Example Using Functions Using TopDown Design and Functions
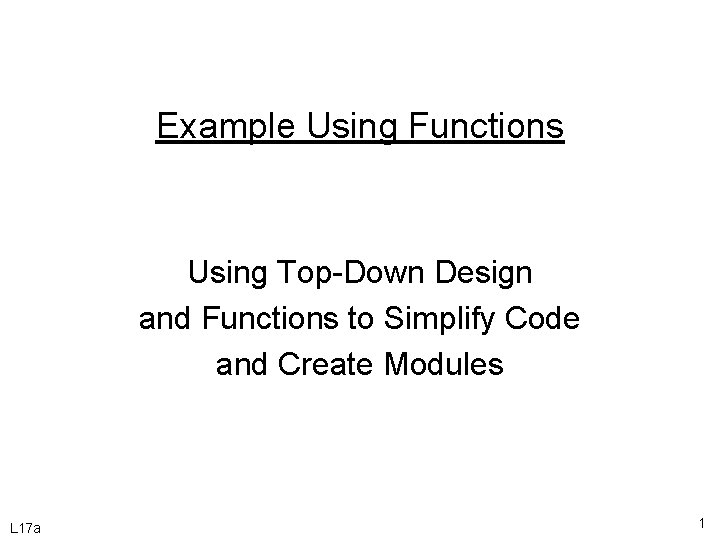
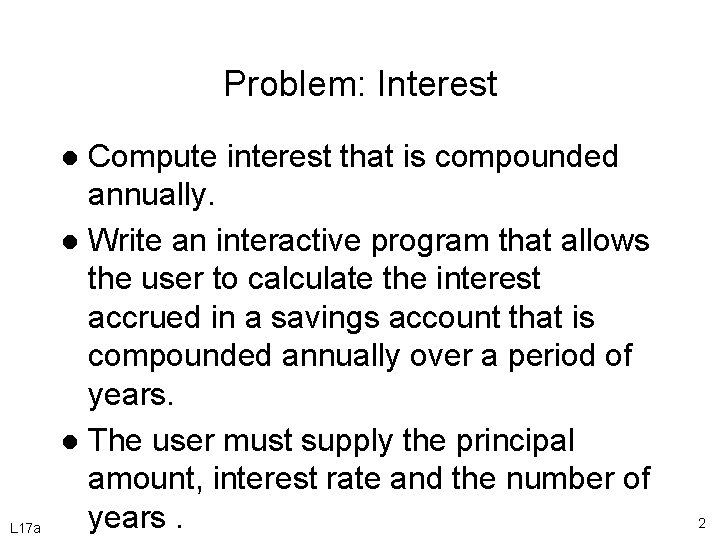
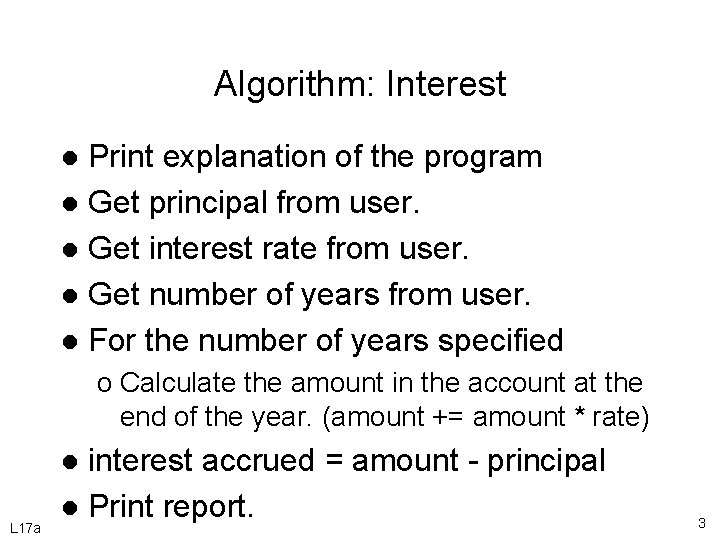
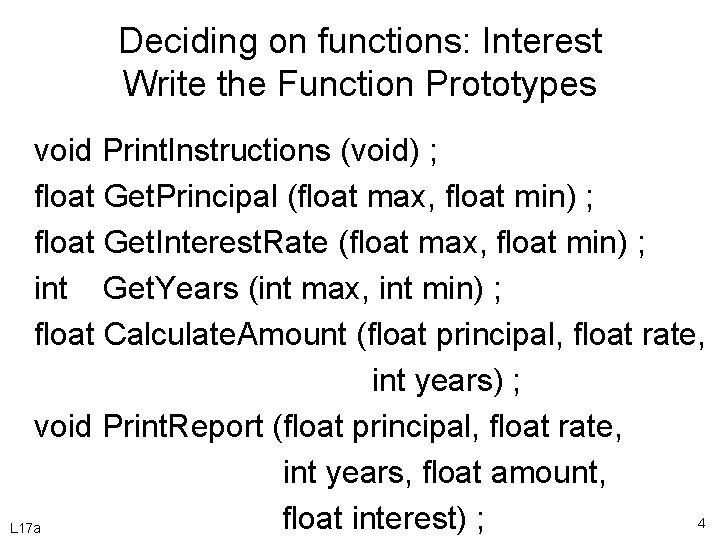
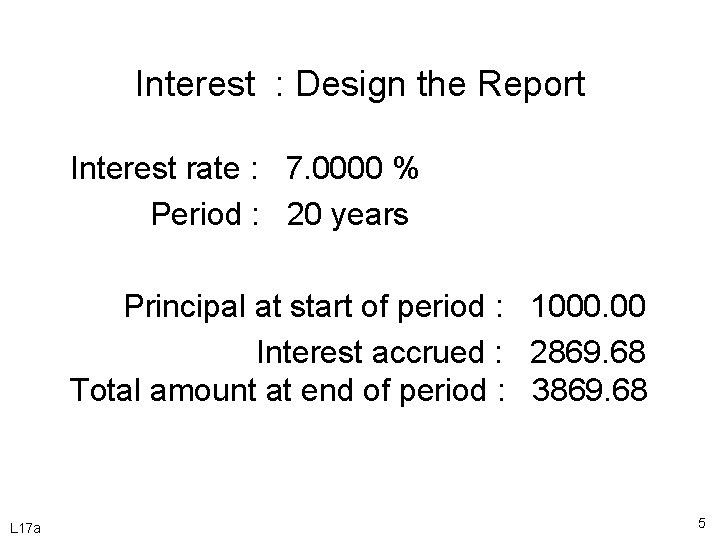
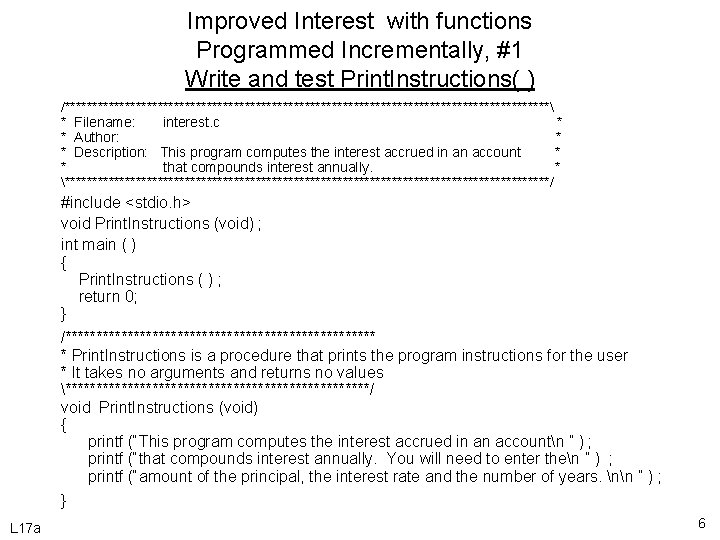
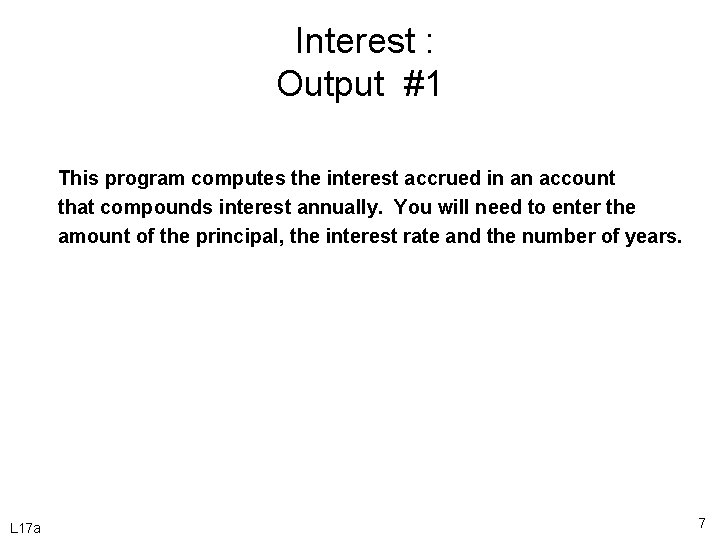
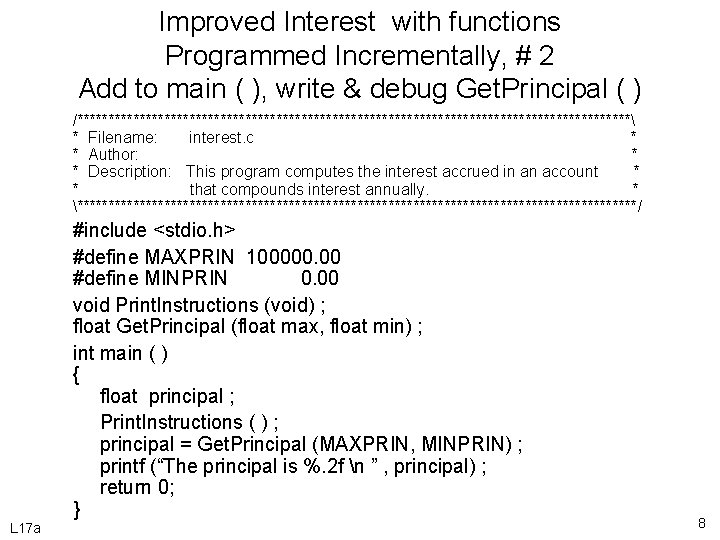
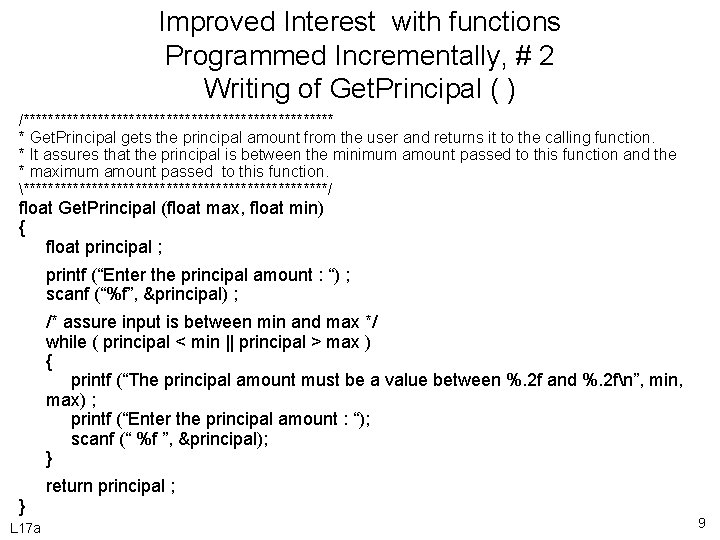
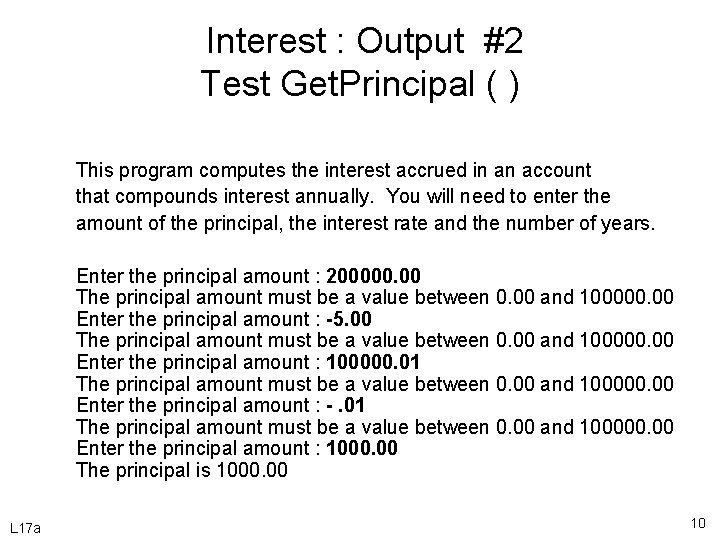
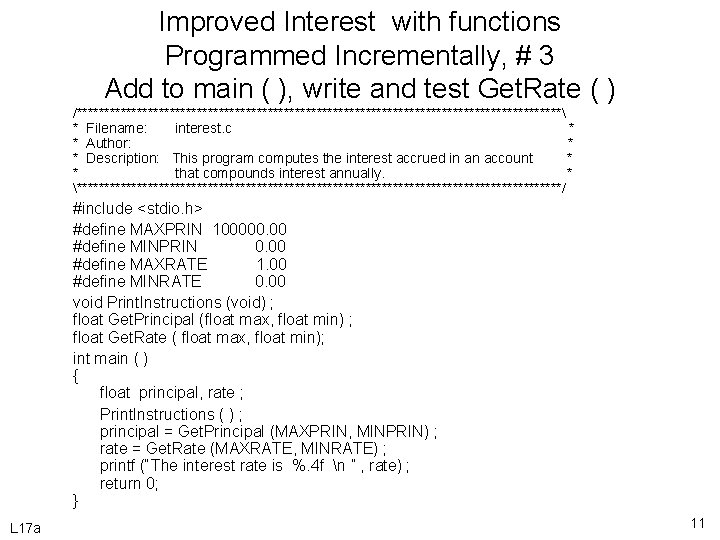
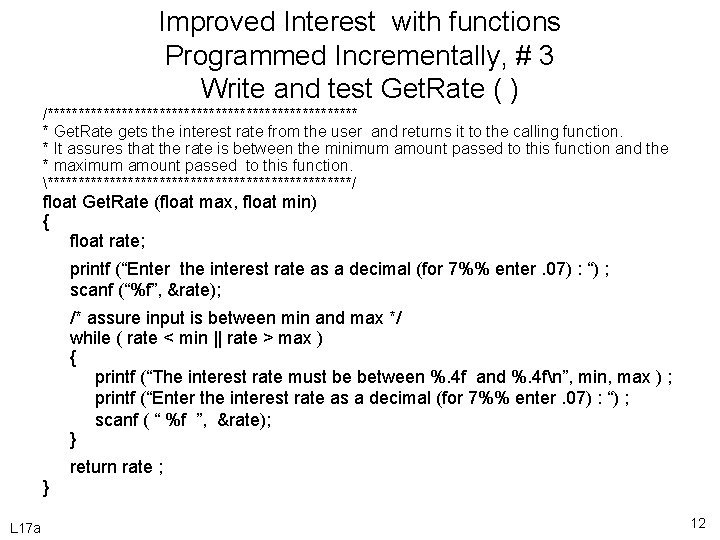
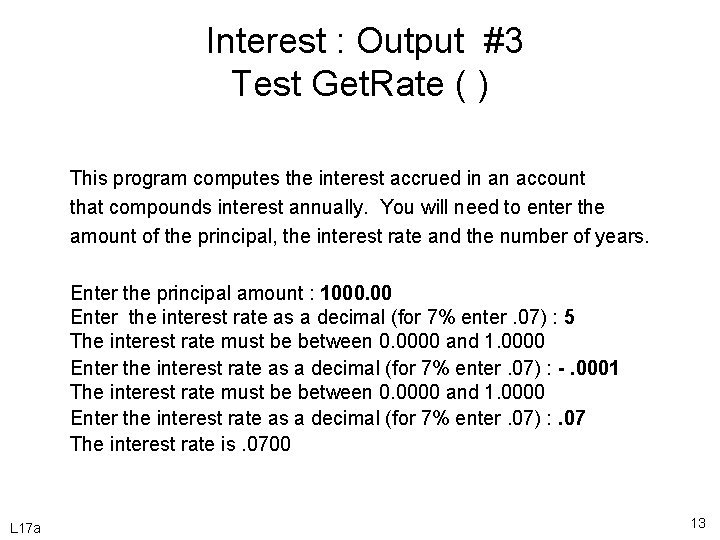
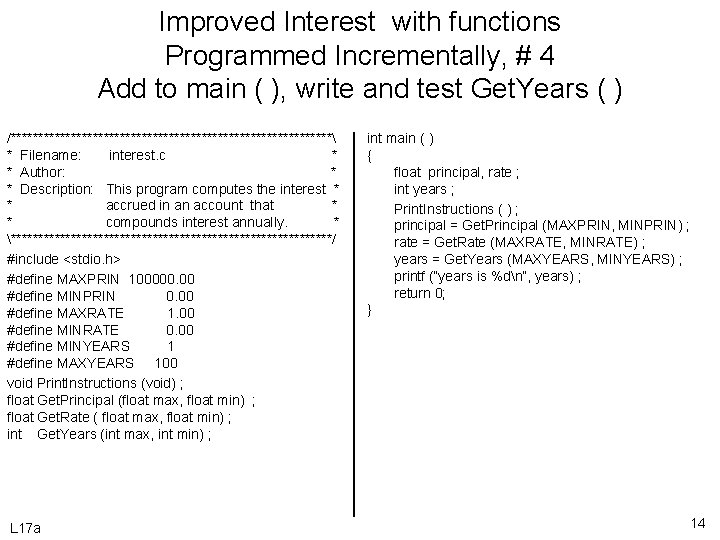
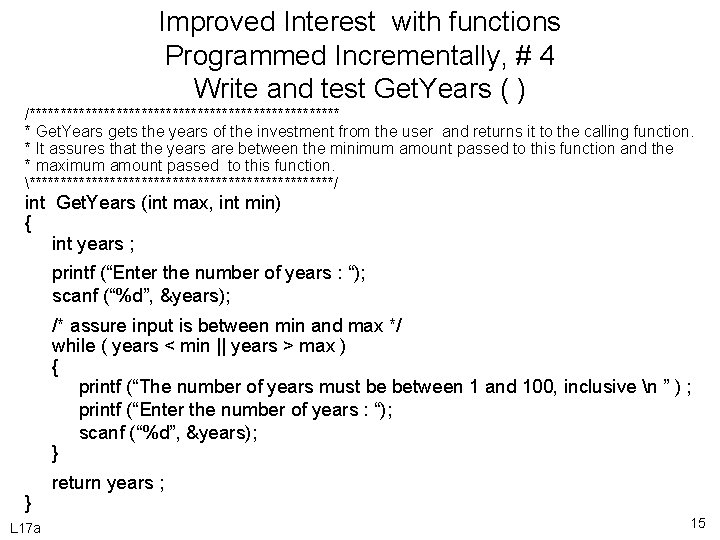
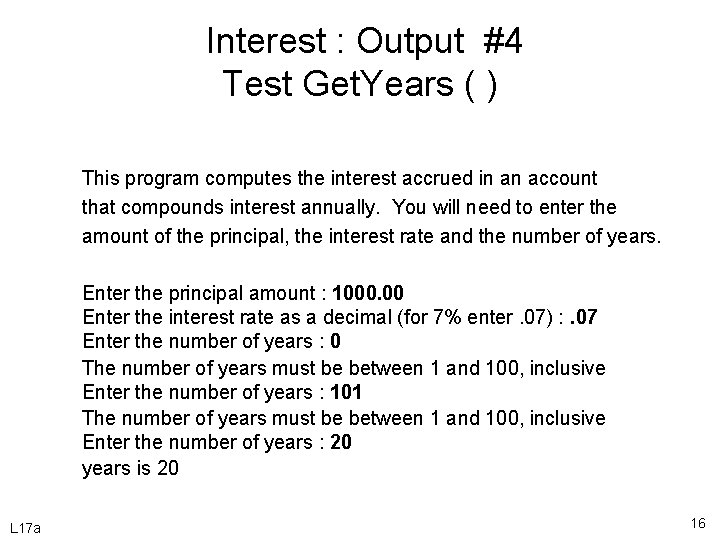
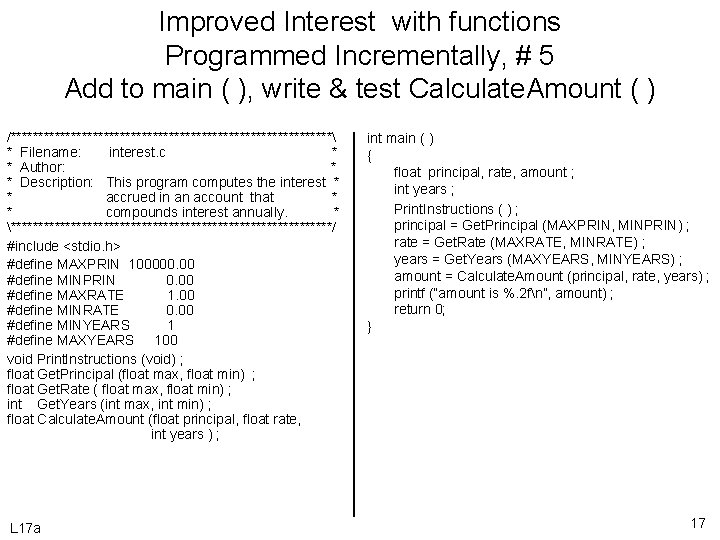
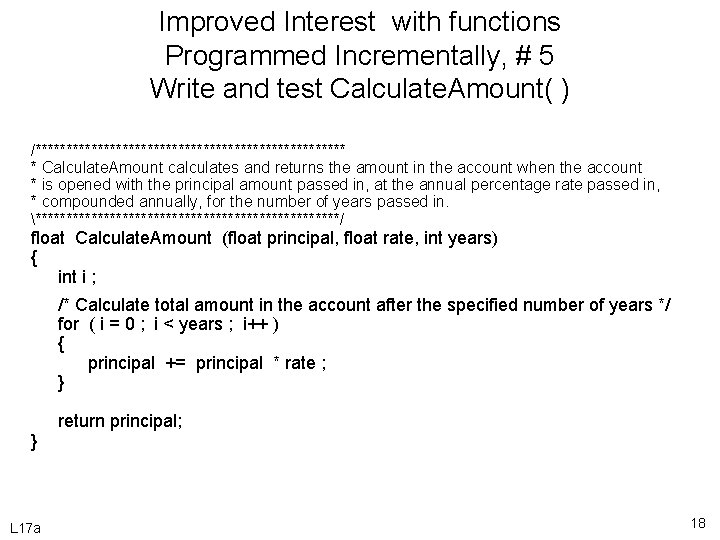
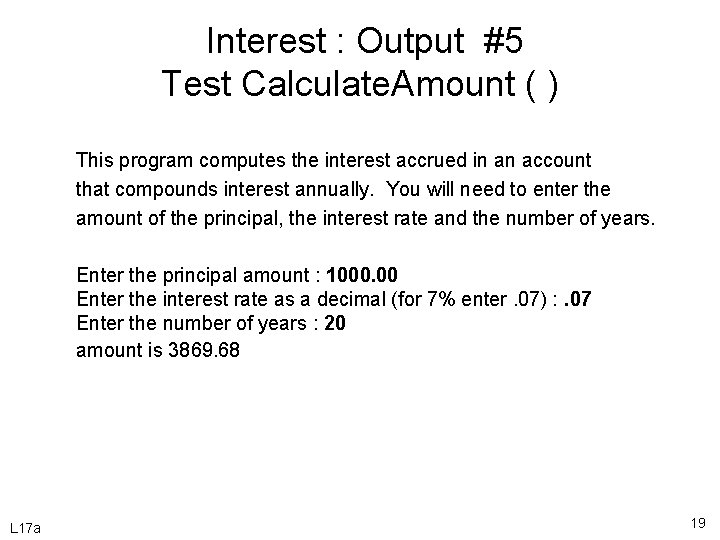
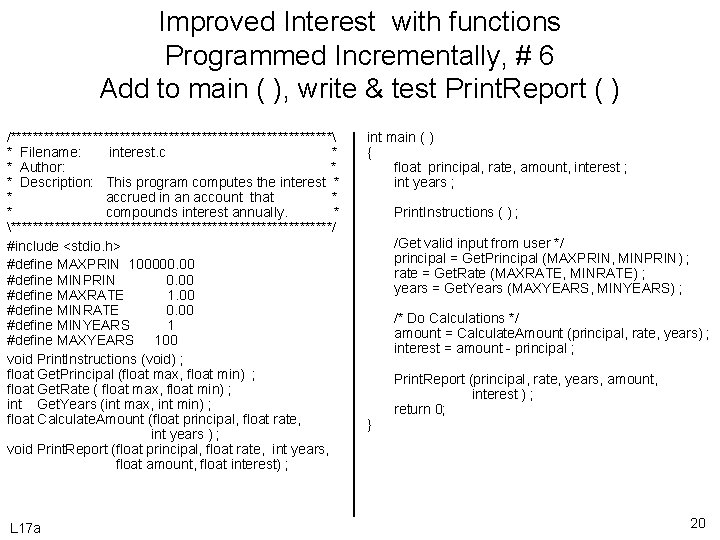
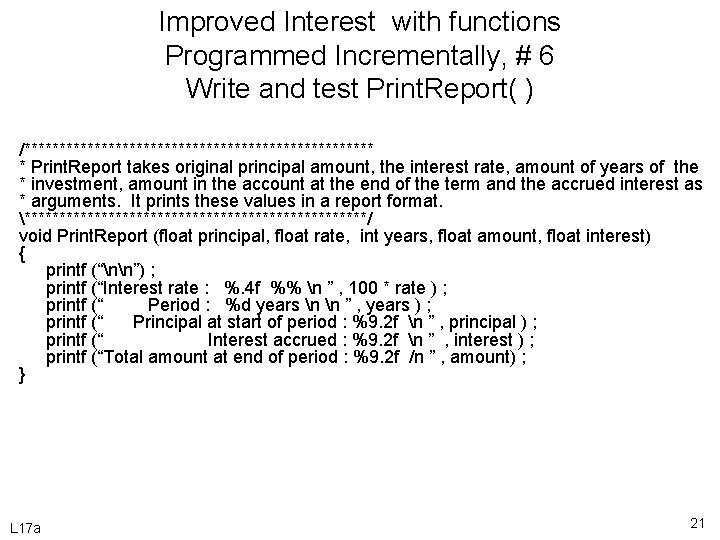
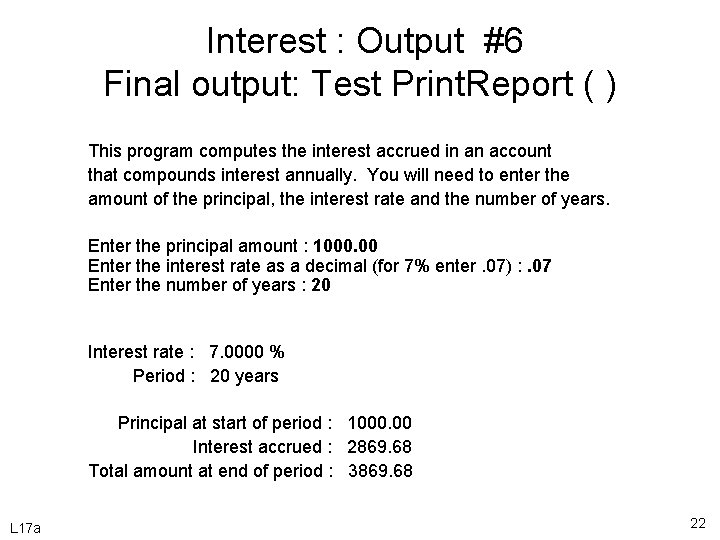
- Slides: 22
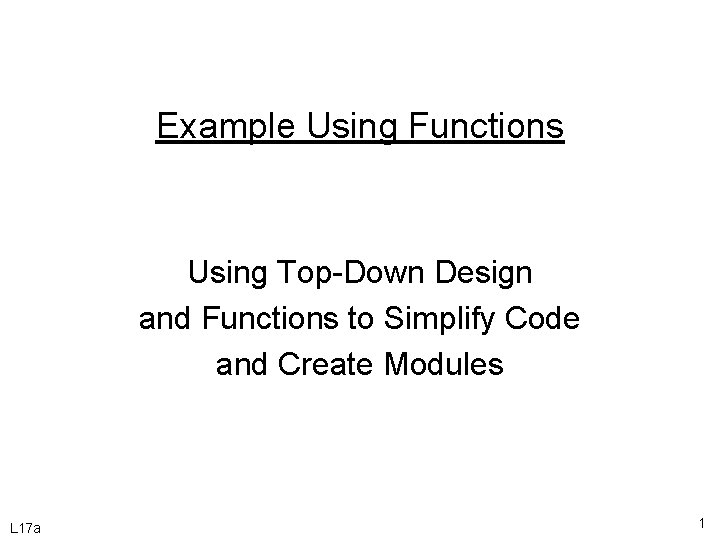
Example Using Functions Using Top-Down Design and Functions to Simplify Code and Create Modules L 17 a 1
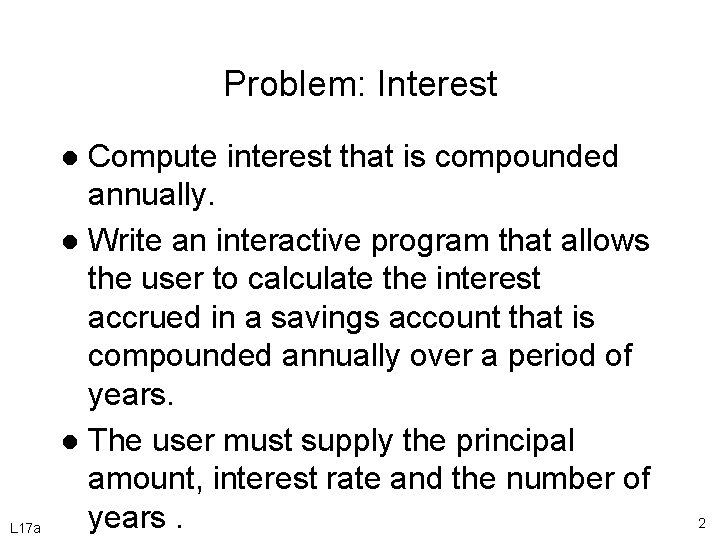
Problem: Interest Compute interest that is compounded annually. l Write an interactive program that allows the user to calculate the interest accrued in a savings account that is compounded annually over a period of years. l The user must supply the principal amount, interest rate and the number of years. l L 17 a 2
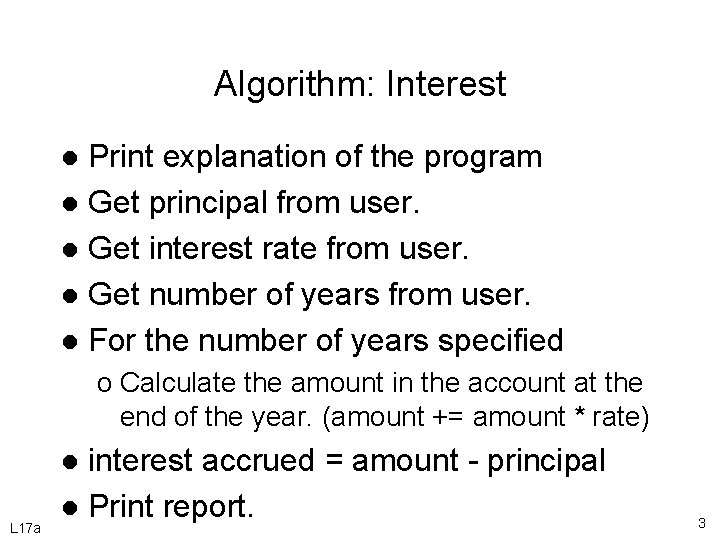
Algorithm: Interest Print explanation of the program l Get principal from user. l Get interest rate from user. l Get number of years from user. l For the number of years specified l o Calculate the amount in the account at the end of the year. (amount += amount * rate) interest accrued = amount - principal l Print report. l L 17 a 3
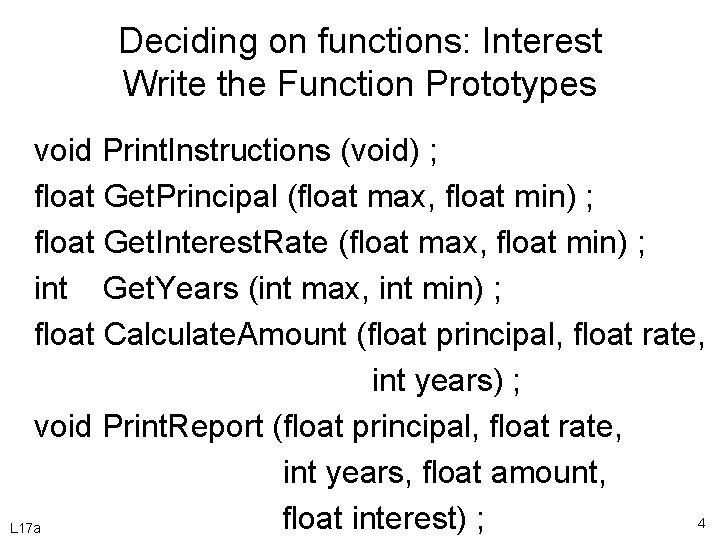
