Exception Handling Errors During the Program Execution Svetlin
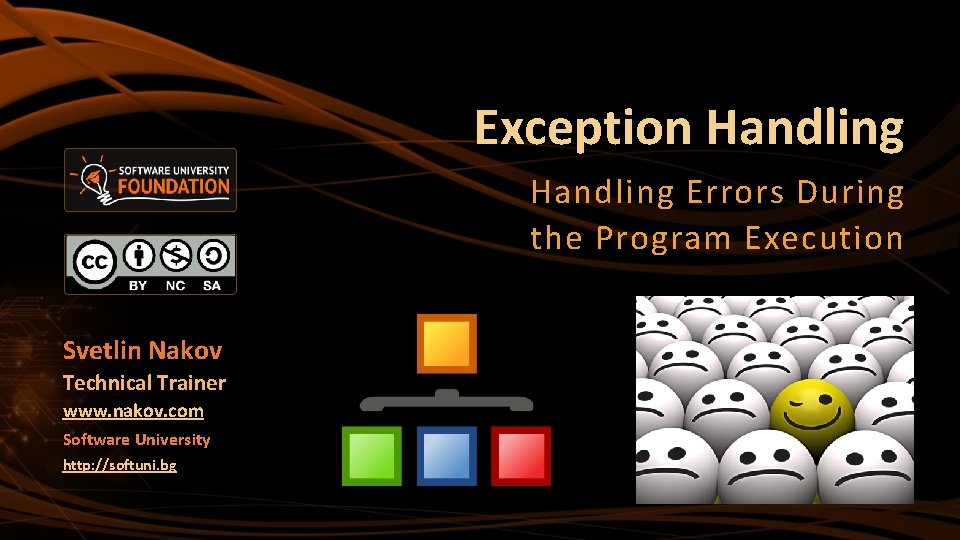
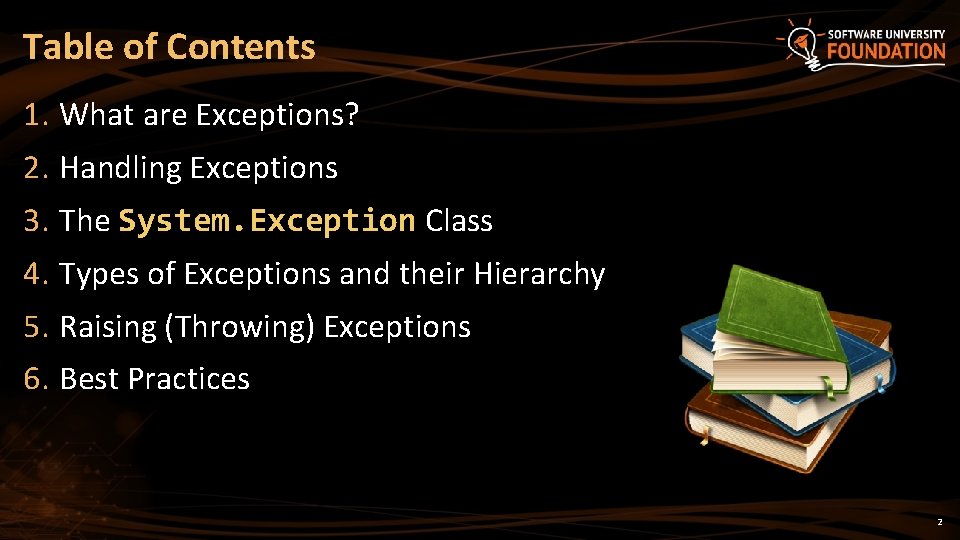
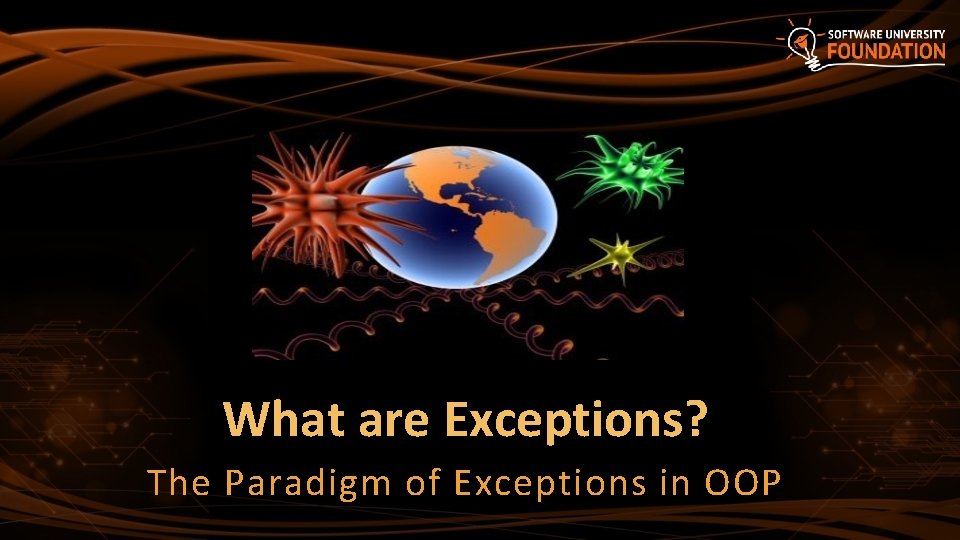
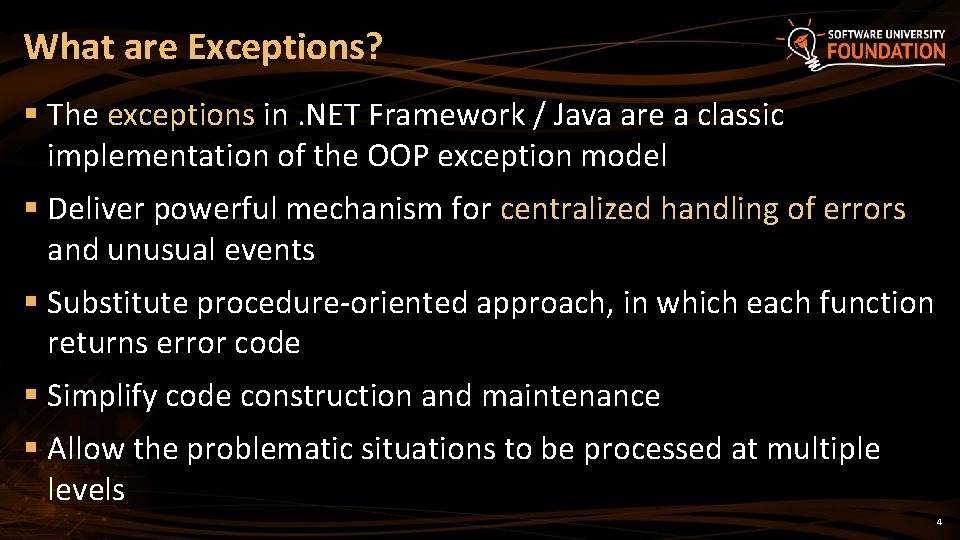
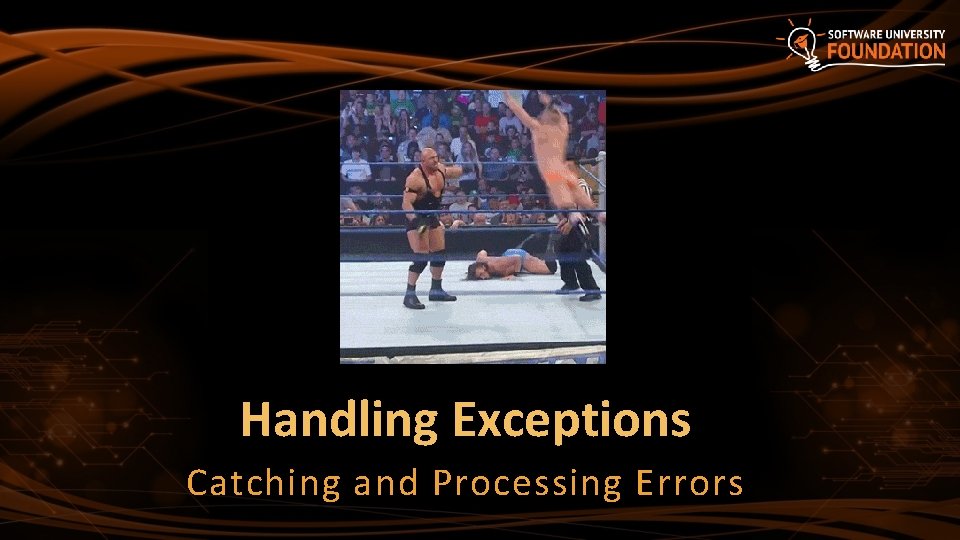
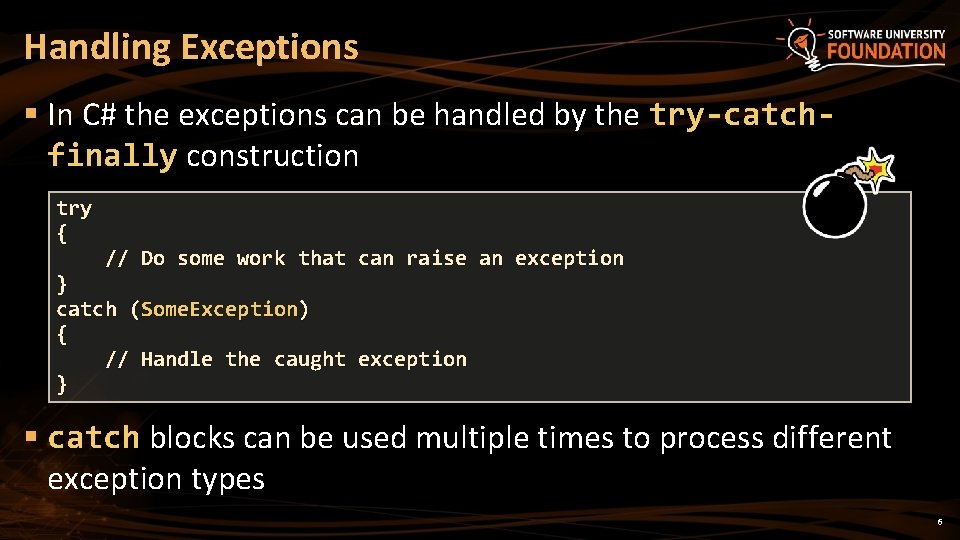
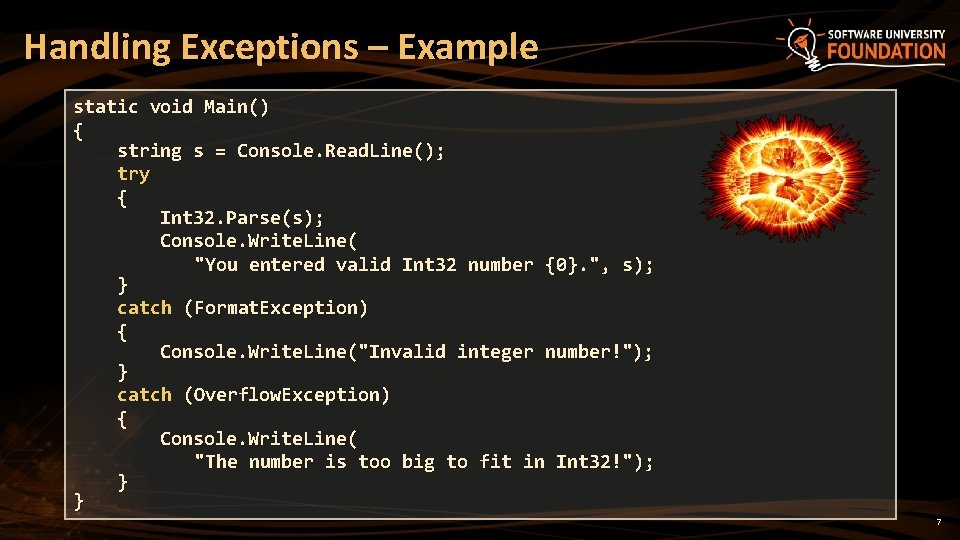
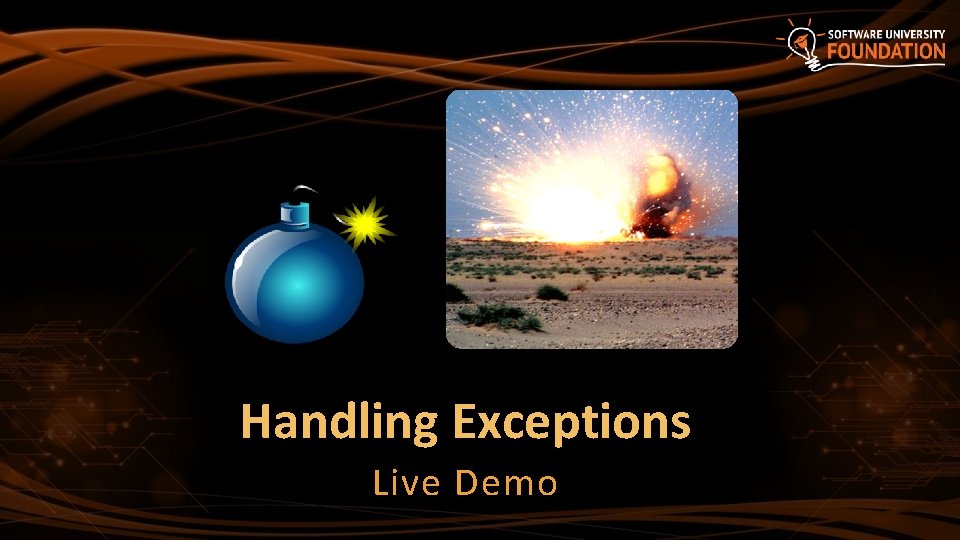
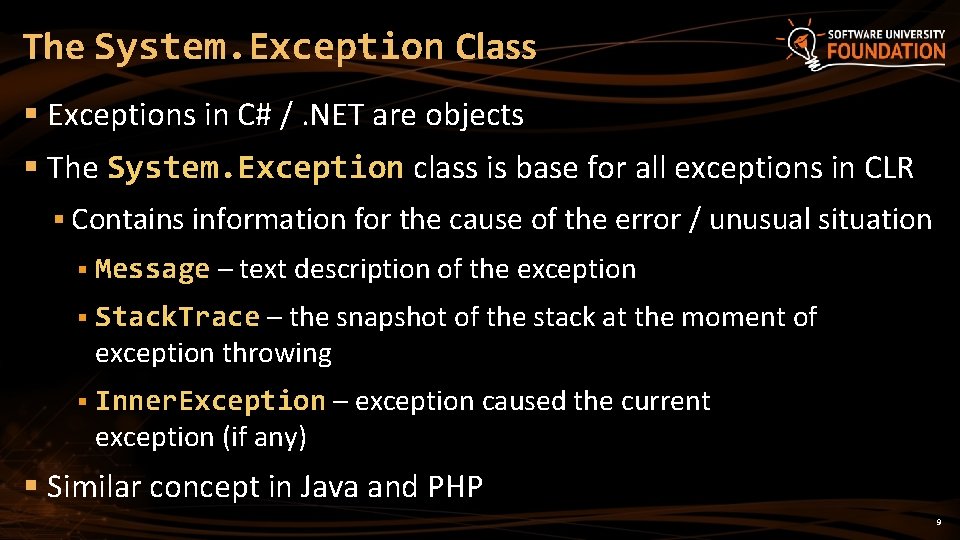
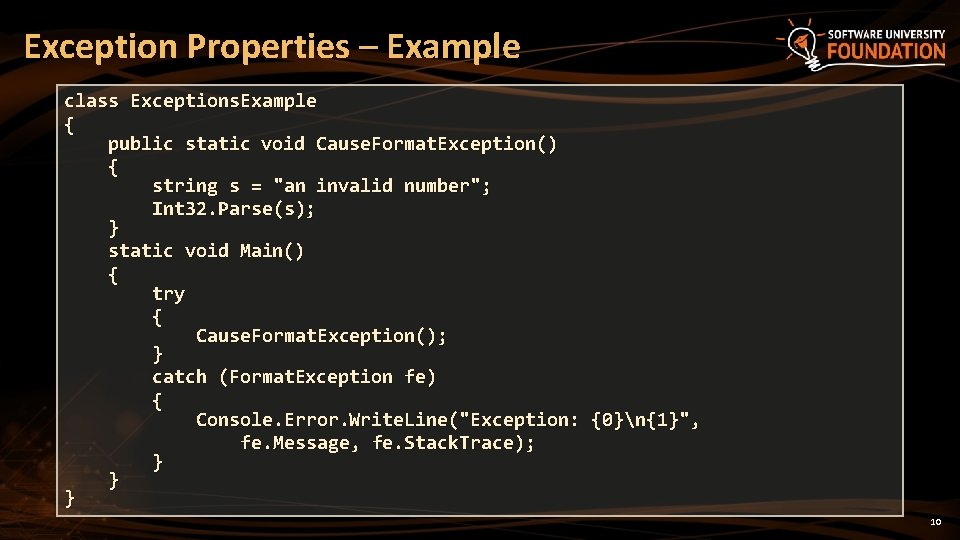
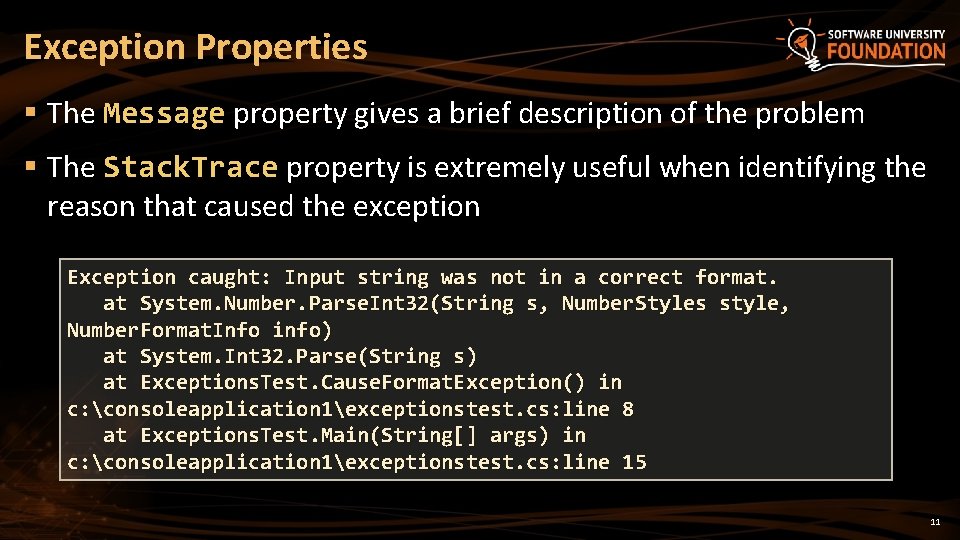
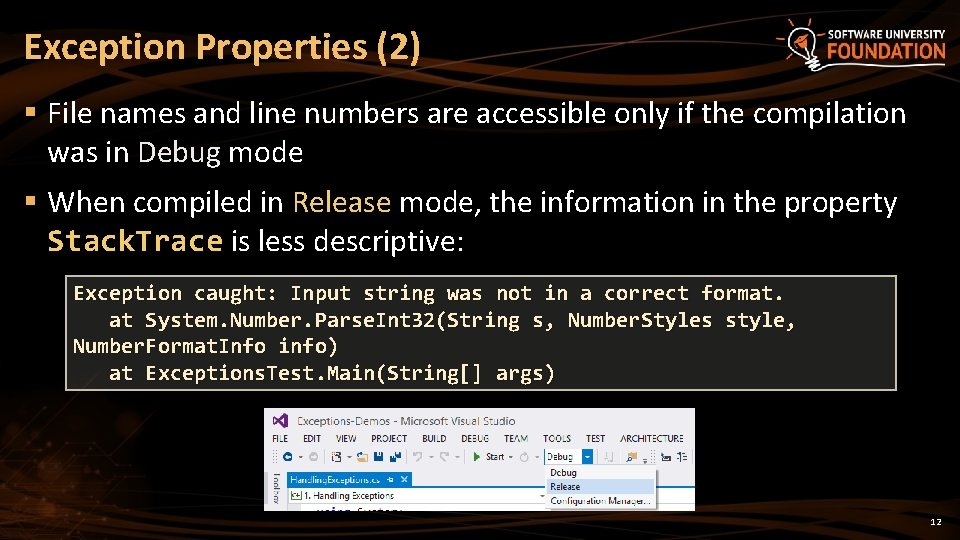
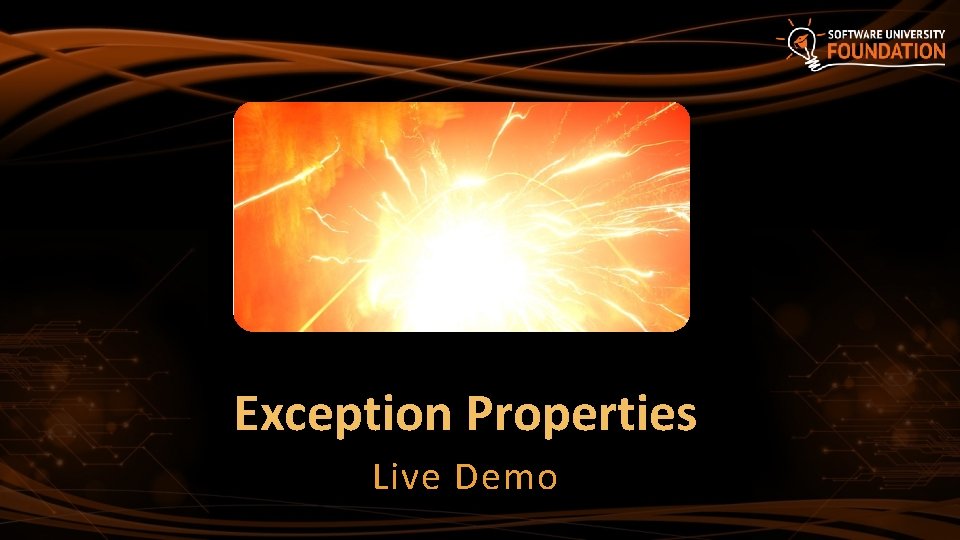
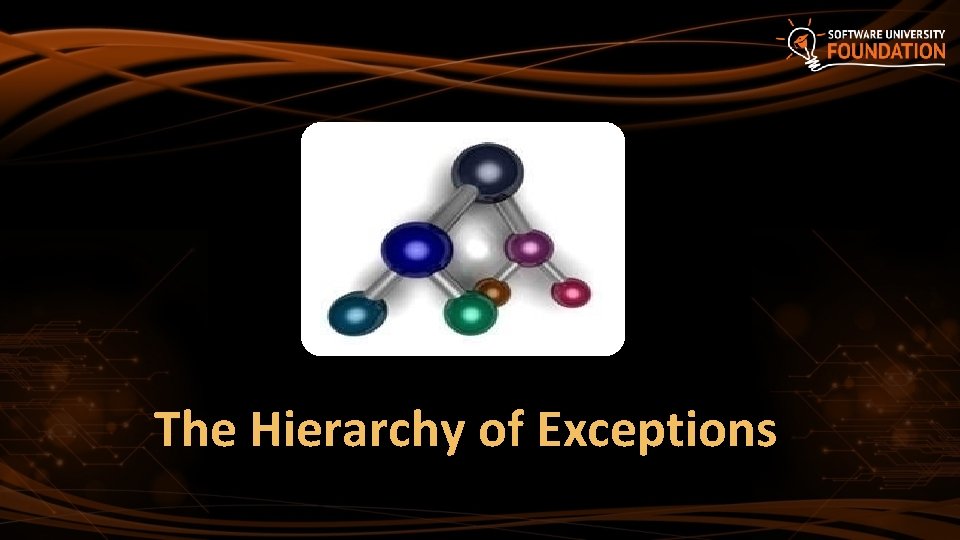
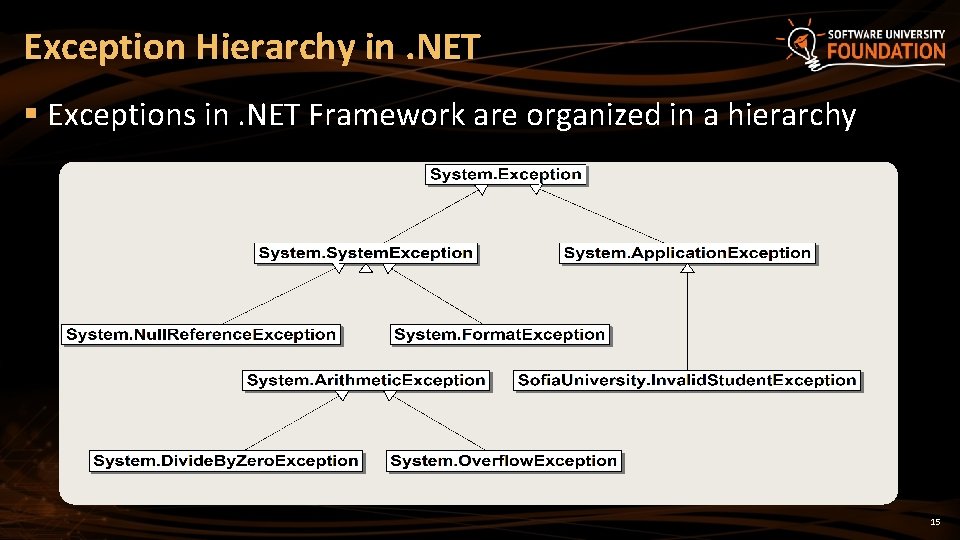
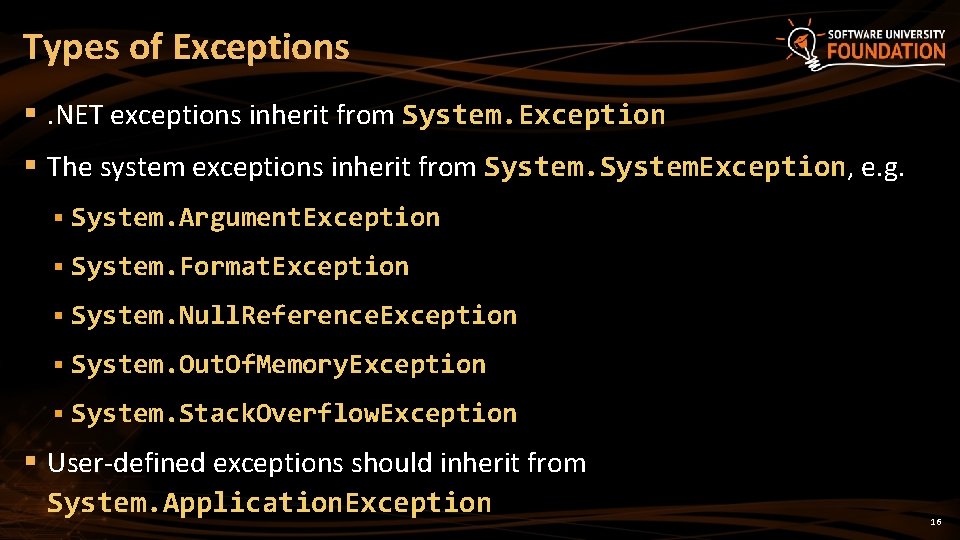
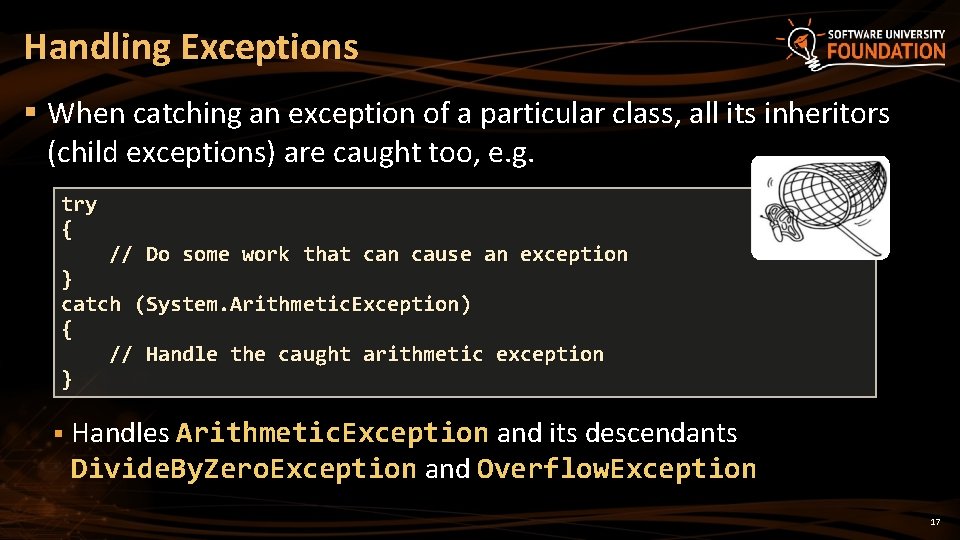
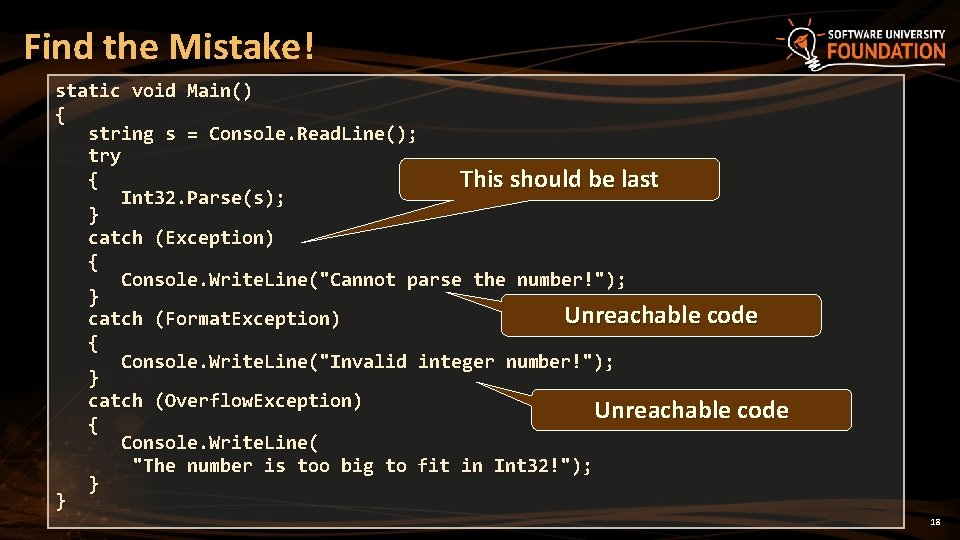
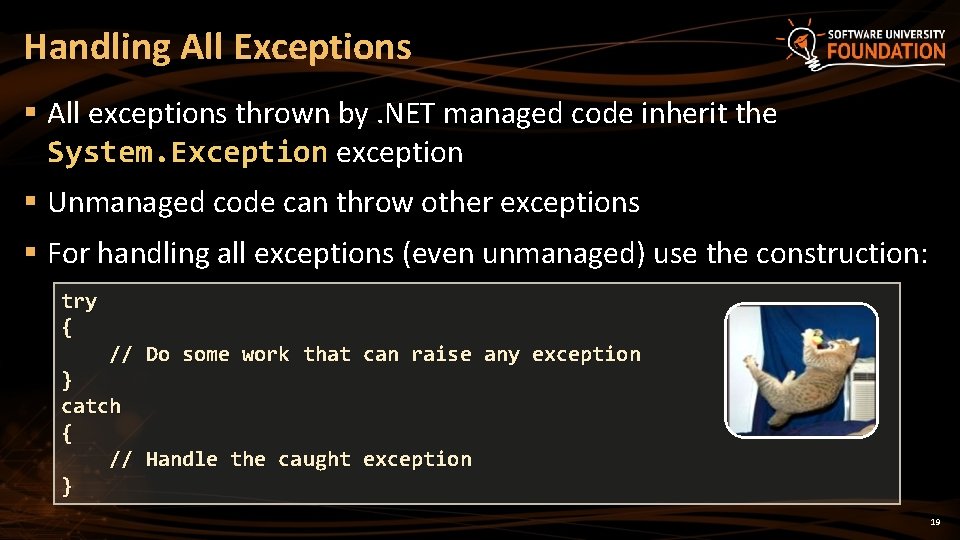
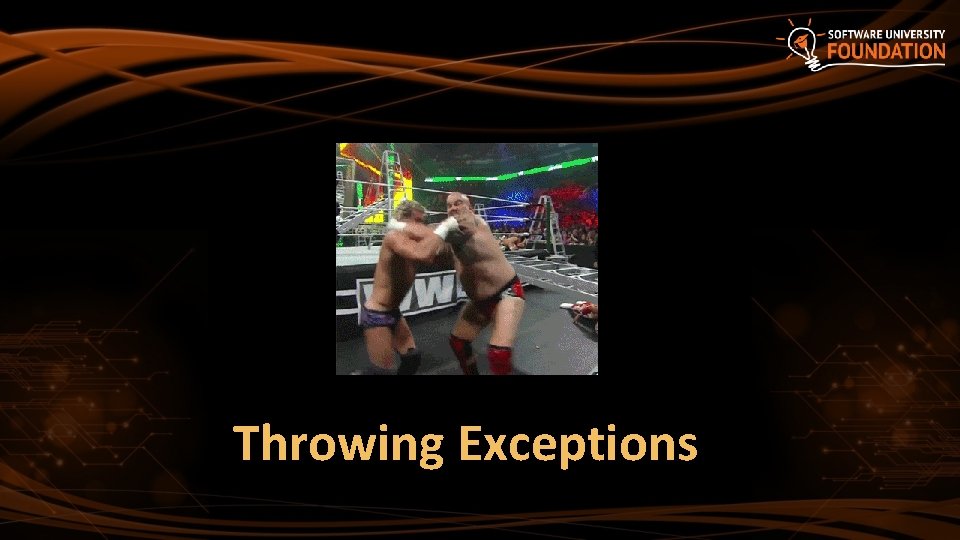
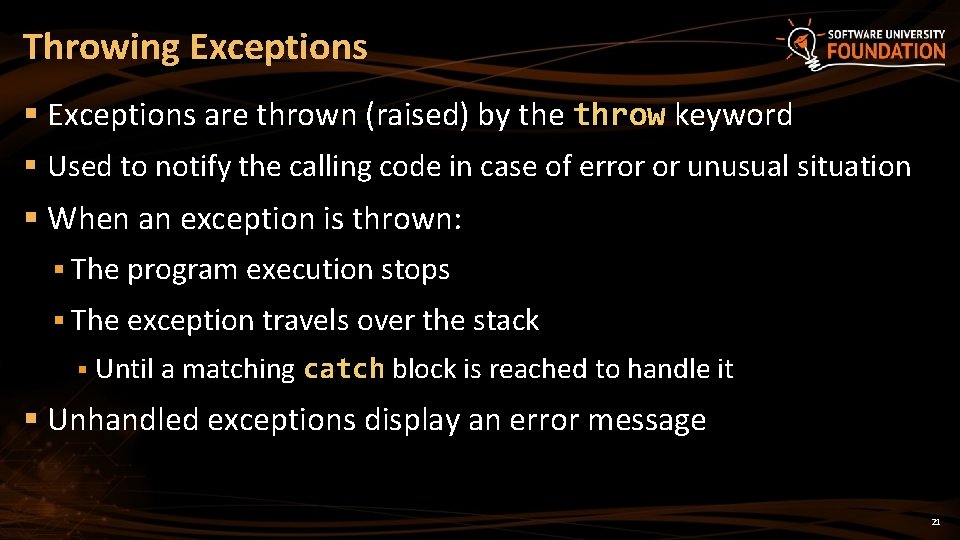
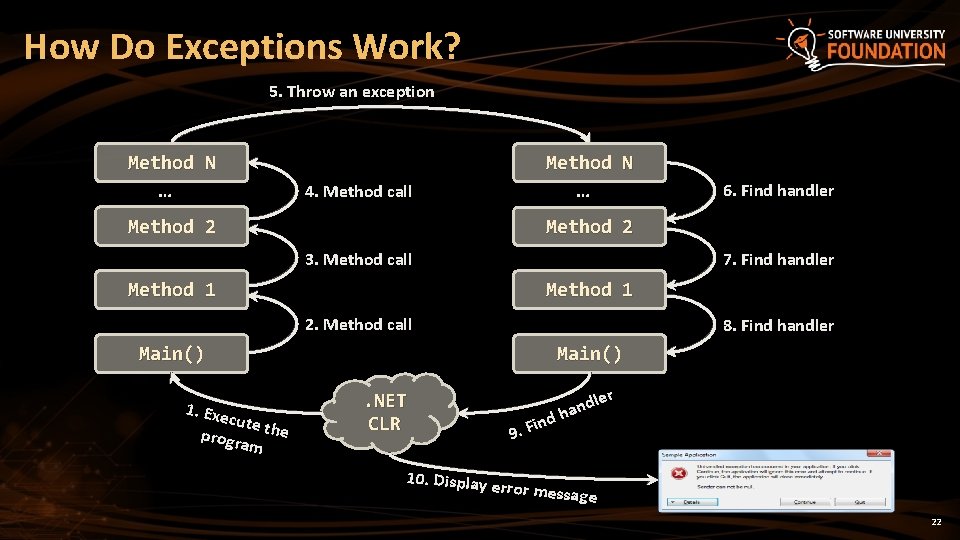
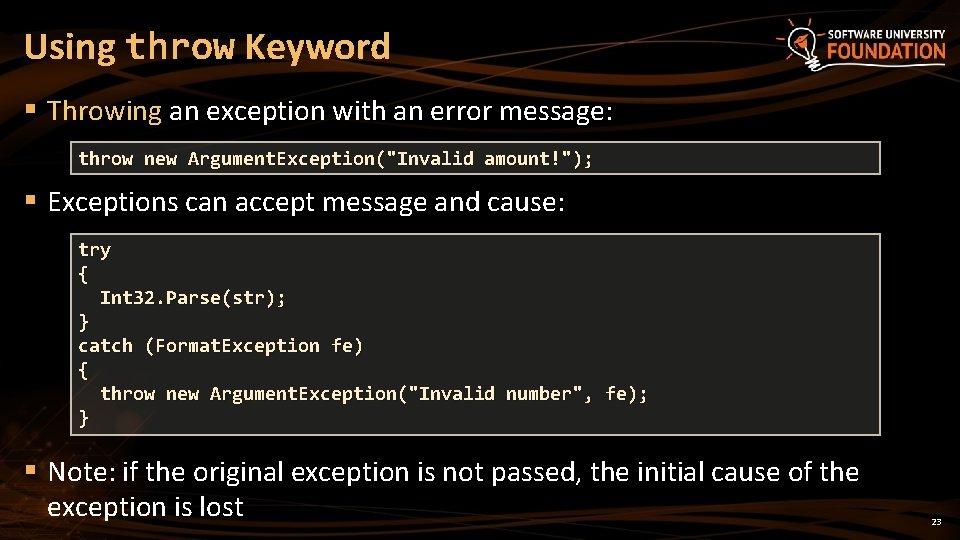
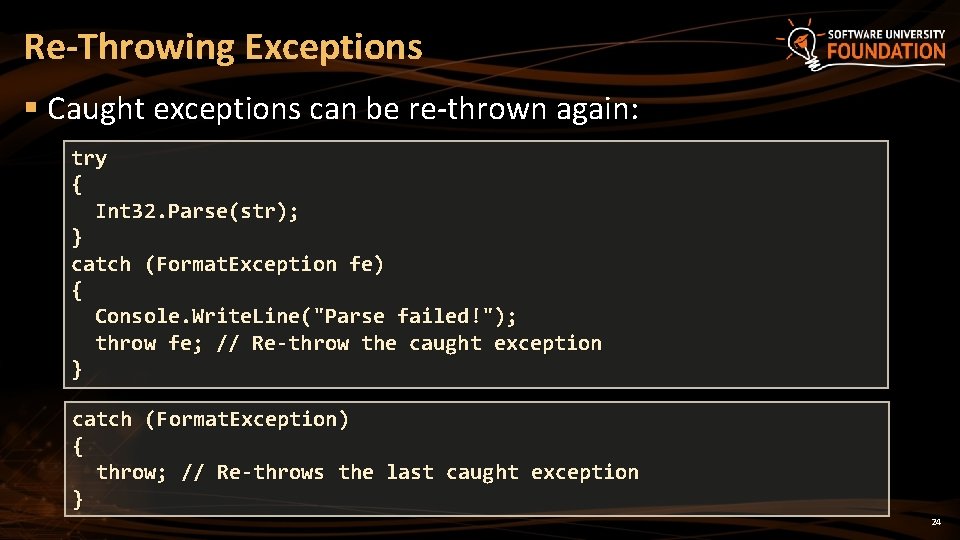
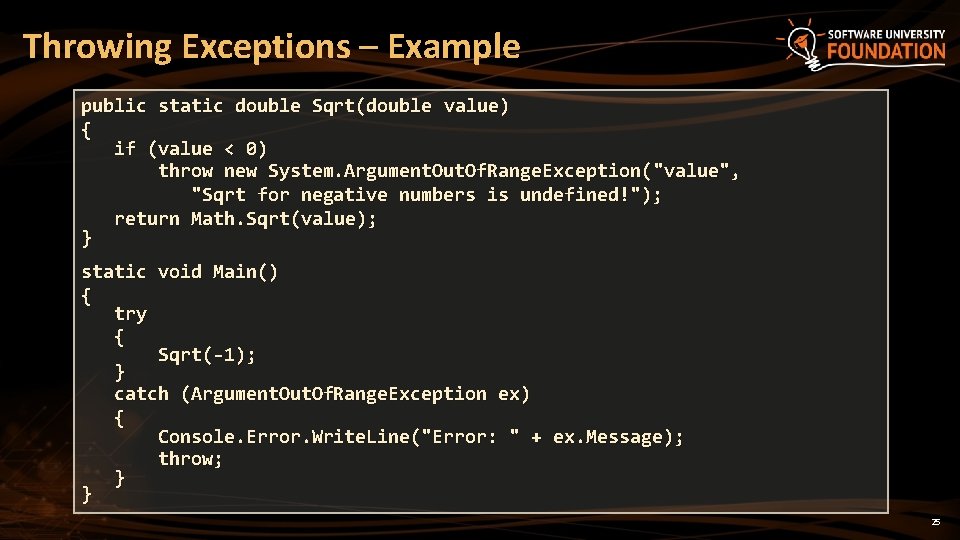
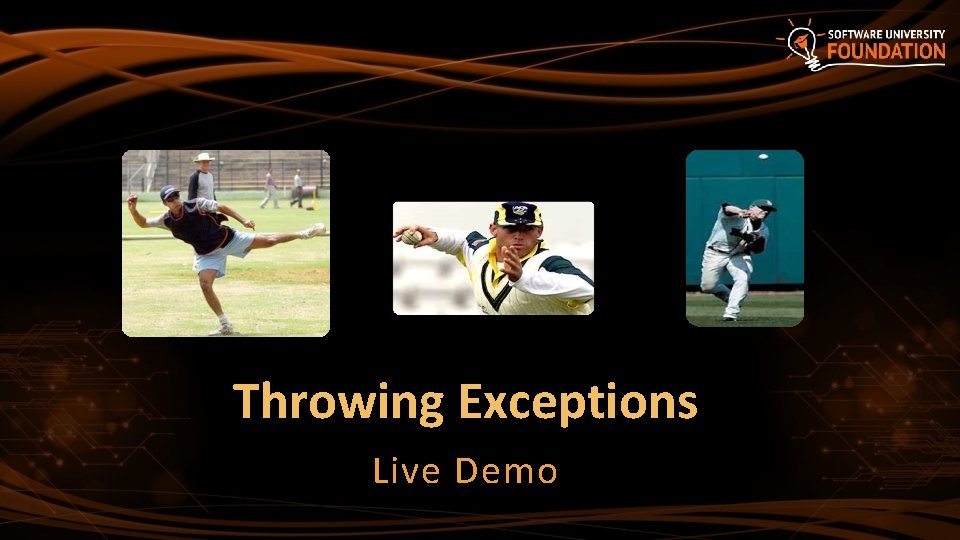
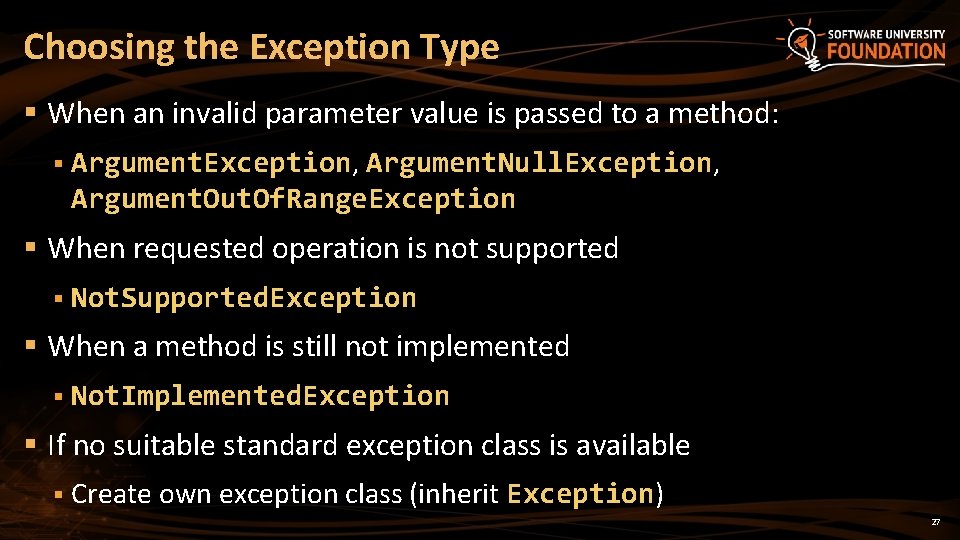
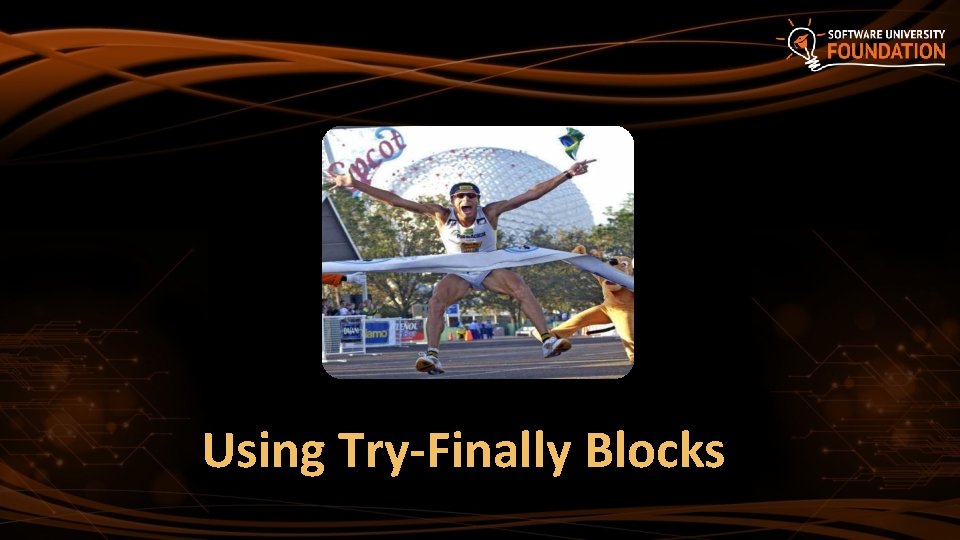
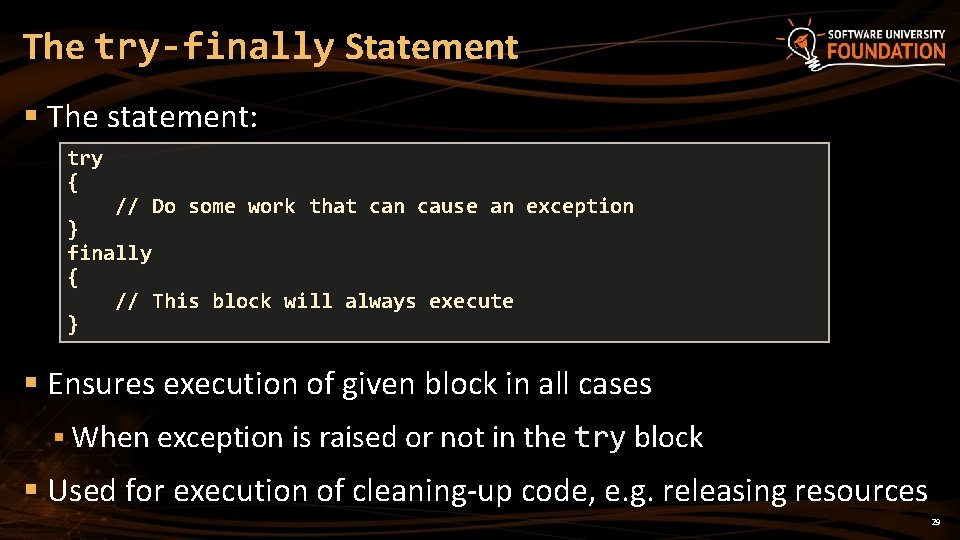
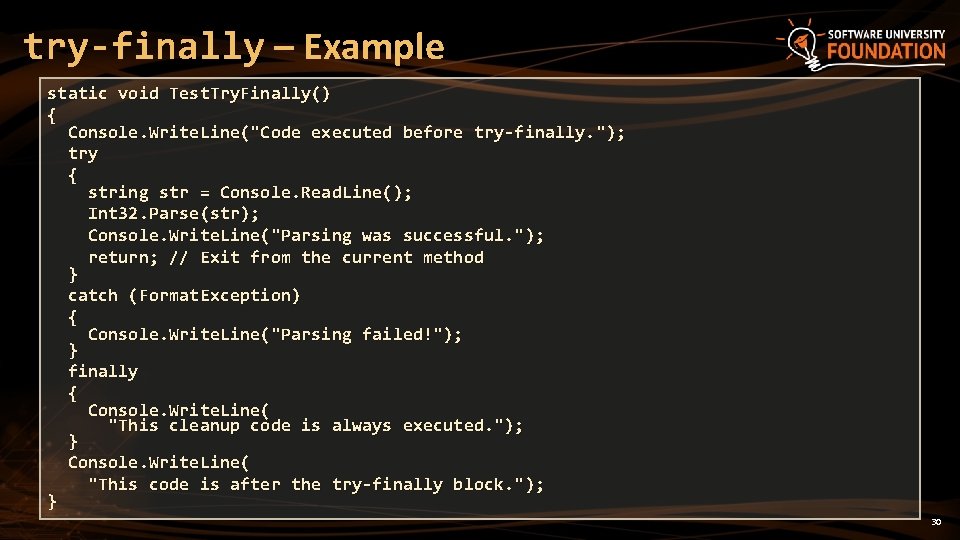
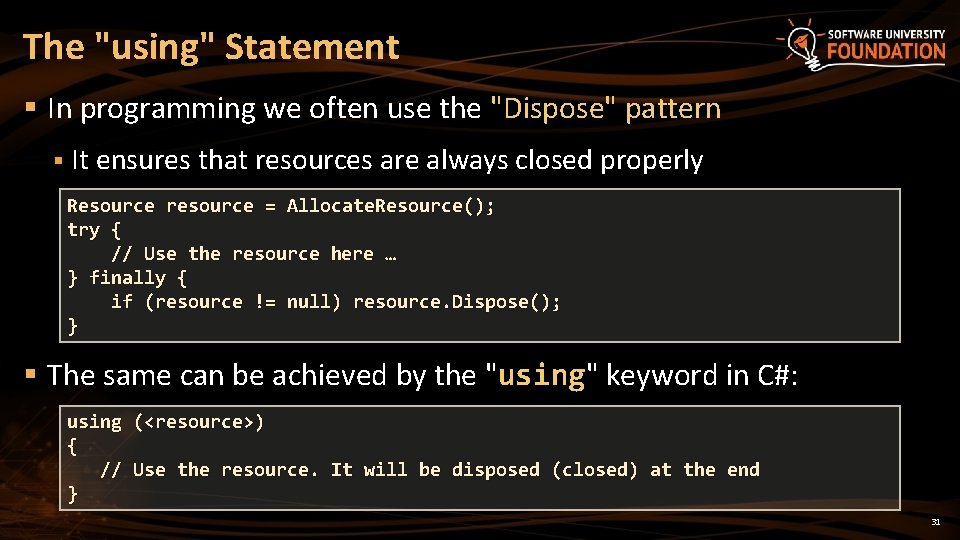
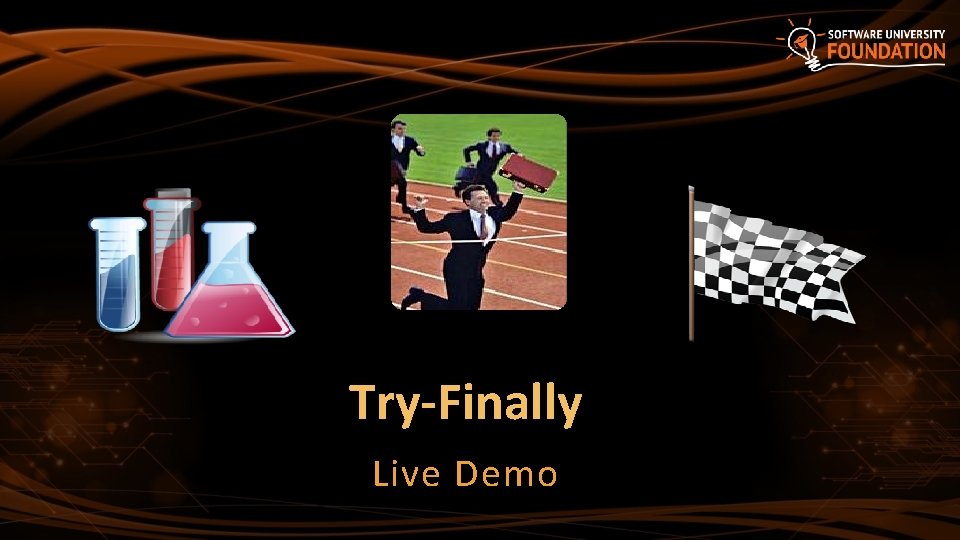
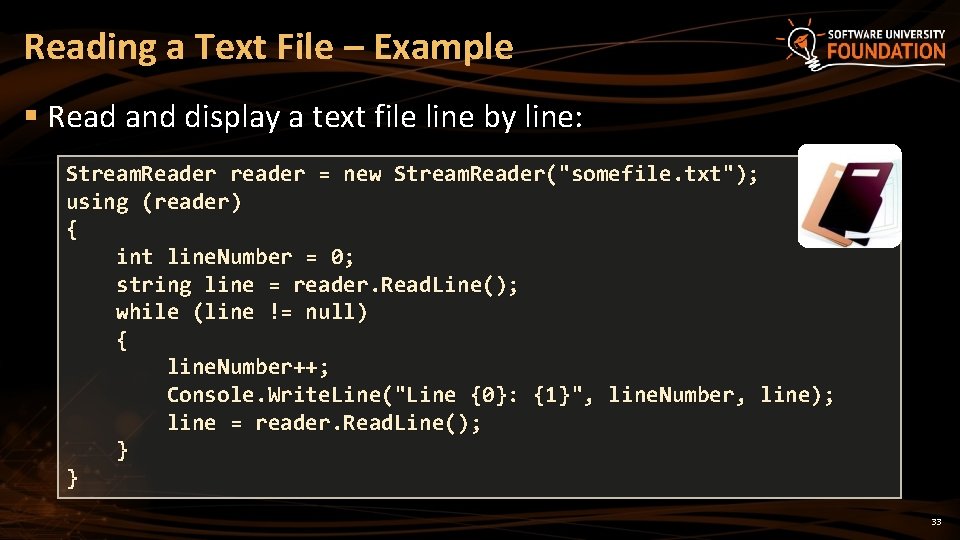
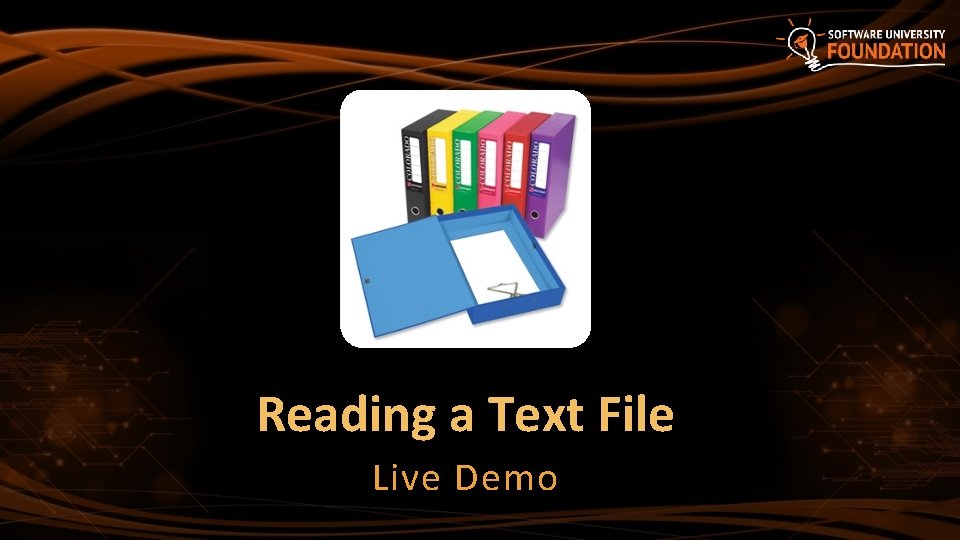
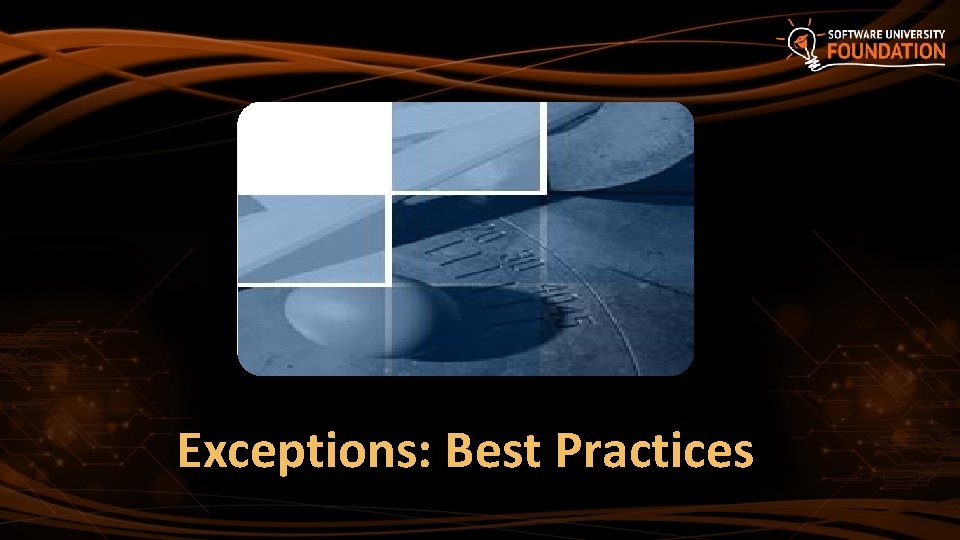
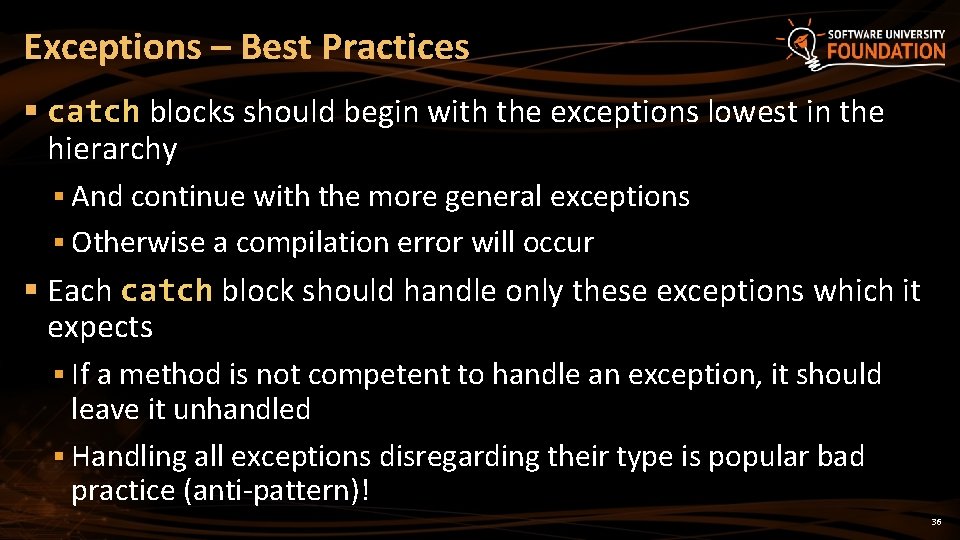
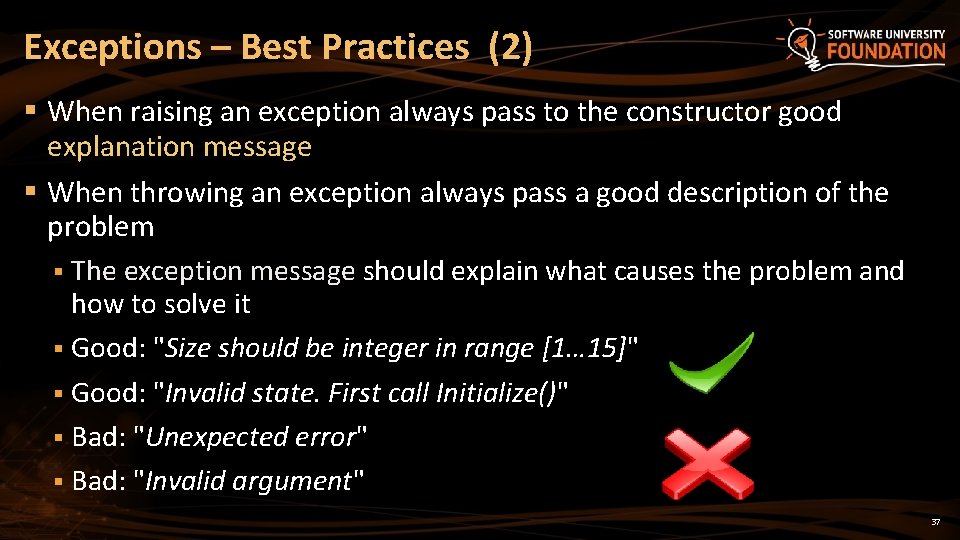
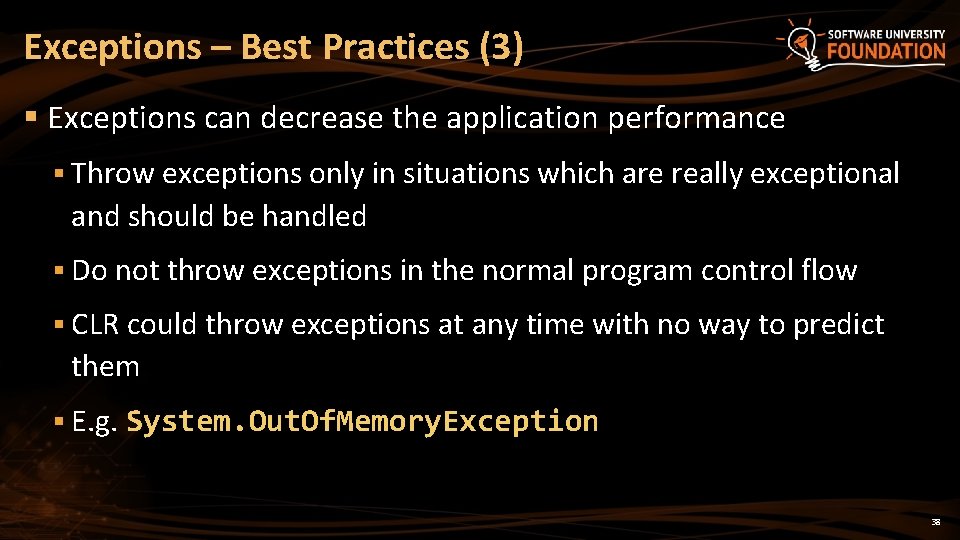
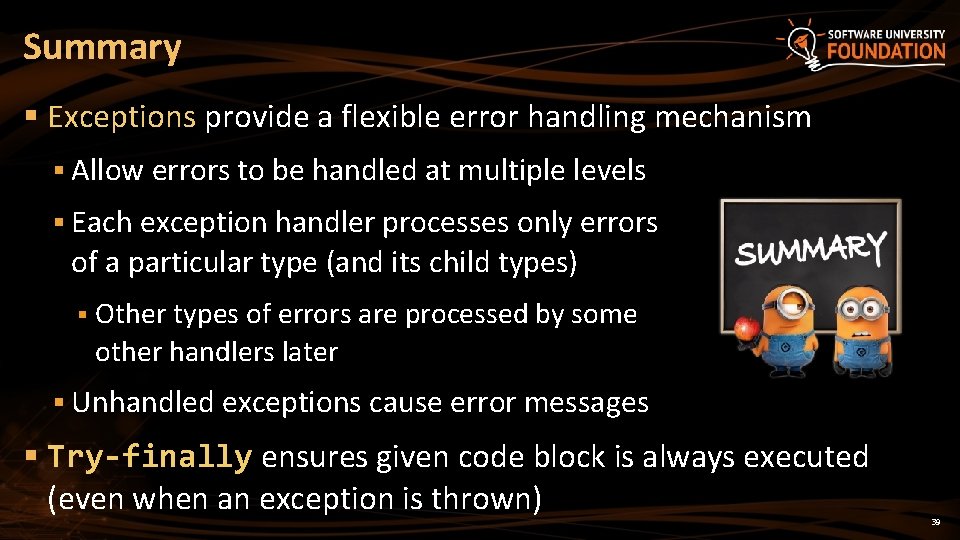
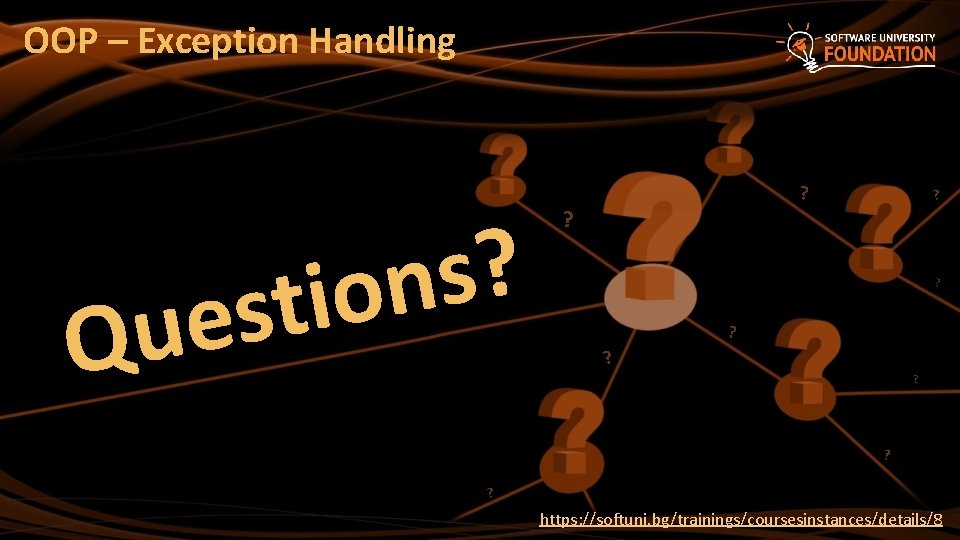
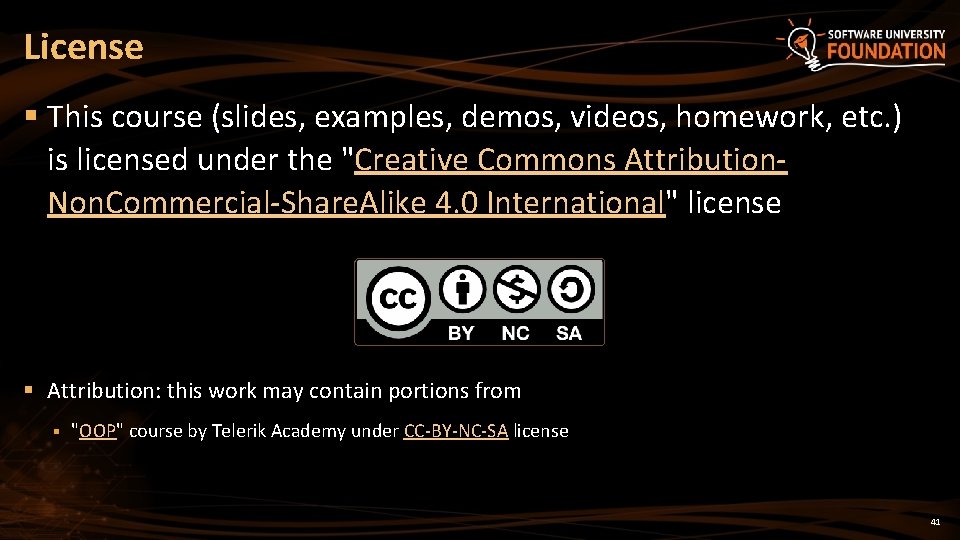
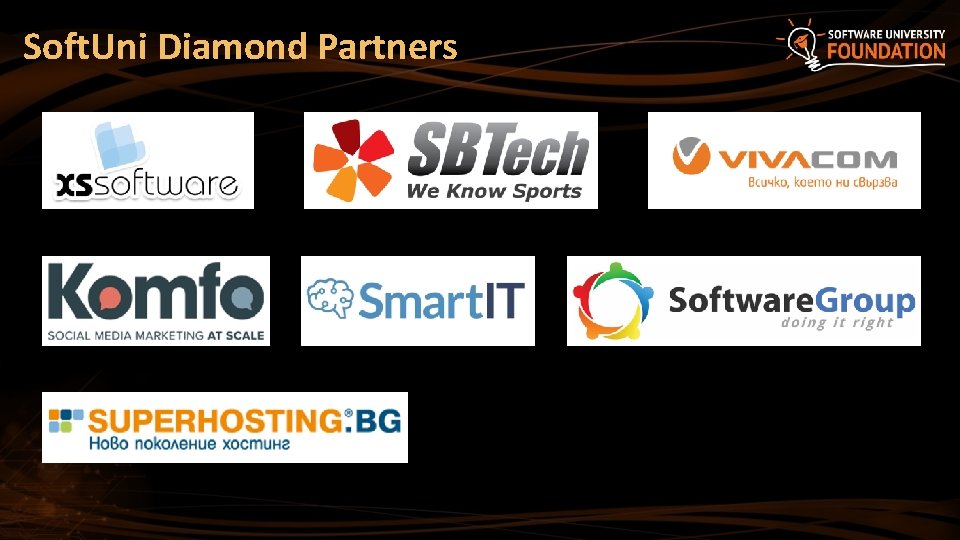
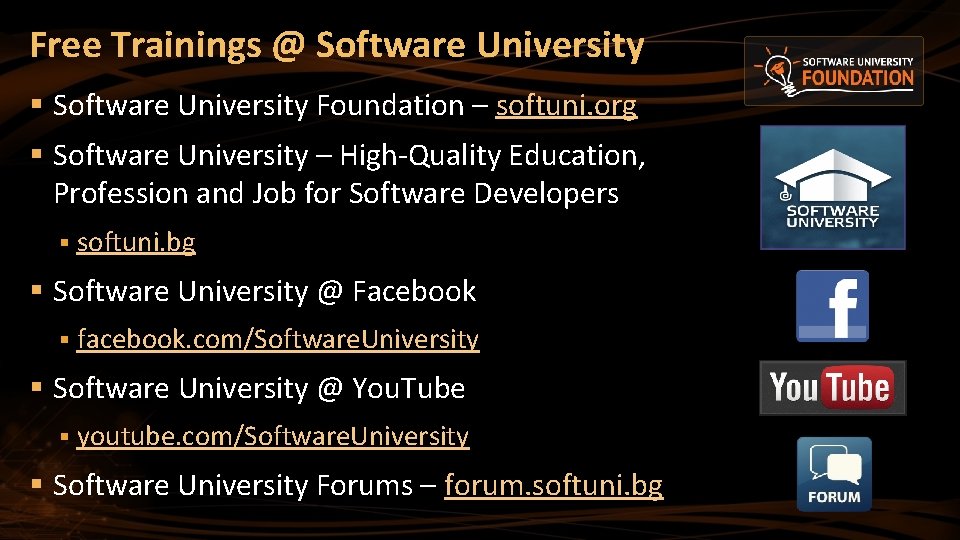
- Slides: 43
