EECS 183 Discussion 5 Katherine Kampf kkampfumich edu
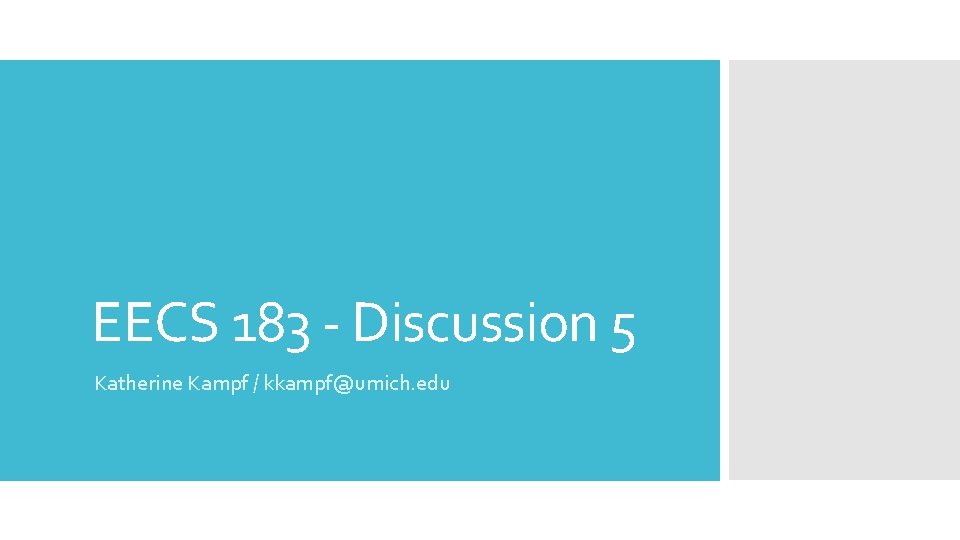
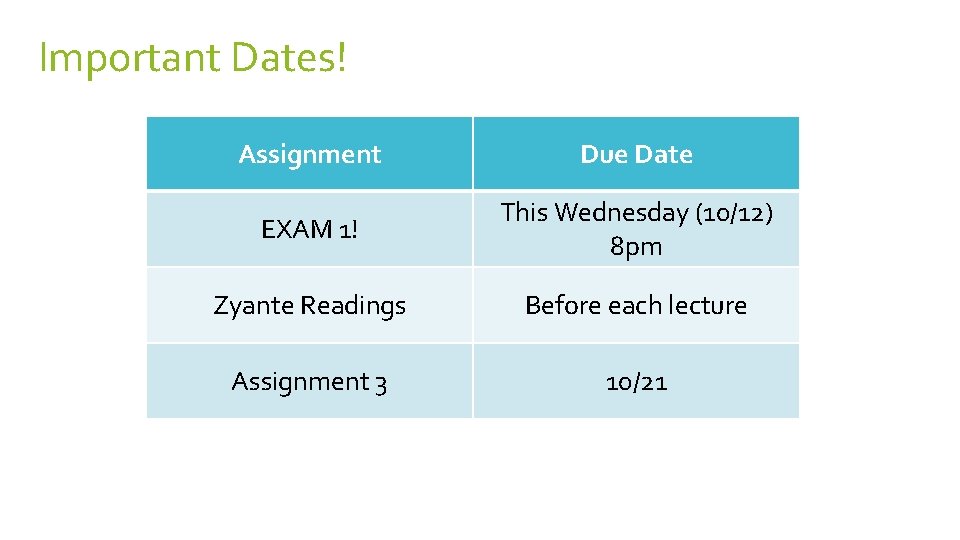
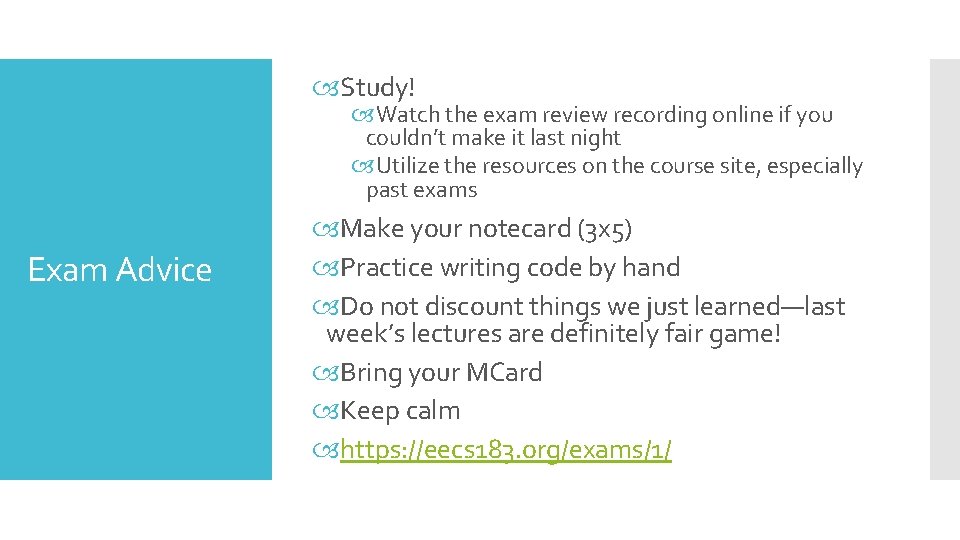
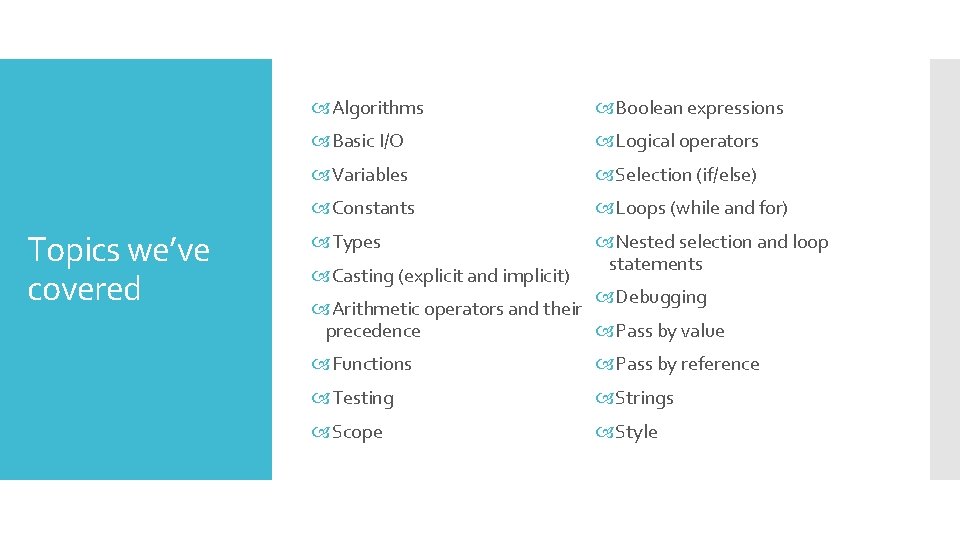
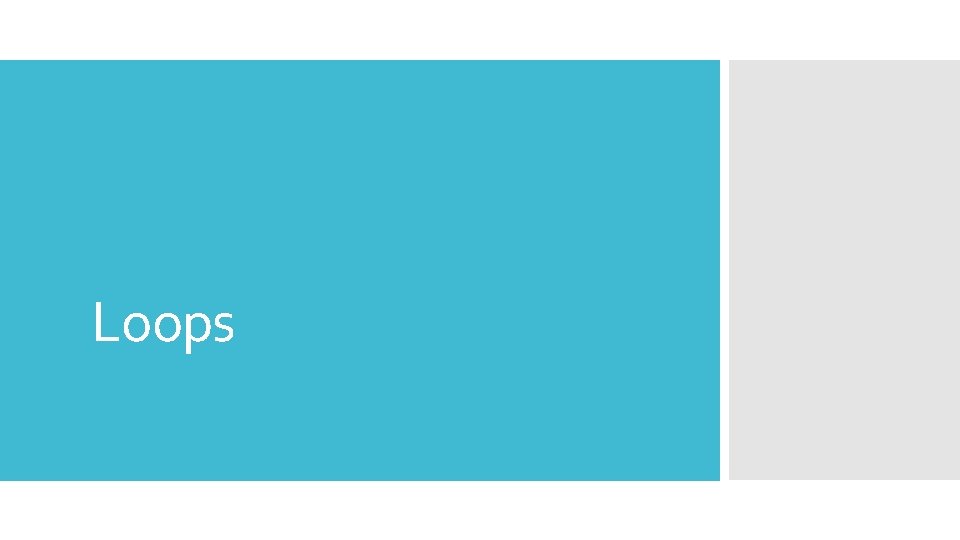
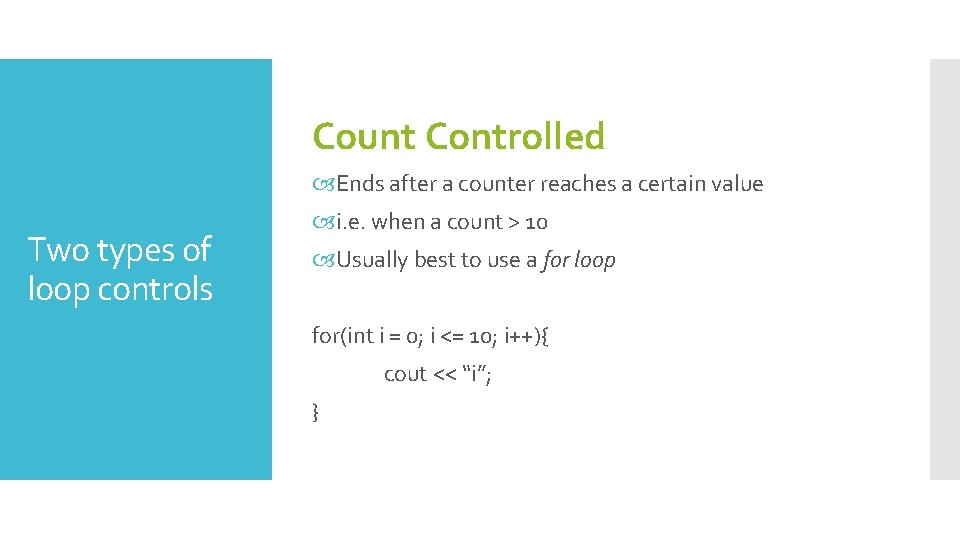
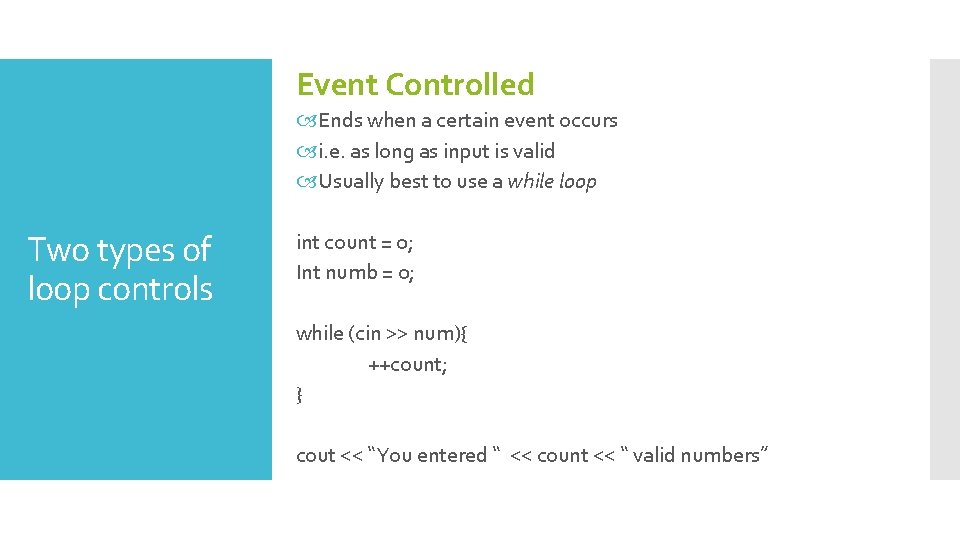
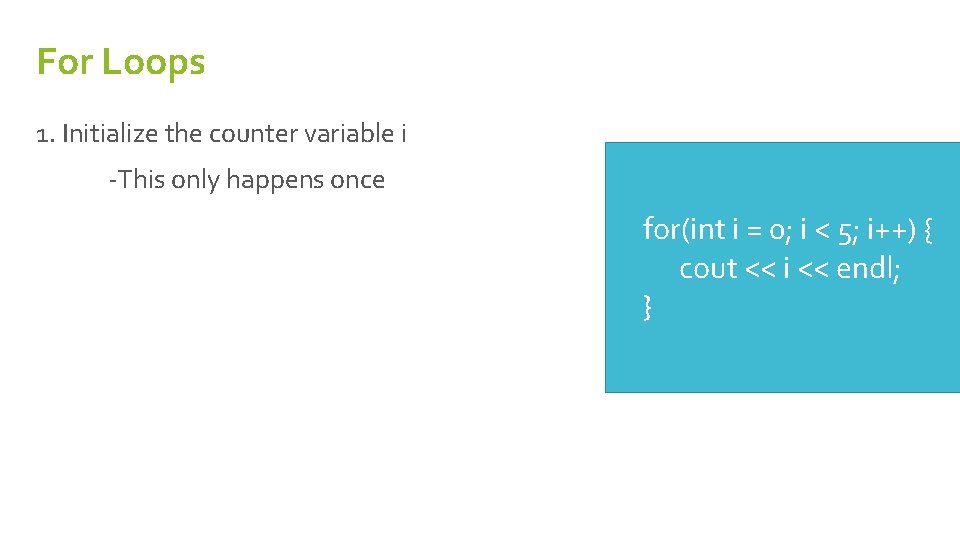
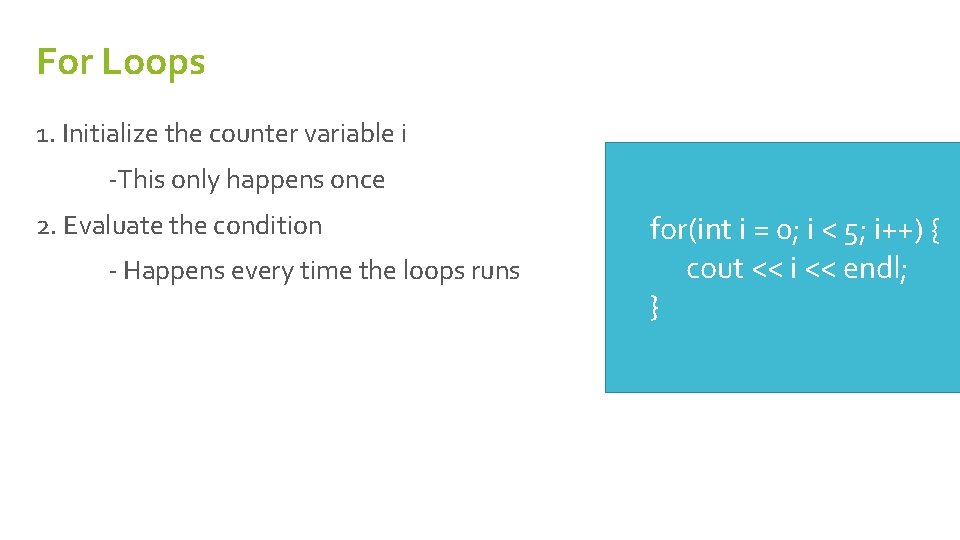
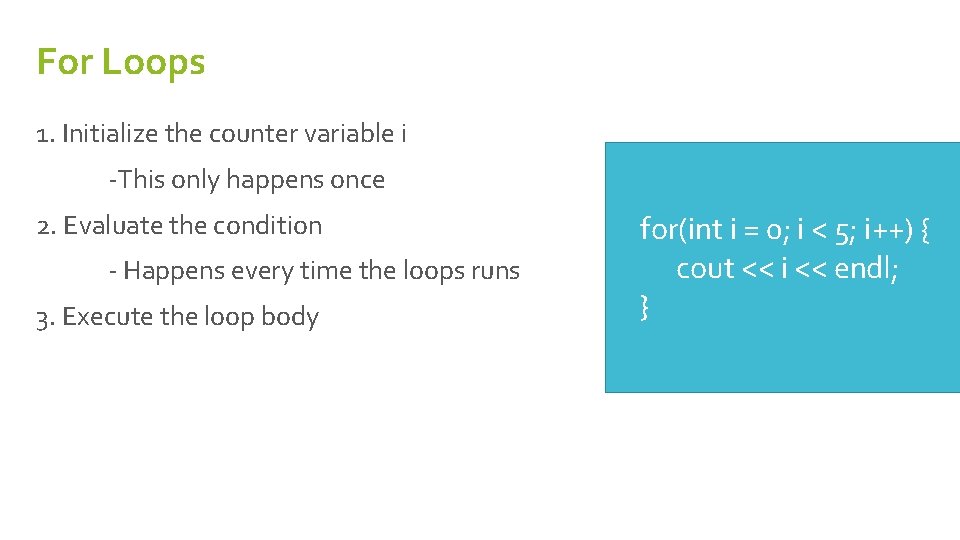
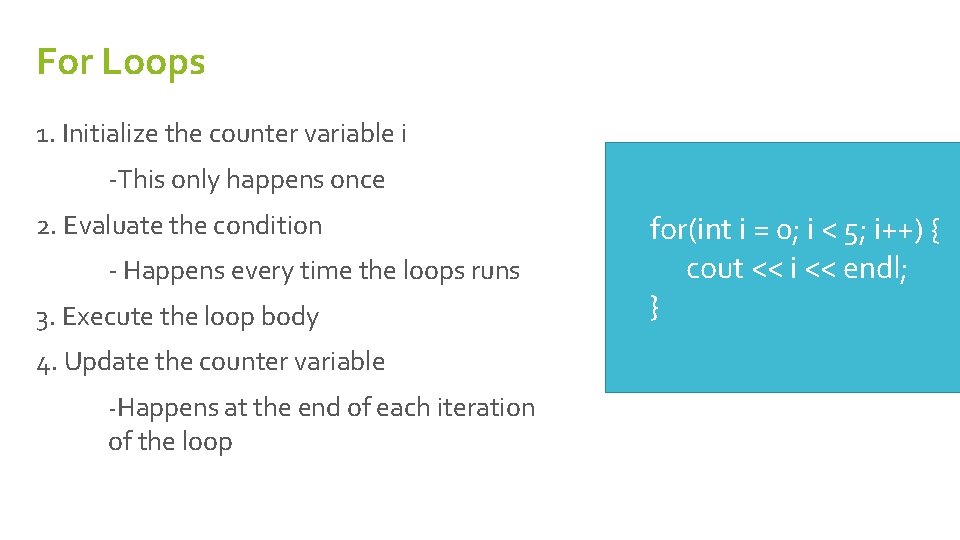
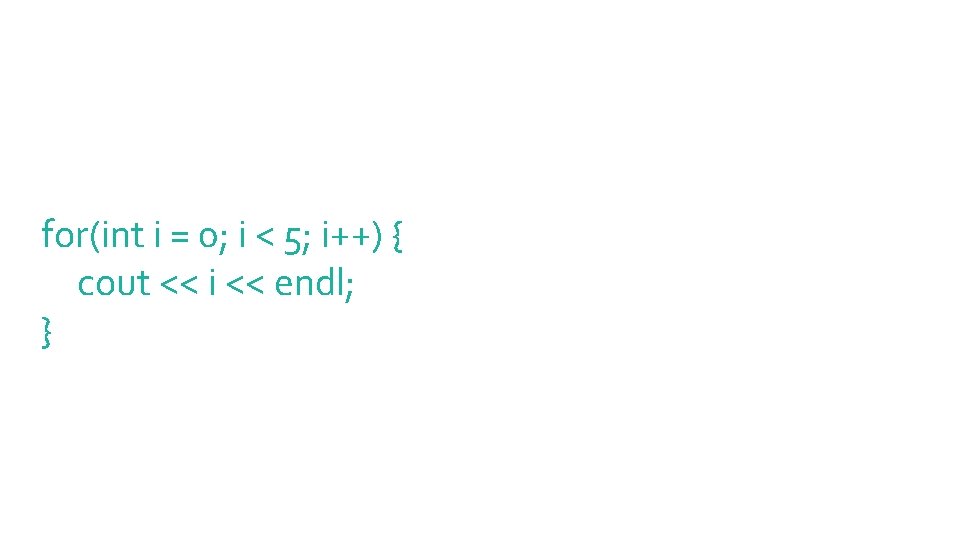
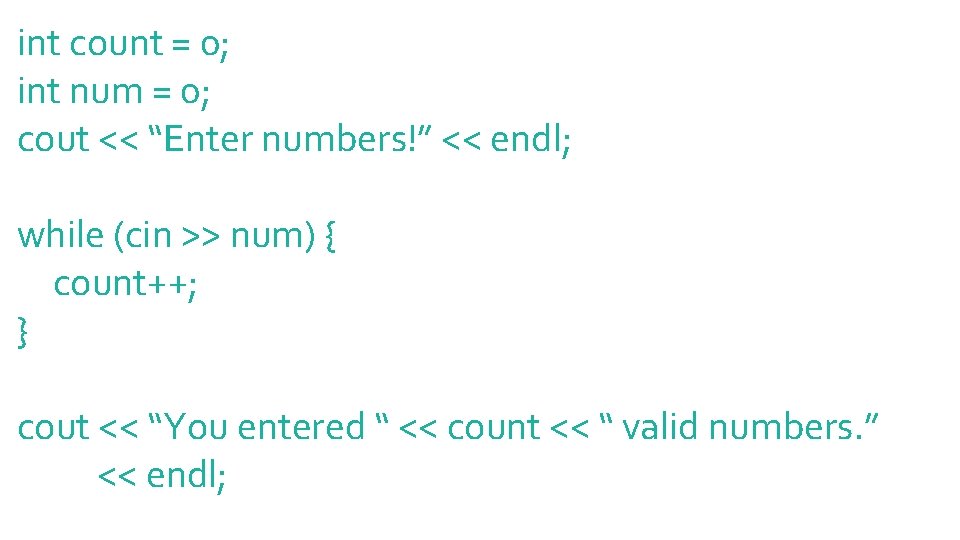
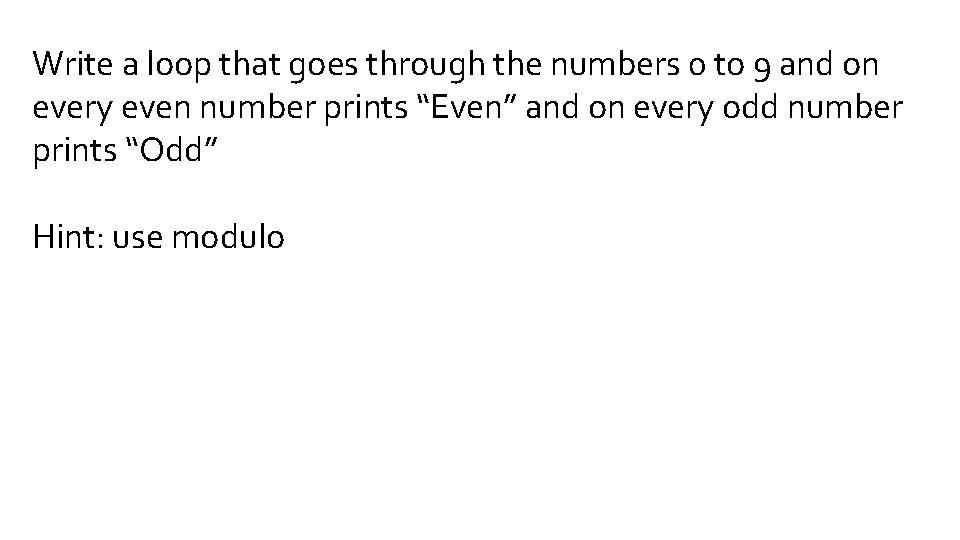
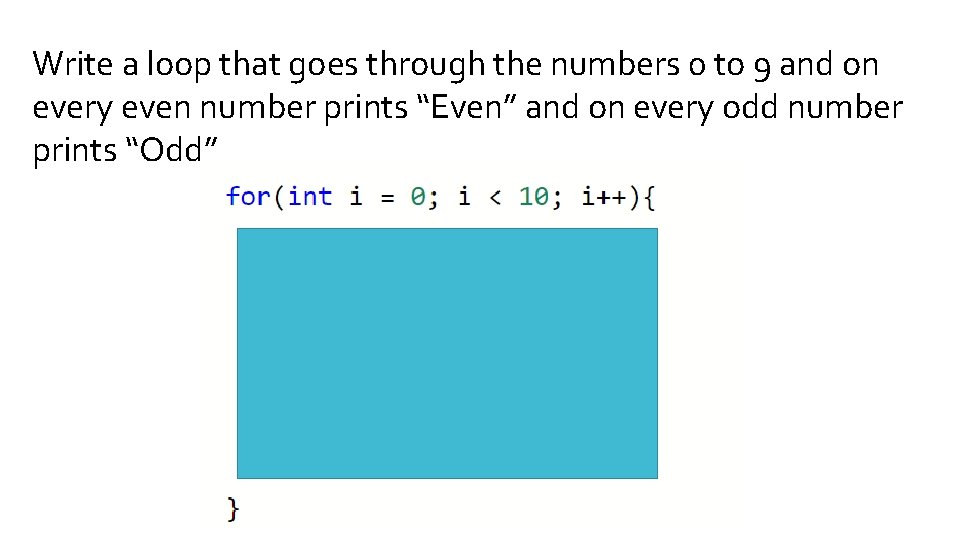
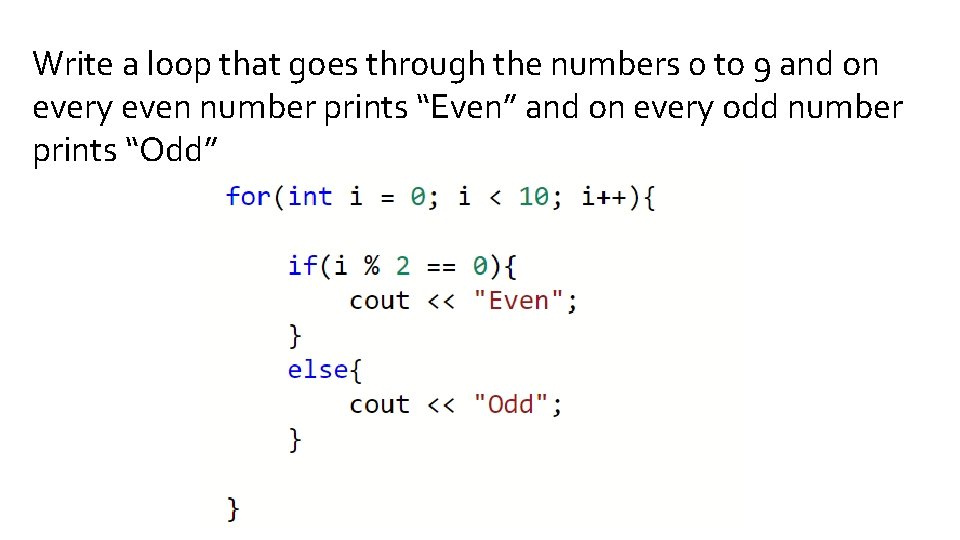
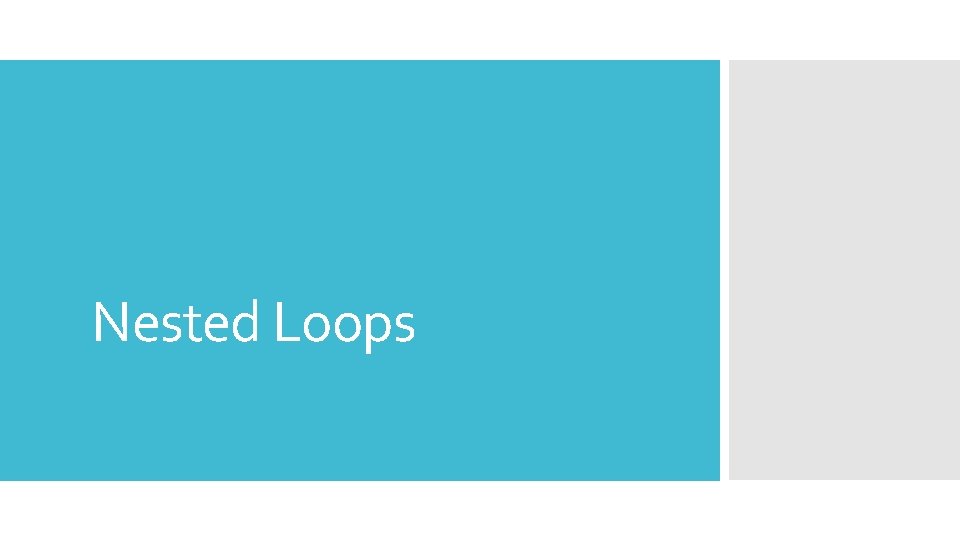
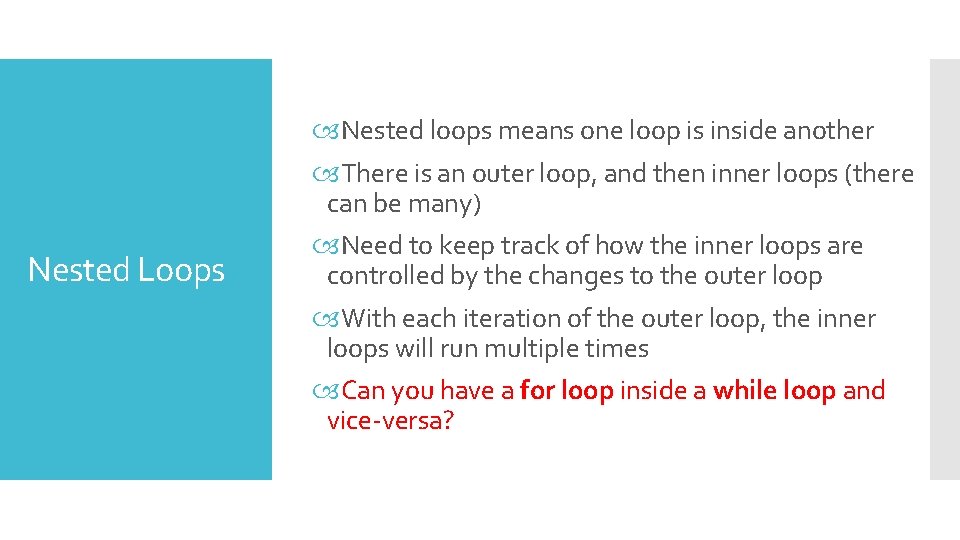
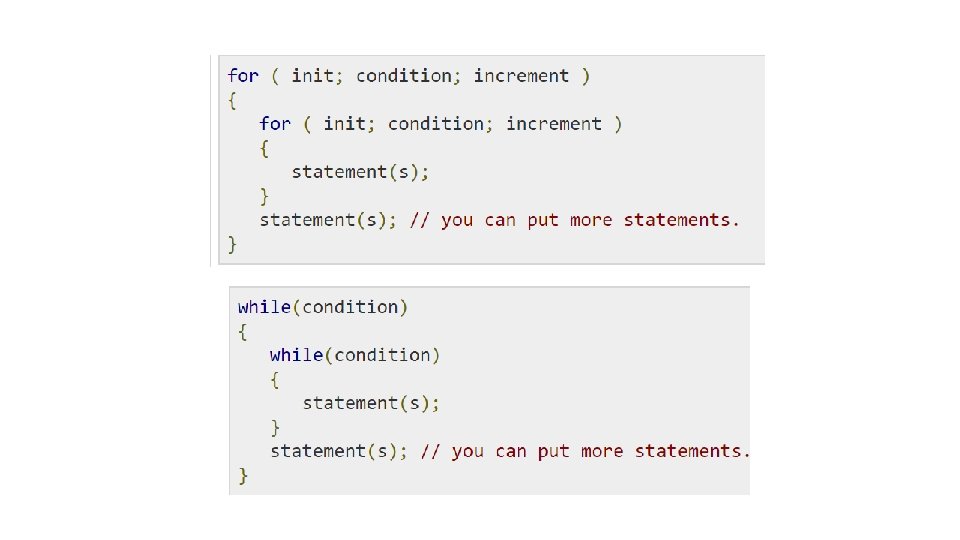
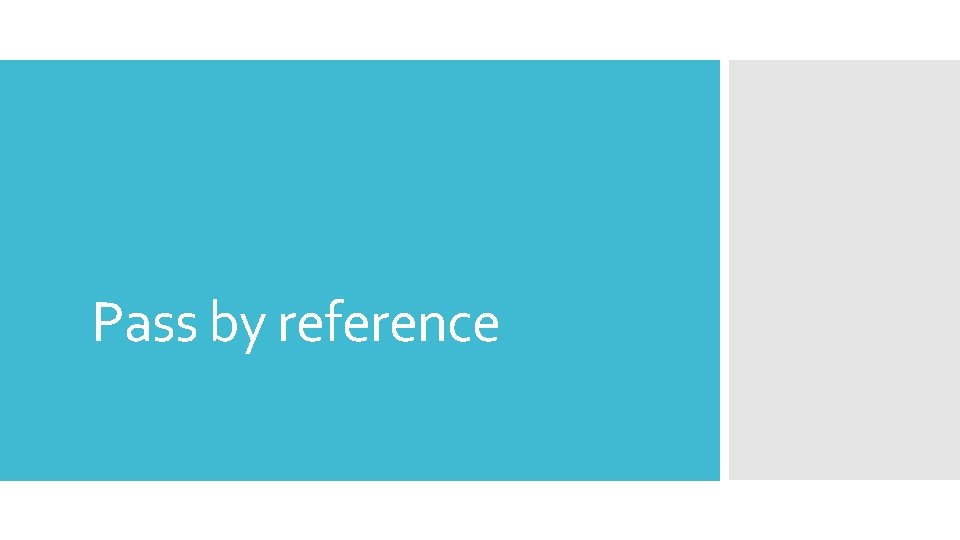
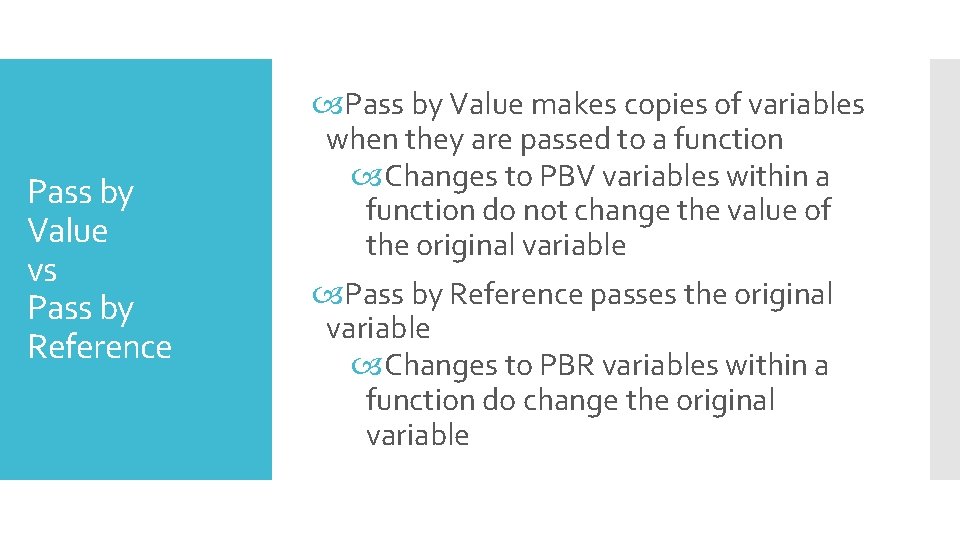
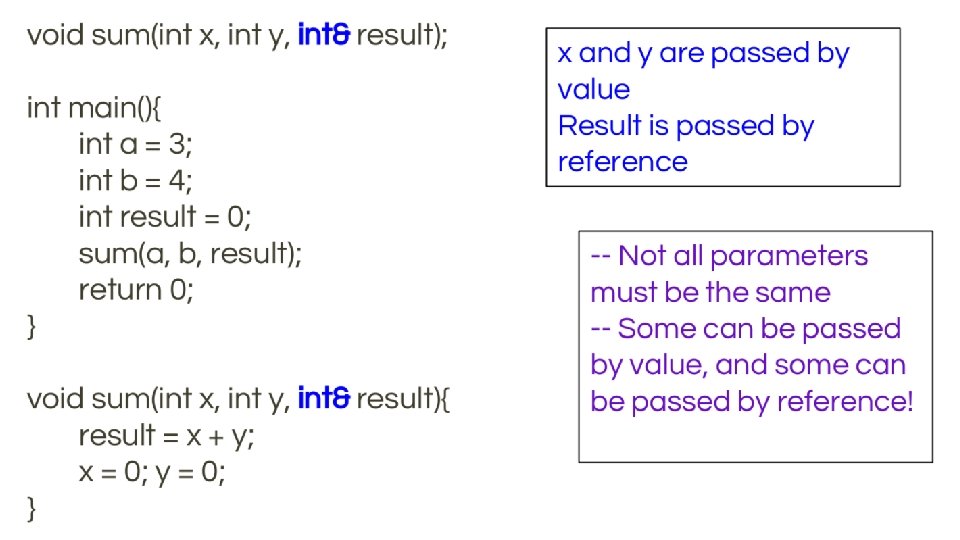
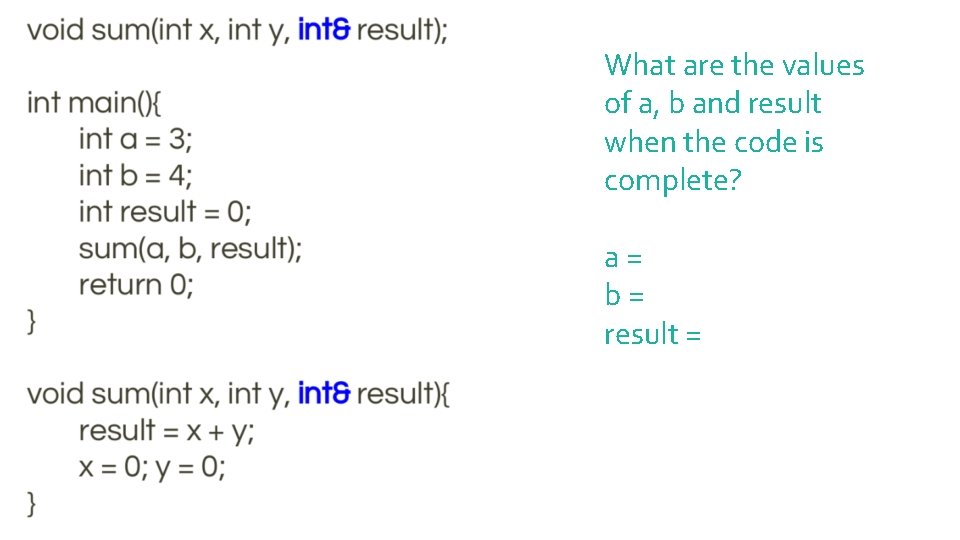
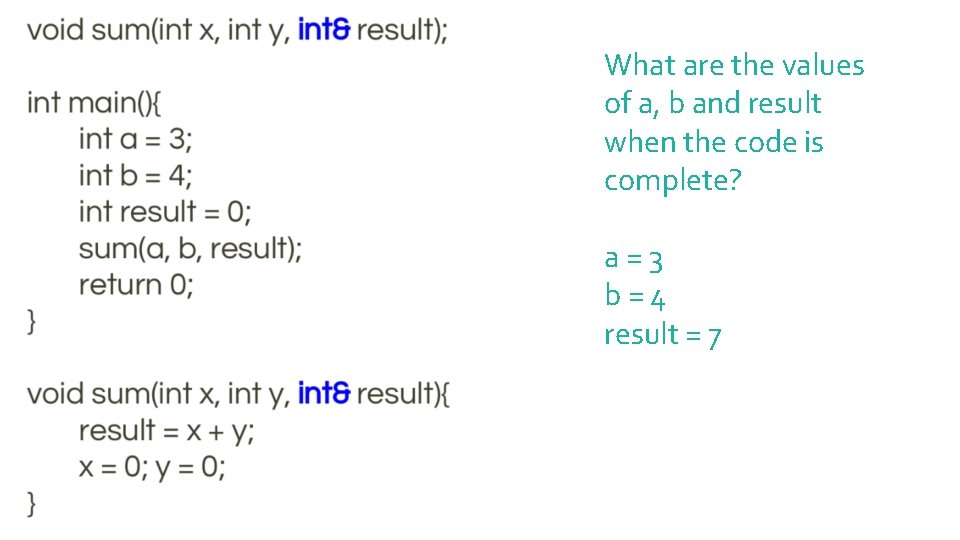
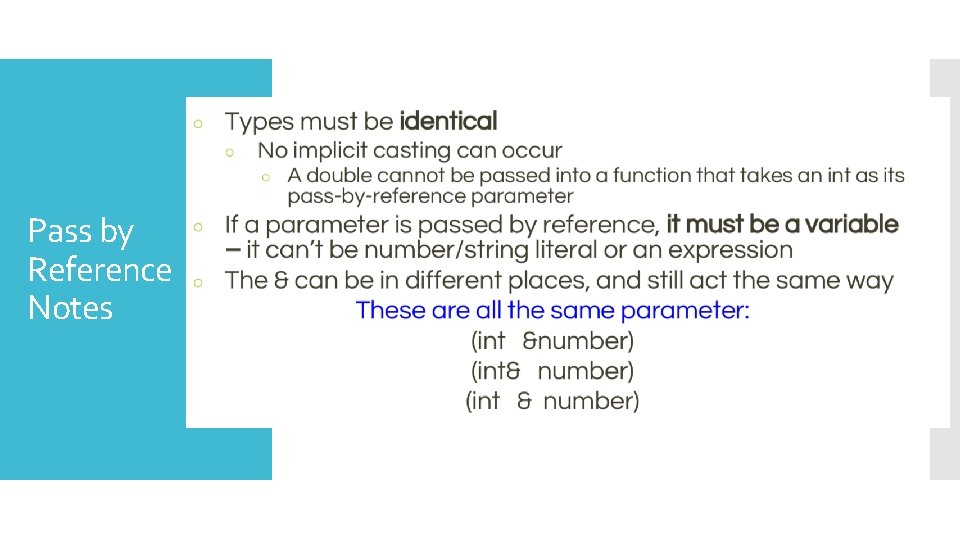
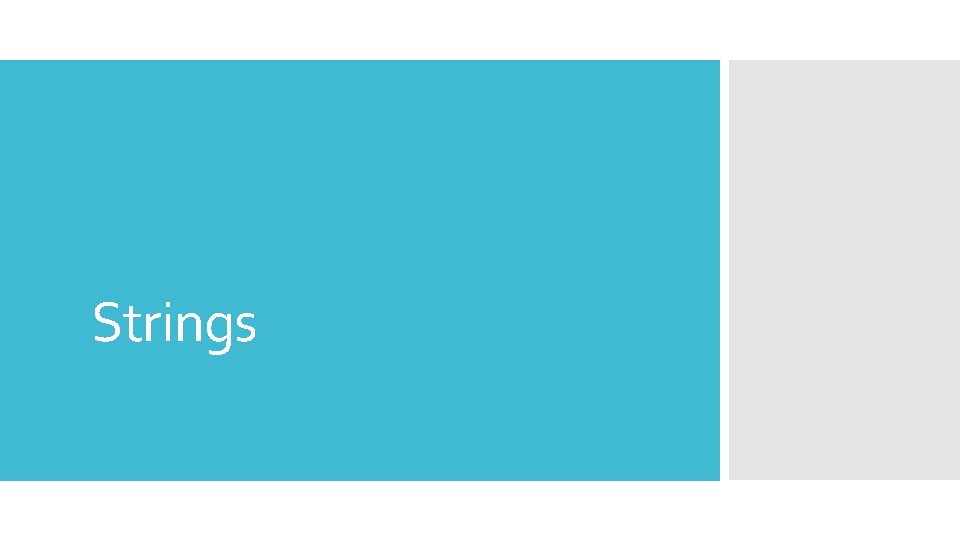
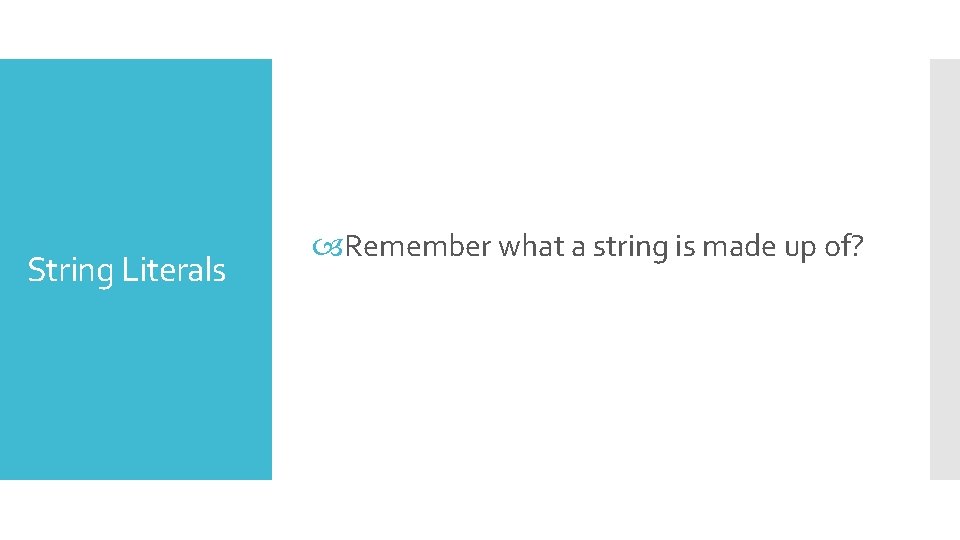
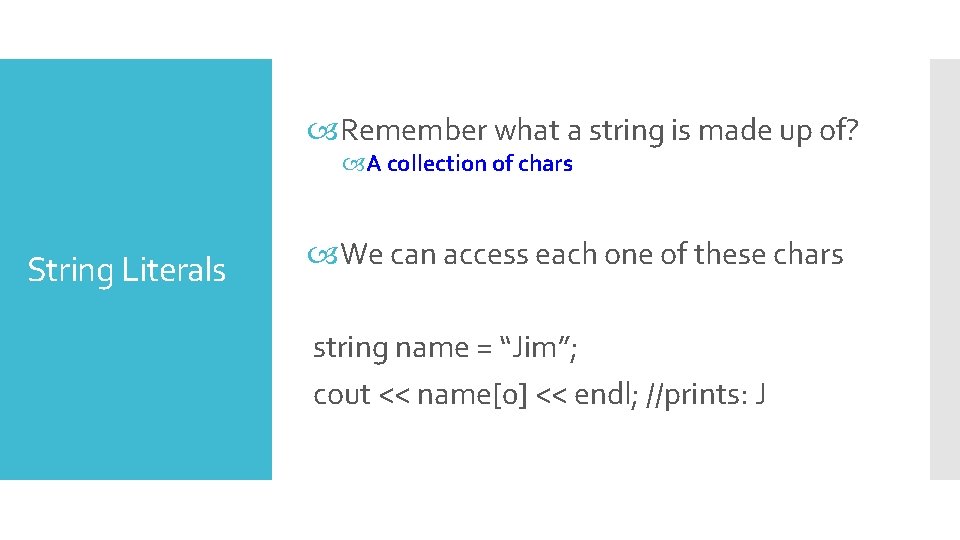
![string name = “Jim”; cout << name[0] << endl; //prints: J Accessing characters of string name = “Jim”; cout << name[0] << endl; //prints: J Accessing characters of](https://slidetodoc.com/presentation_image/6731cfdf865083436f56939d5634a18c/image-29.jpg)