Deciding on functions: Interest Write the Function Prototypes void Print. Instructions (void) ; float Get. Principal (float max, float min) ; float Get. Interest. Rate (float max, float min) ; int Get. Years (int max, int min) ; float Calculate. Amount (float principal, float rate, int years) ; void Print. Report (float principal, float rate, int years, float amount, 4 float interest) ; L 17 a
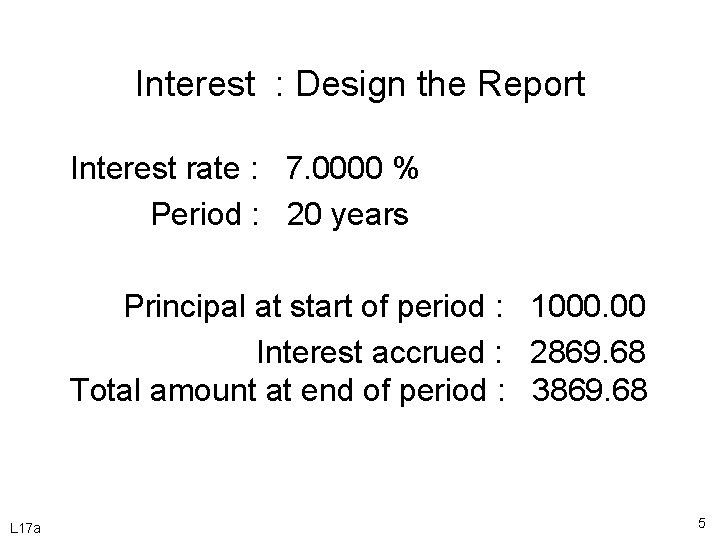
Interest : Design the Report Interest rate : 7. 0000 % Period : 20 years Principal at start of period : 1000. 00 Interest accrued : 2869. 68 Total amount at end of period : 3869. 68 L 17 a 5
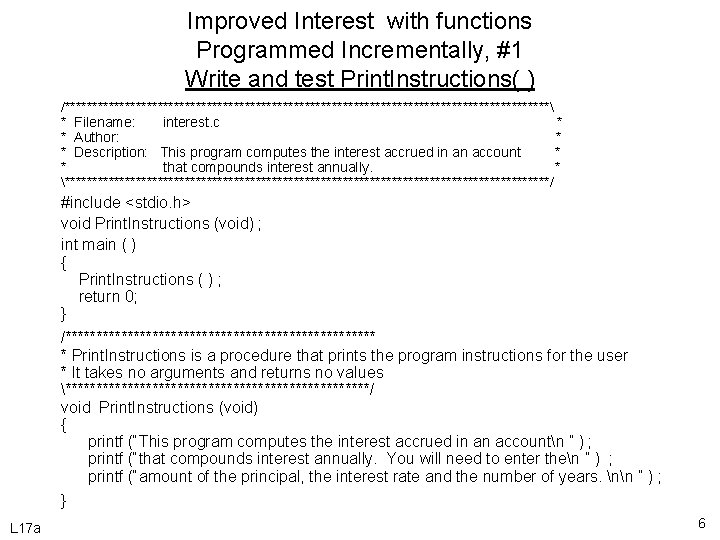
Improved Interest with functions Programmed Incrementally, #1 Write and test Print. Instructions( ) /********************************************* * Filename: interest. c * * Author: * * Description: This program computes the interest accrued in an account * * that compounds interest annually. * *********************************************/ #include <stdio. h> void Print. Instructions (void) ; int main ( ) { Print. Instructions ( ) ; return 0; } /************************* * Print. Instructions is a procedure that prints the program instructions for the user * It takes no arguments and returns no values *************************/ void Print. Instructions (void) { printf (“This program computes the interest accrued in an accountn ” ) ; printf (“that compounds interest annually. You will need to enter then ” ) ; printf (“amount of the principal, the interest rate and the number of years. nn ” ) ; } L 17 a 6
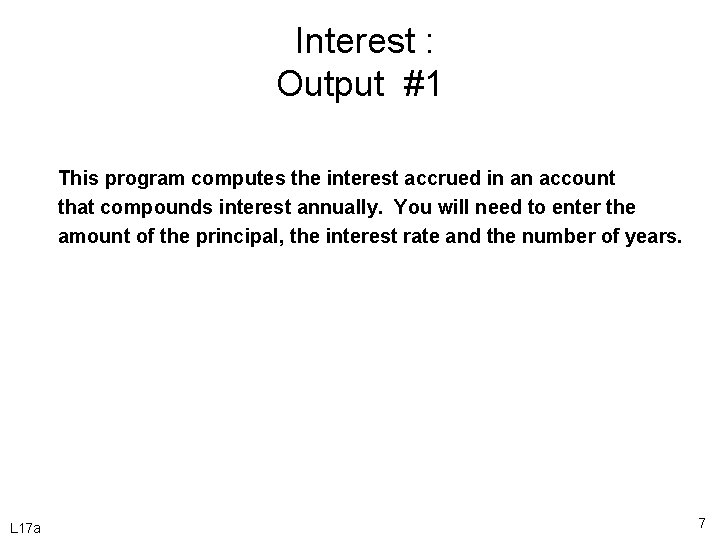
Interest : Output #1 This program computes the interest accrued in an account that compounds interest annually. You will need to enter the amount of the principal, the interest rate and the number of years. L 17 a 7
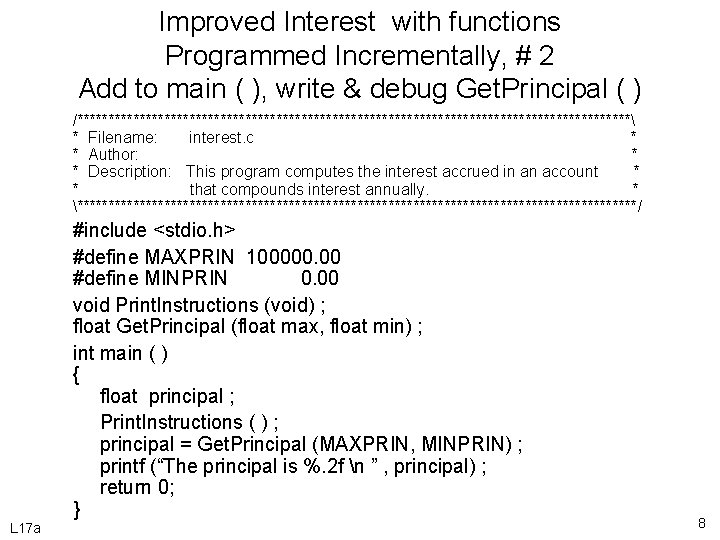
