EKT 150 INTRODUCTION TO COMPUTER PROGRAMMING Function Part
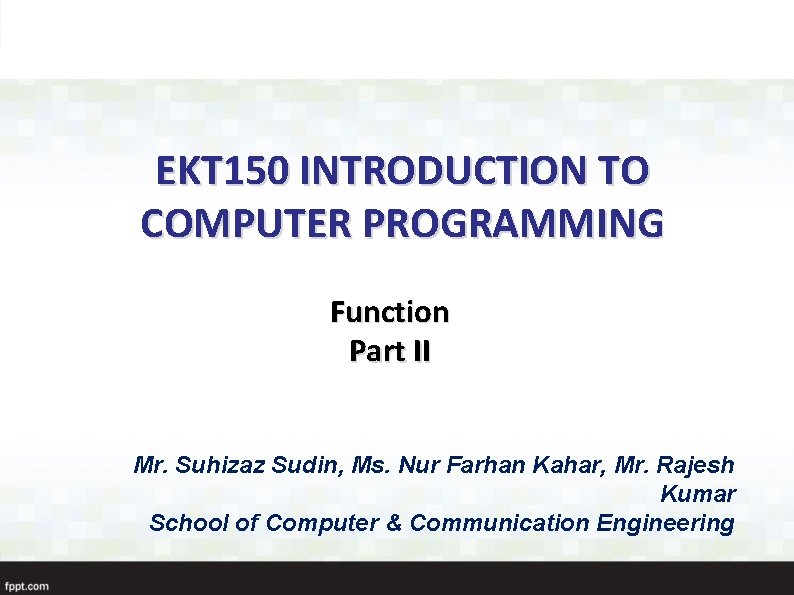
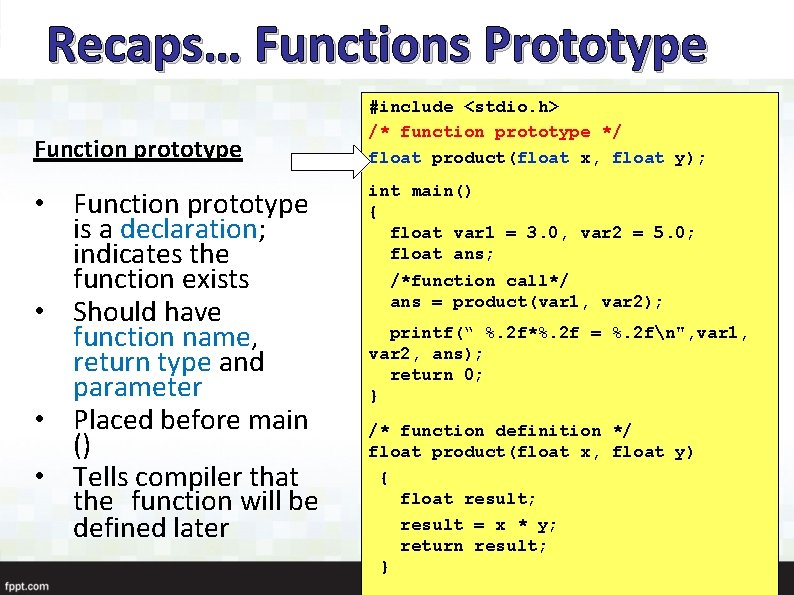
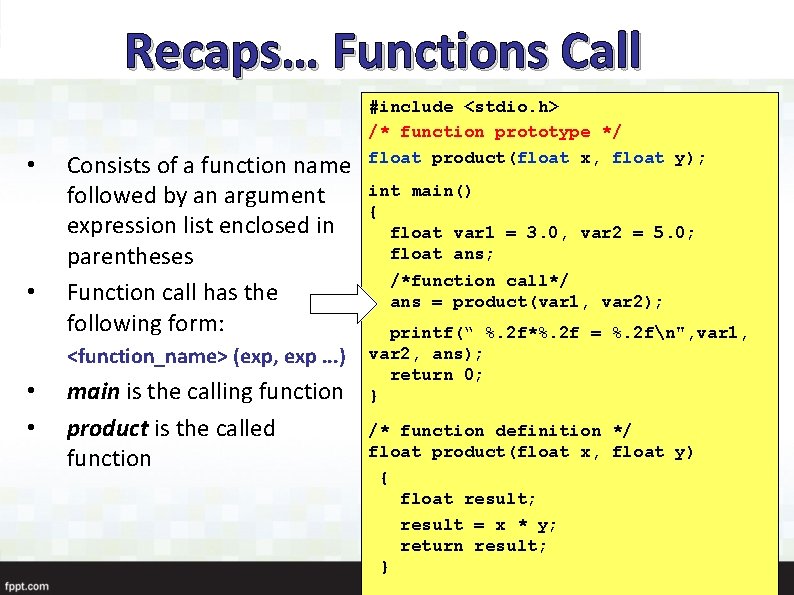
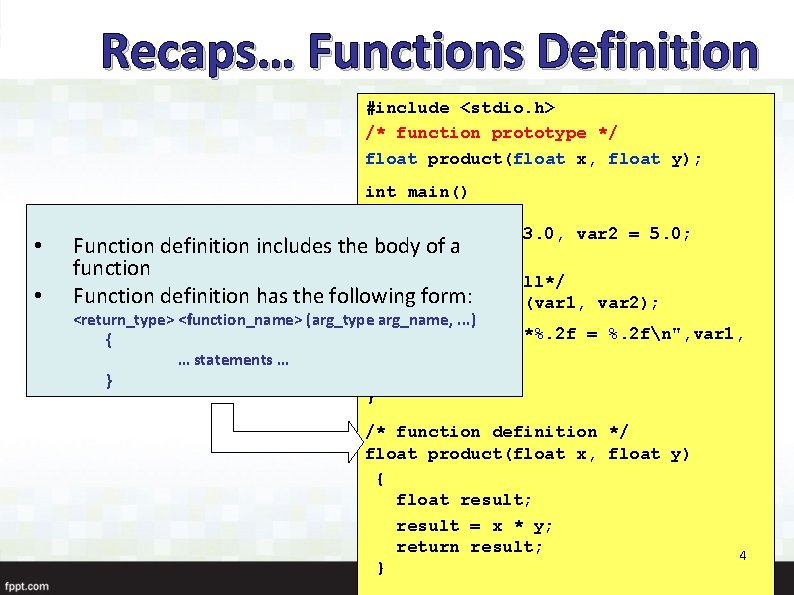
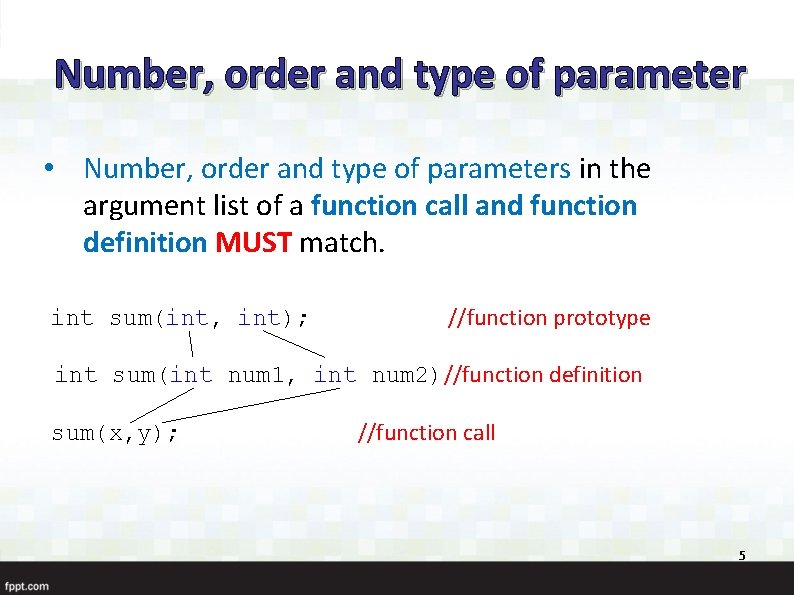
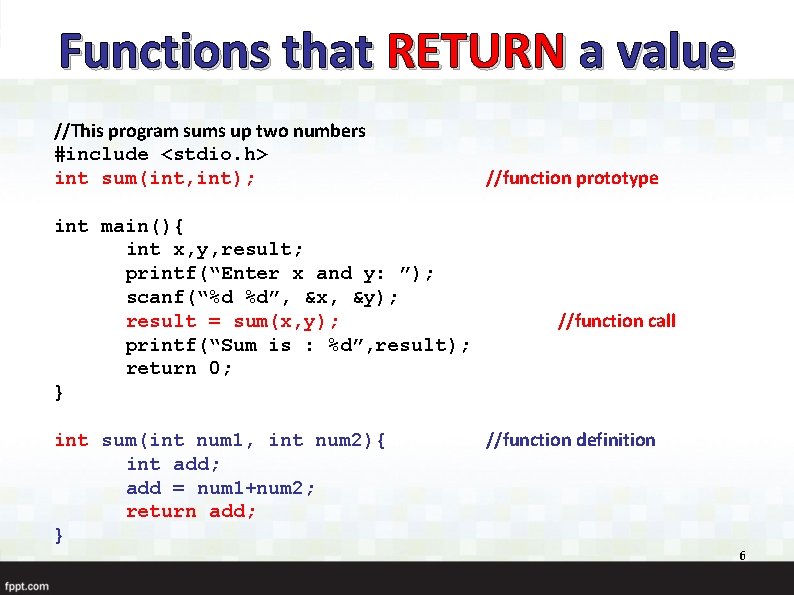
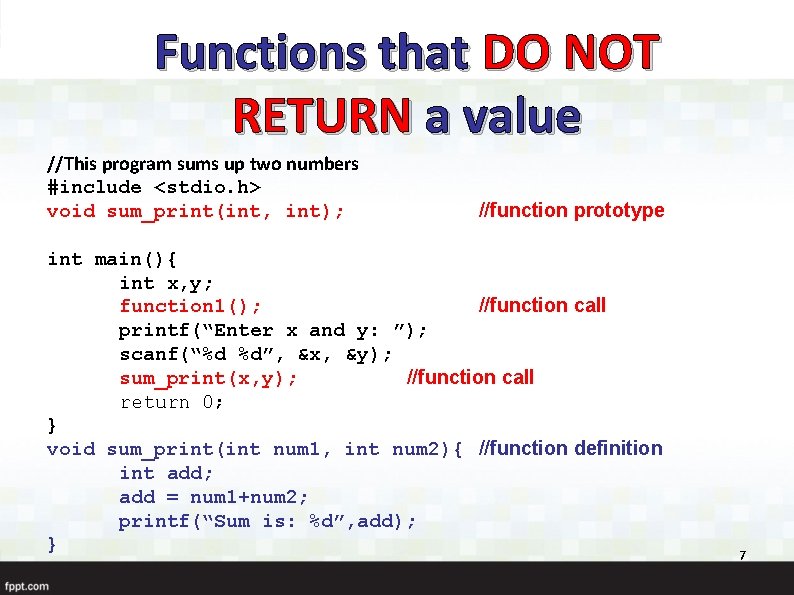
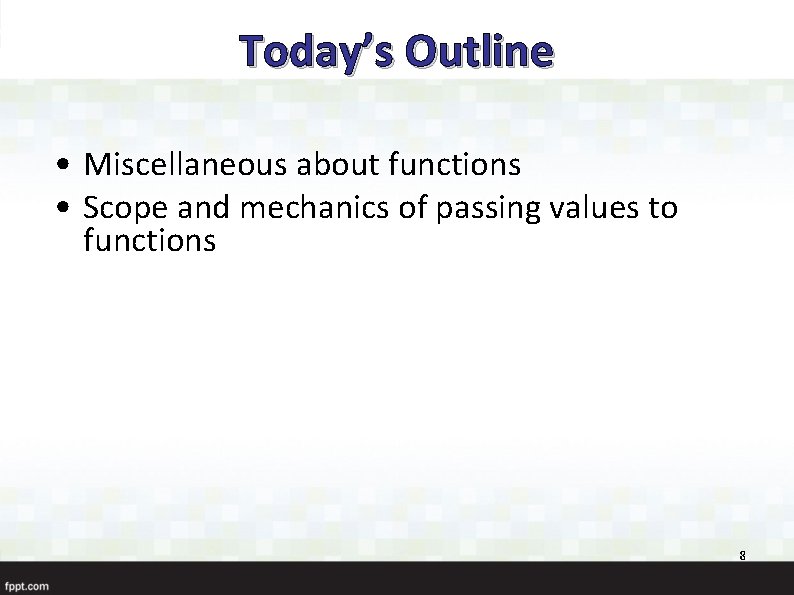
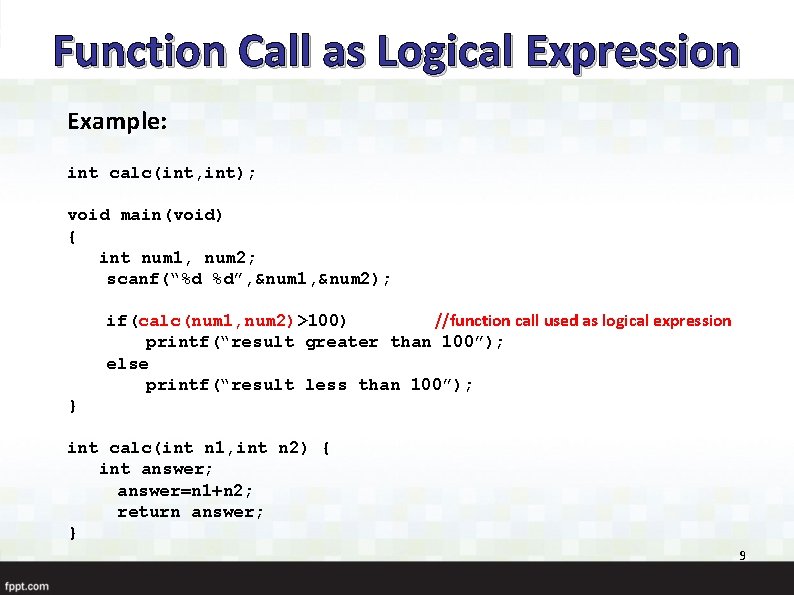
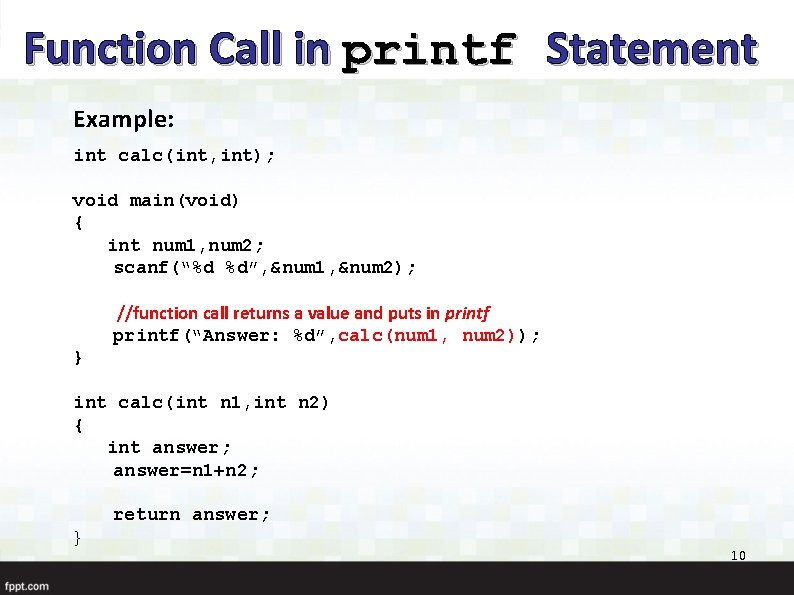
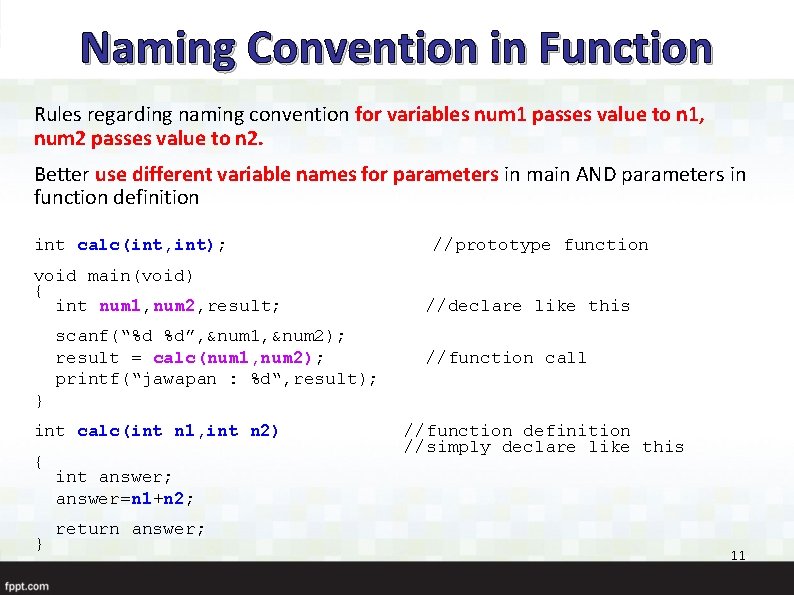
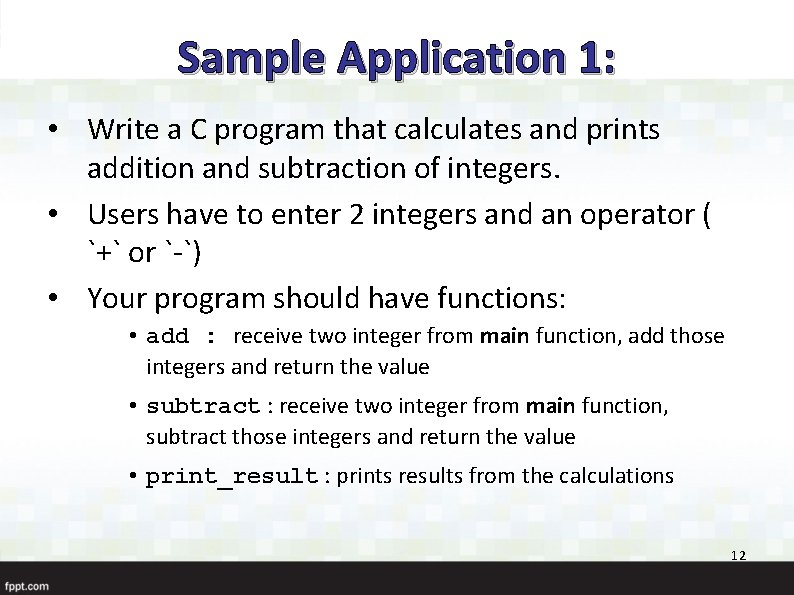
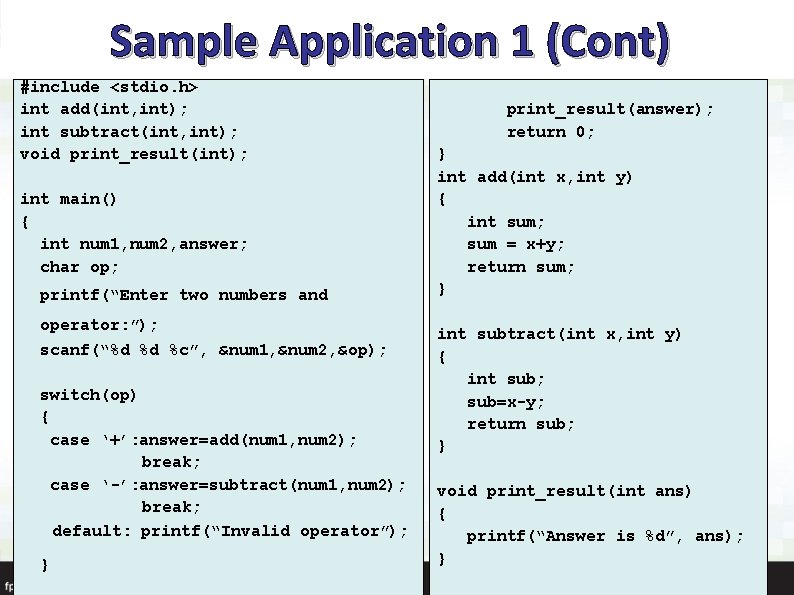
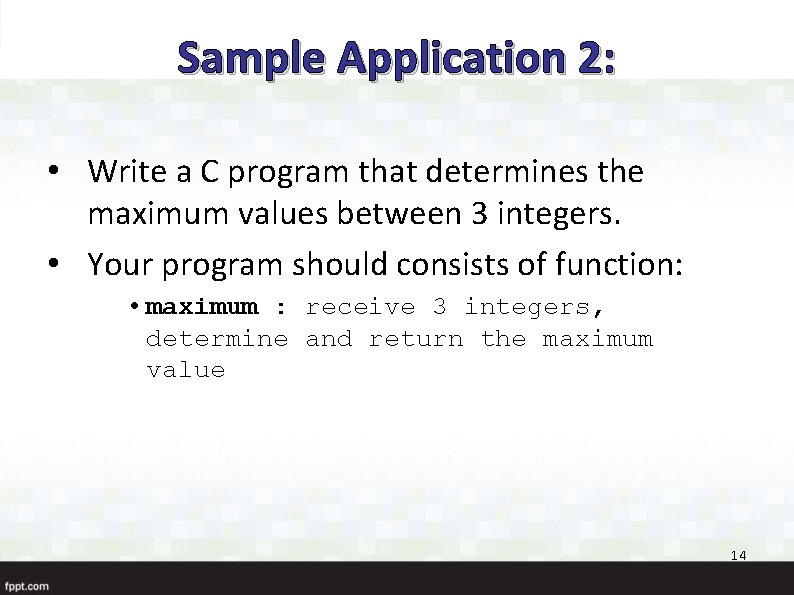
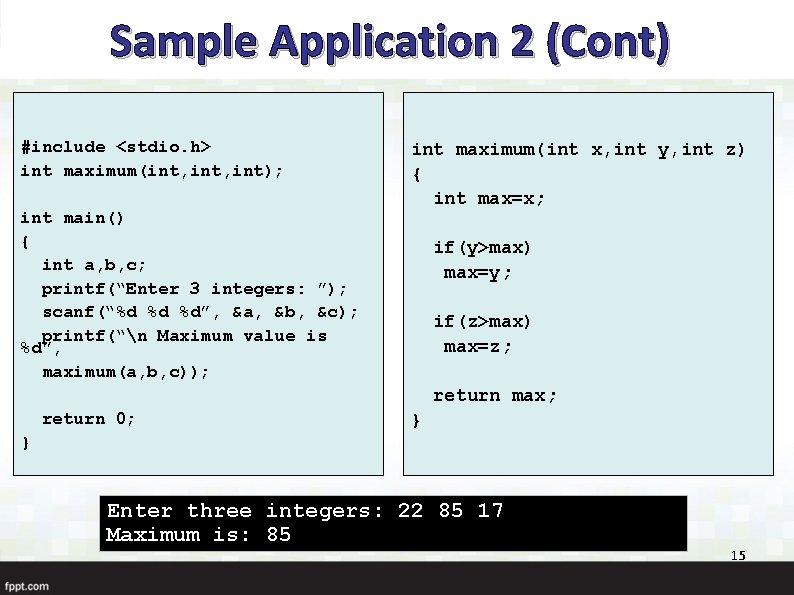
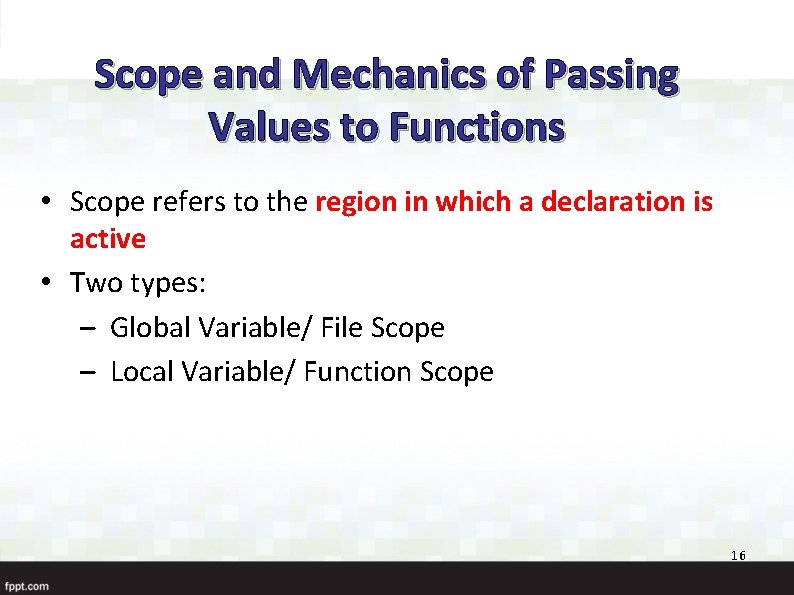
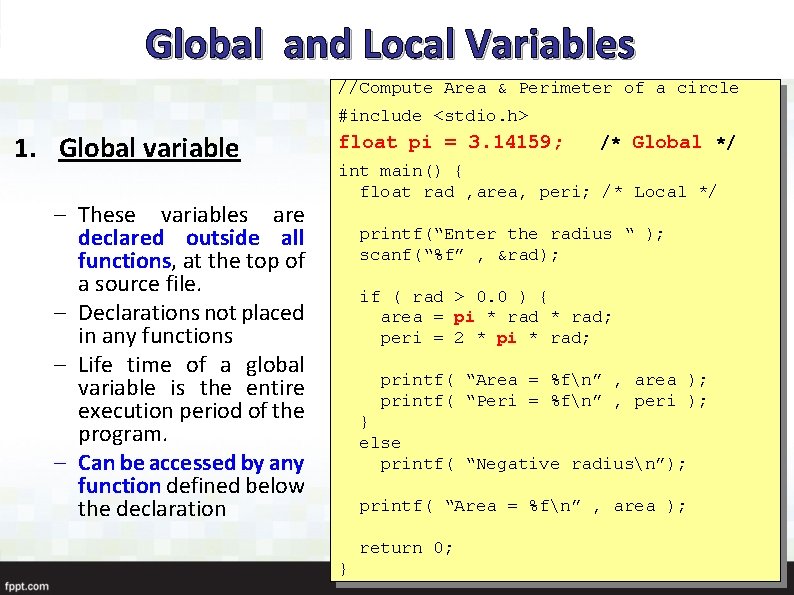
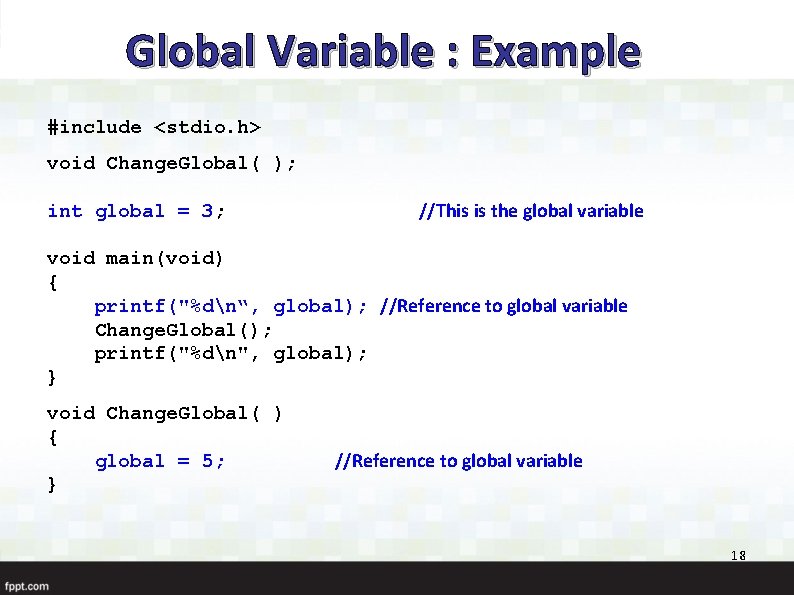
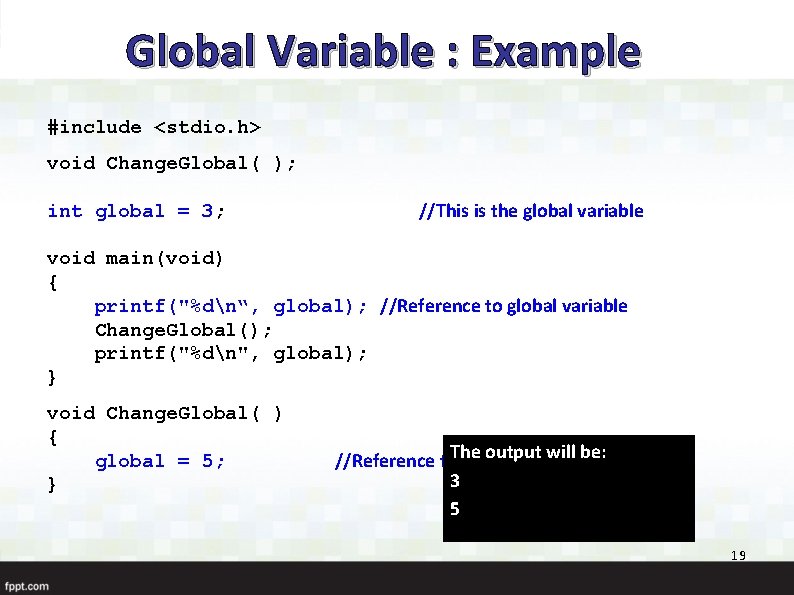
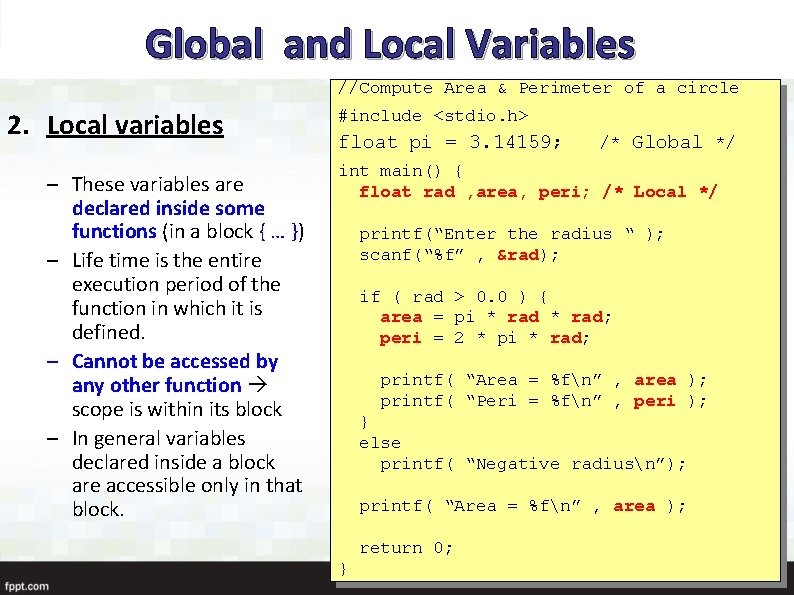
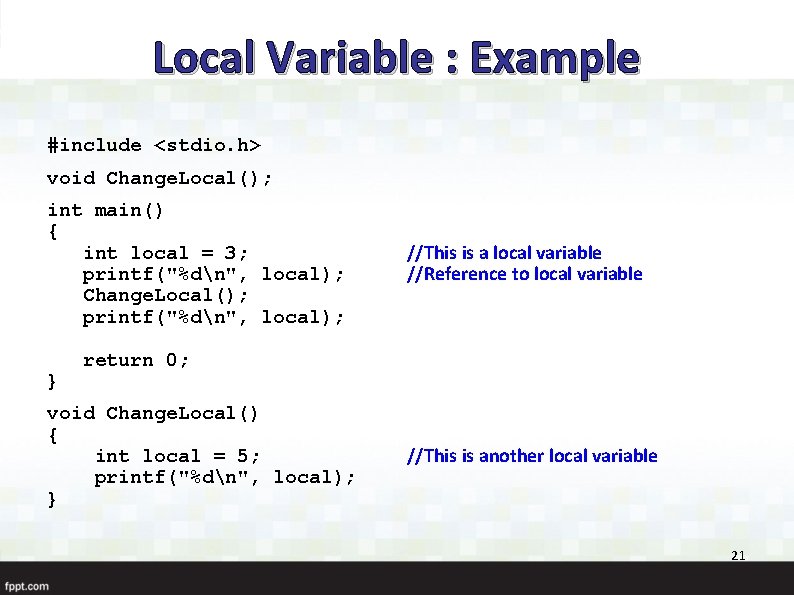
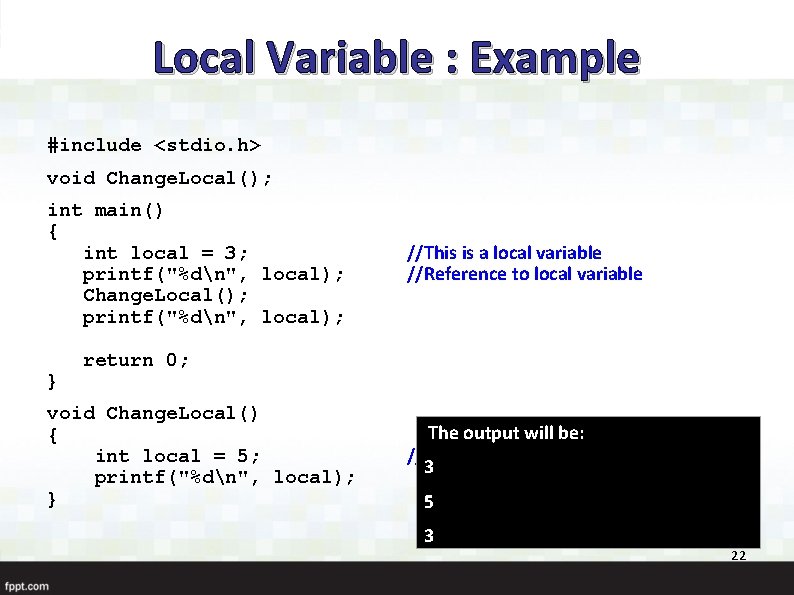
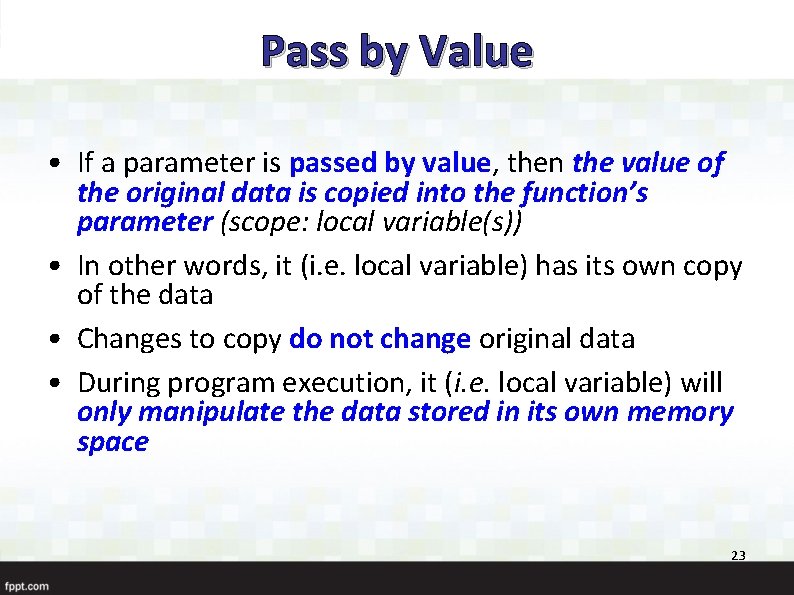
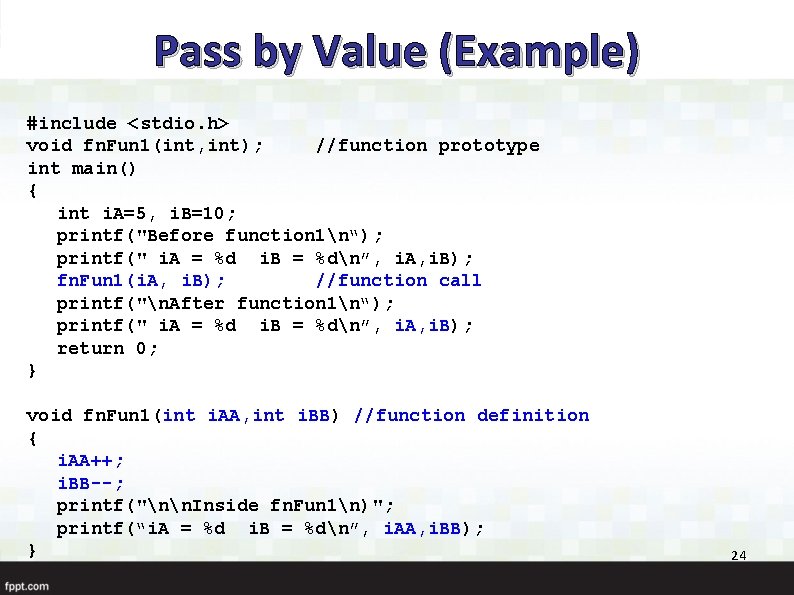
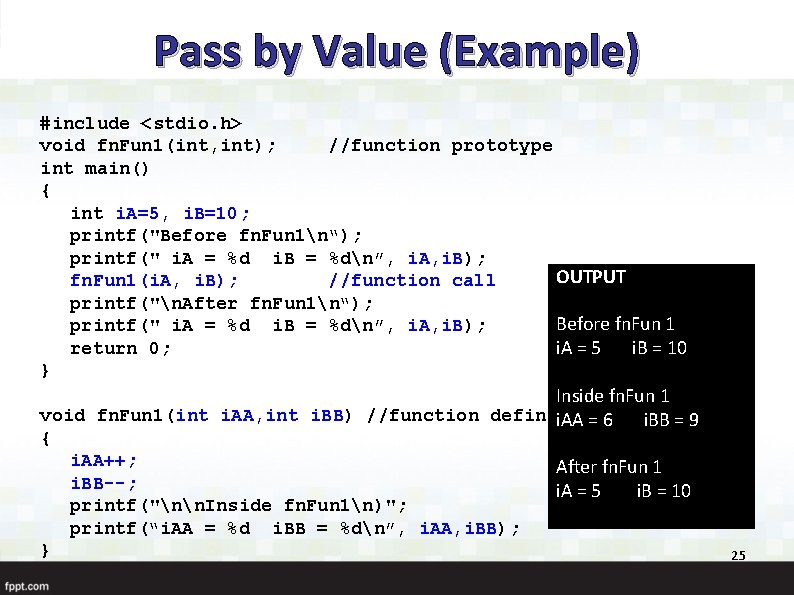
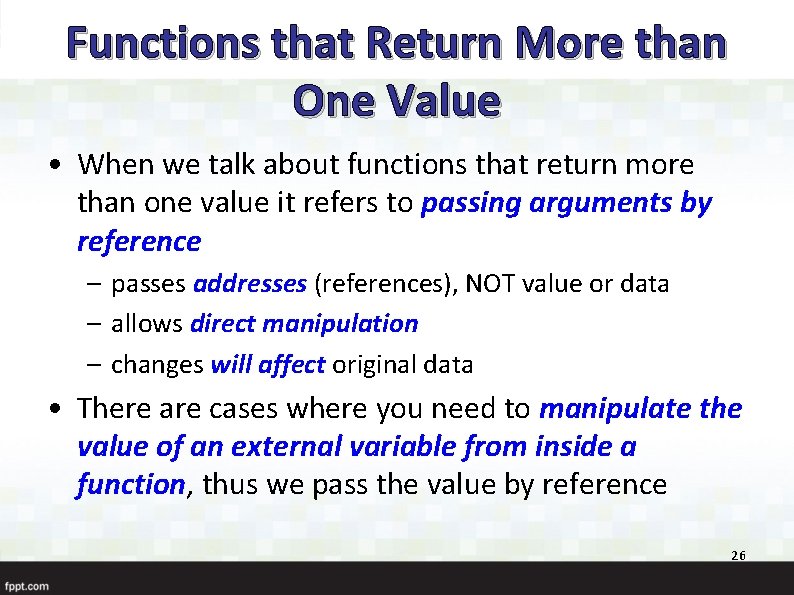
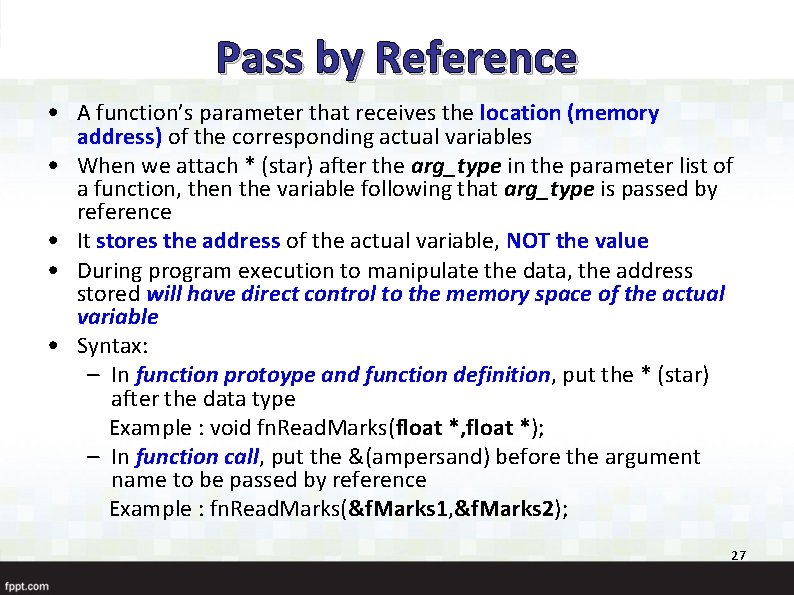
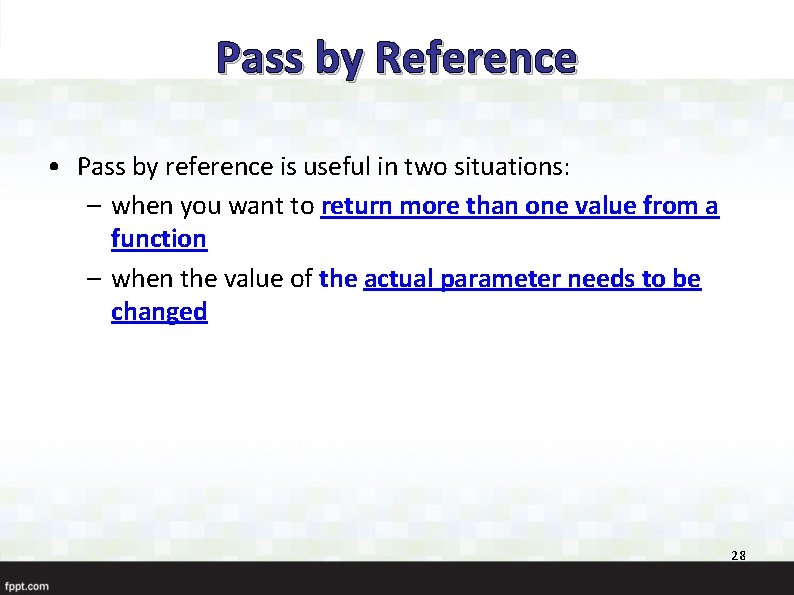
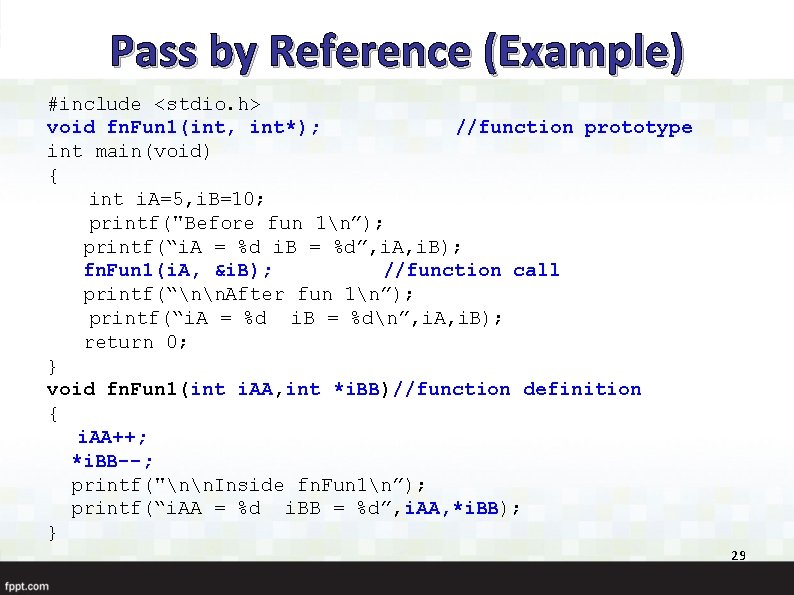
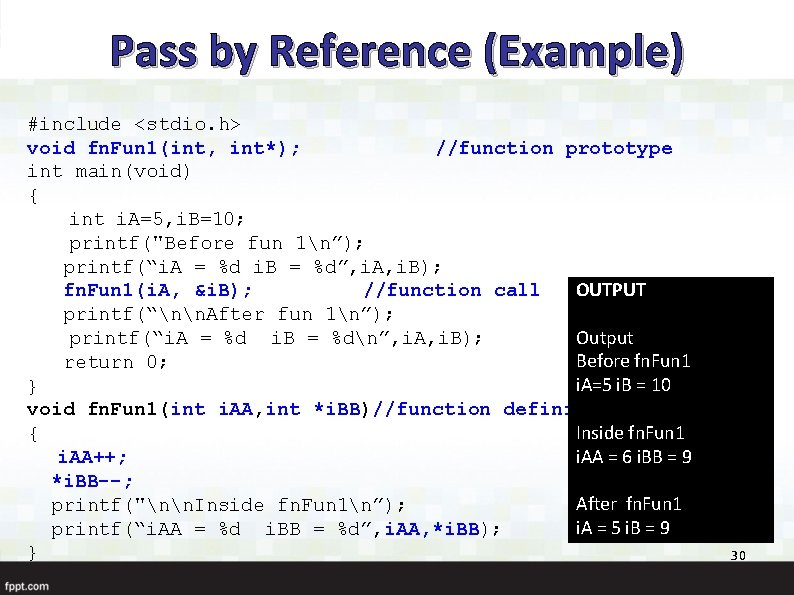
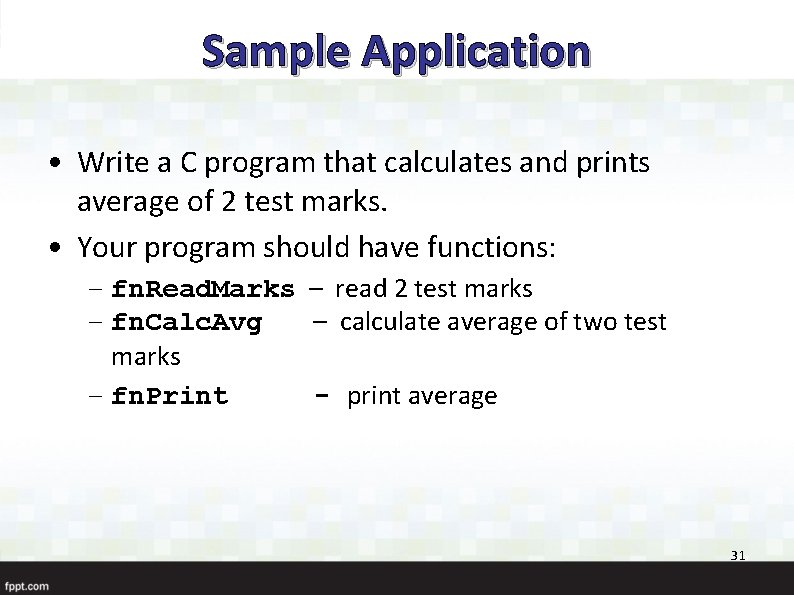
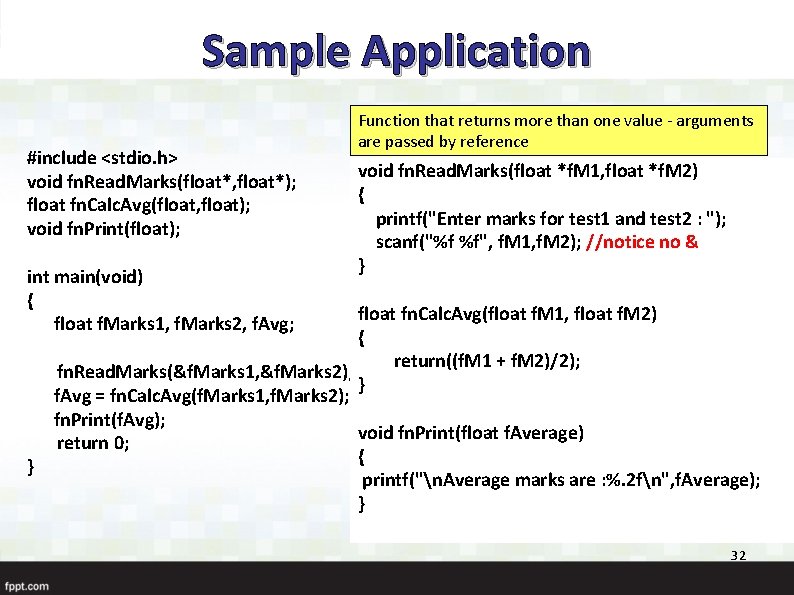
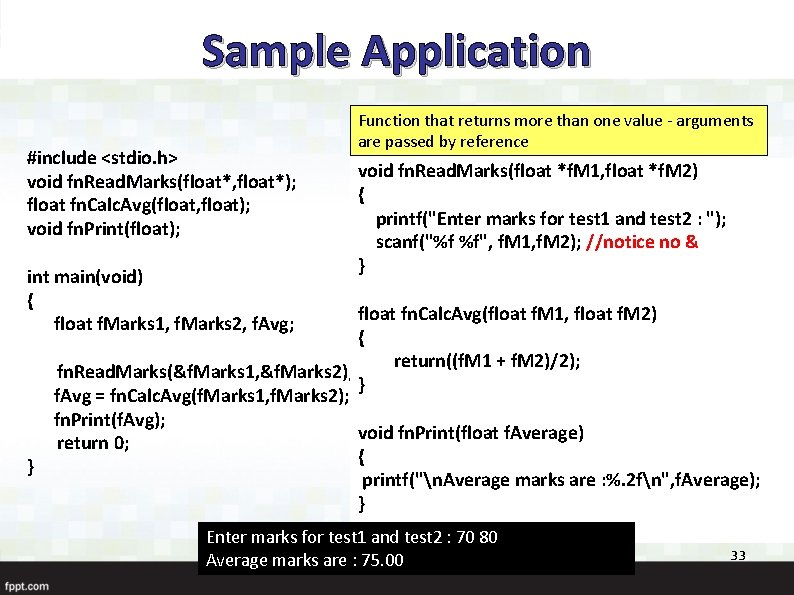
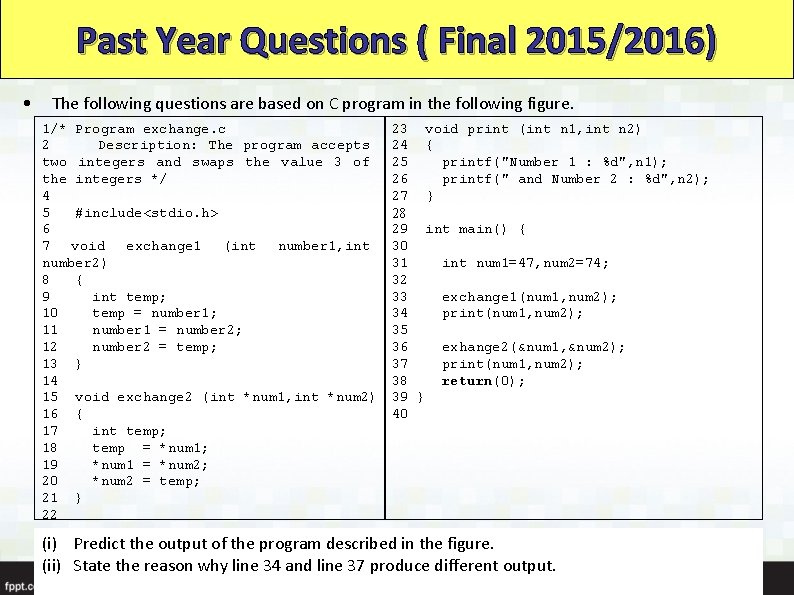
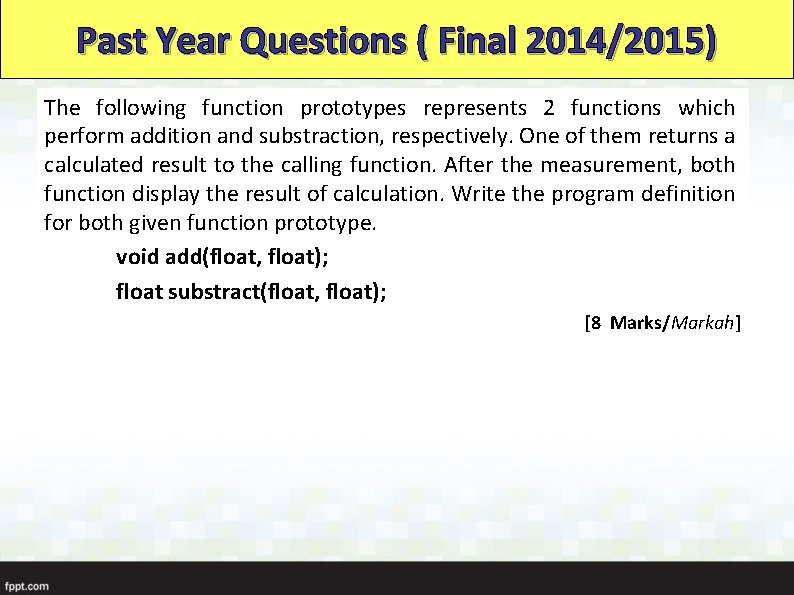
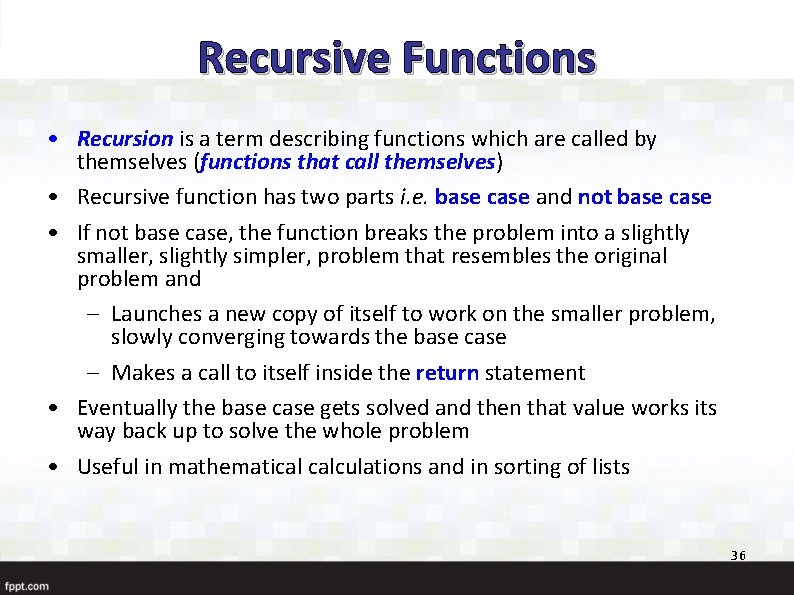
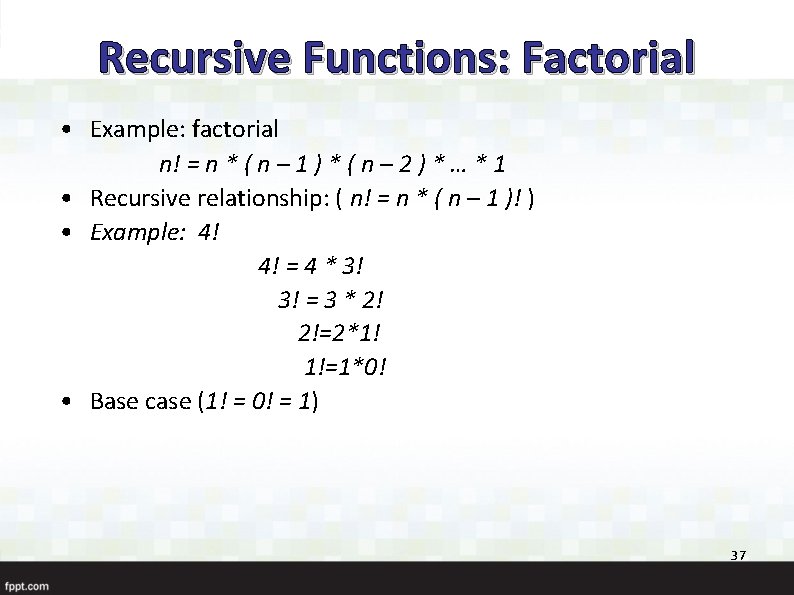
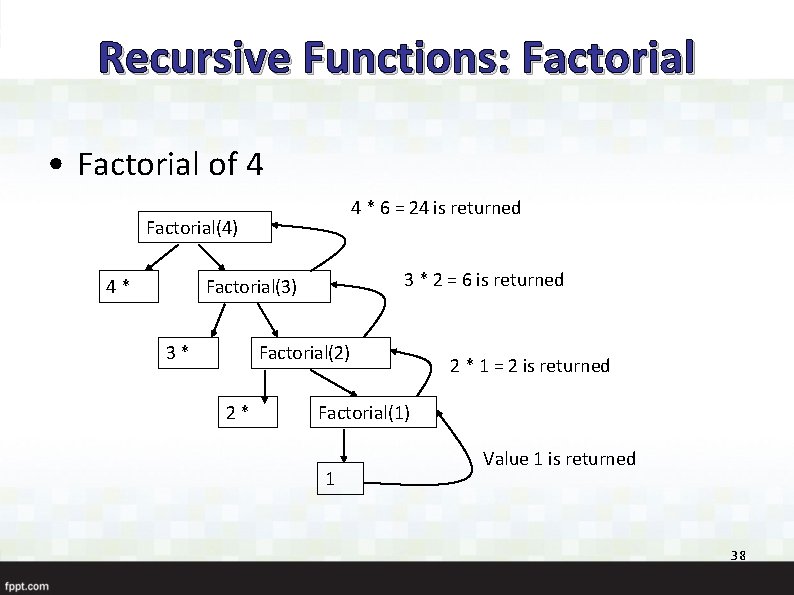
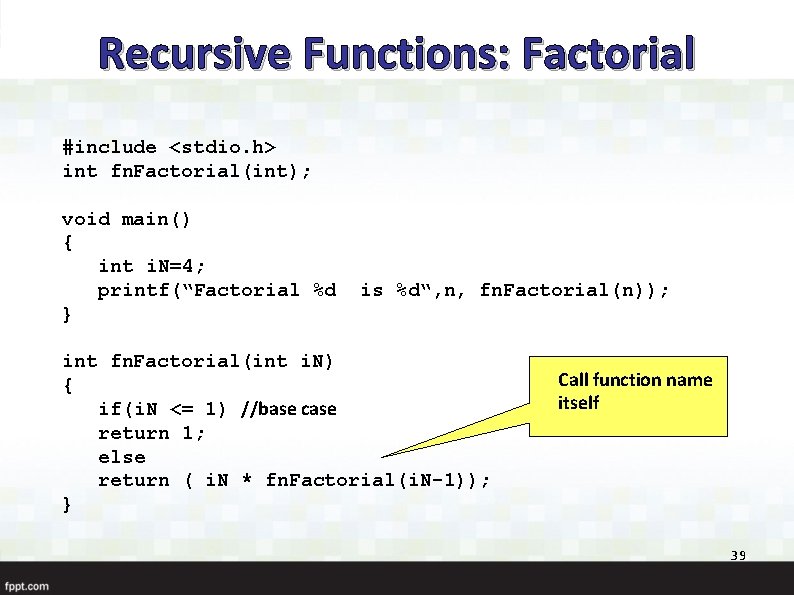
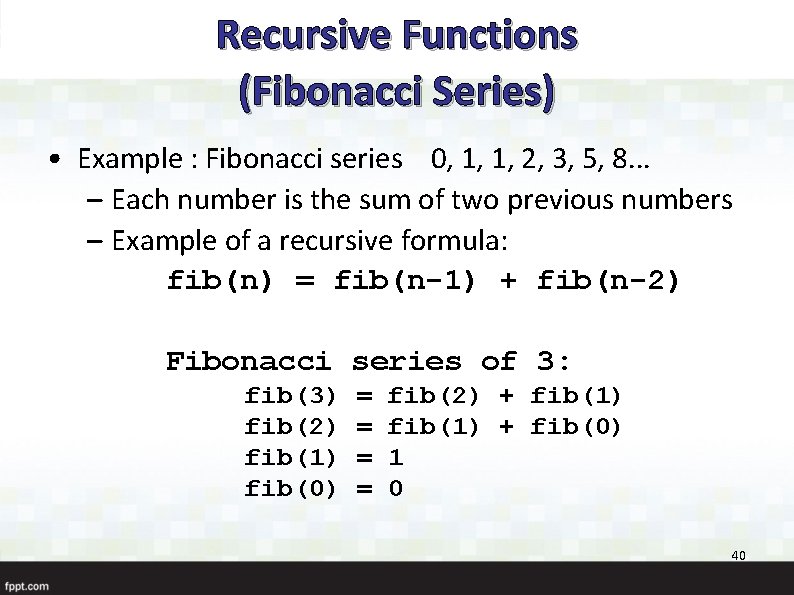
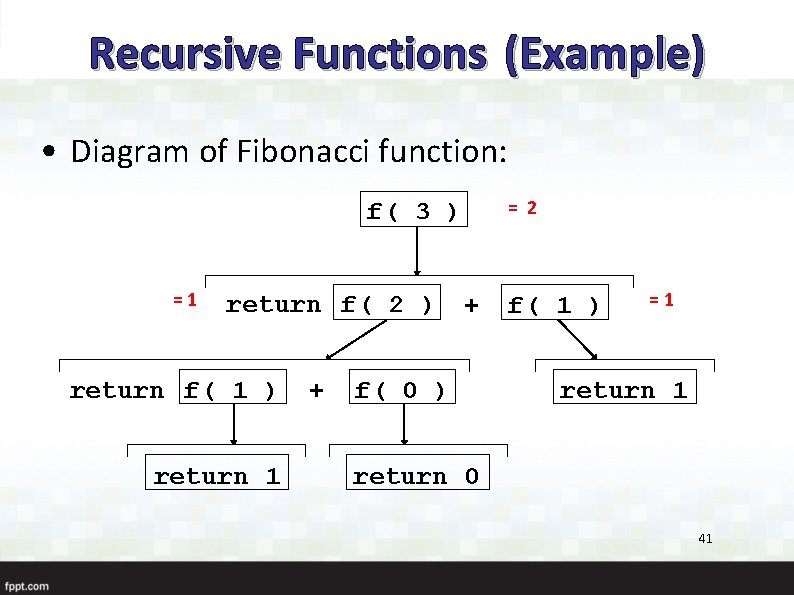
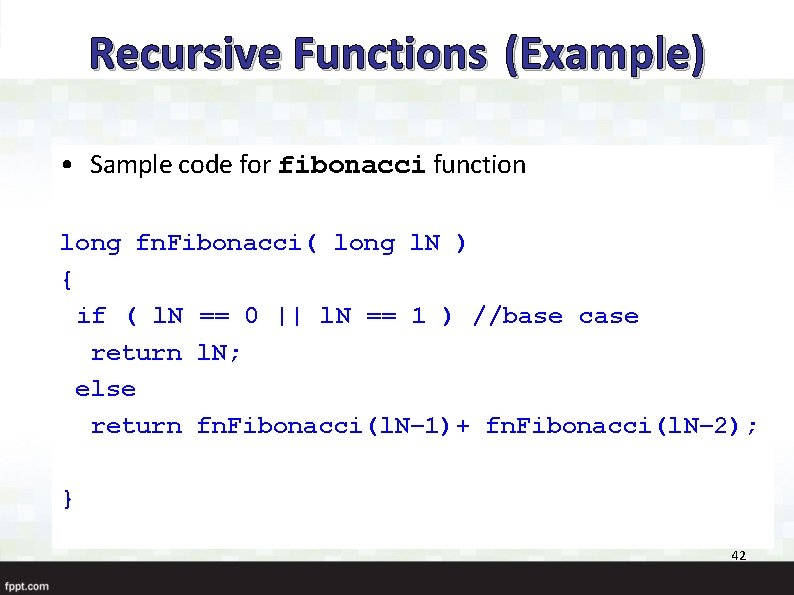
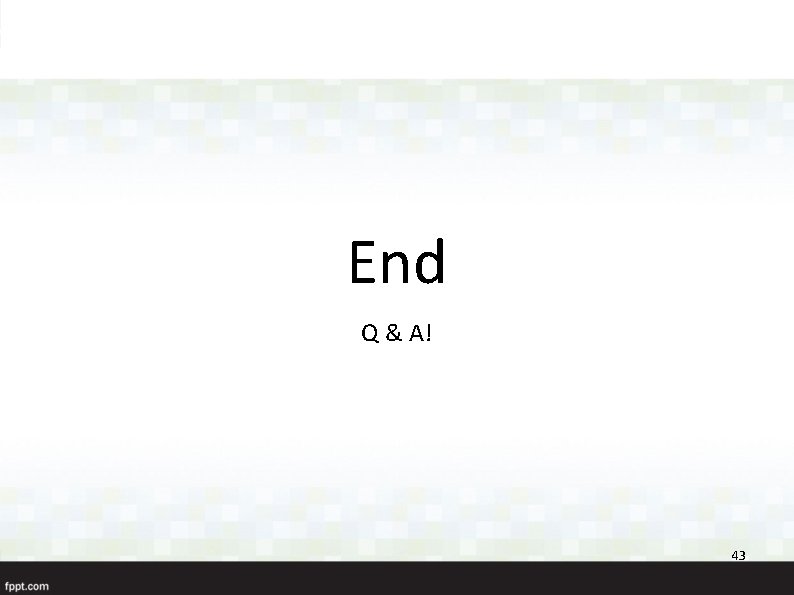
- Slides: 43
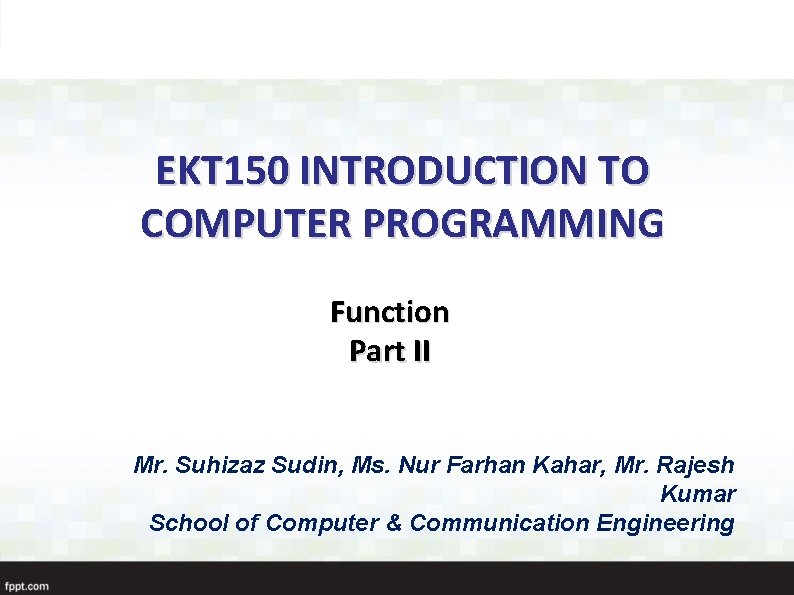
EKT 150 INTRODUCTION TO COMPUTER PROGRAMMING Function Part II Mr. Suhizaz Sudin, Ms. Nur Farhan Kahar, Mr. Rajesh Kumar School of Computer & Communication Engineering
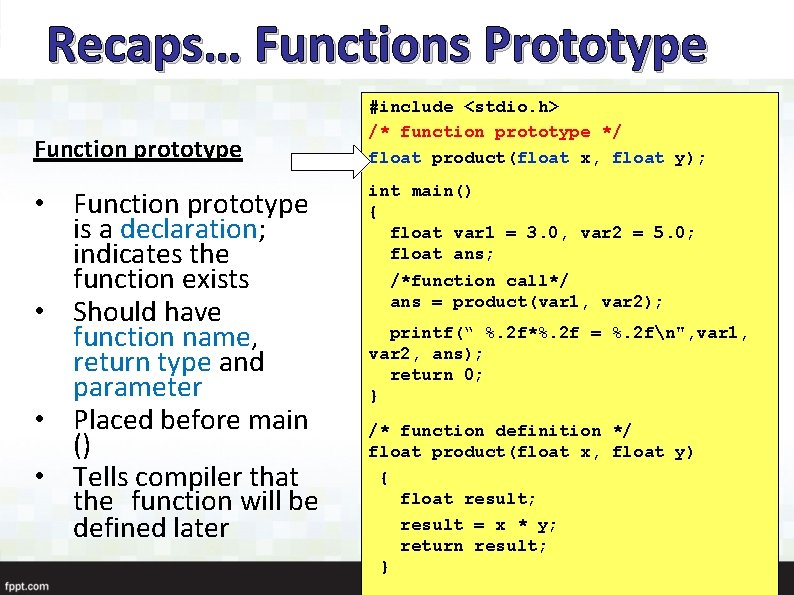
Recaps… Functions Prototype Function prototype • Function prototype is a declaration; indicates the function exists • Should have function name, return type and parameter • Placed before main () • Tells compiler that the function will be defined later #include <stdio. h> /* function prototype */ float product(float x, float y); int main() { float var 1 = 3. 0, var 2 = 5. 0; float ans; /*function call*/ ans = product(var 1, var 2); printf(“ %. 2 f*%. 2 f = %. 2 fn", var 1, var 2, ans); return 0; } /* function definition */ float product(float x, float y) { float result; result = x * y; return result; } 2
