For Loop Tips And Tricks 1 The outermost
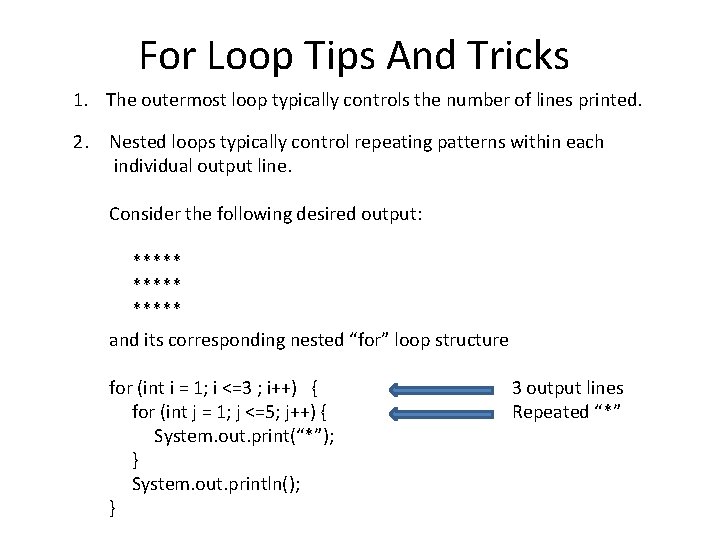
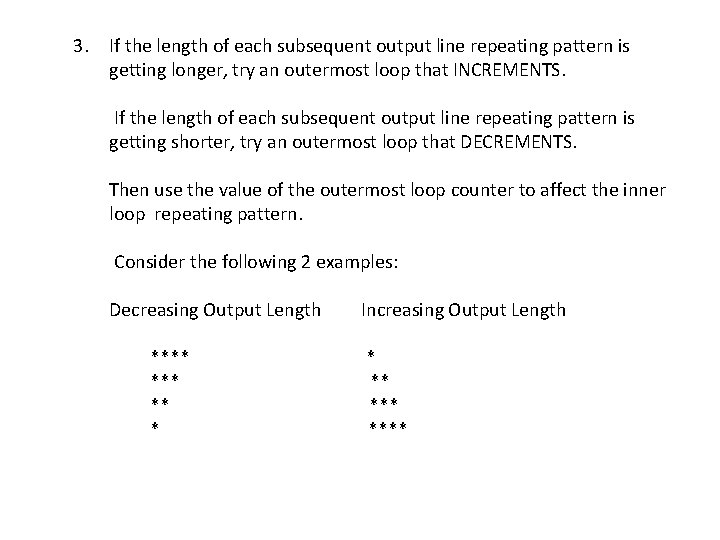
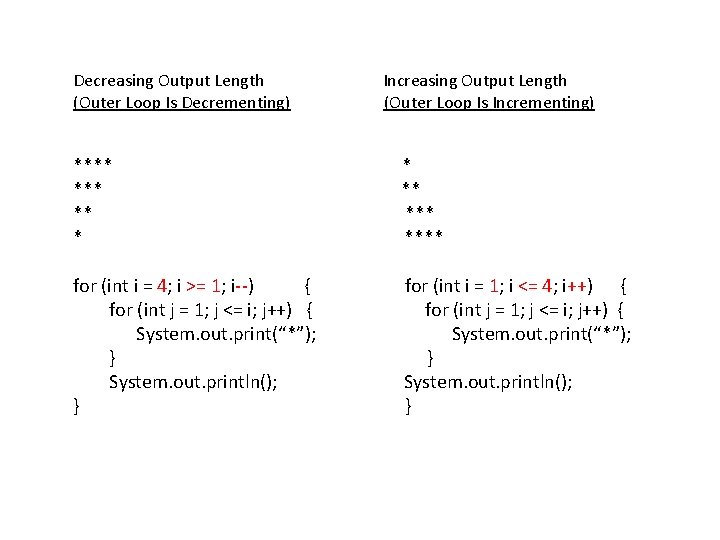
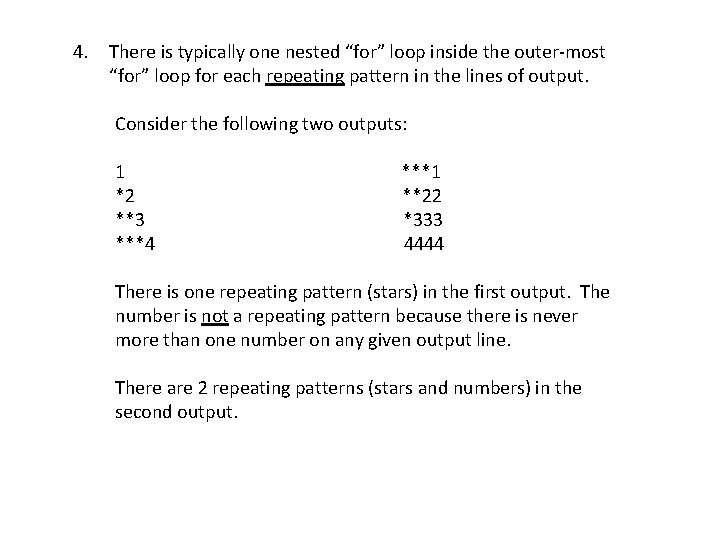
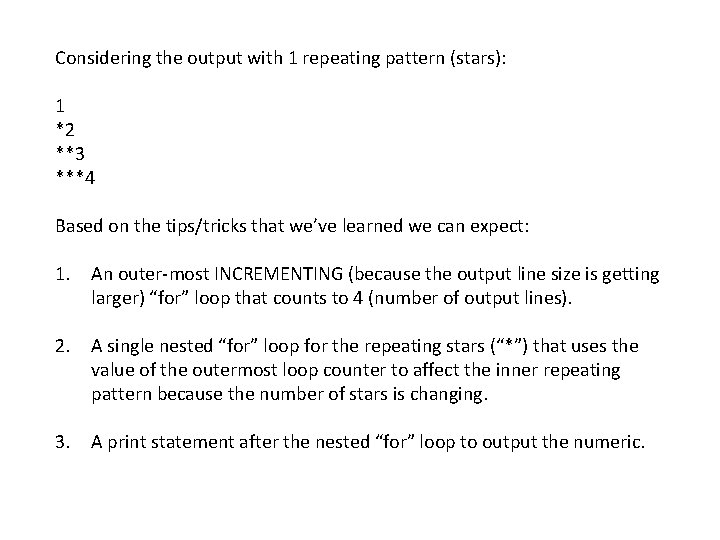
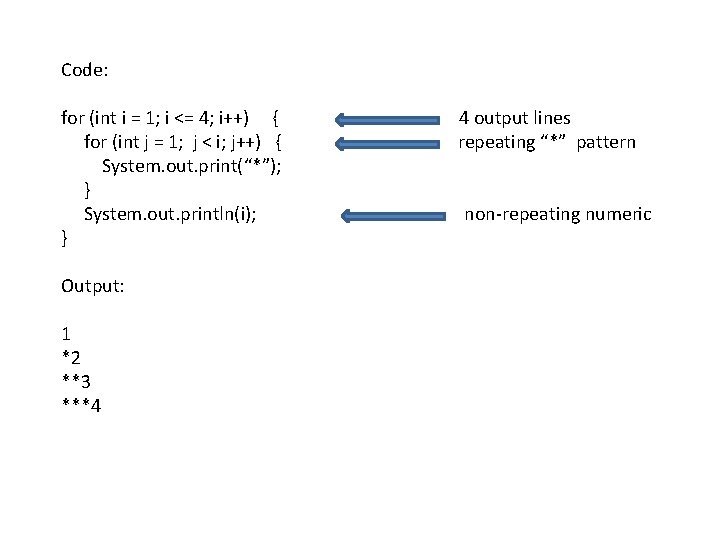
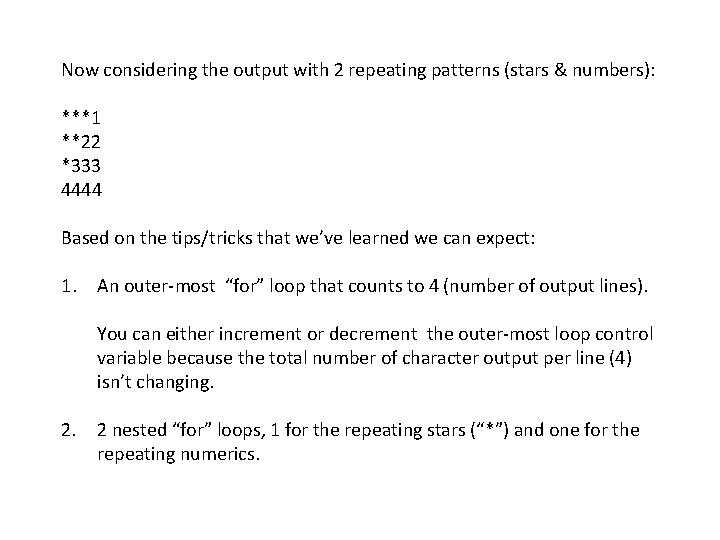
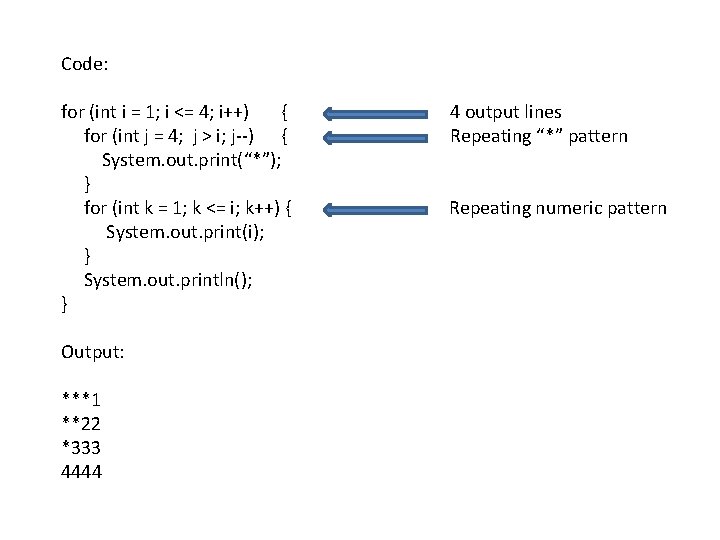
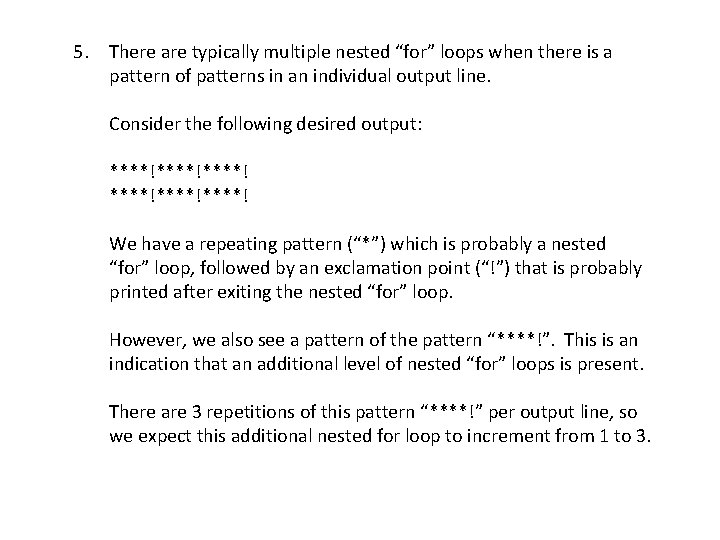
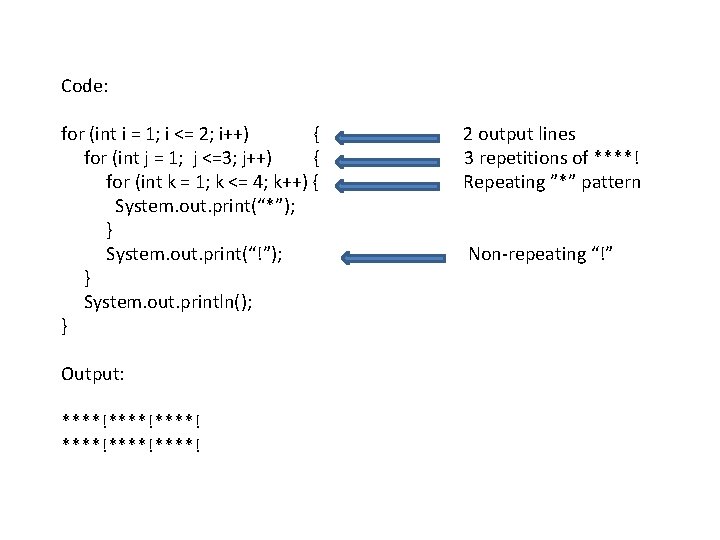
- Slides: 10
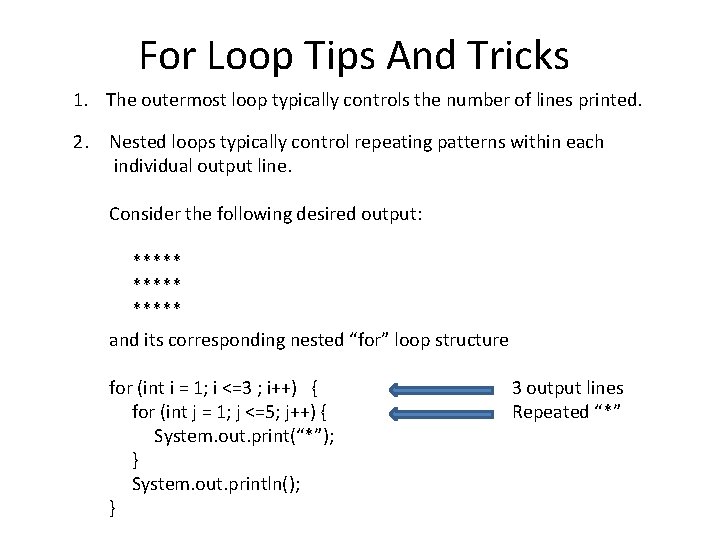