Improved Interest with functions Programmed Incrementally, # 2 Add to main ( ), write & debug Get. Principal ( ) /********************************************* * Filename: interest. c * * Author: * * Description: This program computes the interest accrued in an account * * that compounds interest annually. * *********************************************/ #include <stdio. h> #define MAXPRIN 100000. 00 #define MINPRIN 0. 00 void Print. Instructions (void) ; float Get. Principal (float max, float min) ; int main ( ) { float principal ; Print. Instructions ( ) ; principal = Get. Principal (MAXPRIN, MINPRIN) ; printf (“The principal is %. 2 f n ” , principal) ; return 0; } L 17 a 8
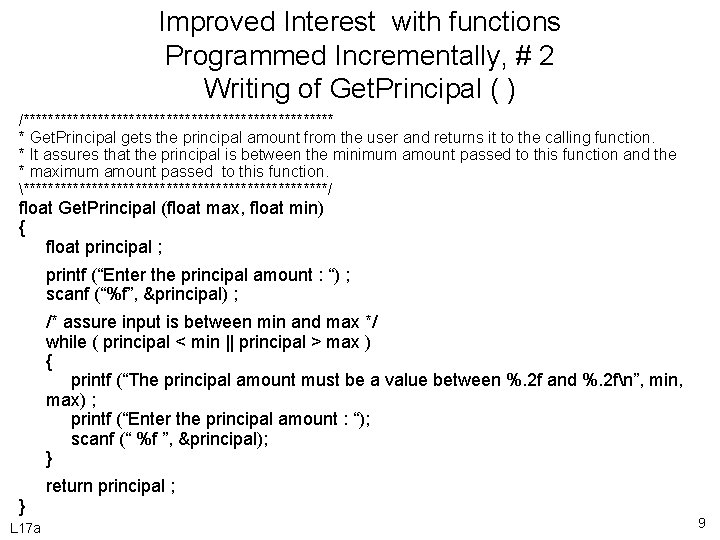
Improved Interest with functions Programmed Incrementally, # 2 Writing of Get. Principal ( ) /************************* * Get. Principal gets the principal amount from the user and returns it to the calling function. * It assures that the principal is between the minimum amount passed to this function and the * maximum amount passed to this function. *************************/ float Get. Principal (float max, float min) { float principal ; printf (“Enter the principal amount : “) ; scanf (“%f”, &principal) ; /* assure input is between min and max */ while ( principal < min || principal > max ) { printf (“The principal amount must be a value between %. 2 f and %. 2 fn”, min, max) ; printf (“Enter the principal amount : “); scanf (“ %f ”, &principal); } } L 17 a return principal ; 9
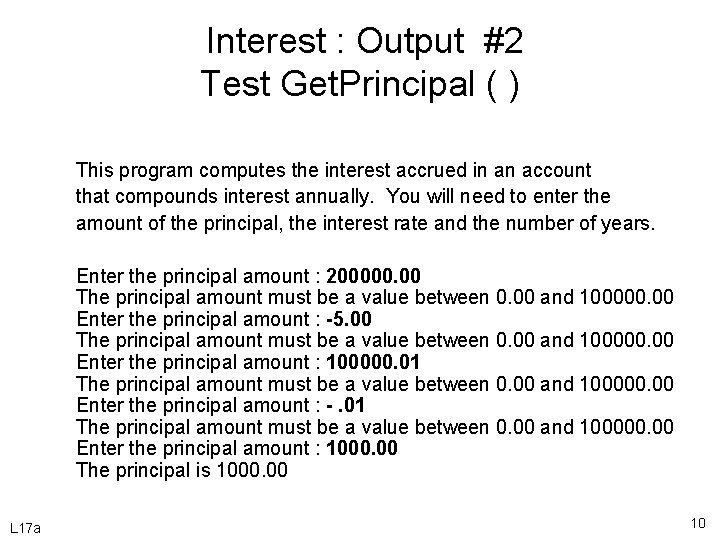
Interest : Output #2 Test Get. Principal ( ) This program computes the interest accrued in an account that compounds interest annually. You will need to enter the amount of the principal, the interest rate and the number of years. Enter the principal amount : 200000. 00 The principal amount must be a value between 0. 00 and 100000. 00 Enter the principal amount : -5. 00 The principal amount must be a value between 0. 00 and 100000. 00 Enter the principal amount : 100000. 01 The principal amount must be a value between 0. 00 and 100000. 00 Enter the principal amount : -. 01 The principal amount must be a value between 0. 00 and 100000. 00 Enter the principal amount : 1000. 00 The principal is 1000. 00 L 17 a 10
