Enumeration Types Enumerated Types An enumerated type is
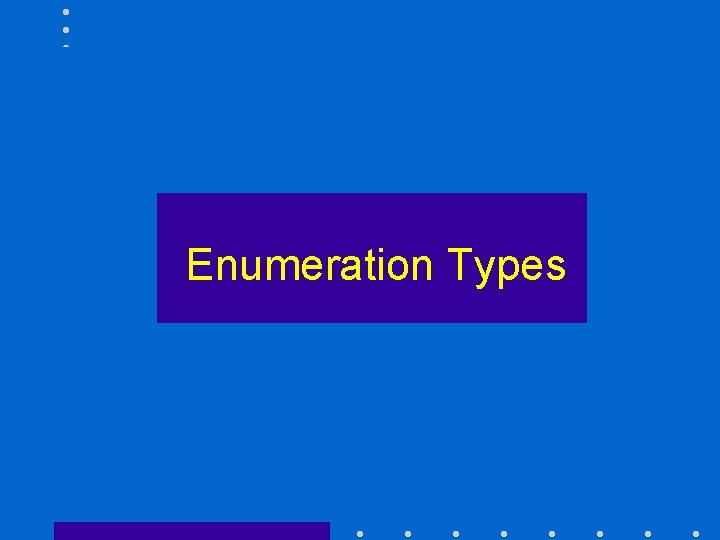
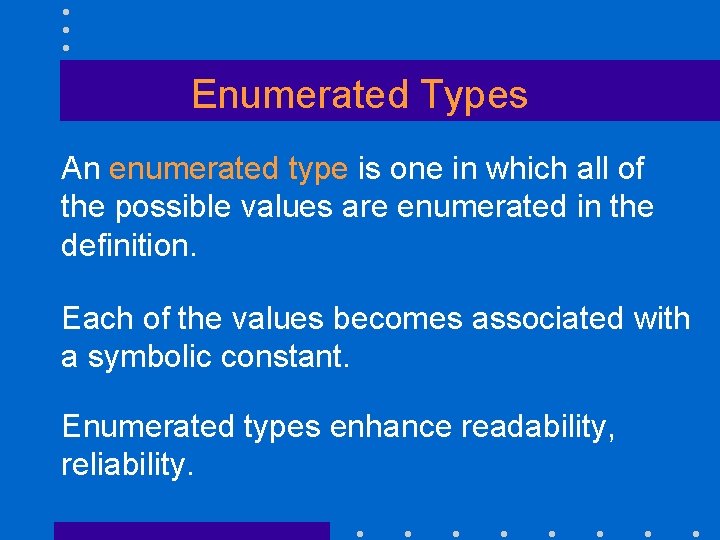
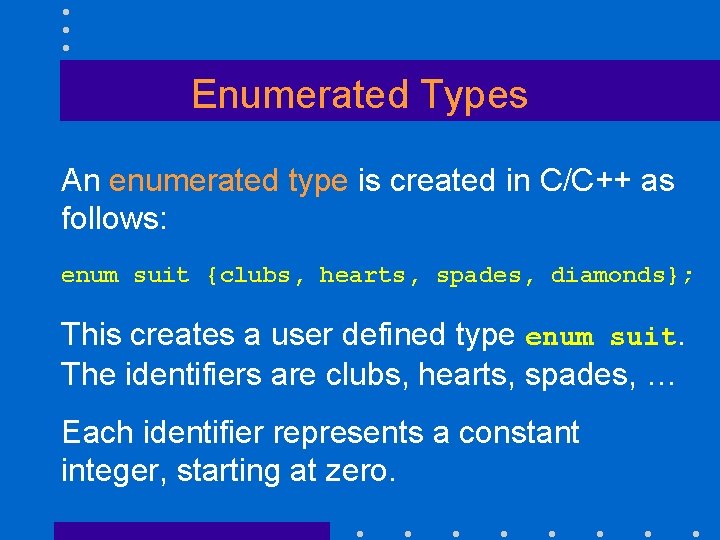
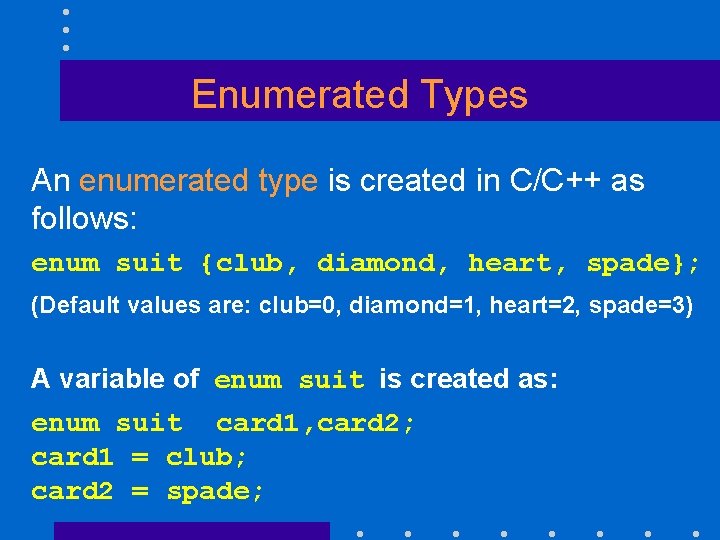
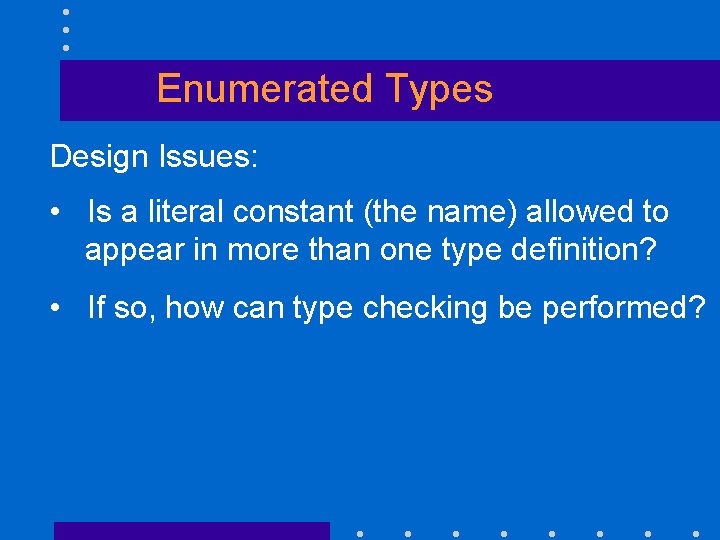
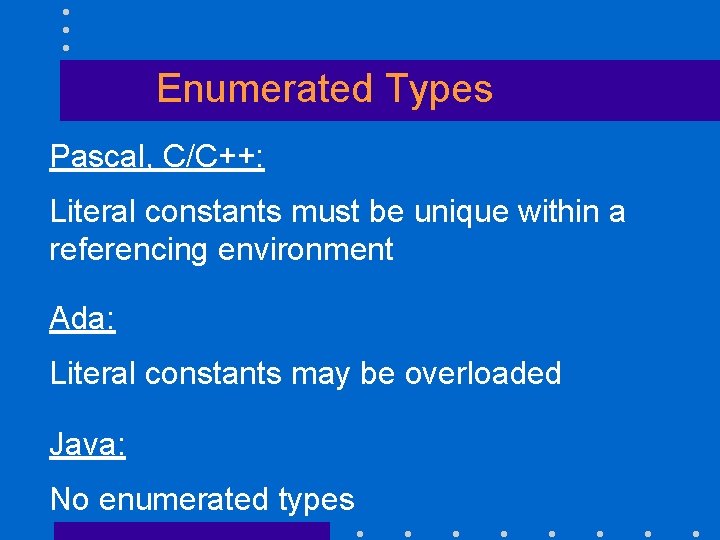
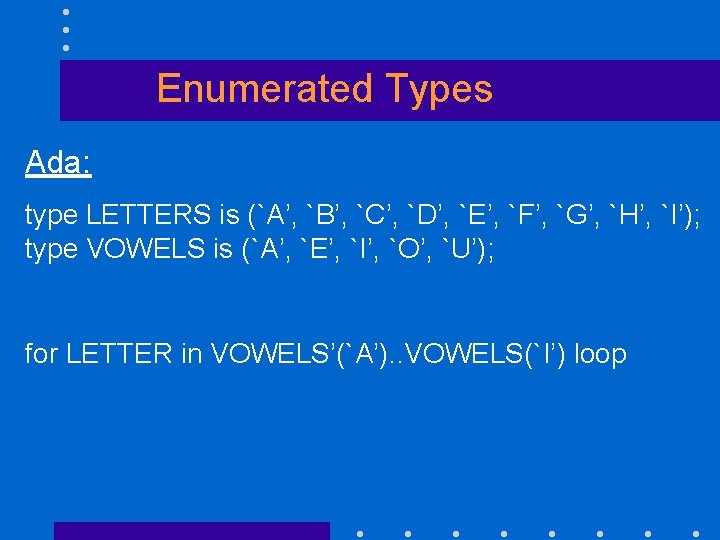
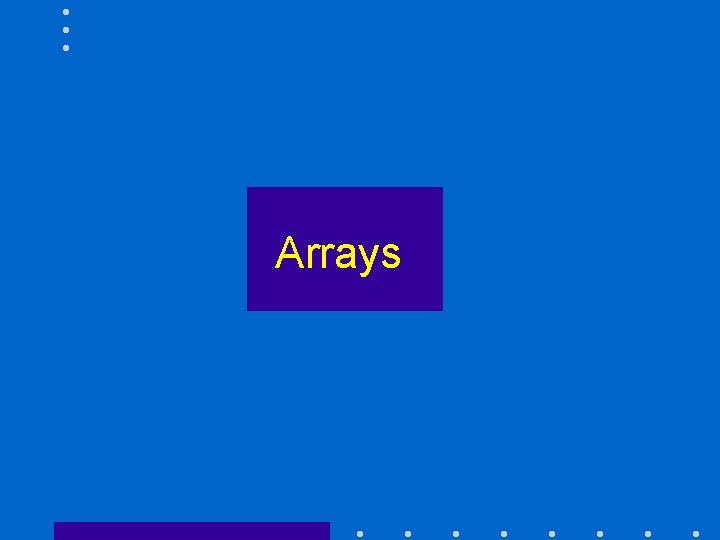
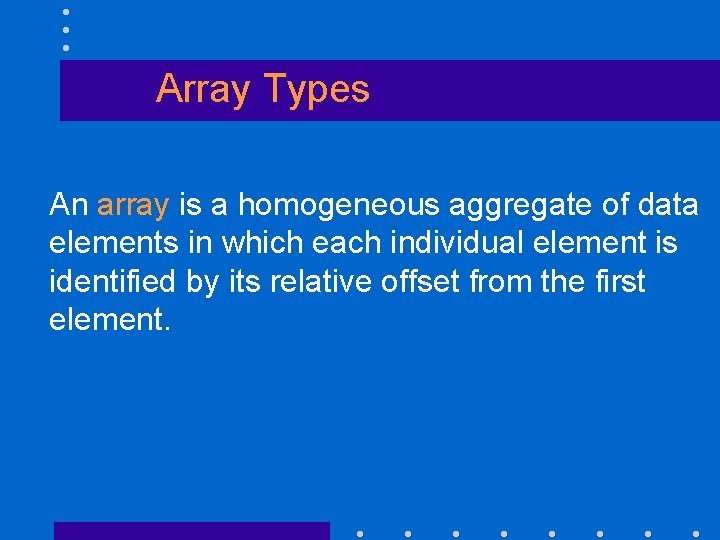
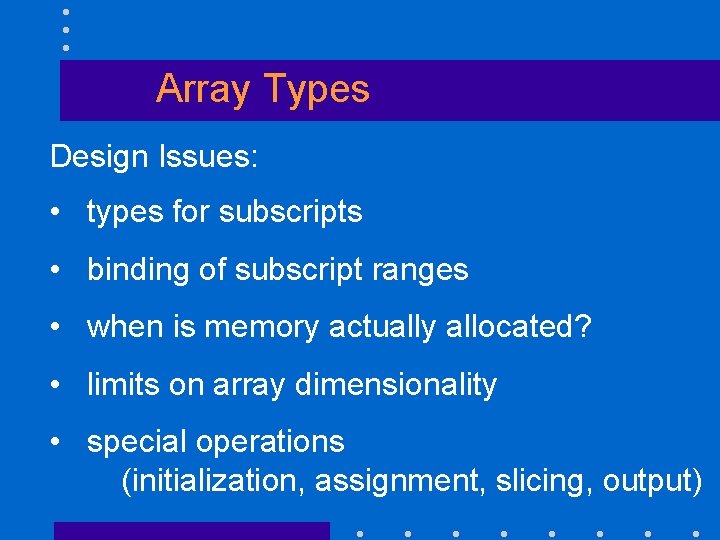
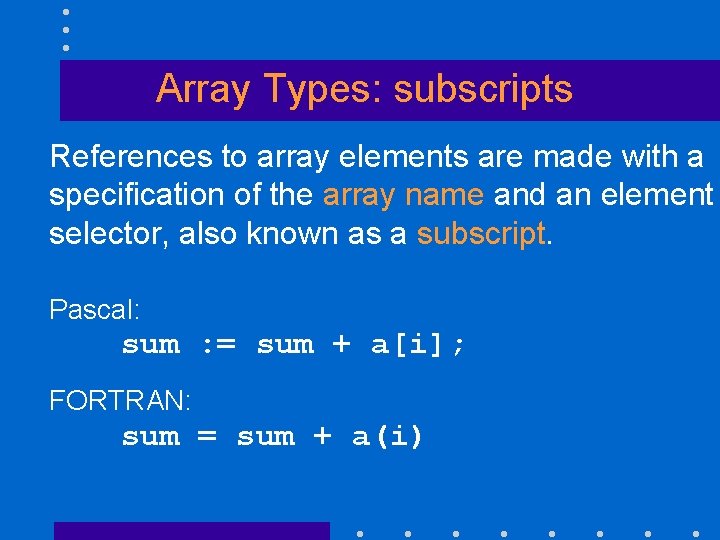
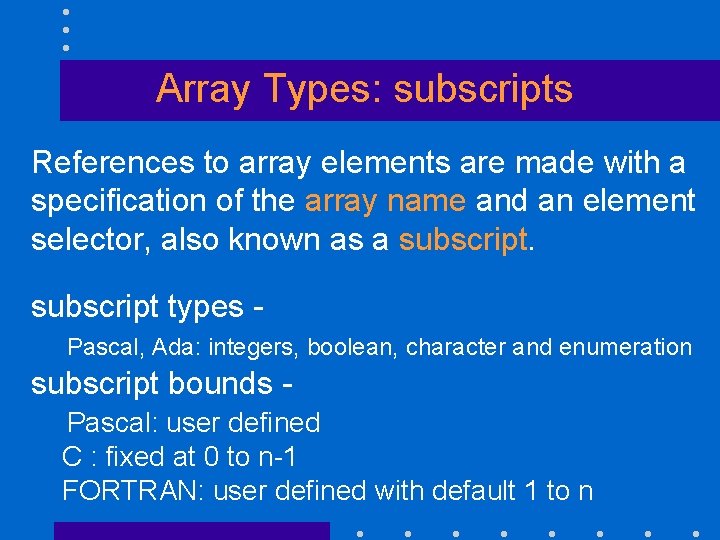
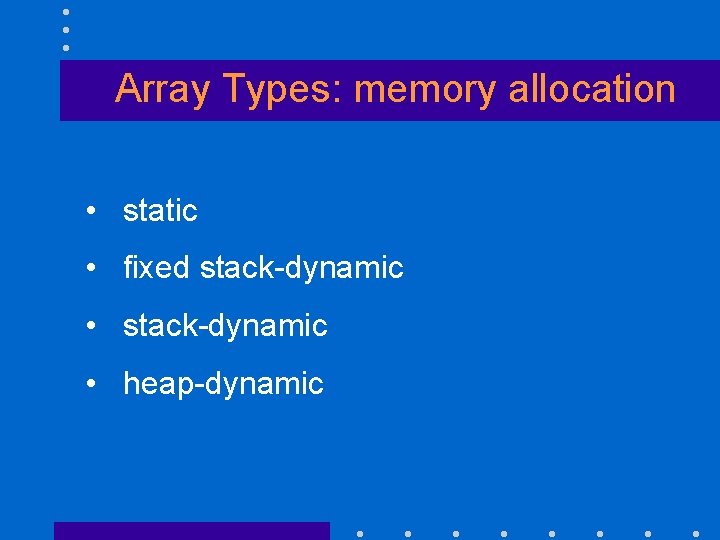
