Entity Framework NET Framework ADO NET Data Set
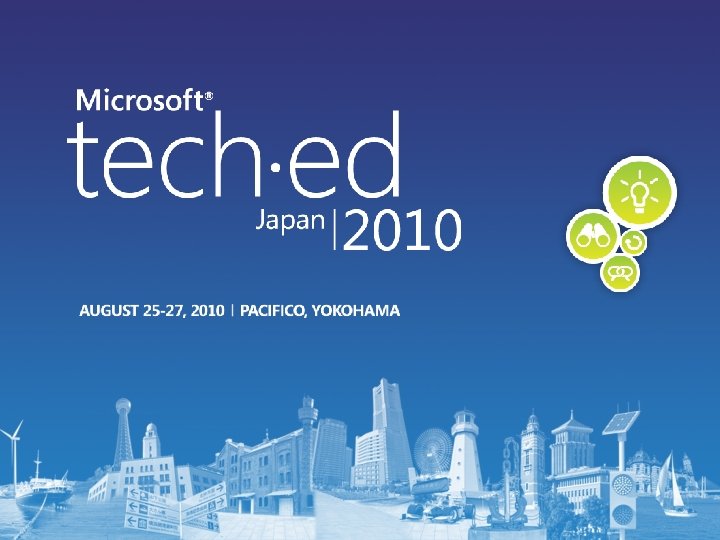
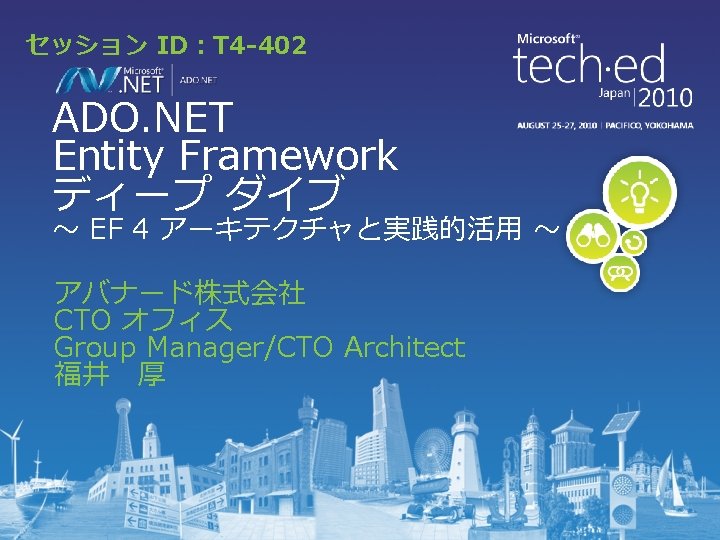
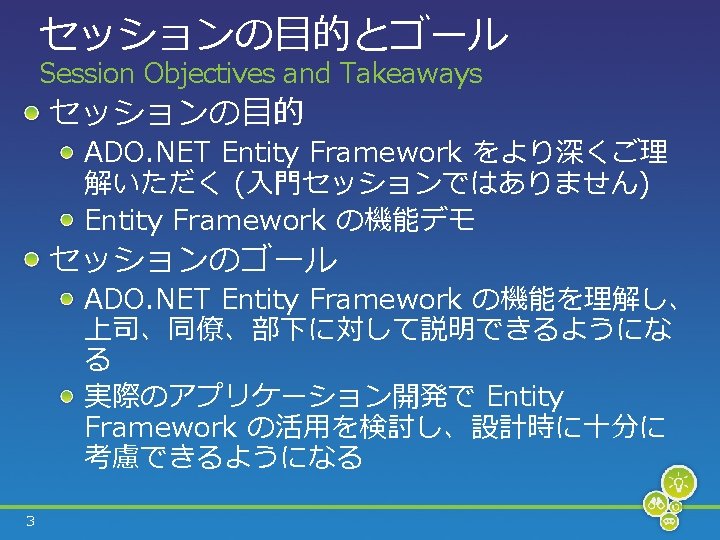
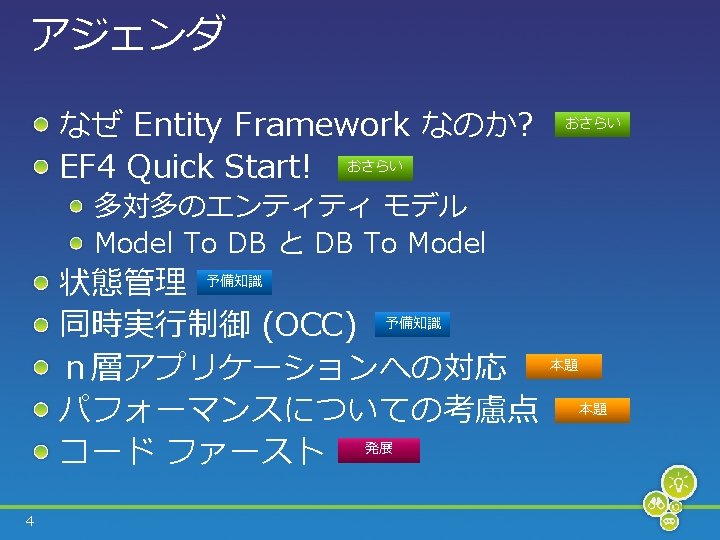
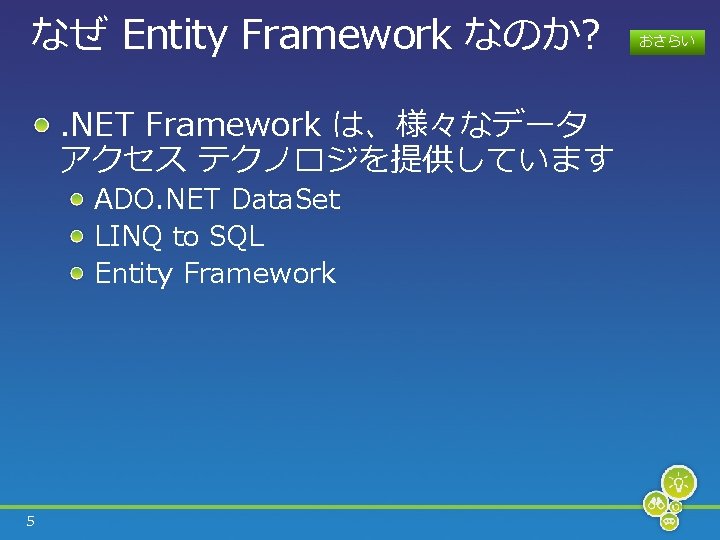
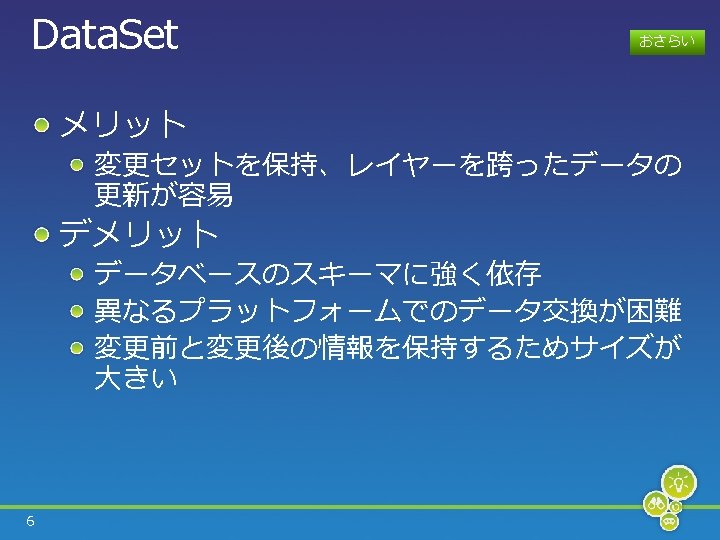
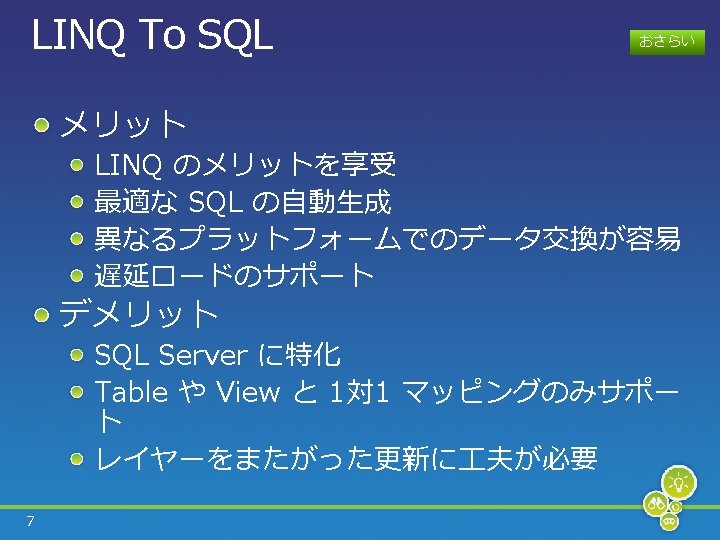
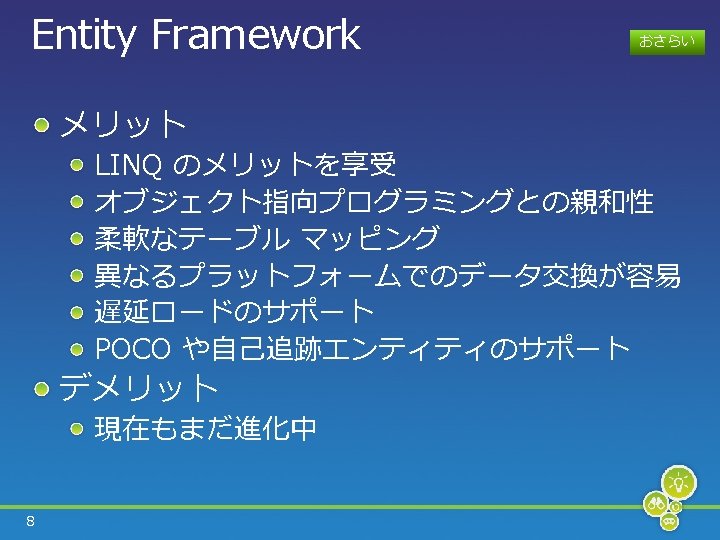
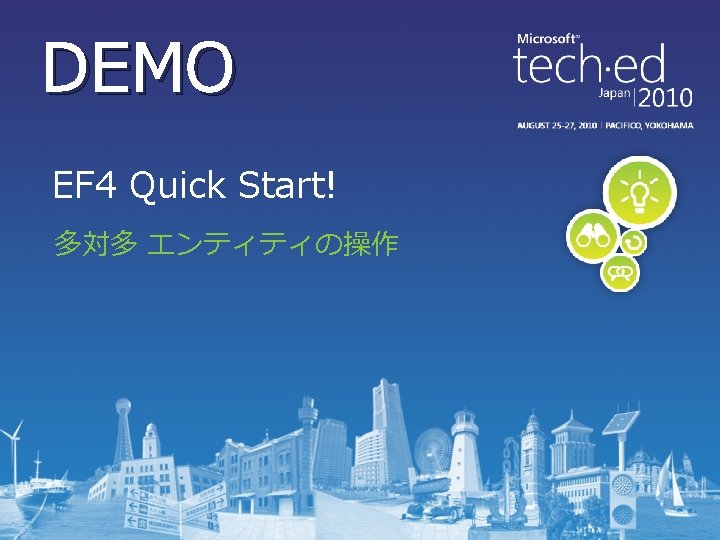
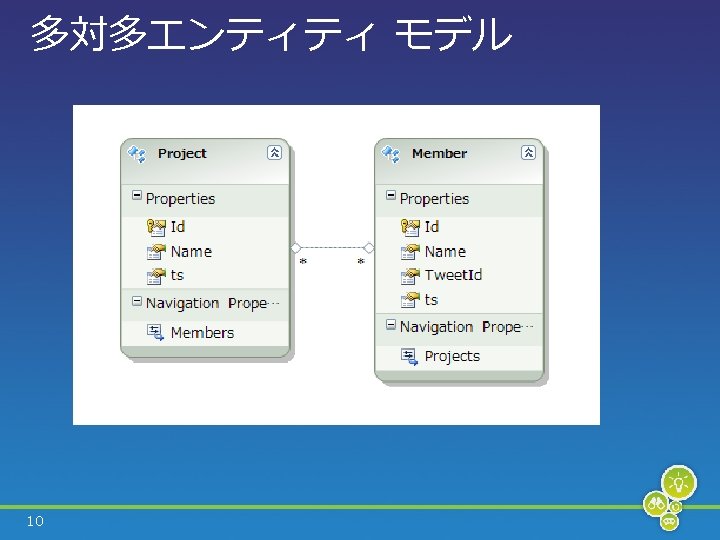
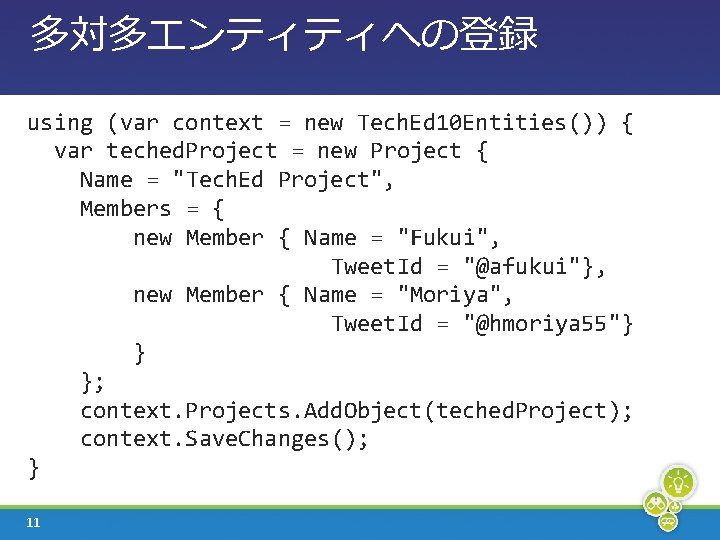
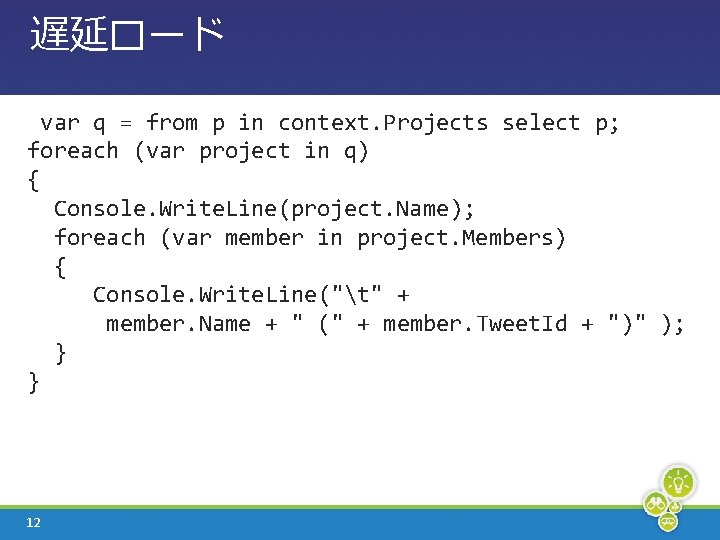
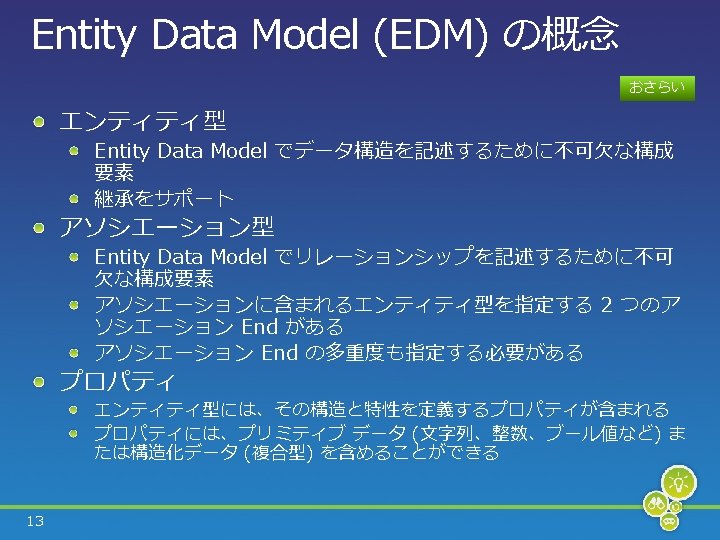
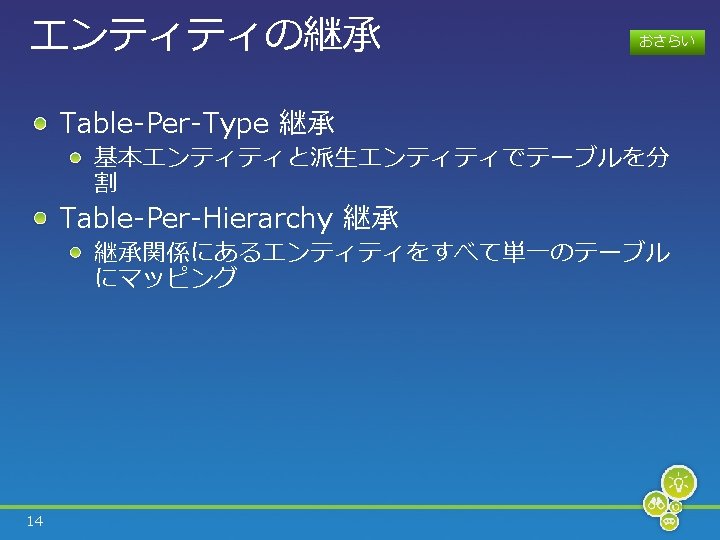
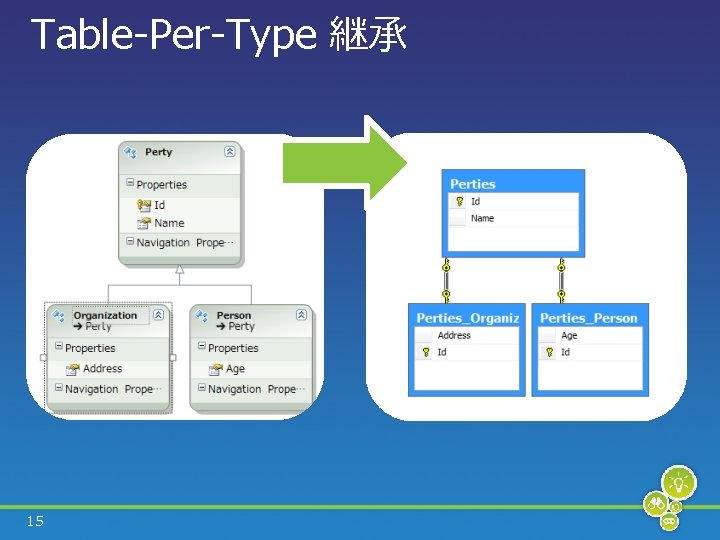
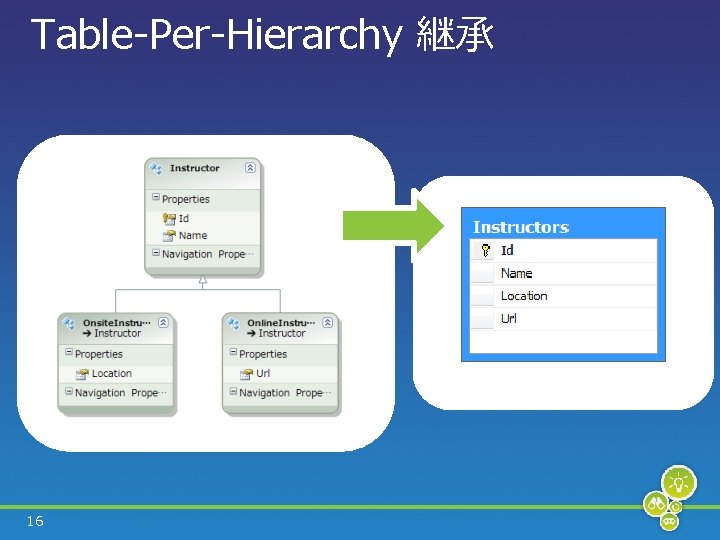
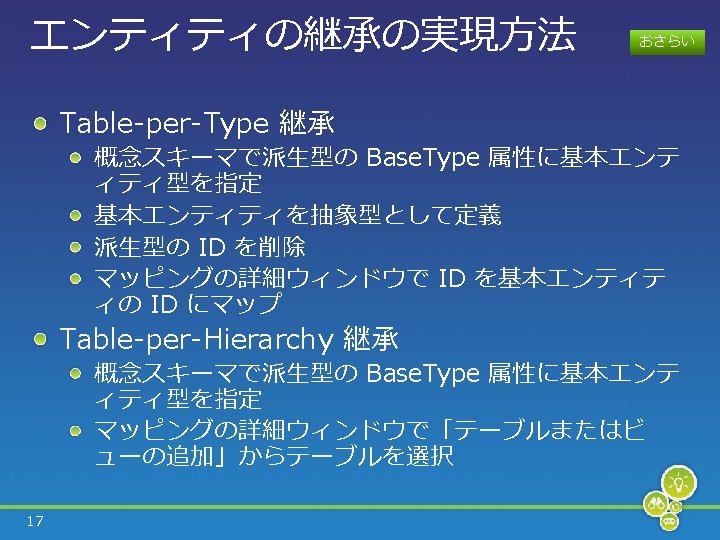
![自動生成される SQL の一部 (Table-Per-Type 継承の例) CREATE TABLE [dbo]. [Parties] ( [Id] int IDENTITY(1, 1) 自動生成される SQL の一部 (Table-Per-Type 継承の例) CREATE TABLE [dbo]. [Parties] ( [Id] int IDENTITY(1, 1)](https://slidetodoc.com/presentation_image_h2/b4b6c174360f3c4ddedb6b37f1229d76/image-18.jpg)
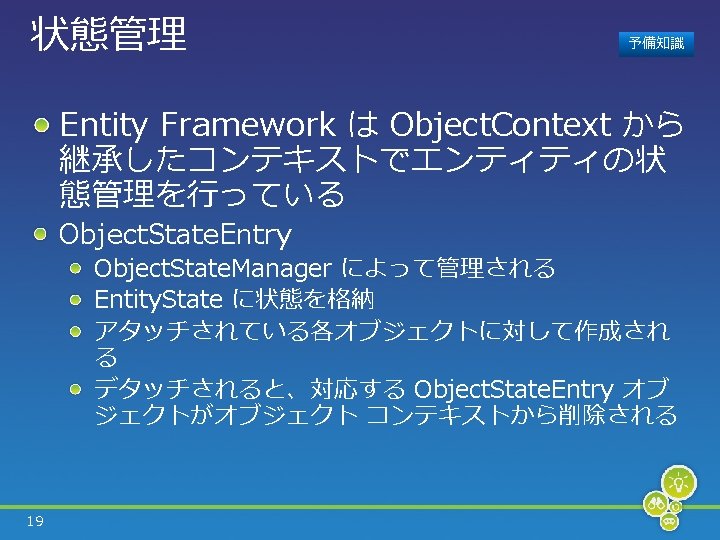
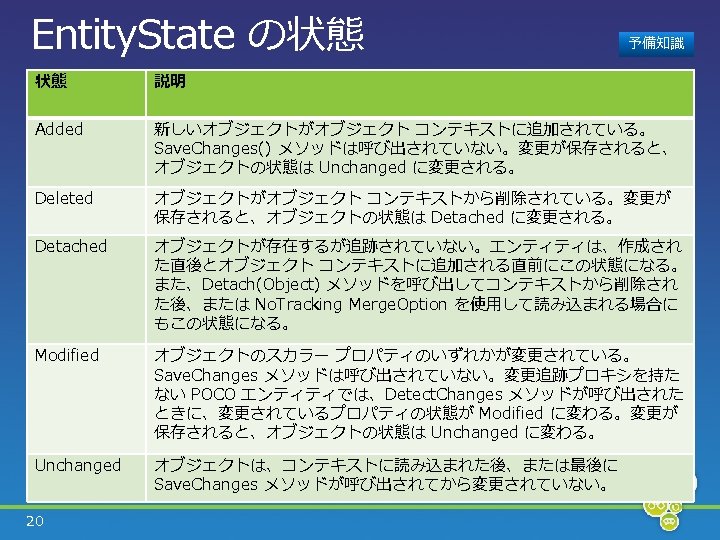
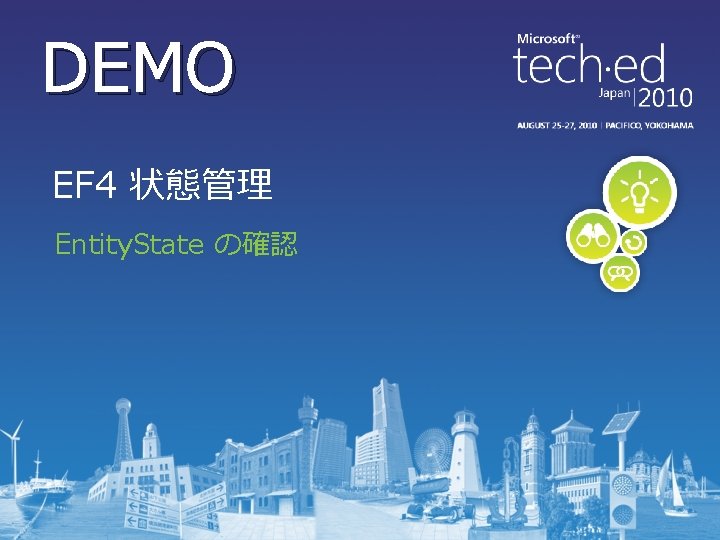
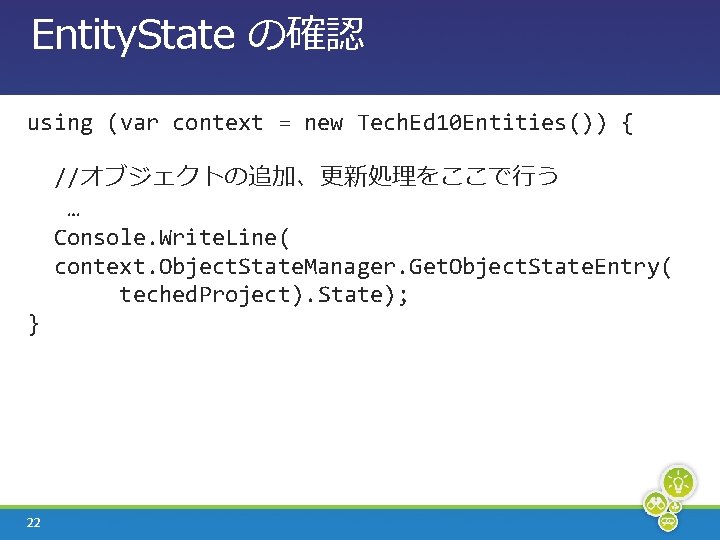
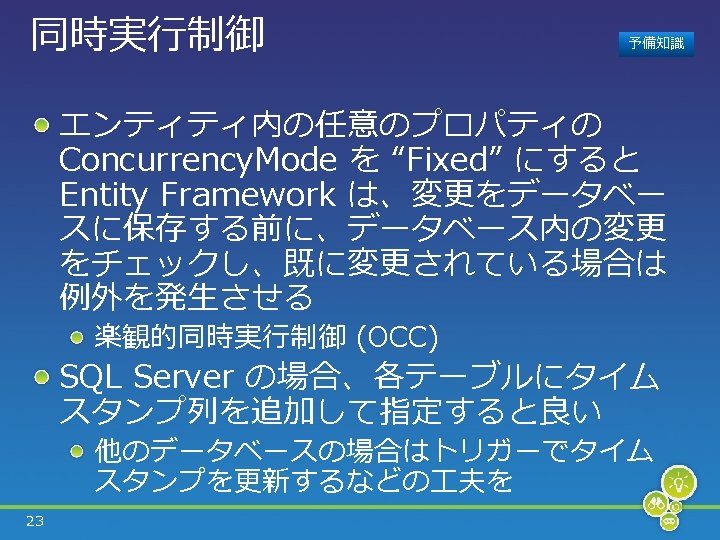
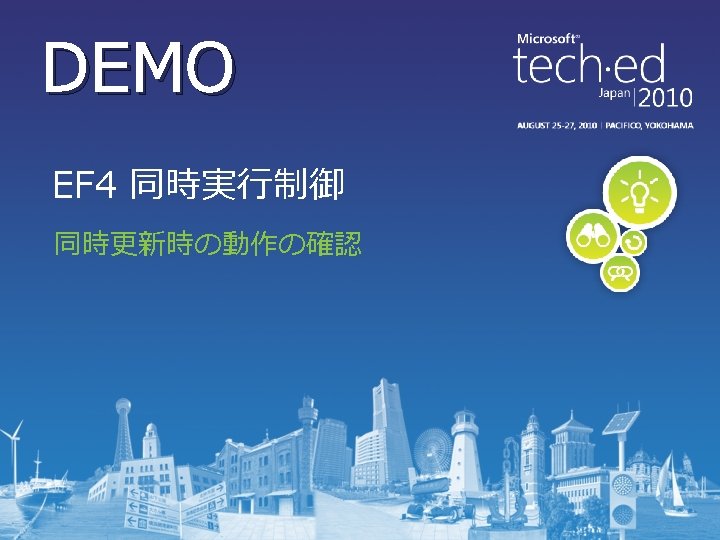
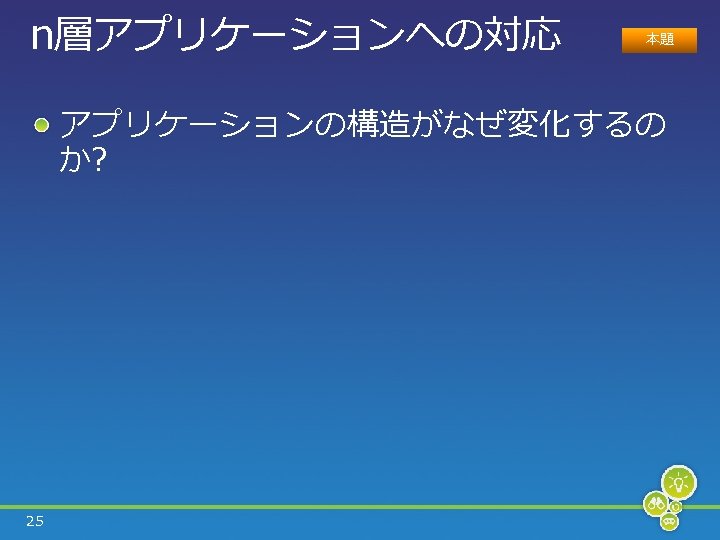
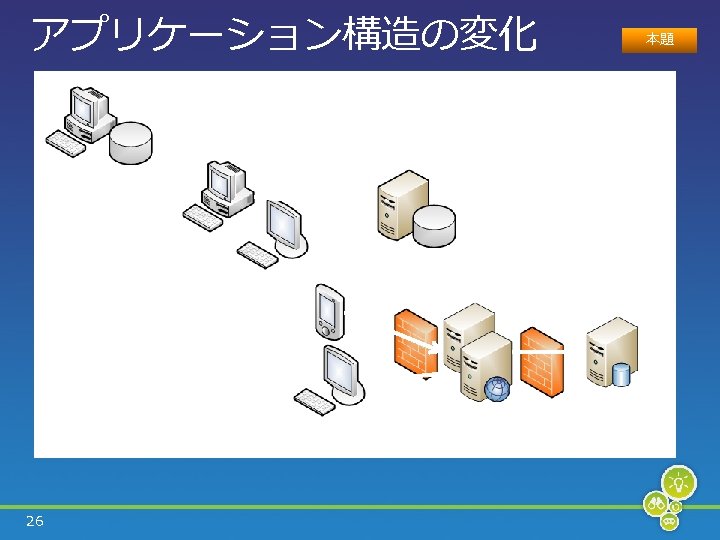