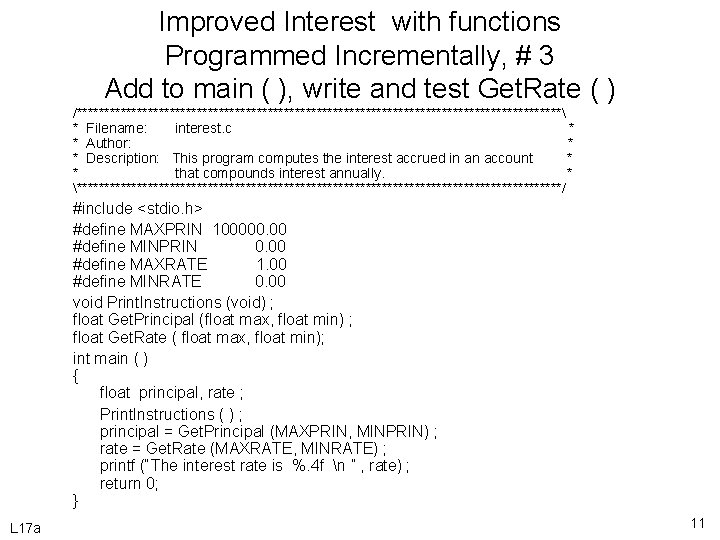
Improved Interest with functions Programmed Incrementally, # 3 Add to main ( ), write and test Get. Rate ( ) /********************************************* * Filename: interest. c * * Author: * * Description: This program computes the interest accrued in an account * * that compounds interest annually. * *********************************************/ #include <stdio. h> #define MAXPRIN 100000. 00 #define MINPRIN 0. 00 #define MAXRATE 1. 00 #define MINRATE 0. 00 void Print. Instructions (void) ; float Get. Principal (float max, float min) ; float Get. Rate ( float max, float min); int main ( ) { float principal, rate ; Print. Instructions ( ) ; principal = Get. Principal (MAXPRIN, MINPRIN) ; rate = Get. Rate (MAXRATE, MINRATE) ; printf (“The interest rate is %. 4 f n ” , rate) ; return 0; } L 17 a 11
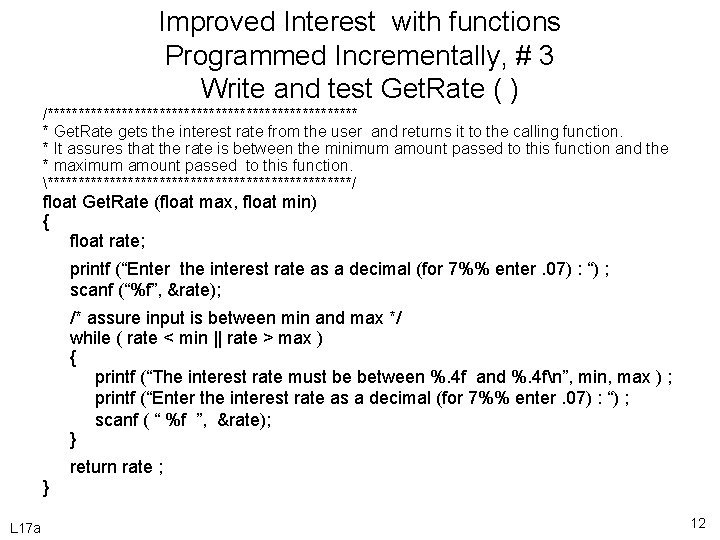
Improved Interest with functions Programmed Incrementally, # 3 Write and test Get. Rate ( ) /************************* * Get. Rate gets the interest rate from the user and returns it to the calling function. * It assures that the rate is between the minimum amount passed to this function and the * maximum amount passed to this function. *************************/ float Get. Rate (float max, float min) { float rate; printf (“Enter the interest rate as a decimal (for 7%% enter. 07) : “) ; scanf (“%f”, &rate); /* assure input is between min and max */ while ( rate < min || rate > max ) { printf (“The interest rate must be between %. 4 f and %. 4 fn”, min, max ) ; printf (“Enter the interest rate as a decimal (for 7%% enter. 07) : “) ; scanf ( “ %f ”, &rate); } } L 17 a return rate ; 12
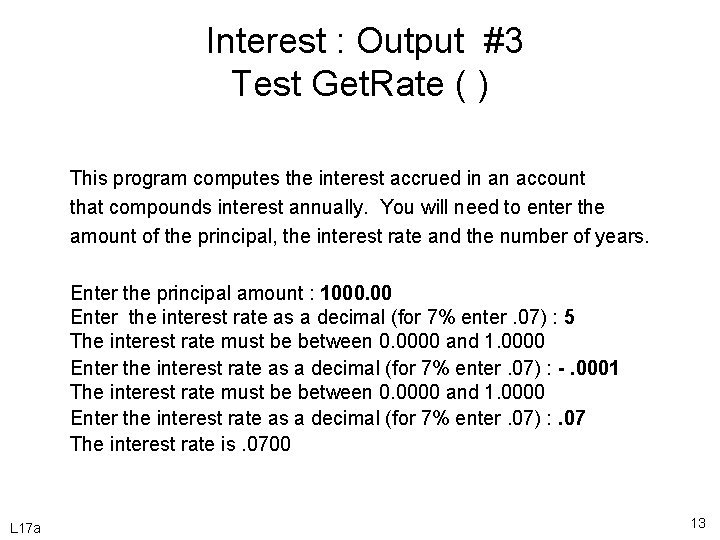
