File class File my Filenew Filec java Demoaa
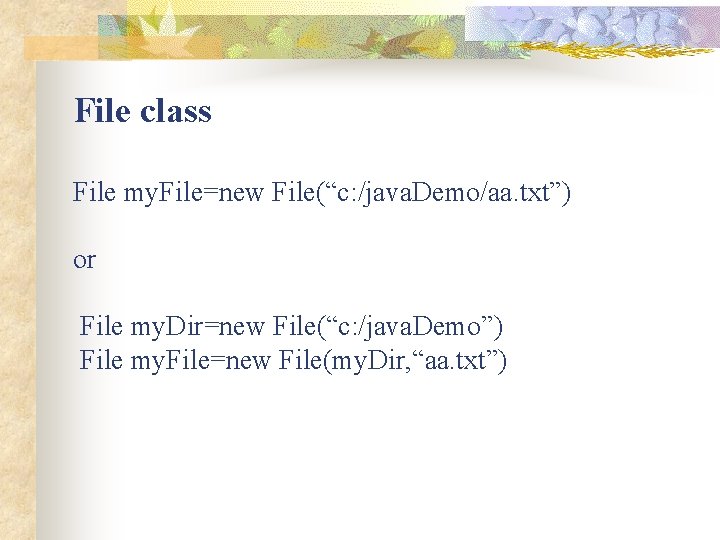
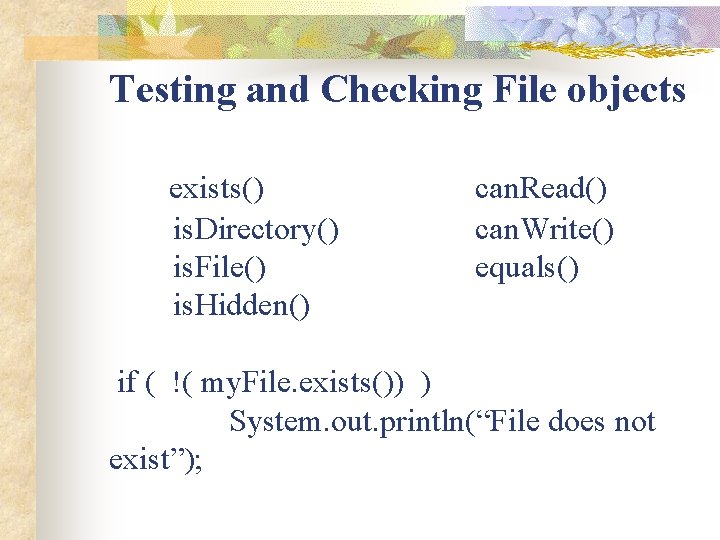
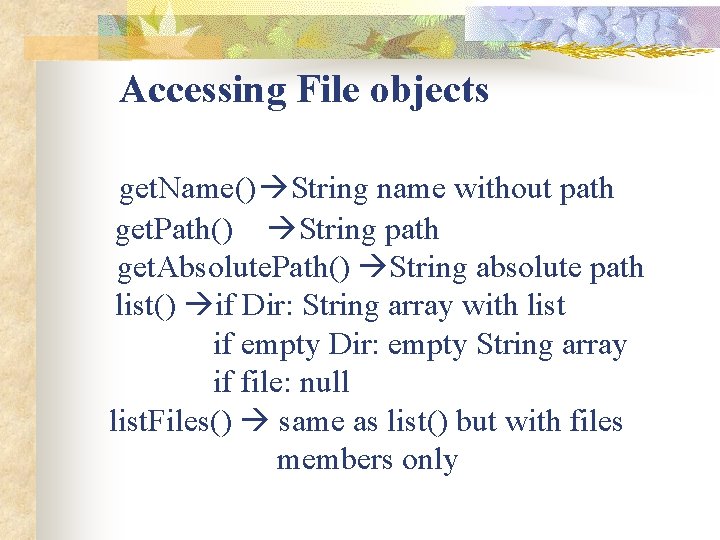
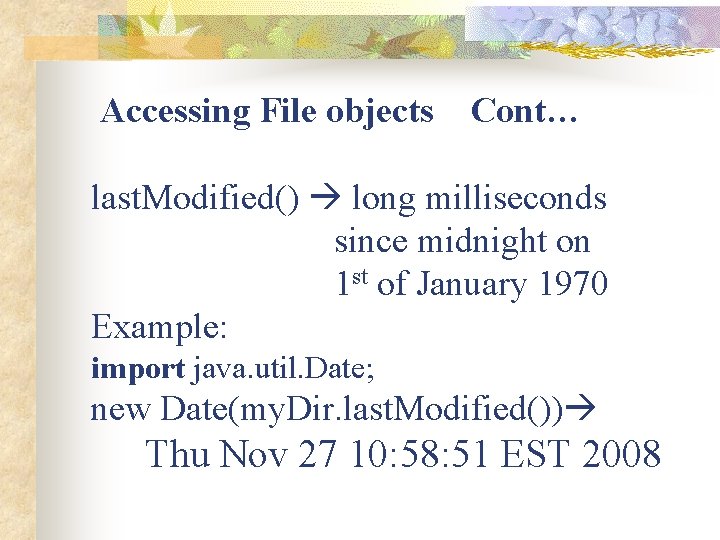
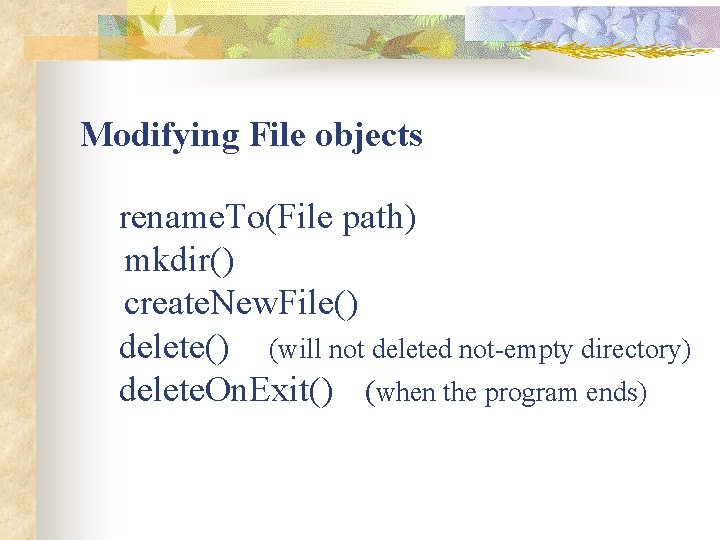
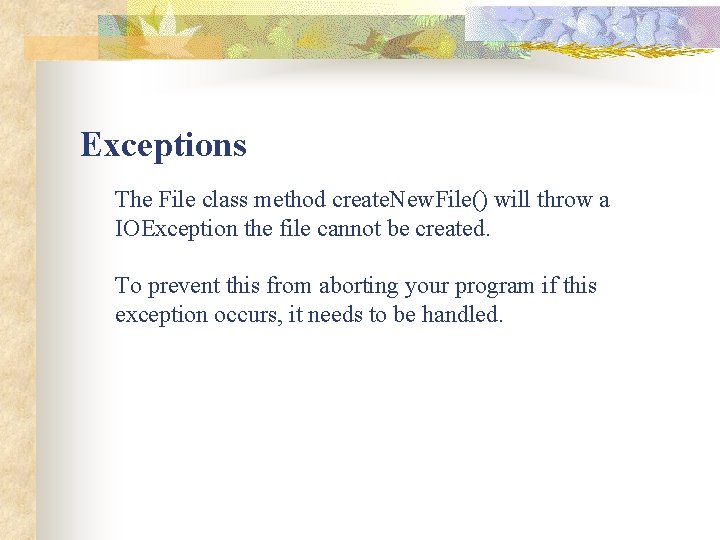
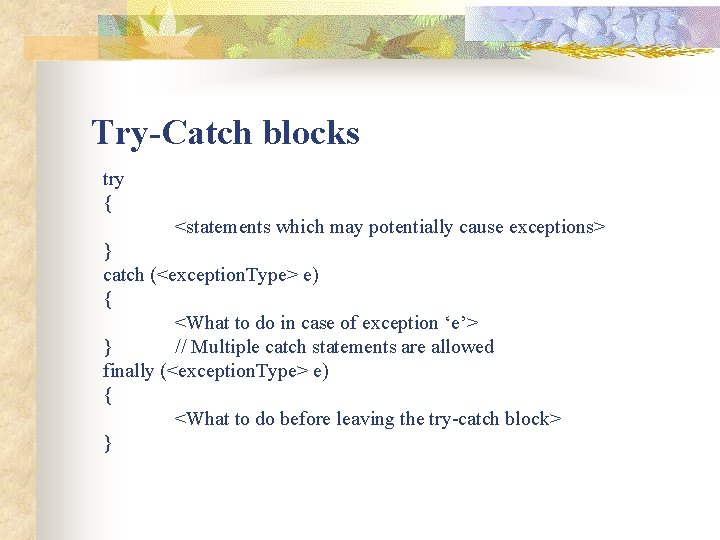
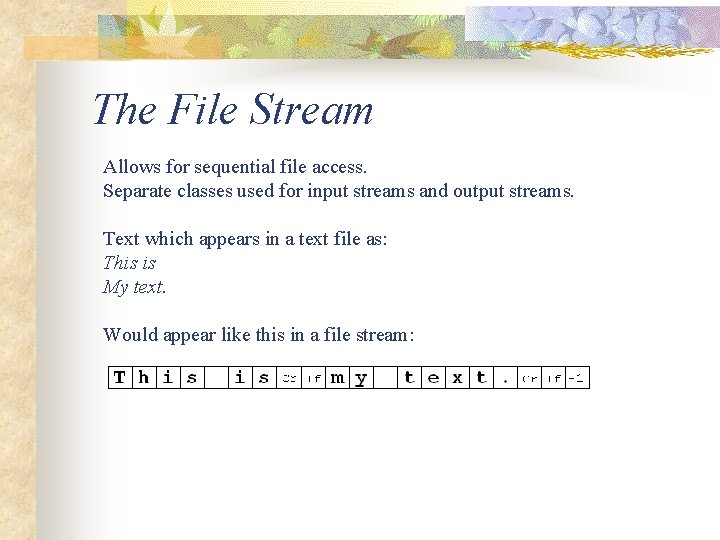
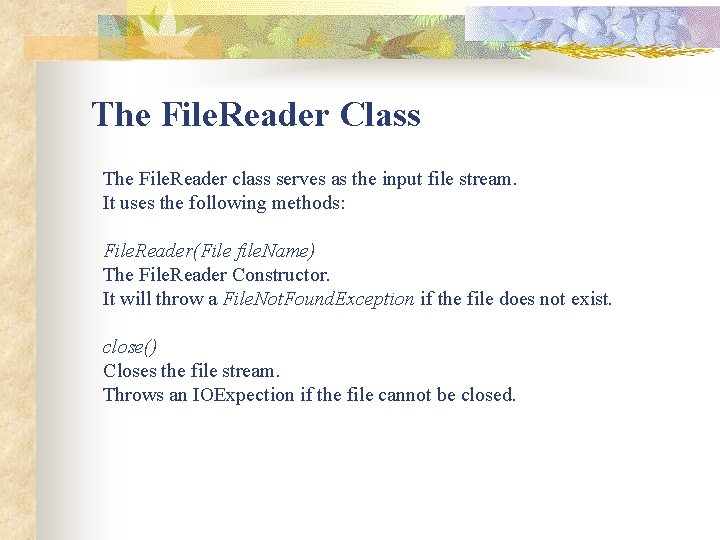
