Engineering Output Engineering Notation Similar to scientific notation
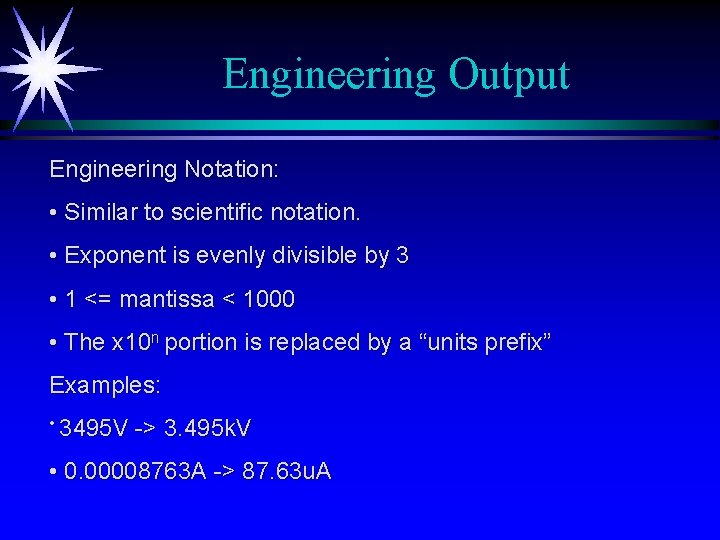
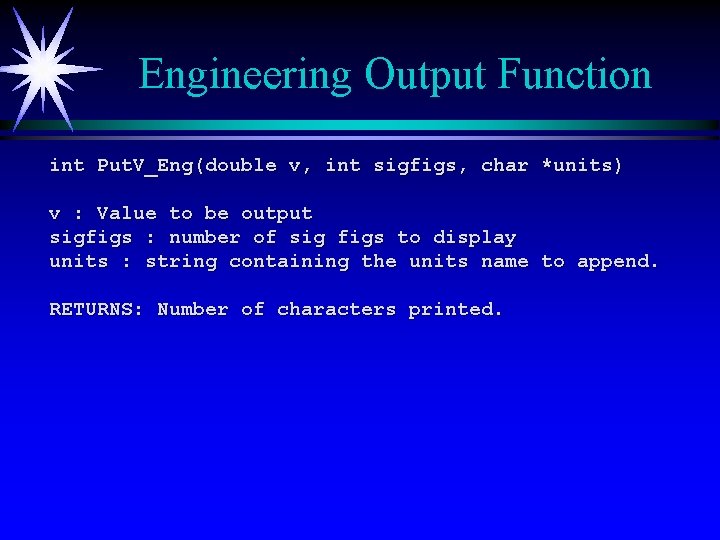
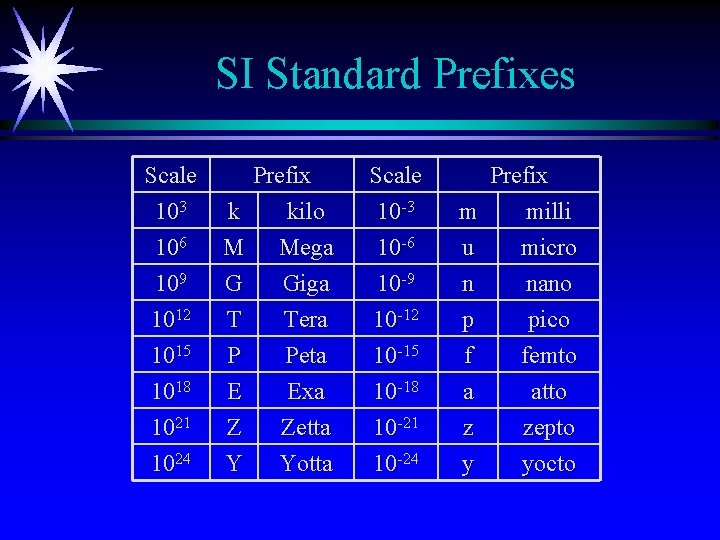
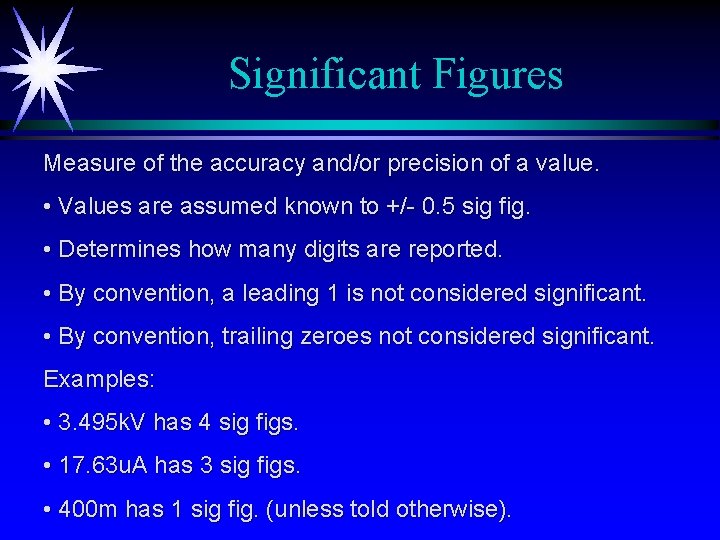
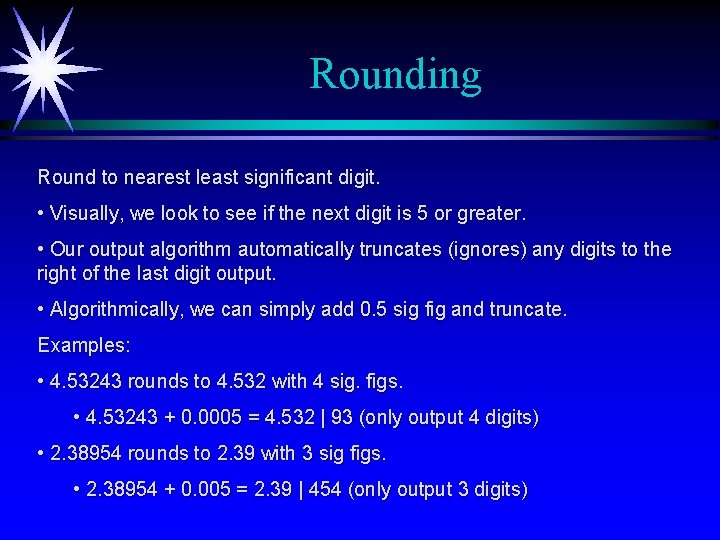
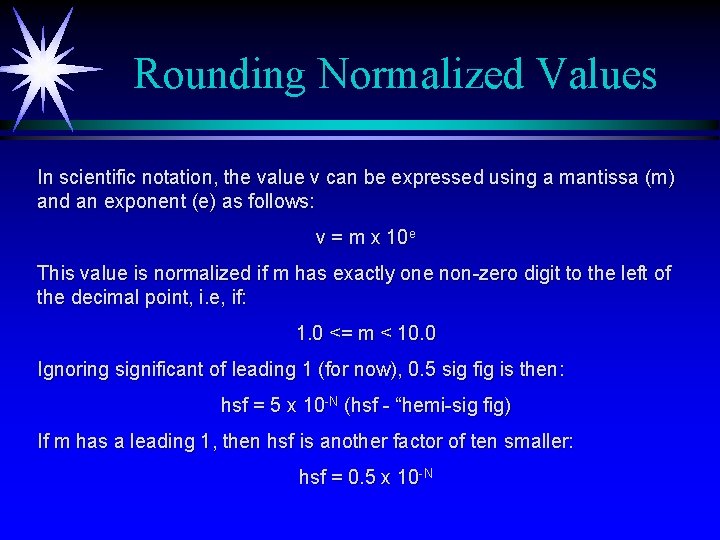
