FOR LOOPS UNISA COS 1511 Tutor Ed Edmundvtutoronline
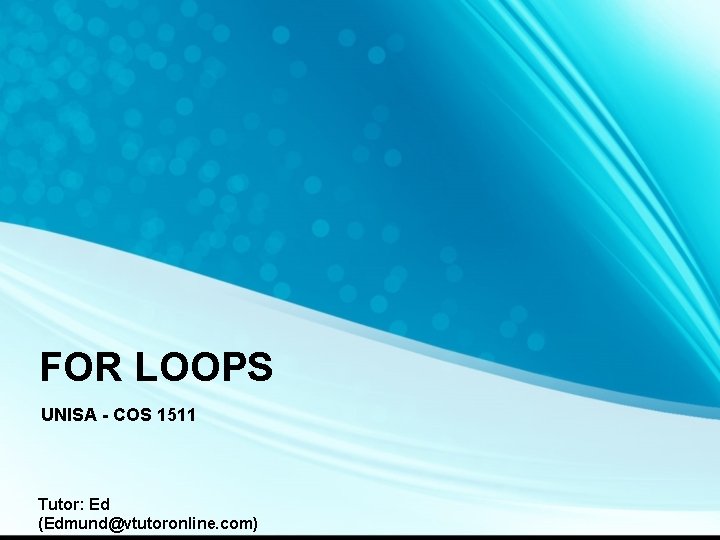
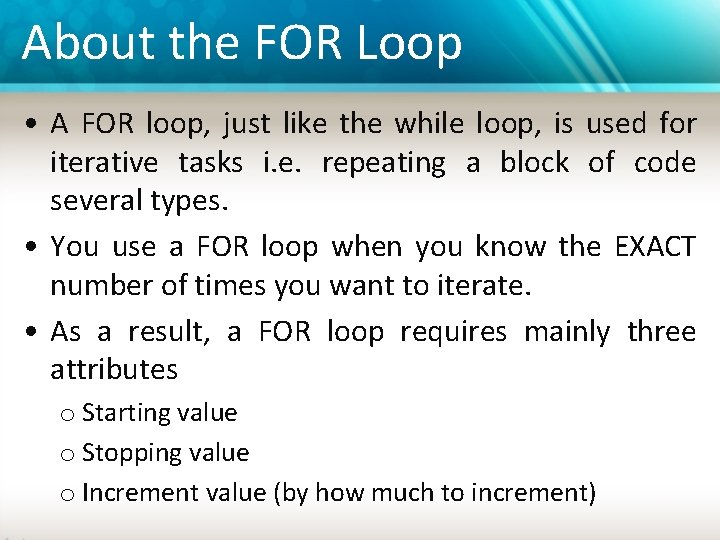
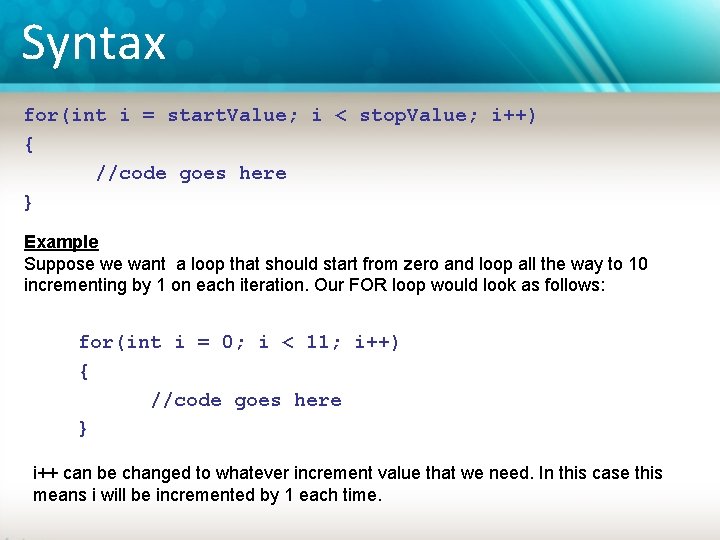
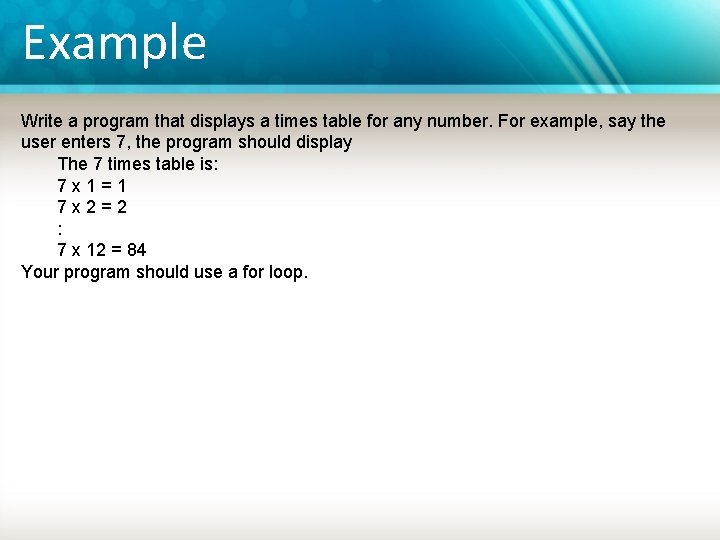
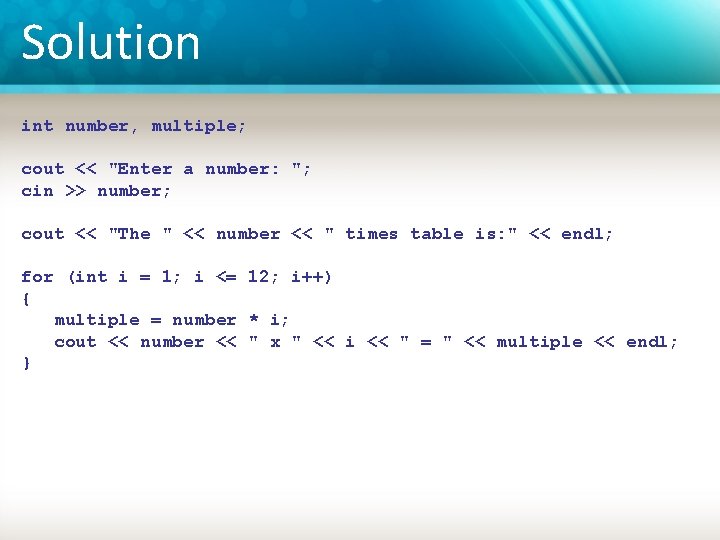
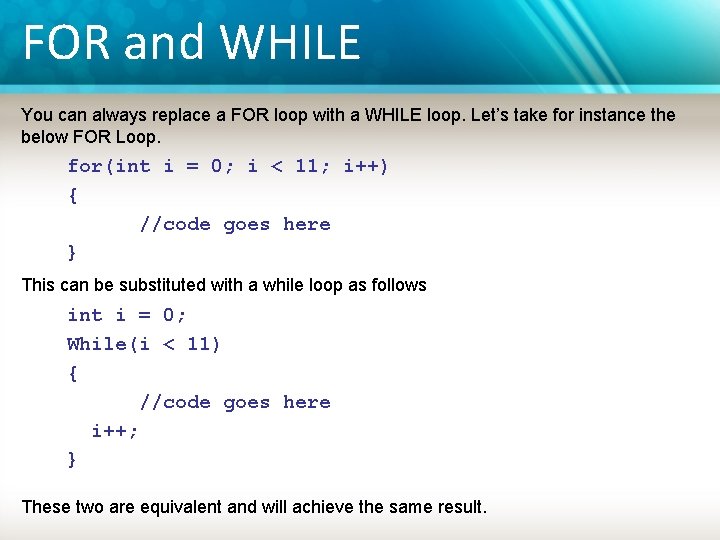
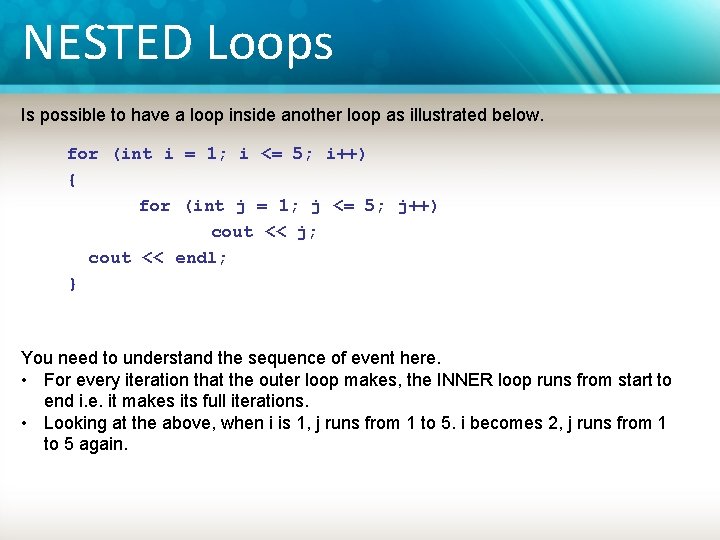
