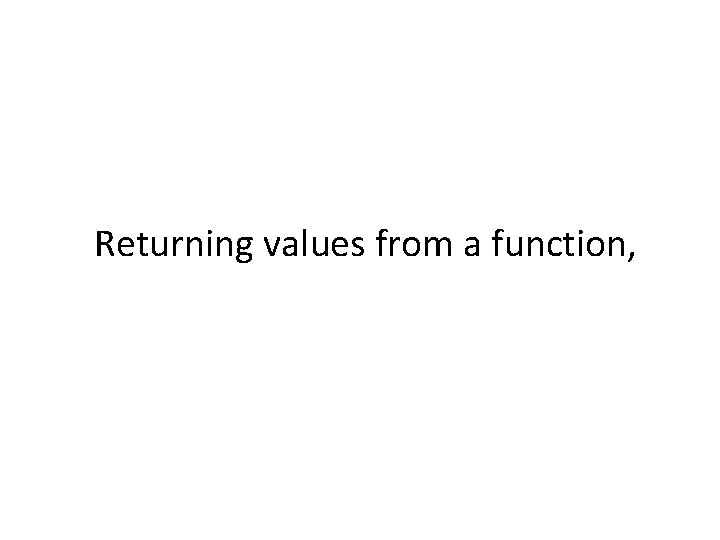
Returning values from a function,
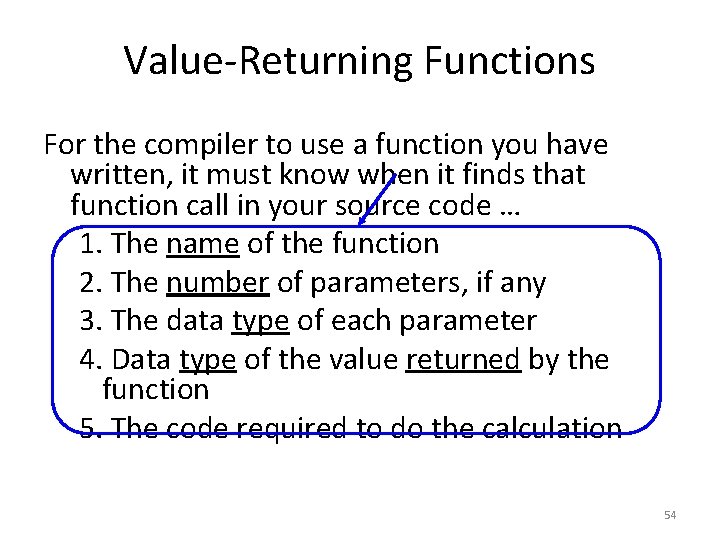
Value-Returning Functions For the compiler to use a function you have written, it must know when it finds that function call in your source code … 1. The name of the function 2. The number of parameters, if any 3. The data type of each parameter 4. Data type of the value returned by the function 5. The code required to do the calculation 54
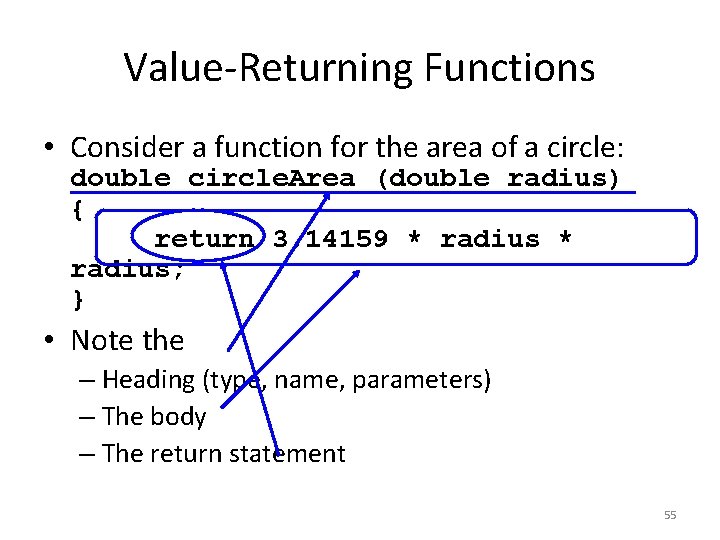
Value-Returning Functions • Consider a function for the area of a circle: double circle. Area (double radius) { return 3. 14159 * radius; } • Note the – Heading (type, name, parameters) – The body – The return statement 55
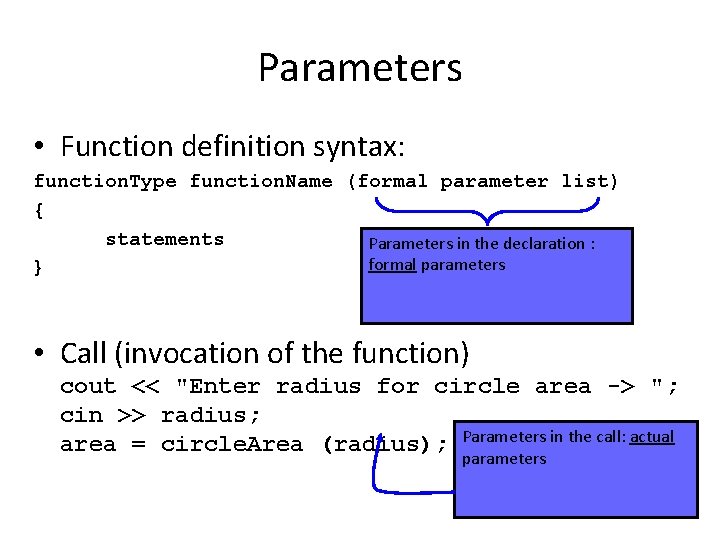
Parameters • Function definition syntax: function. Type function. Name (formal parameter list) { statements Parameters in the declaration : formal parameters } • Call (invocation of the function) cout << "Enter radius for circle area -> "; cin >> radius; area = circle. Area (radius); Parameters in the call: actual parameters 56
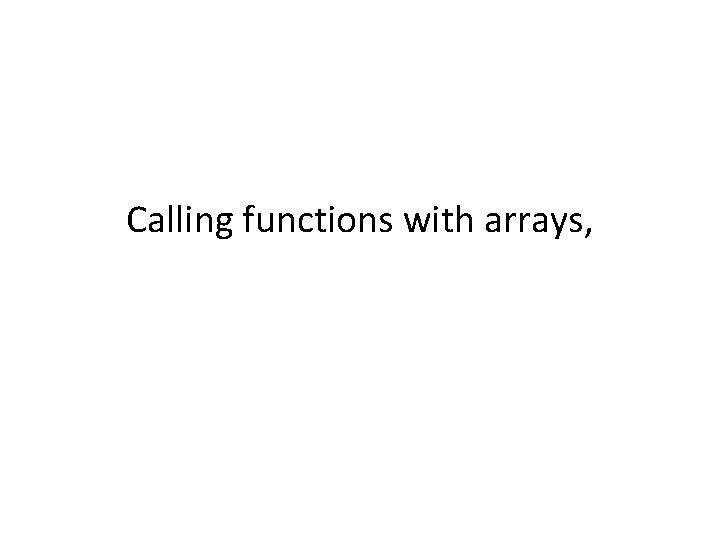
Calling functions with arrays,
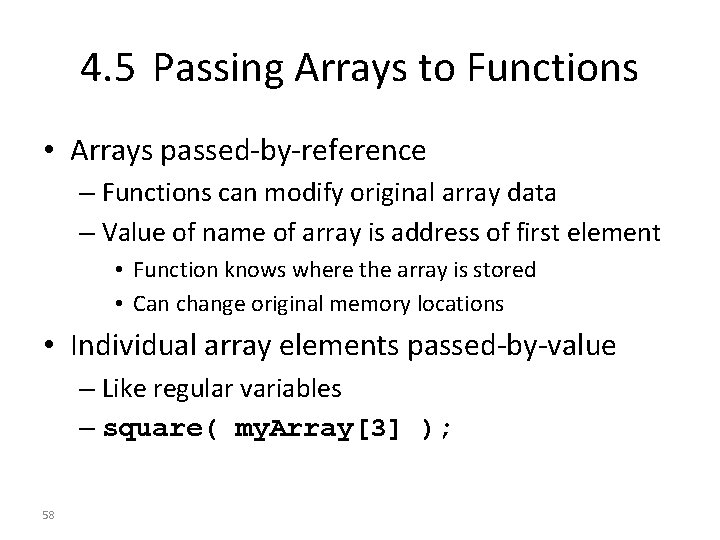
4. 5 Passing Arrays to Functions • Arrays passed-by-reference – Functions can modify original array data – Value of name of array is address of first element • Function knows where the array is stored • Can change original memory locations • Individual array elements passed-by-value – Like regular variables – square( my. Array[3] ); 58
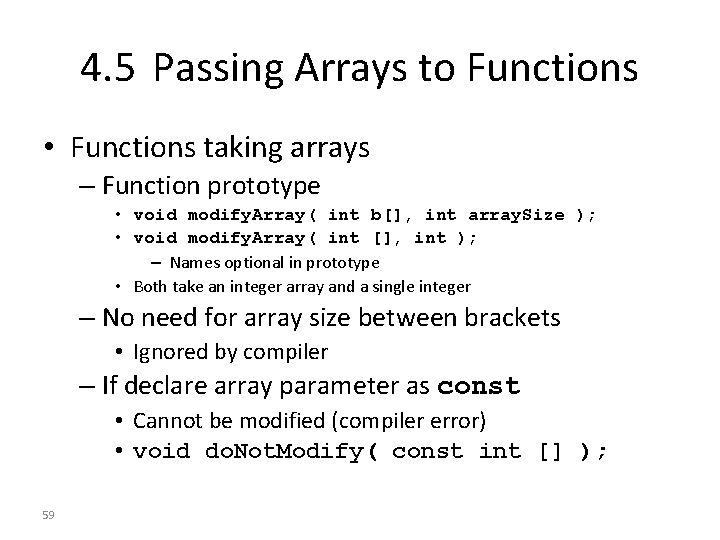