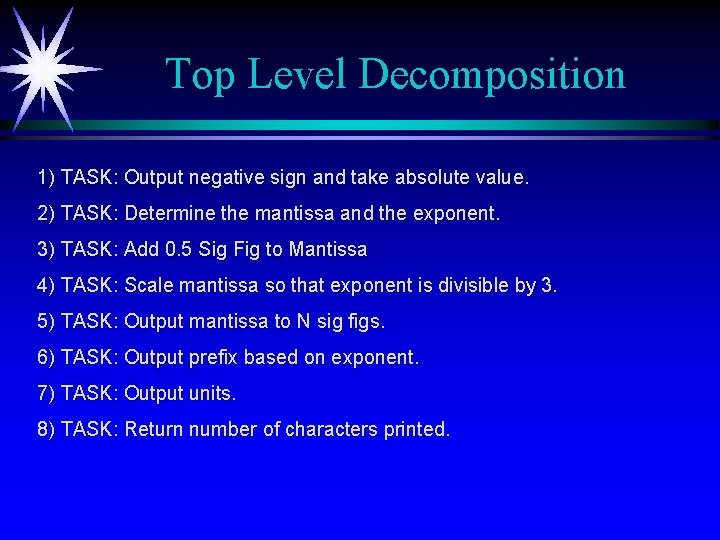
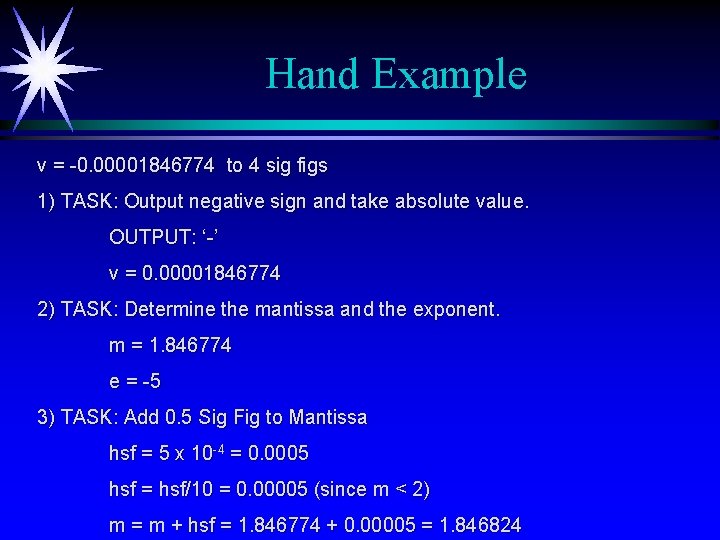
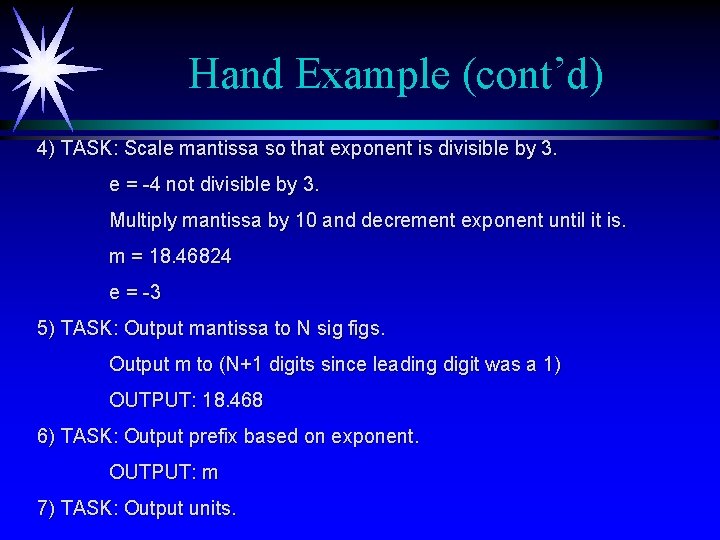
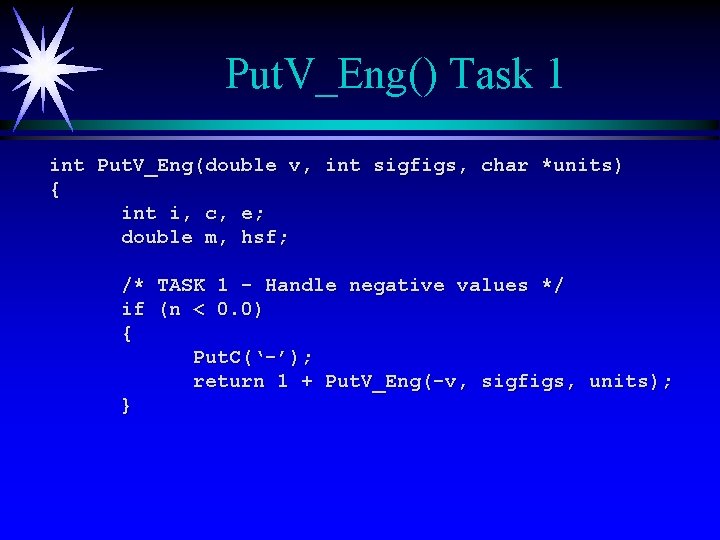
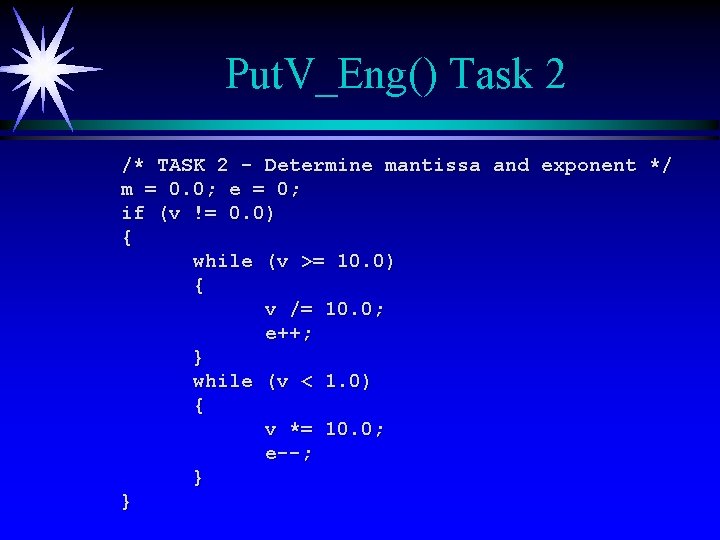
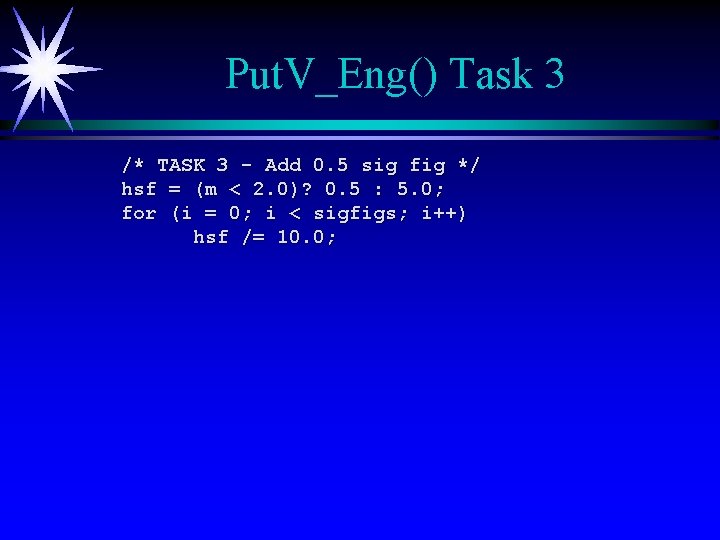
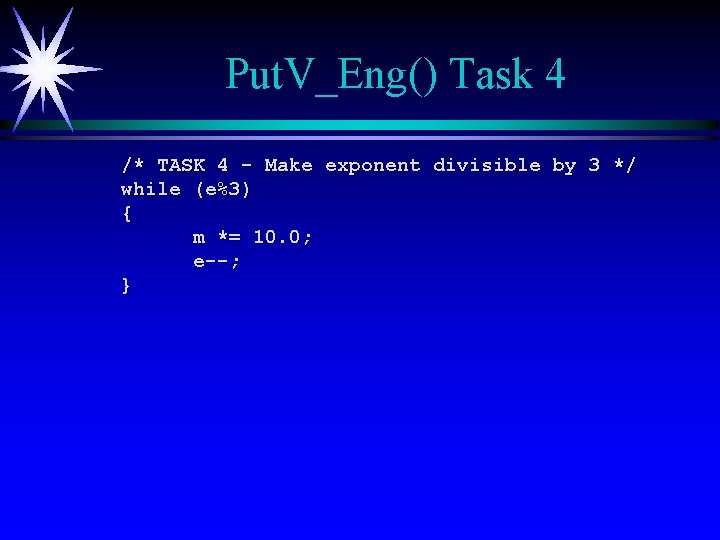
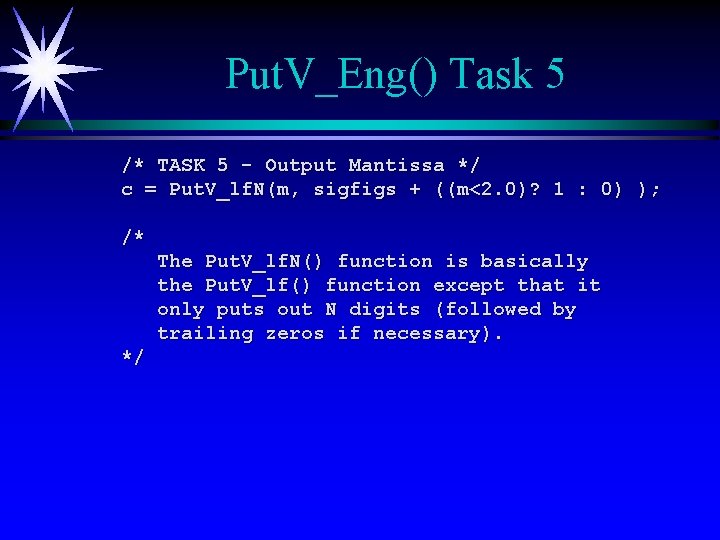