Interest : Output #3 Test Get. Rate ( ) This program computes the interest accrued in an account that compounds interest annually. You will need to enter the amount of the principal, the interest rate and the number of years. Enter the principal amount : 1000. 00 Enter the interest rate as a decimal (for 7% enter. 07) : 5 The interest rate must be between 0. 0000 and 1. 0000 Enter the interest rate as a decimal (for 7% enter. 07) : -. 0001 The interest rate must be between 0. 0000 and 1. 0000 Enter the interest rate as a decimal (for 7% enter. 07) : . 07 The interest rate is. 0700 L 17 a 13
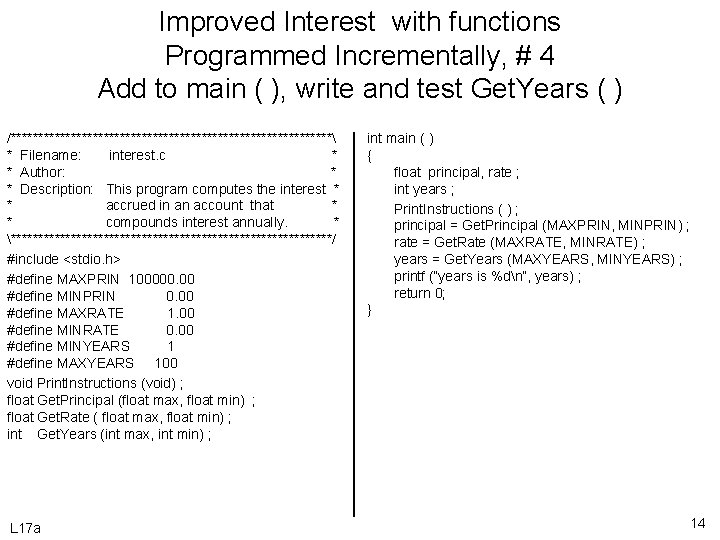
Improved Interest with functions Programmed Incrementally, # 4 Add to main ( ), write and test Get. Years ( ) /****************************** * Filename: interest. c * * Author: * * Description: This program computes the interest * * accrued in an account that * * compounds interest annually. * ******************************/ #include <stdio. h> #define MAXPRIN 100000. 00 #define MINPRIN 0. 00 #define MAXRATE 1. 00 #define MINRATE 0. 00 #define MINYEARS 1 #define MAXYEARS 100 void Print. Instructions (void) ; float Get. Principal (float max, float min) ; float Get. Rate ( float max, float min) ; int Get. Years (int max, int min) ; L 17 a int main ( ) { float principal, rate ; int years ; Print. Instructions ( ) ; principal = Get. Principal (MAXPRIN, MINPRIN) ; rate = Get. Rate (MAXRATE, MINRATE) ; years = Get. Years (MAXYEARS, MINYEARS) ; printf (“years is %dn”, years) ; return 0; } 14
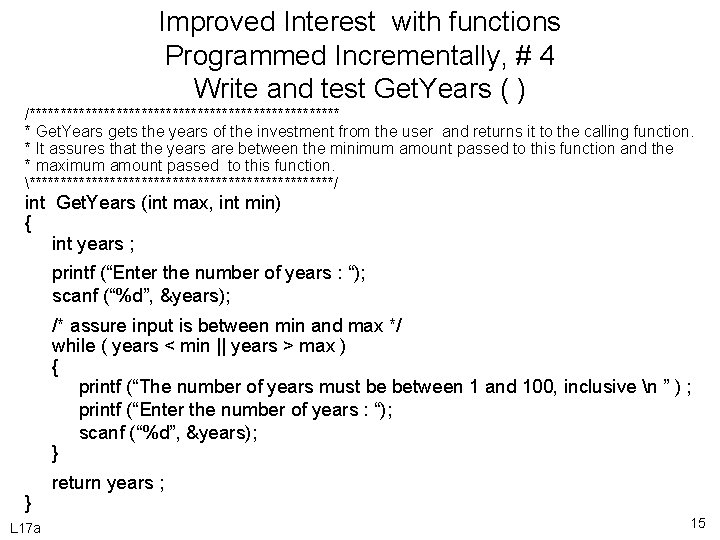
Improved Interest with functions Programmed Incrementally, # 4 Write and test Get. Years ( ) /************************* * Get. Years gets the years of the investment from the user and returns it to the calling function. * It assures that the years are between the minimum amount passed to this function and the * maximum amount passed to this function. *************************/ int