Final Exam Date January 9 th Thursday p
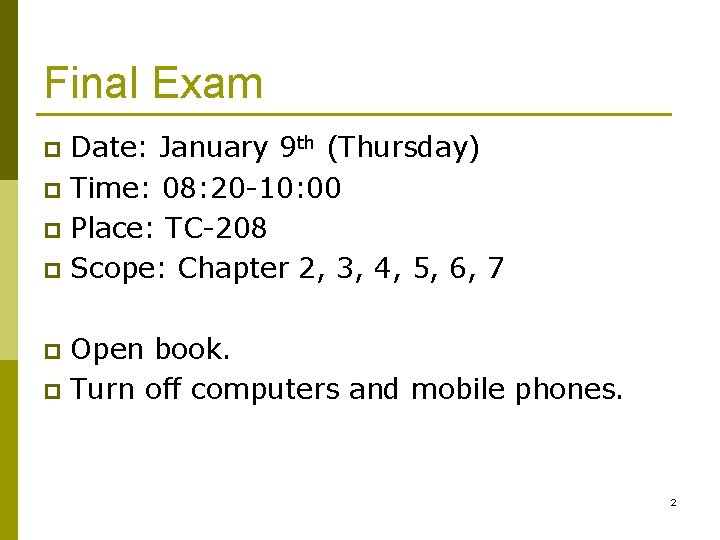
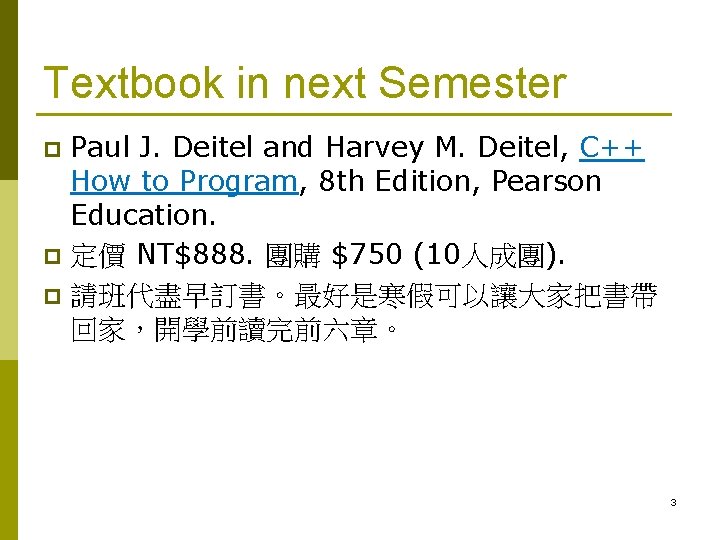
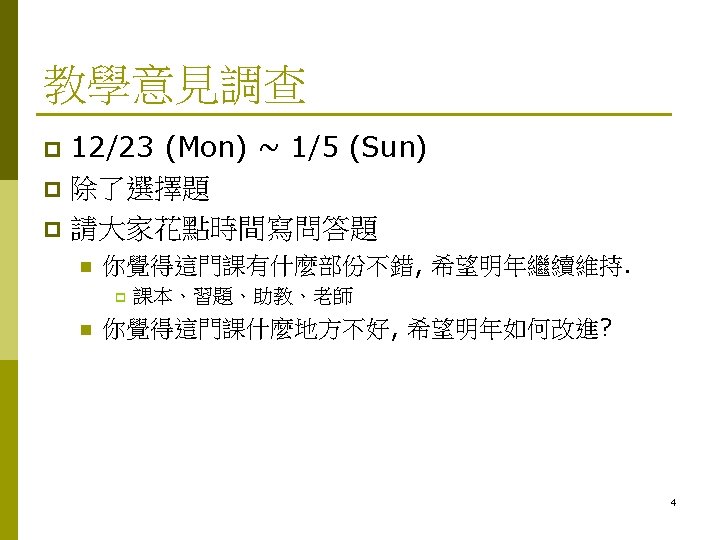
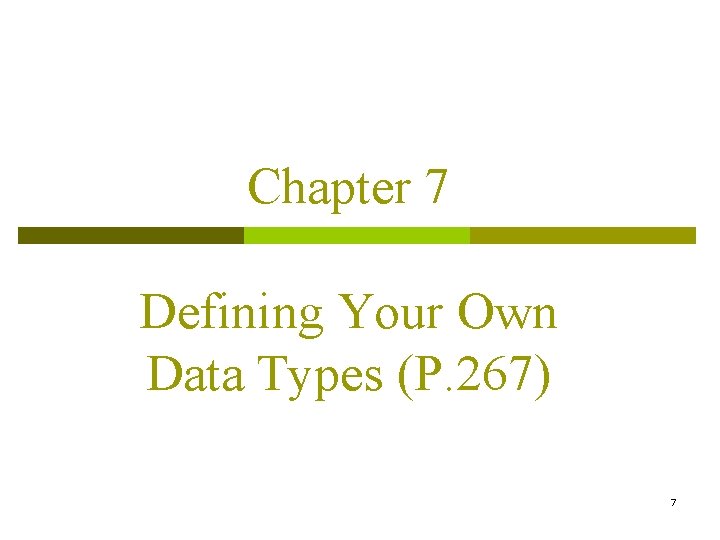
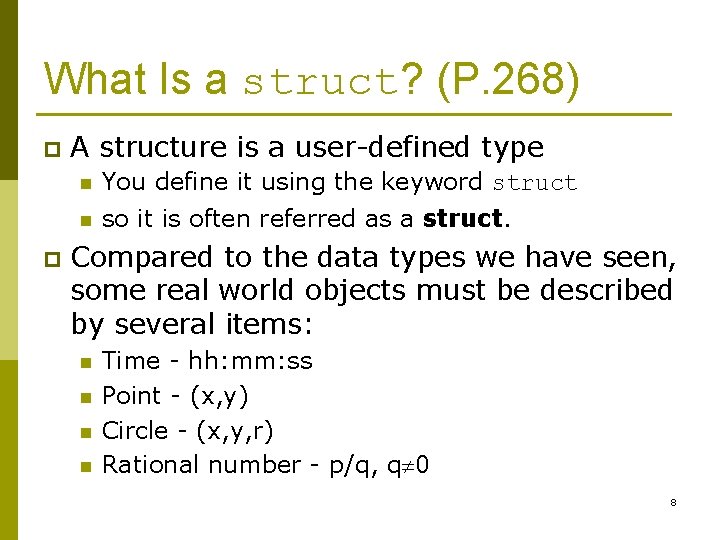
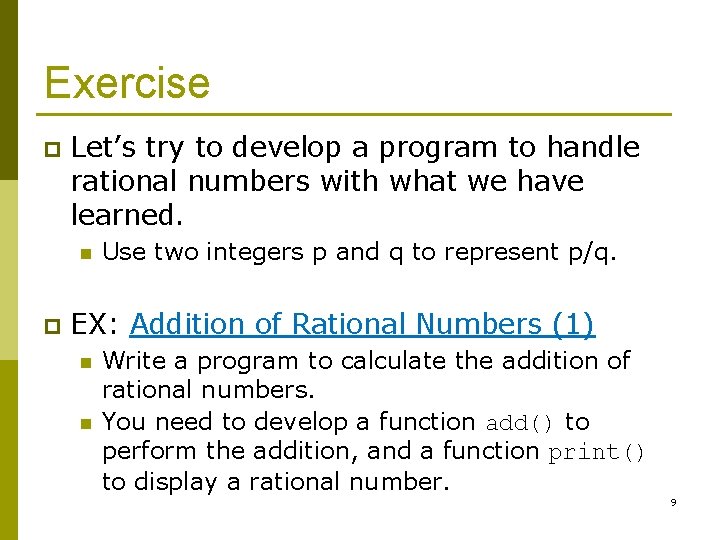
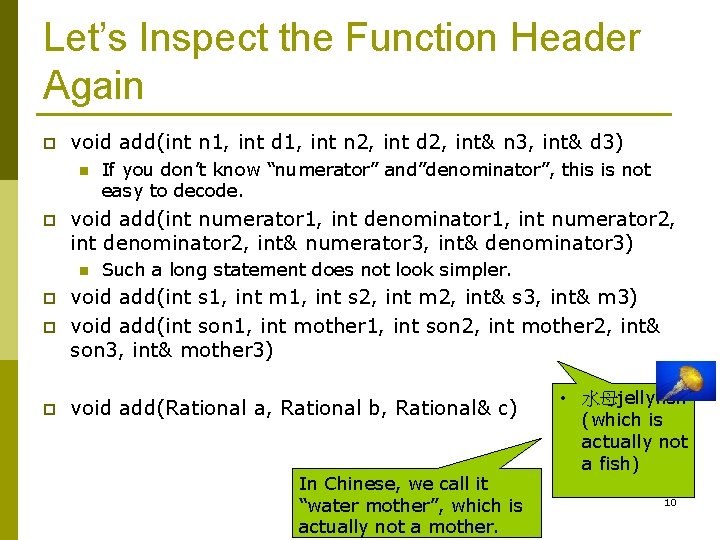
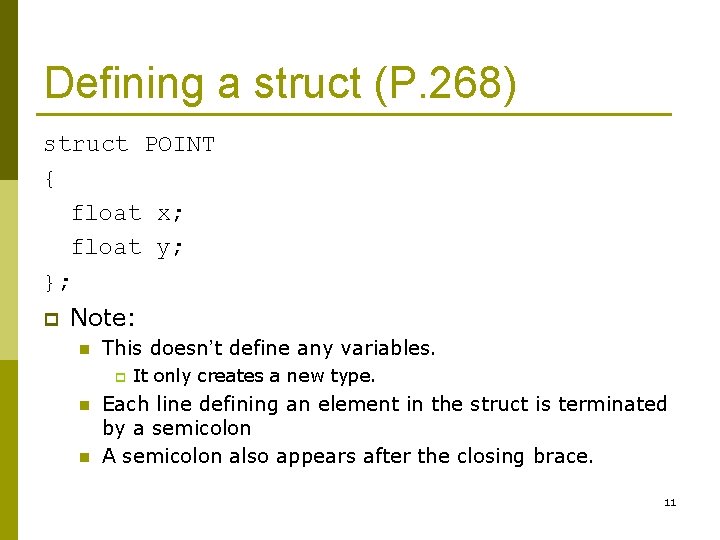
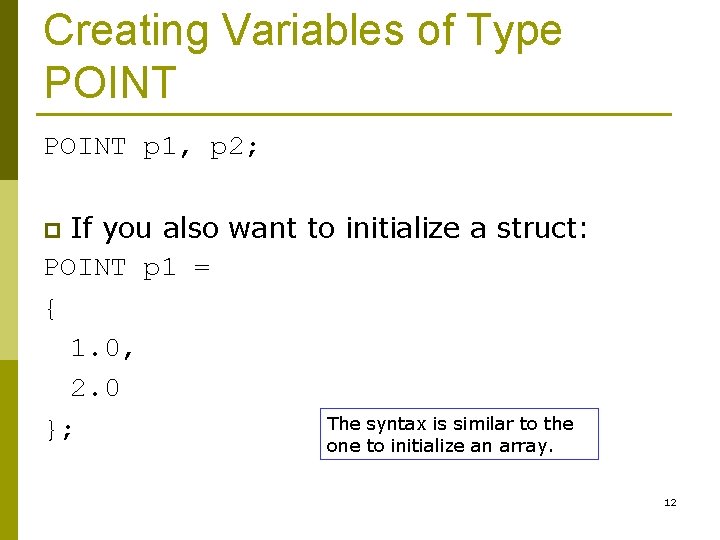
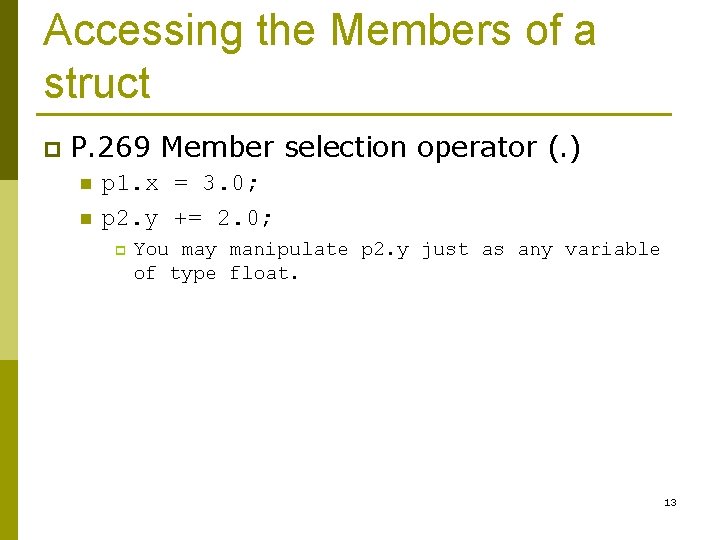
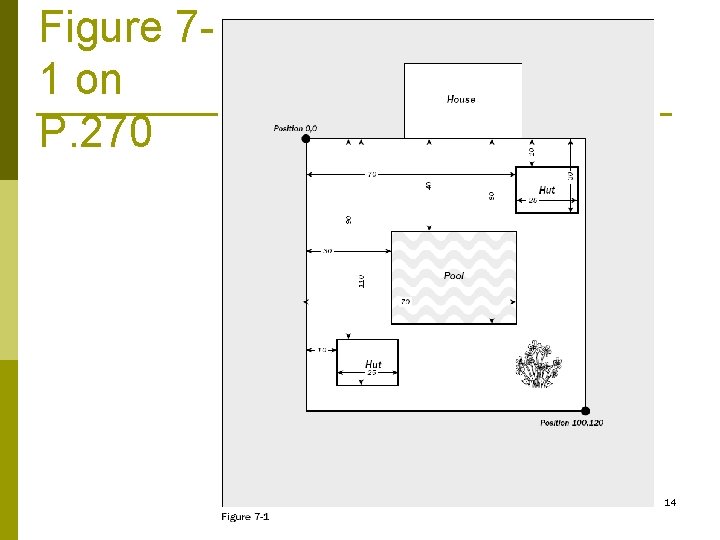
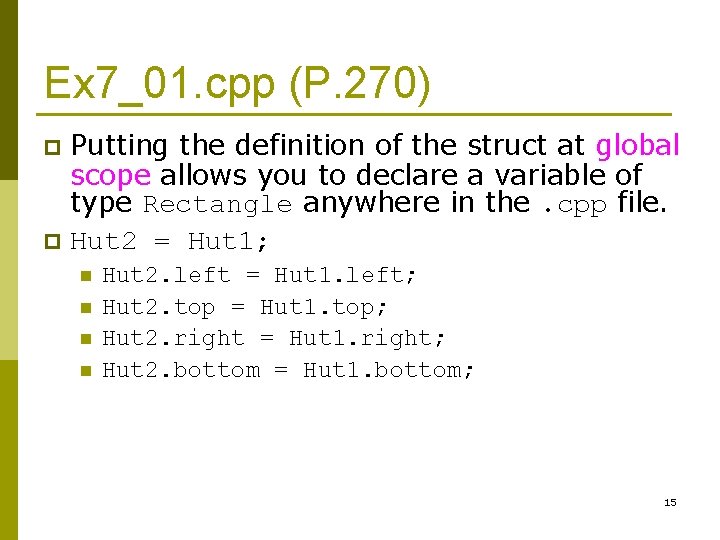
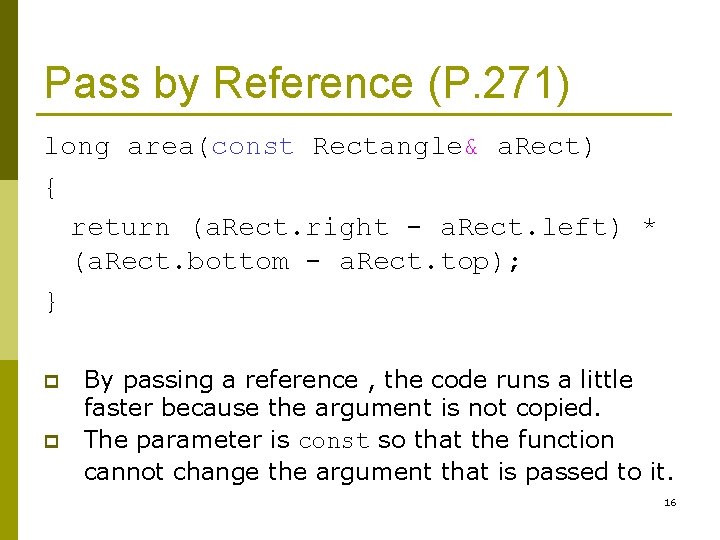
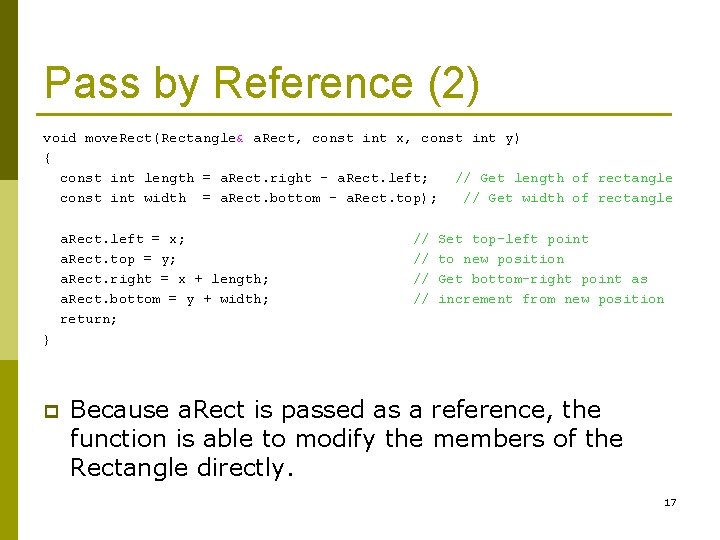
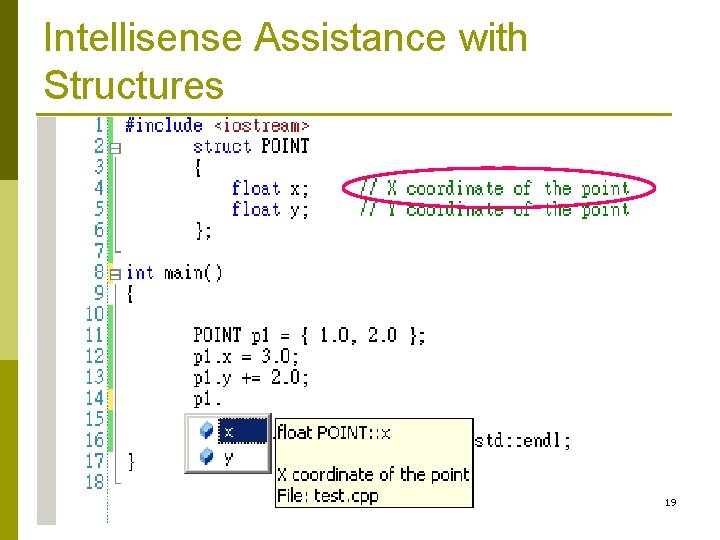
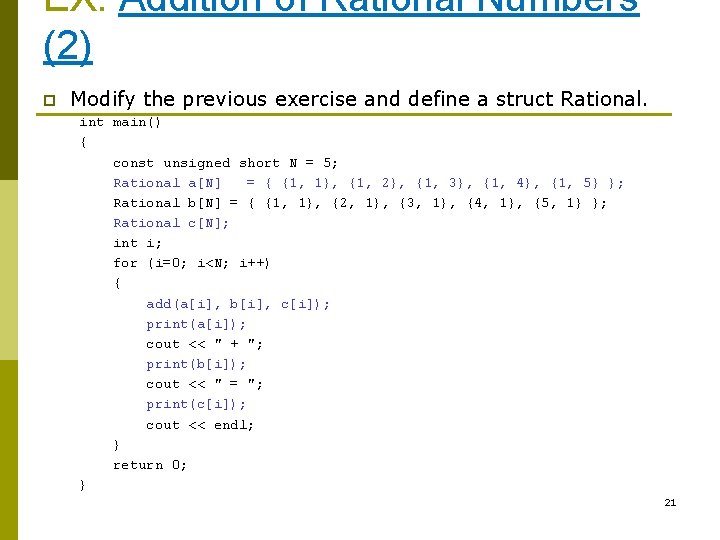
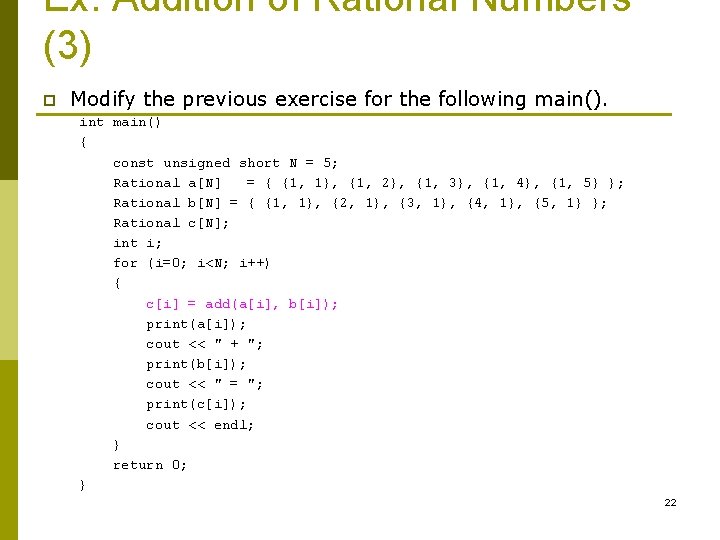
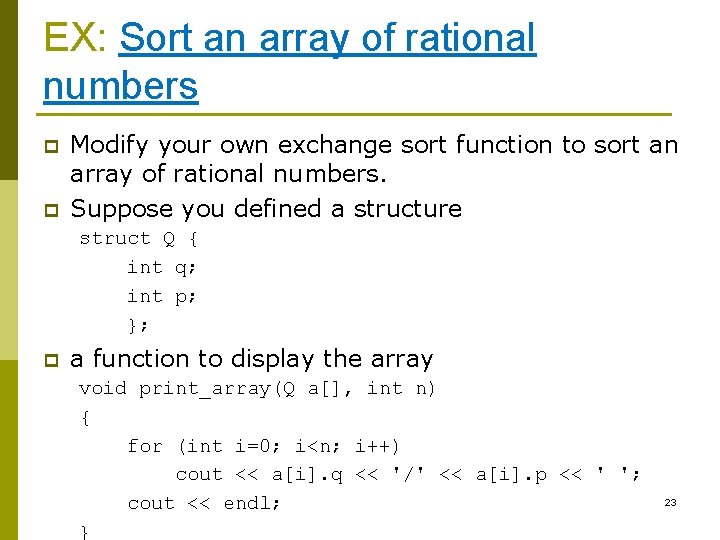
![p and a main program to test it. int main() { Q a[] = p and a main program to test it. int main() { Q a[] =](https://slidetodoc.com/presentation_image_h2/90330dcb96790c9e60adb0ff998e0611/image-19.jpg)
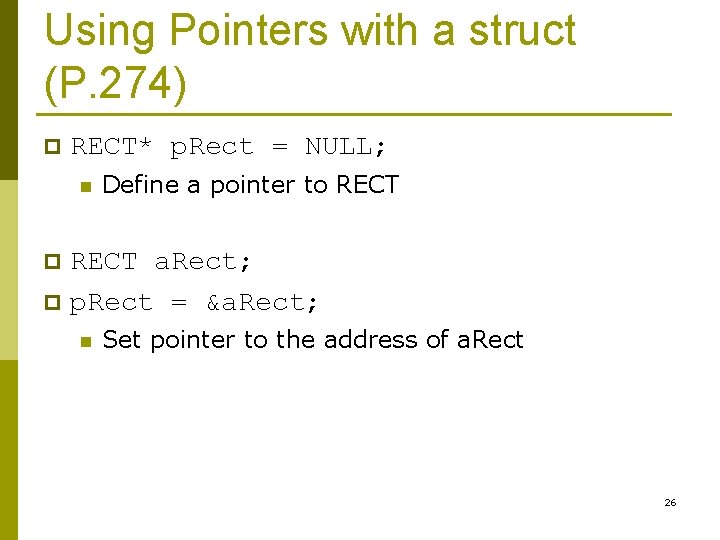
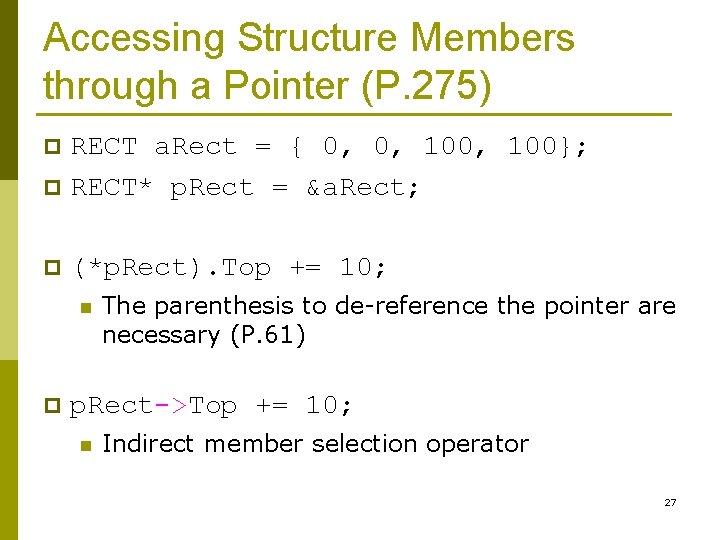
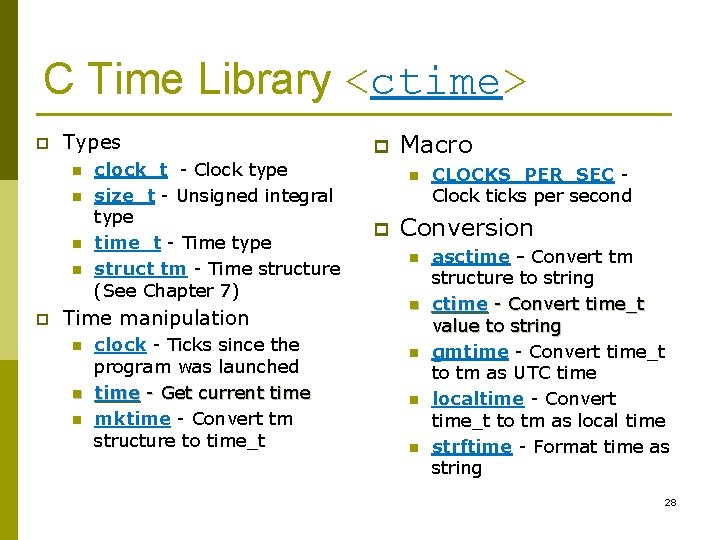
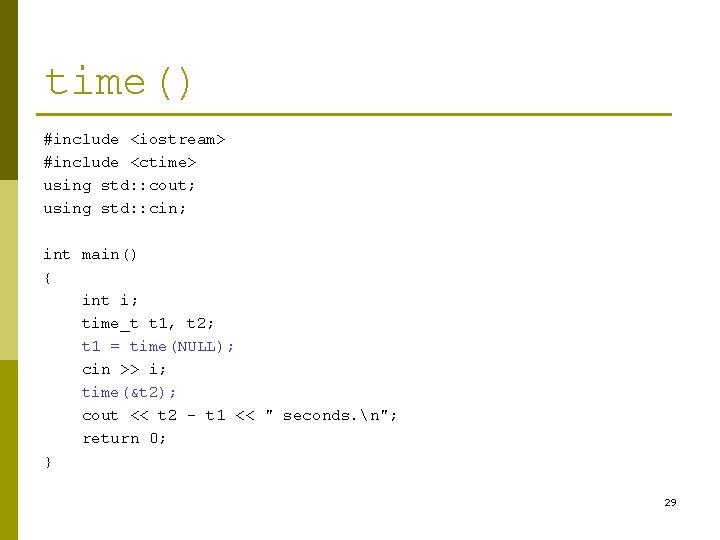
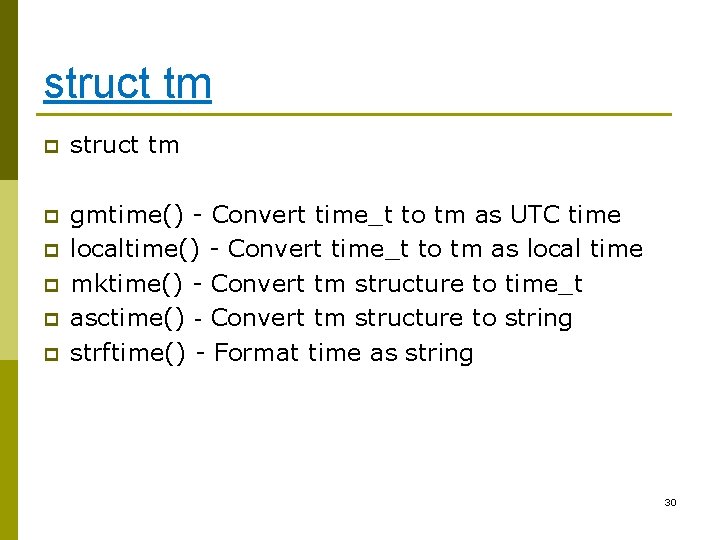
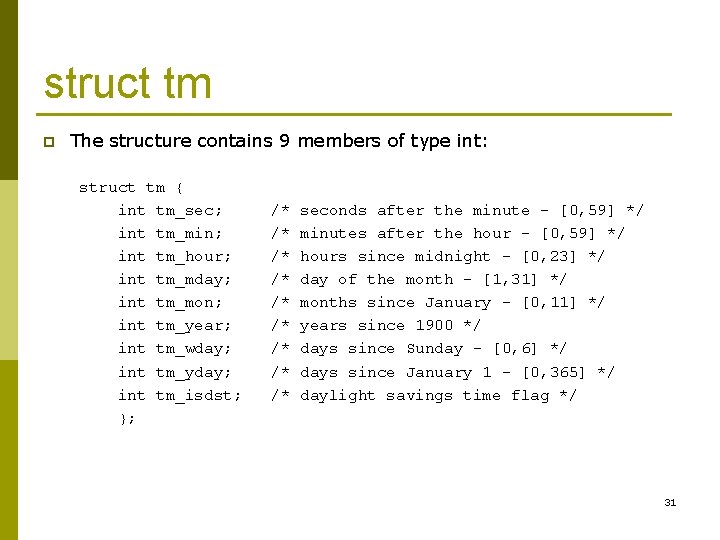
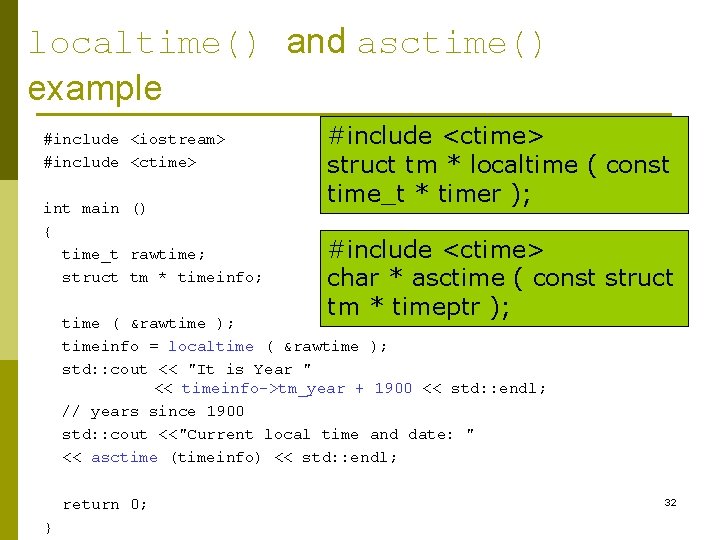
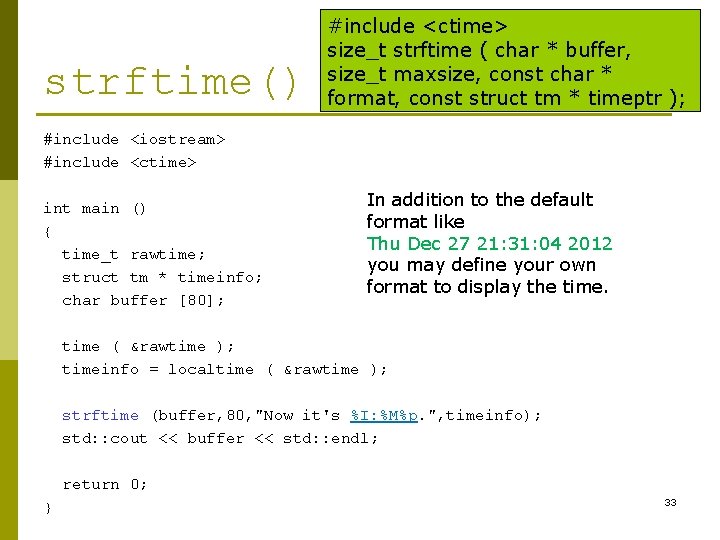
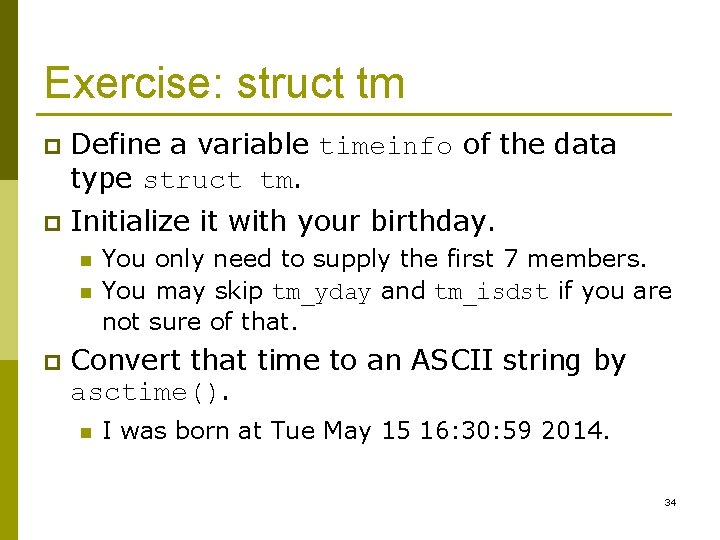
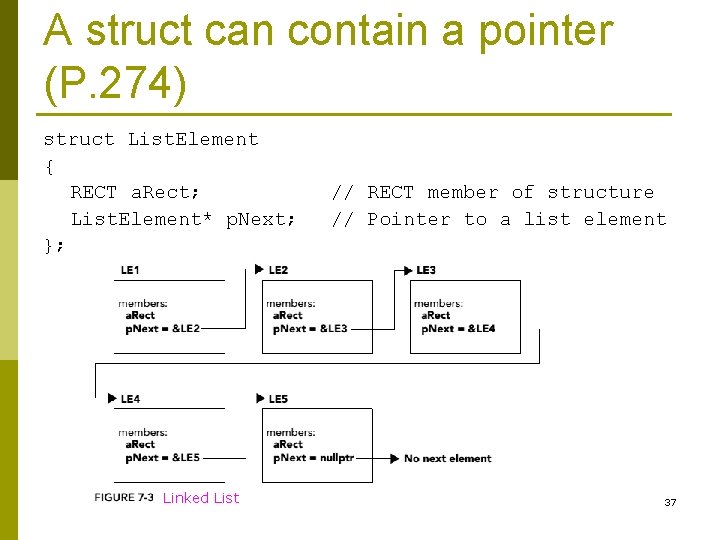
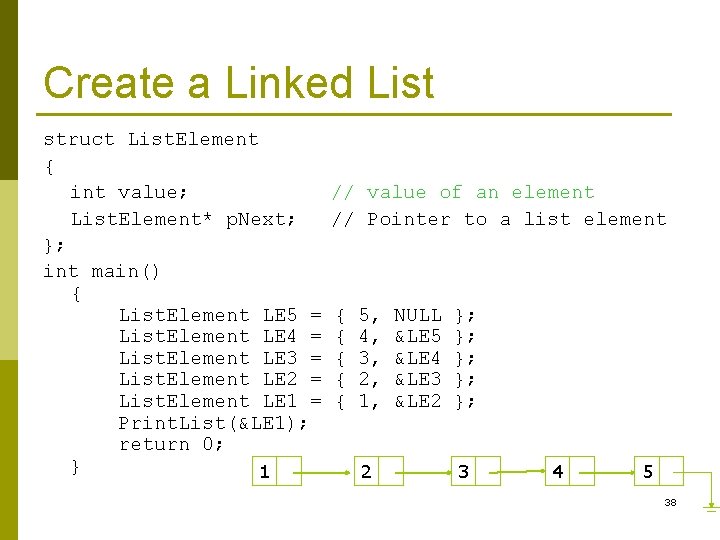
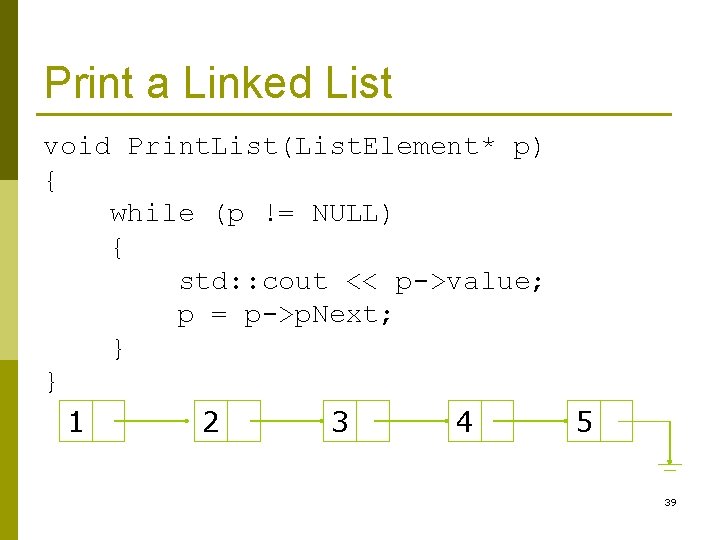
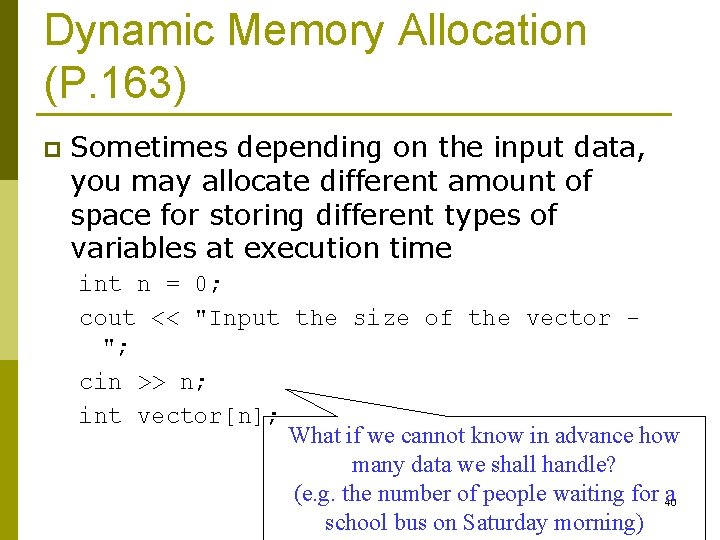
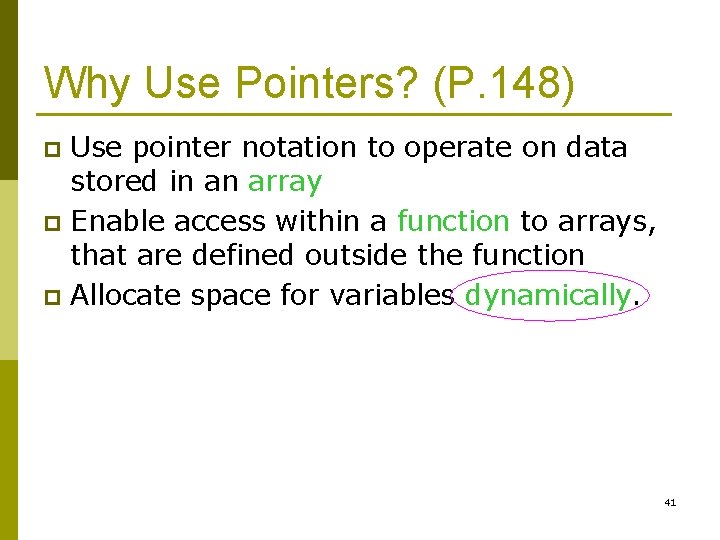
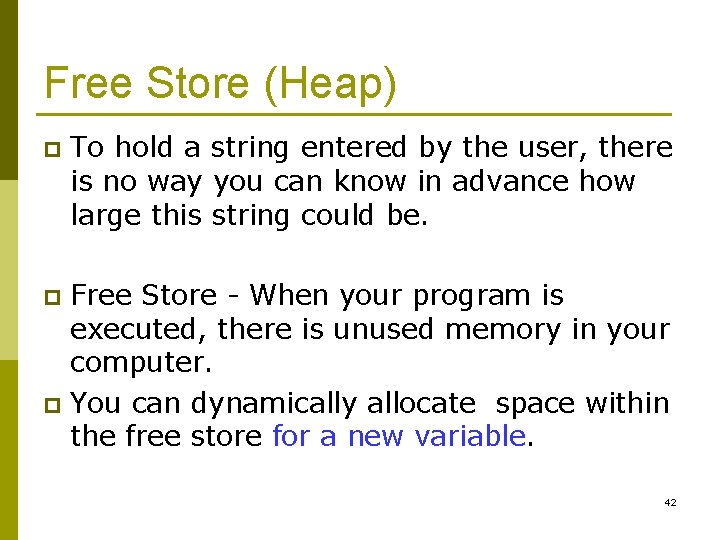
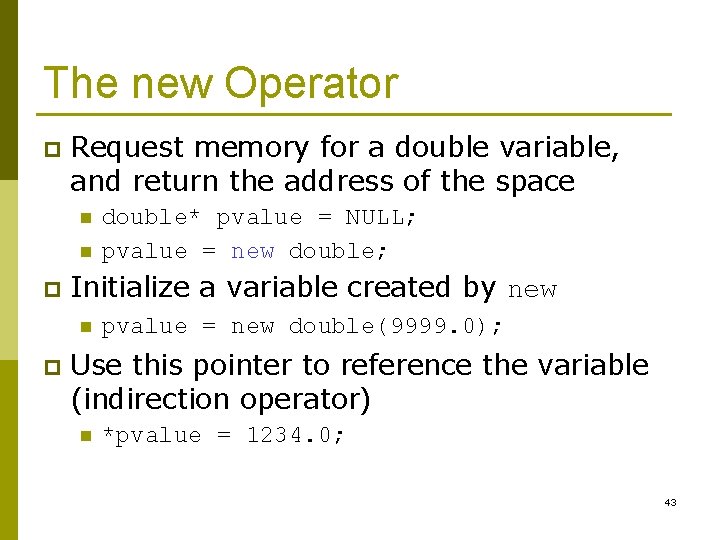
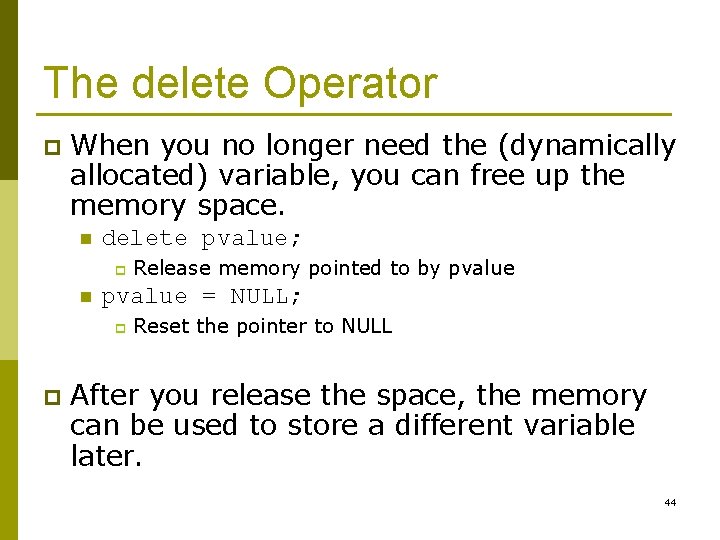
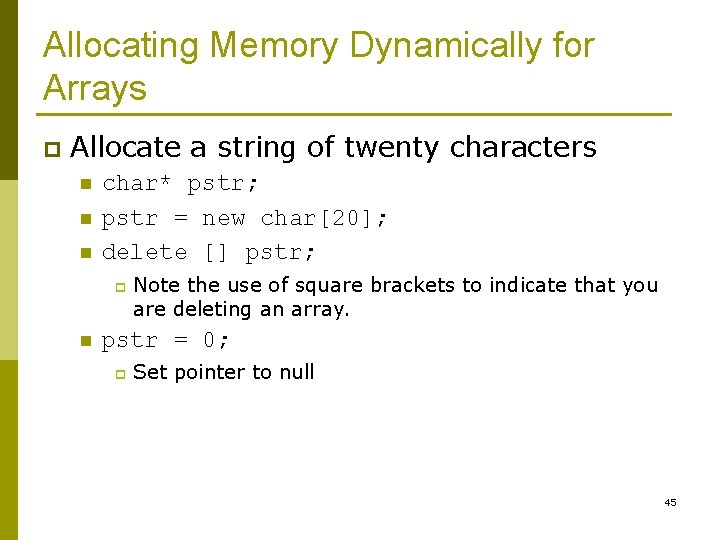
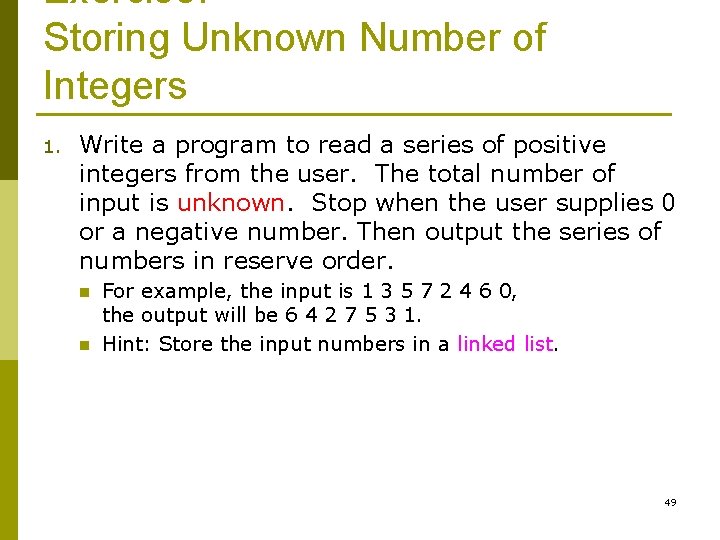
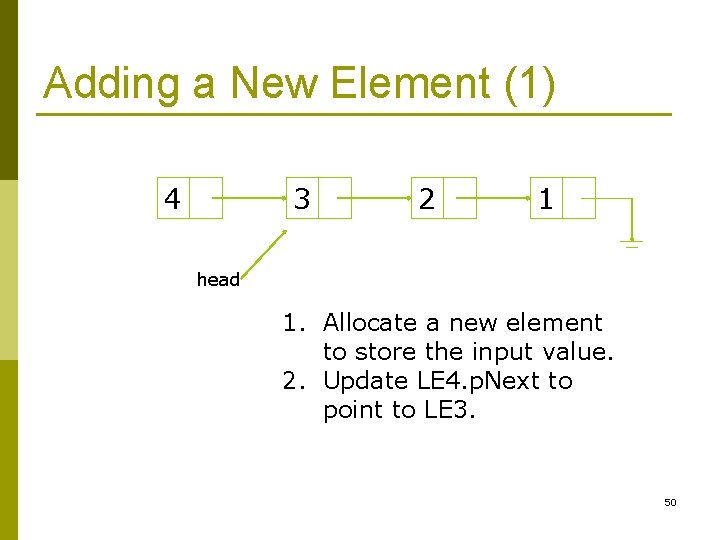
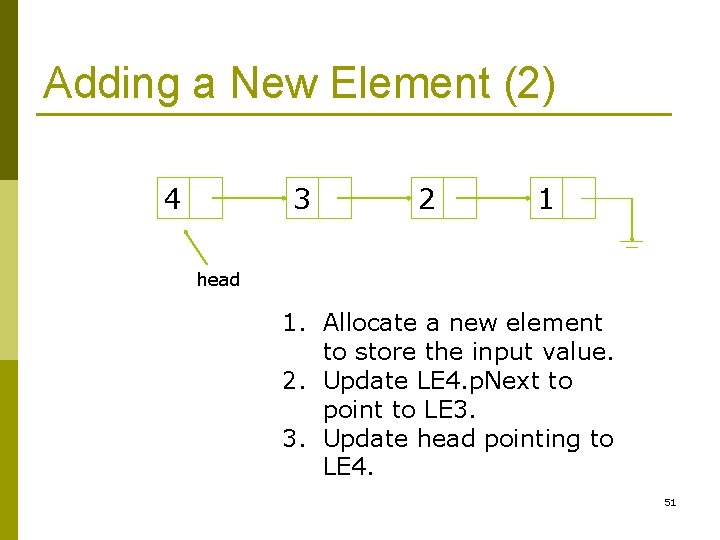
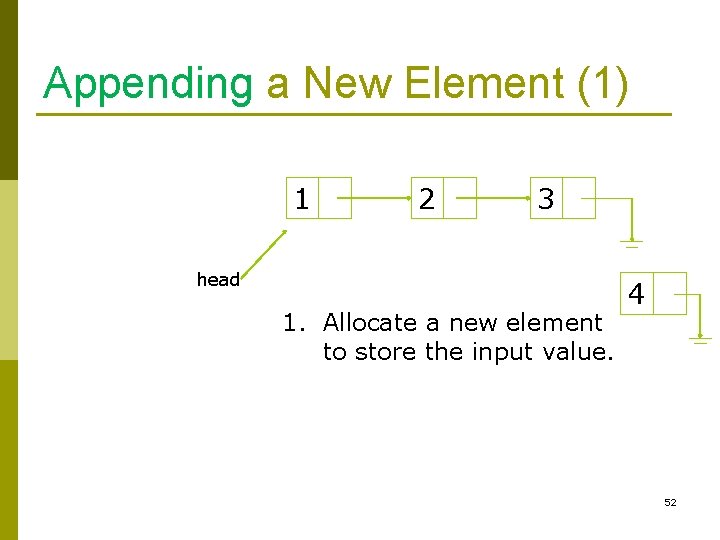
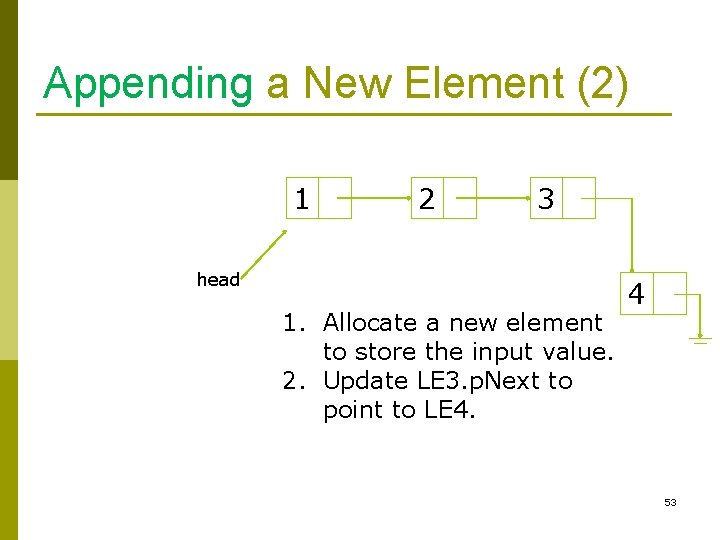
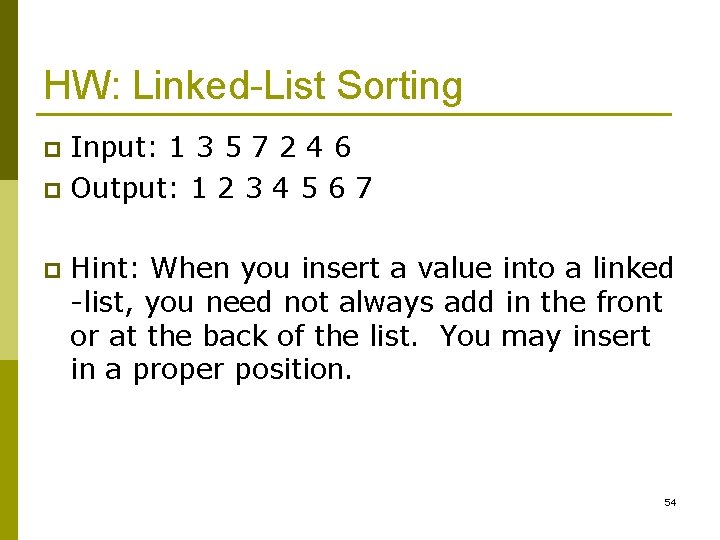
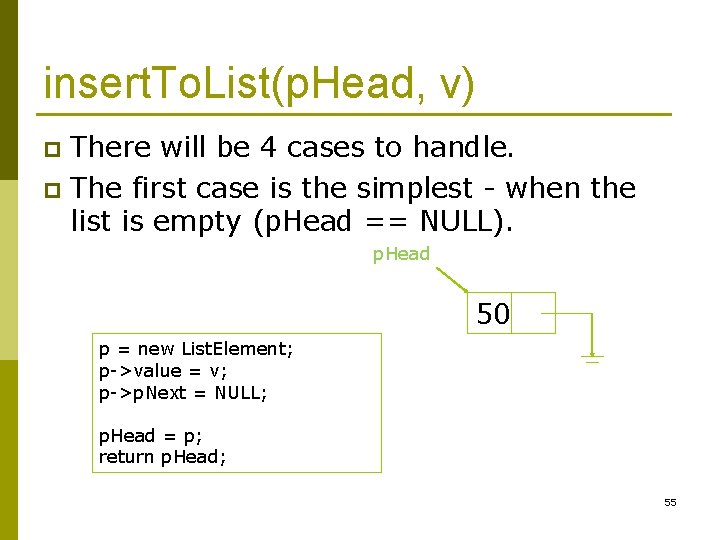
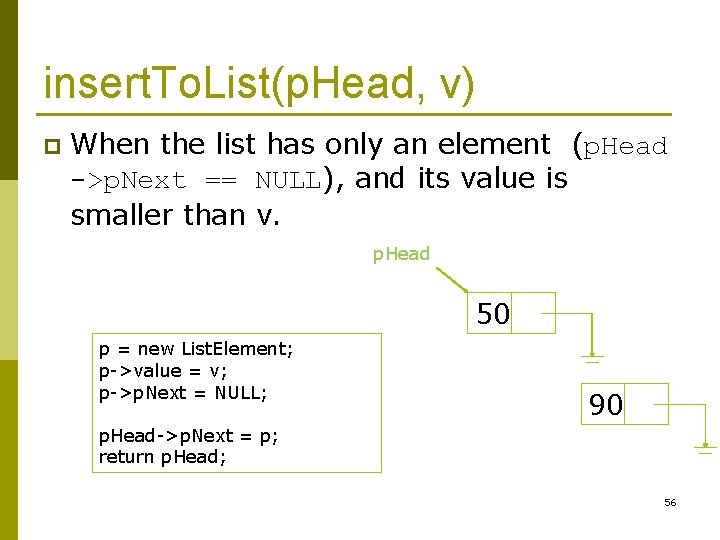
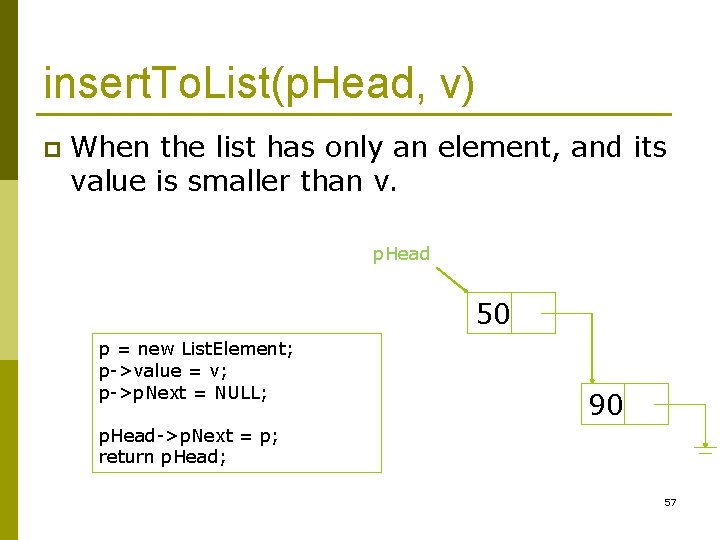
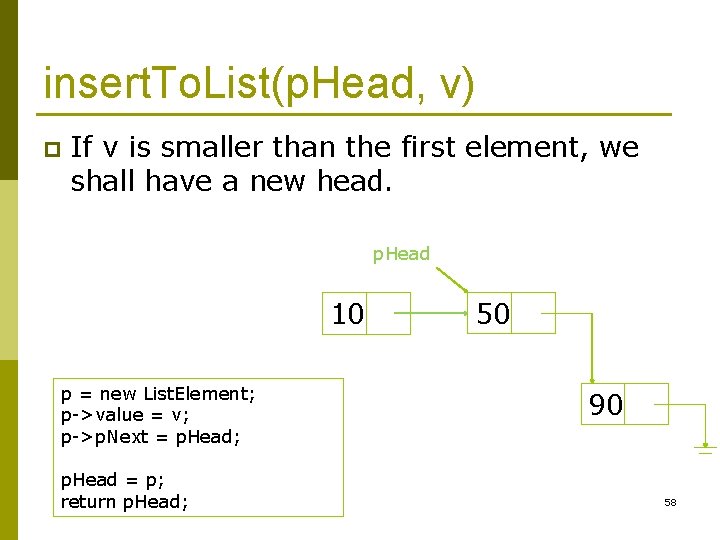
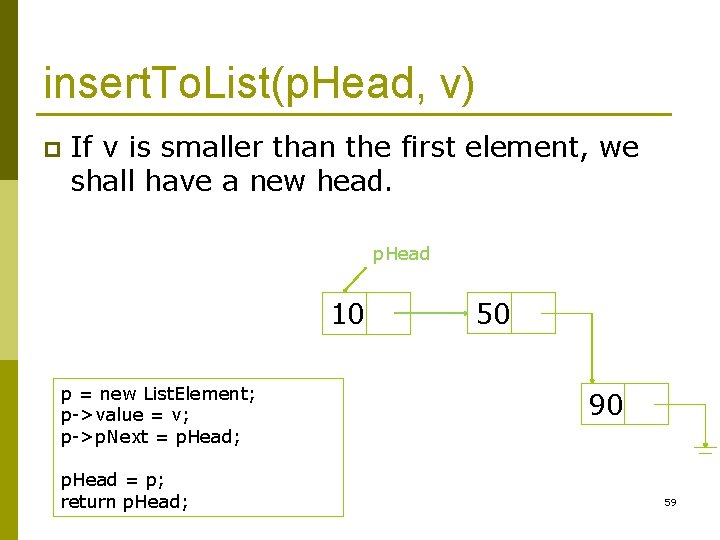
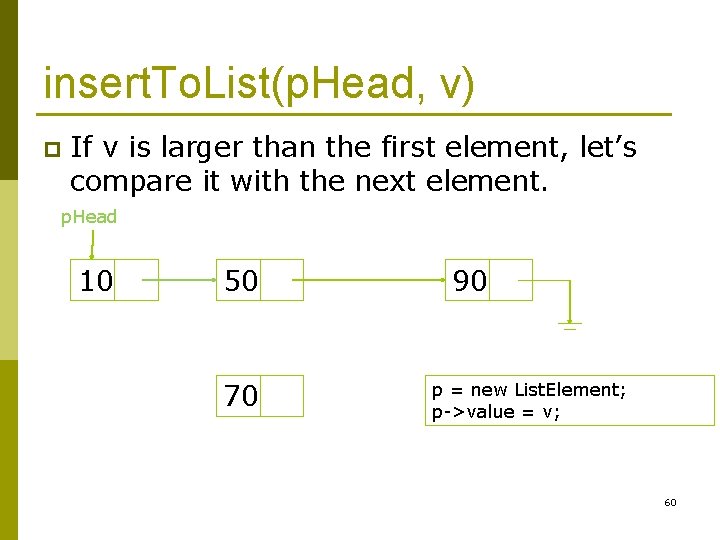
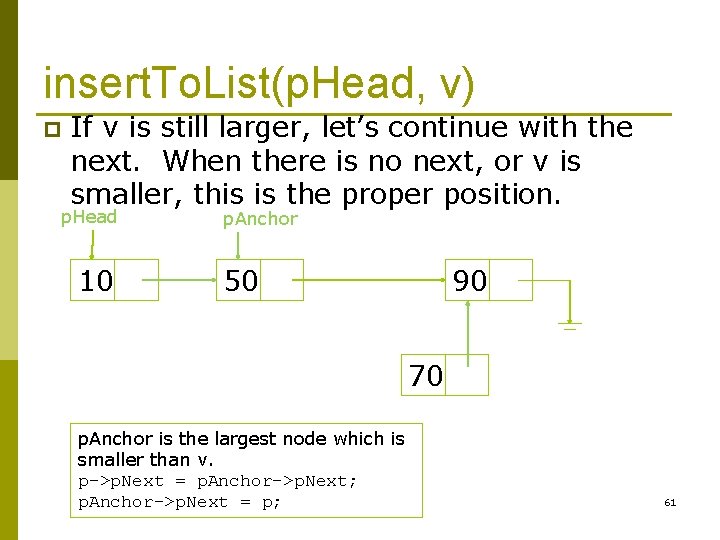
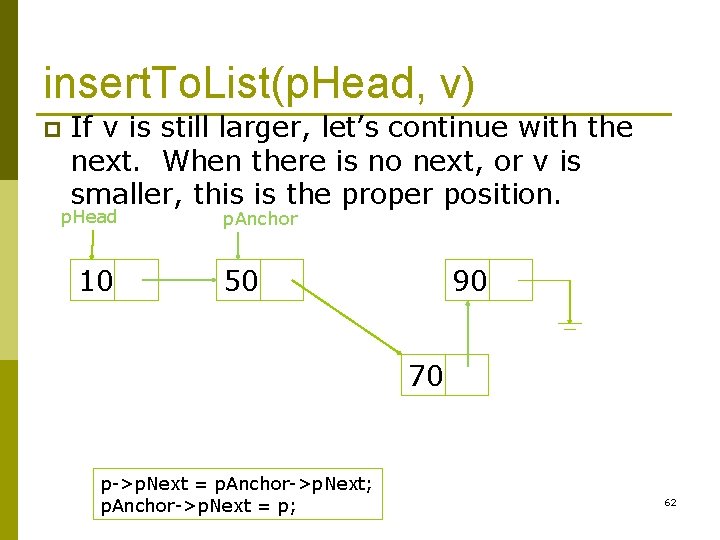
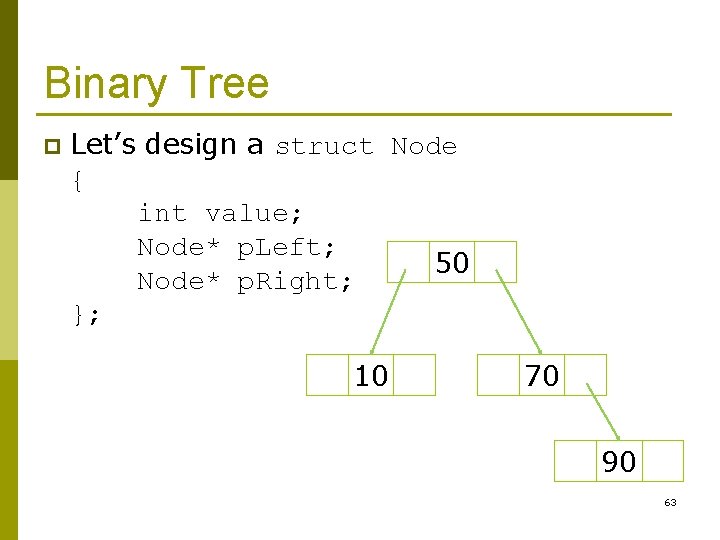
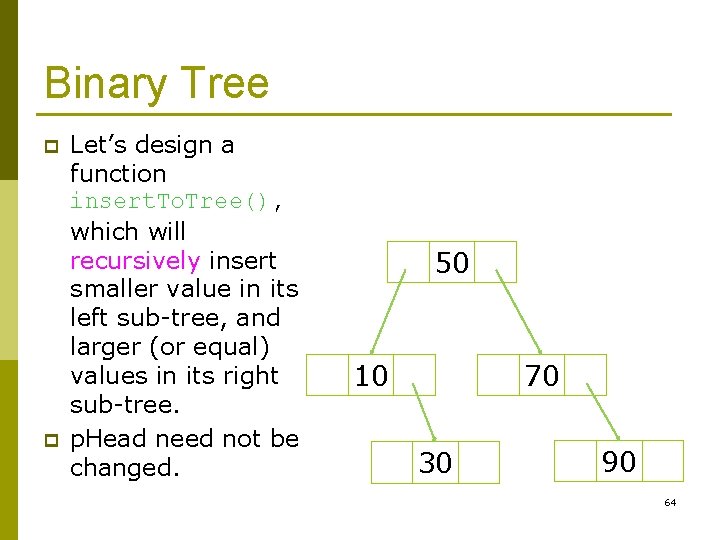
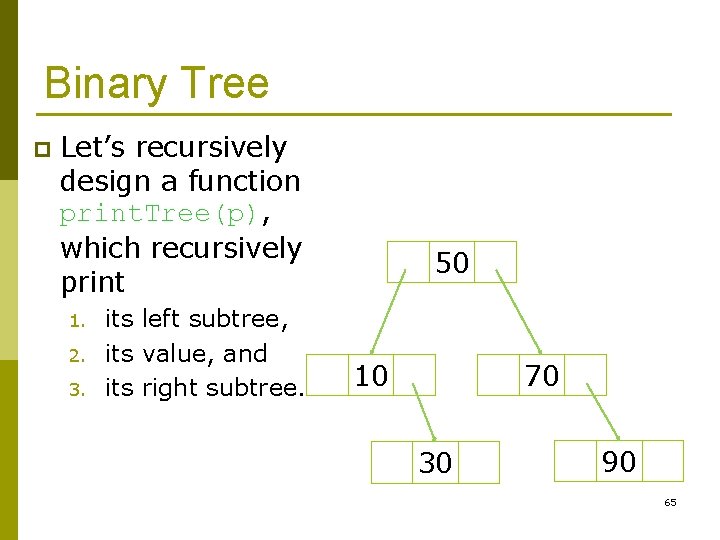
- Slides: 54
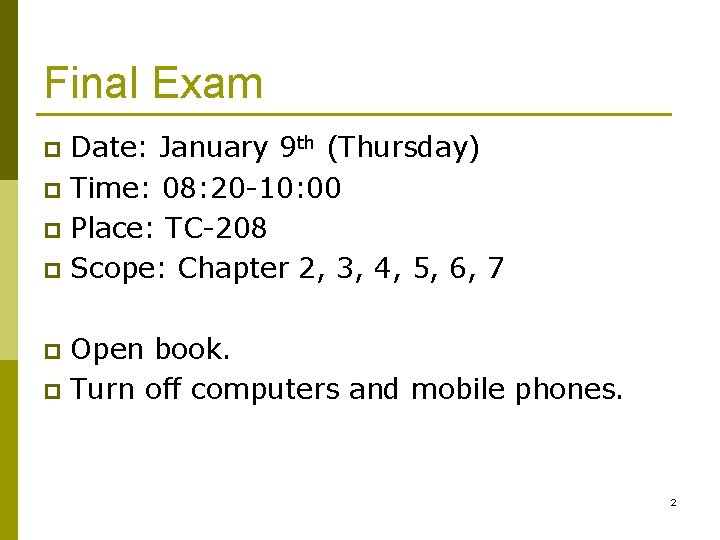
Final Exam Date: January 9 th (Thursday) p Time: 08: 20 -10: 00 p Place: TC-208 p Scope: Chapter 2, 3, 4, 5, 6, 7 p Open book. p Turn off computers and mobile phones. p 2