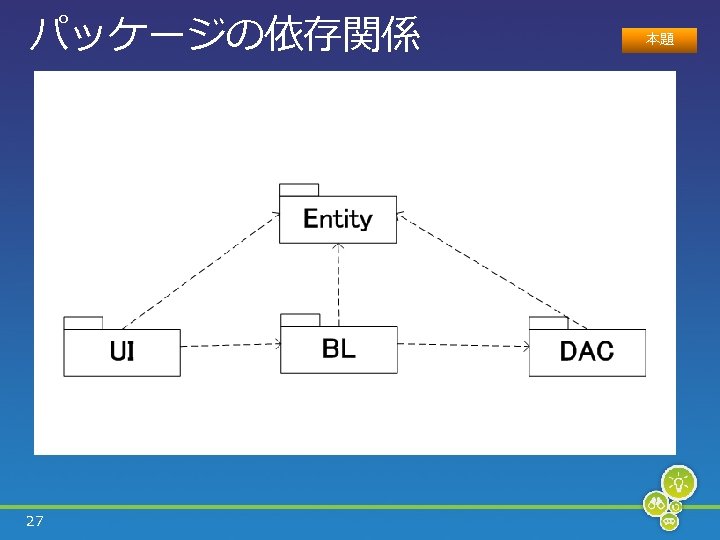
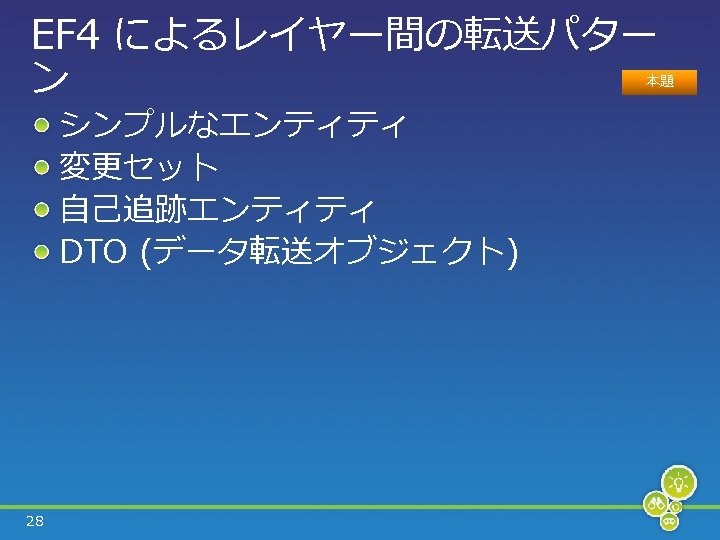
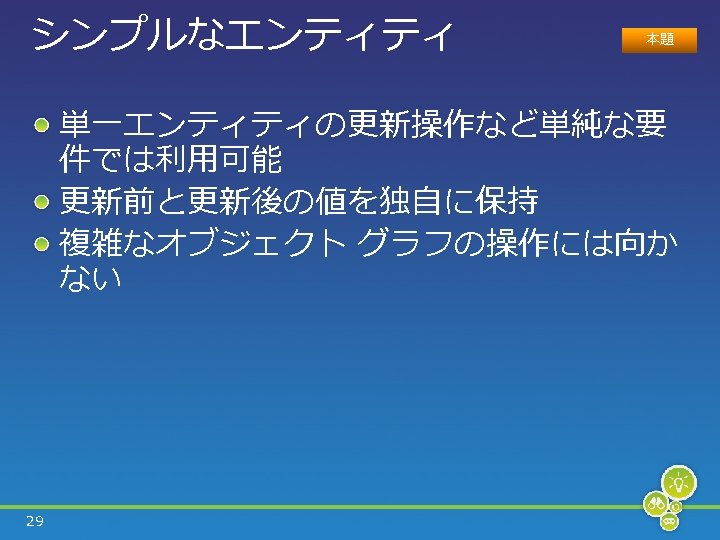
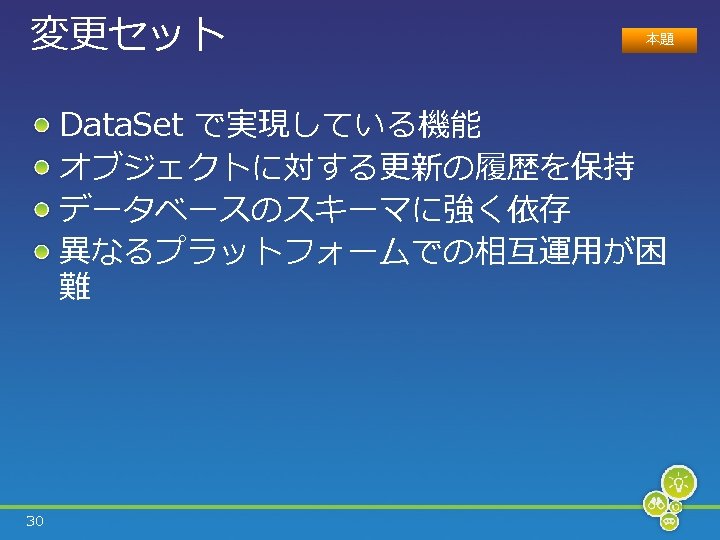
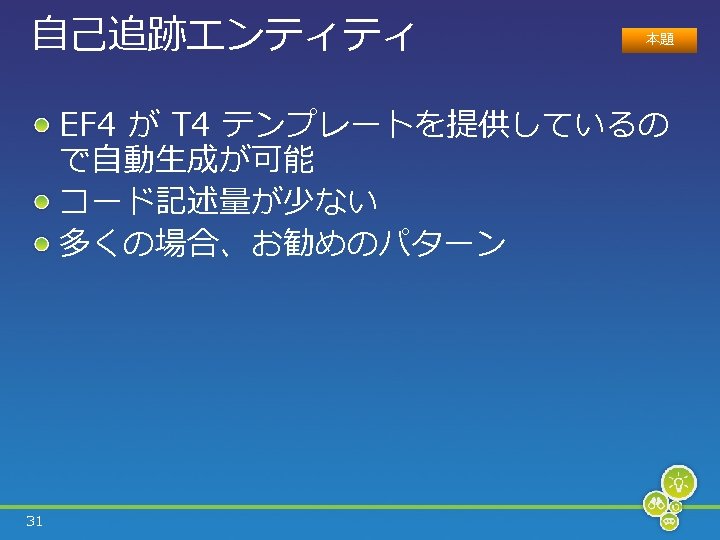
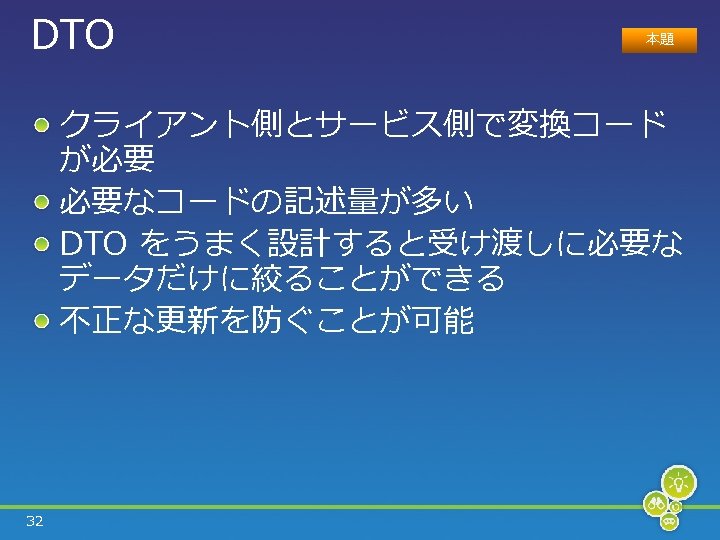
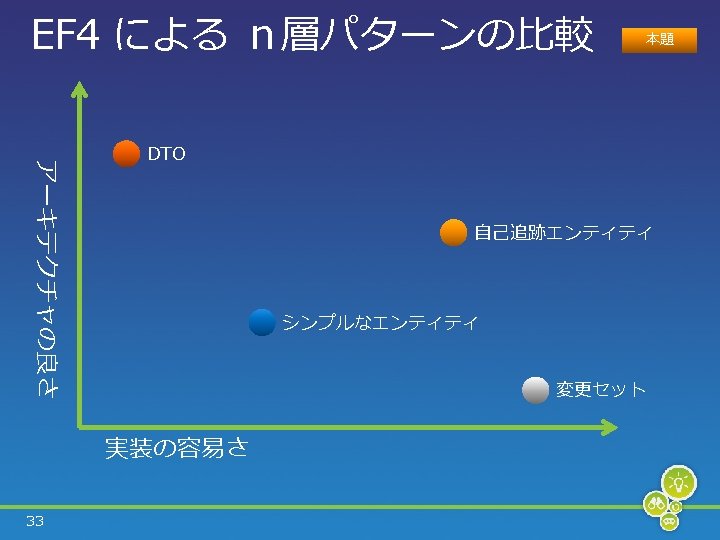
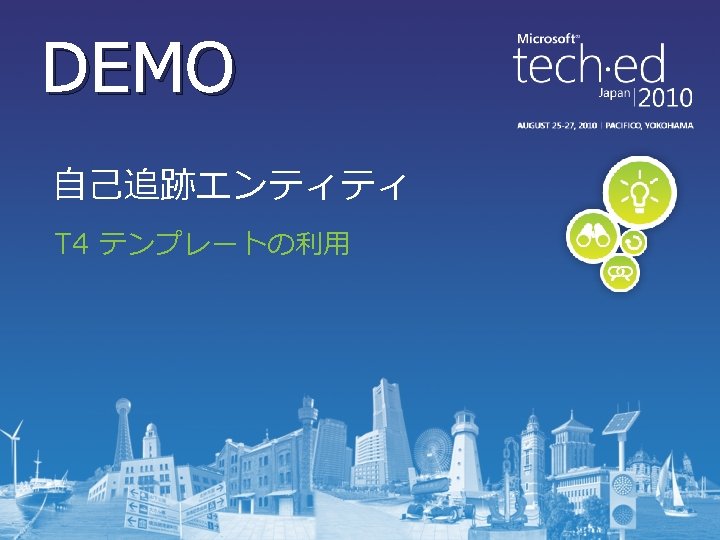
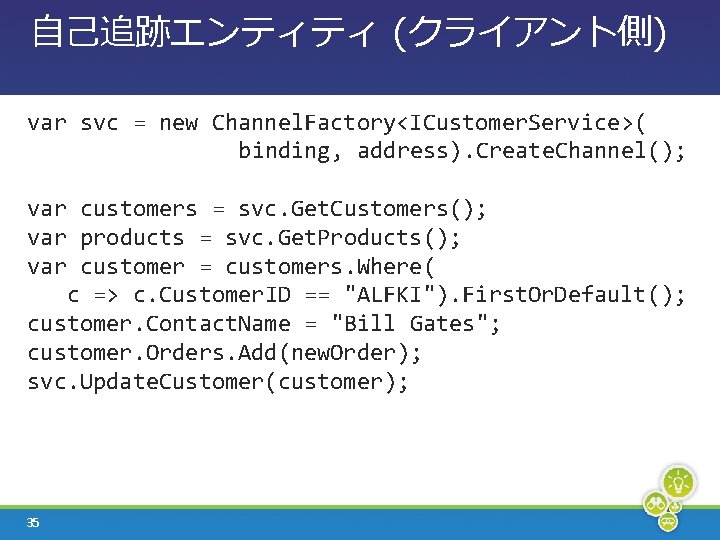
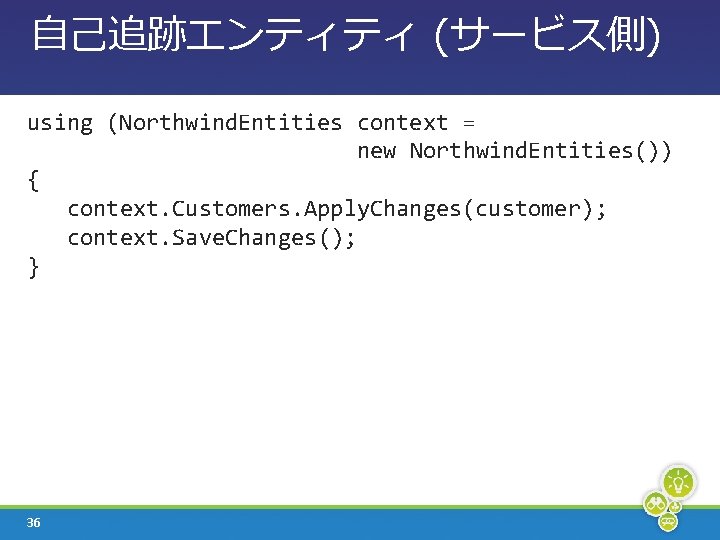
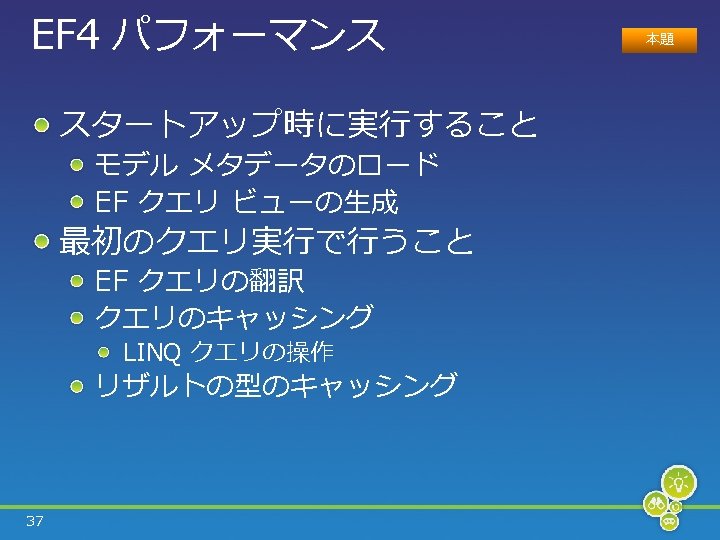
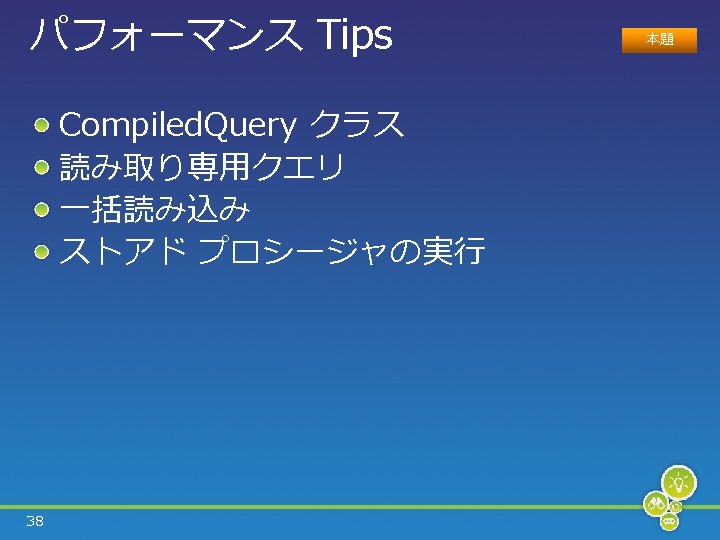
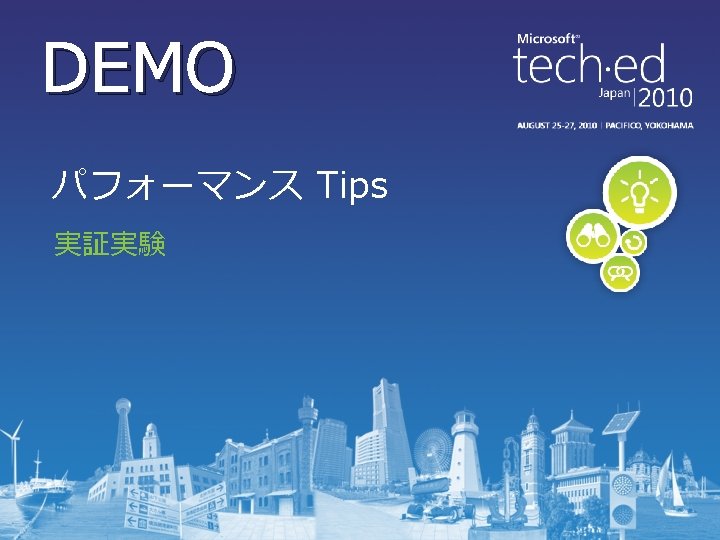
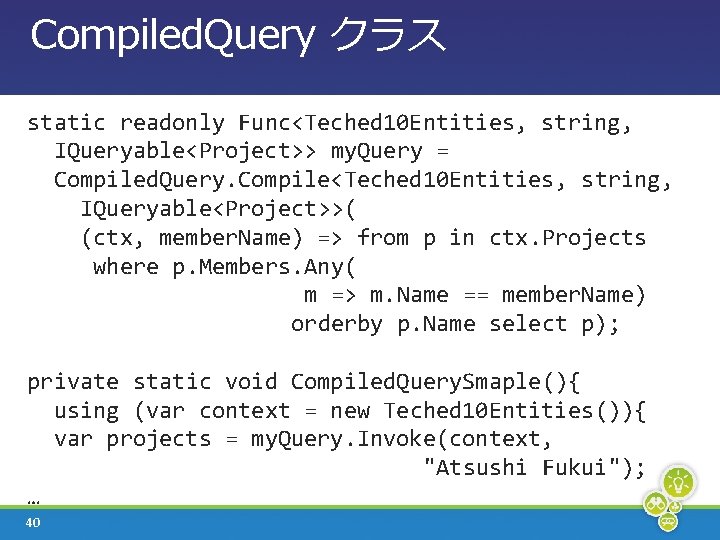
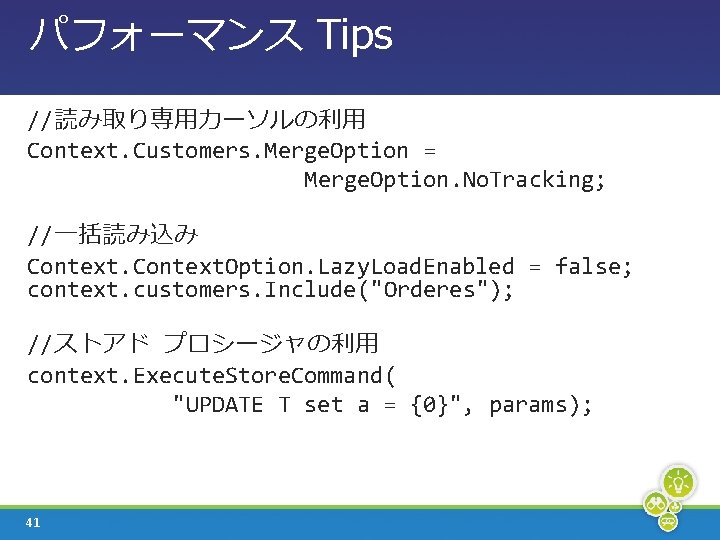
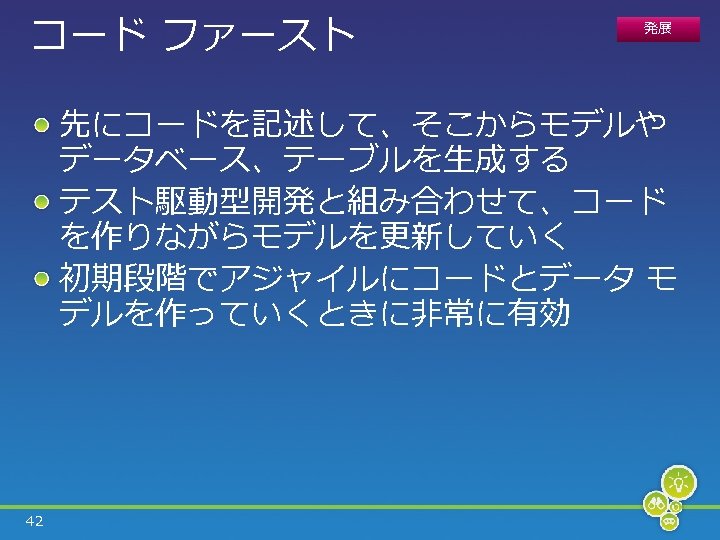
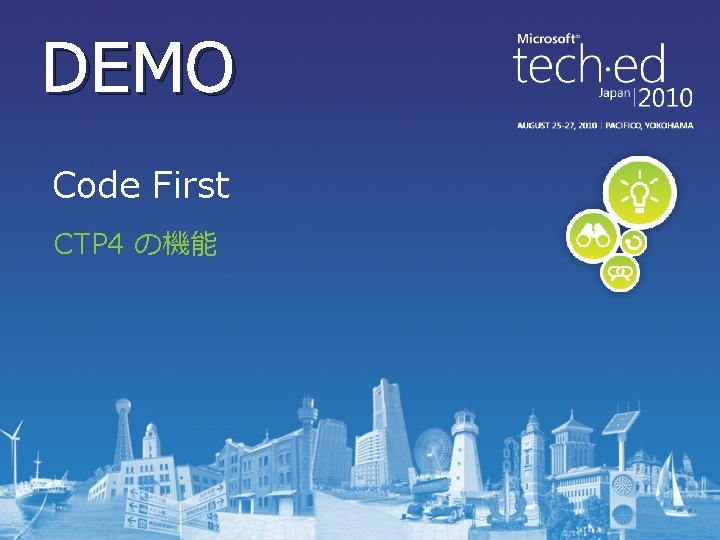
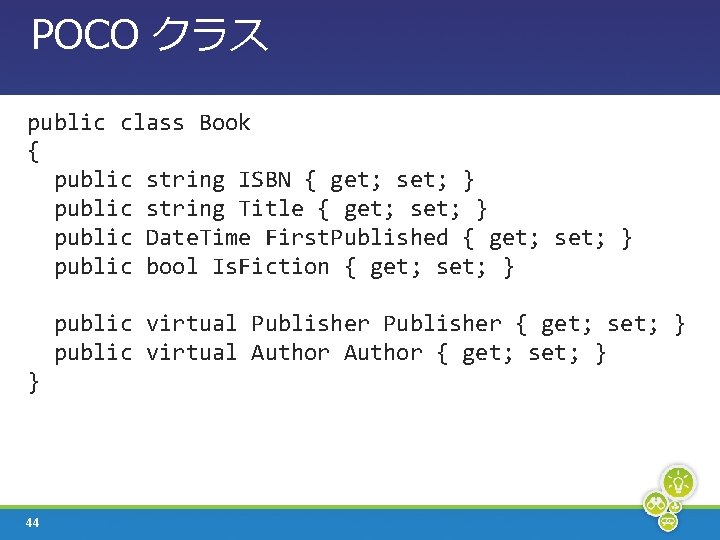
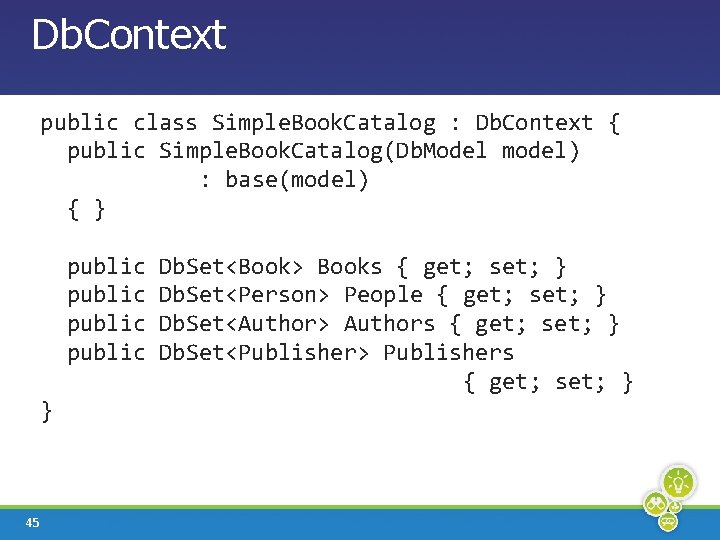
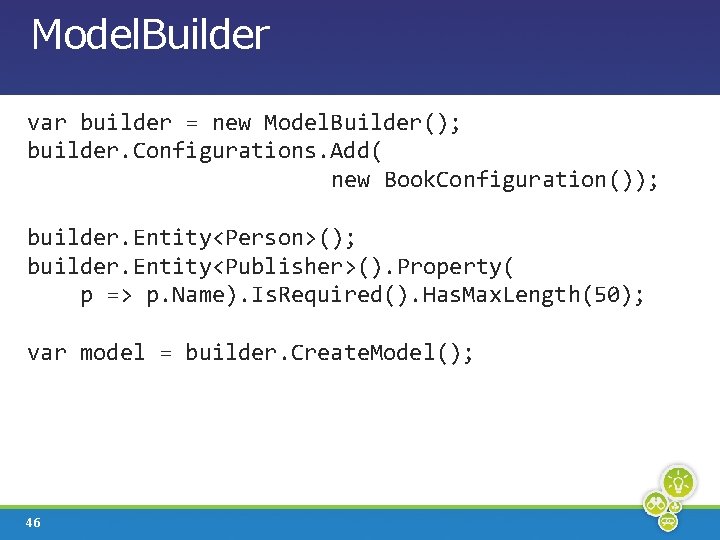
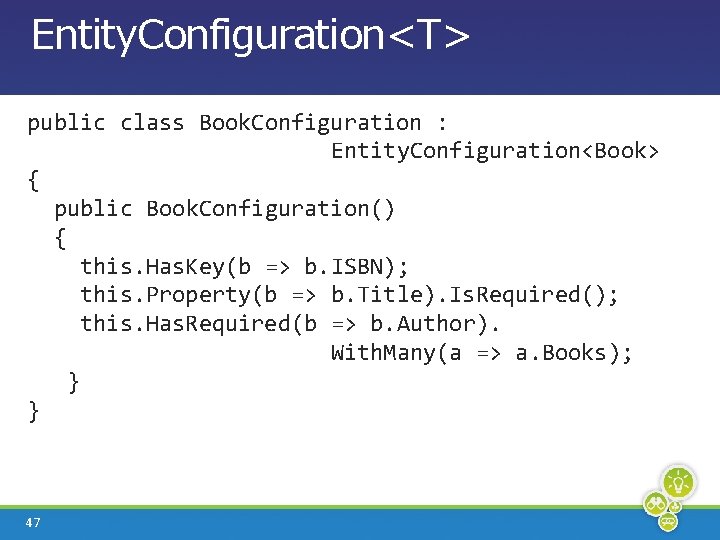
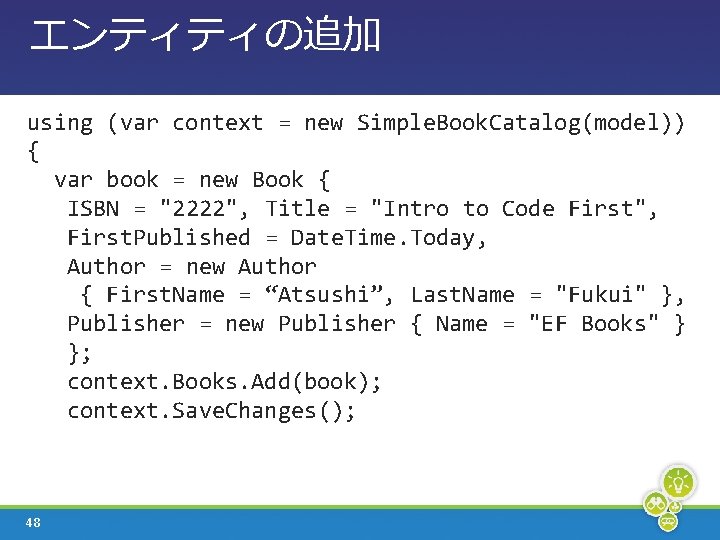
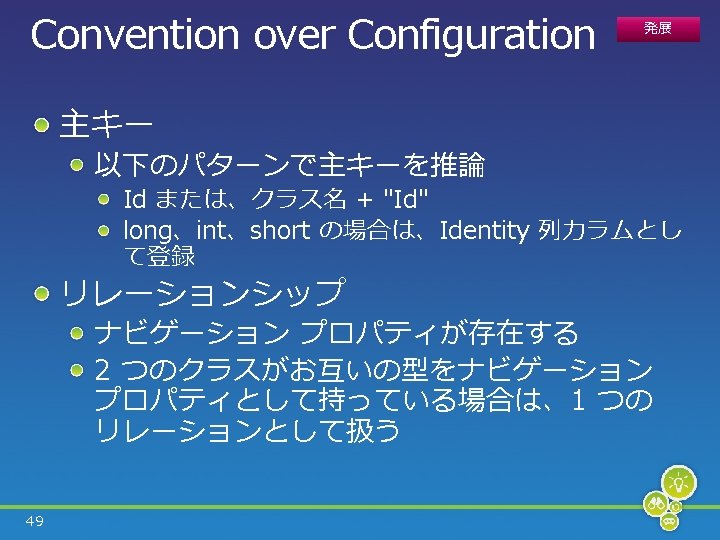
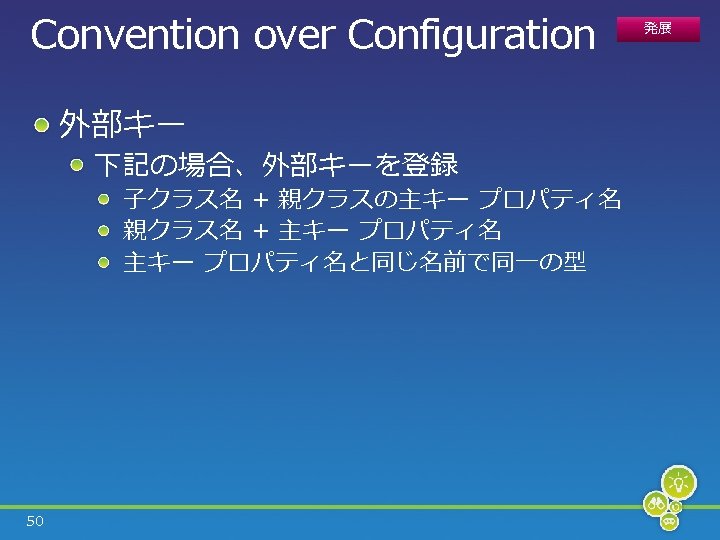
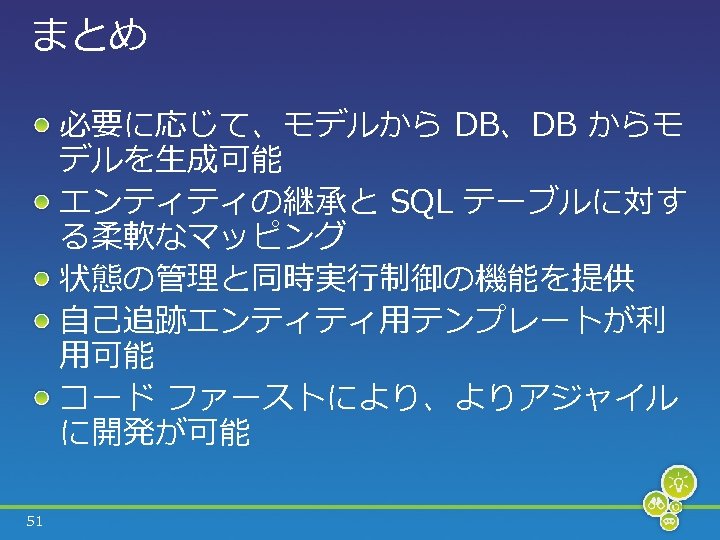
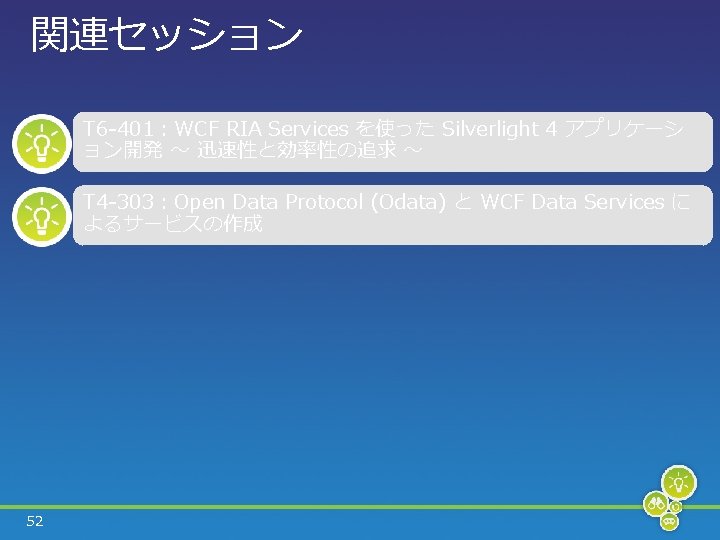
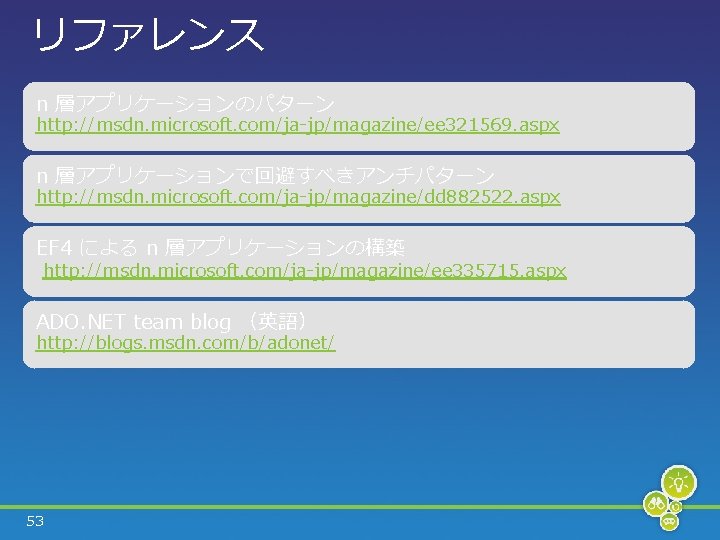
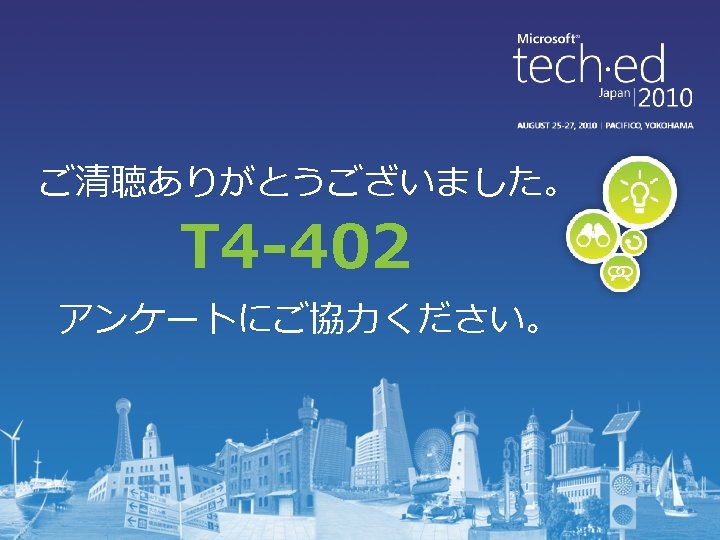
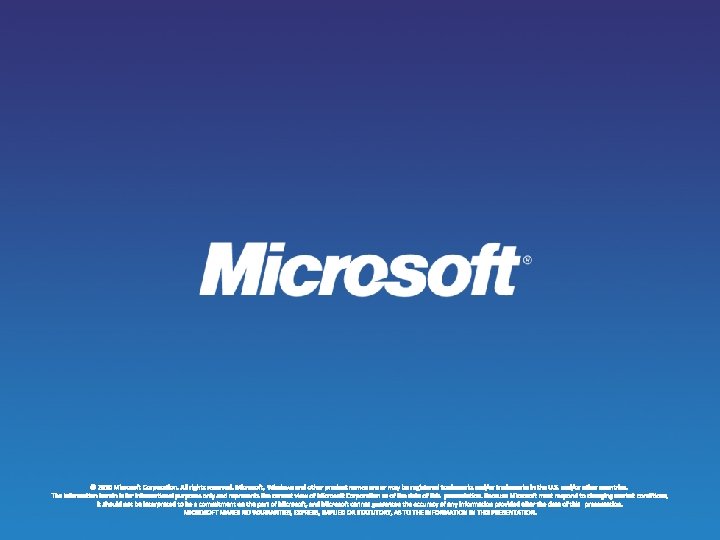
- Slides: 55
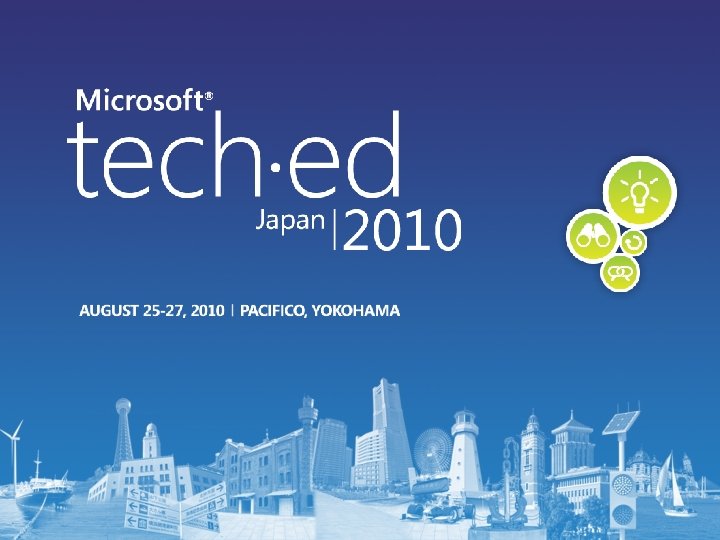
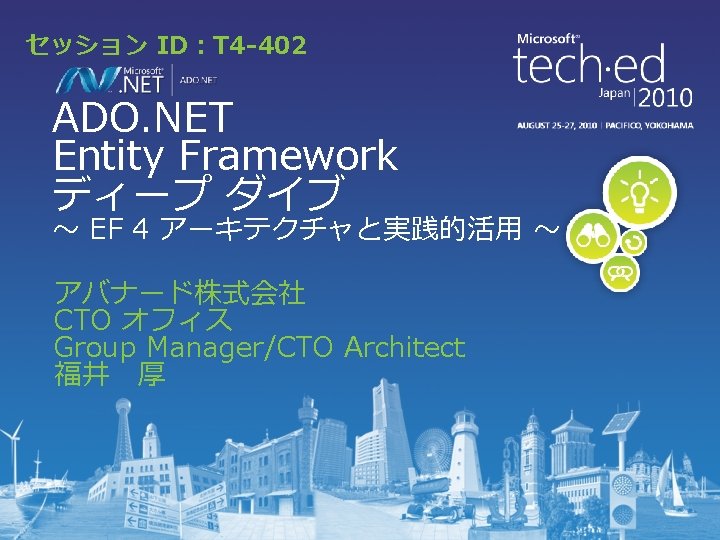
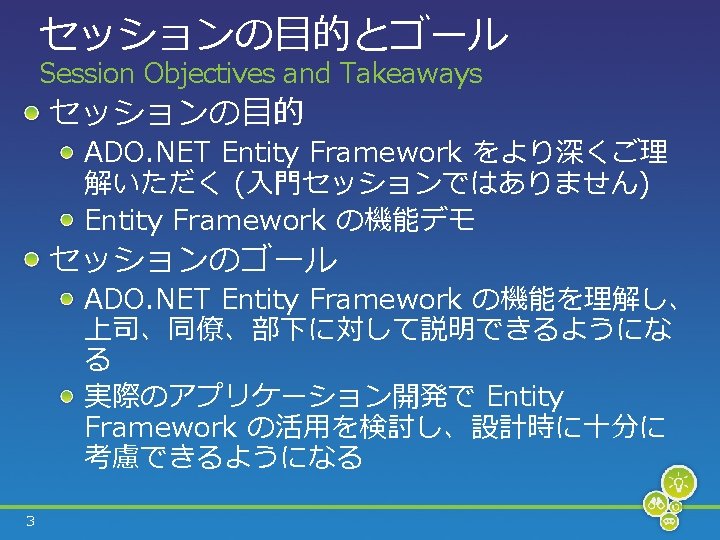
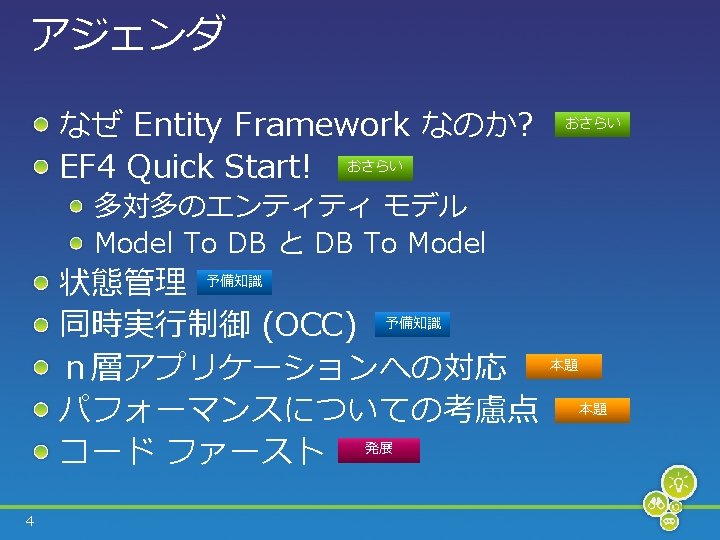