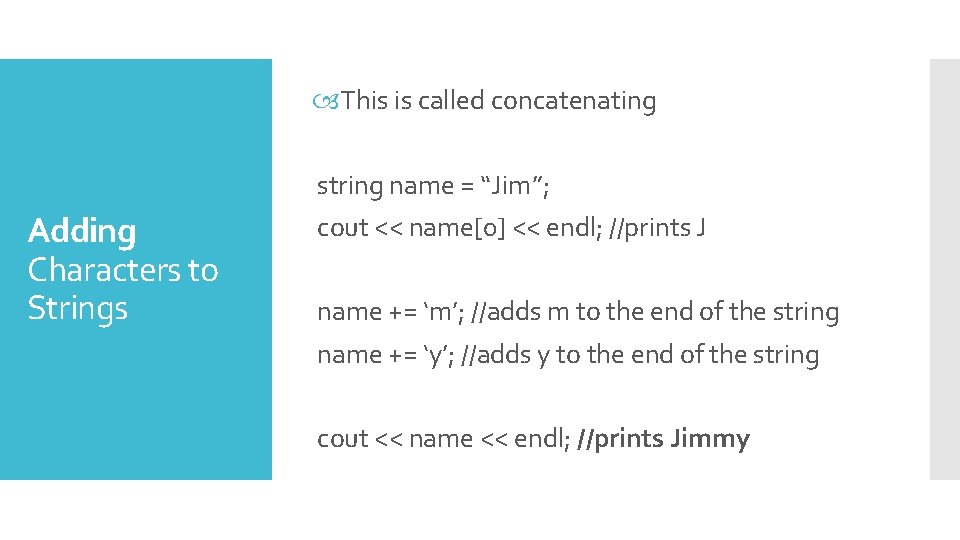
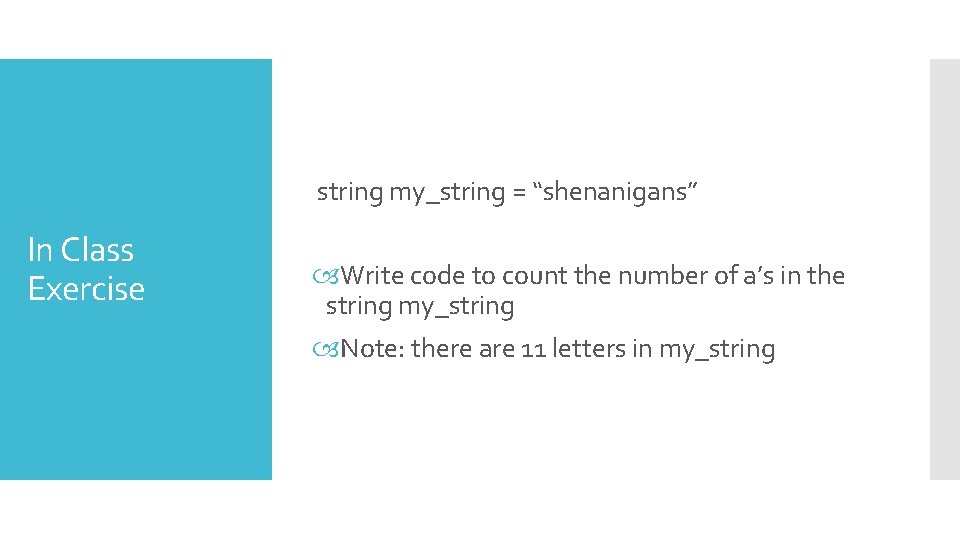
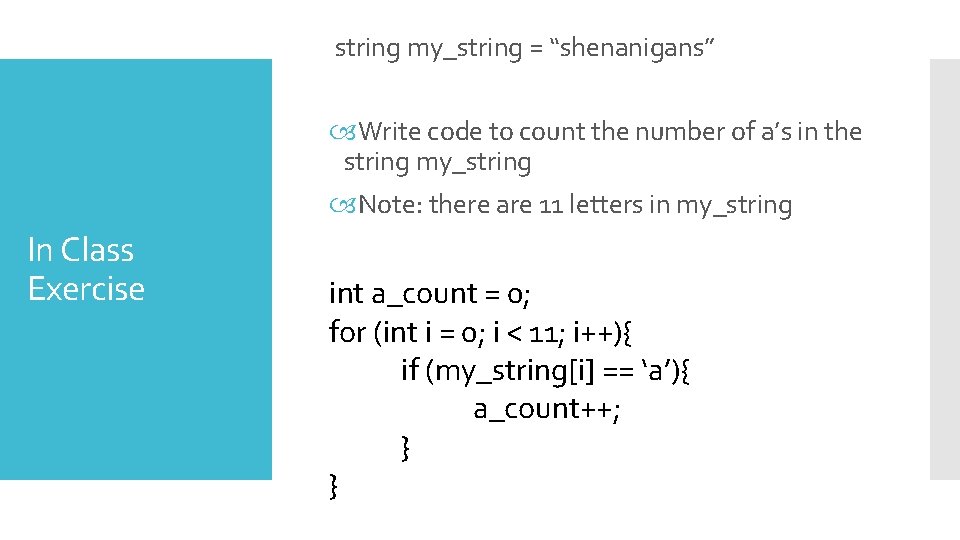
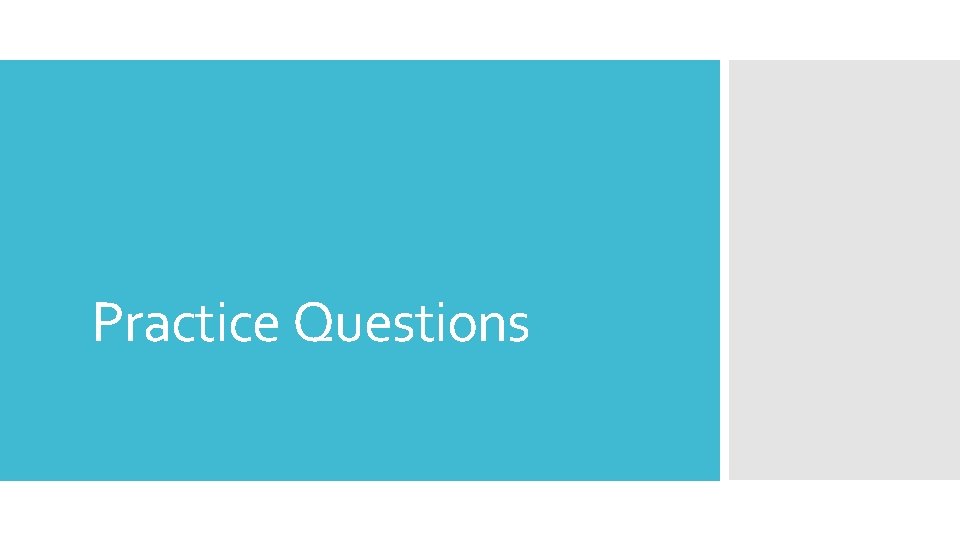
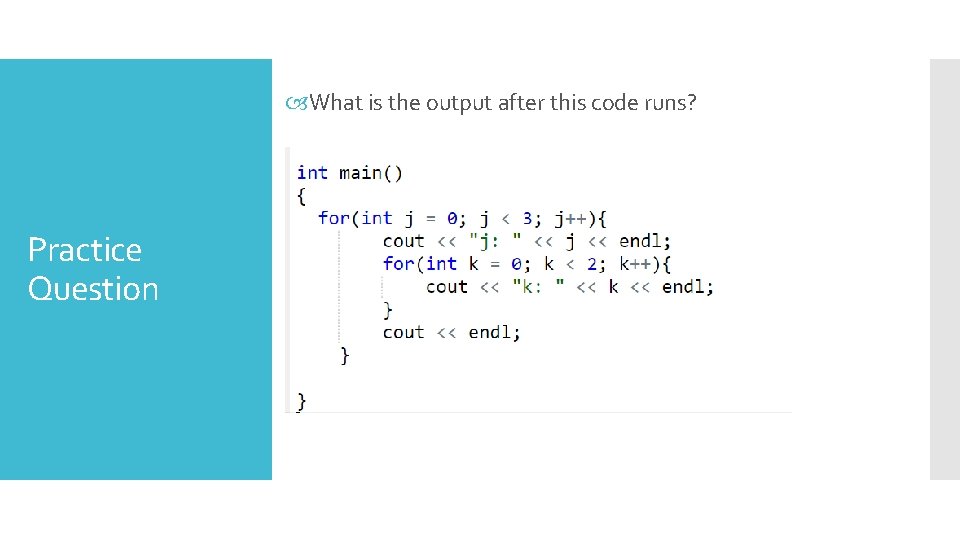
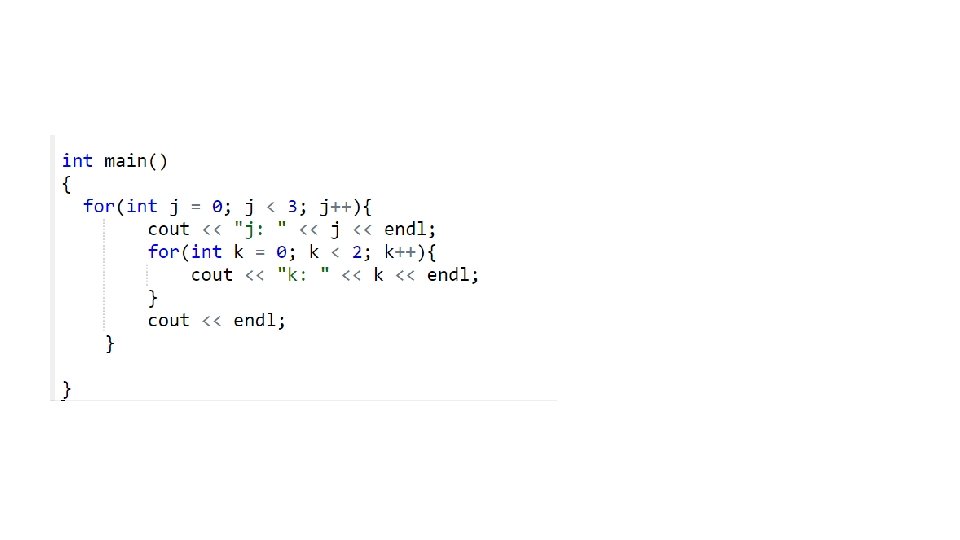
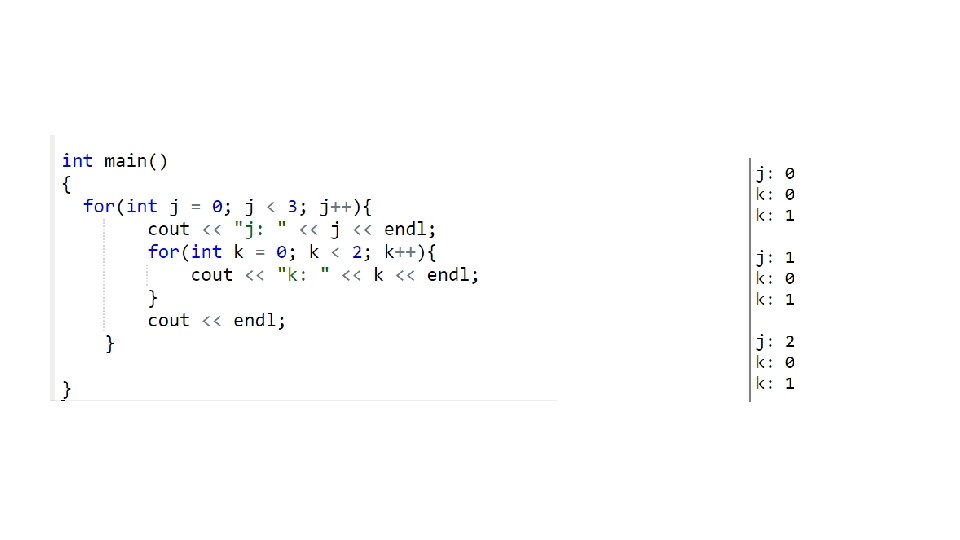
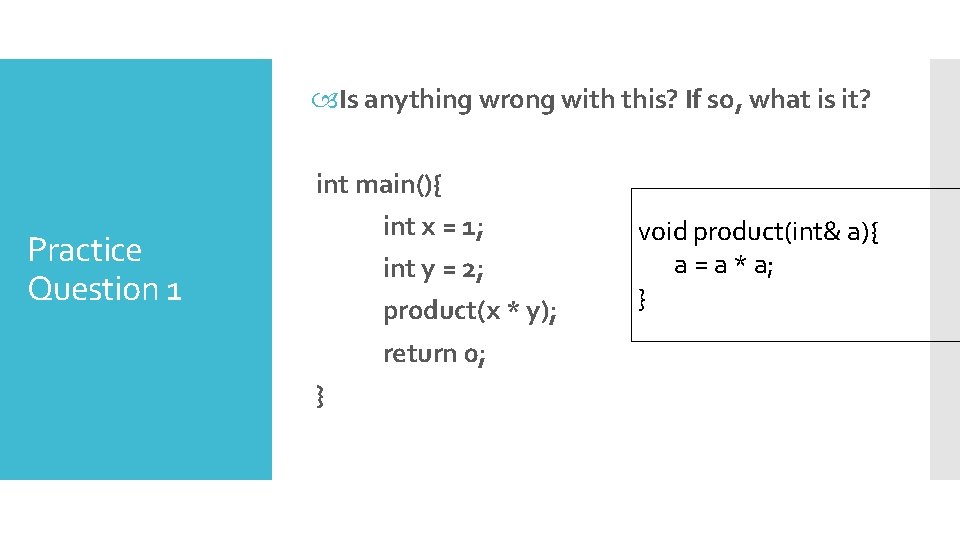
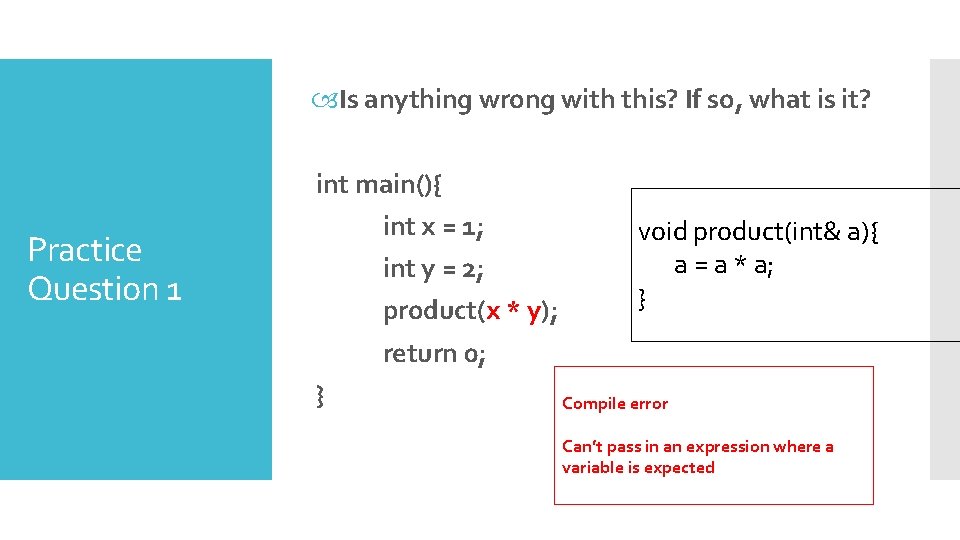
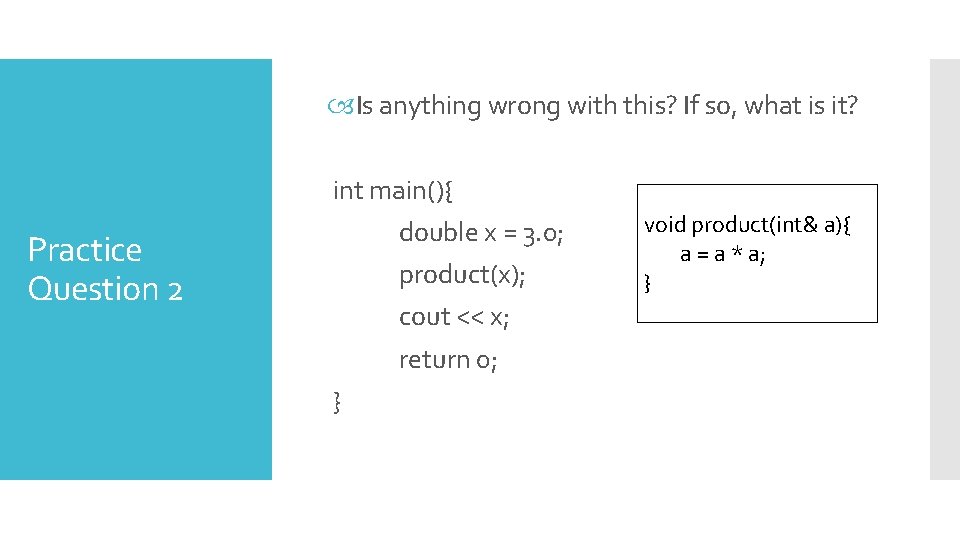
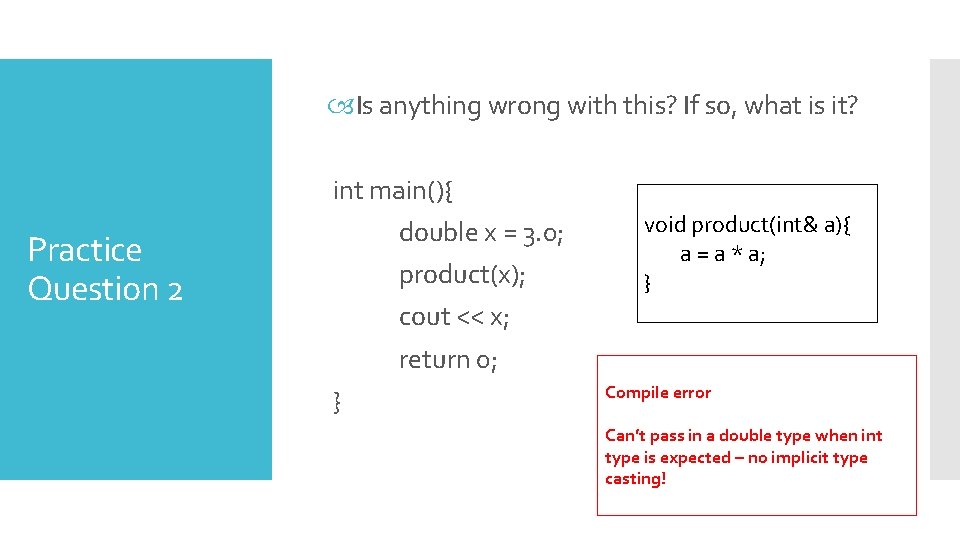
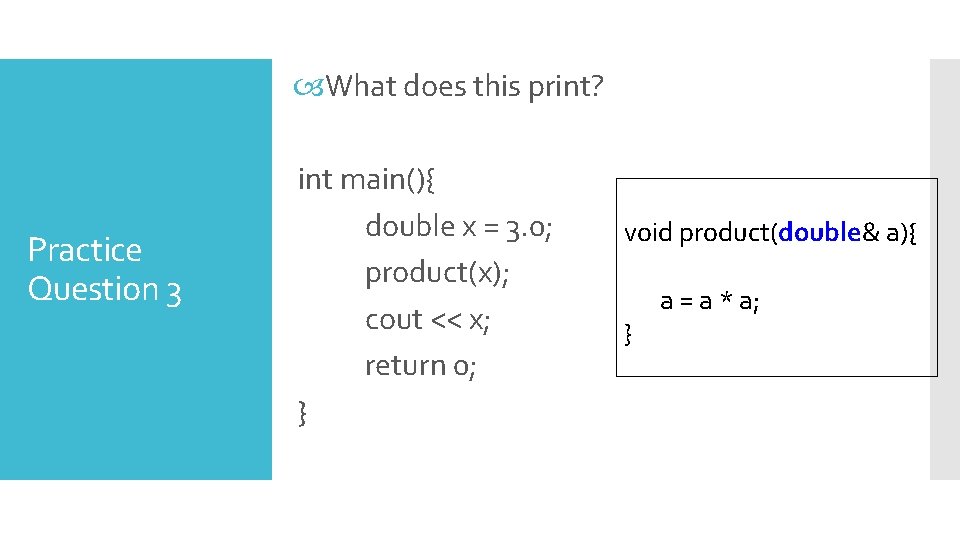
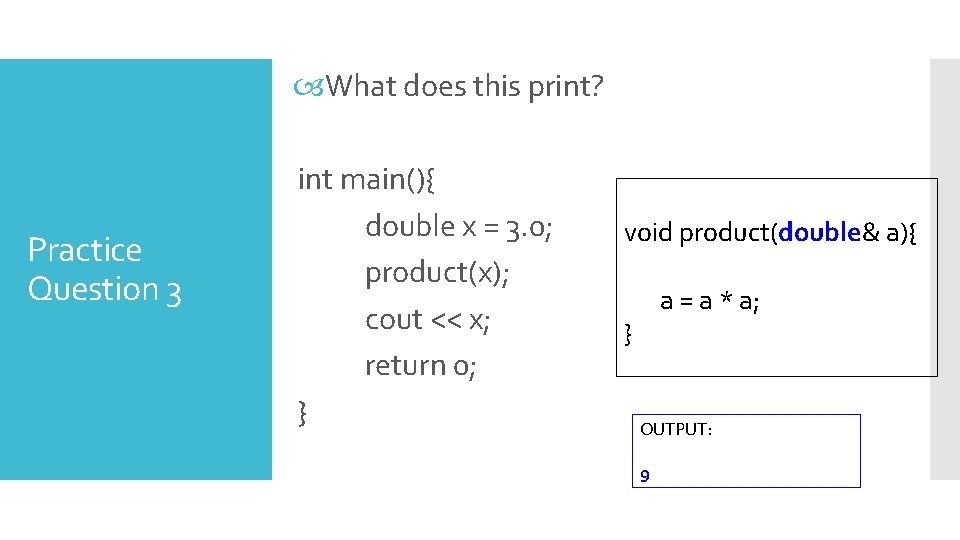
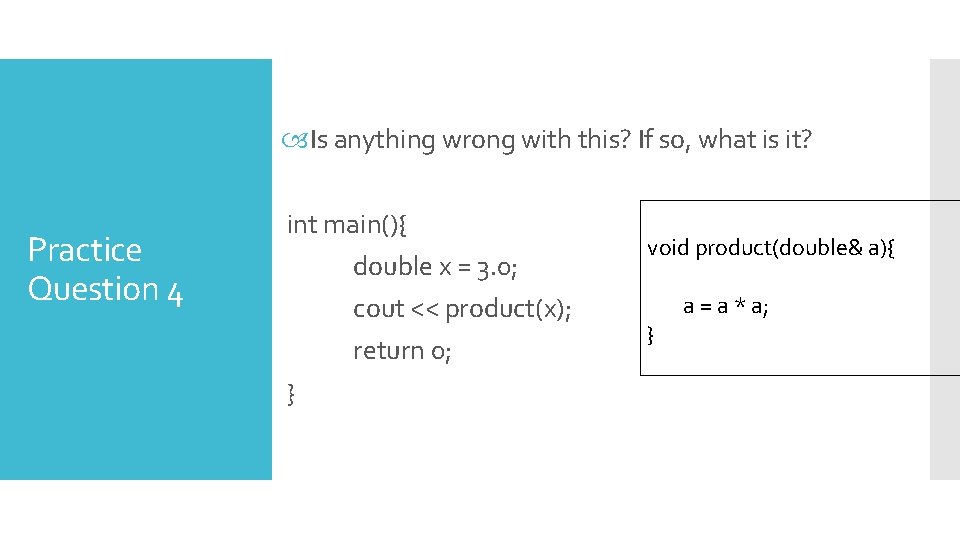
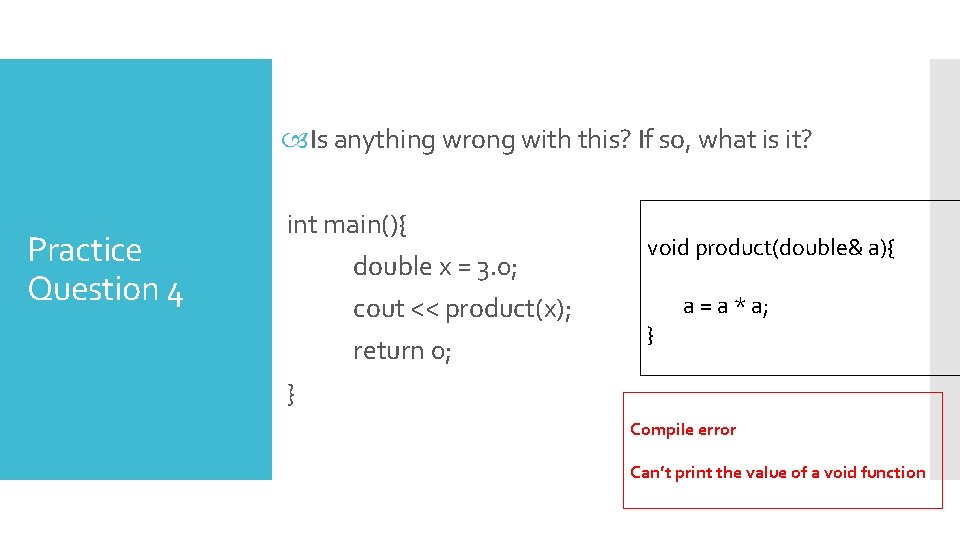
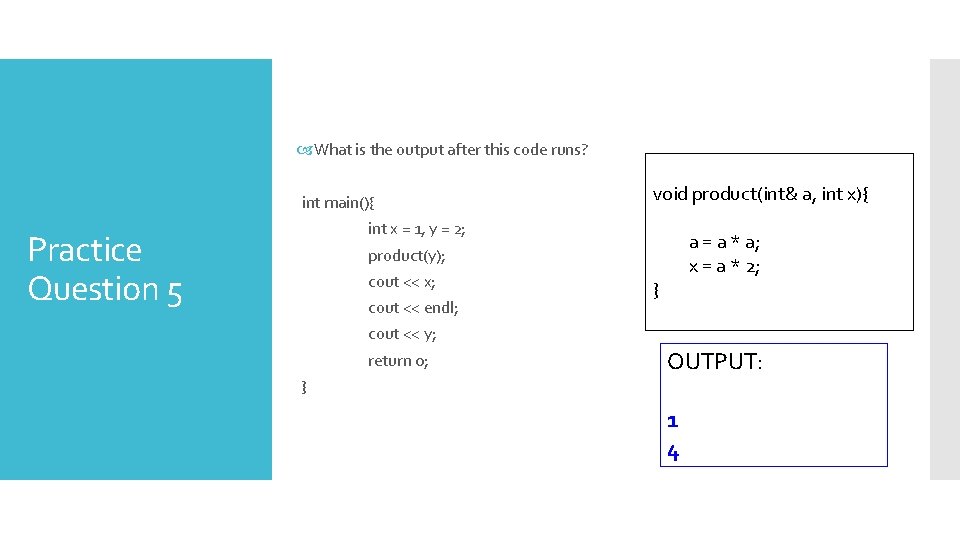
- Slides: 45
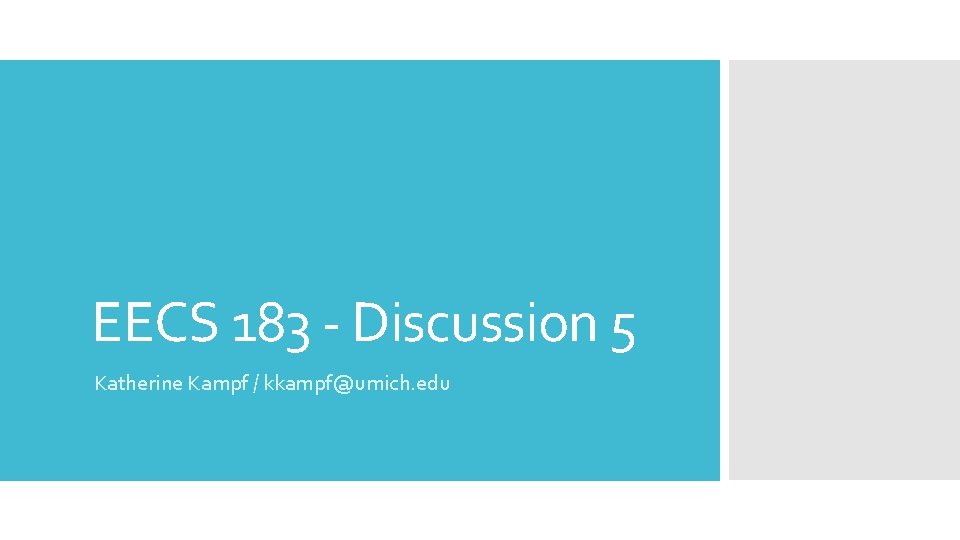
EECS 183 - Discussion 5 Katherine Kampf / kkampf@umich. edu
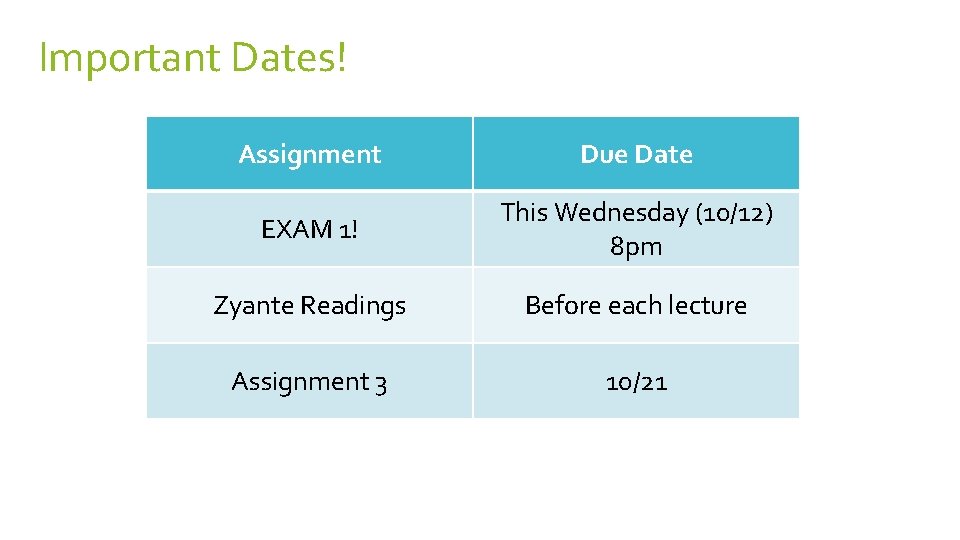
Important Dates! Assignment Due Date EXAM 1! This Wednesday (10/12) 8 pm Zyante Readings Before each lecture Assignment 3 10/21
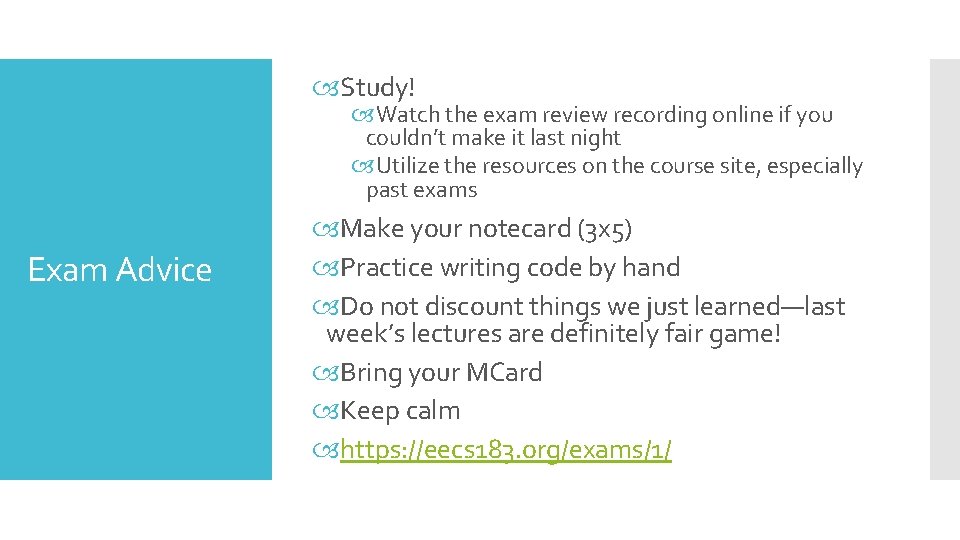
Study! Watch the exam review recording online if you couldn’t make it last night Utilize the resources on the course site, especially past exams Exam Advice Make your notecard (3 x 5) Practice writing code by hand Do not discount things we just learned—last week’s lectures are definitely fair game! Bring your MCard Keep calm https: //eecs 183. org/exams/1/