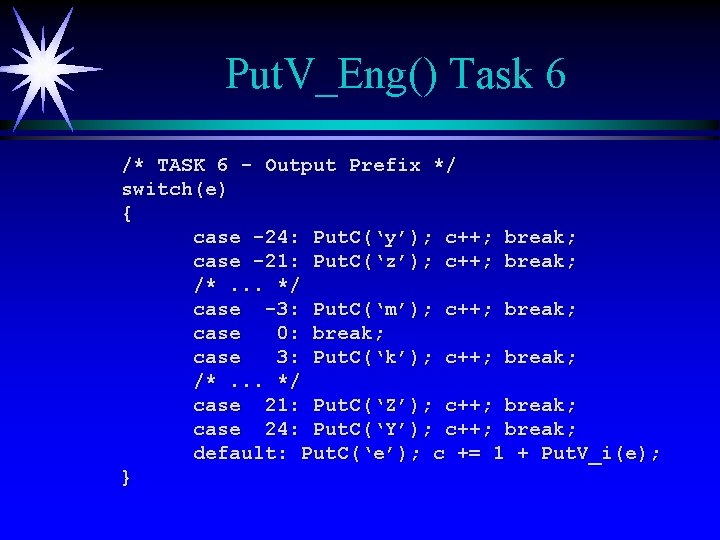
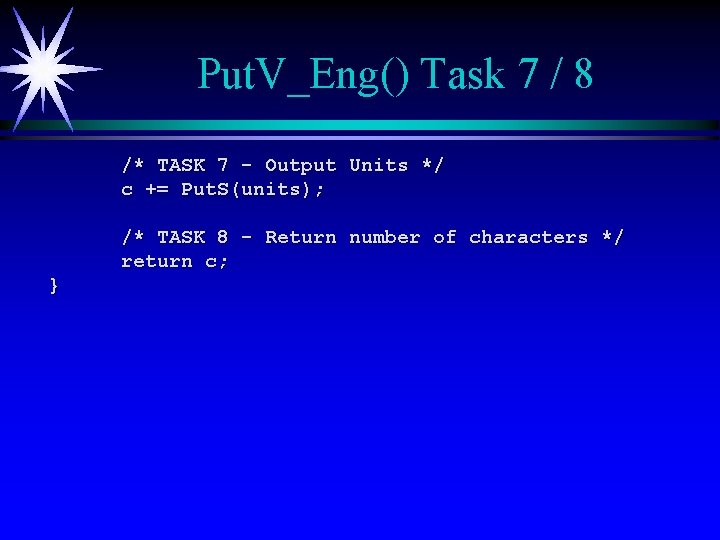
- Slides: 16
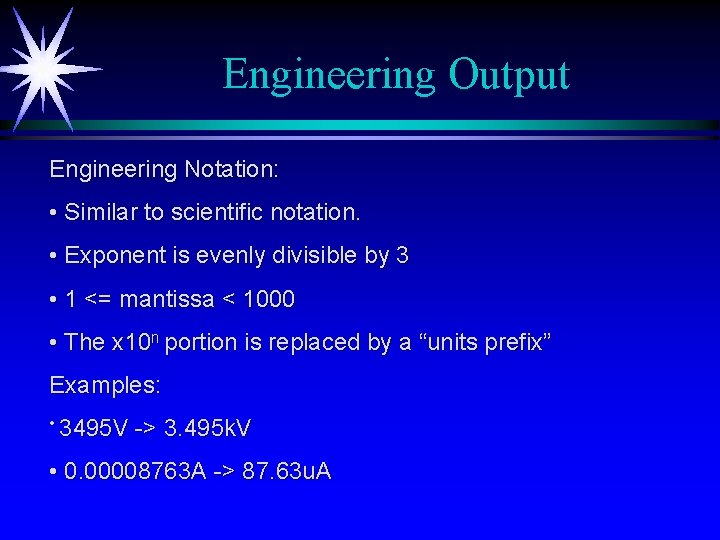
Engineering Output Engineering Notation: • Similar to scientific notation. • Exponent is evenly divisible by 3 • 1 <= mantissa < 1000 • The x 10 n portion is replaced by a “units prefix” Examples: • 3495 V -> 3. 