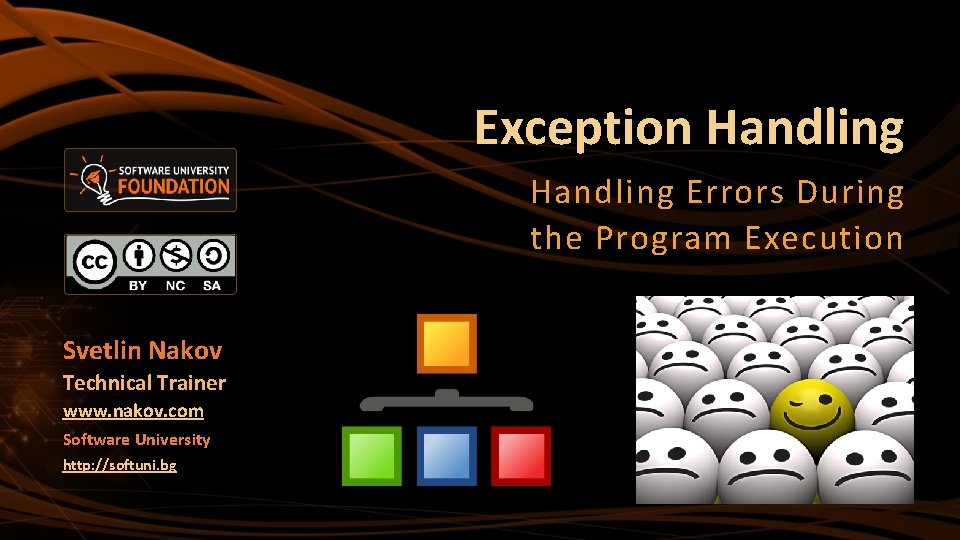
Exception Handling Errors During the Program Execution Svetlin Nakov Technical Trainer www. nakov. com Software University http: //softuni. bg
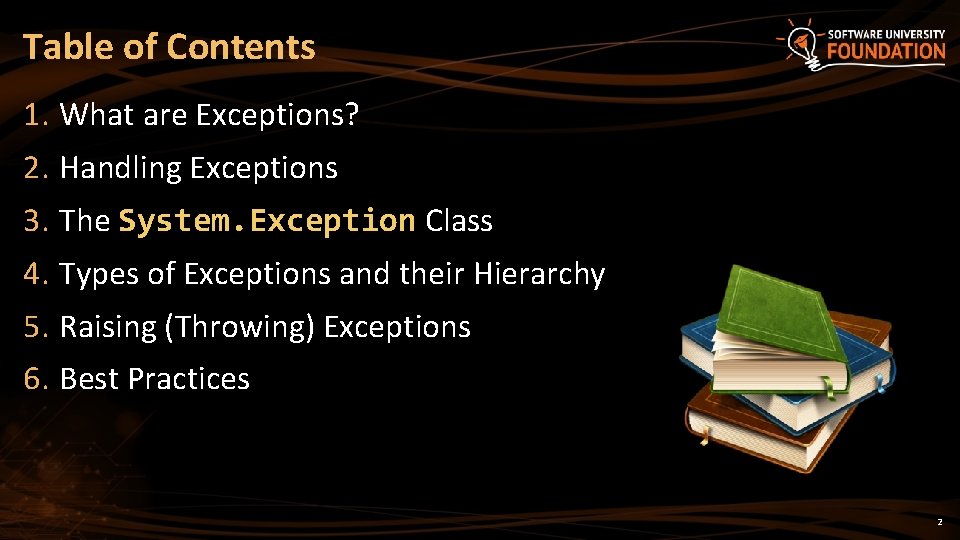
Table of Contents 1. What are Exceptions? 2. Handling Exceptions 3. The System. Exception Class 4. Types of Exceptions and their Hierarchy 5. Raising (Throwing) Exceptions 6. Best Practices 2
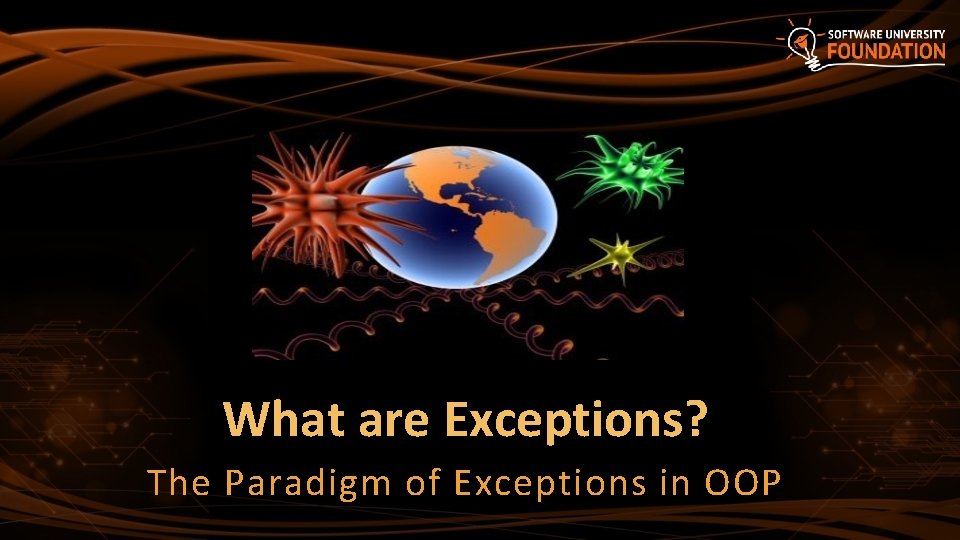
What are Exceptions? The Paradigm of Exceptions in OOP
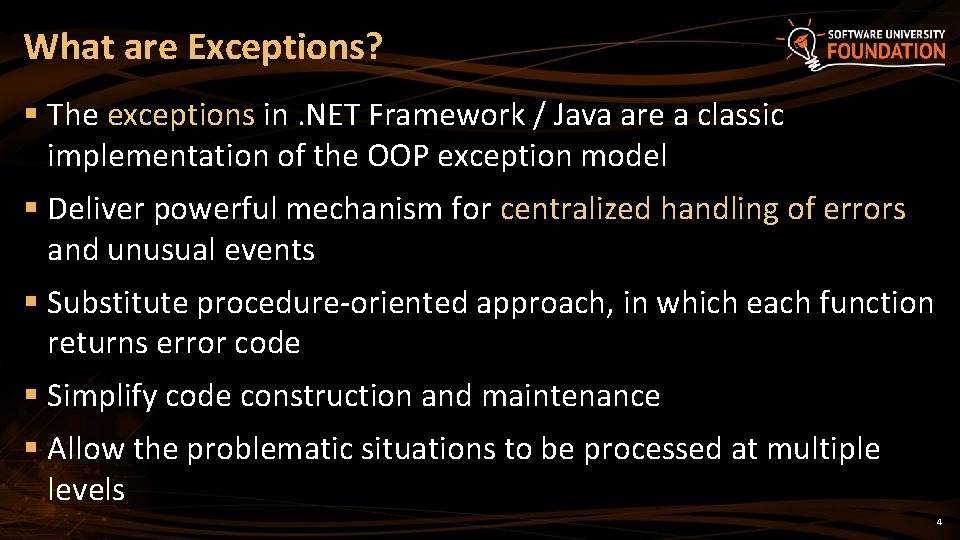
What are Exceptions? § The exceptions in. NET Framework / Java are a classic implementation of the OOP exception model § Deliver powerful mechanism for centralized handling of errors and unusual events § Substitute procedure-oriented approach, in which each function returns error code § Simplify code construction and maintenance § Allow the problematic situations to be processed at multiple levels 4
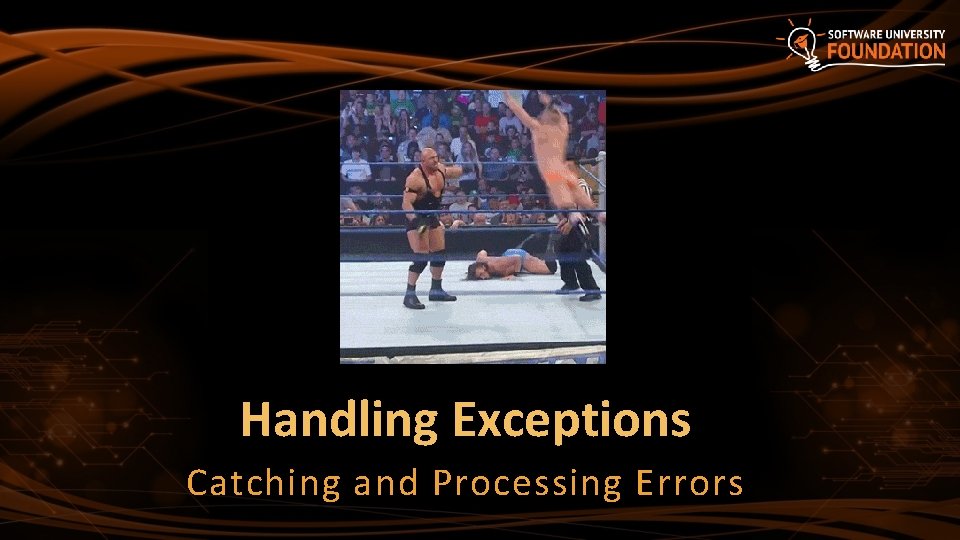
Handling Exceptions Catching and Processing Errors
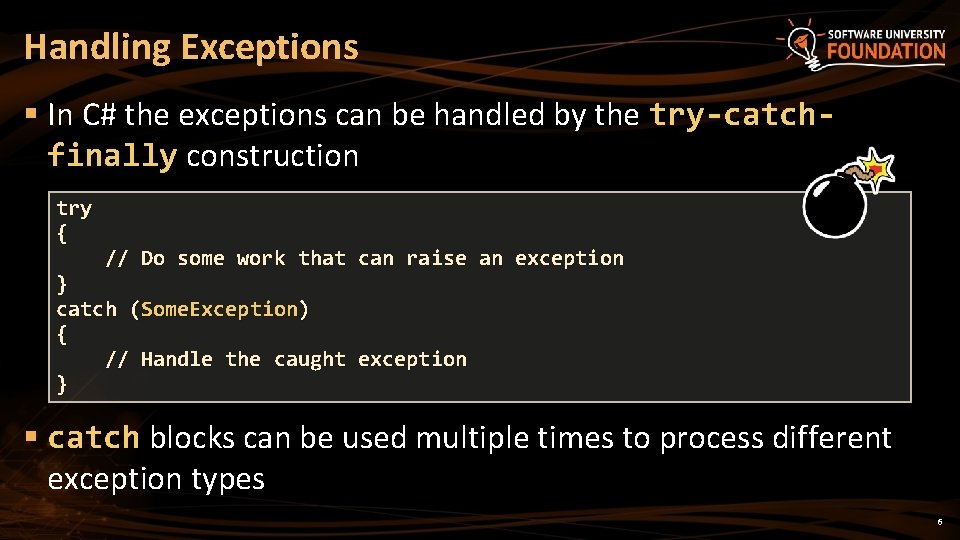
Handling Exceptions § In C# the exceptions can be handled by the try-catchfinally construction try { // Do some work that can raise an exception } catch (Some. Exception) { // Handle the caught exception } § catch blocks can be used multiple times to process different exception types 6
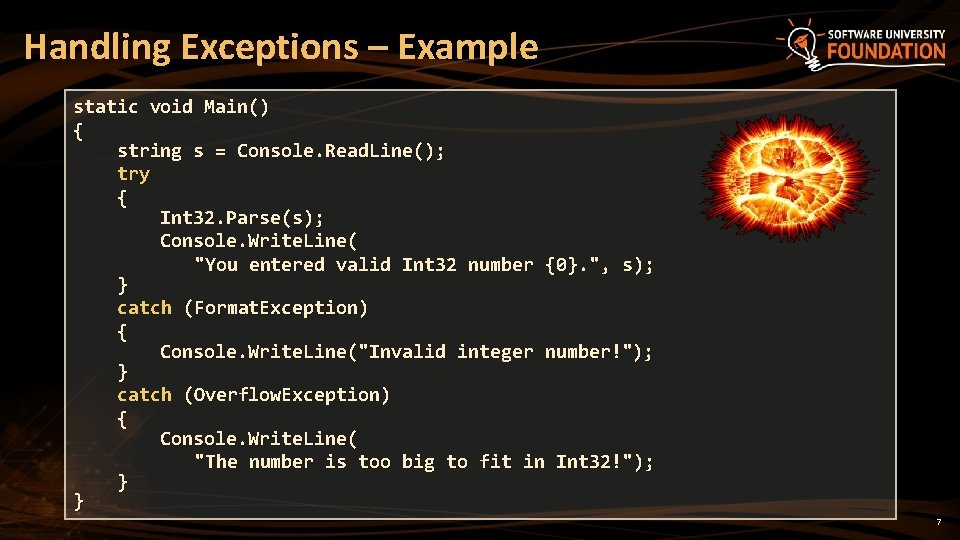
Handling Exceptions – Example static void Main() { string s = Console. Read. Line(); try { Int 32. Parse(s); Console. Write. Line( "You entered valid Int 32 number {0}. ", s); } catch (Format. Exception) { Console. Write. Line("Invalid integer number!"); } catch (Overflow. Exception) { Console. Write. Line( "The number is too big to fit in Int 32!"); } } 7
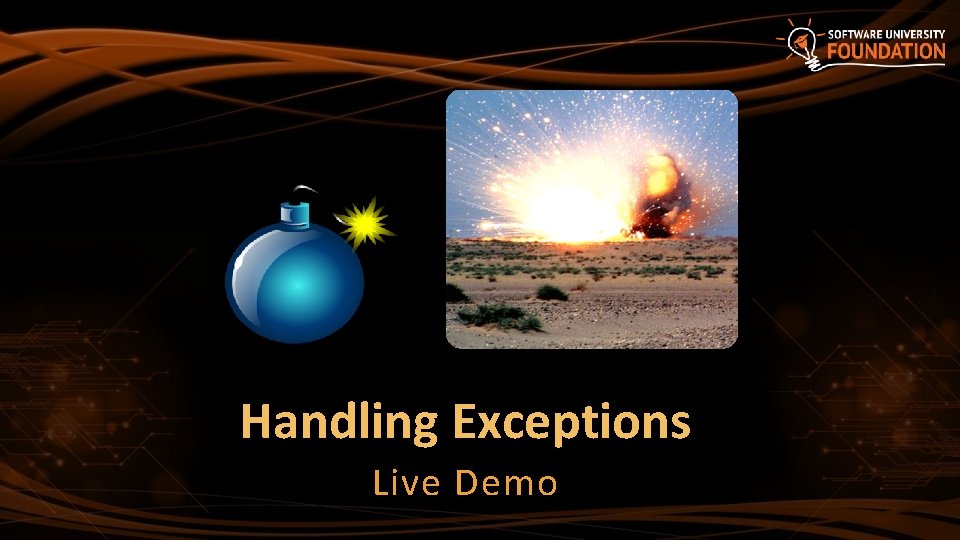
Handling Exceptions Live Demo