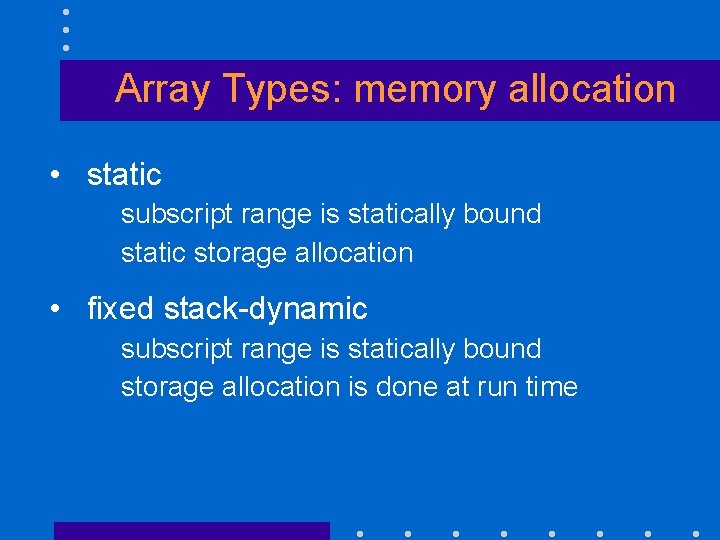
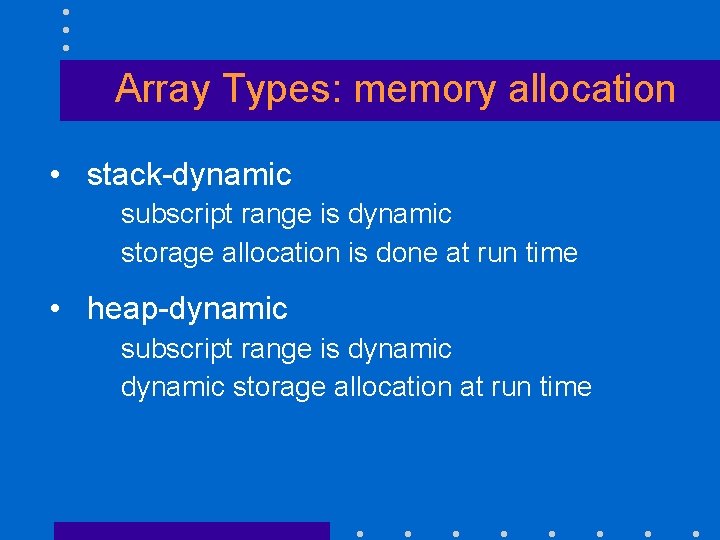
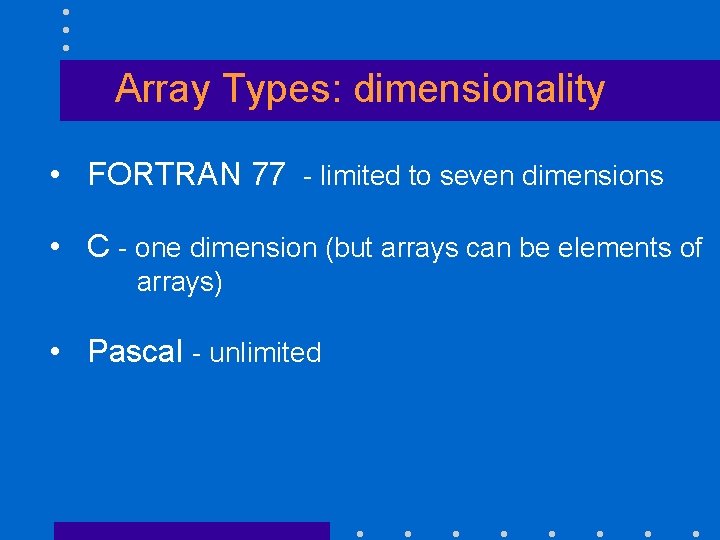
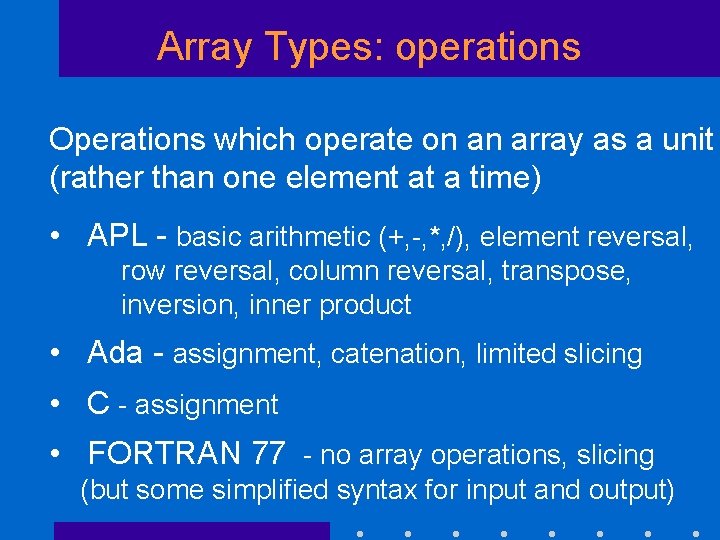
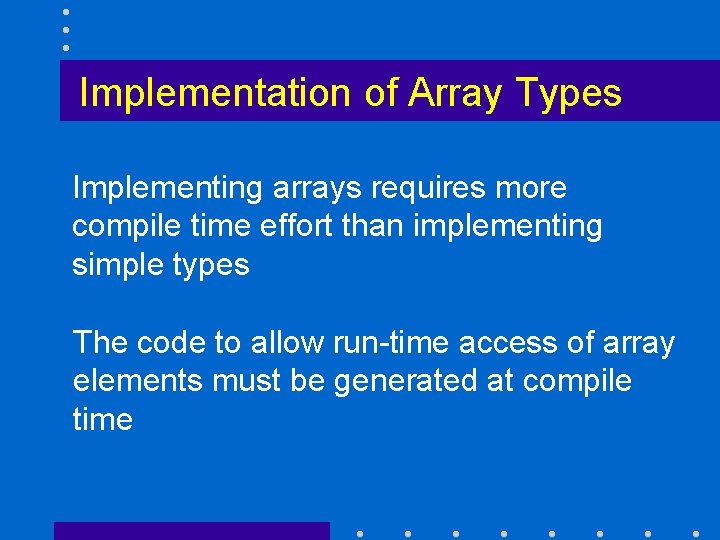
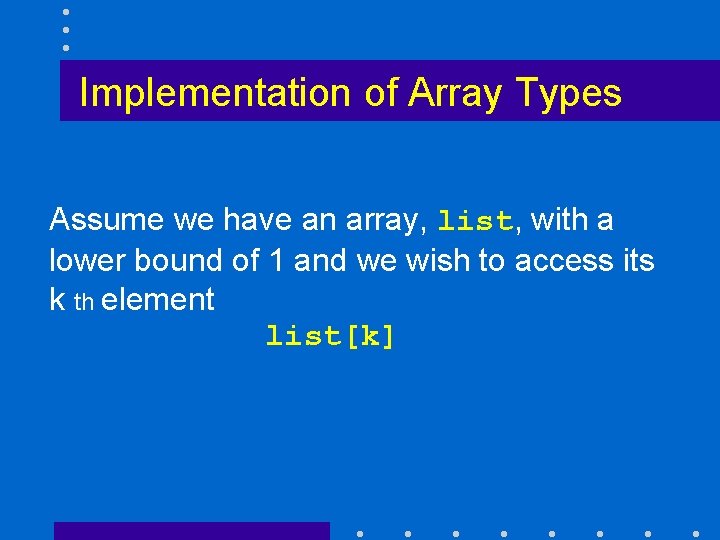
![Implementation of Array Types The computation of the address of list[k] can be computed Implementation of Array Types The computation of the address of list[k] can be computed](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-20.jpg)
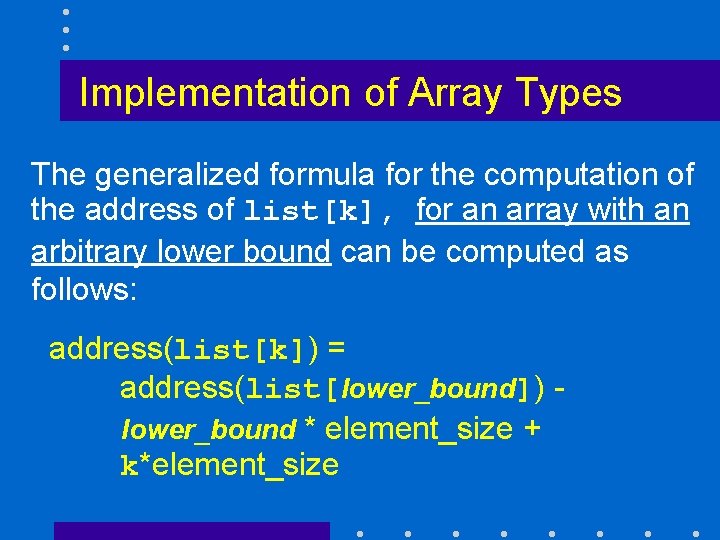
![Implementation of Array Types address(list[k]) = address(list[lower_bound]) lower_bound * element_size + k*element_size list[100] list[101] Implementation of Array Types address(list[k]) = address(list[lower_bound]) lower_bound * element_size + k*element_size list[100] list[101]](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-22.jpg)
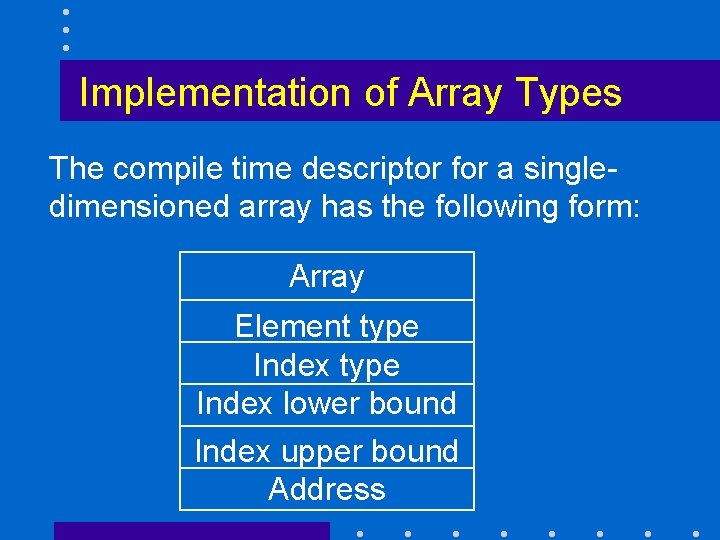
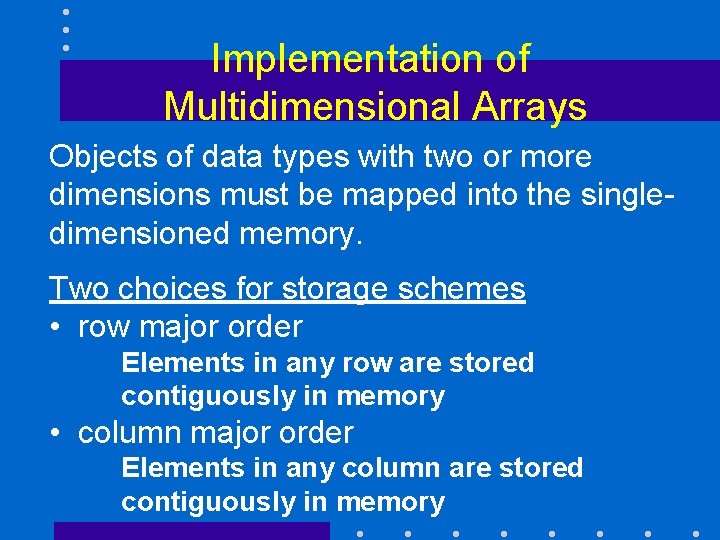
![Implementation of Multidimensional Arrays 3 6 1 A[1][1] = 3 A[2][1] = 6 A[3][1] Implementation of Multidimensional Arrays 3 6 1 A[1][1] = 3 A[2][1] = 6 A[3][1]](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-25.jpg)