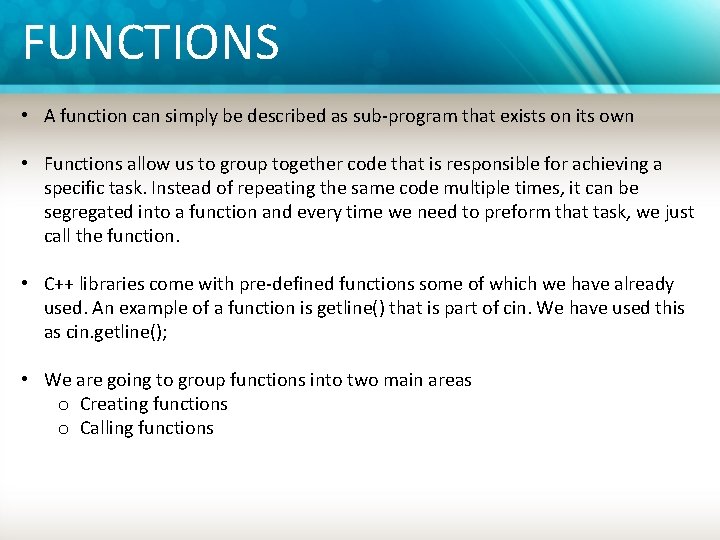
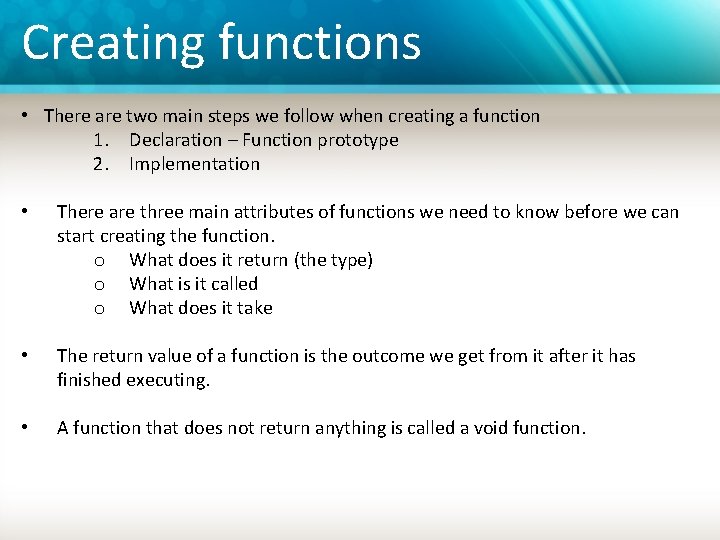
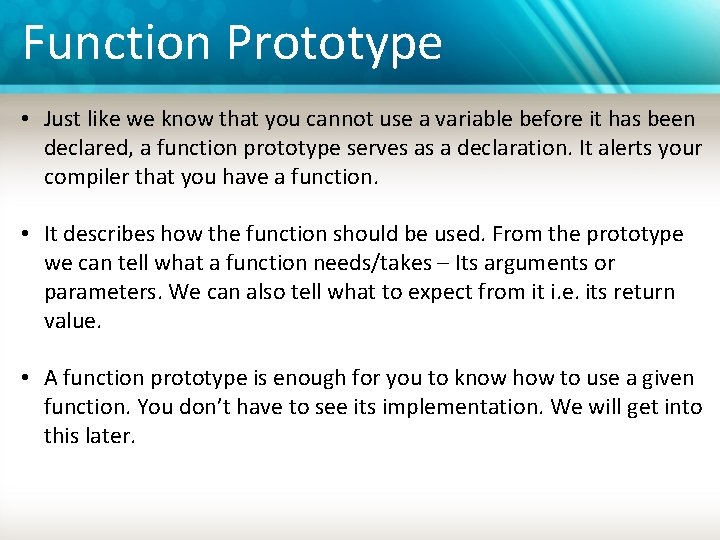
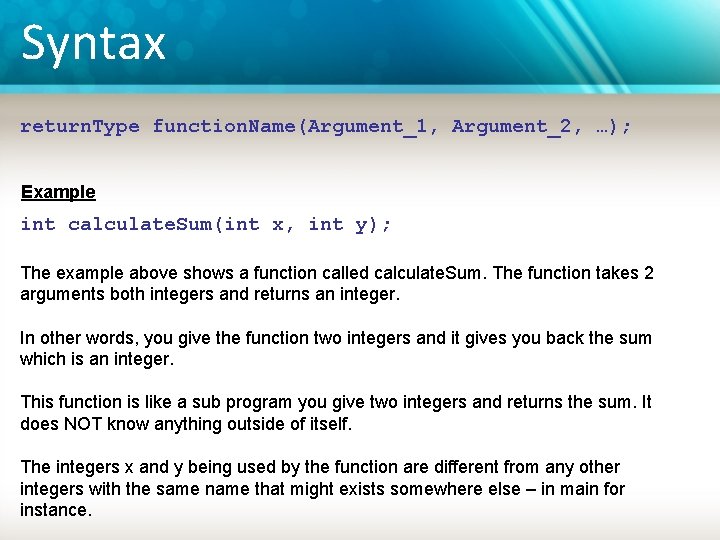
- Slides: 11
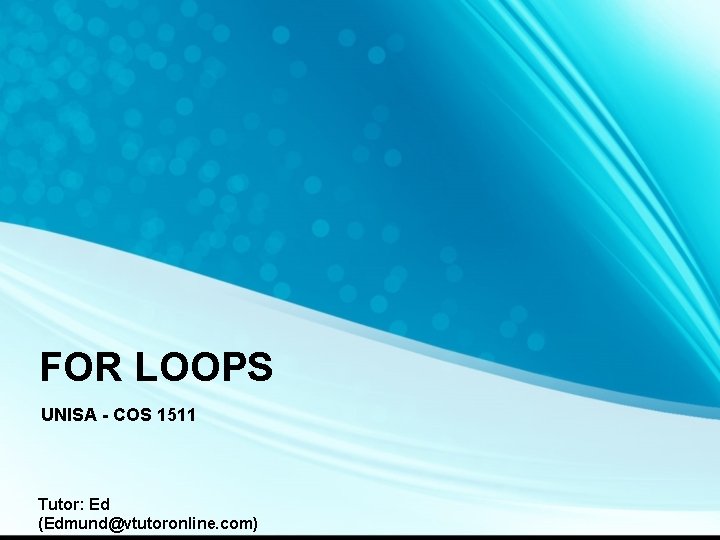
FOR LOOPS UNISA - COS 1511 Tutor: Ed (Edmund@vtutoronline. com)
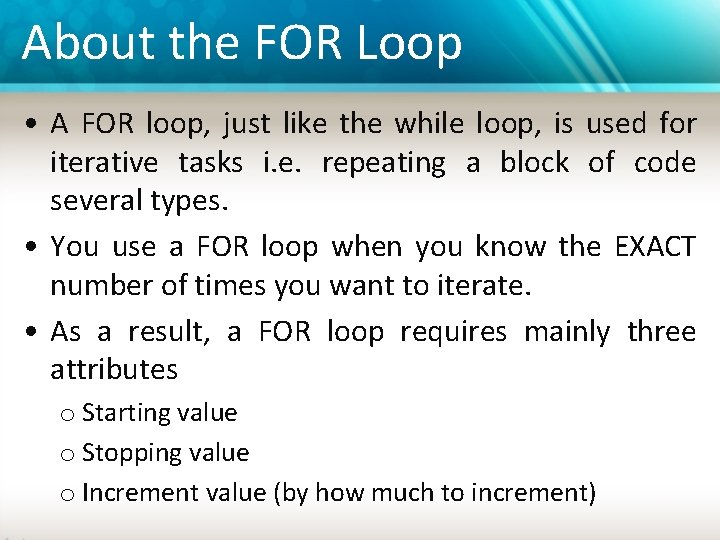