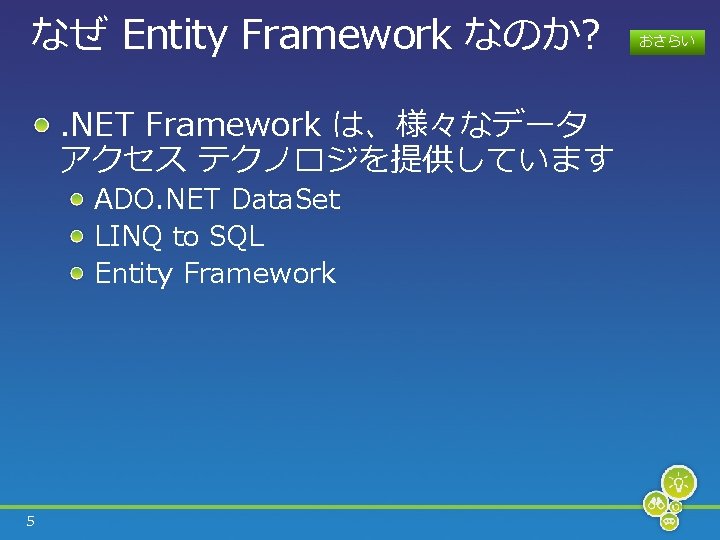
なぜ Entity Framework なのか? . NET Framework は、様々なデータ アクセス テクノロジを提供しています ADO. NET Data. Set LINQ to SQL Entity Framework 5 おさらい
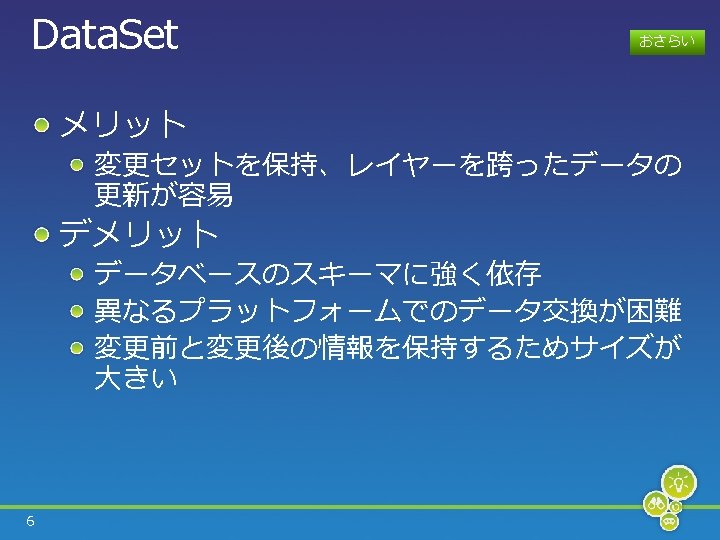
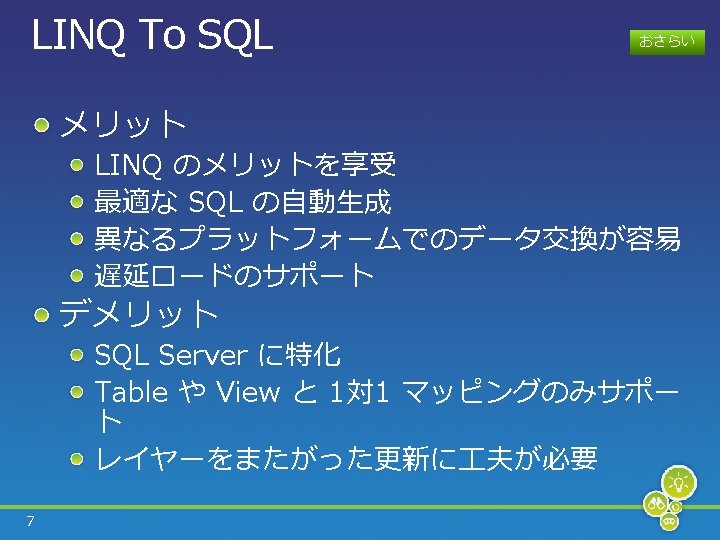
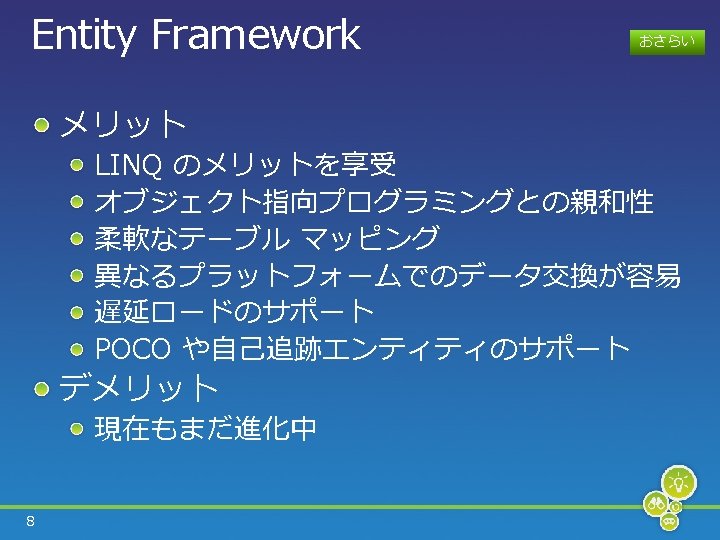
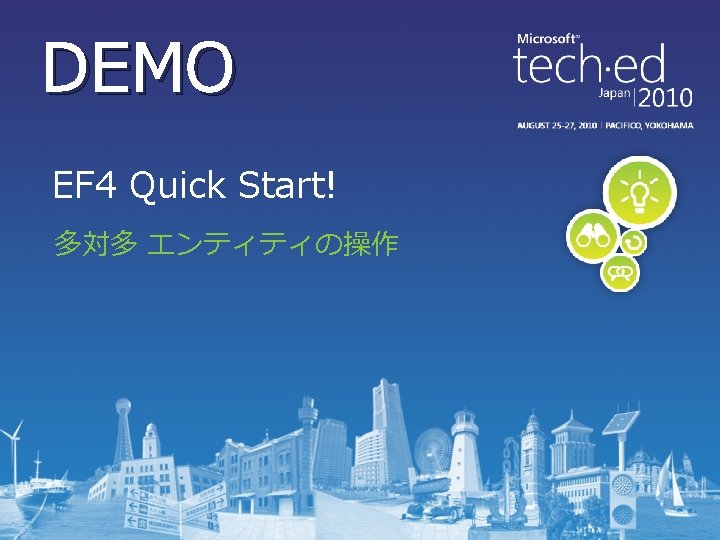
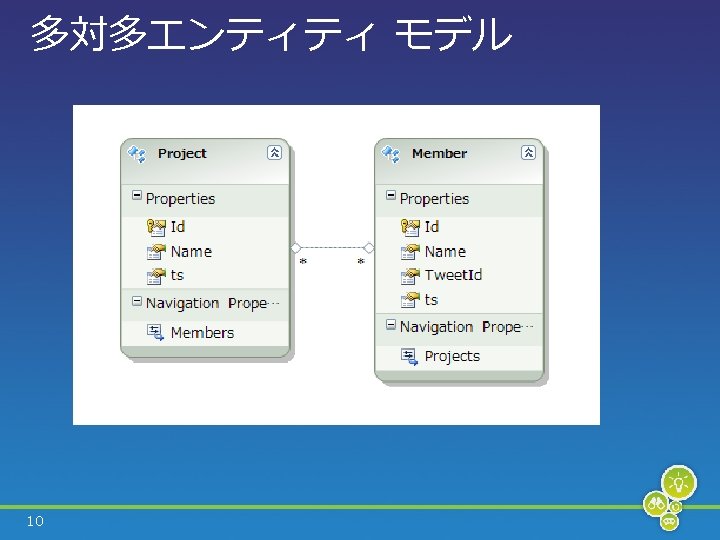
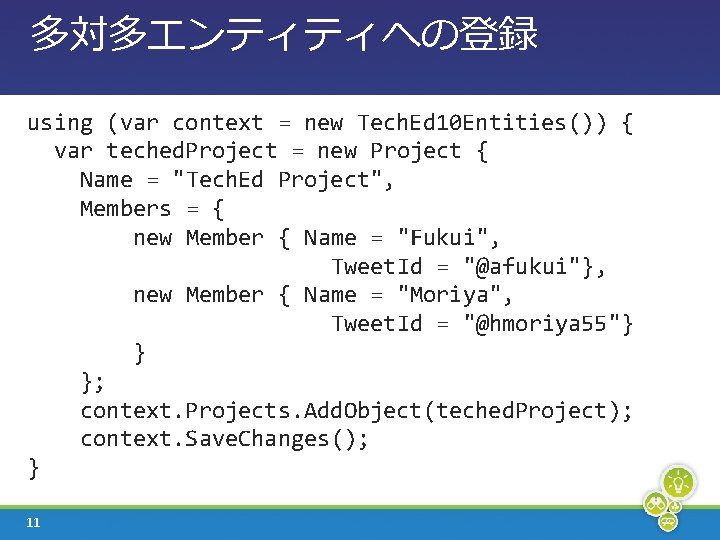
多対多エンティティへの登録 using (var context = new Tech. Ed 10 Entities()) { var teched. Project = new Project { Name = "Tech. Ed Project", Members = { new Member { Name = "Fukui", Tweet. Id = "@afukui"}, new Member { Name = "Moriya", Tweet. Id = "@hmoriya 55"} } }; context. Projects. Add. Object(teched. Project); context. Save. Changes(); } 11
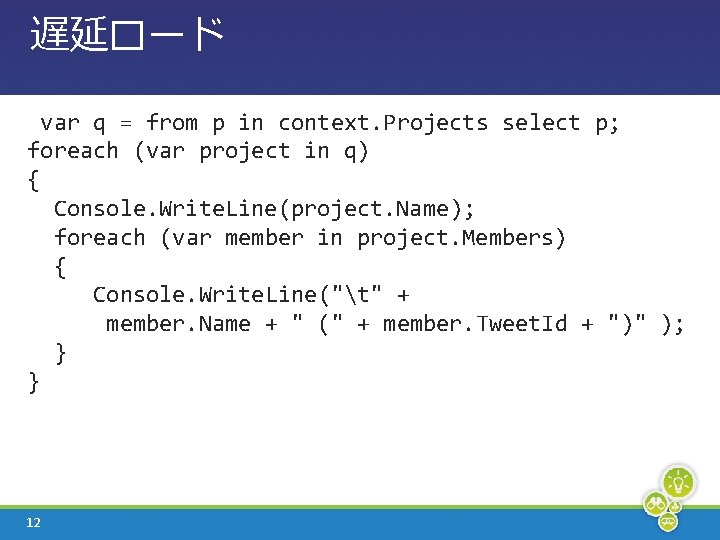
遅延ロード var q = from p in context. Projects select p; foreach (var project in q) { Console. Write. Line(project. Name); foreach (var member in project. Members) { Console. Write. Line("t" + member. Name + " (" + member. Tweet. Id + ")" ); } } 12
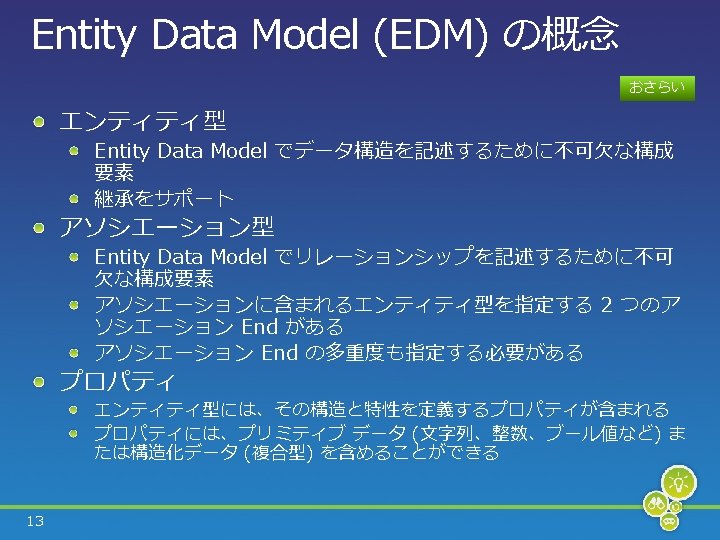
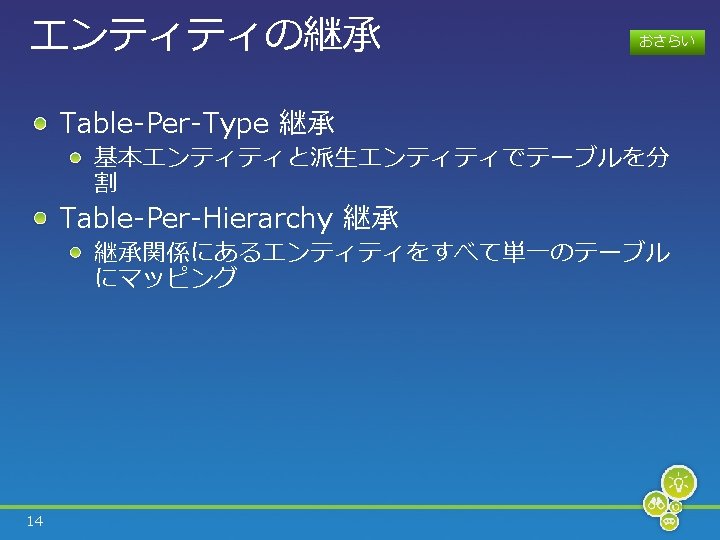
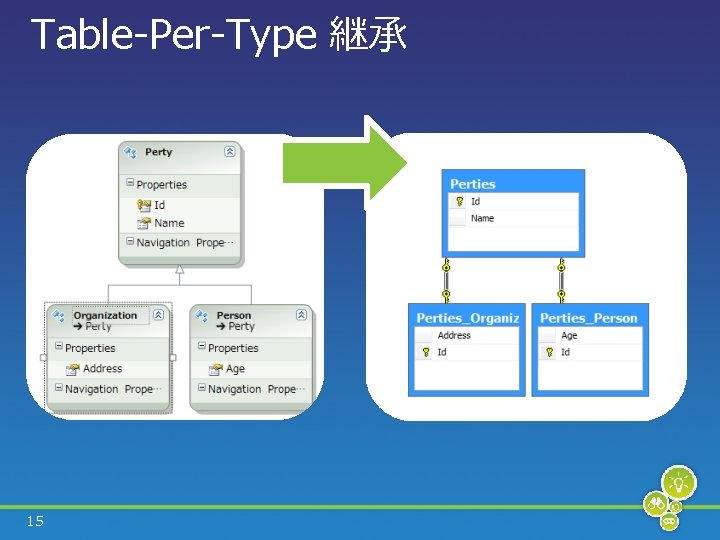
Table-Per-Type 継承 15
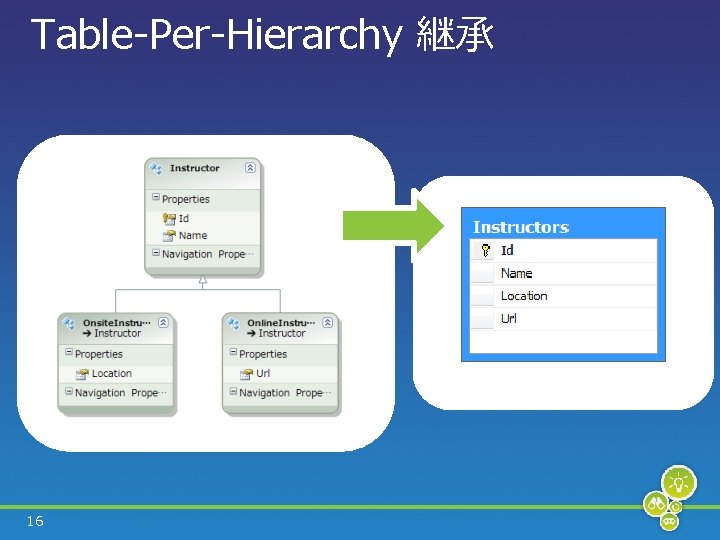
Table-Per-Hierarchy 継承 16
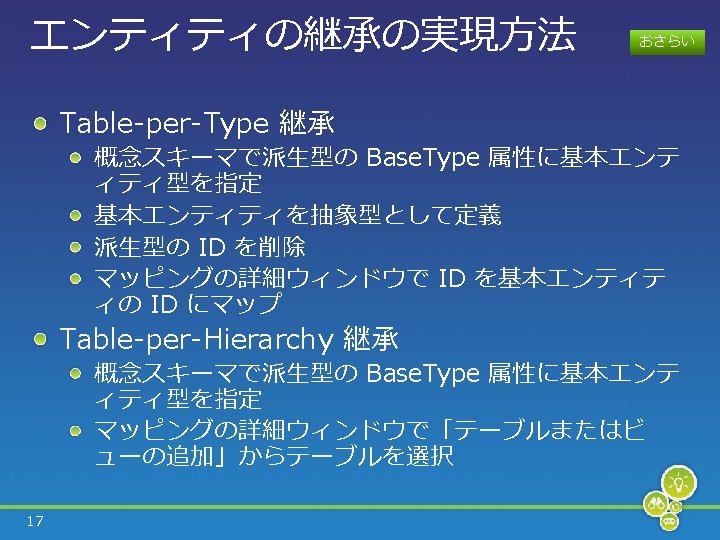