![Implementation of Multidimensional Arrays A[1][1] = 3 A[2][1] = 6 A[3][1] = 1 ROW Implementation of Multidimensional Arrays A[1][1] = 3 A[2][1] = 6 A[3][1] = 1 ROW](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-26.jpg)
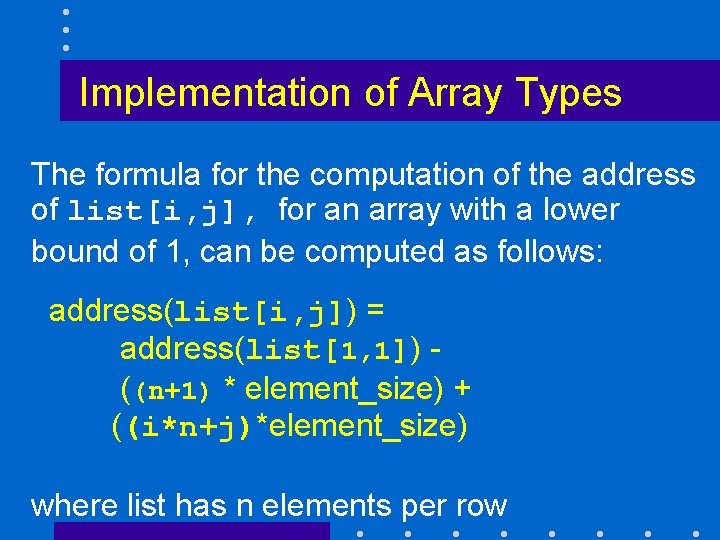
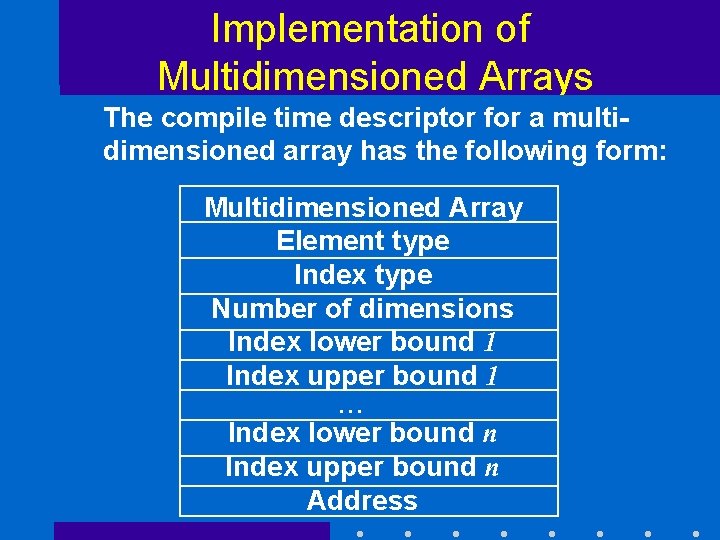
- Slides: 28
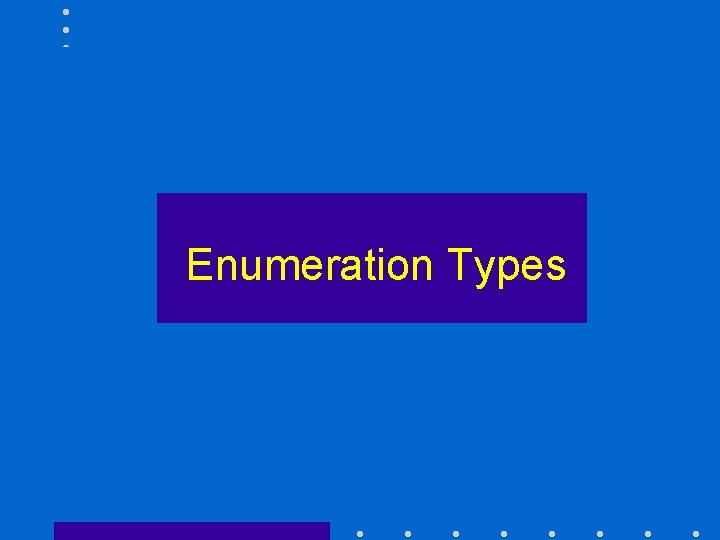
Enumeration Types
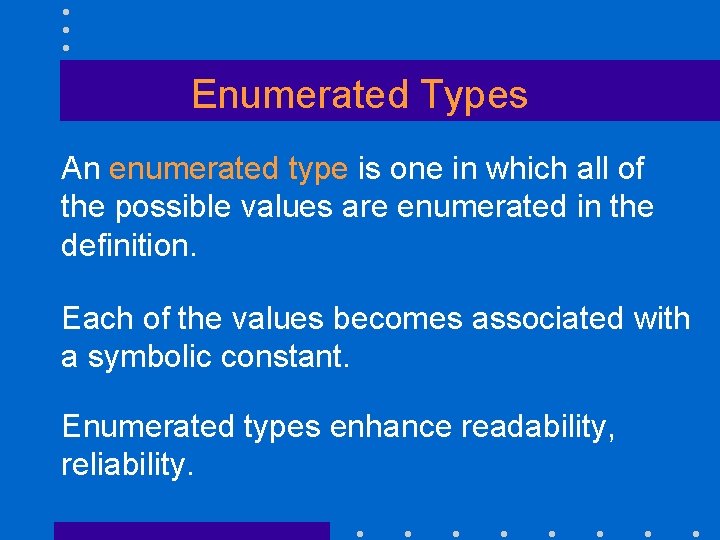
Enumerated Types An enumerated type is one in which all of the possible values are enumerated in the definition. Each of the values becomes associated with a symbolic constant. Enumerated types enhance readability, reliability.