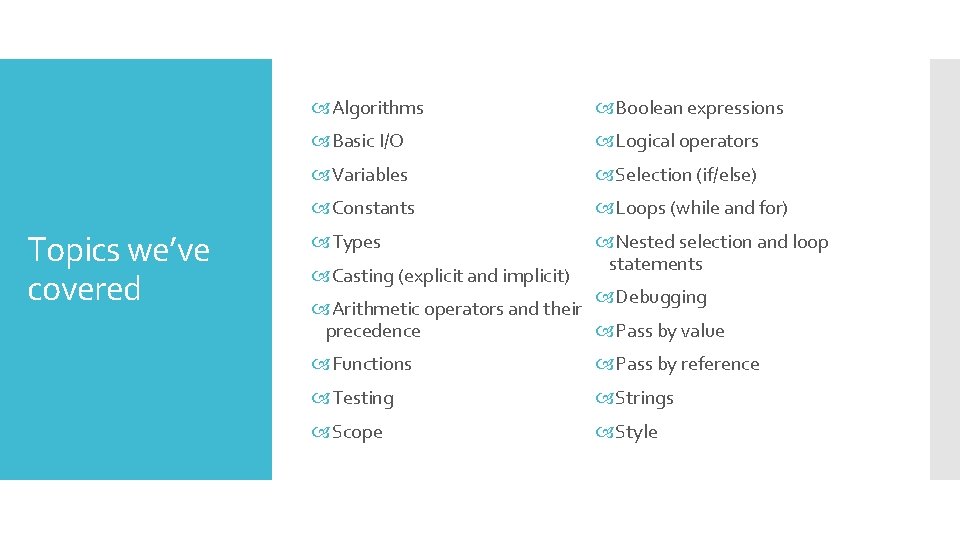
Topics we’ve covered Algorithms Boolean expressions Basic I/O Logical operators Variables Selection (if/else) Constants Loops (while and for) Types Nested selection and loop statements Casting (explicit and implicit) Debugging Arithmetic operators and their precedence Pass by value Functions Pass by reference Testing Strings Scope Style
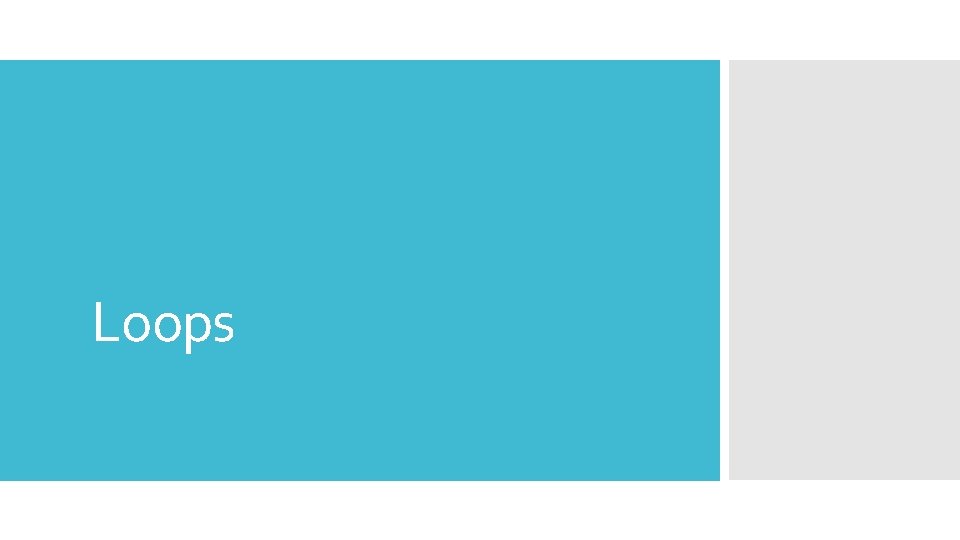
Loops
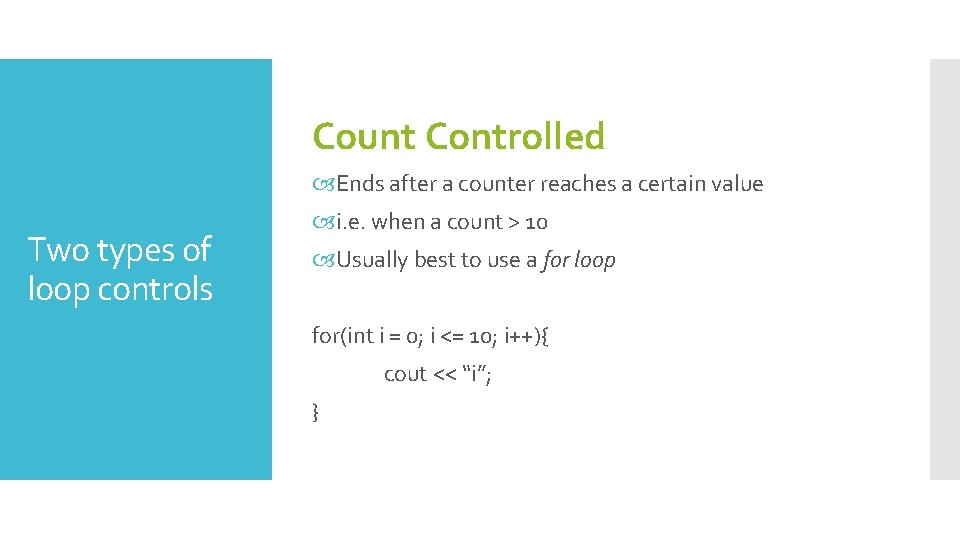
Count Controlled Ends after a counter reaches a certain value Two types of loop controls i. e. when a count > 10 Usually best to use a for loop for(int i = 0; i <= 10; i++){ cout << “i”; }
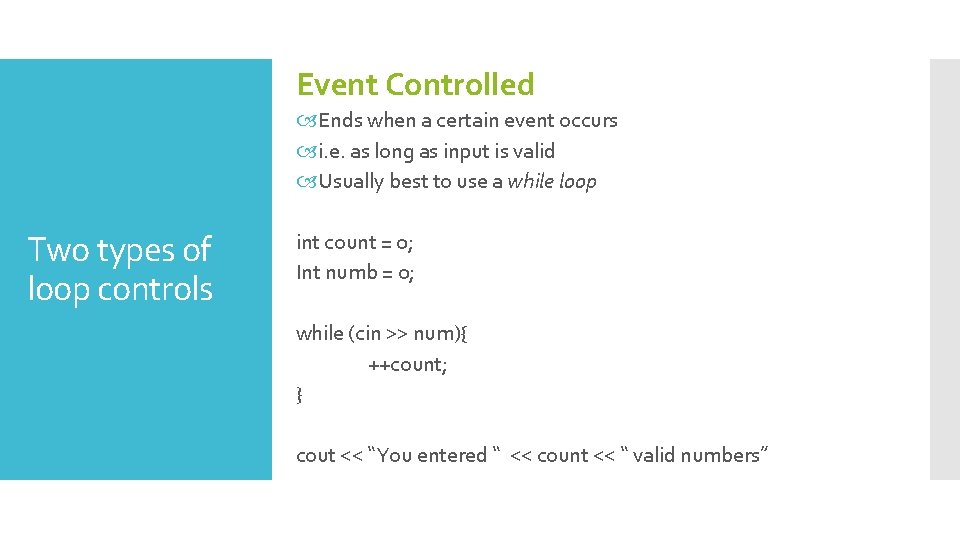
Event Controlled Ends when a certain event occurs i. e. as long as input is valid Usually best to use a while loop Two types of loop controls int count = 0; Int numb = 0; while (cin >> num){ ++count; } cout << “You entered “ << count << “ valid numbers”
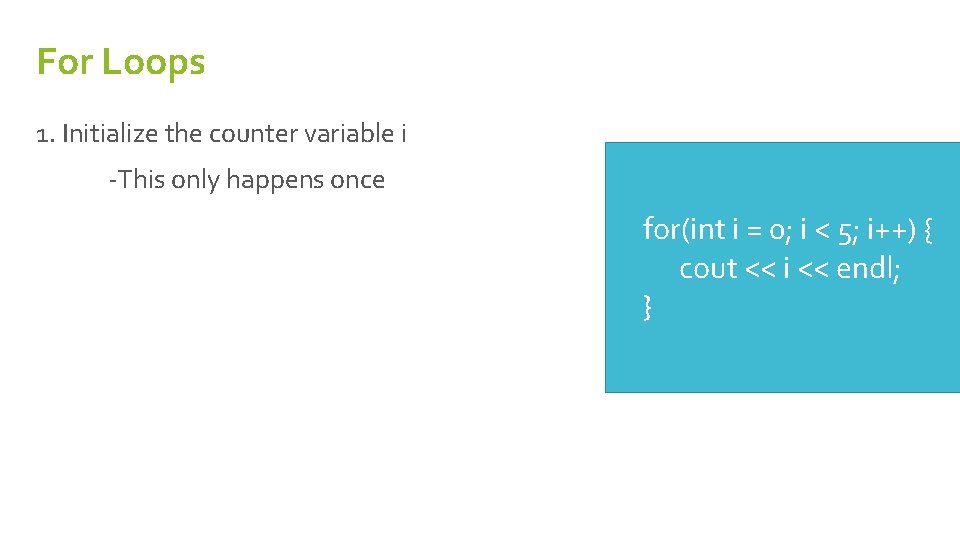
For Loops 1. Initialize the counter variable i -This only happens once for(int i = 0; i < 5; i++) { cout << i << endl; }
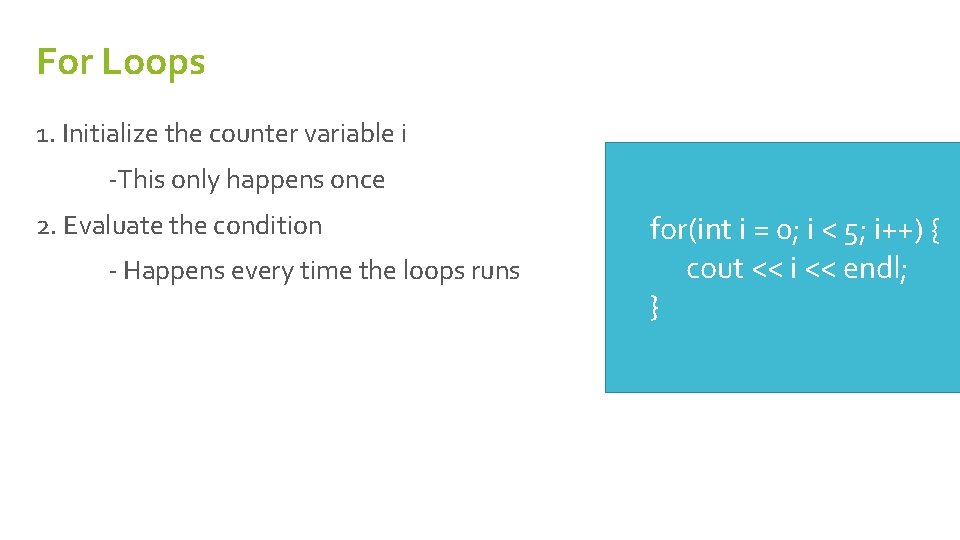