For Loop Tips And Tricks 1. The outermost loop typically controls the number of lines printed. 2. Nested loops typically control repeating patterns within each individual output line. Consider the following desired output: ***** and its corresponding nested “for” loop structure for (int i = 1; i <=3 ; i++) { for (int j = 1; j <=5; j++) { System. out. print(“*”); } System. out. println(); } 3 output lines Repeated “*”
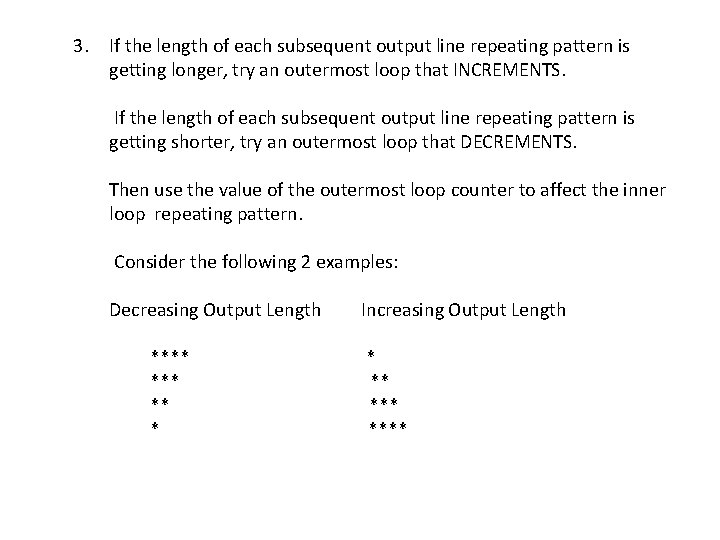
3. If the length of each subsequent output line repeating pattern is getting longer, try an outermost loop that INCREMENTS. If the length of each subsequent output line