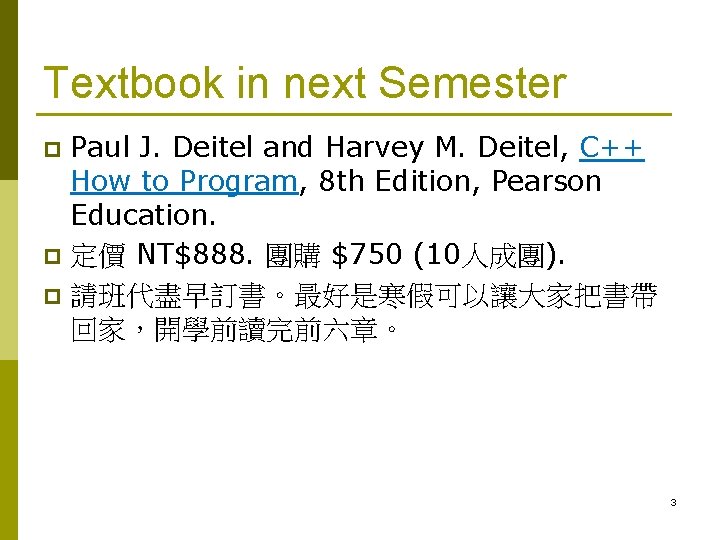
Textbook in next Semester Paul J. Deitel and Harvey M. Deitel, C++ How to Program, 8 th Edition, Pearson Education. p 定價 NT$888. 團購 $750 (10人成團). p 請班代盡早訂書。最好是寒假可以讓大家把書帶 回家,開學前讀完前六章。 p 3
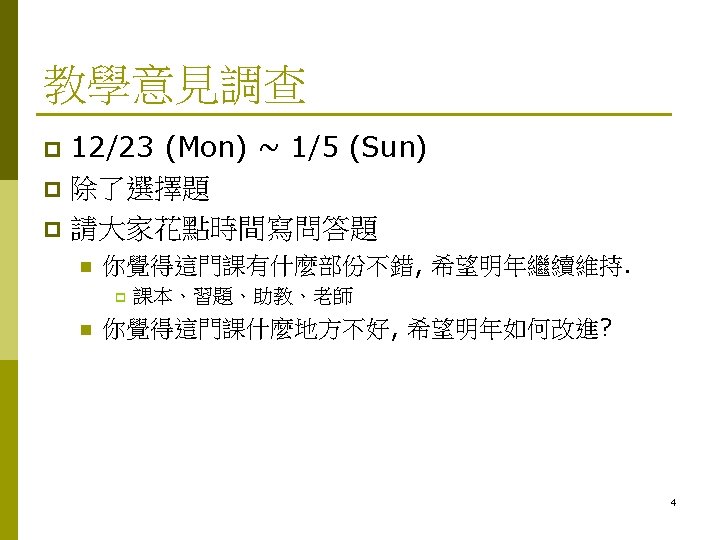
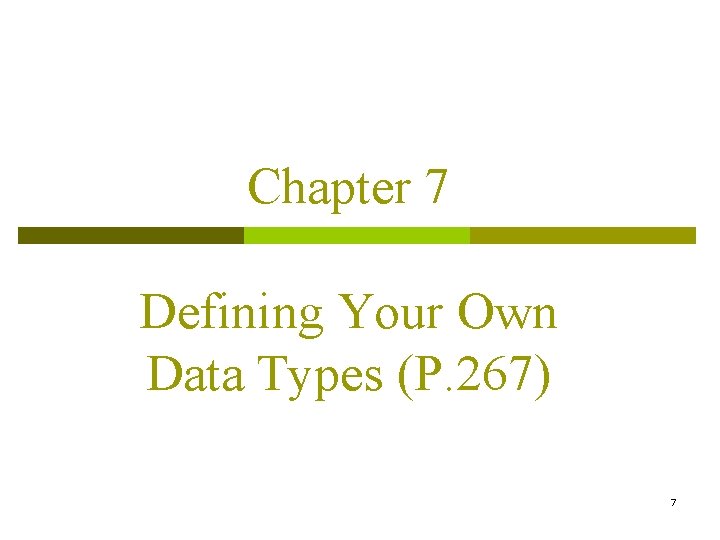
Chapter 7 Defining Your Own Data Types (P. 267) 7
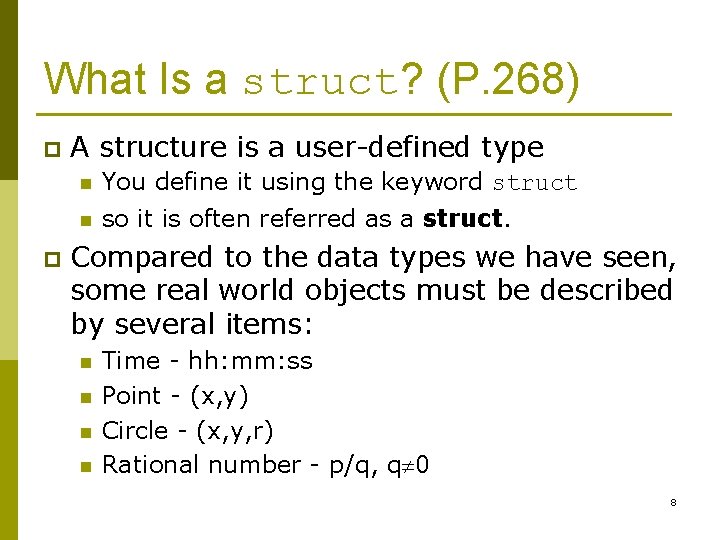
What Is a struct? (P. 268) p p A structure is a user-defined type n You define it using the keyword struct n so it is often referred as a struct. Compared to the data types we have seen, some real world objects must be described by several items: n n Time - hh: mm: ss Point - (x, y) Circle - (x, y, r) Rational number - p/q, q 0 8
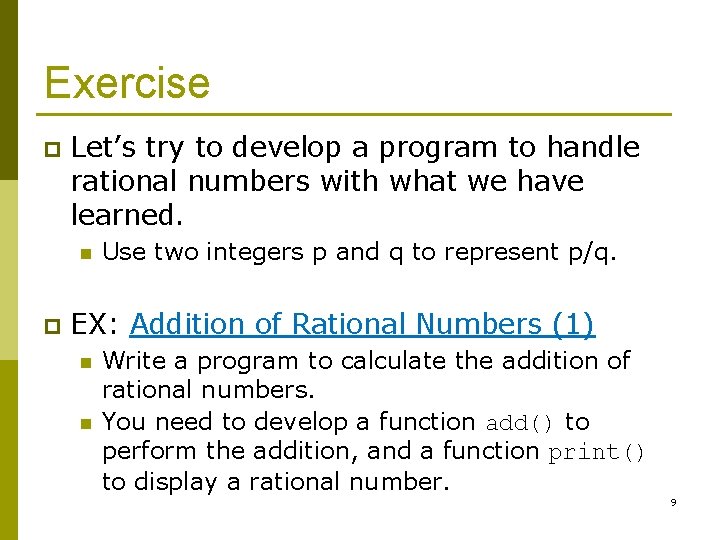
Exercise p Let’s try to develop a program to handle rational numbers with what we have learned. n p Use two integers p and q to represent p/q. EX: Addition of Rational Numbers (1) n n Write a program to calculate the addition of rational numbers. You need to develop a function add() to perform the addition, and a function print() to display a rational number. 9
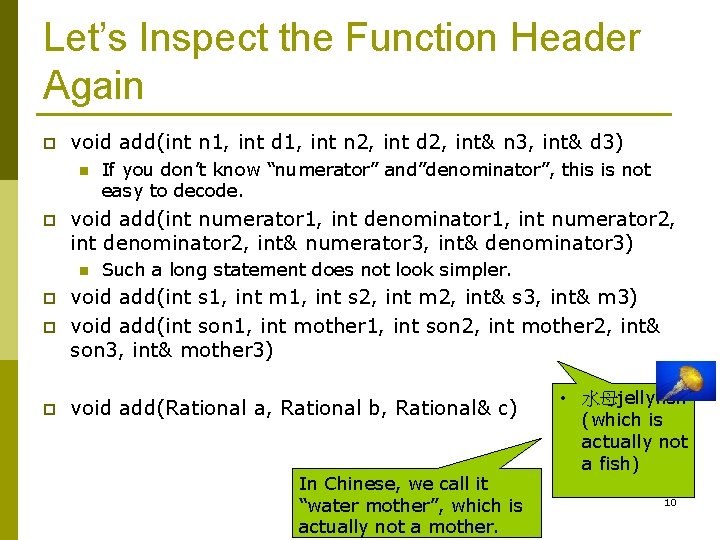
Let’s Inspect the Function Header Again p void add(int n 1, int d 1, int n 2, int d 2, int& n 3, int& d 3) n p If you don’t know “numerator” and”denominator”, this is not easy to decode. void add(int numerator 1, int denominator 1, int numerator 2, int denominator 2, int& numerator 3, int& denominator 3) n Such a long statement does not look simpler. p void add(int s 1, int m 1, int s 2, int m 2, int& s 3, int& m 3) void add(int son 1, int mother 1, int son 2, int mother 2, int& son 3, int& mother 3) p void add(Rational a, Rational b, Rational& c) p In Chinese, we call it “water mother”, which is actually not a mother. • 水母jellyfish (which is actually not a fish) 10
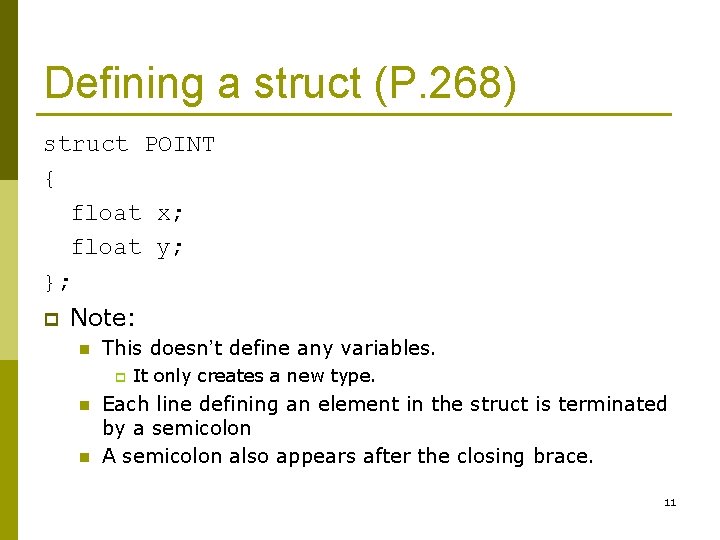
Defining a struct (P. 268) struct POINT { float x; float y; }; p Note: n This doesn’t define any variables. p n n It only creates a new type. Each line defining an element in the struct is terminated by a semicolon A semicolon also appears after the closing brace. 11
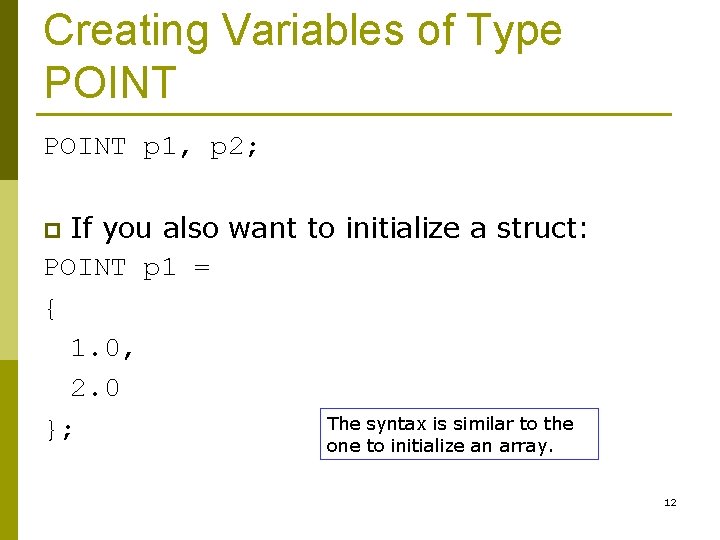
Creating Variables of Type POINT p 1, p 2; If you also want to initialize a struct: POINT p 1 = { 1. 0, 2. 0 The syntax is similar to the }; p one to initialize an array. 12
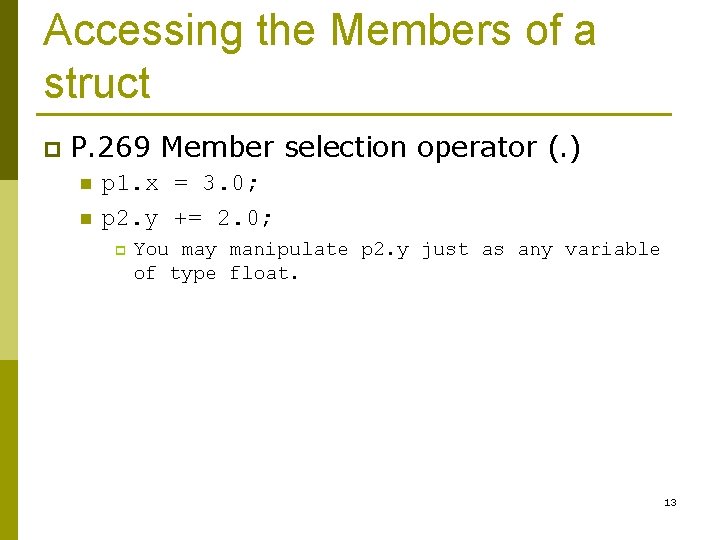
Accessing the Members of a struct p P. 269 Member selection operator (. ) n n p 1. x = 3. 0; p 2. y += 2. 0; p You may manipulate p 2. y just as any variable of type float. 13