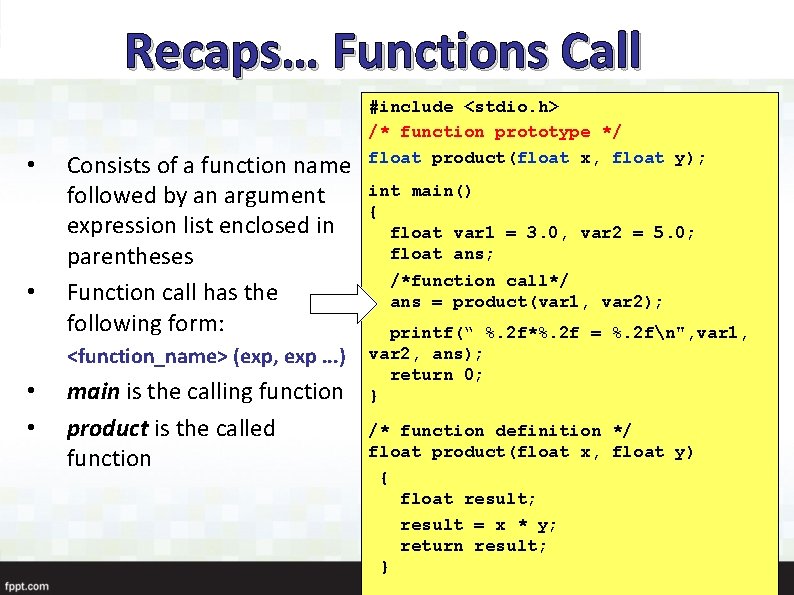
Recaps… Functions Call • • Consists of a function name followed by an argument expression list enclosed in parentheses Function call has the following form: <function_name> (exp, exp. . . ) • • main is the calling function product is the called function #include <stdio. h> /* function prototype */ float product(float x, float y); int main() { float var 1 = 3. 0, var 2 = 5. 0; float ans; /*function call*/ ans = product(var 1, var 2); printf(“ %. 2 f*%. 2 f = %. 2 fn", var 1, var 2, ans); return 0; } /* function definition */ float product(float x, float y) { float result; result = x * y; return result; } 3
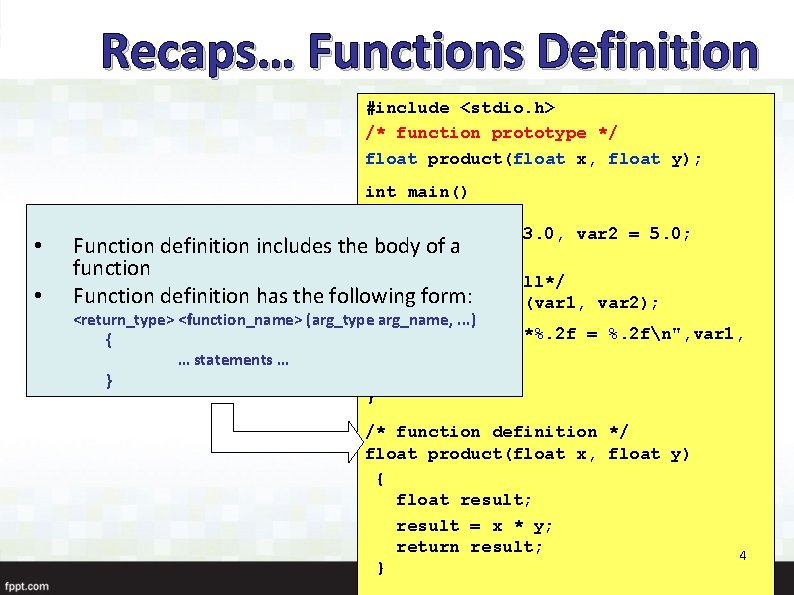
Recaps… Functions Definition #include <stdio. h> /* function prototype */ float product(float x, float y); • • int main() { float var 1 = 3. 0, var 2 = 5. 0; Function definition includes the body of a float ans; function /*function call*/ Function definition has the following form: ans = product(var 1, var 2); <return_type> <function_name> (arg_type arg_name, . . . ) printf(“ %. 2 f*%. 2 f = %. 2 fn", var 1, { var 2, ans); … statements … return 0; } } /* function definition */ float product(float x, float y) { float result; result = x * y; return result; } 4
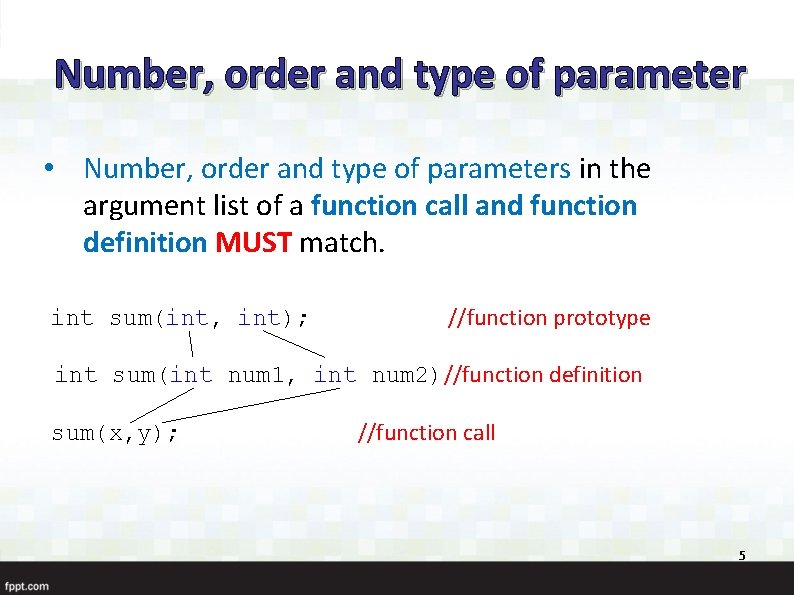
Number, order and type of parameter • Number, order and type of parameters in the argument list of a function call and function definition MUST match. int sum(int, int); //function prototype int sum(int num 1, int num 2)//function definition sum(x, y); //function call 5
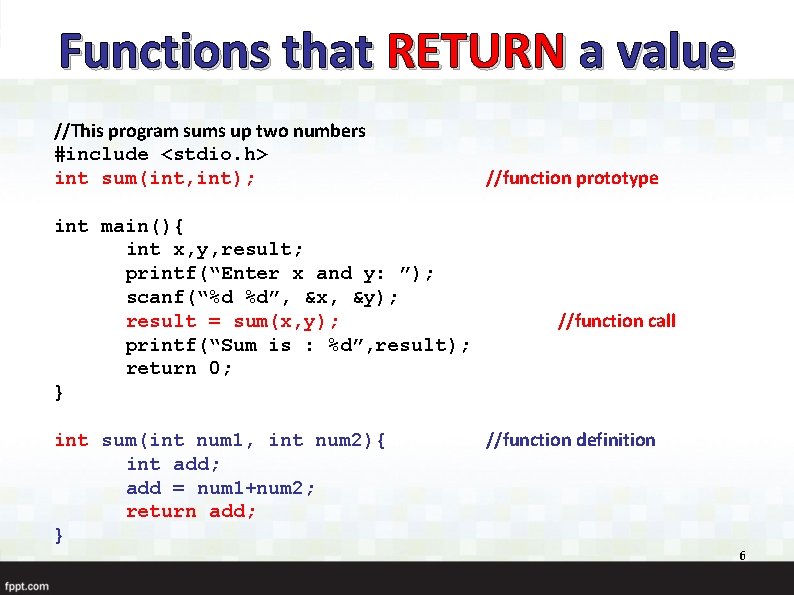
Functions that RETURN a value //This program sums up two numbers #include <stdio. h> int sum(int, int); int main(){ int x, y, result; printf(“Enter x and y: ”); scanf(“%d %d”, &x, &y); result = sum(x, y); printf(“Sum is : %d”, result); return 0; } int sum(int num 1, int num 2){ int add; add = num 1+num 2; return add; } //function prototype //function call //function definition 6
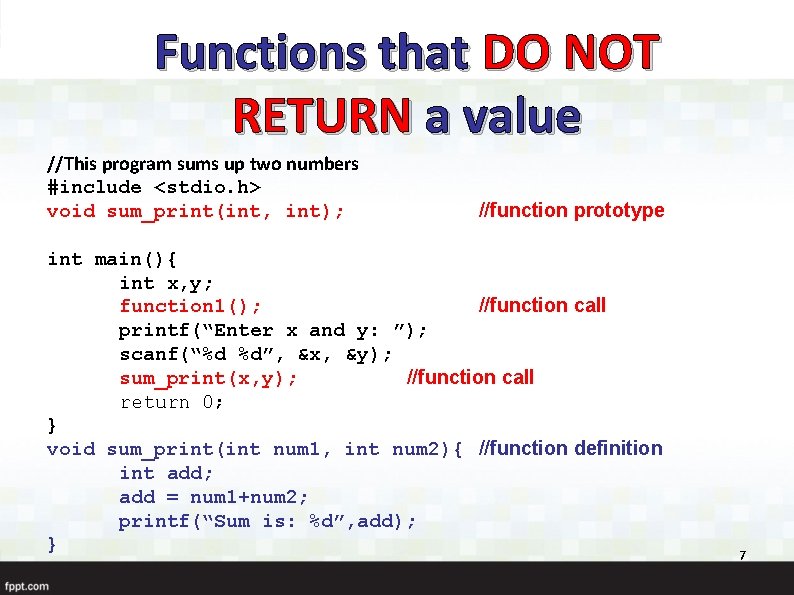
Functions that DO NOT RETURN a value //This program sums up two numbers #include <stdio. h> void sum_print(int, int); //function prototype int main(){ int x, y; function 1(); //function call printf(“Enter x and y: ”); scanf(“%d %d”, &x, &y); sum_print(x, y); //function call return 0; } void sum_print(int num 1, int num 2){ //function definition int add; add = num 1+num 2; printf(“Sum is: %d”, add); } 7
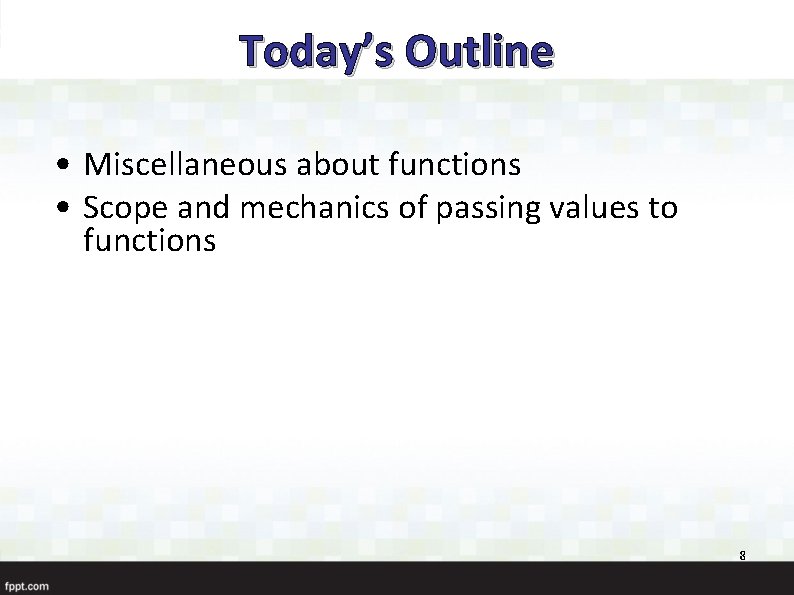
Today’s Outline • Miscellaneous about functions • Scope and mechanics of passing values to functions 8
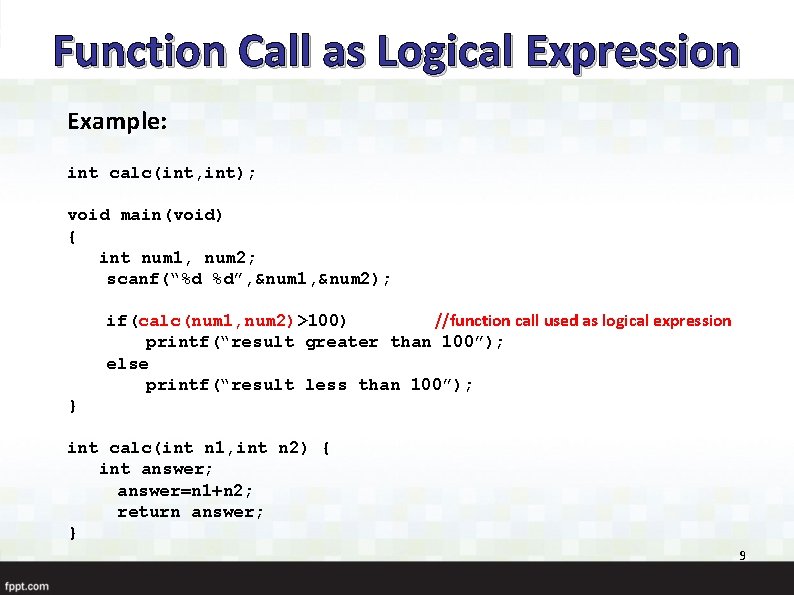