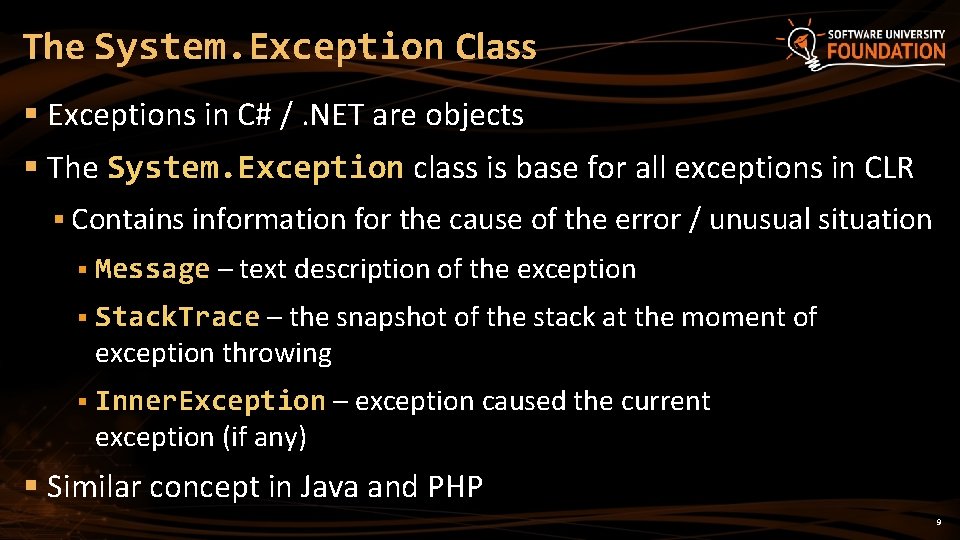
The System. Exception Class § Exceptions in C# /. NET are objects § The System. Exception class is base for all exceptions in CLR § Contains information for the cause of the error / unusual situation § Message – text description of the exception § Stack. Trace – the snapshot of the stack at the moment of exception throwing § Inner. Exception – exception caused the current exception (if any) § Similar concept in Java and PHP 9
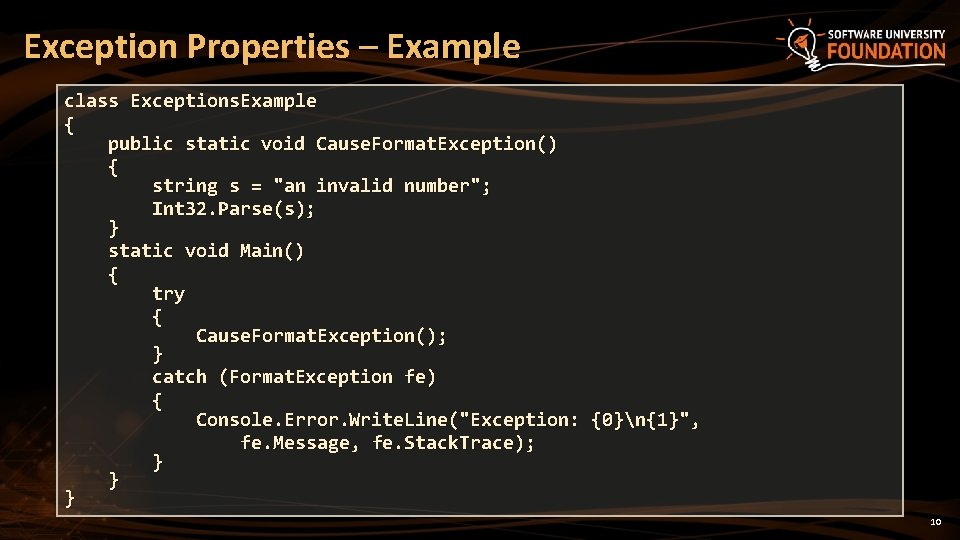
Exception Properties – Example class Exceptions. Example { public static void Cause. Format. Exception() { string s = "an invalid number"; Int 32. Parse(s); } static void Main() { try { Cause. Format. Exception(); } catch (Format. Exception fe) { Console. Error. Write. Line("Exception: {0}n{1}", fe. Message, fe. Stack. Trace); } } } 10
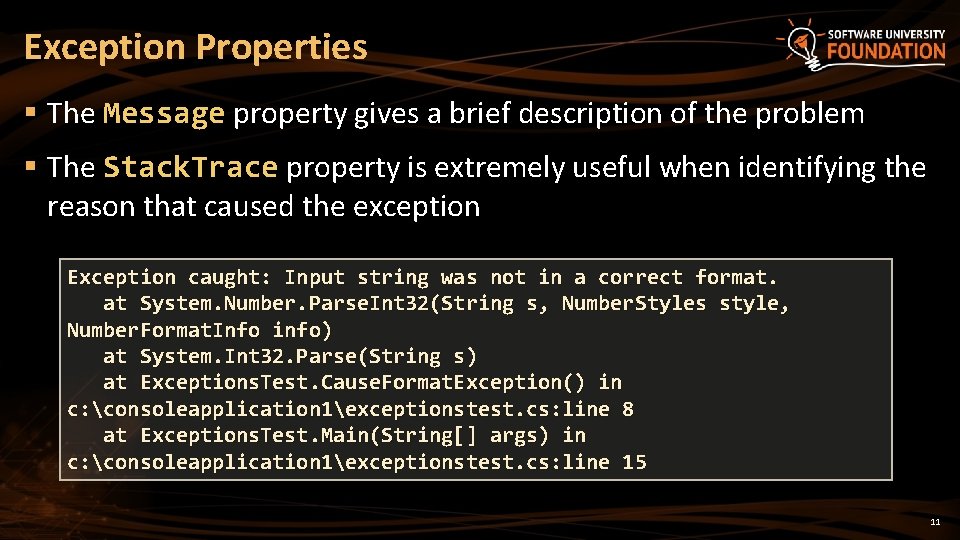
Exception Properties § The Message property gives a brief description of the problem § The Stack. Trace property is extremely useful when identifying the reason that caused the exception Exception caught: Input string was not in a correct format. at System. Number. Parse. Int 32(String s, Number. Styles style, Number. Format. Info info) at System. Int 32. Parse(String s) at Exceptions. Test. Cause. Format. Exception() in c: consoleapplication 1exceptionstest. cs: line 8 at Exceptions. Test. Main(String[] args) in c: consoleapplication 1exceptionstest. cs: line 15 11
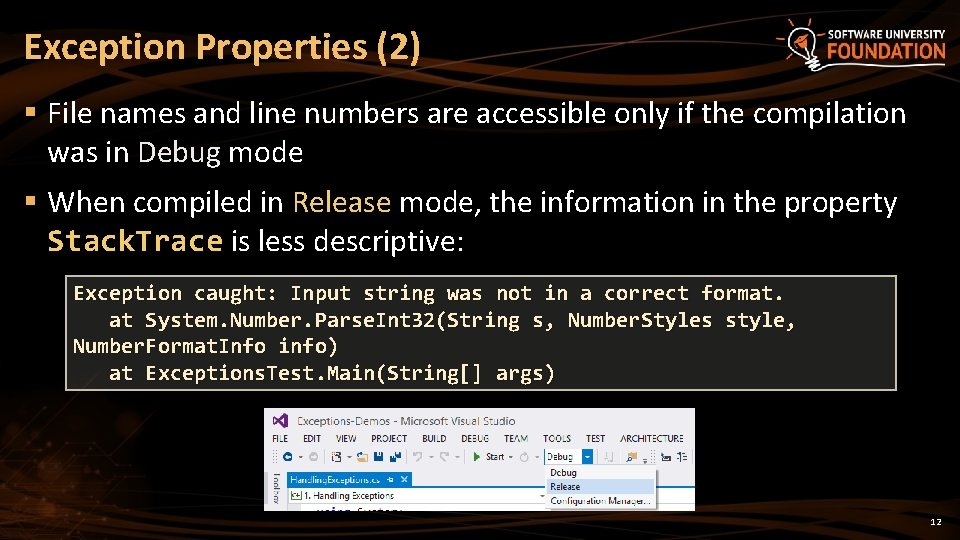
Exception Properties (2) § File names and line numbers are accessible only if the compilation was in Debug mode § When compiled in Release mode, the information in the property Stack. Trace is less descriptive: Exception caught: Input string was not in a correct format. at System. Number. Parse. Int 32(String s, Number. Styles style, Number. Format. Info info) at Exceptions. Test. Main(String[] args) 12