4. 5 Passing Arrays to Functions • Functions taking arrays – Function prototype • void modify. Array( int b[], int array. Size ); • void modify. Array( int [], int ); – Names optional in prototype • Both take an integer array and a single integer – No need for array size between brackets • Ignored by compiler – If declare array parameter as const • Cannot be modified (compiler error) • void do. Not. Modify( const int [] ); 59
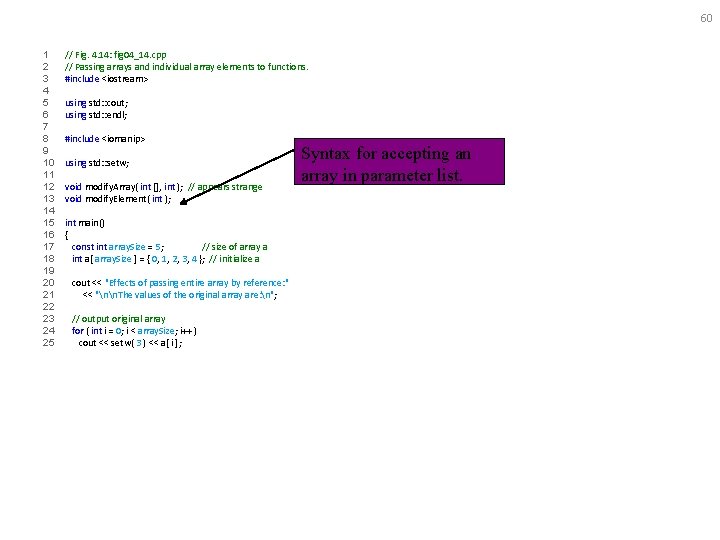
60 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 // Fig. 4. 14: fig 04_14. cpp // Passing arrays and individual array elements to functions. #include <iostream> using std: : cout; using std: : endl; #include <iomanip> using std: : setw; void modify. Array( int [], int ); // appears strange void modify. Element( int ); int main() { const int array. Size = 5; // size of array a int a[ array. Size ] = { 0, 1, 2, 3, 4 }; // initialize a cout << "Effects of passing entire array by reference: " << "nn. The values of the original array are: n"; // output original array for ( int i = 0; i < array. Size; i++ ) cout << setw( 3 ) << a[ i ]; Syntax for accepting an array in parameter list.
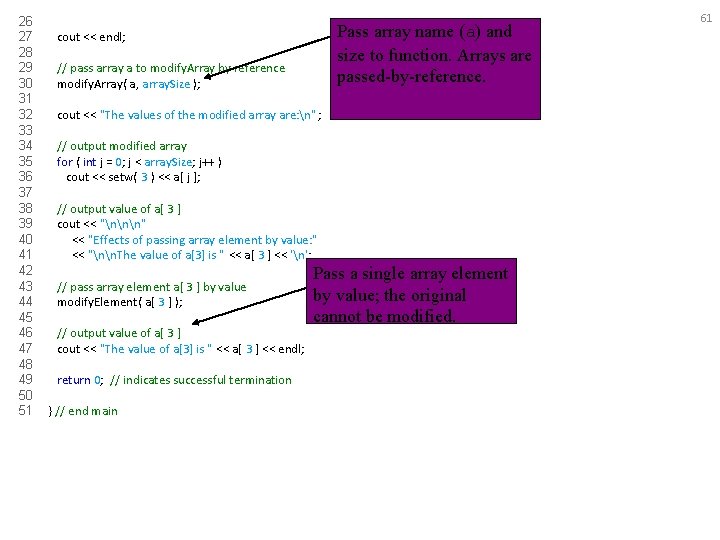
26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 Pass array name (a) and size to function. Arrays are passed-by-reference. cout << endl; // pass array a to modify. Array by reference modify. Array( a, array. Size ); cout << "The values of the modified array are: n" ; // output modified array for ( int j = 0; j < array. Size; j++ ) cout << setw( 3 ) << a[ j ]; // output value of a[ 3 ] cout << "nnn" << "Effects of passing array