About the FOR Loop • A FOR loop, just like the while loop, is used for iterative tasks i. e. repeating a block of code several types. • You use a FOR loop when you know the EXACT number of times you want to iterate. • As a result, a FOR loop requires mainly three attributes o Starting value o Stopping value o Increment value (by how much to increment)
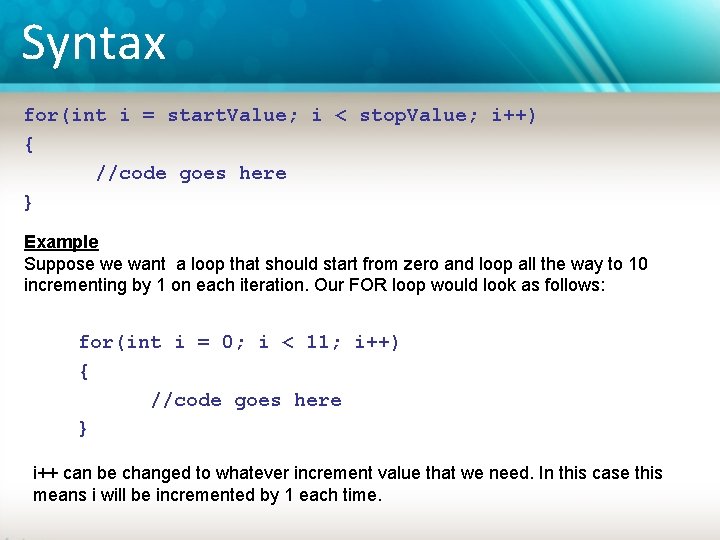
Syntax for(int i = start. Value; i < stop. Value; i++) { //code goes here } Example Suppose we want a loop that should start from zero and loop all the way to 10 incrementing by 1 on each iteration. Our FOR loop would look as follows: for(int i = 0; i < 11; i++) { //code goes here } i++ can be changed to whatever increment