Function Call as Logical Expression Example: int calc(int, int); void main(void) { int num 1, num 2; scanf(“%d %d”, &num 1, &num 2); if(calc(num 1, num 2)>100) //function call used as logical expression printf(“result greater than 100”); else printf(“result less than 100”); } int calc(int n 1, int n 2) { int answer; answer=n 1+n 2; return answer; } 9
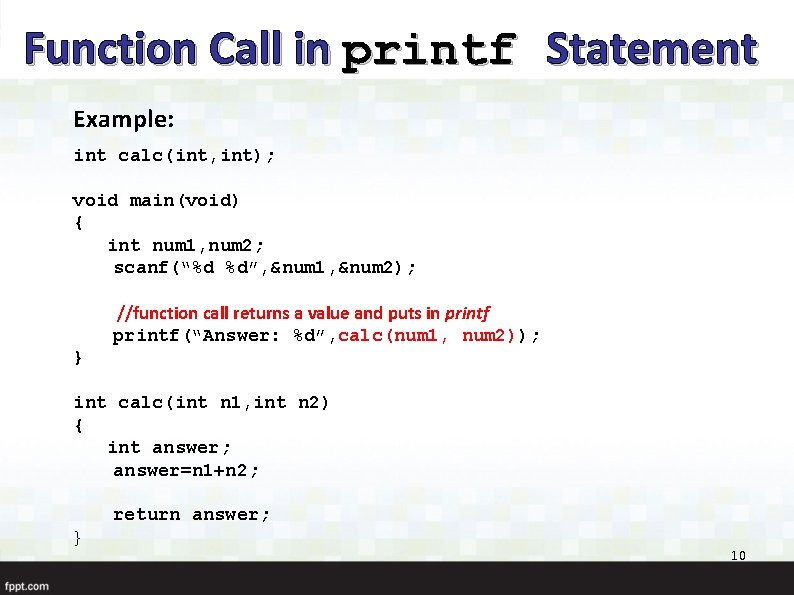
Function Call in printf Statement Example: int calc(int, int); void main(void) { int num 1, num 2; scanf(“%d %d”, &num 1, &num 2); //function call returns a value and puts in printf(“Answer: %d”, calc(num 1, num 2)); } int calc(int n 1, int n 2) { int answer; answer=n 1+n 2; return answer; } 10
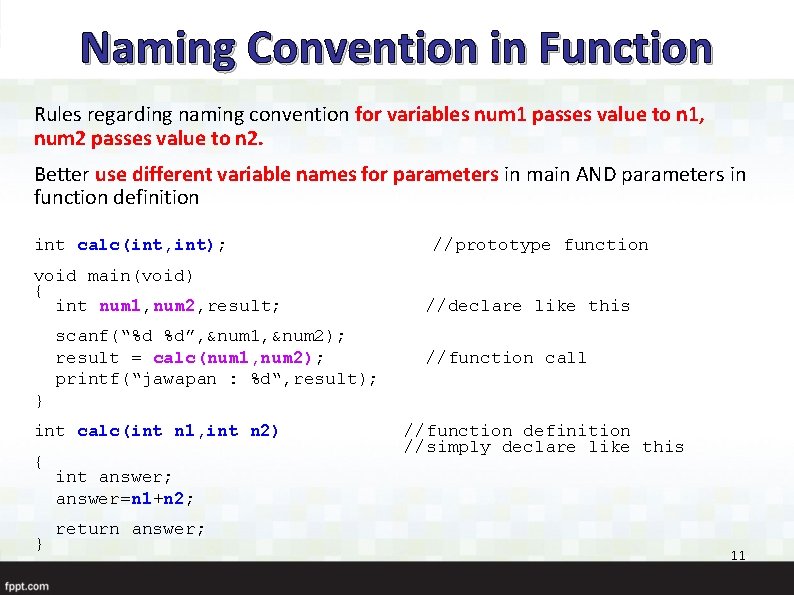
Naming Convention in Function Rules regarding naming convention for variables num 1 passes value to n 1, num 2 passes value to n 2. Better use different variable names for parameters in main AND parameters in function definition int calc(int, int); void main(void) { int num 1, num 2, result; scanf(“%d %d”, &num 1, &num 2); result = calc(num 1, num 2); printf(“jawapan : %d“, result); //prototype function //declare like this //function call } int calc(int n 1, int n 2) { } //function definition //simply declare like this int answer; answer=n 1+n 2; return answer; 11
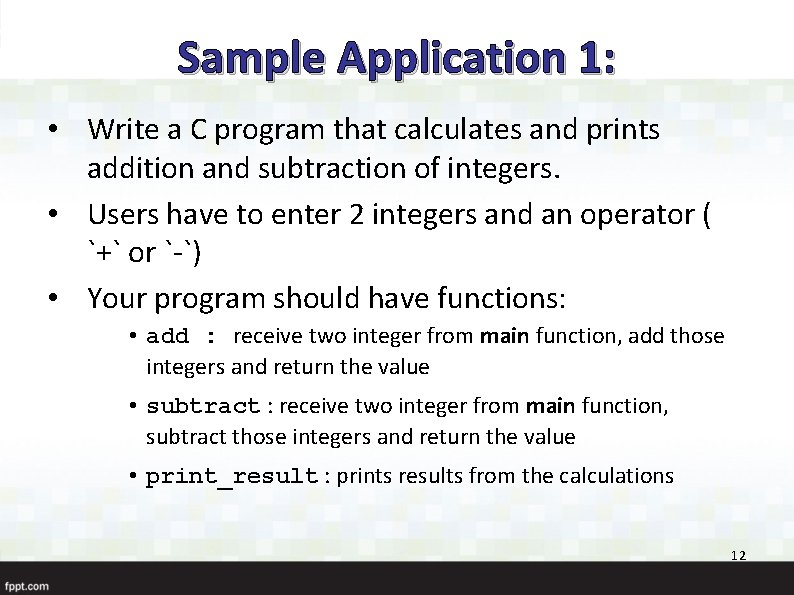
Sample Application 1: • Write a C program that calculates and prints addition and subtraction of integers. • Users have to enter 2 integers and an operator ( `+` or `-`) • Your program should have functions: • add : receive two integer from main function, add those integers and return the value • subtract : receive two integer from main function, subtract those integers and return the value • print_result : prints results from the calculations 12
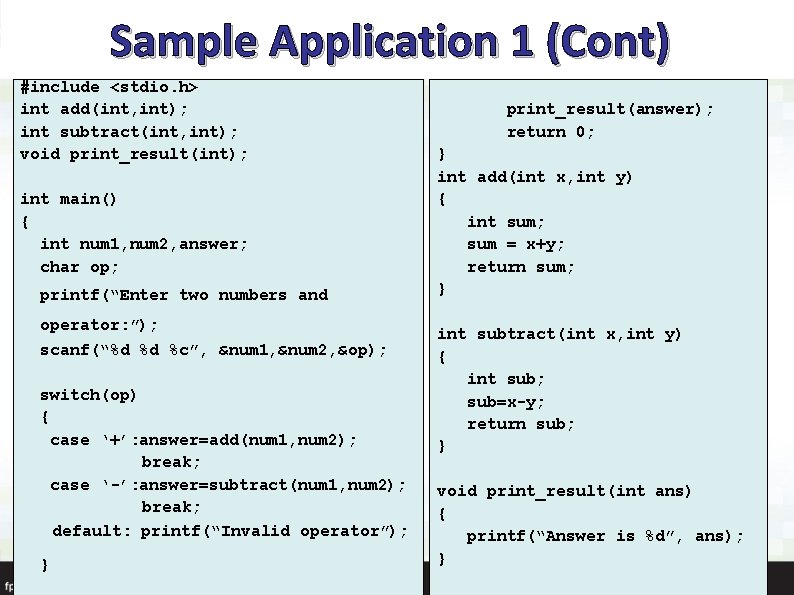
Sample Application 1 (Cont) #include <stdio. h> int add(int, int); int subtract(int, int); void print_result(int); int main() { int num 1, num 2, answer; char op; printf(“Enter two numbers and operator: ”); scanf(“%d %d %c”, &num 1, &num 2, &op); switch(op) { case ‘+’: answer=add(num 1, num 2); break; case ‘-’: answer=subtract(num 1, num 2); break; default: printf(“Invalid operator”); } print_result(answer); return 0; } int add(int x, int y) { int sum; sum = x+y; return sum; } int subtract(int x, int y) { int sub; sub=x-y; return sub; } void print_result(int ans) { printf(“Answer is %d”, ans); 13 }
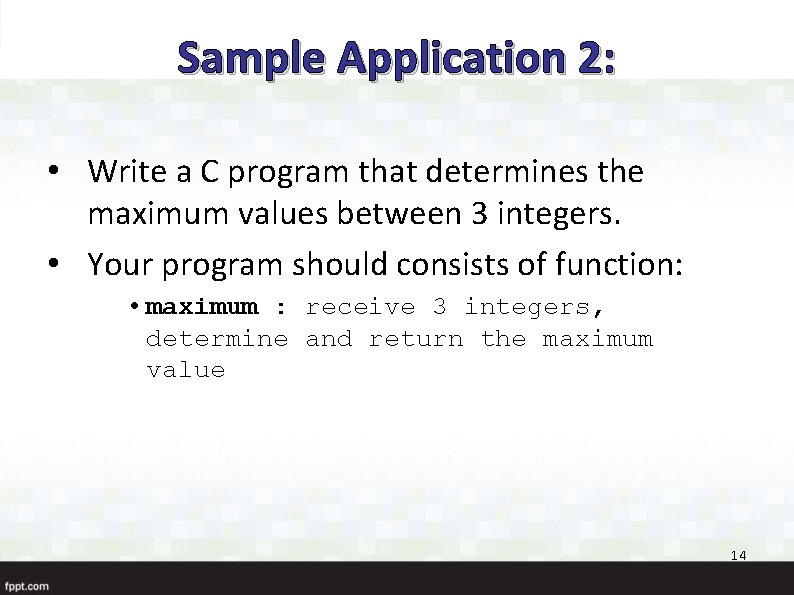
Sample Application 2: • Write a C program that determines the maximum values between 3 integers. • Your program should consists of function: • maximum : receive 3 integers, determine and return the maximum value 14
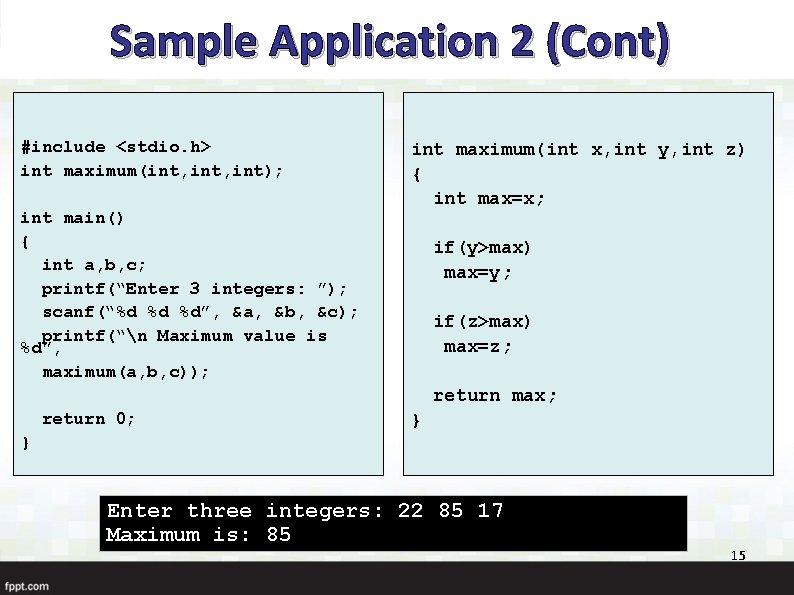