For Loops 1. Initialize the counter variable i -This only happens once 2. Evaluate the condition - Happens every time the loops runs for(int i = 0; i < 5; i++) { cout << i << endl; }
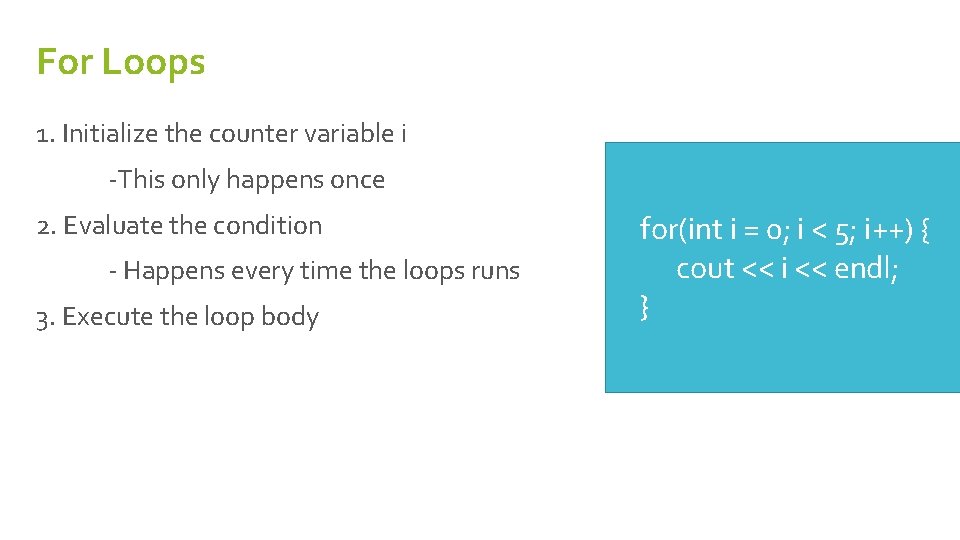
For Loops 1. Initialize the counter variable i -This only happens once 2. Evaluate the condition - Happens every time the loops runs 3. Execute the loop body for(int i = 0; i < 5; i++) { cout << i << endl; }
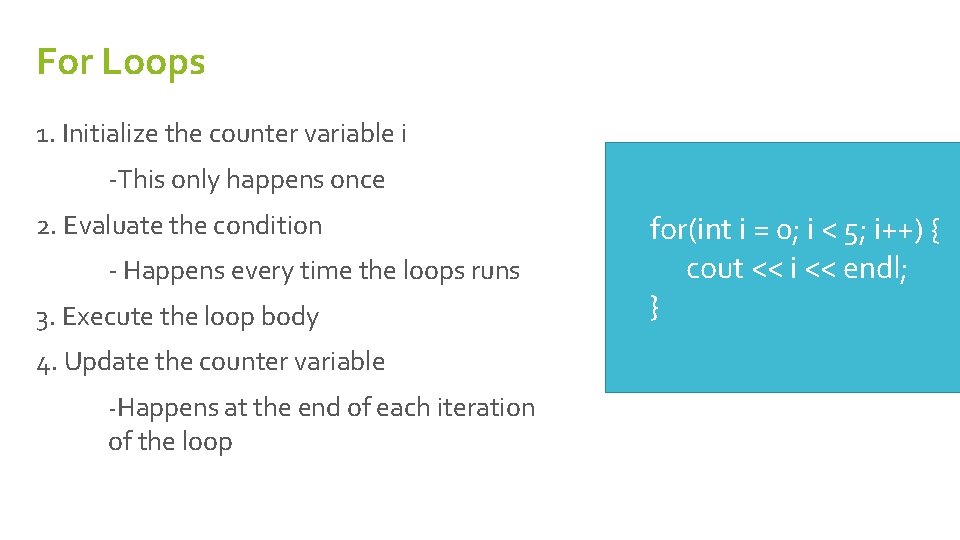
For Loops 1. Initialize the counter variable i -This only happens once 2. Evaluate the condition - Happens every time the loops runs 3. Execute the loop body 4. Update the counter variable -Happens at the end of each iteration of the loop for(int i = 0; i < 5; i++) { cout << i << endl; }
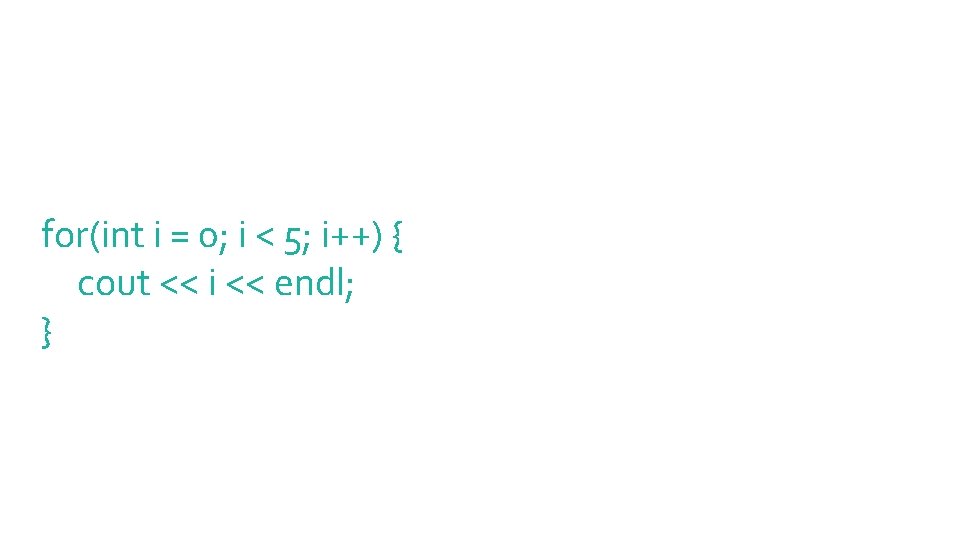
for(int i = 0; i < 5; i++) { cout << i << endl; }
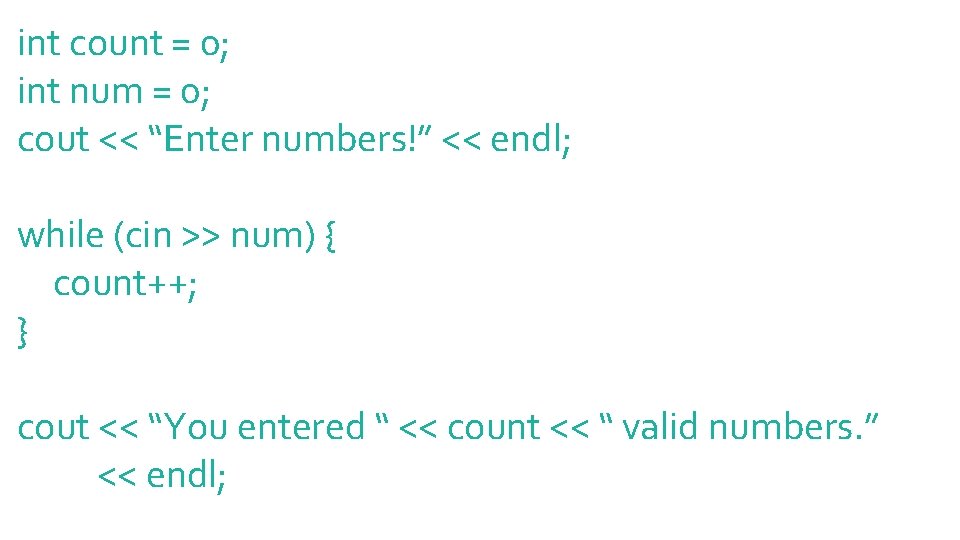
int count = 0; int num = 0; cout << “Enter numbers!” << endl; while (cin >> num) { count++; } cout << “You entered “ << count << “ valid numbers. ” << endl;
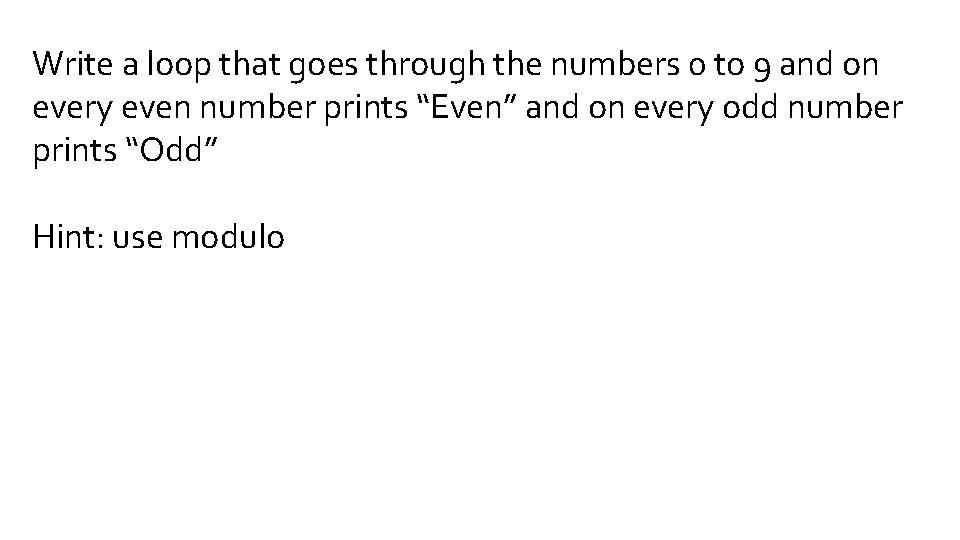
Write a loop that goes through the numbers 0 to 9 and on every even number prints “Even” and on every odd number prints “Odd” Hint: use modulo
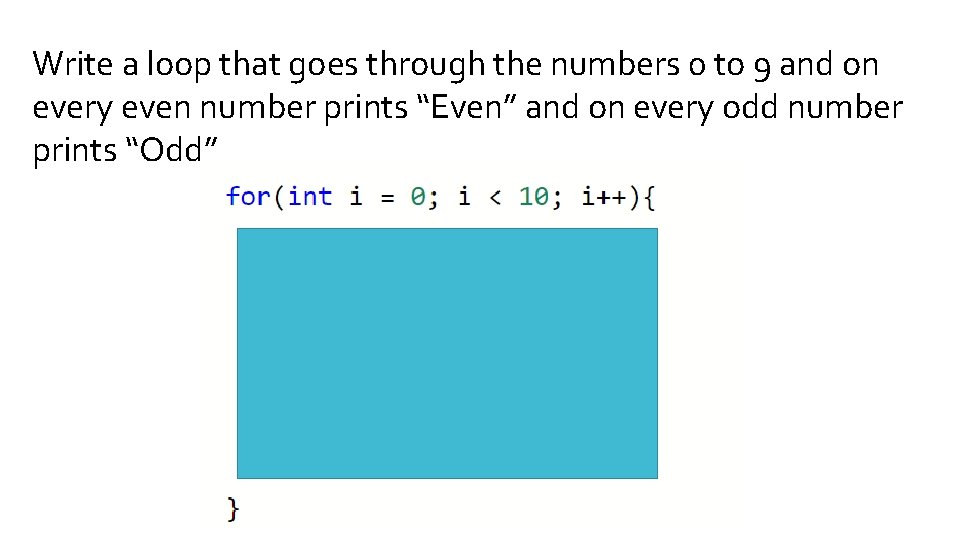
Write a loop that goes through the numbers 0 to 9 and on every even number prints “Even” and on every odd number prints “Odd”
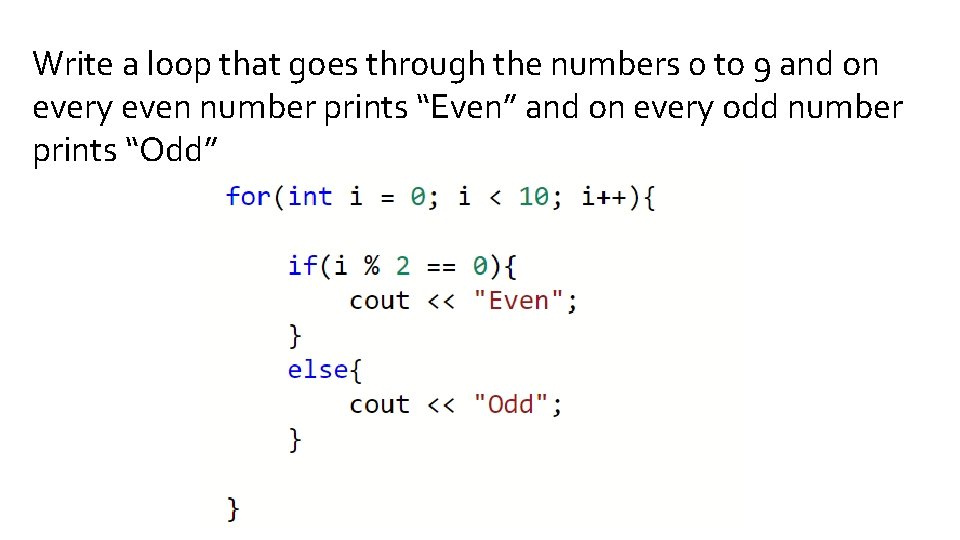
