EECS 122 Communications Networks Socket Programming January 30
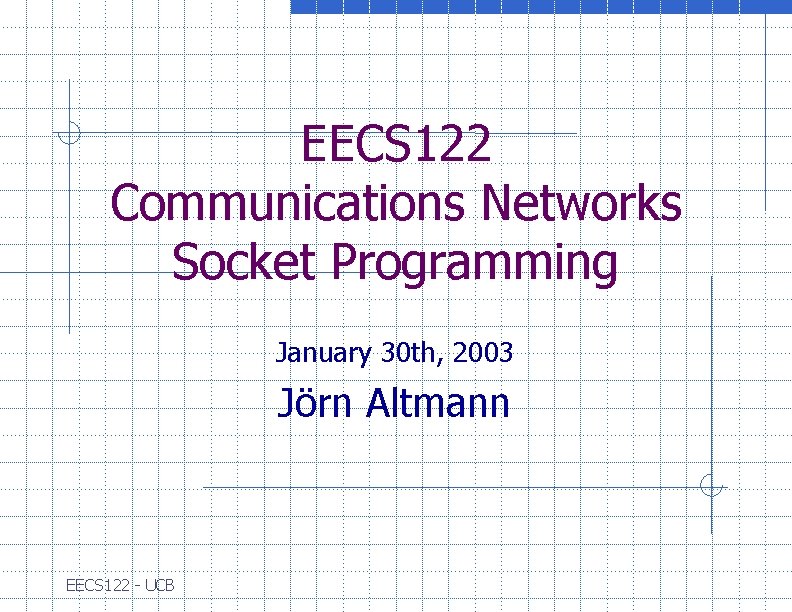
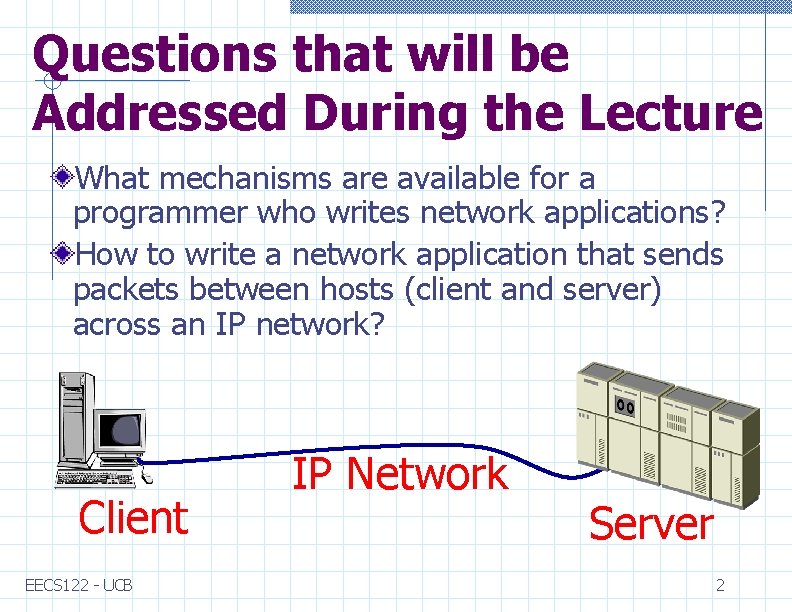
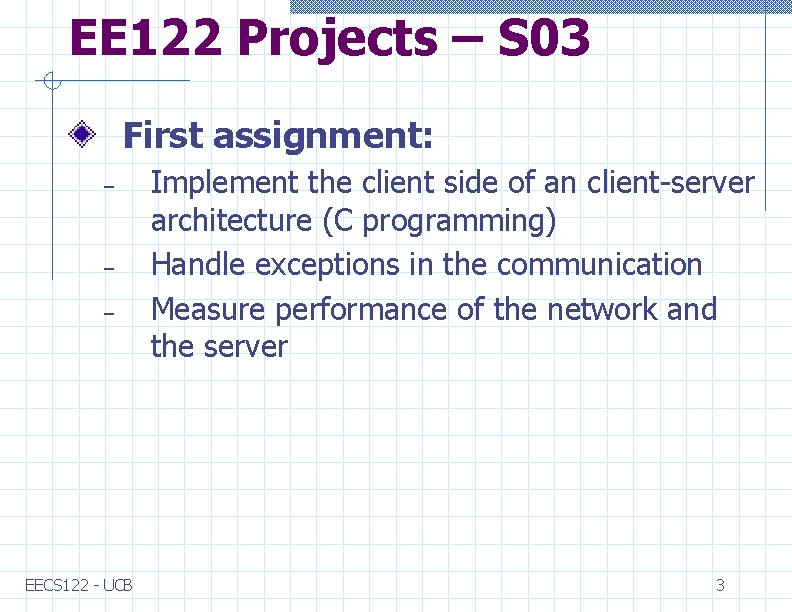
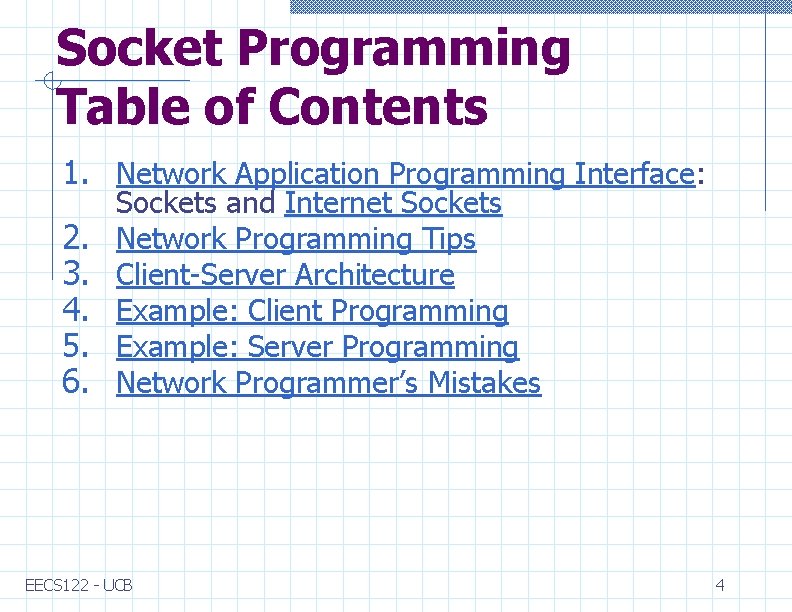
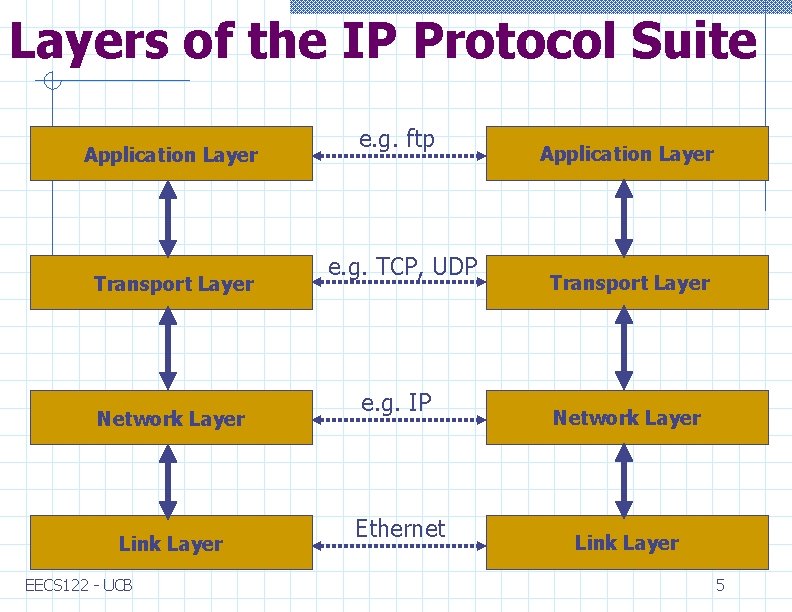
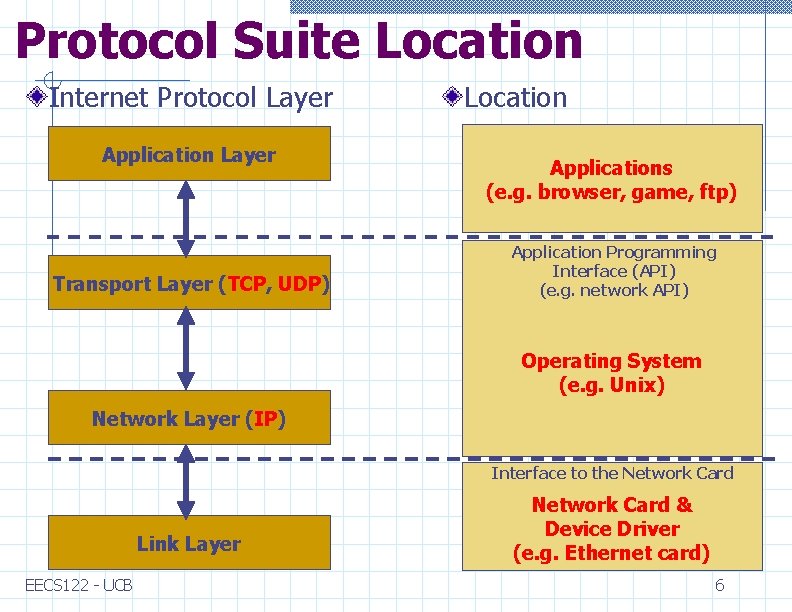
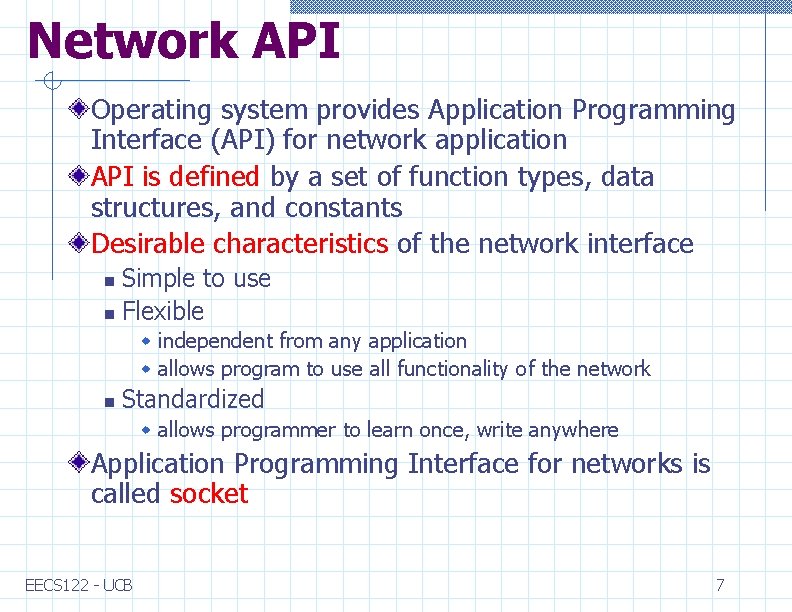
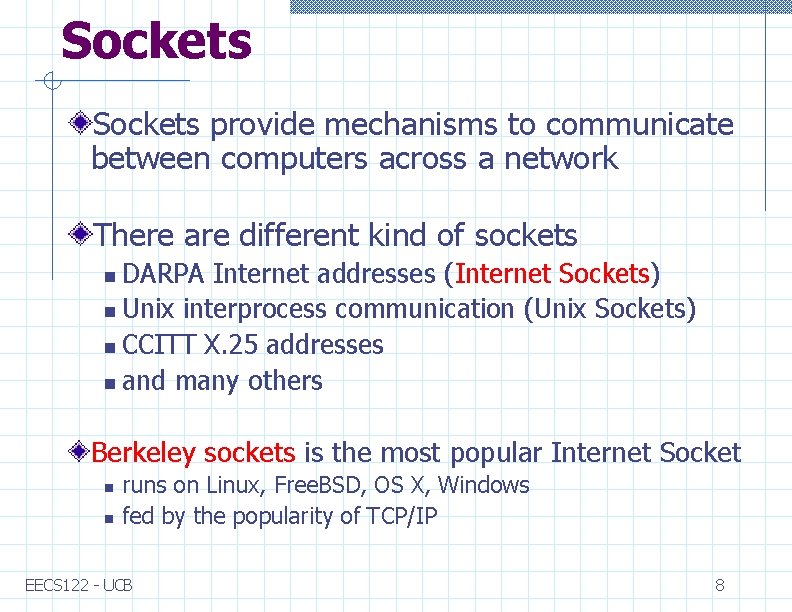
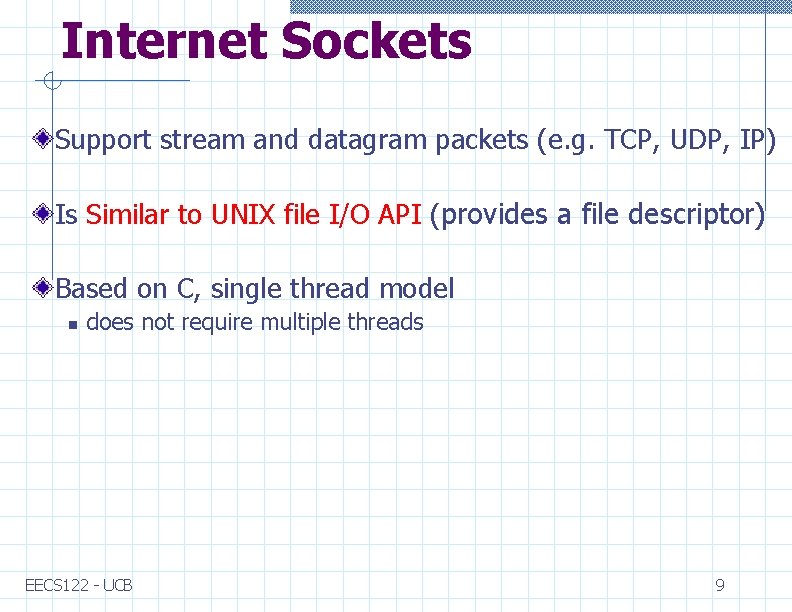
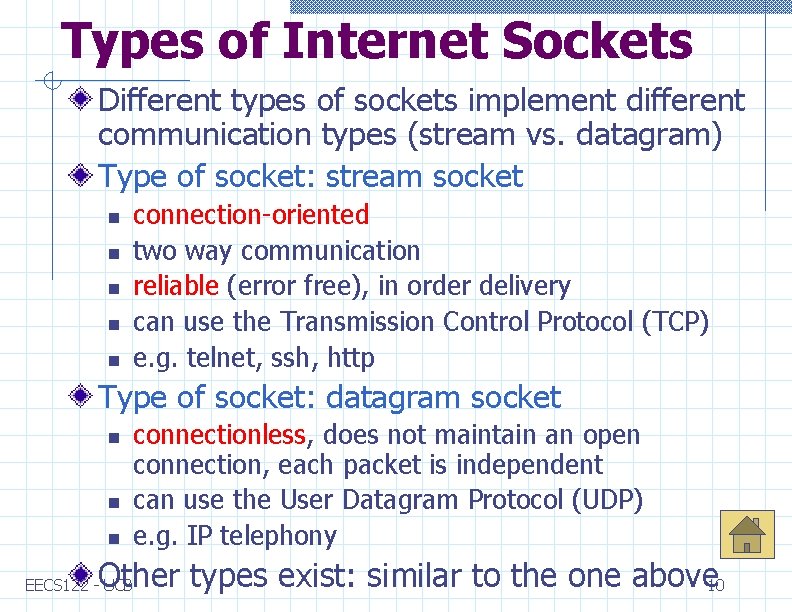
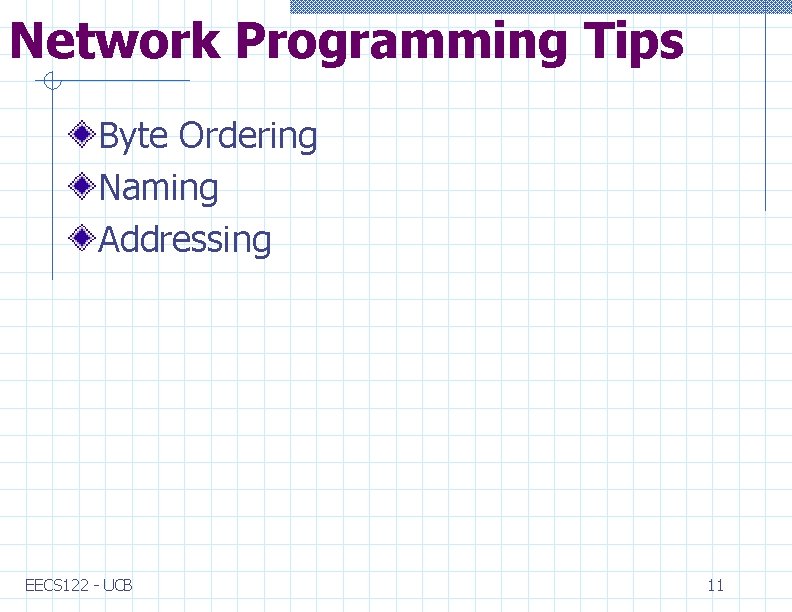
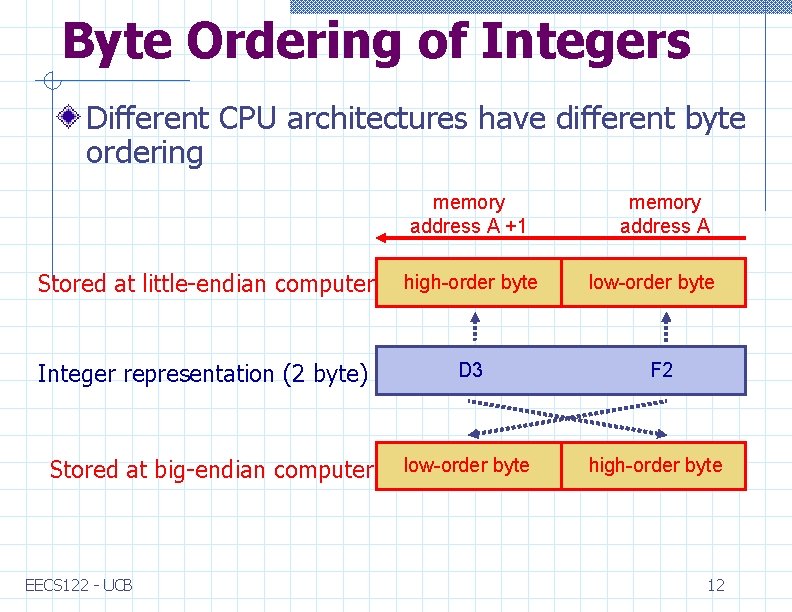
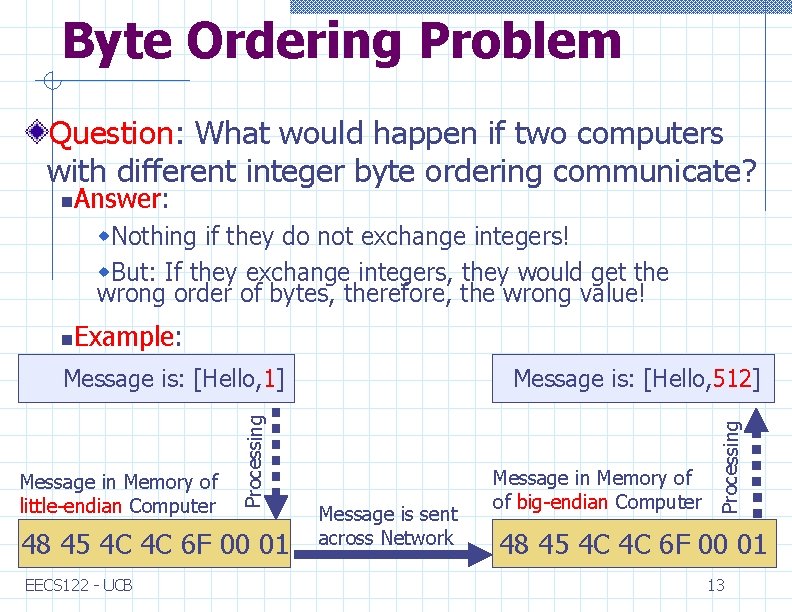
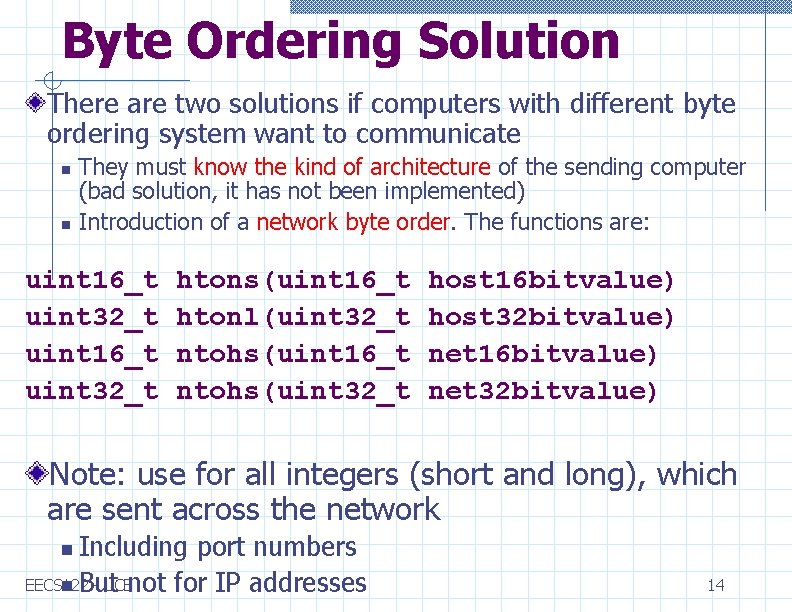
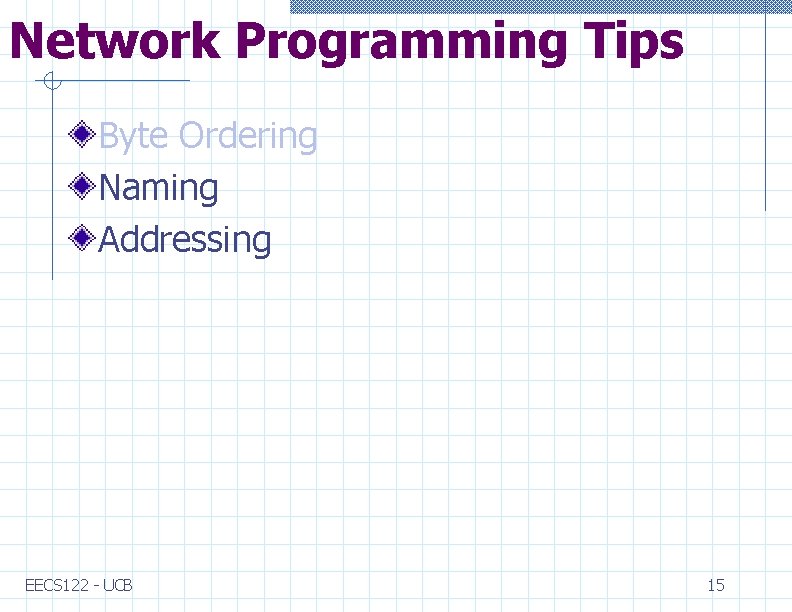
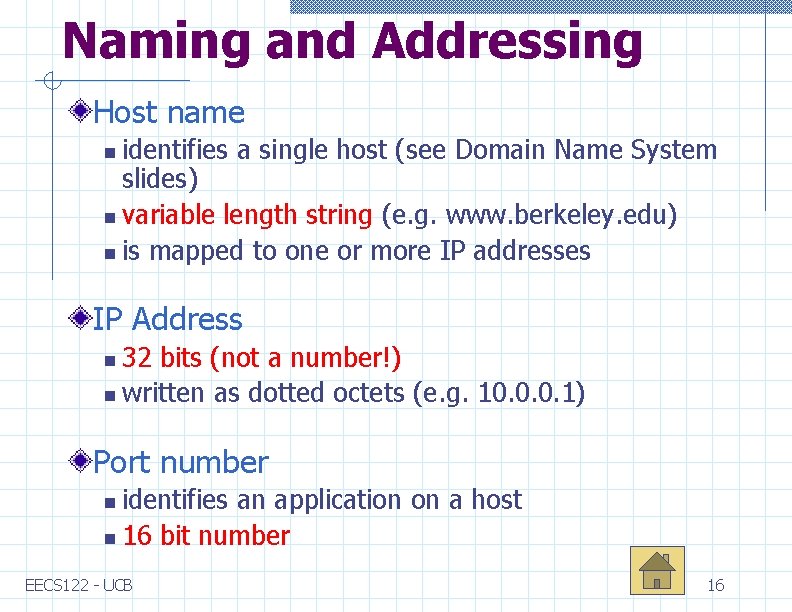
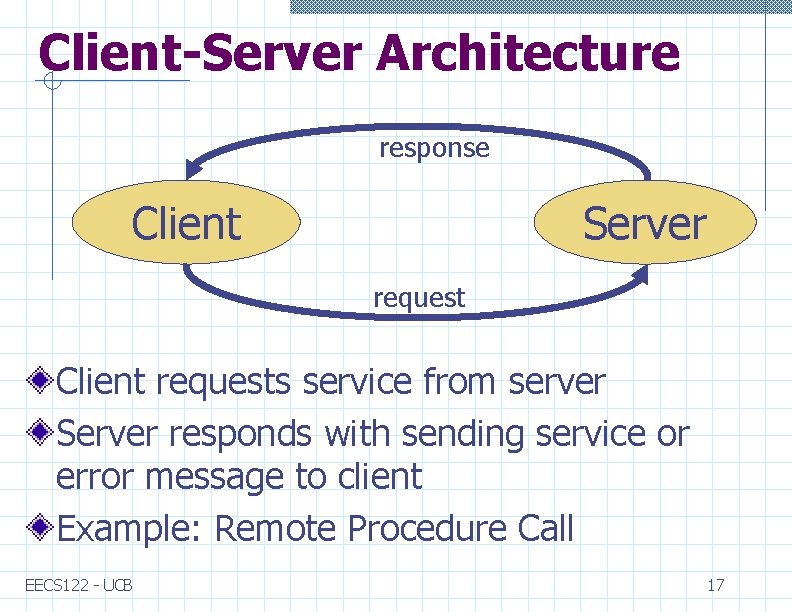
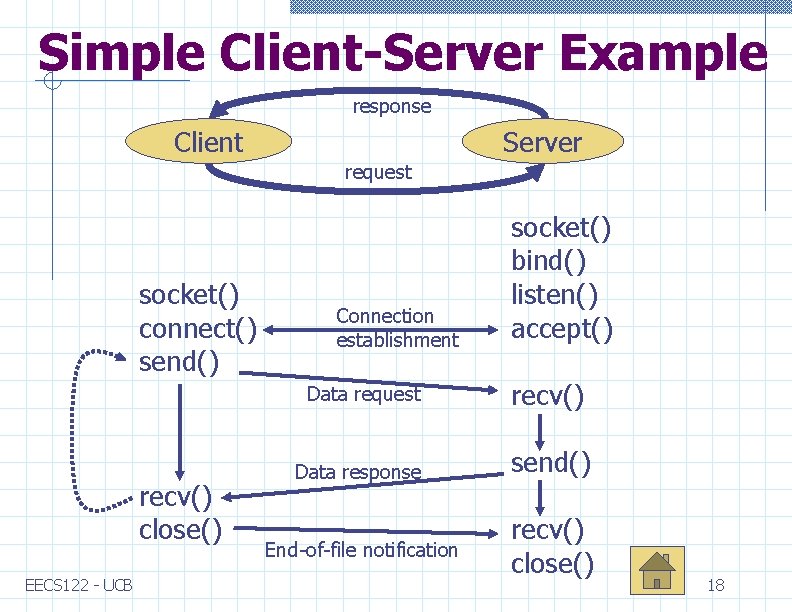
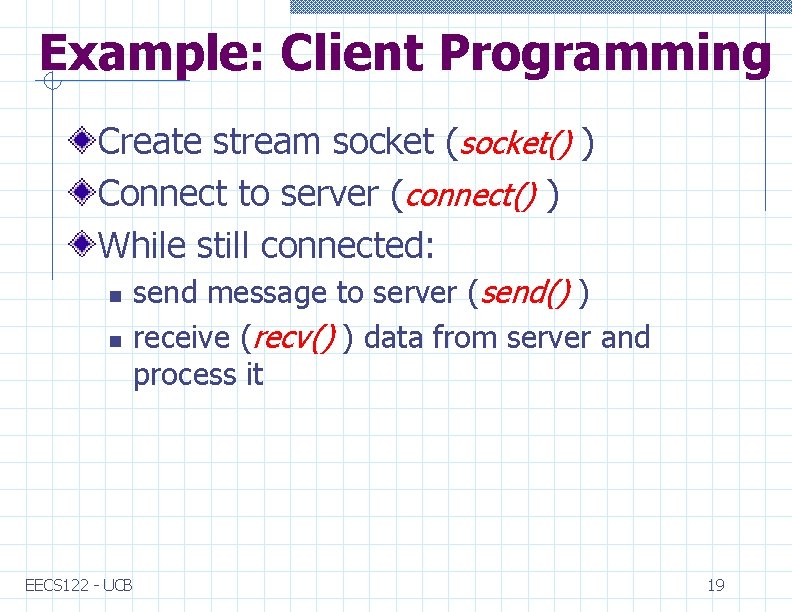
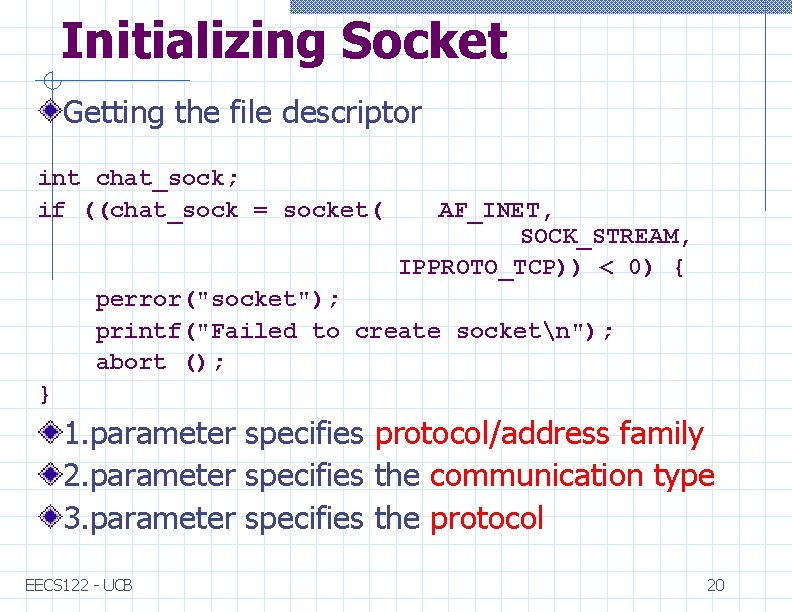
![Connecting to Server struct sockaddr_in sin; struct hostent *host = gethostbyname (argv[1]); unsigned int Connecting to Server struct sockaddr_in sin; struct hostent *host = gethostbyname (argv[1]); unsigned int](https://slidetodoc.com/presentation_image/5169114a8c5c4625783f3757653f85d8/image-21.jpg)
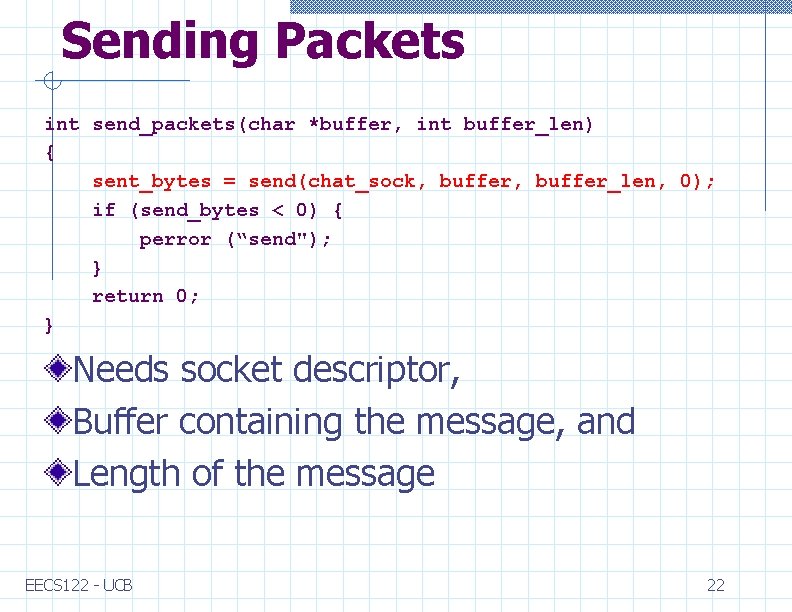
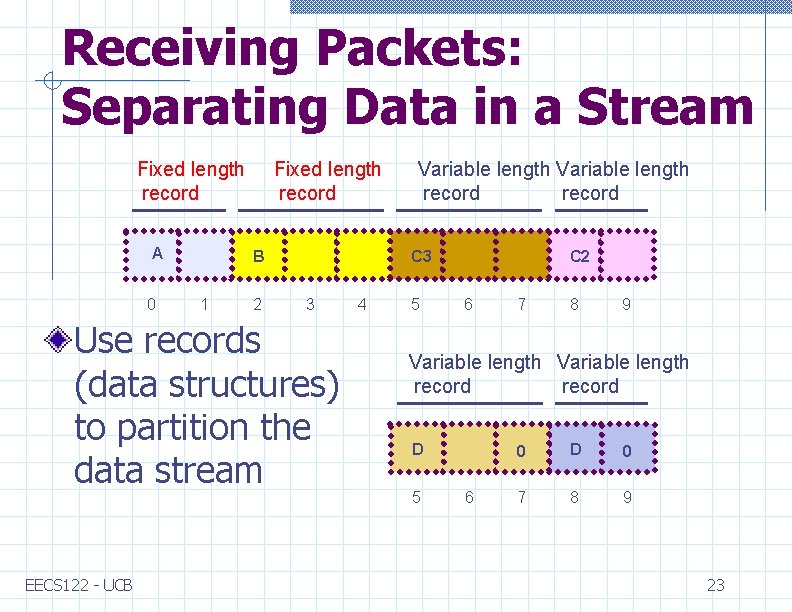
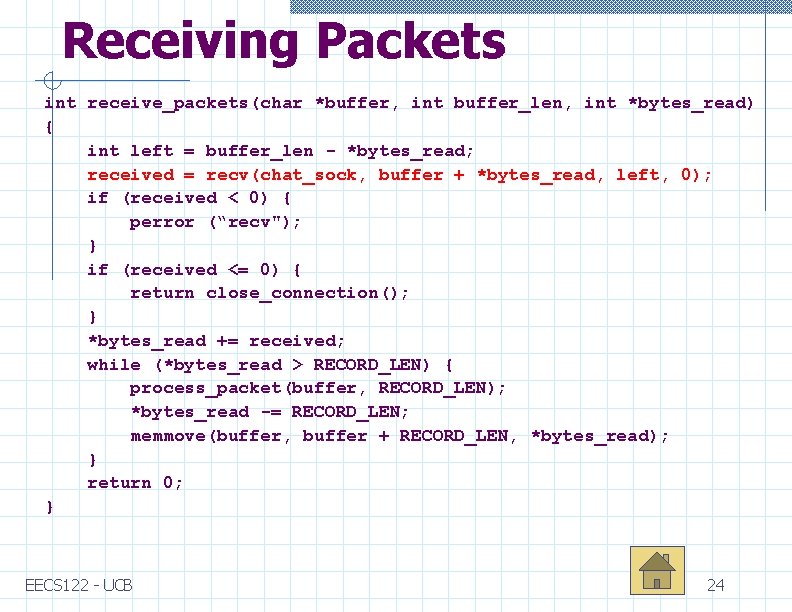
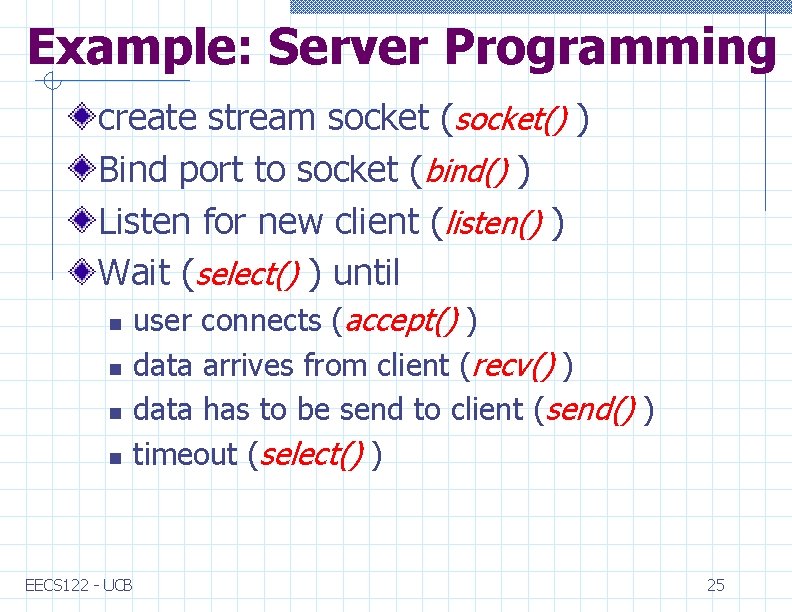
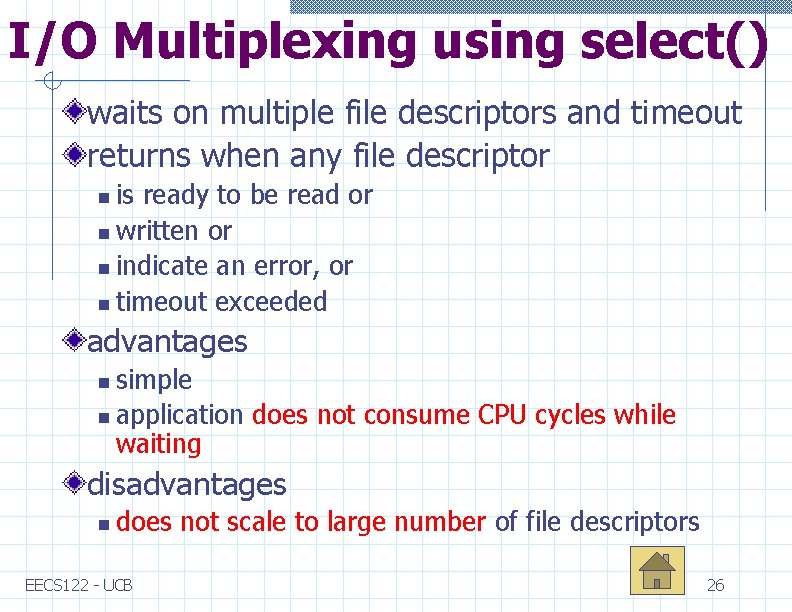
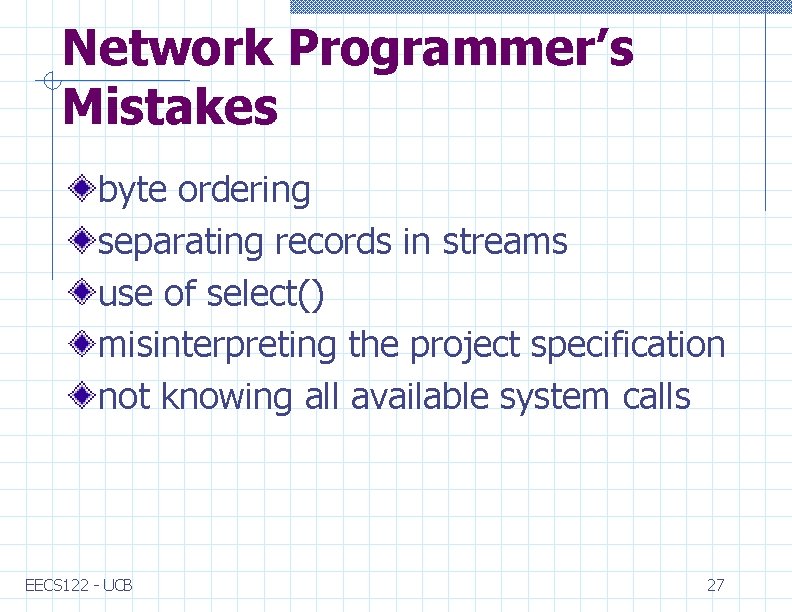
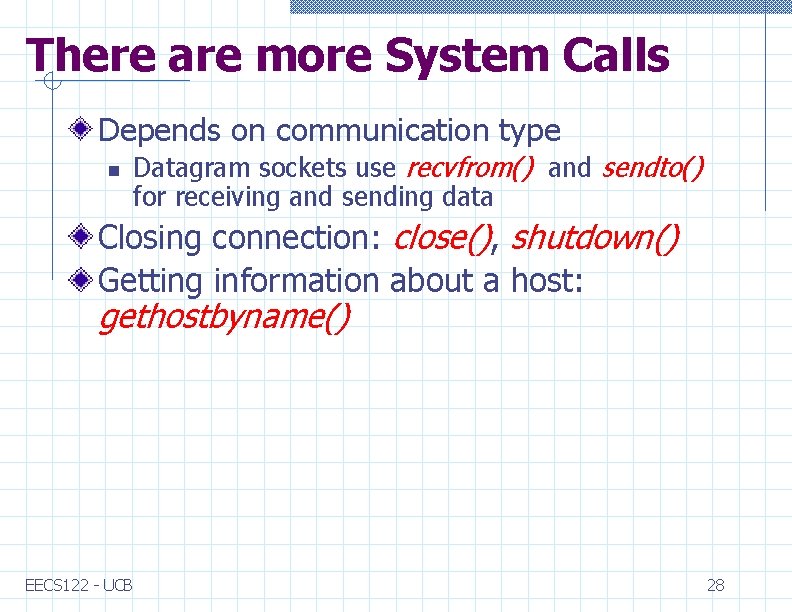
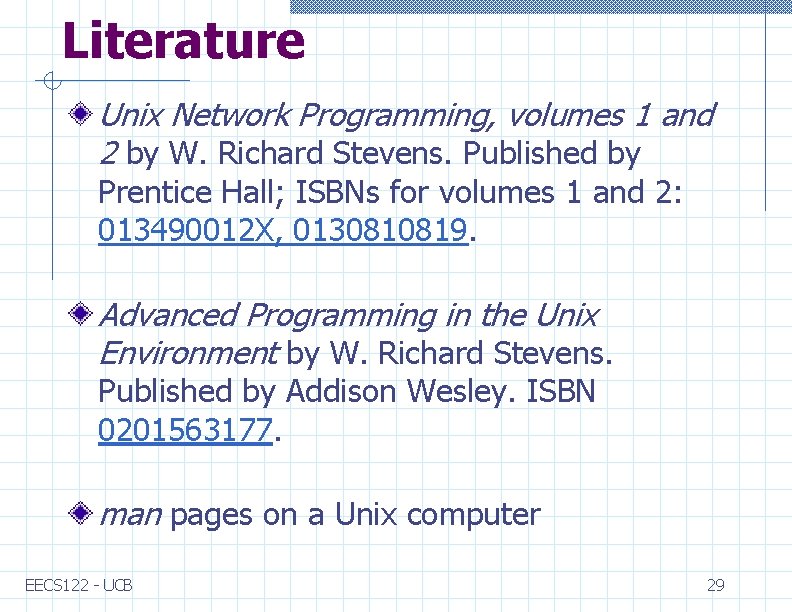
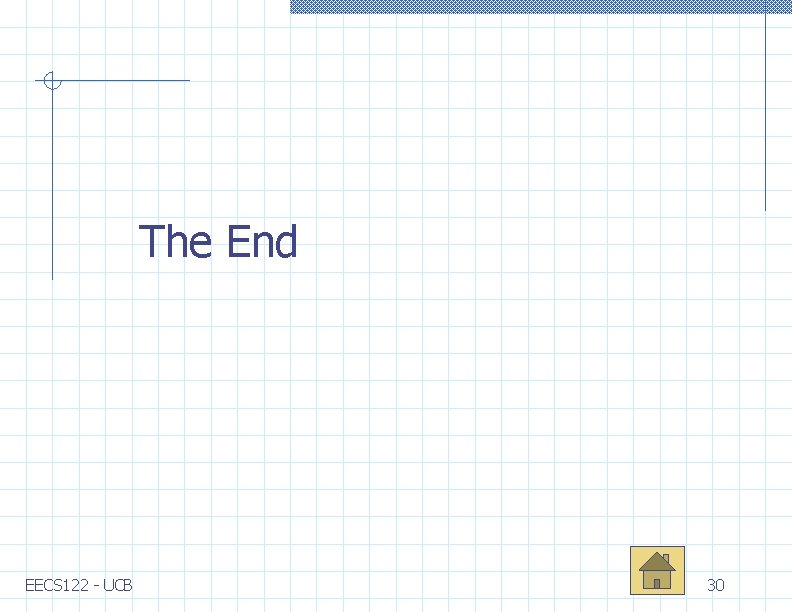
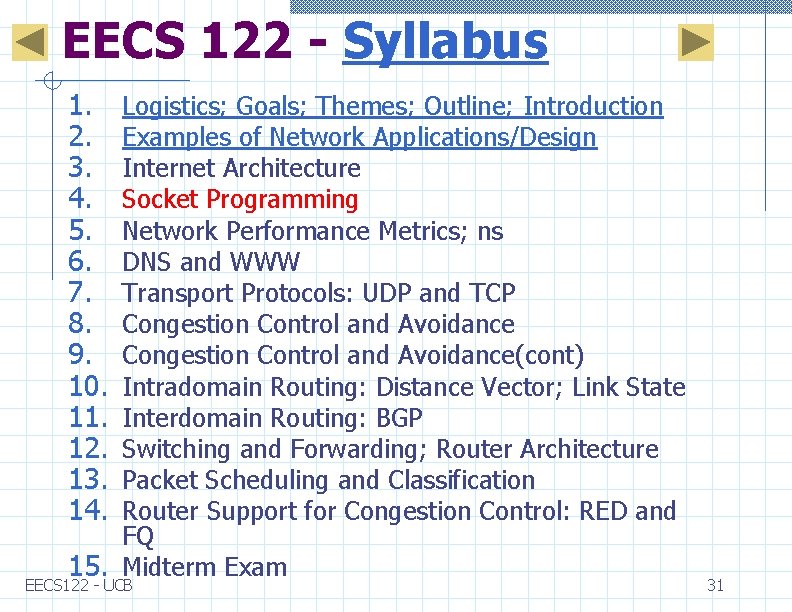
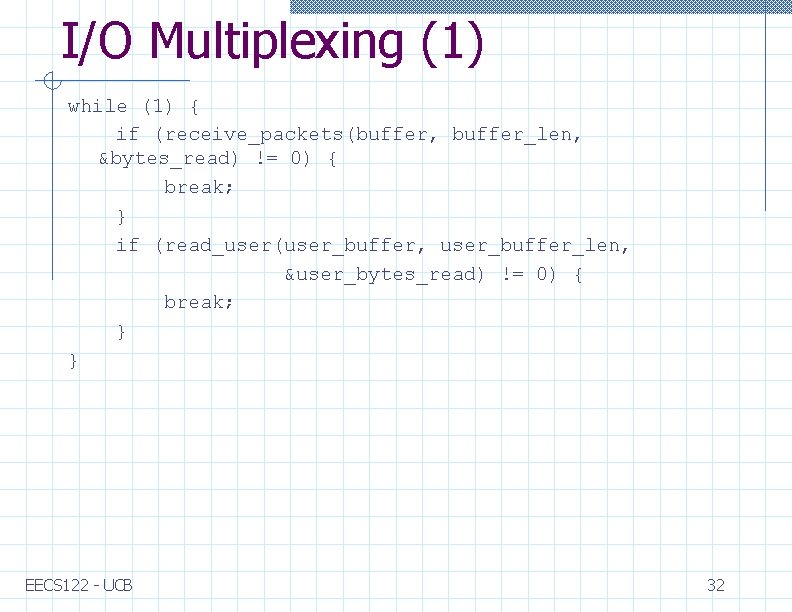
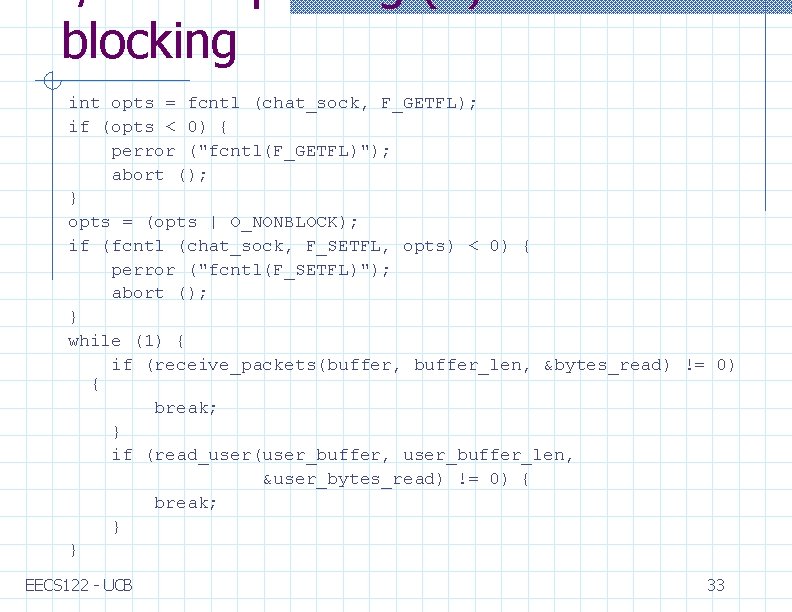
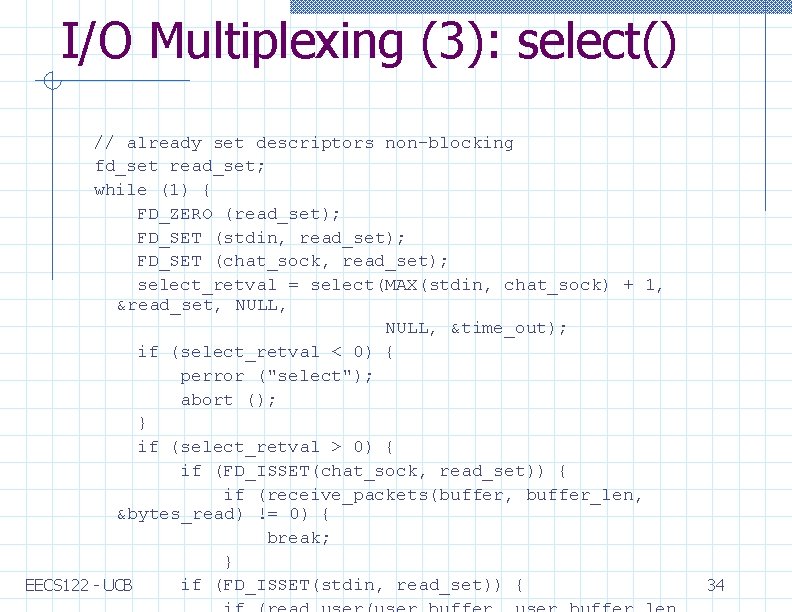
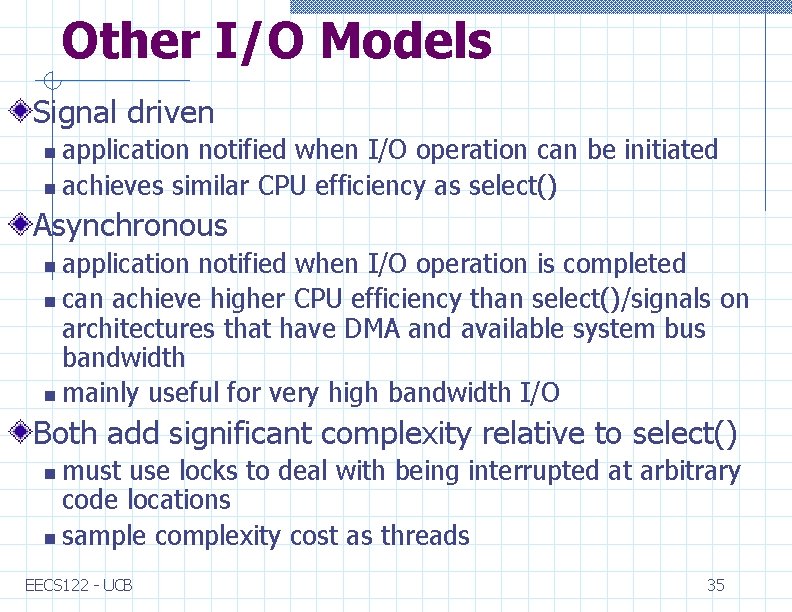
- Slides: 35
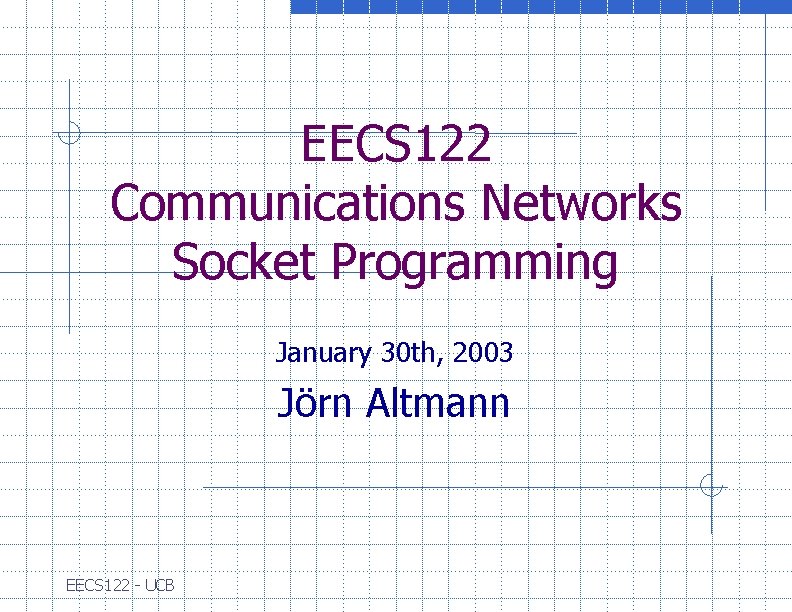