value that we need. In this case this means i will be incremented by 1 each time.
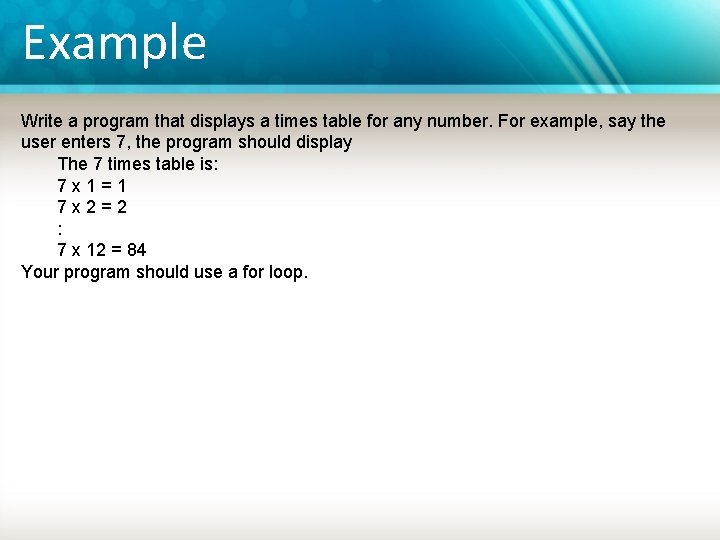
Example Write a program that displays a times table for any number. For example, say the user enters 7, the program should display The 7 times table is: 7 x 1=1 7 x 2=2 : 7 x 12 = 84 Your program should use a for loop.