Sample Application 2 (Cont) #include <stdio. h> int maximum(int, int); int main() { int a, b, c; printf(“Enter 3 integers: ”); scanf(“%d %d %d”, &a, &b, &c); printf(“n Maximum value is %d”, maximum(a, b, c)); int maximum(int x, int y, int z) { int max=x; if(y>max) max=y; if(z>max) max=z; return max; return 0; } } Enter three integers: 22 85 17 Maximum is: 85 15
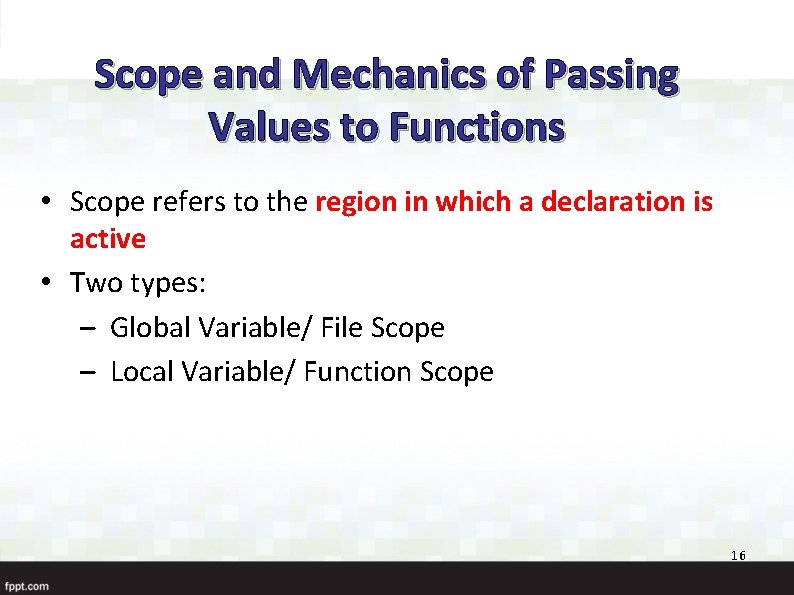
Scope and Mechanics of Passing Values to Functions • Scope refers to the region in which a declaration is active • Two types: – Global Variable/ File Scope – Local Variable/ Function Scope 16
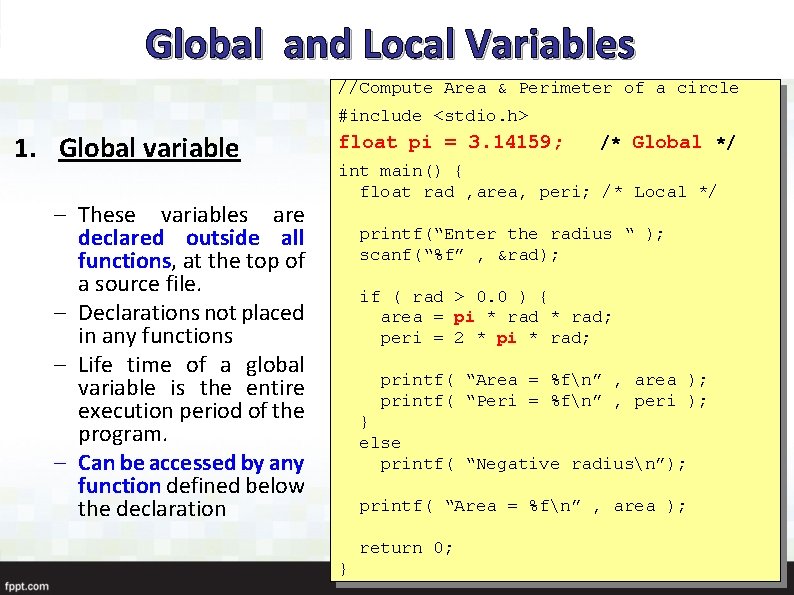
Global and Local Variables //Compute Area & Perimeter of a circle #include <stdio. h> 1. Global variable – These variables are declared outside all functions, at the top of a source file. – Declarations not placed in any functions – Life time of a global variable is the entire execution period of the program. – Can be accessed by any function defined below the declaration float pi = 3. 14159; /* Global */ int main() { float rad , area, peri; /* Local */ printf(“Enter the radius “ ); scanf(“%f” , &rad); if ( rad > 0. 0 ) { area = pi * rad; peri = 2 * pi * rad; printf( “Area = %fn” , area ); printf( “Peri = %fn” , peri ); } else printf( “Negative radiusn”); printf( “Area = %fn” , area ); return 0; } 17
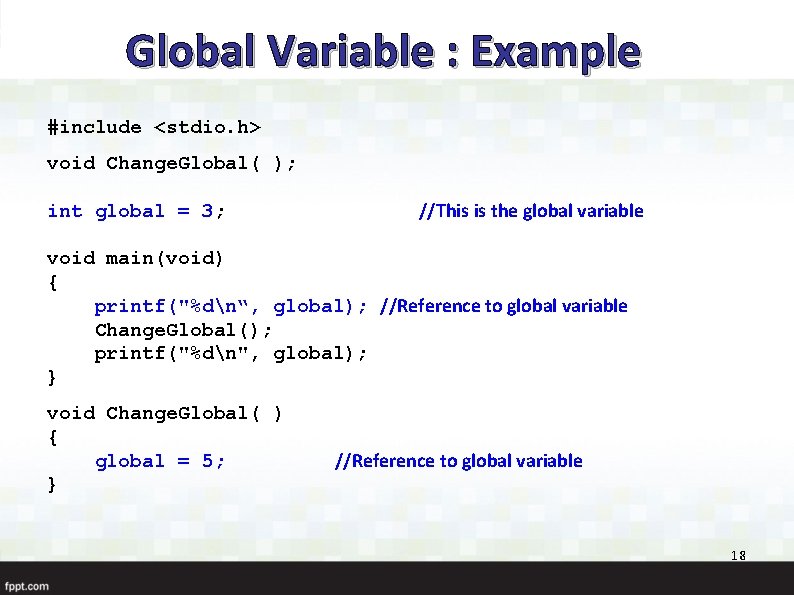
Global Variable : Example #include <stdio. h> void Change. Global( ); int global = 3; //This is the global variable void main(void) { printf("%dn“, global); //Reference to global variable Change. Global(); printf("%dn", global); } void Change. Global( ) { global = 5; } //Reference to global variable 18
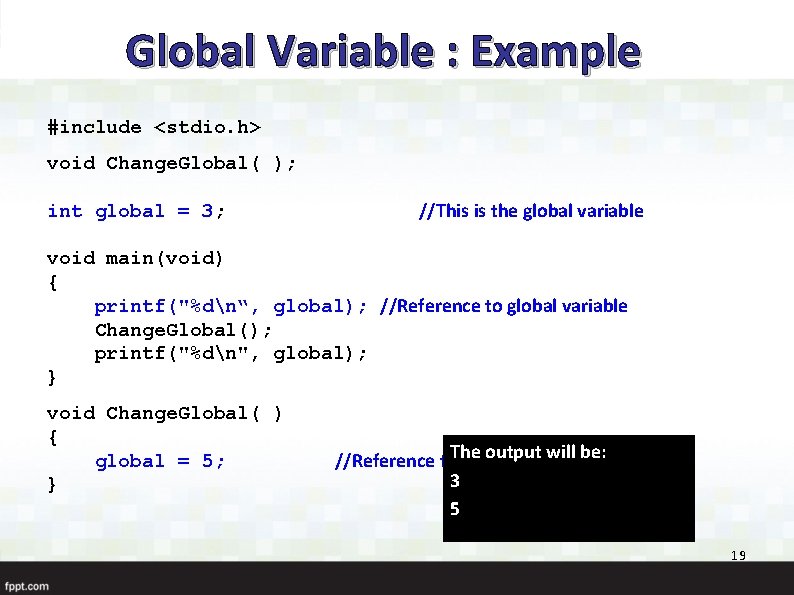
Global Variable : Example #include <stdio. h> void Change. Global( ); int global = 3; //This is the global variable void main(void) { printf("%dn“, global); //Reference to global variable Change. Global(); printf("%dn", global); } void Change. Global( ) { global = 5; } output will be: //Reference to. The global variable 3 5 19
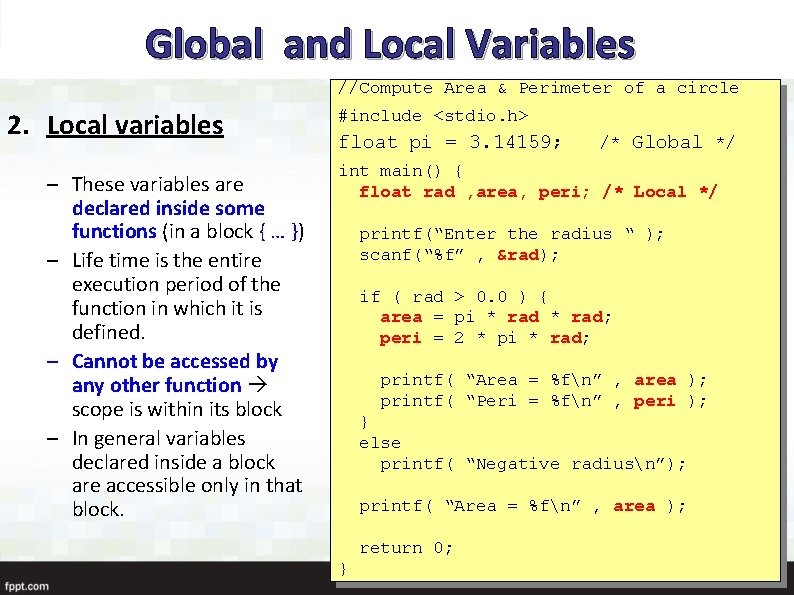
Global and Local Variables //Compute Area & Perimeter of a circle 2. Local variables – These variables are declared inside some functions (in a block { … }) – Life time is the entire execution period of the function in which it is defined. – Cannot be accessed by any other function scope is within its block – In general variables declared inside a block are accessible only in that block. #include <stdio. h> float pi = 3. 14159; /* Global */ int main() { float rad , area, peri; /* Local */ printf(“Enter the radius “ ); scanf(“%f” , &rad); if ( rad > 0. 0 ) { area = pi * rad; peri = 2 * pi * rad; printf( “Area = %fn” , area ); printf( “Peri = %fn” , peri ); } else printf( “Negative radiusn”); printf( “Area = %fn” , area ); return 0; } 20