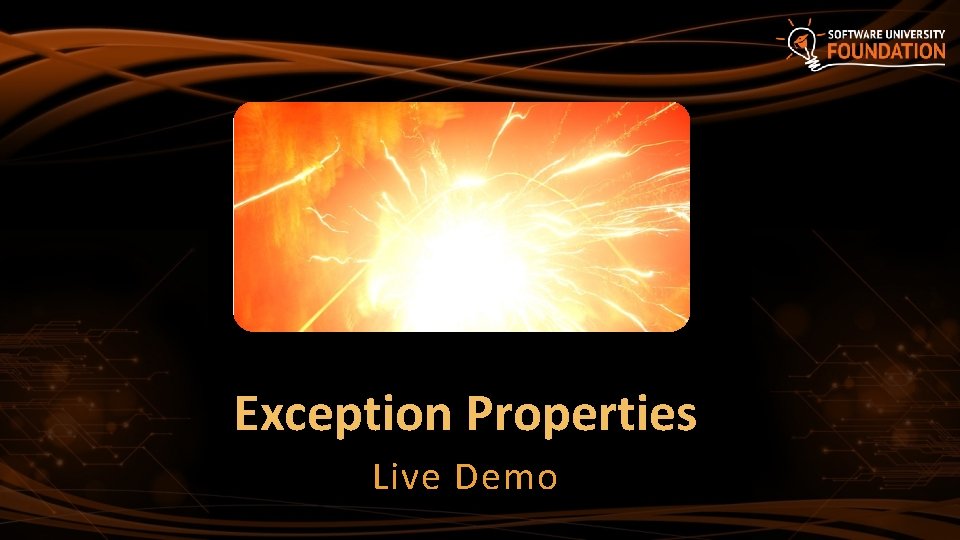
Exception Properties Live Demo
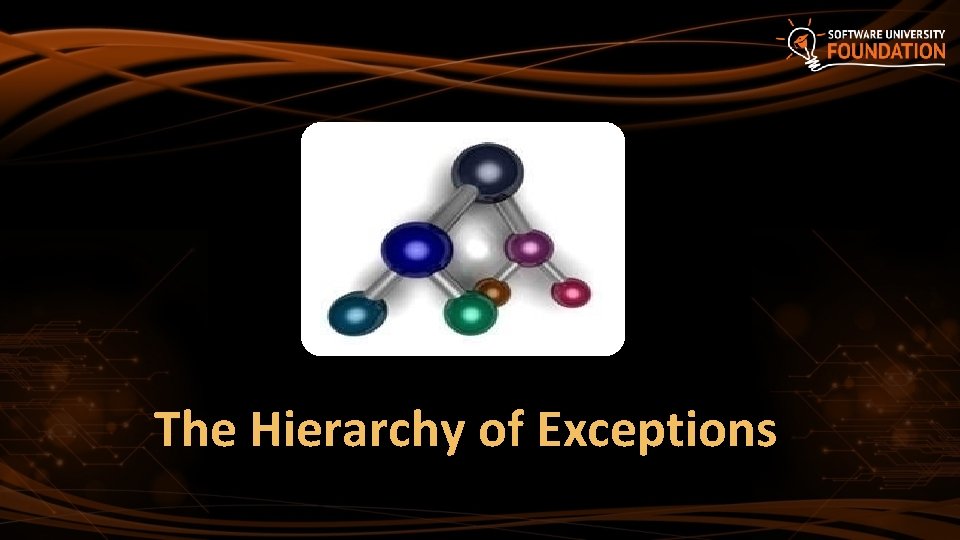
The Hierarchy of Exceptions
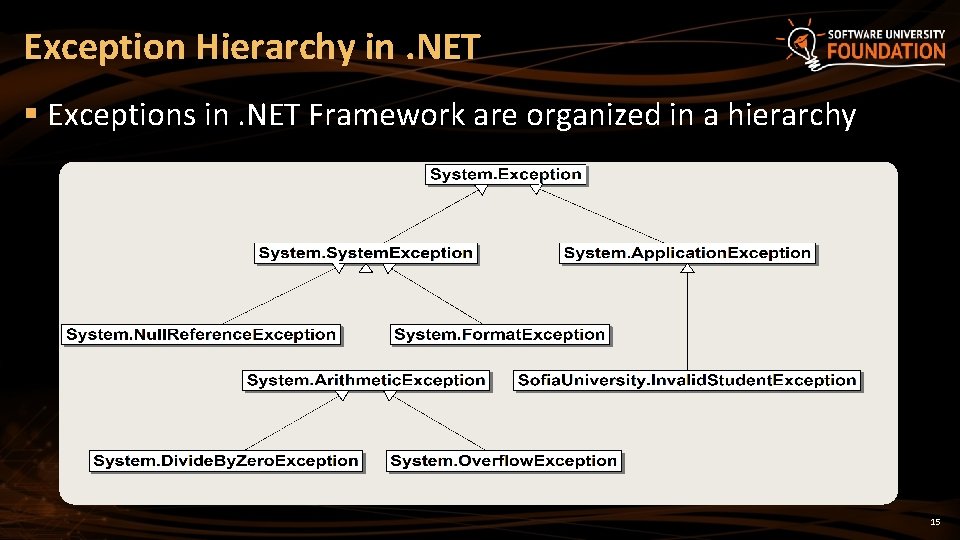
Exception Hierarchy in. NET § Exceptions in. NET Framework are organized in a hierarchy 15
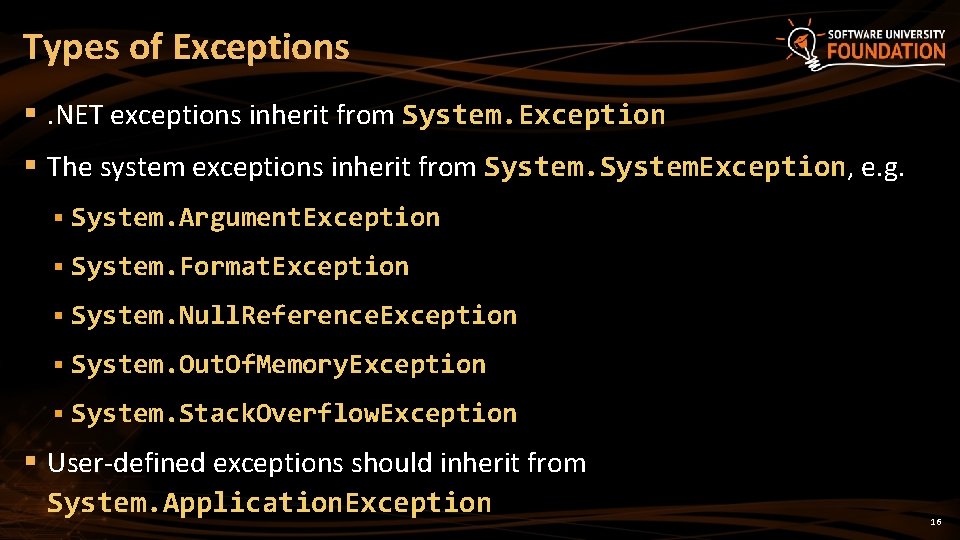
Types of Exceptions §. NET exceptions inherit from System. Exception § The system exceptions inherit from System. Exception, e. g. § System. Argument. Exception § System. Format. Exception § System. Null. Reference. Exception § System. Out. Of. Memory. Exception § System. Stack. Overflow. Exception § User-defined exceptions should inherit from System. Application. Exception 16
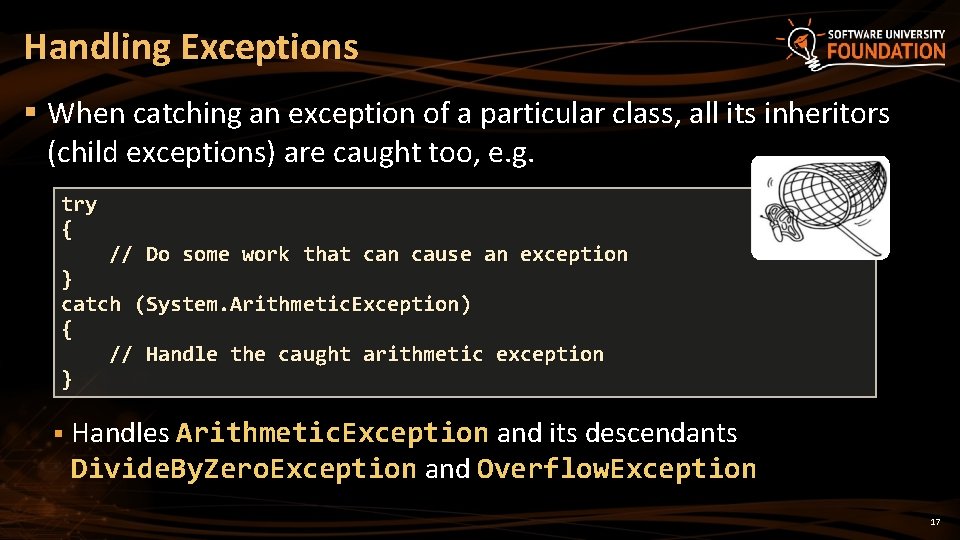
Handling Exceptions § When catching an exception of a particular class, all its inheritors (child exceptions) are caught too, e. g. try { // Do some work that can cause an exception } catch (System. Arithmetic. Exception) { // Handle the caught arithmetic exception } § Handles Arithmetic. Exception and its descendants Divide. By. Zero. Exception and Overflow. Exception 17
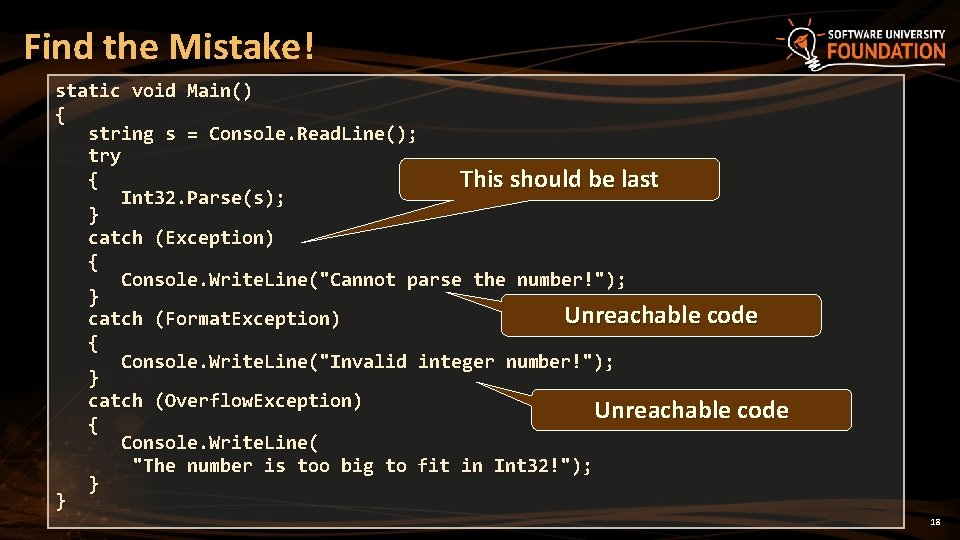
Find the Mistake! static void Main() { string s = Console. Read. Line(); try { This should be last Int 32. Parse(s); } catch (Exception) { Console. Write. Line("Cannot parse the number!"); } Unreachable code catch (Format. Exception) { Console. Write. Line("Invalid integer number!"); } catch (Overflow. Exception) Unreachable code { Console. Write. Line( "The number is too big to fit in Int 32!"); } } 18
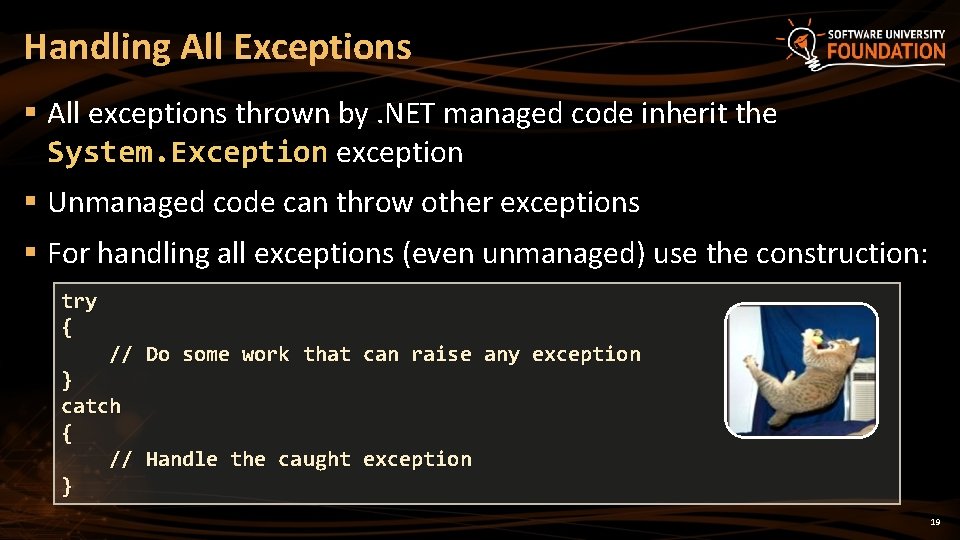
Handling All Exceptions § All exceptions thrown by. NET managed code inherit the System. Exception exception § Unmanaged code can throw other exceptions § For handling all exceptions (even unmanaged) use the construction: try { // Do some work that can raise any exception } catch { // Handle the caught exception } 19