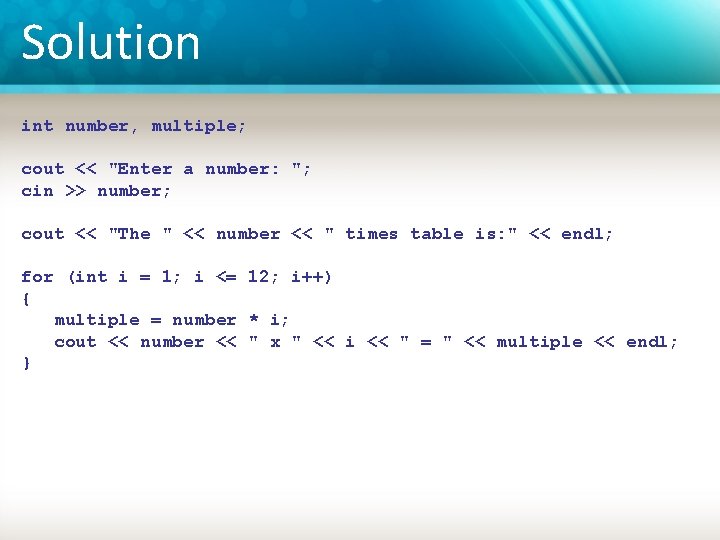
Solution int number, multiple; cout << "Enter a number: "; cin >> number; cout << "The " << number << " times table is: " << endl; for (int i = 1; i <= 12; i++) { multiple = number * i; cout << number << " x " << i << " = " << multiple << endl; }
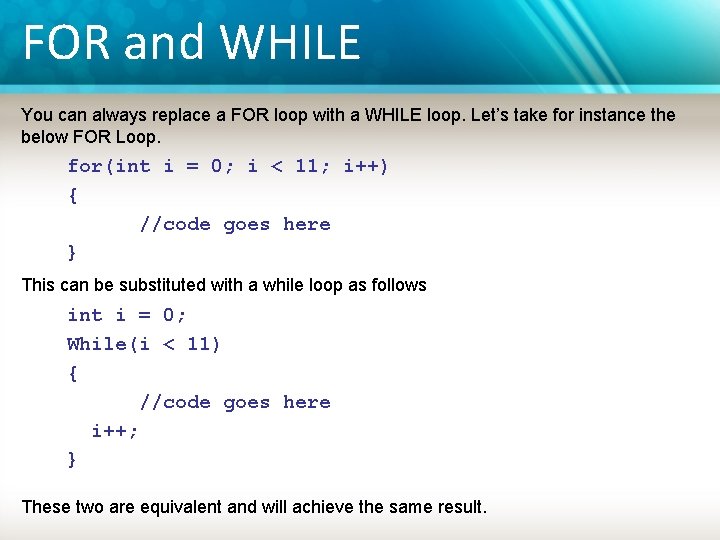
FOR and WHILE You can always replace a FOR loop with a WHILE loop. Let’s take for instance the below FOR Loop. for(int i = 0; i < 11; i++) { //code goes here } This can be substituted with a while loop as follows int i = 0; While(i < 11) { //code goes here i++; } These two are equivalent and will achieve the same result.