Get. Years (int max, int min) { int years ; printf (“Enter the number of years : “); scanf (“%d”, &years); /* assure input is between min and max */ while ( years < min || years > max ) { printf (“The number of years must be between 1 and 100, inclusive n ” ) ; printf (“Enter the number of years : “); scanf (“%d”, &years); } } L 17 a return years ; 15
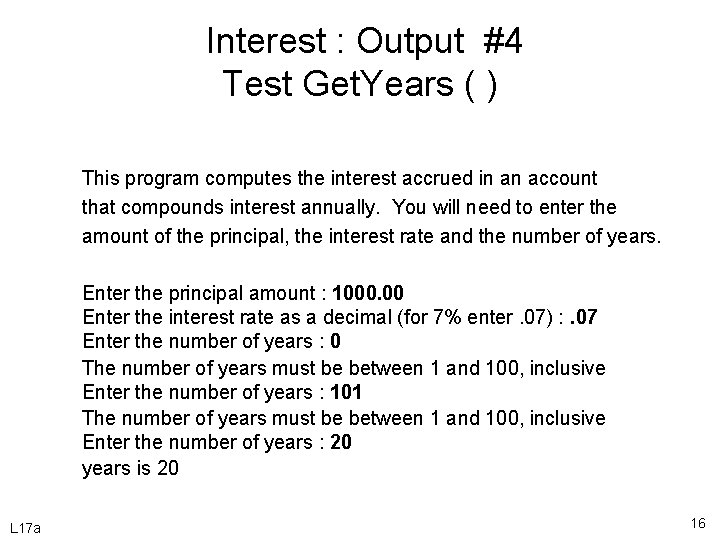
Interest : Output #4 Test Get. Years ( ) This program computes the interest accrued in an account that compounds interest annually. You will need to enter the amount of the principal, the interest rate and the number of years. Enter the principal amount : 1000. 00 Enter the interest rate as a decimal (for 7% enter. 07) : . 07 Enter the number of years : 0 The number of years must be between 1 and 100, inclusive Enter the number of years : 101 The number of years must be between 1 and 100, inclusive Enter the number of years : 20 years is 20 L 17 a 16
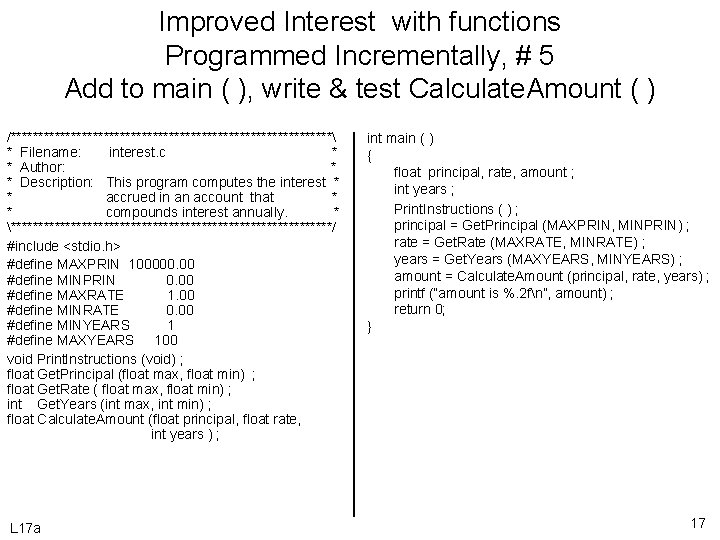
Improved Interest with functions Programmed Incrementally, # 5 Add to main ( ), write & test Calculate. Amount ( ) /****************************** * Filename: interest. c * * Author: * * Description: This program computes the interest * * accrued in an account that * * compounds interest annually. * ******************************/ #include <stdio. h> #define MAXPRIN 100000. 00 #define MINPRIN 0. 00 #define MAXRATE 1. 00 #define MINRATE 0. 00 #define MINYEARS 1 #define MAXYEARS 100 void Print. Instructions (void) ; float Get. Principal (float max, float min) ; float Get. Rate ( float max, float min) ; int Get. Years (int max, int min) ; float Calculate. Amount (float principal, float rate, int years ) ; L 17 a int main ( ) { float principal, rate, amount ; int years ; Print. Instructions ( ) ; principal = Get. Principal (MAXPRIN, MINPRIN) ; rate = Get. Rate (MAXRATE, MINRATE) ; years = Get. Years (MAXYEARS, MINYEARS) ; amount = Calculate. Amount (principal, rate, years) ; printf (“amount is %. 2 fn”, amount) ; return 0; } 17
