Exception Handling Control Structure Overview l Exception Handling
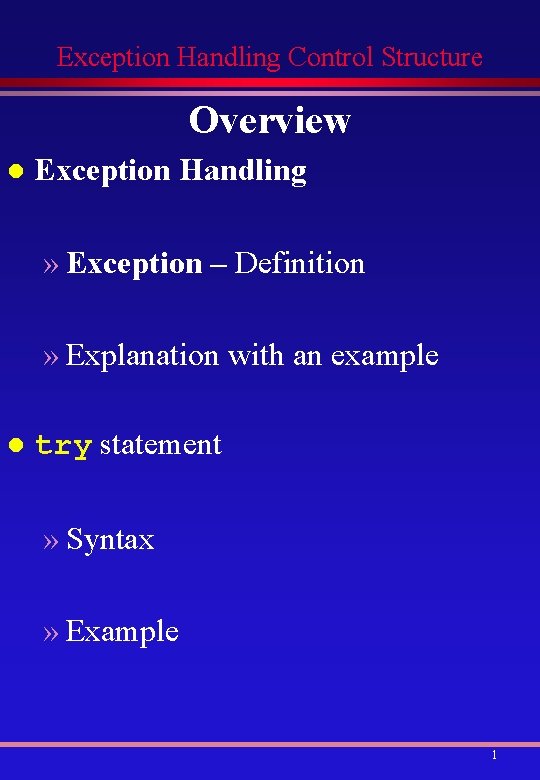
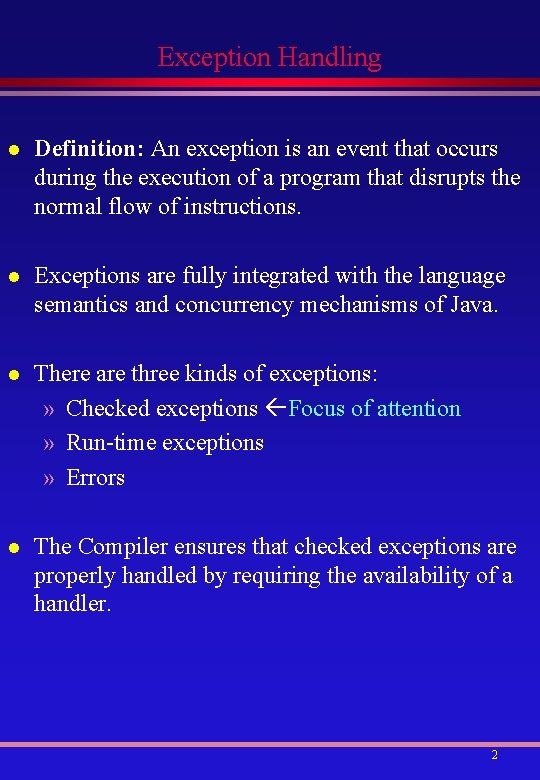
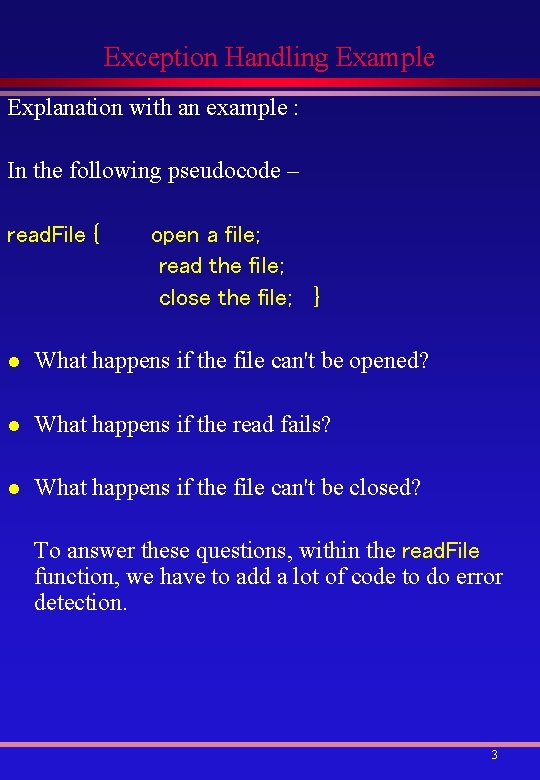
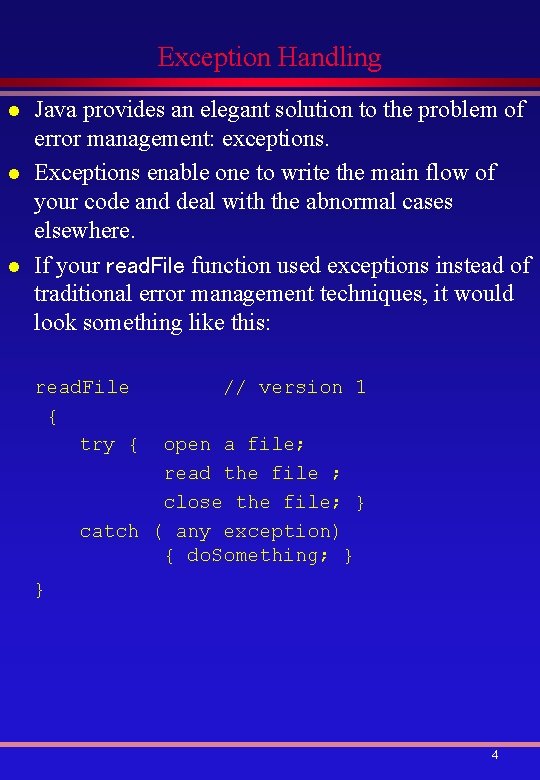
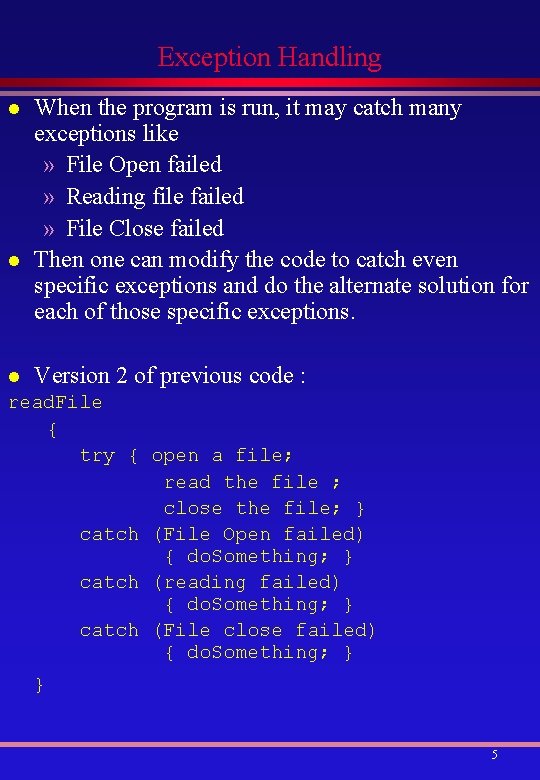
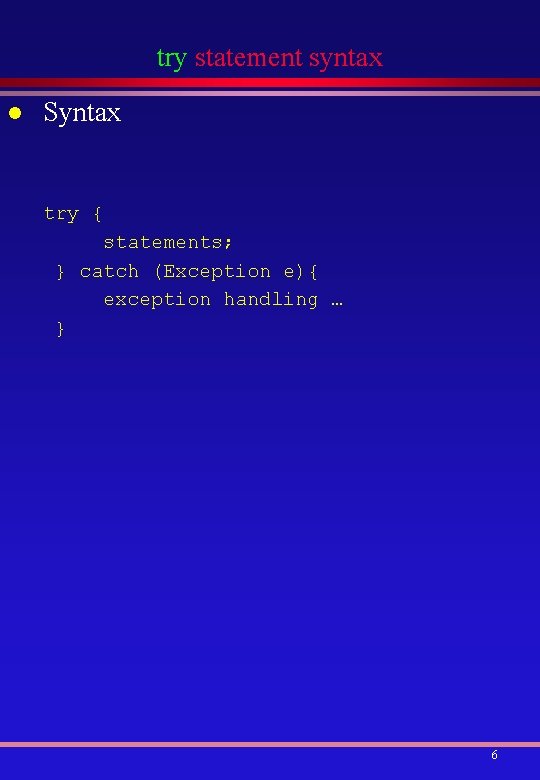
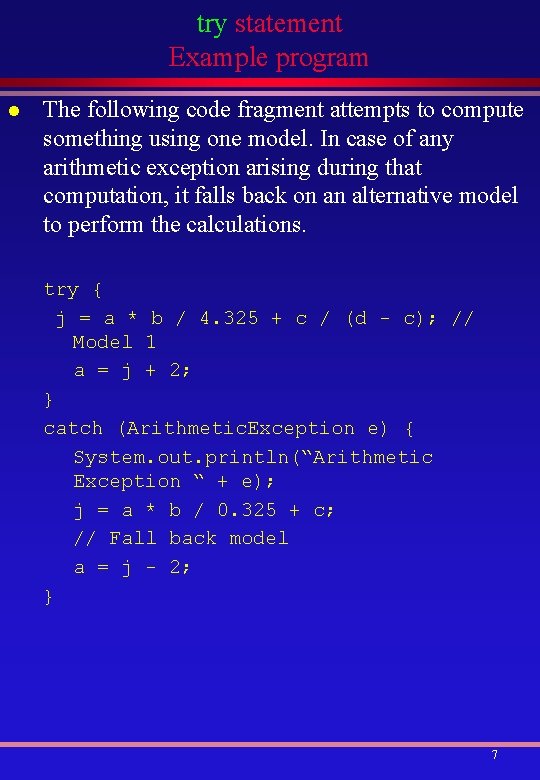
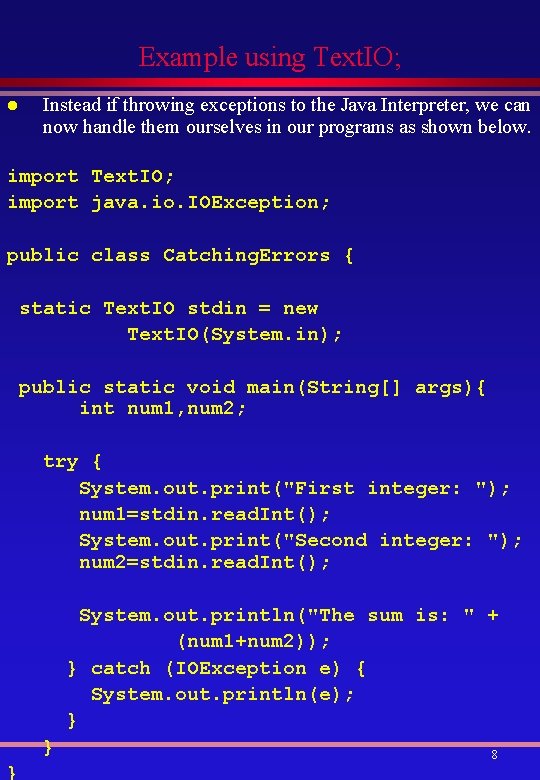
- Slides: 8
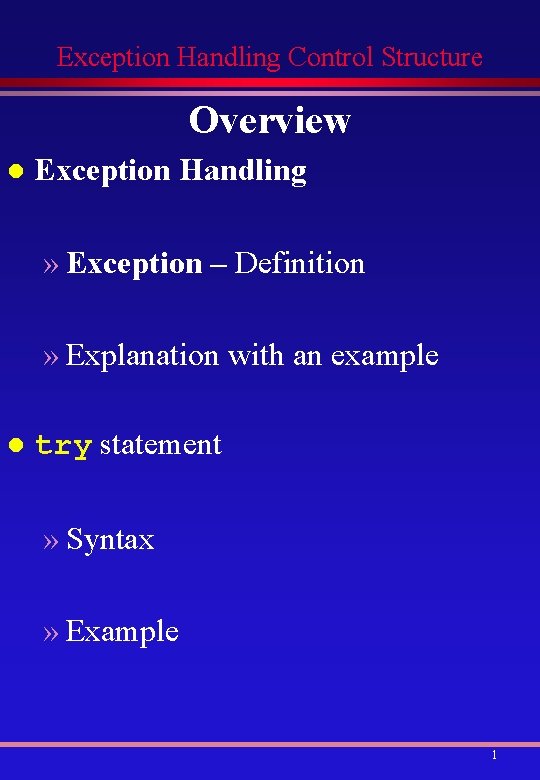
Exception Handling Control Structure Overview l Exception Handling » Exception – Definition » Explanation with an example l try statement » Syntax » Example 1
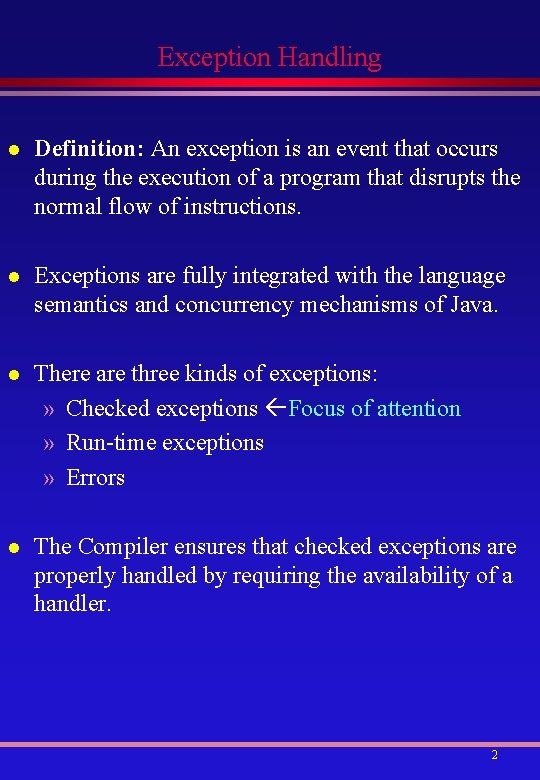