repeating pattern is getting shorter, try an outermost loop that DECREMENTS. Then use the value of the outermost loop counter to affect the inner loop repeating pattern. Consider the following 2 examples: Decreasing Output Length **** ** * Increasing Output Length * ** ****
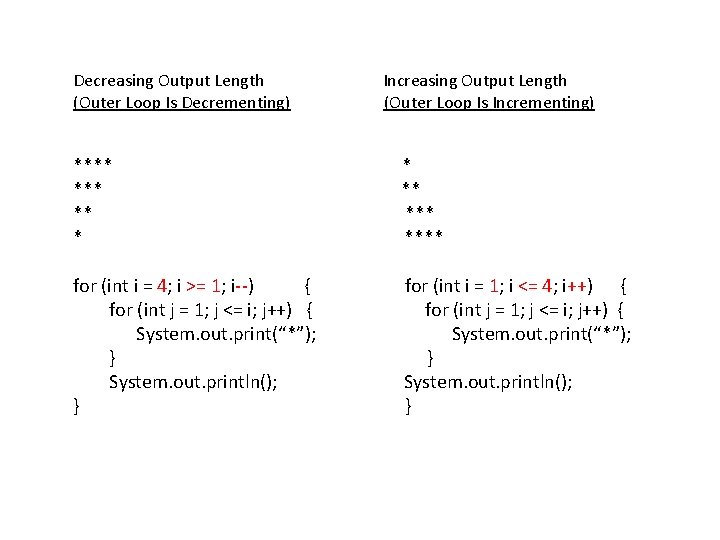
Decreasing Output Length (Outer Loop Is Decrementing) Increasing Output Length (Outer Loop Is Incrementing) **** ** **** for (int i = 4; i >= 1; i--) { for (int j = 1; j <= i; j++) { System. out. print(“*”); } System. out. println(); } for (int i = 1; i <= 