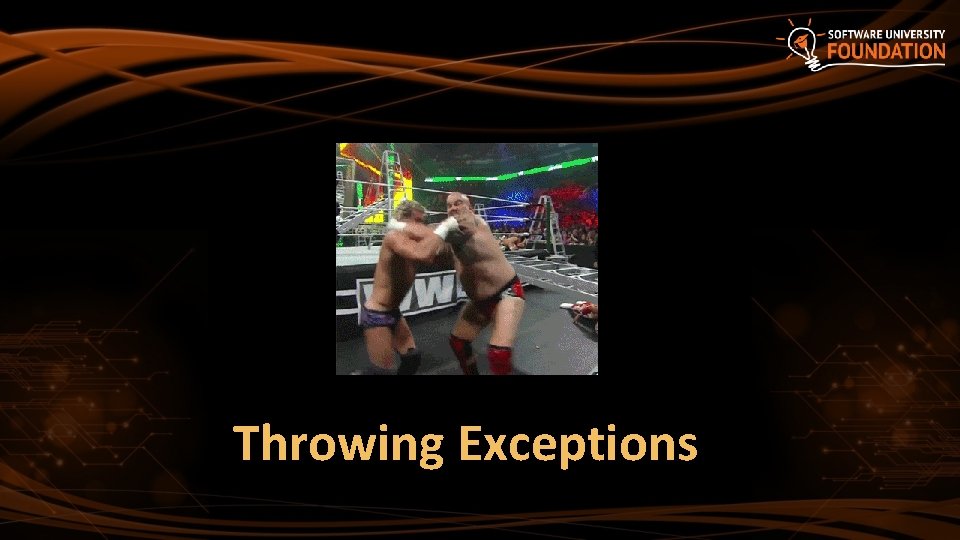
Throwing Exceptions
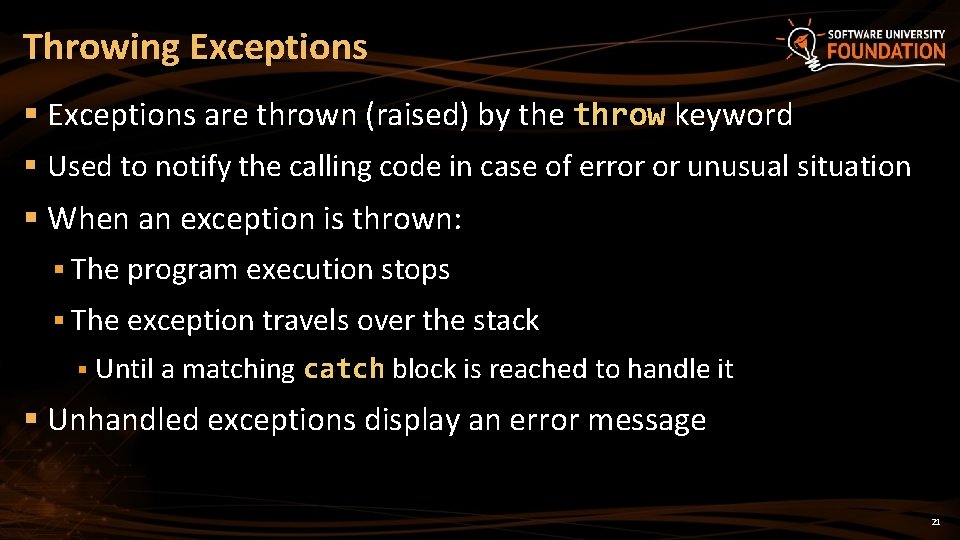
Throwing Exceptions § Exceptions are thrown (raised) by the throw keyword § Used to notify the calling code in case of error or unusual situation § When an exception is thrown: § The program execution stops § The exception travels over the stack § Until a matching catch block is reached to handle it § Unhandled exceptions display an error message 21
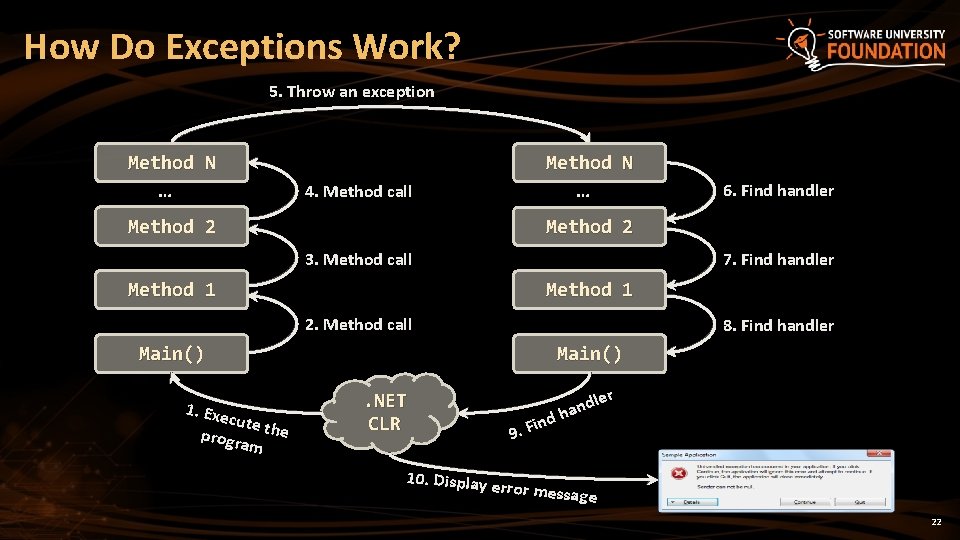
How Do Exceptions Work? 5. Throw an exception Method N … 4. Method call Method 2 Method N … Method 2 3. Method call Method 1 7. Find handler Method 1 2. Method call Main() 1. Exe cute progr the am 6. Find handler 8. Find handler Main(). NET CLR ler d n ha d n i 9. F 10. Display error messa ge 22
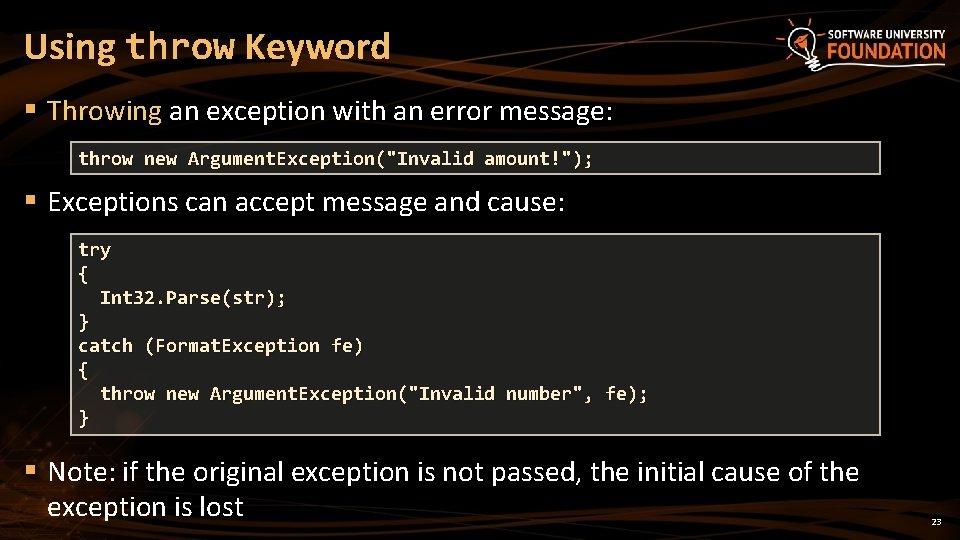
Using throw Keyword § Throwing an exception with an error message: throw new Argument. Exception("Invalid amount!"); § Exceptions can accept message and cause: try { Int 32. Parse(str); } catch (Format. Exception fe) { throw new Argument. Exception("Invalid number", fe); } § Note: if the original exception is not passed, the initial cause of the exception is lost 23
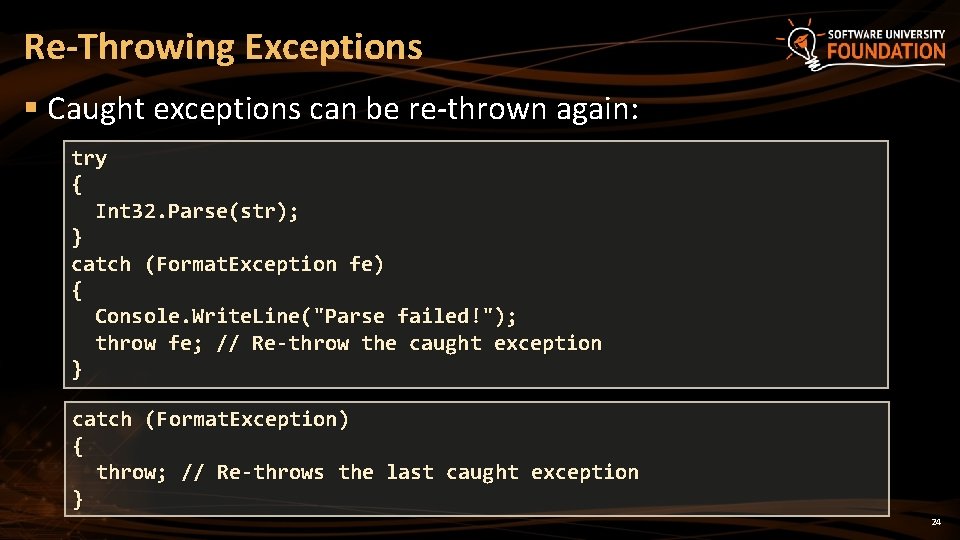
Re-Throwing Exceptions § Caught exceptions can be re-thrown again: try { Int 32. Parse(str); } catch (Format. Exception fe) { Console. Write. Line("Parse failed!"); throw fe; // Re-throw the caught exception } catch (Format. Exception) { throw; // Re-throws the last caught exception } 24
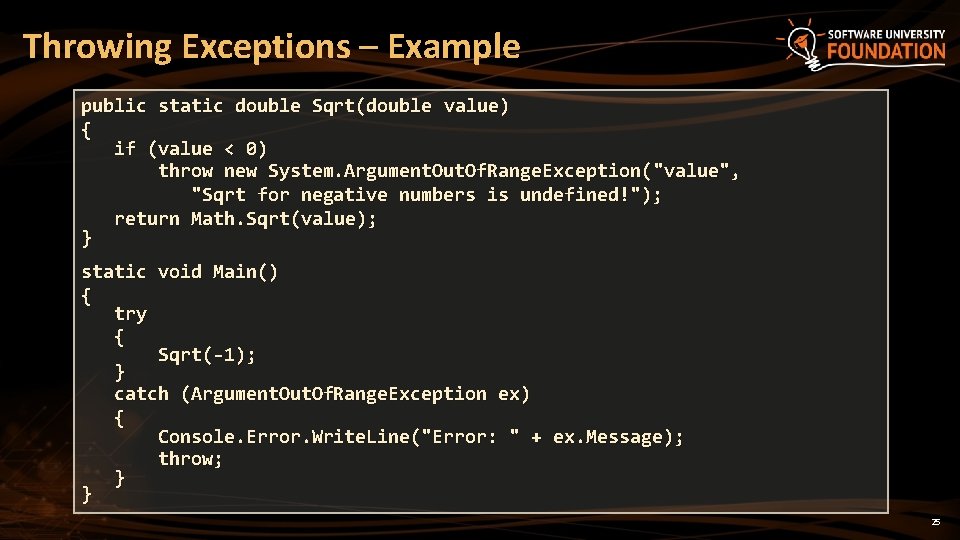