element by value: " << "nn. The value of a[3] is " << a[ 3 ] << 'n'; // pass array element a[ 3 ] by value modify. Element( a[ 3 ] ); // output value of a[ 3 ] cout << "The value of a[3] is " << a[ 3 ] << endl; return 0; // indicates successful termination } // end main Pass a single array element by value; the original cannot be modified. 61
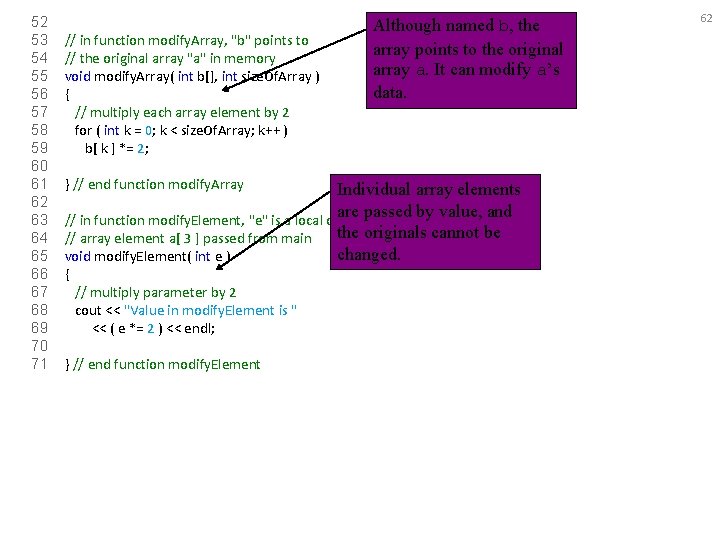
52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 // in function modify. Array, "b" points to // the original array "a" in memory void modify. Array( int b[], int size. Of. Array ) { // multiply each array element by 2 for ( int k = 0; k < size. Of. Array; k++ ) b[ k ] *= 2; } // end function modify. Array Although named b, the array points to the original array a. It can modify a’s data. Individual array elements are passed by value, and // in function modify. Element, "e" is a local copy of the originals cannot be // array element a[ 3 ] passed from main changed. void modify. Element( int e ) { // multiply parameter by 2 cout << "Value in modify. Element is " << ( e *= 2 ) << endl; } // end function modify. Element 62
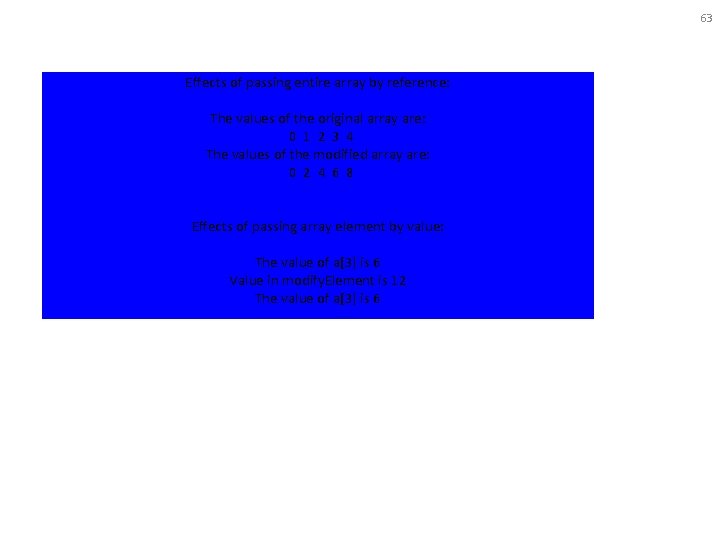
63 Effects of passing entire array by reference: The values of the original array are: 0 1 2 3 4 The values of the modified array are: 0 2 4 6 8 fig 04_14. cpp output (1 of 1) Effects of passing array element by value: The value of a[3] is 6 Value in modify. Element is 12 The value of a[3] is 6
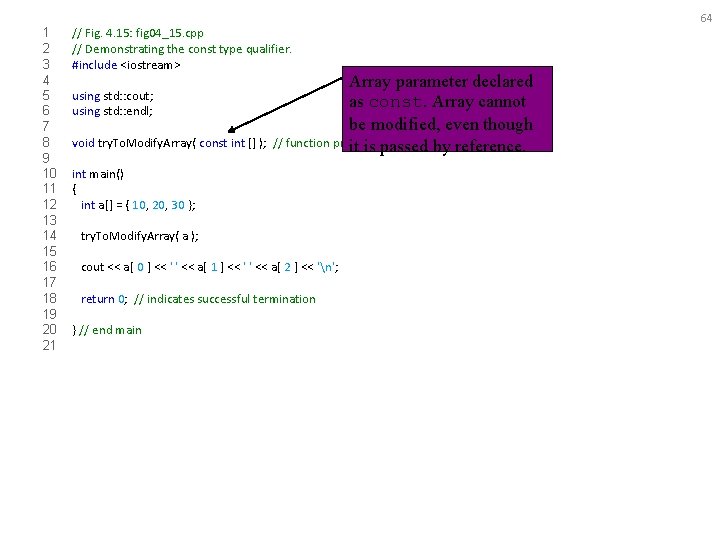