EECS 122 Communications Networks Socket Programming January 30 th, 2003 Jörn Altmann EECS 122 - UCB
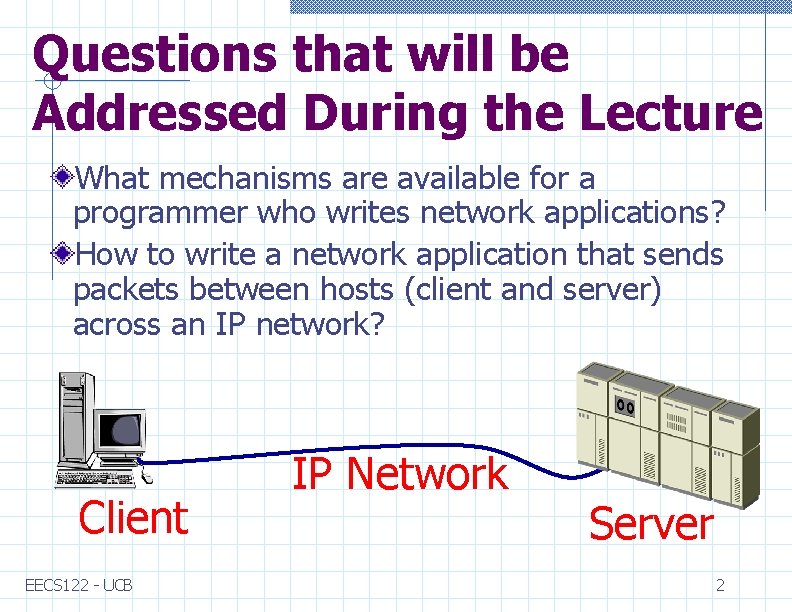
Questions that will be Addressed During the Lecture What mechanisms are available for a programmer who writes network applications? How to write a network application that sends packets between hosts (client and server) across an IP network? Client EECS 122 - UCB IP Network Server 2
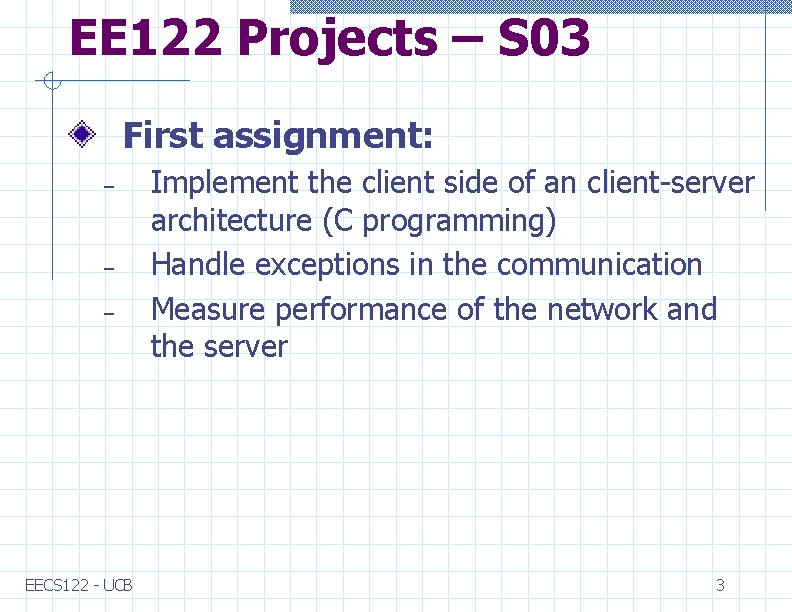
EE 122 Projects – S 03 First assignment: – – – EECS 122 - UCB Implement the client side of an client-server architecture (C programming) Handle exceptions in the communication Measure performance of the network and the server 3
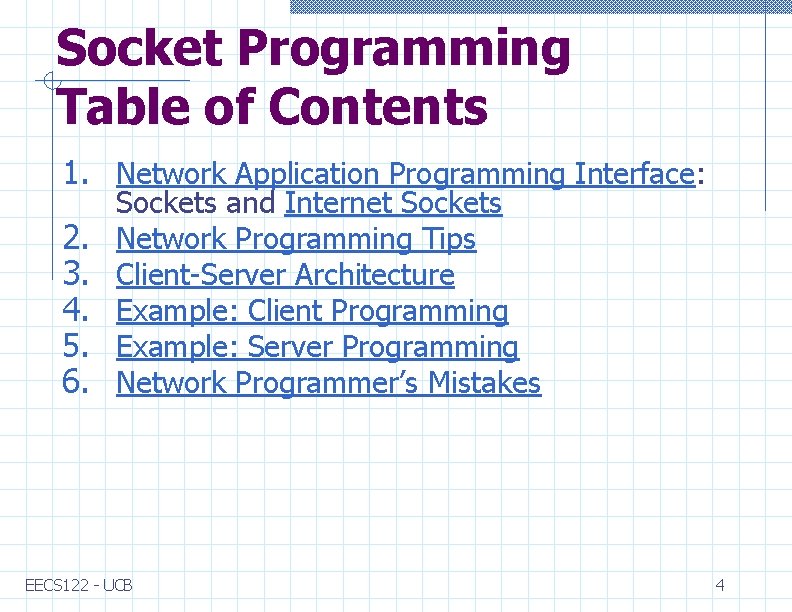
Socket Programming Table of Contents 1. Network Application Programming Interface: 2. 3. 4. 5. 6. Sockets and Internet Sockets Network Programming Tips Client-Server Architecture Example: Client Programming Example: Server Programming Network Programmer’s Mistakes EECS 122 - UCB 4
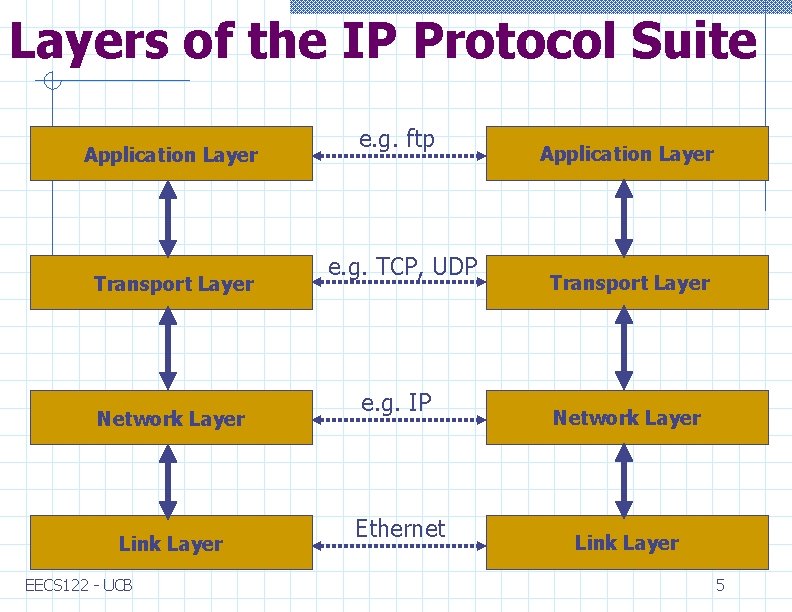
Layers of the IP Protocol Suite Application Layer Transport Layer Network Layer Link Layer EECS 122 - UCB e. g. ftp e. g. TCP, UDP e. g. IP Ethernet Application Layer Transport Layer Network Layer Link Layer 5
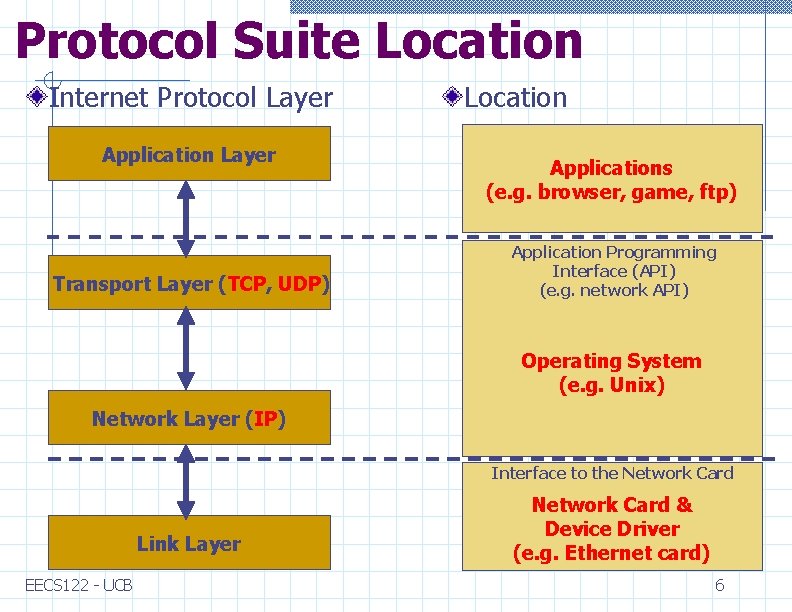
Protocol Suite Location Internet Protocol Layer Application Layer Transport Layer (TCP, UDP) Location Applications (e. g. browser, game, ftp) Application Programming Interface (API) (e. g. network API) Operating System (e. g. Unix) Network Layer (IP) Interface to the Network Card Link Layer EECS 122 - UCB Network Card & Device Driver (e. g. Ethernet card) 6
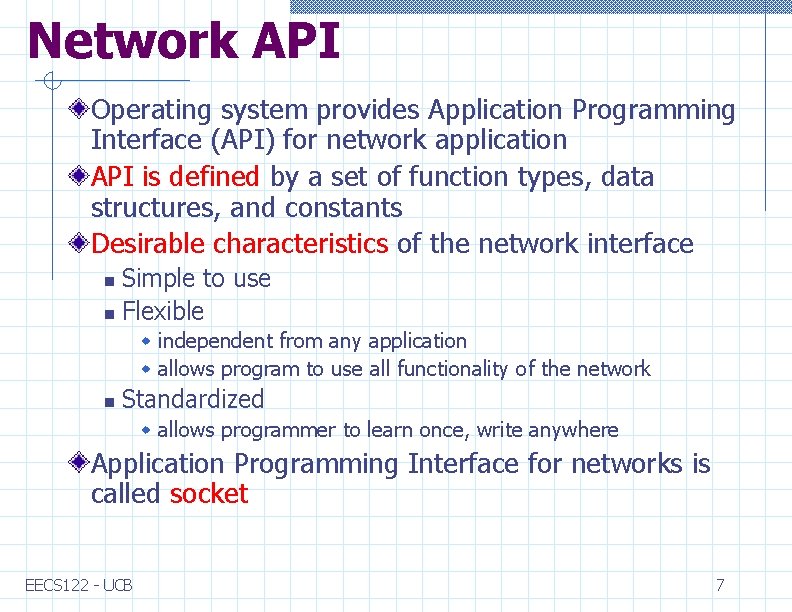