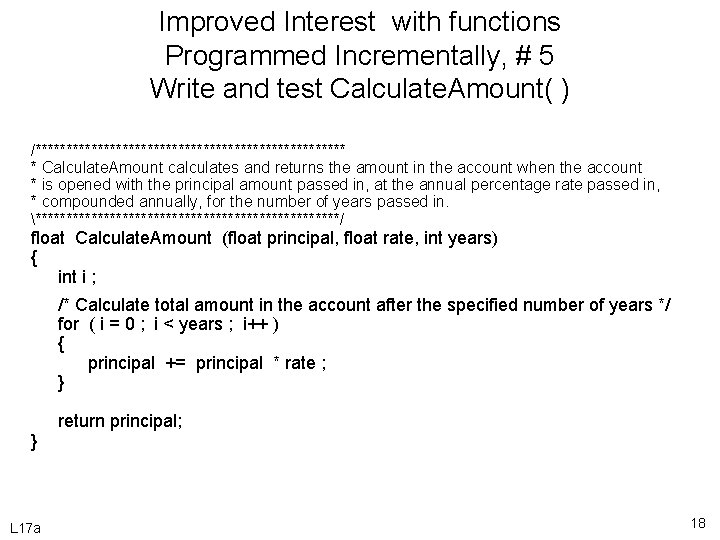
Improved Interest with functions Programmed Incrementally, # 5 Write and test Calculate. Amount( ) /************************* * Calculate. Amount calculates and returns the amount in the account when the account * is opened with the principal amount passed in, at the annual percentage rate passed in, * compounded annually, for the number of years passed in. *************************/ float Calculate. Amount (float principal, float rate, int years) { int i ; /* Calculate total amount in the account after the specified number of years */ for ( i = 0 ; i < years ; i++ ) { principal += principal * rate ; } } L 17 a return principal; 18
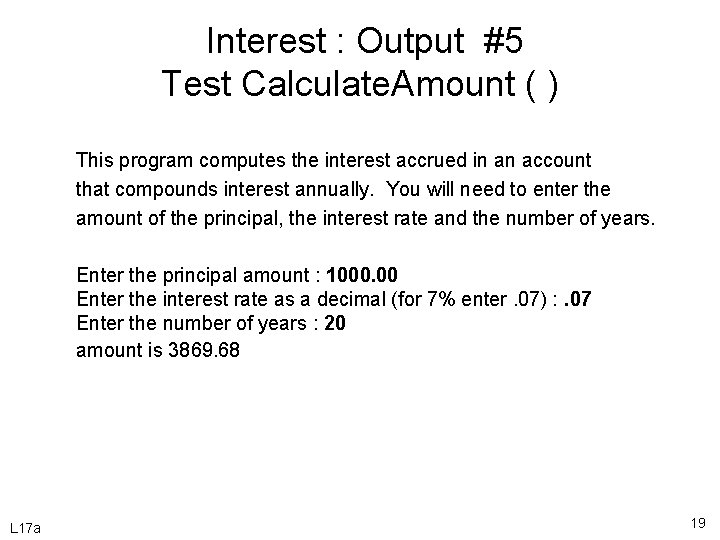
Interest : Output #5 Test Calculate. Amount ( ) This program computes the interest accrued in an account that compounds interest annually. You will need to enter the amount of the principal, the interest rate and the number of years. Enter the principal amount : 1000. 00 Enter the interest rate as a decimal (for 7% enter. 07) : . 07 Enter the number of years : 20 amount is 3869. 68 L 17 a 19
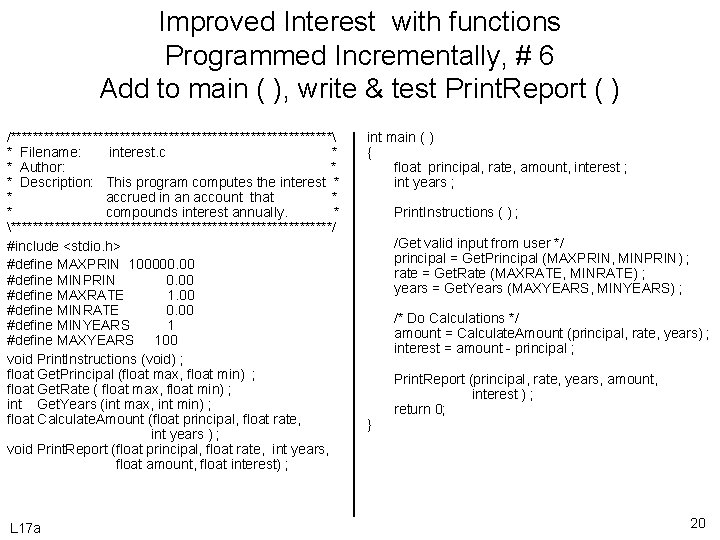
Improved Interest with functions Programmed Incrementally, # 6 Add to main ( ), write & test Print. Report ( ) /****************************** * Filename: interest. c * * Author: * * Description: This program computes the interest * * accrued in an account that * * compounds interest annually. * ******************************/ #include <stdio. h> #define MAXPRIN 100000. 00 #define MINPRIN 0. 00 #define MAXRATE 1. 00 #define MINRATE 0. 