Exception Handling l Definition: An exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. l Exceptions are fully integrated with the language semantics and concurrency mechanisms of Java. l There are three kinds of exceptions: » Checked exceptions Focus of attention » Run-time exceptions » Errors l The Compiler ensures that checked exceptions are properly handled by requiring the availability of a handler. 2
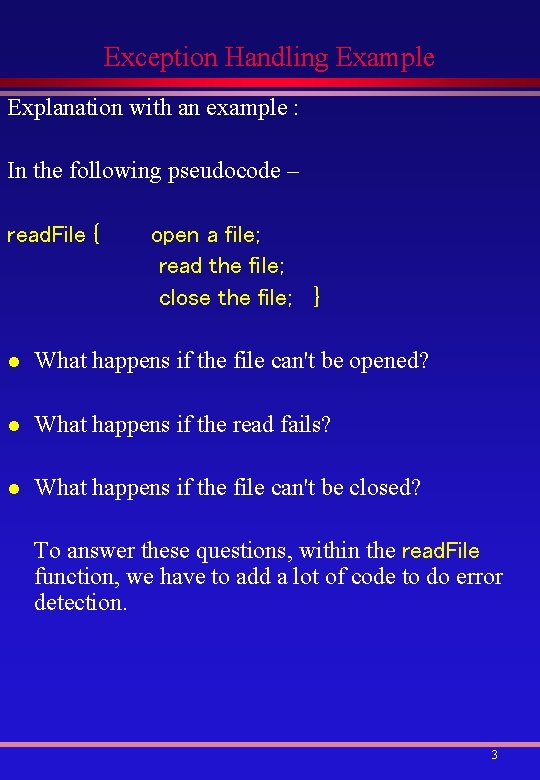
Exception Handling Example Explanation with an example : In the following pseudocode – read. File { open a file; read the file; close the file; } l What happens