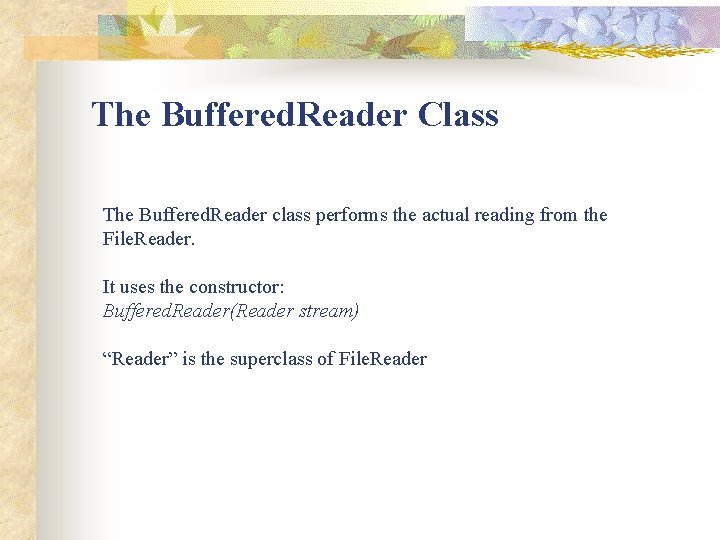
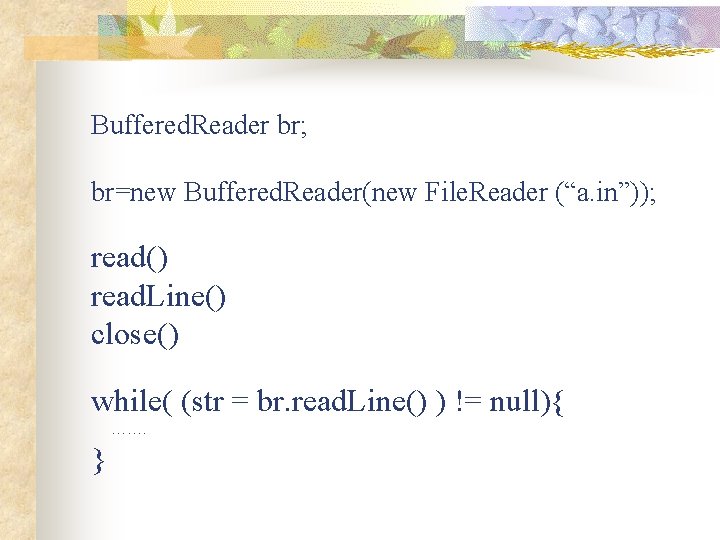
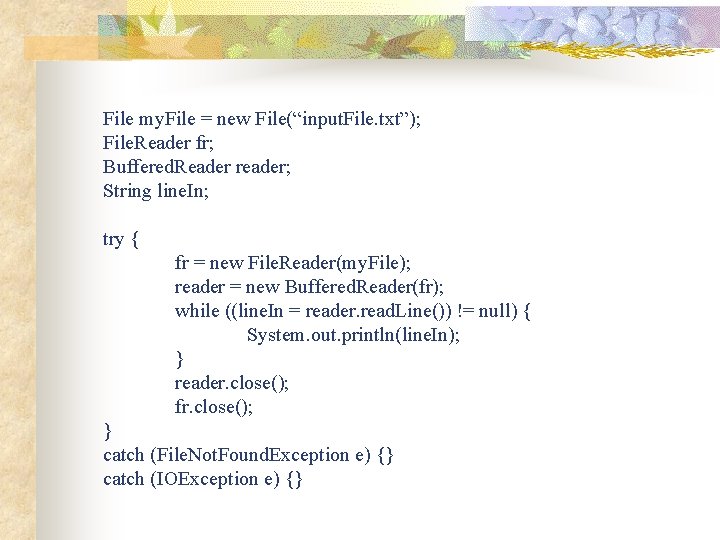
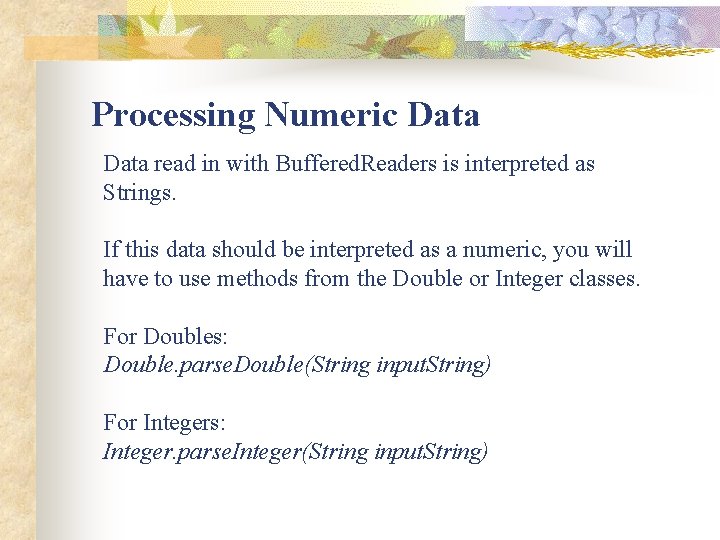
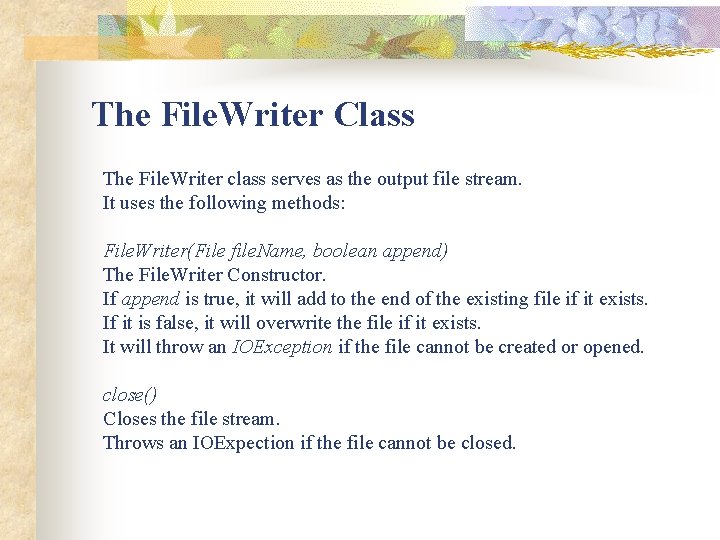
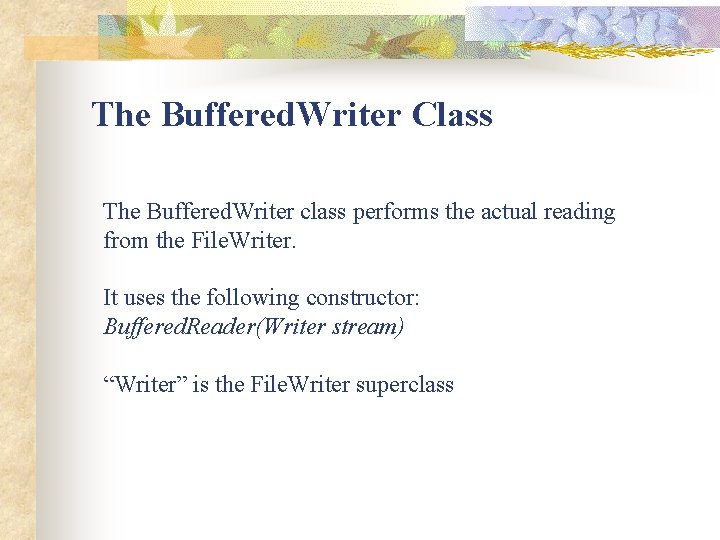
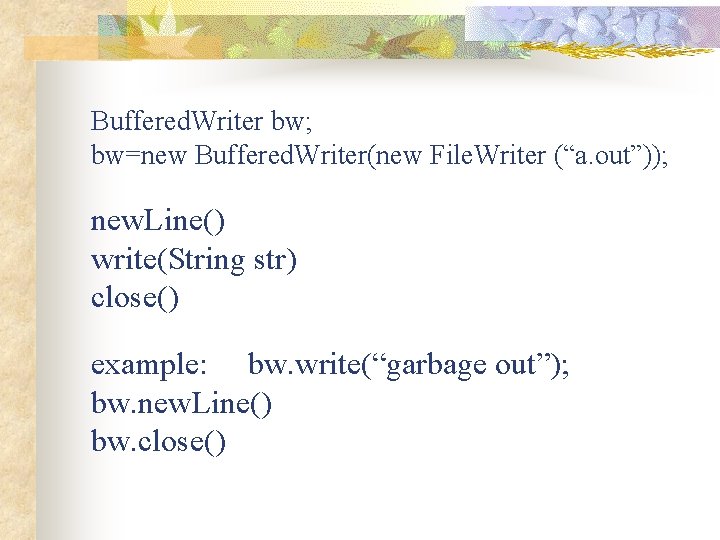
- Slides: 16
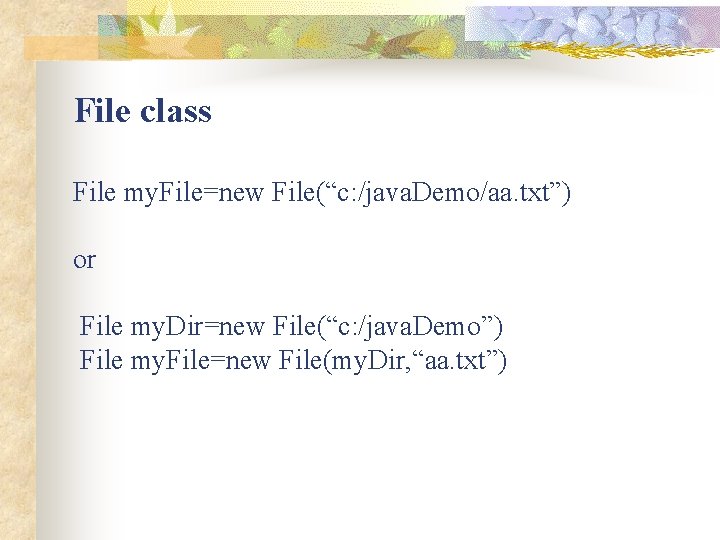
File class File my. File=new File(“c: /java. Demo/aa. txt”) or File my. Dir=new File(“c: /java. Demo”) File my. File=new File(my. Dir, “aa. txt”)