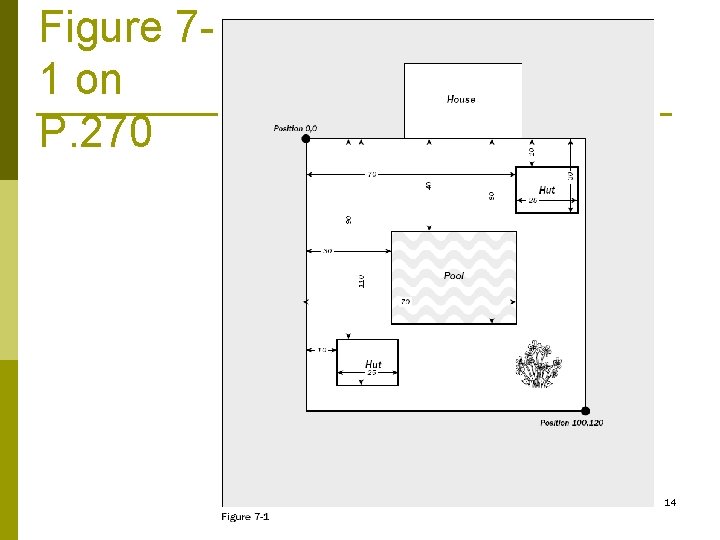
Figure 71 on P. 270 14
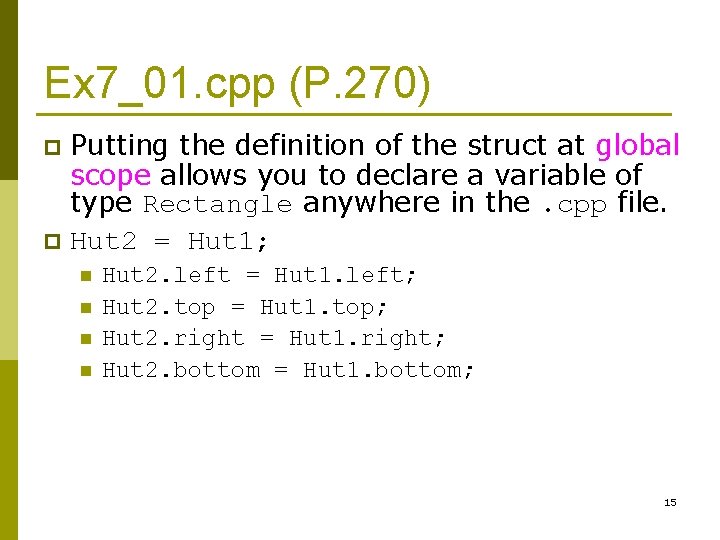
Ex 7_01. cpp (P. 270) Putting the definition of the struct at global scope allows you to declare a variable of type Rectangle anywhere in the. cpp file. p Hut 2 = Hut 1; p n n Hut 2. left = Hut 1. left; Hut 2. top = Hut 1. top; Hut 2. right = Hut 1. right; Hut 2. bottom = Hut 1. bottom; 15
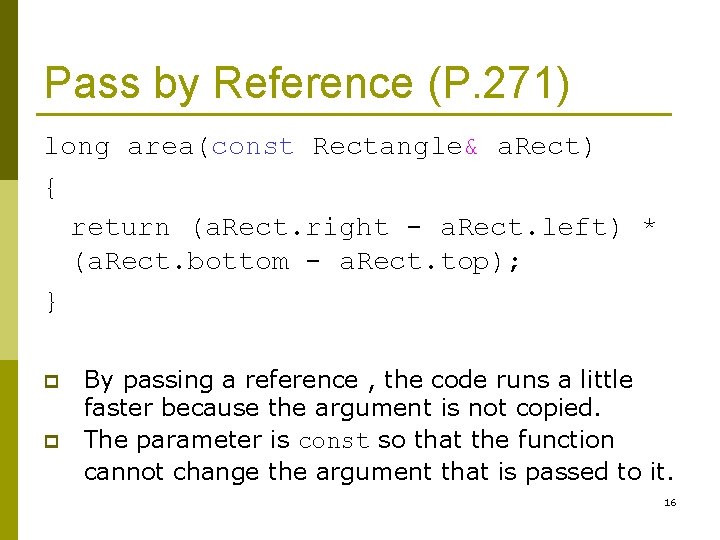
Pass by Reference (P. 271) long area(const Rectangle& a. Rect) { return (a. Rect. right - a. Rect. left) * (a. Rect. bottom - a. Rect. top); } p p By passing a reference , the code runs a little faster because the argument is not copied. The parameter is const so that the function cannot change the argument that is passed to it. 16
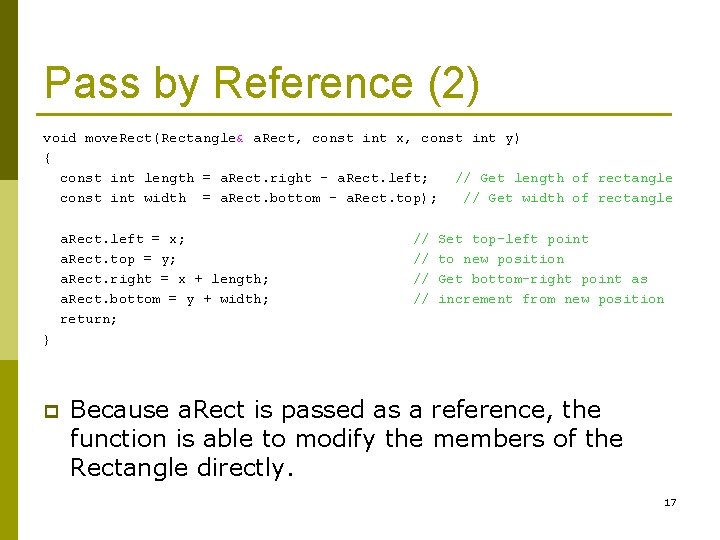
Pass by Reference (2) void move. Rect(Rectangle& a. Rect, const int x, const int y) { const int length = a. Rect. right - a. Rect. left; // Get length of rectangle const int width = a. Rect. bottom - a. Rect. top); // Get width of rectangle a. Rect. left = x; a. Rect. top = y; a. Rect. right = x + length; a. Rect. bottom = y + width; return; // // Set top-left point to new position Get bottom-right point as increment from new position } p Because a. Rect is passed as a reference, the function is able to modify the members of the Rectangle directly. 17
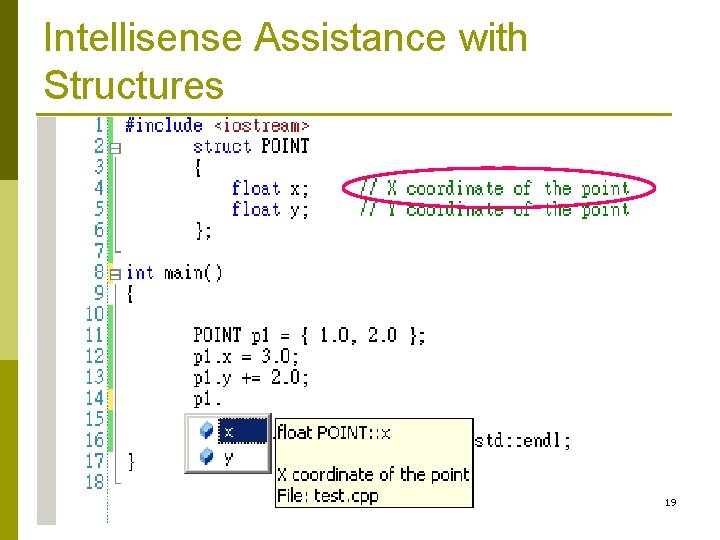
Intellisense Assistance with Structures 19
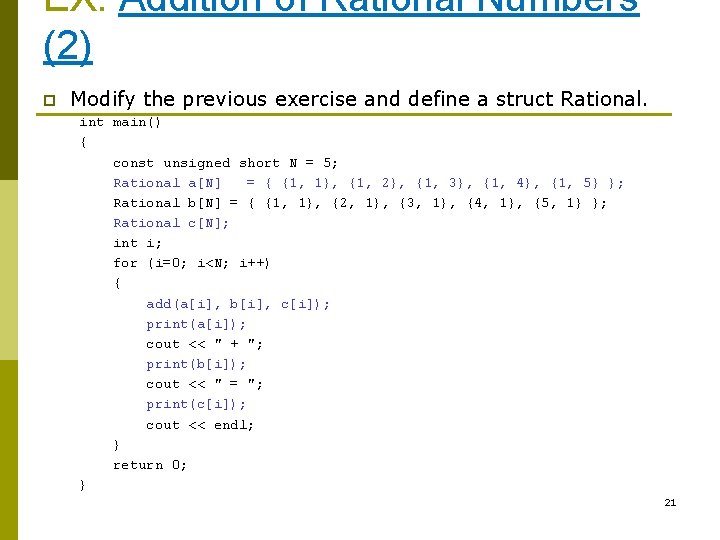
EX: Addition of Rational Numbers (2) p Modify the previous exercise and define a struct Rational. int main() { const unsigned short N = 5; Rational a[N] = { {1, 1}, {1, 2}, {1, 3}, {1, 4}, {1, 5} }; Rational b[N] = { {1, 1}, {2, 1}, {3, 1}, {4, 1}, {5, 1} }; Rational c[N]; int i; for (i=0; i<N; i++) { add(a[i], b[i], c[i]); print(a[i]); cout << " + "; print(b[i]); cout << " = "; print(c[i]); cout << endl; } return 0; } 21
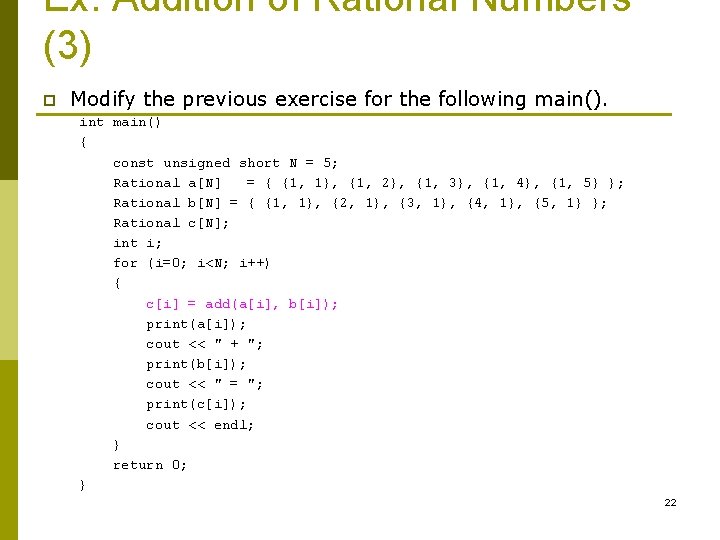
Ex: Addition of Rational Numbers (3) p Modify the previous exercise for the following main(). int main() { const unsigned short N = 5; Rational a[N] = { {1, 1}, {1, 2}, {1, 3}, {1, 4}, {1, 5} }; Rational b[N] = { {1, 1}, {2, 1}, {3, 1}, {4, 1}, {5, 1} }; Rational c[N]; int i; for (i=0; i<N; i++) { c[i] = add(a[i], b[i]); print(a[i]); cout << " + "; print(b[i]); cout << " = "; print(c[i]); cout << endl; } return 0; } 22
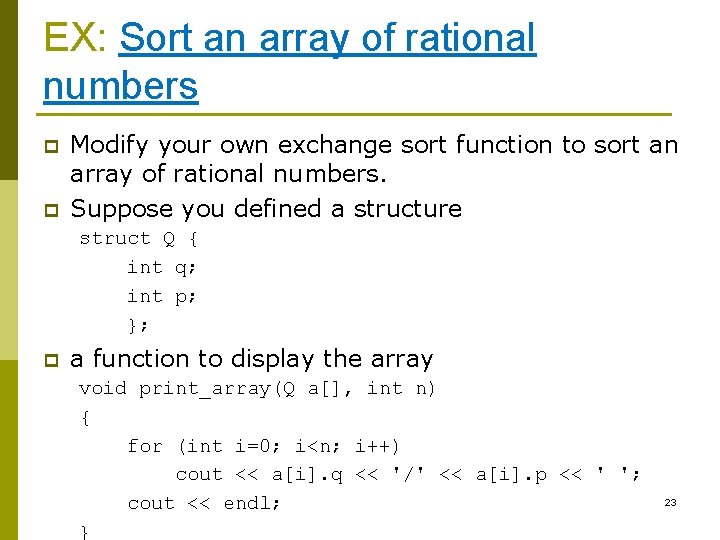