if the file can't be opened? l What happens if the read fails? l What happens if the file can't be closed? To answer these questions, within the read. File function, we have to add a lot of code to do error detection. 3
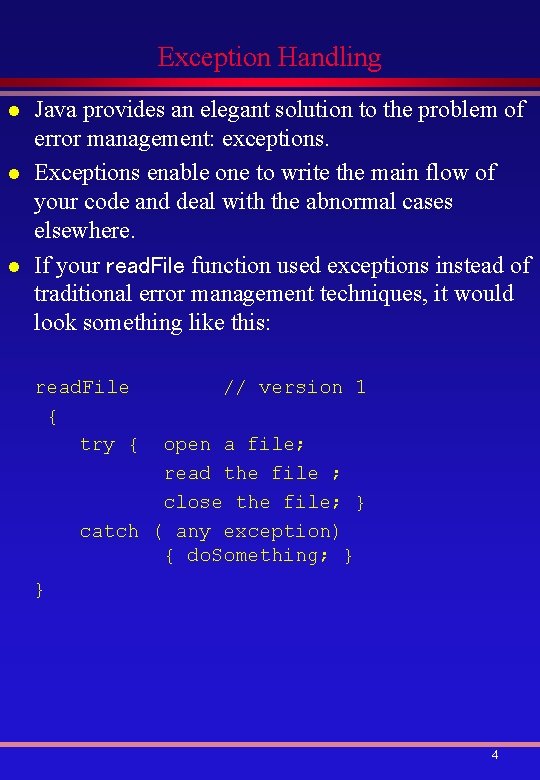
Exception Handling l l l Java provides an elegant solution to the problem of error management: exceptions. Exceptions enable one to write the main flow of your code and deal with the abnormal cases elsewhere. If your read. File function used exceptions instead of traditional error management techniques, it would look something like this: read. File { try { // version 