![自動生成される SQL の一部 TablePerType 継承の例 CREATE TABLE dbo Parties Id int IDENTITY1 1 自動生成される SQL の一部 (Table-Per-Type 継承の例) CREATE TABLE [dbo]. [Parties] ( [Id] int IDENTITY(1, 1)](https://slidetodoc.com/presentation_image_h2/b4b6c174360f3c4ddedb6b37f1229d76/image-18.jpg)
自動生成される SQL の一部 (Table-Per-Type 継承の例) CREATE TABLE [dbo]. [Parties] ( [Id] int IDENTITY(1, 1) NOT NULL, [Name] nvarchar(max) NOT NULL ); GO CREATE TABLE [dbo]. [Parties_Organization] ( [Location] nvarchar(max) NOT NULL, [Id] int NOT NULL ); GO CREATE TABLE [dbo]. [Parties_Person] ( [Age] int NOT NULL, [Id] int NOT NULL ); GO 18
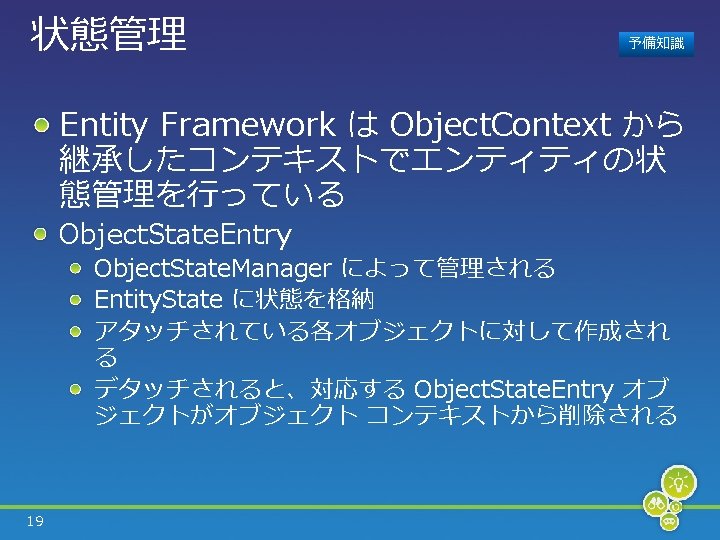
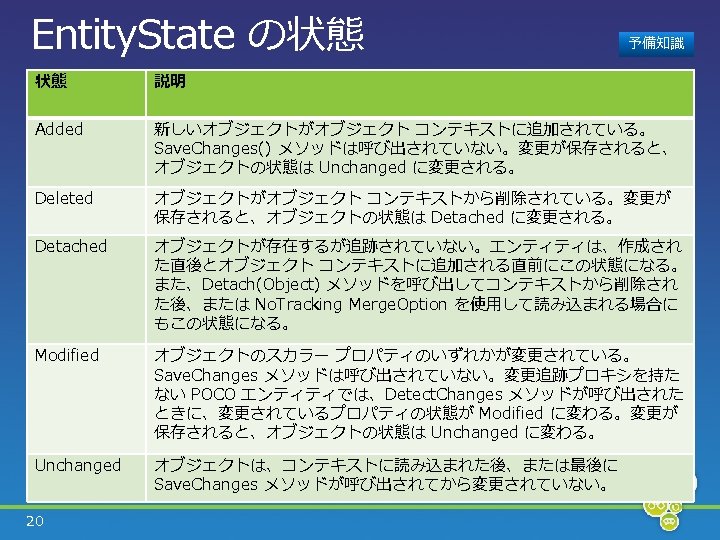
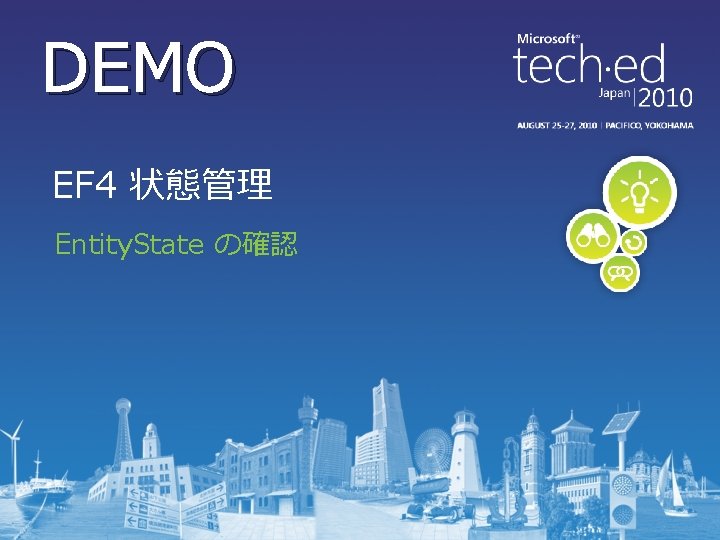
DEMO EF 4 状態管理 Entity. State の確認
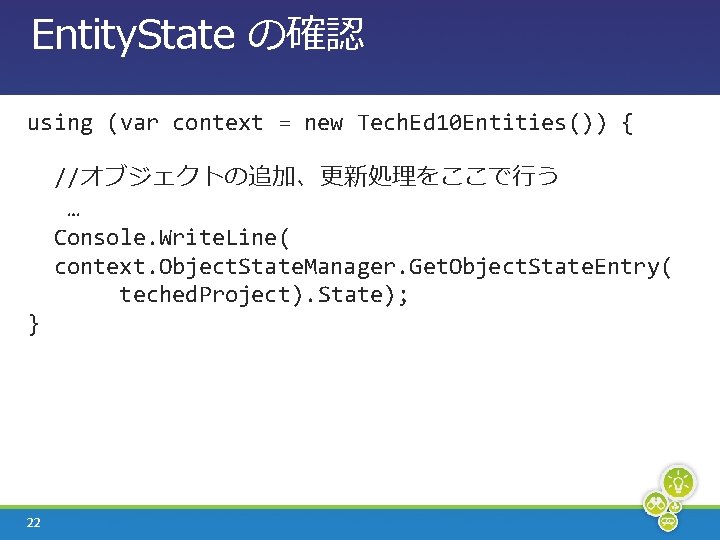
Entity. State の確認 using (var context = new Tech. Ed 10 Entities()) { //オブジェクトの追加、更新処理をここで行う … Console. Write. Line( context. Object. State. Manager. Get. Object. State. Entry( teched. Project). State); } 22
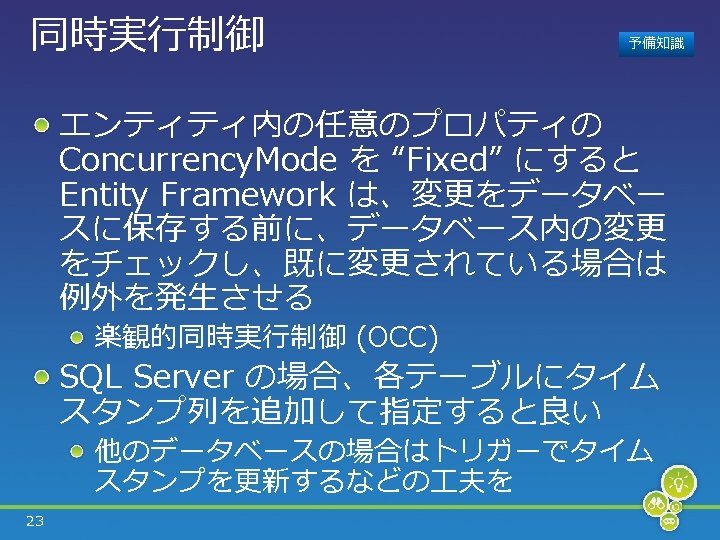
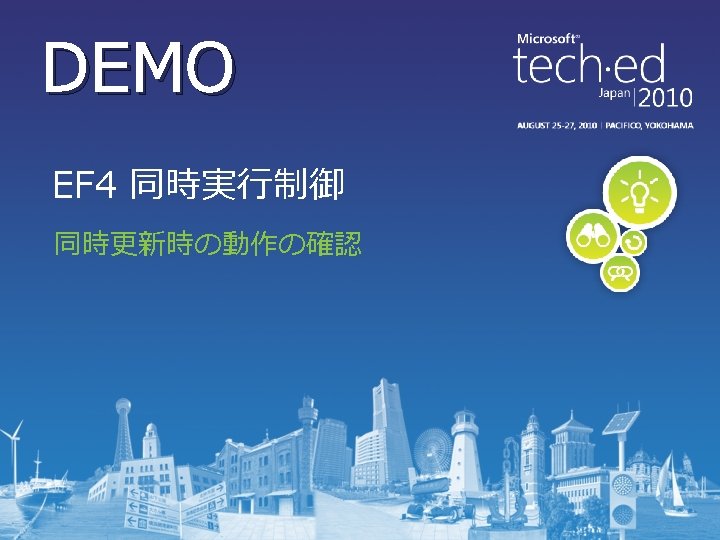
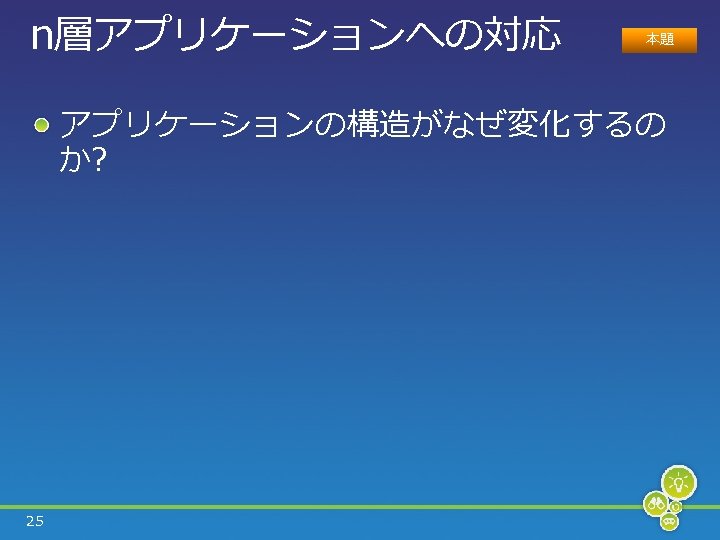
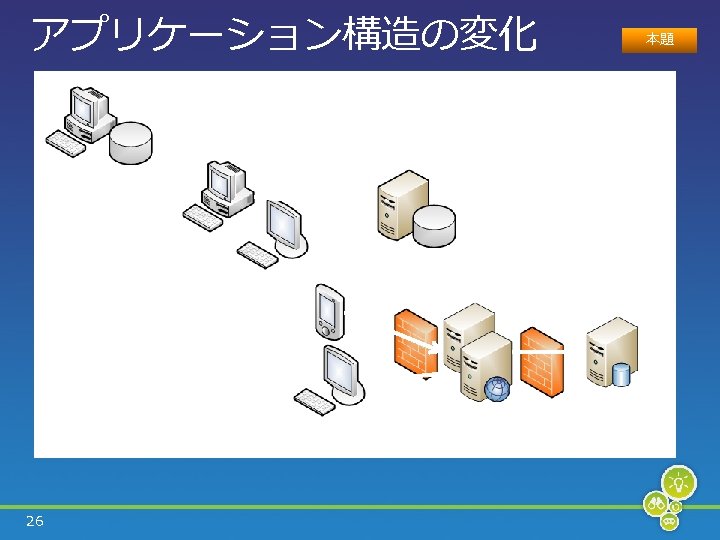
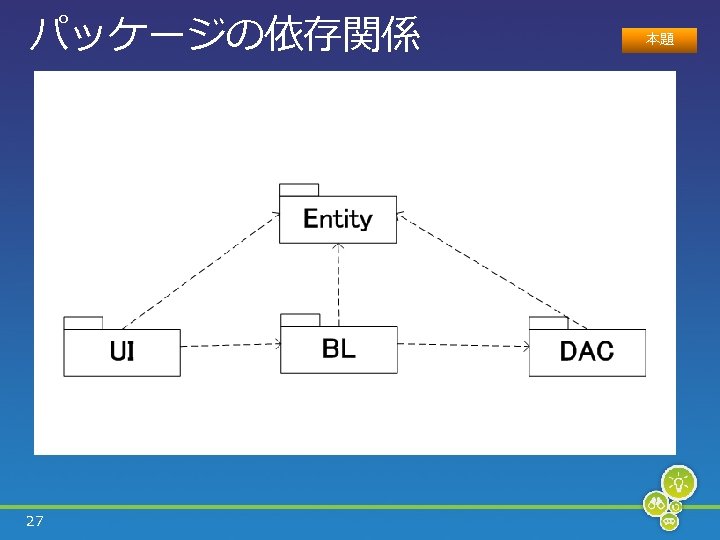
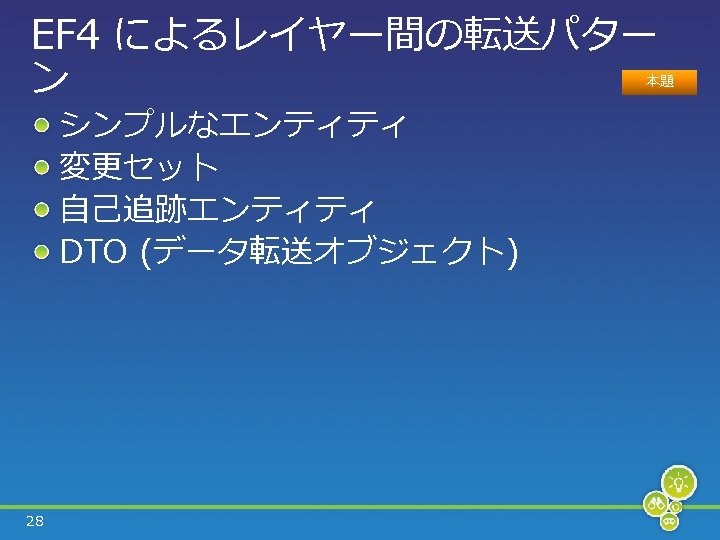
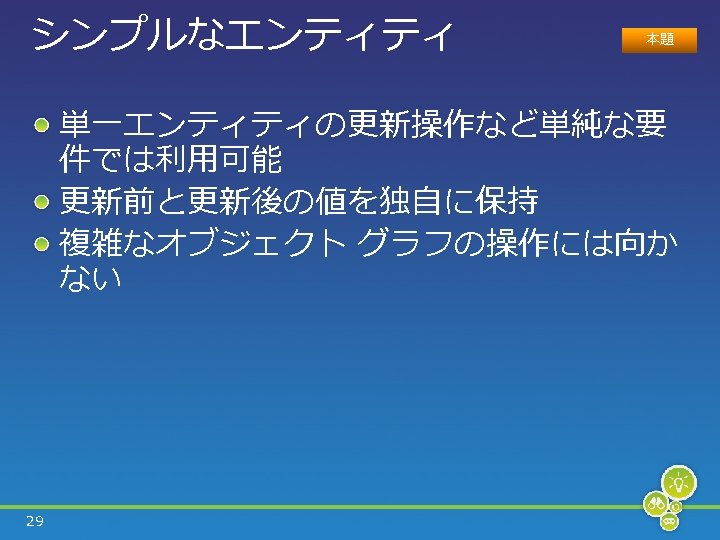
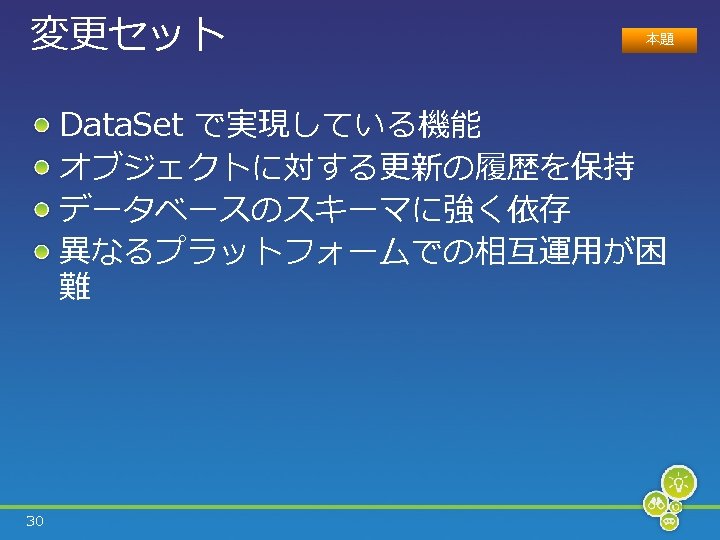
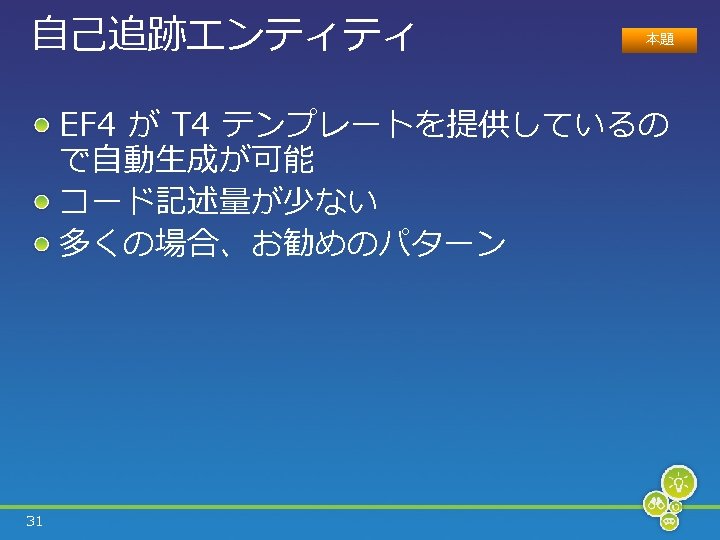
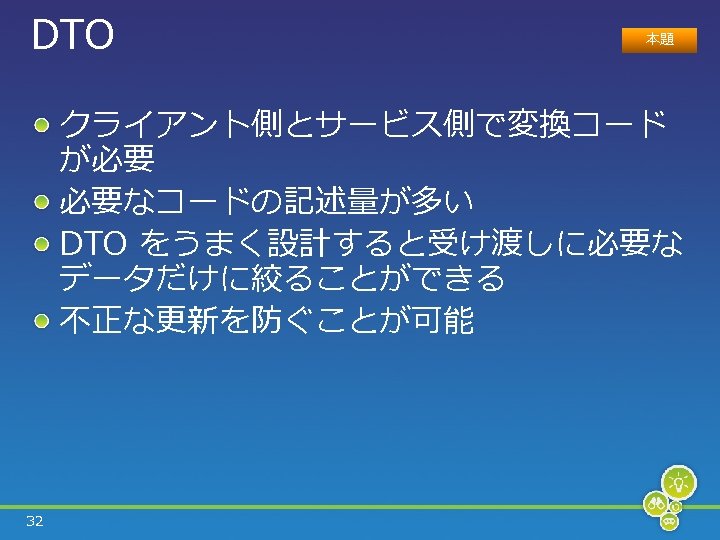
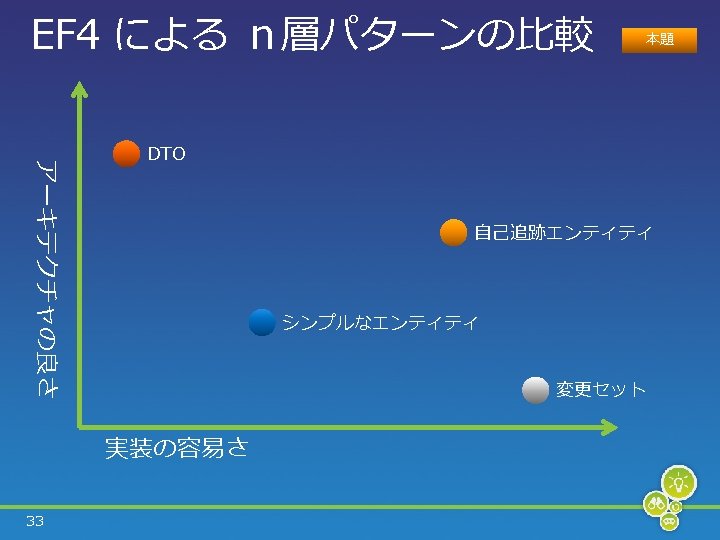