4; i++) { for (int j = 1; j <= i; j++) { System. out. print(“*”); } System. out. println(); }
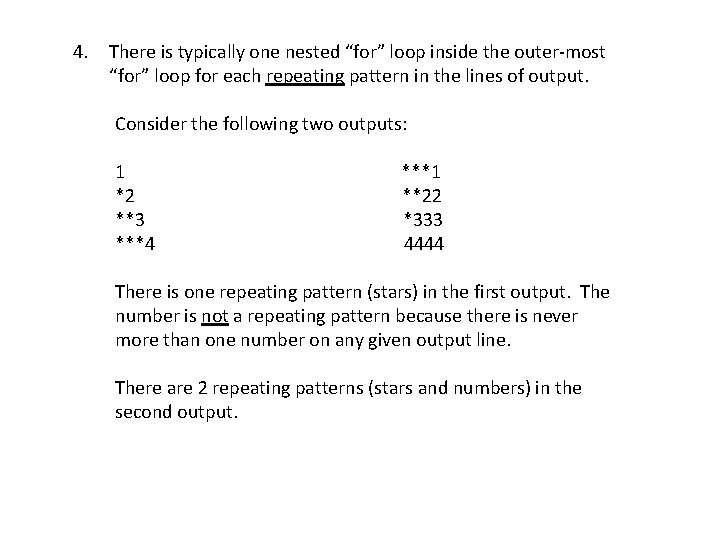
4. There is typically one nested “for” loop inside the outer-most “for” loop for each repeating pattern in the lines of output. Consider the following two outputs: 1 *2 **3 ***4 ***1 **22 *333 4444 There is one repeating pattern (stars) in the first output. The number is not a repeating pattern because there is never more than one number on any given output line. There are 2 repeating patterns (stars and numbers) in the second output.