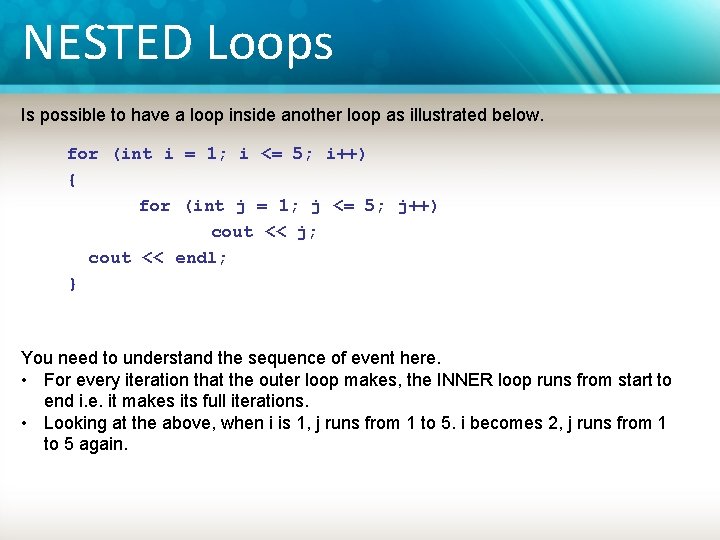
NESTED Loops Is possible to have a loop inside another loop as illustrated below. for (int i = 1; i <= 5; i++) { for (int j = 1; j <= 5; j++) cout << j; cout << endl; } You need to understand the sequence of event here. • For every iteration that the outer loop makes, the INNER loop runs from start to end i. e. it makes its full iterations. • Looking at the above, when i is 1, j runs from 1 to 5. i becomes 2, j runs from 1 to 5 again.
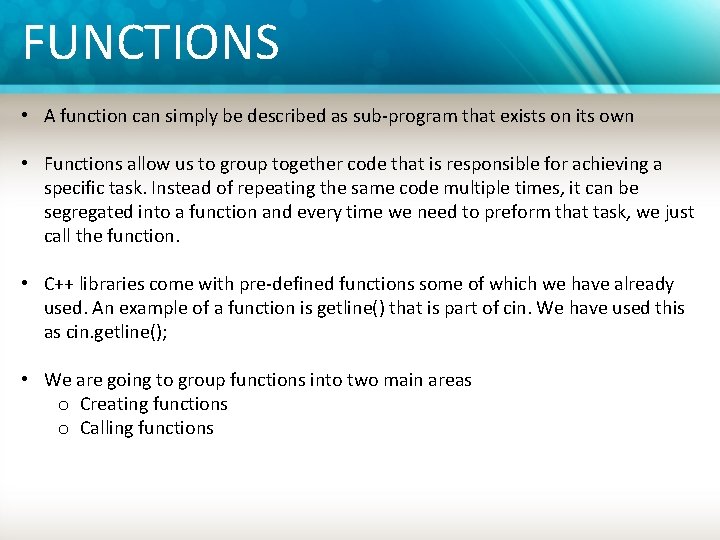
FUNCTIONS • A function can simply be described as sub-program that exists on its own • Functions allow us to group together code that is responsible for achieving a specific task. Instead of repeating the same code multiple times, it can be segregated into a function and every time we need to preform that task, we just call the function. • C++ libraries come with pre-defined functions some of which we have already used. An example of a function is getline() that is part of cin. We have used this as cin. getline(); • We are going to group functions into two main areas o Creating functions o Calling functions
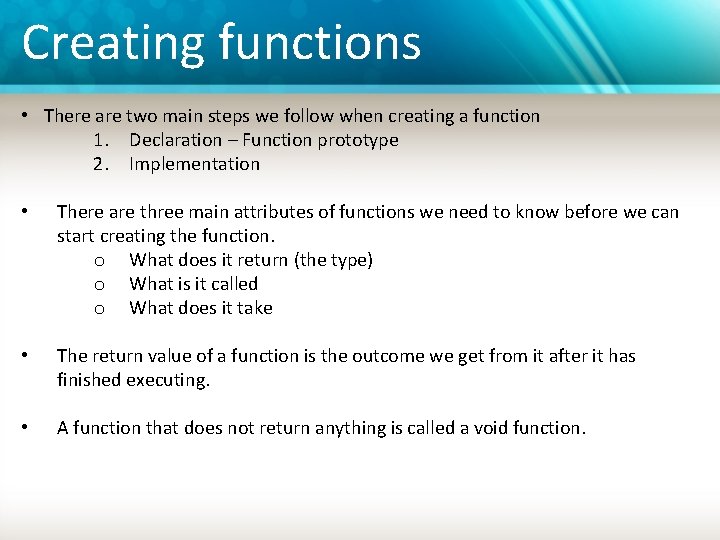
Creating functions • There are two main steps we follow when creating a function 1. Declaration – Function prototype 2. Implementation • There are three main attributes of functions we need to know before we can start creating the function. o What does it return (the type) o What is it called o What does it take • The return value of a function is the outcome we get from it after it has finished executing. • A function that does not return anything is called a void function.