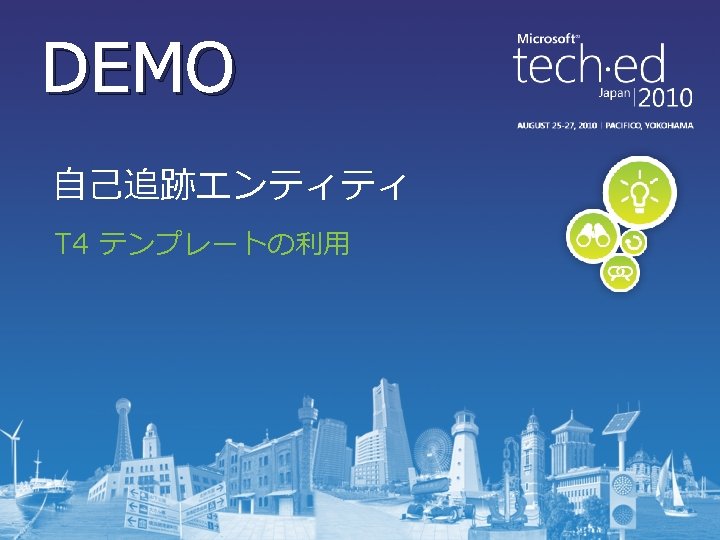
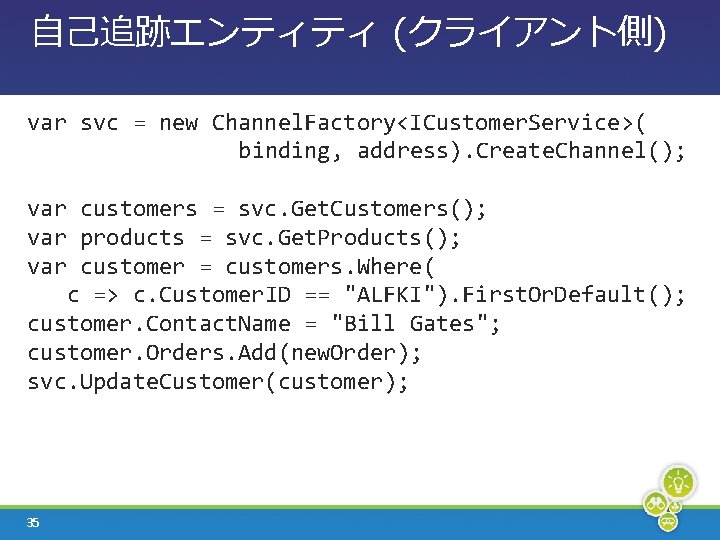
自己追跡エンティティ (クライアント側) var svc = new Channel. Factory<ICustomer. Service>( binding, address). Create. Channel(); var customers = svc. Get. Customers(); var products = svc. Get. Products(); var customer = customers. Where( c => c. Customer. ID == "ALFKI"). First. Or. Default(); customer. Contact. Name = "Bill Gates"; customer. Orders. Add(new. Order); svc. Update. Customer(customer); 35
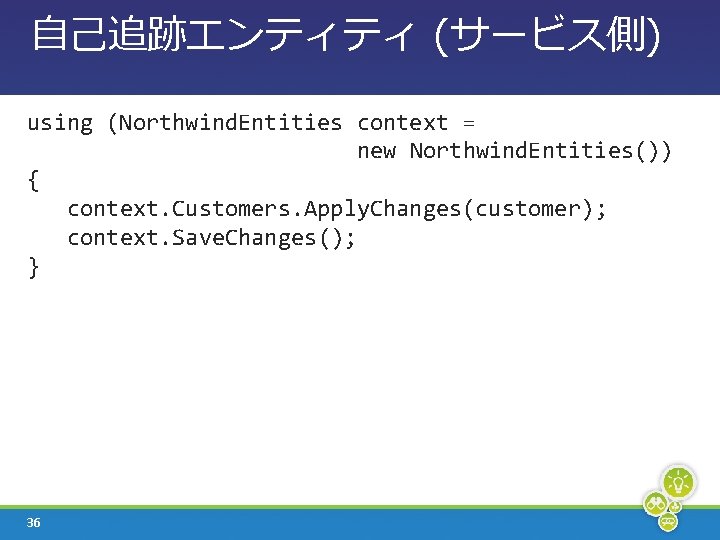
自己追跡エンティティ (サービス側) using (Northwind. Entities context = new Northwind. Entities()) { context. Customers. Apply. Changes(customer); context. Save. Changes(); } 36
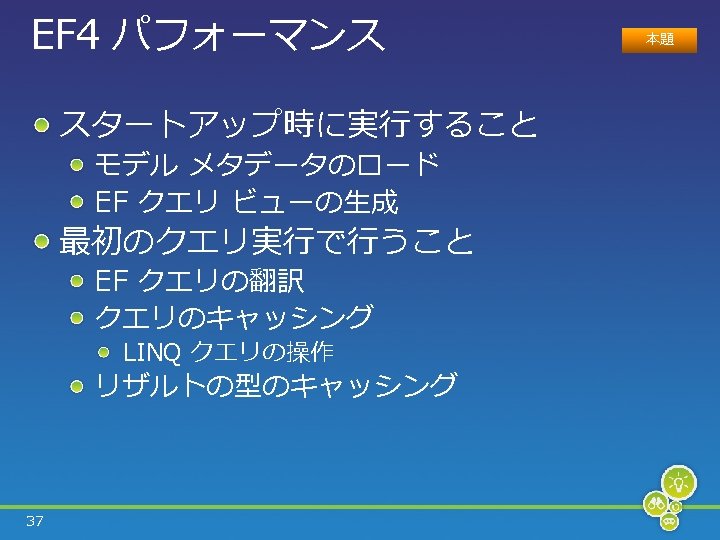
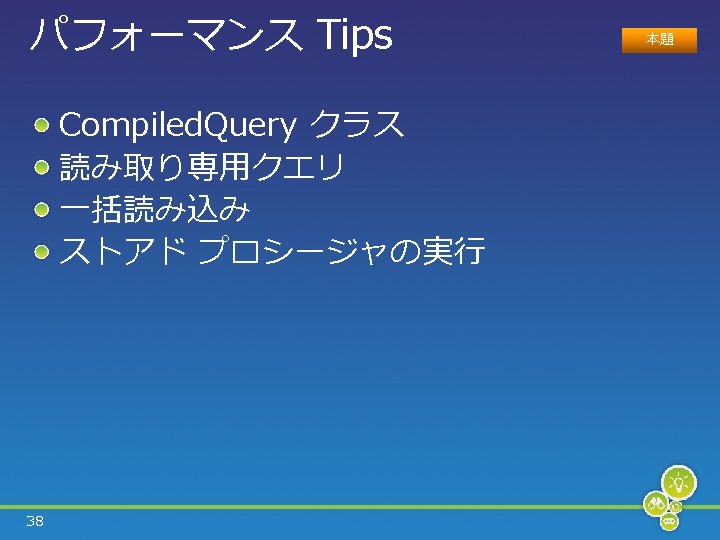
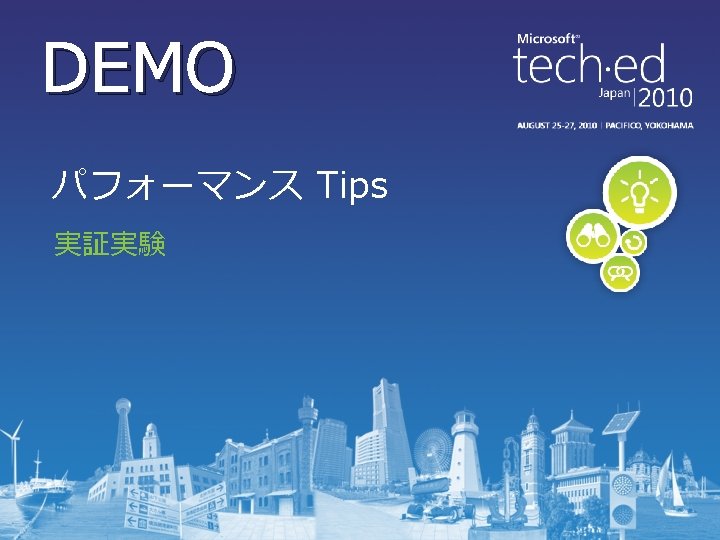
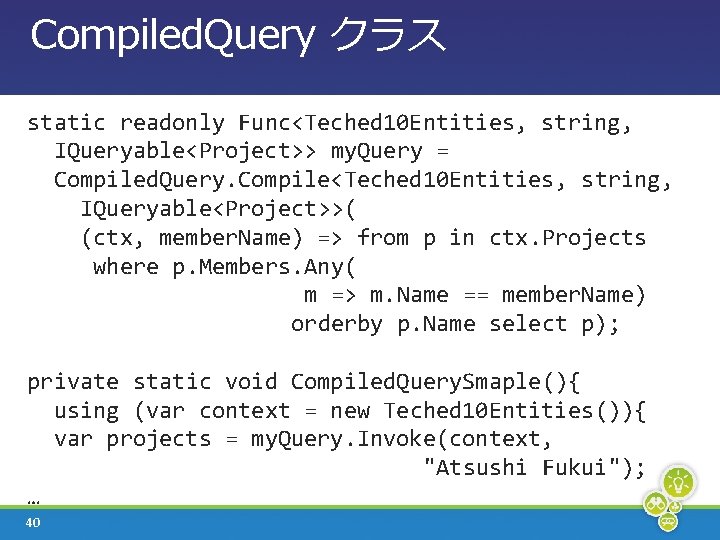
Compiled. Query クラス static readonly Func<Teched 10 Entities, string, IQueryable<Project>> my. Query = Compiled. Query. Compile<Teched 10 Entities, string, IQueryable<Project>>( (ctx, member. Name) => from p in ctx. Projects where p. Members. Any( m => m. Name == member. Name) orderby p. Name select p); private static void Compiled. Query. Smaple(){ using (var context = new Teched 10 Entities()){ var projects = my. Query. Invoke(context, "Atsushi Fukui"); … 40
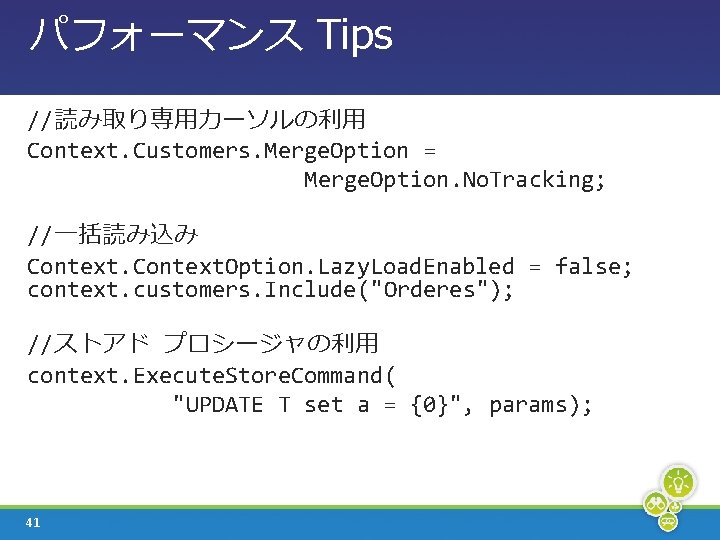