1 open a file; read the file ; close the file; } catch ( any exception) { do. Something; } } 4
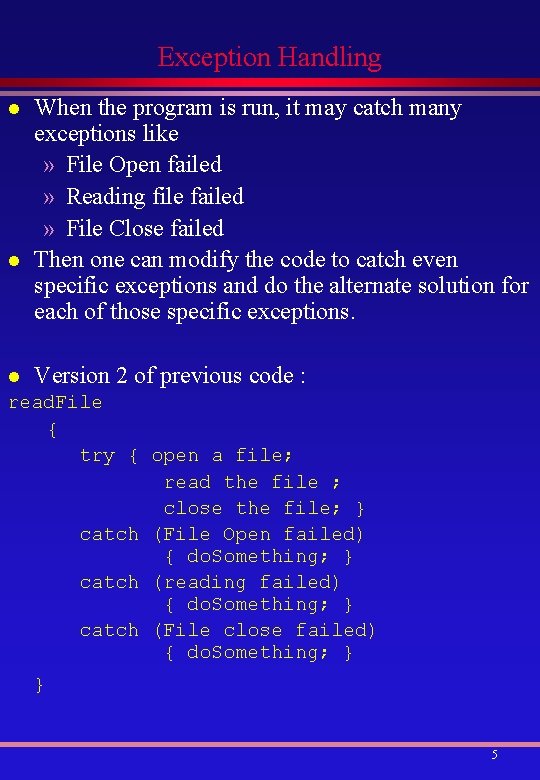
Exception Handling l l l When the program is run, it may catch many exceptions like » File Open failed » Reading file failed » File Close failed Then one can modify the code to catch even specific exceptions and do the alternate solution for each of those specific exceptions. Version 2 of previous code : read. File { try { open a file; read the file ; close the file; } catch (File Open failed) { do. Something; } catch (reading failed) { do. Something; } catch (File close failed) { do. Something; } } 5