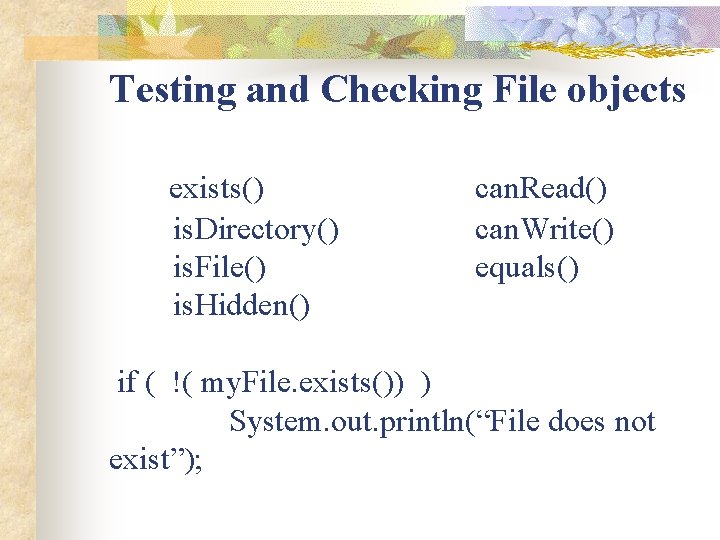
Testing and Checking File objects exists() is. Directory() is. File() is. Hidden() can. Read() can. Write() equals() if ( !( my. File. exists()) ) System. out. println(“File does not exist”);
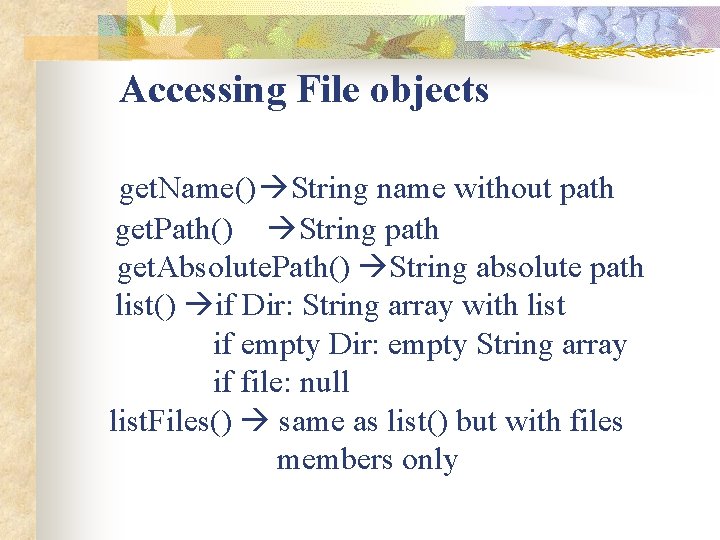
Accessing File objects get. Name() String name without path get. Path() String path get. Absolute. Path() String