Exercise 2 Using for loop Repetition loop n
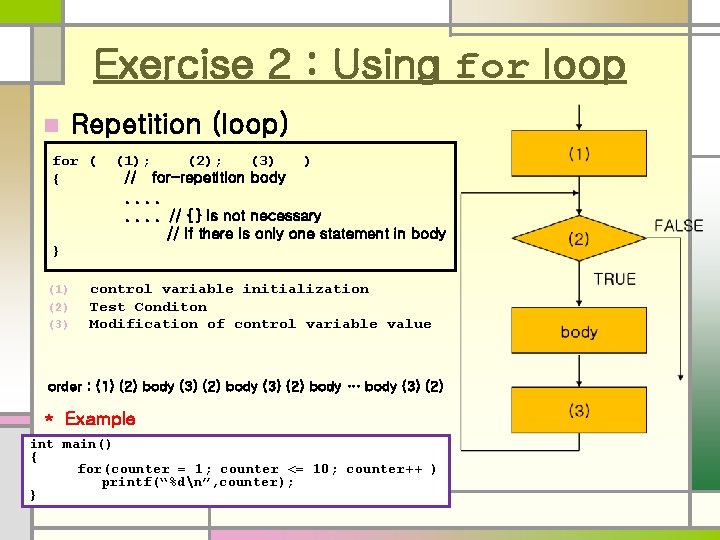
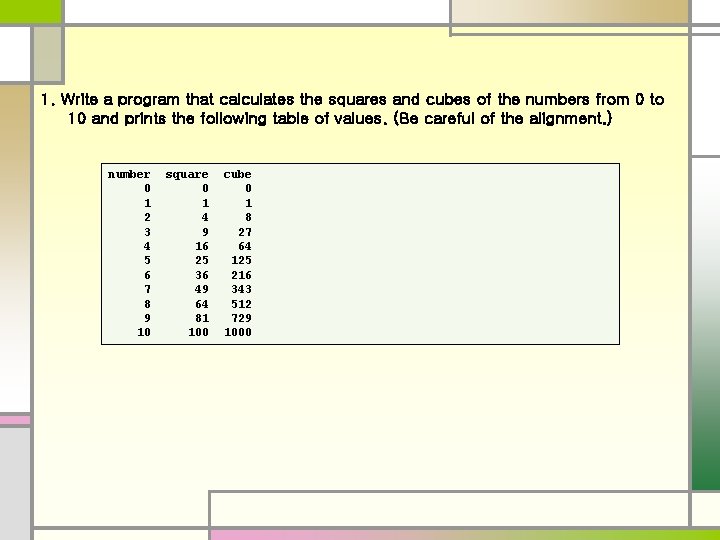
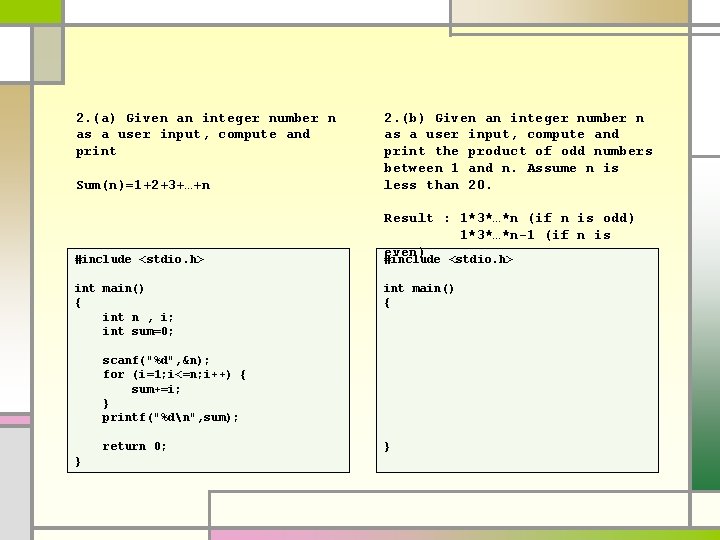
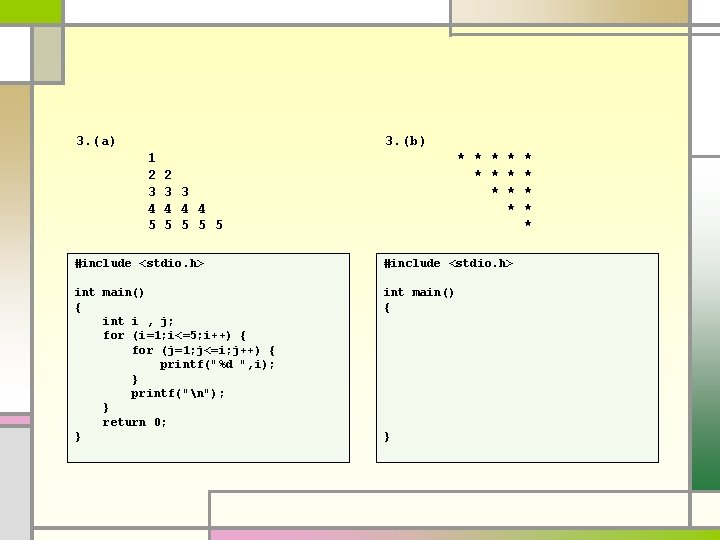
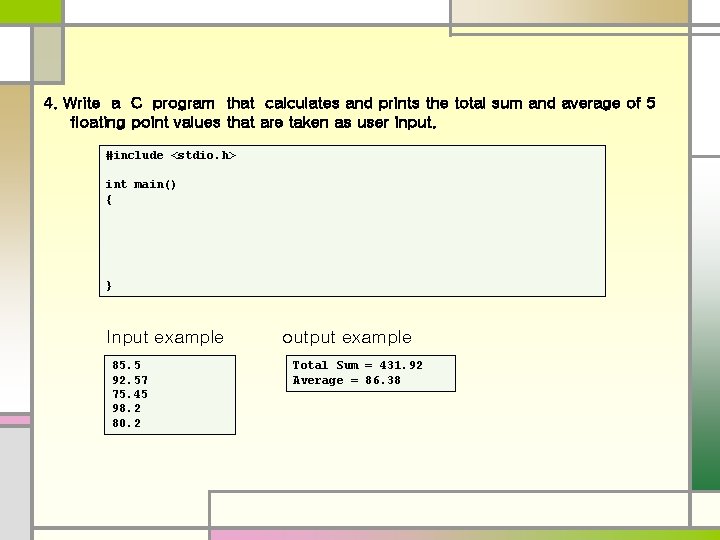
- Slides: 5
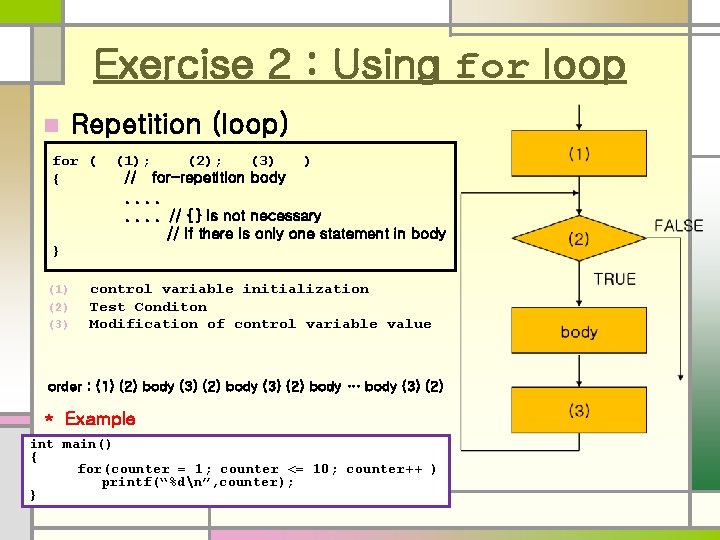
Exercise 2 : Using for loop Repetition (loop) n for ( { (1); (2); (3) ) // for-repetition body. . . . // {} is not necessary // if there is only one statement in body } (1) (2) (3) control variable initialization Test Conditon Modification of control variable value order : (1) (2) body (3) (2) body … body (3) (2) * Example int main() { for(counter = 1; counter <= 10; counter++ ) printf(“%dn”, counter); }