EX: Sort an array of rational numbers p p Modify your own exchange sort function to sort an array of rational numbers. Suppose you defined a structure struct Q { int q; int p; }; p a function to display the array void print_array(Q a[], int n) { for (int i=0; i<n; i++) cout << a[i]. q << '/' << a[i]. p << ' '; cout << endl; } 23
![p and a main program to test it int main Q a p and a main program to test it. int main() { Q a[] =](https://slidetodoc.com/presentation_image_h2/90330dcb96790c9e60adb0ff998e0611/image-19.jpg)
p and a main program to test it. int main() { Q a[] = { {7, 3}, {1, 5}, {6, 5}, {4, 3} }; int size = sizeof(a) / sizeof(a[0]); print_array(a, size); exchange_sort(a, size, cmp); print_array(a, size); The output should be 7/3 1/5 6/5 4/3 return 0; 1/5 6/5 4/3 7/3 } p Now all you need to supply is a cmp() function and a revised exchange_sort() function. 24
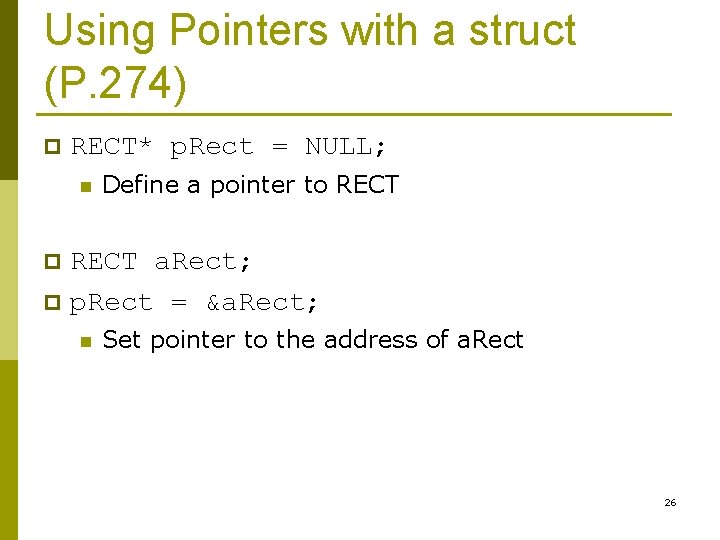
Using Pointers with a struct (P. 274) p RECT* p. Rect = NULL; n Define a pointer to RECT a. Rect; p p. Rect = &a. Rect; p n Set pointer to the address of a. Rect 26
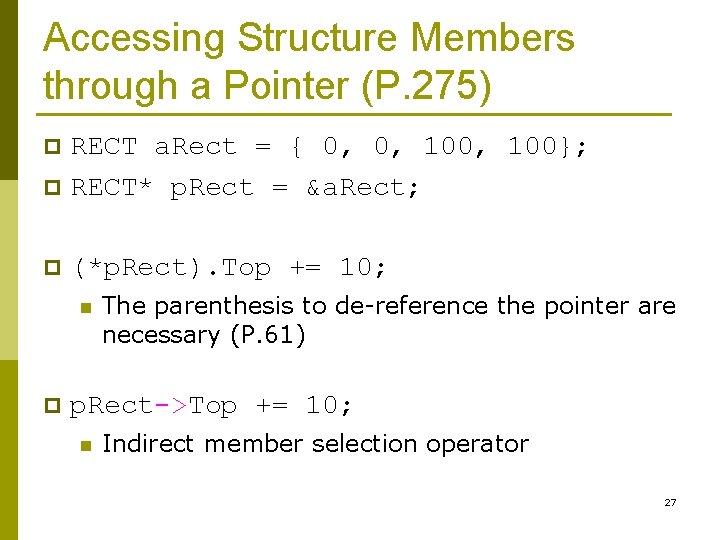
Accessing Structure Members through a Pointer (P. 275) RECT a. Rect = { 0, 0, 100}; p RECT* p. Rect = &a. Rect; p p (*p. Rect). Top += 10; n p The parenthesis to de-reference the pointer are necessary (P. 61) p. Rect->Top += 10; n Indirect member selection operator 27
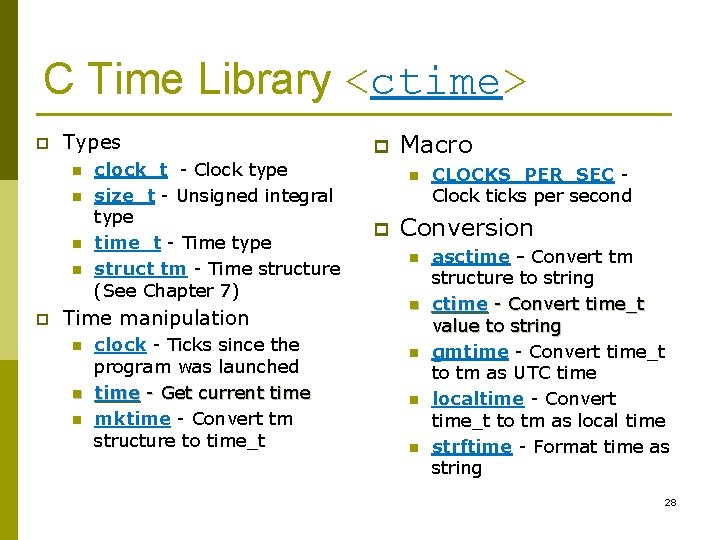
C Time Library <ctime> p Types n n p clock_t - Clock type size_t - Unsigned integral type time_t - Time type struct tm - Time structure (See Chapter 7) Time manipulation n clock - Ticks since the program was launched time - Get current time mktime - Convert tm structure to time_t p Macro n p CLOCKS_PER_SEC Clock ticks per second Conversion n n asctime - Convert tm structure to string ctime - Convert time_t value to string gmtime - Convert time_t to tm as UTC time localtime - Convert time_t to tm as local time strftime - Format time as string 28
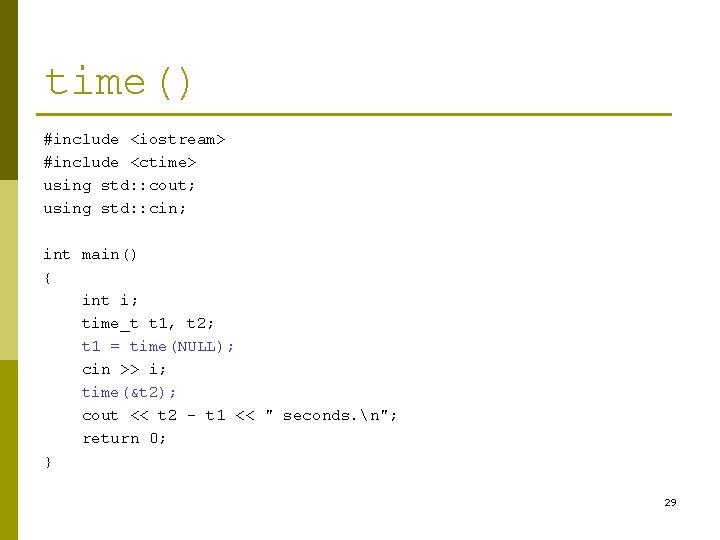
time() #include <iostream> #include <ctime> using std: : cout; using std: : cin; int main() { int i; time_t t 1, t 2; t 1 = time(NULL); cin >> i; time(&t 2); cout << t 2 - t 1 << " seconds. n"; return 0; } 29
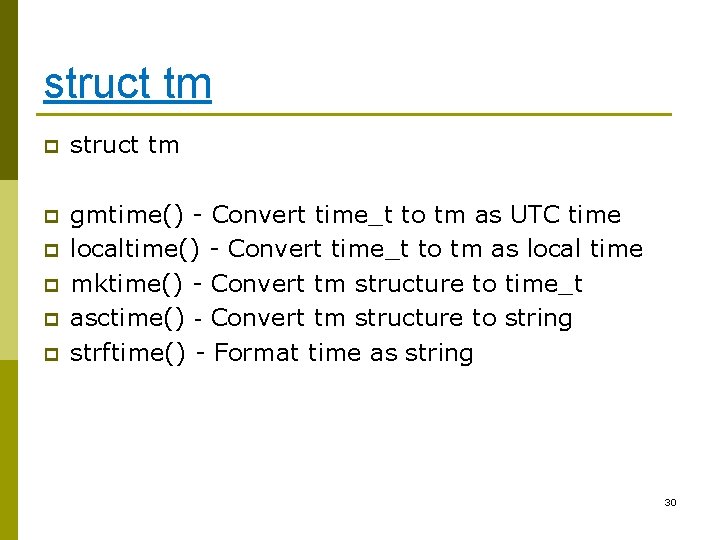
struct tm p gmtime() - Convert time_t to tm as UTC time localtime() - Convert time_t to tm as local time mktime() - Convert tm structure to time_t asctime() - Convert tm structure to string strftime() - Format time as string p p 30
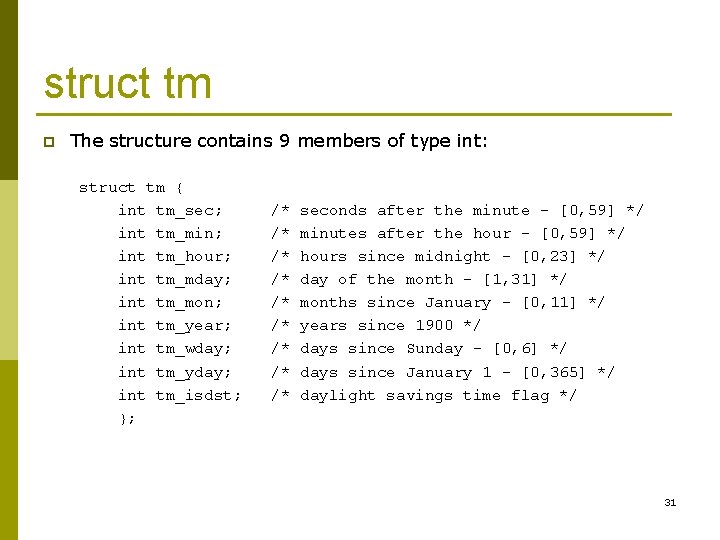