absolute path list() if Dir: String array with list if empty Dir: empty String array if file: null list. Files() same as list() but with files members only
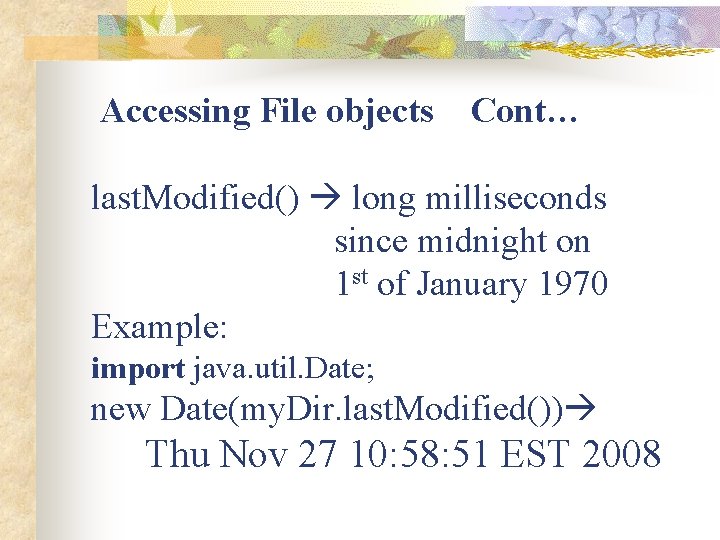
Accessing File objects Cont… last. Modified() long milliseconds since midnight on 1 st of January 1970 Example: import java. util. Date; new Date(my. Dir. last. Modified()) Thu Nov 27 10: 58: 51 EST 2008