パフォーマンス Tips //読み取り専用カーソルの利用 Context. Customers. Merge. Option = Merge. Option. No. Tracking; //一括読み込み Context. Option. Lazy. Load. Enabled = false; context. customers. Include("Orderes"); //ストアド プロシージャの利用 context. Execute. Store. Command( "UPDATE T set a = {0}", params); 41
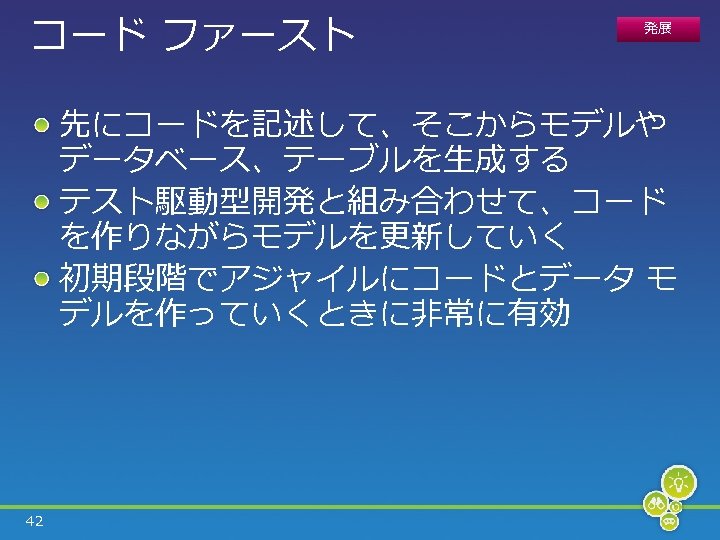
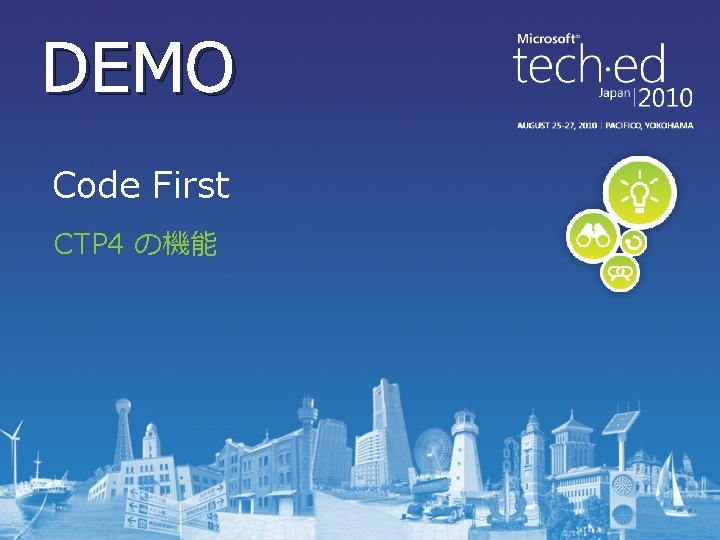
DEMO Code First CTP 4 の機能
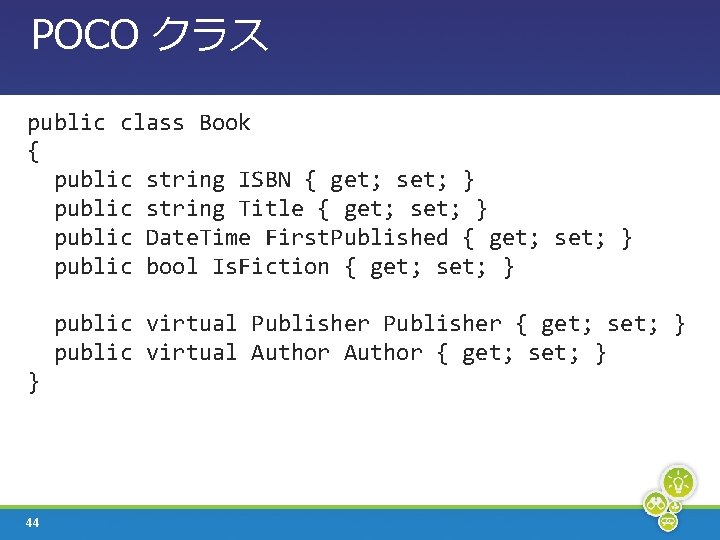
POCO クラス public class Book { public string ISBN { get; set; } public string Title { get; set; } public Date. Time First. Published { get; set; } public bool Is. Fiction { get; set; } public virtual Publisher { get; set; } public virtual Author { get; set; } } 44
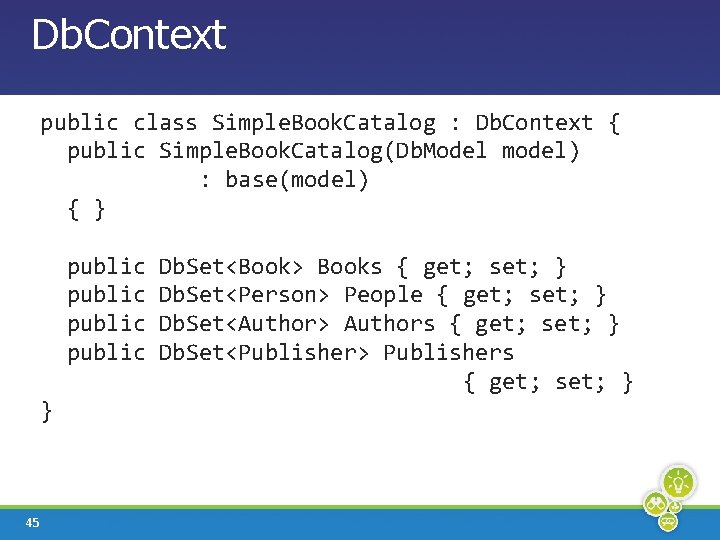
Db. Context public class Simple. Book. Catalog : Db. Context { public Simple. Book. Catalog(Db. Model model) : base(model) { } public } 45 Db. Set<Book> Books { get; set; } Db. Set<Person> People { get; set; } Db. Set<Author> Authors { get; set; } Db. Set<Publisher> Publishers { get; set; }
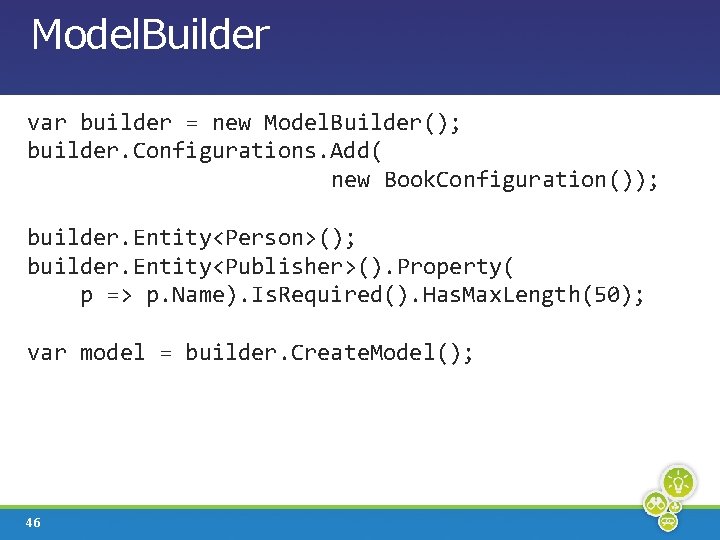
Model. Builder var builder = new Model. Builder(); builder. Configurations. Add( new Book. Configuration()); builder. Entity<Person>(); builder. Entity<Publisher>(). Property( p => p. Name). Is. Required(). Has. Max. Length(50); var model = builder. Create. Model(); 46
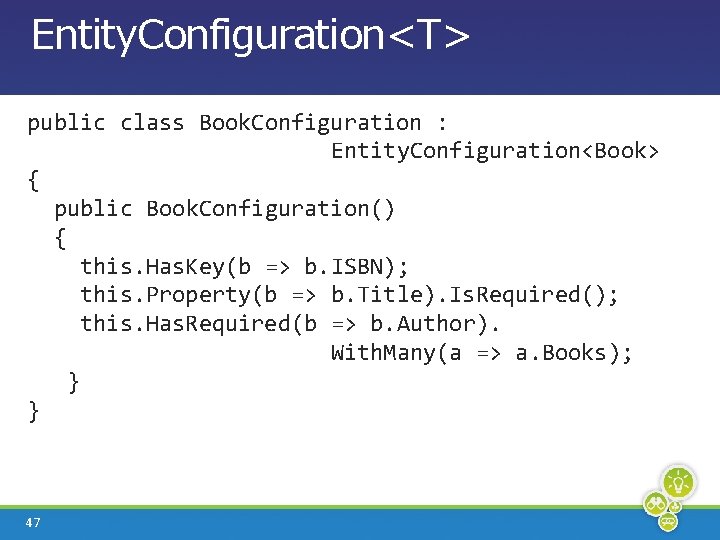