Network API Operating system provides Application Programming Interface (API) for network application API is defined by a set of function types, data structures, and constants Desirable characteristics of the network interface Simple to use n Flexible n w independent from any application w allows program to use all functionality of the network n Standardized w allows programmer to learn once, write anywhere Application Programming Interface for networks is called socket EECS 122 - UCB 7
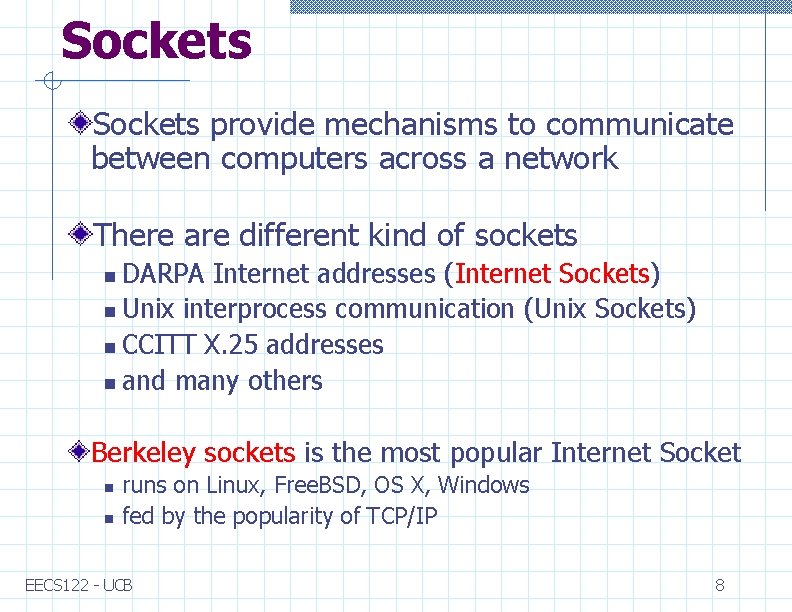
Sockets provide mechanisms to communicate between computers across a network There are different kind of sockets DARPA Internet addresses (Internet Sockets) n Unix interprocess communication (Unix Sockets) n CCITT X. 25 addresses n and many others n Berkeley sockets is the most popular Internet Socket n n runs on Linux, Free. BSD, OS X, Windows fed by the popularity of TCP/IP EECS 122 - UCB 8
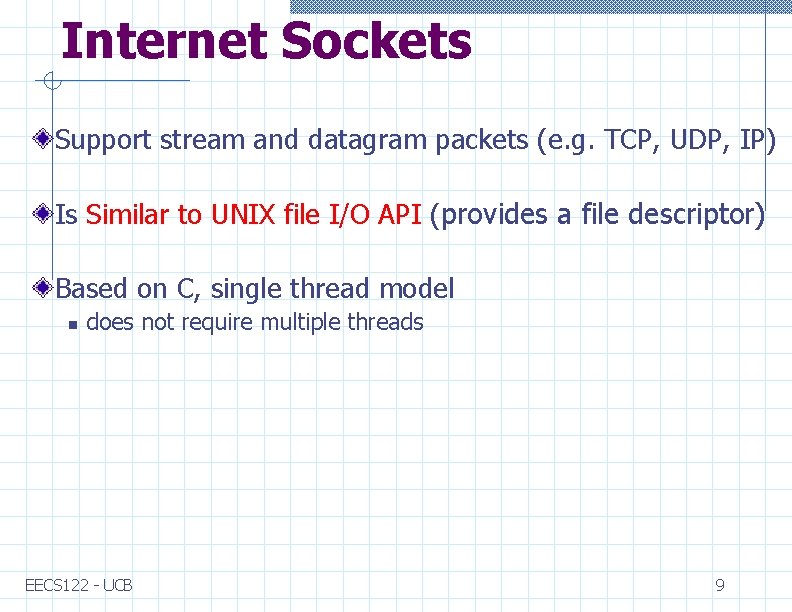
Internet Sockets Support stream and datagram packets (e. g. TCP, UDP, IP) Is Similar to UNIX file I/O API (provides a file descriptor) Based on C, single thread model n does not require multiple threads EECS 122 - UCB 9
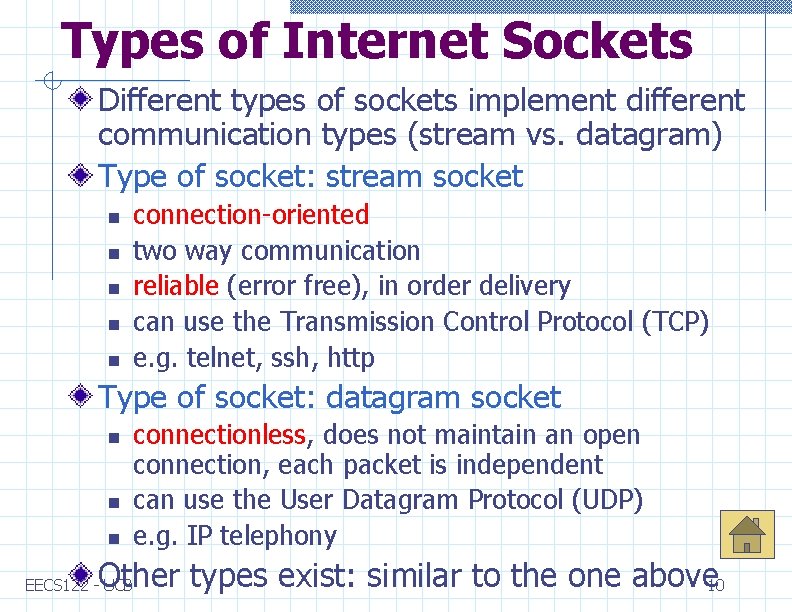
Types of Internet Sockets Different types of sockets implement different communication types (stream vs. datagram) Type of socket: stream socket n n n connection-oriented two way communication reliable (error free), in order delivery can use the Transmission Control Protocol (TCP) e. g. telnet, ssh, http Type of socket: datagram socket n n n connectionless, does not maintain an open connection, each packet is independent can use the User Datagram Protocol (UDP) e. g. IP telephony Other types exist: similar to the one above 10 EECS 122 - UCB
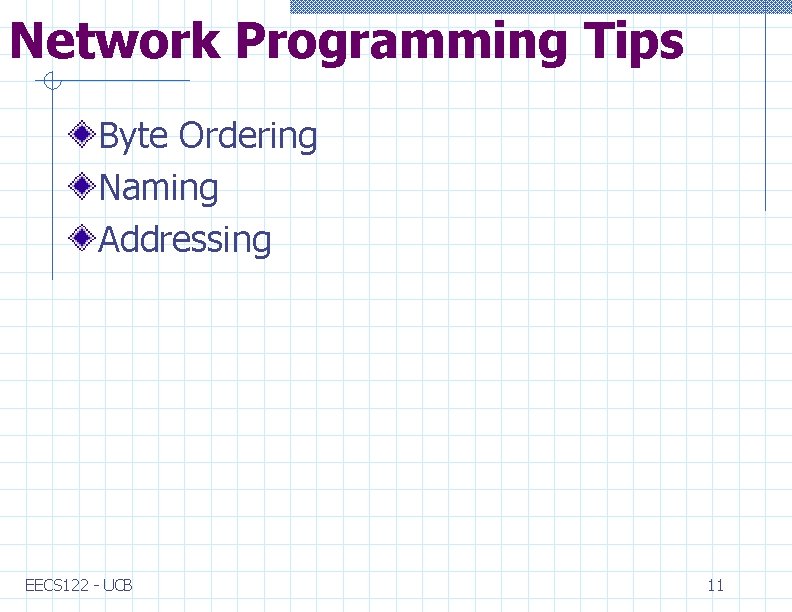
Network Programming Tips Byte Ordering Naming Addressing EECS 122 - UCB 11
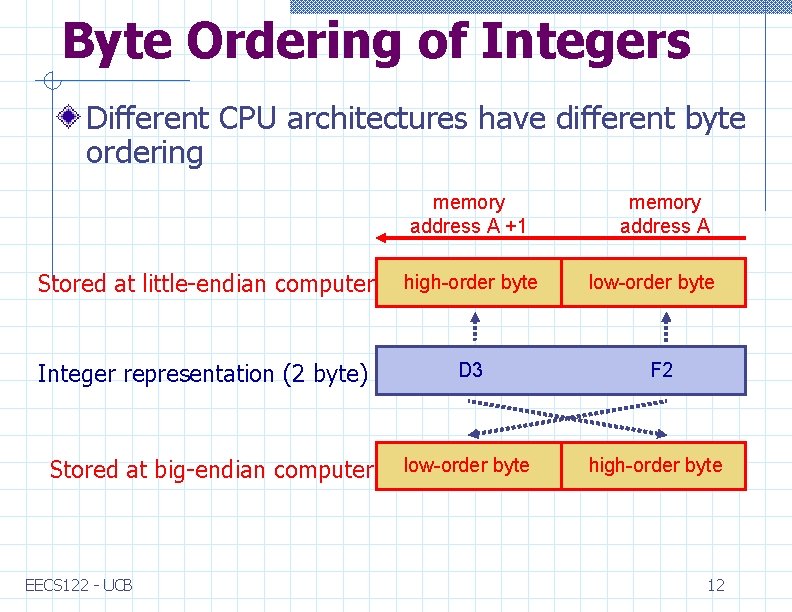