495 k. V • 0. 00008763 A -> 87. 63 u. A
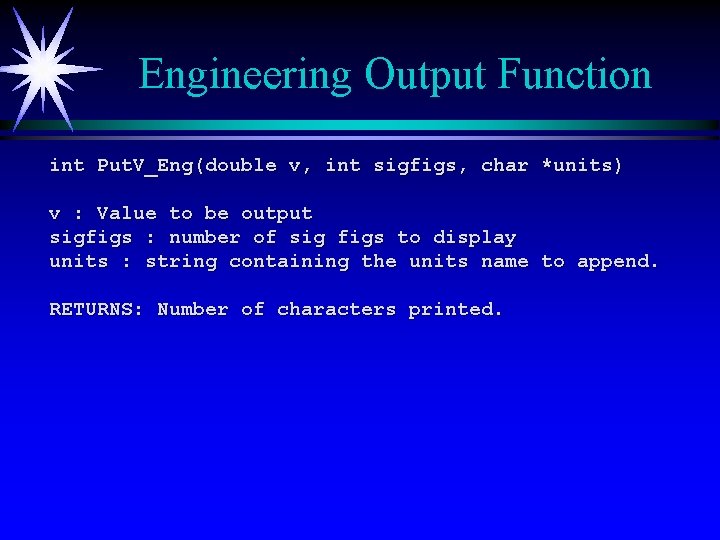
Engineering Output Function int Put. V_Eng(double v, int sigfigs, char *units) v : Value to be output sigfigs : number of sig figs to display units : string containing the units name to append. RETURNS: Number of characters printed.