Entity. Configuration<T> public class Book. Configuration : Entity. Configuration<Book> { public Book. Configuration() { this. Has. Key(b => b. ISBN); this. Property(b => b. Title). Is. Required(); this. Has. Required(b => b. Author). With. Many(a => a. Books); } } 47
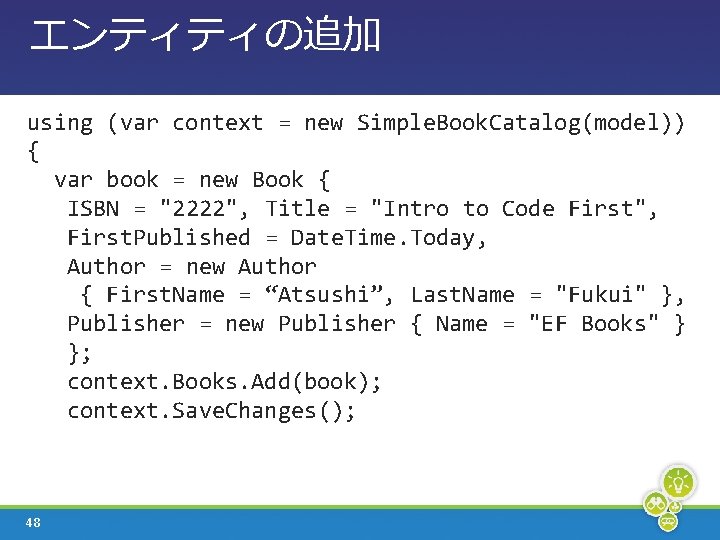
エンティティの追加 using (var context = new Simple. Book. Catalog(model)) { var book = new Book { ISBN = "2222", Title = "Intro to Code First", First. Published = Date. Time. Today, Author = new Author { First. Name = “Atsushi”, Last. Name = "Fukui" }, Publisher = new Publisher { Name = "EF Books" } }; context. Books. Add(book); context. Save. Changes(); 48
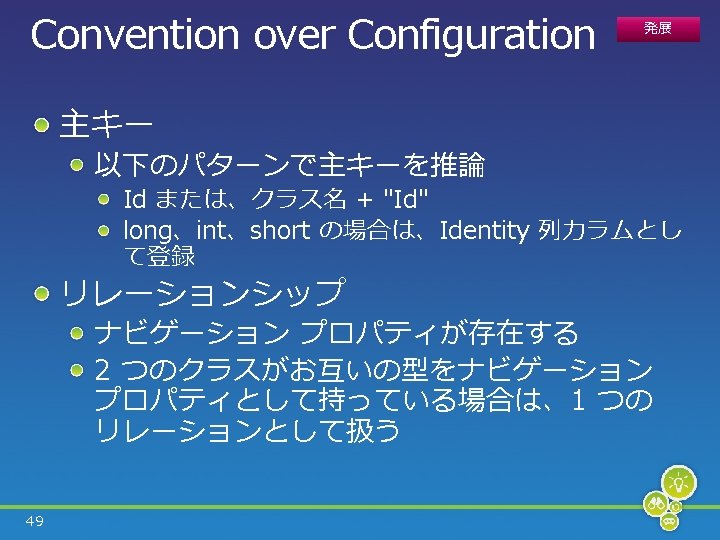
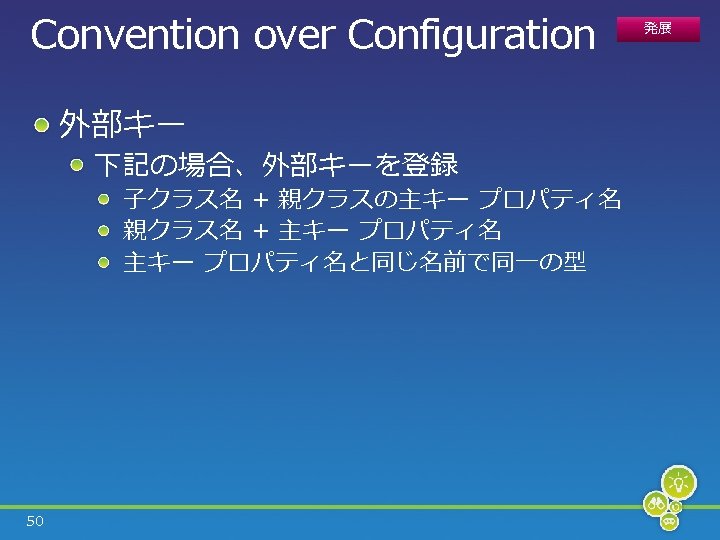
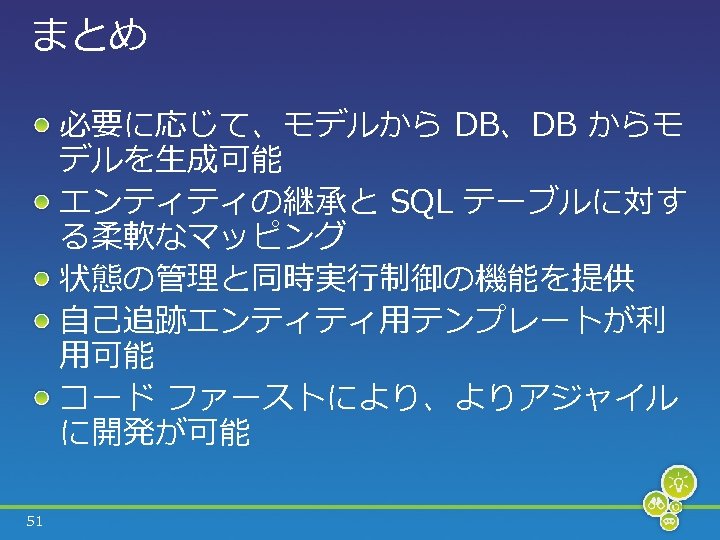
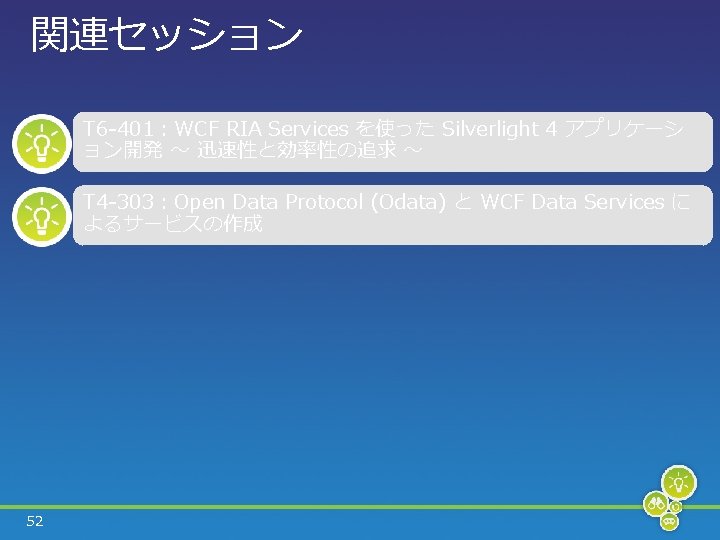
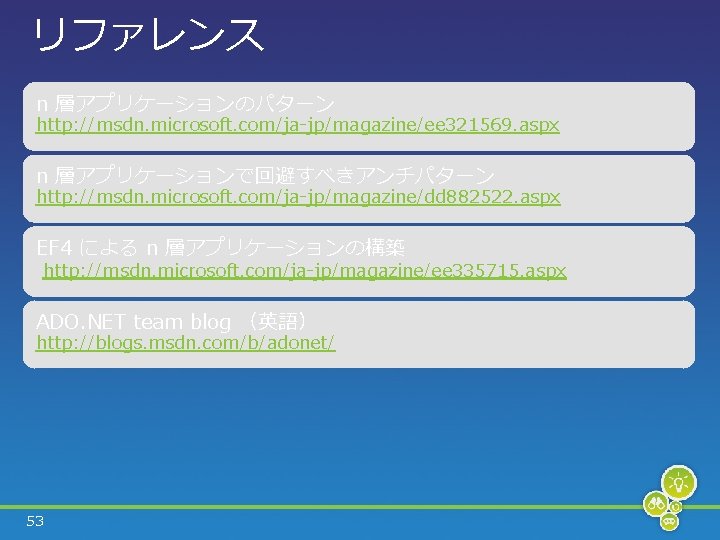
リファレンス n 層アプリケーションのパターン http: //msdn. microsoft. com/ja-jp/magazine/ee 321569. aspx n 層アプリケーションで回避すべきアンチパターン http: //msdn. microsoft. com/ja-jp/magazine/dd 882522. aspx EF 4 による n 層アプリケーションの構築 http: //msdn. microsoft. com/ja-jp/magazine/ee 335715. aspx ADO. NET team blog (英語) http: //blogs. msdn. com/b/adonet/ 53
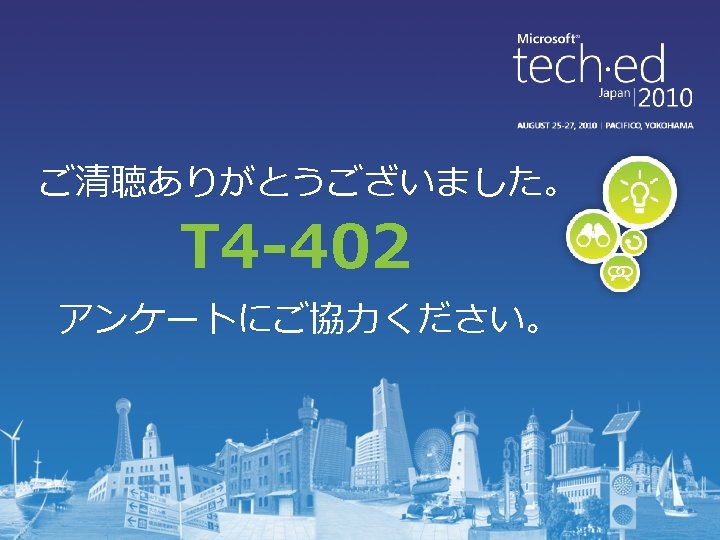
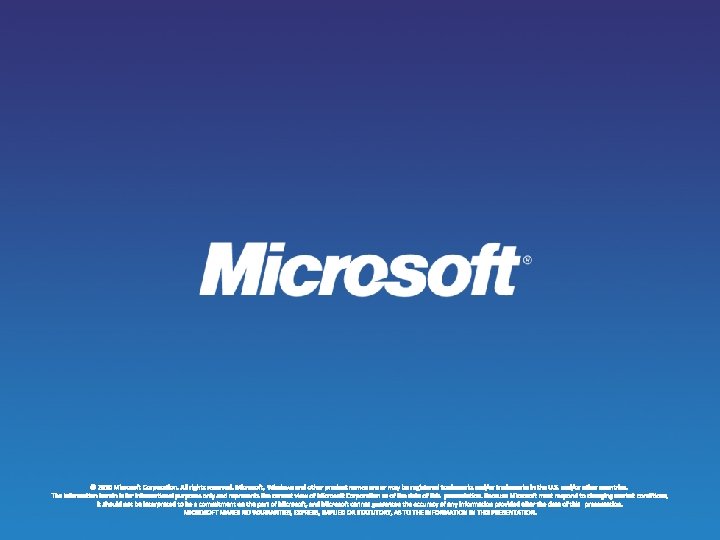
Ado.net vb.net
What is the overlap of data set 1 and data set 2?
Contoh participation constraint
Contoh weak entity
Public interest entity vs listed entity
Public interest entity vs listed entity
Total set awareness set consideration set
Training set validation set test set
Ado.net sql connection
Ado.net object model
¿qué es ado.net?
What are the ado.net objectives
Ado.net objects
2160711
Ado in real life
Which ado.net object contains select command property?
Linq vs ado.net
Ado.net overview
Ado net active directory
Block diagram of ado.net
Connection-oriented
Sqlite ppt
Ado.net c
Weak entity database
Discriminator in weak entity set
Discriminator in weak entity set
The entity set is a *
Nentity
Weak entity set example
Setreasona
Entity framework code first
Microsoft net framework 4
Entity framework disadvantages
Entity framework core
Difference between code first and database first approach
Entity integrity ensures correctness of the data in a table
Data modeling using entity relationship model
Security services x.800
Data preprocessing
Company er diagram
Data entity types
Ternary relationship sql
Entity-relationship data model
Entity relationship data model
When is the concept of a weak entity used in data modeling
Bounded set vs centered set
Crisp set vs fuzzy set
Crisp set vs fuzzy set
Crisp set vs fuzzy set
The function from set a to set b is
Tsupino
Locucao verbal
Allusions in much ado about nothing
Mehr qolur sheri
Shakespeare much ado about nothing
Puns in much ado about nothing