struct tm p The structure contains 9 members of type int: struct tm { int tm_sec; int tm_min; int tm_hour; int tm_mday; int tm_mon; int tm_year; int tm_wday; int tm_yday; int tm_isdst; }; /* /* /* seconds after the minute - [0, 59] */ minutes after the hour - [0, 59] */ hours since midnight - [0, 23] */ day of the month - [1, 31] */ months since January - [0, 11] */ years since 1900 */ days since Sunday - [0, 6] */ days since January 1 - [0, 365] */ daylight savings time flag */ 31
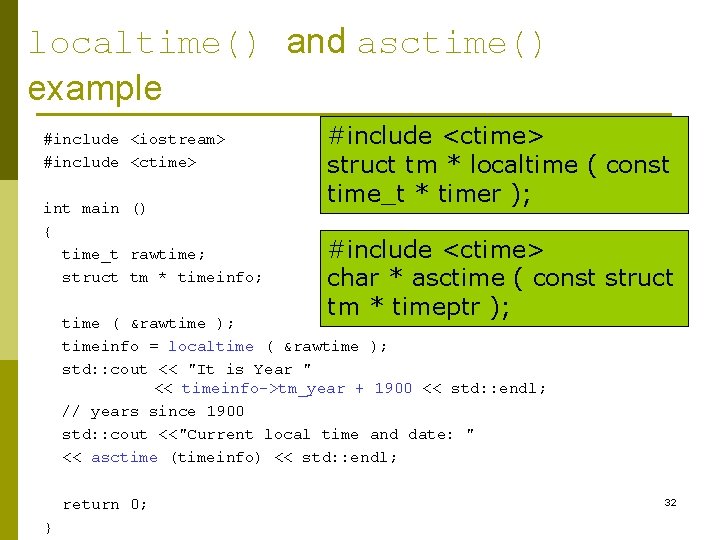
localtime() and asctime() example #include <iostream> #include <ctime> int main () { time_t rawtime; struct tm * timeinfo; #include <ctime> struct tm * localtime ( const time_t * timer ); #include <ctime> char * asctime ( const struct tm * timeptr ); time ( &rawtime ); timeinfo = localtime ( &rawtime ); std: : cout << "It is Year " << timeinfo->tm_year + 1900 << std: : endl; // years since 1900 std: : cout <<"Current local time and date: " << asctime (timeinfo) << std: : endl; return 0; } 32
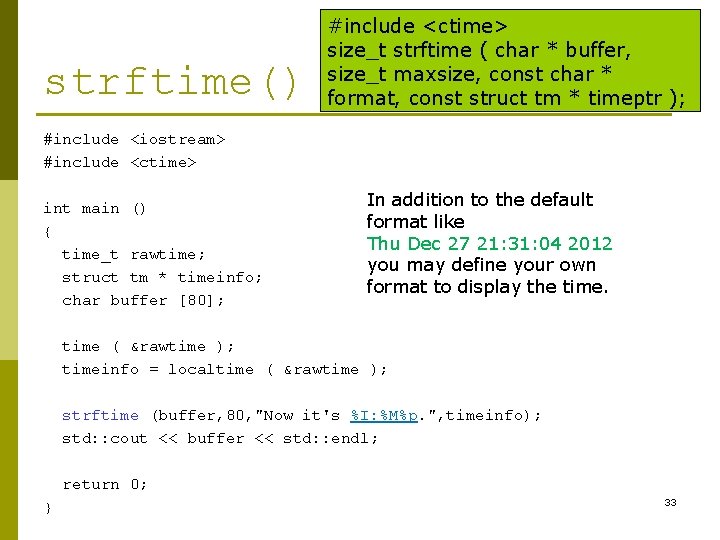
strftime() #include <ctime> size_t strftime ( char * buffer, size_t maxsize, const char * format, const struct tm * timeptr ); #include <iostream> #include <ctime> int main () { time_t rawtime; struct tm * timeinfo; char buffer [80]; In addition to the default format like Thu Dec 27 21: 31: 04 2012 you may define your own format to display the time ( &rawtime ); timeinfo = localtime ( &rawtime ); strftime (buffer, 80, "Now it's %I: %M%p. ", timeinfo); std: : cout << buffer << std: : endl; return 0; } 33
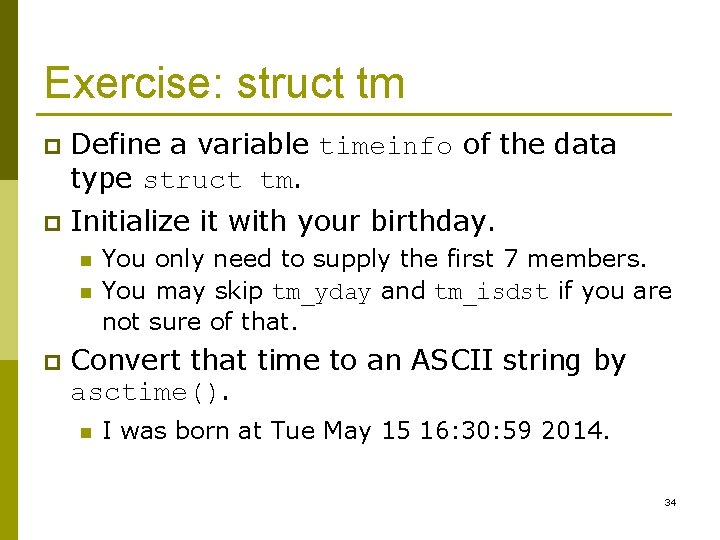
Exercise: struct tm p Define a variable timeinfo of the data type struct tm. p Initialize it with your birthday. n n p You only need to supply the first 7 members. You may skip tm_yday and tm_isdst if you are not sure of that. Convert that time to an ASCII string by asctime(). n I was born at Tue May 15 16: 30: 59 2014. 34
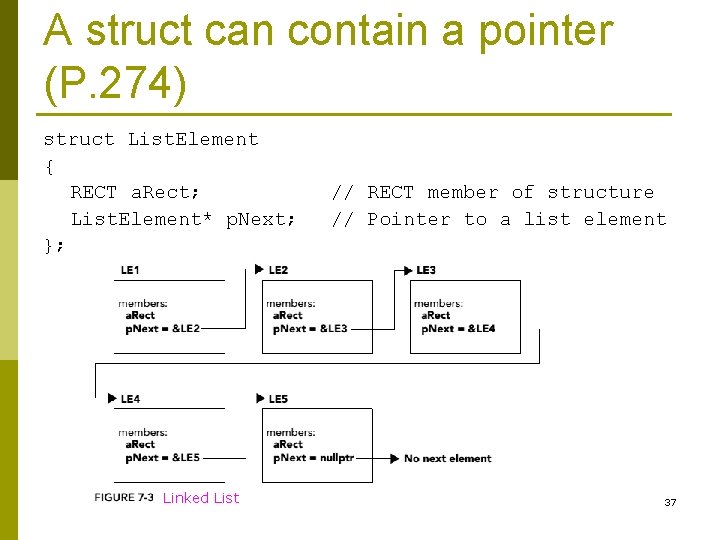
A struct can contain a pointer (P. 274) struct List. Element { RECT a. Rect; List. Element* p. Next; }; Linked List // RECT member of structure // Pointer to a list element 37
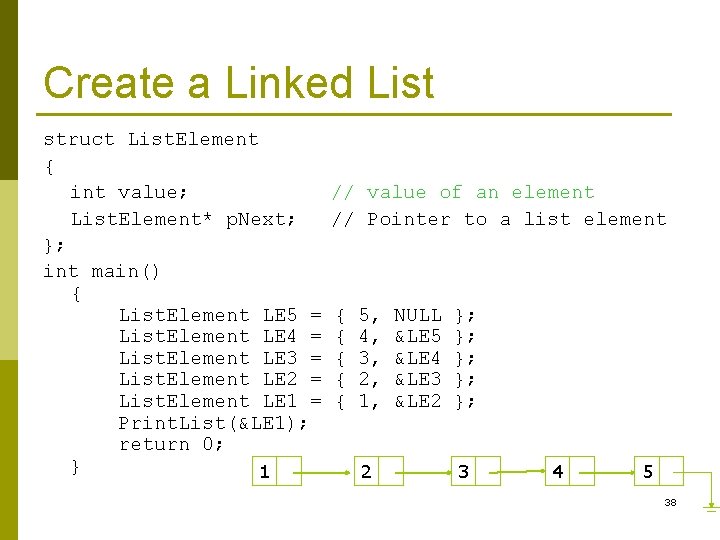
Create a Linked List struct List. Element { int value; List. Element* p. Next; }; int main() { List. Element LE 5 = List. Element LE 4 = List. Element LE 3 = List. Element LE 2 = List. Element LE 1 = Print. List(&LE 1); return 0; } 1 // value of an element // Pointer to a list element { { { 5, 4, 3, 2, 1, 2 NULL &LE 5 &LE 4 &LE 3 &LE 2 }; }; }; 3 4 5 38
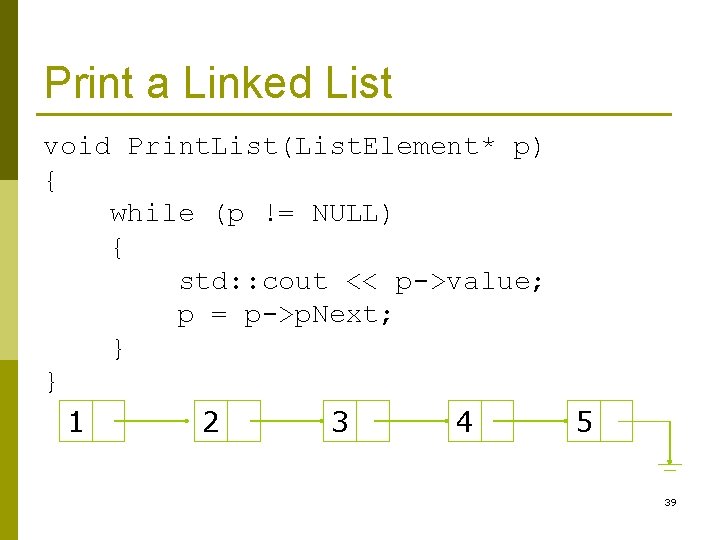
Print a Linked List void Print. List(List. Element* p) { while (p != NULL) { std: : cout << p->value; p = p->p. Next; } } 1 2 3 4 5 39