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 // Fig. 4. 15: fig 04_15. cpp // Demonstrating the const type qualifier. #include <iostream> using std: : cout; using std: : endl; void try. To. Modify. Array( const int [] ); Array parameter declared as const. Array cannot be modified, even though // function prototype it is passed by reference. int main() { int a[] = { 10, 20, 30 }; try. To. Modify. Array( a ); cout << a[ 0 ] << ' ' << a[ 1 ] << ' ' << a[ 2 ] << 'n'; return 0; // indicates successful termination } // end main 64
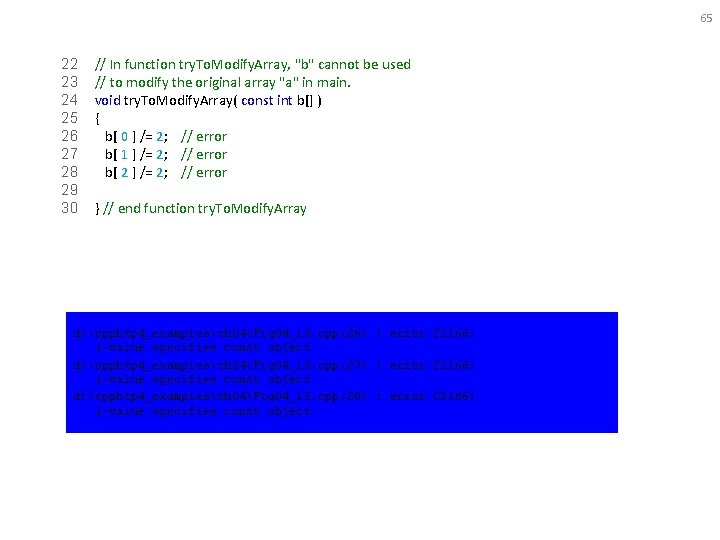
65 22 23 24 25 26 27 28 29 30 // In function try. To. Modify. Array, "b" cannot be used // to modify the original array "a" in main. void try. To. Modify. Array( const int b[] ) { b[ 0 ] /= 2; // error b[ 1 ] /= 2; // error b[ 2 ] /= 2; // error } // end function try. To. Modify. Array d: cpphtp 4_examplesch 04Fig 04_15. cpp(26) : error C 2166: l-value specifies const object d: cpphtp 4_examplesch 04Fig 04_15. cpp(27) : error C 2166: l-value specifies const object d: cpphtp 4_examplesch 04Fig 04_15. cpp(28) : error C 2166: l-value specifies const object
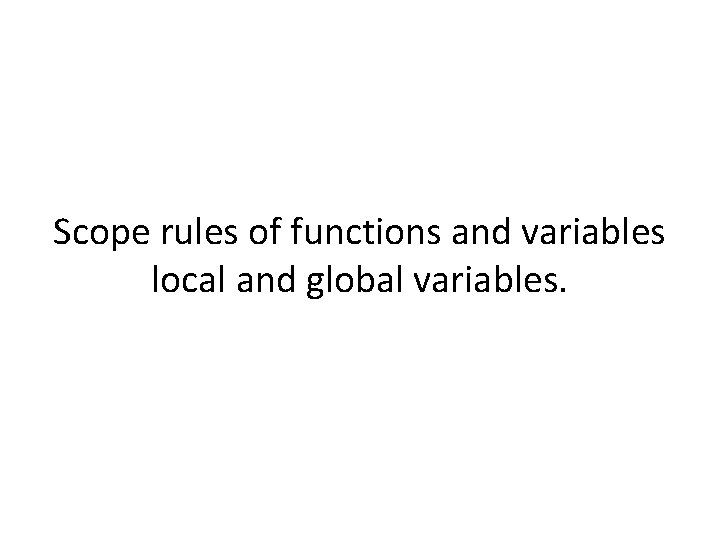
Scope rules of functions and variables local and global variables.
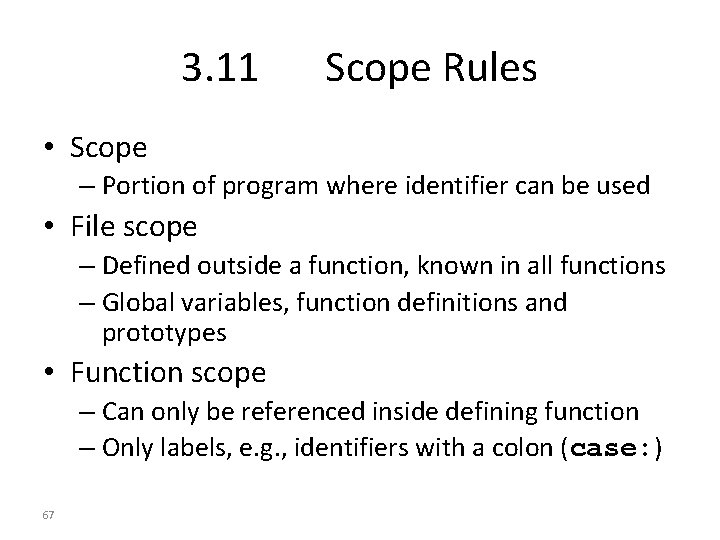
3. 11 Scope Rules • Scope – Portion of program where identifier can be used • File scope – Defined outside a function, known in all functions – Global variables, function definitions and prototypes • Function scope – Can only be referenced inside defining function – Only labels, e. g. , identifiers with a colon (case: ) 67
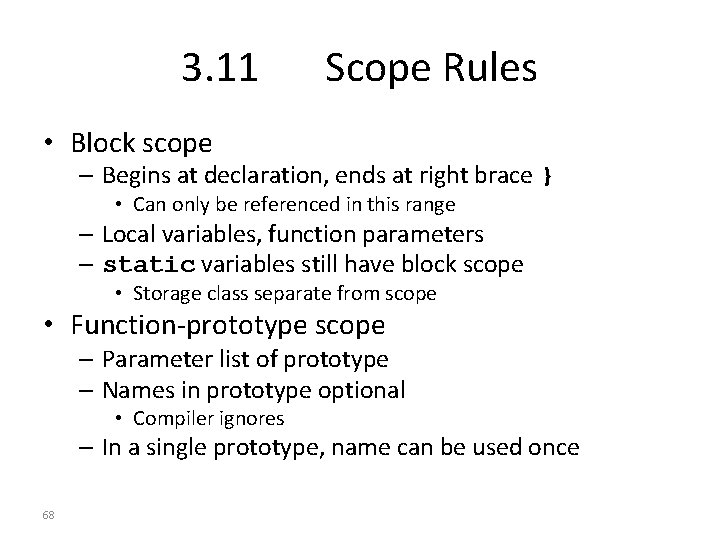