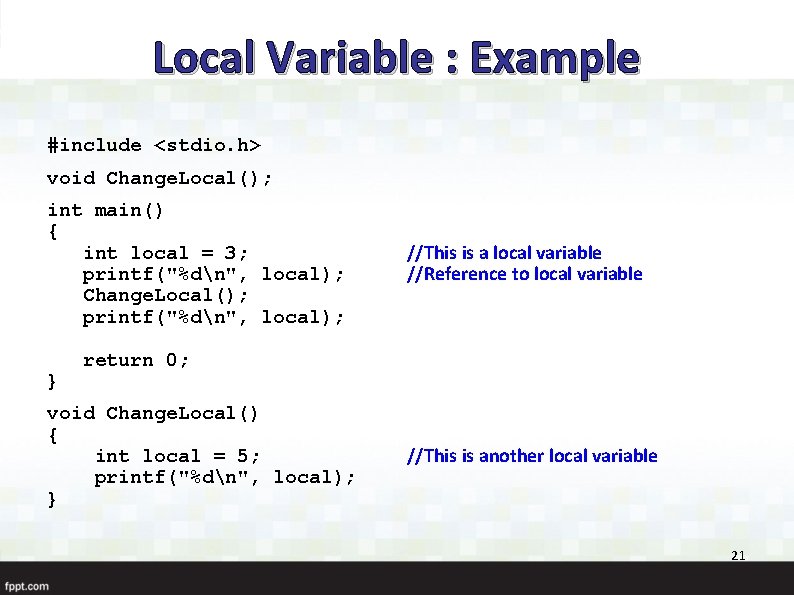
Local Variable : Example #include <stdio. h> void Change. Local(); int main() { int local = 3; printf("%dn", local); Change. Local(); printf("%dn", local); } //This is a local variable //Reference to local variable return 0; void Change. Local() { int local = 5; printf("%dn", local); } //This is another local variable 21
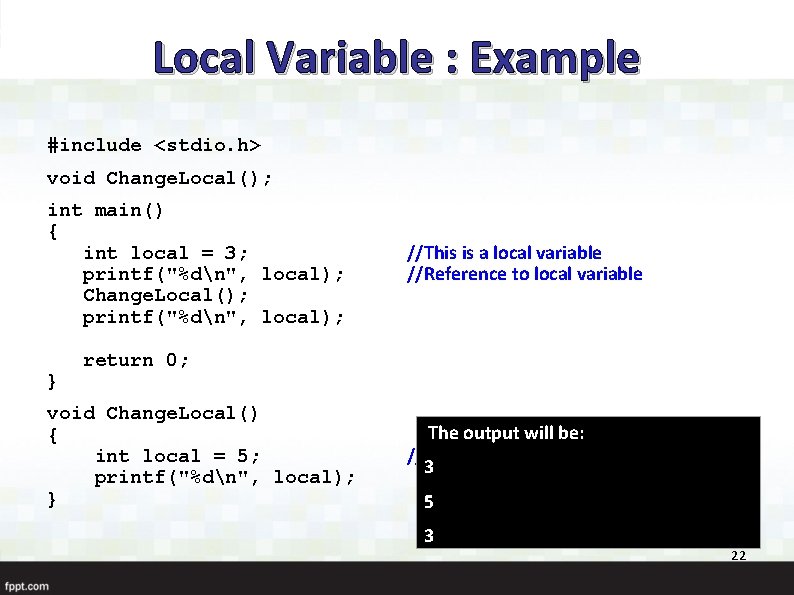
Local Variable : Example #include <stdio. h> void Change. Local(); int main() { int local = 3; printf("%dn", local); Change. Local(); printf("%dn", local); } //This is a local variable //Reference to local variable return 0; void Change. Local() { int local = 5; printf("%dn", local); } The output will be: //This is another local variable 3 5 3 22
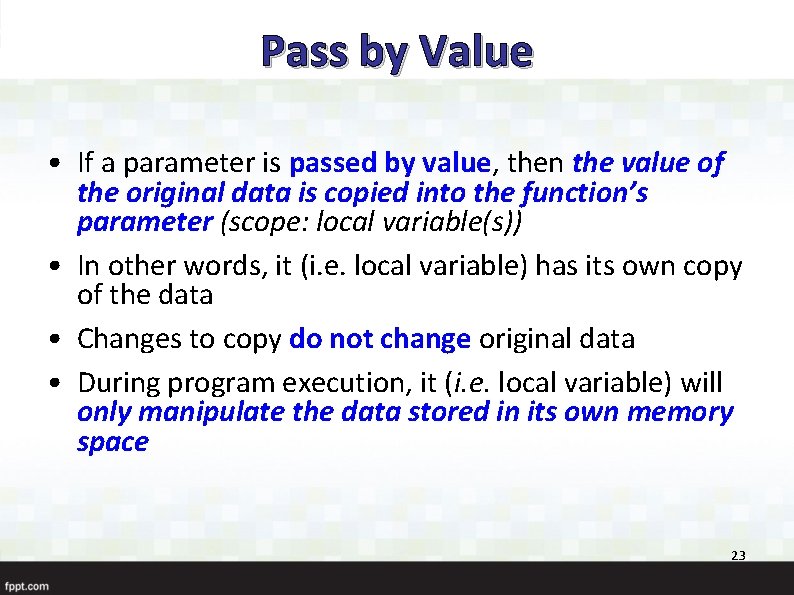
Pass by Value • If a parameter is passed by value, then the value of the original data is copied into the function’s parameter (scope: local variable(s)) • In other words, it (i. e. local variable) has its own copy of the data • Changes to copy do not change original data • During program execution, it (i. e. local variable) will only manipulate the data stored in its own memory space 23
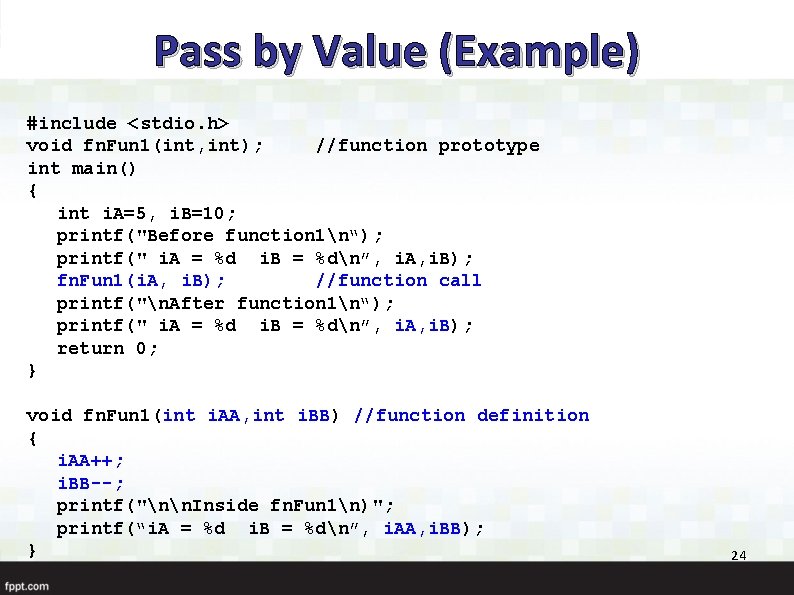
Pass by Value (Example) #include <stdio. h> void fn. Fun 1(int, int); //function prototype int main() { int i. A=5, i. B=10; printf("Before function 1n“); printf(" i. A = %d i. B = %dn”, i. A, i. B); fn. Fun 1(i. A, i. B); //function call printf("n. After function 1n“); printf(" i. A = %d i. B = %dn”, i. A, i. B); return 0; } void fn. Fun 1(int i. AA, int i. BB) //function definition { i. AA++; i. BB--; printf("nn. Inside fn. Fun 1n)"; printf(“i. A = %d i. B = %dn”, i. AA, i. BB); } 24
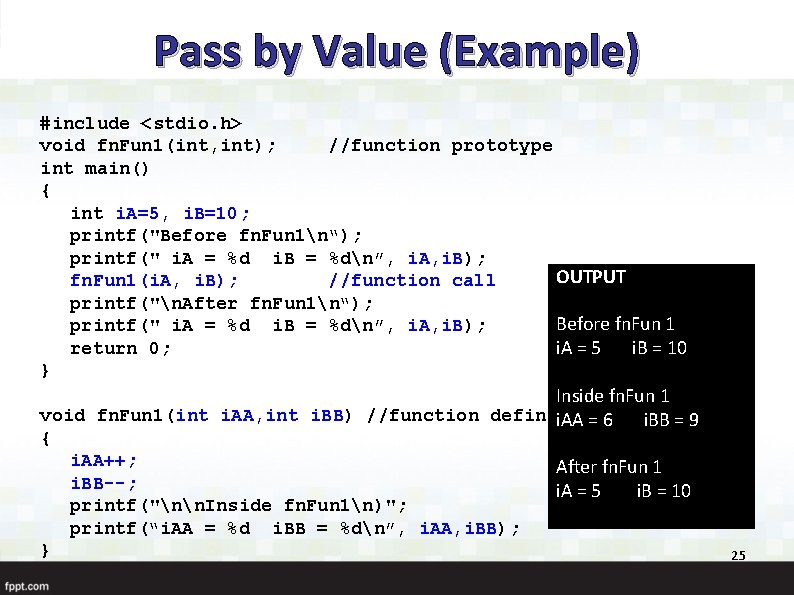
Pass by Value (Example) #include <stdio. h> void fn. Fun 1(int, int); //function prototype int main() { int i. A=5, i. B=10; printf("Before fn. Fun 1n“); printf(" i. A = %d i. B = %dn”, i. A, i. B); OUTPUT fn. Fun 1(i. A, i. B); //function call printf("n. After fn. Fun 1n“); Before fn. Fun 1 printf(" i. A = %d i. B = %dn”, i. A, i. B); return 0; i. A = 5 i. B = 10 } Inside fn. Fun 1 void fn. Fun 1(int i. AA, int i. BB) //function definition i. AA = 6 i. BB = 9 { i. AA++; i. BB--; printf("nn. Inside fn. Fun 1n)"; printf(“i. AA = %d i. BB = %dn”, i. AA, i. BB); } After fn. Fun 1 i. A = 5 i. B = 10 25
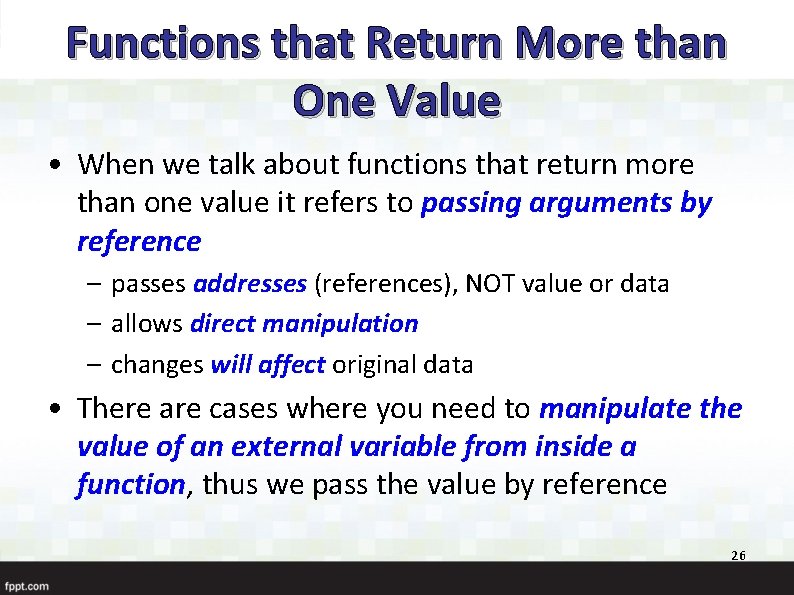