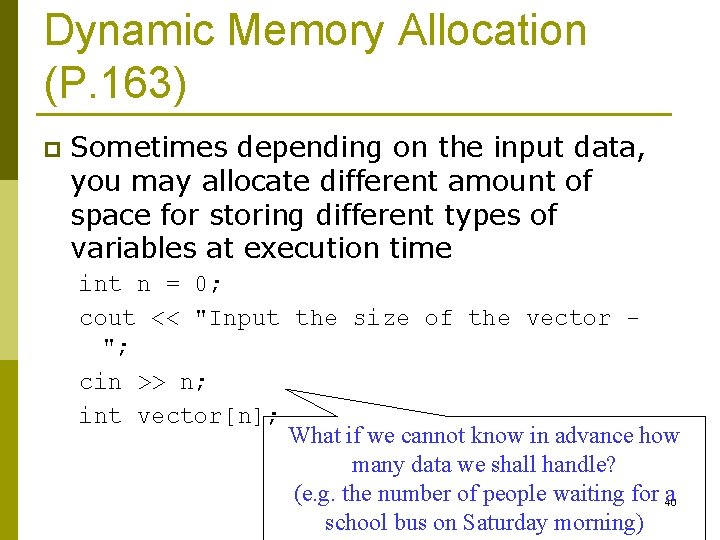
Dynamic Memory Allocation (P. 163) p Sometimes depending on the input data, you may allocate different amount of space for storing different types of variables at execution time int n = 0; cout << "Input the size of the vector "; cin >> n; int vector[n]; What if we cannot know in advance how many data we shall handle? (e. g. the number of people waiting for 40 a school bus on Saturday morning)
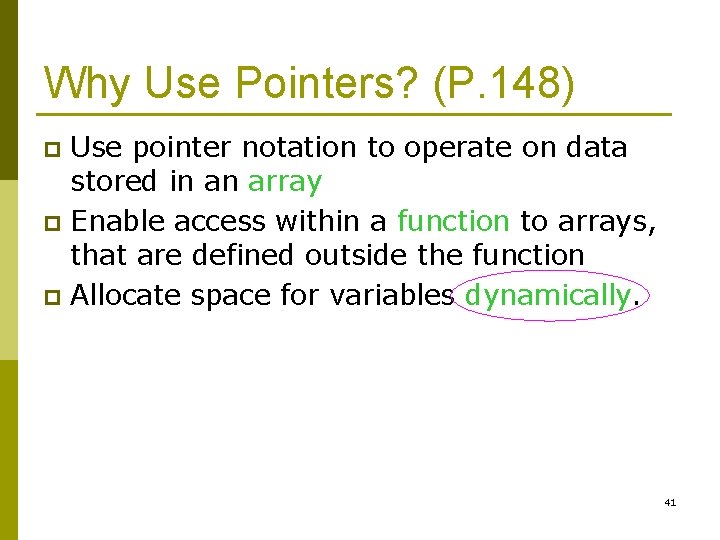
Why Use Pointers? (P. 148) Use pointer notation to operate on data stored in an array p Enable access within a function to arrays, that are defined outside the function p Allocate space for variables dynamically. p 41
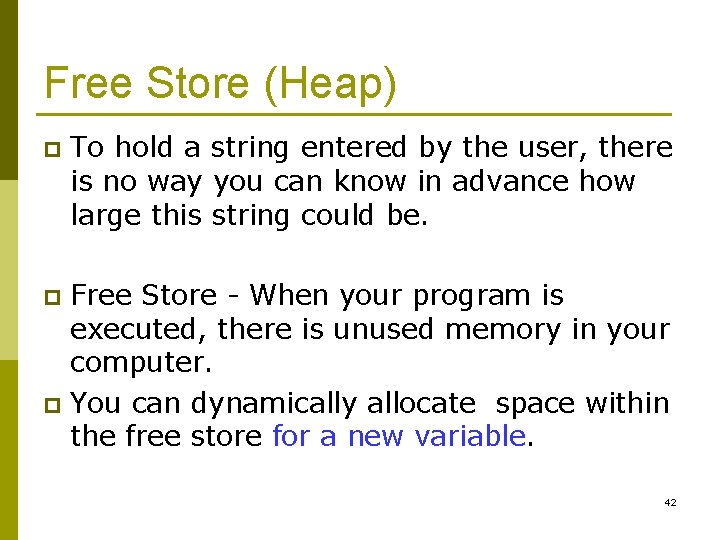
Free Store (Heap) p To hold a string entered by the user, there is no way you can know in advance how large this string could be. Free Store - When your program is executed, there is unused memory in your computer. p You can dynamically allocate space within the free store for a new variable. p 42
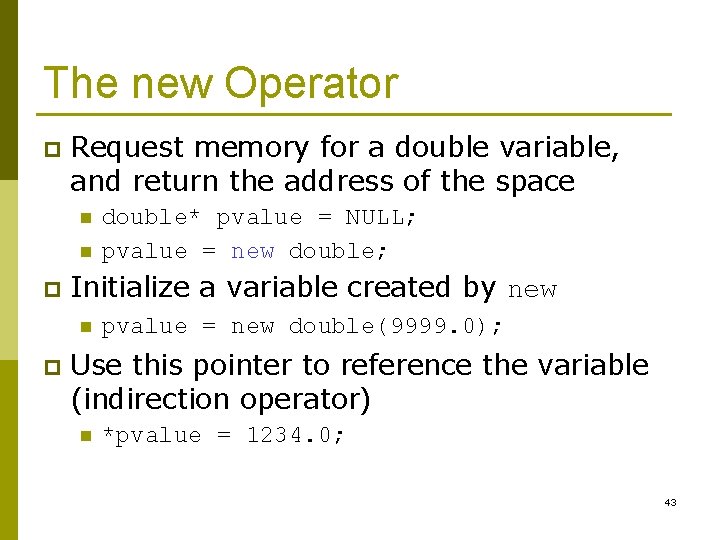
The new Operator p Request memory for a double variable, and return the address of the space n n p Initialize a variable created by new n p double* pvalue = NULL; pvalue = new double(9999. 0); Use this pointer to reference the variable (indirection operator) n *pvalue = 1234. 0; 43
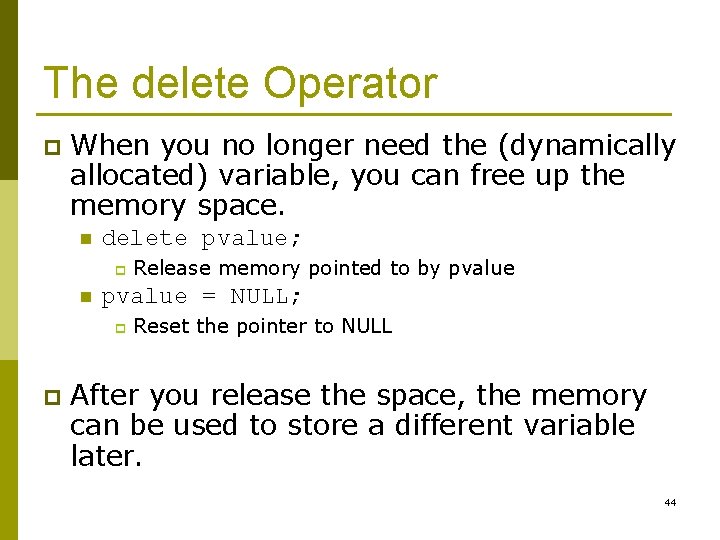
The delete Operator p When you no longer need the (dynamically allocated) variable, you can free up the memory space. n delete pvalue; p n pvalue = NULL; p p Release memory pointed to by pvalue Reset the pointer to NULL After you release the space, the memory can be used to store a different variable later. 44
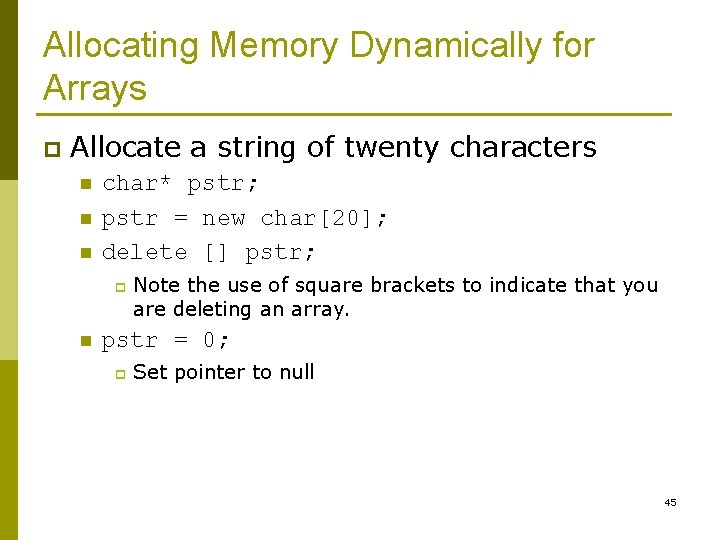
Allocating Memory Dynamically for Arrays p Allocate a string of twenty characters n n n char* pstr; pstr = new char[20]; delete [] pstr; p n Note the use of square brackets to indicate that you are deleting an array. pstr = 0; p Set pointer to null 45
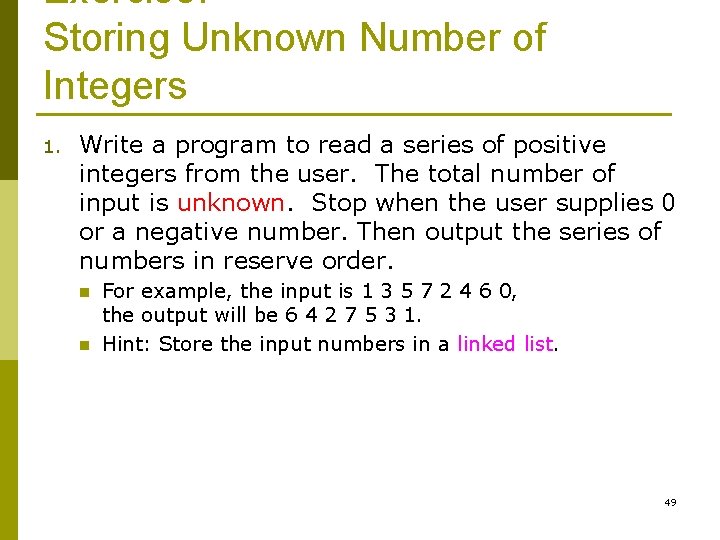
Exercise: Storing Unknown Number of Integers 1. Write a program to read a series of positive integers from the user. The total number of input is unknown. Stop when the user supplies 0 or a negative number. Then output the series of numbers in reserve order. n n For example, the input is 1 3 5 7 2 4 6 0, the output will be 6 4 2 7 5 3 1. Hint: Store the input numbers in a linked list. 49
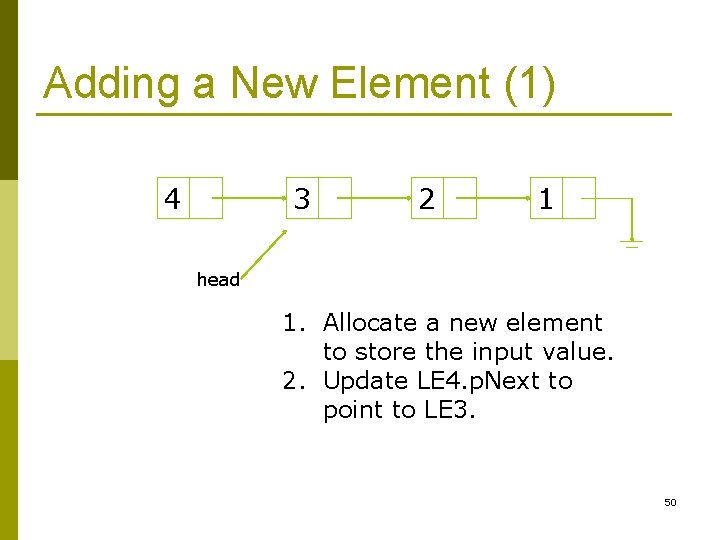
Adding a New Element (1) 4 3 2 1 head 1. Allocate a new element to store the input value. 2. Update LE 4. p. Next to point to LE 3. 3. Update head pointing to LE 4. 50