3. 11 Scope Rules • Block scope – Begins at declaration, ends at right brace } • Can only be referenced in this range – Local variables, function parameters – static variables still have block scope • Storage class separate from scope • Function-prototype scope – Parameter list of prototype – Names in prototype optional • Compiler ignores – In a single prototype, name can be used once 68
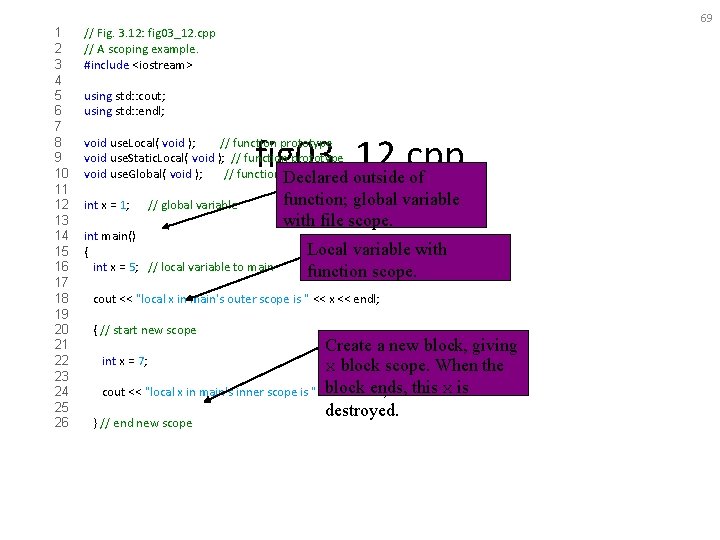
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 69 // Fig. 3. 12: fig 03_12. cpp // A scoping example. #include <iostream> using std: : cout; using std: : endl; fig 03_12. cpp outside of function; global variable (1 of 5) with file scope. void use. Local( void ); // function prototype void use. Static. Local( void ); // function prototype void use. Global( void ); // function Declared prototype int x = 1; // global variable int main() { int x = 5; // local variable to main Local variable with function scope. cout << "local x in main's outer scope is " << x << endl; { // start new scope Create a new block, giving int x = 7; x block scope. When the block ends, this x is cout << "local x in main's inner scope is " << x << endl; destroyed. } // end new scope
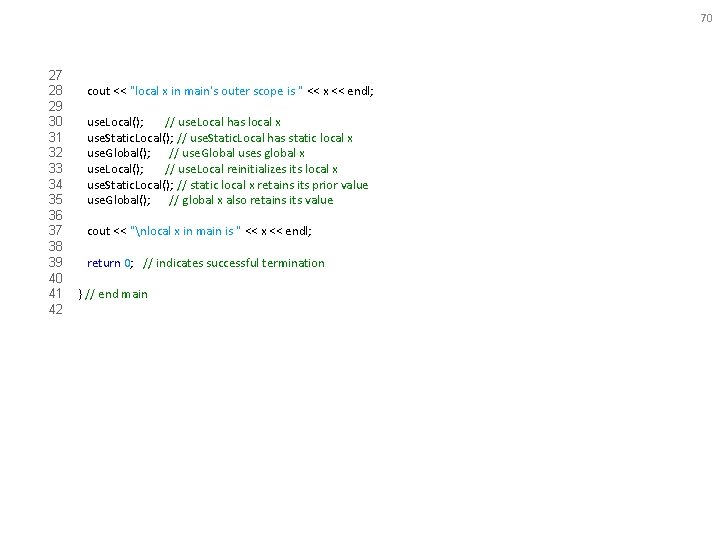
70 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 cout << "local x in main's outer scope is " << x << endl; use. Local(); // use. Local has local x use. Static. Local(); // use. Static. Local has static local x use. Global(); // use. Global uses global x use. Local(); // use. Local reinitializes its local x use. Static. Local(); // static local x retains its prior value use. Global(); // global x also retains its value cout << "nlocal x in main is " << x << endl; return 0; // indicates successful termination } // end main