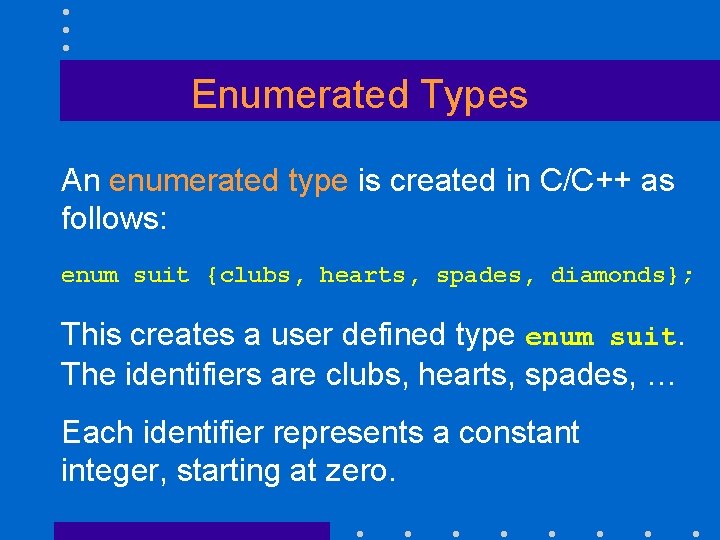
Enumerated Types An enumerated type is created in C/C++ as follows: enum suit {clubs, hearts, spades, diamonds}; This creates a user defined type enum suit. The identifiers are clubs, hearts, spades, … Each identifier represents a constant integer, starting at zero.
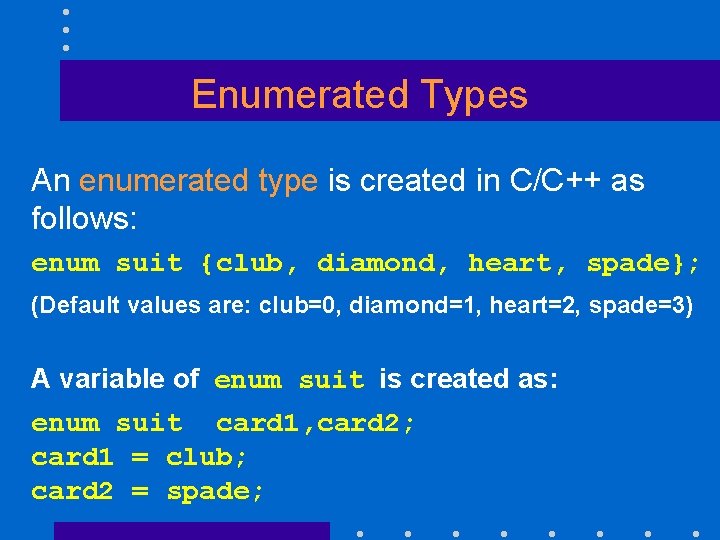
Enumerated Types An enumerated type is created in C/C++ as follows: enum suit {club, diamond, heart, spade}; (Default values are: club=0, diamond=1, heart=2, spade=3) A variable of enum suit is created as: enum suit card 1, card 2; card 1 = club; card 2 = spade;
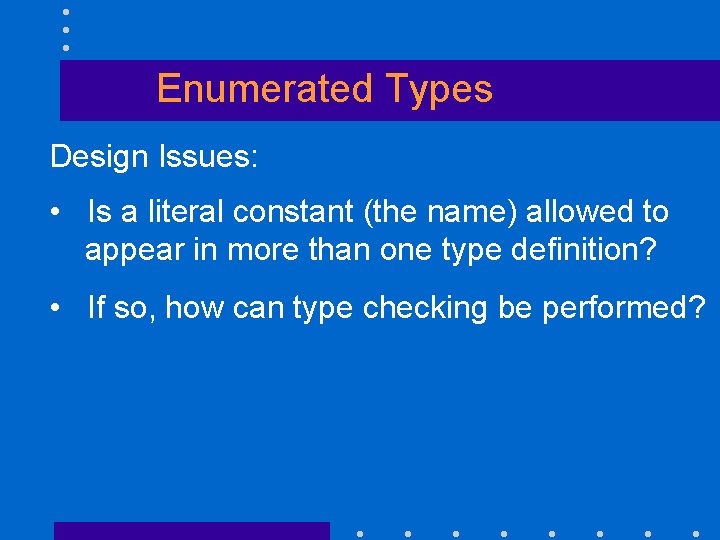
Enumerated Types Design Issues: • Is a literal constant (the name) allowed to appear in more than one type definition? • If so, how can type checking be performed?
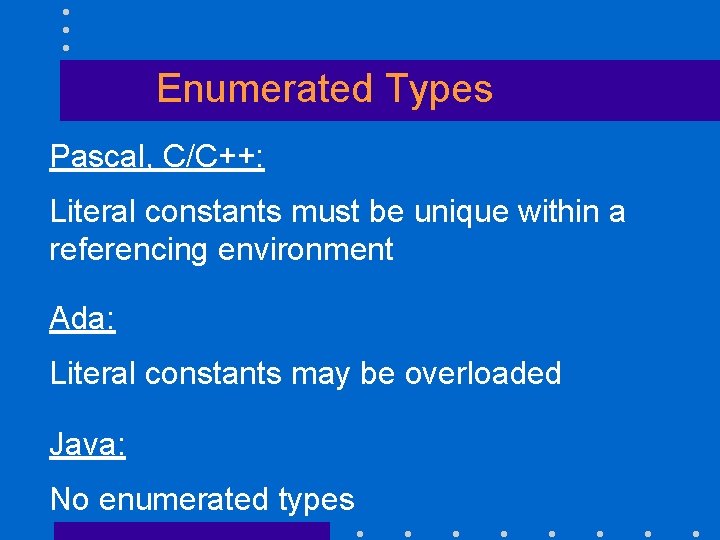
Enumerated Types Pascal, C/C++: Literal constants must be unique within a referencing environment Ada: Literal constants may be overloaded Java: No enumerated types
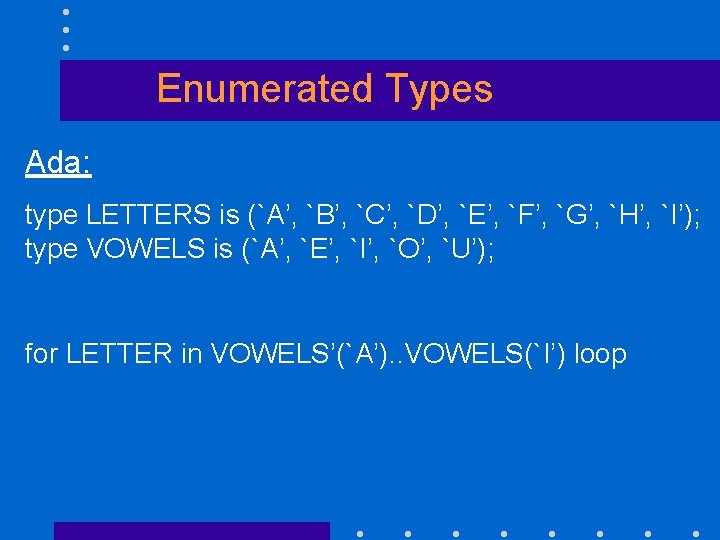
Enumerated Types Ada: type LETTERS is (`A’, `B’, `C’, `D’, `E’, `F’, `G’, `H’, `I’); type VOWELS is (`A’, `E’, `I’, `O’, `U’); for LETTER in VOWELS’(`A’). . VOWELS(`I’) loop
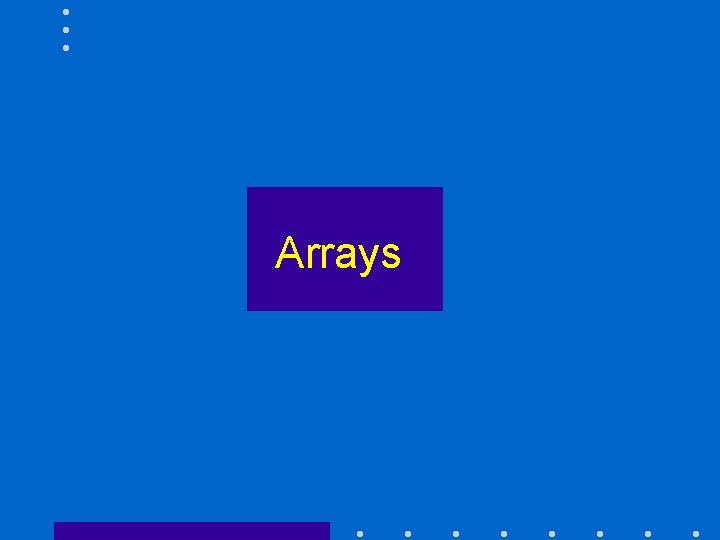
Arrays
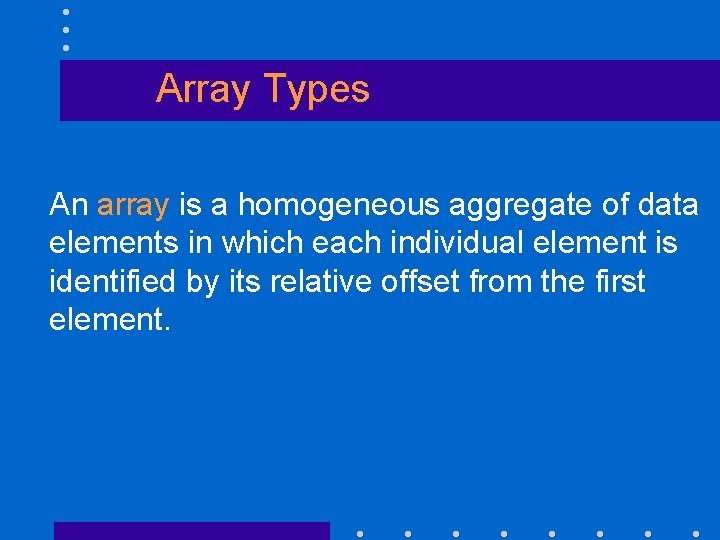
Array Types An array is a homogeneous aggregate of data elements in which each individual element is identified by its relative offset from the first element.