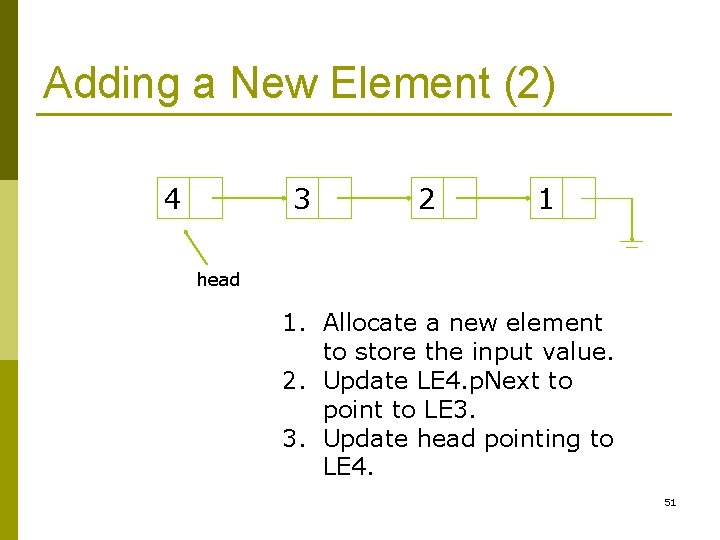
Adding a New Element (2) 4 3 2 1 head 1. Allocate a new element to store the input value. 2. Update LE 4. p. Next to point to LE 3. 3. Update head pointing to LE 4. 51
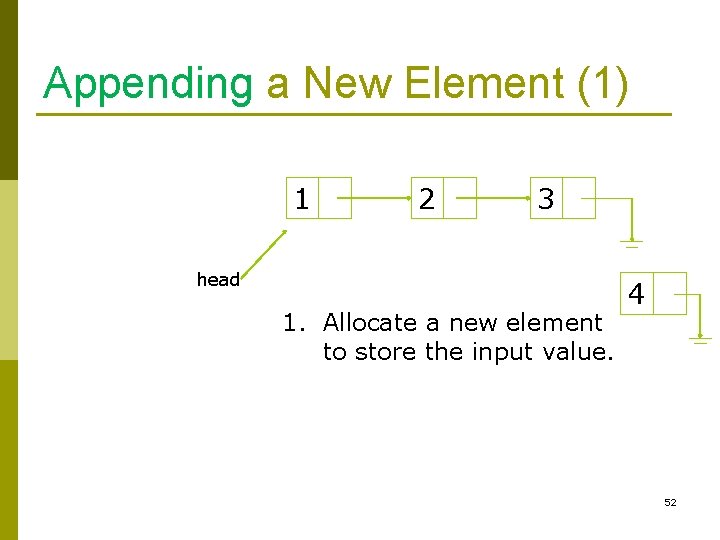
Appending a New Element (1) 1 2 3 head 1. Allocate a new element to store the input value. 2. Update LE 3. p. Next to point to LE 4. 3. Update head pointing to LE 4. 4 52
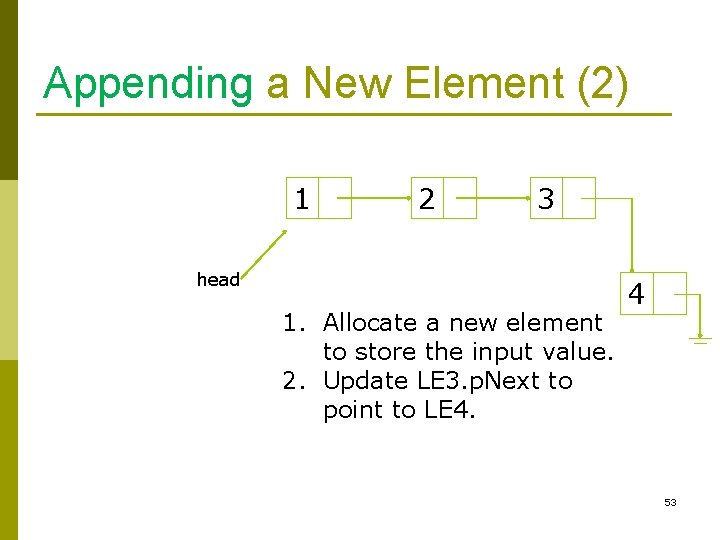
Appending a New Element (2) 1 2 3 head 1. Allocate a new element to store the input value. 2. Update LE 3. p. Next to point to LE 4. 3. Update head pointing to LE 4. 4 53
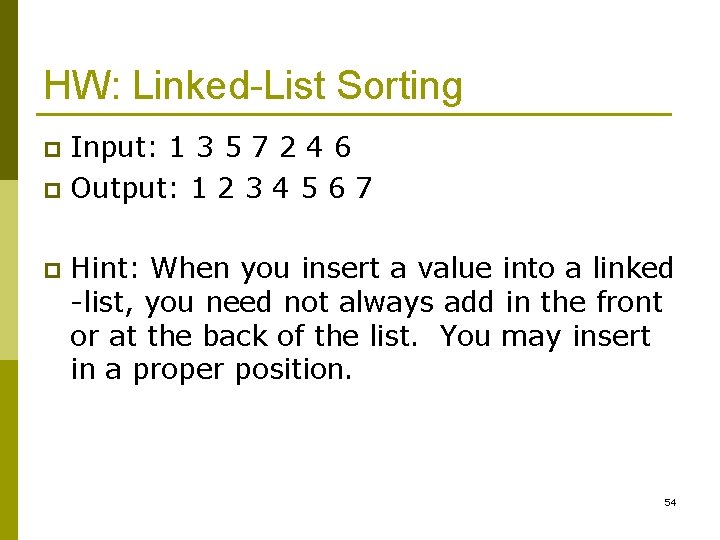
HW: Linked-List Sorting Input: 1 3 5 7 2 4 6 p Output: 1 2 3 4 5 6 7 p p Hint: When you insert a value into a linked -list, you need not always add in the front or at the back of the list. You may insert in a proper position. 54
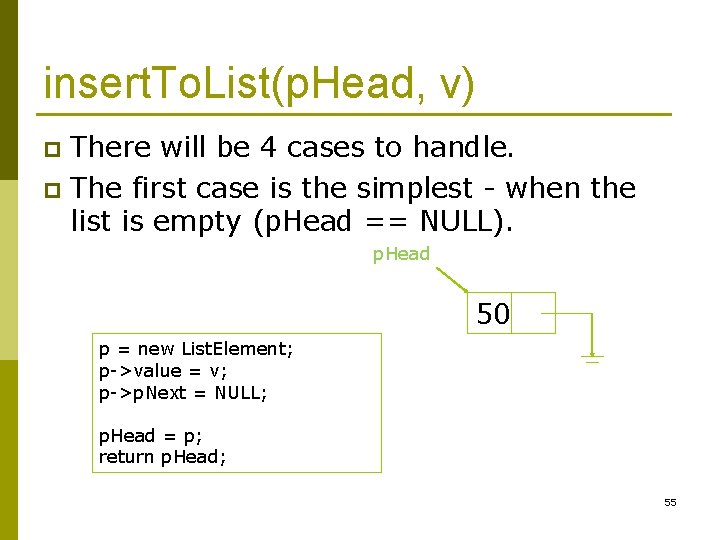
insert. To. List(p. Head, v) There will be 4 cases to handle. p The first case is the simplest - when the list is empty (p. Head == NULL). p p. Head 50 p = new List. Element; p->value = v; p->p. Next = NULL; p. Head = p; return p. Head; 55
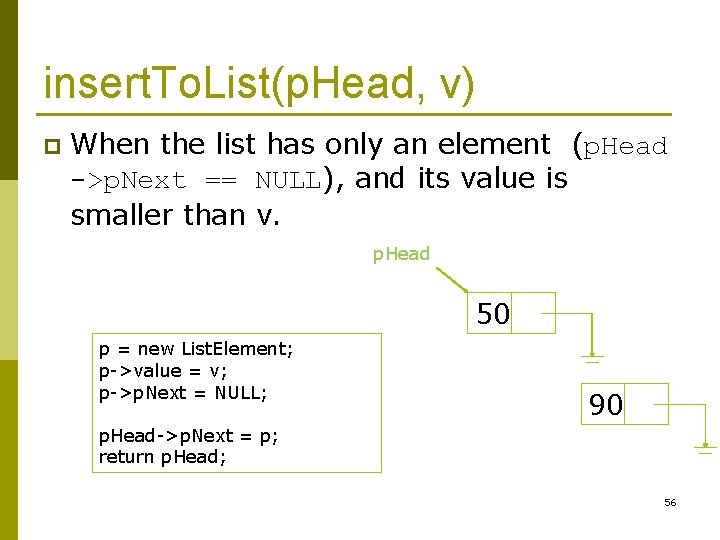
insert. To. List(p. Head, v) p When the list has only an element (p. Head ->p. Next == NULL), and its value is smaller than v. p. Head 50 p = new List. Element; p->value = v; p->p. Next = NULL; 90 p. Head->p. Next = p; return p. Head; 56
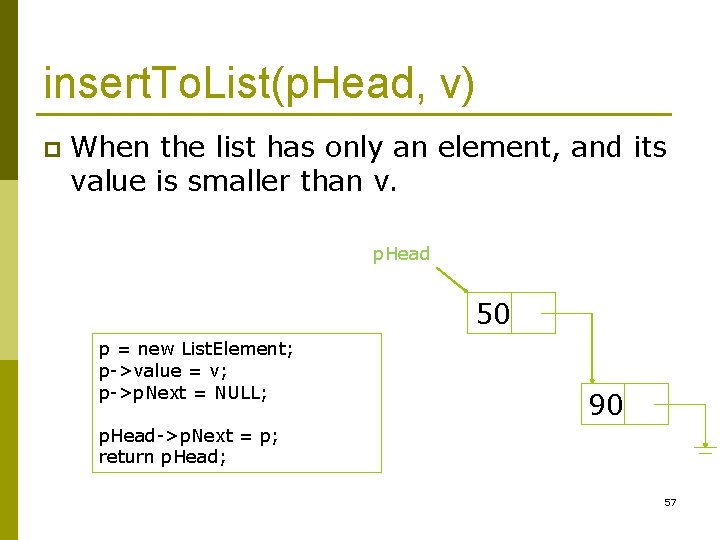
insert. To. List(p. Head, v) p When the list has only an element, and its value is smaller than v. p. Head 50 p = new List. Element; p->value = v; p->p. Next = NULL; 90 p. Head->p. Next = p; return p. Head; 57
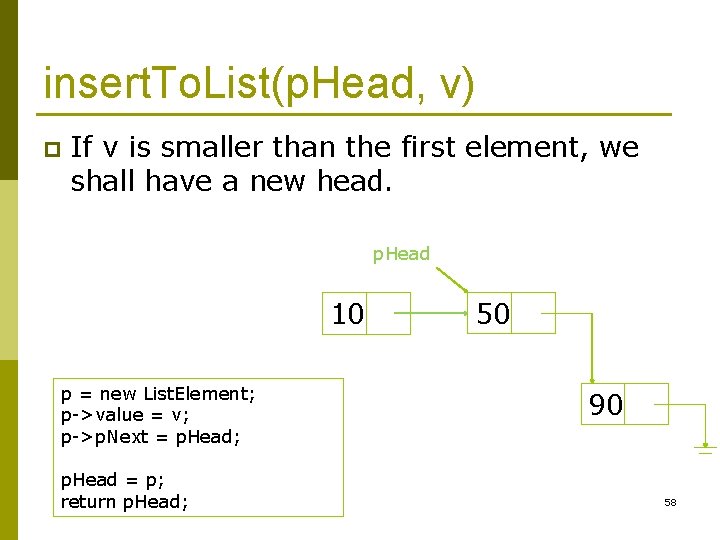
insert. To. List(p. Head, v) p If v is smaller than the first element, we shall have a new head. p. Head 10 p = new List. Element; p->value = v; p->p. Next = p. Head; p. Head = p; return p. Head; 50 90 58
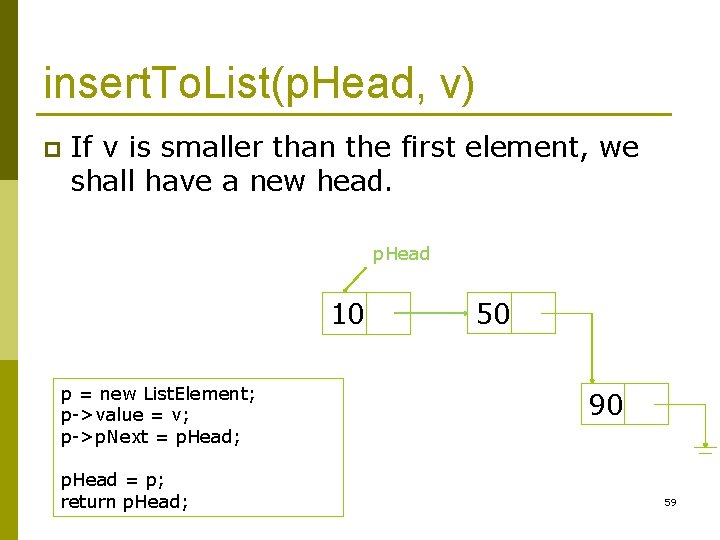
insert. To. List(p. Head, v) p If v is smaller than the first element, we shall have a new head. p. Head 10 p = new List. Element; p->value = v; p->p. Next = p. Head; p. Head = p; return p. Head; 50 90 59
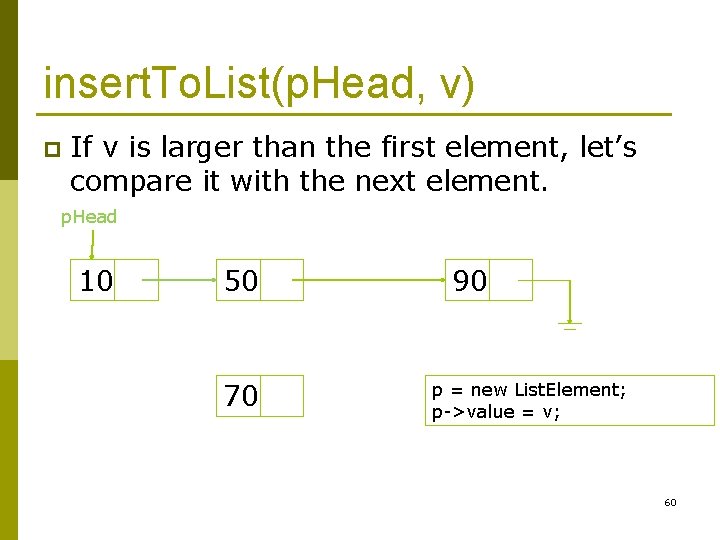
insert. To. List(p. Head, v) p If v is larger than the first element, let’s compare it with the next element. p. Head 10 50 70 90 p = new List. Element; p->value = v; 60
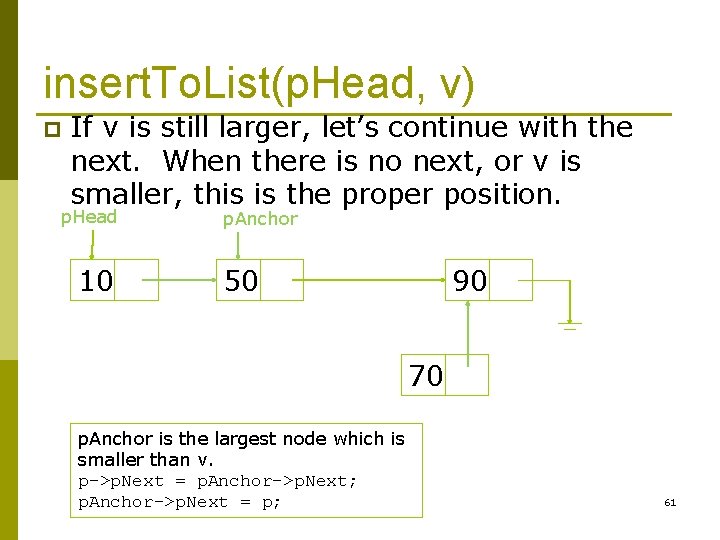