Throwing Exceptions – Example public static double Sqrt(double value) { if (value < 0) throw new System. Argument. Out. Of. Range. Exception( "value", "Sqrt for negative numbers is undefined!"); return Math. Sqrt(value); } static void Main() { try { Sqrt(-1); } catch (Argument. Out. Of. Range. Exception ex) { Console. Error. Write. Line("Error: " + ex. Message); throw; } } 25
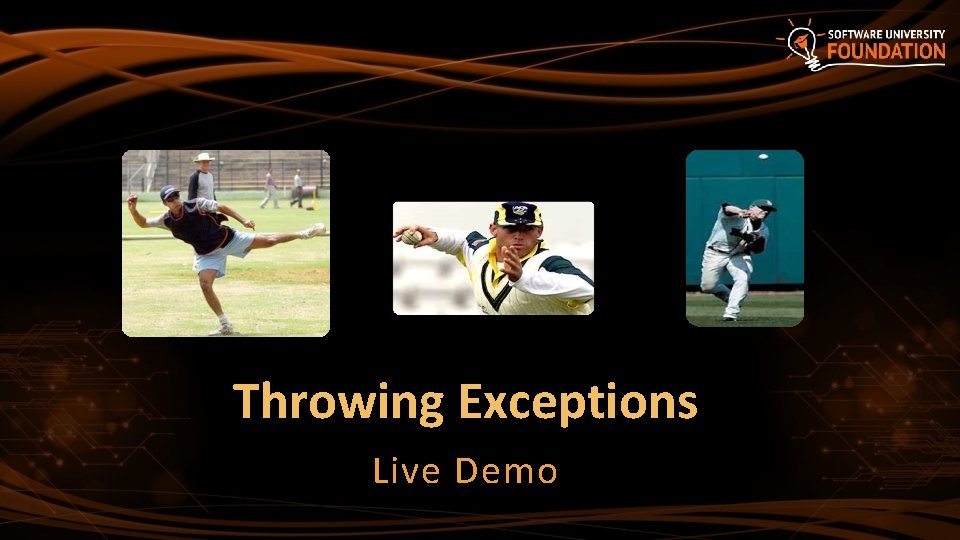
Throwing Exceptions Live Demo
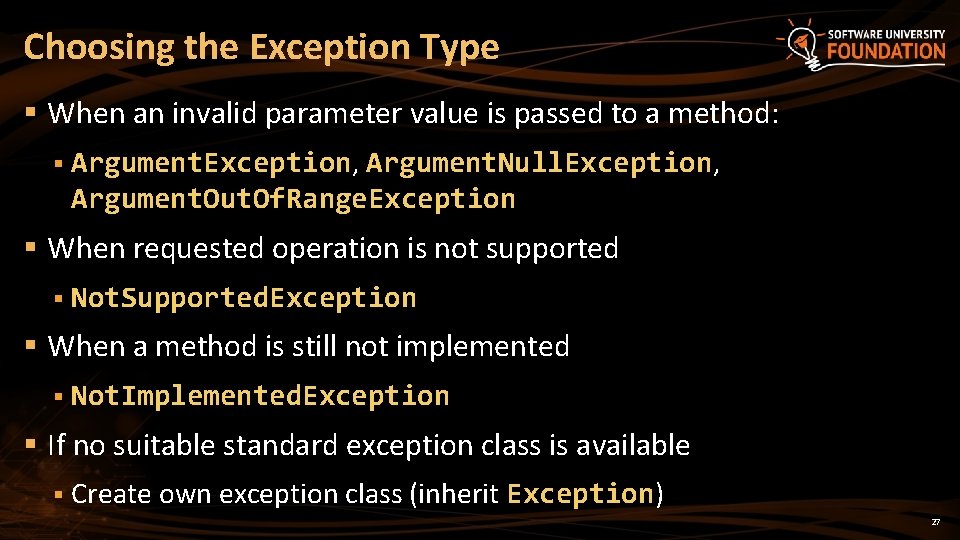
Choosing the Exception Type § When an invalid parameter value is passed to a method: § Argument. Exception, Argument. Null. Exception, Argument. Out. Of. Range. Exception § When requested operation is not supported § Not. Supported. Exception § When a method is still not implemented § Not. Implemented. Exception § If no suitable standard exception class is available § Create own exception class (inherit Exception) 27
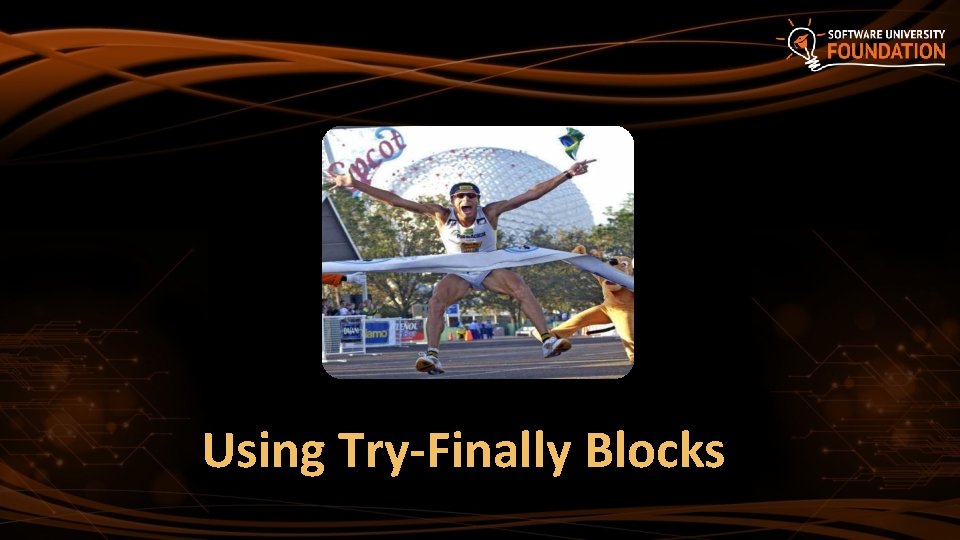
Using Try-Finally Blocks
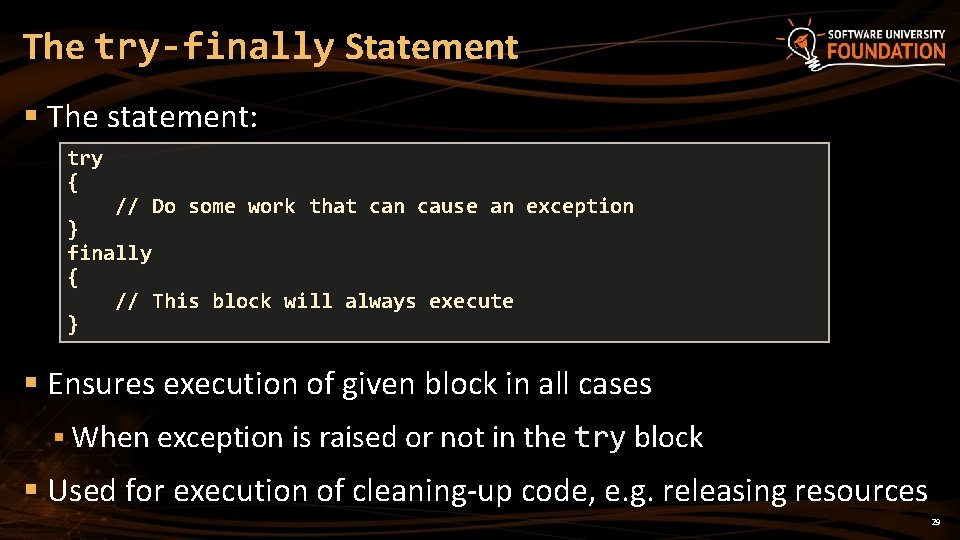
The try-finally Statement § The statement: try { // Do some work that can cause an exception } finally { // This block will always execute } § Ensures execution of given block in all cases § When exception is raised or not in the try block § Used for execution of cleaning-up code, e. g. releasing resources 29
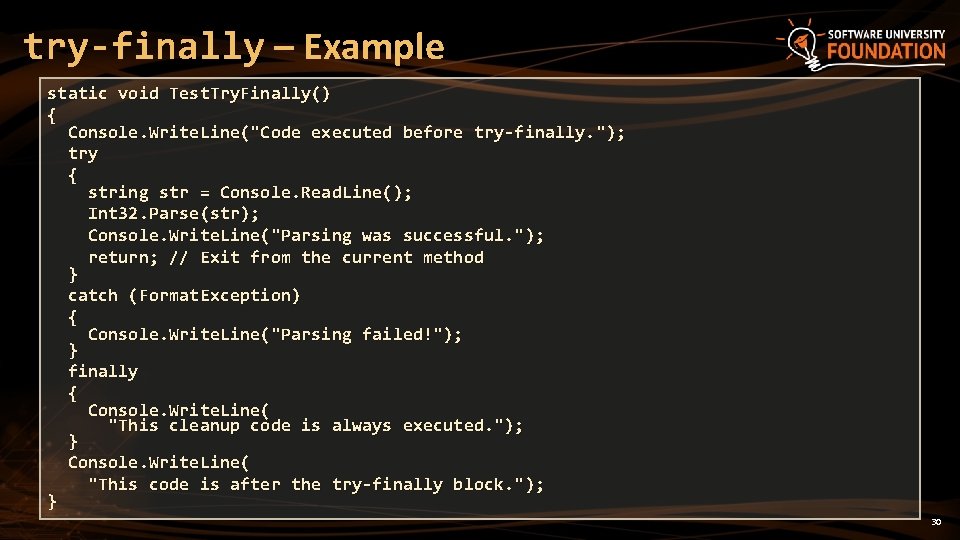
try-finally – Example static void Test. Try. Finally() { Console. Write. Line("Code executed before try-finally. "); try { string str = Console. Read. Line(); Int 32. Parse(str); Console. Write. Line("Parsing was successful. "); return; // Exit from the current method } catch (Format. Exception) { Console. Write. Line("Parsing failed!"); } finally { Console. Write. Line( "This cleanup code is always executed. "); } Console. Write. Line( "This code is after the try-finally block. "); } 30
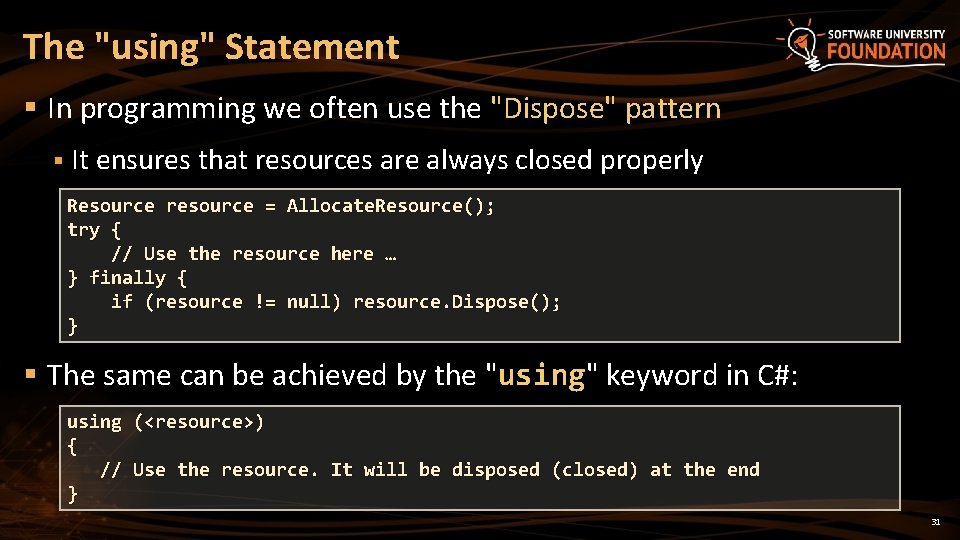