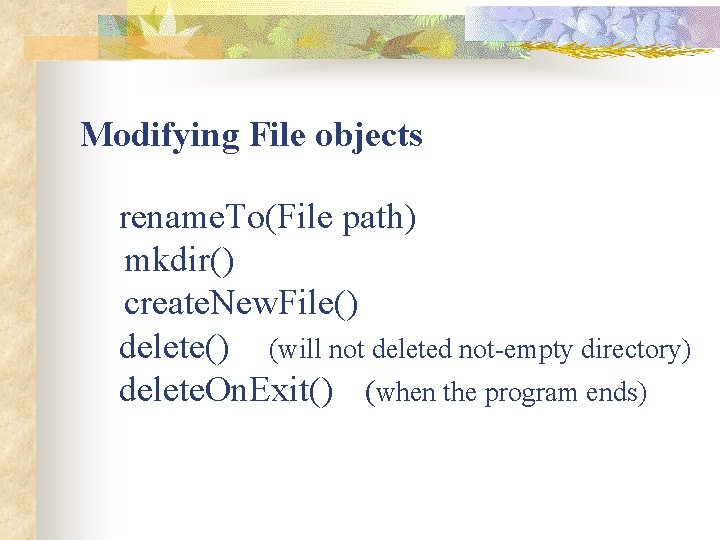
Modifying File objects rename. To(File path) mkdir() create. New. File() delete() (will not deleted not-empty directory) delete. On. Exit() (when the program ends)
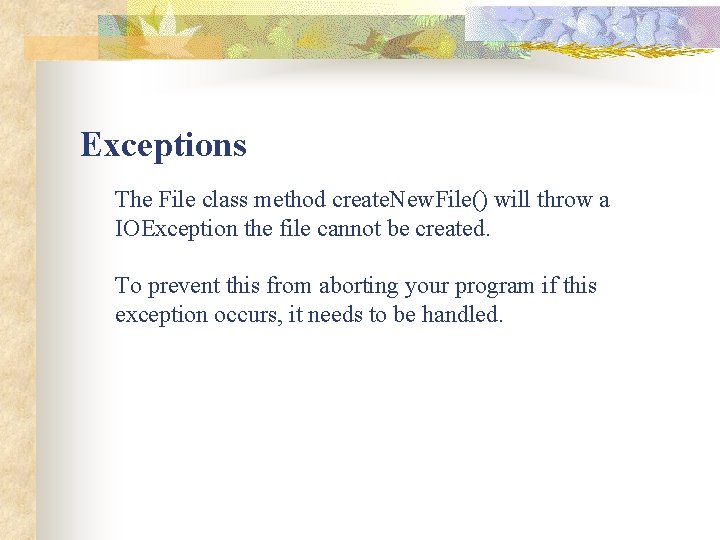
Exceptions The File class method create. New. File() will throw a IOException the file cannot be created. To prevent this from aborting your program if this exception occurs, it needs to be handled.