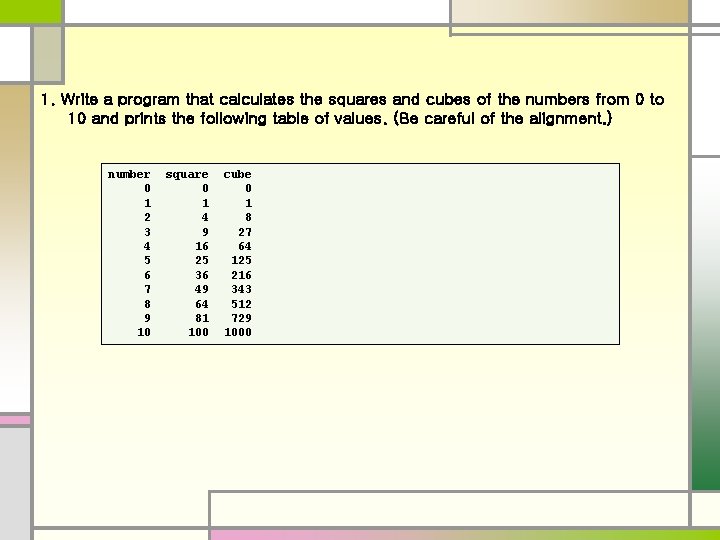
1. Write a program that calculates the squares and cubes of the numbers from 0 to 10 and prints the following table of values. (Be careful of the alignment. ) number 0 1 2 3 4 5 6 7 8 9 10 square 0 1 4 9 16 25 36 49 64 81 100 cube 0 1 8 27 64 125 216 343 512 729 1000
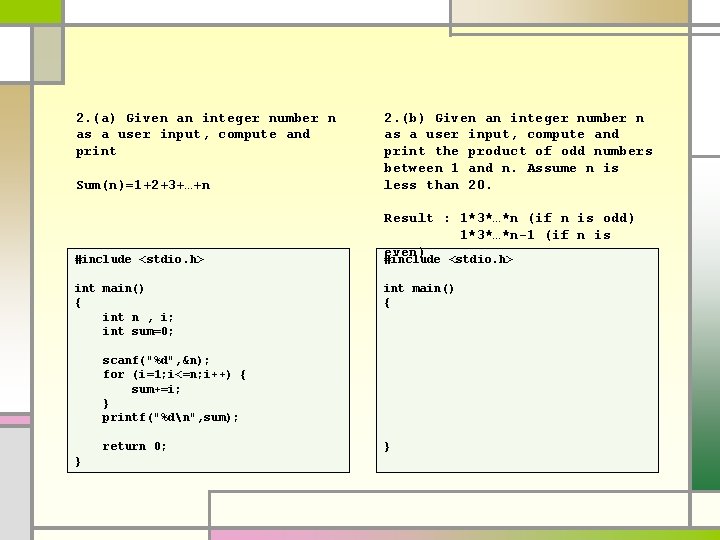
2. (a) Given an integer number n as a user input, compute and print Sum(n)=1+2+3+…+n 2. (b) Given an integer number n as a user input, compute and print the product of odd numbers between 1 and n. Assume n is less than 20. #include <stdio. h> Result : 1*3*…*n (if n is odd) 1*3*…*n-1 (if n is even) #include <stdio. h> int