Write a loop that goes through the numbers 0 to 9 and on every even number prints “Even” and on every odd number prints “Odd”
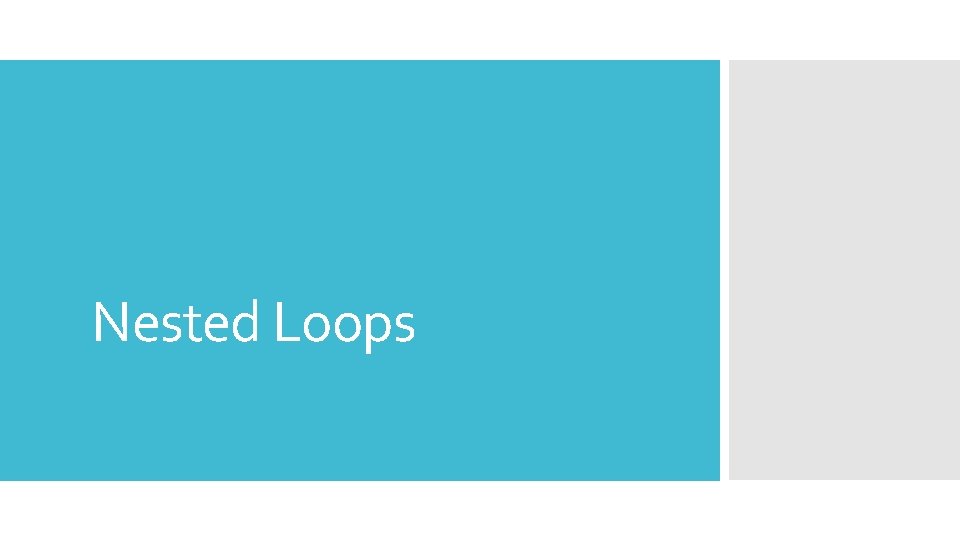
Nested Loops
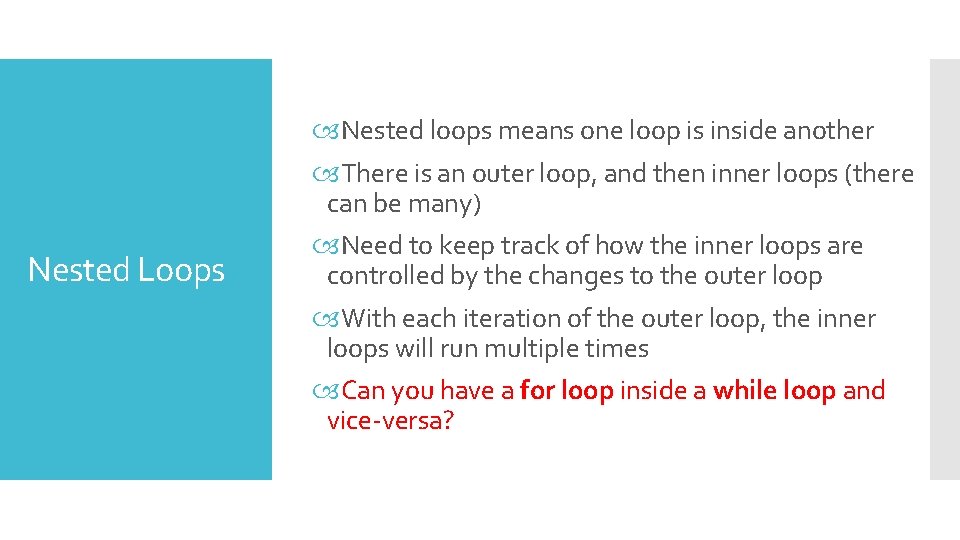
Nested Loops Nested loops means one loop is inside another There is an outer loop, and then inner loops (there can be many) Need to keep track of how the inner loops are controlled by the changes to the outer loop With each iteration of the outer loop, the inner loops will run multiple times Can you have a for loop inside a while loop and vice-versa?
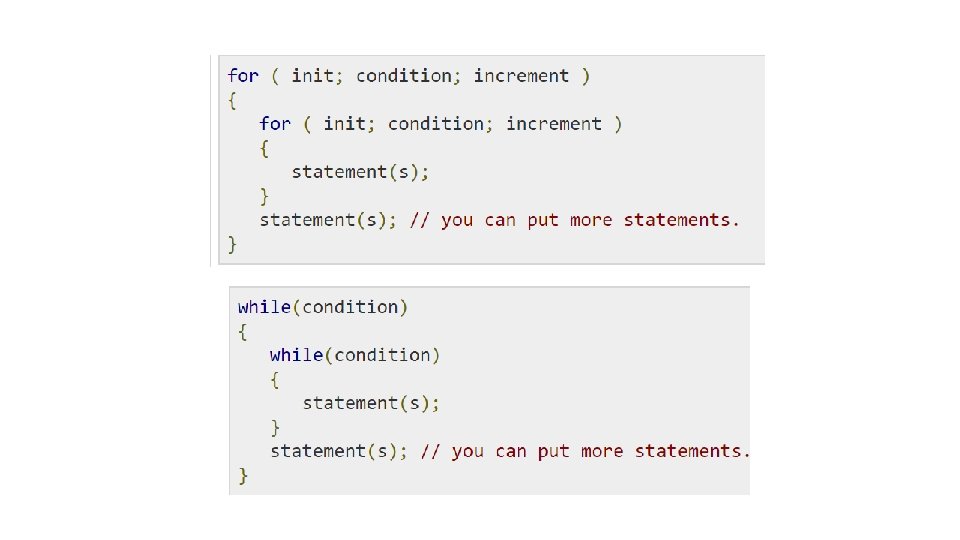
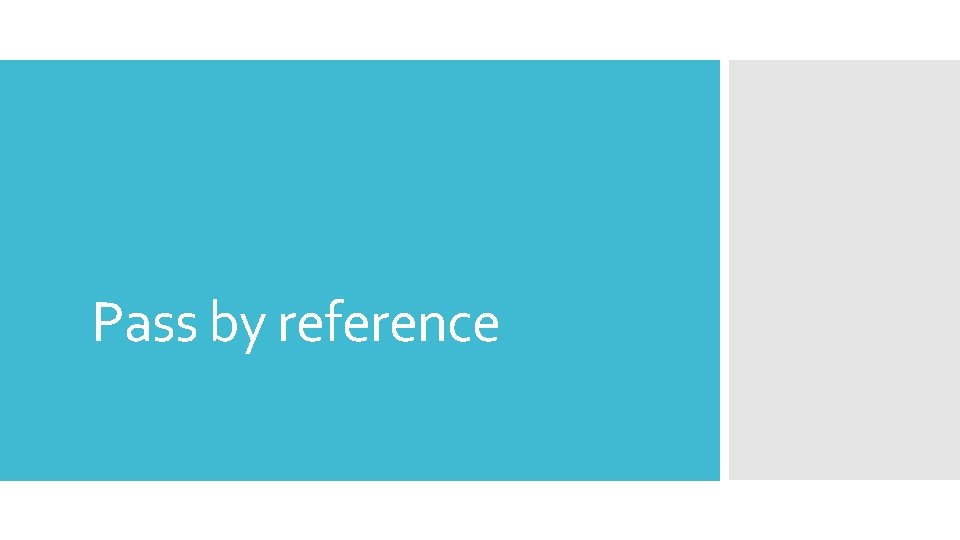
Pass by reference
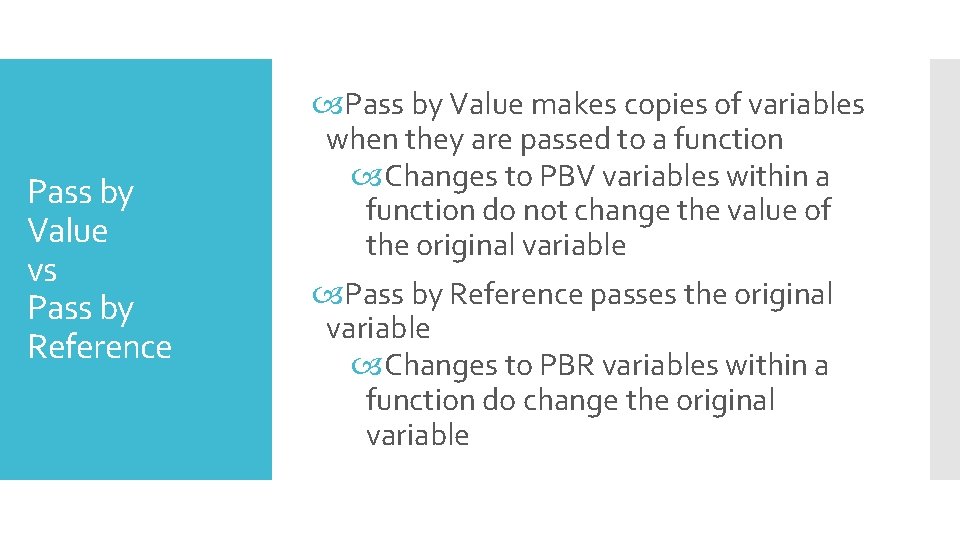
Pass by Value vs Pass by Reference Pass by Value makes copies of variables when they are passed to a function Changes to PBV variables within a function do not change the value of the original variable Pass by Reference passes the original variable Changes to PBR variables within a function do change the original variable
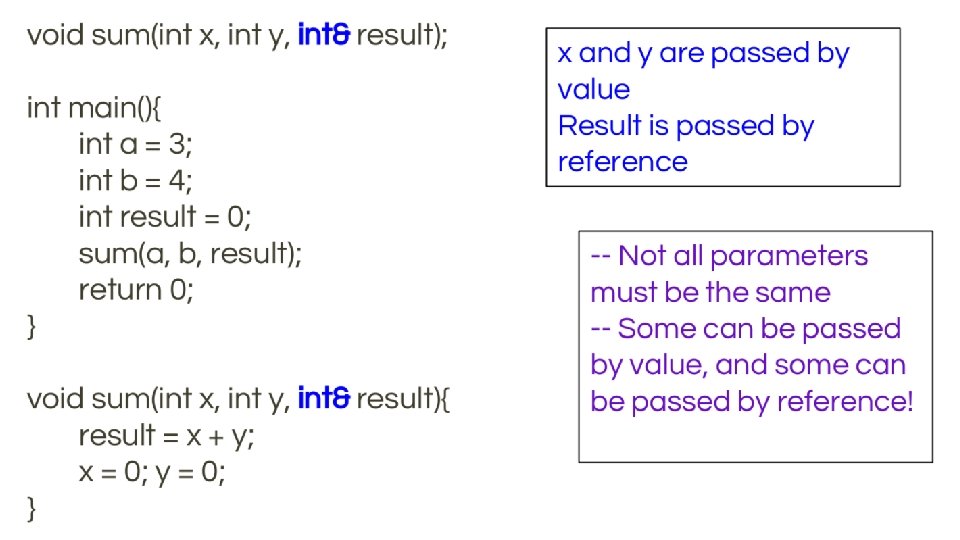
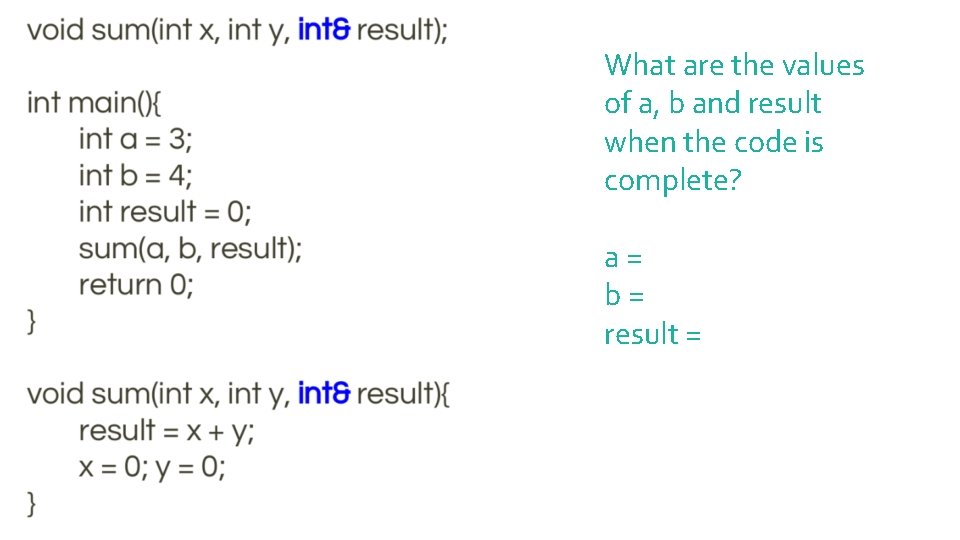
What are the values of a, b and result when the code is complete? a= b= result =
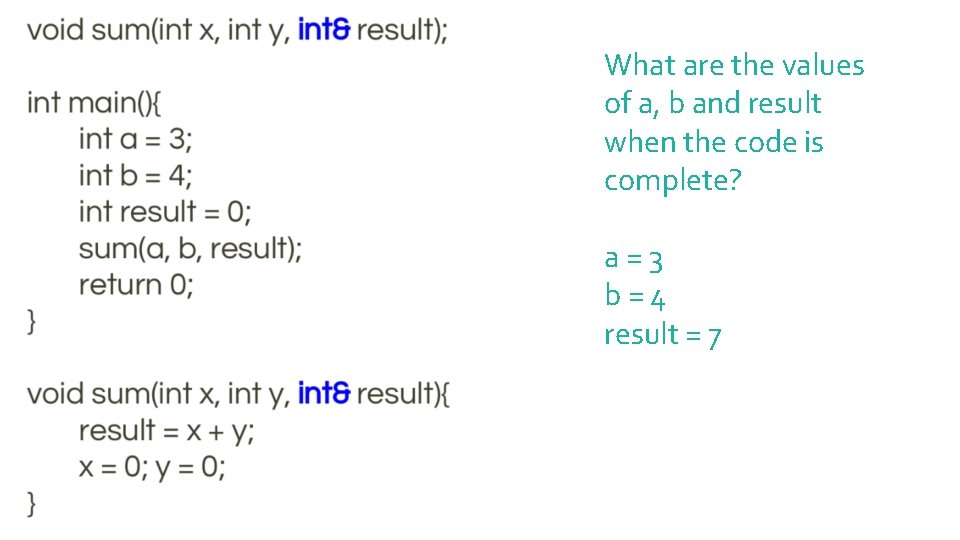
What are the values of a, b and result when the code is complete? a=3 b=4 result = 7