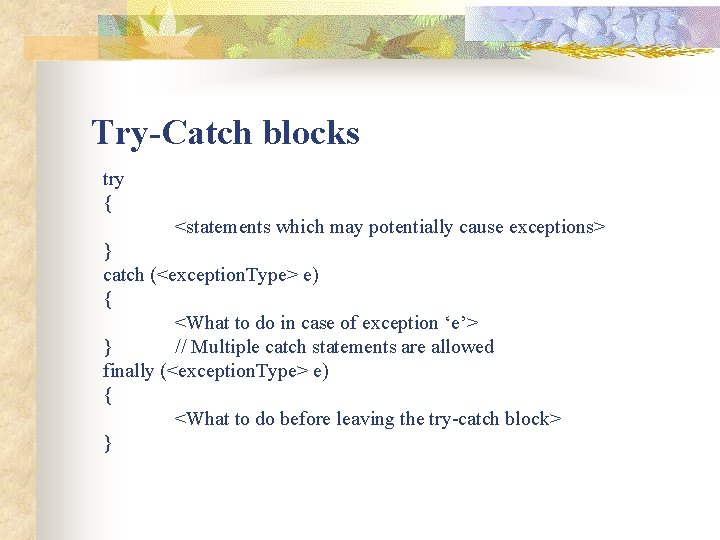
Try-Catch blocks try { <statements which may potentially cause exceptions> } catch (<exception. Type> e) { <What to do in case of exception ‘e’> } // Multiple catch statements are allowed finally (<exception. Type> e) { <What to do before leaving the try-catch block> }
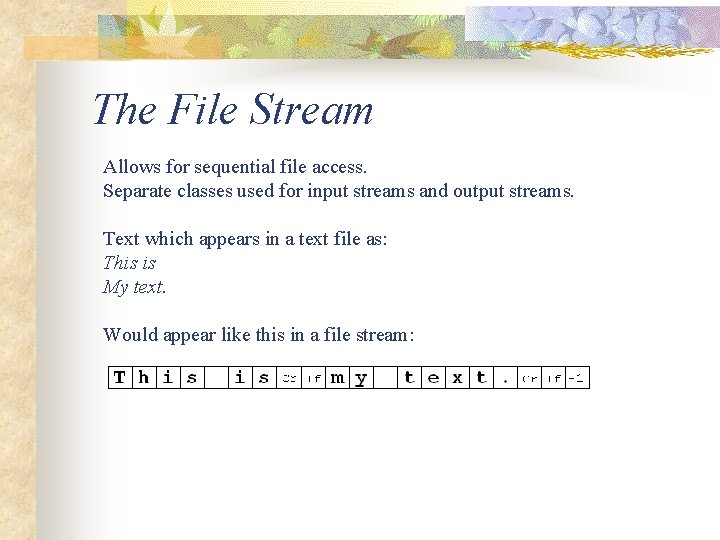
The File Stream Allows for sequential file access. Separate classes used for input streams and output streams. Text which appears in a text file as: This is My text. Would appear like this in a file stream:
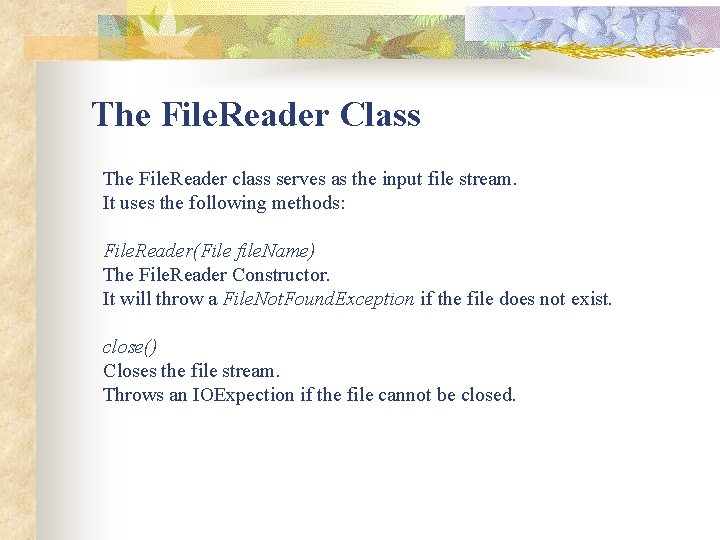
The File. Reader Class The File. Reader class serves as the input file stream. It uses the following methods: File. Reader(File file. Name) The File. Reader Constructor. It will throw a File. Not. Found. Exception if the file does not exist. close() Closes the file stream. Throws an IOExpection if the file cannot be closed.