Byte Ordering of Integers Different CPU architectures have different byte ordering memory address A +1 memory address A Stored at little-endian computer high-order byte Integer representation (2 byte) D 3 F 2 low-order byte high-order byte Stored at big-endian computer EECS 122 - UCB low-order byte 12
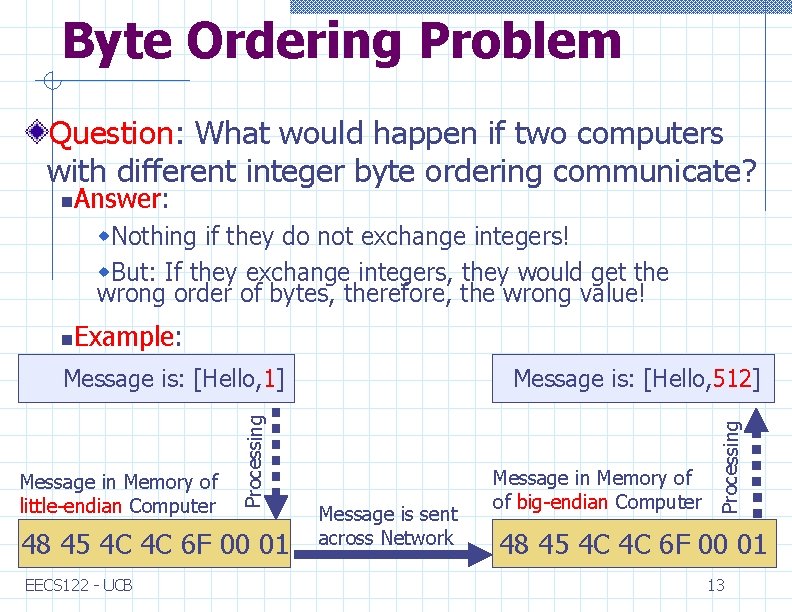
Byte Ordering Problem Question: What would happen if two computers with different integer byte ordering communicate? n Answer: w. Nothing if they do not exchange integers! w. But: If they exchange integers, they would get the wrong order of bytes, therefore, the wrong value! Example: Message in Memory of little-endian Computer Processing Message is: [Hello, 1] 48 45 4 C 4 C 6 F 00 01 EECS 122 - UCB Message is: [Hello, 512] Message is sent across Network Message in Memory of of big-endian Computer Processing n 48 45 4 C 4 C 6 F 00 01 13
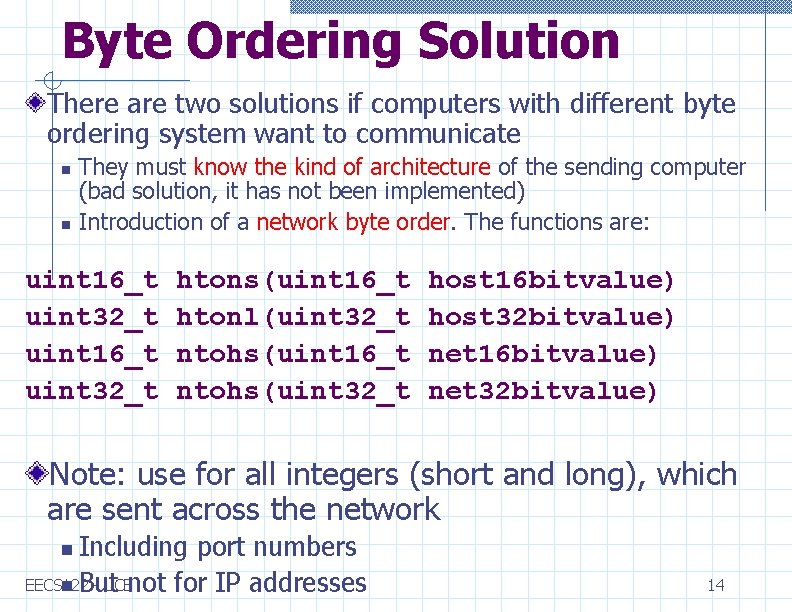
Byte Ordering Solution There are two solutions if computers with different byte ordering system want to communicate n n They must know the kind of architecture of the sending computer (bad solution, it has not been implemented) Introduction of a network byte order. The functions are: uint 16_t uint 32_t htons(uint 16_t htonl(uint 32_t ntohs(uint 16_t ntohs(uint 32_t host 16 bitvalue) host 32 bitvalue) net 16 bitvalue) net 32 bitvalue) Note: use for all integers (short and long), which are sent across the network Including port numbers EECS 122 - UCBnot for IP addresses n But n 14
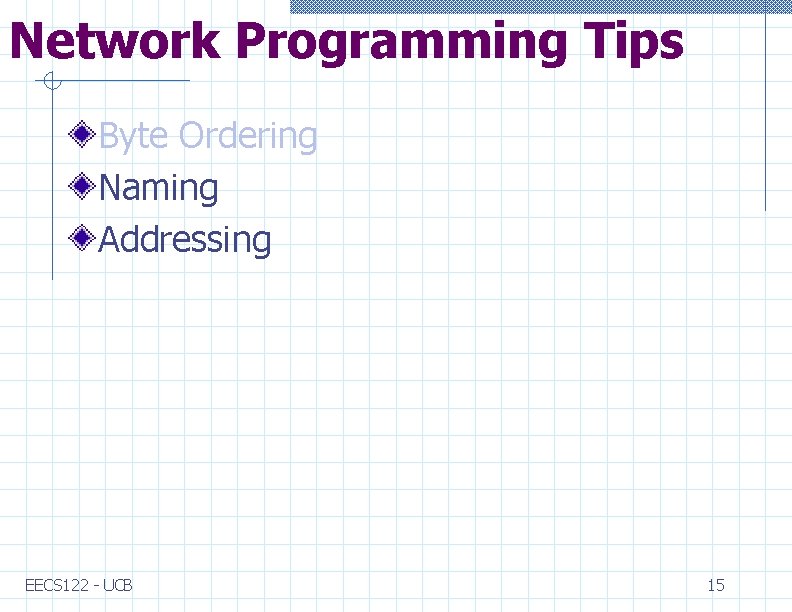
Network Programming Tips Byte Ordering Naming Addressing EECS 122 - UCB 15
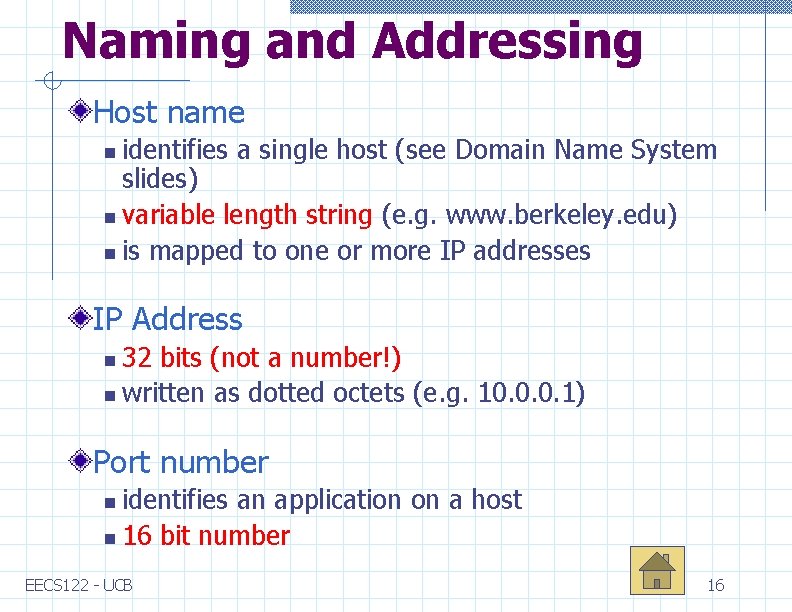
Naming and Addressing Host name identifies a single host (see Domain Name System slides) n variable length string (e. g. www. berkeley. edu) n is mapped to one or more IP addresses n IP Address 32 bits (not a number!) n written as dotted octets (e. g. 10. 0. 0. 1) n Port number identifies an application on a host n 16 bit number n EECS 122 - UCB 16