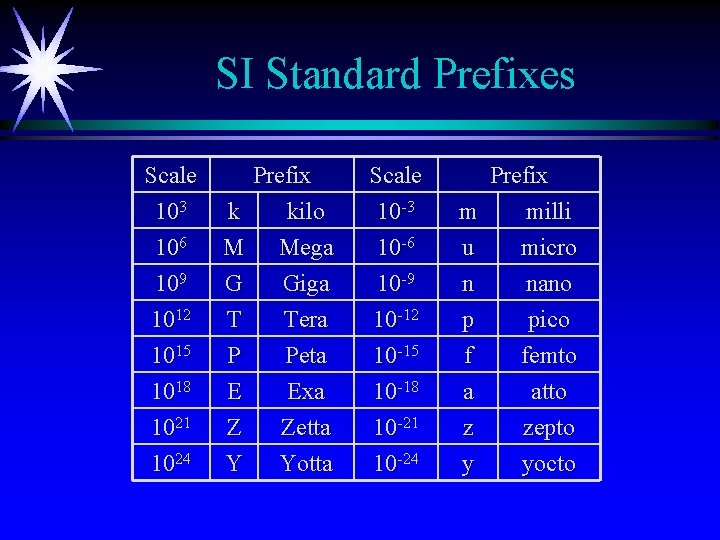
SI Standard Prefixes Scale 103 106 109 Prefix k kilo M Mega G Giga Scale 10 -3 10 -6 10 -9 1012 1015 1018 1021 1024 T P E Z Y 10 -12 10 -15 10 -18 10 -21 10 -24 Tera Peta Exa Zetta Yotta Prefix m milli u micro n nano p pico f femto a atto z zepto y yocto
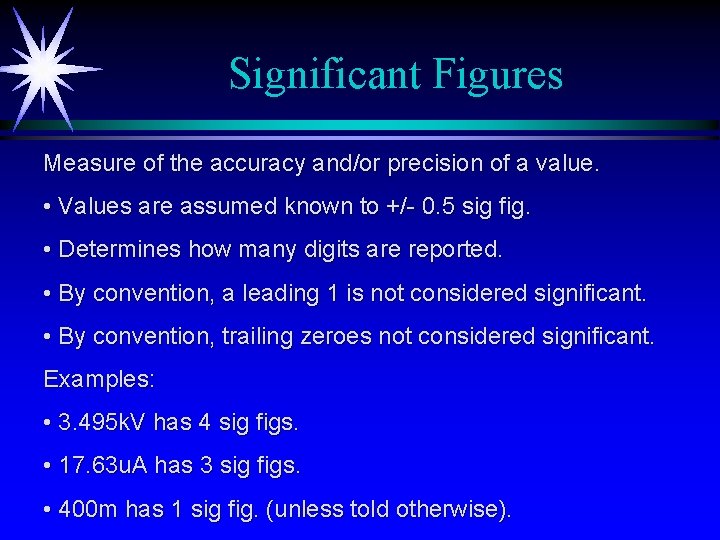
Significant Figures Measure of the accuracy and/or precision of a value. • Values are assumed known to +/- 0. 5 sig fig. • Determines how many digits are reported. • By convention, a leading 1 is not considered significant. • By convention, trailing zeroes not considered significant. Examples: • 3. 495 k. V has 4 sig figs. • 17. 63 u. A has 3 sig figs. • 400 m has 1 sig fig. (unless told otherwise).
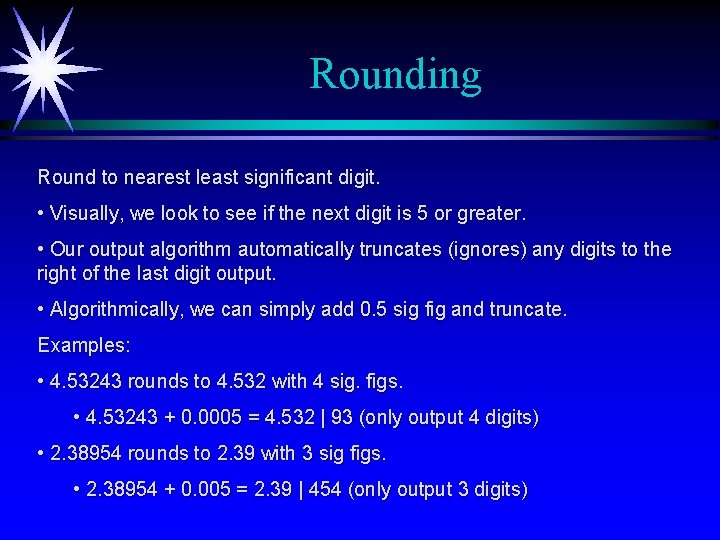
Rounding Round to nearest least significant digit. • Visually, we look to see if the next digit is 5 or greater. • Our output algorithm automatically truncates (ignores) any digits to the right of the last digit output. • Algorithmically, we can simply add 0. 5 sig fig and truncate. Examples: • 4. 53243 rounds to 4. 532 with 4 sig. figs. • 4. 53243 + 0. 0005 = 4. 532 | 93 (only output 4 digits) • 2. 38954 rounds to 2. 39 with 3 sig figs. • 2. 38954 + 0. 005 = 2. 39 | 454 (only output 3 digits)
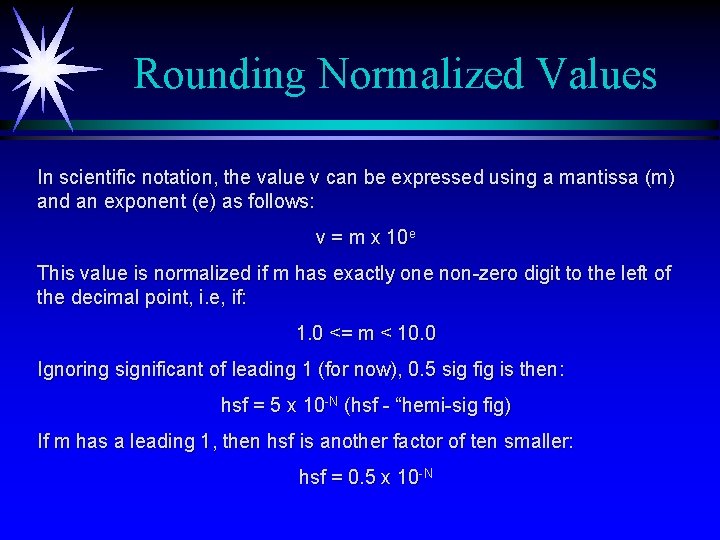