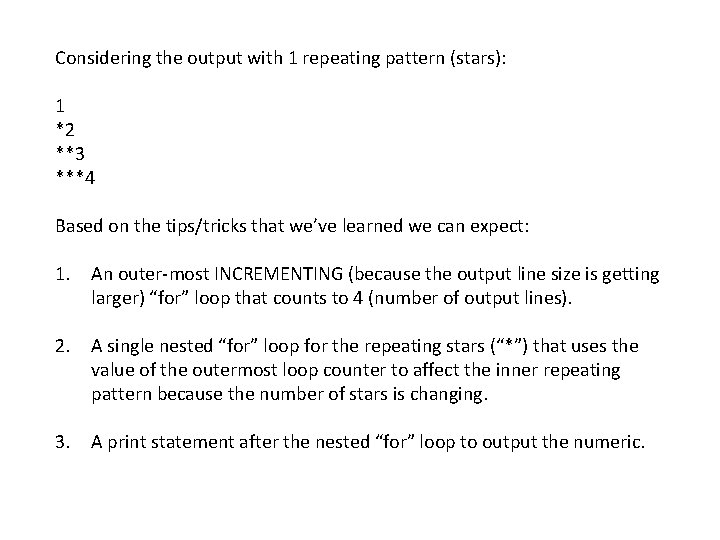
Considering the output with 1 repeating pattern (stars): 1 *2 **3 ***4 Based on the tips/tricks that we’ve learned we can expect: 1. An outer-most INCREMENTING (because the output line size is getting larger) “for” loop that counts to 4 (number of output lines). 2. A single nested “for” loop for the repeating stars (“*”) that uses the value of the outermost loop counter to affect the inner repeating pattern because the number of stars is changing. 3. A print statement after the nested “for” loop to output the numeric.