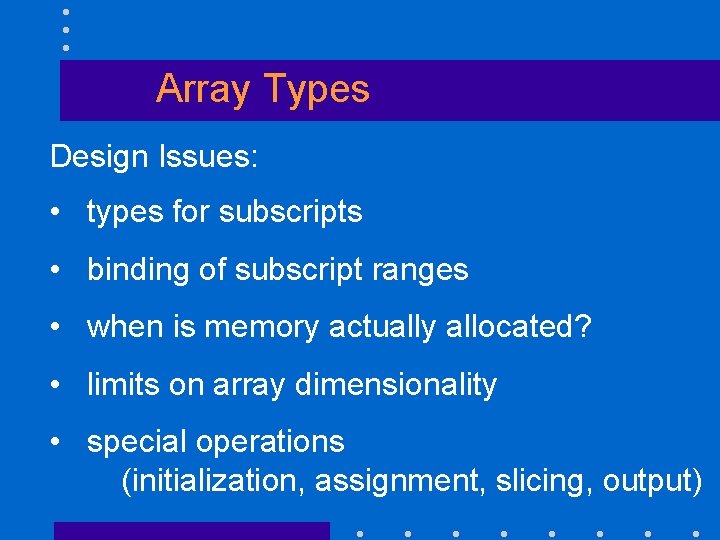
Array Types Design Issues: • types for subscripts • binding of subscript ranges • when is memory actually allocated? • limits on array dimensionality • special operations (initialization, assignment, slicing, output)
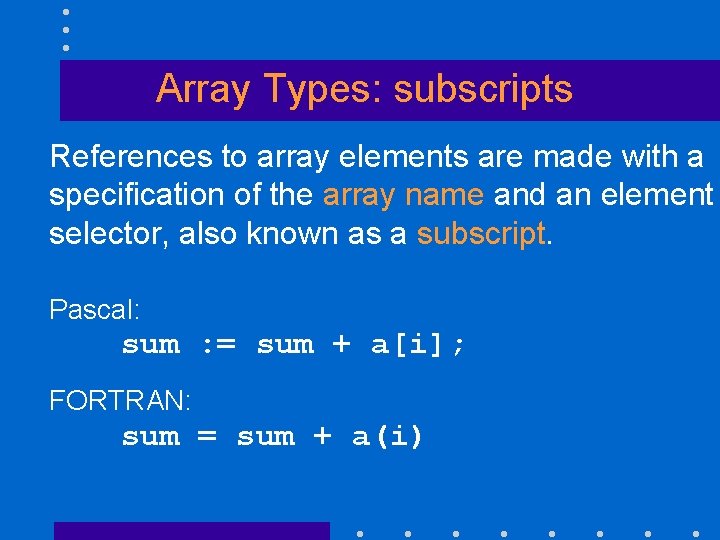
Array Types: subscripts References to array elements are made with a specification of the array name and an element selector, also known as a subscript. Pascal: sum : = sum + a[i]; FORTRAN: sum = sum + a(i)
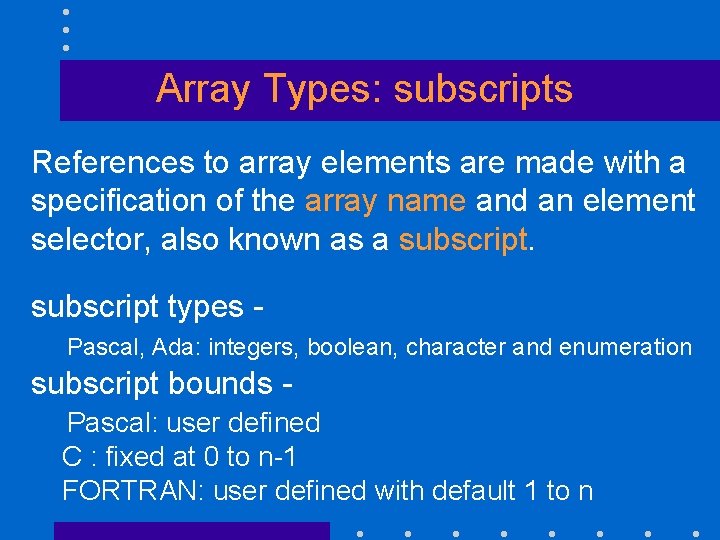
Array Types: subscripts References to array elements are made with a specification of the array name and an element selector, also known as a subscript types Pascal, Ada: integers, boolean, character and enumeration subscript bounds Pascal: user defined C : fixed at 0 to n-1 FORTRAN: user defined with default 1 to n
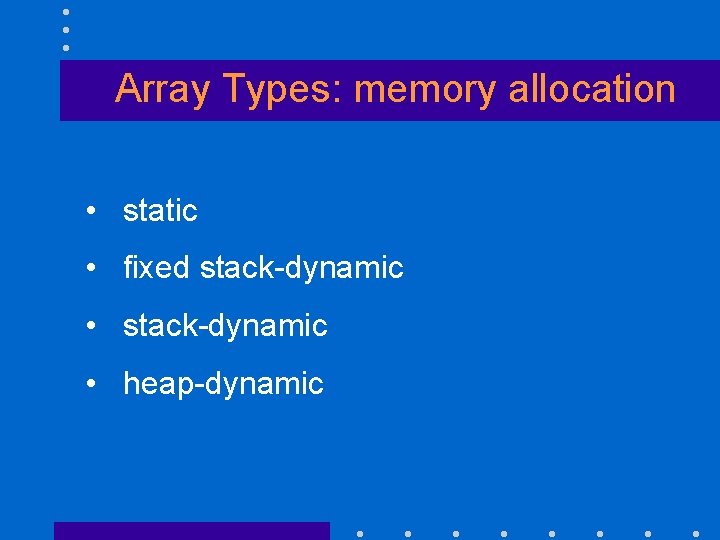
Array Types: memory allocation • static • fixed stack-dynamic • heap-dynamic
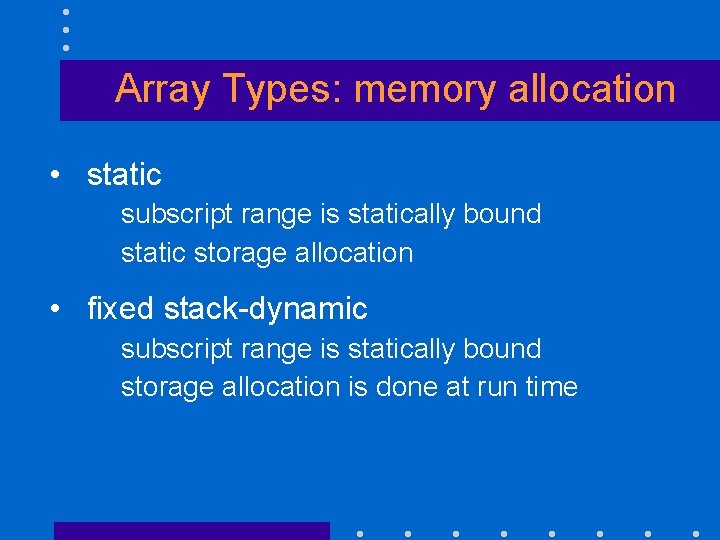
Array Types: memory allocation • static subscript range is statically bound static storage allocation • fixed stack-dynamic subscript range is statically bound storage allocation is done at run time
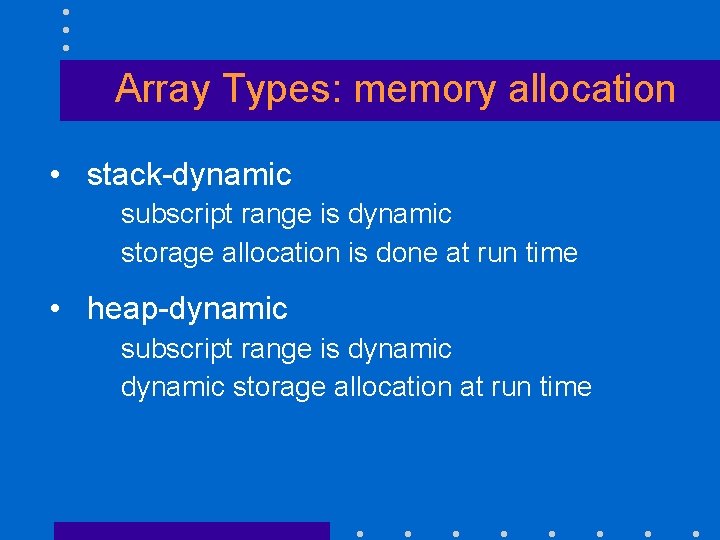
Array Types: memory allocation • stack-dynamic subscript range is dynamic storage allocation is done at run time • heap-dynamic subscript range is dynamic storage allocation at run time
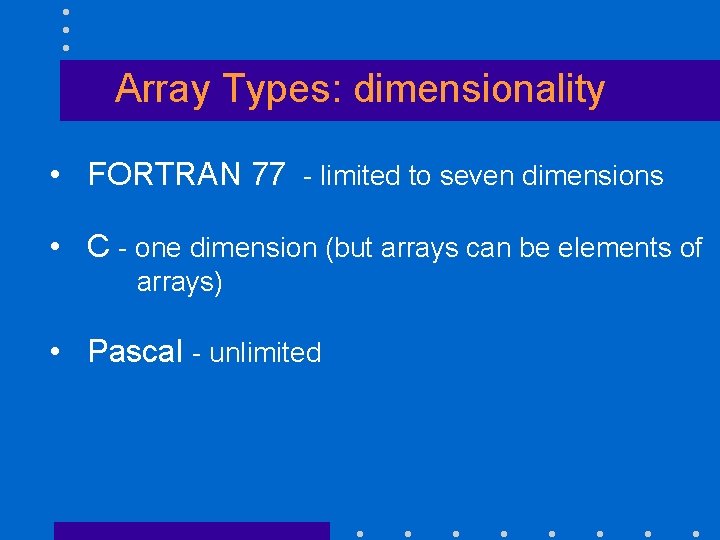
Array Types: dimensionality • FORTRAN 77 - limited to seven dimensions • C - one dimension (but arrays can be elements of arrays) • Pascal - unlimited
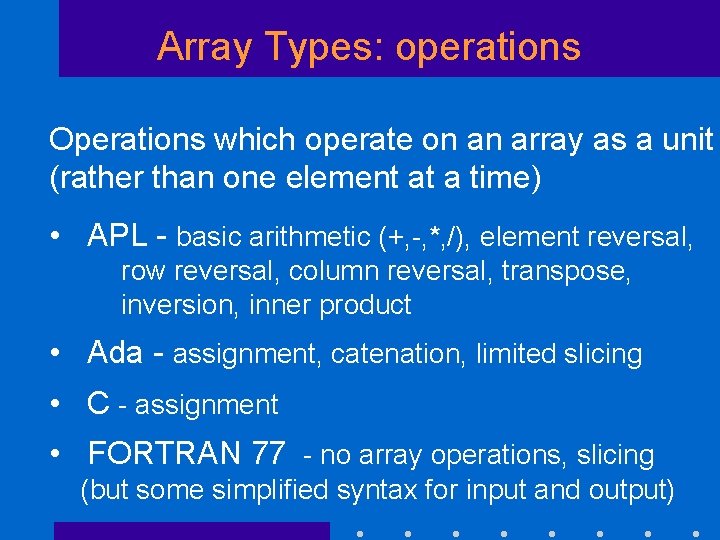
Array Types: operations Operations which operate on an array as a unit (rather than one element at a time) • APL - basic arithmetic (+, -, *, /), element reversal, row reversal, column reversal, transpose, inversion, inner product • Ada - assignment, catenation, limited slicing • C - assignment • FORTRAN 77 - no array operations, slicing (but some simplified syntax for input and output)