Rounding Normalized Values In scientific notation, the value v can be expressed using a mantissa (m) and an exponent (e) as follows: v = m x 10 e This value is normalized if m has exactly one non-zero digit to the left of the decimal point, i. e, if: 1. 0 <= m < 10. 0 Ignoring significant of leading 1 (for now), 0. 5 sig fig is then: hsf = 5 x 10 -N (hsf - “hemi-sig fig) If m has a leading 1, then hsf is another factor of ten smaller: hsf = 0. 5 x 10 -N
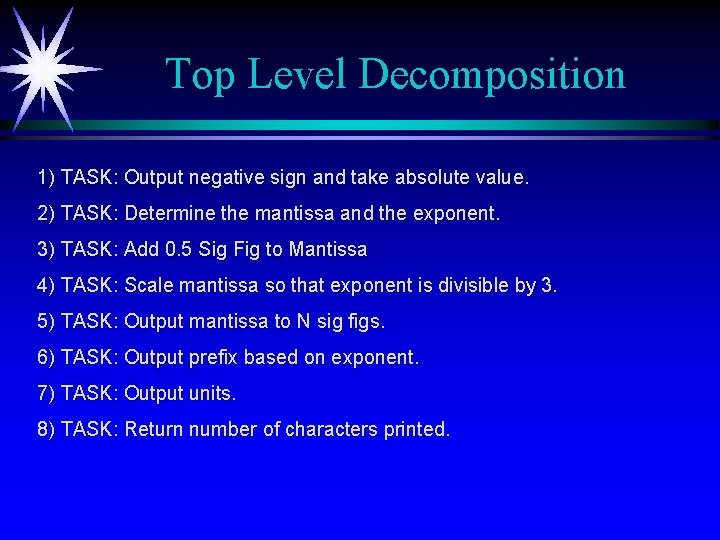
Top Level Decomposition 1) TASK: Output negative sign and take absolute value. 2) TASK: Determine the mantissa and the exponent. 3) TASK: Add 0. 5 Sig Fig to Mantissa 4) TASK: Scale mantissa so that exponent is divisible by 3. 5) TASK: Output mantissa to N sig figs. 6) TASK: Output prefix based on exponent. 7) TASK: Output units. 8) TASK: Return number of characters printed.
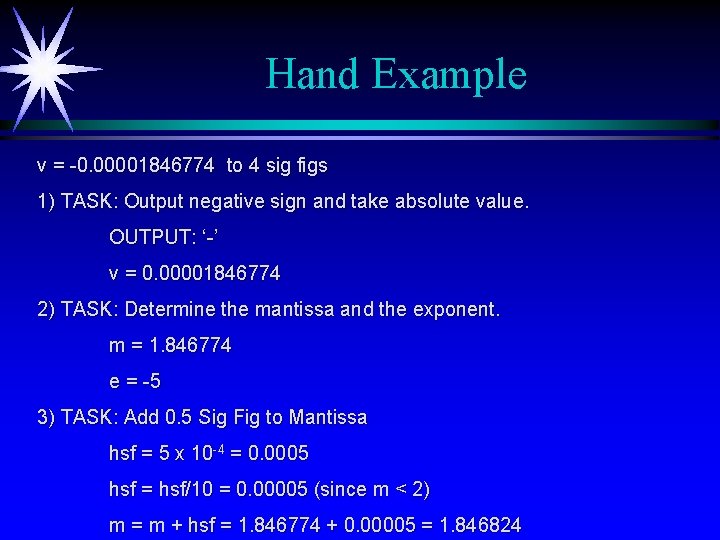
Hand Example v = -0. 00001846774 to 4 sig figs 1) TASK: Output negative sign and take absolute value. OUTPUT: ‘-’ v = 0. 00001846774 2) TASK: Determine the mantissa and the exponent. m = 1. 846774 e = -5 3) TASK: Add 0. 5 Sig Fig to Mantissa hsf = 5 x 10 -4 = 0. 0005 hsf = hsf/10 = 0. 00005 (since m < 2) m = m + hsf = 1. 846774 + 0. 00005 = 1. 846824
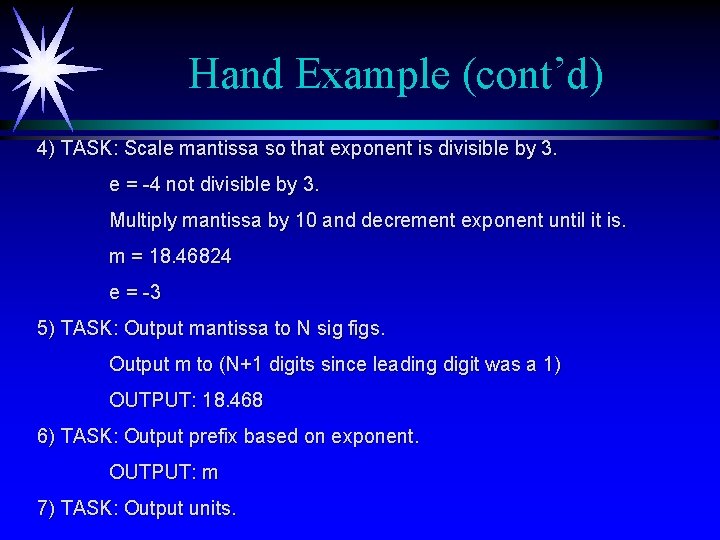
Hand Example (cont’d) 4) TASK: Scale mantissa so that exponent is divisible by 3. e = -4 not divisible by 3. Multiply mantissa by 10 and decrement exponent until it is. m = 18. 46824 e = -3 5) TASK: Output mantissa to N sig figs. Output m to (N+1 digits since leading digit was a 1) OUTPUT: 18. 468 6) TASK: Output prefix based on exponent. OUTPUT: m 7) TASK: Output units.