The "using" Statement § In programming we often use the "Dispose" pattern § It ensures that resources are always closed properly Resource resource = Allocate. Resource(); try { // Use the resource here … } finally { if (resource != null) resource. Dispose(); } § The same can be achieved by the "using" keyword in C#: using (<resource>) { // Use the resource. It will be disposed (closed) at the end } 31
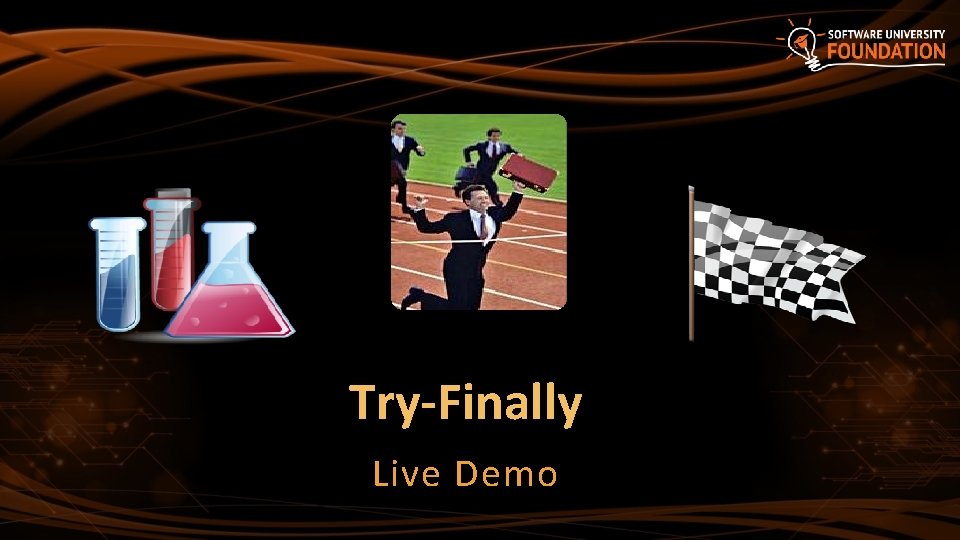
Try-Finally Live Demo
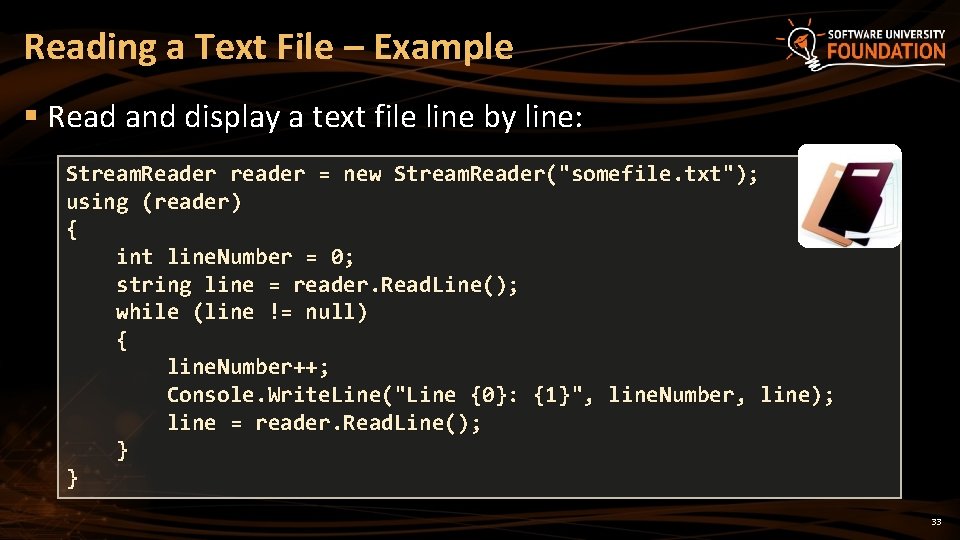
Reading a Text File – Example § Read and display a text file line by line: Stream. Reader reader = new Stream. Reader("somefile. txt"); using (reader) { int line. Number = 0; string line = reader. Read. Line(); while (line != null) { line. Number++; Console. Write. Line("Line {0}: {1}", line. Number, line); line = reader. Read. Line(); } } 33
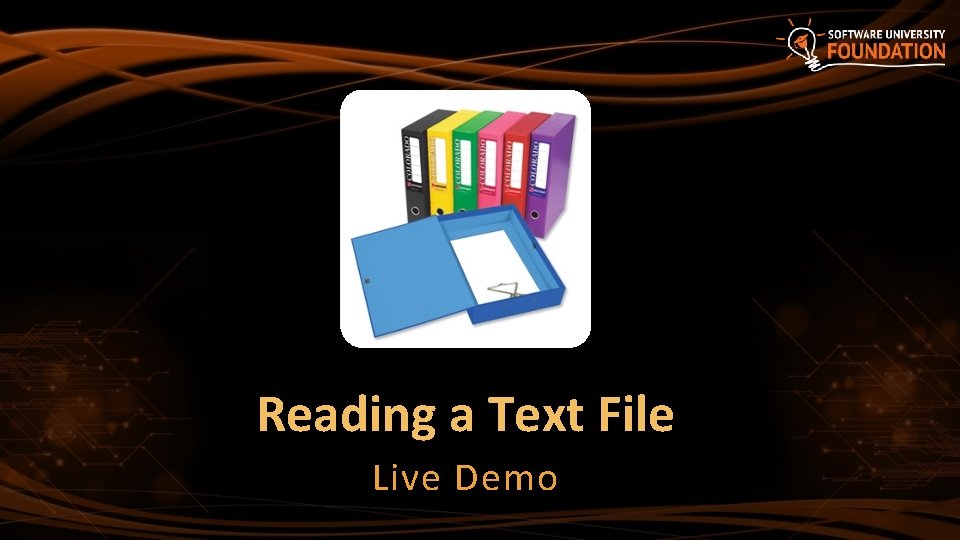
Reading a Text File Live Demo
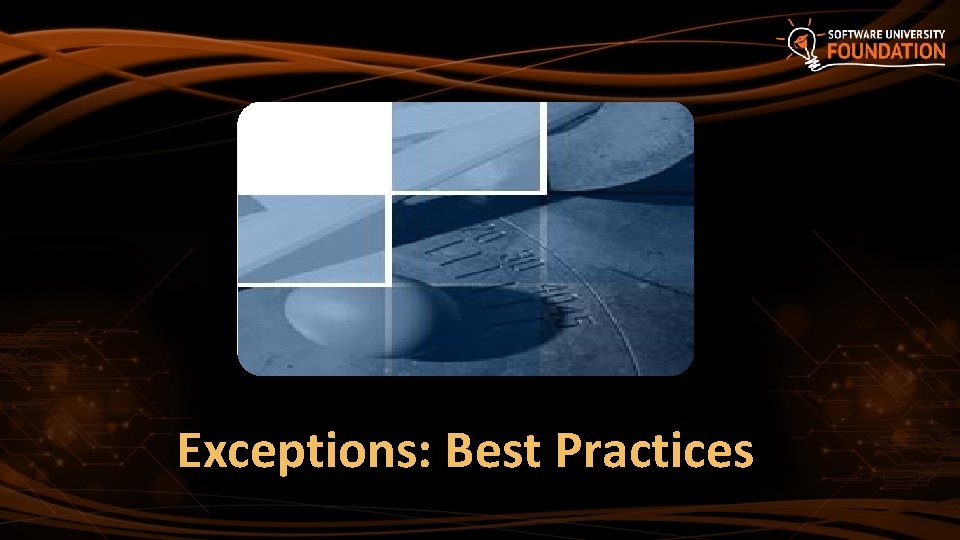
Exceptions: Best Practices
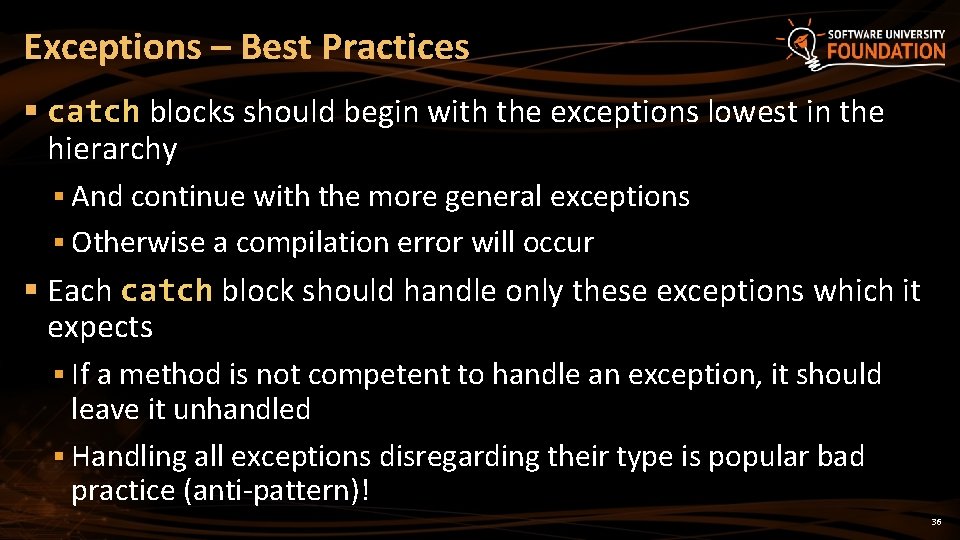
Exceptions – Best Practices § catch blocks should begin with the exceptions lowest in the hierarchy § And continue with the more general exceptions § Otherwise a compilation error will occur § Each catch block should handle only these exceptions which it expects § If a method is not competent to handle an exception, it should leave it unhandled § Handling all exceptions disregarding their type is popular bad practice (anti-pattern)! 36
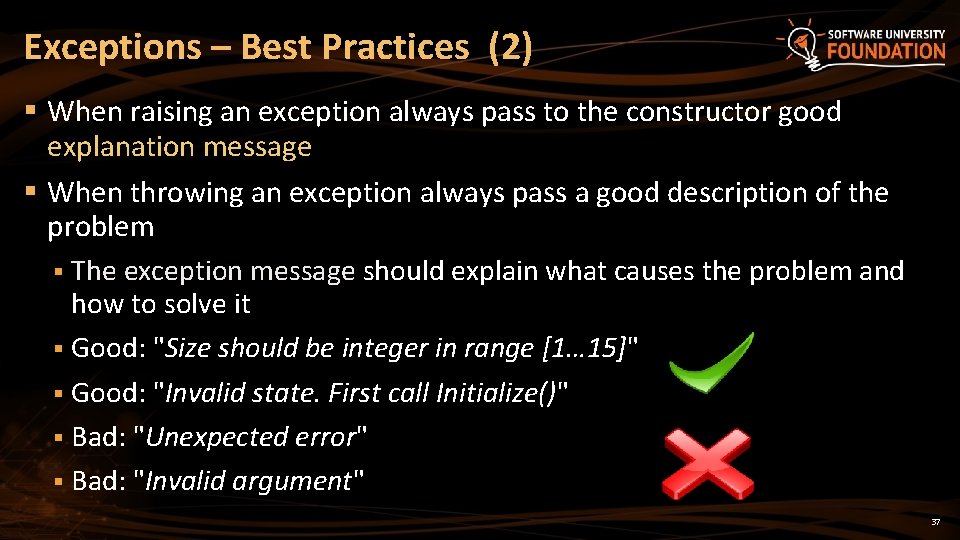
Exceptions – Best Practices (2) § When raising an exception always pass to the constructor good explanation message § When throwing an exception always pass a good description of the problem § The exception message should explain what causes the problem and how to solve it § Good: "Size should be integer in range [1… 15]" § Good: "Invalid state. First call Initialize()" § Bad: "Unexpected error" § Bad: "Invalid argument" 37