main() { int n , i; int sum=0; int main() { scanf("%d", &n); for (i=1; i<=n; i++) { sum+=i; } printf("%dn", sum); return 0; } }
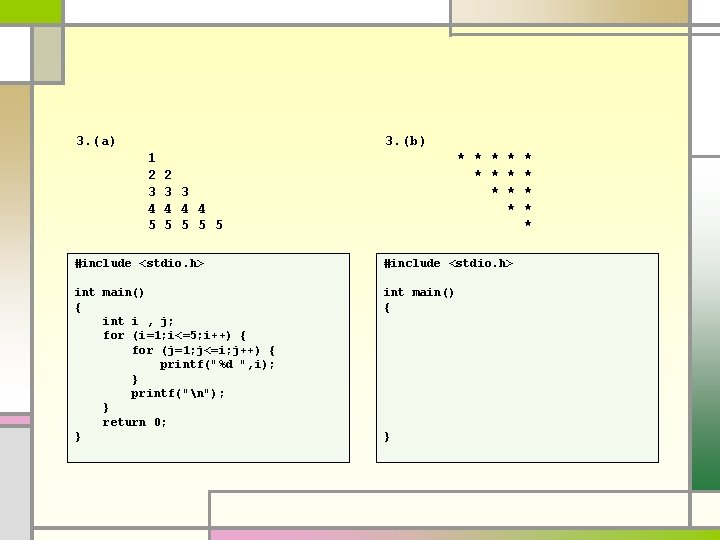
3. (a) 3. (b) 1 2 3 4 5 * * * * * 2 3 3 4 4 4 5 5 #include <stdio. h> int main() { int i , j; for (i=1; i<=5; i++) { for (j=1; j<=i; j++) { printf("%d ", i); } printf("n"); } return 0; } int main() { } * * *
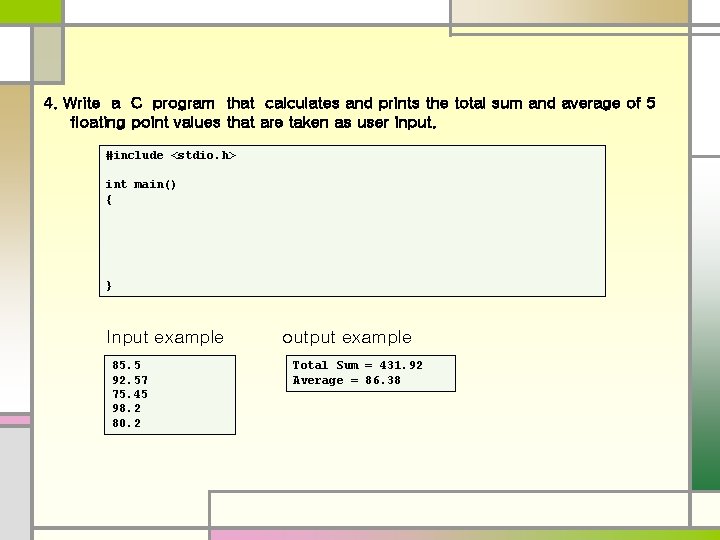
4. Write a C program that calculates and prints the total sum and average of 5 floating point values that are taken as user input. #include <stdio. h> int main() { } Input example 85. 5 92. 57 75. 45 98. 2 80. 2 output example Total Sum = 431. 92 Average = 86. 38