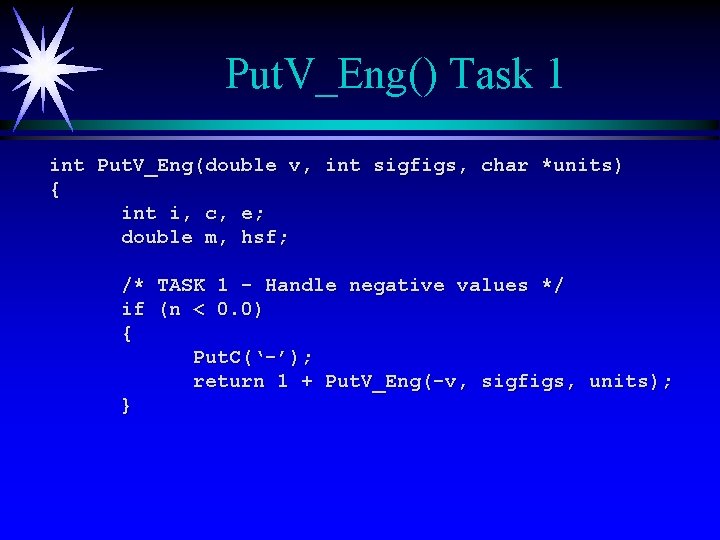
Put. V_Eng() Task 1 int Put. V_Eng(double v, int sigfigs, char *units) { int i, c, e; double m, hsf; /* if { TASK (n < 1 - Handle negative values */ 0. 0) Put. C(‘-’); return 1 + Put. V_Eng(-v, sigfigs, units); }
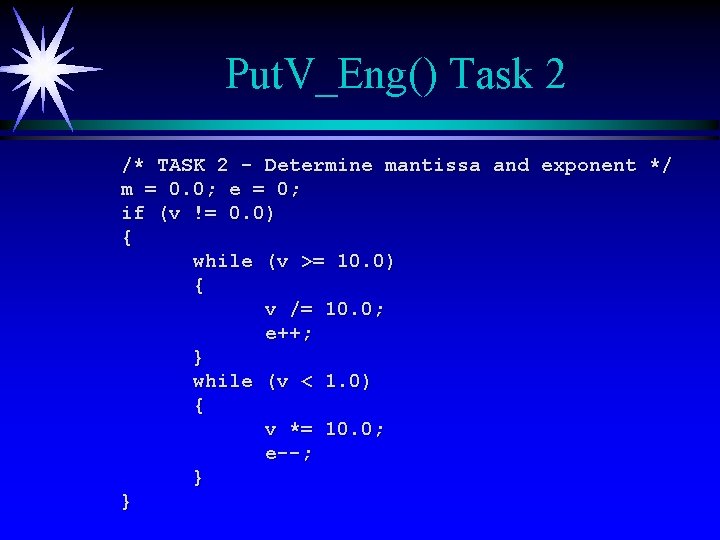
Put. V_Eng() Task 2 /* TASK 2 - Determine mantissa and exponent */ m = 0. 0; e = 0; if (v != 0. 0) { while (v >= 10. 0) { v /= 10. 0; e++; } while (v < 1. 0) { v *= 10. 0; e--; } }
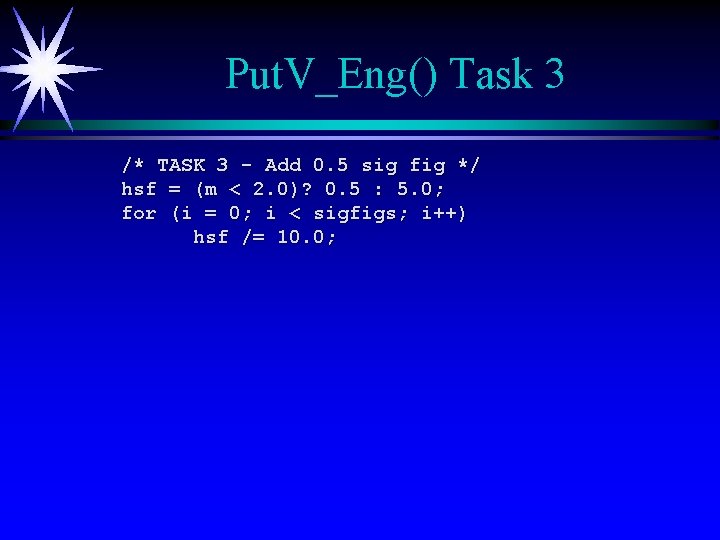
Put. V_Eng() Task 3 /* TASK 3 - Add 0. 5 sig fig */ hsf = (m < 2. 0)? 0. 5 : 5. 0; for (i = 0; i < sigfigs; i++) hsf /= 10. 0;
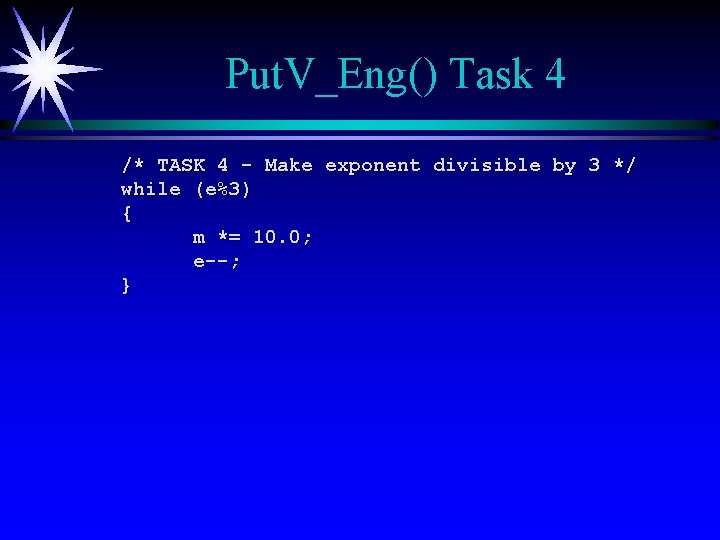
Put. V_Eng() Task 4 /* TASK 4 - Make exponent divisible by 3 */ while (e%3) { m *= 10. 0; e--; }
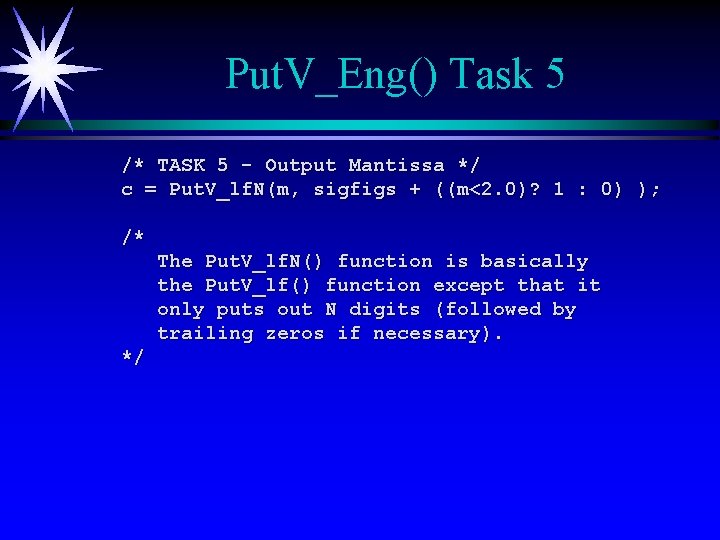
Put. V_Eng() Task 5 /* TASK 5 - Output Mantissa */ c = Put. V_lf. N(m, sigfigs + ((m<2. 0)? 1 : 0) ); /* The Put. V_lf. N() function is basically the Put. V_lf() function except that it only puts out N digits (followed by trailing zeros if necessary). */
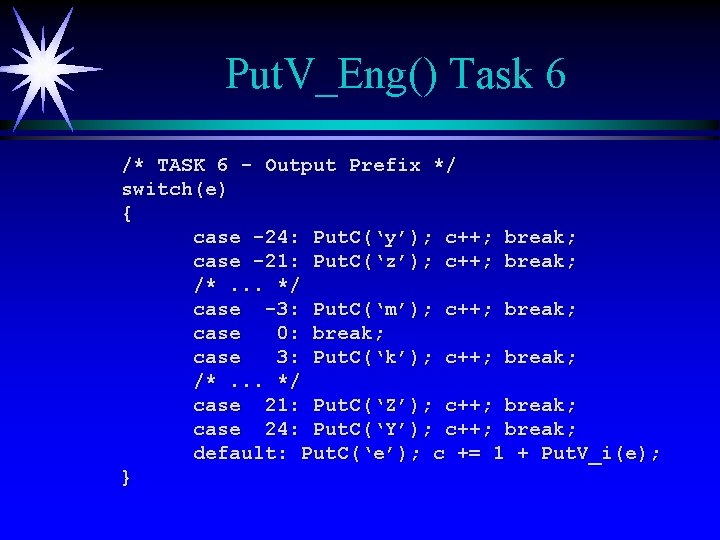