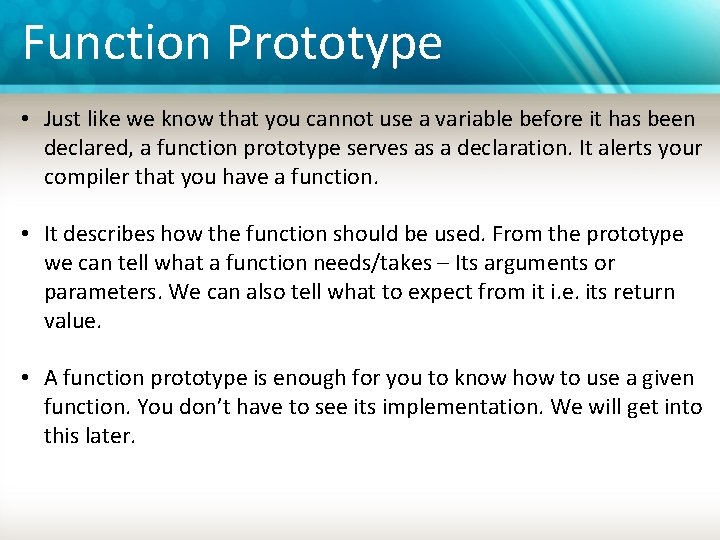
Function Prototype • Just like we know that you cannot use a variable before it has been declared, a function prototype serves as a declaration. It alerts your compiler that you have a function. • It describes how the function should be used. From the prototype we can tell what a function needs/takes – Its arguments or parameters. We can also tell what to expect from it i. e. its return value. • A function prototype is enough for you to know how to use a given function. You don’t have to see its implementation. We will get into this later.
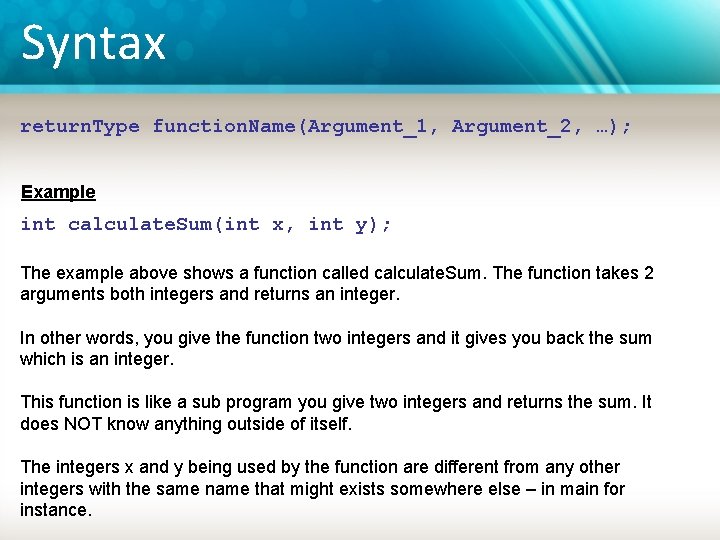
Syntax return. Type function. Name(Argument_1, Argument_2, …); Example int calculate. Sum(int x, int y); The example above shows a function called calculate. Sum. The function takes 2 arguments both integers and returns an integer. In other words, you give the function two integers and it gives you back the sum which is an integer. This function is like a sub program you give two integers and returns the sum. It does NOT know anything outside of itself. The integers x and y being used by the function are different from any other integers with the same name that might exists somewhere else – in main for instance.