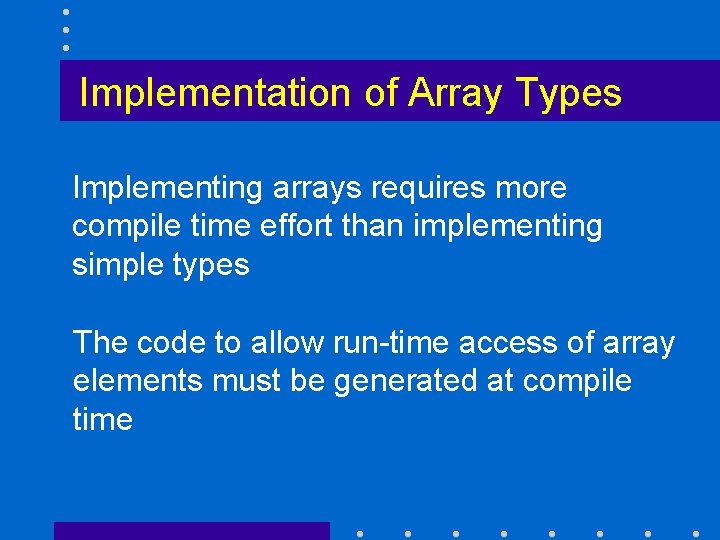
Implementation of Array Types Implementing arrays requires more compile time effort than implementing simple types The code to allow run-time access of array elements must be generated at compile time
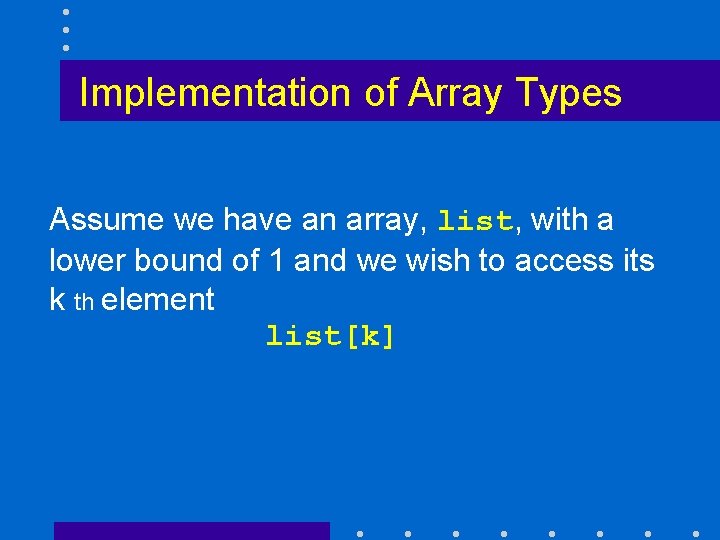
Implementation of Array Types Assume we have an array, list, with a lower bound of 1 and we wish to access its k th element list[k]
![Implementation of Array Types The computation of the address of listk can be computed Implementation of Array Types The computation of the address of list[k] can be computed](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-20.jpg)
Implementation of Array Types The computation of the address of list[k] can be computed as follows: address(list[k]) = address(list[1]) + (k-1)*element_size This simplifies to: address(list[k]) = address(list[1]) - element_size + k*element_size
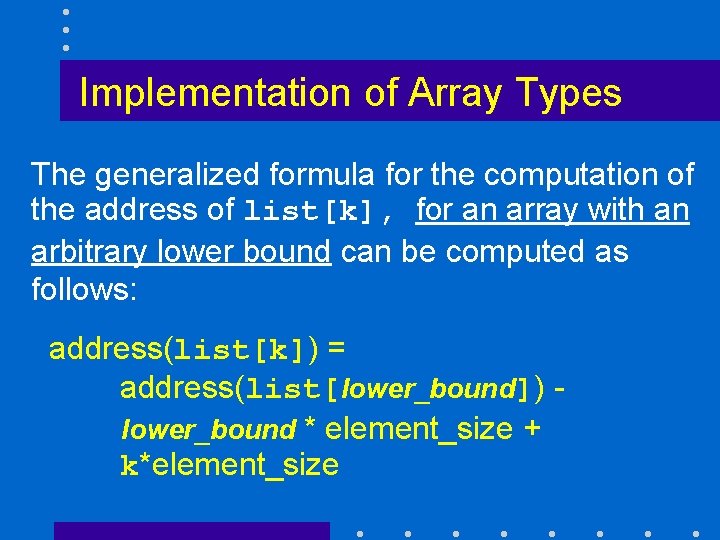
Implementation of Array Types The generalized formula for the computation of the address of list[k], for an array with an arbitrary lower bound can be computed as follows: address(list[k]) = address(list[lower_bound]) lower_bound * element_size + k*element_size
![Implementation of Array Types addresslistk addresslistlowerbound lowerbound elementsize kelementsize list100 list101 Implementation of Array Types address(list[k]) = address(list[lower_bound]) lower_bound * element_size + k*element_size list[100] list[101]](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-22.jpg)
Implementation of Array Types address(list[k]) = address(list[lower_bound]) lower_bound * element_size + k*element_size list[100] list[101] … list[107] list[108]
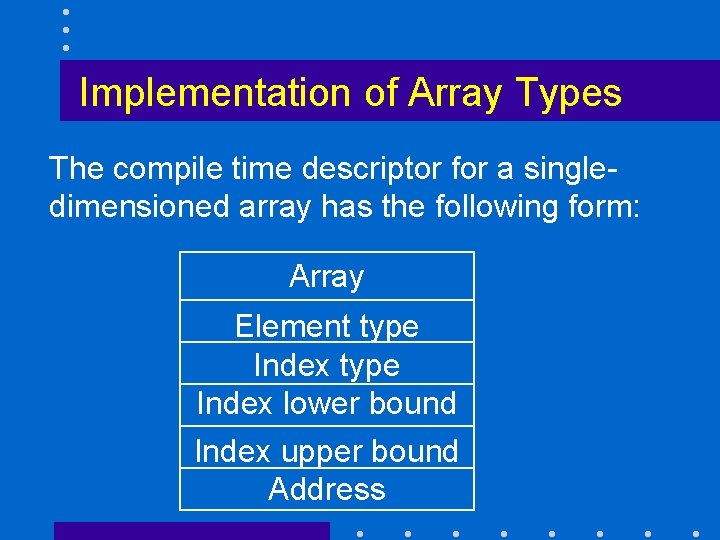
Implementation of Array Types The compile time descriptor for a singledimensioned array has the following form: Array Element type Index lower bound Index upper bound Address