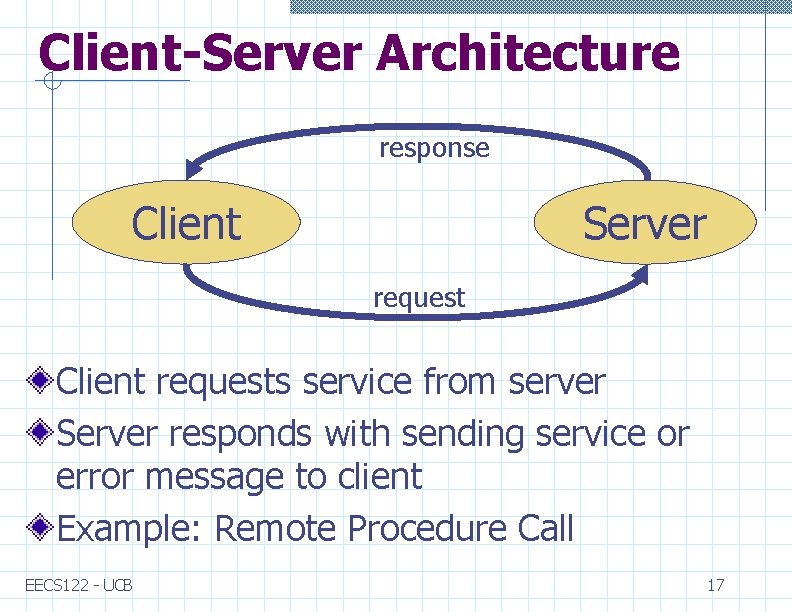
Client-Server Architecture response Client Server request Client requests service from server Server responds with sending service or error message to client Example: Remote Procedure Call EECS 122 - UCB 17
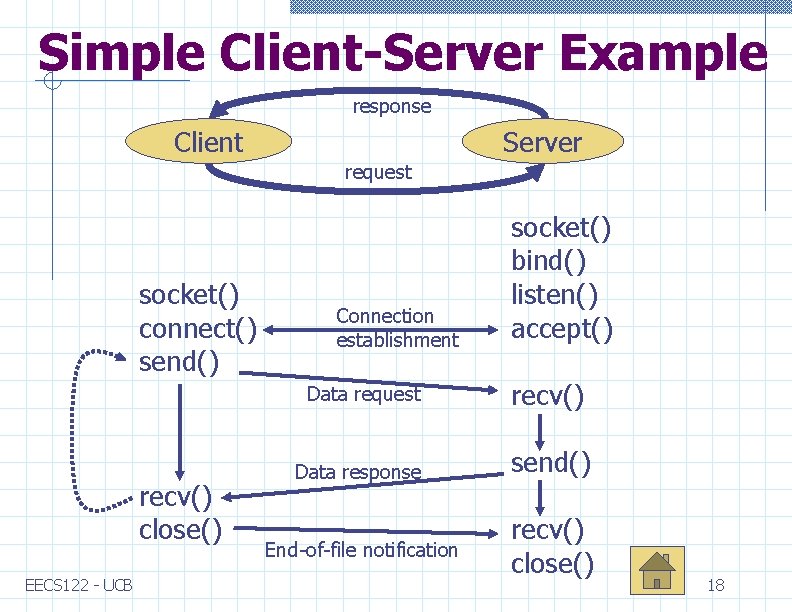
Simple Client-Server Example response Client Server request socket() connect() send() recv() close() EECS 122 - UCB Connection establishment socket() bind() listen() accept() Data request recv() Data response send() End-of-file notification recv() close() 18
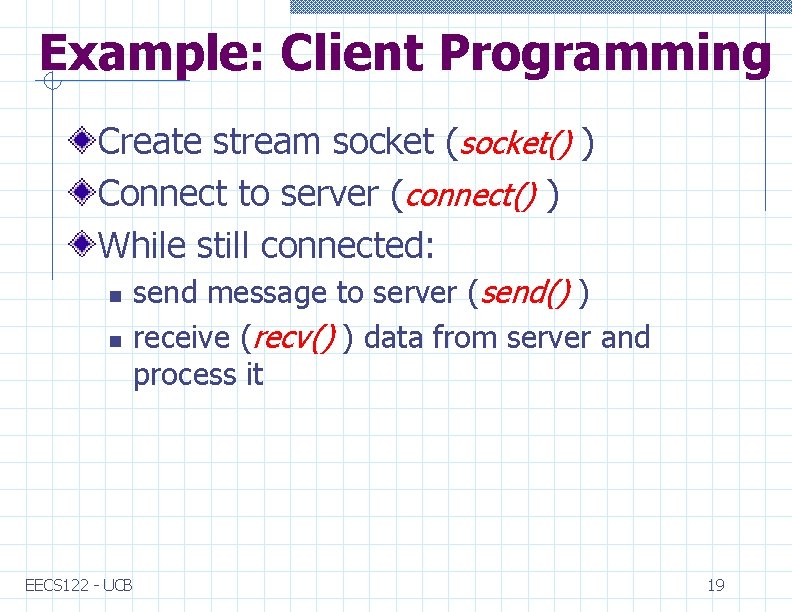
Example: Client Programming Create stream socket (socket() ) Connect to server (connect() ) While still connected: n n send message to server (send() ) receive (recv() ) data from server and process it EECS 122 - UCB 19
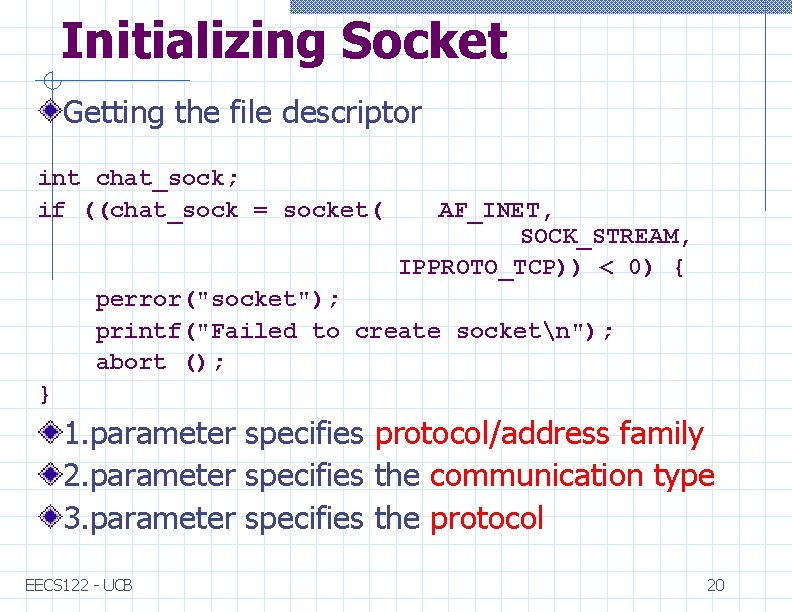
Initializing Socket Getting the file descriptor int chat_sock; if ((chat_sock = socket( AF_INET, SOCK_STREAM, IPPROTO_TCP)) < 0) { perror("socket"); printf("Failed to create socketn"); abort (); } 1. parameter specifies protocol/address family 2. parameter specifies the communication type 3. parameter specifies the protocol EECS 122 - UCB 20
![Connecting to Server struct sockaddrin sin struct hostent host gethostbyname argv1 unsigned int Connecting to Server struct sockaddr_in sin; struct hostent *host = gethostbyname (argv[1]); unsigned int](https://slidetodoc.com/presentation_image/5169114a8c5c4625783f3757653f85d8/image-21.jpg)