Functions that Return More than One Value • When we talk about functions that return more than one value it refers to passing arguments by reference – passes addresses (references), NOT value or data – allows direct manipulation – changes will affect original data • There are cases where you need to manipulate the value of an external variable from inside a function, thus we pass the value by reference 26
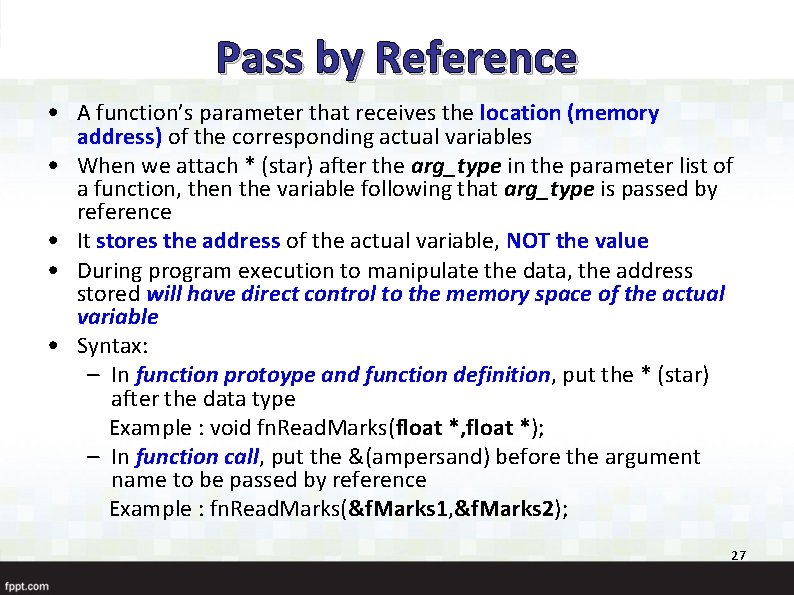
Pass by Reference • A function’s parameter that receives the location (memory address) of the corresponding actual variables • When we attach * (star) after the arg_type in the parameter list of a function, then the variable following that arg_type is passed by reference • It stores the address of the actual variable, NOT the value • During program execution to manipulate the data, the address stored will have direct control to the memory space of the actual variable • Syntax: – In function protoype and function definition, put the * (star) after the data type Example : void fn. Read. Marks(float *, float *); – In function call, put the &(ampersand) before the argument name to be passed by reference Example : fn. Read. Marks(&f. Marks 1, &f. Marks 2); 27
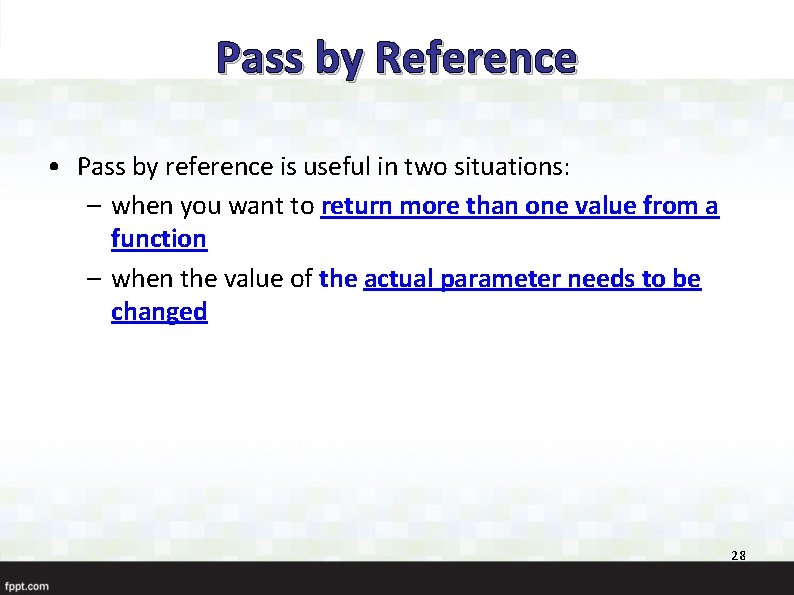
Pass by Reference • Pass by reference is useful in two situations: – when you want to return more than one value from a function – when the value of the actual parameter needs to be changed 28
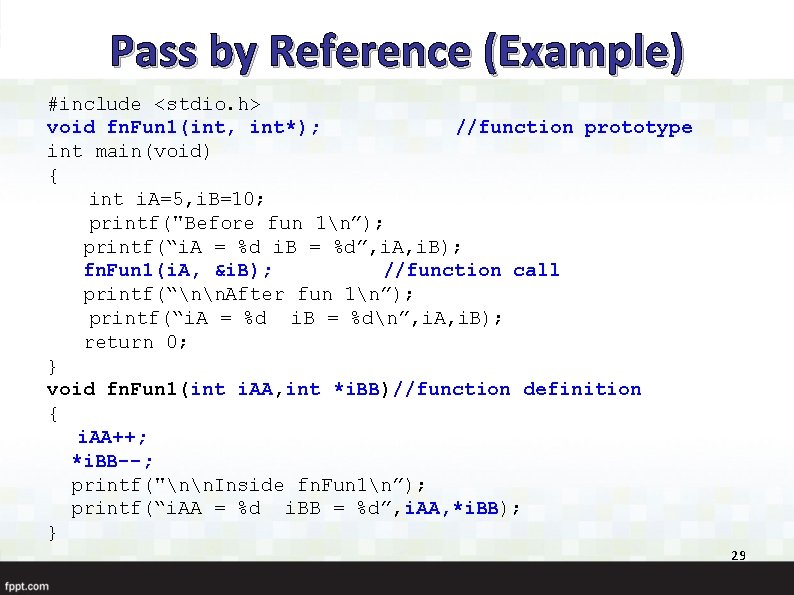
Pass by Reference (Example) #include <stdio. h> void fn. Fun 1(int, int*); //function prototype int main(void) { int i. A=5, i. B=10; printf("Before fun 1n”); printf(“i. A = %d i. B = %d”, i. A, i. B); fn. Fun 1(i. A, &i. B); //function call printf(“nn. After fun 1n”); printf(“i. A = %d i. B = %dn”, i. A, i. B); return 0; } void fn. Fun 1(int i. AA, int *i. BB)//function definition { i. AA++; *i. BB--; printf("nn. Inside fn. Fun 1n”); printf(“i. AA = %d i. BB = %d”, i. AA, *i. BB); } 29
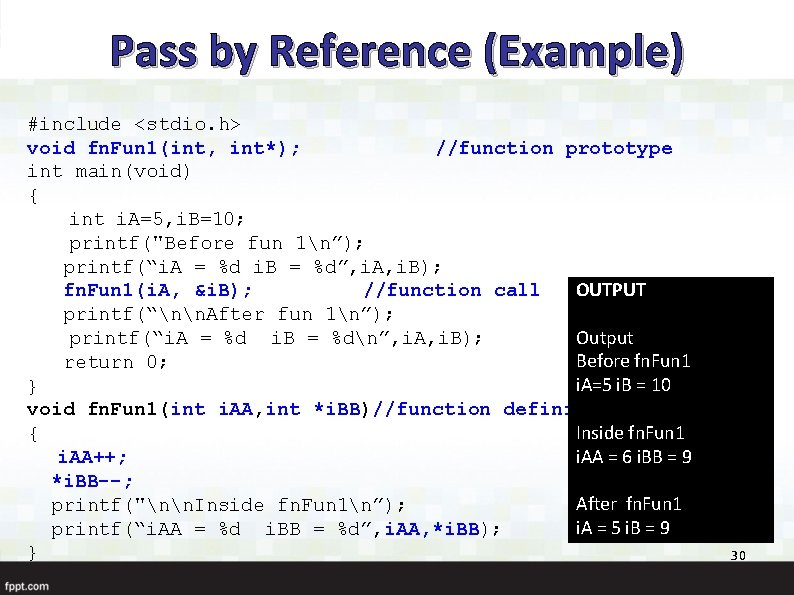
Pass by Reference (Example) #include <stdio. h> void fn. Fun 1(int, int*); //function prototype int main(void) { int i. A=5, i. B=10; printf("Before fun 1n”); printf(“i. A = %d i. B = %d”, i. A, i. B); OUTPUT fn. Fun 1(i. A, &i. B); //function call printf(“nn. After fun 1n”); Output printf(“i. A = %d i. B = %dn”, i. A, i. B); Before fn. Fun 1 return 0; i. A=5 i. B = 10 } void fn. Fun 1(int i. AA, int *i. BB)//function definition Inside fn. Fun 1 { i. AA = 6 i. BB = 9 i. AA++; *i. BB--; After fn. Fun 1 printf("nn. Inside fn. Fun 1n”); i. A = 5 i. B = 9 printf(“i. AA = %d i. BB = %d”, i. AA, *i. BB); } 30
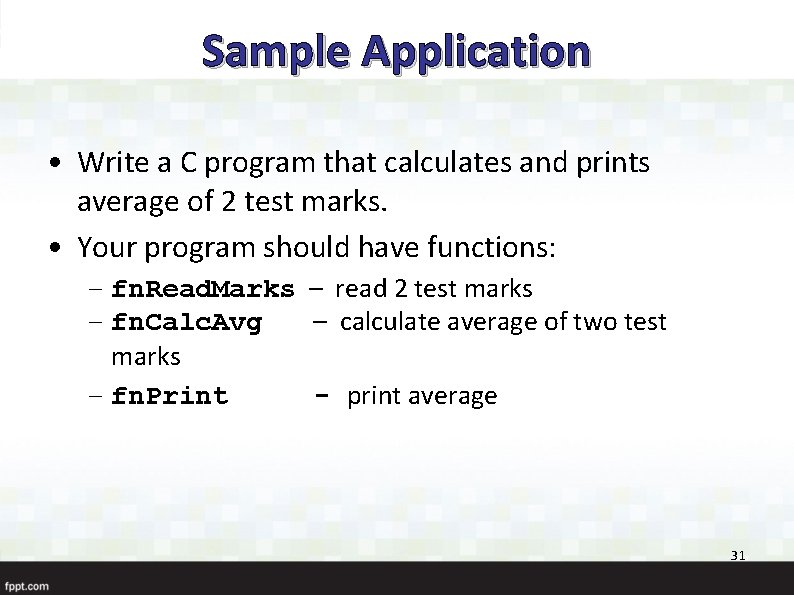
Sample Application • Write a C program that calculates and prints average of 2 test marks. • Your program should have functions: – fn. Read. Marks – read 2 test marks – fn. Calc. Avg – calculate average of two test marks – fn. Print - print average 31