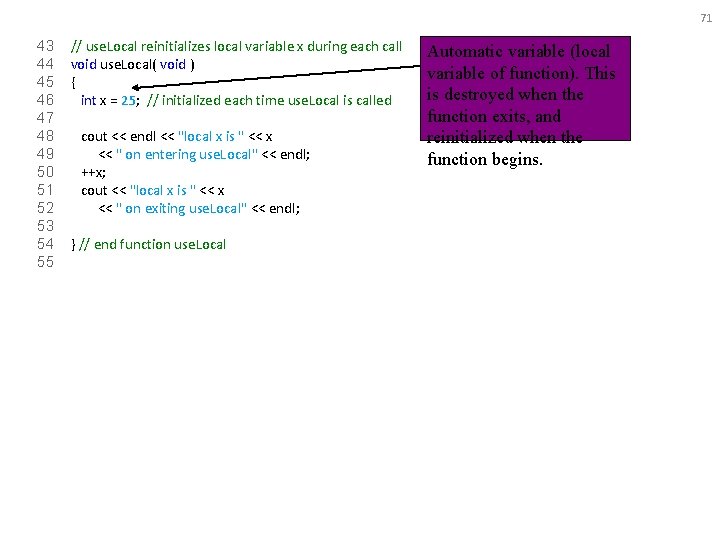
71 43 44 45 46 47 48 49 50 51 52 53 54 55 // use. Local reinitializes local variable x during each call void use. Local( void ) { int x = 25; // initialized each time use. Local is called cout << endl << "local x is " << x << " on entering use. Local" << endl; ++x; cout << "local x is " << x << " on exiting use. Local" << endl; } // end function use. Local Automatic variable (local variable of function). This is destroyed when the function exits, and reinitialized when the function begins.
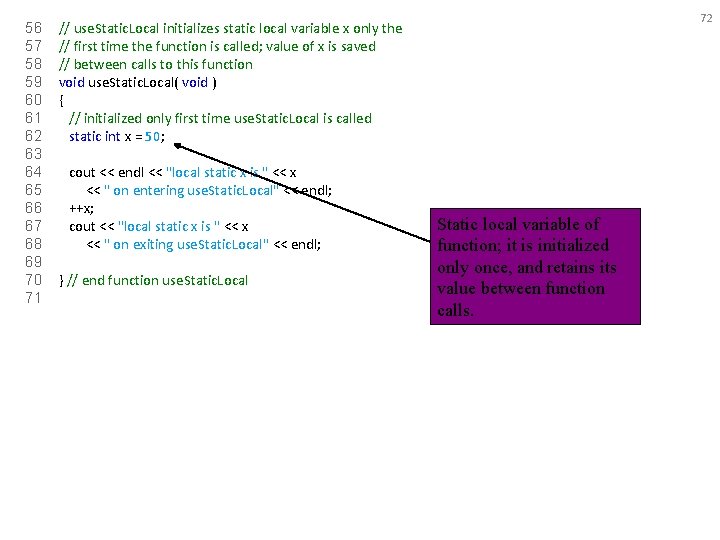
56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 // use. Static. Local initializes static local variable x only the // first time the function is called; value of x is saved // between calls to this function void use. Static. Local( void ) { // initialized only first time use. Static. Local is called static int x = 50; cout << endl << "local static x is " << x << " on entering use. Static. Local" << endl; ++x; cout << "local static x is " << x << " on exiting use. Static. Local" << endl; } // end function use. Static. Local Static local variable of function; it is initialized only once, and retains its value between function calls.
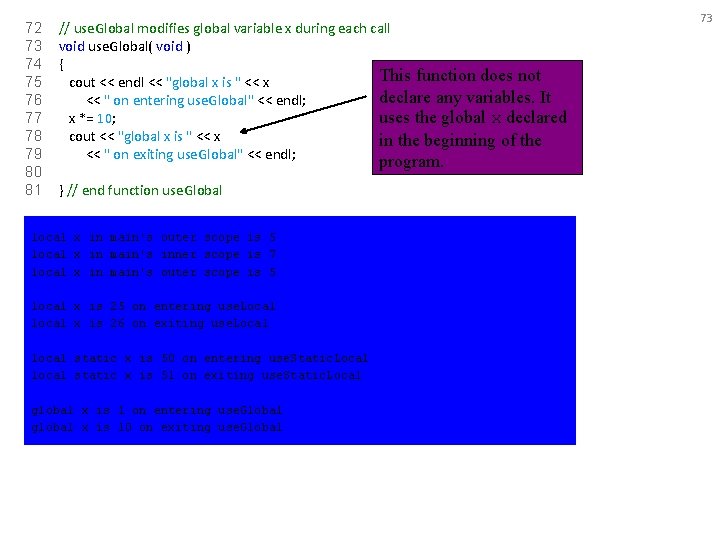
72 73 74 75 76 77 78 79 80 81 // use. Global modifies global variable x during each call void use. Global( void ) { This function does not cout << endl << "global x is " << x declare any variables. It << " on entering use. Global" << endl; uses the global x declared