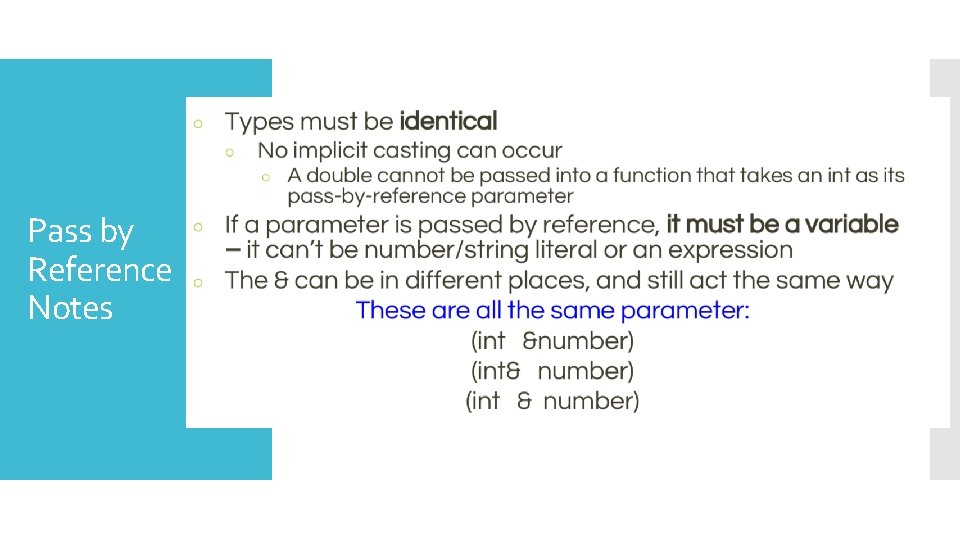
Pass by Reference Notes
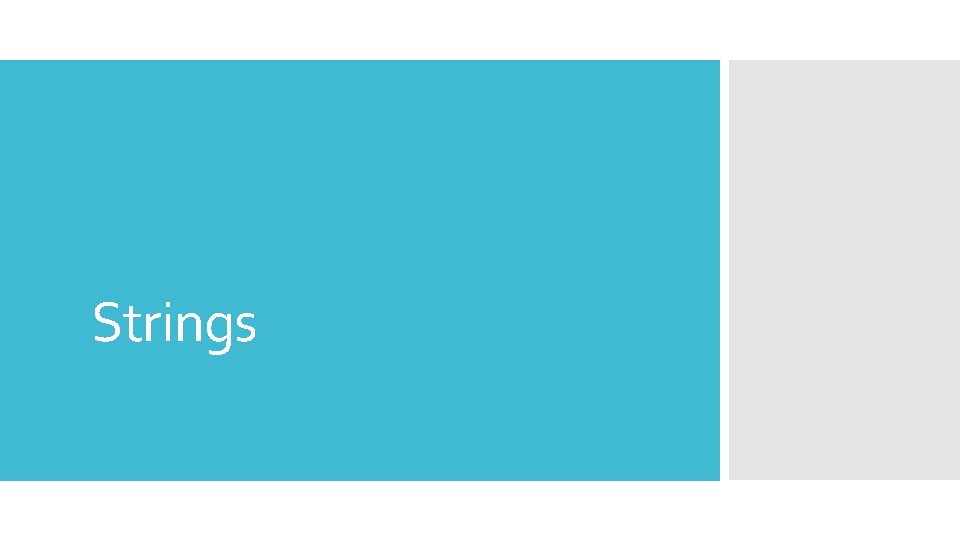
Strings
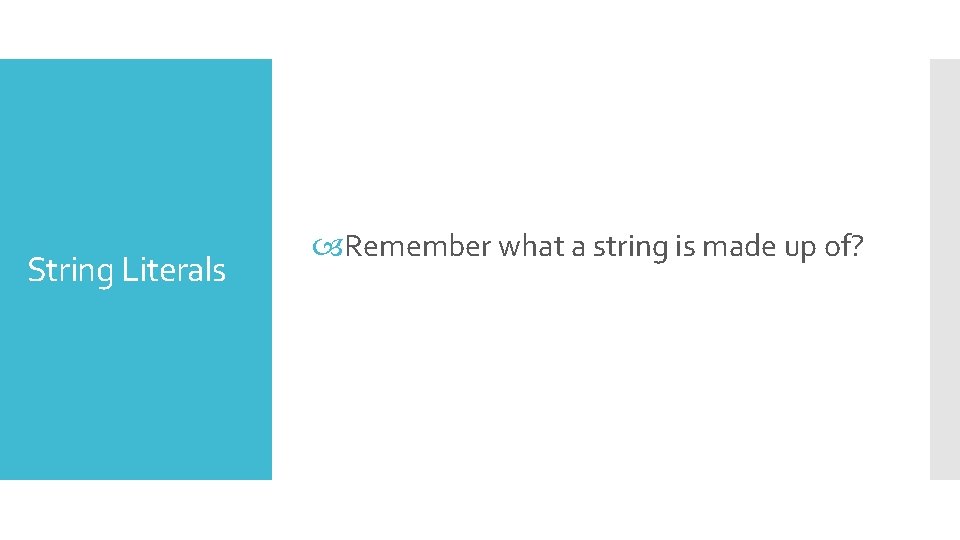
String Literals Remember what a string is made up of?
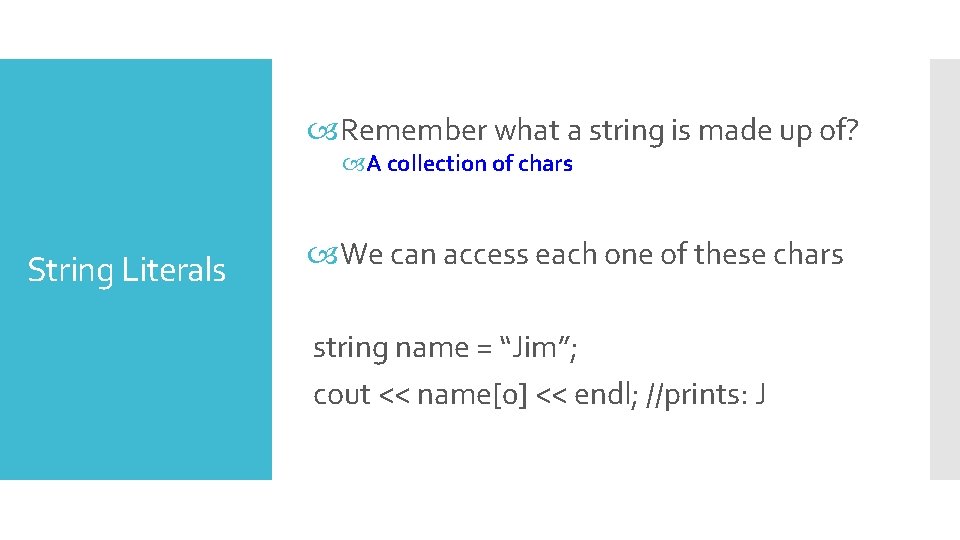
Remember what a string is made up of? A collection of chars String Literals We can access each one of these chars string name = “Jim”; cout << name[0] << endl; //prints: J
![string name Jim cout name0 endl prints J Accessing characters of string name = “Jim”; cout << name[0] << endl; //prints: J Accessing characters of](https://slidetodoc.com/presentation_image/6731cfdf865083436f56939d5634a18c/image-29.jpg)
string name = “Jim”; cout << name[0] << endl; //prints: J Accessing characters of strings Strings are what we call: 0 indexed The first char of the string is at index 0 The second char of the string is at index 1 And so on… The index is inside the brackets: [1]
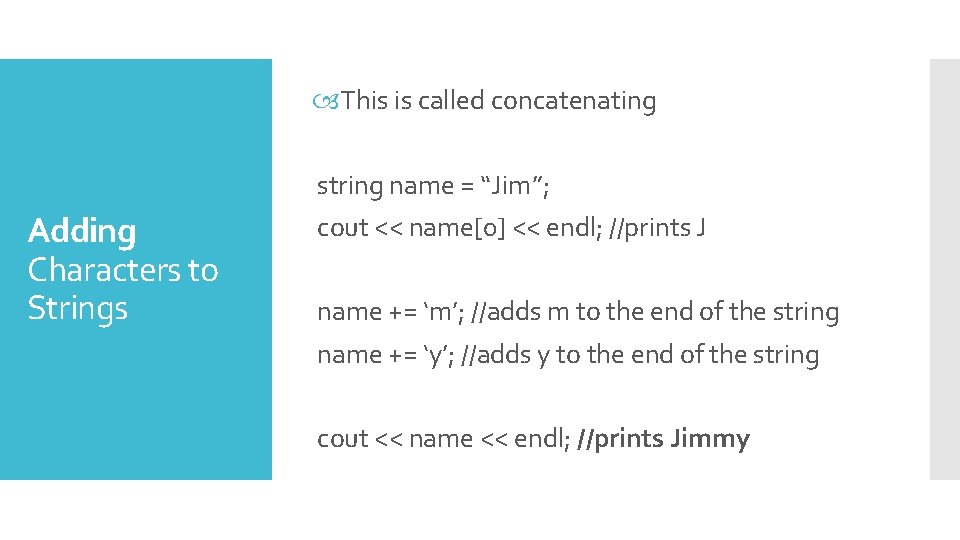
This is called concatenating string name = “Jim”; Adding Characters to Strings cout << name[0] << endl; //prints J name += ‘m’; //adds m to the end of the string name += ‘y’; //adds y to the end of the string cout << name << endl; //prints Jimmy
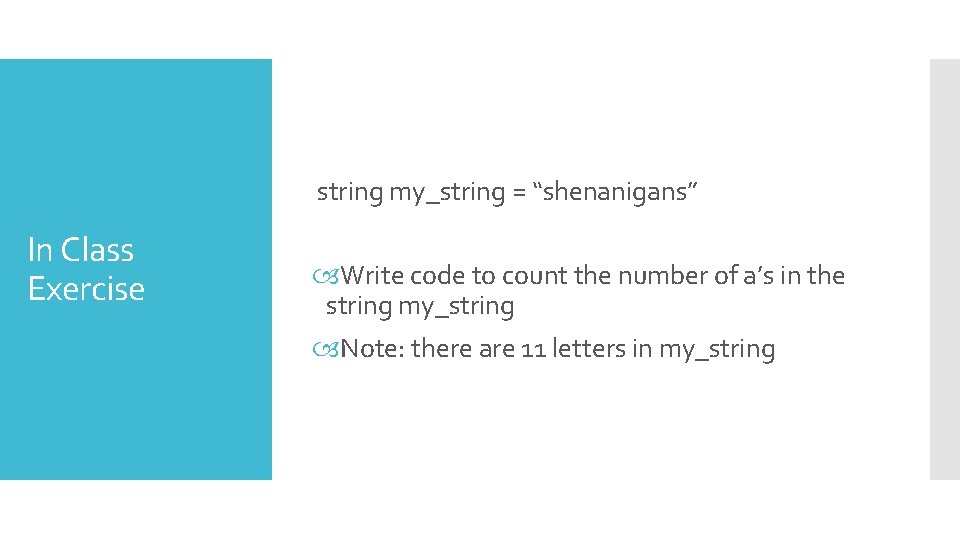
string my_string = “shenanigans” In Class Exercise Write code to count the number of a’s in the string my_string Note: there are 11 letters in my_string