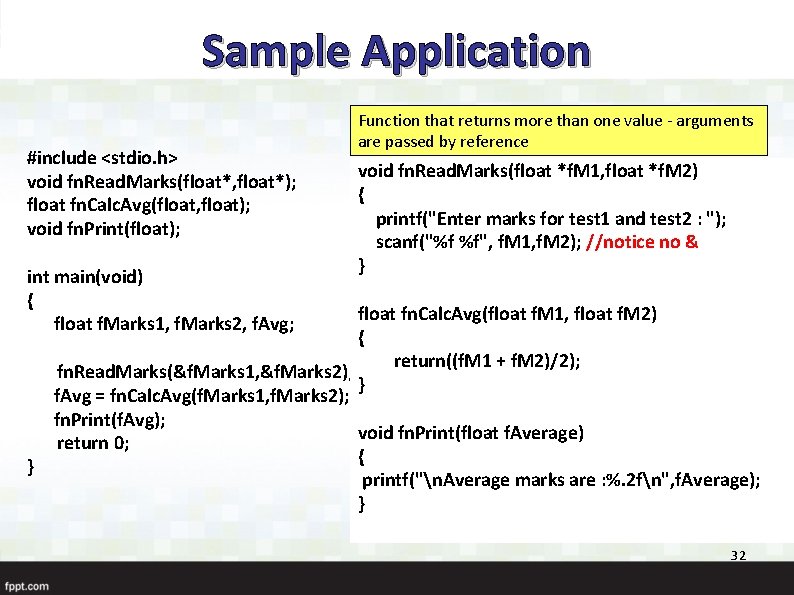
Sample Application #include <stdio. h> void fn. Read. Marks(float*, float*); float fn. Calc. Avg(float, float); void fn. Print(float); int main(void) { float f. Marks 1, f. Marks 2, f. Avg; } Function that returns more than one value - arguments are passed by reference void fn. Read. Marks(float *f. M 1, float *f. M 2) { printf("Enter marks for test 1 and test 2 : "); scanf("%f %f", f. M 1, f. M 2); //notice no & } float fn. Calc. Avg(float f. M 1, float f. M 2) { return((f. M 1 + f. M 2)/2); fn. Read. Marks(&f. Marks 1, &f. Marks 2); } f. Avg = fn. Calc. Avg(f. Marks 1, f. Marks 2); fn. Print(f. Avg); void fn. Print(float f. Average) return 0; { printf("n. Average marks are : %. 2 fn", f. Average); } 32
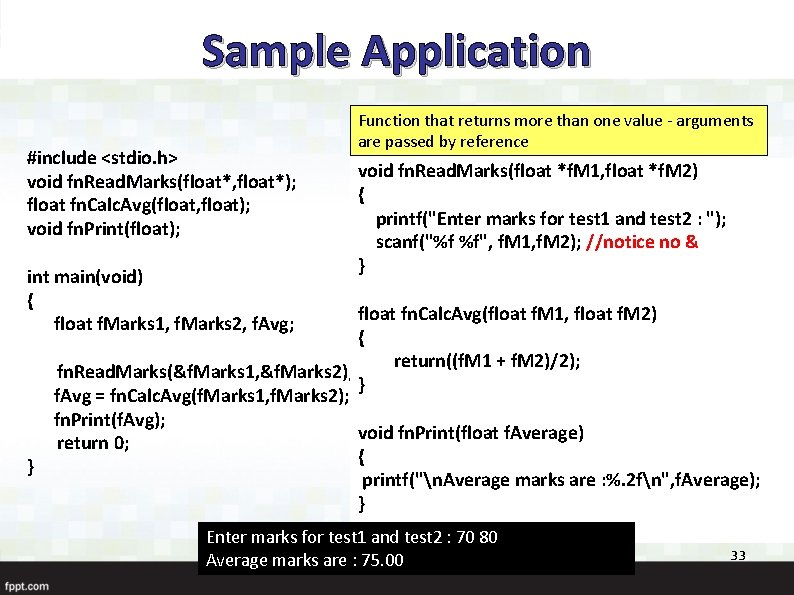
Sample Application #include <stdio. h> void fn. Read. Marks(float*, float*); float fn. Calc. Avg(float, float); void fn. Print(float); int main(void) { float f. Marks 1, f. Marks 2, f. Avg; } Function that returns more than one value - arguments are passed by reference void fn. Read. Marks(float *f. M 1, float *f. M 2) { printf("Enter marks for test 1 and test 2 : "); scanf("%f %f", f. M 1, f. M 2); //notice no & } float fn. Calc. Avg(float f. M 1, float f. M 2) { return((f. M 1 + f. M 2)/2); fn. Read. Marks(&f. Marks 1, &f. Marks 2); } f. Avg = fn. Calc. Avg(f. Marks 1, f. Marks 2); fn. Print(f. Avg); void fn. Print(float f. Average) return 0; { printf("n. Average marks are : %. 2 fn", f. Average); } Enter marks for test 1 and test 2 : 70 80 Average marks are : 75. 00 33
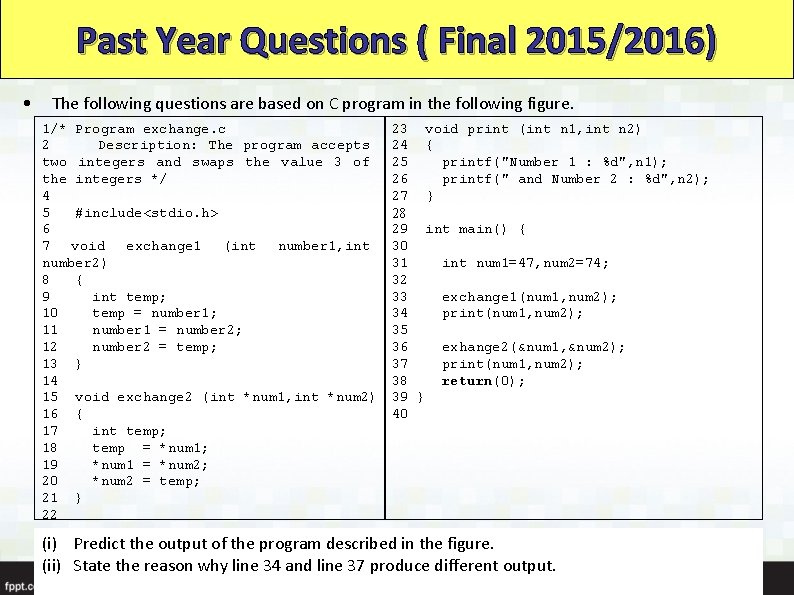
Past Year Questions ( Final 2015/2016) • The following questions are based on C program in the following figure. 1/* Program exchange. c 2 Description: The program accepts two integers and swaps the value 3 of the integers */ 4 5 #include<stdio. h> 6 7 void exchange 1 (int number 1, int number 2) 8 { 9 int temp; 10 temp = number 1; 11 number 1 = number 2; 12 number 2 = temp; 13 } 14 15 void exchange 2 (int *num 1, int *num 2) 16 { 17 int temp; 18 temp = *num 1; 19 *num 1 = *num 2; 20 *num 2 = temp; 21 } 22 23 void print (int n 1, int n 2) 24 { 25 printf("Number 1 : %d", n 1); 26 printf(" and Number 2 : %d", n 2); 27 } 28 29 int main() { 30 31 int num 1=47, num 2=74; 32 33 exchange 1(num 1, num 2); 34 print(num 1, num 2); 35 36 exhange 2(&num 1, &num 2); 37 print(num 1, num 2); 38 return(0); 39 } 40 (i) Predict the output of the program described in the figure. (ii) State the reason why line 34 and line 37 produce different output.