x *= 10; cout << "global x is " << x in the beginning of the << " on exiting use. Global" << endl; program. } // end function use. Global local x in main's outer scope is 5 local x in main's inner scope is 7 local x in main's outer scope is 5 local x is 25 on entering use. Local local x is 26 on exiting use. Local local static x is 50 on entering use. Static. Local local static x is 51 on exiting use. Static. Local global x is 1 on entering use. Global global x is 10 on exiting use. Global 73
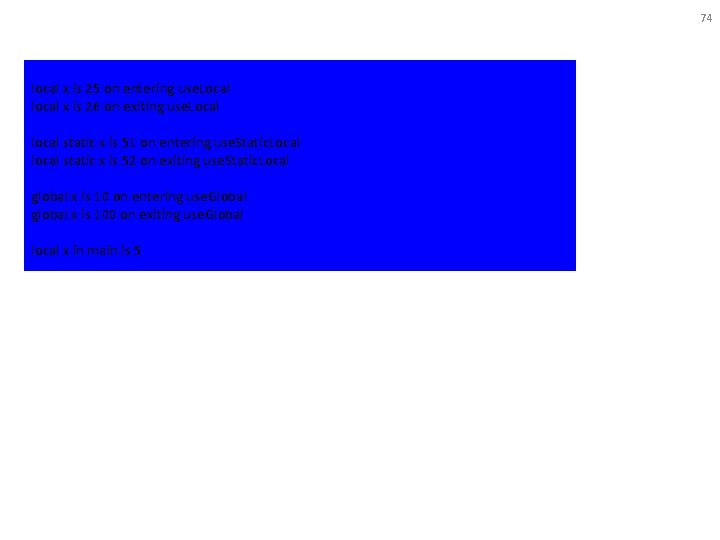
74 local x is 25 on entering use. Local local x is 26 on exiting use. Local local static x is 51 on entering use. Static. Local local static x is 52 on exiting use. Static. Local global x is 10 on entering use. Global global x is 100 on exiting use. Global local x in main is 5
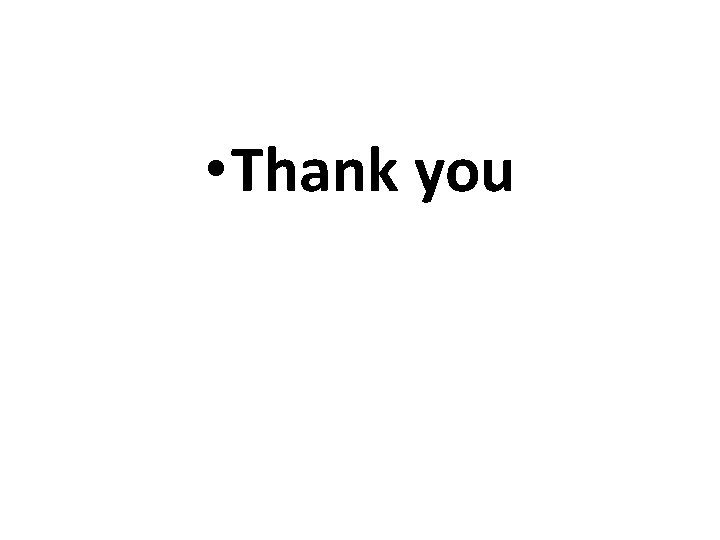
• Thank you
Absolute value function to piecewise function
How to solve evaluating functions
Evaluating functions and operations on functions
Introduction to quadratic function
Introduction to piecewise functions
Rational function rules
Parent function project
Codomain
Section 3 introduction to functions
Identify the parent function.
Introduction to logarithmic functions
Rational functions parent function
Asymptote transformations
Exponetial parent function
Function vocabulary math
Essay structure introduction
Why study thermodynamics
Pressure is state function or path function
Logarithmic parent function
Linear function vs nonlinear function
Pressure is state function or path function
Function and relation
Unit 4 linear equations
How to identify a polynomial function