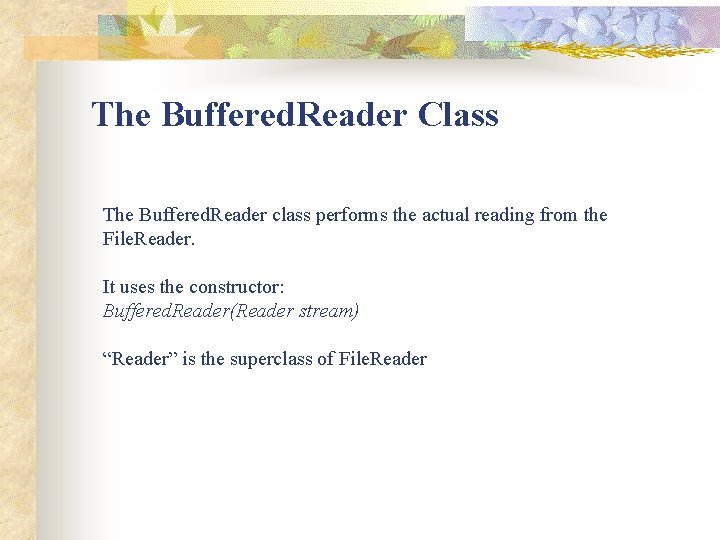
The Buffered. Reader Class The Buffered. Reader class performs the actual reading from the File. Reader. It uses the constructor: Buffered. Reader(Reader stream) “Reader” is the superclass of File. Reader
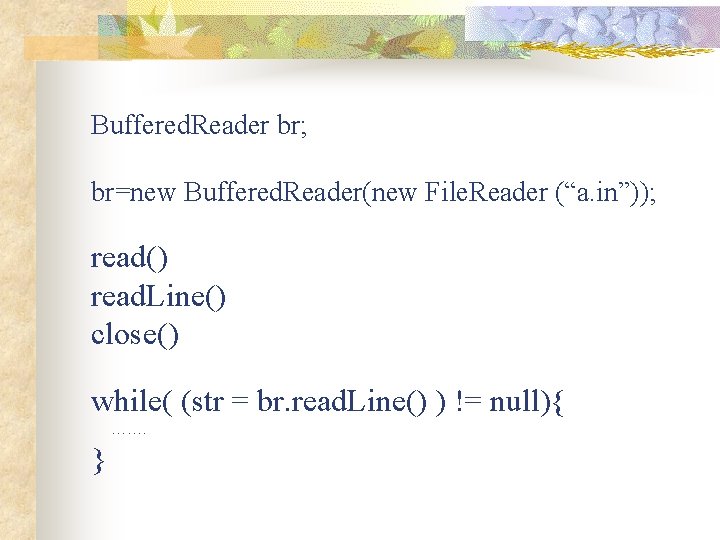
Buffered. Reader br; br=new Buffered. Reader(new File. Reader (“a. in”)); read() read. Line() close() while( (str = br. read. Line() ) != null){ ……. }
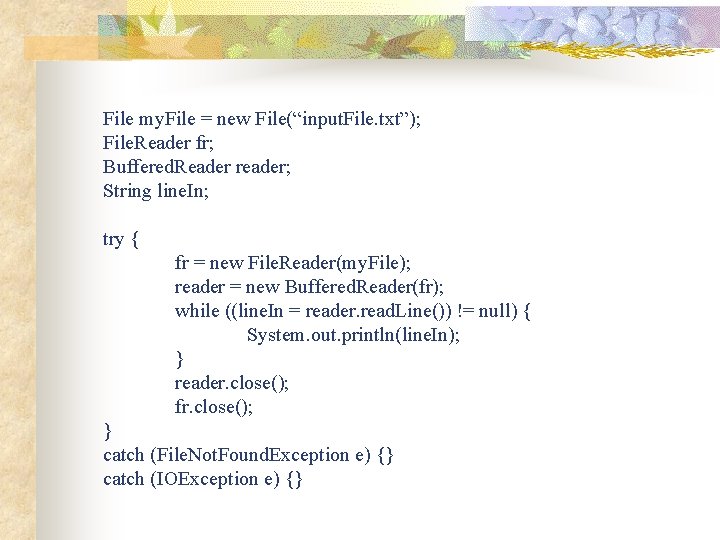
File my. File = new File(“input. File. txt”); File. Reader fr; Buffered. Reader reader; String line. In; try { fr = new File. Reader(my. File); reader = new Buffered. Reader(fr); while ((line. In = reader. read. Line()) != null) { System. out. println(line. In); } reader. close(); fr. close(); } catch (File. Not. Found. Exception e) {} catch (IOException e) {}
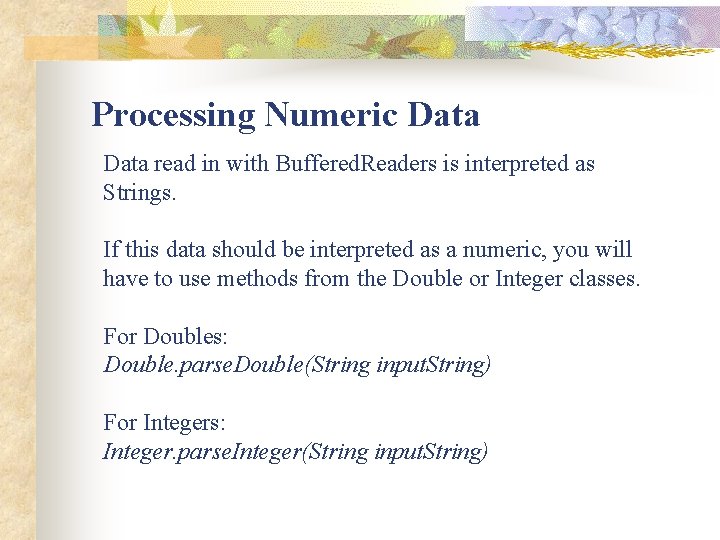
Processing Numeric Data read in with Buffered. Readers is interpreted as Strings. If this data should be interpreted as a numeric, you will have to use methods from the Double or Integer classes. For Doubles: Double. parse. Double(String input. String) For Integers: Integer. parse. Integer(String input. String)