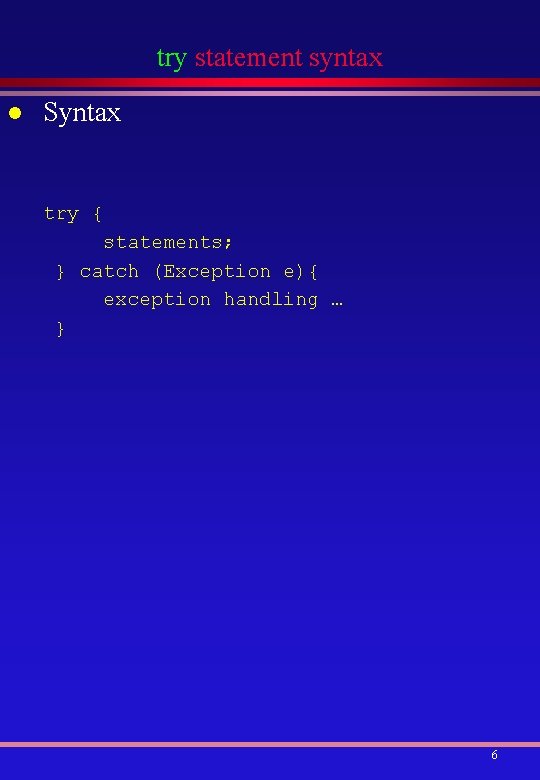
try statement syntax l Syntax try { statements; } catch (Exception e){ exception handling … } 6
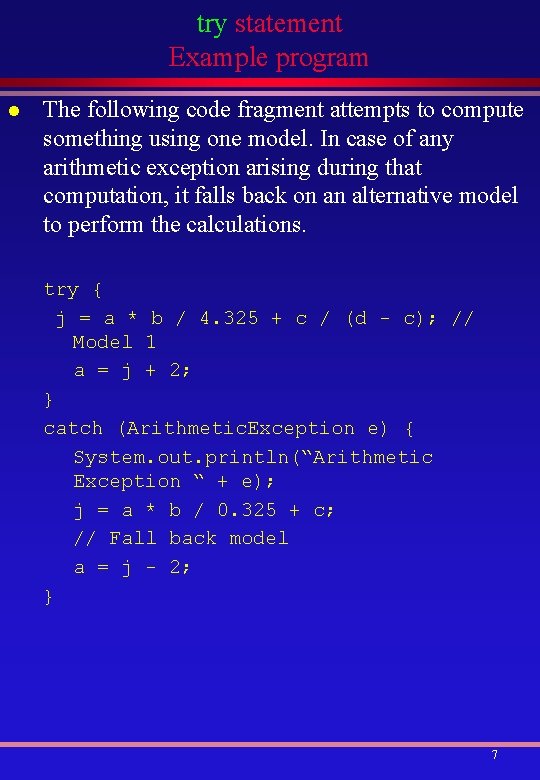
try statement Example program l The following code fragment attempts to compute something using one model. In case of any arithmetic exception arising during that computation, it falls back on an alternative model to perform the calculations. try { j = a * b / 4. 325 + c / (d - c); // Model 1 a = j + 2; } catch (Arithmetic. Exception e) { System. out. println(“Arithmetic Exception “ + e); j = a * b / 0. 325 + c; // Fall back model a = j - 2; } 7