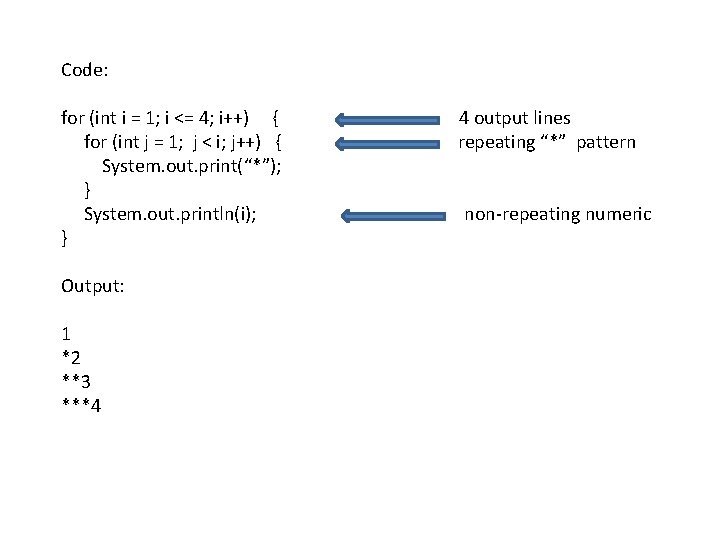
Code: for (int i = 1; i <= 4; i++) { for (int j = 1; j < i; j++) { System. out. print(“*”); } System. out. println(i); } Output: 1 *2 **3 ***4 4 output lines repeating “*” pattern non-repeating numeric
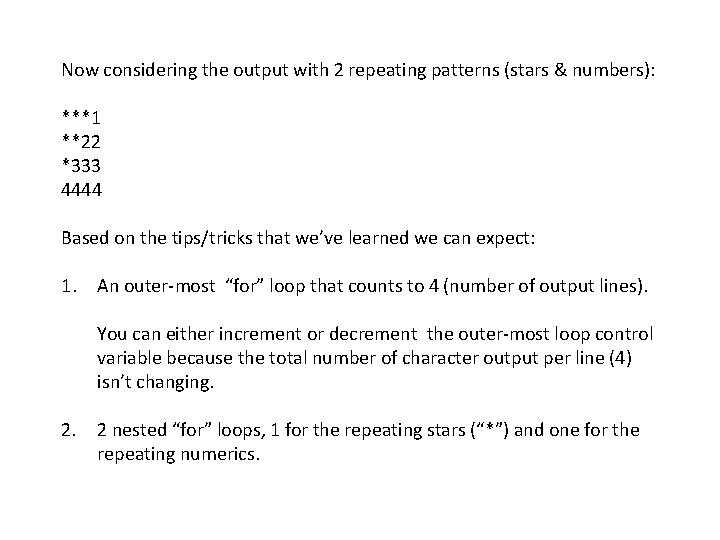
Now considering the output with 2 repeating patterns (stars & numbers): ***1 **22 *333 4444 Based on the tips/tricks that we’ve learned we can expect: 1. An outer-most “for” loop that counts to 4 (number of output lines). You can either increment or decrement the outer-most loop control variable because the total number of character output per line (4) isn’t changing. 2. 2 nested “for” loops, 1 for the repeating stars (“*”) and one for the repeating numerics.