A rational function is a function of the form
How a predicate function become a propositional function?
Quadratic cubic linear
Find the inverse of function f. a. b. c. d.
Composite exponential function
Composite fx
Rumus penerimaan
Polynomial function parent function
State function and path function in thermodynamics
College algebra polynomials
Function prototype and function definition
Article writing school magazine
Persuasive topics for year 9
Thematic essays
Behavioural training topics
Skill 21 draw conclusions about who what when where
What is competency based interview
Surprising reversal strategy
Theme of love in the great gatsby
Thematic statement structure
Toastmasters table topics questions
Subjective and objective writing
Solo talk examples
Software project management topics
Sociolinguistics topics for presentation
Smaw topics
Pertanyaan untuk pemateri seminar
Adventist youth ministries
Primary six science topics
Customer service topics for discussion
Problem solution essay thesis
Philosophical chairs topics
Problem solution persuasive speech
Leadership management topics
Leaflet writting
In an outline the relationship of topics
Literacy test essay examples
Features of a newspaper
Multi genre project topics
Introduction of value education
Types of information systems
Vts-0fxyt-e -site:youtube.com
Safety topics for february
Scope of operations research
Kubernetes topics
Hosa extemporaneous health poster
Original oratory topics
Sample lac session topics
Example history ia
Ib english b identities
Current topics in sports nutrition
Questions about leisure activities