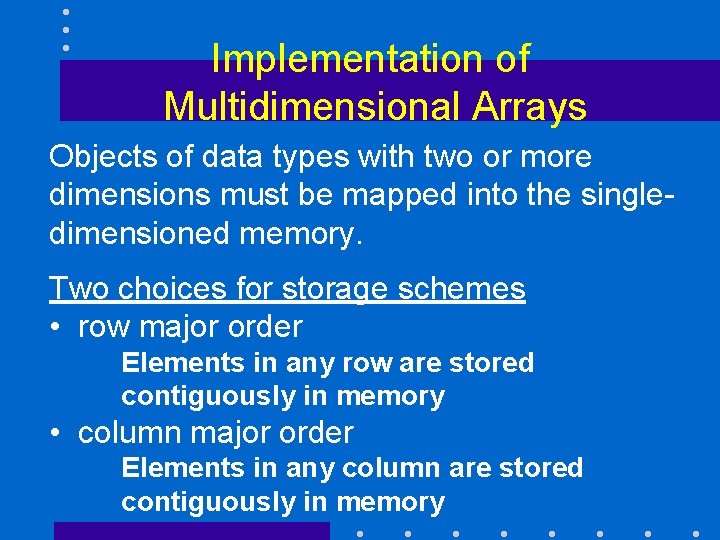
Implementation of Multidimensional Arrays Objects of data types with two or more dimensions must be mapped into the singledimensioned memory. Two choices for storage schemes • row major order Elements in any row are stored contiguously in memory • column major order Elements in any column are stored contiguously in memory
![Implementation of Multidimensional Arrays 3 6 1 A11 3 A21 6 A31 Implementation of Multidimensional Arrays 3 6 1 A[1][1] = 3 A[2][1] = 6 A[3][1]](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-25.jpg)
Implementation of Multidimensional Arrays 3 6 1 A[1][1] = 3 A[2][1] = 6 A[3][1] = 1 4 2 3 A[1][2] = 4 A[2][2] = 2 A[3][2] = 3 7 5 8 A[1][3] = 7 A[2][3] = 5 A[3][3] = 8
![Implementation of Multidimensional Arrays A11 3 A21 6 A31 1 ROW Implementation of Multidimensional Arrays A[1][1] = 3 A[2][1] = 6 A[3][1] = 1 ROW](https://slidetodoc.com/presentation_image/1e8fa21bc385c884e77e577042ee9d2a/image-26.jpg)
Implementation of Multidimensional Arrays A[1][1] = 3 A[2][1] = 6 A[3][1] = 1 ROW MAJOR ORDER : A[1][2] = 4 A[2][2] = 2 A[3][2] = 3 A[1][1] = 3 A[1][2] = 4 A[1][3] = 7 A[2][1] = 6 A[2][2] = 2 A[2][3] = 5 A[3][1] = 1 A[1][3] = 7 A[2][3] = 5 A[3][3] = 8 COLUMN A[1][1] = 3 MAJOR ORDER : A[2][1] = 6 A[3][1] = 1 A[1][2] = 4 A[2][2] = 2 A[3][2] = 3 A[1][3] = 7 A[2][3] = 5
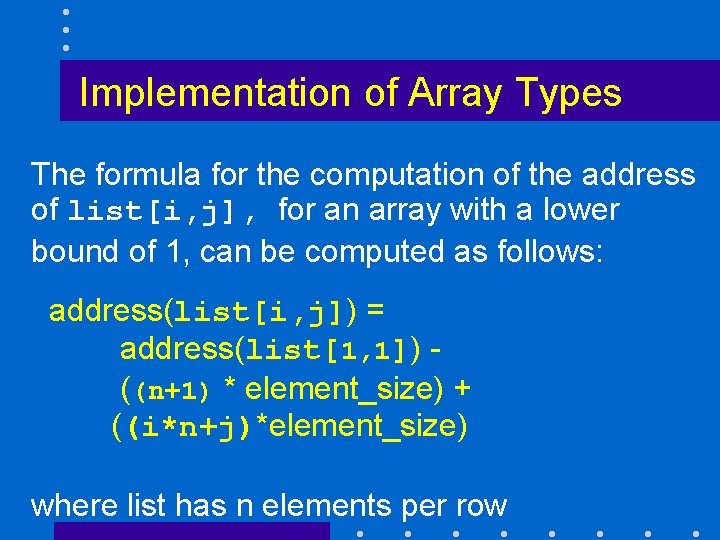
Implementation of Array Types The formula for the computation of the address of list[i, j], for an array with a lower bound of 1, can be computed as follows: address(list[i, j]) = address(list[1, 1]) ((n+1) * element_size) + ((i*n+j)*element_size) where list has n elements per row
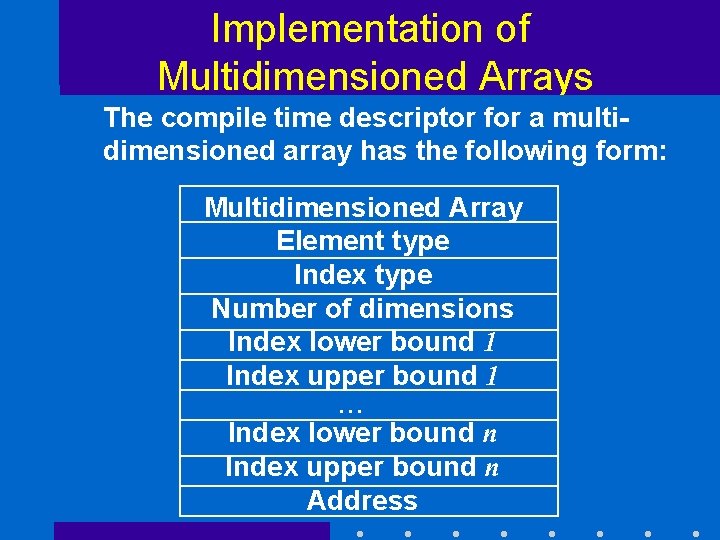
Implementation of Multidimensioned Arrays The compile time descriptor for a multidimensioned array has the following form: Multidimensioned Array Element type Index type Number of dimensions Index lower bound 1 Index upper bound 1 . . . Index lower bound n Index upper bound n Address