Connecting to Server struct sockaddr_in sin; struct hostent *host = gethostbyname (argv[1]); unsigned int server_address = *(unsigned long *) host->h_addr_list[0]; unsigned short server_port = atoi (argv[2]); memset (&sin, 0, sizeof (sin)); sin_family = AF_INET; sin_addr. s_addr = server_address; sin_port = htons (server_port); if (connect(chat_sock, (struct sockaddr *) &sin, sizeof (sin)) < 0) { perror("connect"); printf("Cannot connect to servern"); abort(); } EECS 122 - UCB 21
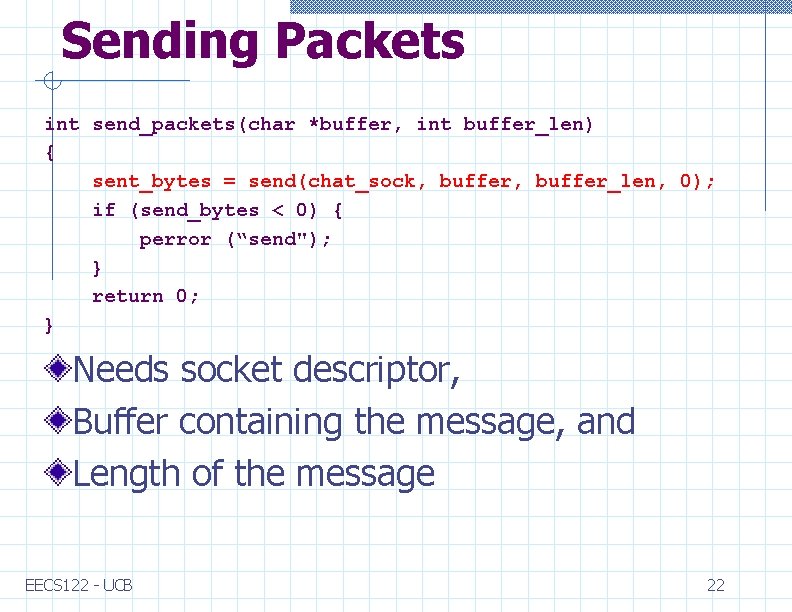
Sending Packets int send_packets(char *buffer, int buffer_len) { sent_bytes = send(chat_sock, buffer_len, 0); if (send_bytes < 0) { perror (“send"); } return 0; } Needs socket descriptor, Buffer containing the message, and Length of the message EECS 122 - UCB 22
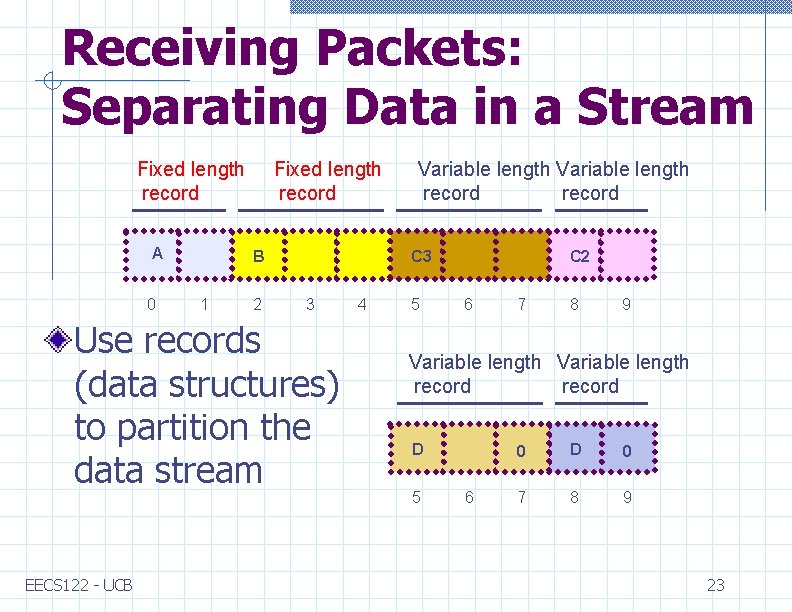
Receiving Packets: Separating Data in a Stream Fixed length record A 0 B 1 2 C 3 3 Use records (data structures) to partition the data stream EECS 122 - UCB Variable length record 4 5 C 2 6 7 8 9 Variable length record D 5 6 0 D 0 7 8 9 23
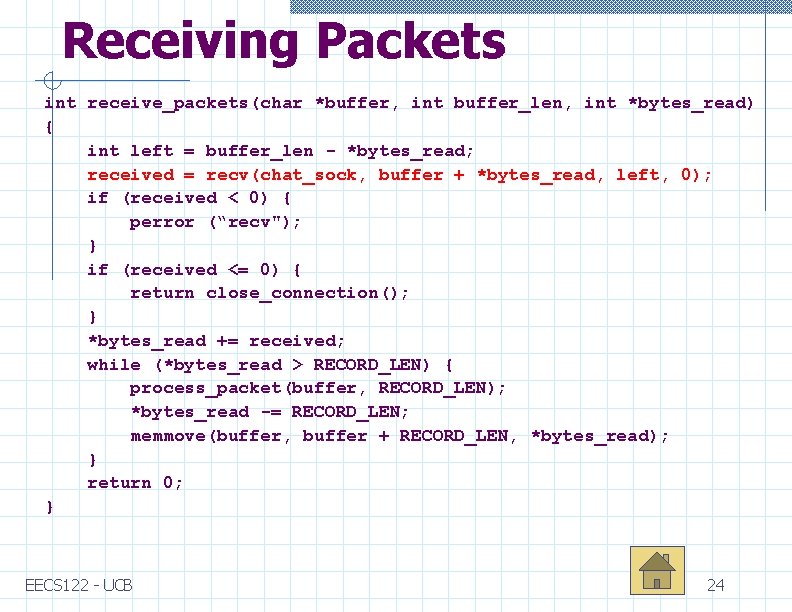
Receiving Packets int receive_packets(char *buffer, int buffer_len, int *bytes_read) { int left = buffer_len - *bytes_read; received = recv(chat_sock, buffer + *bytes_read, left, 0); if (received < 0) { perror (“recv"); } if (received <= 0) { return close_connection(); } *bytes_read += received; while (*bytes_read > RECORD_LEN) { process_packet(buffer, RECORD_LEN); *bytes_read -= RECORD_LEN; memmove(buffer, buffer + RECORD_LEN, *bytes_read); } return 0; } EECS 122 - UCB 24
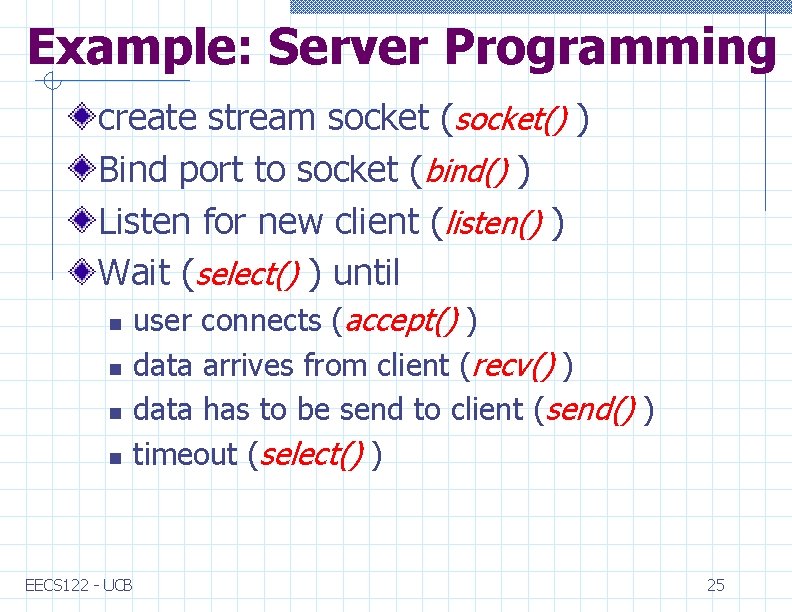
Example: Server Programming create stream socket (socket() ) Bind port to socket (bind() ) Listen for new client (listen() ) Wait (select() ) until n n user connects (accept() ) data arrives from client (recv() ) data has to be send to client (send() ) timeout (select() ) EECS 122 - UCB 25
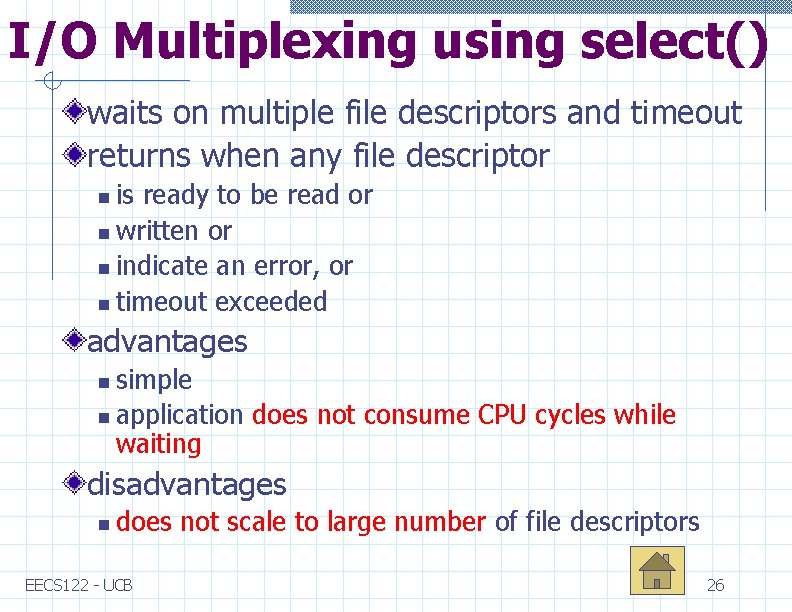