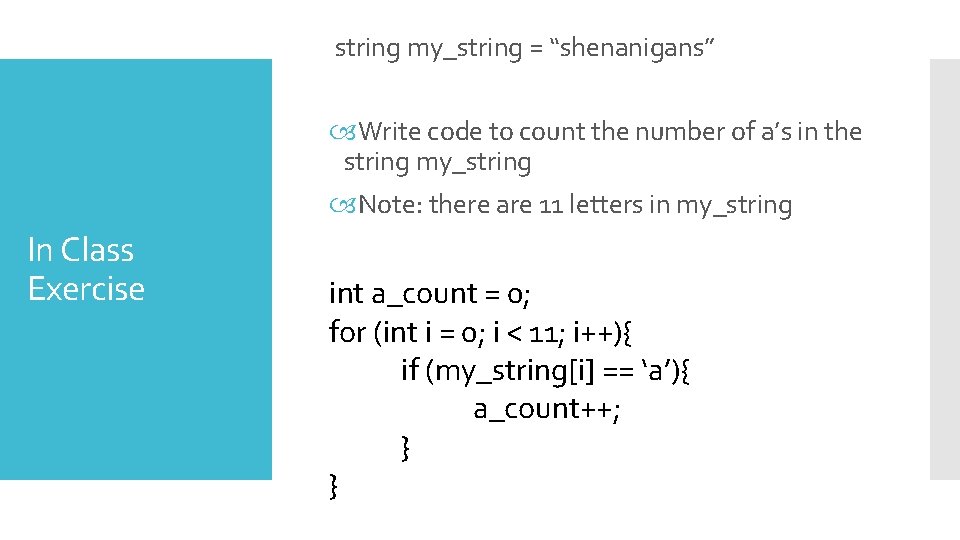
string my_string = “shenanigans” Write code to count the number of a’s in the string my_string Note: there are 11 letters in my_string In Class Exercise int a_count = 0; for (int i = 0; i < 11; i++){ if (my_string[i] == ‘a’){ a_count++; } }
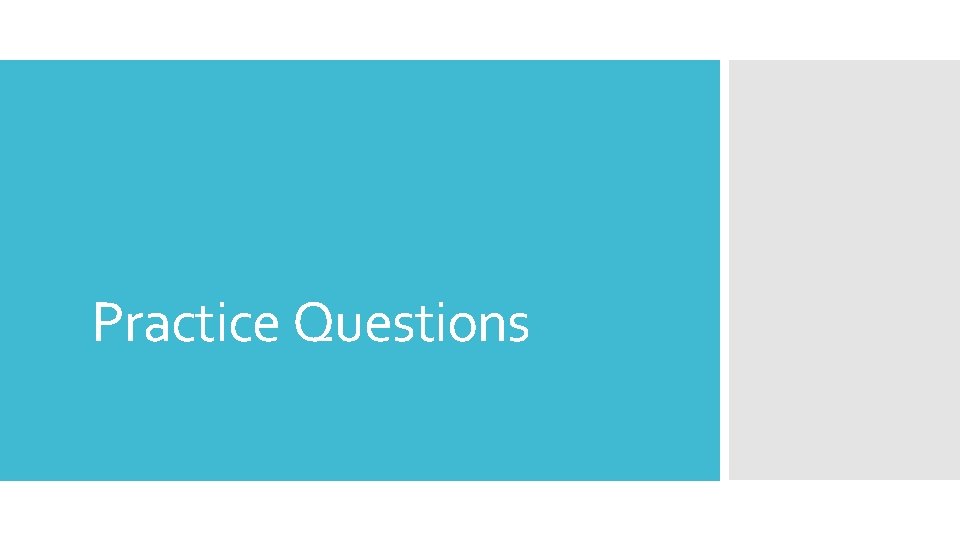
Practice Questions
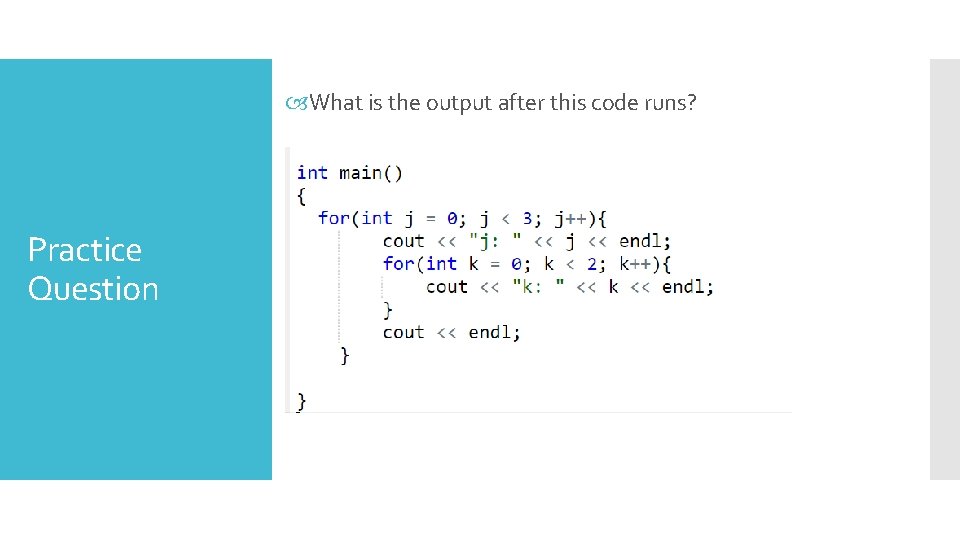
What is the output after this code runs? Practice Question
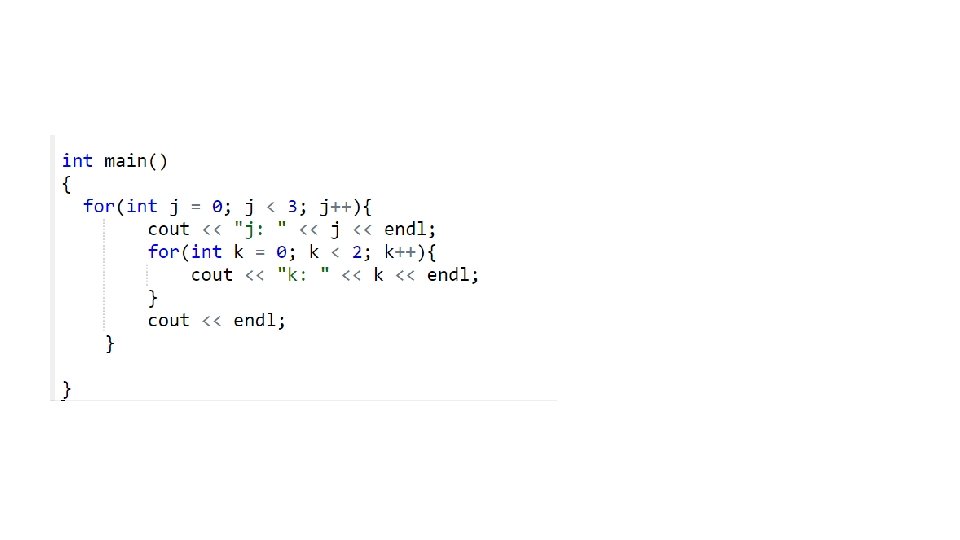
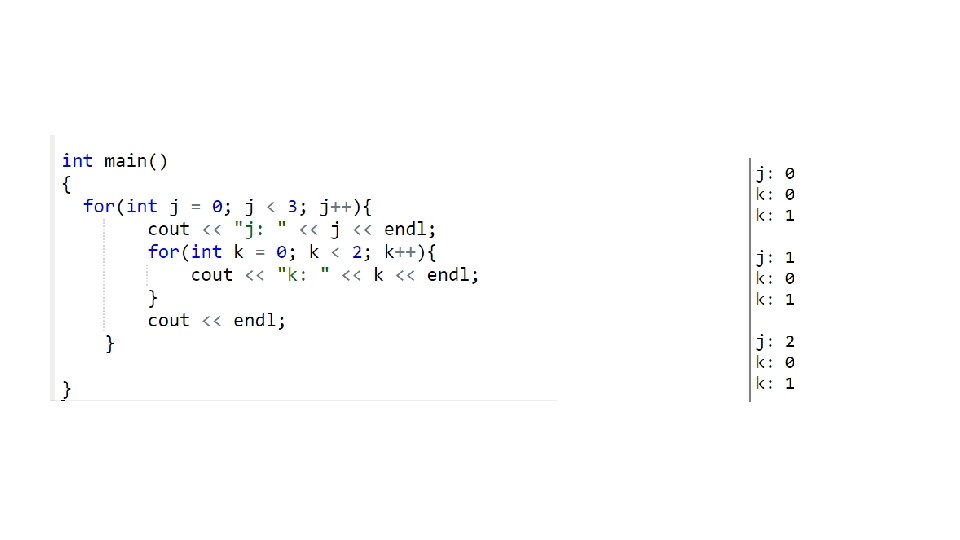
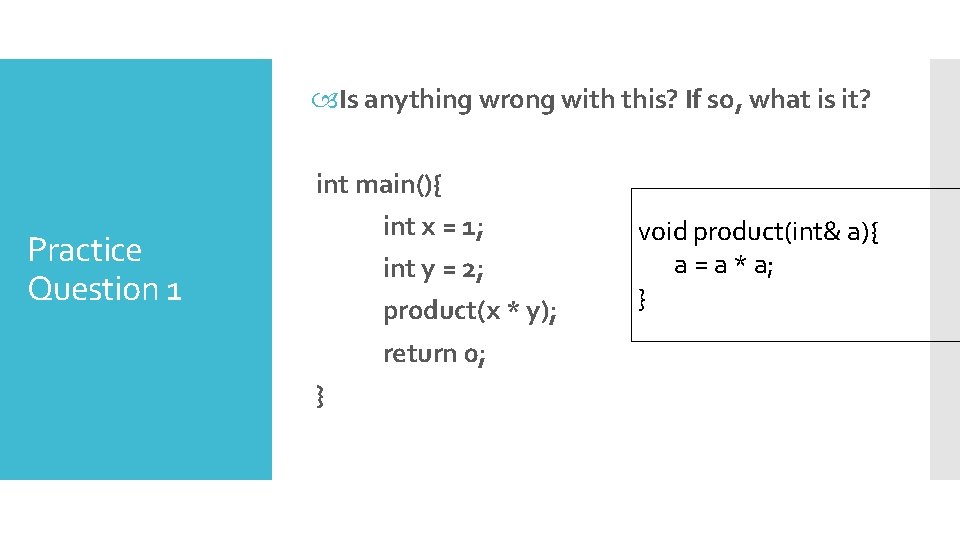
Is anything wrong with this? If so, what is it? int main(){ int x = 1; Practice Question 1 int y = 2; product(x * y); return 0; } void product(int& a){ a = a * a; }
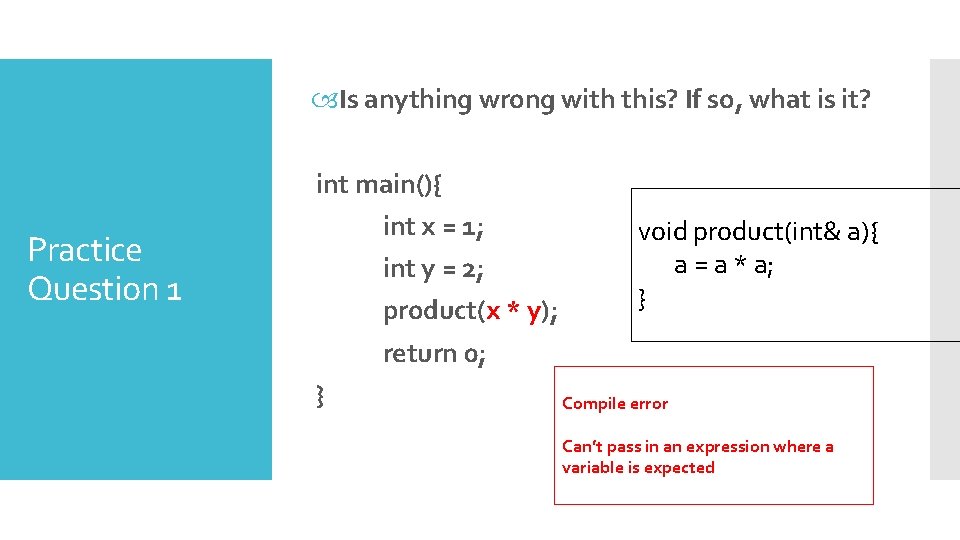
Is anything wrong with this? If so, what is it? int main(){ int x = 1; Practice Question 1 int y = 2; product(x * y); void product(int& a){ a = a * a; } return 0; } Compile error Can’t pass in an expression where a variable is expected
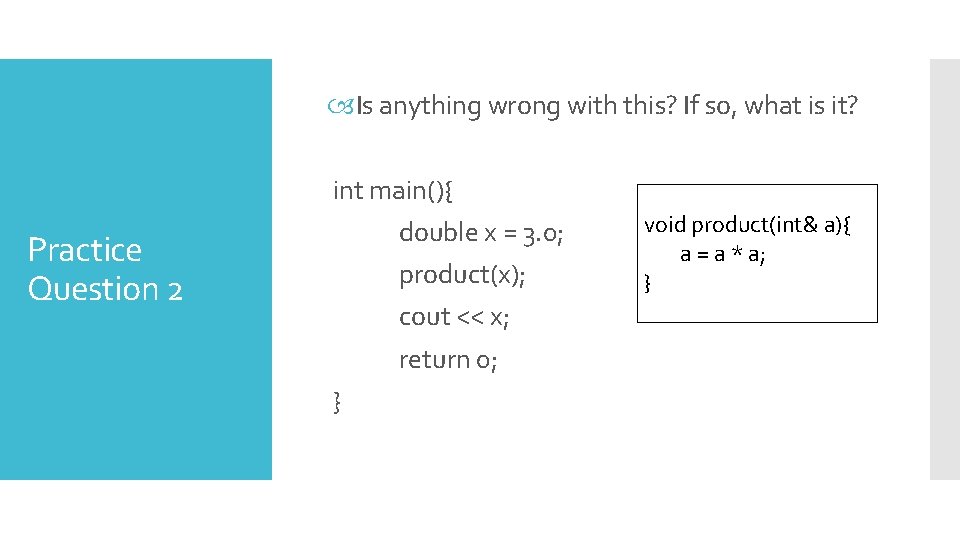
Is anything wrong with this? If so, what is it? int main(){ double x = 3. 0; Practice Question 2 product(x); cout << x; return 0; } void product(int& a){ a = a * a; }
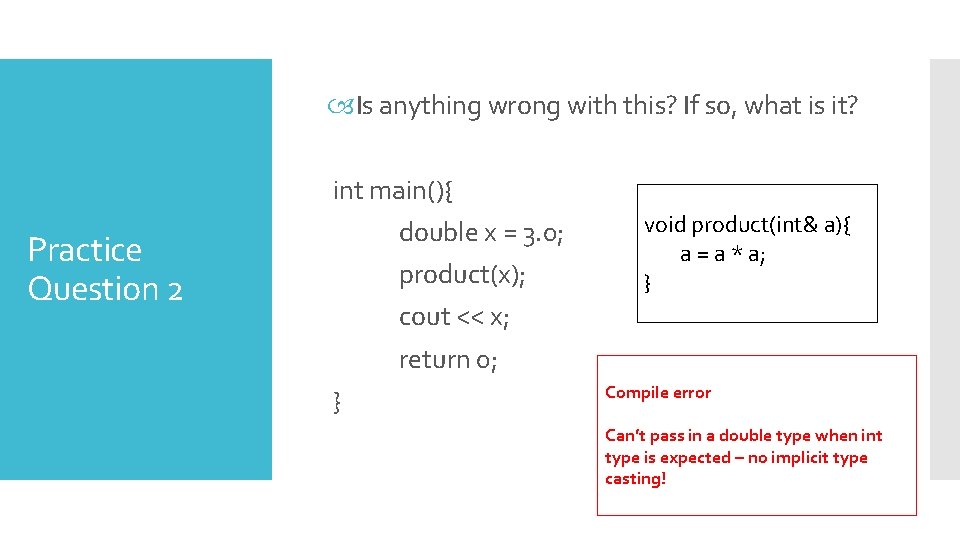