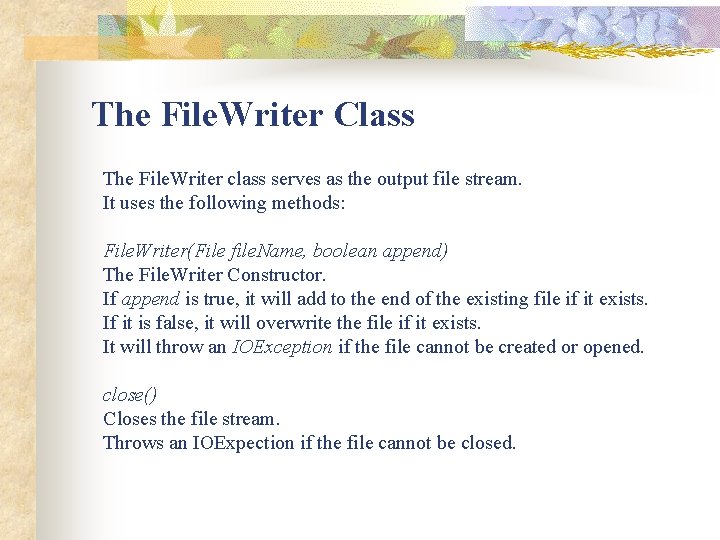
The File. Writer Class The File. Writer class serves as the output file stream. It uses the following methods: File. Writer(File file. Name, boolean append) The File. Writer Constructor. If append is true, it will add to the end of the existing file if it exists. If it is false, it will overwrite the file if it exists. It will throw an IOException if the file cannot be created or opened. close() Closes the file stream. Throws an IOExpection if the file cannot be closed.
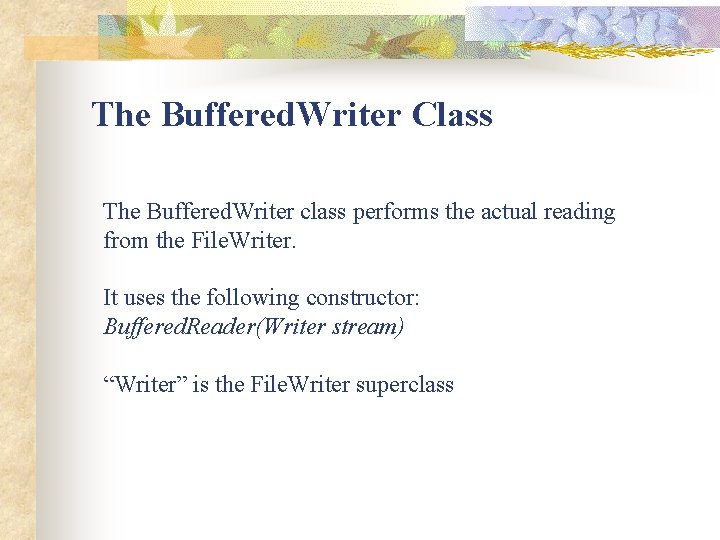
The Buffered. Writer Class The Buffered. Writer class performs the actual reading from the File. Writer. It uses the following constructor: Buffered. Reader(Writer stream) “Writer” is the File. Writer superclass
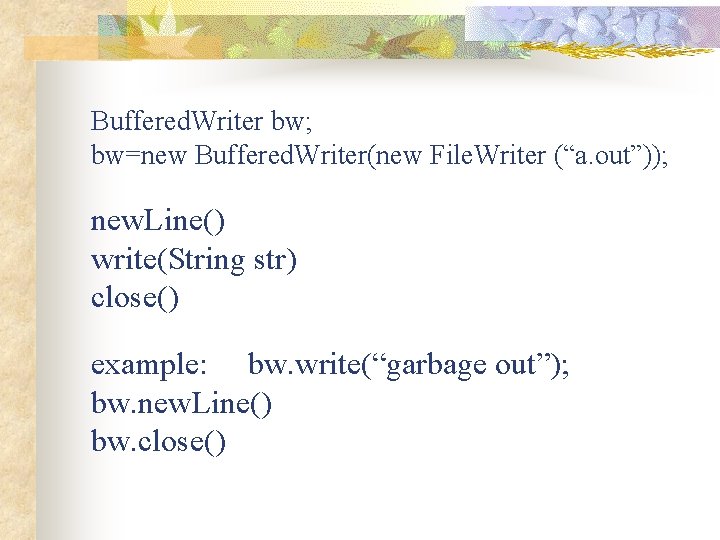
Buffered. Writer bw; bw=new Buffered. Writer(new File. Writer (“a. out”)); new. Line() write(String str) close() example: bw. write(“garbage out”); bw. new. Line() bw. close()