I/O Multiplexing using select() waits on multiple file descriptors and timeout returns when any file descriptor is ready to be read or n written or n indicate an error, or n timeout exceeded n advantages simple n application does not consume CPU cycles while waiting n disadvantages n does not scale to large number of file descriptors EECS 122 - UCB 26
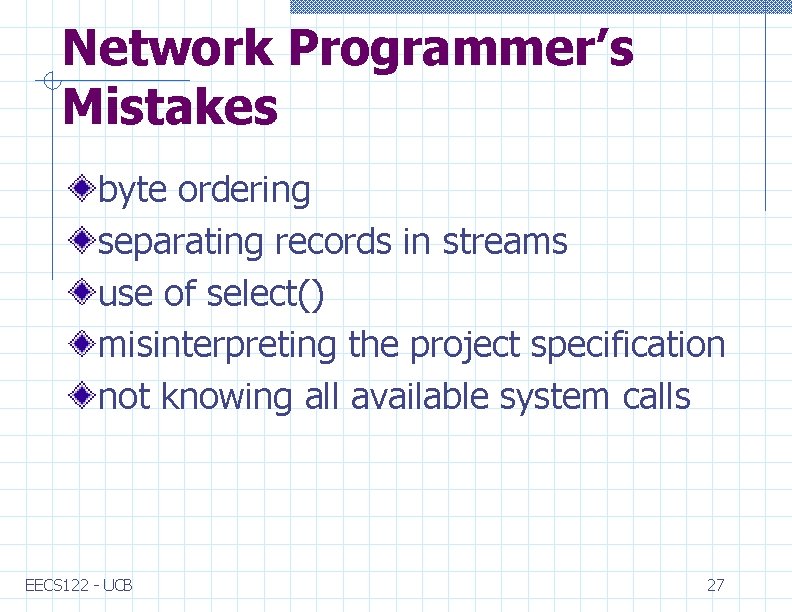
Network Programmer’s Mistakes byte ordering separating records in streams use of select() misinterpreting the project specification not knowing all available system calls EECS 122 - UCB 27
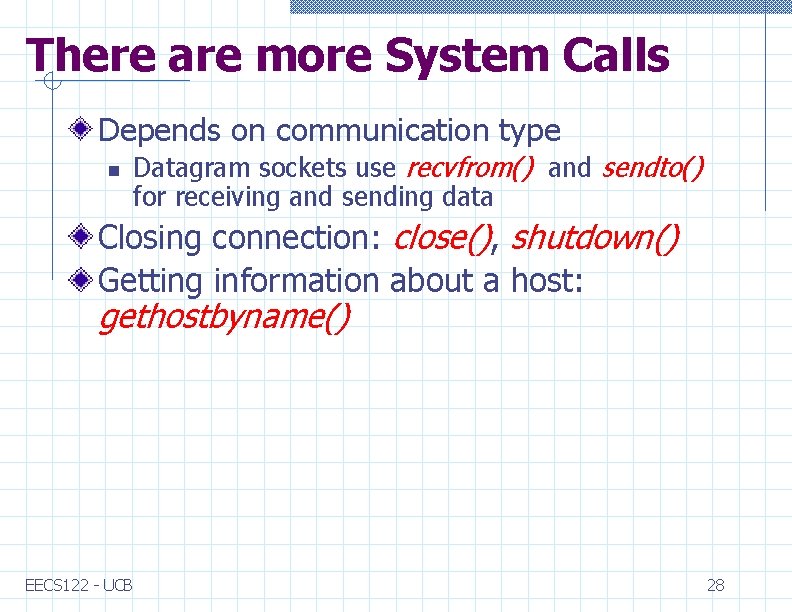
There are more System Calls Depends on communication type n Datagram sockets use recvfrom() and sendto() for receiving and sending data Closing connection: close(), shutdown() Getting information about a host: gethostbyname() EECS 122 - UCB 28
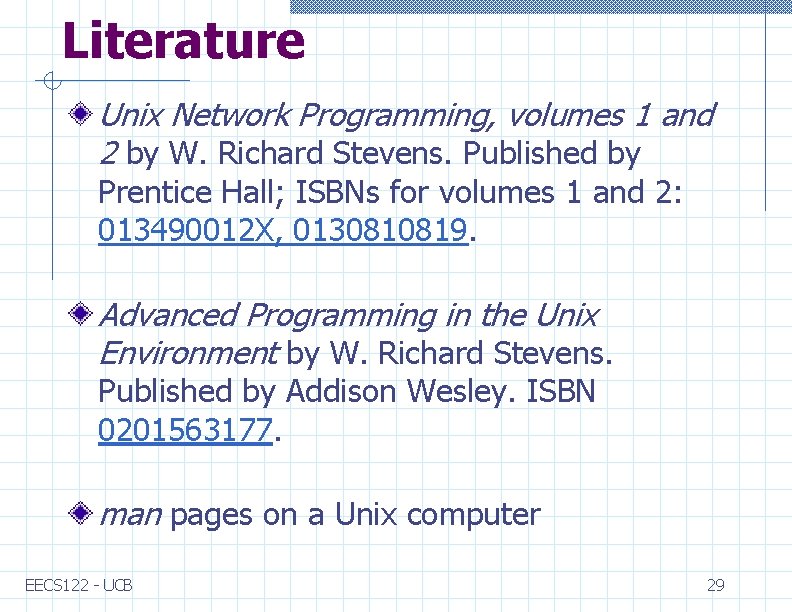
Literature Unix Network Programming, volumes 1 and 2 by W. Richard Stevens. Published by Prentice Hall; ISBNs for volumes 1 and 2: 013490012 X, 0130810819. Advanced Programming in the Unix Environment by W. Richard Stevens. Published by Addison Wesley. ISBN 0201563177. man pages on a Unix computer EECS 122 - UCB 29
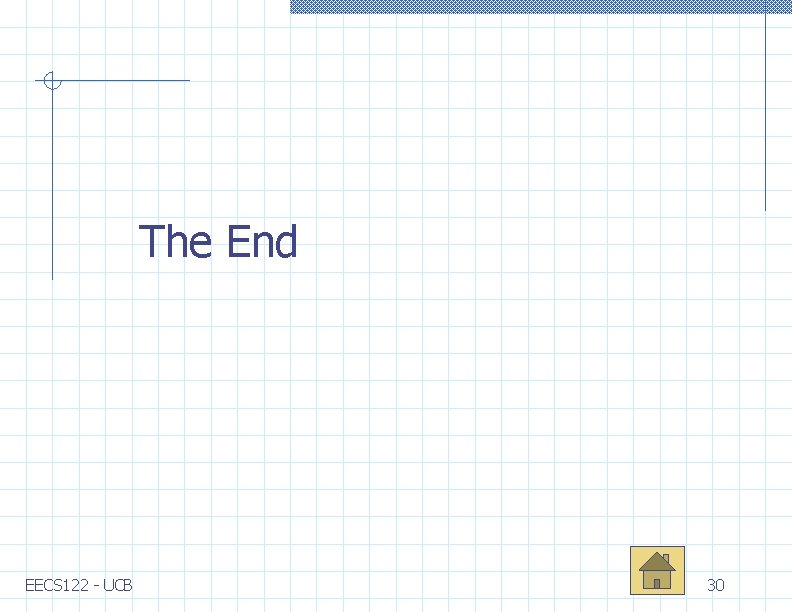
The End EECS 122 - UCB 30
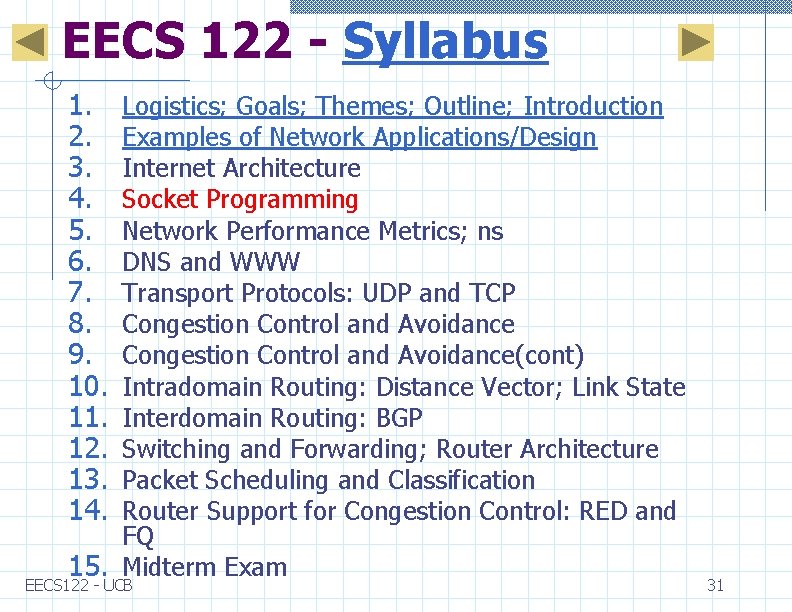
EECS 122 - Syllabus 1. 2. 3. 4. 5. 6. 7. 8. 9. 10. 11. 12. 13. 14. Logistics; Goals; Themes; Outline; Introduction Examples of Network Applications/Design Internet Architecture Socket Programming Network Performance Metrics; ns DNS and WWW Transport Protocols: UDP and TCP Congestion Control and Avoidance(cont) Intradomain Routing: Distance Vector; Link State Interdomain Routing: BGP Switching and Forwarding; Router Architecture Packet Scheduling and Classification Router Support for Congestion Control: RED and FQ 15. Midterm Exam EECS 122 - UCB 31