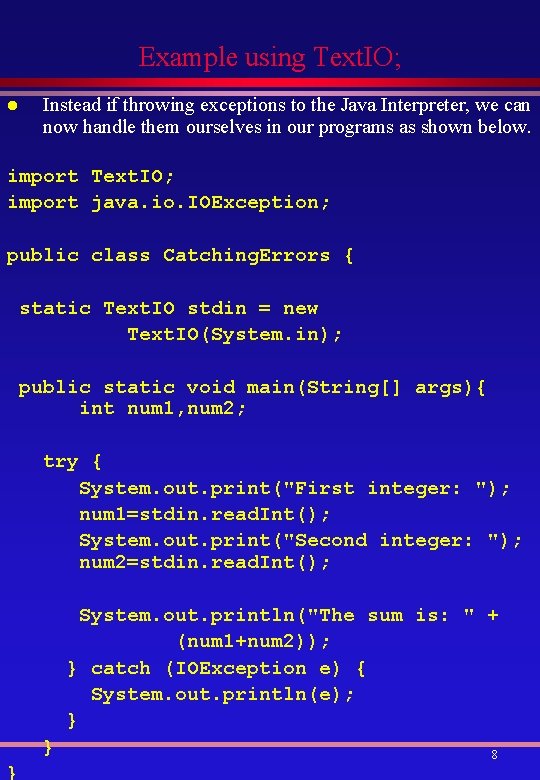
Example using Text. IO; Instead if throwing exceptions to the Java Interpreter, we can now handle them ourselves in our programs as shown below. l import Text. IO; import java. io. IOException; public class Catching. Errors { static Text. IO stdin = new Text. IO(System. in); public static void main(String[] args){ int num 1, num 2; try { System. out. print("First integer: "); num 1=stdin. read. Int(); System. out. print("Second integer: "); num 2=stdin. read. Int(); System. out. println("The sum is: " + (num 1+num 2)); } catch (IOException e) { System. out. println(e); } } } 8