Put. V_Eng() Task 6 /* TASK 6 - Output Prefix */ switch(e) { case -24: Put. C(‘y’); c++; break; case -21: Put. C(‘z’); c++; break; /*. . . */ case -3: Put. C(‘m’); c++; break; case 0: break; case 3: Put. C(‘k’); c++; break; /*. . . */ case 21: Put. C(‘Z’); c++; break; case 24: Put. C(‘Y’); c++; break; default: Put. C(‘e’); c += 1 + Put. V_i(e); }
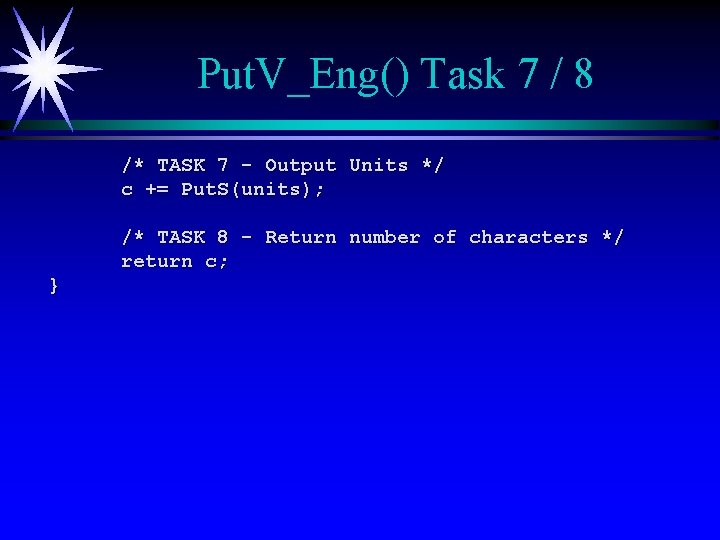
Put. V_Eng() Task 7 / 8 /* TASK 7 - Output Units */ c += Put. S(units); /* TASK 8 - Return number of characters */ return c; }
What are prefixes in science
Similar disuelve a similar
Propiedades fisicoquímicas del agua
Similar disuelve a similar
Members of an avian species of identical plumage congregate
Scientific inquiry vs scientific method
How is a scientific law different from a scientific theory?
Scientific notation
71 000 in scientific notation
Metric conversion scientific notation
97 700 000 000 000 000 000 000 in scientific notation
Atlantic pacific rule sig figs
Incorrect scientific notation examples
How to write in scientific notation
Express 4,980,000, 000 in scientific notation
Rules of scientific notation
149 600 000 in scientific notation