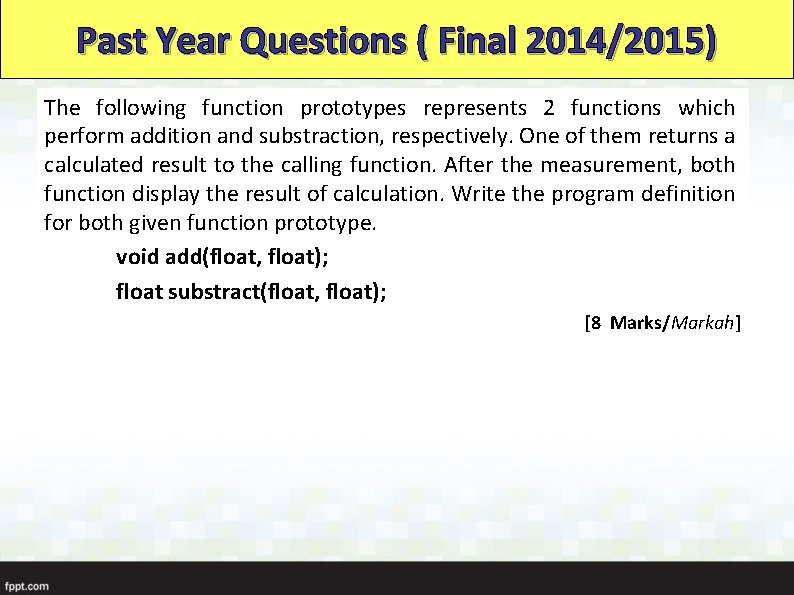
Past Year Questions ( Final 2014/2015) The following function prototypes represents 2 functions which perform addition and substraction, respectively. One of them returns a calculated result to the calling function. After the measurement, both function display the result of calculation. Write the program definition for both given function prototype. void add(float, float); float substract(float, float); [8 Marks/Markah]
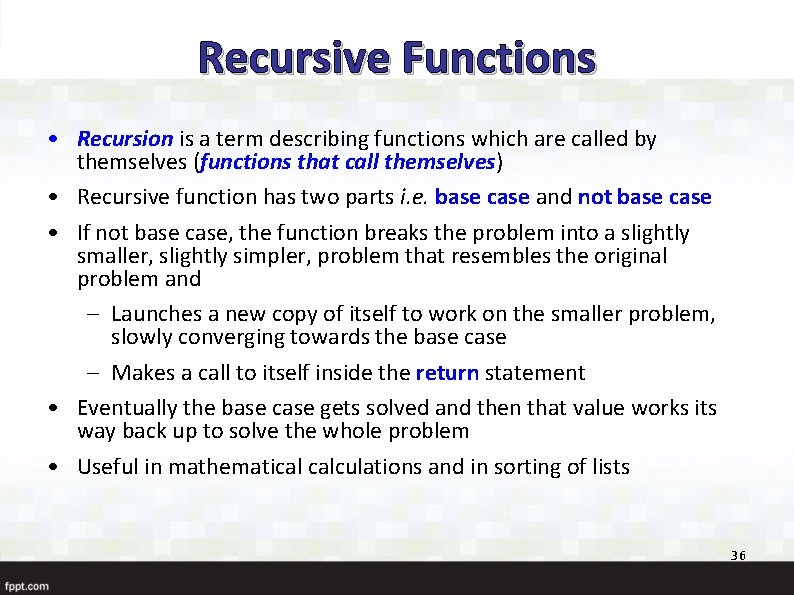
Recursive Functions • Recursion is a term describing functions which are called by themselves (functions that call themselves) • Recursive function has two parts i. e. base case and not base case • If not base case, the function breaks the problem into a slightly smaller, slightly simpler, problem that resembles the original problem and – Launches a new copy of itself to work on the smaller problem, slowly converging towards the base case – Makes a call to itself inside the return statement • Eventually the base case gets solved and then that value works its way back up to solve the whole problem • Useful in mathematical calculations and in sorting of lists 36
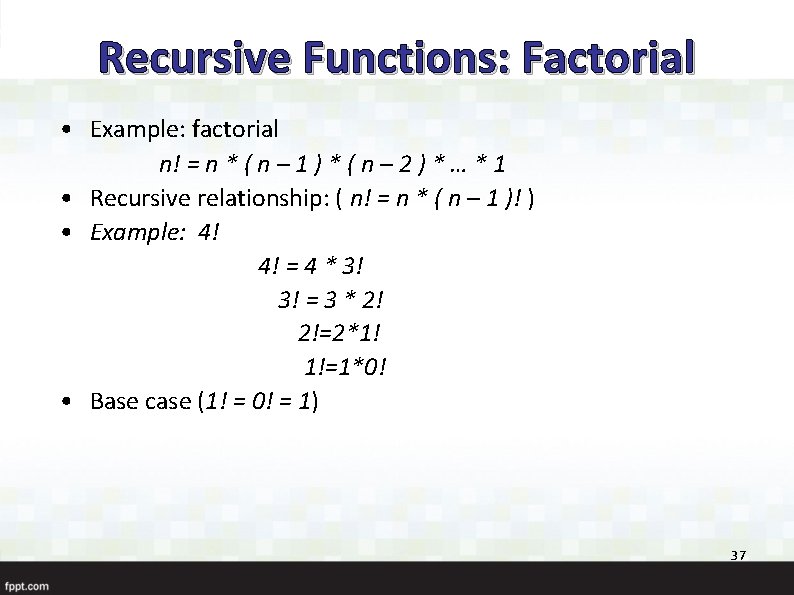
Recursive Functions: Factorial • Example: factorial n! = n * ( n – 1 ) * ( n – 2 ) * … * 1 • Recursive relationship: ( n! = n * ( n – 1 )! ) • Example: 4! 4! = 4 * 3! 3! = 3 * 2! 2!=2*1! 1!=1*0! • Base case (1! = 0! = 1) 37
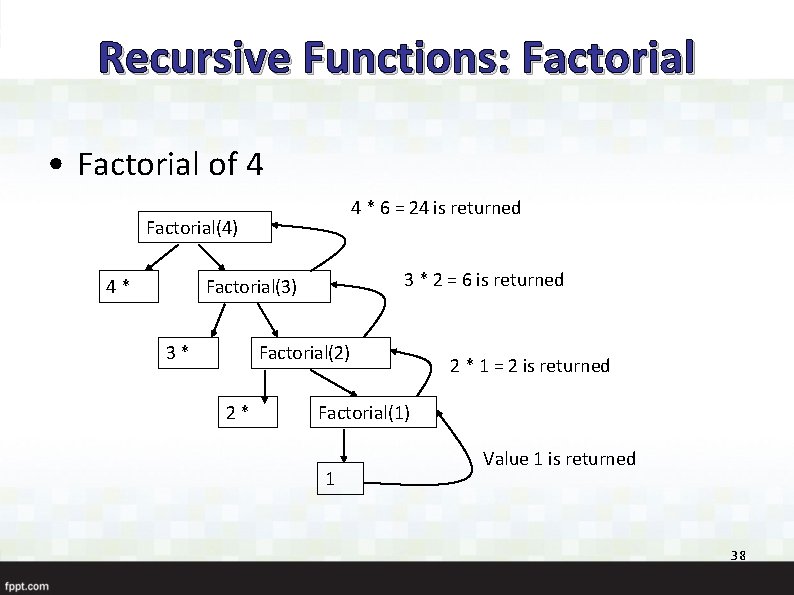
Recursive Functions: Factorial • Factorial of 4 4 * 6 = 24 is returned Factorial(4) 4 * 3 * 2 = 6 is returned Factorial(3) 3 * Factorial(2) 2 * 1 = 2 is returned Factorial(1) 1 Value 1 is returned 38
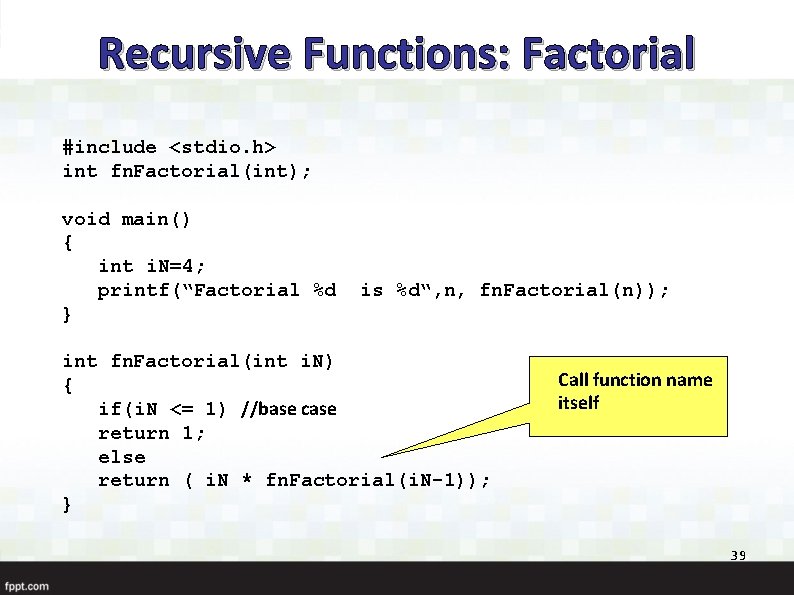
Recursive Functions: Factorial #include <stdio. h> int fn. Factorial(int); void main() { int i. N=4; printf(“Factorial %d } is %d“, n, fn. Factorial(n)); int fn. Factorial(int i. N) { if(i. N <= 1) //base case return 1; else return ( i. N * fn. Factorial(i. N-1)); } Call function name itself 39
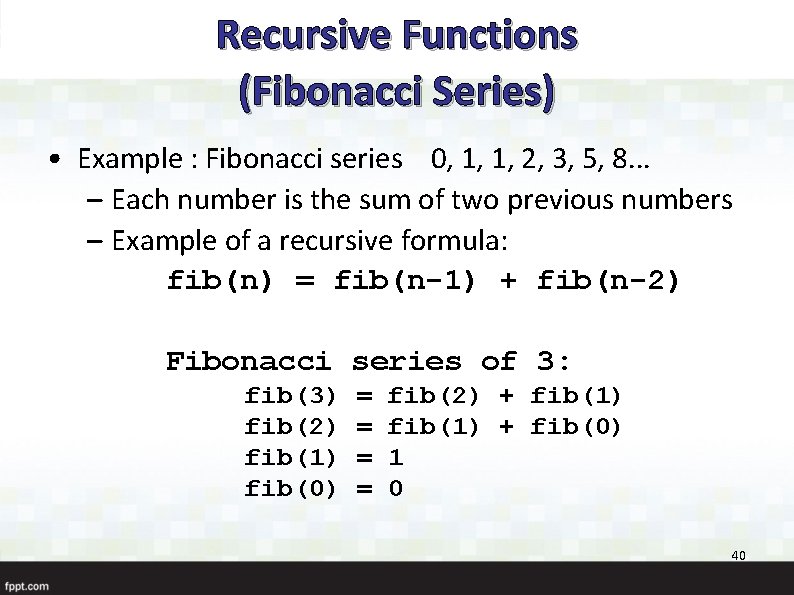
Recursive Functions (Fibonacci Series) • Example : Fibonacci series 0, 1, 1, 2, 3, 5, 8. . . – Each number is the sum of two previous numbers – Example of a recursive formula: fib(n) = fib(n-1) + fib(n-2) Fibonacci series of 3: fib(3) fib(2) fib(1) fib(0) = = fib(2) + fib(1) + fib(0) 1 0 40
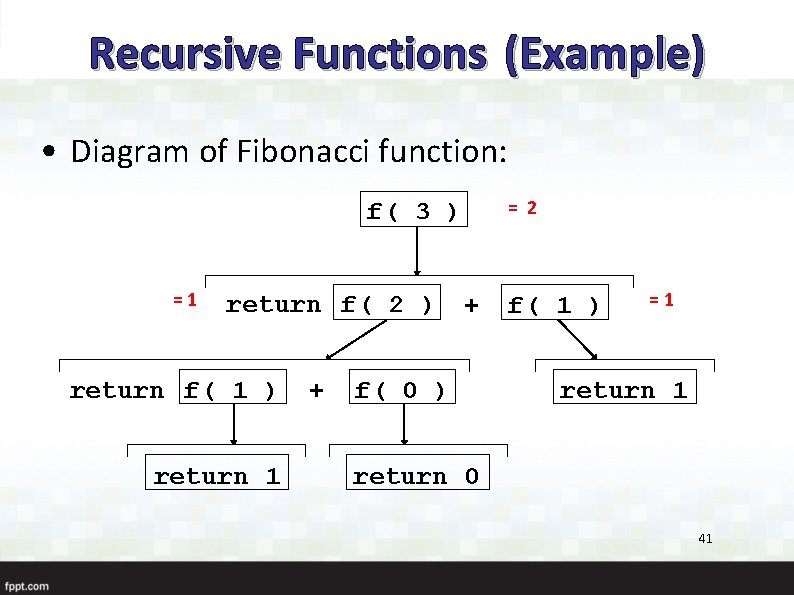
Recursive Functions (Example) • Diagram of Fibonacci function: = 2 f( 3 ) =1 return f( 2 ) return f( 1 ) return 1 + + f( 0 ) f( 1 ) =1 return 0 41
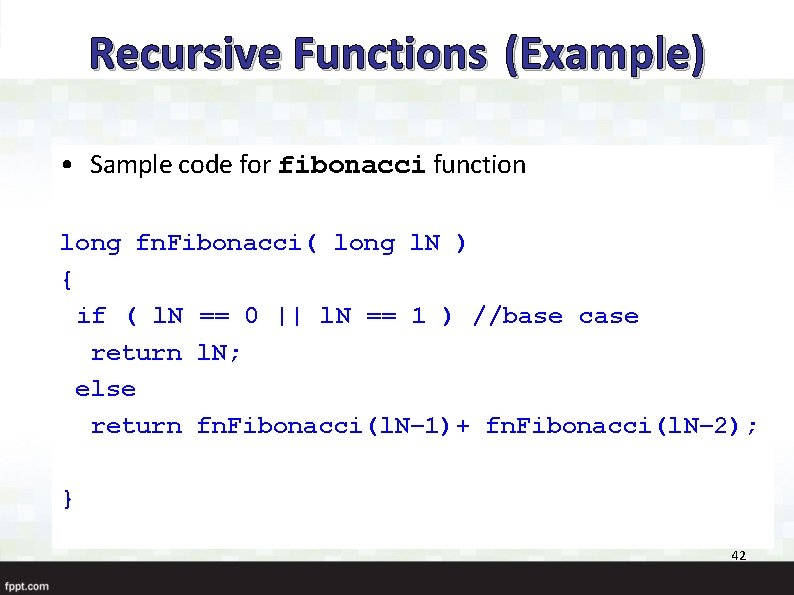
Recursive Functions (Example) • Sample code for fibonacci function long fn. Fibonacci( long l. N ) { if ( l. N == 0 || l. N == 1 ) //base case return l. N; else return fn. Fibonacci(l. N– 1)+ fn. Fibonacci(l. N– 2); } 42
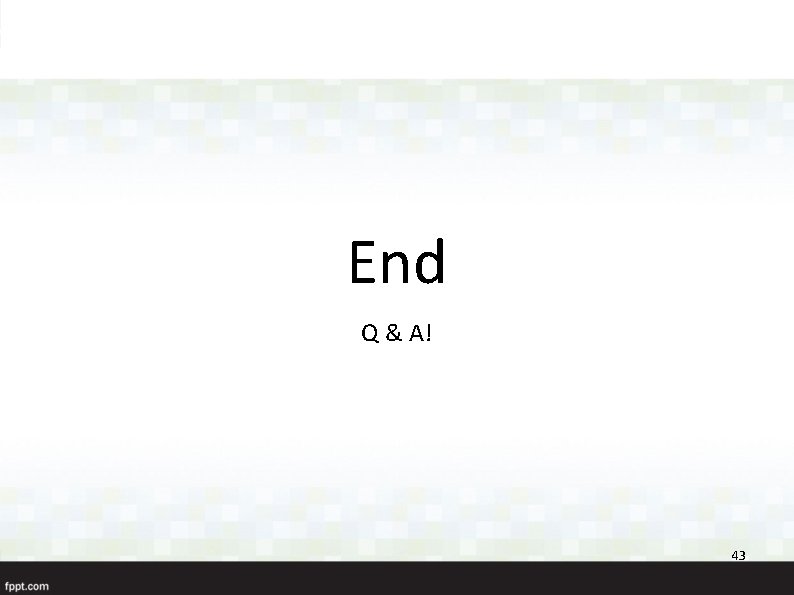
End Q & A! 43