Is anything wrong with this? If so, what is it? int main(){ double x = 3. 0; Practice Question 2 product(x); void product(int& a){ a = a * a; } cout << x; return 0; } Compile error Can’t pass in a double type when int type is expected – no implicit type casting!
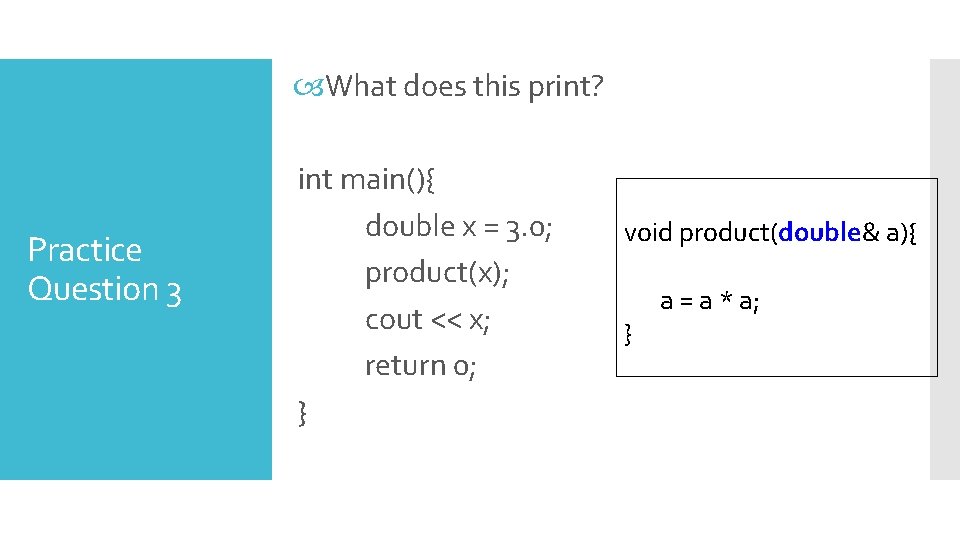
What does this print? Practice Question 3 int main(){ double x = 3. 0; product(x); cout << x; return 0; } void product(double& a){ } a = a * a;
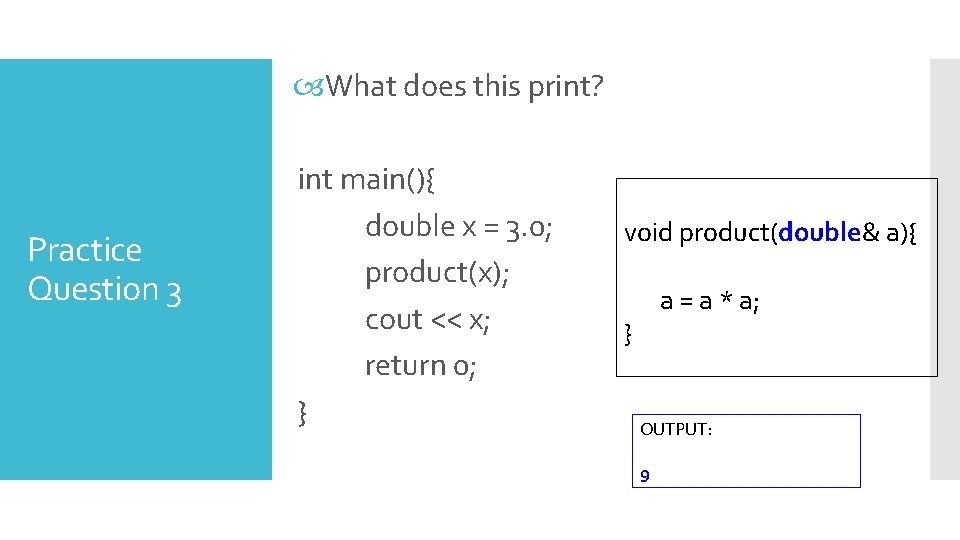
What does this print? Practice Question 3 int main(){ double x = 3. 0; product(x); cout << x; return 0; } void product(double& a){ a = a * a; } OUTPUT: 9
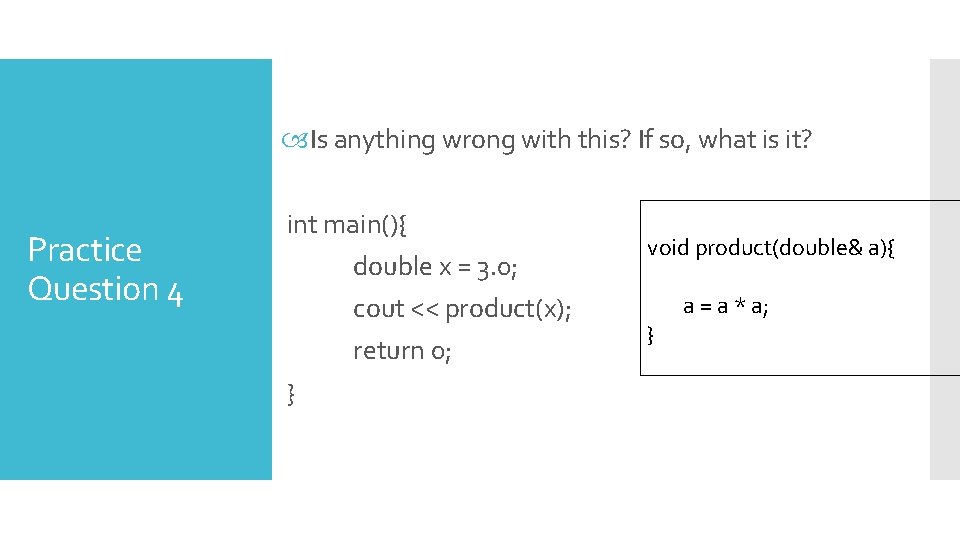
Is anything wrong with this? If so, what is it? Practice Question 4 int main(){ double x = 3. 0; cout << product(x); return 0; } void product(double& a){ } a = a * a;
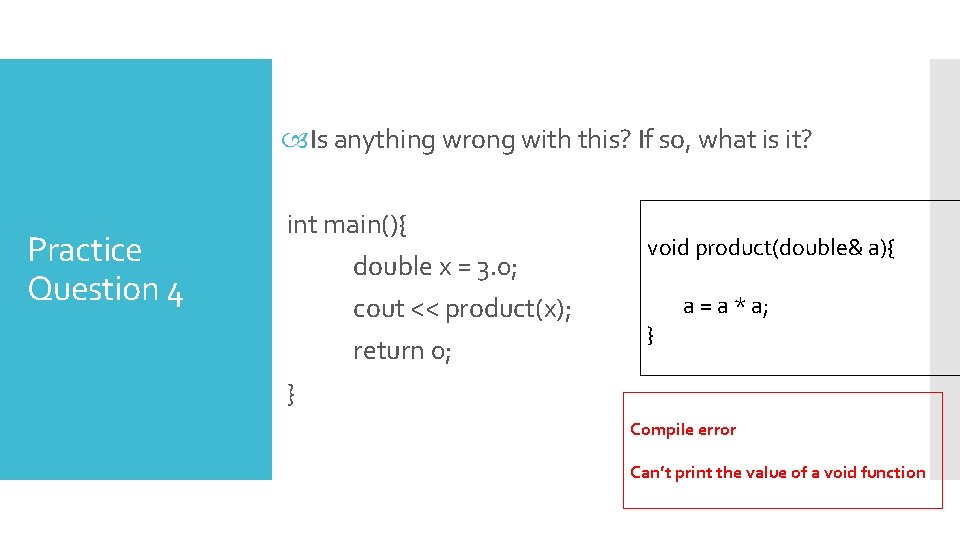
Is anything wrong with this? If so, what is it? Practice Question 4 int main(){ double x = 3. 0; cout << product(x); return 0; void product(double& a){ } a = a * a; } Compile error Can’t print the value of a void function
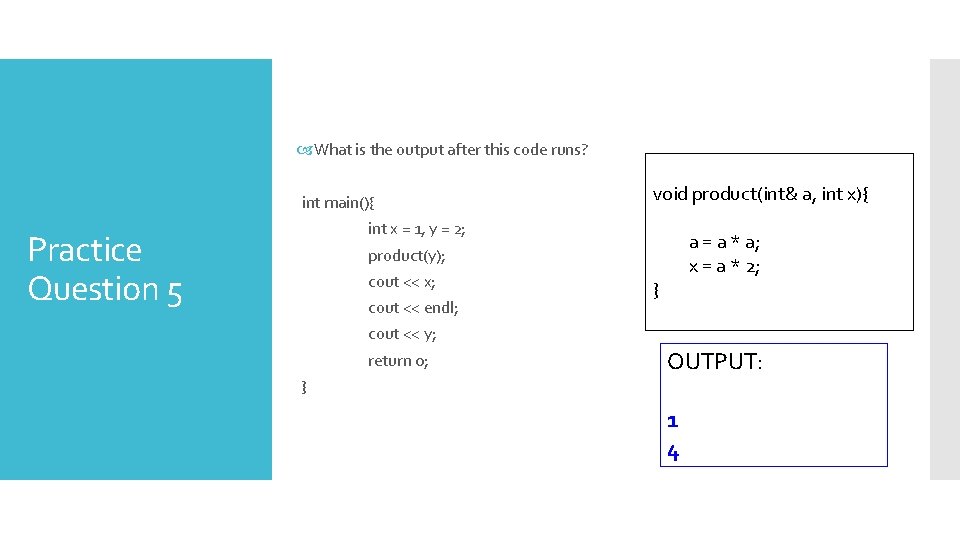
What is the output after this code runs? int main(){ void product(int& a, int x){ int x = 1, y = 2; Practice Question 5 a = a * a; x = a * 2; product(y); cout << x; cout << endl; } cout << y; return 0; } OUTPUT: 1 4