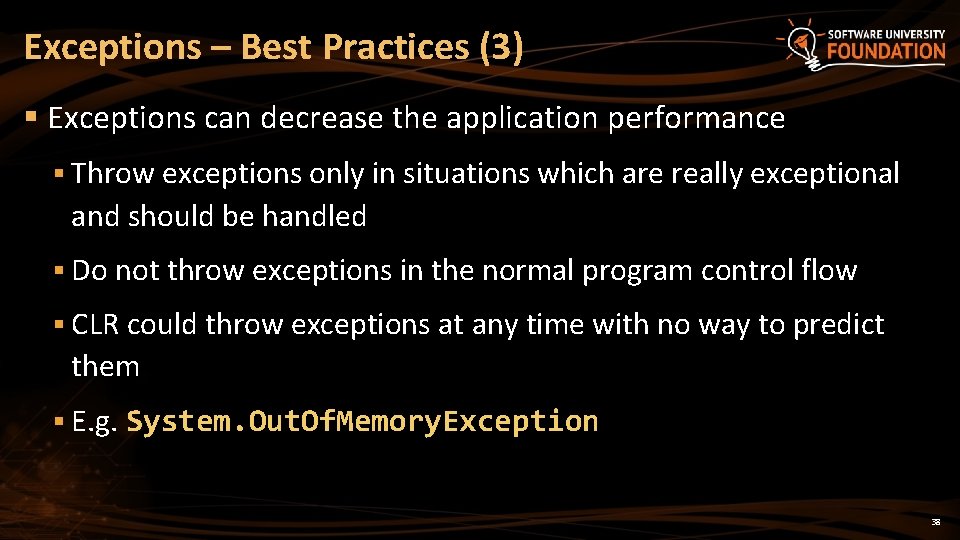
Exceptions – Best Practices (3) § Exceptions can decrease the application performance § Throw exceptions only in situations which are really exceptional and should be handled § Do not throw exceptions in the normal program control flow § CLR could throw exceptions at any time with no way to predict them § E. g. System. Out. Of. Memory. Exception 38
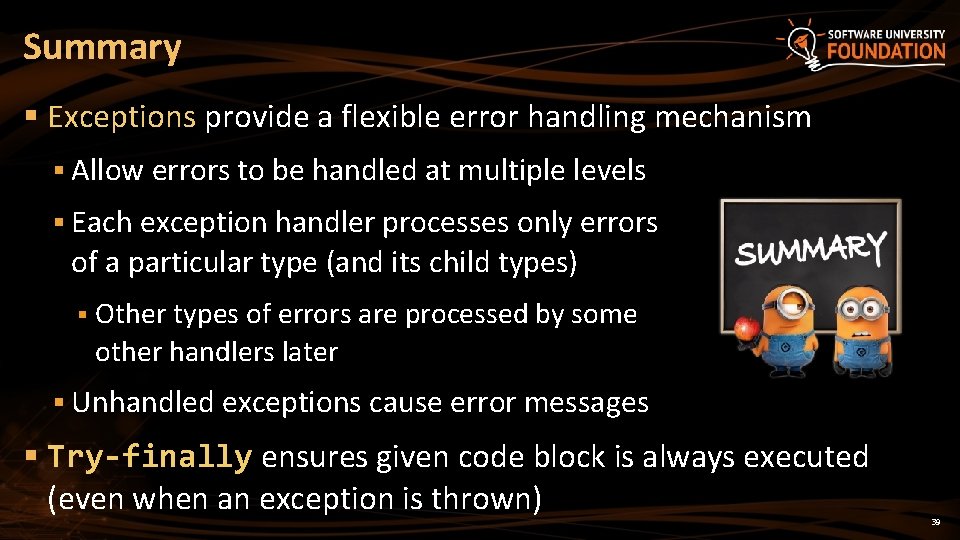
Summary § Exceptions provide a flexible error handling mechanism § Allow errors to be handled at multiple levels § Each exception handler processes only errors of a particular type (and its child types) § Other types of errors are processed by some other handlers later § Unhandled exceptions cause error messages § Try-finally ensures given code block is always executed (even when an exception is thrown) 39
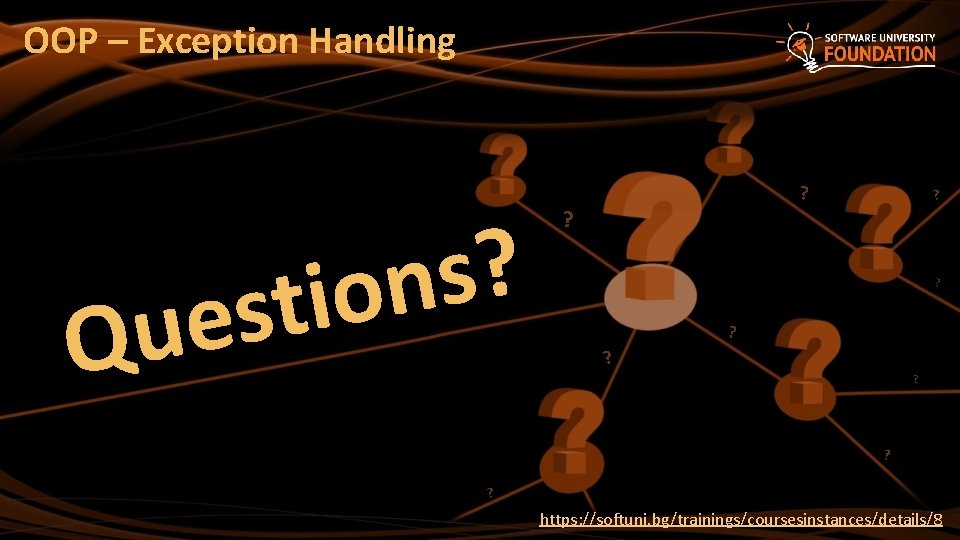
OOP – Exception Handling ? s n o i t s e u Q ? ? ? https: //softuni. bg/trainings/coursesinstances/details/8
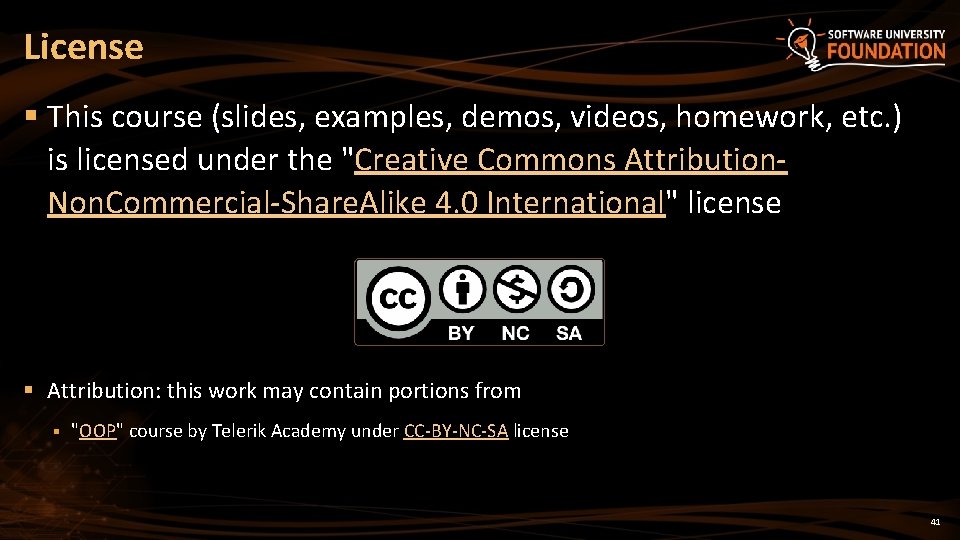
License § This course (slides, examples, demos, videos, homework, etc. ) is licensed under the "Creative Commons Attribution. Non. Commercial-Share. Alike 4. 0 International" license § Attribution: this work may contain portions from § "OOP" course by Telerik Academy under CC-BY-NC-SA license 41
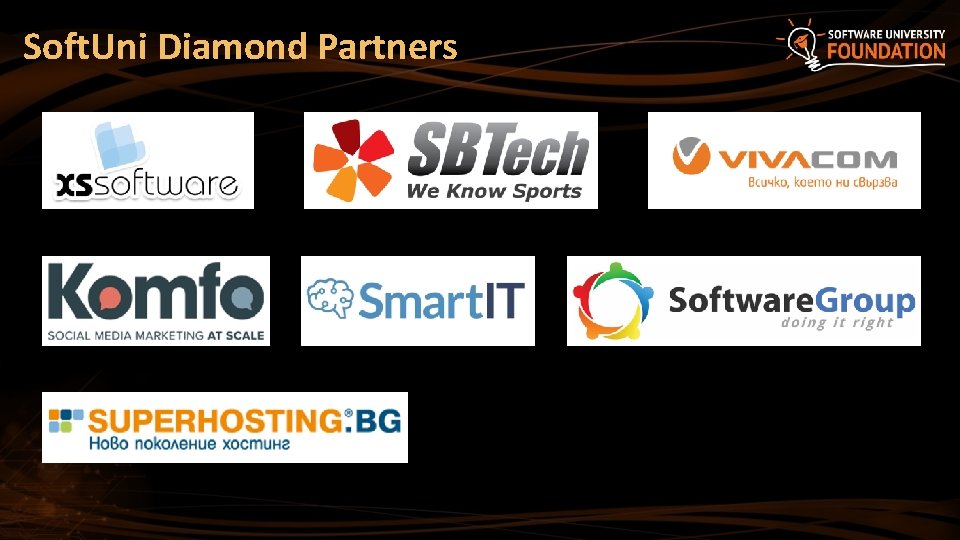
Soft. Uni Diamond Partners
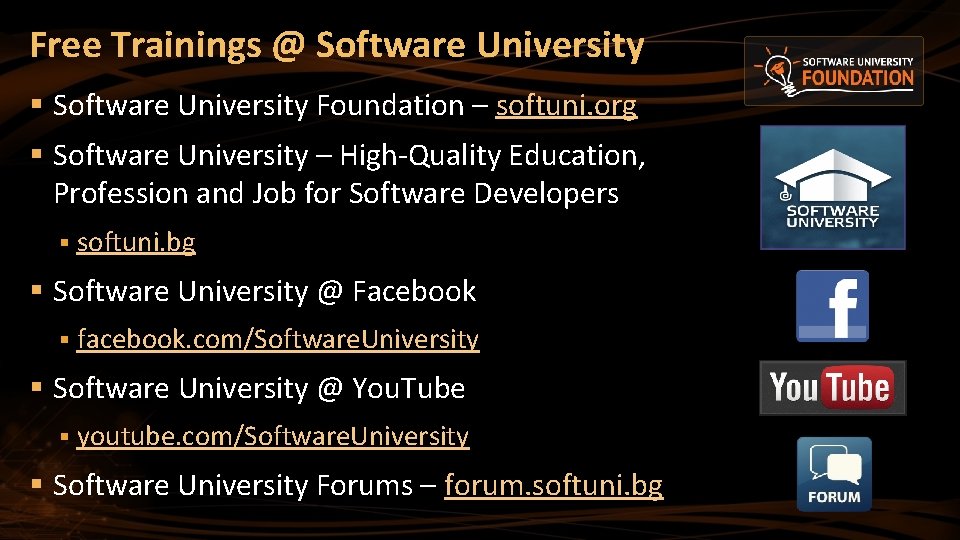
Free Trainings @ Software University § Software University Foundation – softuni. org § Software University – High-Quality Education, Profession and Job for Software Developers § softuni. bg § Software University @ Facebook § facebook. com/Software. University § Software University @ You. Tube § youtube. com/Software. University § Software University Forums – forum. softuni. bg