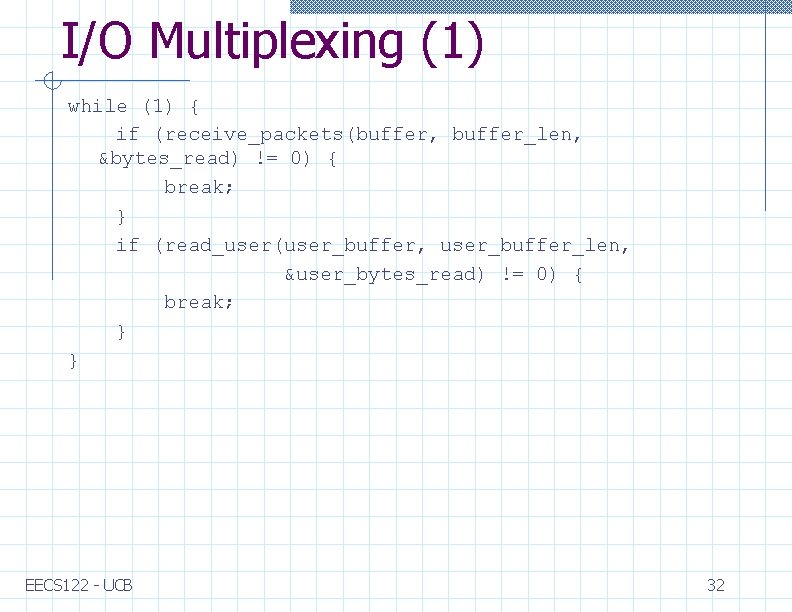
I/O Multiplexing (1) while (1) { if (receive_packets(buffer, buffer_len, &bytes_read) != 0) { break; } if (read_user(user_buffer, user_buffer_len, &user_bytes_read) != 0) { break; } } EECS 122 - UCB 32
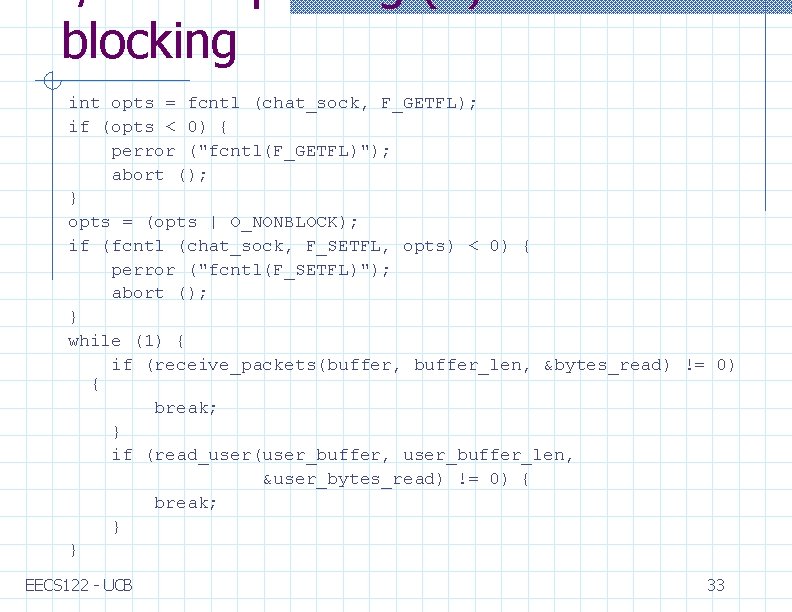
blocking int opts = fcntl (chat_sock, F_GETFL); if (opts < 0) { perror ("fcntl(F_GETFL)"); abort (); } opts = (opts | O_NONBLOCK); if (fcntl (chat_sock, F_SETFL, opts) < 0) { perror ("fcntl(F_SETFL)"); abort (); } while (1) { if (receive_packets(buffer, buffer_len, &bytes_read) != 0) { break; } if (read_user(user_buffer, user_buffer_len, &user_bytes_read) != 0) { break; } } EECS 122 - UCB 33
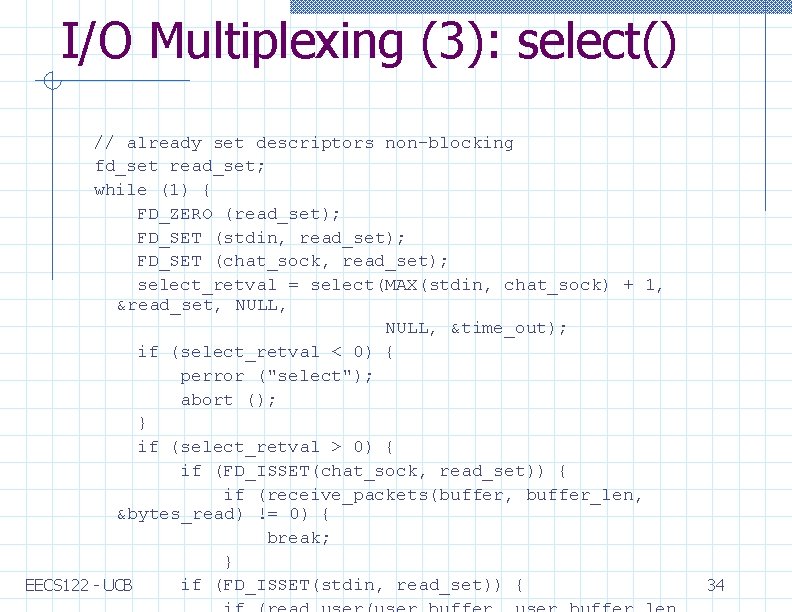
I/O Multiplexing (3): select() // already set descriptors non-blocking fd_set read_set; while (1) { FD_ZERO (read_set); FD_SET (stdin, read_set); FD_SET (chat_sock, read_set); select_retval = select(MAX(stdin, chat_sock) + 1, &read_set, NULL, &time_out); if (select_retval < 0) { perror ("select"); abort (); } if (select_retval > 0) { if (FD_ISSET(chat_sock, read_set)) { if (receive_packets(buffer, buffer_len, &bytes_read) != 0) { break; } if (FD_ISSET(stdin, read_set)) { EECS 122 - UCB 34
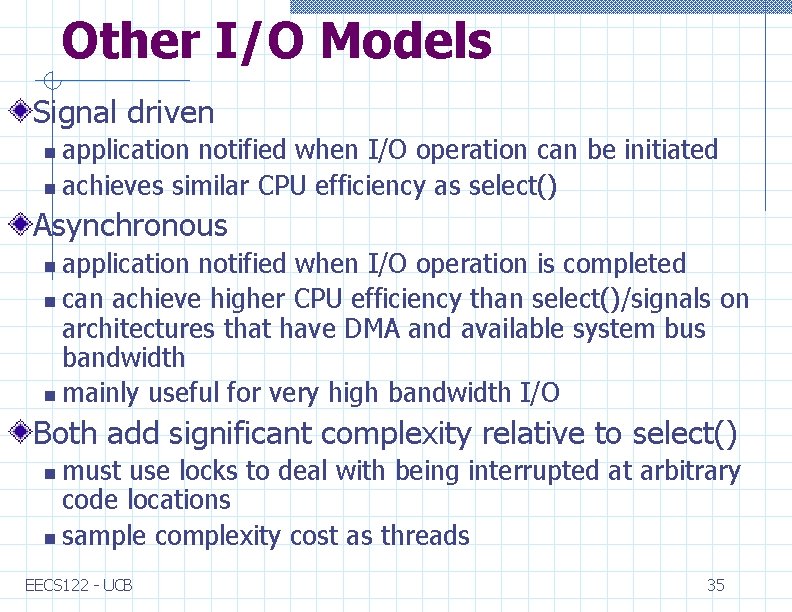
Other I/O Models Signal driven application notified when I/O operation can be initiated n achieves similar CPU efficiency as select() n Asynchronous application notified when I/O operation is completed n can achieve higher CPU efficiency than select()/signals on architectures that have DMA and available system bus bandwidth n mainly useful for very high bandwidth I/O n Both add significant complexity relative to select() must use locks to deal with being interrupted at arbitrary code locations n sample complexity cost as threads n EECS 122 - UCB 35