insert. To. List(p. Head, v) p If v is still larger, let’s continue with the next. When there is no next, or v is smaller, this is the proper position. p. Head 10 p. Anchor 50 90 70 p. Anchor is the largest node which is smaller than v. p->p. Next = p. Anchor->p. Next; p. Anchor->p. Next = p; 61
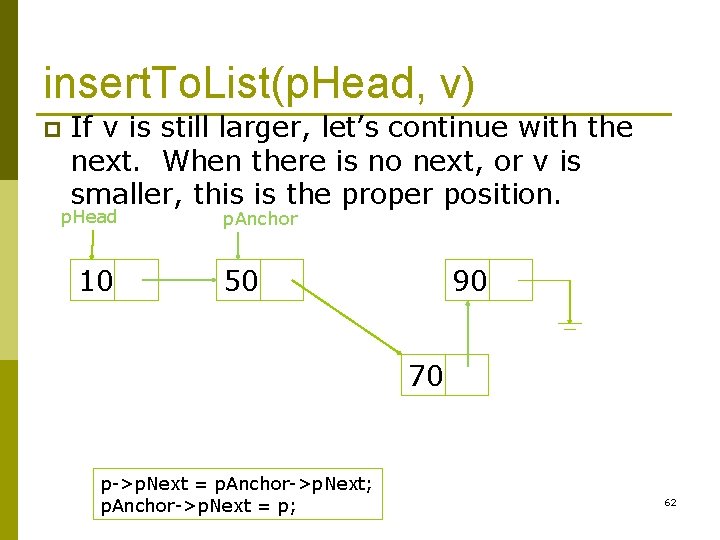
insert. To. List(p. Head, v) p If v is still larger, let’s continue with the next. When there is no next, or v is smaller, this is the proper position. p. Head 10 p. Anchor 50 90 70 p->p. Next = p. Anchor->p. Next; p. Anchor->p. Next = p; 62
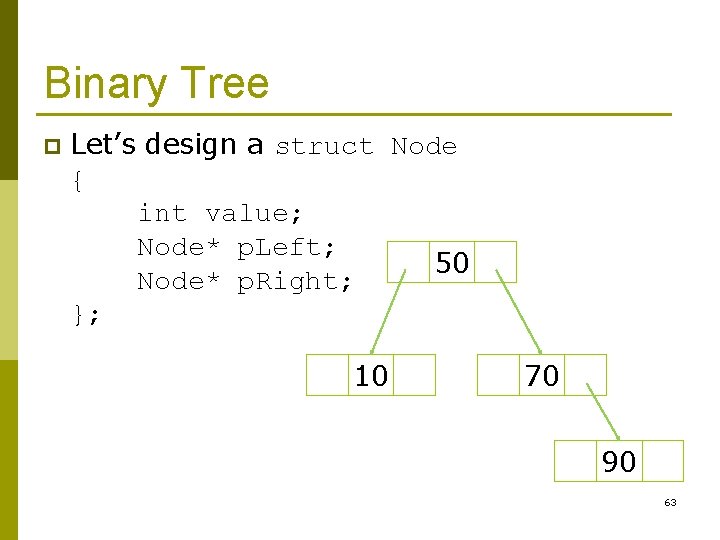
Binary Tree p Let’s design a struct Node { int value; Node* p. Left; 50 Node* p. Right; }; 10 70 90 63
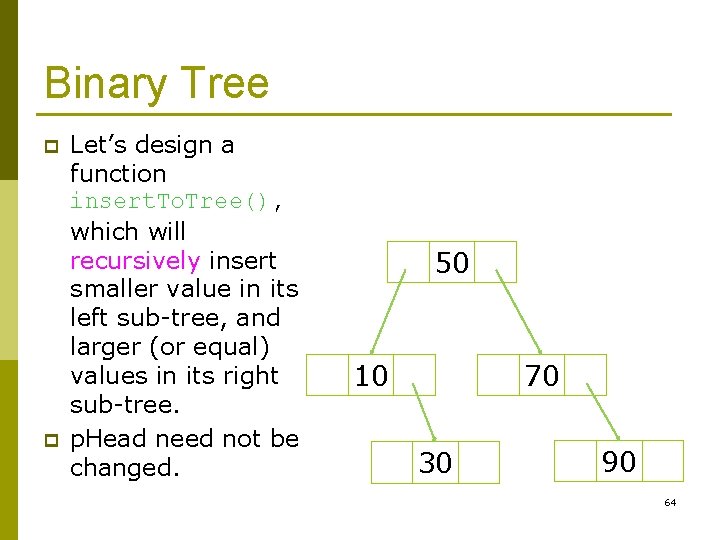
Binary Tree p p Let’s design a function insert. To. Tree(), which will recursively insert smaller value in its left sub-tree, and larger (or equal) values in its right sub-tree. p. Head need not be changed. 50 10 70 30 90 64
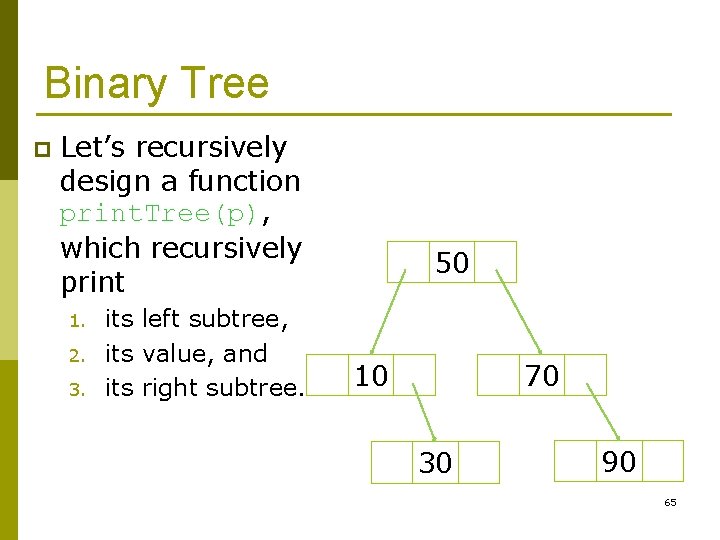
Binary Tree p Let’s recursively design a function print. Tree(p), which recursively print 1. 2. 3. its left subtree, its value, and its right subtree. 50 10 70 30 90 65
His birth date was on 25 january 1759
Types of dividend policy
Homemade dividend
Gamsat exam date
Latin 1 final exam
Entrepreneurship 1 final exam review
Pols 1101 final exam study guide
Hbs final exam practice test
Operating system final exam
English 10 b final exam
Romeo and juliet exam review
Visual basic final exam
Stoichiometry exam
Pointing
Ap world history final exam
Speech final exam
Civics and economics final exam
Heat transfer final exam
World history fall final review answers
English semester 2 final exam
Enviromental science final exam
Wipo dl 101
Ied final exam
Semester 1 final exam study guide us history
Business communication final exam
Computer vision final exam
Drc uic
Chem 130 final exam
Physics semester 1 final exam study guide answers
Final exam instructions
Physics 101 final exam
Final exam review algebra 1
Amdm final exam answers
Earth science final exam answers
Street law final exam
Enc 1101 final exam
Of mice and men final exam
Mat1033c
World history spring final exam review answers
Operating systems final exam
Psyc 1504 final exam
Software engineering final exam
Salute report example
Human body systems final exam
The crucible final test review
Ap gov review final exam review
Personal finance final exam review
Gold coast exam
Earth science final
Simile in writing
Social 10-1 final exam
Human resource management questions for exams
Sbu finals schedule
World history semester 2 exam review
Usf final exam matrix