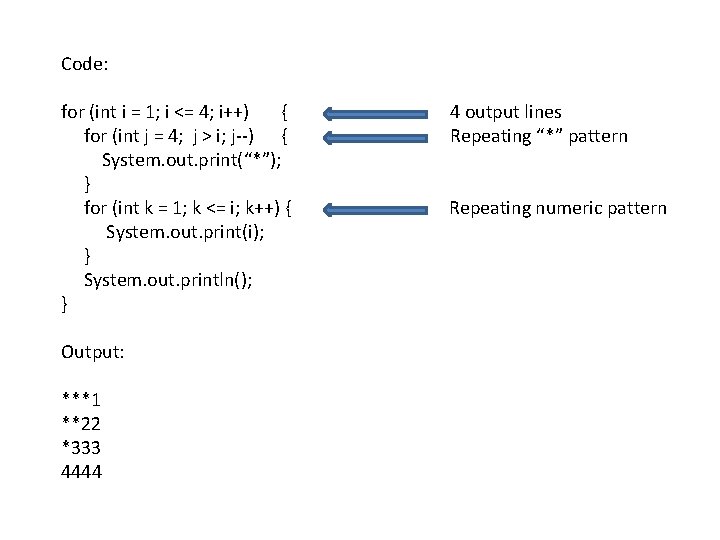
Code: for (int i = 1; i <= 4; i++) { for (int j = 4; j > i; j--) { System. out. print(“*”); } for (int k = 1; k <= i; k++) { System. out. print(i); } System. out. println(); } Output: ***1 **22 *333 4444 4 output lines Repeating “*” pattern Repeating numeric pattern
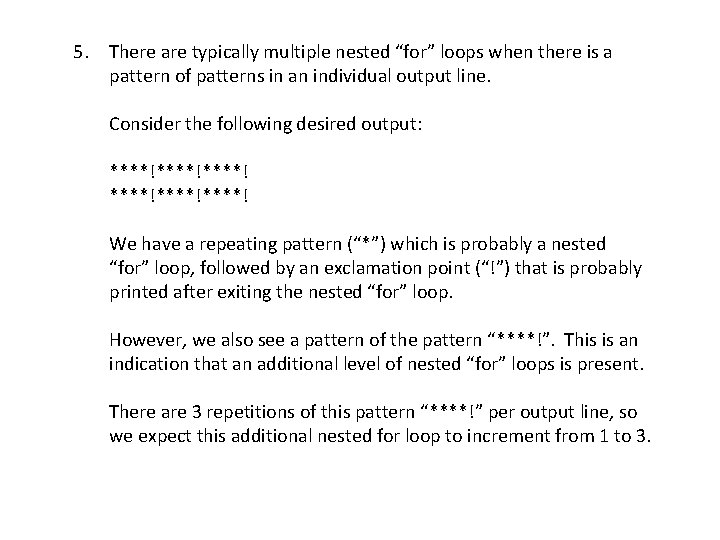
5. There are typically multiple nested “for” loops when there is a pattern of patterns in an individual output line. Consider the following desired output: ****!****!****! We have a repeating pattern (“*”) which is probably a nested “for” loop, followed by an exclamation point (“!”) that is probably printed after exiting the nested “for” loop. However, we also see a pattern of the pattern “****!”. This is an indication that an additional level of nested “for” loops is present. There are 3 repetitions of this pattern “****!” per output line, so we expect this additional nested for loop to increment from 1 to 3.
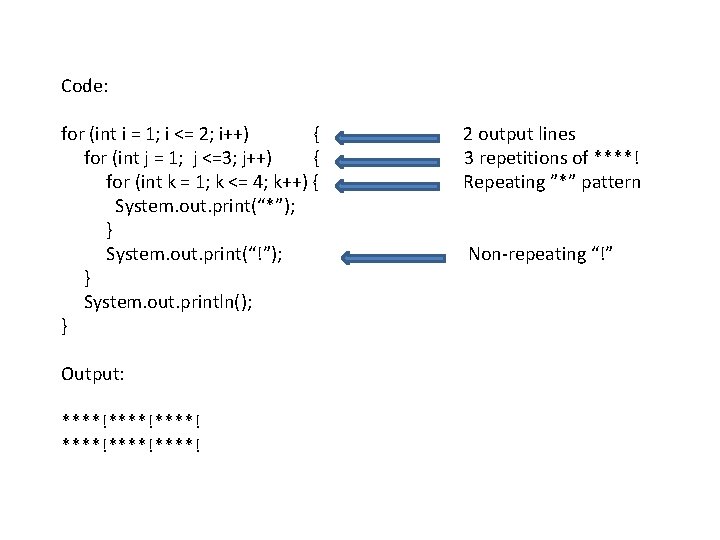
Code: for (int i = 1; i <= 2; i++) { for (int j = 1; j <=3; j++) { for (int k = 1; k <= 4; k++) { System. out. print(“*”); } System. out. print(“!”); } System. out. println(); } Output: ****!****!****! 2 output lines 3 repetitions of ****! Repeating ”*” pattern Non-repeating “!”