00 #define MINYEARS 1 #define MAXYEARS 100 void Print. Instructions (void) ; float Get. Principal (float max, float min) ; float Get. Rate ( float max, float min) ; int Get. Years (int max, int min) ; float Calculate. Amount (float principal, float rate, int years ) ; void Print. Report (float principal, float rate, int years, float amount, float interest) ; L 17 a int main ( ) { float principal, rate, amount, interest ; int years ; Print. Instructions ( ) ; /Get valid input from user */ principal = Get. Principal (MAXPRIN, MINPRIN) ; rate = Get. Rate (MAXRATE, MINRATE) ; years = Get. Years (MAXYEARS, MINYEARS) ; /* Do Calculations */ amount = Calculate. Amount (principal, rate, years) ; interest = amount - principal ; } Print. Report (principal, rate, years, amount, interest ) ; return 0; 20
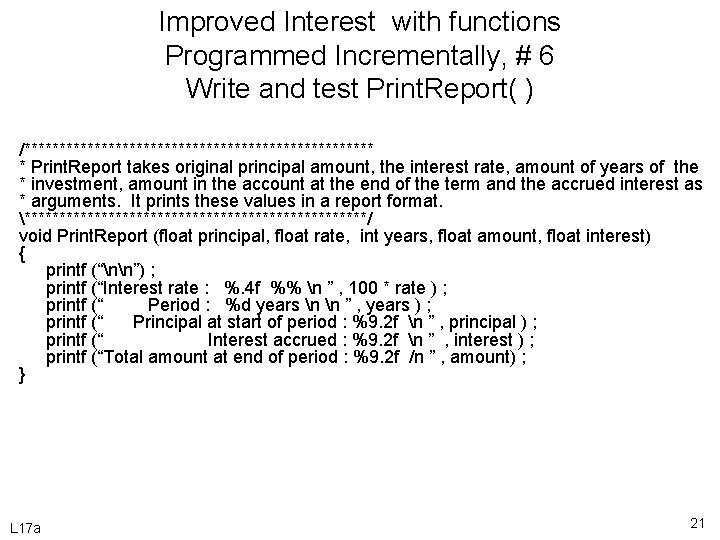
Improved Interest with functions Programmed Incrementally, # 6 Write and test Print. Report( ) /************************* * Print. Report takes original principal amount, the interest rate, amount of years of the * investment, amount in the account at the end of the term and the accrued interest as * arguments. It prints these values in a report format. *************************/ void Print. Report (float principal, float rate, int years, float amount, float interest) { printf (“nn”) ; printf (“Interest rate : %. 4 f %% n ” , 100 * rate ) ; printf (“ Period : %d years n n ” , years ) ; printf (“ Principal at start of period : %9. 2 f n ” , principal ) ; printf (“ Interest accrued : %9. 2 f n ” , interest ) ; printf (“Total amount at end of period : %9. 2 f /n ” , amount) ; } L 17 a 21
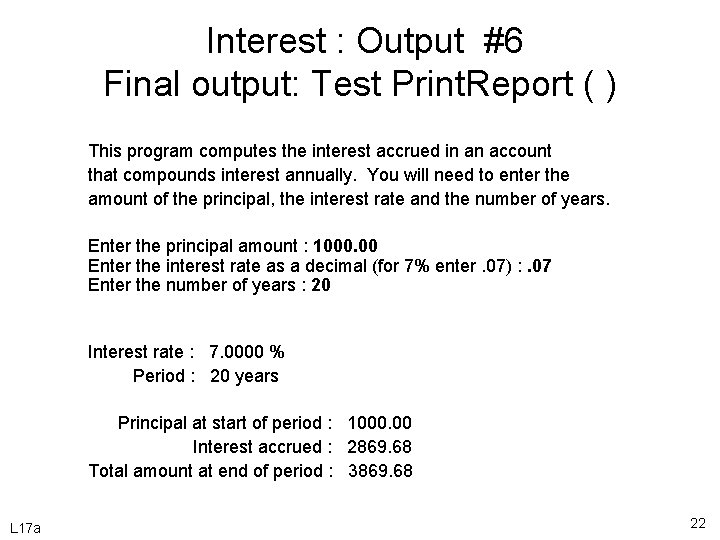
Interest : Output #6 Final output: Test Print. Report ( ) This program computes the interest accrued in an account that compounds interest annually. You will need to enter the amount of the principal, the interest rate and the number of years. Enter the principal amount : 1000. 00 Enter the interest rate as a decimal (for 7% enter. 07) : . 07 Enter the number of years : 20 Interest rate : 7. 0000 % Period : 20 years Principal at start of period : 1000. 00 Interest accrued : 2869. 68 Total amount at end of period : 3869. 68 L 17 a 22